Computer Organization CS 224 Fall 2012 Lessons 5
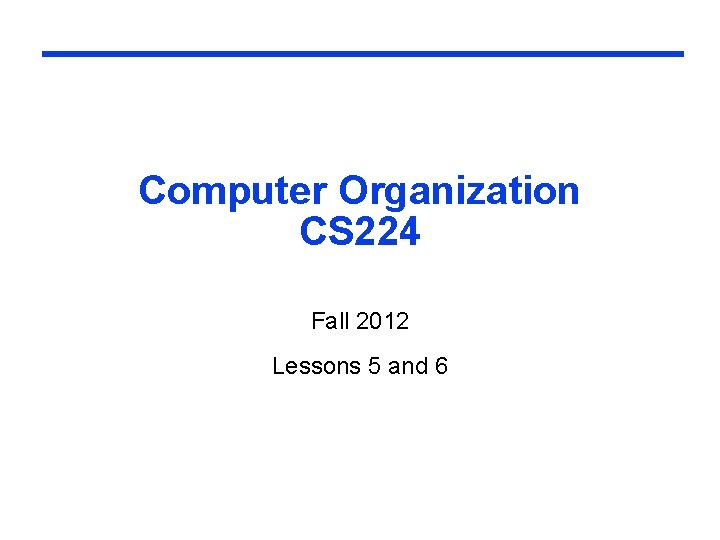
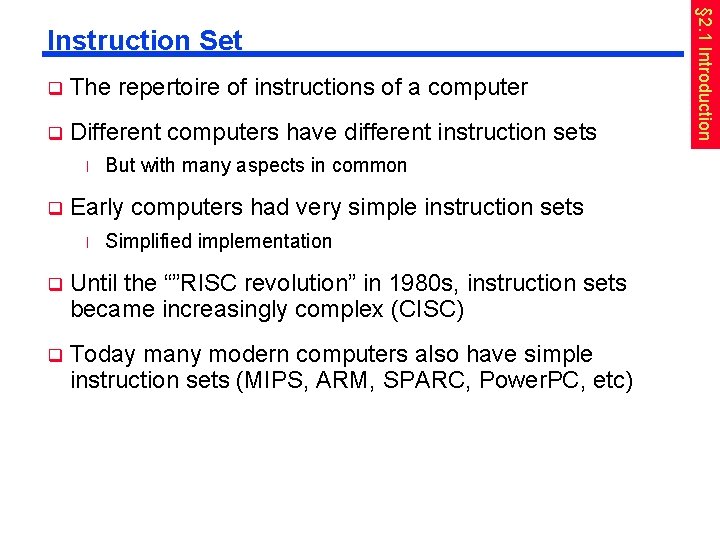
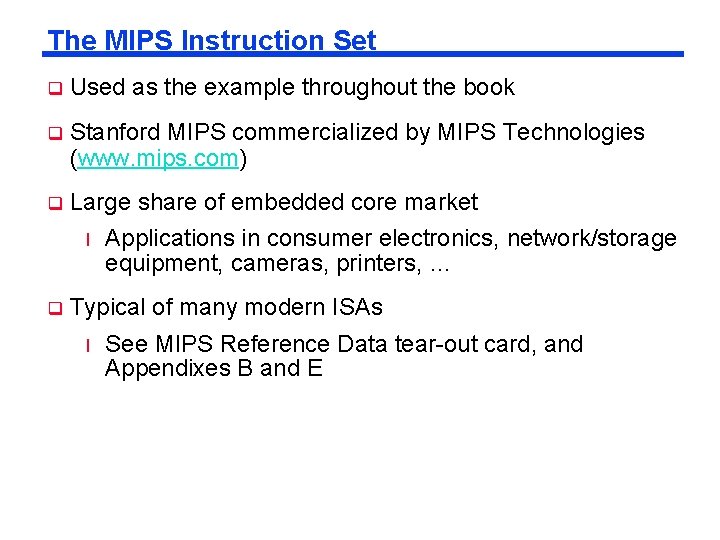
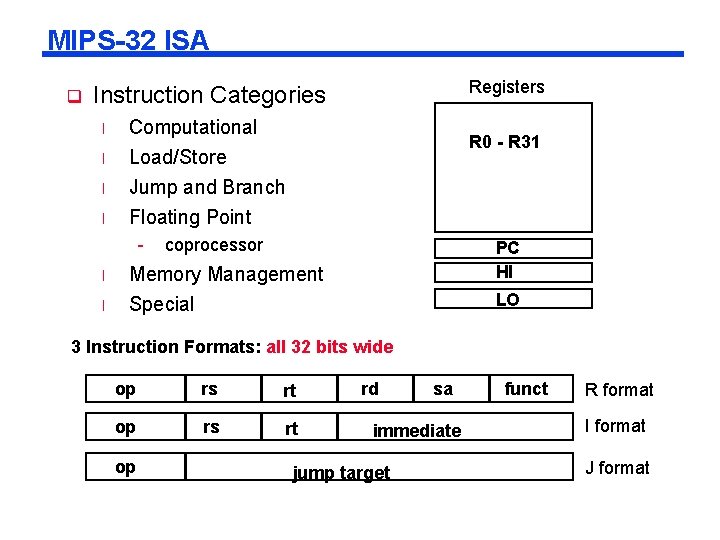
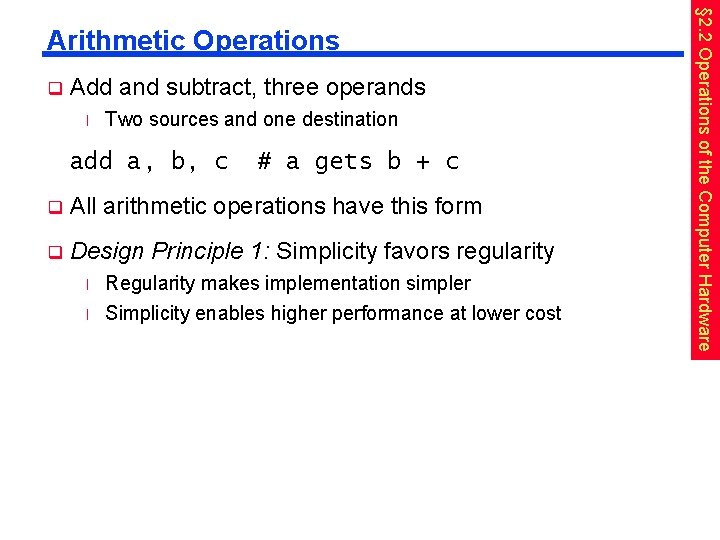
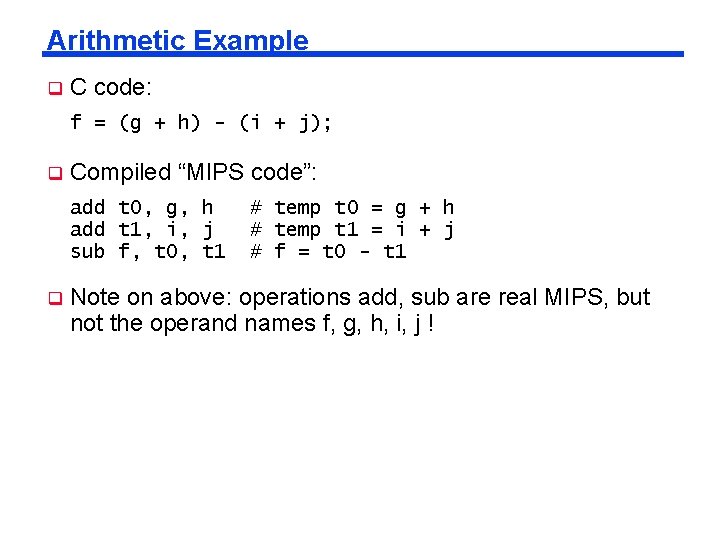
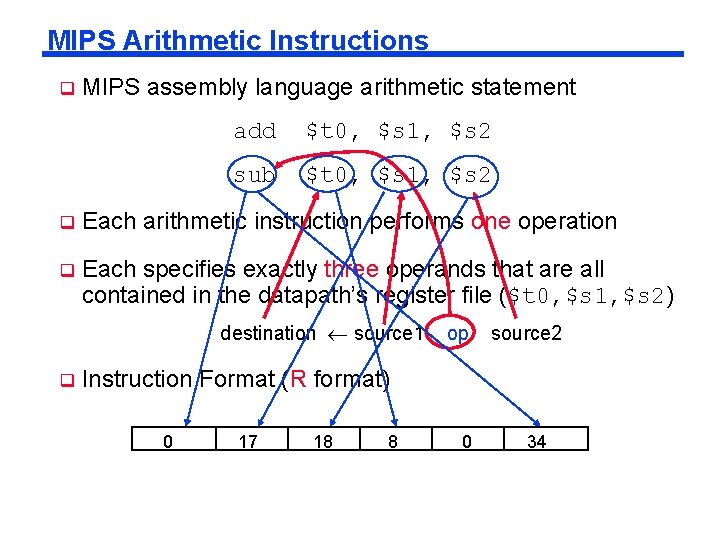
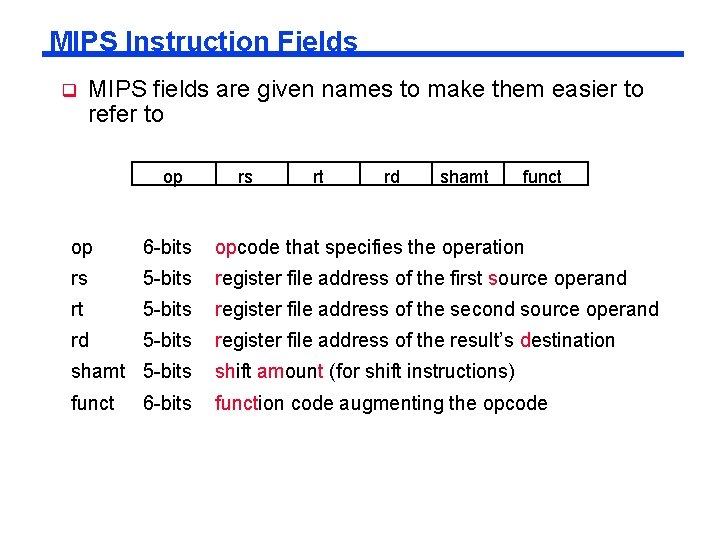
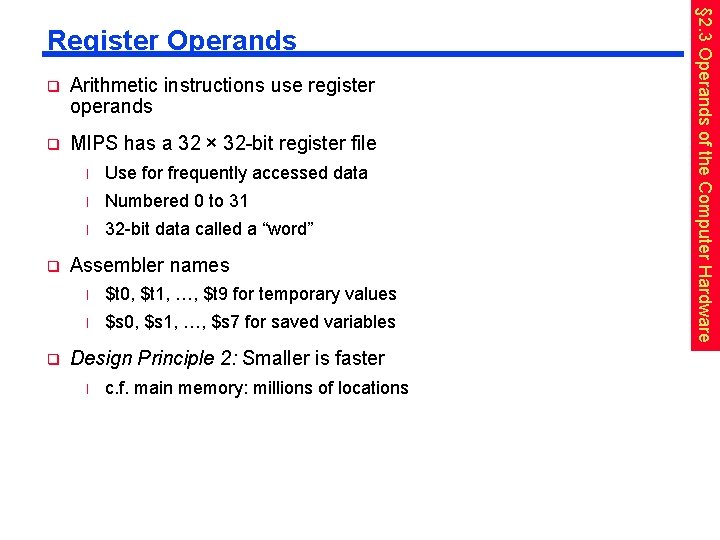
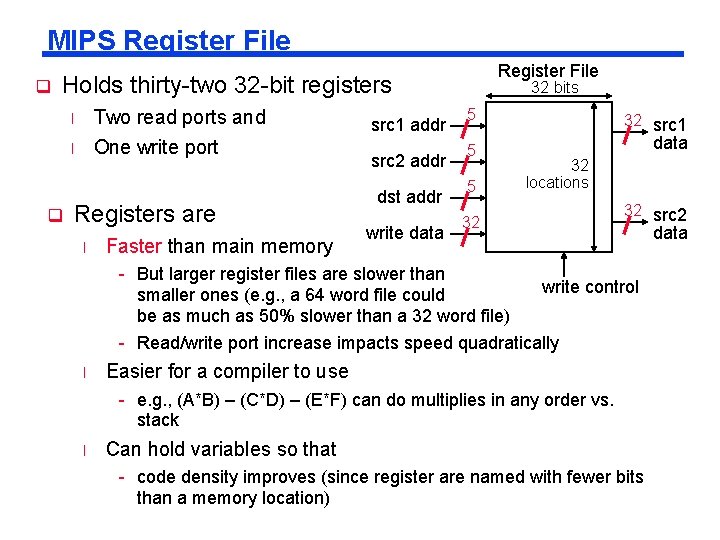
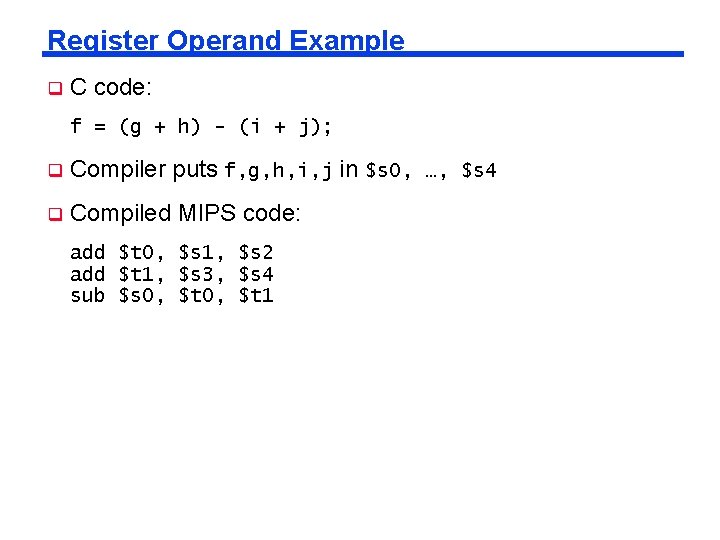
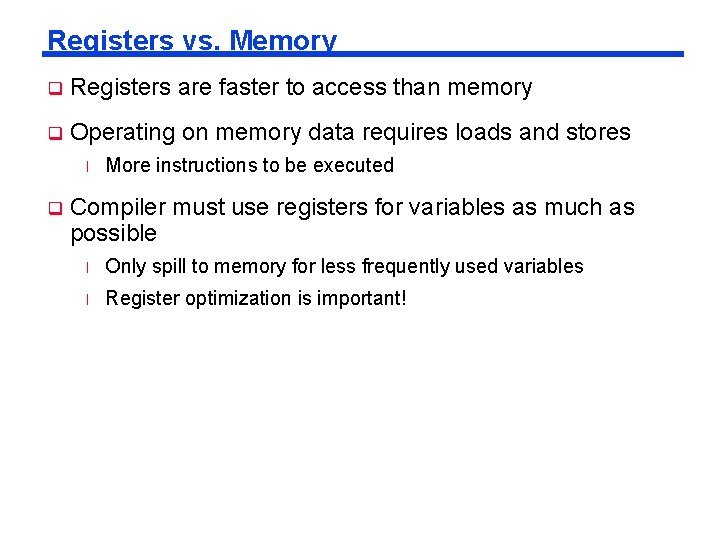
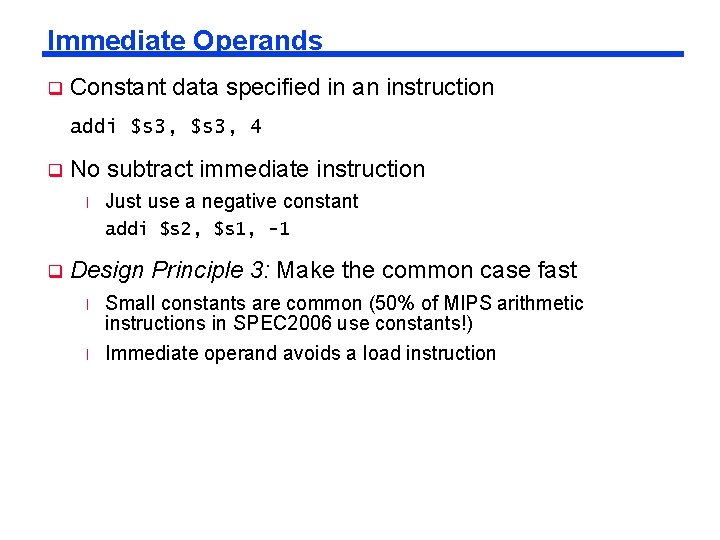
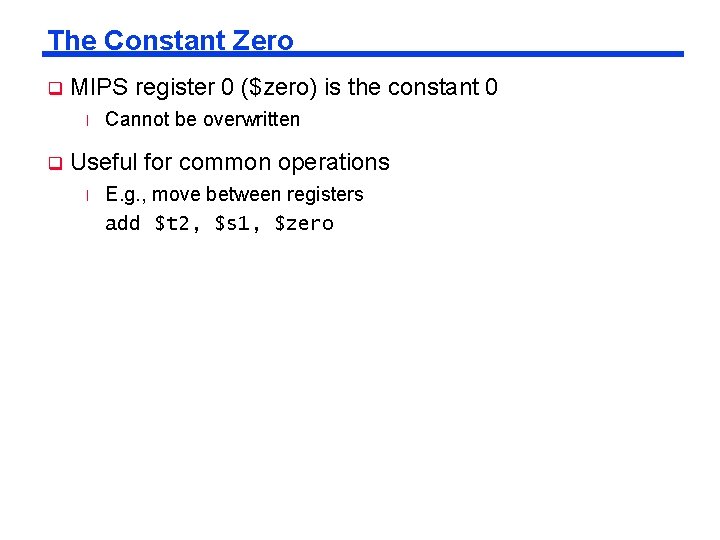
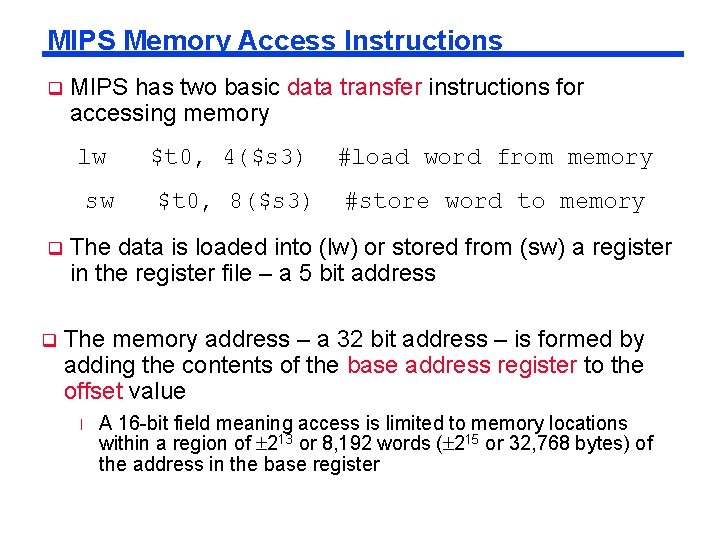
![Memory Operand Example 1 q C code: g = h + A[8]; l q Memory Operand Example 1 q C code: g = h + A[8]; l q](https://slidetodoc.com/presentation_image_h2/0c7a7a2b048aa81803cbc8e22a62966e/image-16.jpg)
![Memory Operand Example 2 q C code: A[12] = h + A[8]; l q Memory Operand Example 2 q C code: A[12] = h + A[8]; l q](https://slidetodoc.com/presentation_image_h2/0c7a7a2b048aa81803cbc8e22a62966e/image-17.jpg)
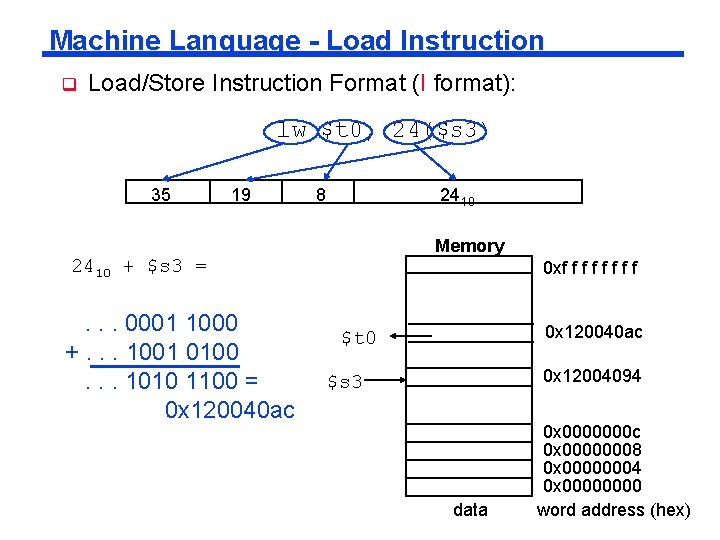
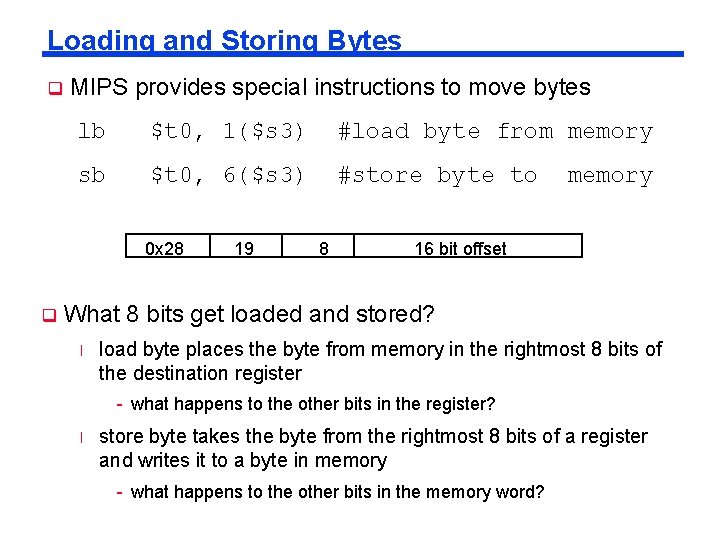
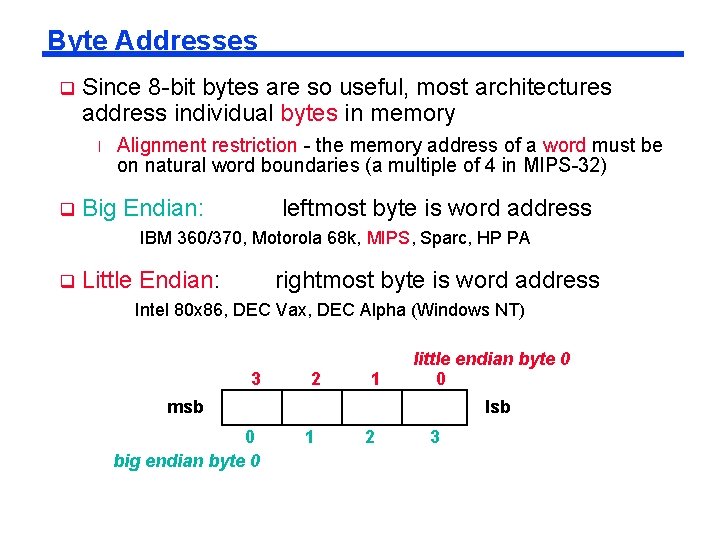
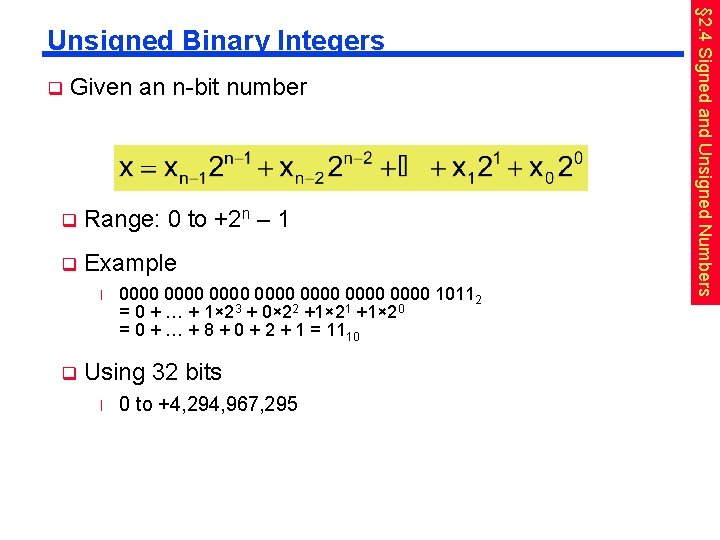
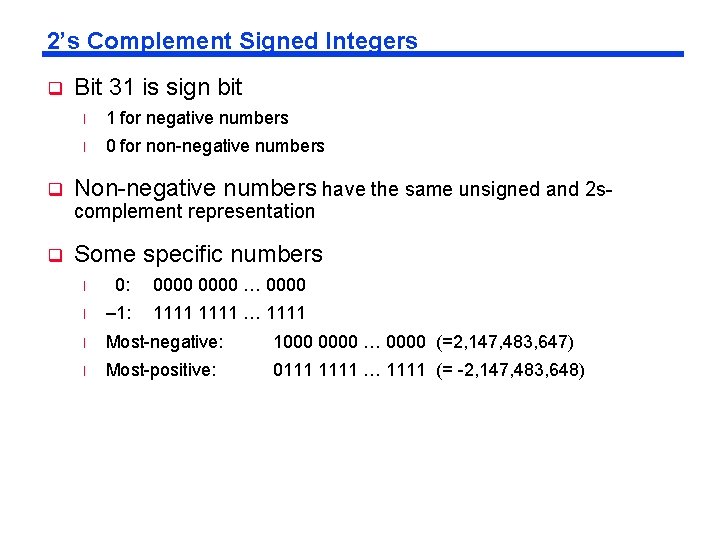
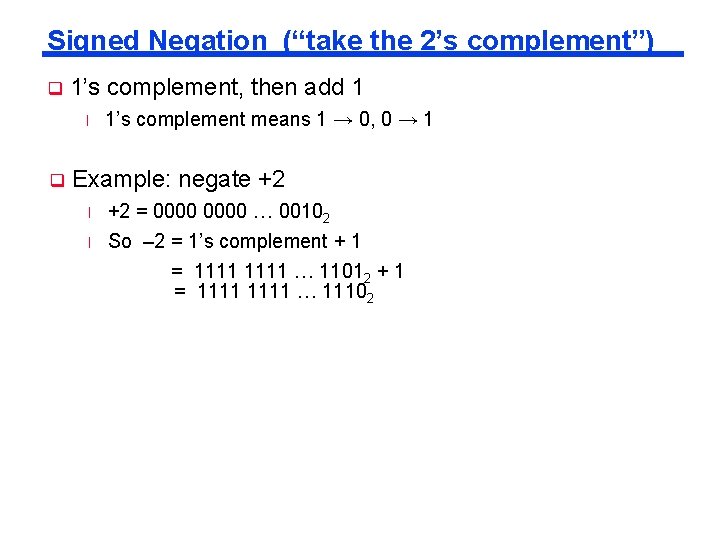
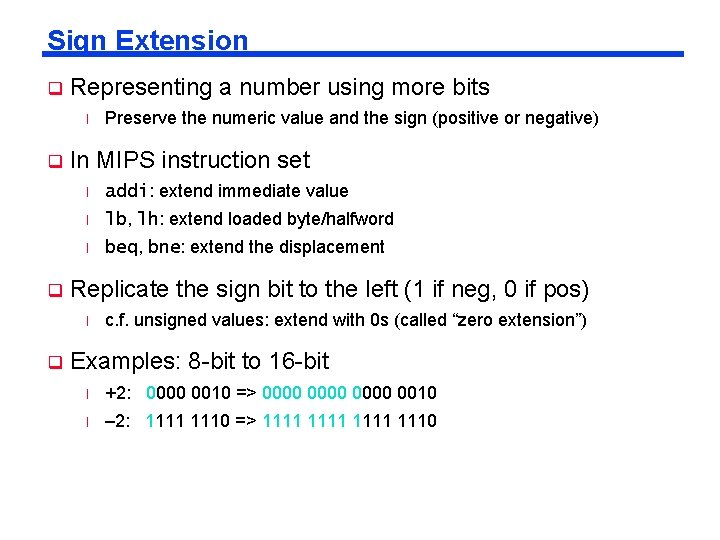
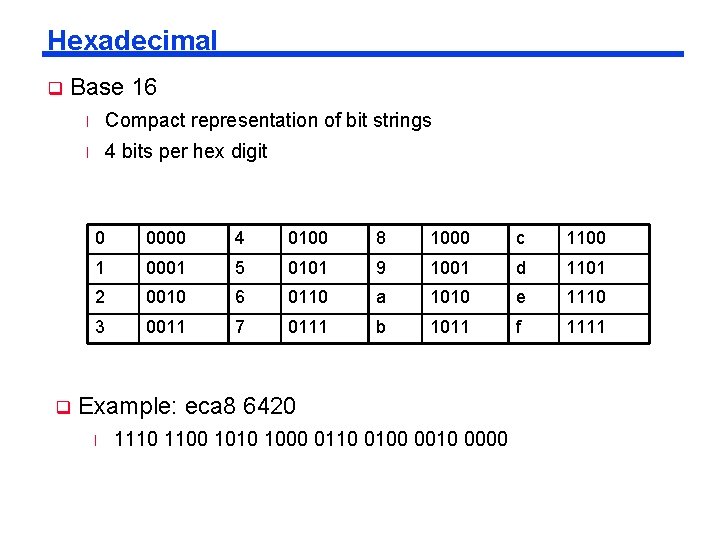
- Slides: 25
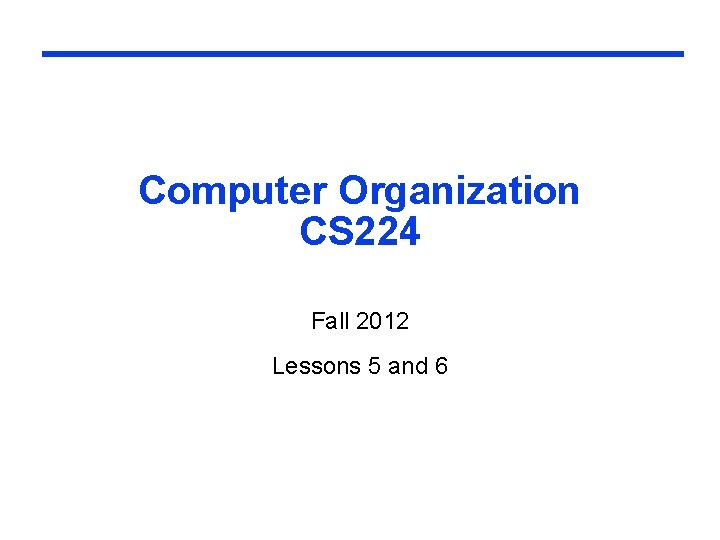
Computer Organization CS 224 Fall 2012 Lessons 5 and 6
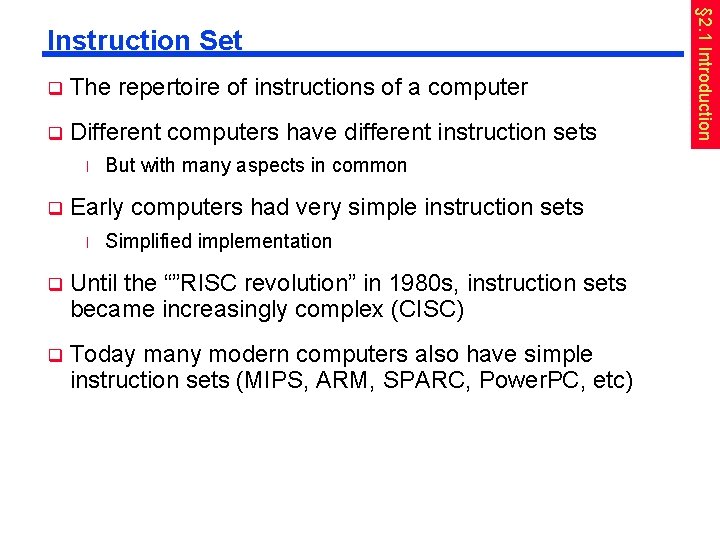
q The repertoire of instructions of a computer q Different computers have different instruction sets l q But with many aspects in common Early computers had very simple instruction sets l Simplified implementation q Until the “”RISC revolution” in 1980 s, instruction sets became increasingly complex (CISC) q Today many modern computers also have simple instruction sets (MIPS, ARM, SPARC, Power. PC, etc) § 2. 1 Introduction Instruction Set
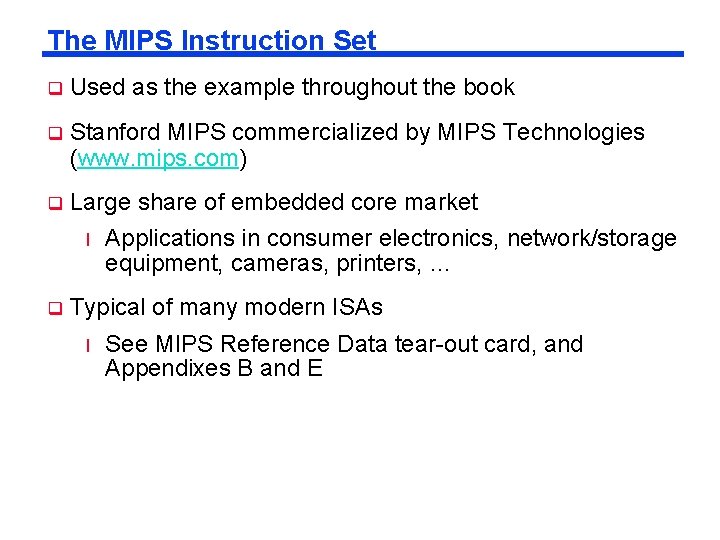
The MIPS Instruction Set q Used as the example throughout the book q Stanford MIPS commercialized by MIPS Technologies (www. mips. com) q Large share of embedded core market l q Applications in consumer electronics, network/storage equipment, cameras, printers, … Typical of many modern ISAs l See MIPS Reference Data tear-out card, and Appendixes B and E
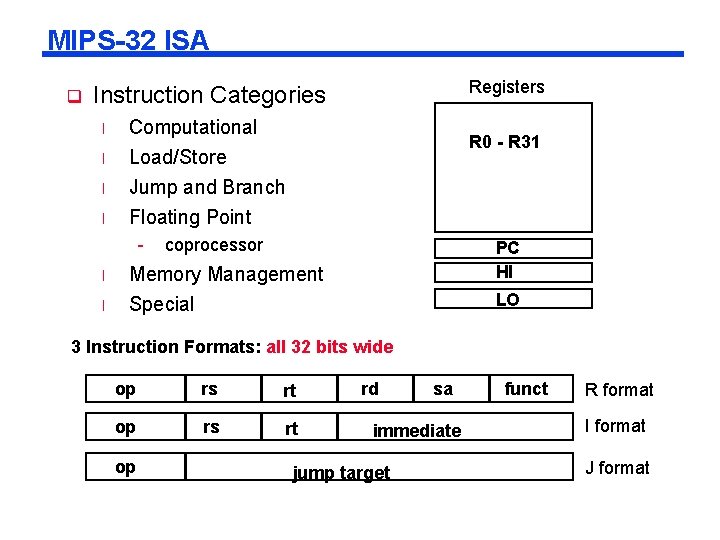
MIPS-32 ISA q Registers Instruction Categories l Computational Load/Store Jump and Branch l Floating Point l l - R 0 - R 31 coprocessor l Memory Management PC HI l Special LO 3 Instruction Formats: all 32 bits wide op rs rt op rd sa immediate jump target funct R format I format J format
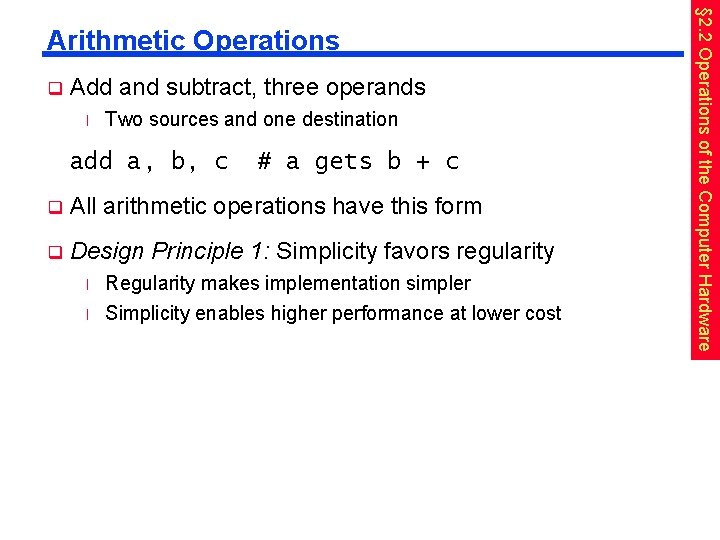
q Add and subtract, three operands l Two sources and one destination add a, b, c # a gets b + c q All arithmetic operations have this form q Design Principle 1: Simplicity favors regularity l Regularity makes implementation simpler l Simplicity enables higher performance at lower cost § 2. 2 Operations of the Computer Hardware Arithmetic Operations
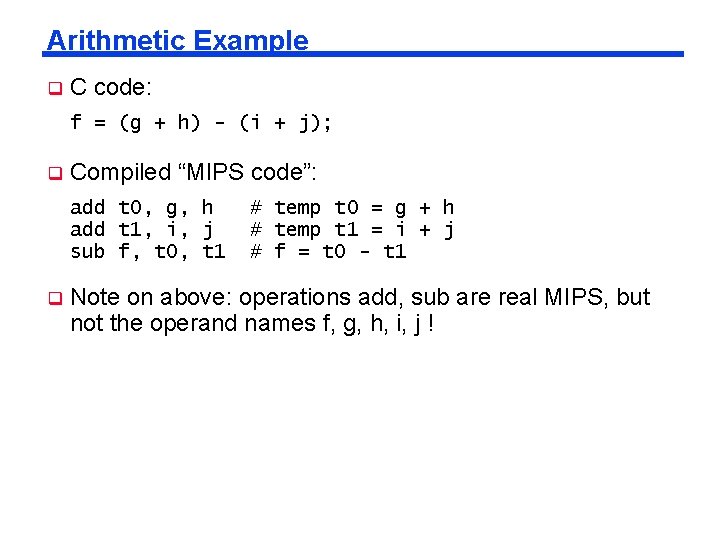
Arithmetic Example q C code: f = (g + h) - (i + j); q Compiled “MIPS code”: add t 0, g, h add t 1, i, j sub f, t 0, t 1 q # temp t 0 = g + h # temp t 1 = i + j # f = t 0 - t 1 Note on above: operations add, sub are real MIPS, but not the operand names f, g, h, i, j !
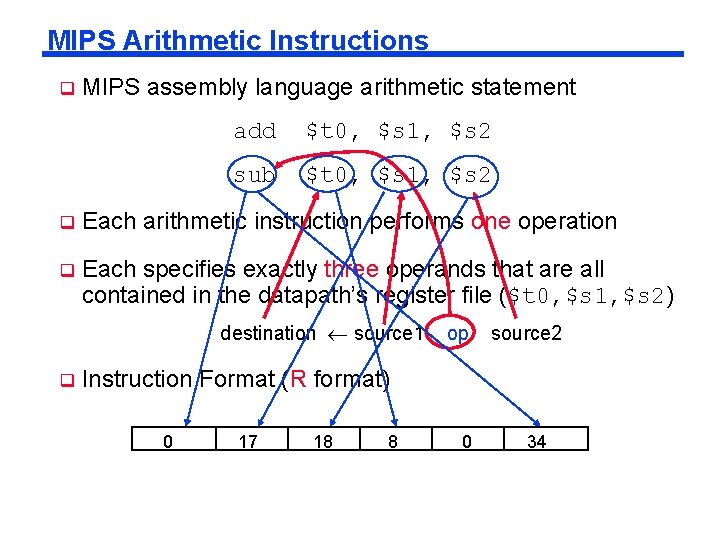
MIPS Arithmetic Instructions q MIPS assembly language arithmetic statement add $t 0, $s 1, $s 2 sub $t 0, $s 1, $s 2 q Each arithmetic instruction performs one operation q Each specifies exactly three operands that are all contained in the datapath’s register file ($t 0, $s 1, $s 2) destination source 1 q op source 2 Instruction Format (R format) 0 17 18 8 0 34
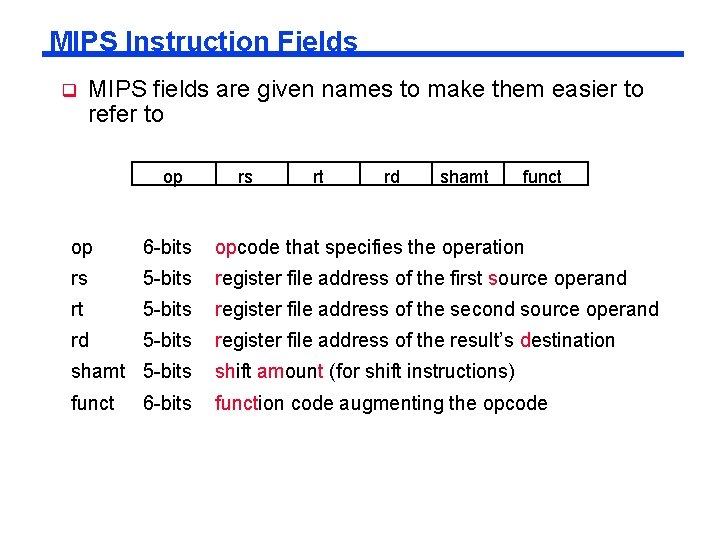
MIPS Instruction Fields q MIPS fields are given names to make them easier to refer to op rs rt rd shamt funct op 6 -bits opcode that specifies the operation rs 5 -bits register file address of the first source operand rt 5 -bits register file address of the second source operand rd 5 -bits register file address of the result’s destination shamt 5 -bits shift amount (for shift instructions) function code augmenting the opcode 6 -bits
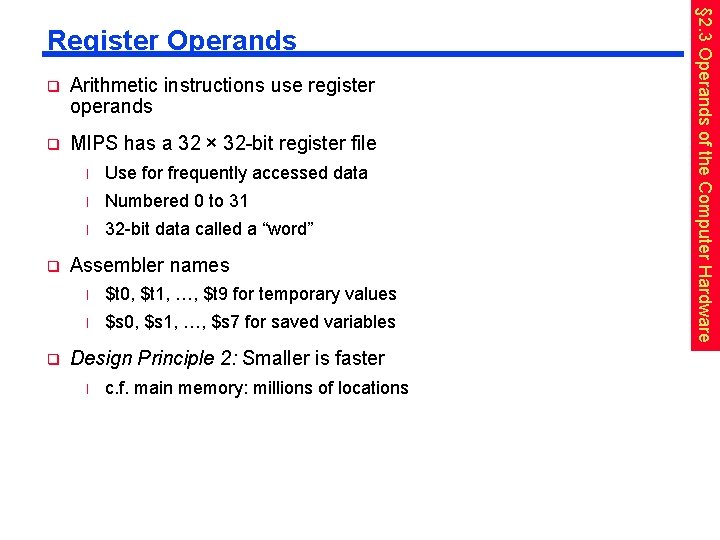
q Arithmetic instructions use register operands q MIPS has a 32 × 32 -bit register file q q l Use for frequently accessed data l Numbered 0 to 31 l 32 -bit data called a “word” Assembler names l $t 0, $t 1, …, $t 9 for temporary values l $s 0, $s 1, …, $s 7 for saved variables Design Principle 2: Smaller is faster l c. f. main memory: millions of locations § 2. 3 Operands of the Computer Hardware Register Operands
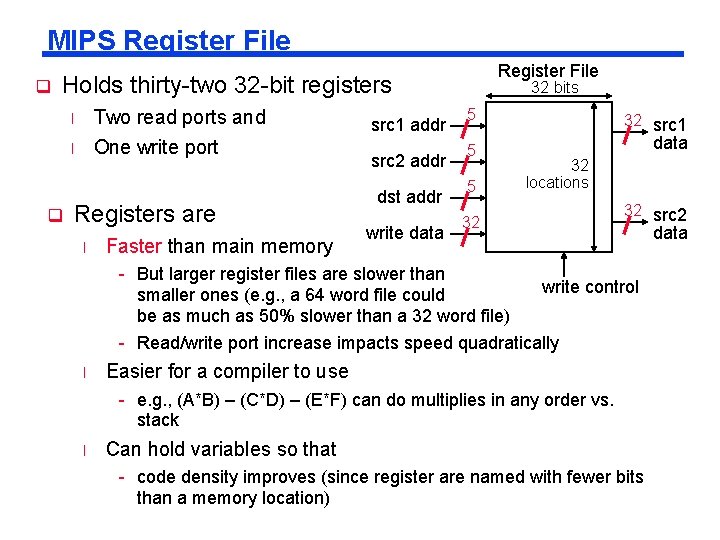
MIPS Register File q Register File Holds thirty-two 32 -bit registers Two read ports and One write port l l q Registers are l Faster than main memory src 1 addr src 2 addr dst addr write data 32 bits 5 5 5 32 src 1 data 32 locations 32 32 src 2 - But larger register files are slower than write control smaller ones (e. g. , a 64 word file could be as much as 50% slower than a 32 word file) - Read/write port increase impacts speed quadratically l Easier for a compiler to use - e. g. , (A*B) – (C*D) – (E*F) can do multiplies in any order vs. stack l Can hold variables so that - code density improves (since register are named with fewer bits than a memory location) data
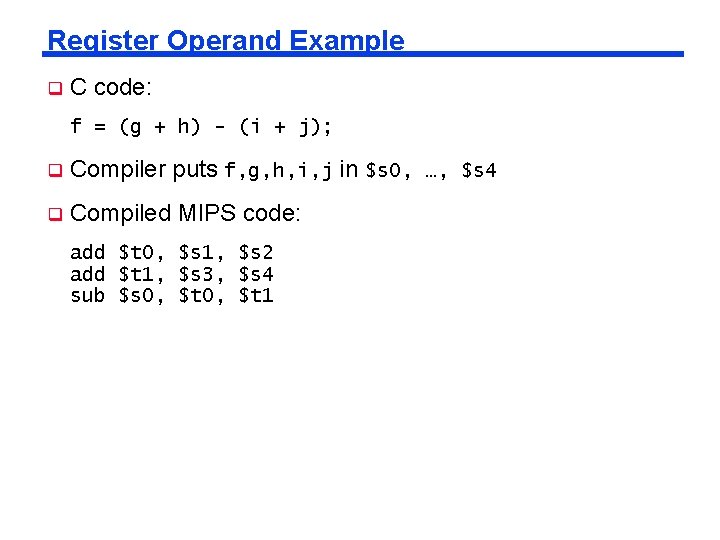
Register Operand Example q C code: f = (g + h) - (i + j); q Compiler puts f, g, h, i, j in $s 0, …, $s 4 q Compiled MIPS code: add $t 0, $s 1, $s 2 add $t 1, $s 3, $s 4 sub $s 0, $t 1
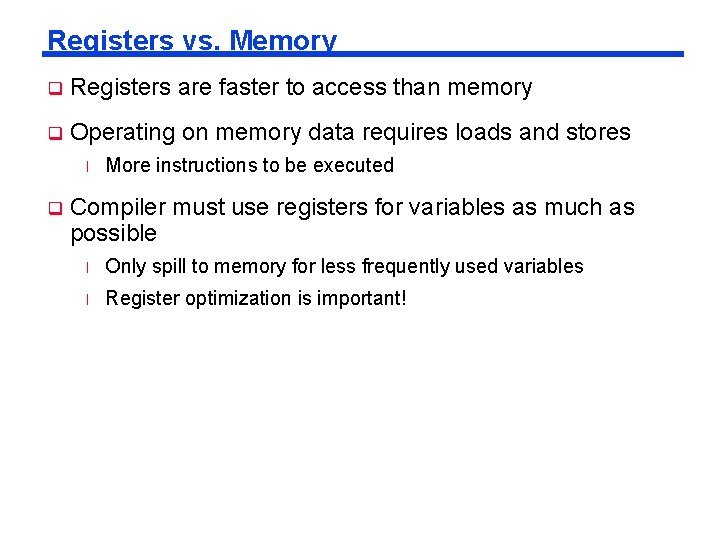
Registers vs. Memory q Registers are faster to access than memory q Operating on memory data requires loads and stores l q More instructions to be executed Compiler must use registers for variables as much as possible l Only spill to memory for less frequently used variables l Register optimization is important!
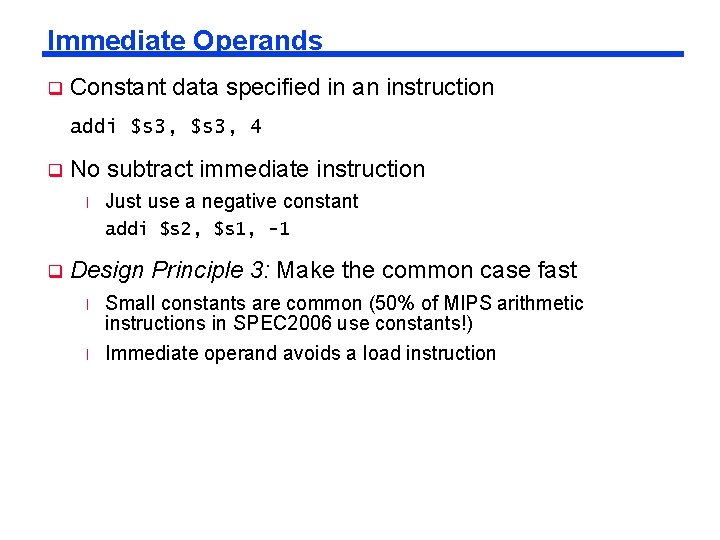
Immediate Operands q Constant data specified in an instruction addi $s 3, 4 q No subtract immediate instruction l Just use a negative constant addi $s 2, $s 1, -1 q Design Principle 3: Make the common case fast l Small constants are common (50% of MIPS arithmetic instructions in SPEC 2006 use constants!) l Immediate operand avoids a load instruction
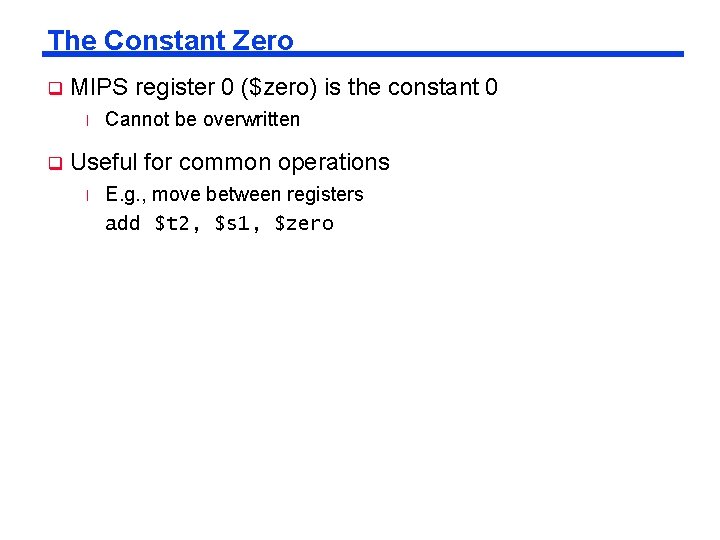
The Constant Zero q MIPS register 0 ($zero) is the constant 0 l q Cannot be overwritten Useful for common operations l E. g. , move between registers add $t 2, $s 1, $zero
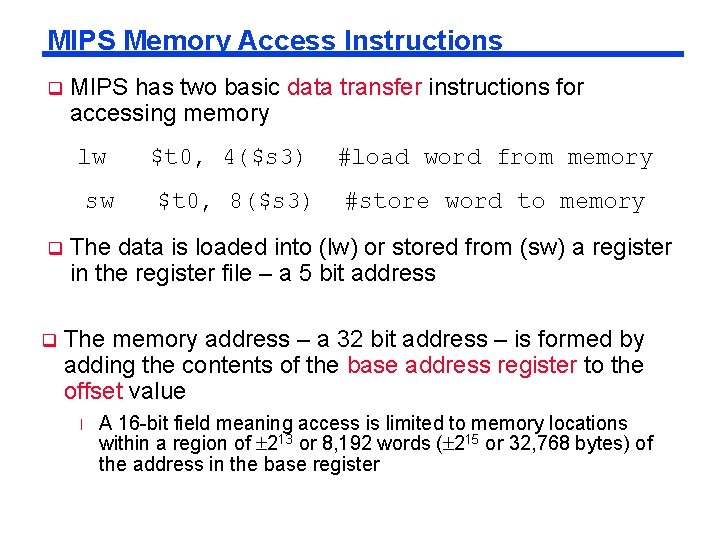
MIPS Memory Access Instructions q MIPS has two basic data transfer instructions for accessing memory lw sw q q $t 0, 4($s 3) $t 0, 8($s 3) #load word from memory #store word to memory The data is loaded into (lw) or stored from (sw) a register in the register file – a 5 bit address The memory address – a 32 bit address – is formed by adding the contents of the base address register to the offset value l A 16 -bit field meaning access is limited to memory locations within a region of 213 or 8, 192 words ( 215 or 32, 768 bytes) of the address in the base register
![Memory Operand Example 1 q C code g h A8 l q Memory Operand Example 1 q C code: g = h + A[8]; l q](https://slidetodoc.com/presentation_image_h2/0c7a7a2b048aa81803cbc8e22a62966e/image-16.jpg)
Memory Operand Example 1 q C code: g = h + A[8]; l q g in $s 1, h in $s 2, base address of A in $s 3 Compiled MIPS code: l Index 8 requires offset of 32 bytes - 4 bytes per word lw $t 0, 32($s 3) add $s 1, $s 2, $t 0 offset # load word base register
![Memory Operand Example 2 q C code A12 h A8 l q Memory Operand Example 2 q C code: A[12] = h + A[8]; l q](https://slidetodoc.com/presentation_image_h2/0c7a7a2b048aa81803cbc8e22a62966e/image-17.jpg)
Memory Operand Example 2 q C code: A[12] = h + A[8]; l q h in $s 2, base address of A in $s 3 Compiled MIPS code: l Index 8 requires offset of 32 bytes l Index 12 requires offset of 48 bytes lw $t 0, 32($s 3) add $t 0, $s 2, $t 0 sw $t 0, 48($s 3) # load word # store word
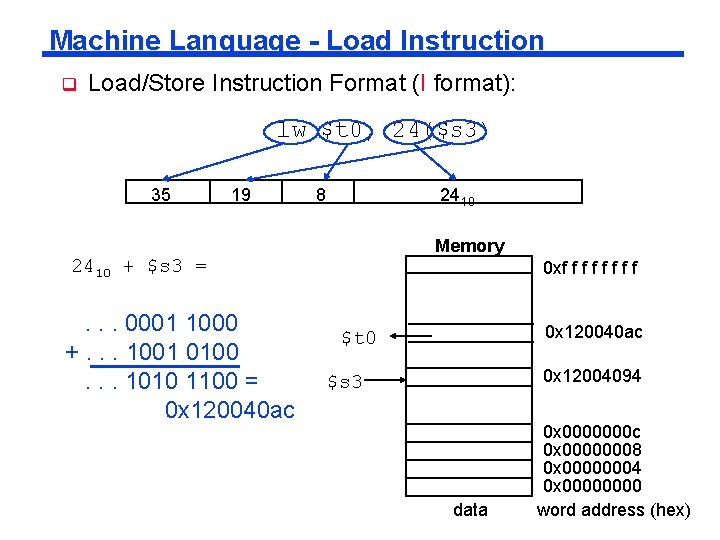
Machine Language - Load Instruction q Load/Store Instruction Format (I format): lw $t 0, 24($s 3) 35 19 8 24 10 Memory 2410 + $s 3 = . . . 0001 1000 +. . . 1001 0100. . . 1010 1100 = 0 x 120040 ac 0 xf f f f 0 x 120040 ac $t 0 0 x 12004094 $s 3 data 0 x 0000000 c 0 x 00000008 0 x 00000004 0 x 0000 word address (hex)
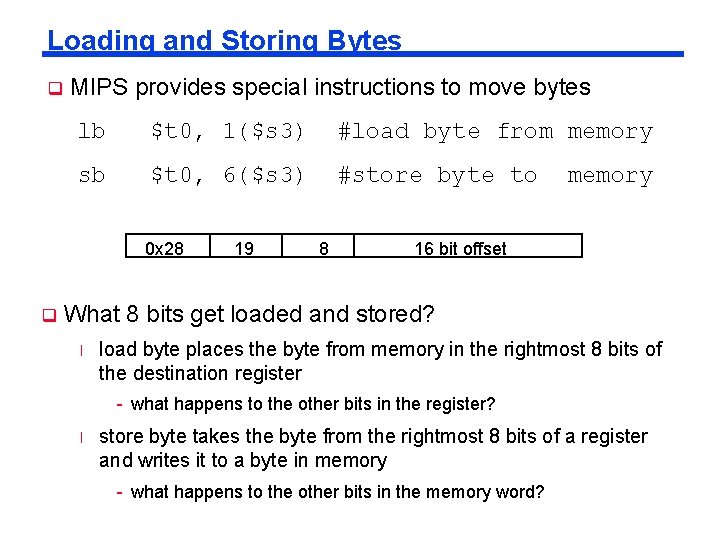
Loading and Storing Bytes q MIPS provides special instructions to move bytes lb $t 0, 1($s 3) #load byte from memory sb $t 0, 6($s 3) #store byte to 0 x 28 q 19 8 memory 16 bit offset What 8 bits get loaded and stored? l load byte places the byte from memory in the rightmost 8 bits of the destination register - what happens to the other bits in the register? l store byte takes the byte from the rightmost 8 bits of a register and writes it to a byte in memory - what happens to the other bits in the memory word?
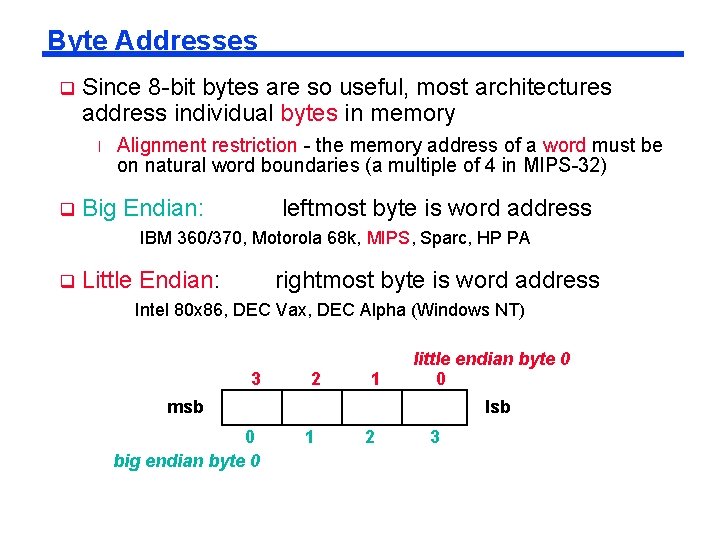
Byte Addresses q Since 8 -bit bytes are so useful, most architectures address individual bytes in memory l q Alignment restriction - the memory address of a word must be on natural word boundaries (a multiple of 4 in MIPS-32) Big Endian: leftmost byte is word address IBM 360/370, Motorola 68 k, MIPS, Sparc, HP PA q Little Endian: rightmost byte is word address Intel 80 x 86, DEC Vax, DEC Alpha (Windows NT) 3 2 1 little endian byte 0 0 msb 0 big endian byte 0 lsb 1 2 3
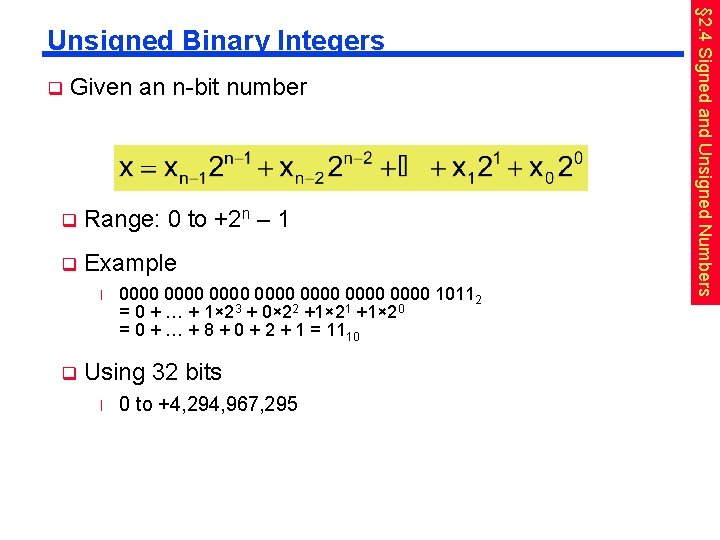
q Given an n-bit number q Range: 0 to +2 n – 1 q Example l q 0000 0000 10112 = 0 + … + 1× 23 + 0× 22 +1× 21 +1× 20 = 0 + … + 8 + 0 + 2 + 1 = 1110 Using 32 bits l 0 to +4, 294, 967, 295 § 2. 4 Signed and Unsigned Numbers Unsigned Binary Integers
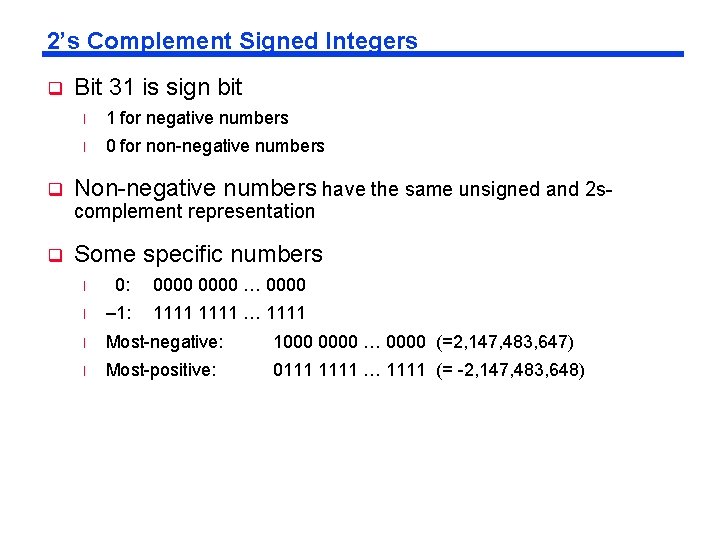
2’s Complement Signed Integers q q Bit 31 is sign bit l 1 for negative numbers l 0 for non-negative numbers Non-negative numbers have the same unsigned and 2 scomplement representation q Some specific numbers l 0: 0000 … 0000 l – 1: 1111 … 1111 l Most-negative: 1000 0000 … 0000 (=2, 147, 483, 647) l Most-positive: 0111 1111 … 1111 (= -2, 147, 483, 648)
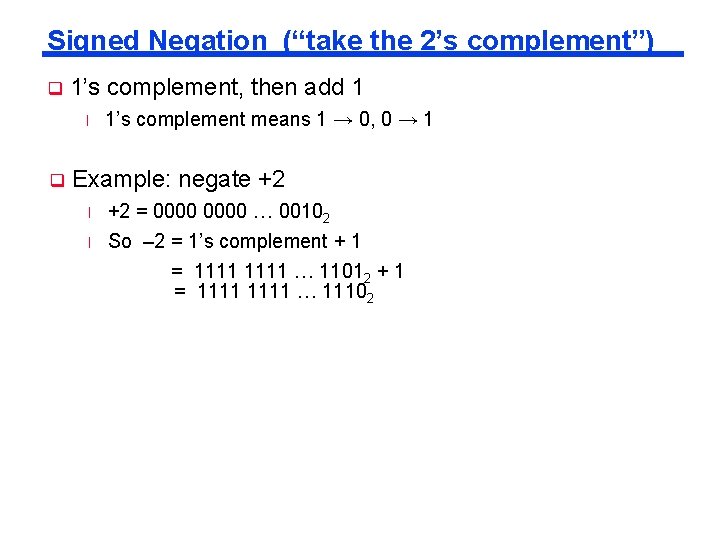
Signed Negation (“take the 2’s complement”) q 1’s complement, then add 1 l q 1’s complement means 1 → 0, 0 → 1 Example: negate +2 l l +2 = 0000 … 00102 So – 2 = 1’s complement + 1 = 1111 … 11012 + 1 = 1111 … 11102
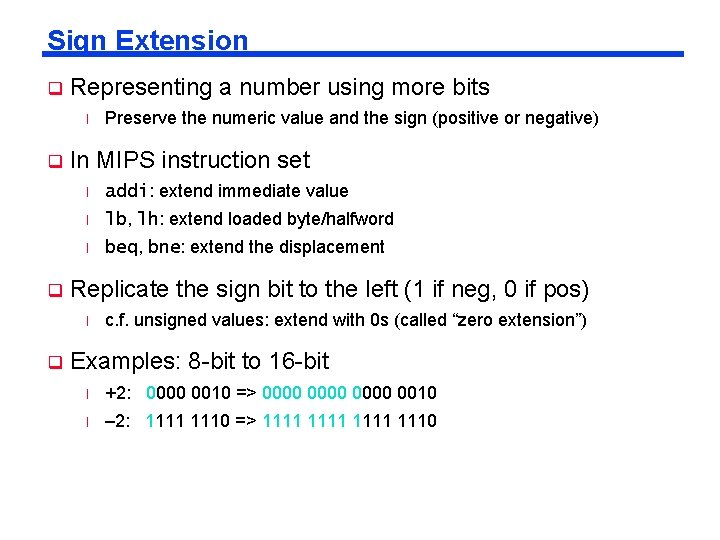
Sign Extension q Representing a number using more bits l q q In MIPS instruction set l addi: extend immediate value l lb, lh: extend loaded byte/halfword l beq, bne: extend the displacement Replicate the sign bit to the left (1 if neg, 0 if pos) l q Preserve the numeric value and the sign (positive or negative) c. f. unsigned values: extend with 0 s (called “zero extension”) Examples: 8 -bit to 16 -bit l +2: 0000 0010 => 0000 0010 l – 2: 1111 1110 => 1111 1110
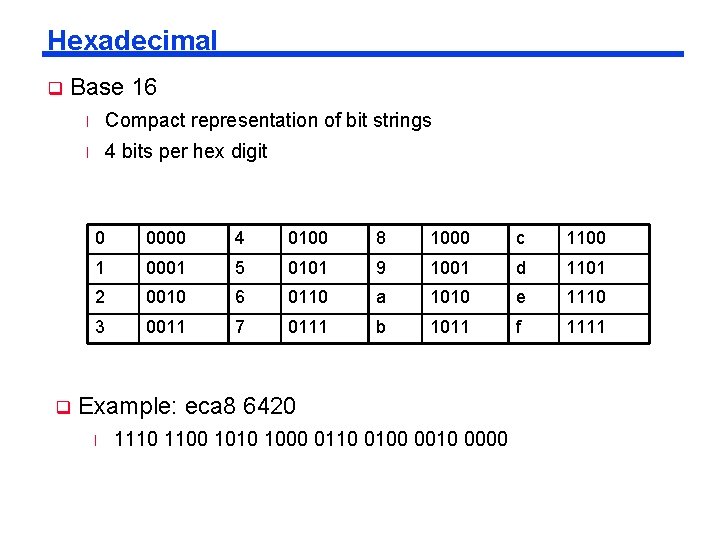
Hexadecimal q Base 16 q l Compact representation of bit strings l 4 bits per hex digit 0 0000 4 0100 8 1000 c 1100 1 0001 5 0101 9 1001 d 1101 2 0010 6 0110 a 1010 e 1110 3 0011 7 0111 b 1011 f 1111 Example: eca 8 6420 l 1110 1100 1010 1000 0110 0100 0010 0000
Cs-224 computer organization
Process organization in computer organization
Basic structure of a computer system
Difference computer organization and architecture
Design of basic computer
Basic computer organization
Plastic shrinkage cracking repair
Edu224
Embolie pulmonaire item
Asw 224
Ece 224
Bivariate linear regression
Din 800 kg carbid un sudor obtine 224
Cs 224
Binary 10110
Cs 224w
Soc 224
Km 224
Alkan alken alkin asitlik sıralaması
Oksimerkürasyon
Km 224
Mat 224
Ece 224
Dea number
224+48
224+48