Computer Organization CS 224 Fall 2012 Lessons 9
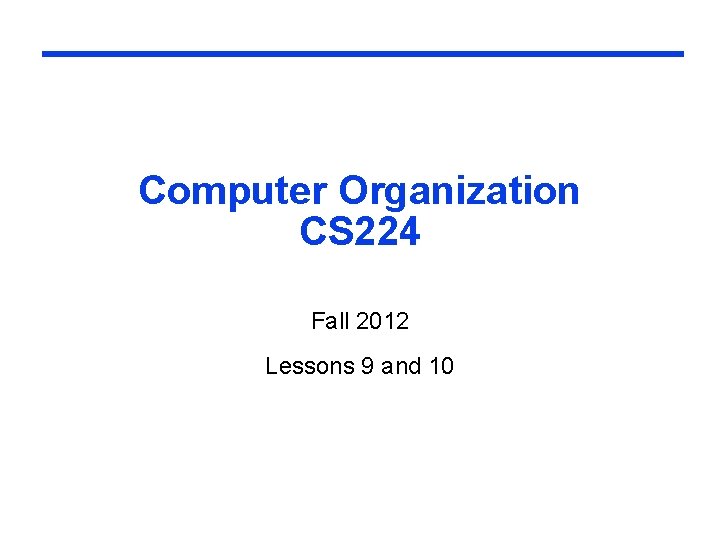
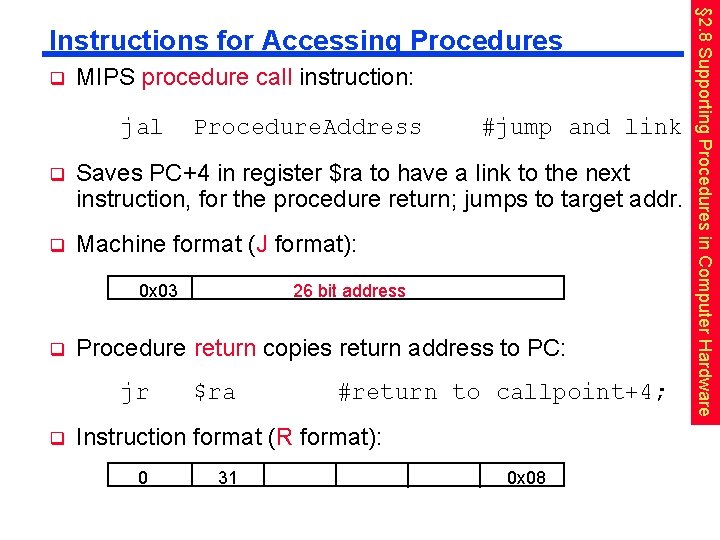
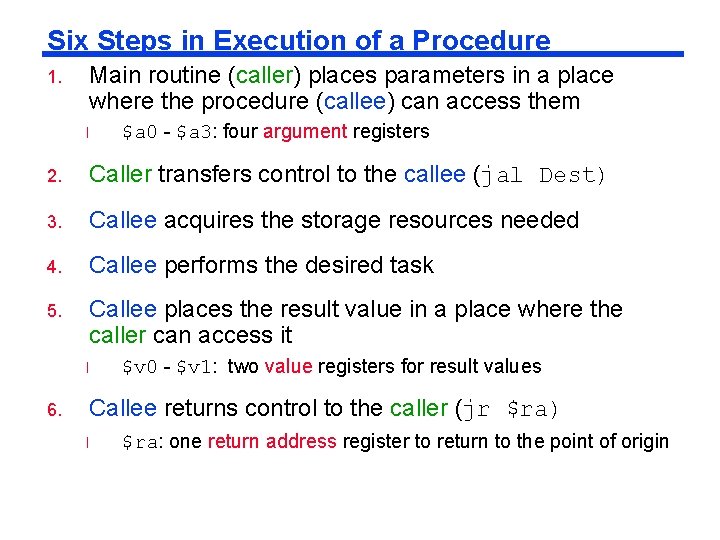
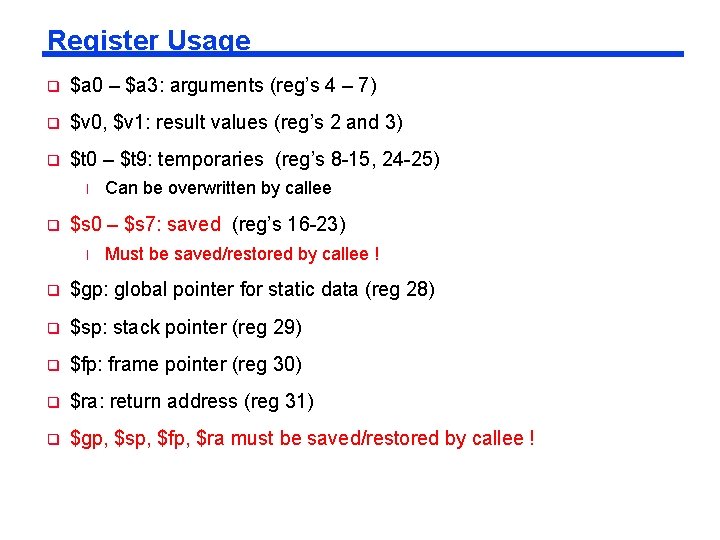
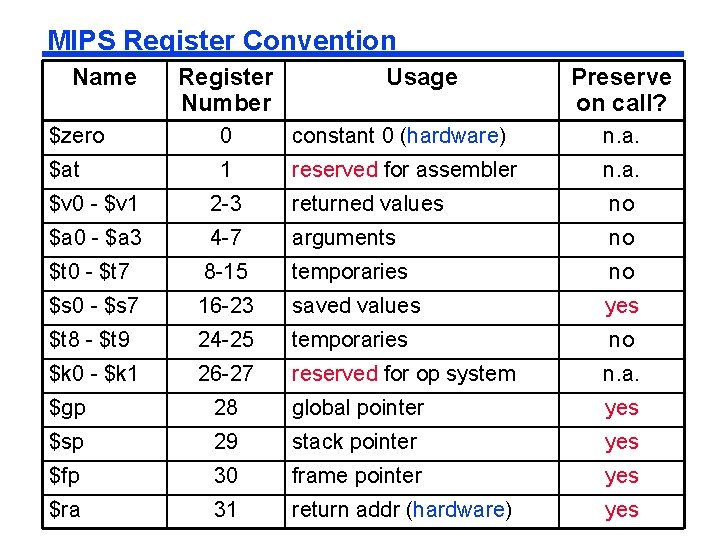
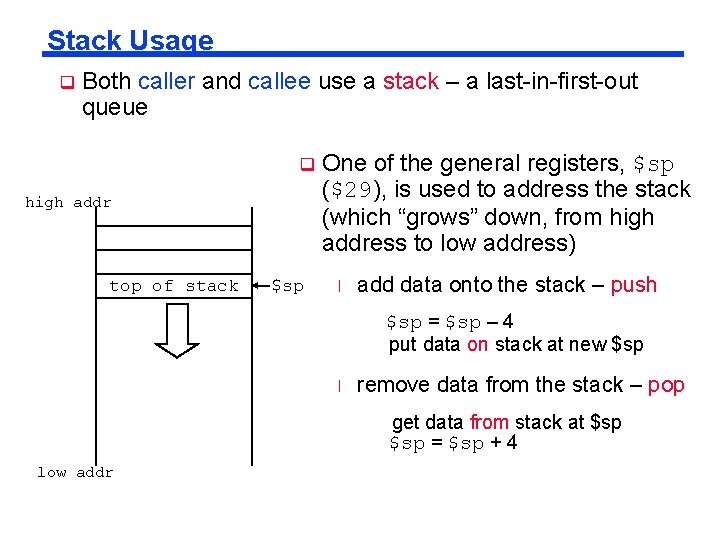
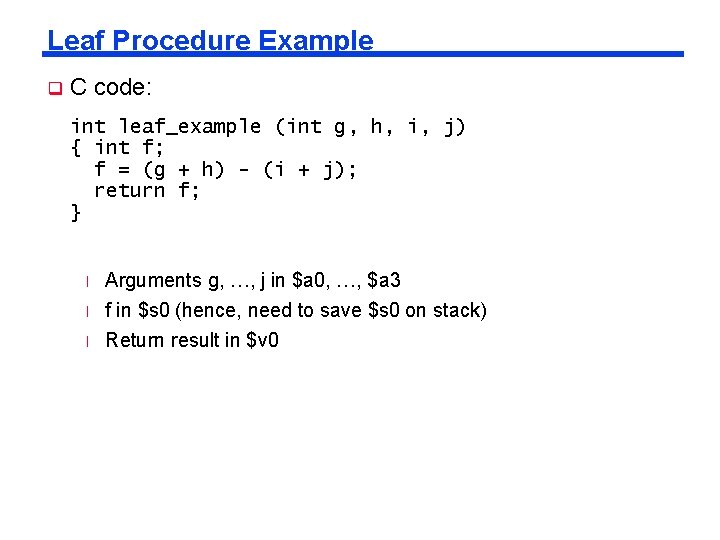
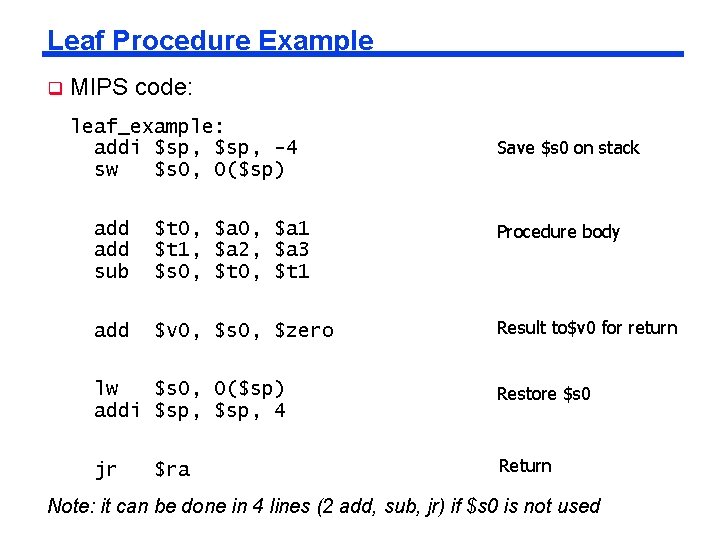
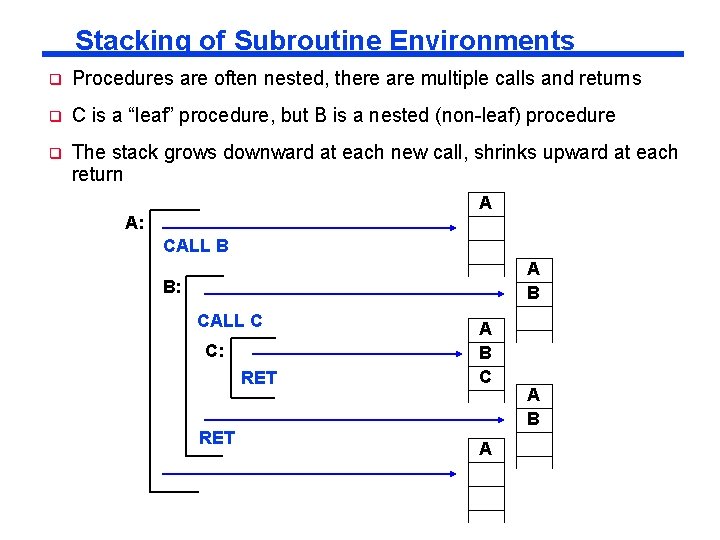
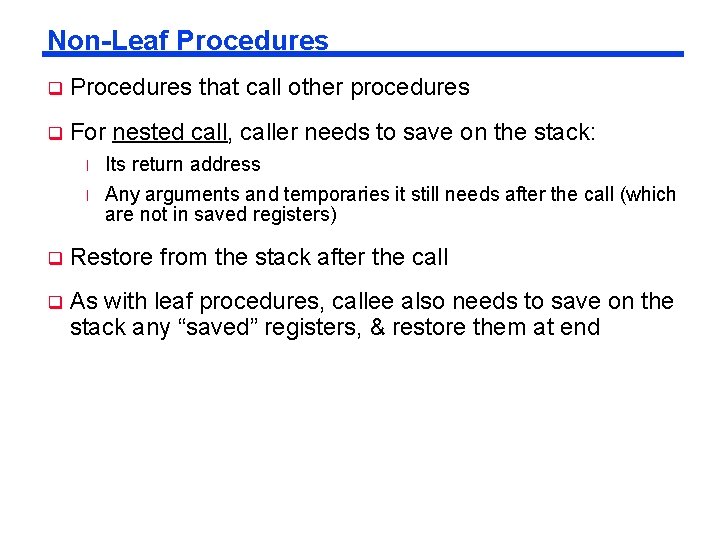
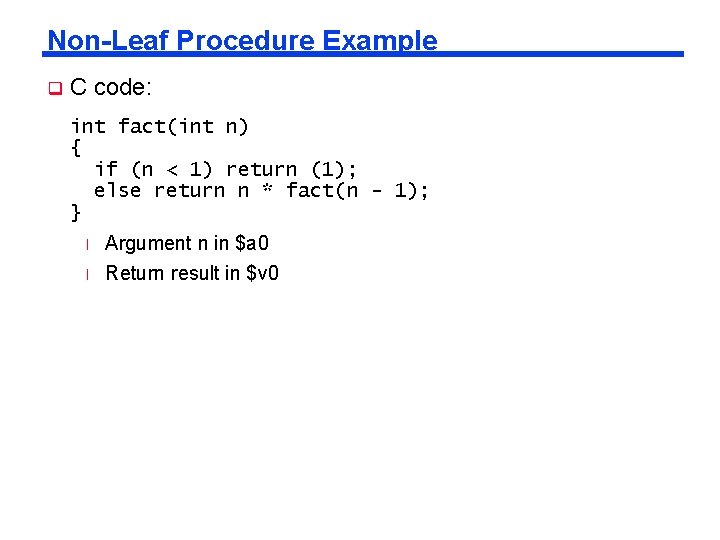
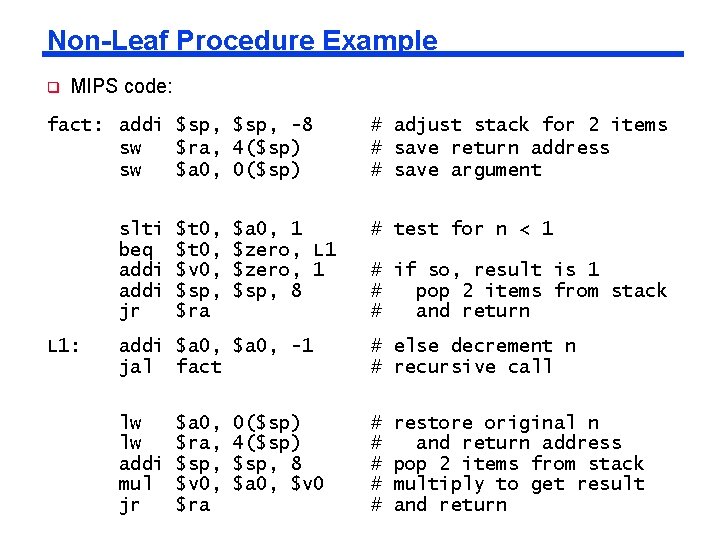
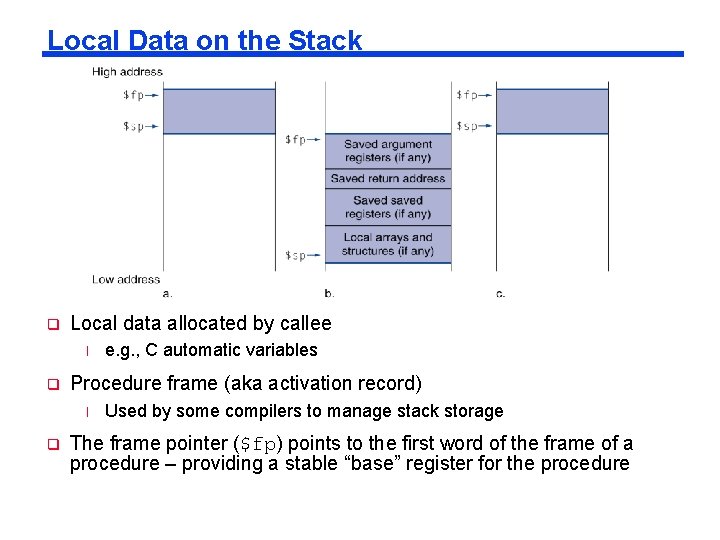
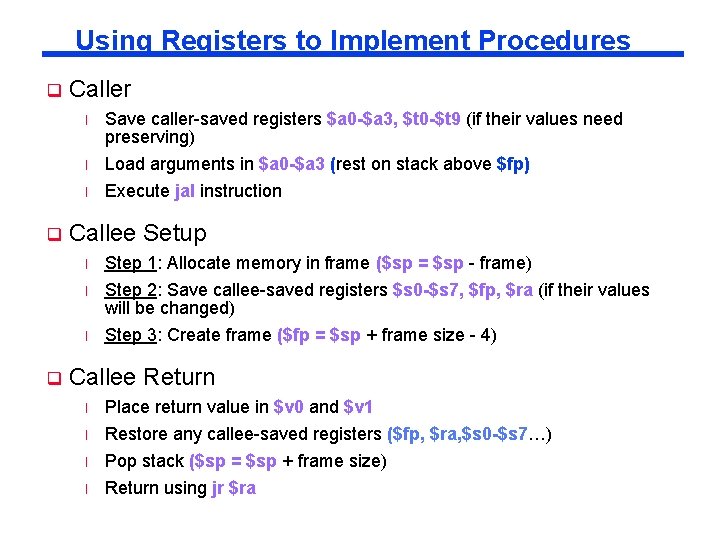
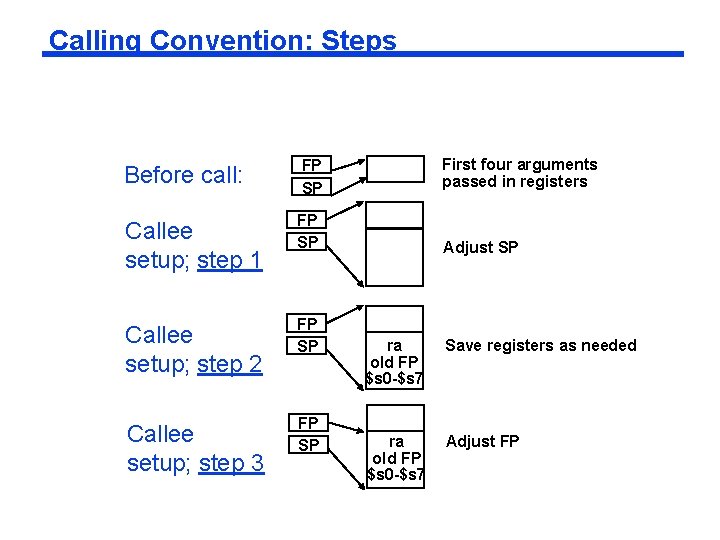
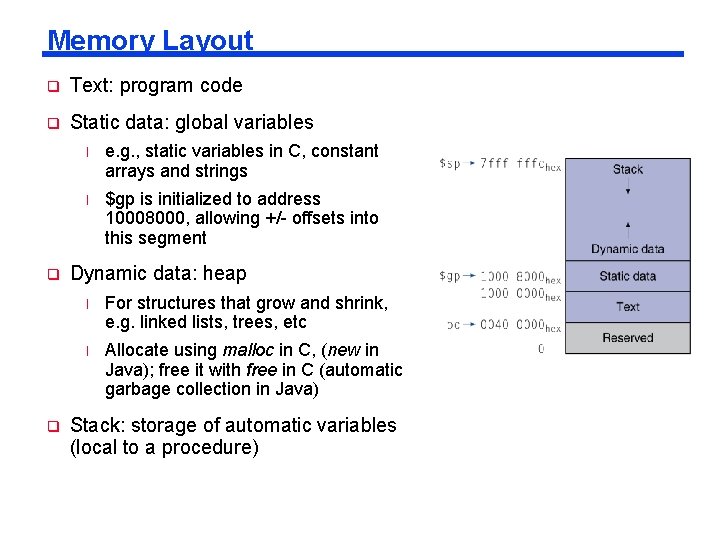
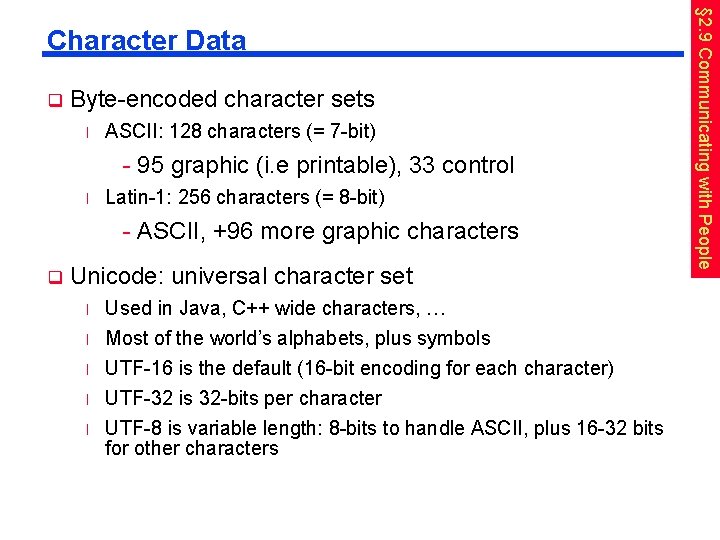
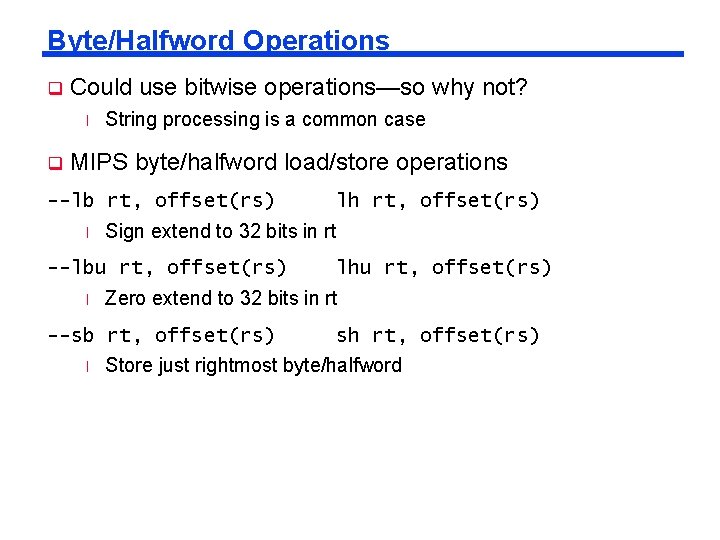
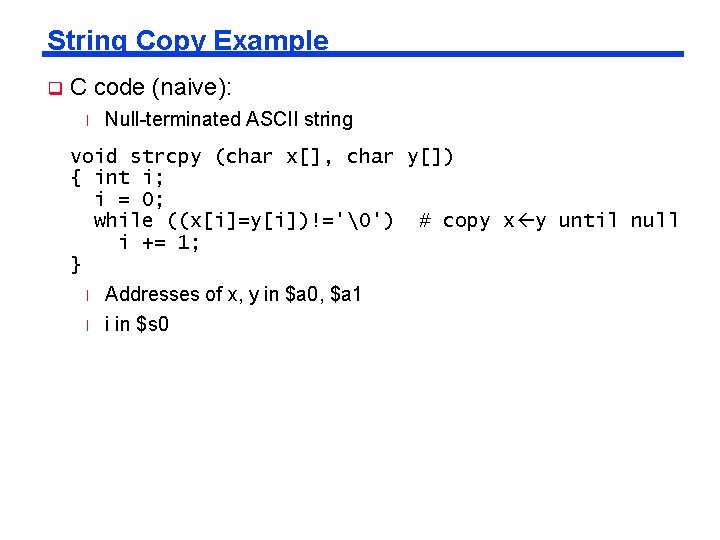
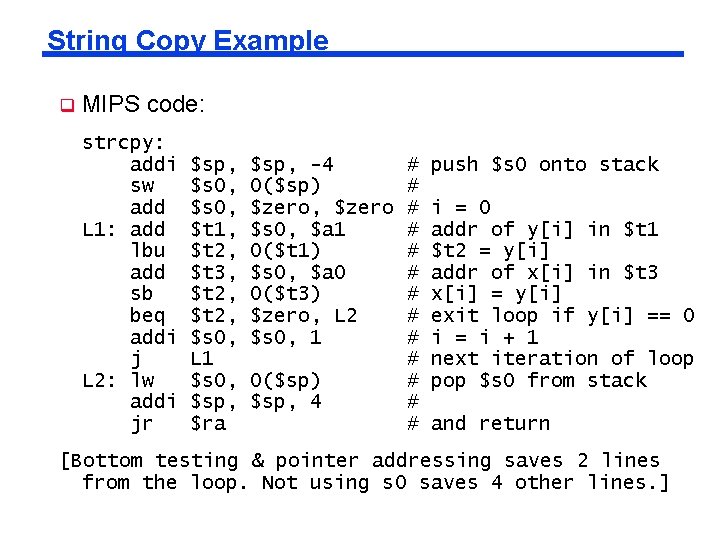
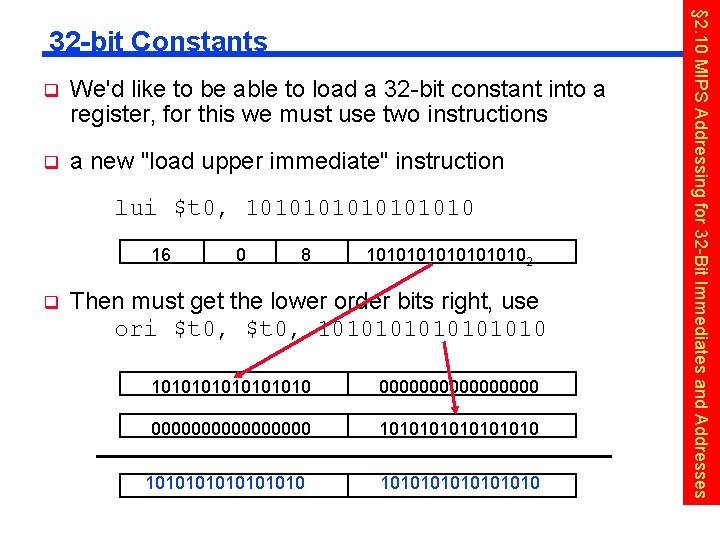
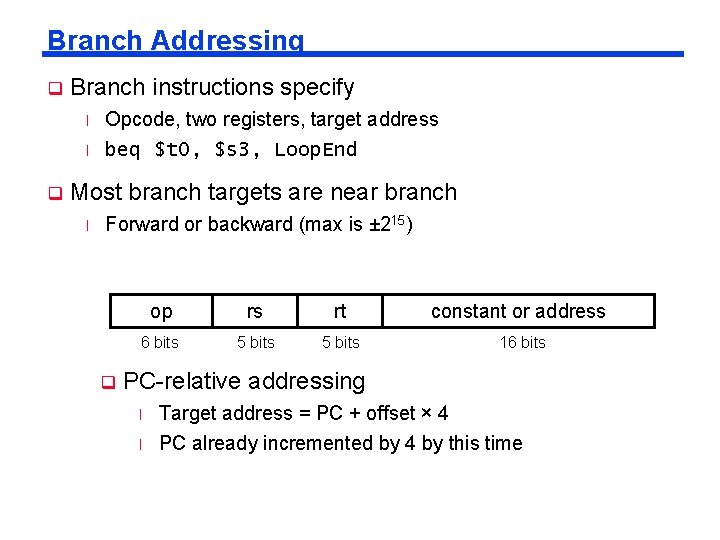
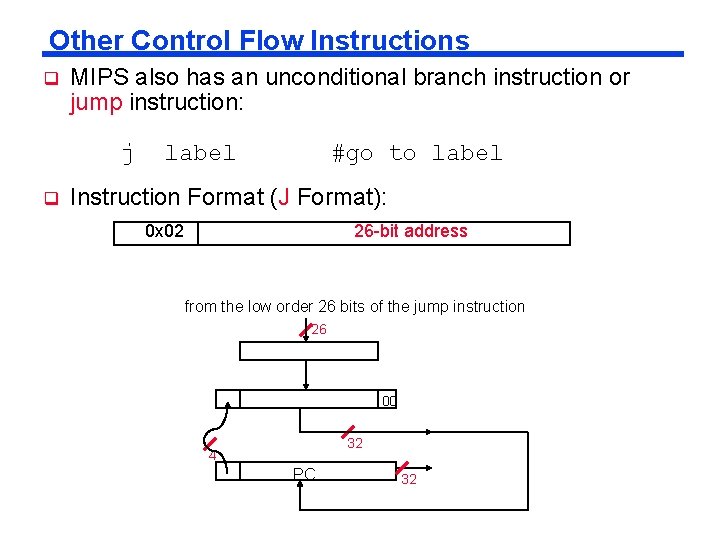
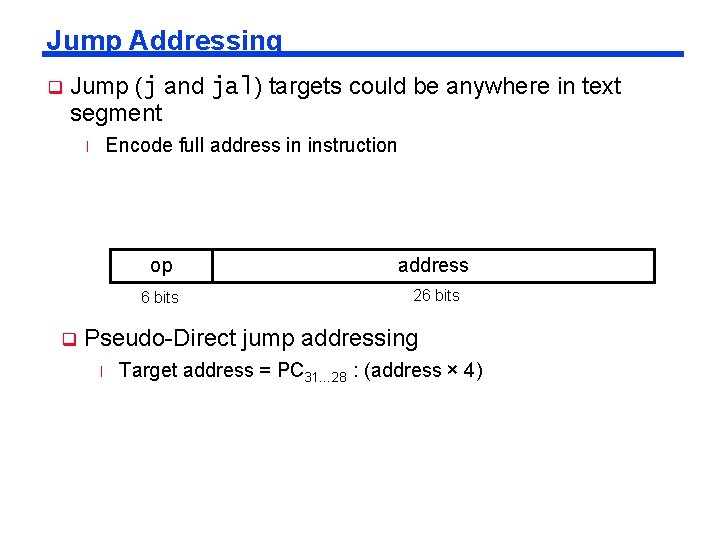
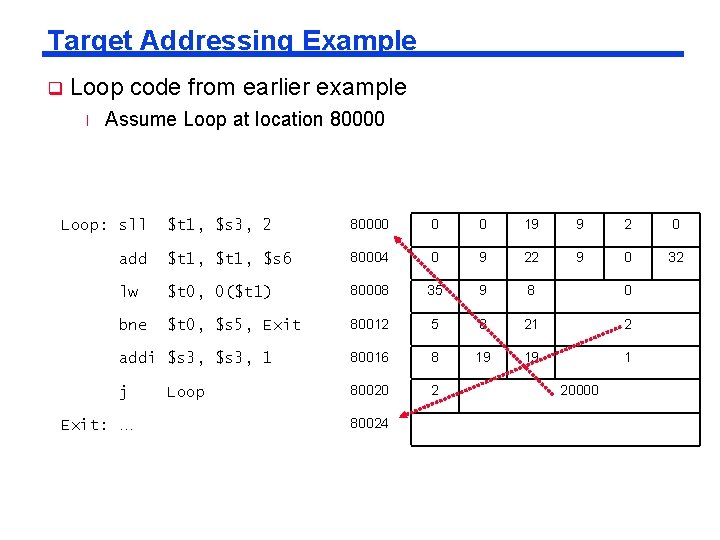
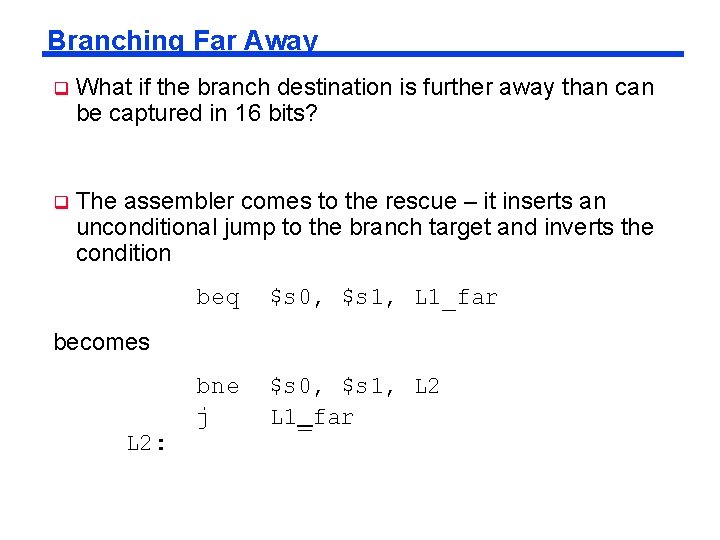
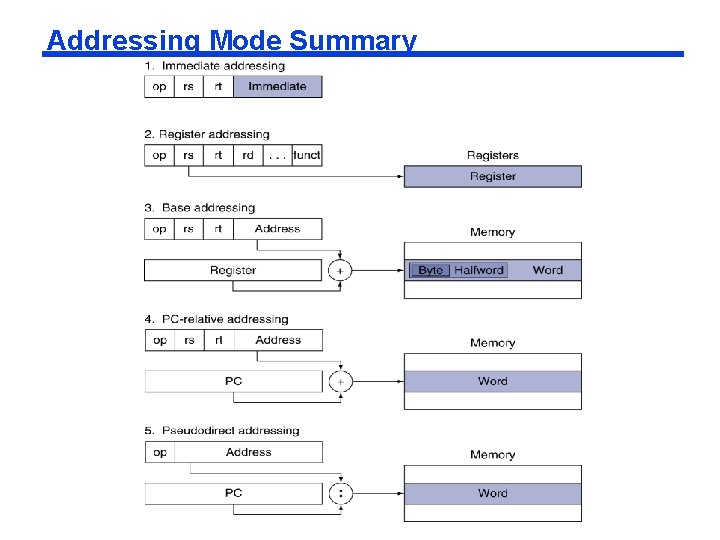
- Slides: 27
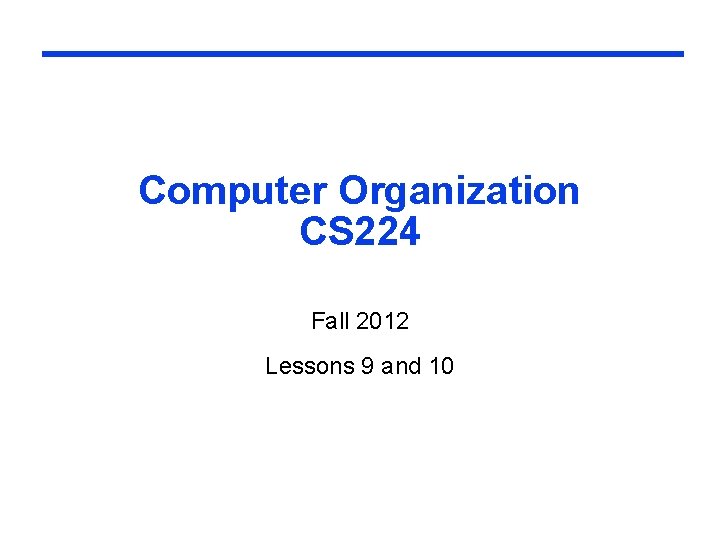
Computer Organization CS 224 Fall 2012 Lessons 9 and 10
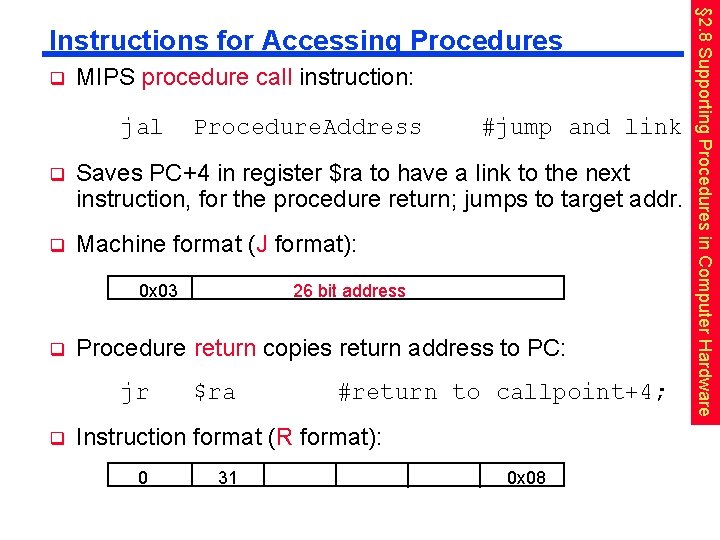
q MIPS procedure call instruction: jal Procedure. Address #jump and link q Saves PC+4 in register $ra to have a link to the next instruction, for the procedure return; jumps to target addr. q Machine format (J format): 0 x 03 q Procedure return copies return address to PC: jr q 26 bit address $ra #return to callpoint+4; Instruction format (R format): 0 31 0 x 08 § 2. 8 Supporting Procedures in Computer Hardware Instructions for Accessing Procedures
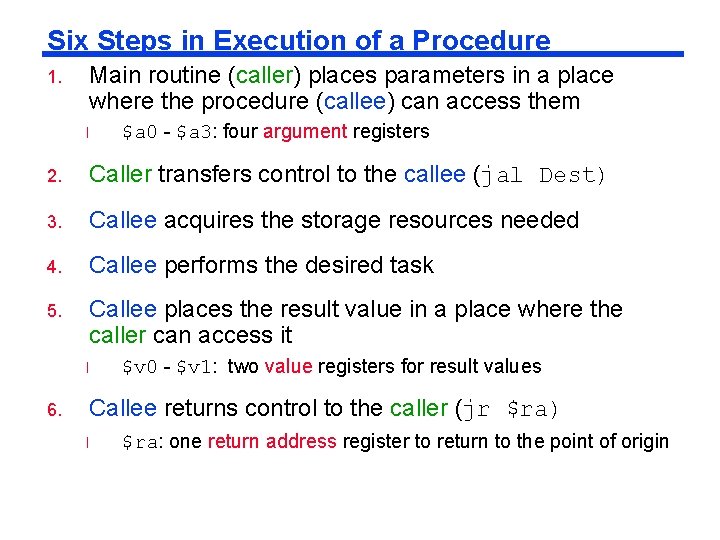
Six Steps in Execution of a Procedure 1. Main routine (caller) places parameters in a place where the procedure (callee) can access them l $a 0 - $a 3: four argument registers 2. Caller transfers control to the callee (jal Dest) 3. Callee acquires the storage resources needed 4. Callee performs the desired task 5. Callee places the result value in a place where the caller can access it l 6. $v 0 - $v 1: two value registers for result values Callee returns control to the caller (jr $ra) l $ra: one return address register to return to the point of origin
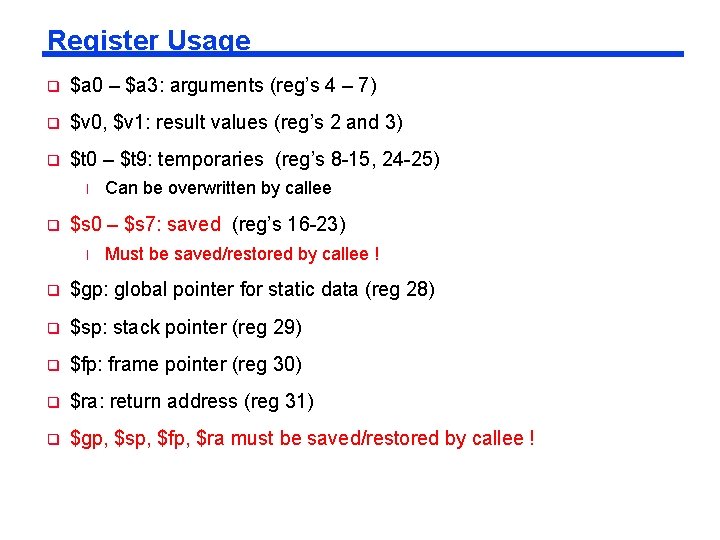
Register Usage q $a 0 – $a 3: arguments (reg’s 4 – 7) q $v 0, $v 1: result values (reg’s 2 and 3) q $t 0 – $t 9: temporaries (reg’s 8 -15, 24 -25) l q Can be overwritten by callee $s 0 – $s 7: saved (reg’s 16 -23) l Must be saved/restored by callee ! q $gp: global pointer for static data (reg 28) q $sp: stack pointer (reg 29) q $fp: frame pointer (reg 30) q $ra: return address (reg 31) q $gp, $sp, $fp, $ra must be saved/restored by callee !
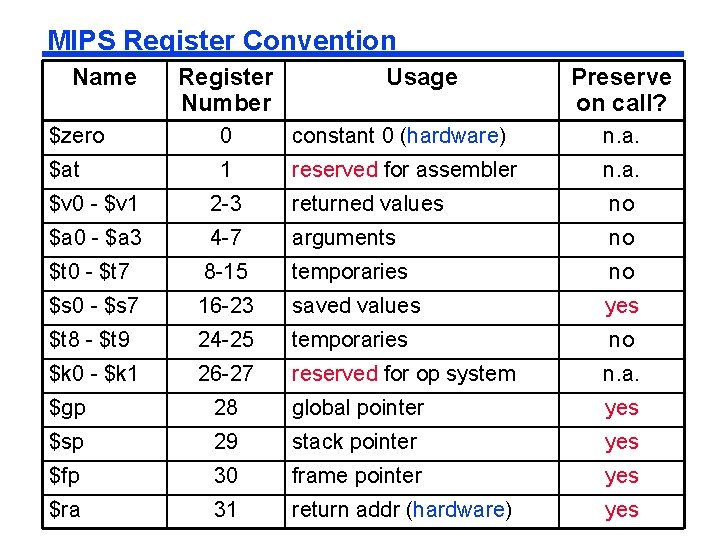
MIPS Register Convention Name Register Number Usage Preserve on call? $zero 0 constant 0 (hardware) n. a. $at 1 reserved for assembler n. a. $v 0 - $v 1 2 -3 returned values no $a 0 - $a 3 4 -7 arguments no $t 0 - $t 7 8 -15 temporaries no $s 0 - $s 7 16 -23 saved values yes $t 8 - $t 9 24 -25 temporaries no $k 0 - $k 1 26 -27 reserved for op system n. a. $gp 28 global pointer yes $sp 29 stack pointer yes $fp 30 frame pointer yes $ra 31 return addr (hardware) yes
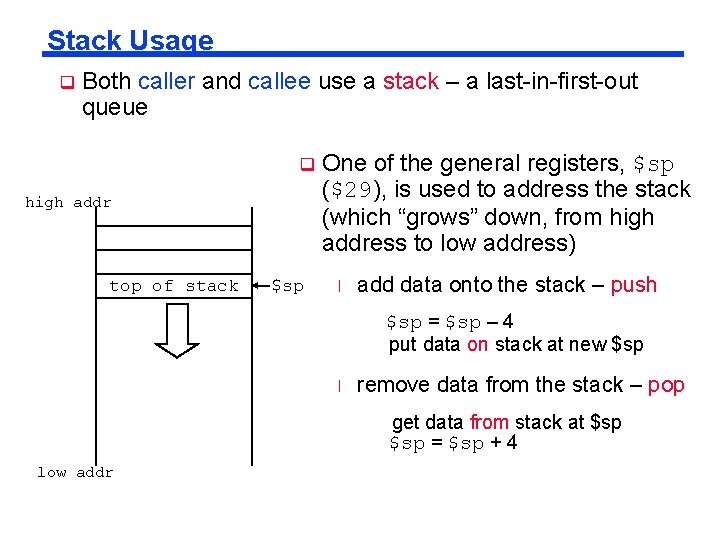
Stack Usage q Both caller and callee use a stack – a last-in-first-out queue q high addr top of stack $sp One of the general registers, $sp ($29), is used to address the stack (which “grows” down, from high address to low address) l add data onto the stack – push $sp = $sp – 4 put data on stack at new $sp l remove data from the stack – pop get data from stack at $sp = $sp + 4 low addr
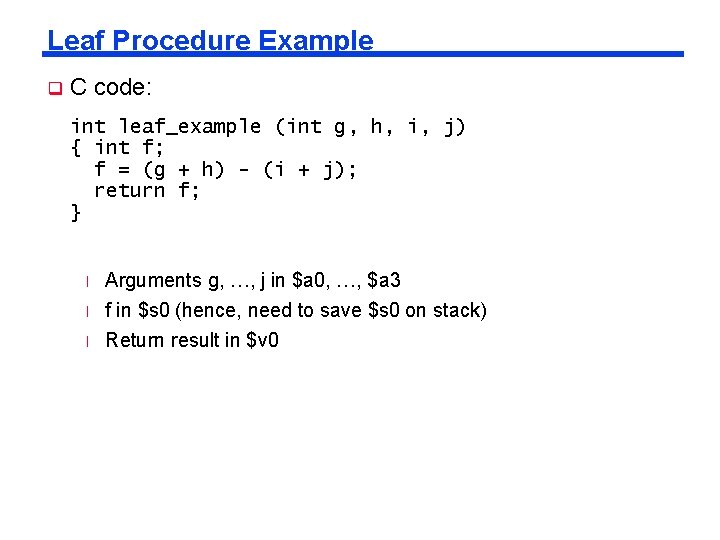
Leaf Procedure Example q C code: int leaf_example (int g, h, i, j) { int f; f = (g + h) - (i + j); return f; } l Arguments g, …, j in $a 0, …, $a 3 l f in $s 0 (hence, need to save $s 0 on stack) Return result in $v 0 l
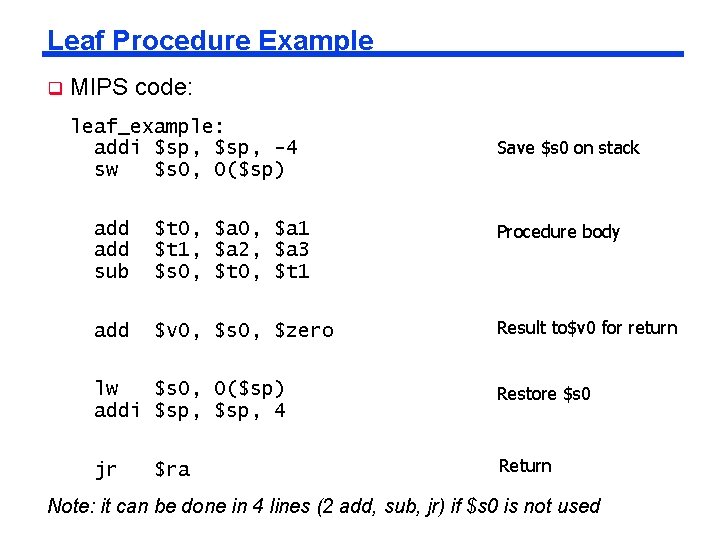
Leaf Procedure Example q MIPS code: leaf_example: addi $sp, -4 sw $s 0, 0($sp) Save $s 0 on stack add sub $t 0, $a 1 $t 1, $a 2, $a 3 $s 0, $t 1 Procedure body add $v 0, $s 0, $zero Result to$v 0 for return lw $s 0, 0($sp) addi $sp, 4 Restore $s 0 jr Return $ra Note: it can be done in 4 lines (2 add, sub, jr) if $s 0 is not used
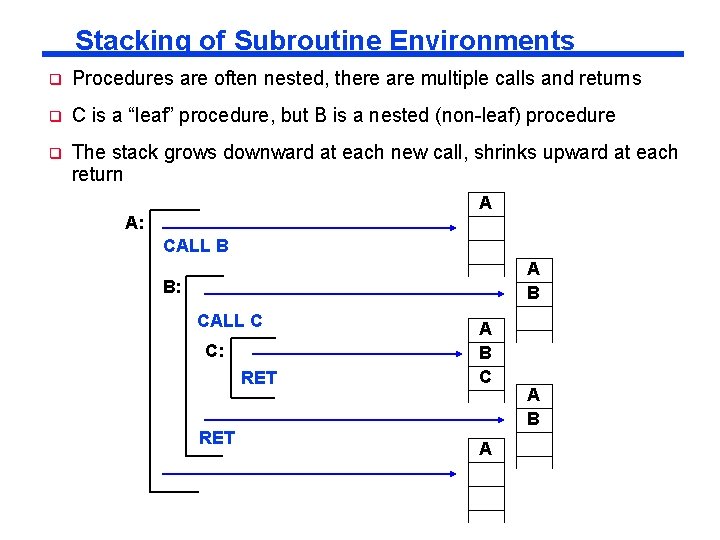
Stacking of Subroutine Environments q Procedures are often nested, there are multiple calls and returns q C is a “leaf” procedure, but B is a nested (non-leaf) procedure q The stack grows downward at each new call, shrinks upward at each return A A: CALL B A B B: CALL C C: RET A B C A A B
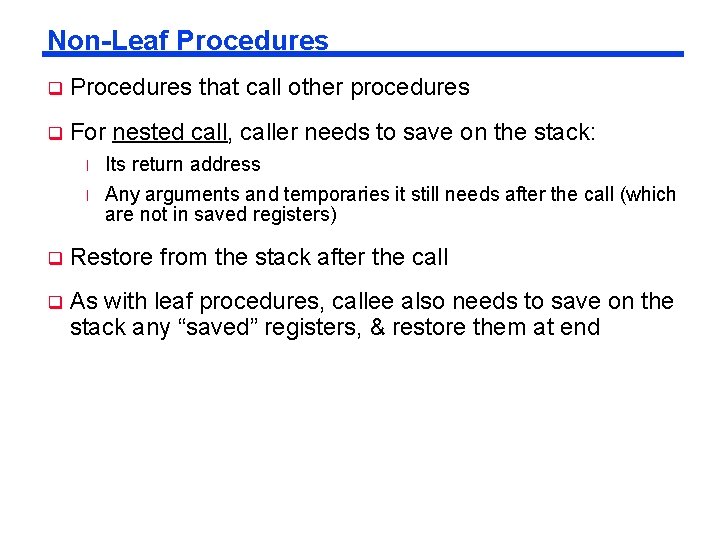
Non-Leaf Procedures q Procedures that call other procedures q For nested call, caller needs to save on the stack: l l Its return address Any arguments and temporaries it still needs after the call (which are not in saved registers) q Restore from the stack after the call q As with leaf procedures, callee also needs to save on the stack any “saved” registers, & restore them at end
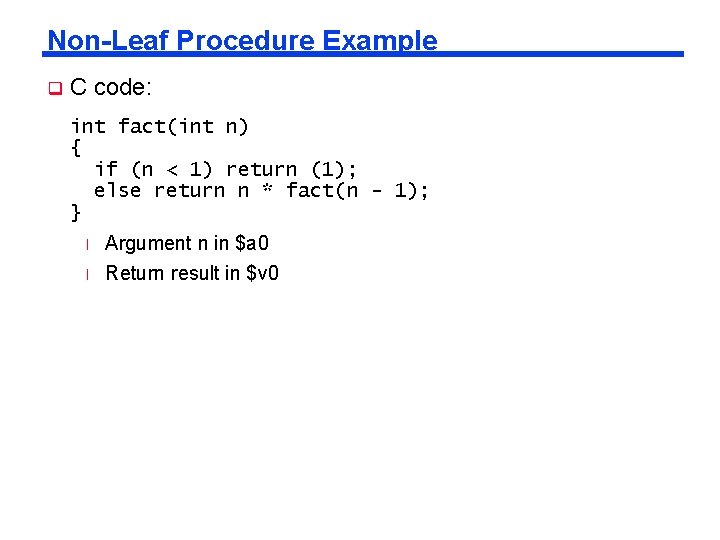
Non-Leaf Procedure Example q C code: int fact(int n) { if (n < 1) return (1); else return n * fact(n - 1); } l Argument n in $a 0 l Return result in $v 0
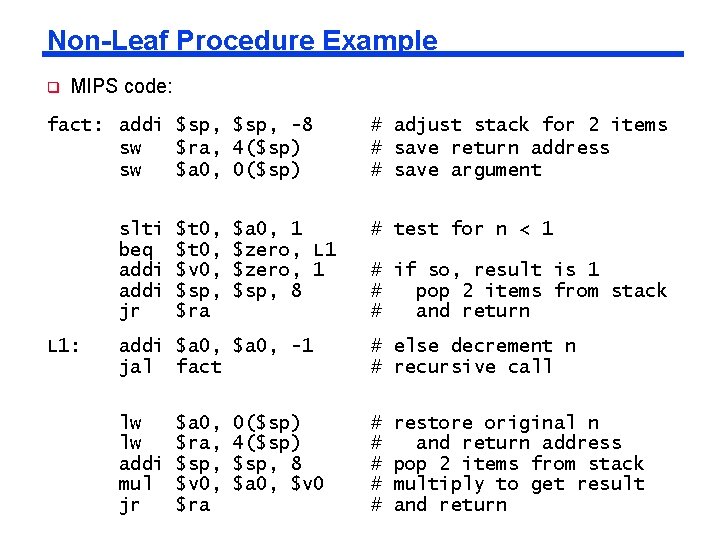
Non-Leaf Procedure Example q MIPS code: fact: addi $sp, -8 sw $ra, 4($sp) sw $a 0, 0($sp) slti beq addi jr L 1: $t 0, $v 0, $sp, $ra $a 0, 1 $zero, L 1 $zero, 1 $sp, 8 # adjust stack for 2 items # save return address # save argument # test for n < 1 # if so, result is 1 # pop 2 items from stack # and return addi $a 0, -1 jal fact # else decrement n # recursive call lw lw addi mul jr # # # $a 0, $ra, $sp, $v 0, $ra 0($sp) 4($sp) $sp, 8 $a 0, $v 0 restore original n and return address pop 2 items from stack multiply to get result and return
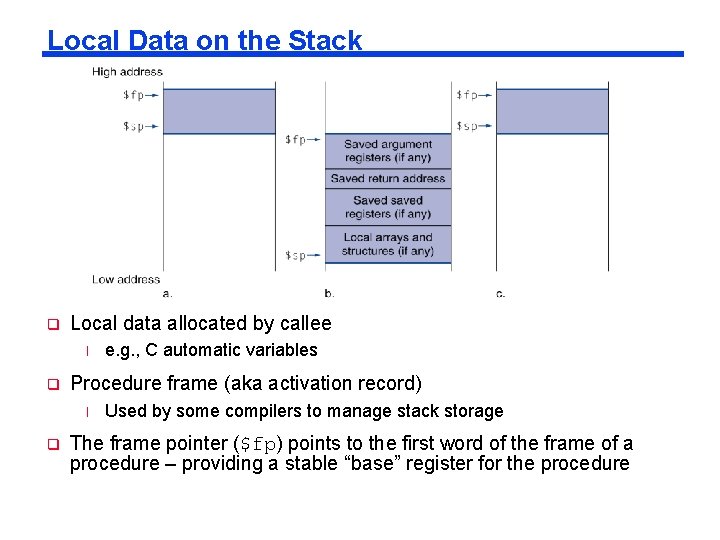
Local Data on the Stack q Local data allocated by callee l q Procedure frame (aka activation record) l q e. g. , C automatic variables Used by some compilers to manage stack storage The frame pointer ($fp) points to the first word of the frame of a procedure – providing a stable “base” register for the procedure
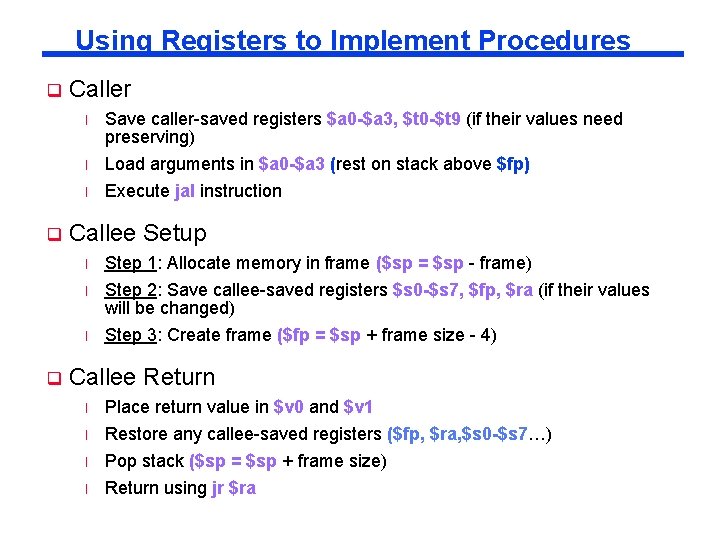
Using Registers to Implement Procedures q Caller l l l q Callee Setup l Step 1: Allocate memory in frame ($sp = $sp - frame) Step 2: Save callee-saved registers $s 0 -$s 7, $fp, $ra (if their values will be changed) l Step 3: Create frame ($fp = $sp + frame size - 4) l q Save caller-saved registers $a 0 -$a 3, $t 0 -$t 9 (if their values need preserving) Load arguments in $a 0 -$a 3 (rest on stack above $fp) Execute jal instruction Callee Return l Place return value in $v 0 and $v 1 l Restore any callee-saved registers ($fp, $ra, $s 0 -$s 7…) l Pop stack ($sp = $sp + frame size) l Return using jr $ra
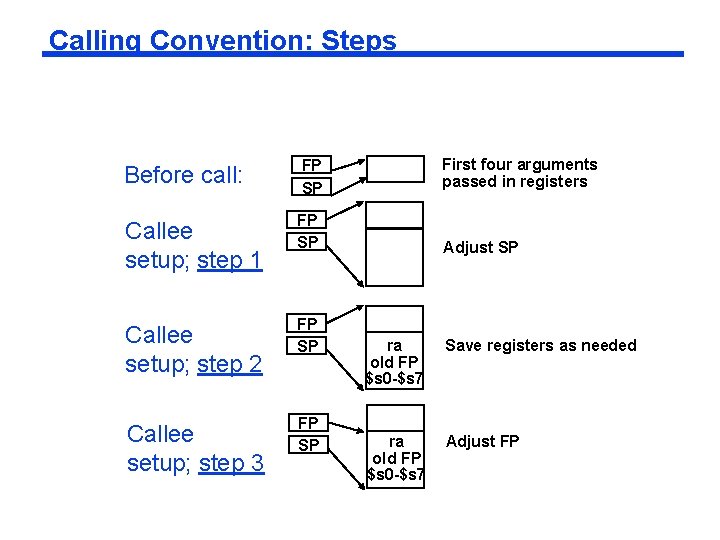
Calling Convention: Steps Before call: FP SP First four arguments passed in registers Callee setup; step 1 FP SP Adjust SP Callee setup; step 2 FP SP Callee setup; step 3 FP SP ra old FP $s 0 -$s 7 Save registers as needed ra old FP $s 0 -$s 7 Adjust FP
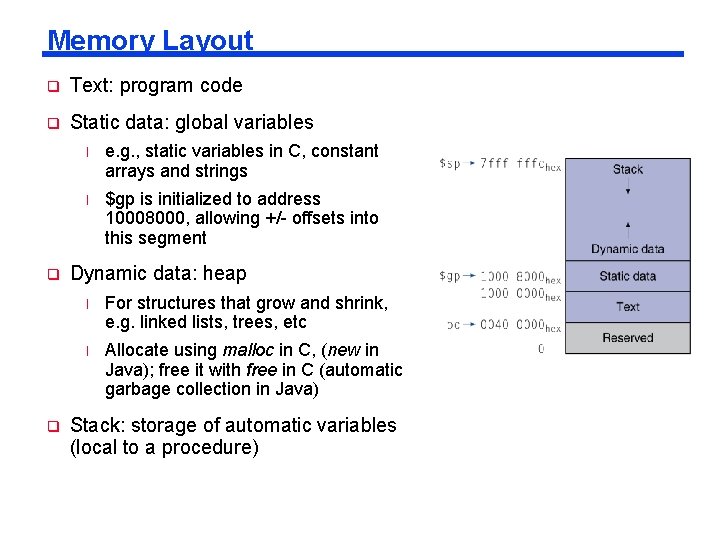
Memory Layout q Text: program code q Static data: global variables q q l e. g. , static variables in C, constant arrays and strings l $gp is initialized to address 10008000, allowing +/- offsets into this segment Dynamic data: heap l For structures that grow and shrink, e. g. linked lists, trees, etc l Allocate using malloc in C, (new in Java); free it with free in C (automatic garbage collection in Java) Stack: storage of automatic variables (local to a procedure)
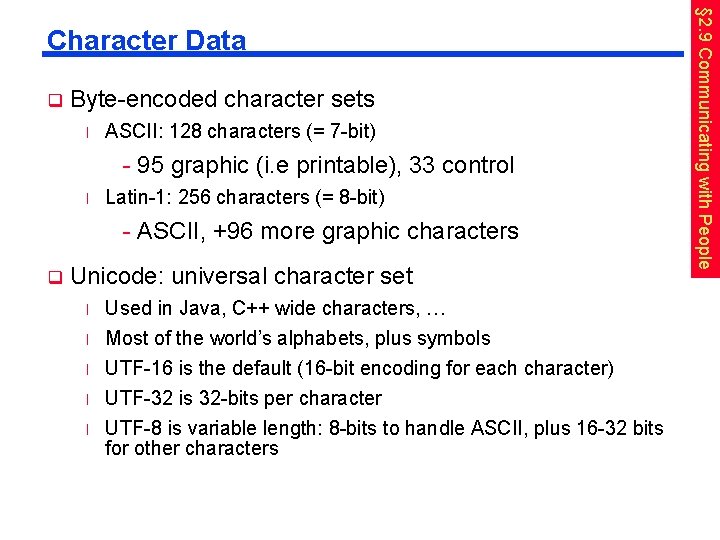
q Byte-encoded character sets l ASCII: 128 characters (= 7 -bit) - 95 graphic (i. e printable), 33 control l Latin-1: 256 characters (= 8 -bit) - ASCII, +96 more graphic characters q Unicode: universal character set l Used in Java, C++ wide characters, … l Most of the world’s alphabets, plus symbols UTF-16 is the default (16 -bit encoding for each character) UTF-32 is 32 -bits per character UTF-8 is variable length: 8 -bits to handle ASCII, plus 16 -32 bits for other characters l l l § 2. 9 Communicating with People Character Data
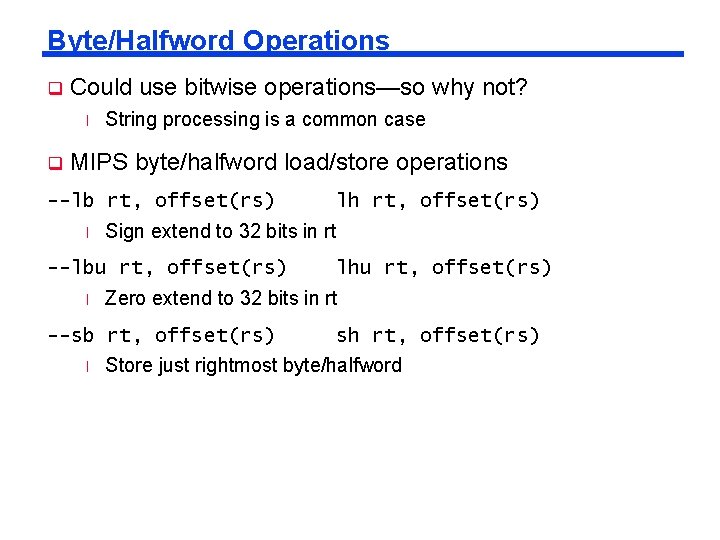
Byte/Halfword Operations q Could use bitwise operations—so why not? l q String processing is a common case MIPS byte/halfword load/store operations --lb rt, offset(rs) l Sign extend to 32 bits in rt --lbu rt, offset(rs) l lhu rt, offset(rs) Zero extend to 32 bits in rt --sb rt, offset(rs) l lh rt, offset(rs) sh rt, offset(rs) Store just rightmost byte/halfword
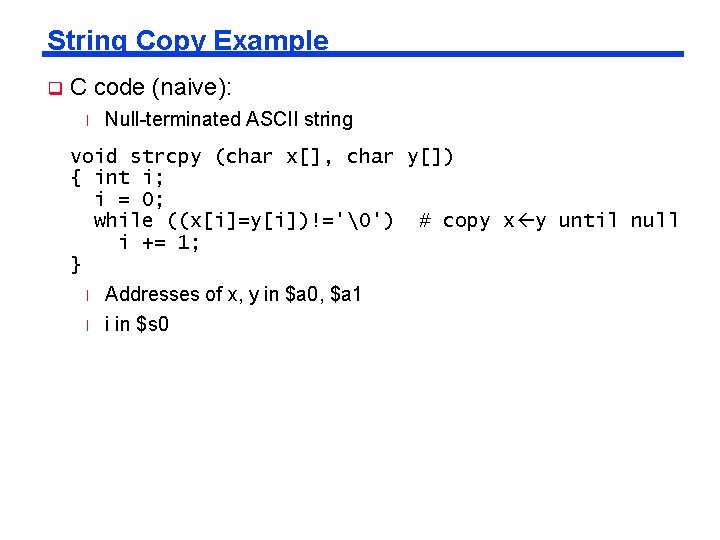
String Copy Example q C code (naive): l Null-terminated ASCII string void strcpy (char x[], char y[]) { int i; i = 0; while ((x[i]=y[i])!='