CS 1101 Programming Methodology http www comp nus
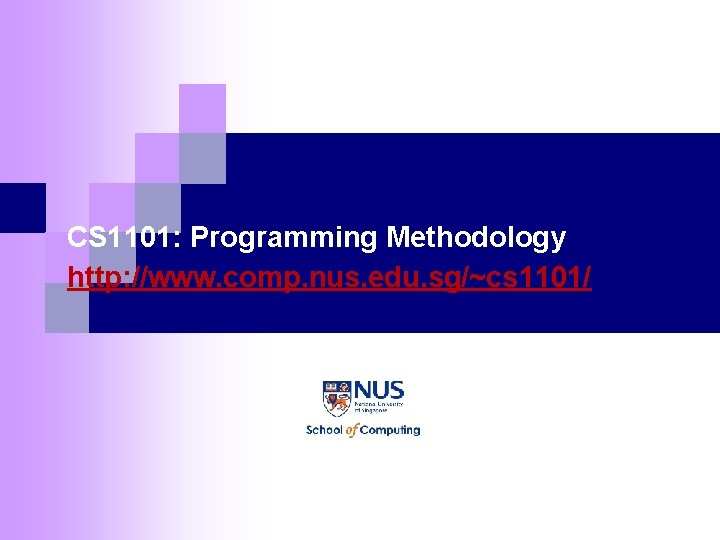
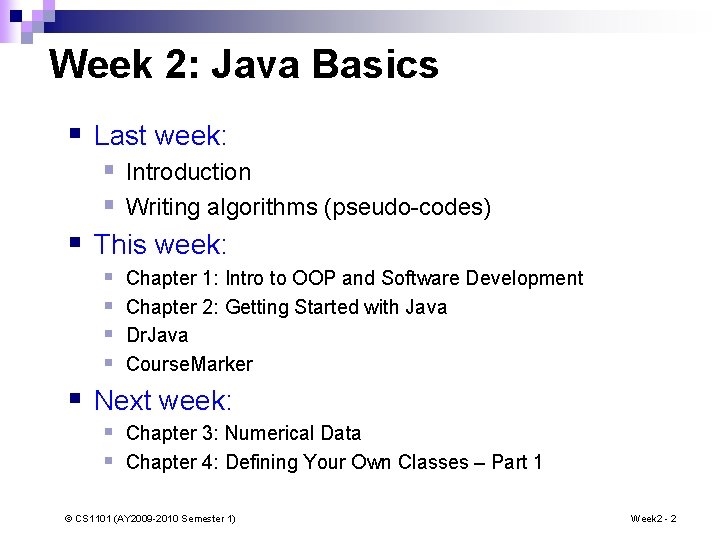
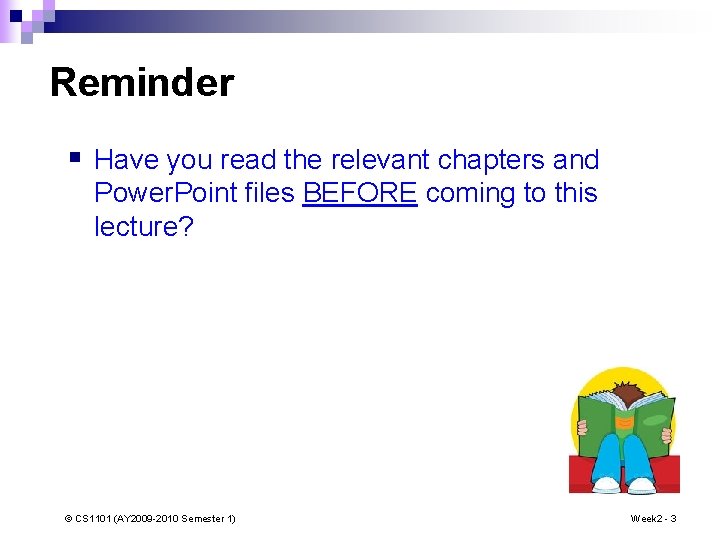
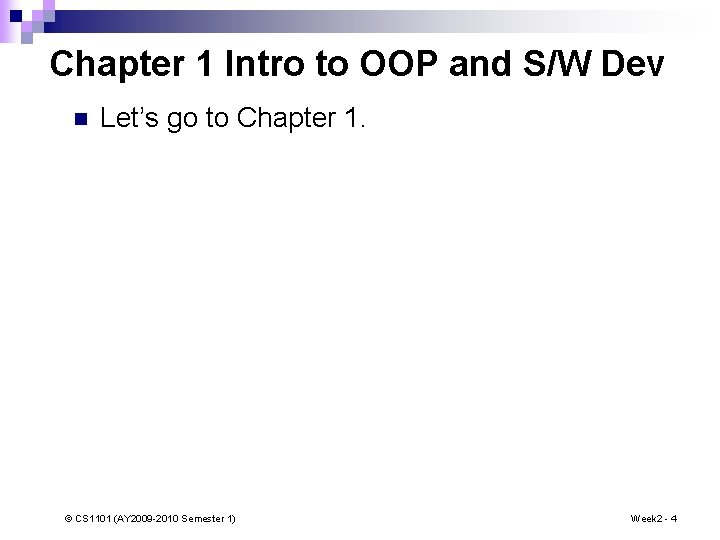
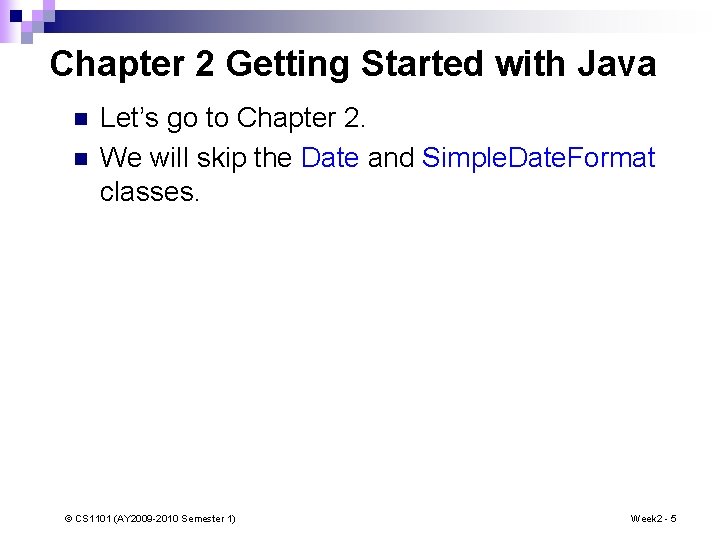
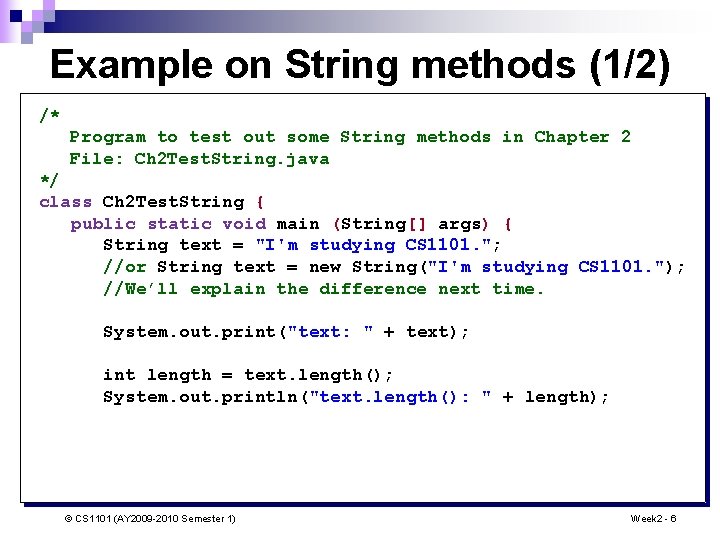
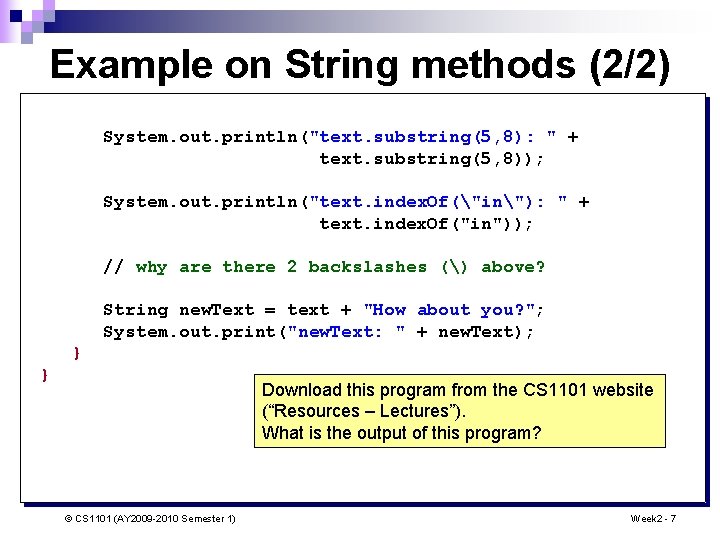
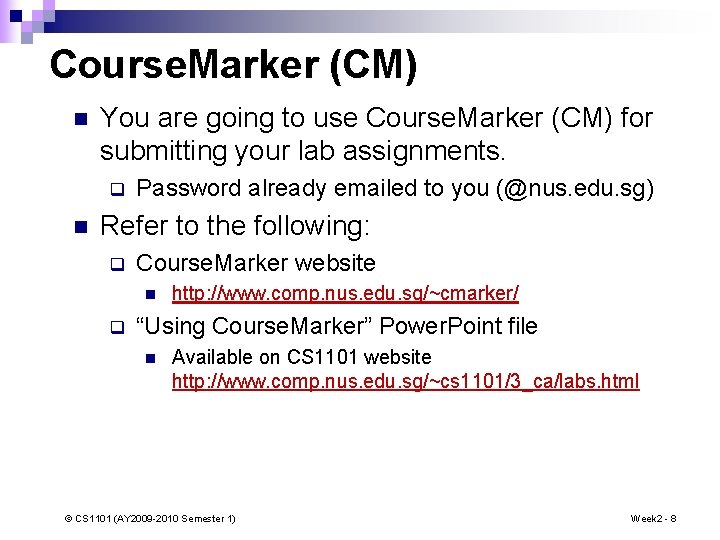
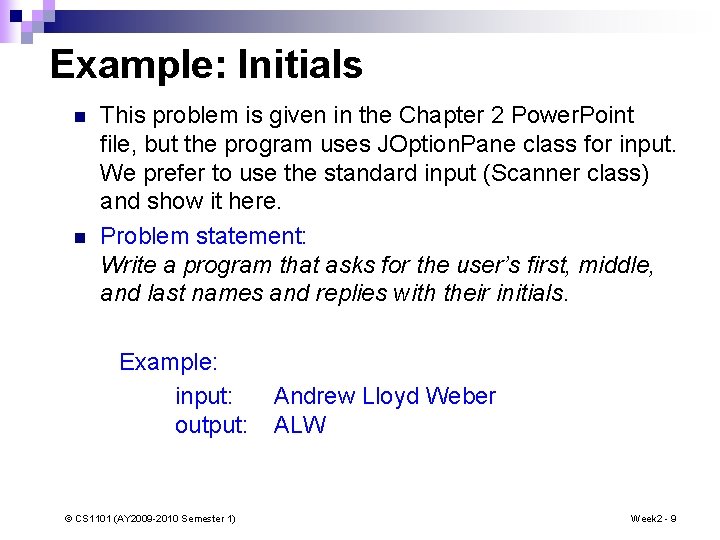
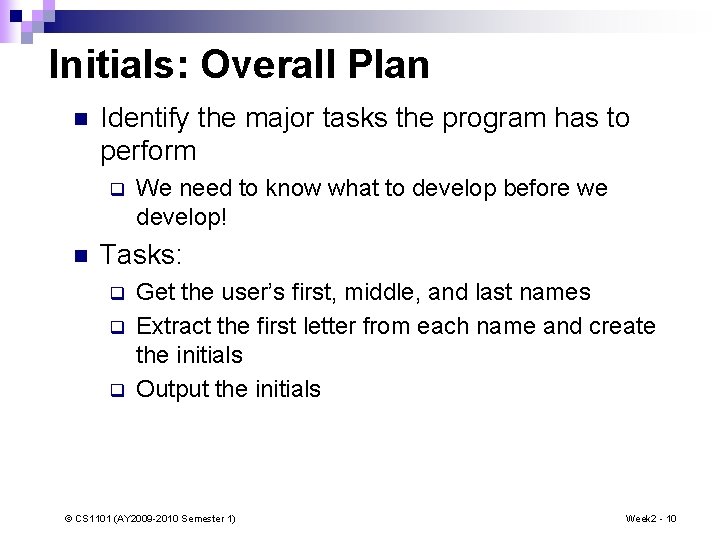
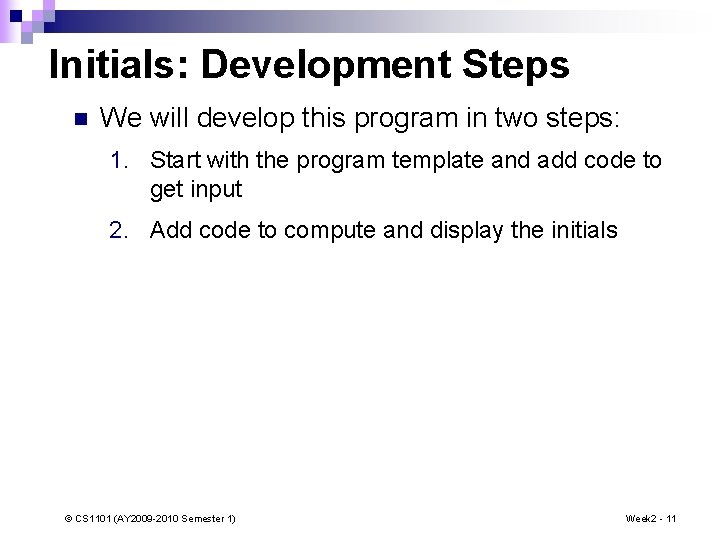
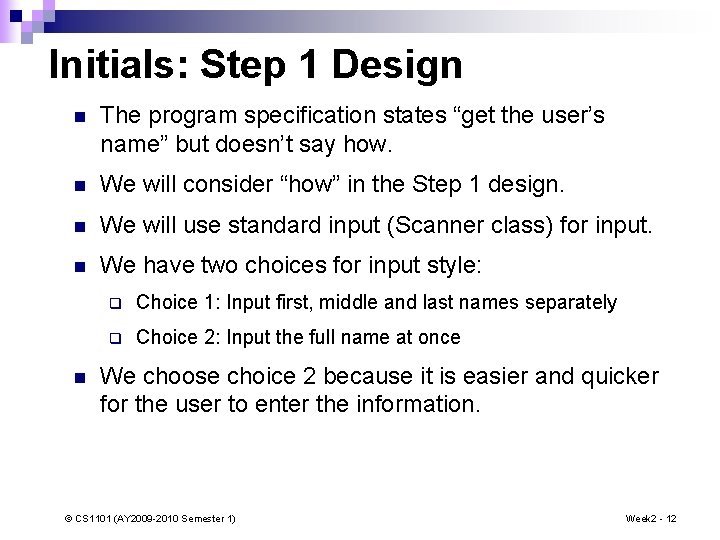
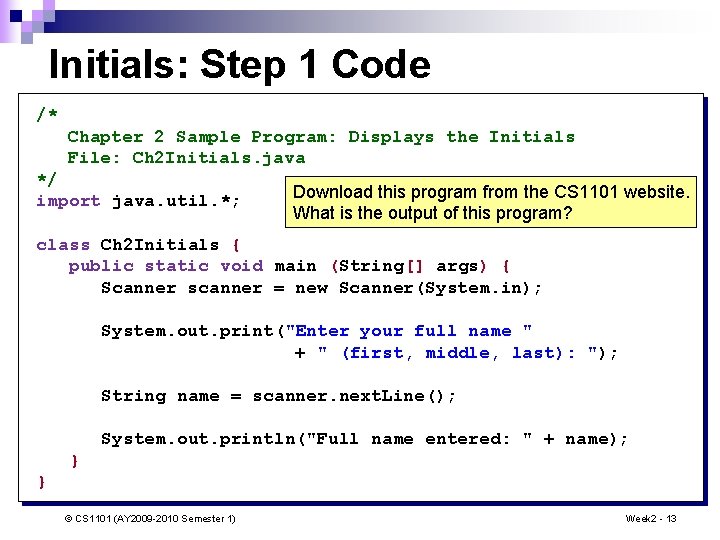
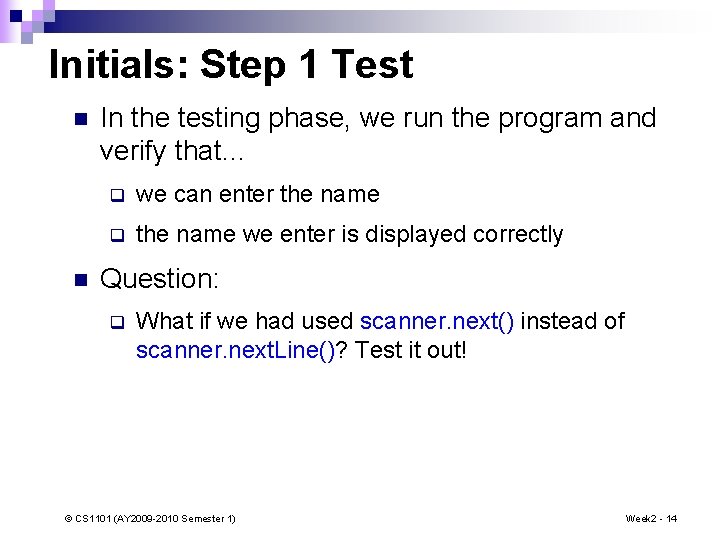
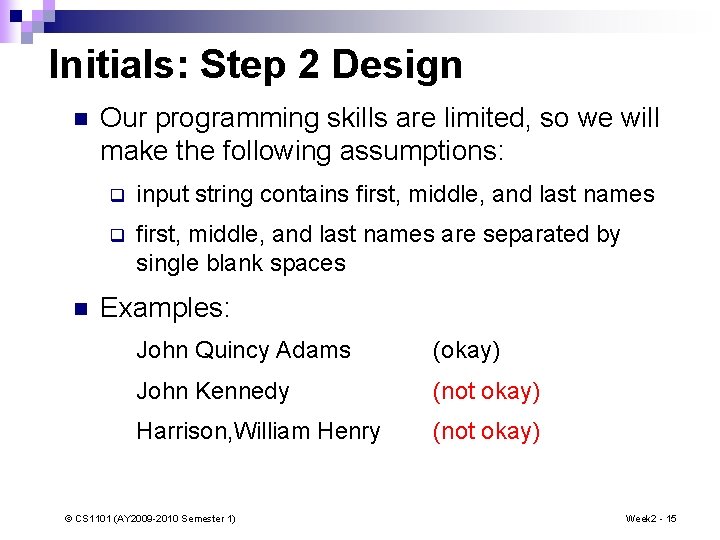
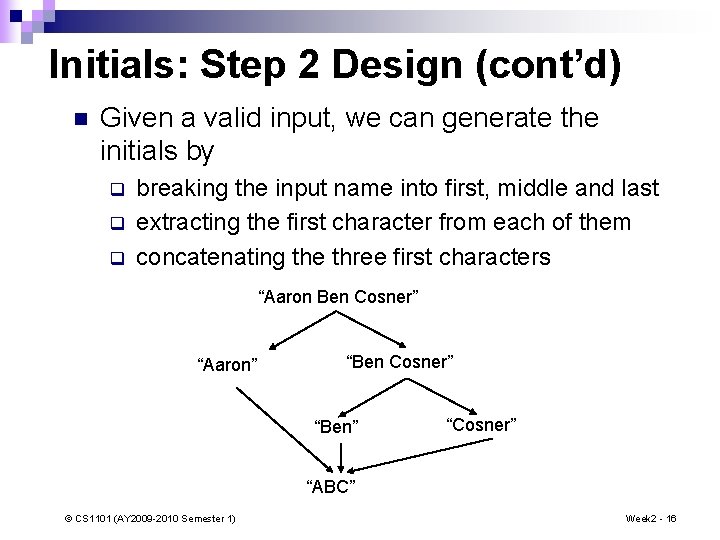
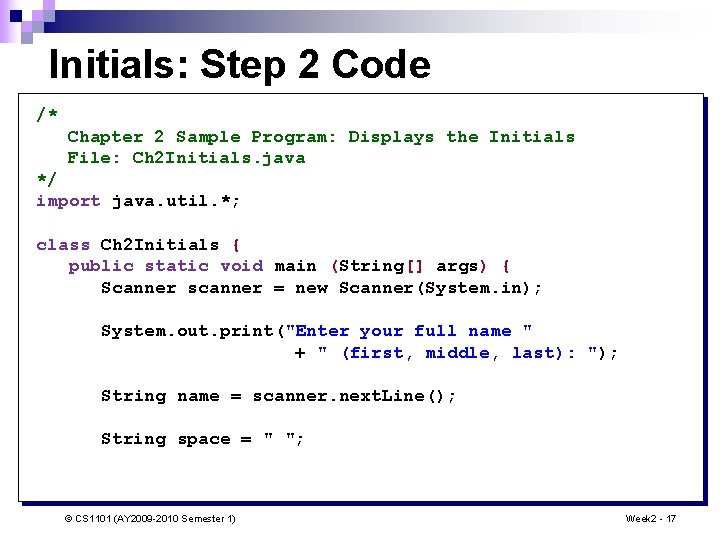
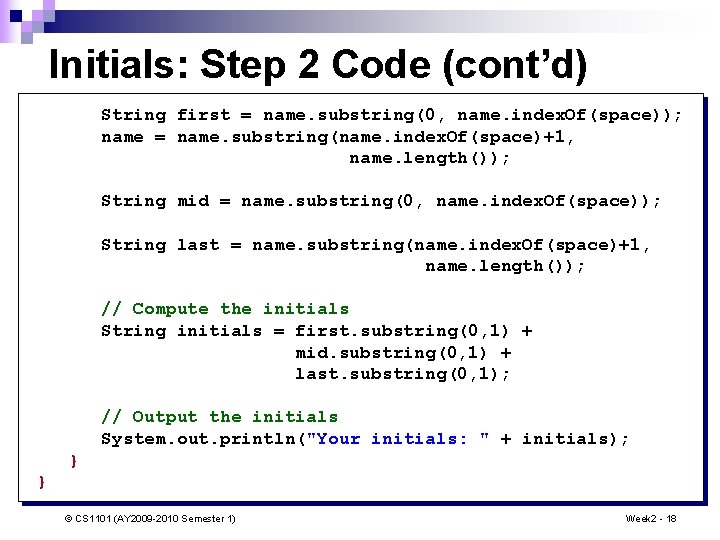
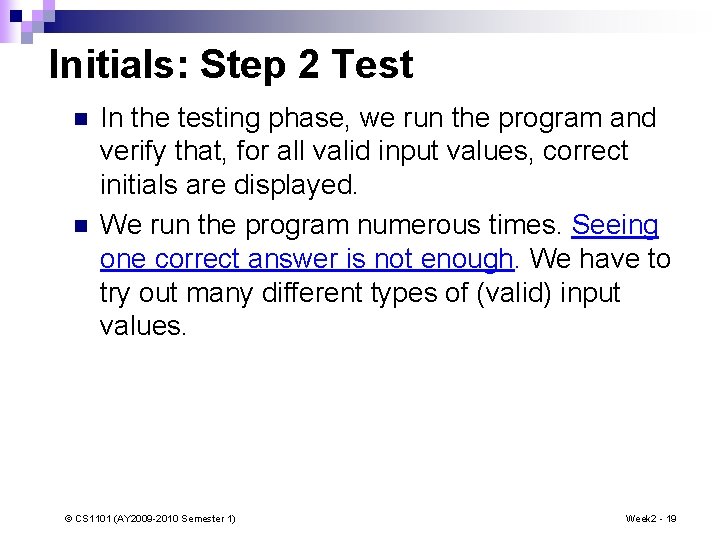
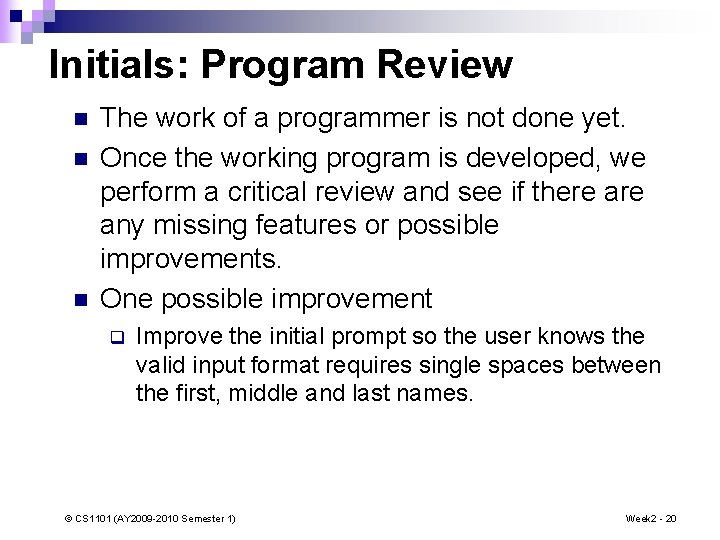
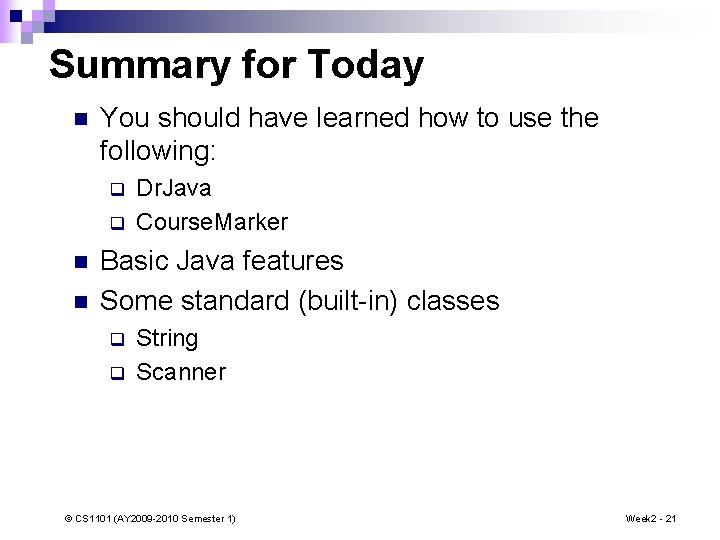
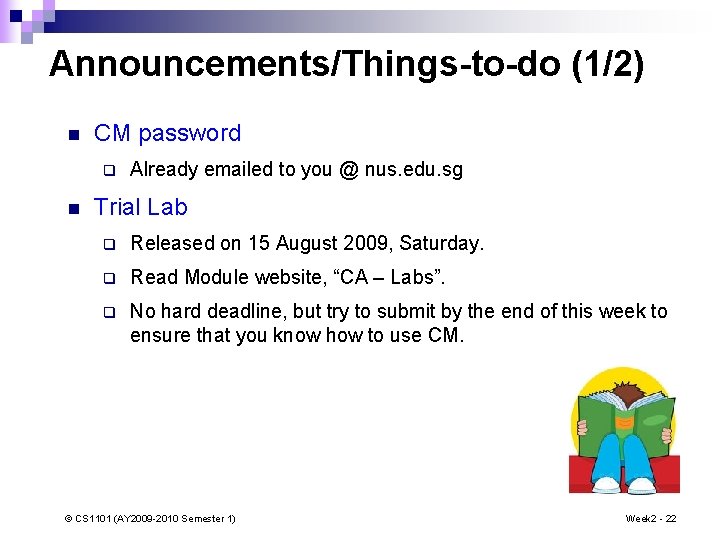
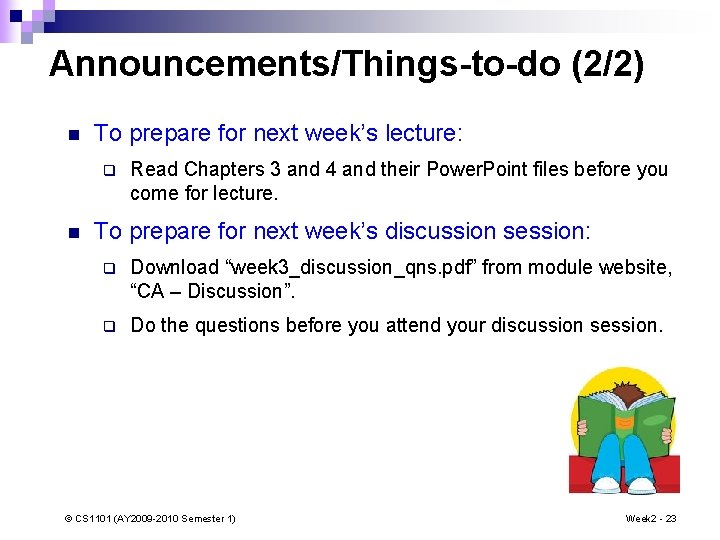
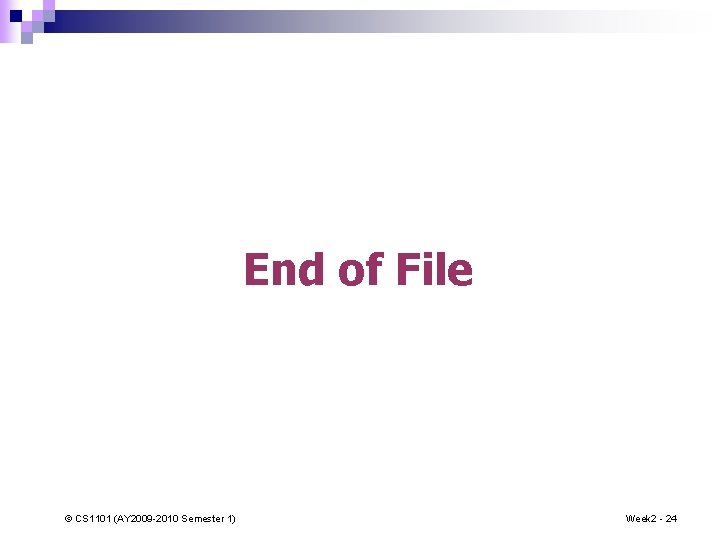
- Slides: 24
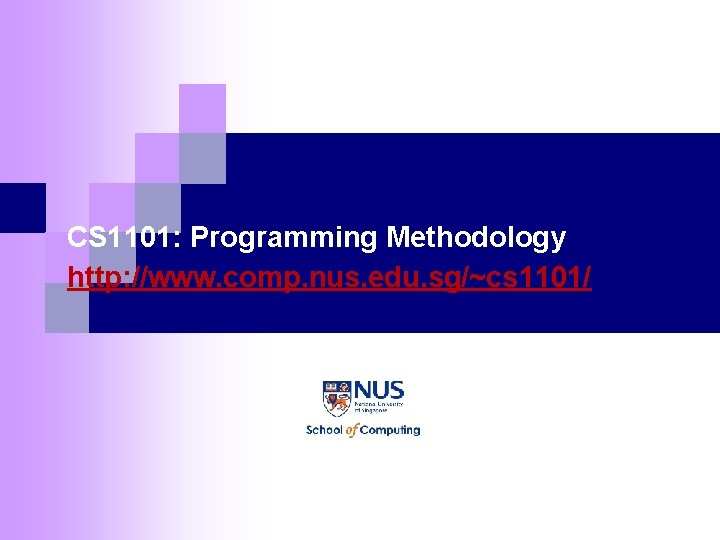
CS 1101: Programming Methodology http: //www. comp. nus. edu. sg/~cs 1101/
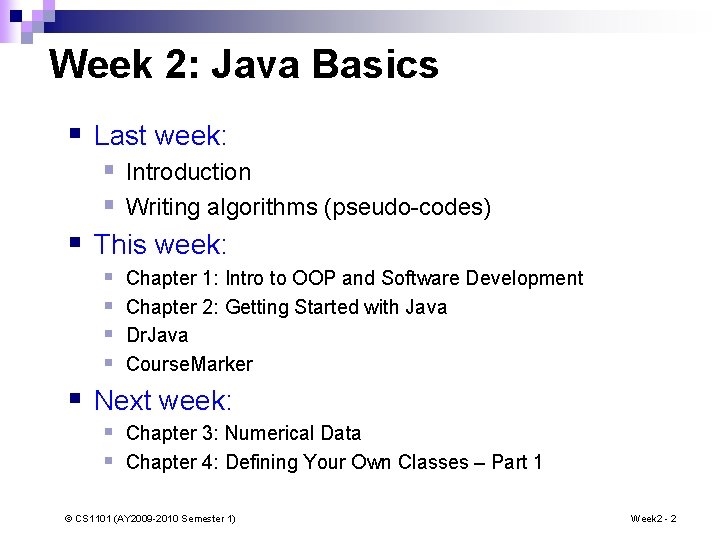
Week 2: Java Basics § Last week: § Introduction § Writing algorithms (pseudo-codes) § This week: § § Chapter 1: Intro to OOP and Software Development Chapter 2: Getting Started with Java Dr. Java Course. Marker § Next week: § Chapter 3: Numerical Data § Chapter 4: Defining Your Own Classes – Part 1 © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 2
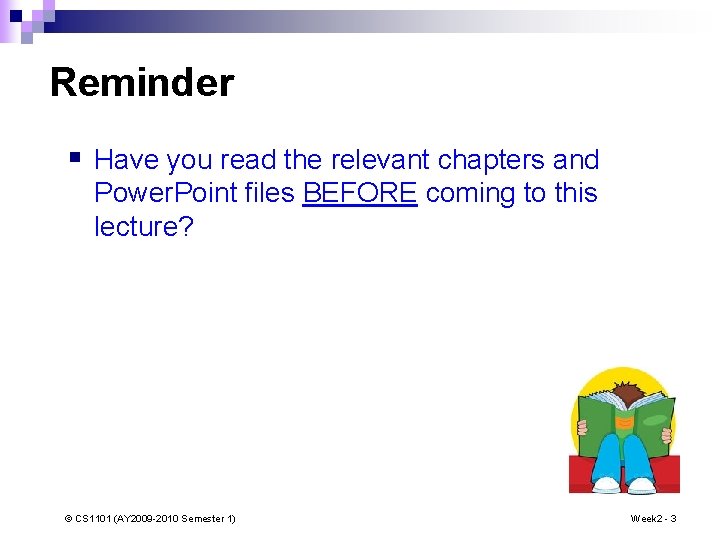
Reminder § Have you read the relevant chapters and Power. Point files BEFORE coming to this lecture? © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 3
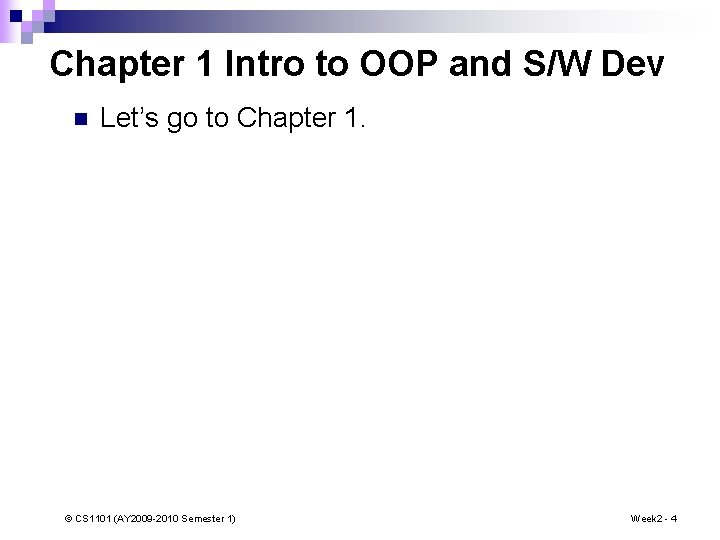
Chapter 1 Intro to OOP and S/W Dev n Let’s go to Chapter 1. © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 4
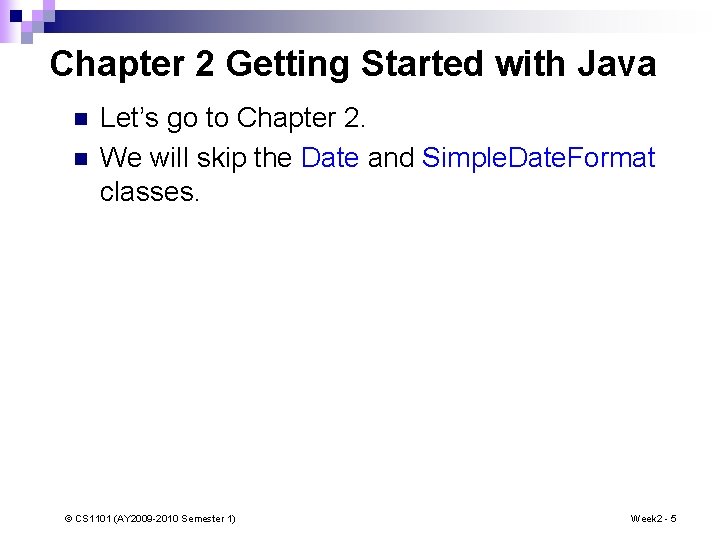
Chapter 2 Getting Started with Java n n Let’s go to Chapter 2. We will skip the Date and Simple. Date. Format classes. © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 5
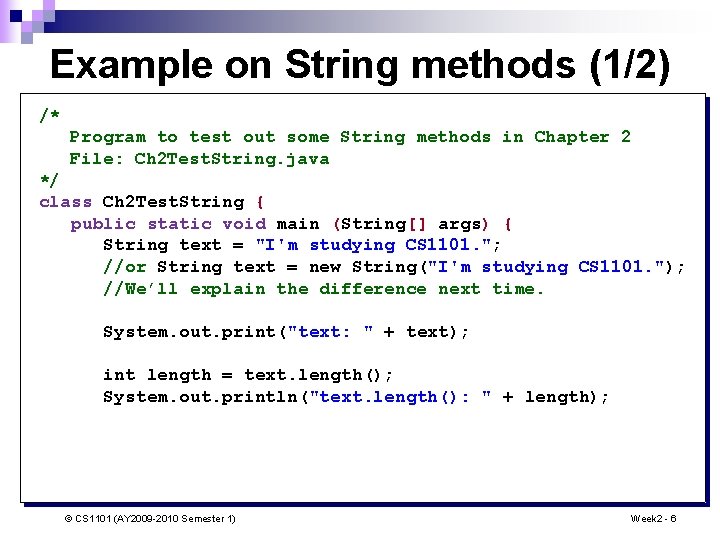
Example on String methods (1/2) /* Program to test out some String methods in Chapter 2 File: Ch 2 Test. String. java */ class Ch 2 Test. String { public static void main (String[] args) { String text = "I'm studying CS 1101. "; //or String text = new String("I'm studying CS 1101. "); //We’ll explain the difference next time. System. out. print("text: " + text); int length = text. length(); System. out. println("text. length(): " + length); © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 6
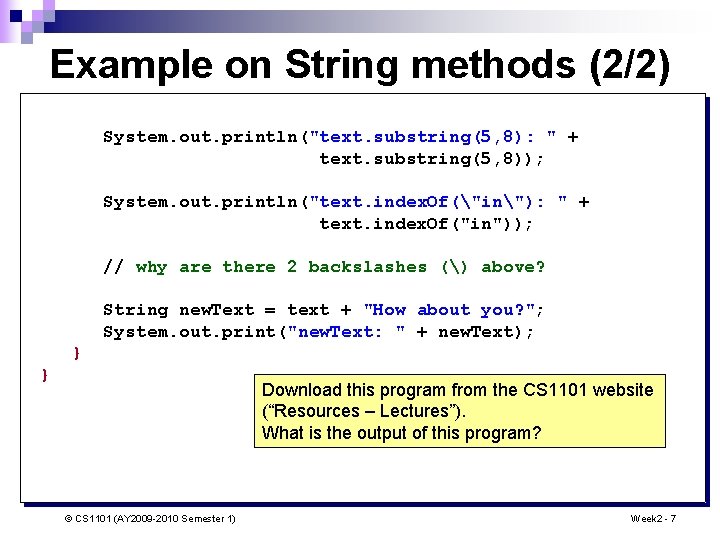
Example on String methods (2/2) System. out. println("text. substring(5, 8): " + text. substring(5, 8)); System. out. println("text. index. Of("in"): " + text. index. Of("in")); // why are there 2 backslashes () above? String new. Text = text + "How about you? "; System. out. print("new. Text: " + new. Text); } } Download this program from the CS 1101 website (“Resources – Lectures”). What is the output of this program? © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 7
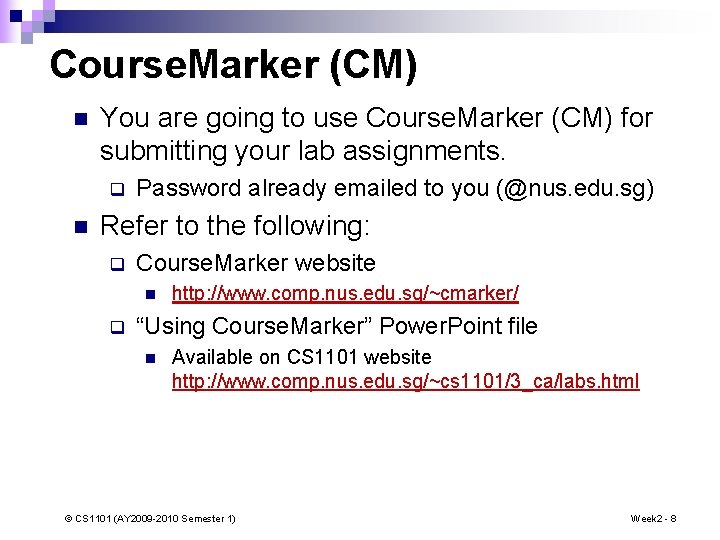
Course. Marker (CM) n You are going to use Course. Marker (CM) for submitting your lab assignments. q n Password already emailed to you (@nus. edu. sg) Refer to the following: q Course. Marker website n q http: //www. comp. nus. edu. sg/~cmarker/ “Using Course. Marker” Power. Point file n Available on CS 1101 website http: //www. comp. nus. edu. sg/~cs 1101/3_ca/labs. html © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 8
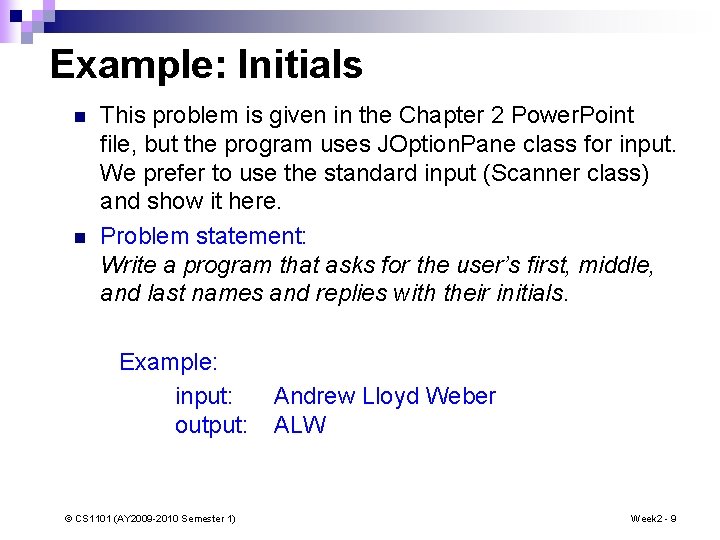
Example: Initials n n This problem is given in the Chapter 2 Power. Point file, but the program uses JOption. Pane class for input. We prefer to use the standard input (Scanner class) and show it here. Problem statement: Write a program that asks for the user’s first, middle, and last names and replies with their initials. Example: input: output: © CS 1101 (AY 2009 -2010 Semester 1) Andrew Lloyd Weber ALW Week 2 - 9
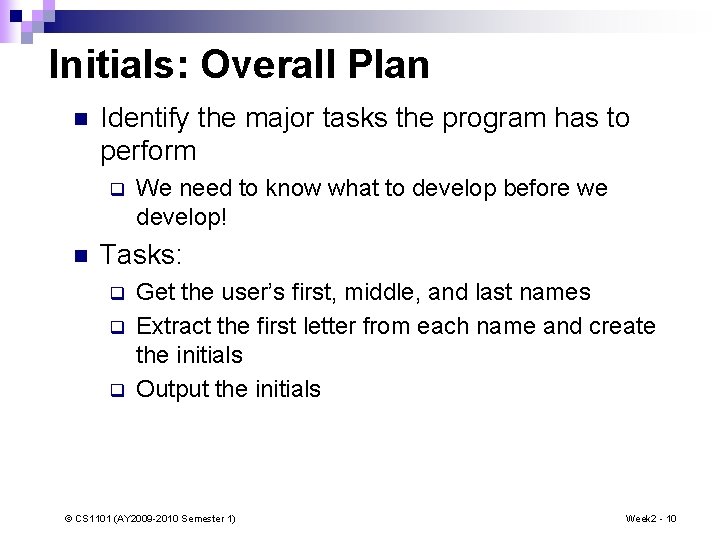
Initials: Overall Plan n Identify the major tasks the program has to perform q n We need to know what to develop before we develop! Tasks: q q q Get the user’s first, middle, and last names Extract the first letter from each name and create the initials Output the initials © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 10
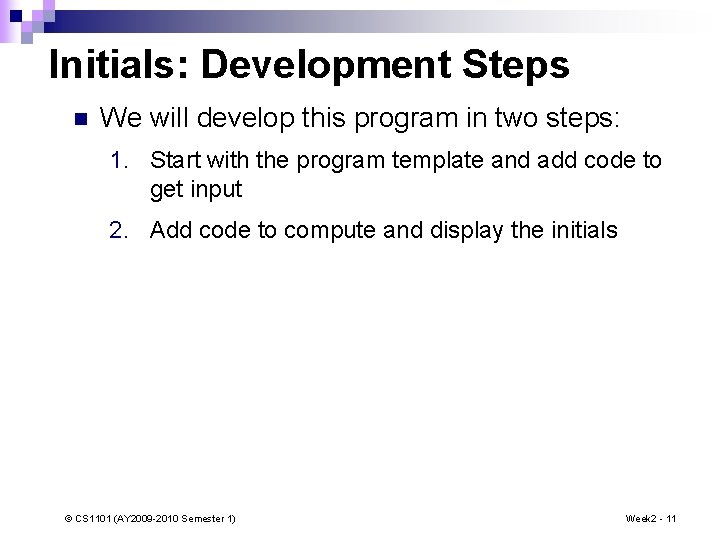
Initials: Development Steps n We will develop this program in two steps: 1. Start with the program template and add code to get input 2. Add code to compute and display the initials © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 11
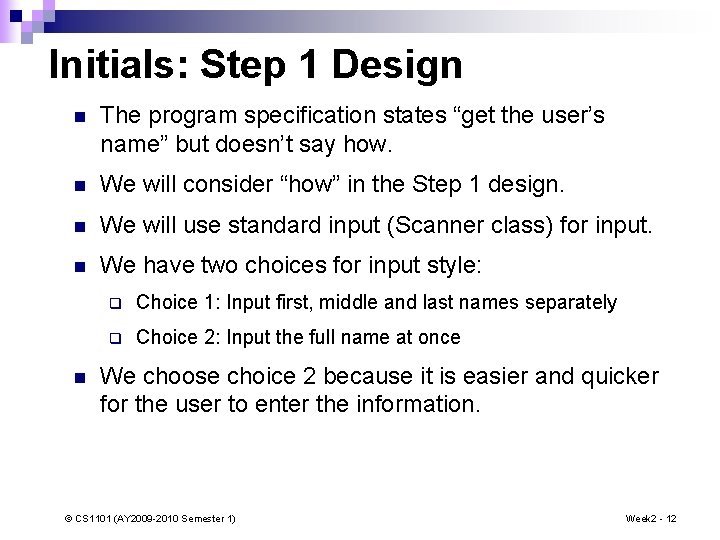
Initials: Step 1 Design n The program specification states “get the user’s name” but doesn’t say how. n We will consider “how” in the Step 1 design. n We will use standard input (Scanner class) for input. n We have two choices for input style: n q Choice 1: Input first, middle and last names separately q Choice 2: Input the full name at once We choose choice 2 because it is easier and quicker for the user to enter the information. © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 12
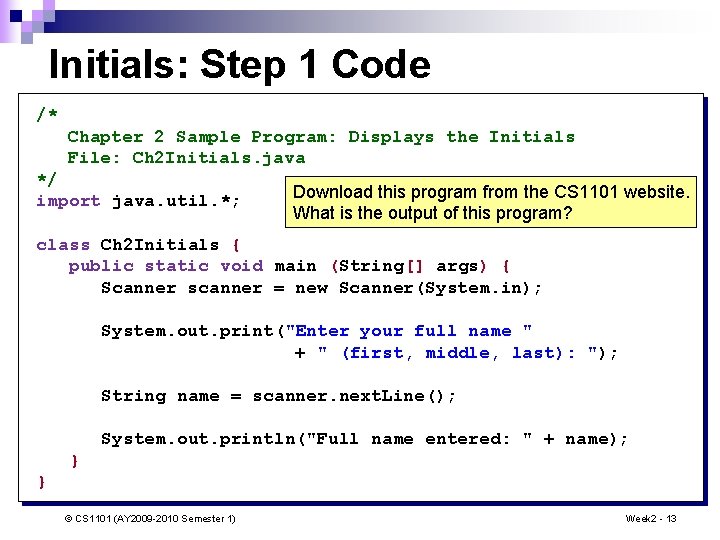
Initials: Step 1 Code /* Chapter 2 Sample Program: Displays the Initials File: Ch 2 Initials. java */ import java. util. *; Download this program from the CS 1101 website. What is the output of this program? class Ch 2 Initials { public static void main (String[] args) { Scanner scanner = new Scanner(System. in); System. out. print("Enter your full name " + " (first, middle, last): "); String name = scanner. next. Line(); System. out. println("Full name entered: " + name); } } © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 13
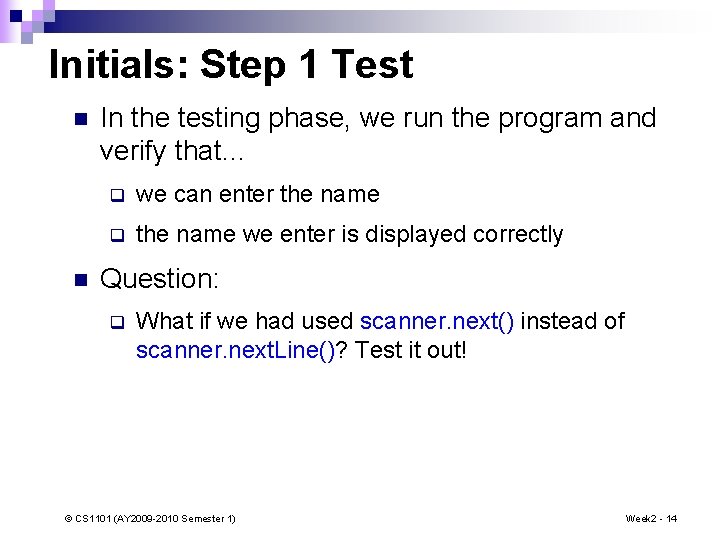
Initials: Step 1 Test n n In the testing phase, we run the program and verify that… q we can enter the name q the name we enter is displayed correctly Question: q What if we had used scanner. next() instead of scanner. next. Line()? Test it out! © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 14
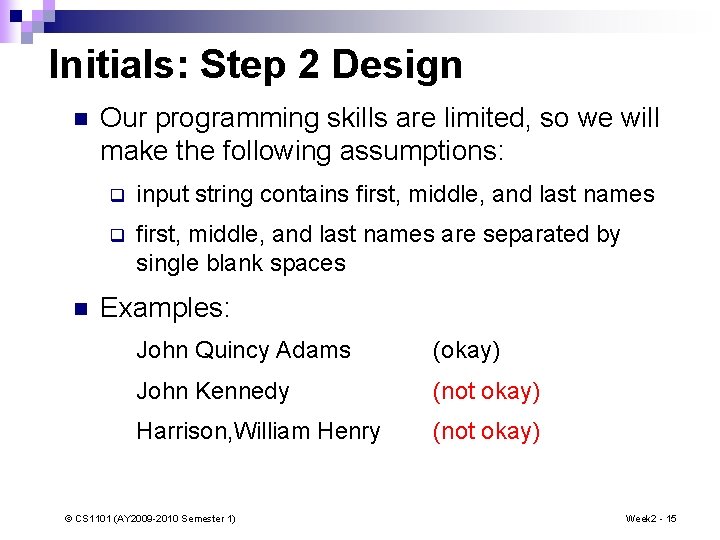
Initials: Step 2 Design n n Our programming skills are limited, so we will make the following assumptions: q input string contains first, middle, and last names q first, middle, and last names are separated by single blank spaces Examples: John Quincy Adams (okay) John Kennedy (not okay) Harrison, William Henry (not okay) © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 15
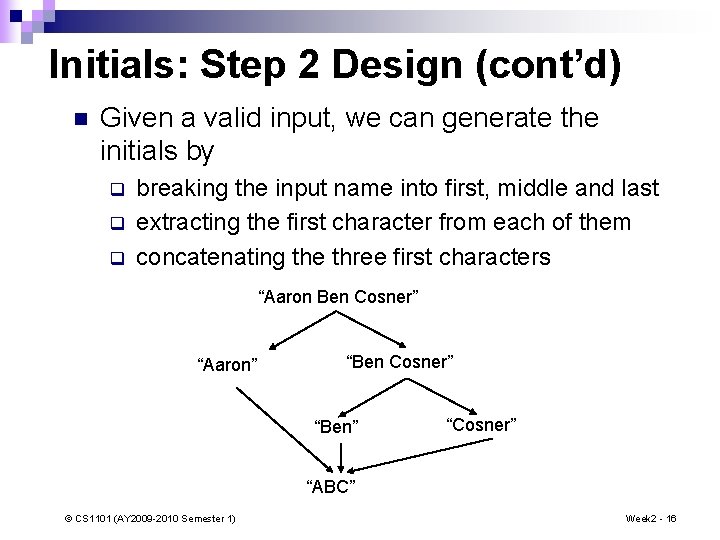
Initials: Step 2 Design (cont’d) n Given a valid input, we can generate the initials by q q q breaking the input name into first, middle and last extracting the first character from each of them concatenating the three first characters “Aaron Ben Cosner” “Aaron” “Ben Cosner” “Ben” “Cosner” “ABC” © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 16
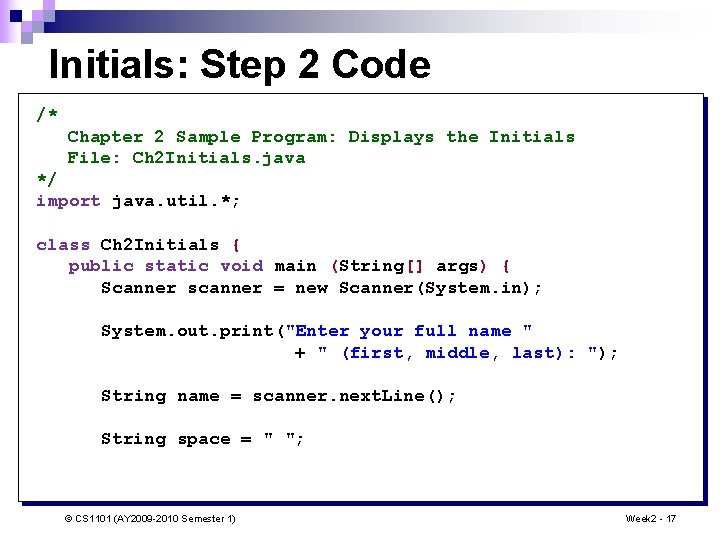
Initials: Step 2 Code /* Chapter 2 Sample Program: Displays the Initials File: Ch 2 Initials. java */ import java. util. *; class Ch 2 Initials { public static void main (String[] args) { Scanner scanner = new Scanner(System. in); System. out. print("Enter your full name " + " (first, middle, last): "); String name = scanner. next. Line(); String space = " "; © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 17
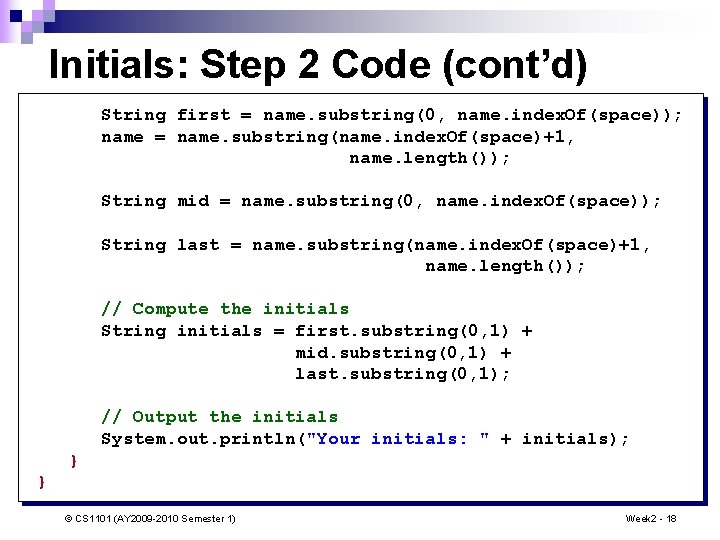
Initials: Step 2 Code (cont’d) String first = name. substring(0, name. index. Of(space)); name = name. substring(name. index. Of(space)+1, name. length()); String mid = name. substring(0, name. index. Of(space)); String last = name. substring(name. index. Of(space)+1, name. length()); // Compute the initials String initials = first. substring(0, 1) + mid. substring(0, 1) + last. substring(0, 1); // Output the initials System. out. println("Your initials: " + initials); } } © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 18
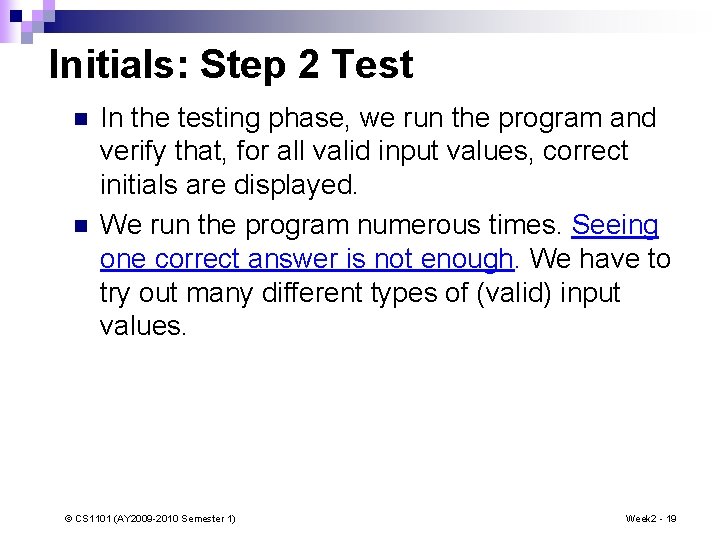
Initials: Step 2 Test n n In the testing phase, we run the program and verify that, for all valid input values, correct initials are displayed. We run the program numerous times. Seeing one correct answer is not enough. We have to try out many different types of (valid) input values. © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 19
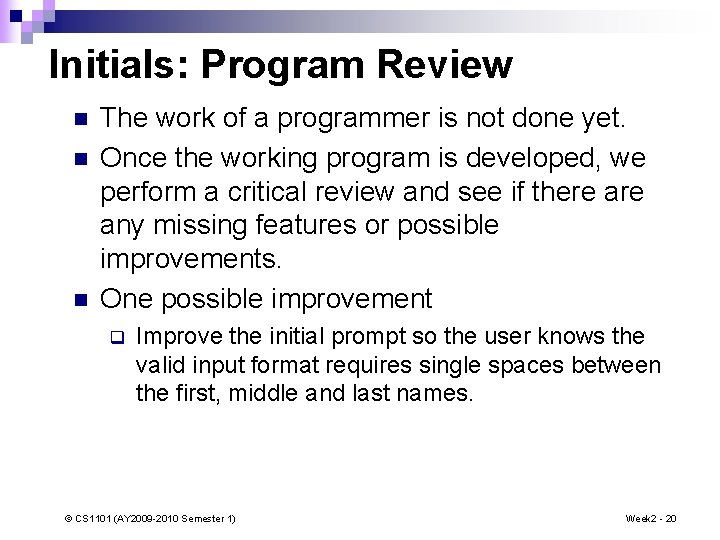
Initials: Program Review n n n The work of a programmer is not done yet. Once the working program is developed, we perform a critical review and see if there any missing features or possible improvements. One possible improvement q Improve the initial prompt so the user knows the valid input format requires single spaces between the first, middle and last names. © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 20
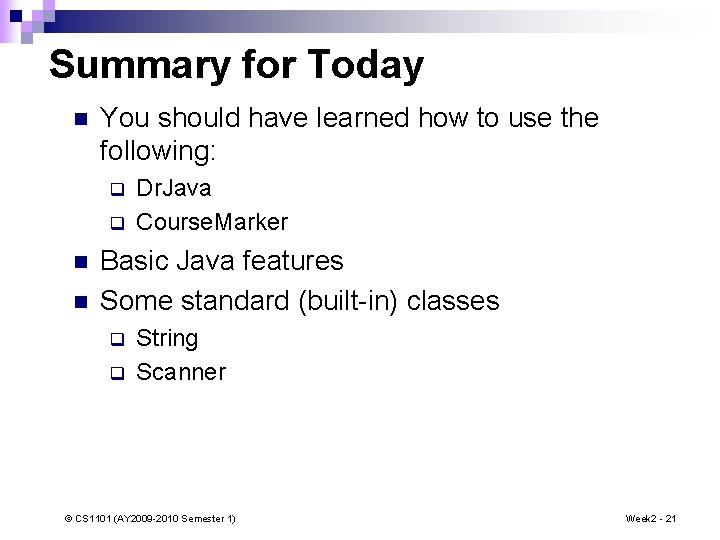
Summary for Today n You should have learned how to use the following: q q n n Dr. Java Course. Marker Basic Java features Some standard (built-in) classes q q String Scanner © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 21
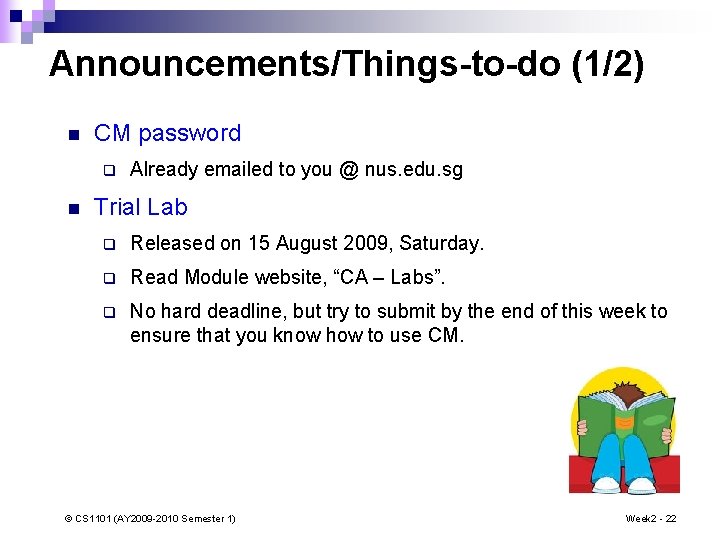
Announcements/Things-to-do (1/2) n CM password q n Already emailed to you @ nus. edu. sg Trial Lab q Released on 15 August 2009, Saturday. q Read Module website, “CA – Labs”. q No hard deadline, but try to submit by the end of this week to ensure that you know how to use CM. © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 22
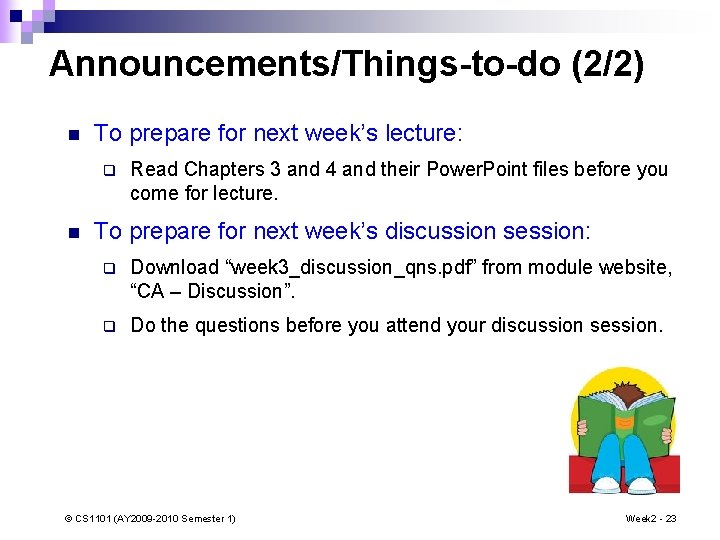
Announcements/Things-to-do (2/2) n To prepare for next week’s lecture: q n Read Chapters 3 and 4 and their Power. Point files before you come for lecture. To prepare for next week’s discussion session: q Download “week 3_discussion_qns. pdf” from module website, “CA – Discussion”. q Do the questions before you attend your discussion session. © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 23
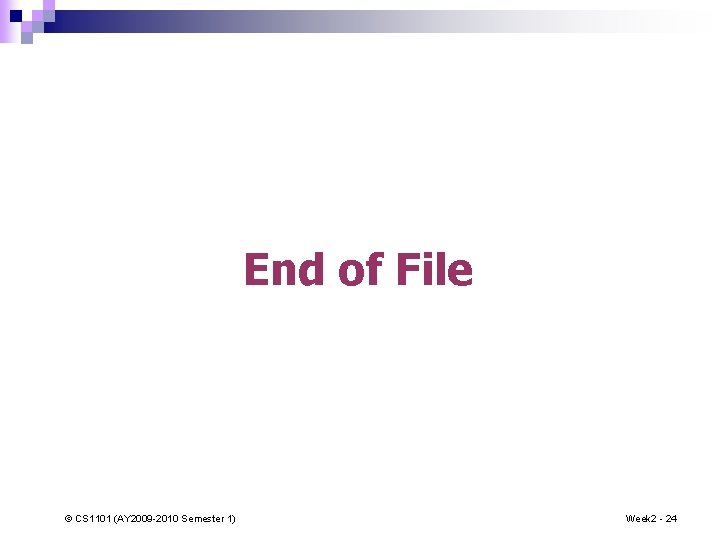
End of File © CS 1101 (AY 2009 -2010 Semester 1) Week 2 - 24
Cs1101
Fundamentals of patrolling
Cs 1101 final
Cs 1101 programming fundamentals
Cs 1101 programming fundamentals
Sequence detector 1101
29cfr1926.1101
Shanshan lian uga
Enc 1101 final exam
1101 sequence detector using mealy
Cst 1101
11 from binary to decimal
Cst 1101
Sls 1101
Pols 1101 final exam study guide
¿qué número decimal representa la expresión 1101?
Afi 51-1101
Enc 1101 fiu
Complementos circunstanciales
Flowgarithm
Cs1101s practical assessment
Zastępcze elementy geometryczne
1010 1101
Cs 1101 final
Jfdi academy