CS 1101 Programming Methodology http www comp nus
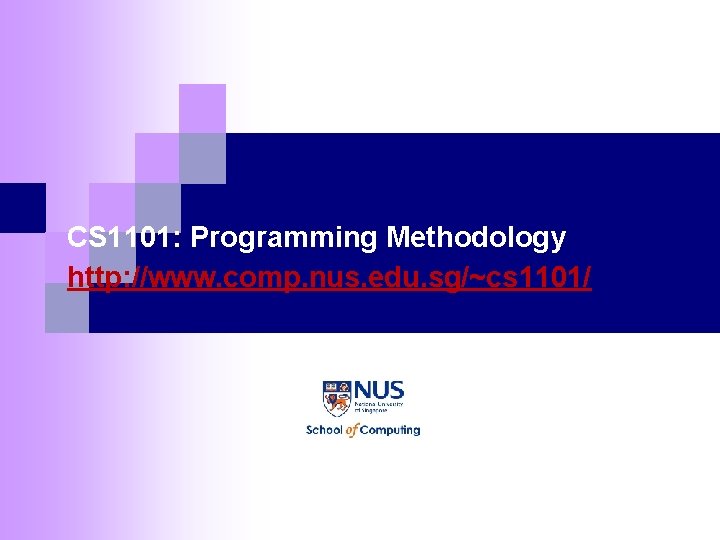
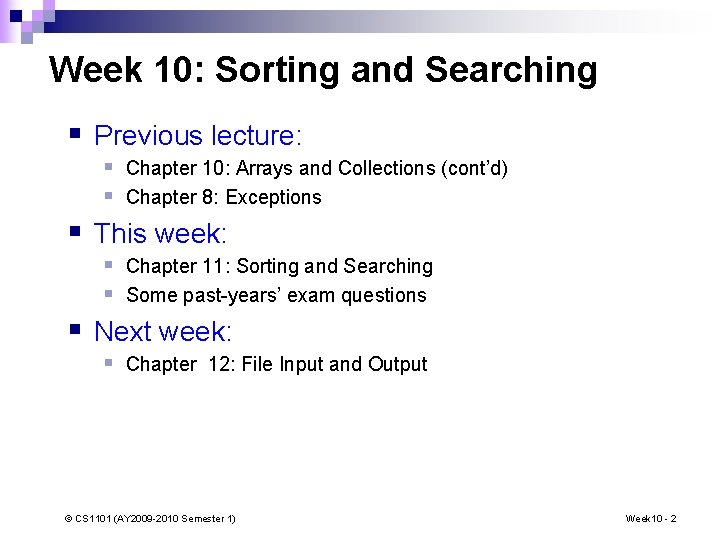
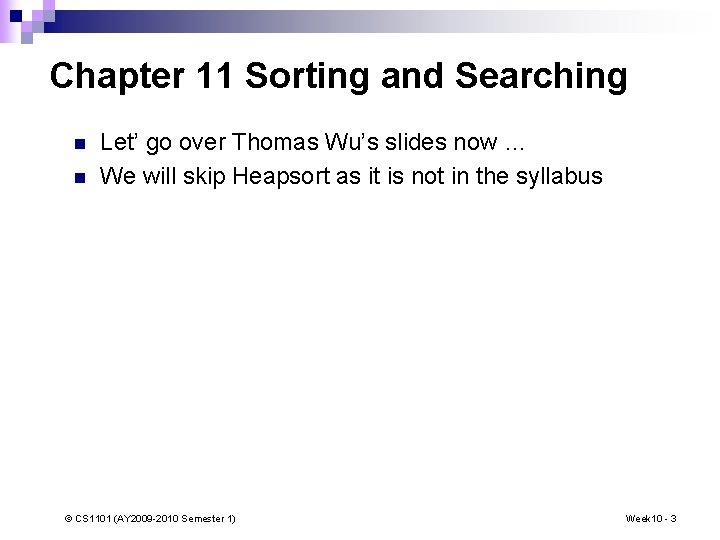
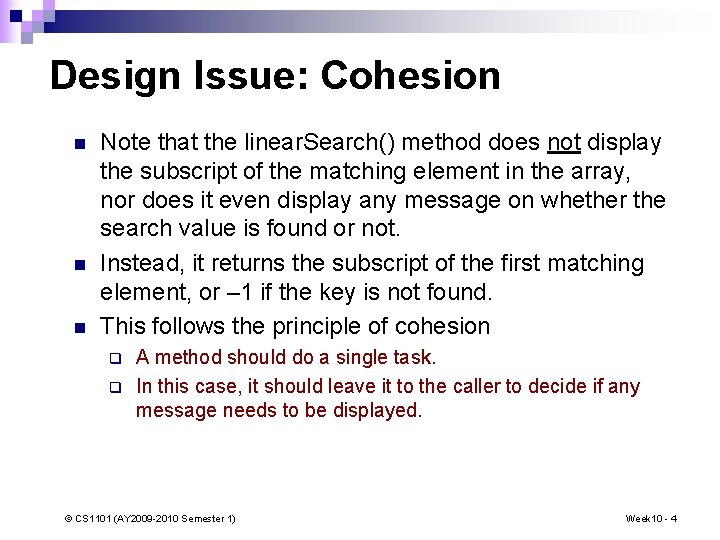
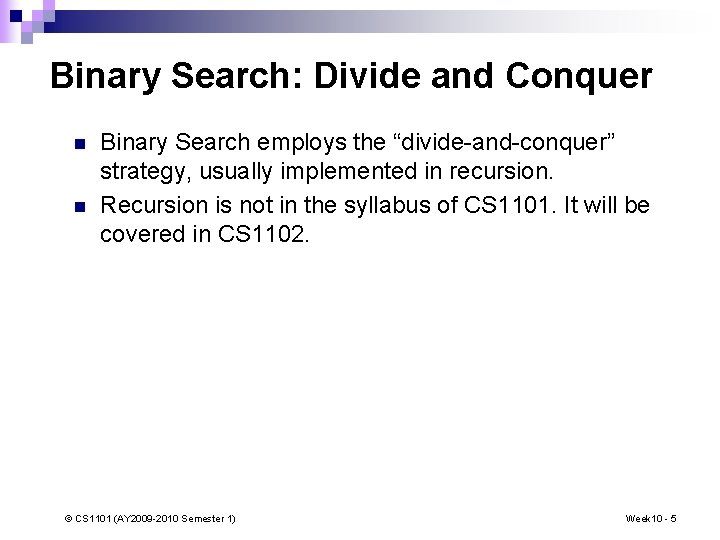
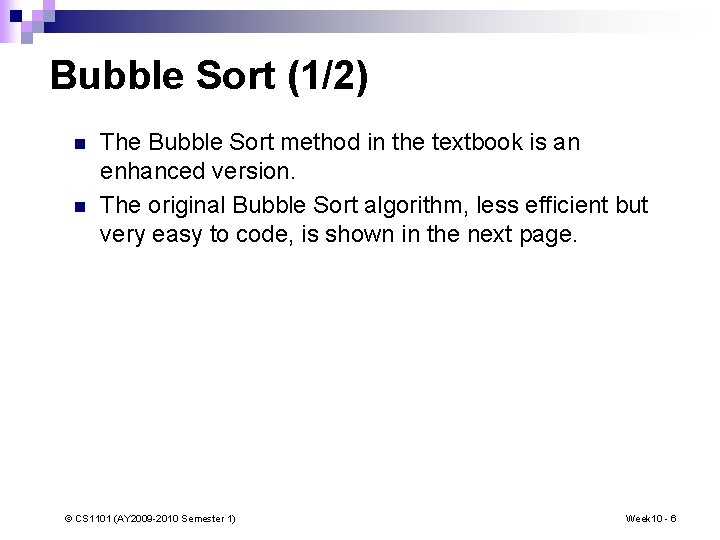
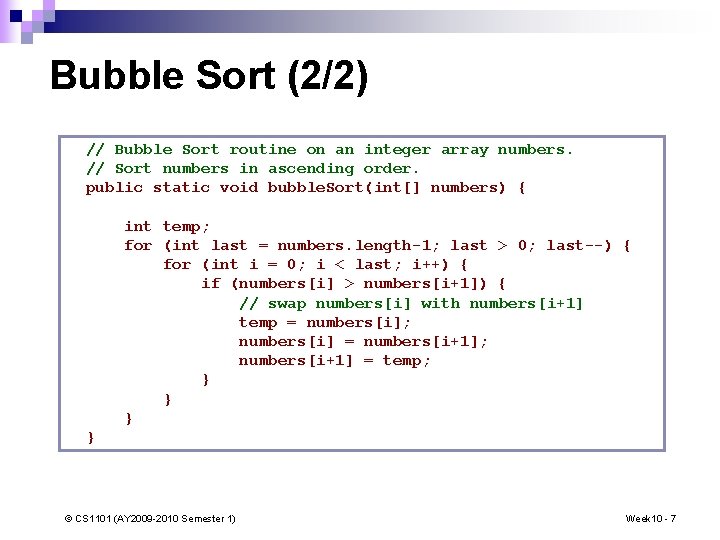
![Insertion Sort (1/6) n Algorithm basis ¨ n On pass i, n Insert v[i] Insertion Sort (1/6) n Algorithm basis ¨ n On pass i, n Insert v[i]](https://slidetodoc.com/presentation_image_h2/c9f96522800be5d60eda148bbb1d31ca/image-8.jpg)
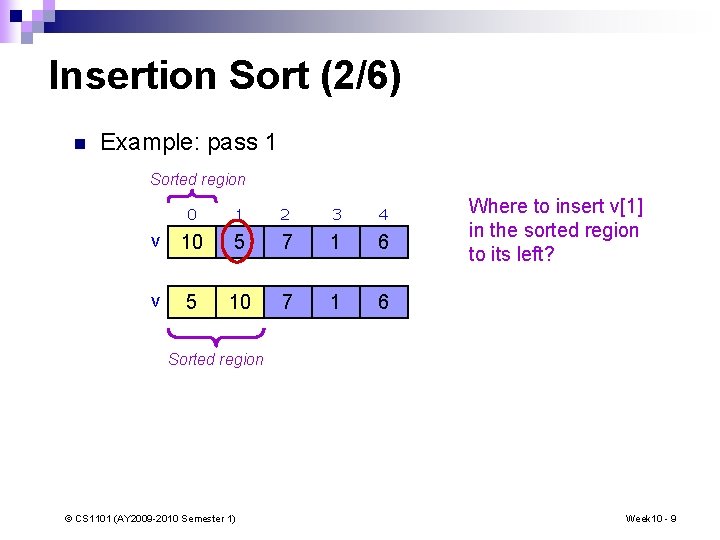
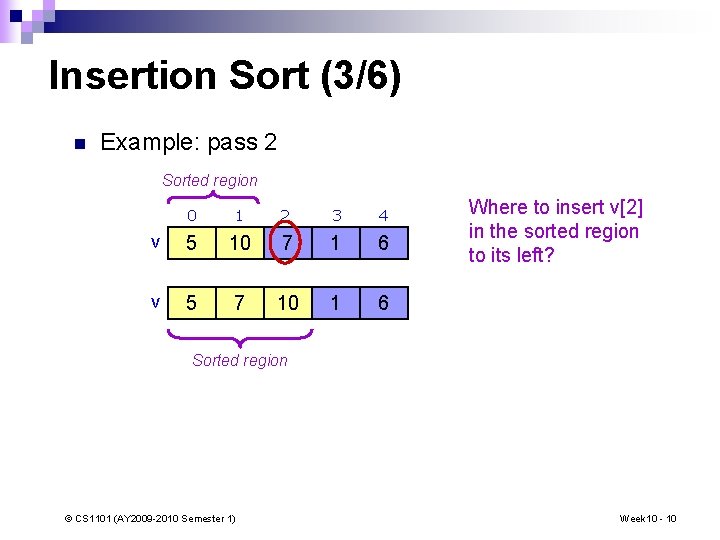
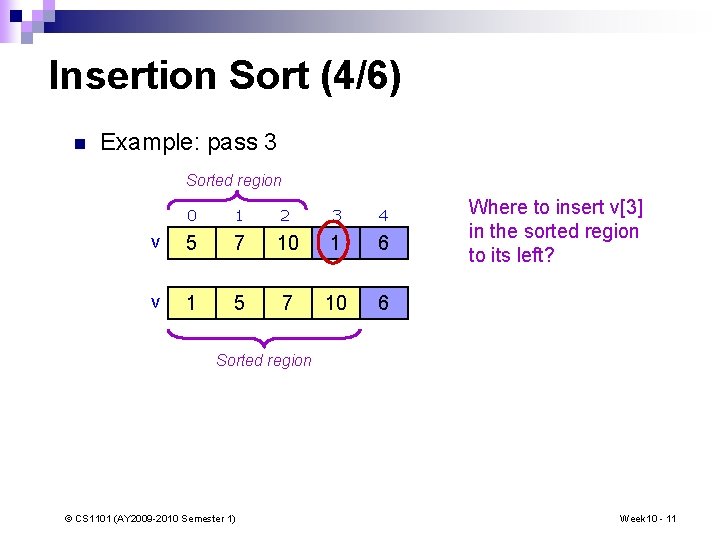
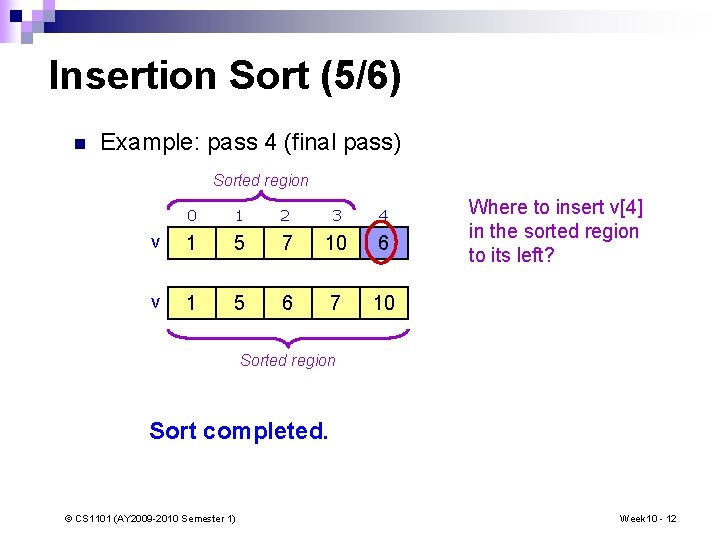
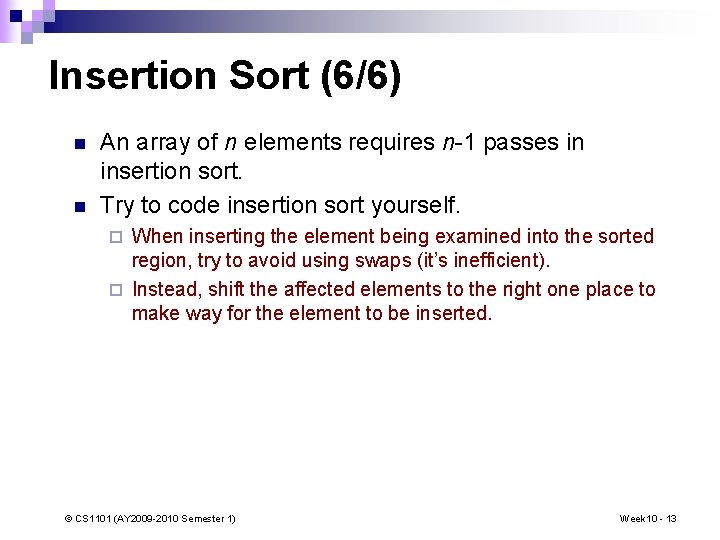
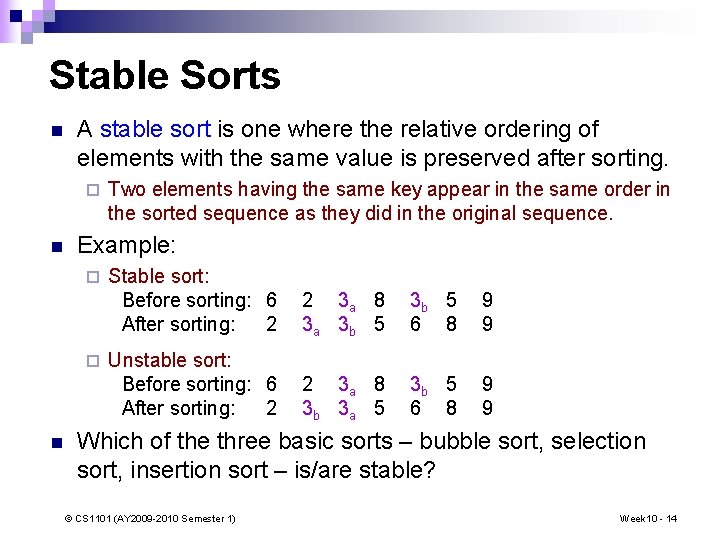
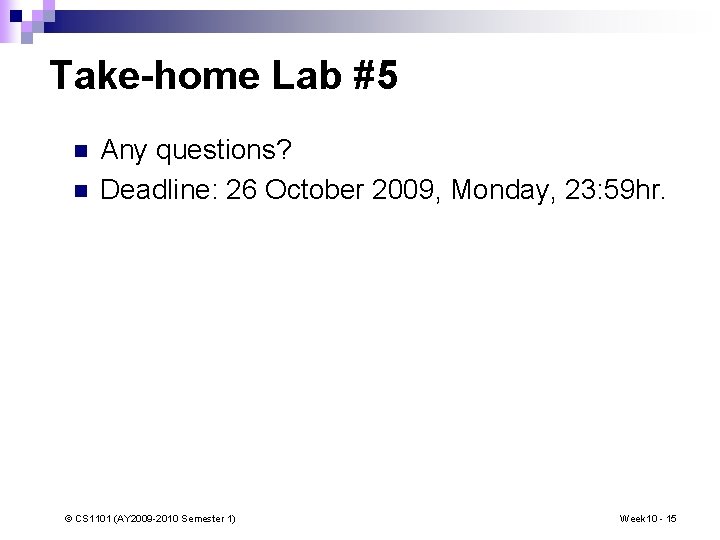
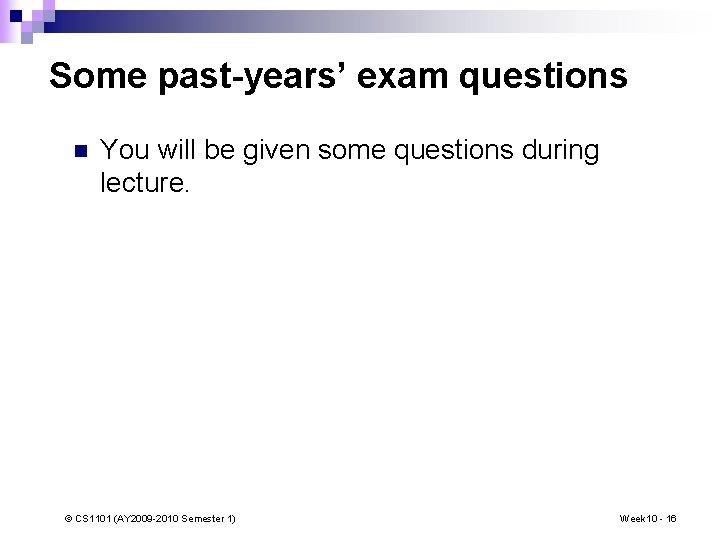
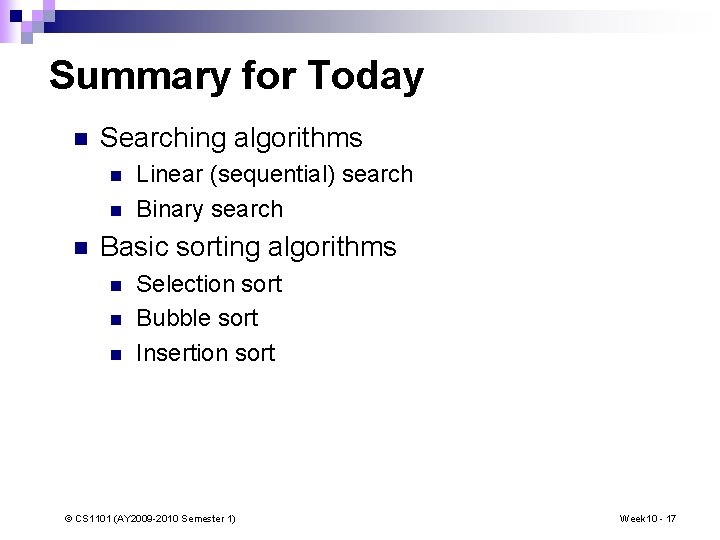
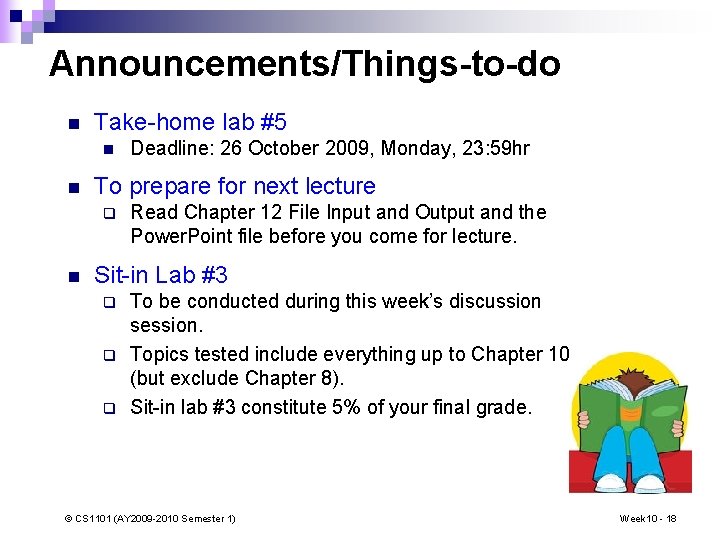
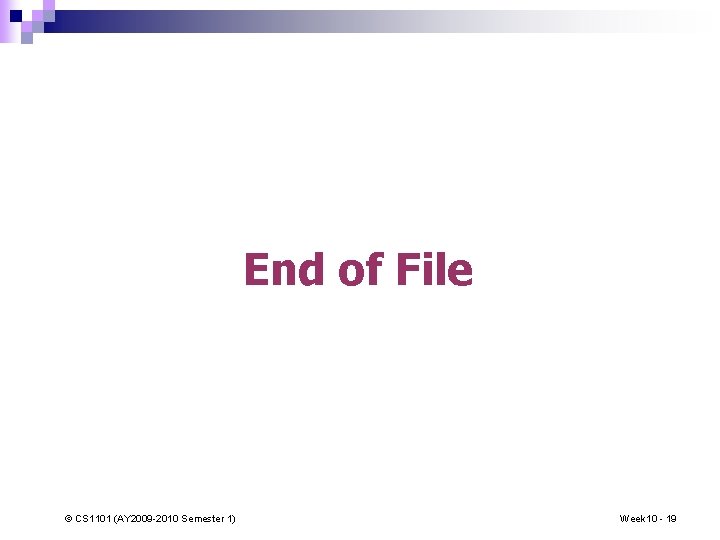
- Slides: 19
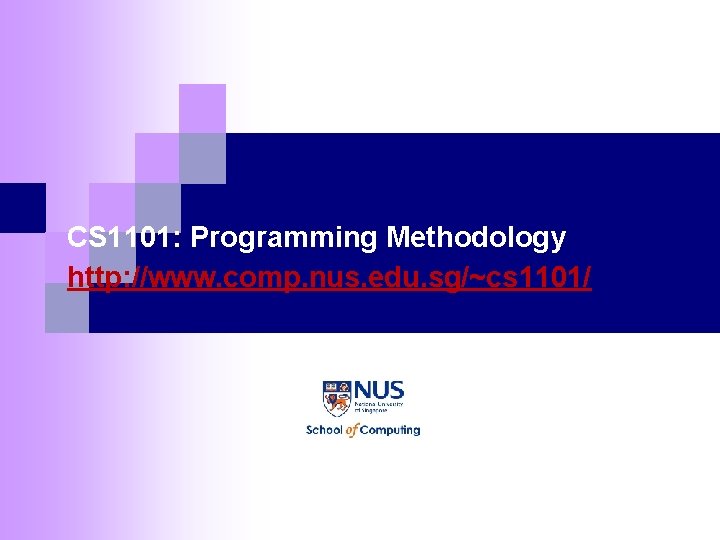
CS 1101: Programming Methodology http: //www. comp. nus. edu. sg/~cs 1101/
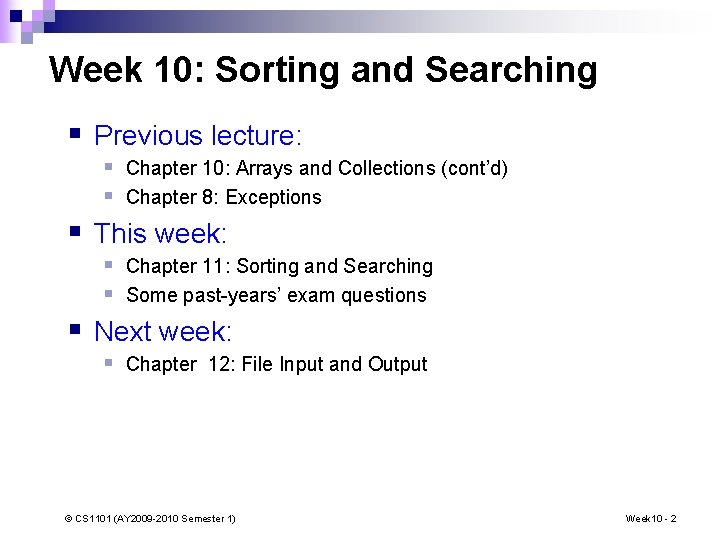
Week 10: Sorting and Searching § Previous lecture: § Chapter 10: Arrays and Collections (cont’d) § Chapter 8: Exceptions § This week: § Chapter 11: Sorting and Searching § Some past-years’ exam questions § Next week: § Chapter 12: File Input and Output © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 2
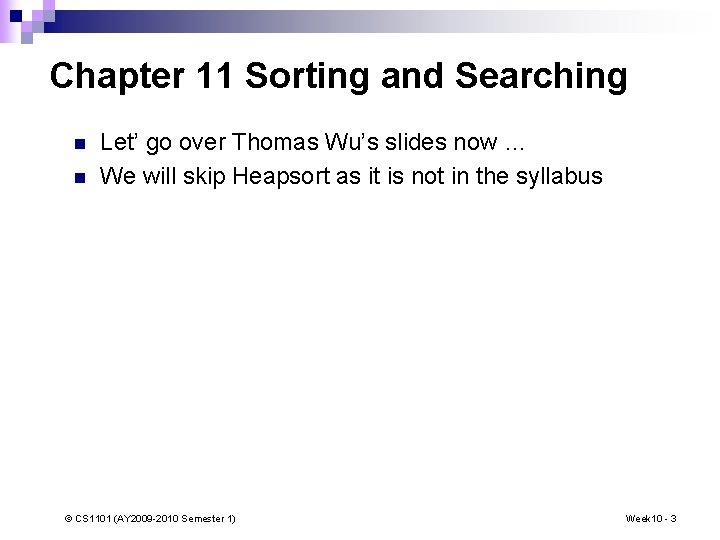
Chapter 11 Sorting and Searching n n Let’ go over Thomas Wu’s slides now … We will skip Heapsort as it is not in the syllabus © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 3
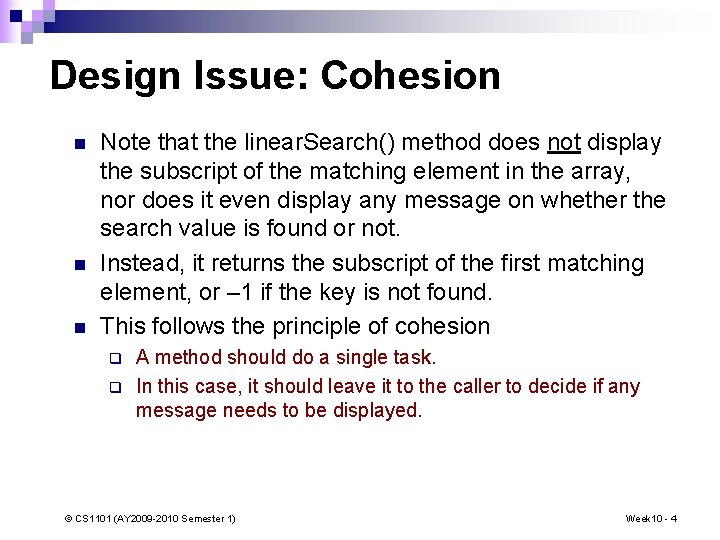
Design Issue: Cohesion n Note that the linear. Search() method does not display the subscript of the matching element in the array, nor does it even display any message on whether the search value is found or not. Instead, it returns the subscript of the first matching element, or – 1 if the key is not found. This follows the principle of cohesion q q A method should do a single task. In this case, it should leave it to the caller to decide if any message needs to be displayed. © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 4
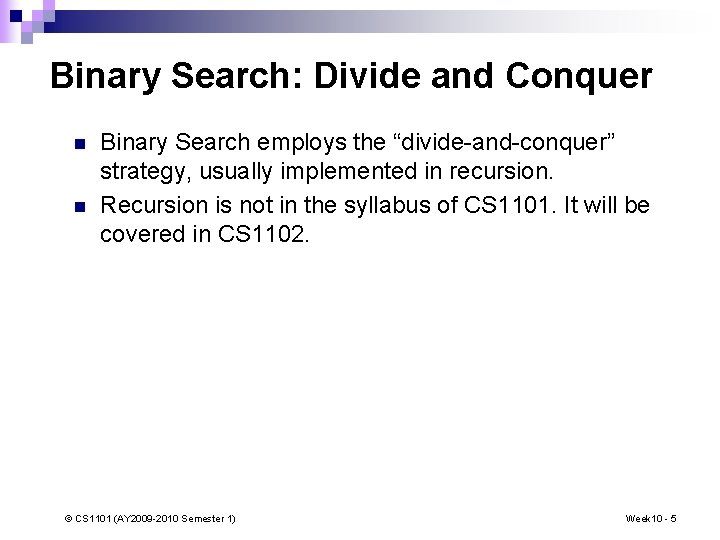
Binary Search: Divide and Conquer n n Binary Search employs the “divide-and-conquer” strategy, usually implemented in recursion. Recursion is not in the syllabus of CS 1101. It will be covered in CS 1102. © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 5
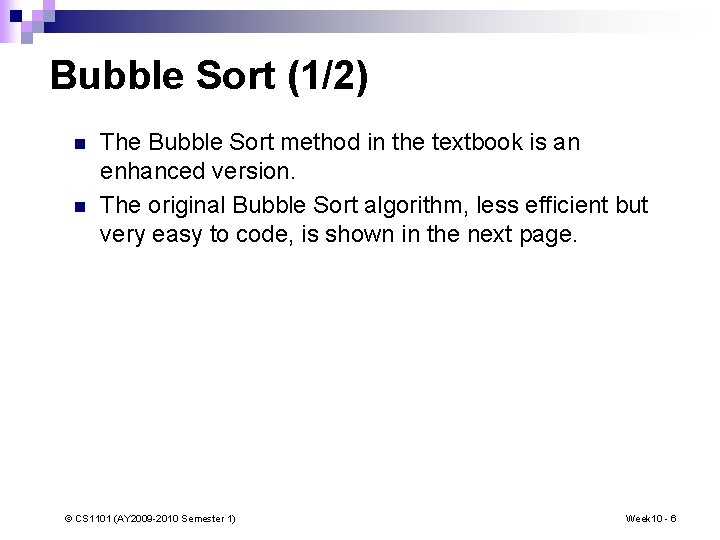
Bubble Sort (1/2) n n The Bubble Sort method in the textbook is an enhanced version. The original Bubble Sort algorithm, less efficient but very easy to code, is shown in the next page. © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 6
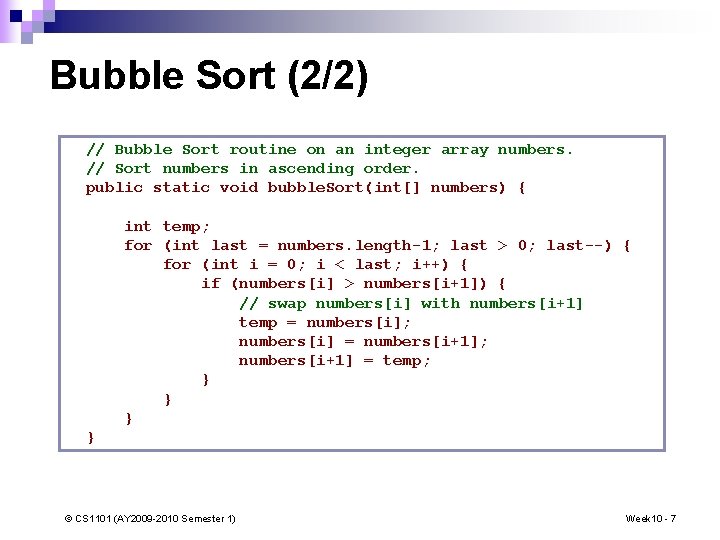
Bubble Sort (2/2) // Bubble Sort routine on an integer array numbers. // Sort numbers in ascending order. public static void bubble. Sort(int[] numbers) { int temp; for (int last = numbers. length-1; last > 0; last--) { for (int i = 0; i < last; i++) { if (numbers[i] > numbers[i+1]) { // swap numbers[i] with numbers[i+1] temp = numbers[i]; numbers[i] = numbers[i+1]; numbers[i+1] = temp; } } © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 7
![Insertion Sort 16 n Algorithm basis n On pass i n Insert vi Insertion Sort (1/6) n Algorithm basis ¨ n On pass i, n Insert v[i]](https://slidetodoc.com/presentation_image_h2/c9f96522800be5d60eda148bbb1d31ca/image-8.jpg)
Insertion Sort (1/6) n Algorithm basis ¨ n On pass i, n Insert v[i] into the correct position in the sorted region to its left: v[0]…v[i-1]. Example – pass 1 (assume n = 5) Compare v[1] with v[0] ¨ If v[1] < v[0], move v[1] to the front of v[0] (shifting needed) ¨ The sorted region now consists of two elements: v[0] and v[1]. ¨ © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 8
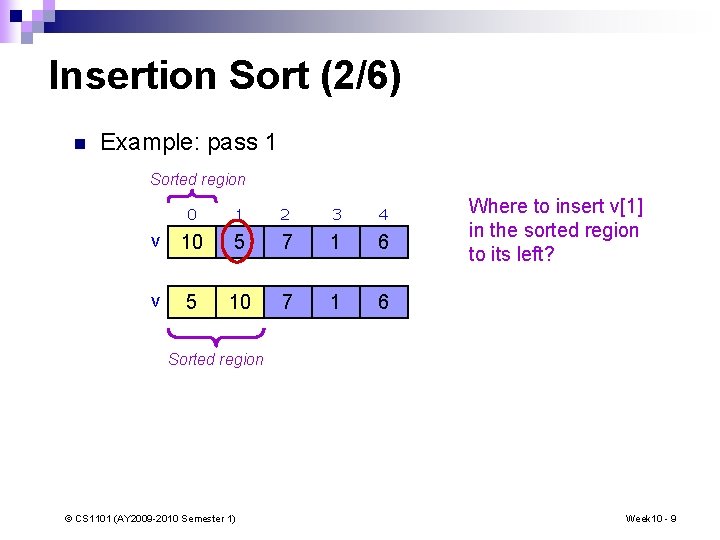
Insertion Sort (2/6) n Example: pass 1 Sorted region 0 1 2 3 4 v 10 5 7 1 6 v 5 10 7 1 6 Where to insert v[1] in the sorted region to its left? Sorted region © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 9
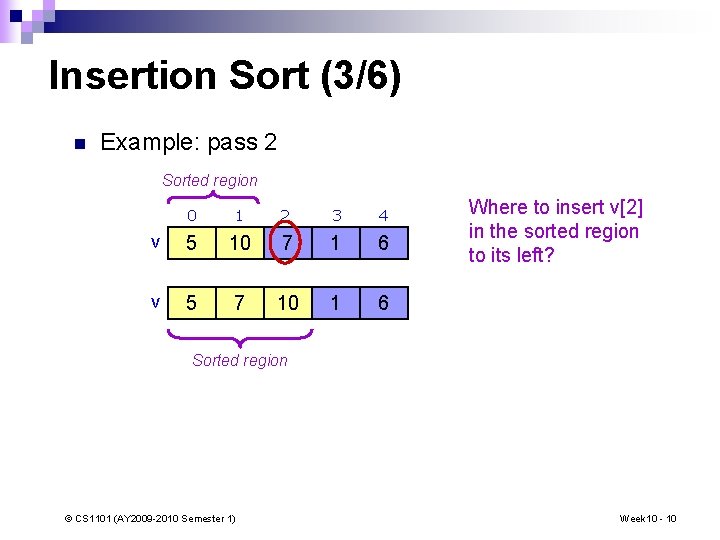
Insertion Sort (3/6) n Example: pass 2 Sorted region 0 1 2 3 4 v 5 10 7 1 6 v 5 7 10 1 6 Where to insert v[2] in the sorted region to its left? Sorted region © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 10
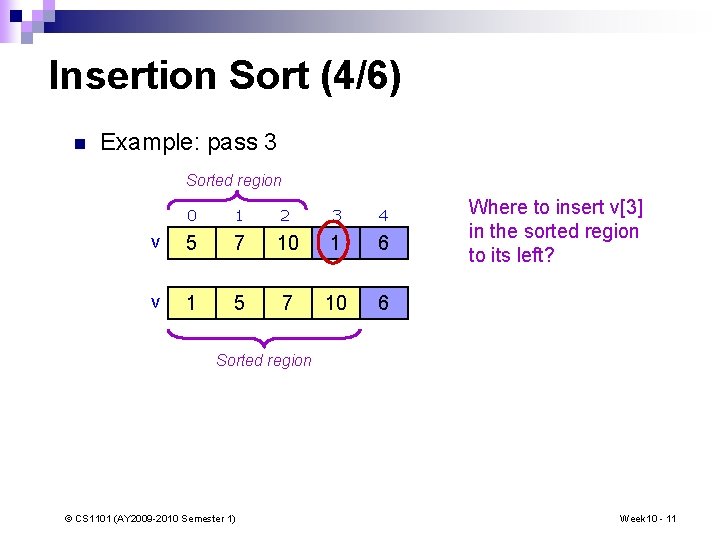
Insertion Sort (4/6) n Example: pass 3 Sorted region 0 1 2 3 4 v 5 7 10 1 6 v 1 5 7 10 6 Where to insert v[3] in the sorted region to its left? Sorted region © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 11
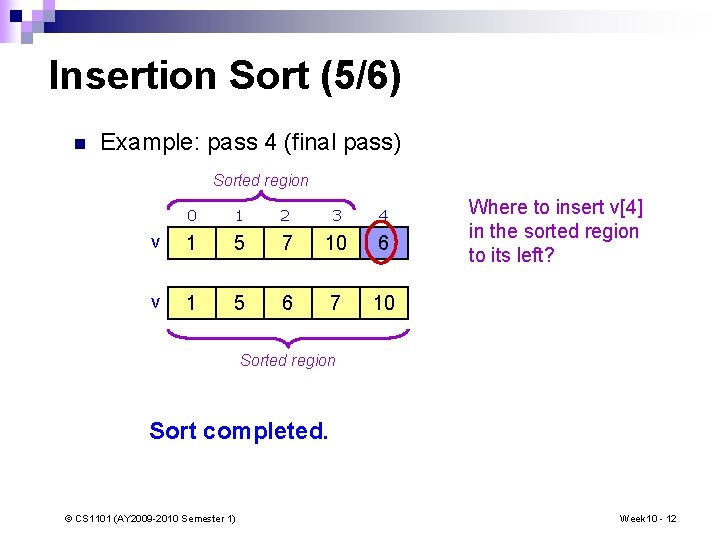
Insertion Sort (5/6) n Example: pass 4 (final pass) Sorted region 0 1 2 3 4 v 1 5 7 10 6 v 1 5 6 7 10 Where to insert v[4] in the sorted region to its left? Sorted region Sort completed. © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 12
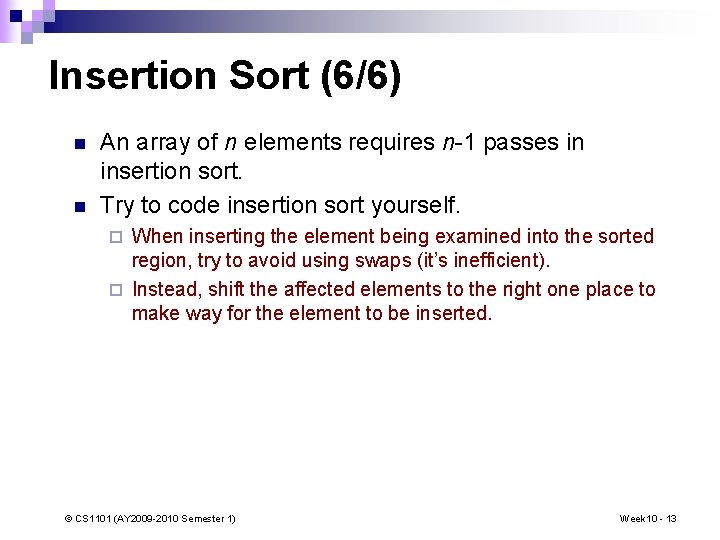
Insertion Sort (6/6) n n An array of n elements requires n-1 passes in insertion sort. Try to code insertion sort yourself. When inserting the element being examined into the sorted region, try to avoid using swaps (it’s inefficient). ¨ Instead, shift the affected elements to the right one place to make way for the element to be inserted. ¨ © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 13
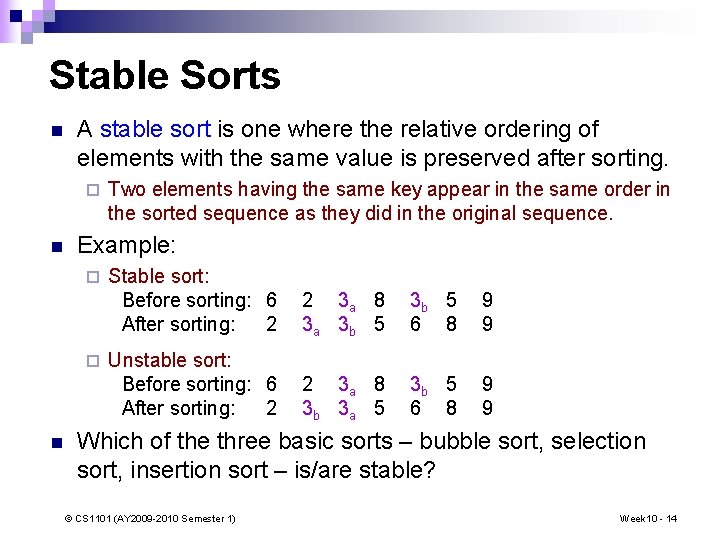
Stable Sorts n A stable sort is one where the relative ordering of elements with the same value is preserved after sorting. ¨ n Example: ¨ ¨ n Two elements having the same key appear in the same order in the sorted sequence as they did in the original sequence. Stable sort: Before sorting: 6 After sorting: 2 2 3 a 8 3 a 3 b 5 6 8 9 9 Unstable sort: Before sorting: 6 After sorting: 2 2 3 a 8 3 b 3 a 5 3 b 5 6 8 9 9 Which of the three basic sorts – bubble sort, selection sort, insertion sort – is/are stable? © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 14
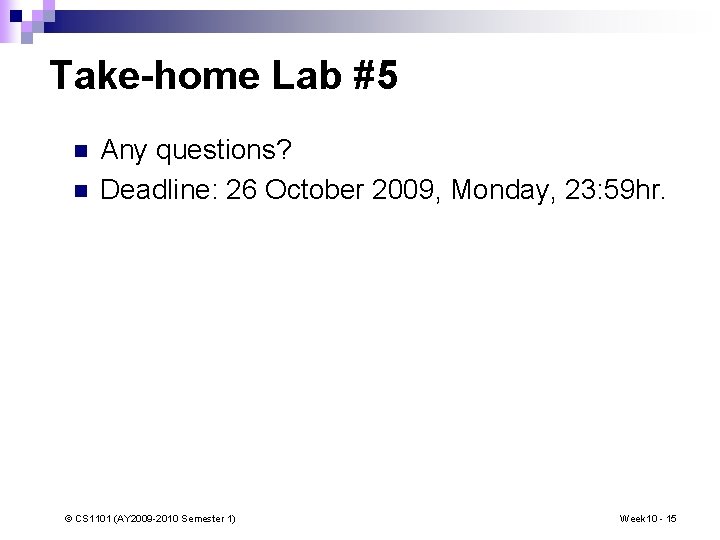
Take-home Lab #5 n n Any questions? Deadline: 26 October 2009, Monday, 23: 59 hr. © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 15
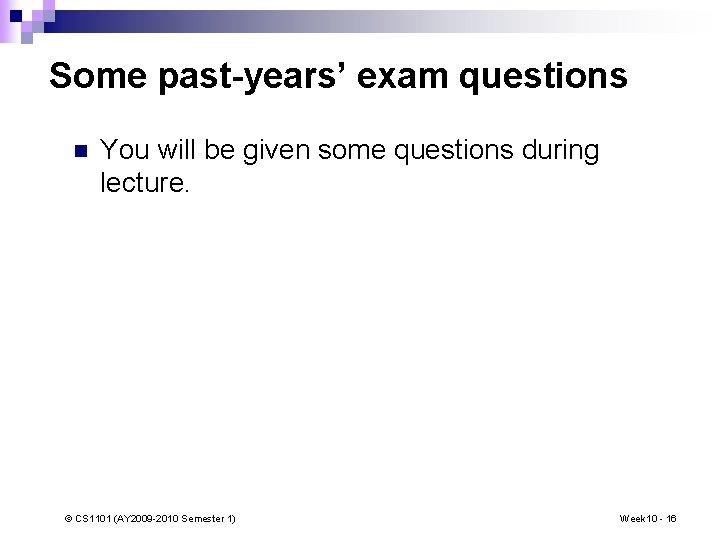
Some past-years’ exam questions n You will be given some questions during lecture. © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 16
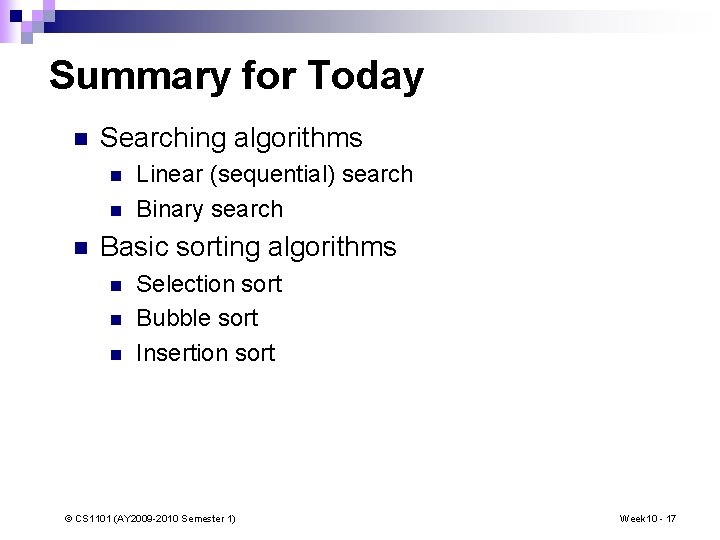
Summary for Today n Searching algorithms n n n Linear (sequential) search Binary search Basic sorting algorithms n n n Selection sort Bubble sort Insertion sort © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 17
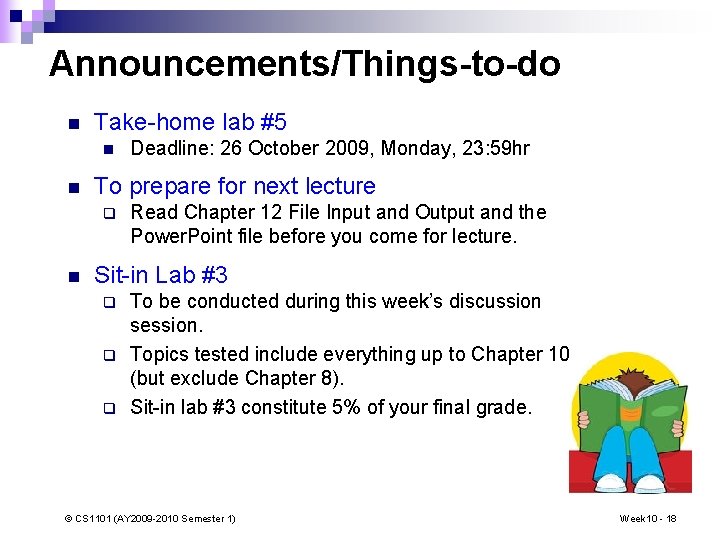
Announcements/Things-to-do n Take-home lab #5 n n To prepare for next lecture q n Deadline: 26 October 2009, Monday, 23: 59 hr Read Chapter 12 File Input and Output and the Power. Point file before you come for lecture. Sit-in Lab #3 q q q To be conducted during this week’s discussion session. Topics tested include everything up to Chapter 10 (but exclude Chapter 8). Sit-in lab #3 constitute 5% of your final grade. © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 18
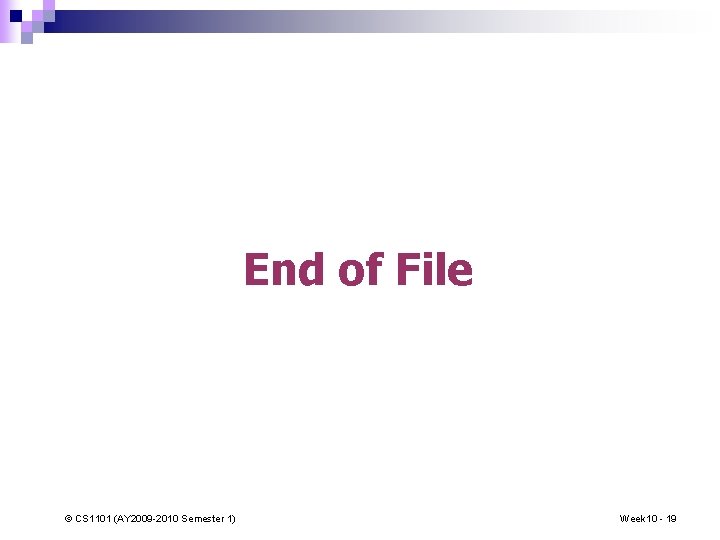
End of File © CS 1101 (AY 2009 -2010 Semester 1) Week 10 - 19
Cs 1101 final exam
Cs 1101 programming fundamentals final exam
Cs 1101 programming fundamentals final exam
Cs 1101 programming fundamentals
Cs 1101 programming fundamentals
Sequence detector 1101
29 cfr 1926 class asbestps
Uga pols 1101
Enc 1101 final exam
Sequence detector 1101
Cst 1101
11 from binary to decimal
Cst 1101
Sls 1101
Pols 1101 final exam
¿qué número decimal representa la expresión 1101?
Afi 51-1101
Enc 1101 fiu
Componentes del sintagma nominal
Cst 1101