COSC 3306 Programming Paradigms Lecture 3 Design Specifications
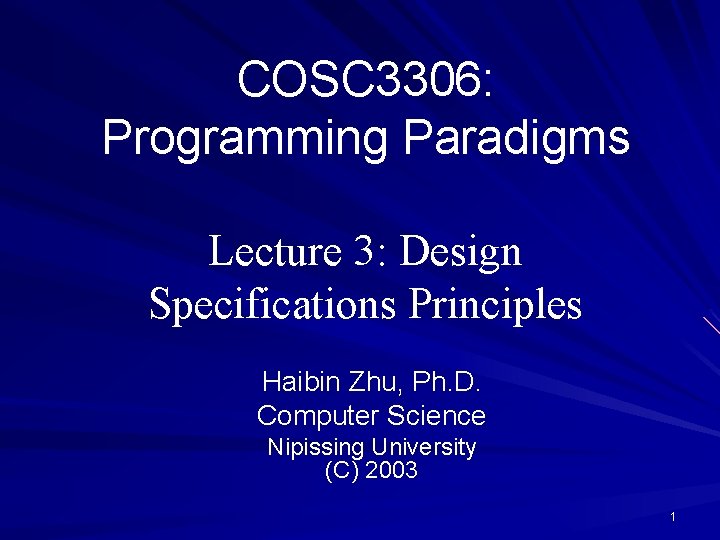
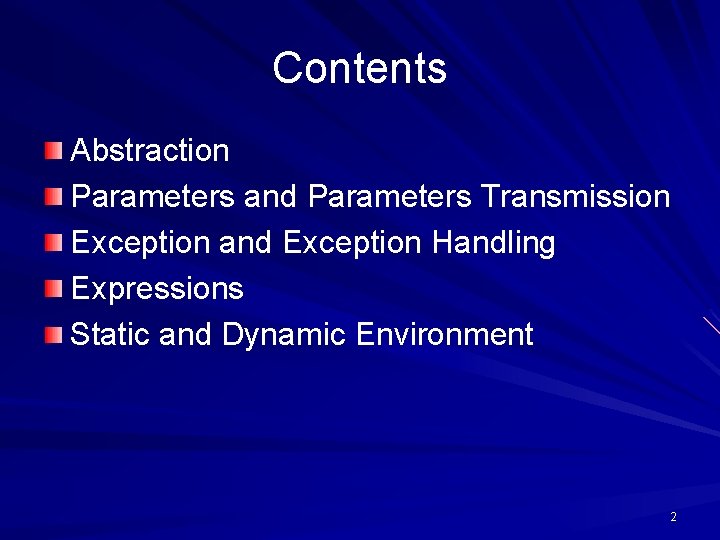
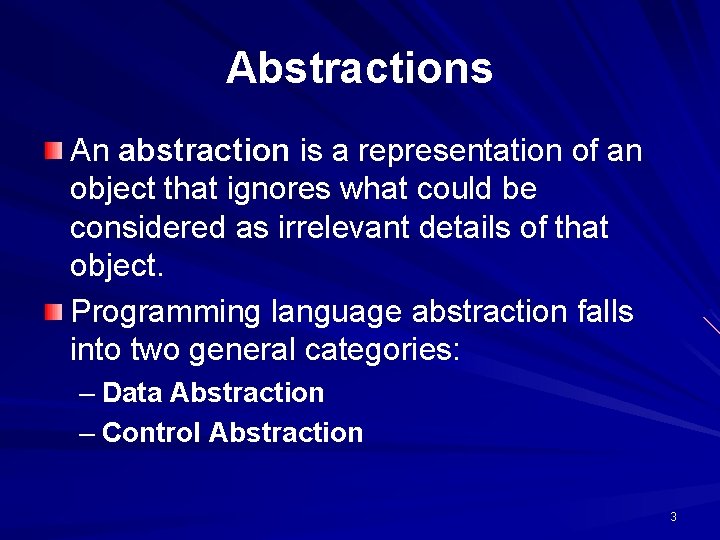
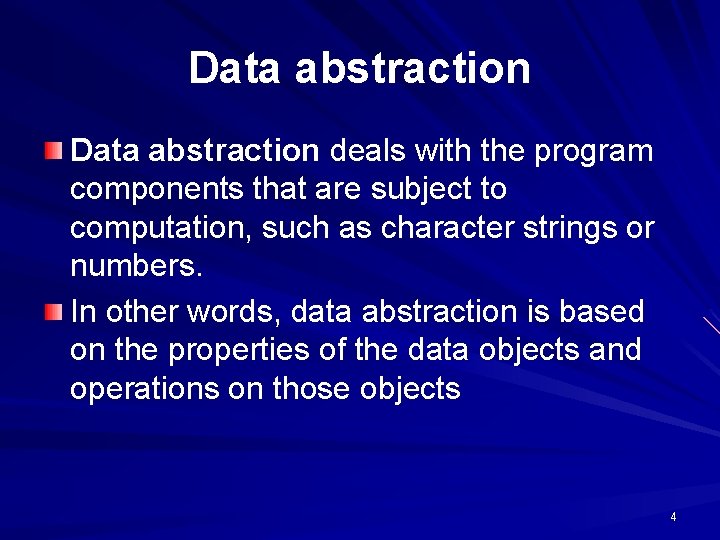
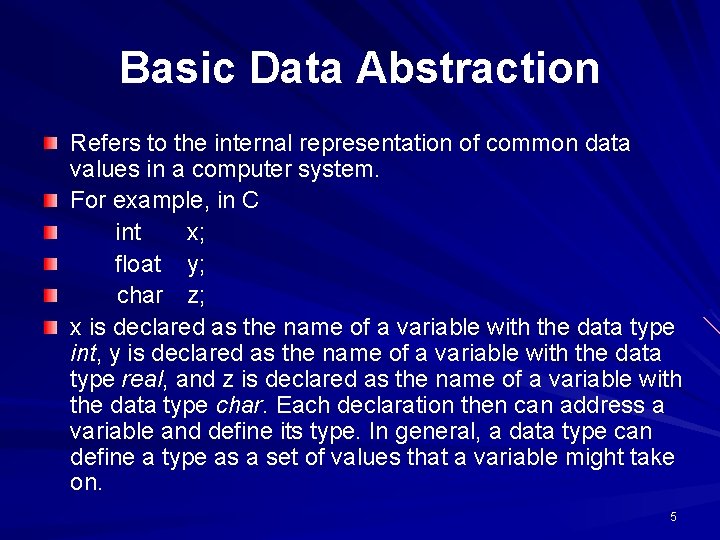
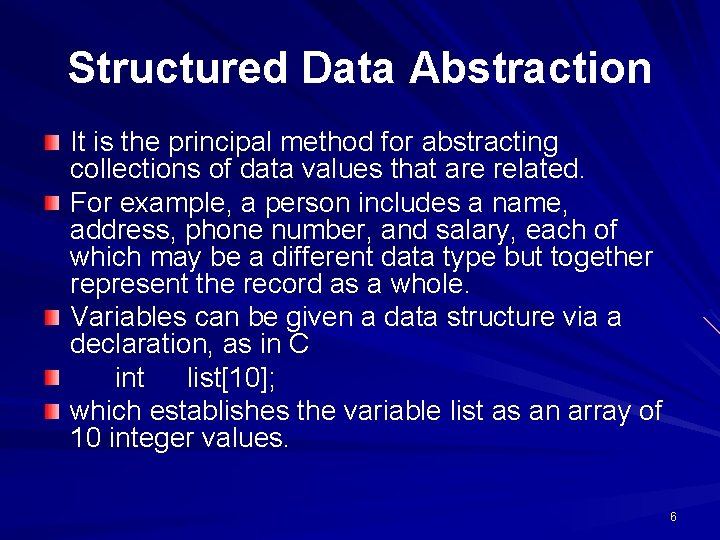
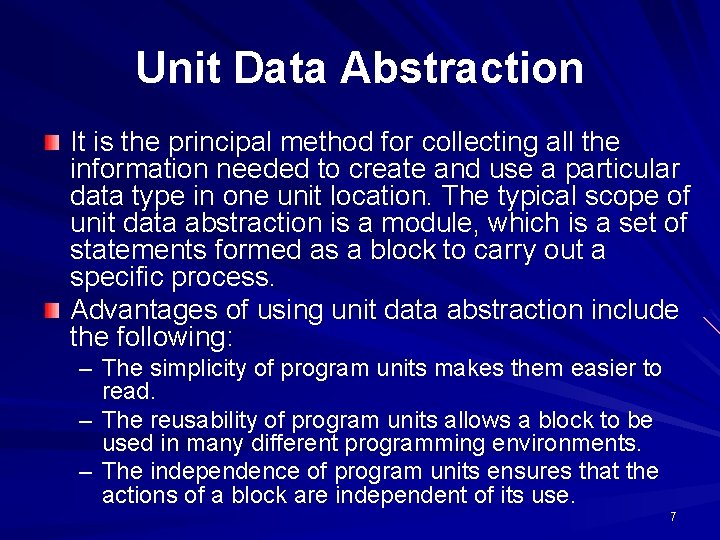
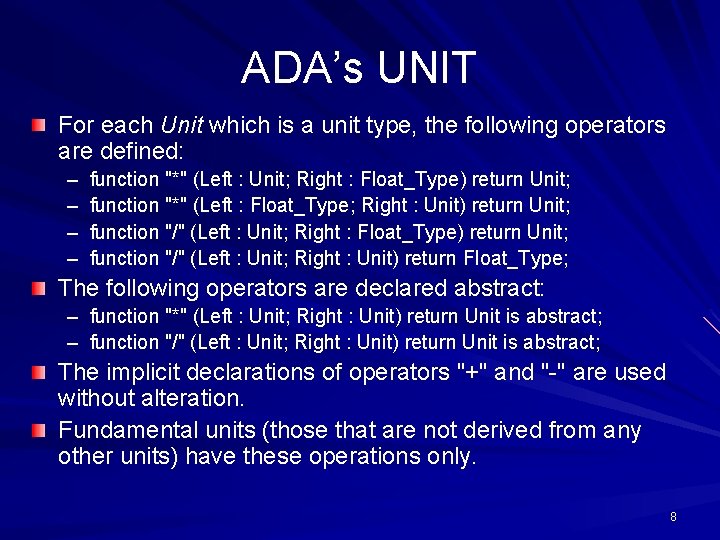
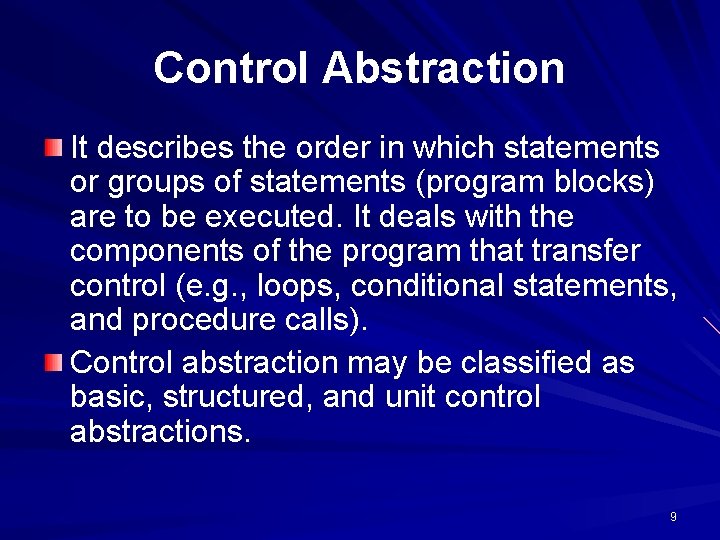
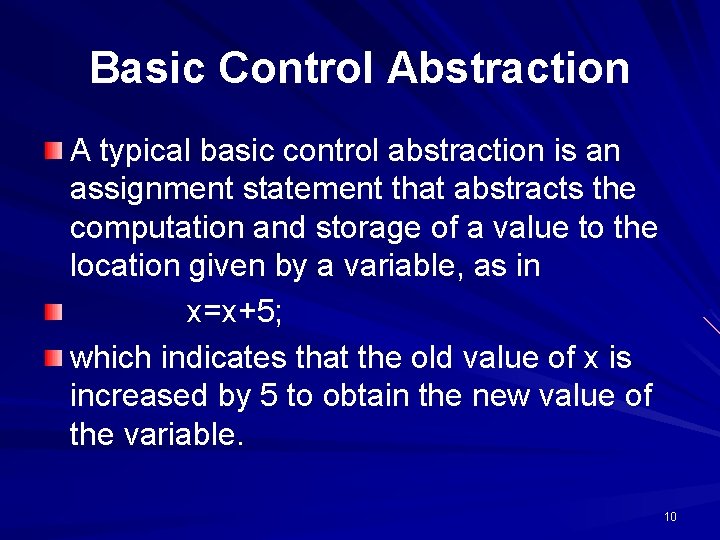
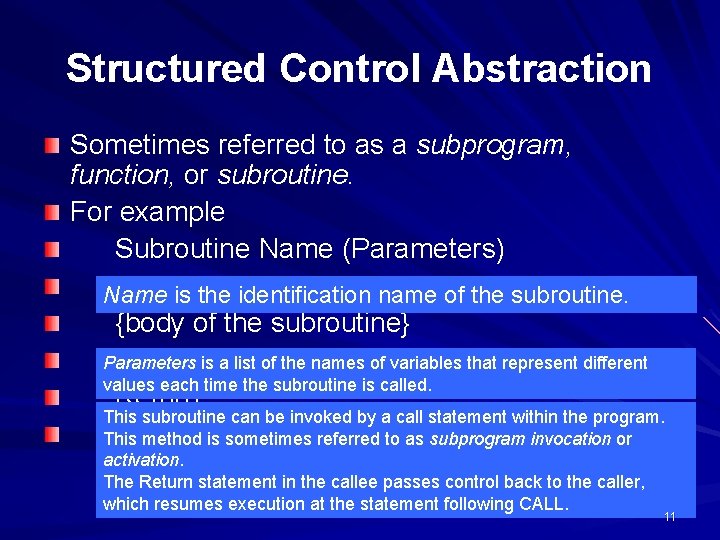
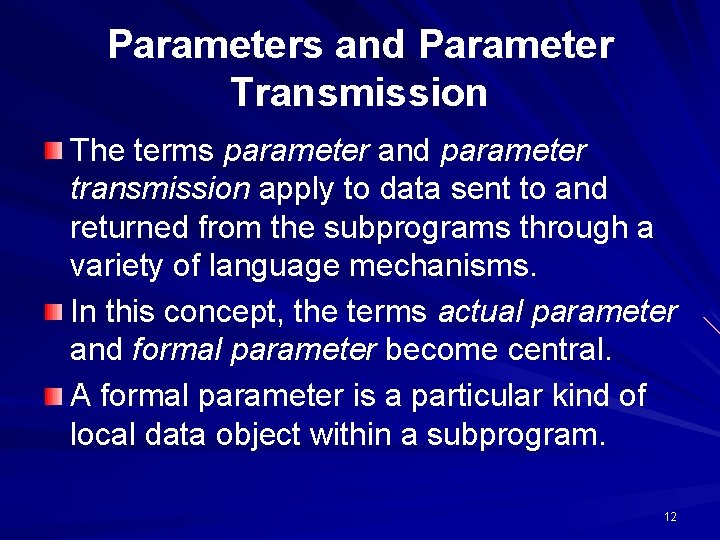
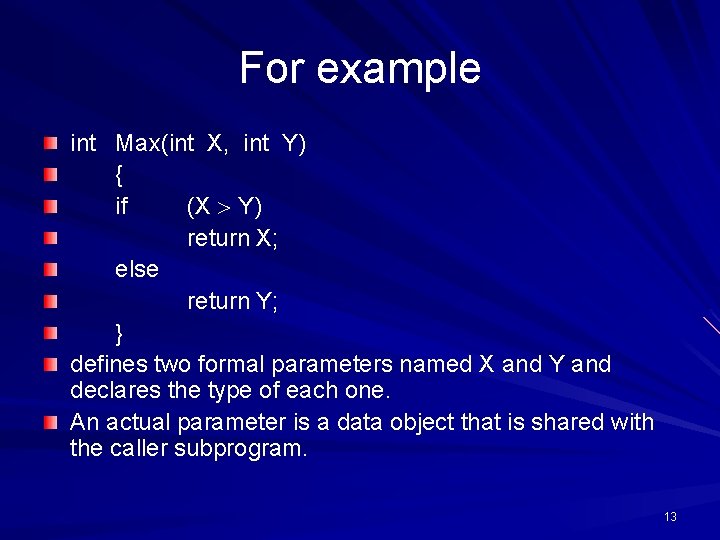
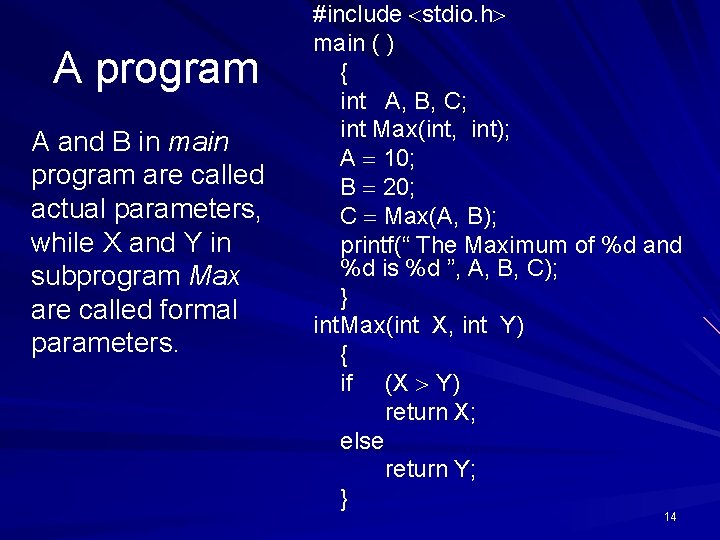
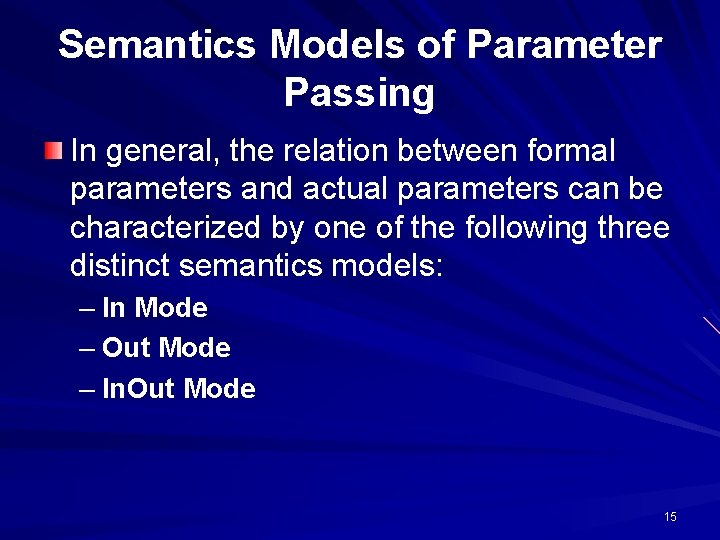
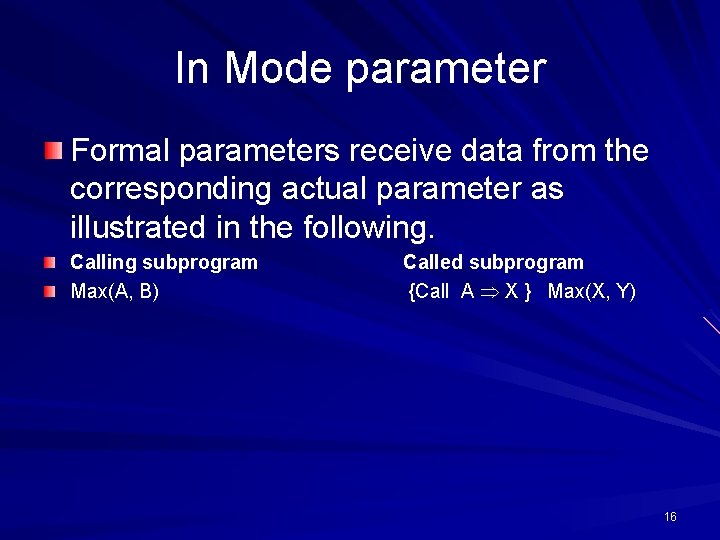
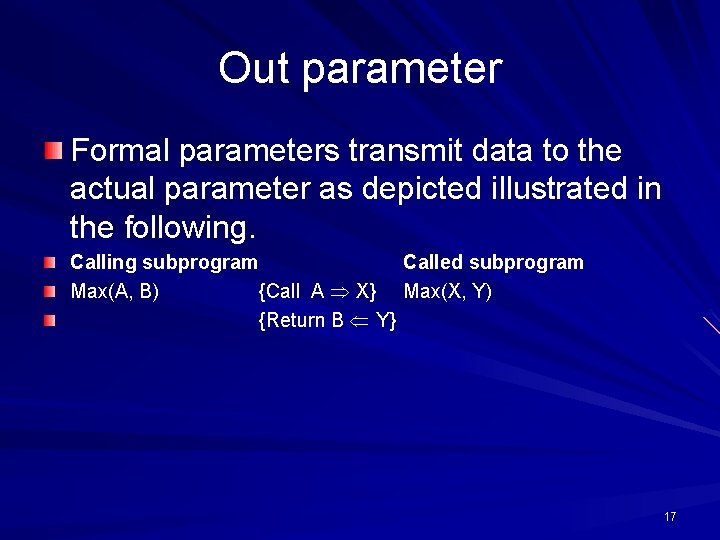
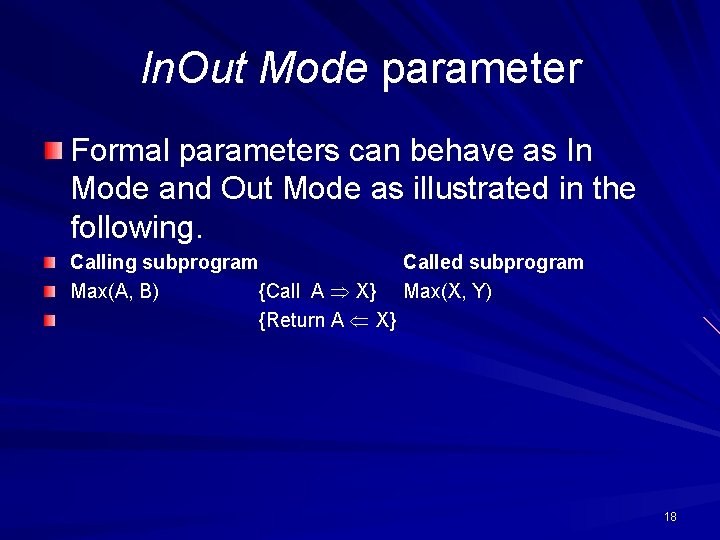
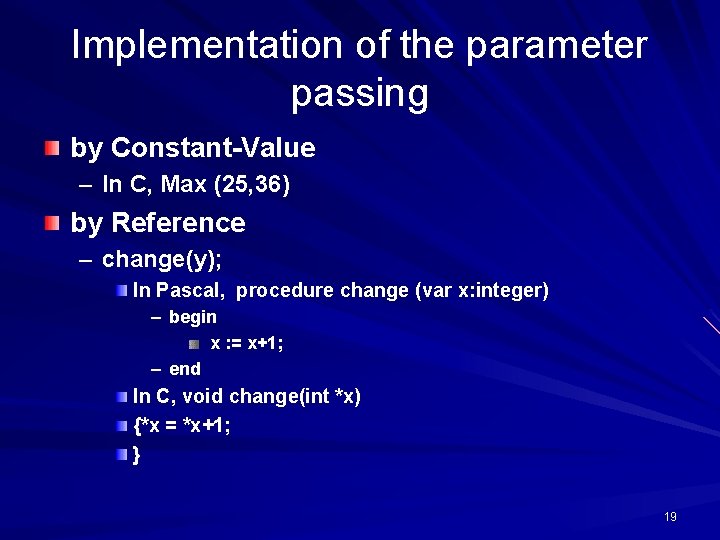
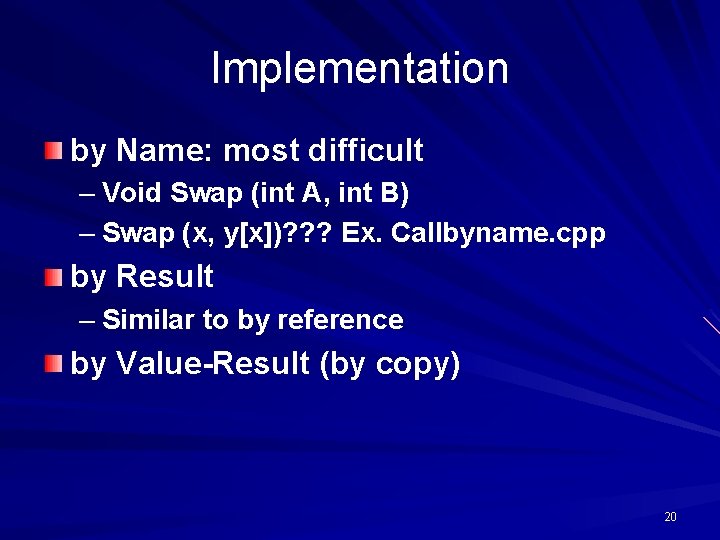
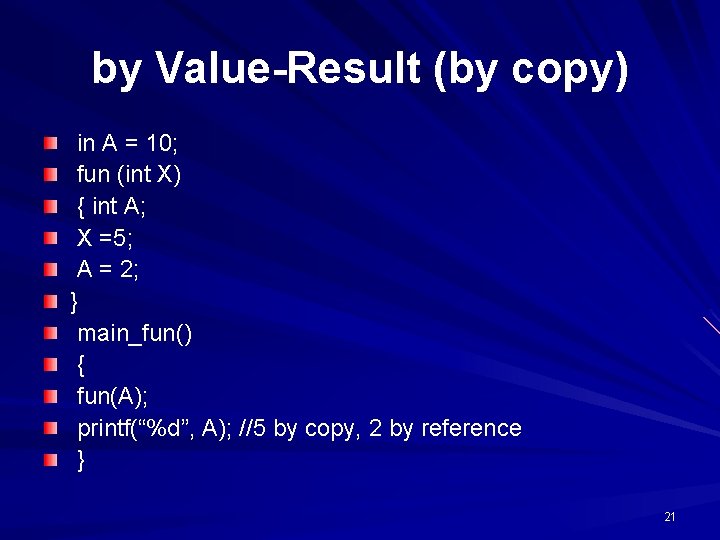
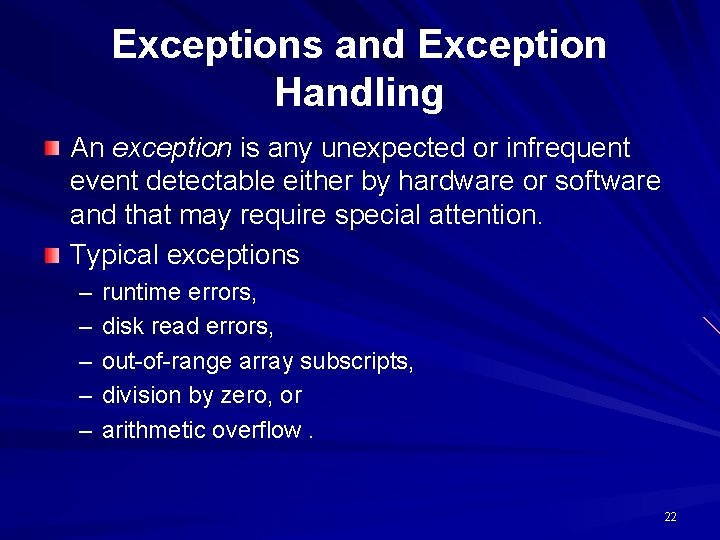
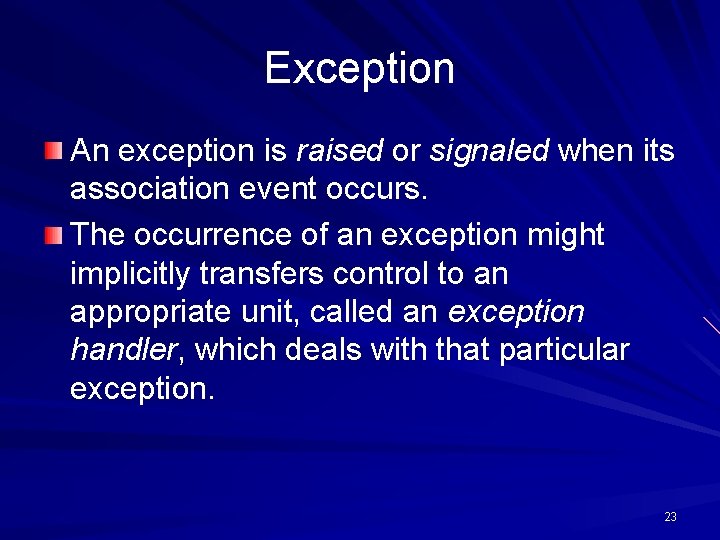
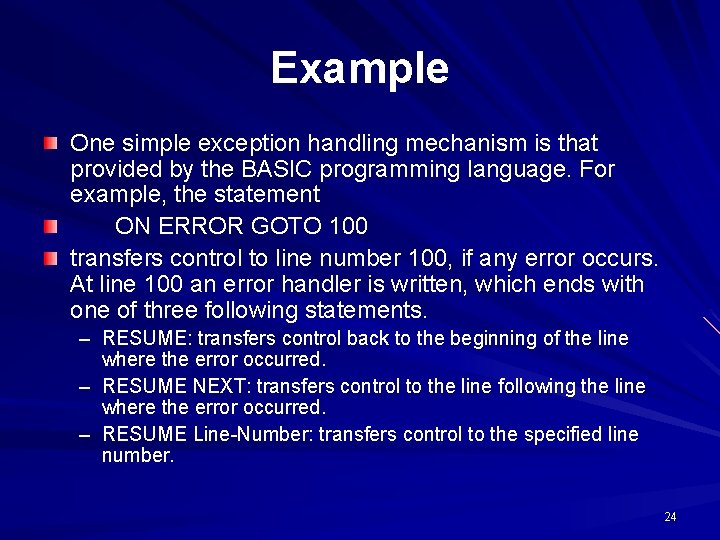
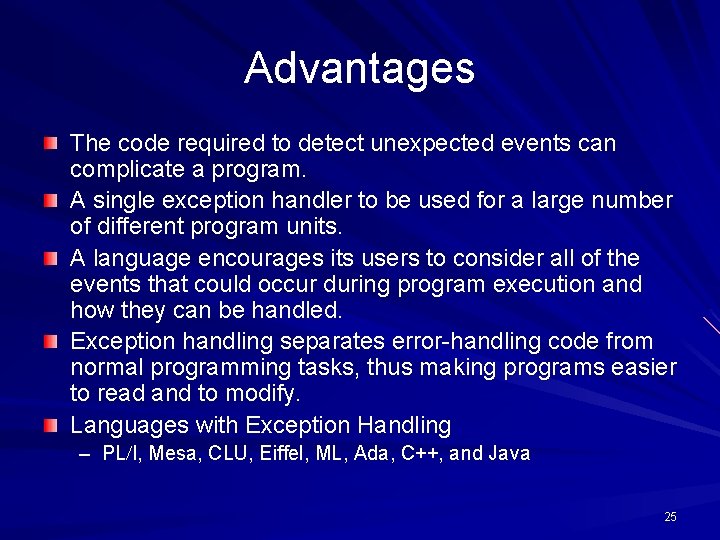
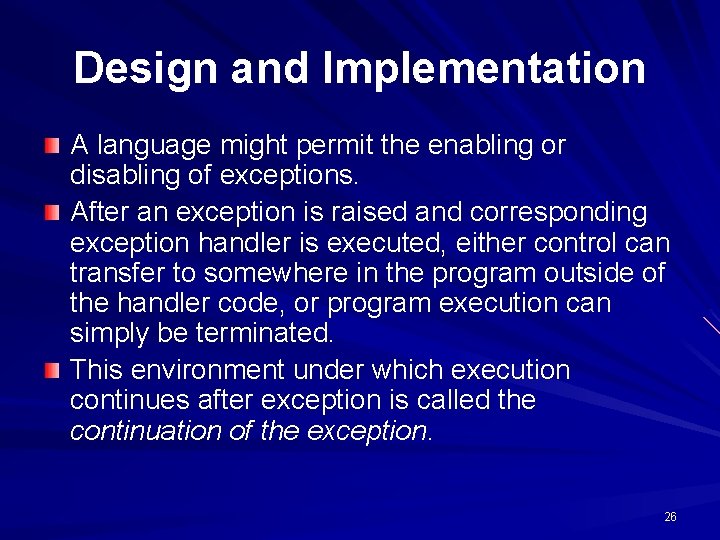
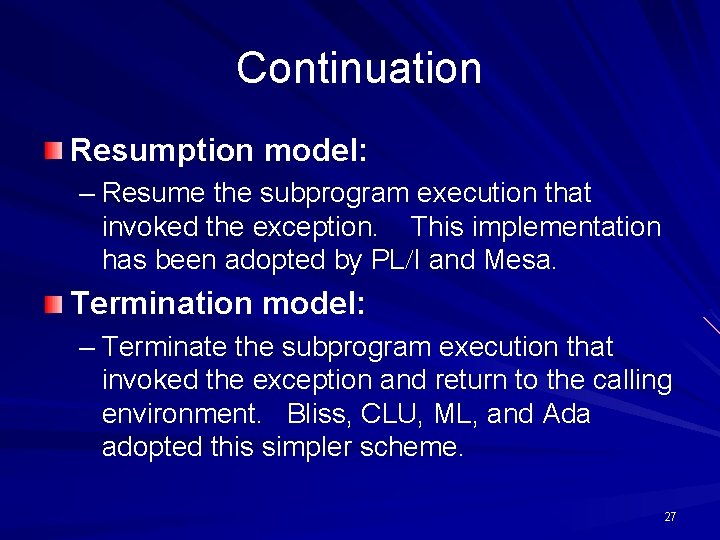
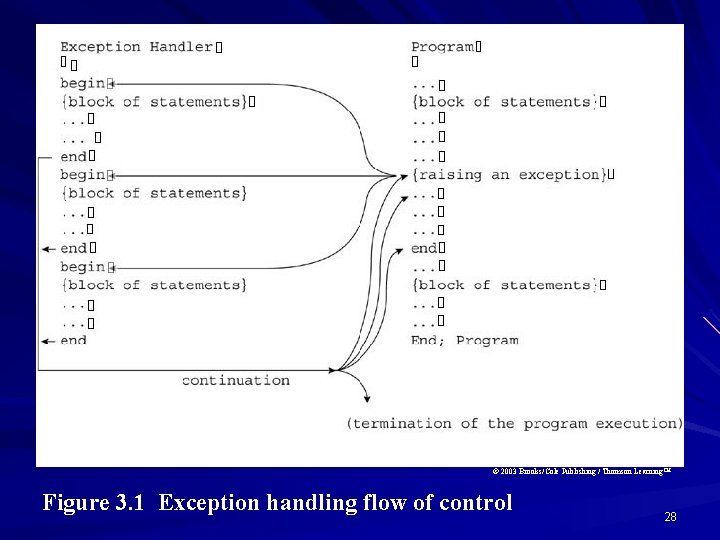
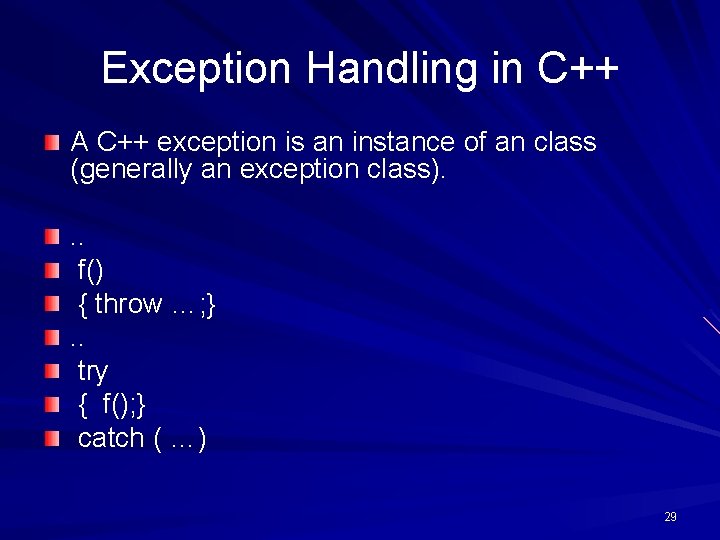
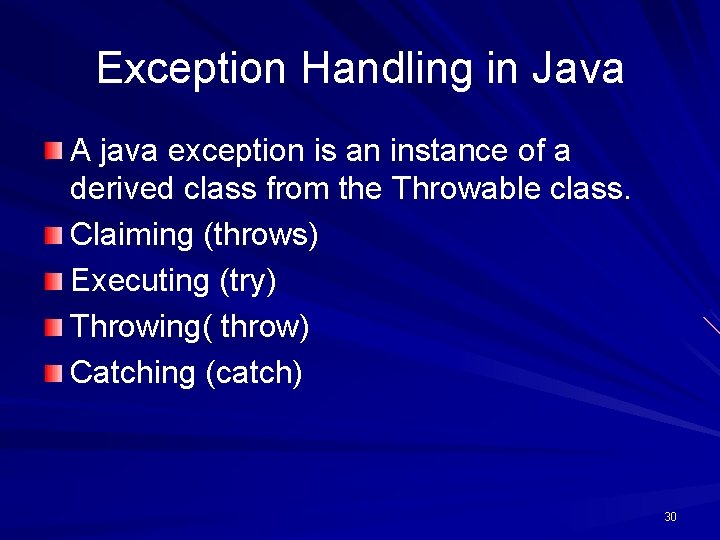
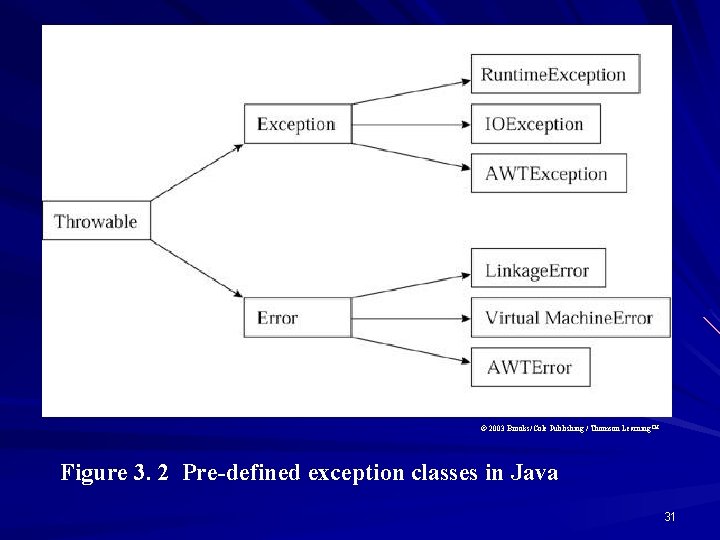
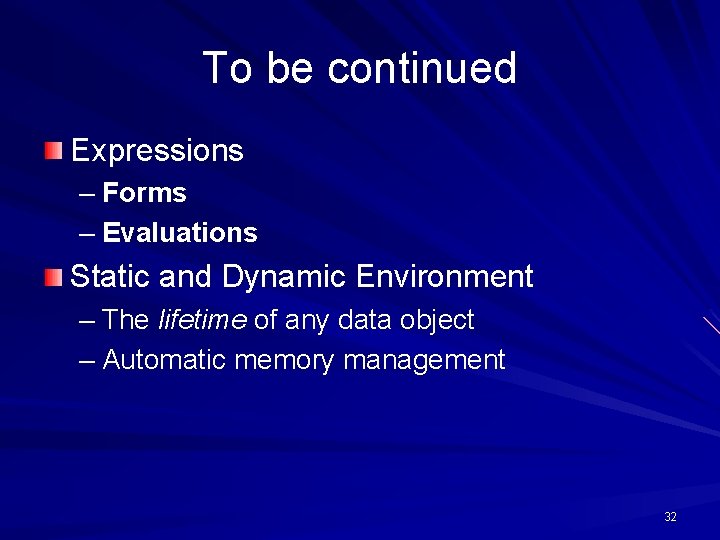
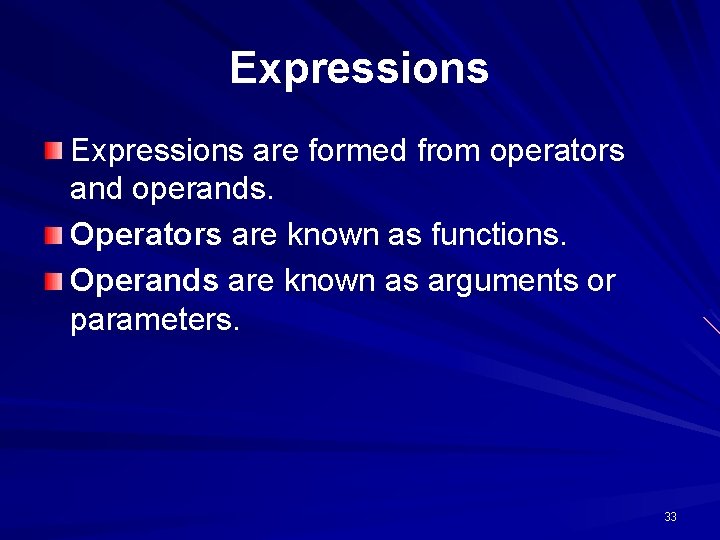
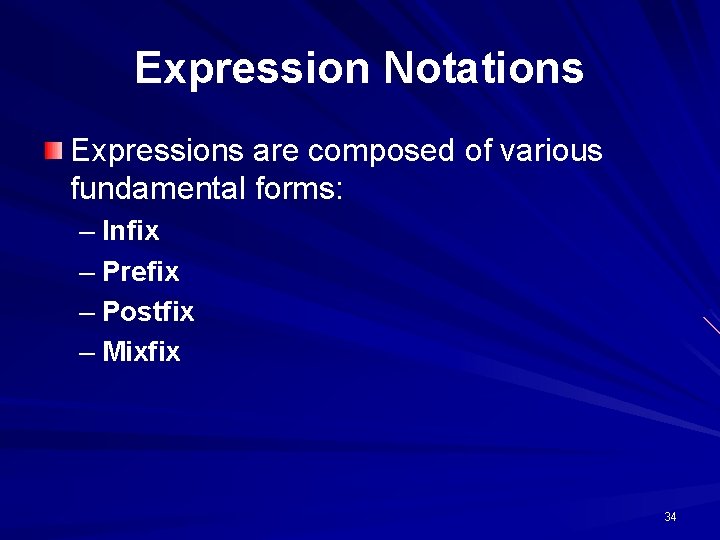
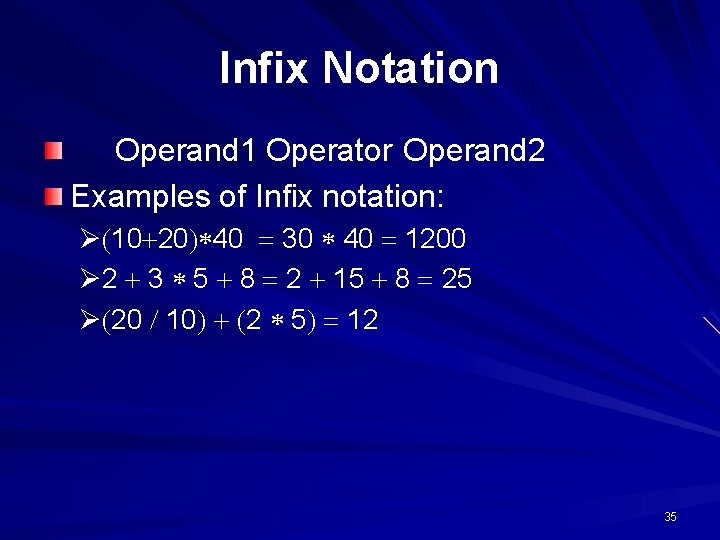
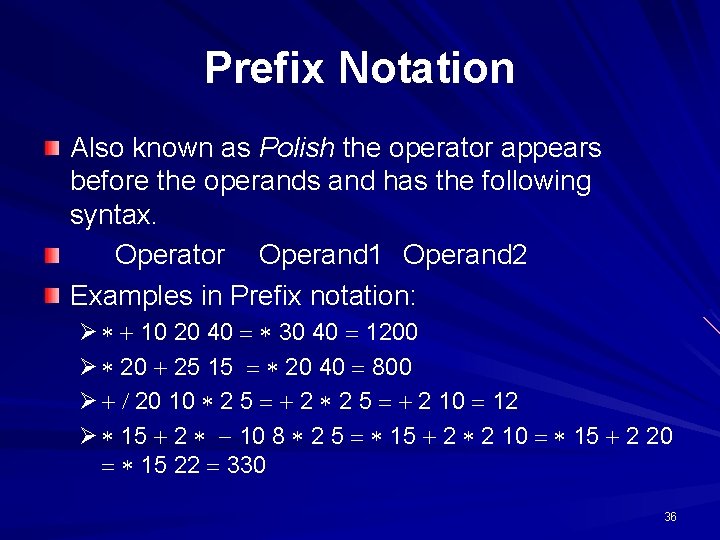
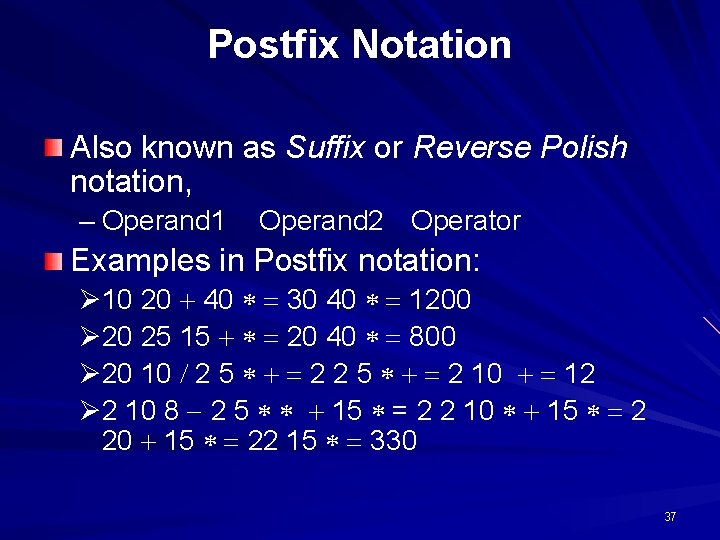
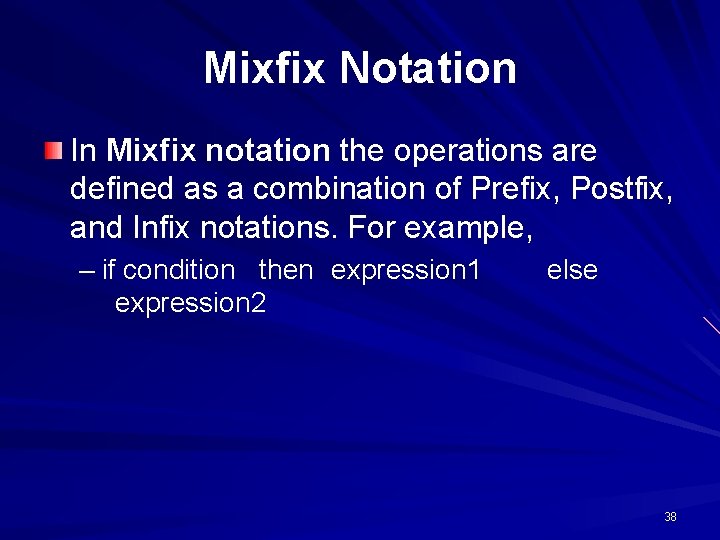
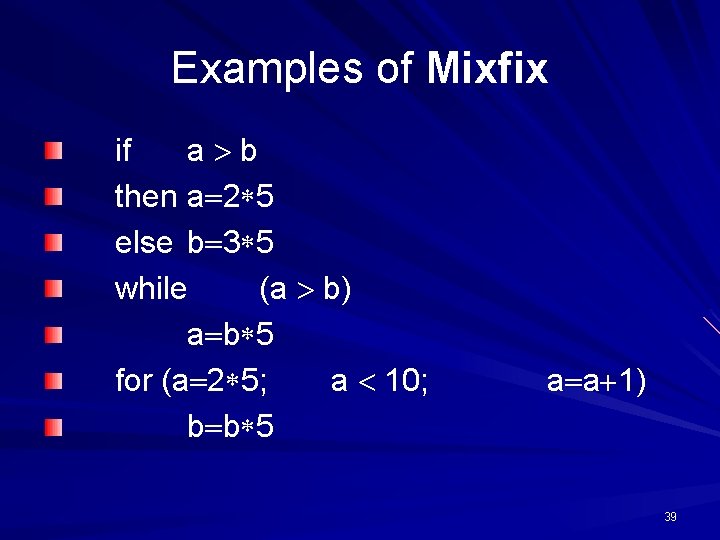
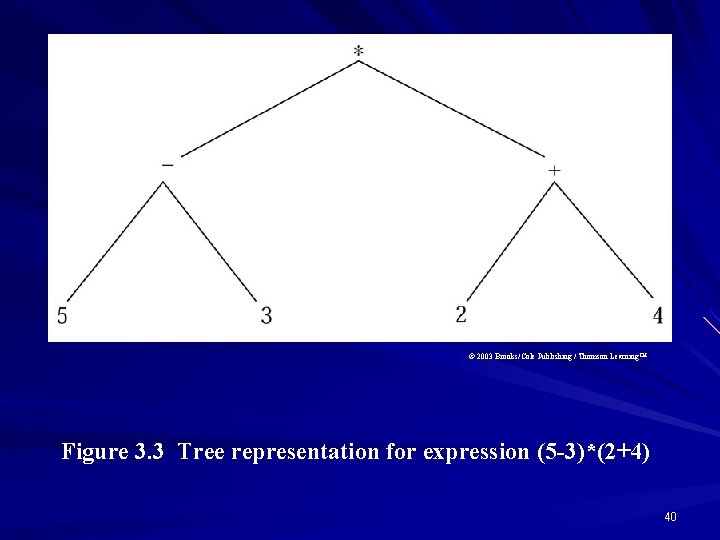
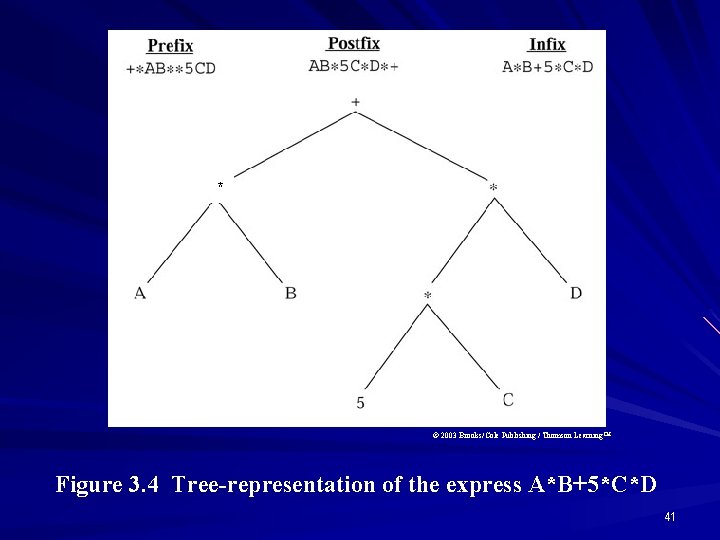
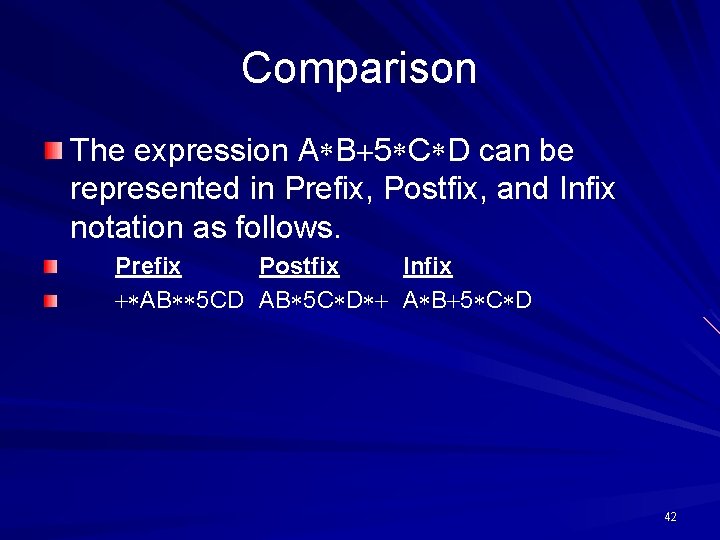
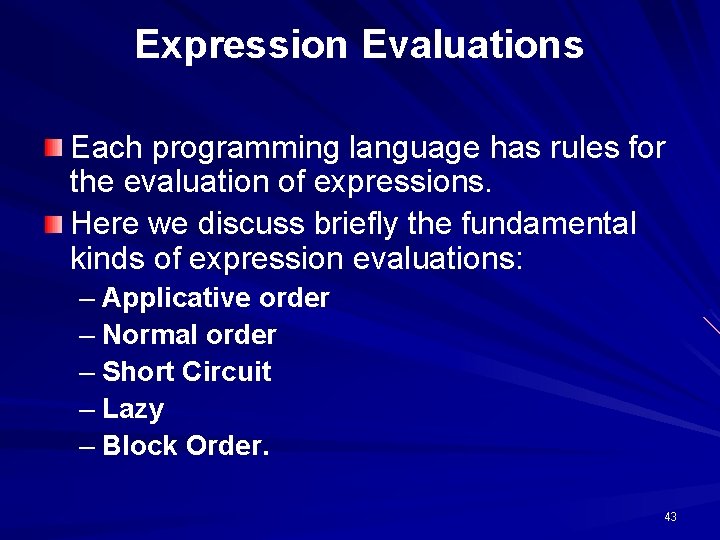
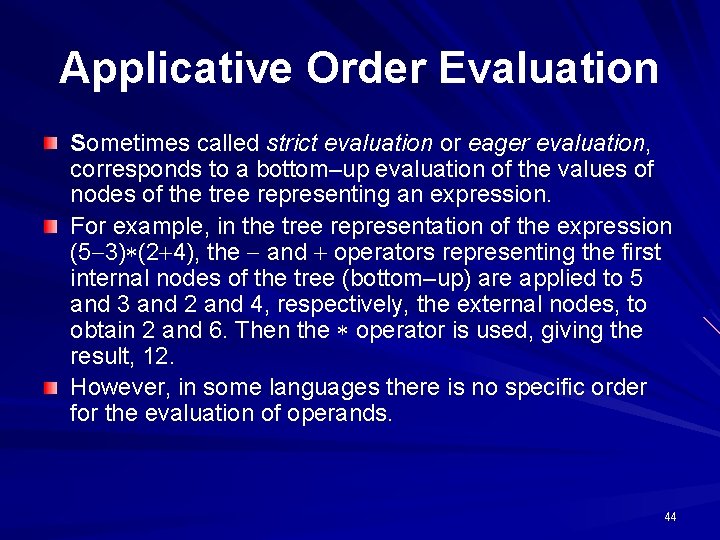
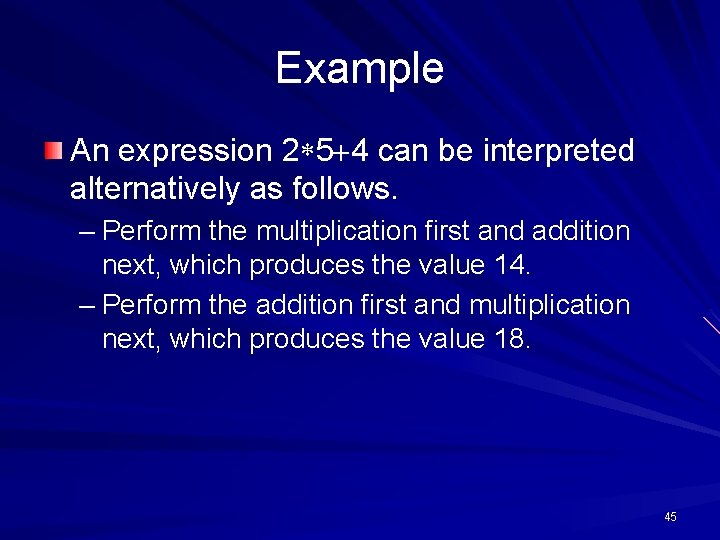
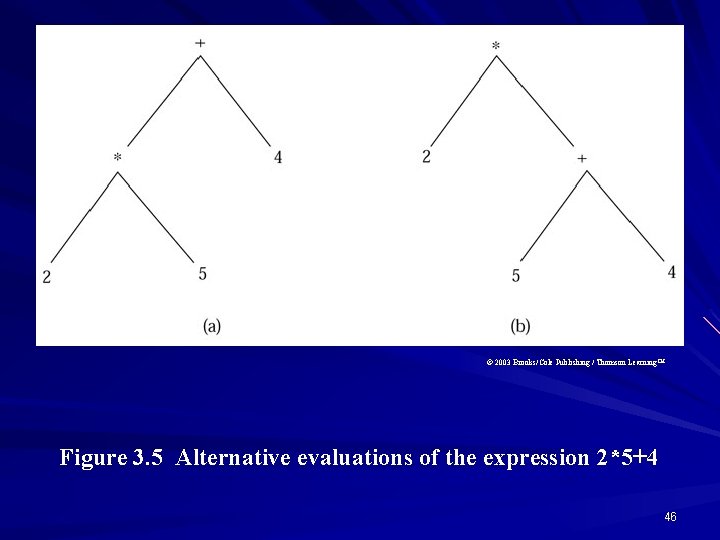
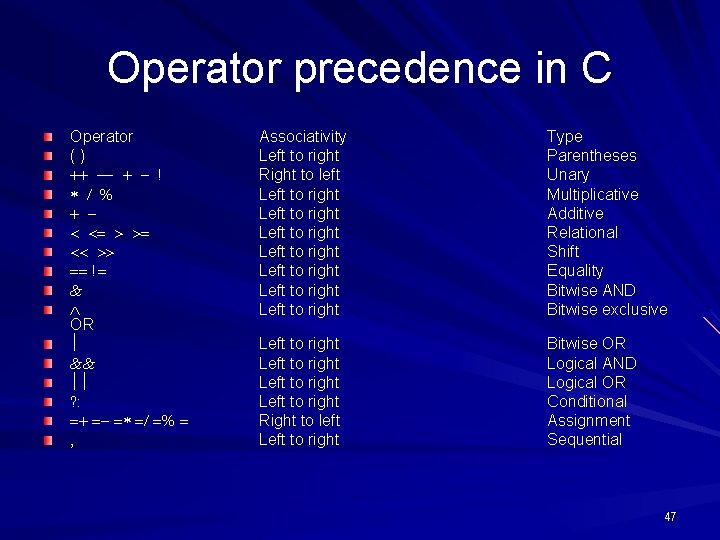
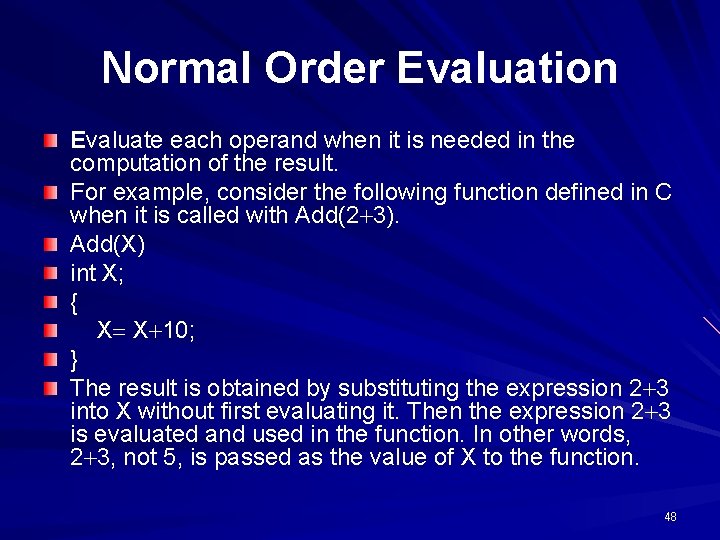
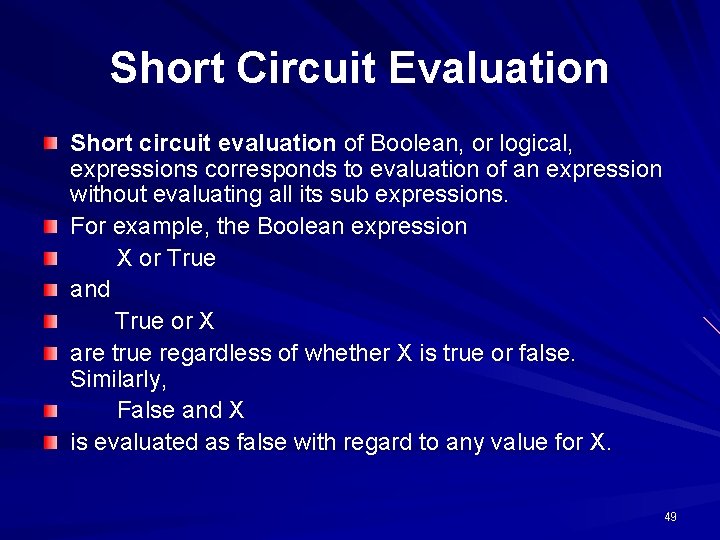
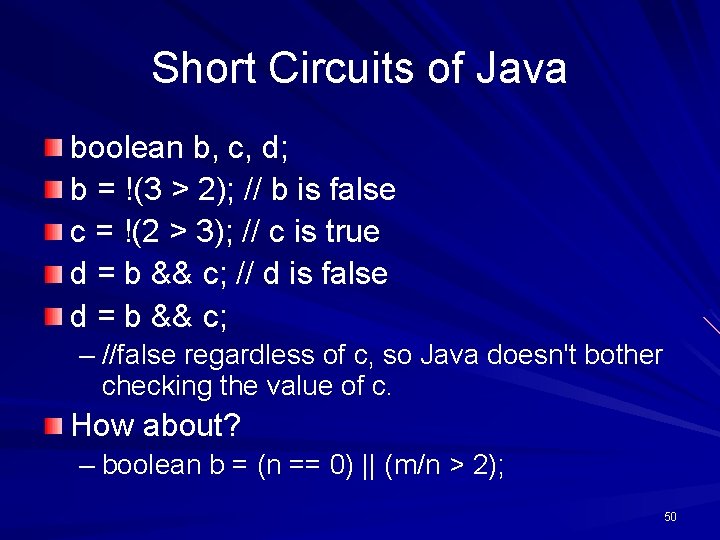
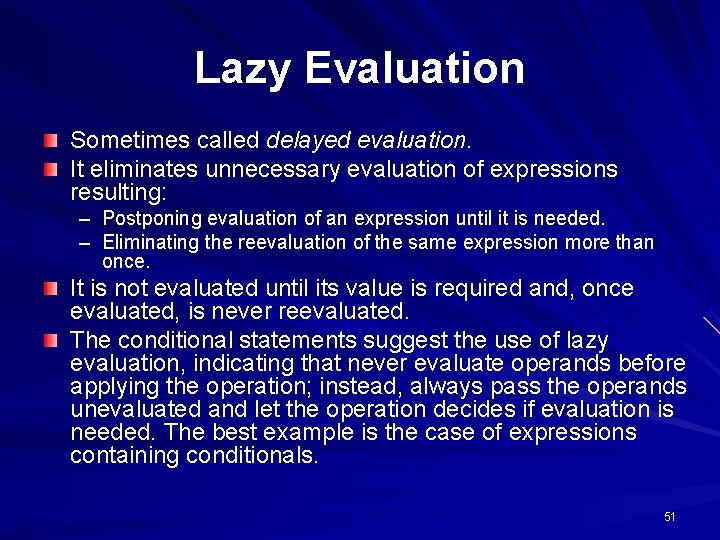
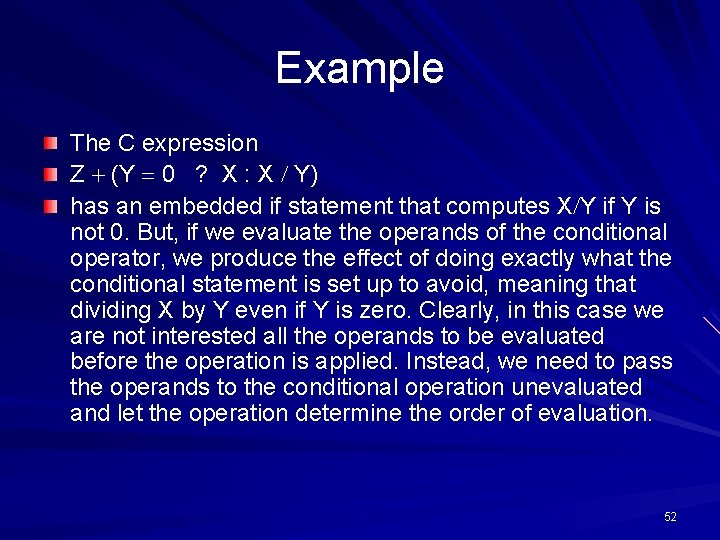
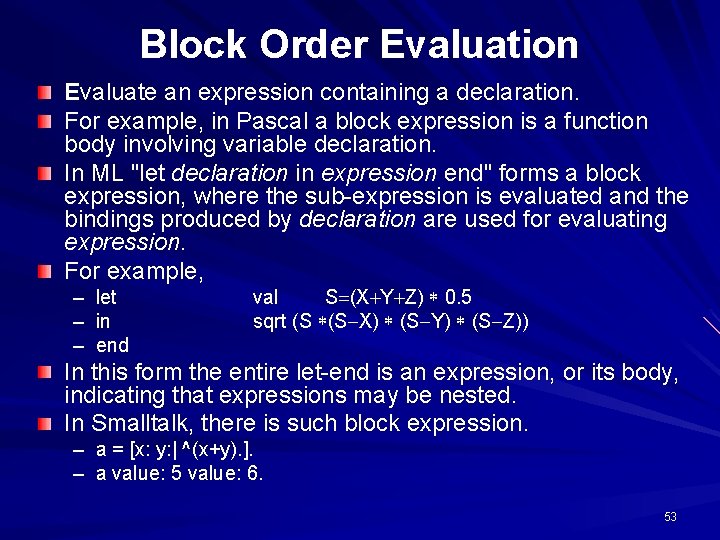
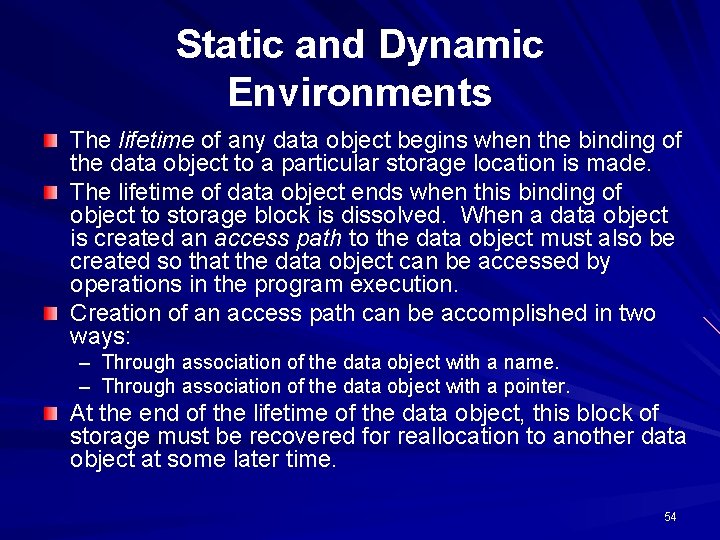
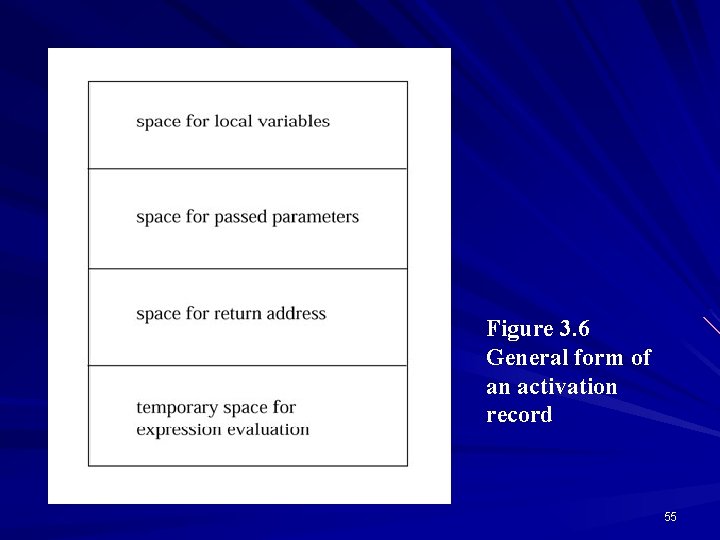
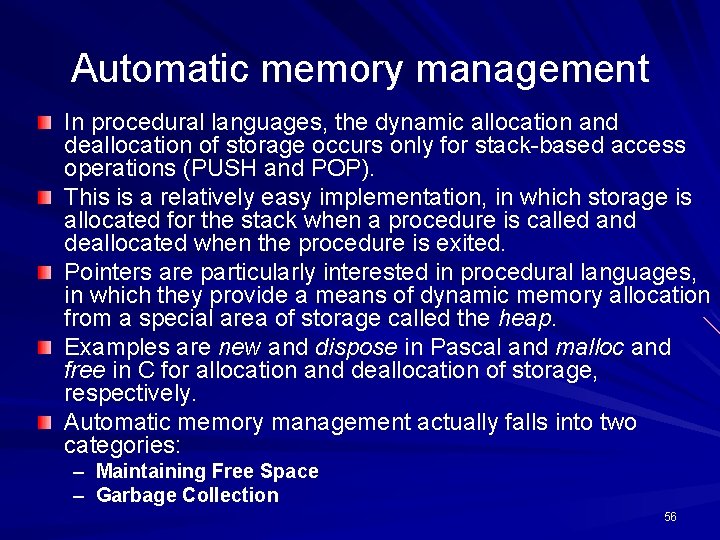
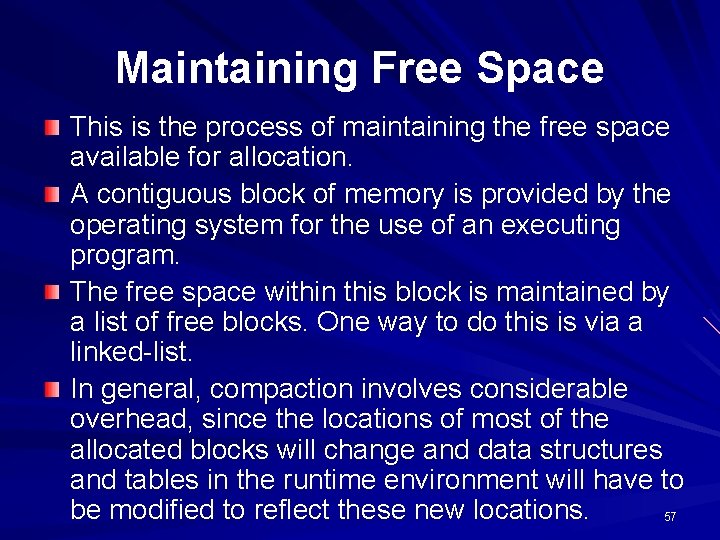
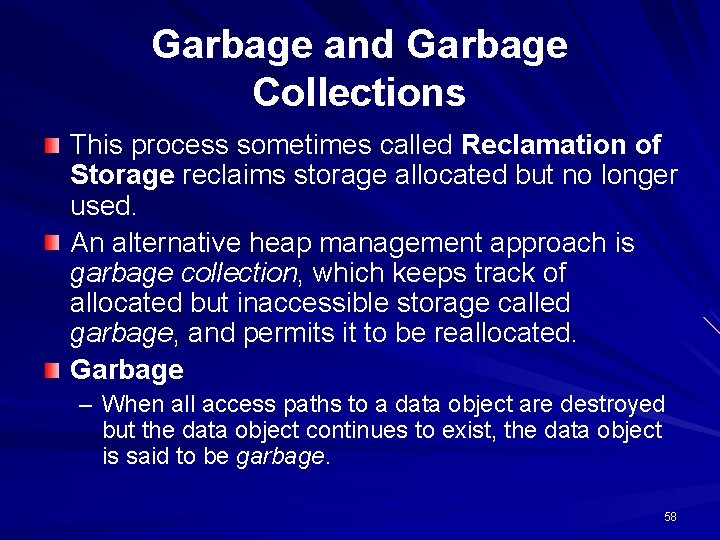
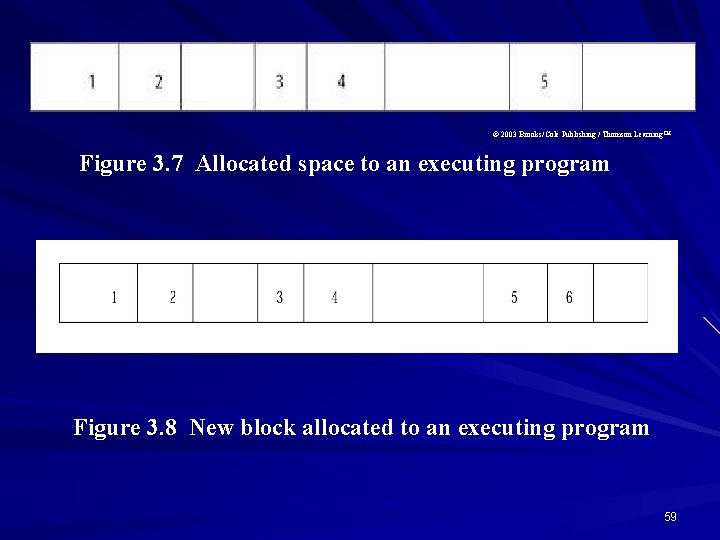
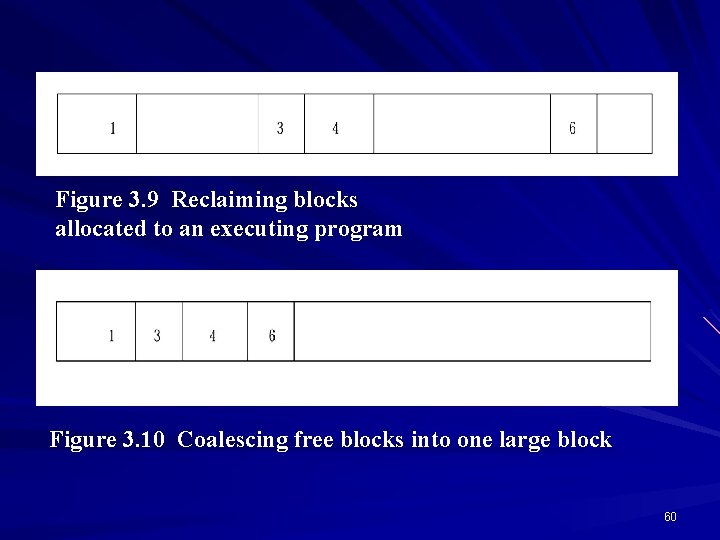
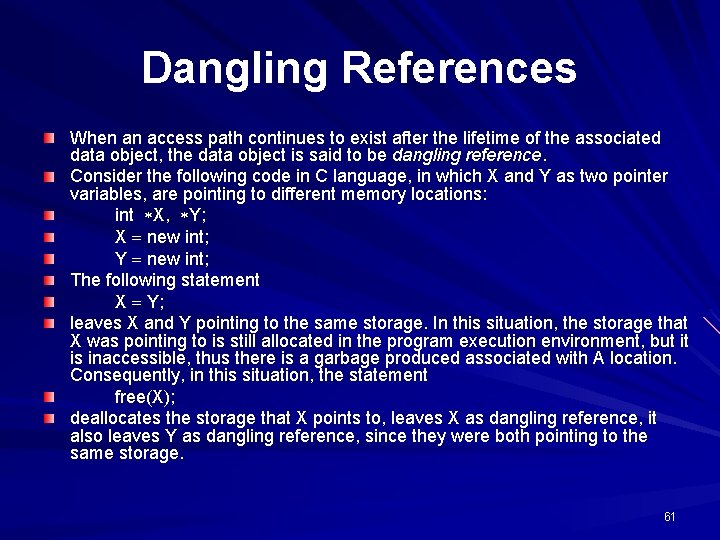
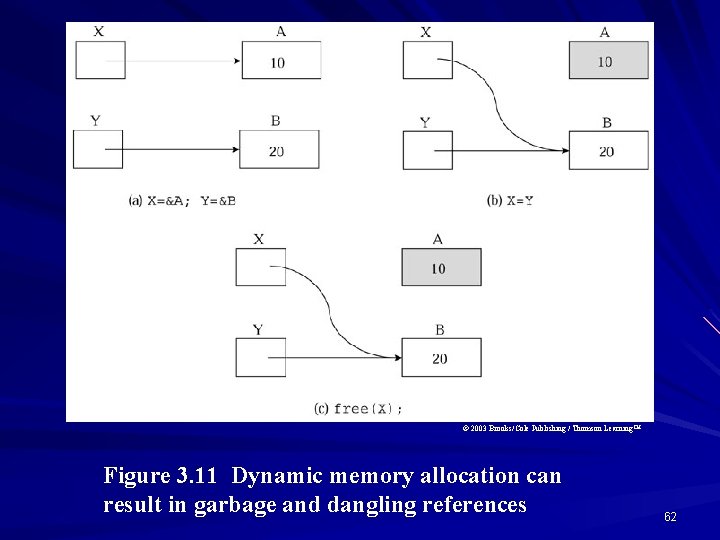
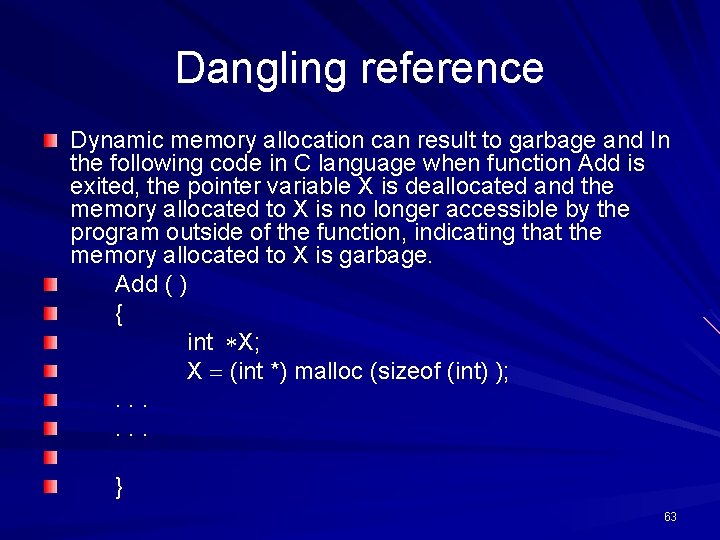
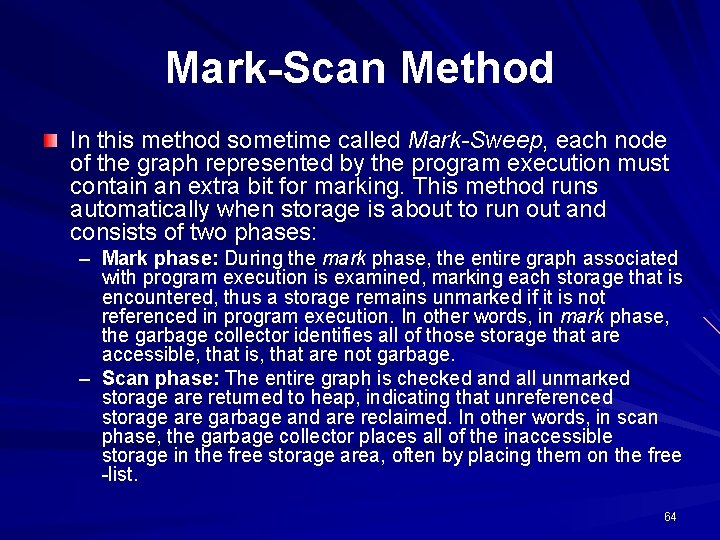
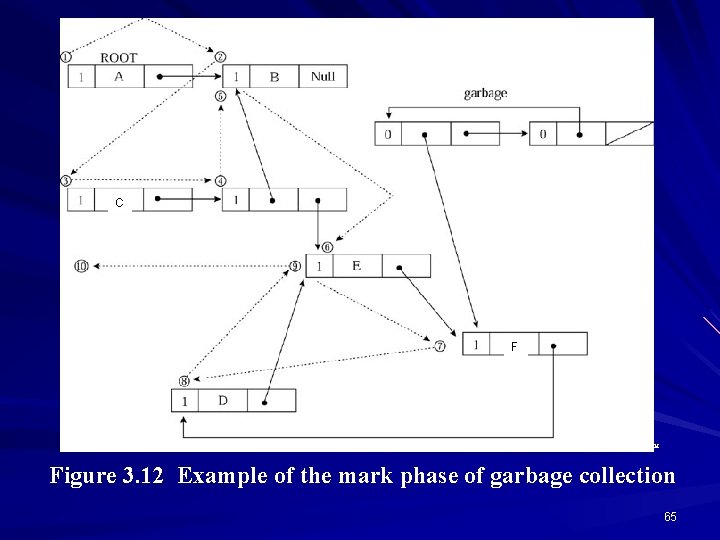
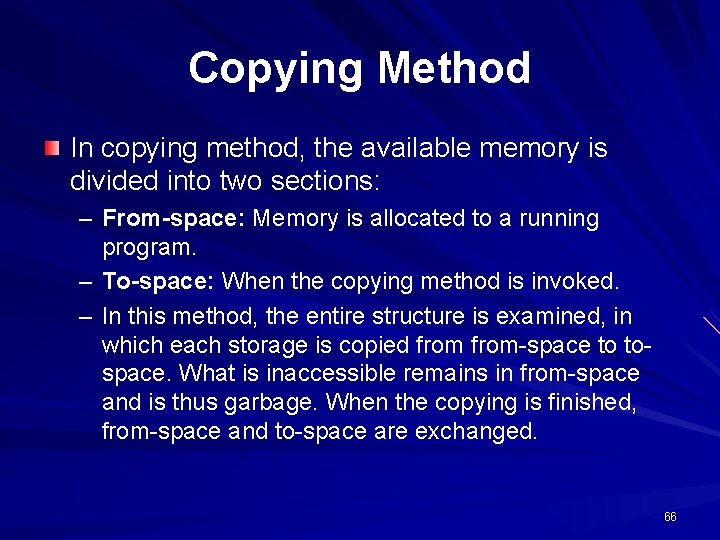
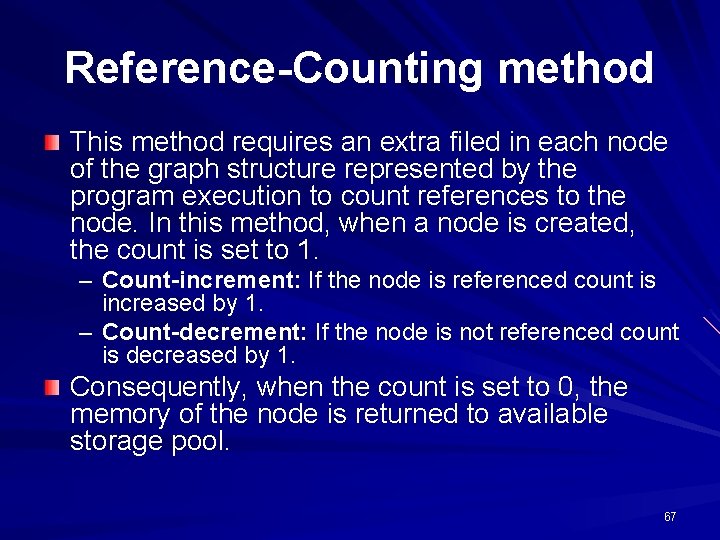
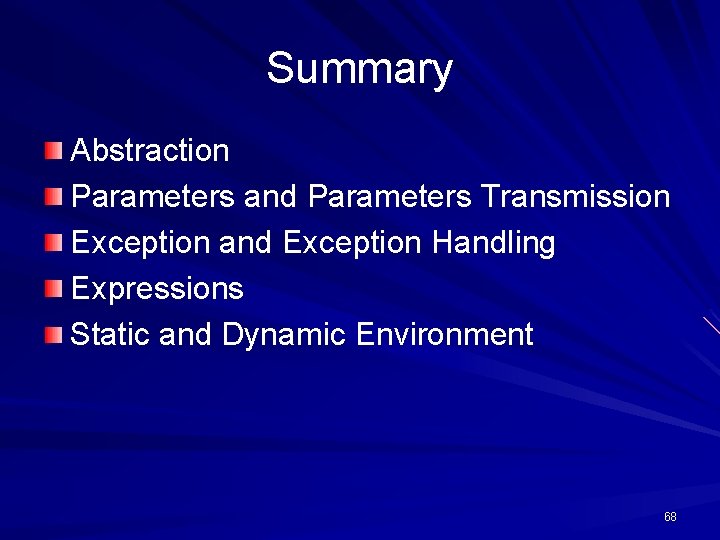
- Slides: 68
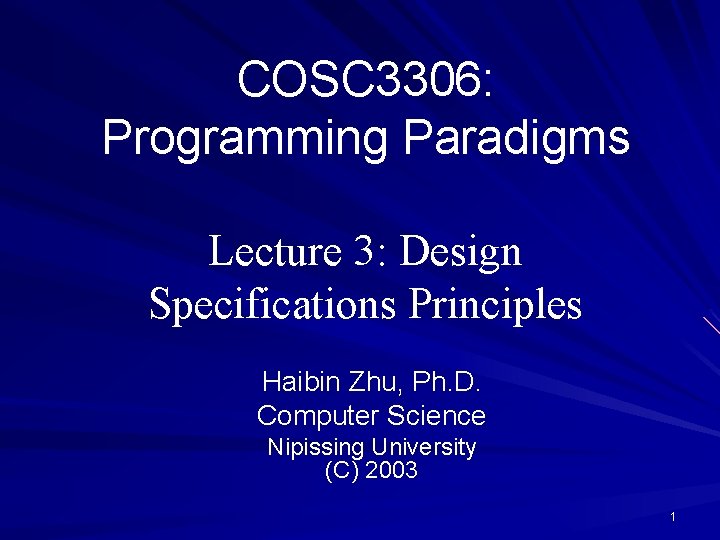
COSC 3306: Programming Paradigms Lecture 3: Design Specifications Principles Haibin Zhu, Ph. D. Computer Science Nipissing University (C) 2003 1
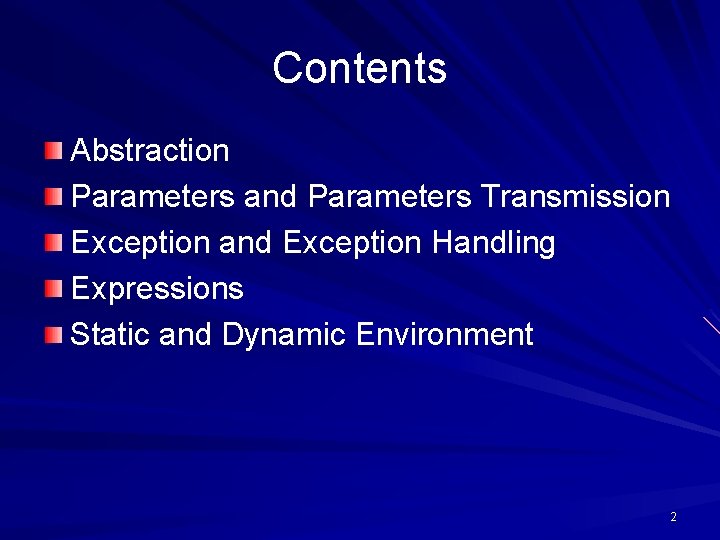
Contents Abstraction Parameters and Parameters Transmission Exception and Exception Handling Expressions Static and Dynamic Environment 2
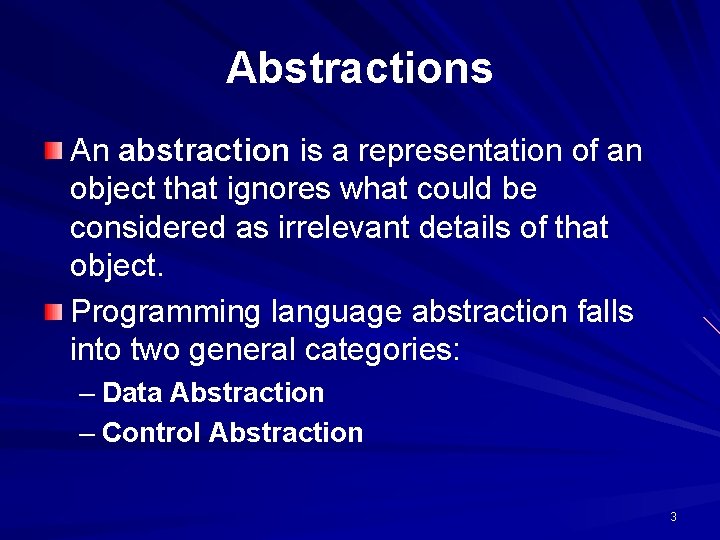
Abstractions An abstraction is a representation of an object that ignores what could be considered as irrelevant details of that object. Programming language abstraction falls into two general categories: – Data Abstraction – Control Abstraction 3
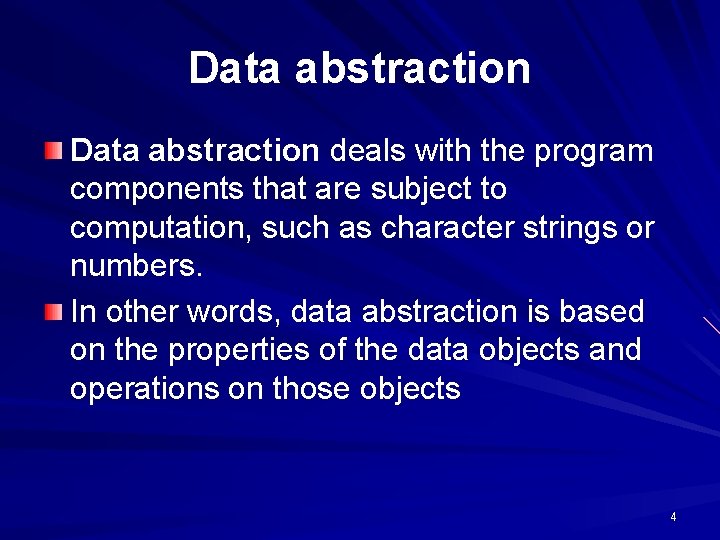
Data abstraction deals with the program components that are subject to computation, such as character strings or numbers. In other words, data abstraction is based on the properties of the data objects and operations on those objects 4
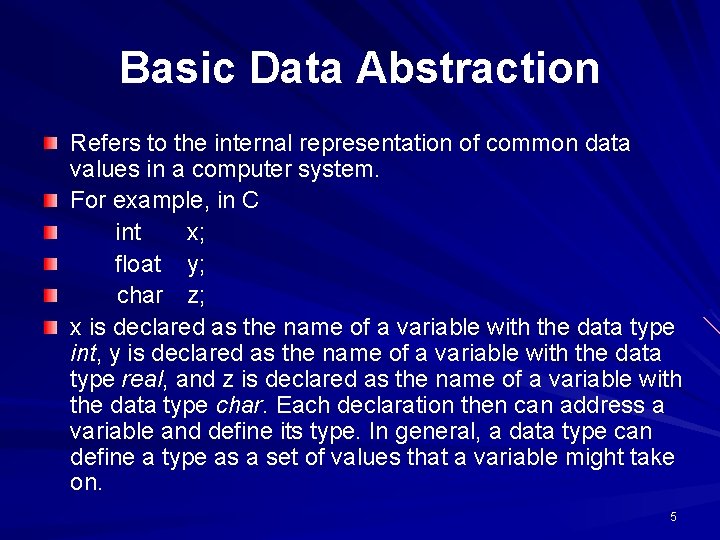
Basic Data Abstraction Refers to the internal representation of common data values in a computer system. For example, in C int x; float y; char z; x is declared as the name of a variable with the data type int, y is declared as the name of a variable with the data type real, and z is declared as the name of a variable with the data type char. Each declaration then can address a variable and define its type. In general, a data type can define a type as a set of values that a variable might take on. 5
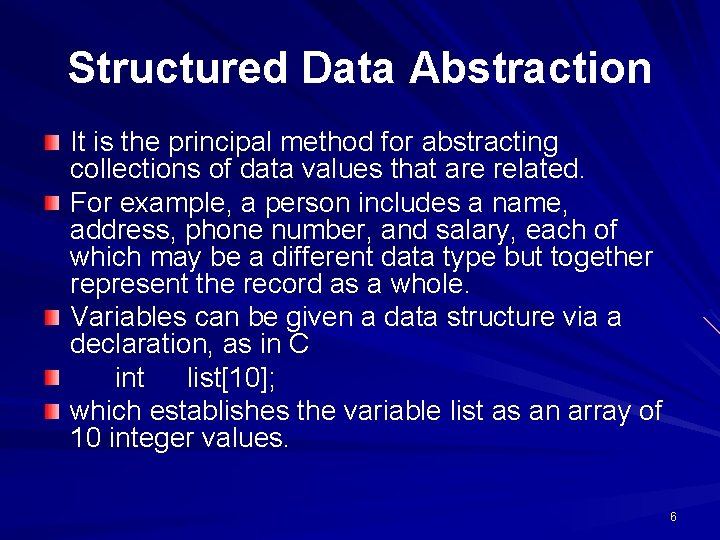
Structured Data Abstraction It is the principal method for abstracting collections of data values that are related. For example, a person includes a name, address, phone number, and salary, each of which may be a different data type but together represent the record as a whole. Variables can be given a data structure via a declaration, as in C int list[10]; which establishes the variable list as an array of 10 integer values. 6
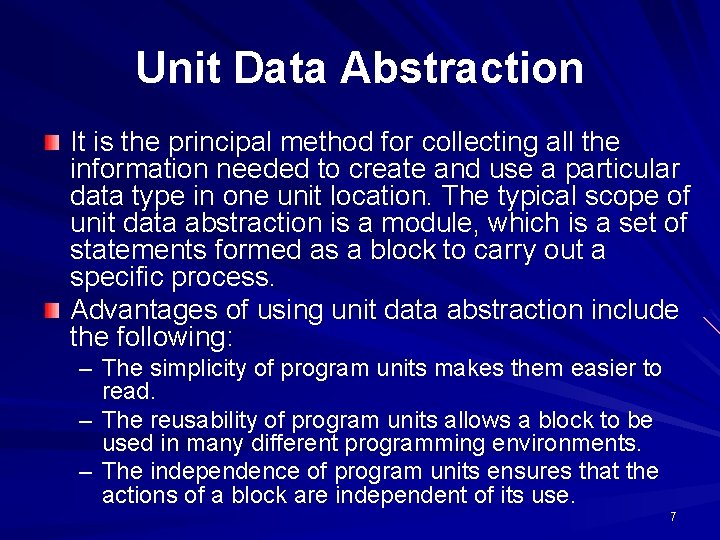
Unit Data Abstraction It is the principal method for collecting all the information needed to create and use a particular data type in one unit location. The typical scope of unit data abstraction is a module, which is a set of statements formed as a block to carry out a specific process. Advantages of using unit data abstraction include the following: – The simplicity of program units makes them easier to read. – The reusability of program units allows a block to be used in many different programming environments. – The independence of program units ensures that the actions of a block are independent of its use. 7
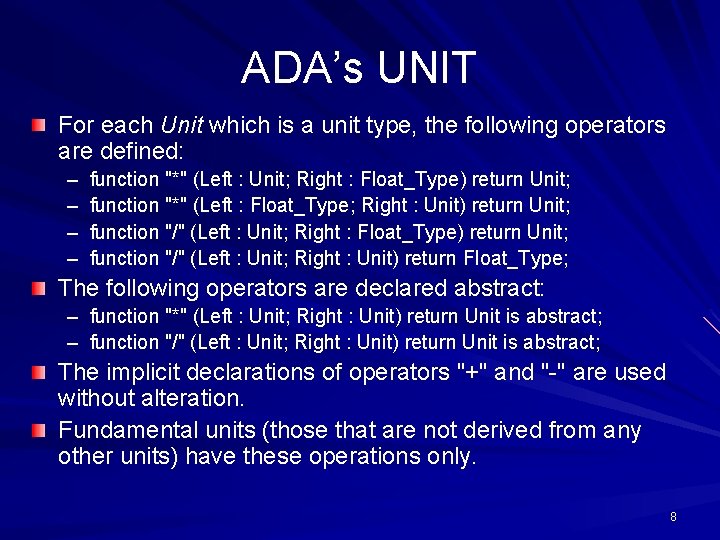
ADA’s UNIT For each Unit which is a unit type, the following operators are defined: – – function "*" (Left : Unit; Right : Float_Type) return Unit; function "*" (Left : Float_Type; Right : Unit) return Unit; function "/" (Left : Unit; Right : Float_Type) return Unit; function "/" (Left : Unit; Right : Unit) return Float_Type; The following operators are declared abstract: – function "*" (Left : Unit; Right : Unit) return Unit is abstract; – function "/" (Left : Unit; Right : Unit) return Unit is abstract; The implicit declarations of operators "+" and "-" are used without alteration. Fundamental units (those that are not derived from any other units) have these operations only. 8
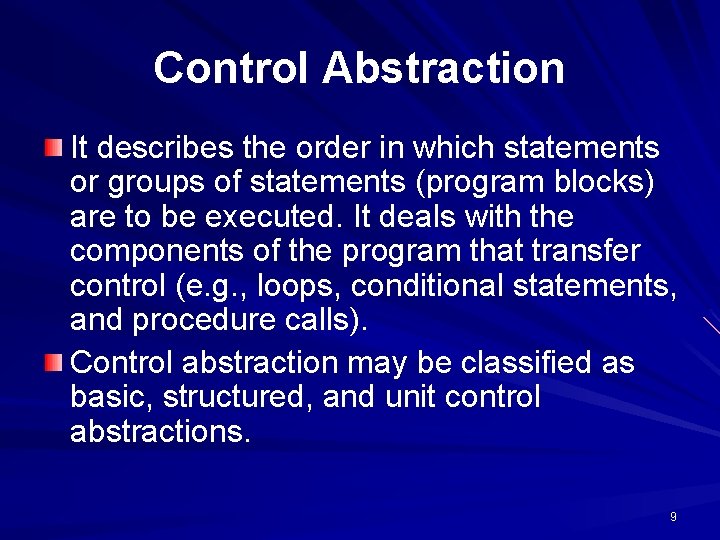
Control Abstraction It describes the order in which statements or groups of statements (program blocks) are to be executed. It deals with the components of the program that transfer control (e. g. , loops, conditional statements, and procedure calls). Control abstraction may be classified as basic, structured, and unit control abstractions. 9
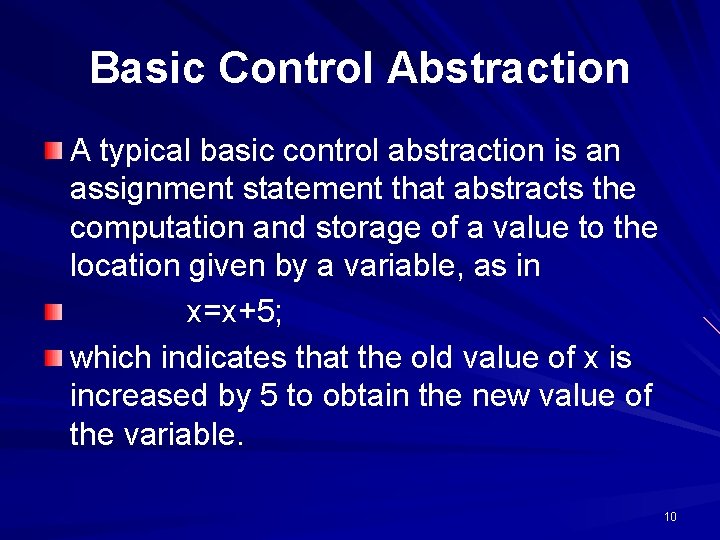
Basic Control Abstraction A typical basic control abstraction is an assignment statement that abstracts the computation and storage of a value to the location given by a variable, as in x=x+5; which indicates that the old value of x is increased by 5 to obtain the new value of the variable. 10
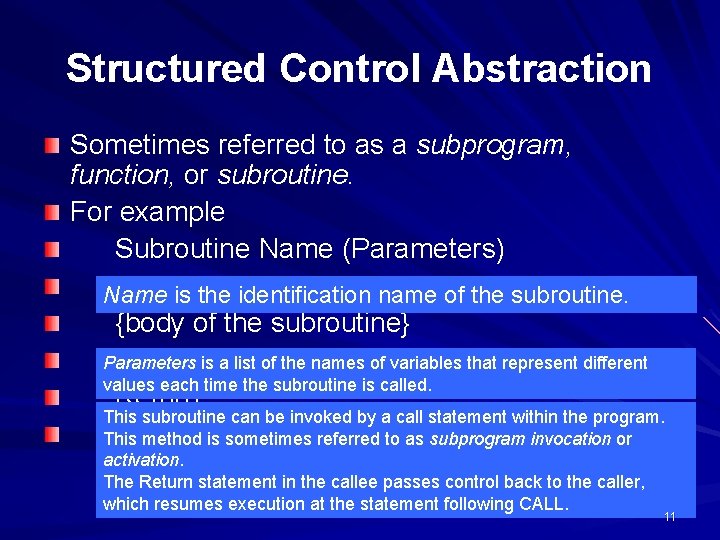
Structured Control Abstraction Sometimes referred to as a subprogram, function, or subroutine. For example Subroutine Name (Parameters) … is the identification name of the subroutine. Name {body of the subroutine} Parameters is a list of the names of variables that represent different … values each time the subroutine is called. Return This subroutine can be invoked by a call statement within the program. End This method is sometimes referred to as subprogram invocation or activation. The Return statement in the callee passes control back to the caller, which resumes execution at the statement following CALL. 11
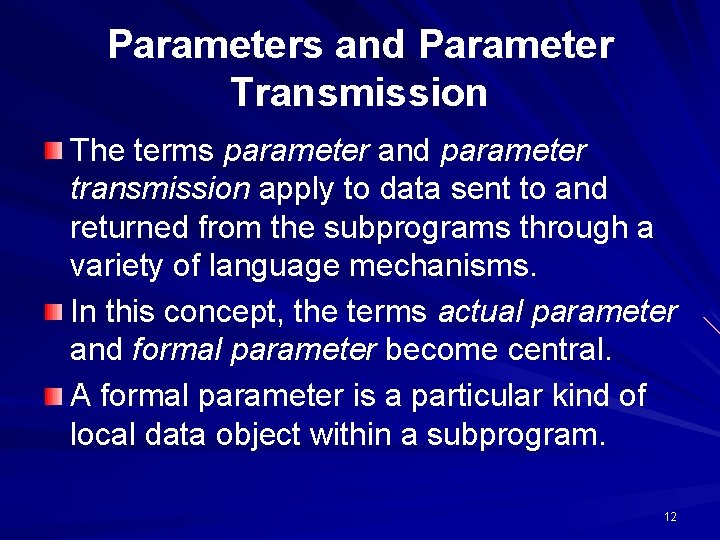
Parameters and Parameter Transmission The terms parameter and parameter transmission apply to data sent to and returned from the subprograms through a variety of language mechanisms. In this concept, the terms actual parameter and formal parameter become central. A formal parameter is a particular kind of local data object within a subprogram. 12
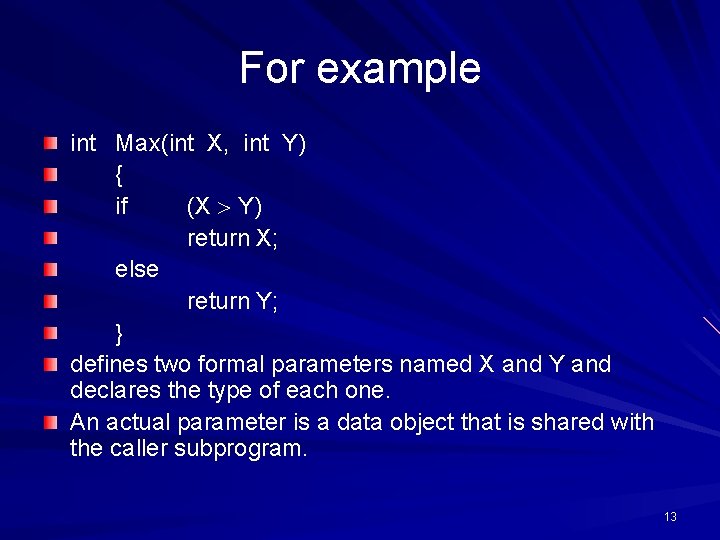
For example int Max(int X, int Y) { if (X Y) return X; else return Y; } defines two formal parameters named X and Y and declares the type of each one. An actual parameter is a data object that is shared with the caller subprogram. 13
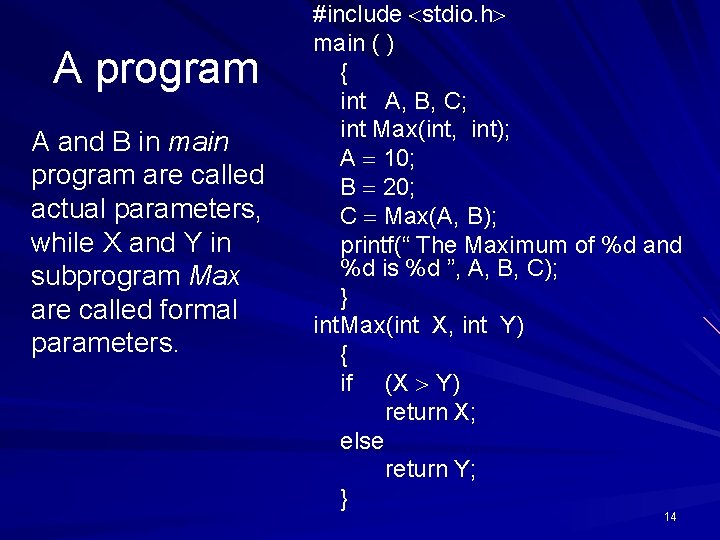
A program A and B in main program are called actual parameters, while X and Y in subprogram Max are called formal parameters. #include stdio. h main ( ) { int A, B, C; int Max(int, int); A 10; B 20; C Max(A, B); printf(“ The Maximum of %d and %d is %d ”, A, B, C); } int Max(int X, int Y) { if (X Y) return X; else return Y; } 14
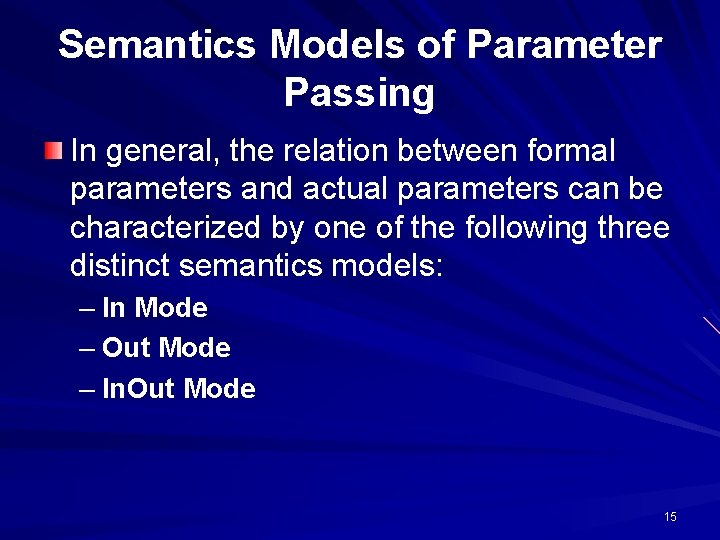
Semantics Models of Parameter Passing In general, the relation between formal parameters and actual parameters can be characterized by one of the following three distinct semantics models: – In Mode – Out Mode – In. Out Mode 15
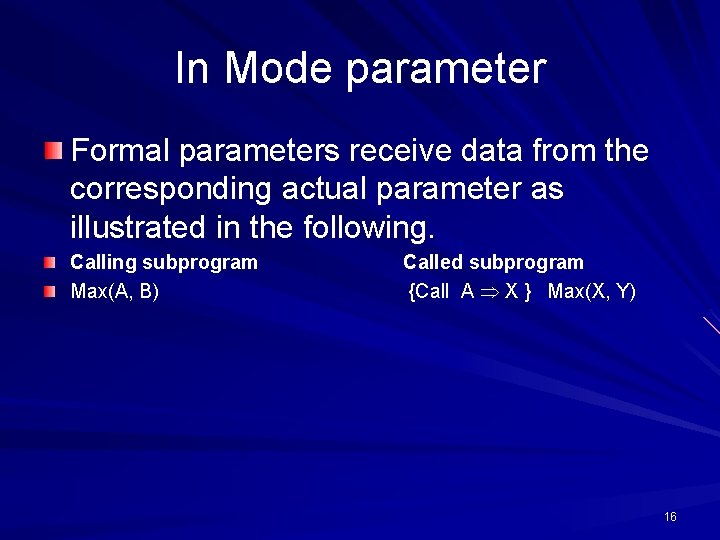
In Mode parameter Formal parameters receive data from the corresponding actual parameter as illustrated in the following. Calling subprogram Max(A, B) Called subprogram {Call A X } Max(X, Y) 16
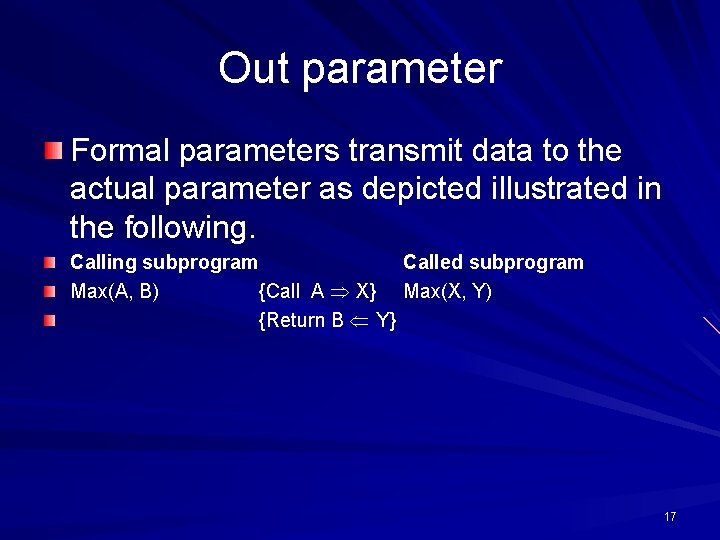
Out parameter Formal parameters transmit data to the actual parameter as depicted illustrated in the following. Calling subprogram Called subprogram Max(A, B) {Call A X} Max(X, Y) {Return B Y} 17
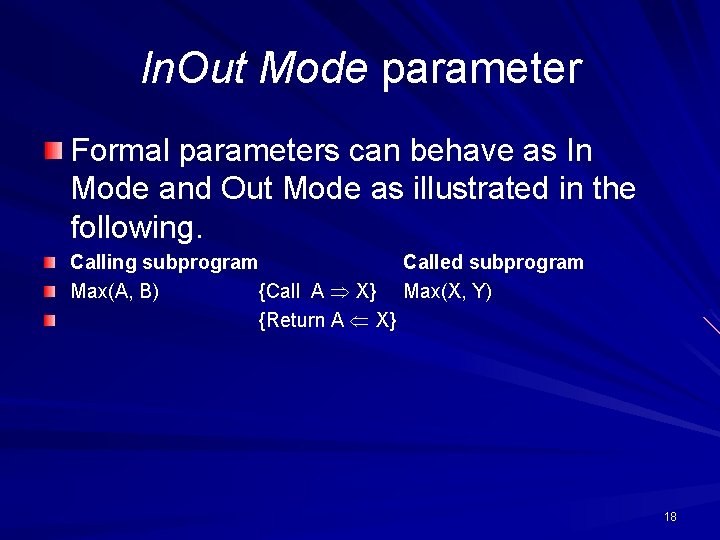
In. Out Mode parameter Formal parameters can behave as In Mode and Out Mode as illustrated in the following. Calling subprogram Called subprogram Max(A, B) {Call A X} Max(X, Y) {Return A X} 18
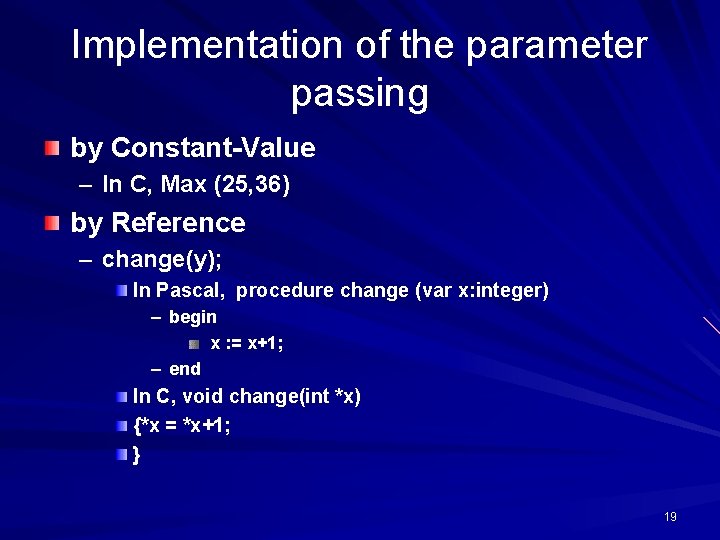
Implementation of the parameter passing by Constant-Value – In C, Max (25, 36) by Reference – change(y); In Pascal, procedure change (var x: integer) – begin x : = x+1; – end In C, void change(int *x) {*x = *x+1; } 19
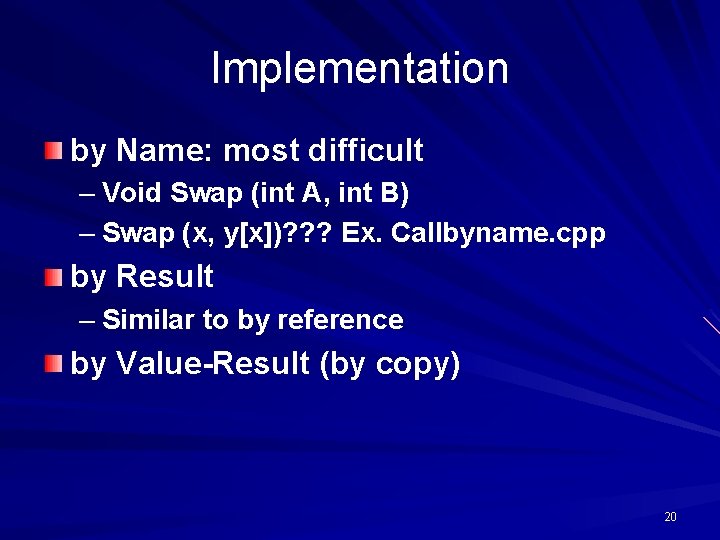
Implementation by Name: most difficult – Void Swap (int A, int B) – Swap (x, y[x])? ? ? Ex. Callbyname. cpp by Result – Similar to by reference by Value-Result (by copy) 20
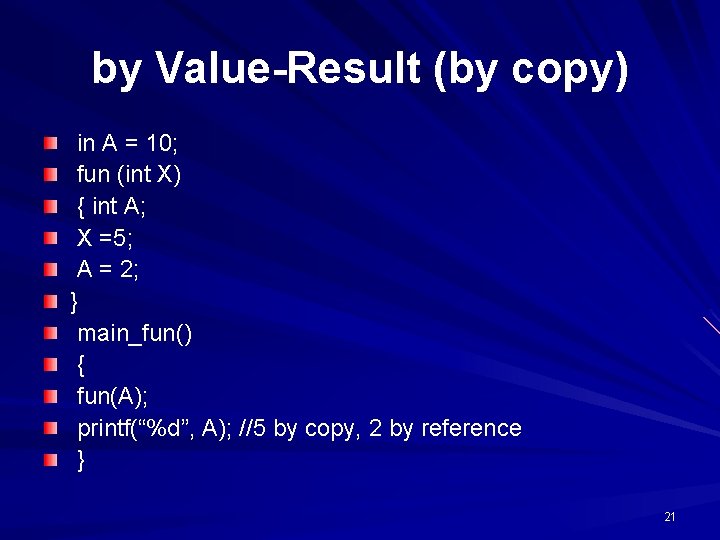
by Value-Result (by copy) in A = 10; fun (int X) { int A; X =5; A = 2; } main_fun() { fun(A); printf(“%d”, A); //5 by copy, 2 by reference } 21
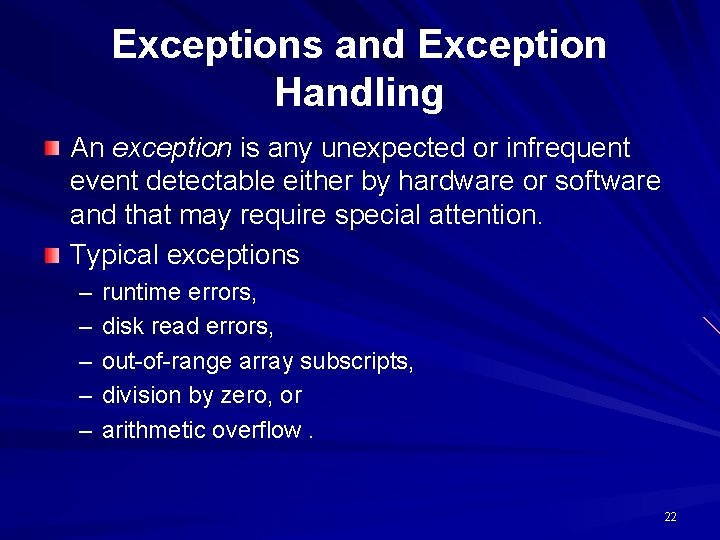
Exceptions and Exception Handling An exception is any unexpected or infrequent event detectable either by hardware or software and that may require special attention. Typical exceptions – – – runtime errors, disk read errors, out-of-range array subscripts, division by zero, or arithmetic overflow. 22
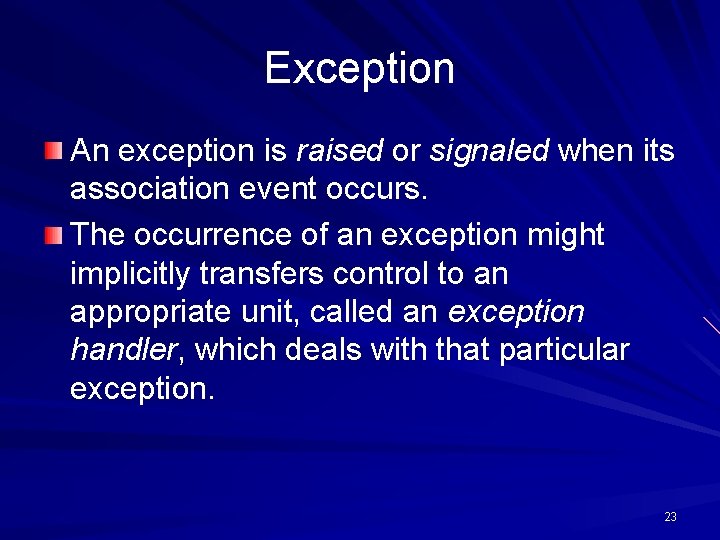
Exception An exception is raised or signaled when its association event occurs. The occurrence of an exception might implicitly transfers control to an appropriate unit, called an exception handler, which deals with that particular exception. 23
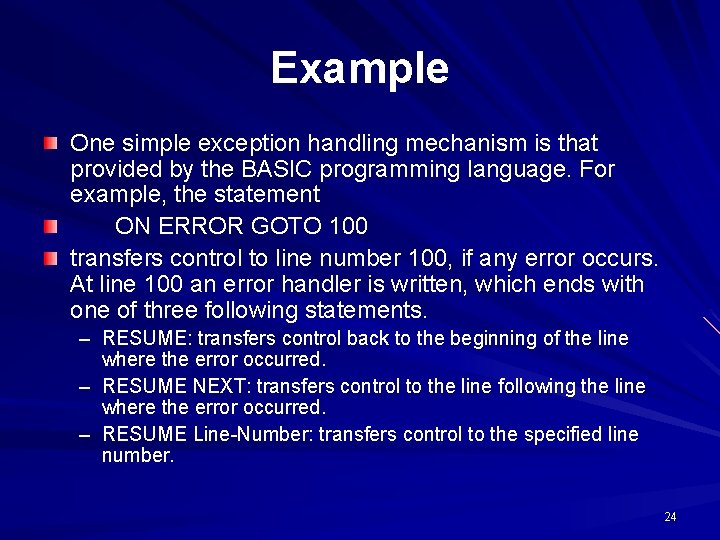
Example One simple exception handling mechanism is that provided by the BASIC programming language. For example, the statement ON ERROR GOTO 100 transfers control to line number 100, if any error occurs. At line 100 an error handler is written, which ends with one of three following statements. – RESUME: transfers control back to the beginning of the line where the error occurred. – RESUME NEXT: transfers control to the line following the line where the error occurred. – RESUME Line-Number: transfers control to the specified line number. 24
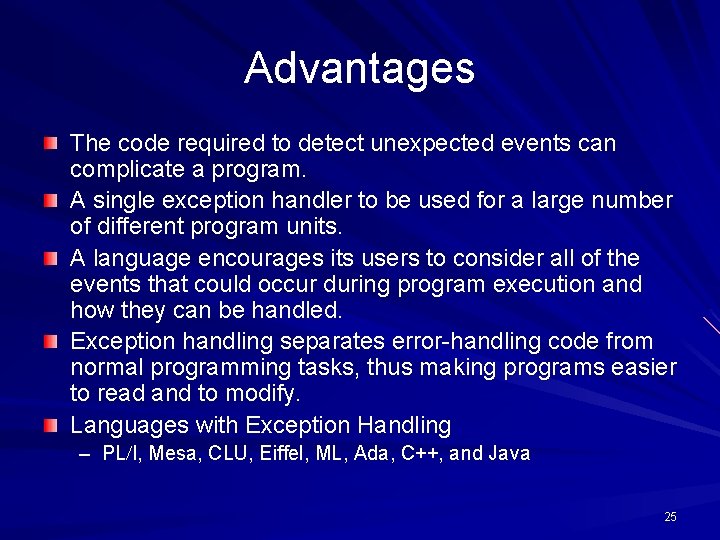
Advantages The code required to detect unexpected events can complicate a program. A single exception handler to be used for a large number of different program units. A language encourages its users to consider all of the events that could occur during program execution and how they can be handled. Exception handling separates error-handling code from normal programming tasks, thus making programs easier to read and to modify. Languages with Exception Handling – PL I, Mesa, CLU, Eiffel, ML, Ada, C++, and Java 25
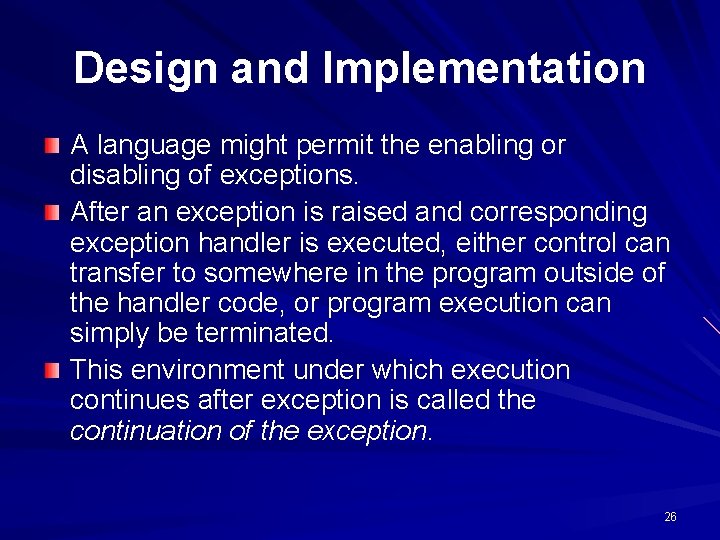
Design and Implementation A language might permit the enabling or disabling of exceptions. After an exception is raised and corresponding exception handler is executed, either control can transfer to somewhere in the program outside of the handler code, or program execution can simply be terminated. This environment under which execution continues after exception is called the continuation of the exception. 26
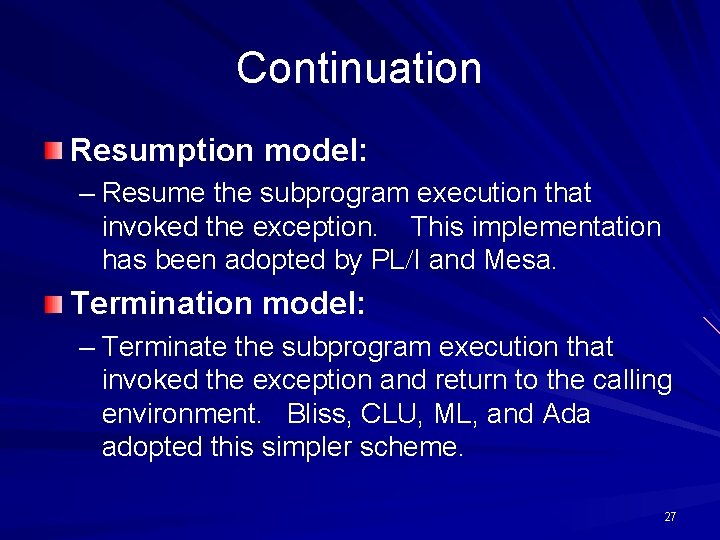
Continuation Resumption model: – Resume the subprogram execution that invoked the exception. This implementation has been adopted by PL I and Mesa. Termination model: – Terminate the subprogram execution that invoked the exception and return to the calling environment. Bliss, CLU, ML, and Ada adopted this simpler scheme. 27
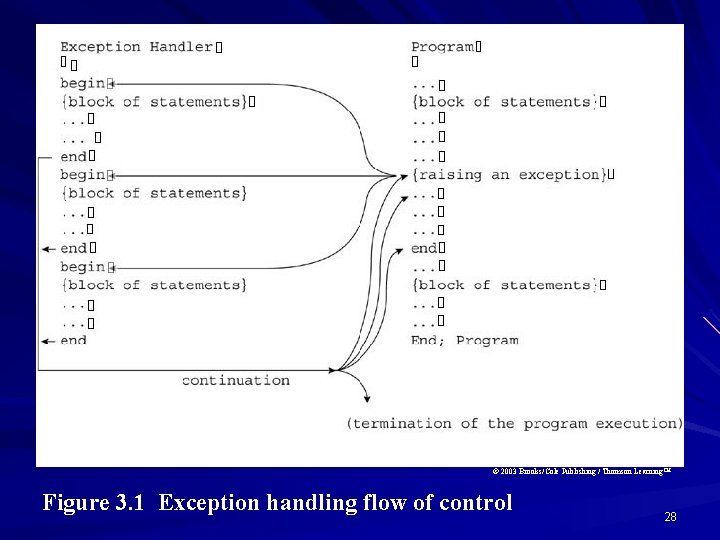
© 2003 Brooks/Cole Publishing / Thomson Learning™ Figure 3. 1 Exception handling flow of control 28
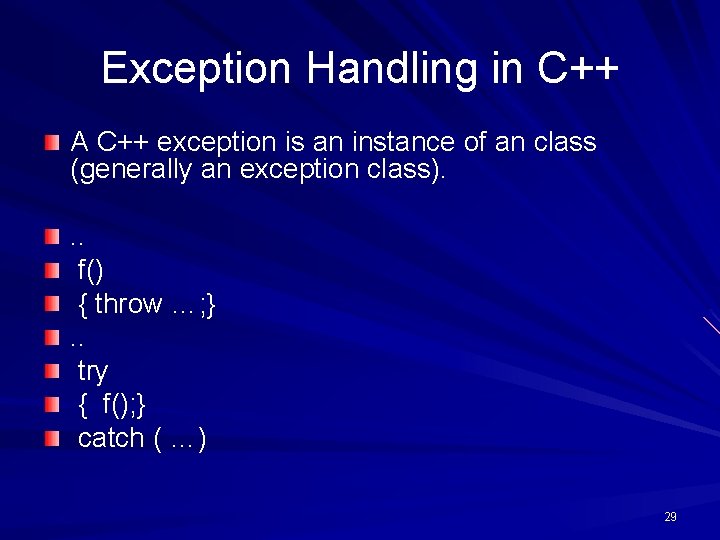
Exception Handling in C++ A C++ exception is an instance of an class (generally an exception class). . . f() { throw …; }. . try { f(); } catch ( …) 29
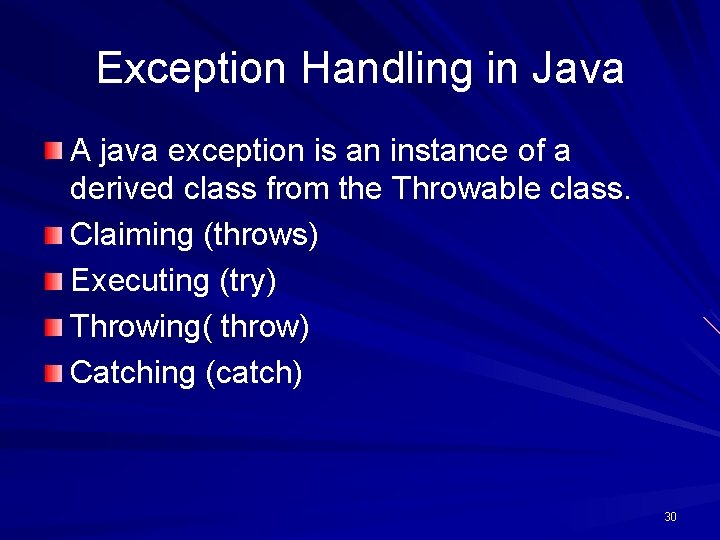
Exception Handling in Java A java exception is an instance of a derived class from the Throwable class. Claiming (throws) Executing (try) Throwing( throw) Catching (catch) 30
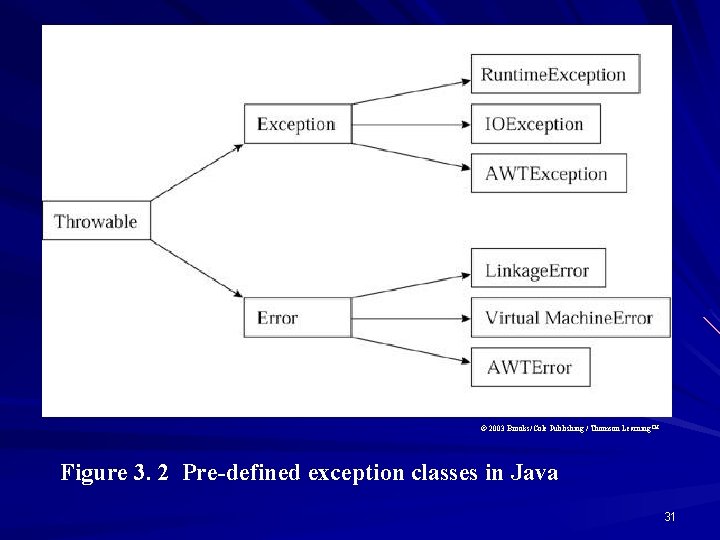
© 2003 Brooks/Cole Publishing / Thomson Learning™ Figure 3. 2 Pre-defined exception classes in Java 31
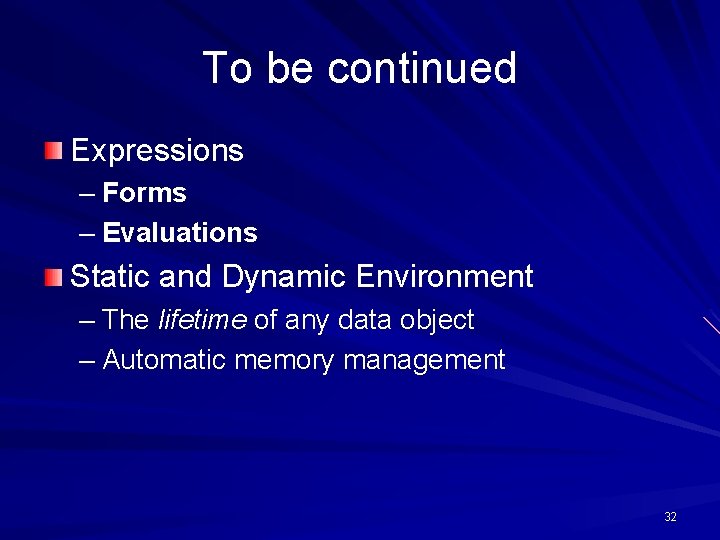
To be continued Expressions – Forms – Evaluations Static and Dynamic Environment – The lifetime of any data object – Automatic memory management 32
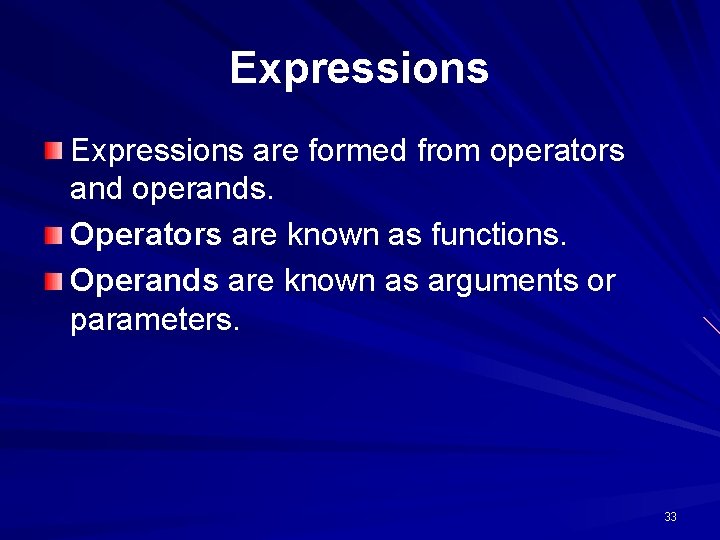
Expressions are formed from operators and operands. Operators are known as functions. Operands are known as arguments or parameters. 33
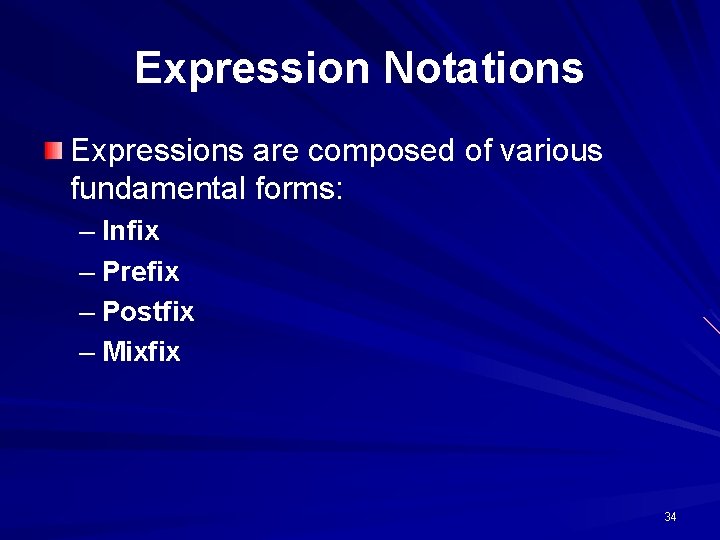
Expression Notations Expressions are composed of various fundamental forms: – Infix – Prefix – Postfix – Mixfix 34
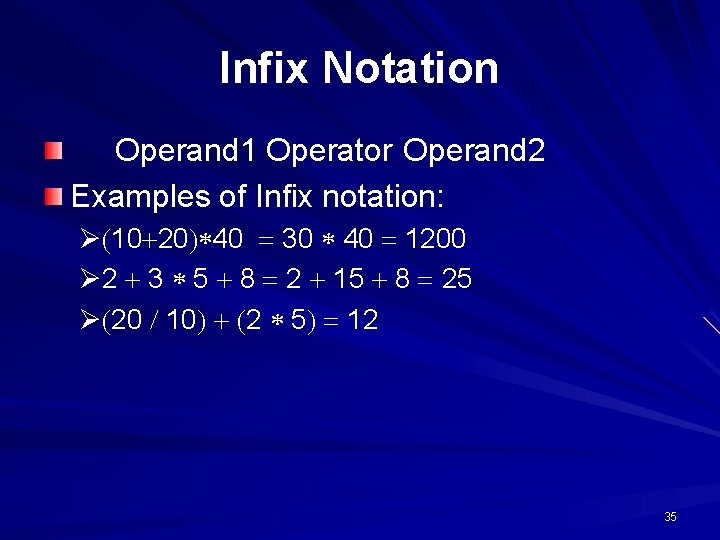
Infix Notation Operand 1 Operator Operand 2 Examples of Infix notation: Ø 10 20 40 30 40 1200 Ø 2 3 5 8 2 15 8 25 Ø 20 10 2 5 12 35
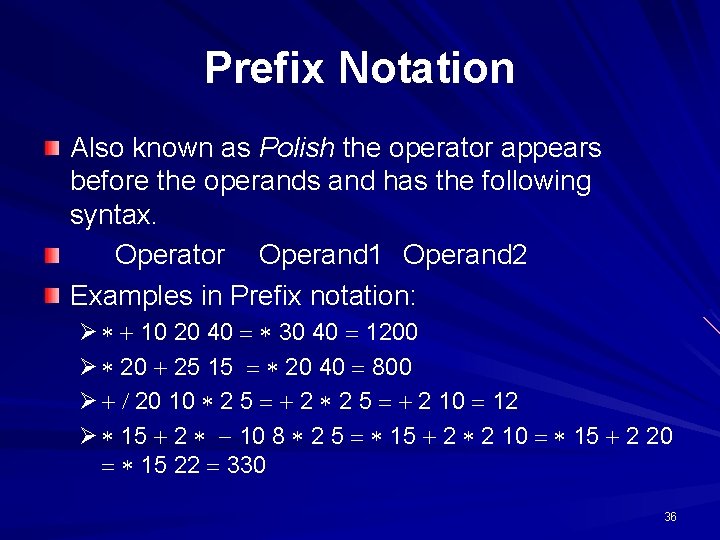
Prefix Notation Also known as Polish the operator appears before the operands and has the following syntax. Operator Operand 1 Operand 2 Examples in Prefix notation: Ø 10 20 40 30 40 1200 Ø 20 25 15 20 40 800 Ø 20 10 2 5 2 10 12 Ø 15 2 10 8 2 5 15 2 2 10 15 2 20 15 22 330 36
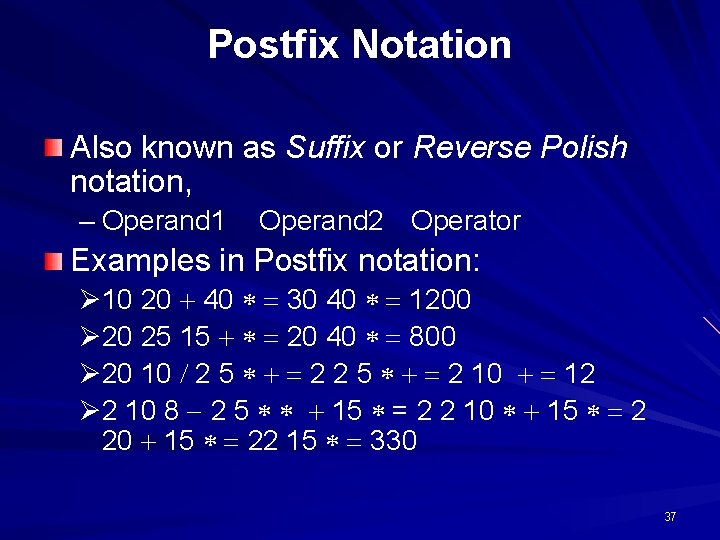
Postfix Notation Also known as Suffix or Reverse Polish notation, – Operand 1 Operand 2 Operator Examples in Postfix notation: Ø 10 20 40 30 40 1200 Ø 20 25 15 20 40 800 Ø 20 10 2 5 2 10 12 Ø 2 10 8 2 5 15 = 2 2 10 15 2 20 15 22 15 330 37
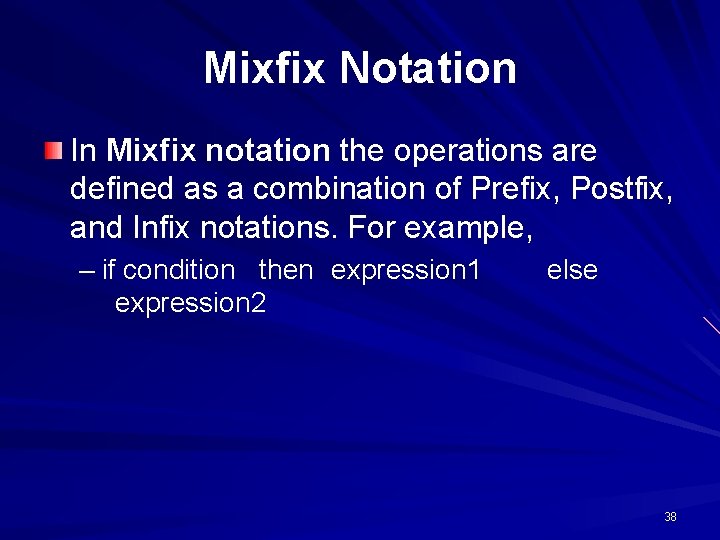
Mixfix Notation In Mixfix notation the operations are defined as a combination of Prefix, Postfix, and Infix notations. For example, – if condition then expression 1 expression 2 else 38
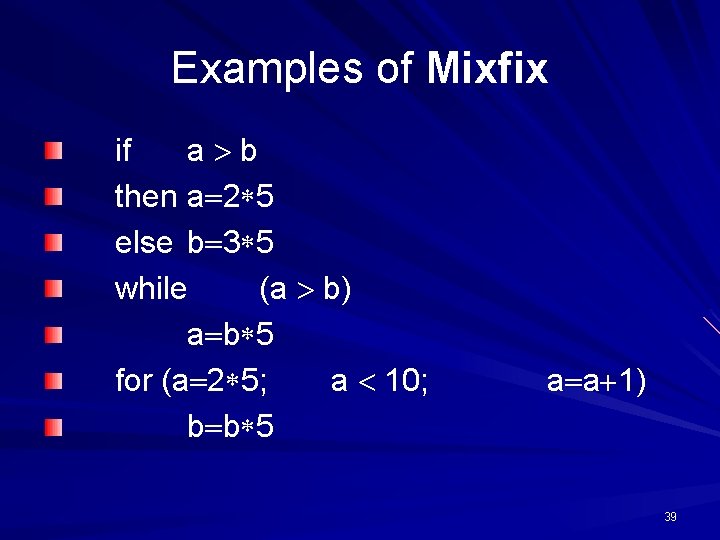
Examples of Mixfix if a b then a 2 5 else b 3 5 while (a b) a b 5 for (a 2 5; a 10; b b 5 a a 1) 39
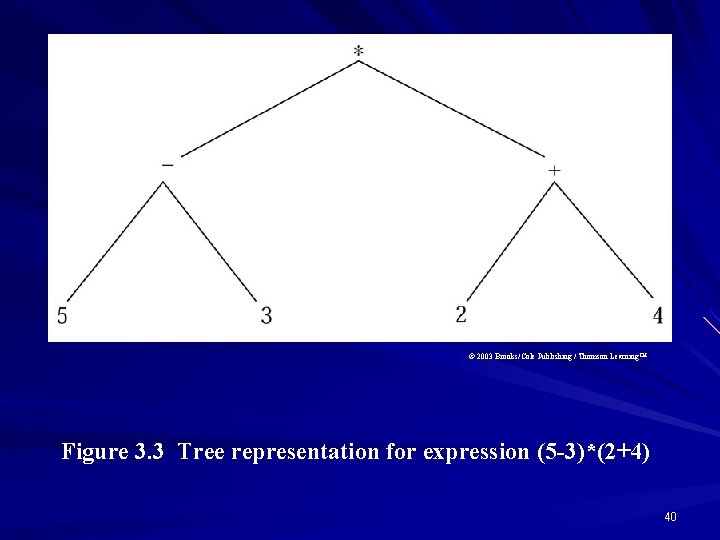
© 2003 Brooks/Cole Publishing / Thomson Learning™ Figure 3. 3 Tree representation for expression (5 -3)*(2+4) 40
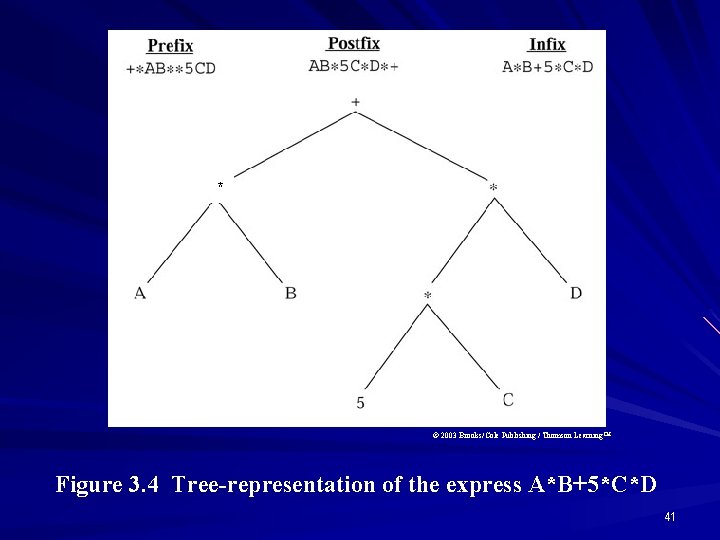
* © 2003 Brooks/Cole Publishing / Thomson Learning™ Figure 3. 4 Tree-representation of the express A*B+5*C*D 41
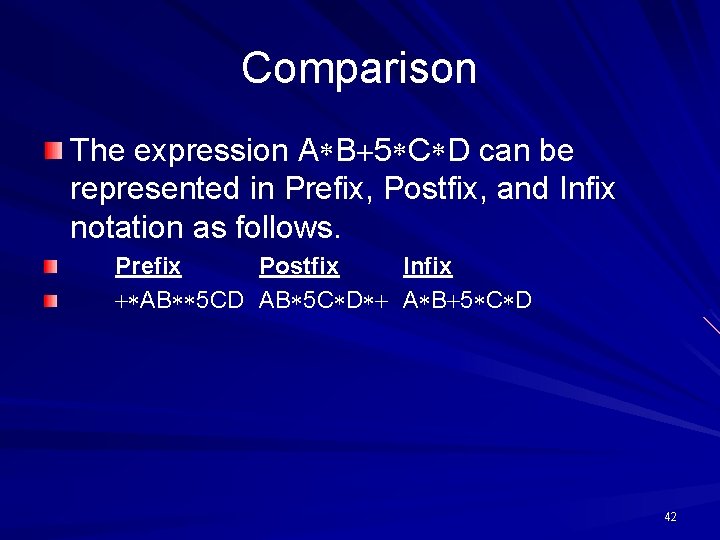
Comparison The expression A B 5 C D can be represented in Prefix, Postfix, and Infix notation as follows. Prefix Postfix Infix AB 5 CD AB 5 C D A B 5 C D 42
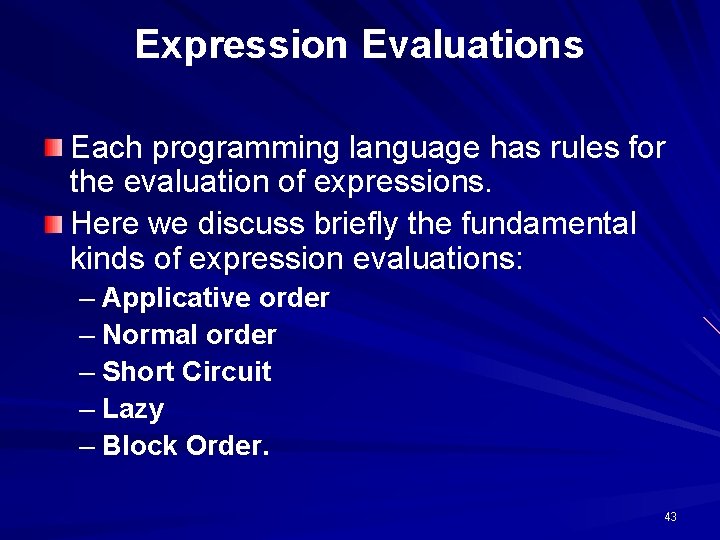
Expression Evaluations Each programming language has rules for the evaluation of expressions. Here we discuss briefly the fundamental kinds of expression evaluations: – Applicative order – Normal order – Short Circuit – Lazy – Block Order. 43
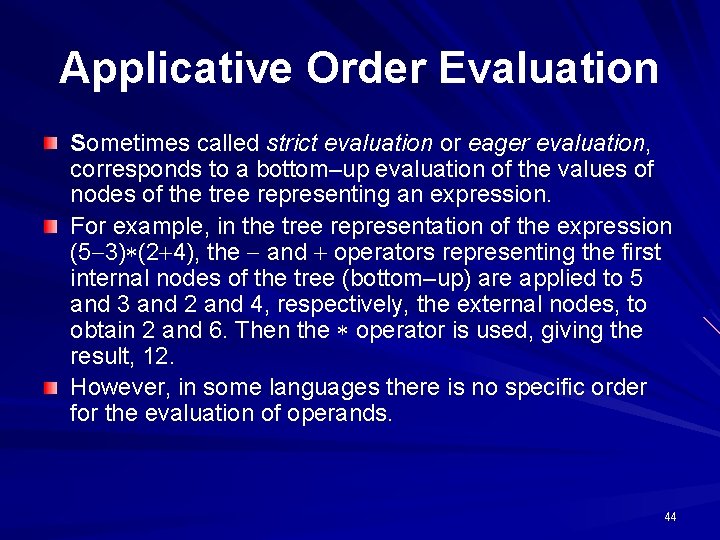
Applicative Order Evaluation Sometimes called strict evaluation or eager evaluation, corresponds to a bottom–up evaluation of the values of nodes of the tree representing an expression. For example, in the tree representation of the expression (5 3) (2 4), the and operators representing the first internal nodes of the tree (bottom–up) are applied to 5 and 3 and 2 and 4, respectively, the external nodes, to obtain 2 and 6. Then the operator is used, giving the result, 12. However, in some languages there is no specific order for the evaluation of operands. 44
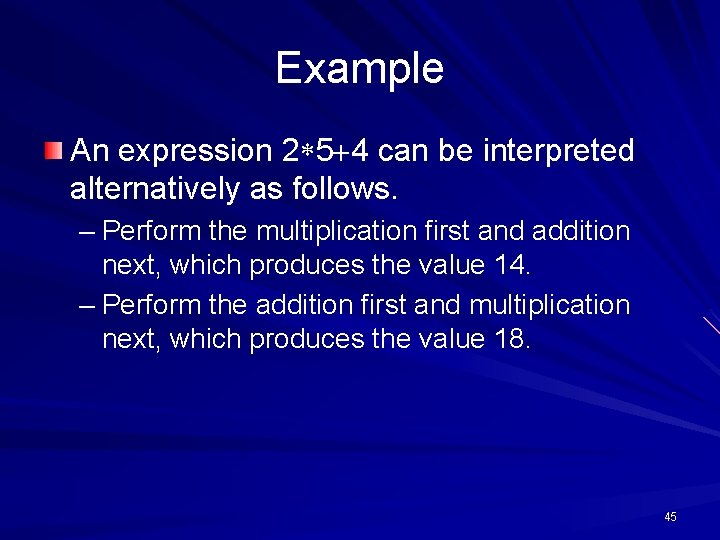
Example An expression 2 5 4 can be interpreted alternatively as follows. – Perform the multiplication first and addition next, which produces the value 14. – Perform the addition first and multiplication next, which produces the value 18. 45
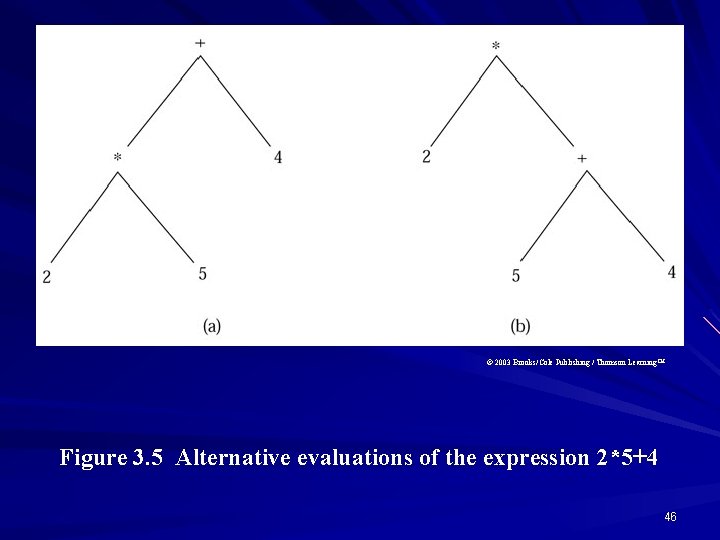
© 2003 Brooks/Cole Publishing / Thomson Learning™ Figure 3. 5 Alternative evaluations of the expression 2*5+4 46
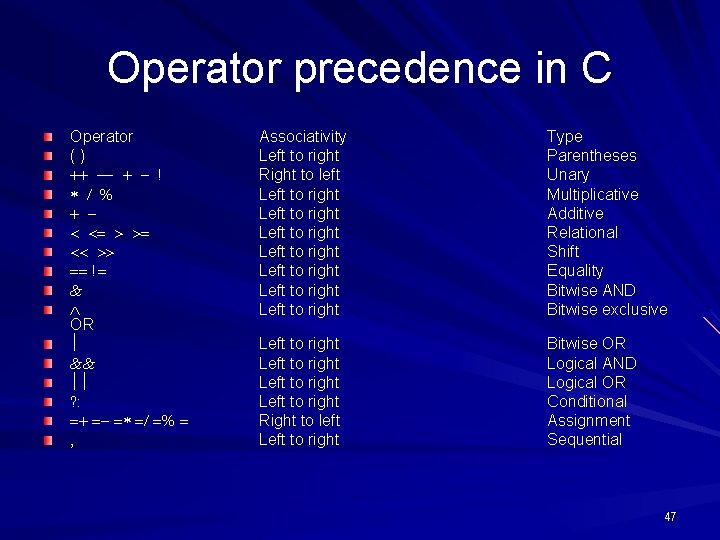
Operator precedence in C Operator () OR Associativity Left to right Right to left Left to right Left to right Type Parentheses Unary Multiplicative Additive Relational Shift Equality Bitwise AND Bitwise exclusive Left to right Right to left Left to right Bitwise OR Logical AND Logical OR Conditional Assignment Sequential 47
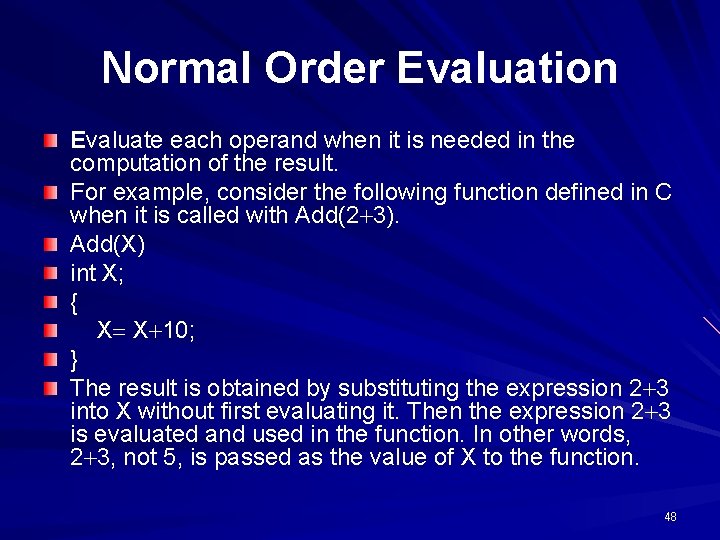
Normal Order Evaluation Evaluate each operand when it is needed in the computation of the result. For example, consider the following function defined in C when it is called with Add(2 3). Add(X) int X; { X X 10; } The result is obtained by substituting the expression 2 3 into X without first evaluating it. Then the expression 2 3 is evaluated and used in the function. In other words, 2 3, not 5, is passed as the value of X to the function. 48
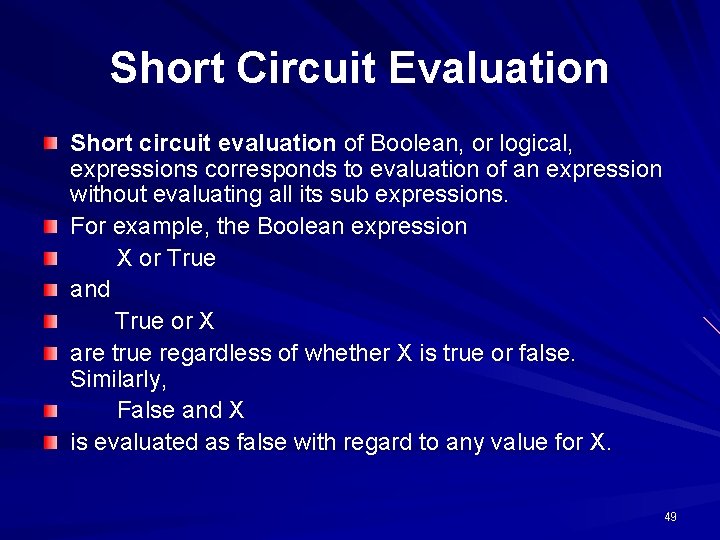
Short Circuit Evaluation Short circuit evaluation of Boolean, or logical, expressions corresponds to evaluation of an expression without evaluating all its sub expressions. For example, the Boolean expression X or True and True or X are true regardless of whether X is true or false. Similarly, False and X is evaluated as false with regard to any value for X. 49
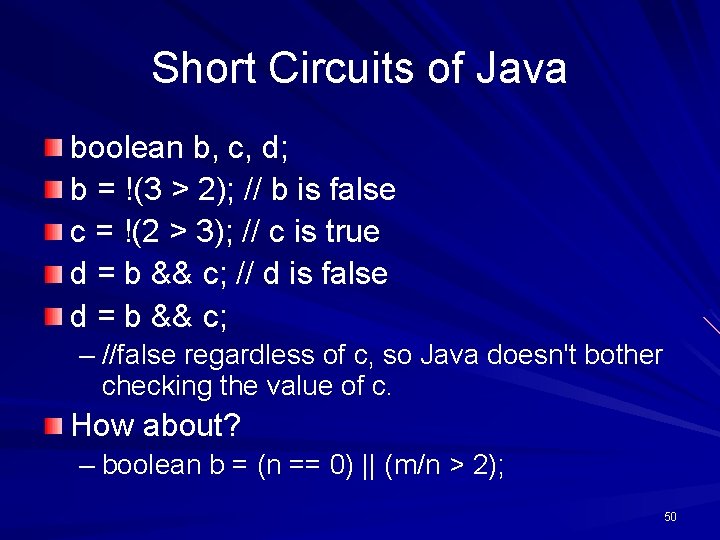
Short Circuits of Java boolean b, c, d; b = !(3 > 2); // b is false c = !(2 > 3); // c is true d = b && c; // d is false d = b && c; – //false regardless of c, so Java doesn't bother checking the value of c. How about? – boolean b = (n == 0) || (m/n > 2); 50
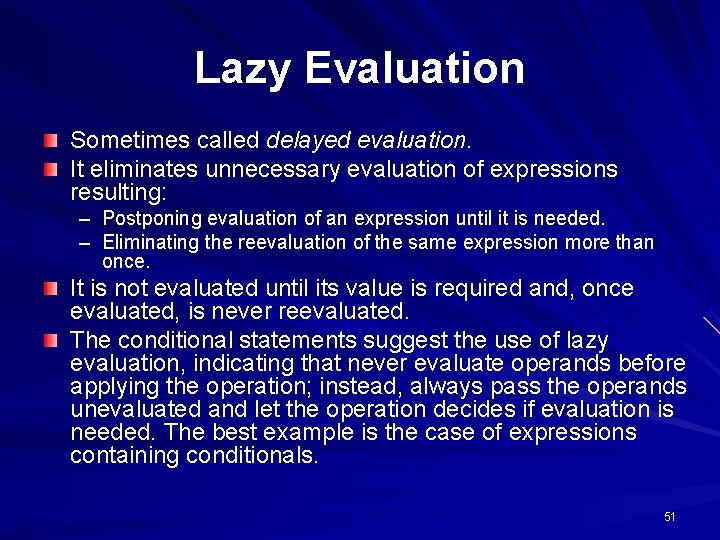
Lazy Evaluation Sometimes called delayed evaluation. It eliminates unnecessary evaluation of expressions resulting: – Postponing evaluation of an expression until it is needed. – Eliminating the reevaluation of the same expression more than once. It is not evaluated until its value is required and, once evaluated, is never reevaluated. The conditional statements suggest the use of lazy evaluation, indicating that never evaluate operands before applying the operation; instead, always pass the operands unevaluated and let the operation decides if evaluation is needed. The best example is the case of expressions containing conditionals. 51
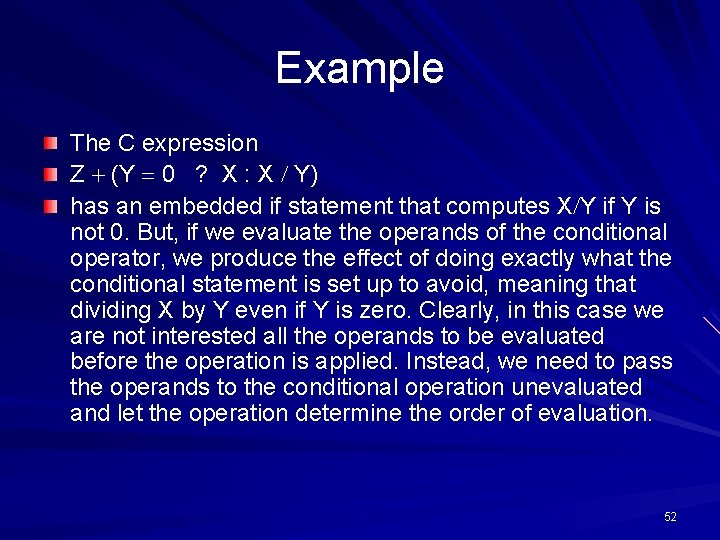
Example The C expression Z (Y 0 ? X : X Y) has an embedded if statement that computes X Y if Y is not 0. But, if we evaluate the operands of the conditional operator, we produce the effect of doing exactly what the conditional statement is set up to avoid, meaning that dividing X by Y even if Y is zero. Clearly, in this case we are not interested all the operands to be evaluated before the operation is applied. Instead, we need to pass the operands to the conditional operation unevaluated and let the operation determine the order of evaluation. 52
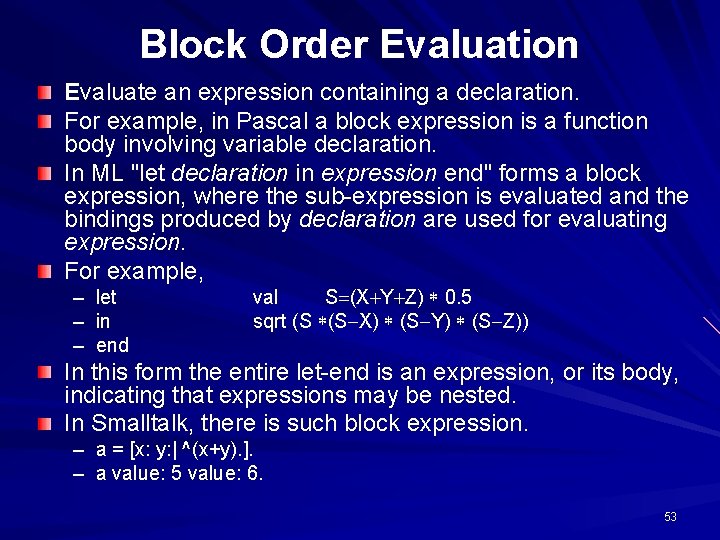
Block Order Evaluation Evaluate an expression containing a declaration. For example, in Pascal a block expression is a function body involving variable declaration. In ML "let declaration in expression end" forms a block expression, where the sub-expression is evaluated and the bindings produced by declaration are used for evaluating expression. For example, – – – let in end val S (X Y Z) 0. 5 sqrt (S (S X) (S Y) (S Z)) In this form the entire let-end is an expression, or its body, indicating that expressions may be nested. In Smalltalk, there is such block expression. – a = [x: y: | ^(x+y). ]. – a value: 5 value: 6. 53
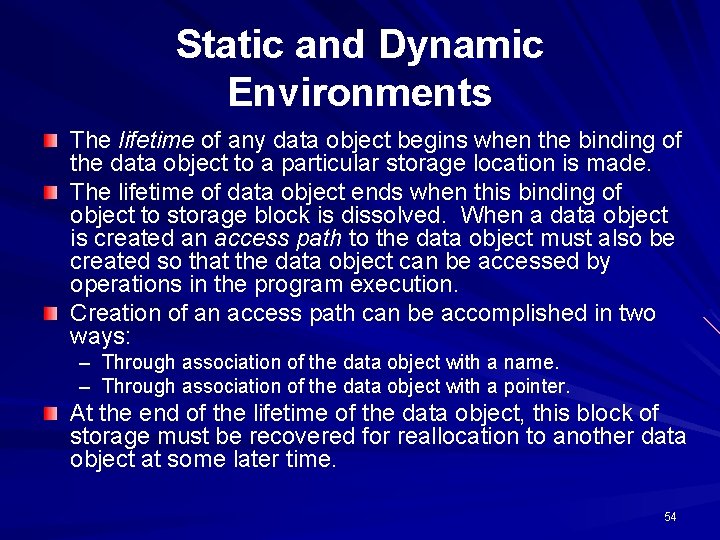
Static and Dynamic Environments The lifetime of any data object begins when the binding of the data object to a particular storage location is made. The lifetime of data object ends when this binding of object to storage block is dissolved. When a data object is created an access path to the data object must also be created so that the data object can be accessed by operations in the program execution. Creation of an access path can be accomplished in two ways: – Through association of the data object with a name. – Through association of the data object with a pointer. At the end of the lifetime of the data object, this block of storage must be recovered for reallocation to another data object at some later time. 54
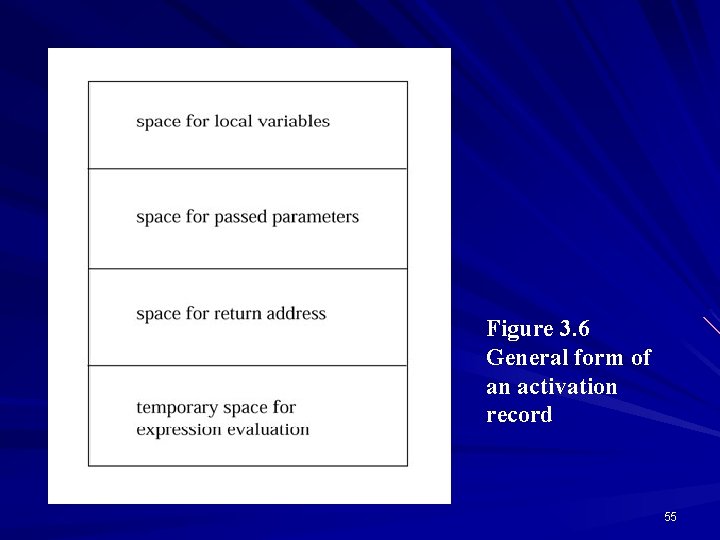
Figure 3. 6 General form of an activation record © 2003 Brooks/Cole Publishing / Thomson Learning™ 55
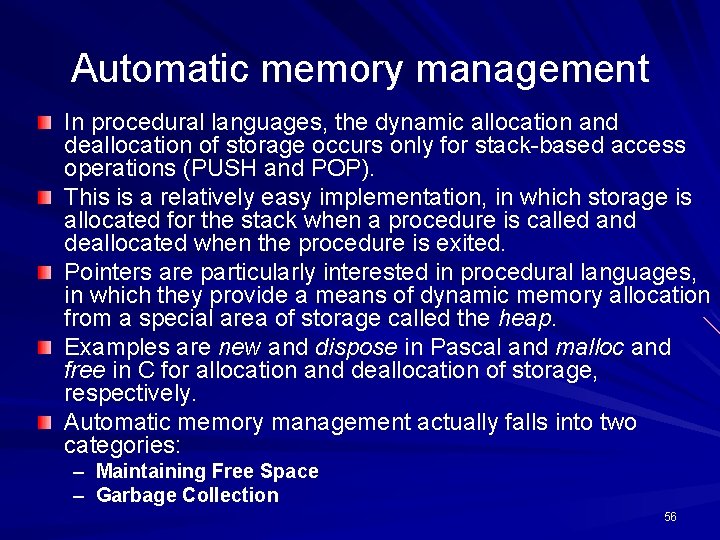
Automatic memory management In procedural languages, the dynamic allocation and deallocation of storage occurs only for stack-based access operations (PUSH and POP). This is a relatively easy implementation, in which storage is allocated for the stack when a procedure is called and deallocated when the procedure is exited. Pointers are particularly interested in procedural languages, in which they provide a means of dynamic memory allocation from a special area of storage called the heap. Examples are new and dispose in Pascal and malloc and free in C for allocation and deallocation of storage, respectively. Automatic memory management actually falls into two categories: – Maintaining Free Space – Garbage Collection 56
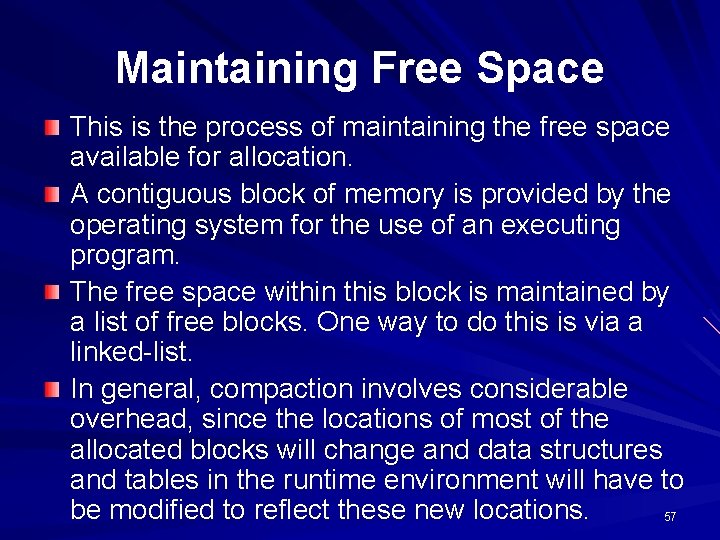
Maintaining Free Space This is the process of maintaining the free space available for allocation. A contiguous block of memory is provided by the operating system for the use of an executing program. The free space within this block is maintained by a list of free blocks. One way to do this is via a linked-list. In general, compaction involves considerable overhead, since the locations of most of the allocated blocks will change and data structures and tables in the runtime environment will have to be modified to reflect these new locations. 57
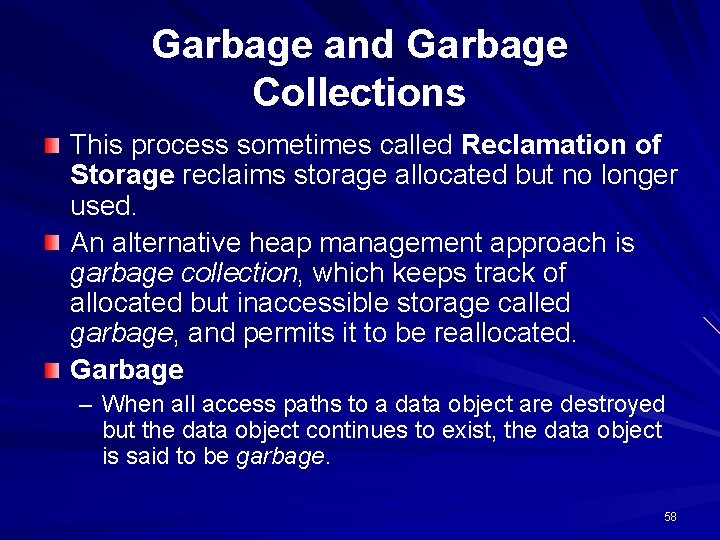
Garbage and Garbage Collections This process sometimes called Reclamation of Storage reclaims storage allocated but no longer used. An alternative heap management approach is garbage collection, which keeps track of allocated but inaccessible storage called garbage, and permits it to be reallocated. Garbage – When all access paths to a data object are destroyed but the data object continues to exist, the data object is said to be garbage. 58
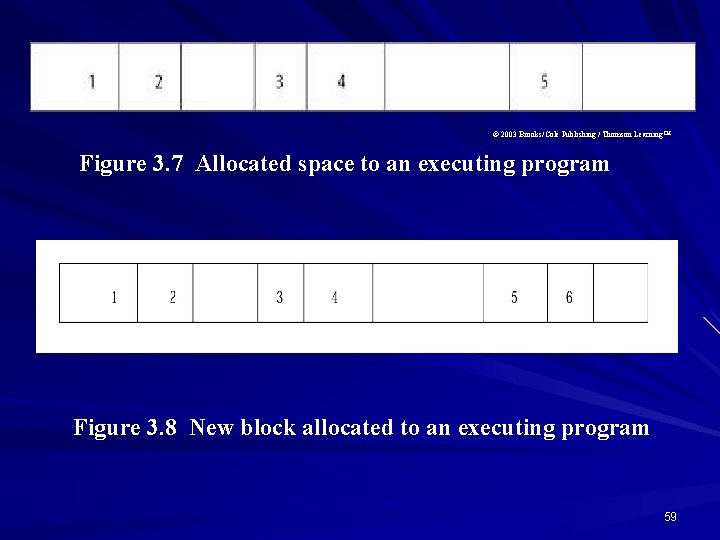
© 2003 Brooks/Cole Publishing / Thomson Learning™ Figure 3. 7 Allocated space to an executing program © 2003 Brooks/Cole Publishing / Thomson Learning™ Figure 3. 8 New block allocated to an executing program 59
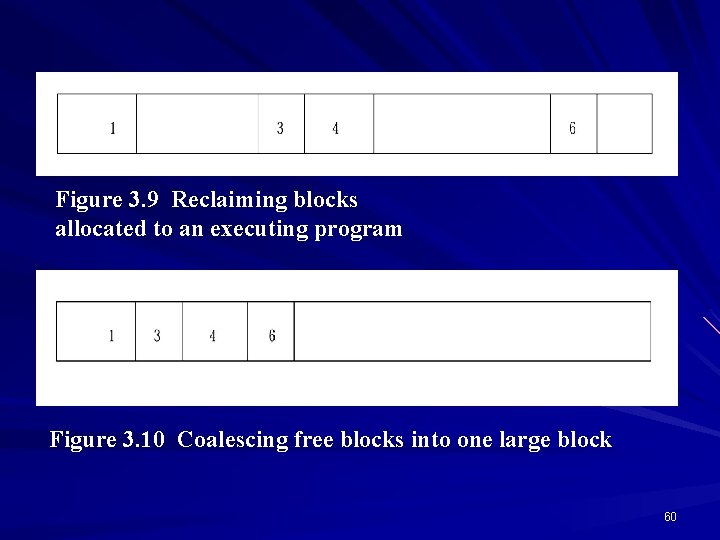
© 2003 Brooks/Cole Publishing / Thomson Learning™ Figure 3. 9 Reclaiming blocks allocated to an executing program © 2003 Brooks/Cole Publishing / Thomson Learning™ Figure 3. 10 Coalescing free blocks into one large block 60
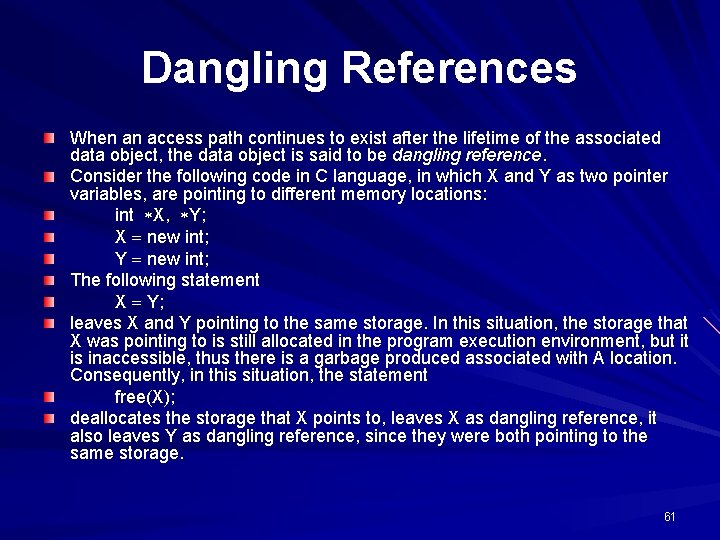
Dangling References When an access path continues to exist after the lifetime of the associated data object, the data object is said to be dangling reference. Consider the following code in C language, in which X and Y as two pointer variables, are pointing to different memory locations: int X, Y; X new int; Y new int; The following statement X Y; leaves X and Y pointing to the same storage. In this situation, the storage that X was pointing to is still allocated in the program execution environment, but it is inaccessible, thus there is a garbage produced associated with A location. Consequently, in this situation, the statement free(X); deallocates the storage that X points to, leaves X as dangling reference, it also leaves Y as dangling reference, since they were both pointing to the same storage. 61
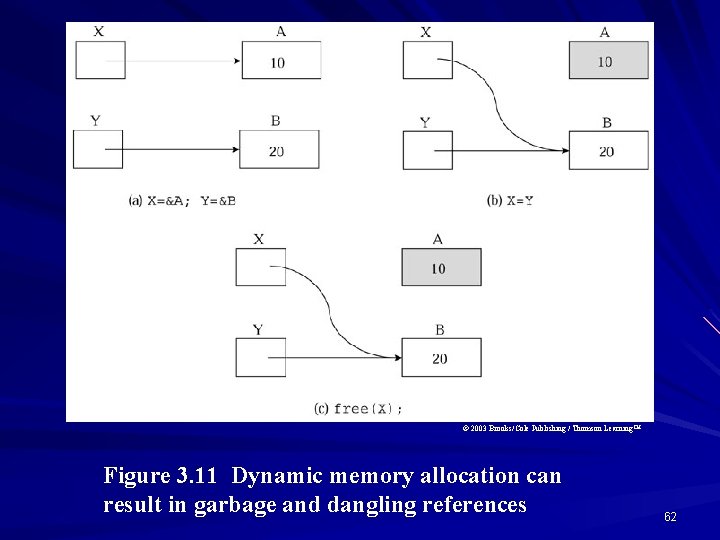
© 2003 Brooks/Cole Publishing / Thomson Learning™ Figure 3. 11 Dynamic memory allocation can result in garbage and dangling references 62
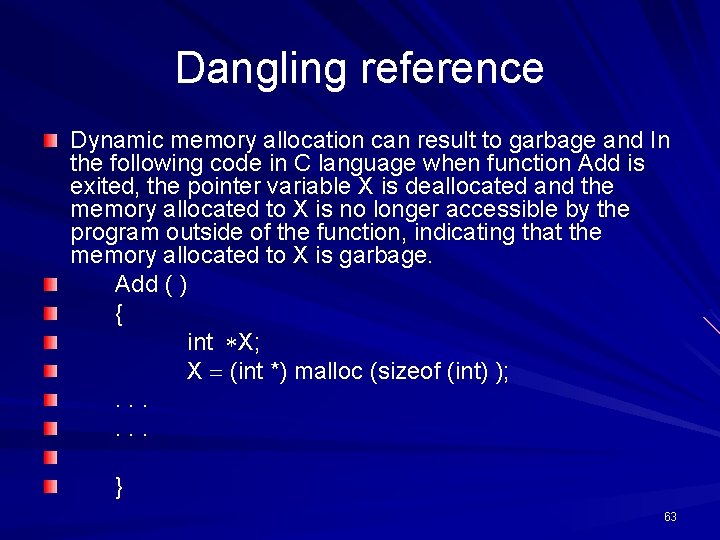
Dangling reference Dynamic memory allocation can result to garbage and In the following code in C language when function Add is exited, the pointer variable X is deallocated and the memory allocated to X is no longer accessible by the program outside of the function, indicating that the memory allocated to X is garbage. Add ( ) { int X; X (int *) malloc (sizeof (int) ); . . . } 63
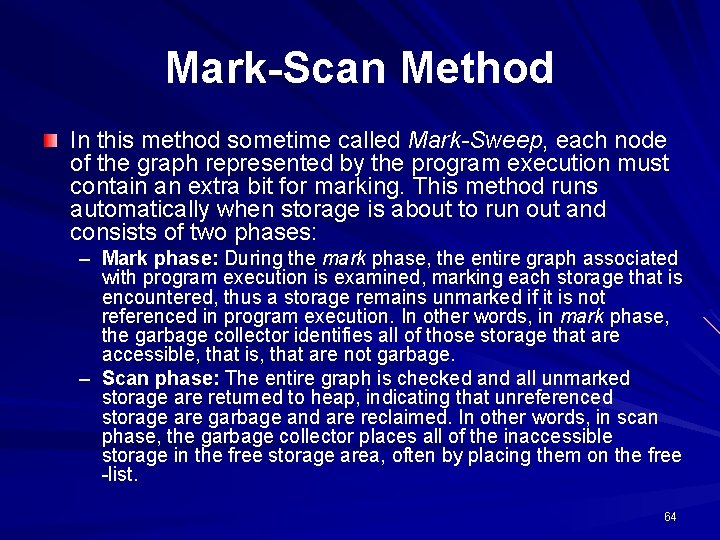
Mark-Scan Method In this method sometime called Mark-Sweep, each node of the graph represented by the program execution must contain an extra bit for marking. This method runs automatically when storage is about to run out and consists of two phases: – Mark phase: During the mark phase, the entire graph associated with program execution is examined, marking each storage that is encountered, thus a storage remains unmarked if it is not referenced in program execution. In other words, in mark phase, the garbage collector identifies all of those storage that are accessible, that is, that are not garbage. – Scan phase: The entire graph is checked and all unmarked storage are returned to heap, indicating that unreferenced storage are garbage and are reclaimed. In other words, in scan phase, the garbage collector places all of the inaccessible storage in the free storage area, often by placing them on the free -list. 64
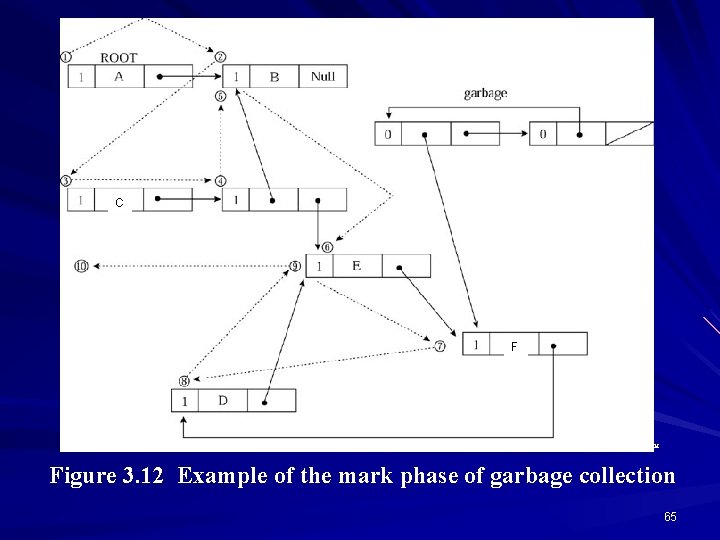
C F © 2003 Brooks/Cole Publishing / Thomson Learning™ Figure 3. 12 Example of the mark phase of garbage collection 65
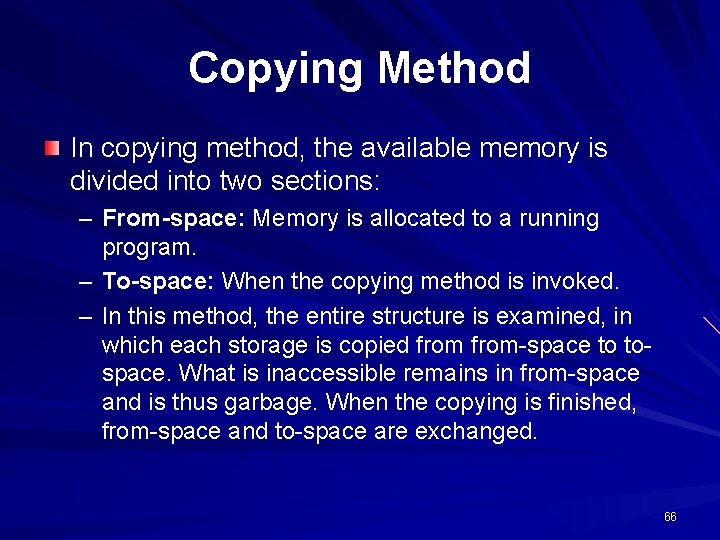
Copying Method In copying method, the available memory is divided into two sections: – From-space: Memory is allocated to a running program. – To-space: When the copying method is invoked. – In this method, the entire structure is examined, in which each storage is copied from-space to tospace. What is inaccessible remains in from-space and is thus garbage. When the copying is finished, from-space and to-space are exchanged. 66
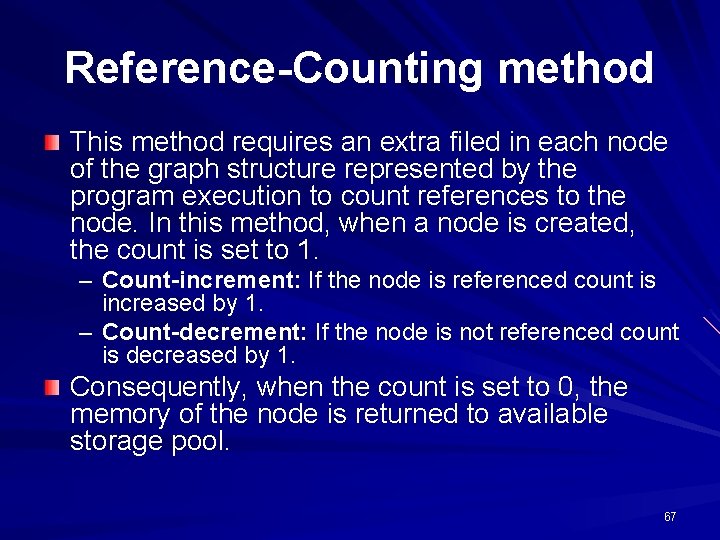
Reference-Counting method This method requires an extra filed in each node of the graph structure represented by the program execution to count references to the node. In this method, when a node is created, the count is set to 1. – Count-increment: If the node is referenced count is increased by 1. – Count-decrement: If the node is not referenced count is decreased by 1. Consequently, when the count is set to 0, the memory of the node is returned to available storage pool. 67
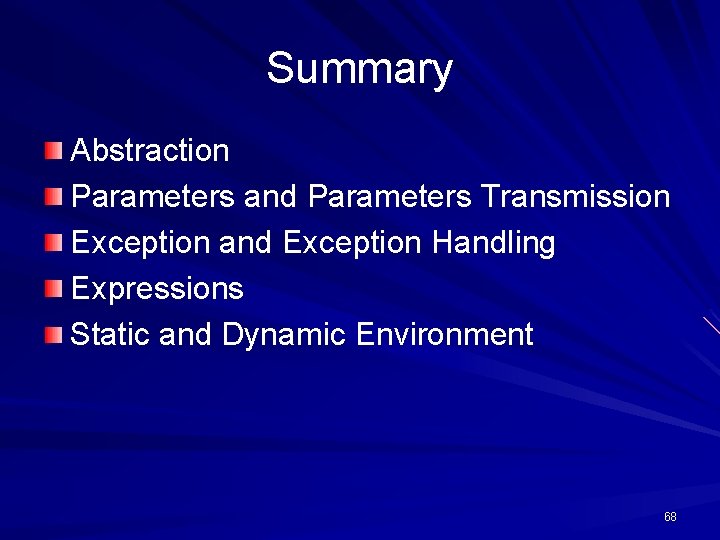
Summary Abstraction Parameters and Parameters Transmission Exception and Exception Handling Expressions Static and Dynamic Environment 68
Binding in programming paradigms
Ktu programming paradigms notes
R programming language paradigms
01:640:244 lecture notes - lecture 15: plat, idah, farad
Design specification
C data types with examples
Cosc 4p61
Cosc 4p42
Cosc 3p91
Cosc 1306
Cosc 1306
Cosc 4368
Cosc 4p41
Cosc 4p41
Cosc
Cosc 3340
Cosc 320
Cosc parameters
Cosc 2p12
Adt functional programming
Cosc 3340
Cosc 3p92
Cosc 121
Cosc 1p02
Cosc 2p12
Cosc 3p92
1 bit alu
Cosc 121
Cosc 121
Cosc 121
Cosc 121
Cosc -cos d
Cosc 1306
Cosc 3p94
Cosc101
Cosc 3340
Cosc 3340
Hall policy paradigms
Paradigms of cognitive psychology
Paradigm and principles
Vertical
Paradigms of interaction in hci
Distributed systems: principles and paradigms
Message ordering paradigms
Distributed systems principles and paradigms
Different computing paradigms
3 paradigms
Interpretivist approach to consumer behavior
Enemy centered paradigm
Alternative paradigm of development ppt
Issues for multimedia authoring
Decide evaluation framework
Biological paradigm of psychopathology
Php paradigms
Paradigms and principles
Distributed systems principles and paradigms
Paradigms of development
Distributed systems principles and paradigms
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
Components of system programming
Linear vs integer programming
Perbedaan linear programming dan integer programming
Eurocode 2 lap length table
Urban design lecture
Elements of design in interior design ppt
Lecture hall background
Game design lecture
Computer aided drug design lecture notes