COMP 2710 Software Construction Midterm Exam 1 Review
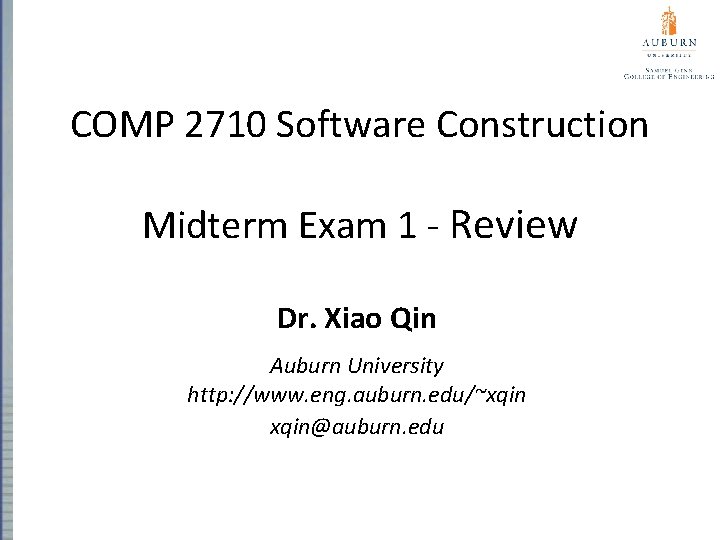
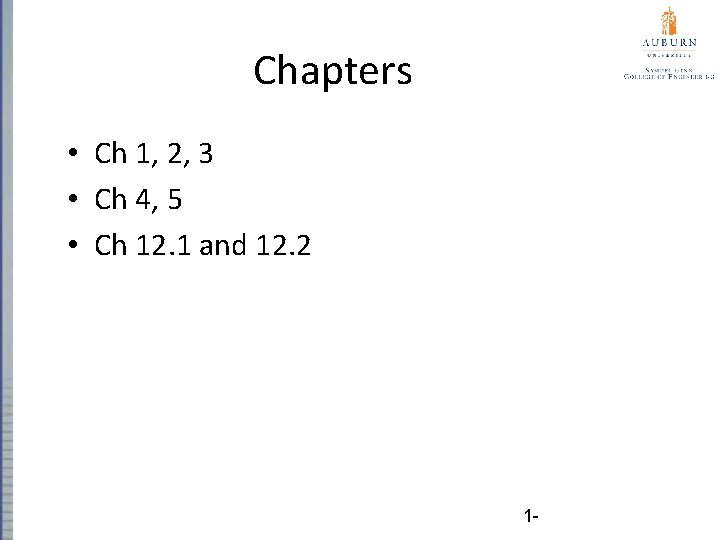
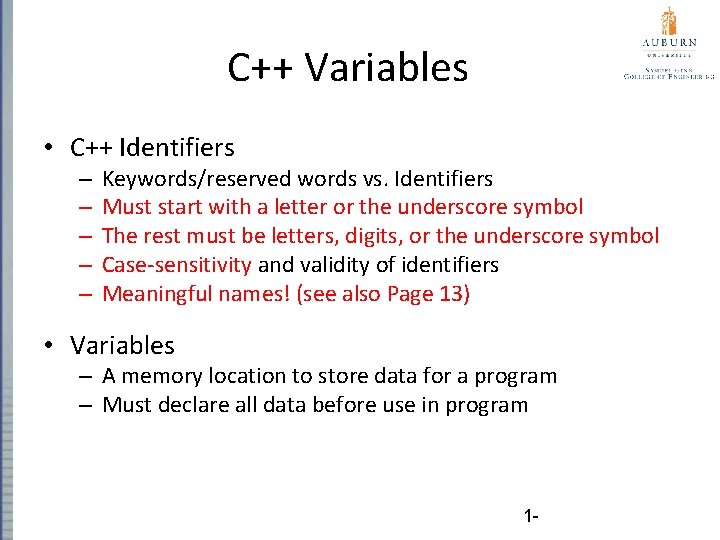
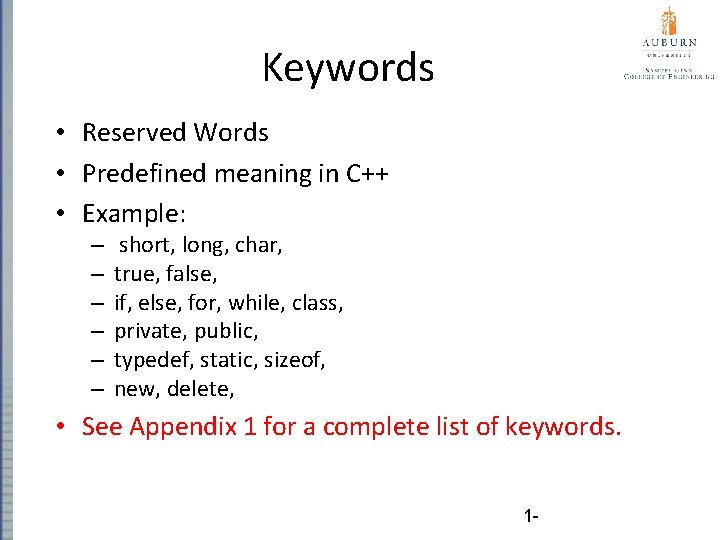
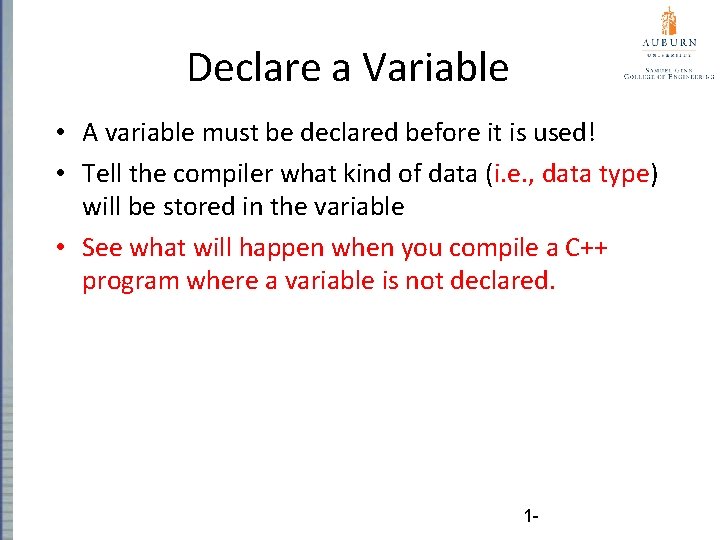
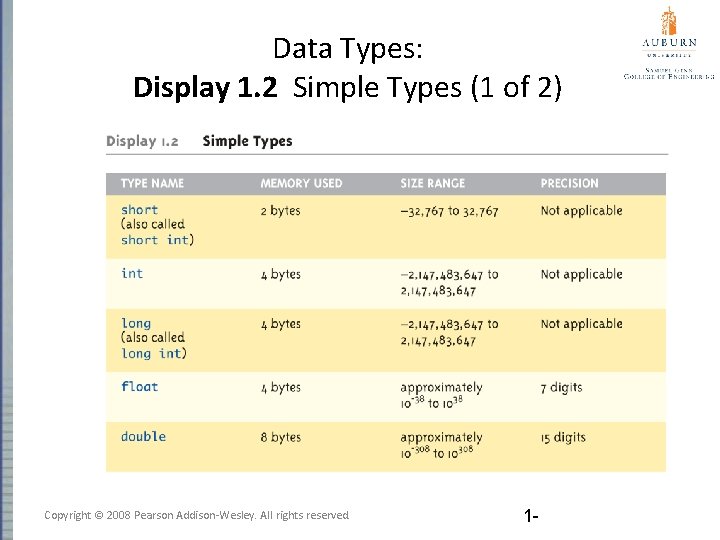
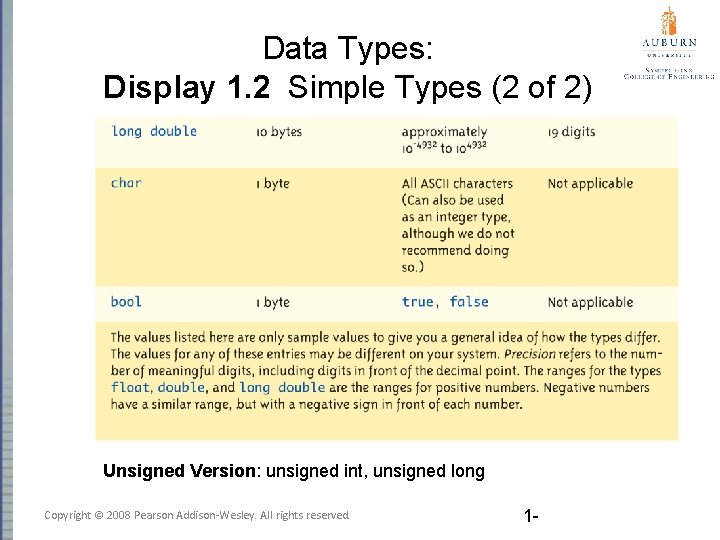
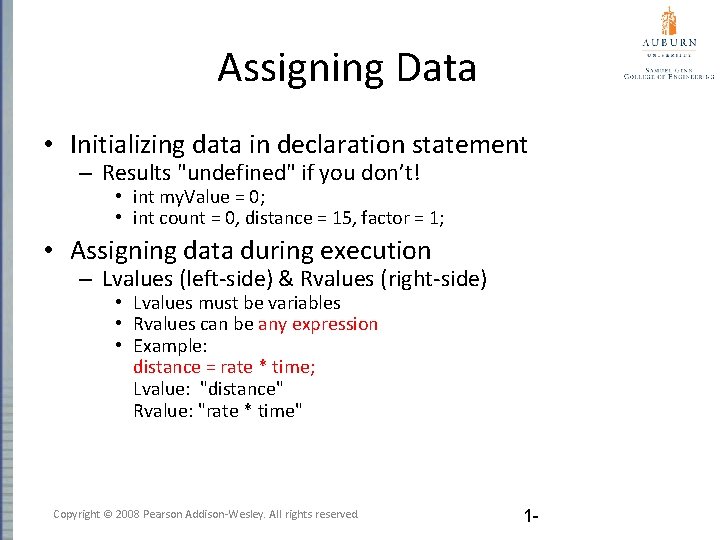
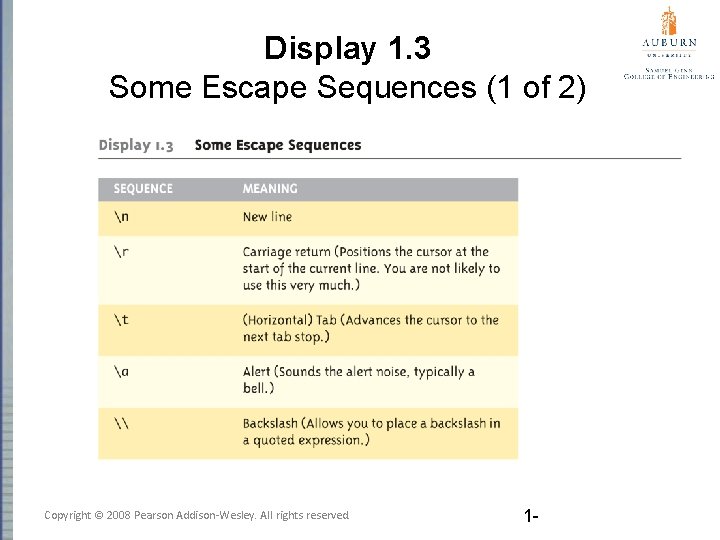
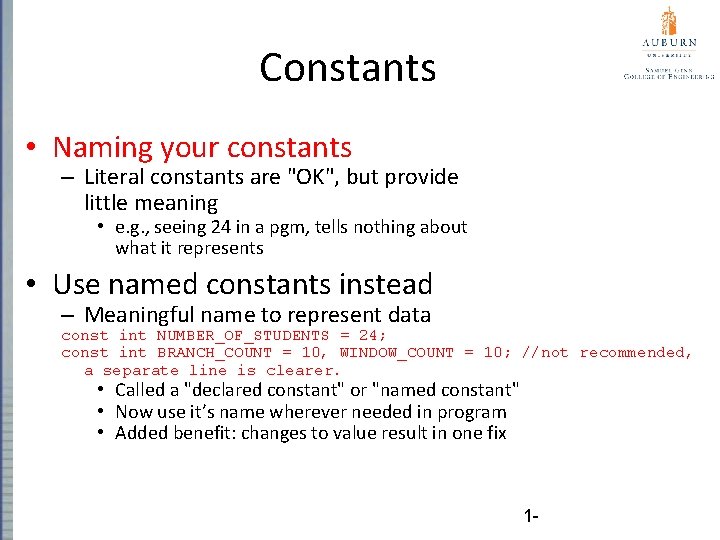
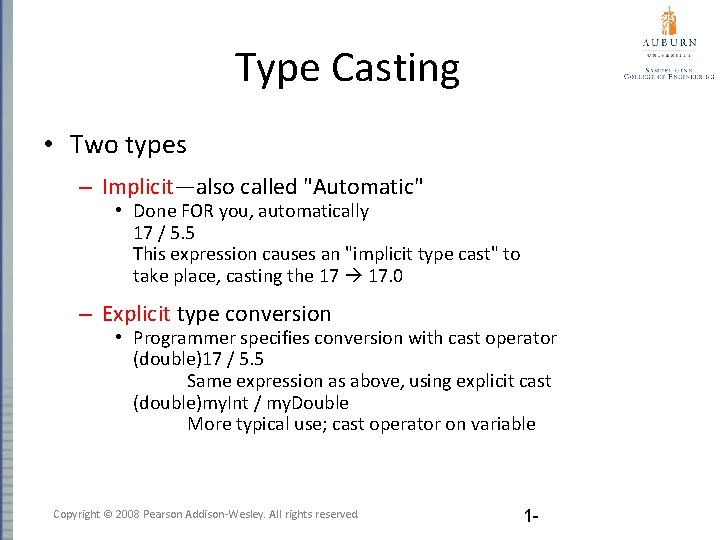
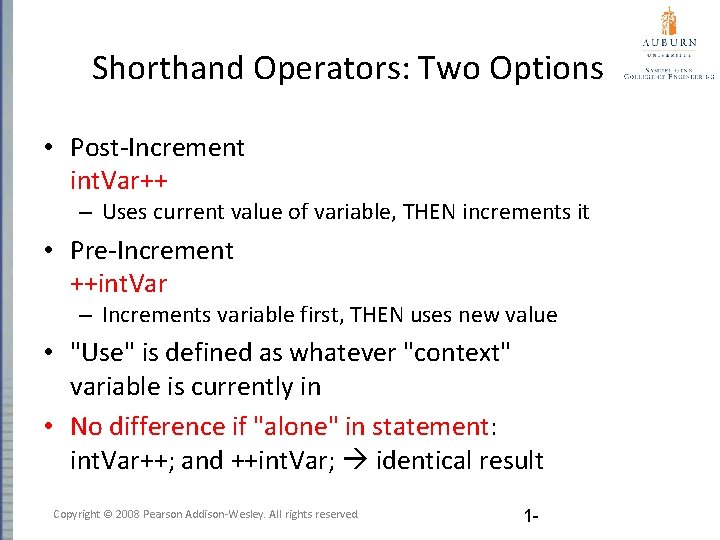
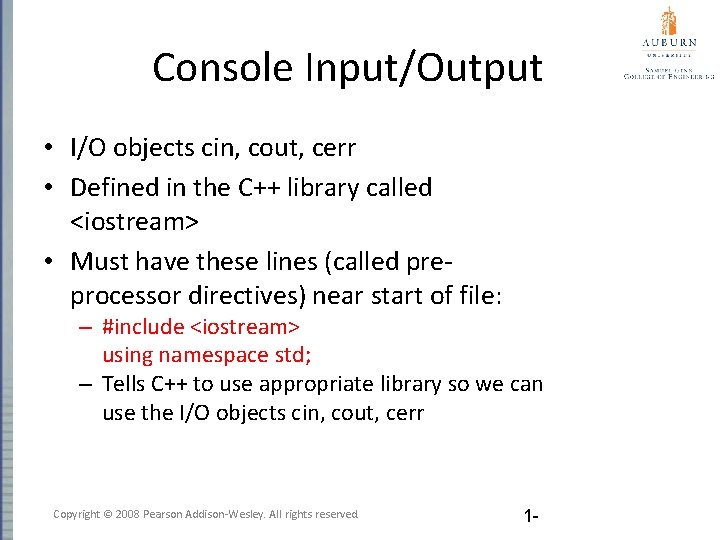
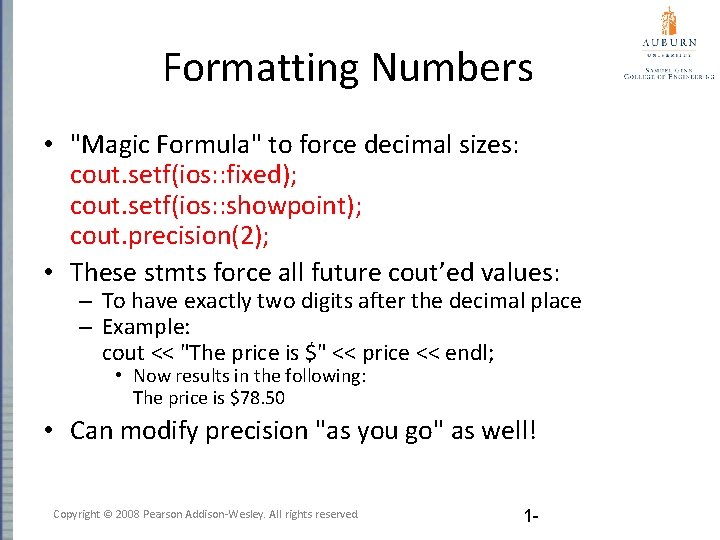
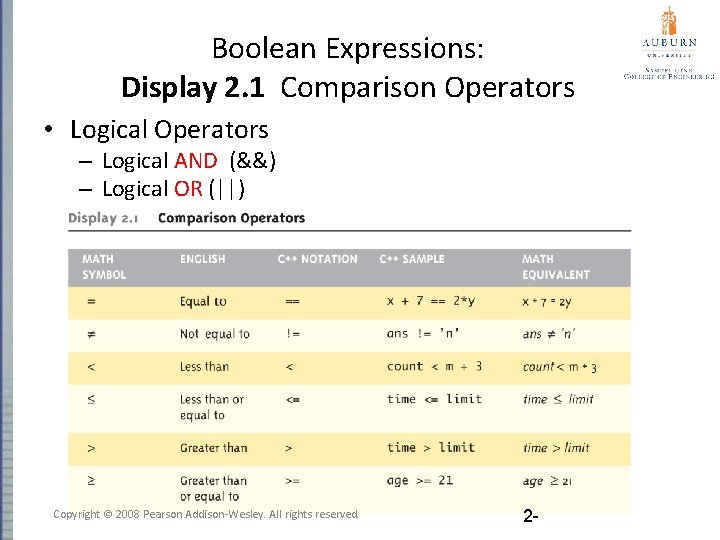
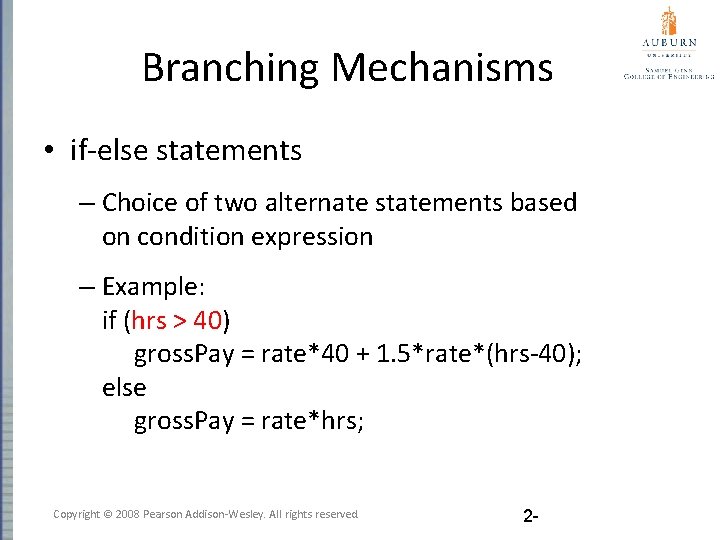
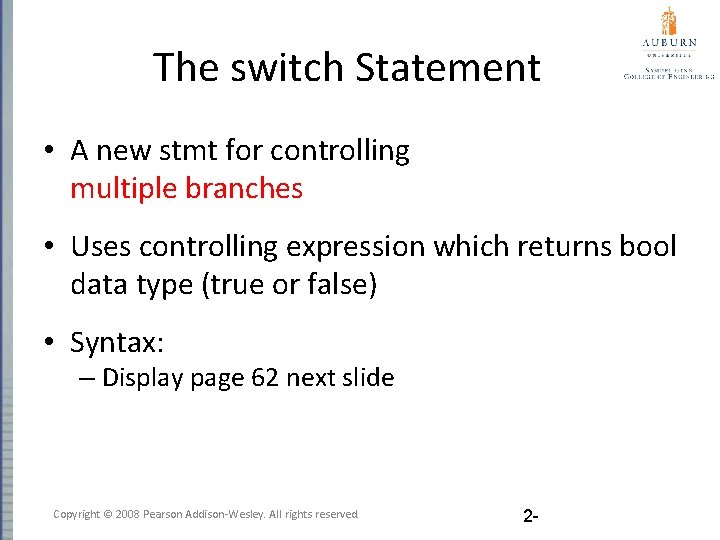
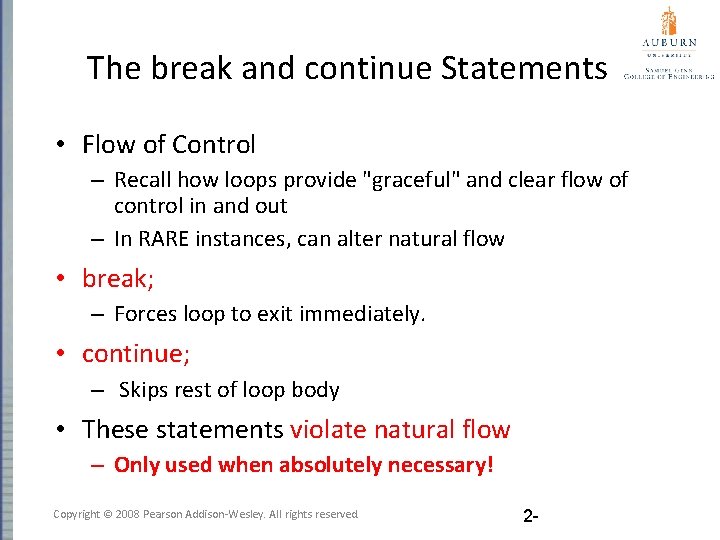
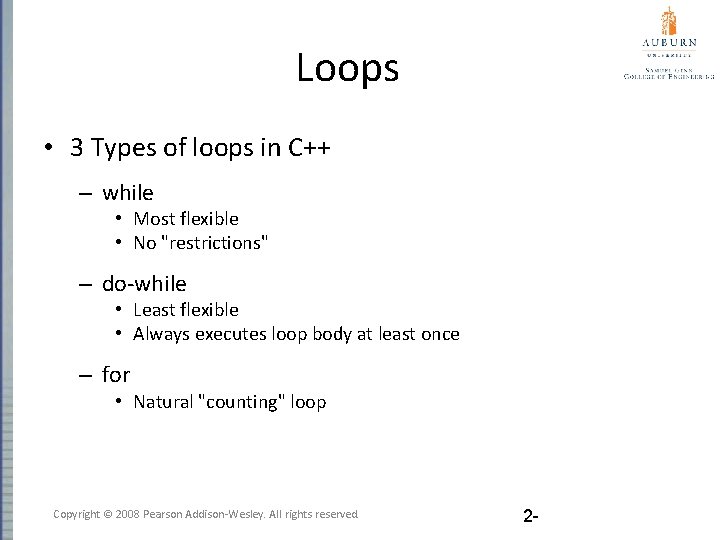
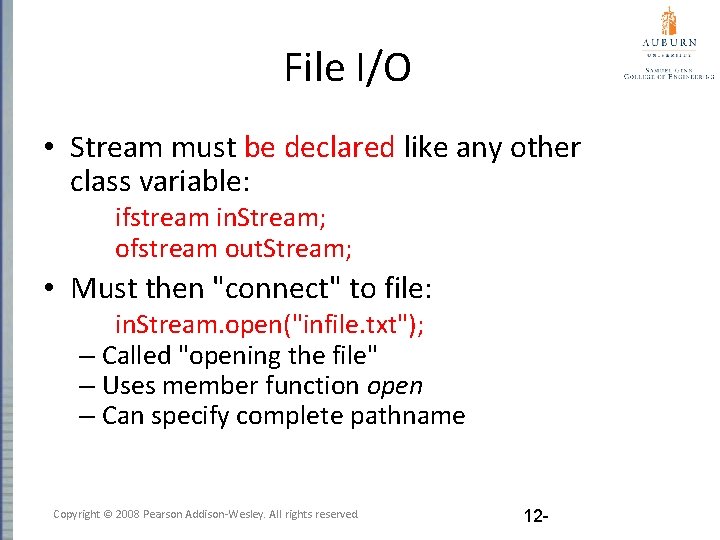
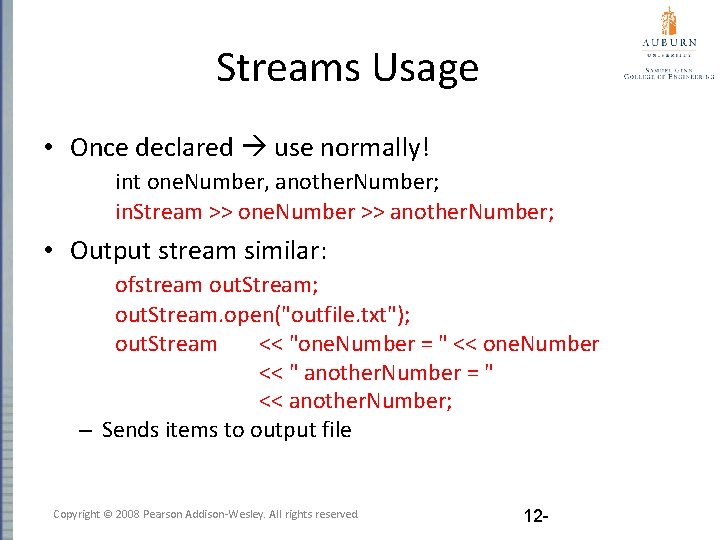
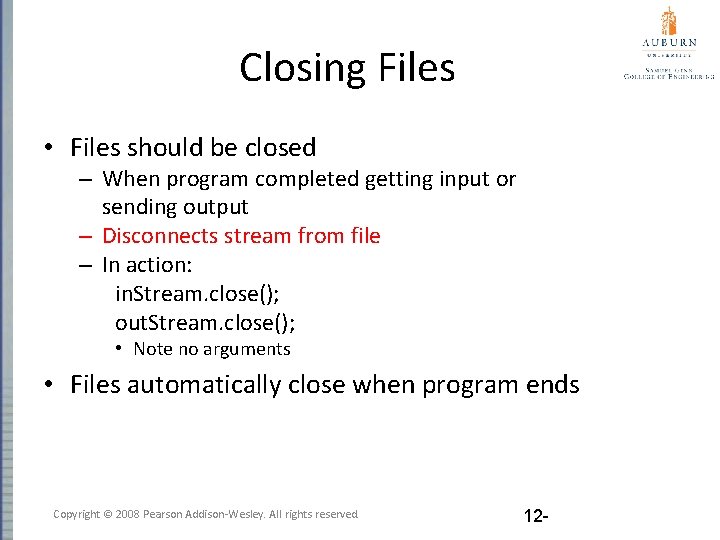
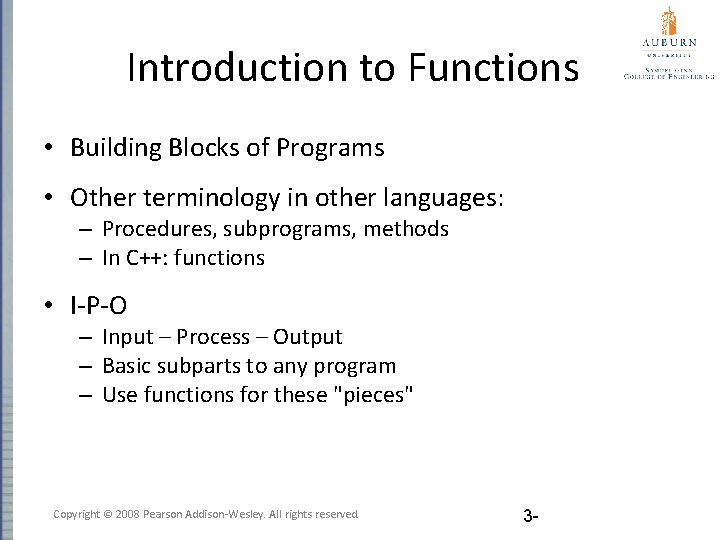
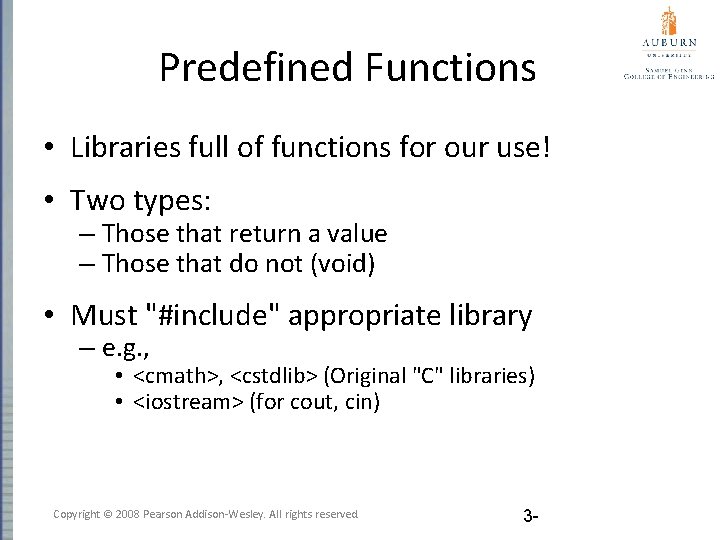
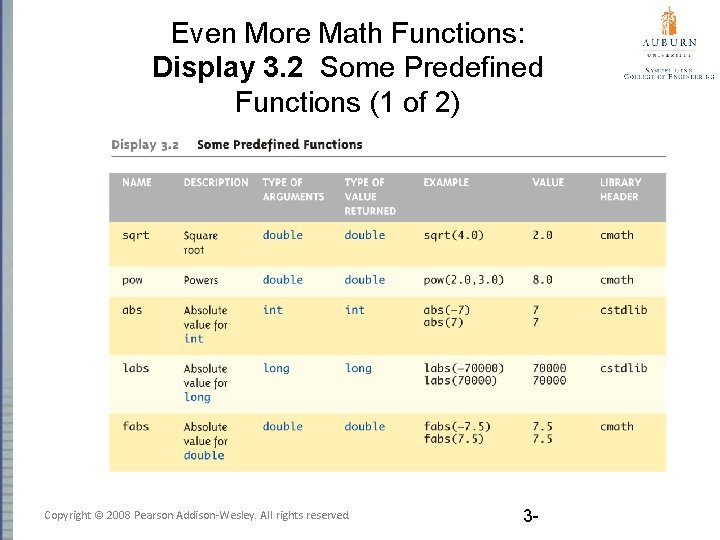
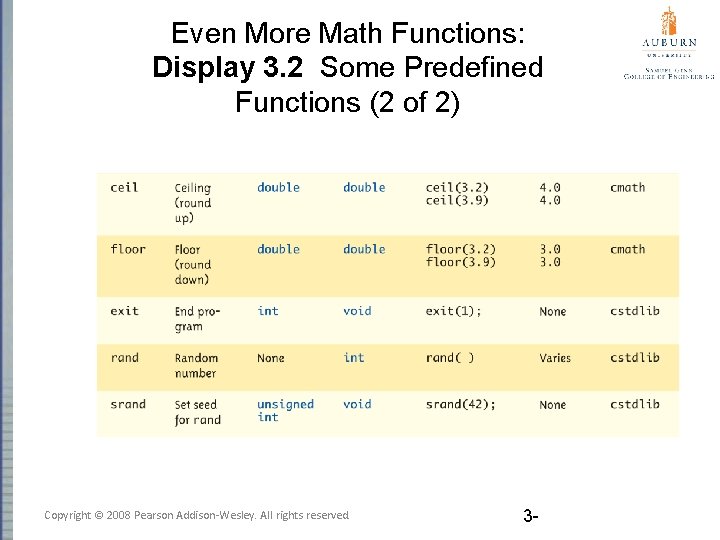
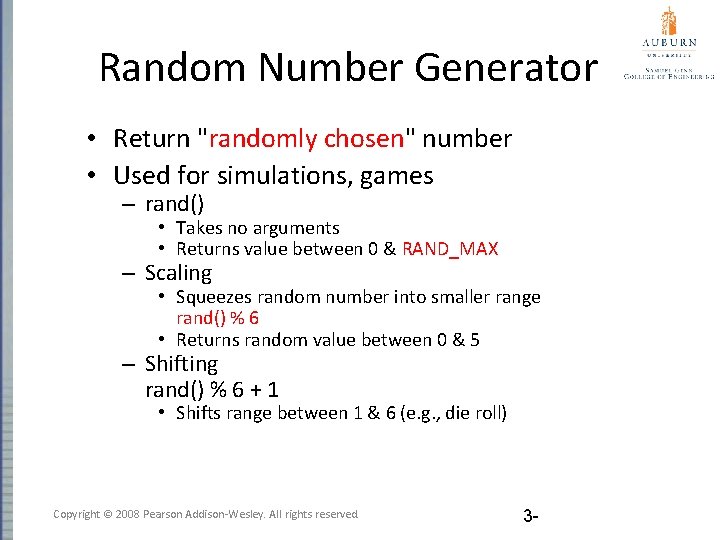
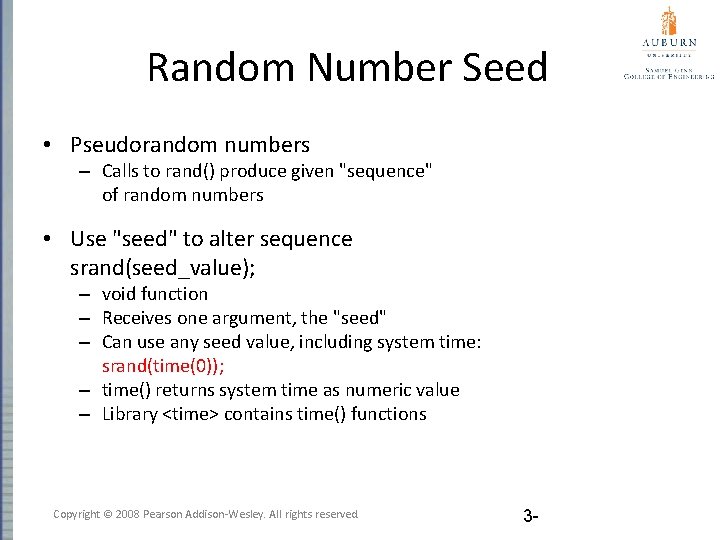
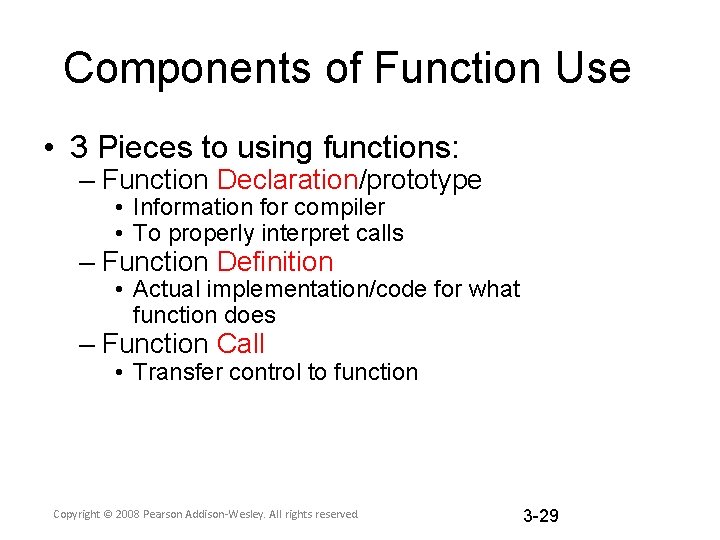
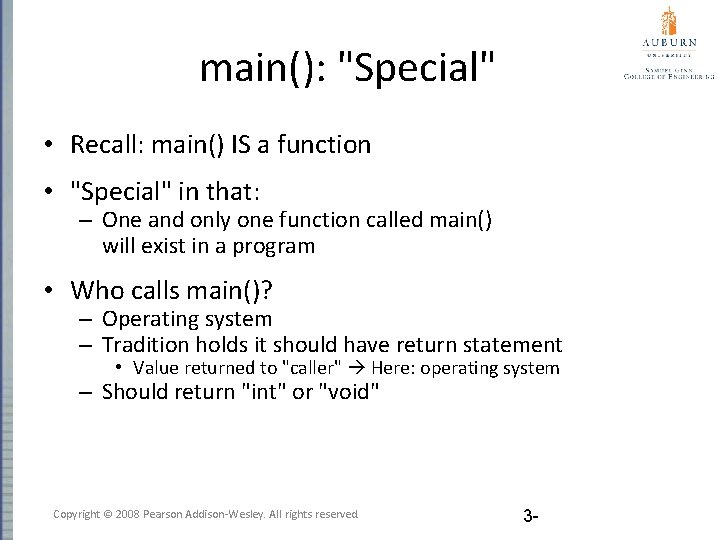
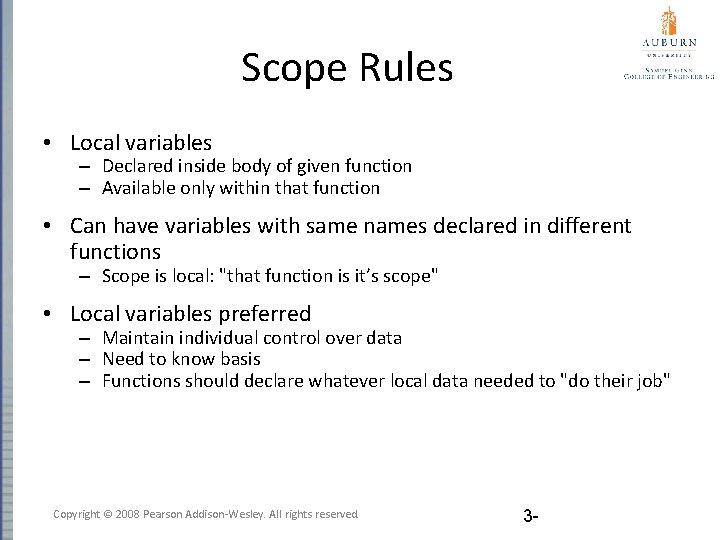
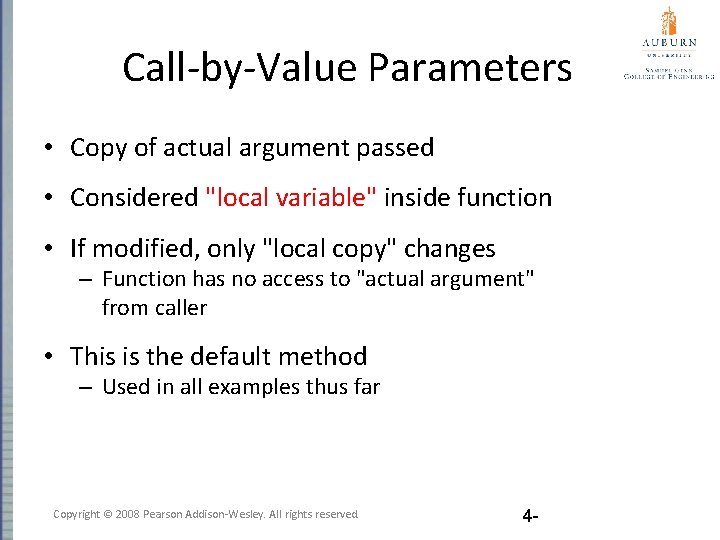
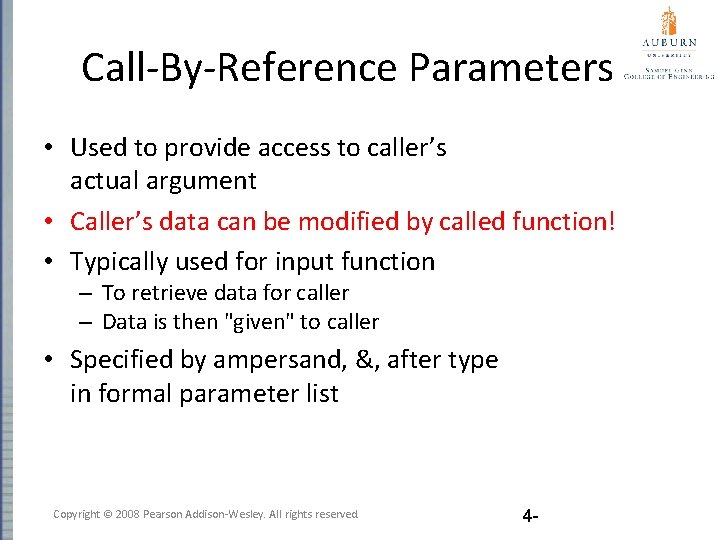
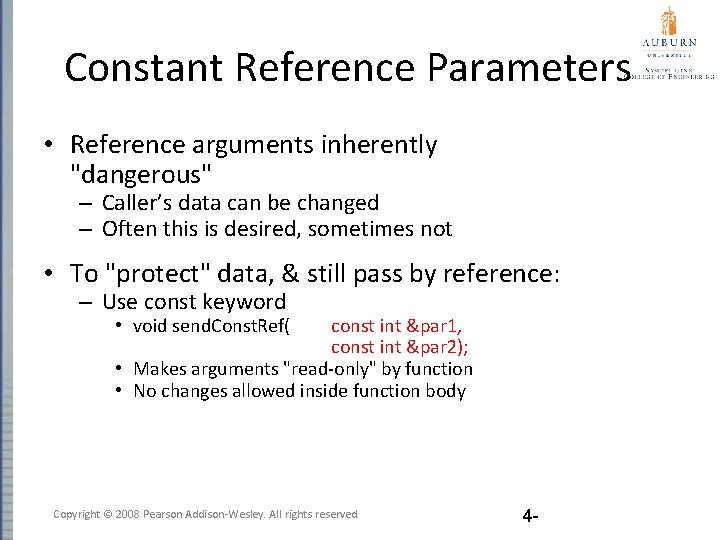
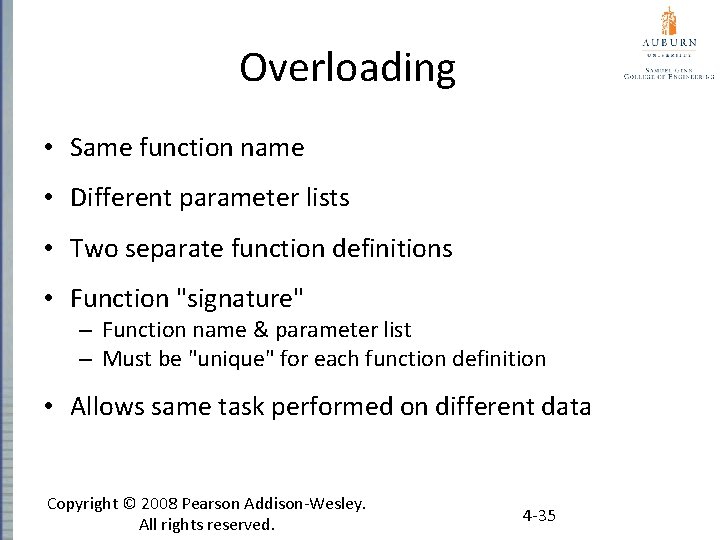
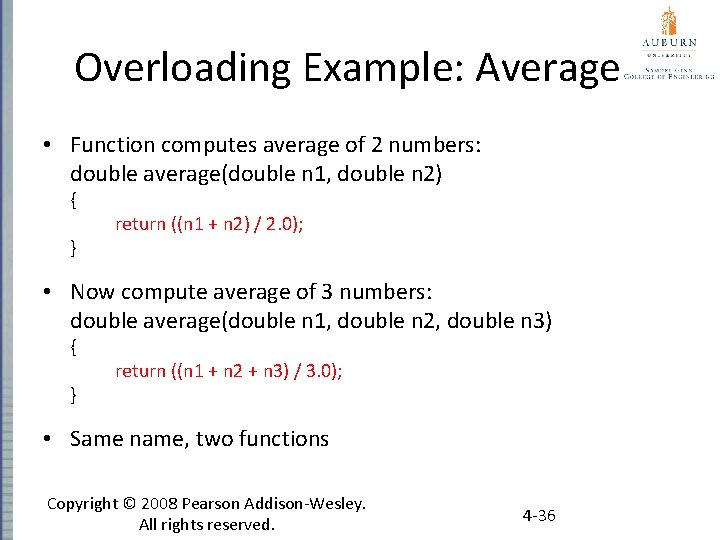
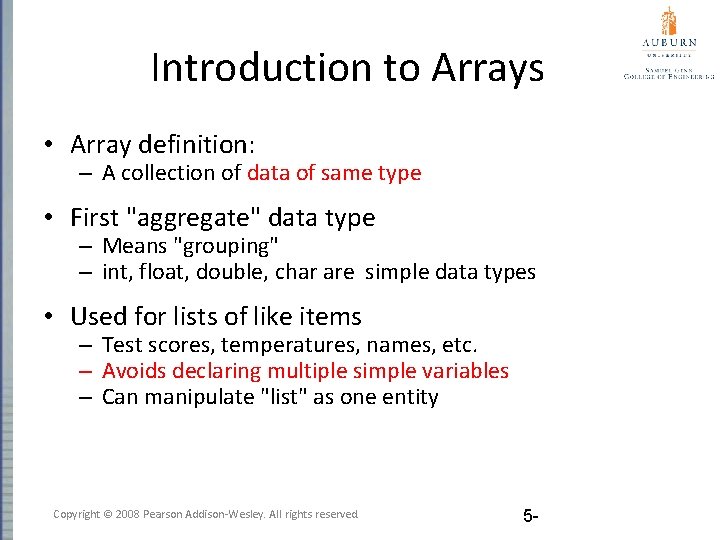
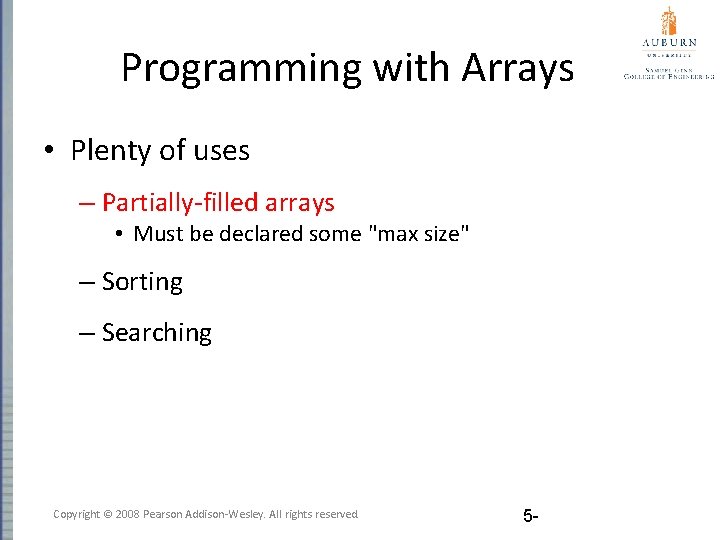
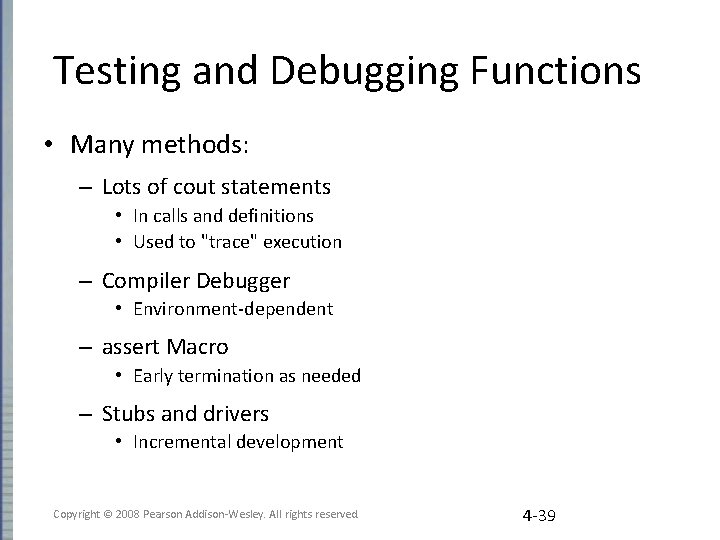
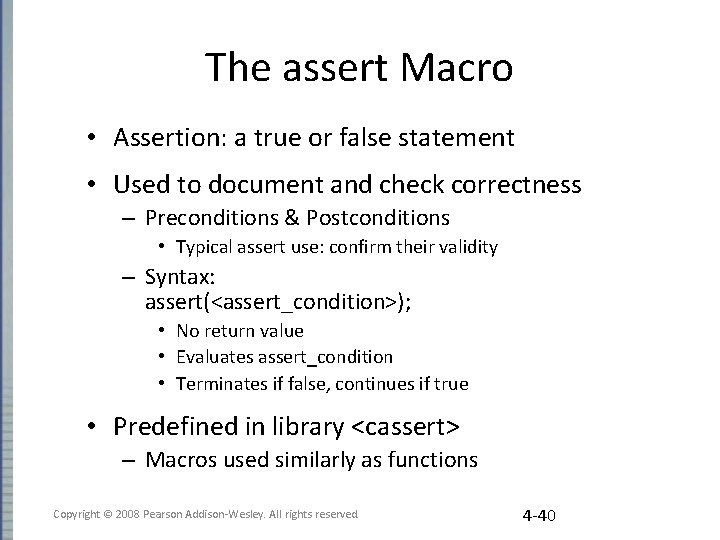
- Slides: 40
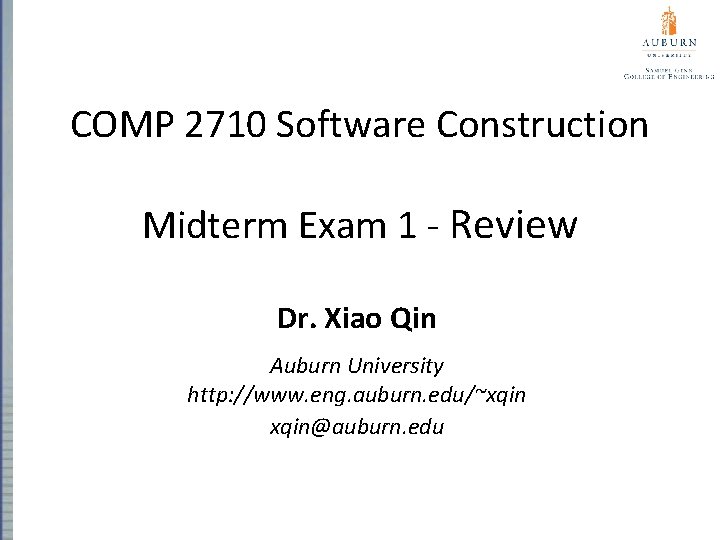
COMP 2710 Software Construction Midterm Exam 1 - Review Dr. Xiao Qin Auburn University http: //www. eng. auburn. edu/~xqin@auburn. edu
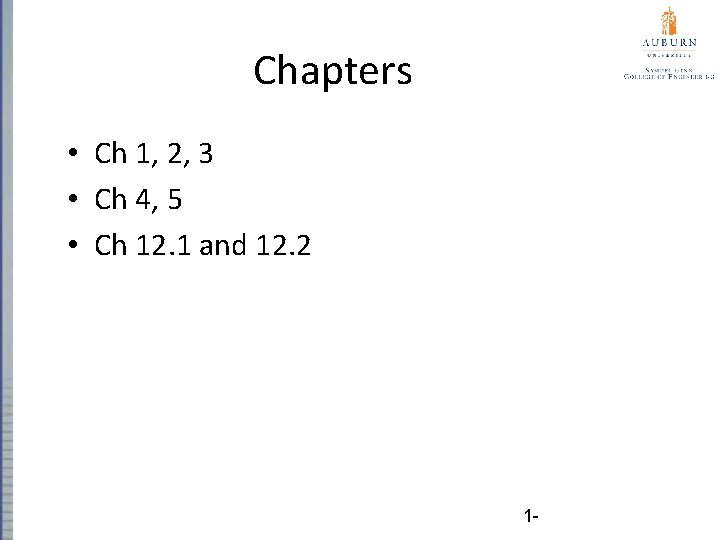
Chapters • Ch 1, 2, 3 • Ch 4, 5 • Ch 12. 1 and 12. 2 1 -
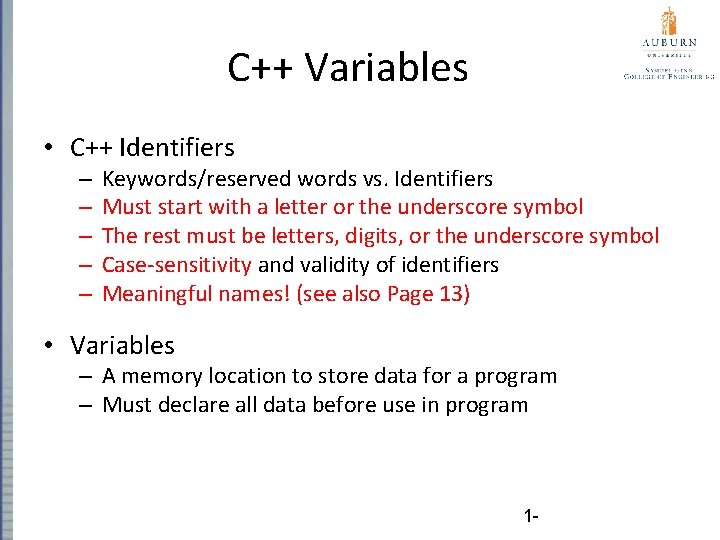
C++ Variables • C++ Identifiers – – – Keywords/reserved words vs. Identifiers Must start with a letter or the underscore symbol The rest must be letters, digits, or the underscore symbol Case-sensitivity and validity of identifiers Meaningful names! (see also Page 13) • Variables – A memory location to store data for a program – Must declare all data before use in program 1 -
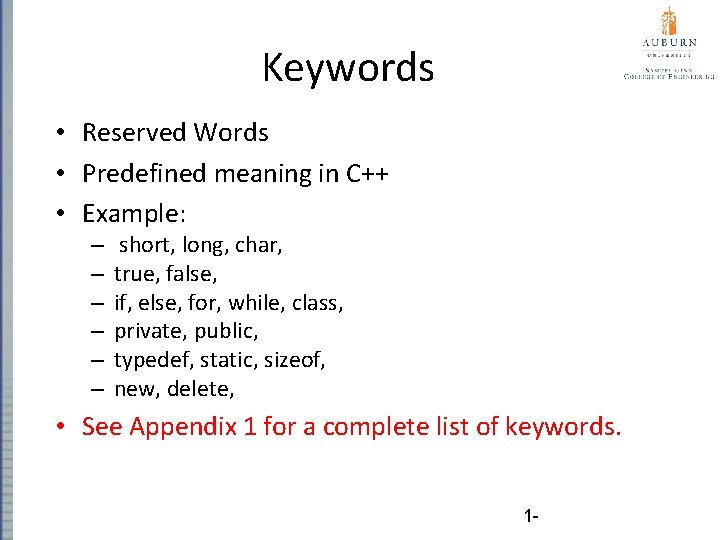
Keywords • Reserved Words • Predefined meaning in C++ • Example: – – – short, long, char, true, false, if, else, for, while, class, private, public, typedef, static, sizeof, new, delete, • See Appendix 1 for a complete list of keywords. 1 -
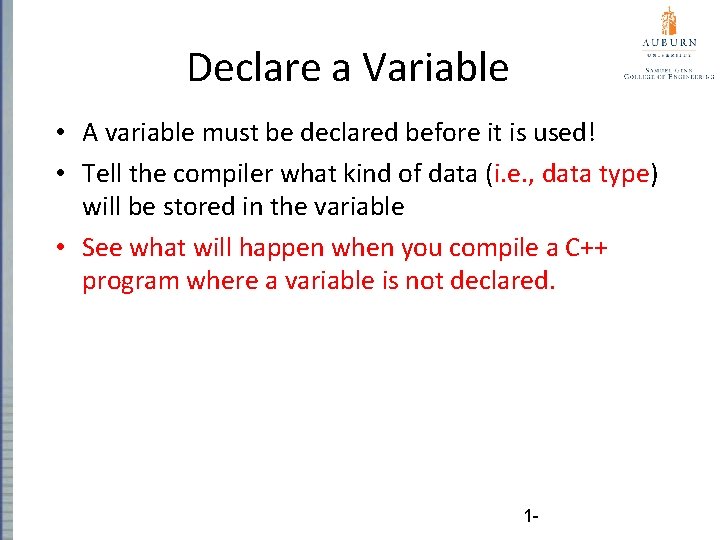
Declare a Variable • A variable must be declared before it is used! • Tell the compiler what kind of data (i. e. , data type) will be stored in the variable • See what will happen when you compile a C++ program where a variable is not declared. 1 -
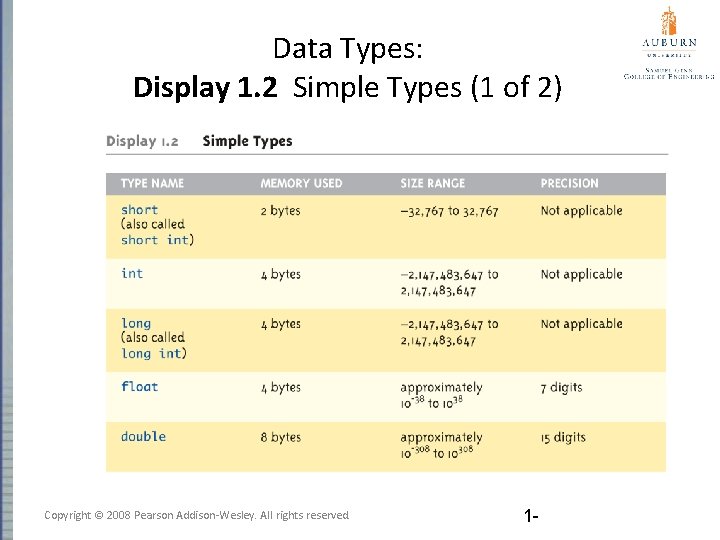
Data Types: Display 1. 2 Simple Types (1 of 2) Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 1 -
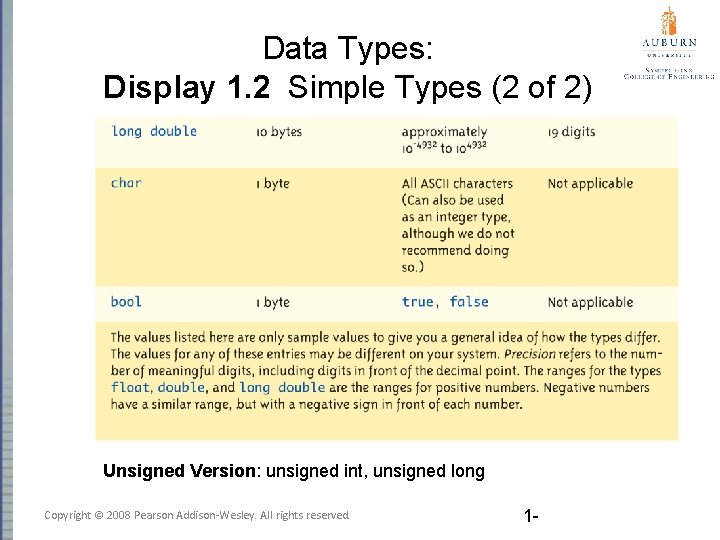
Data Types: Display 1. 2 Simple Types (2 of 2) Unsigned Version: unsigned int, unsigned long Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 1 -
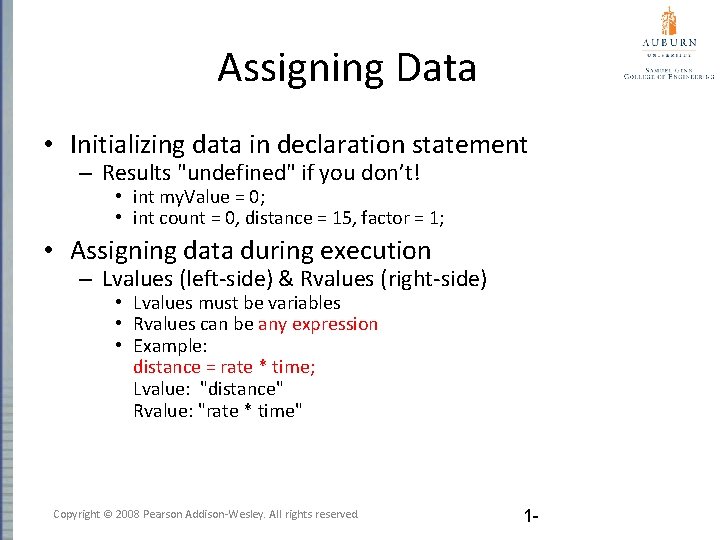
Assigning Data • Initializing data in declaration statement – Results "undefined" if you don’t! • int my. Value = 0; • int count = 0, distance = 15, factor = 1; • Assigning data during execution – Lvalues (left-side) & Rvalues (right-side) • Lvalues must be variables • Rvalues can be any expression • Example: distance = rate * time; Lvalue: "distance" Rvalue: "rate * time" Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 1 -
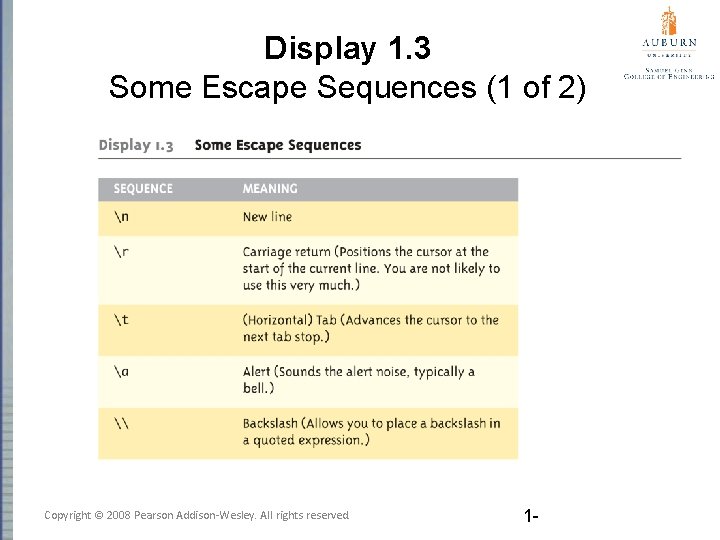
Display 1. 3 Some Escape Sequences (1 of 2) Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 1 -
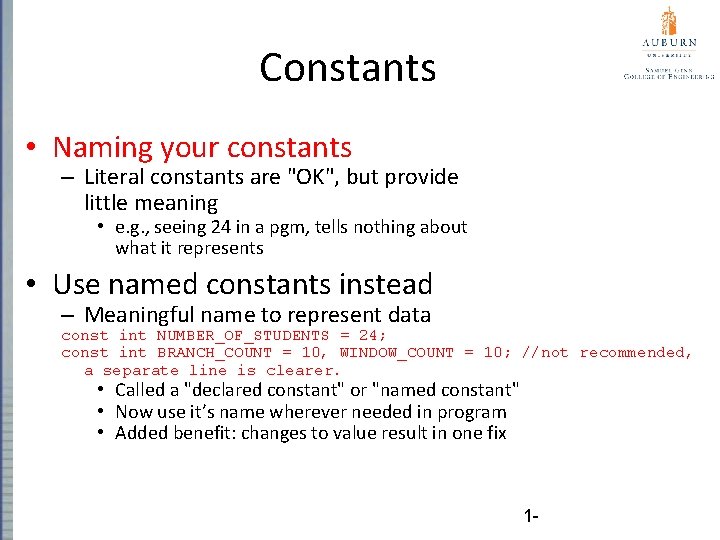
Constants • Naming your constants – Literal constants are "OK", but provide little meaning • e. g. , seeing 24 in a pgm, tells nothing about what it represents • Use named constants instead – Meaningful name to represent data const int NUMBER_OF_STUDENTS = 24; const int BRANCH_COUNT = 10, WINDOW_COUNT = 10; //not recommended, a separate line is clearer. • Called a "declared constant" or "named constant" • Now use it’s name wherever needed in program • Added benefit: changes to value result in one fix 1 -
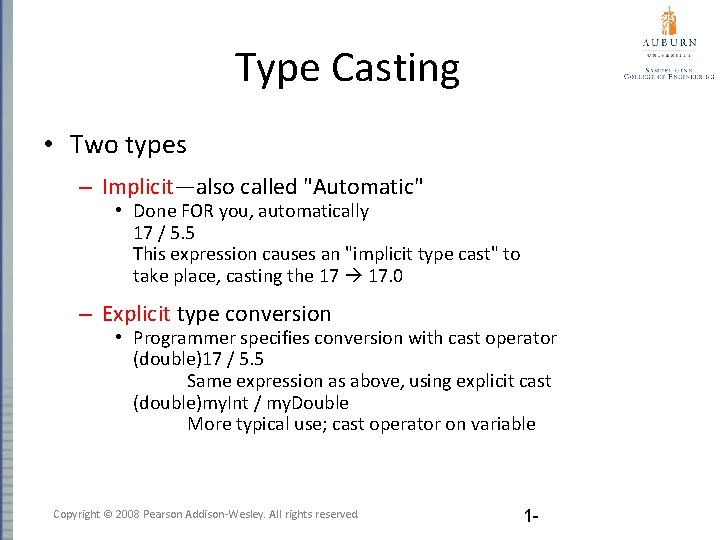
Type Casting • Two types – Implicit—also called "Automatic" • Done FOR you, automatically 17 / 5. 5 This expression causes an "implicit type cast" to take place, casting the 17 17. 0 – Explicit type conversion • Programmer specifies conversion with cast operator (double)17 / 5. 5 Same expression as above, using explicit cast (double)my. Int / my. Double More typical use; cast operator on variable Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 1 -
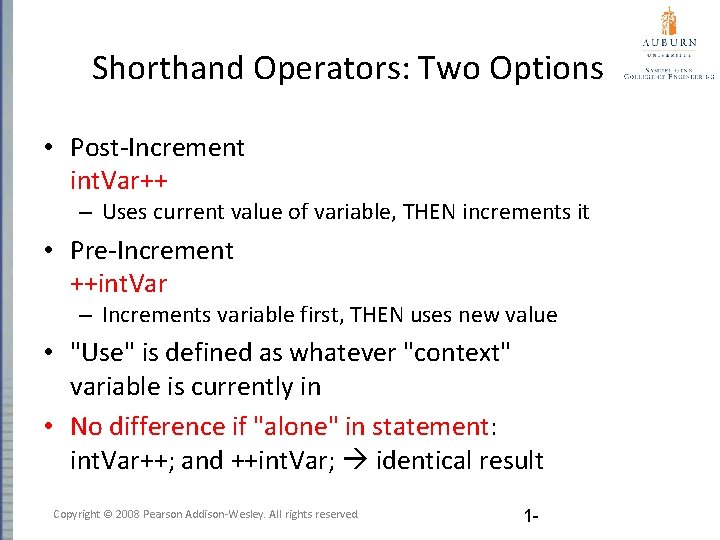
Shorthand Operators: Two Options • Post-Increment int. Var++ – Uses current value of variable, THEN increments it • Pre-Increment ++int. Var – Increments variable first, THEN uses new value • "Use" is defined as whatever "context" variable is currently in • No difference if "alone" in statement: int. Var++; and ++int. Var; identical result Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 1 -
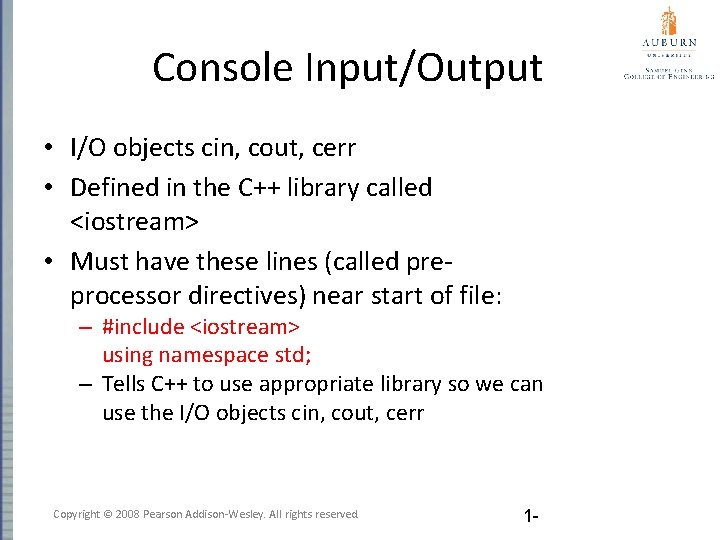
Console Input/Output • I/O objects cin, cout, cerr • Defined in the C++ library called <iostream> • Must have these lines (called preprocessor directives) near start of file: – #include <iostream> using namespace std; – Tells C++ to use appropriate library so we can use the I/O objects cin, cout, cerr Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 1 -
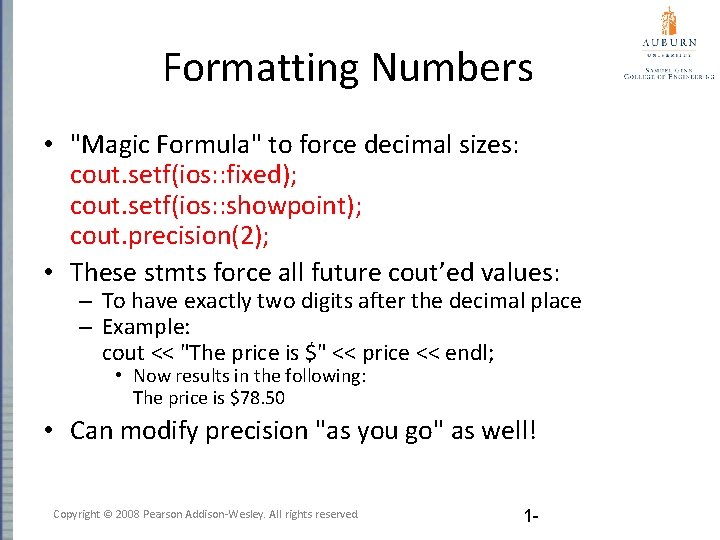
Formatting Numbers • "Magic Formula" to force decimal sizes: cout. setf(ios: : fixed); cout. setf(ios: : showpoint); cout. precision(2); • These stmts force all future cout’ed values: – To have exactly two digits after the decimal place – Example: cout << "The price is $" << price << endl; • Now results in the following: The price is $78. 50 • Can modify precision "as you go" as well! Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 1 -
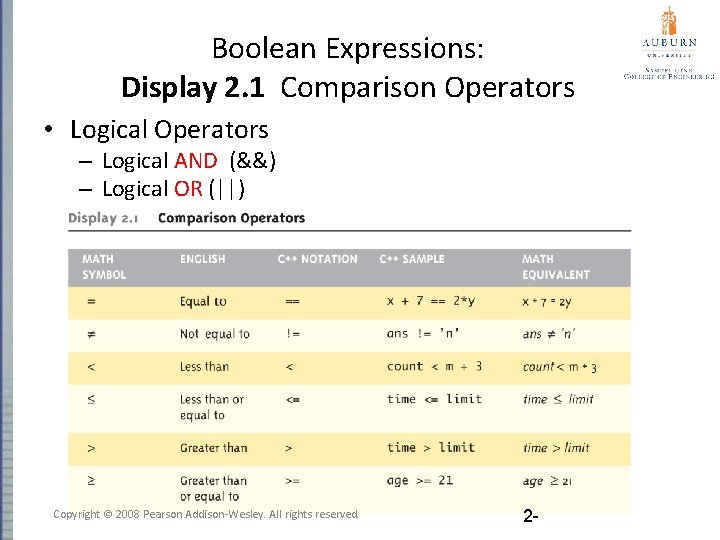
Boolean Expressions: Display 2. 1 Comparison Operators • Logical Operators – Logical AND (&&) – Logical OR (||) Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 2 -
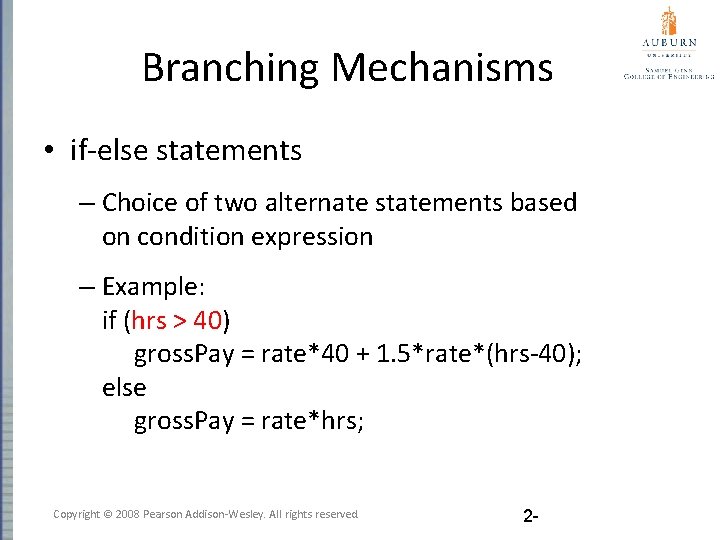
Branching Mechanisms • if-else statements – Choice of two alternate statements based on condition expression – Example: if (hrs > 40) gross. Pay = rate*40 + 1. 5*rate*(hrs-40); else gross. Pay = rate*hrs; Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 2 -
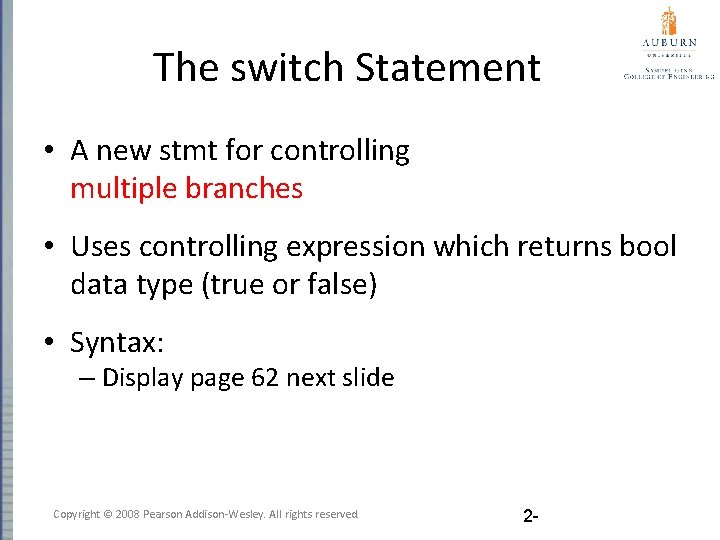
The switch Statement • A new stmt for controlling multiple branches • Uses controlling expression which returns bool data type (true or false) • Syntax: – Display page 62 next slide Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 2 -
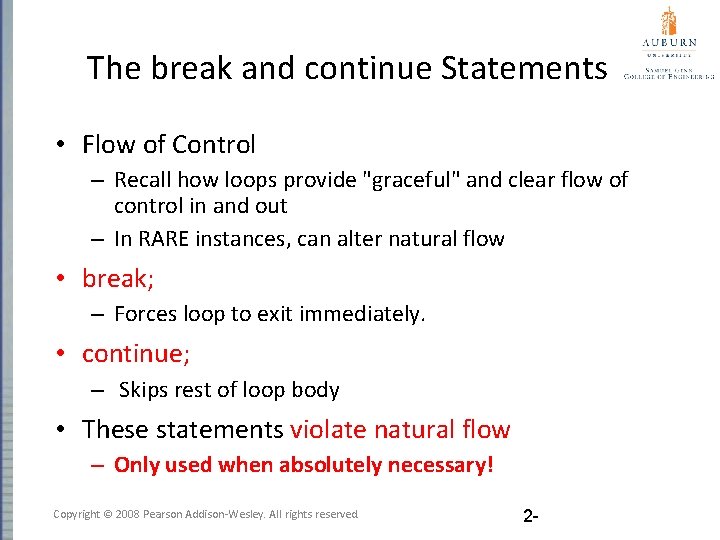
The break and continue Statements • Flow of Control – Recall how loops provide "graceful" and clear flow of control in and out – In RARE instances, can alter natural flow • break; – Forces loop to exit immediately. • continue; – Skips rest of loop body • These statements violate natural flow – Only used when absolutely necessary! Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 2 -
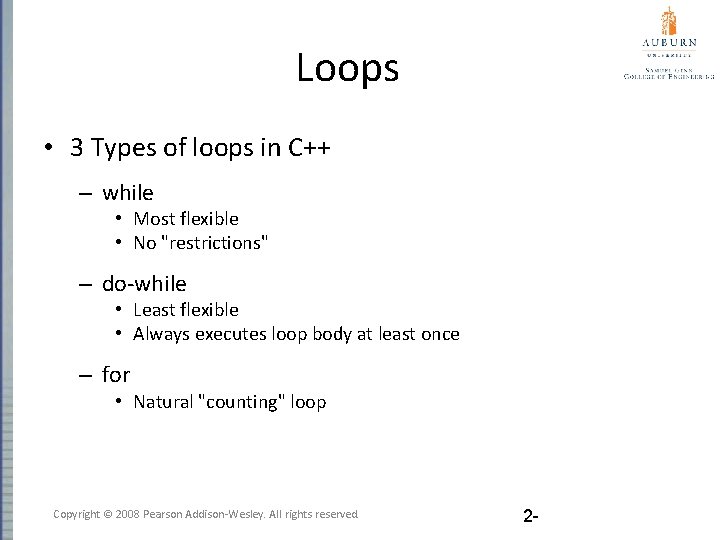
Loops • 3 Types of loops in C++ – while • Most flexible • No "restrictions" – do-while • Least flexible • Always executes loop body at least once – for • Natural "counting" loop Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 2 -
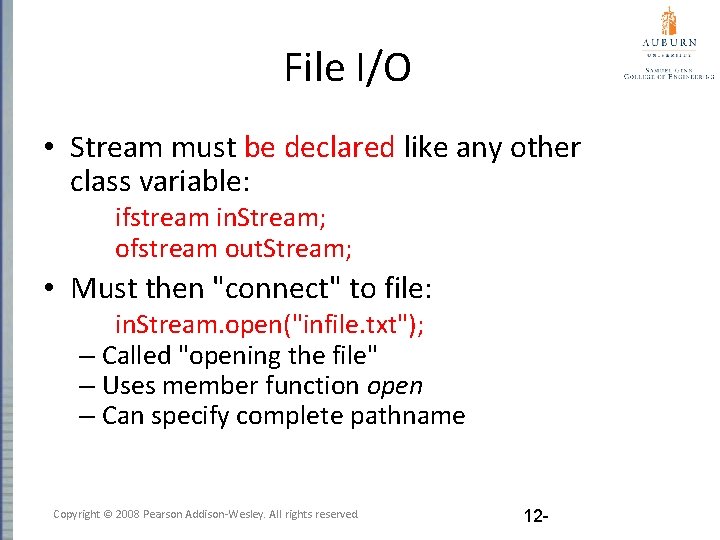
File I/O • Stream must be declared like any other class variable: ifstream in. Stream; ofstream out. Stream; • Must then "connect" to file: in. Stream. open("infile. txt"); – Called "opening the file" – Uses member function open – Can specify complete pathname Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 12 -
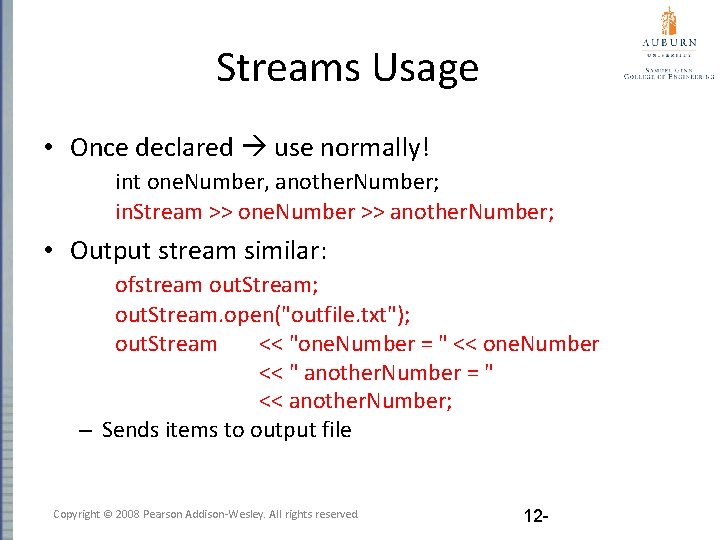
Streams Usage • Once declared use normally! int one. Number, another. Number; in. Stream >> one. Number >> another. Number; • Output stream similar: ofstream out. Stream; out. Stream. open("outfile. txt"); out. Stream << "one. Number = " << one. Number << " another. Number = " << another. Number; – Sends items to output file Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 12 -
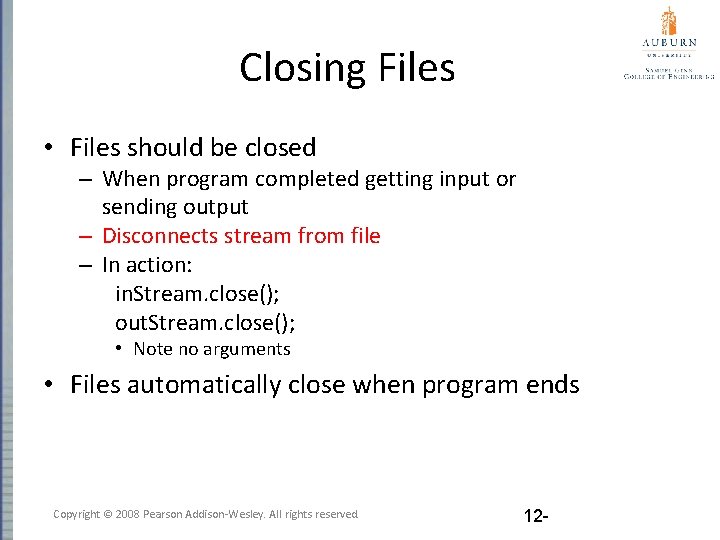
Closing Files • Files should be closed – When program completed getting input or sending output – Disconnects stream from file – In action: in. Stream. close(); out. Stream. close(); • Note no arguments • Files automatically close when program ends Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 12 -
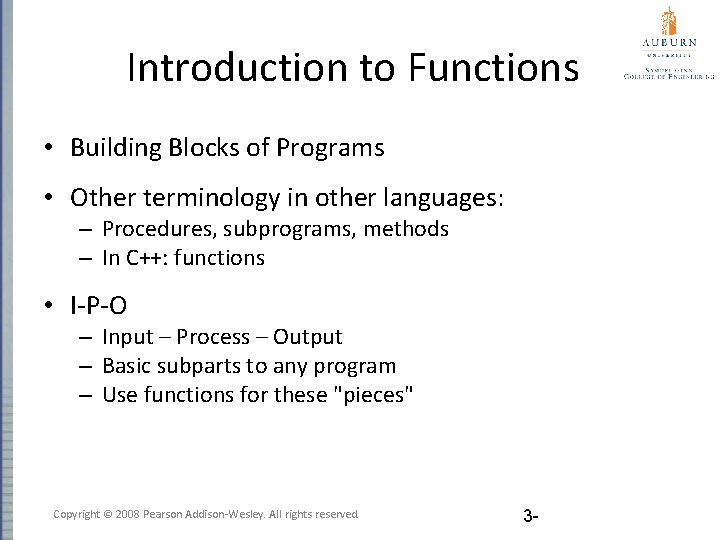
Introduction to Functions • Building Blocks of Programs • Other terminology in other languages: – Procedures, subprograms, methods – In C++: functions • I-P-O – Input – Process – Output – Basic subparts to any program – Use functions for these "pieces" Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 3 -
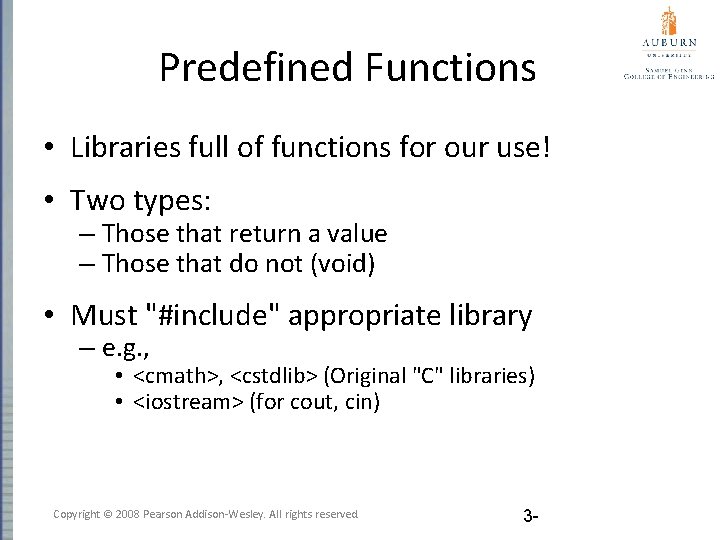
Predefined Functions • Libraries full of functions for our use! • Two types: – Those that return a value – Those that do not (void) • Must "#include" appropriate library – e. g. , • <cmath>, <cstdlib> (Original "C" libraries) • <iostream> (for cout, cin) Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 3 -
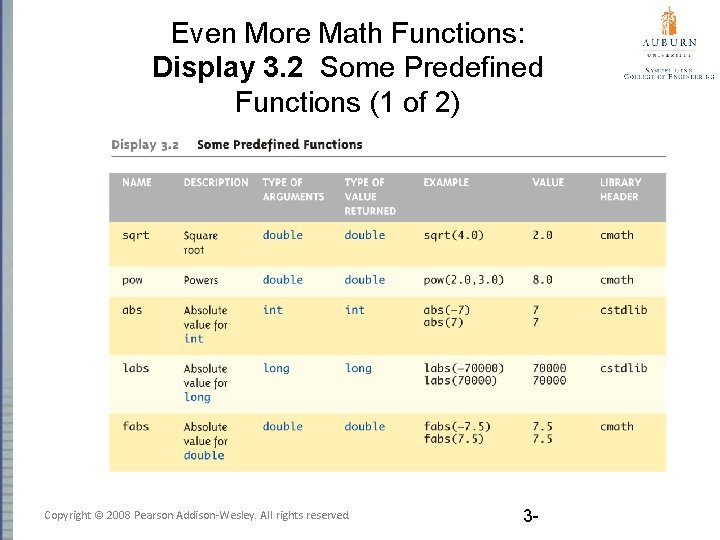
Even More Math Functions: Display 3. 2 Some Predefined Functions (1 of 2) Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 3 -
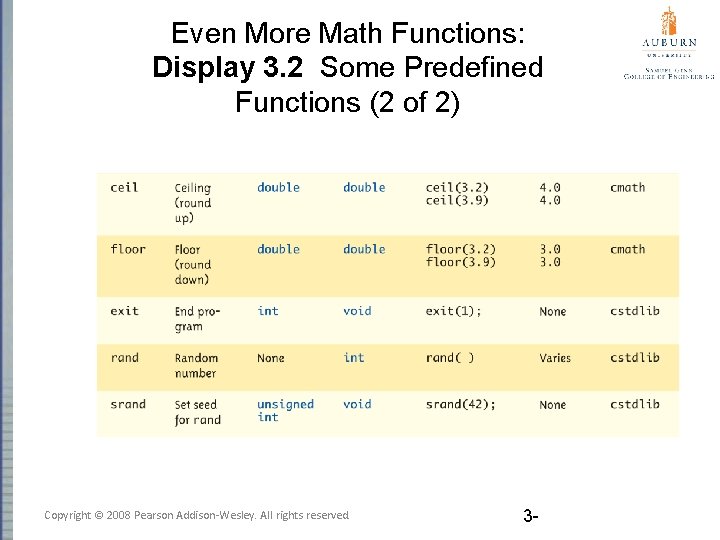
Even More Math Functions: Display 3. 2 Some Predefined Functions (2 of 2) Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 3 -
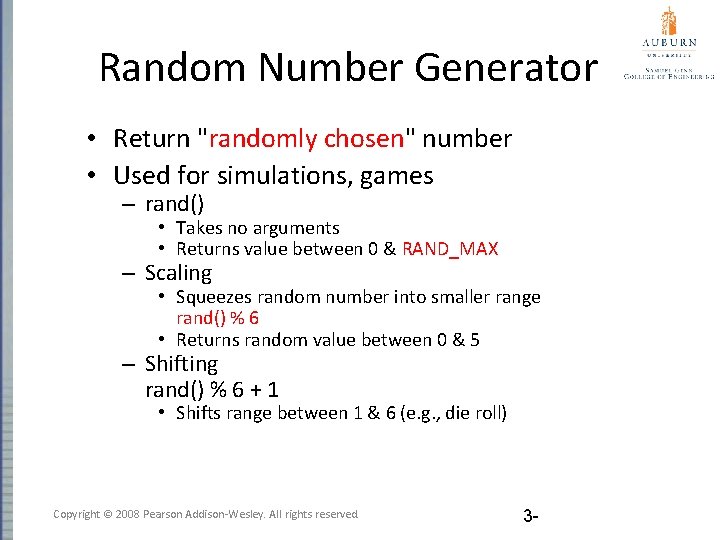
Random Number Generator • Return "randomly chosen" number • Used for simulations, games – rand() • Takes no arguments • Returns value between 0 & RAND_MAX – Scaling • Squeezes random number into smaller range rand() % 6 • Returns random value between 0 & 5 – Shifting rand() % 6 + 1 • Shifts range between 1 & 6 (e. g. , die roll) Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 3 -
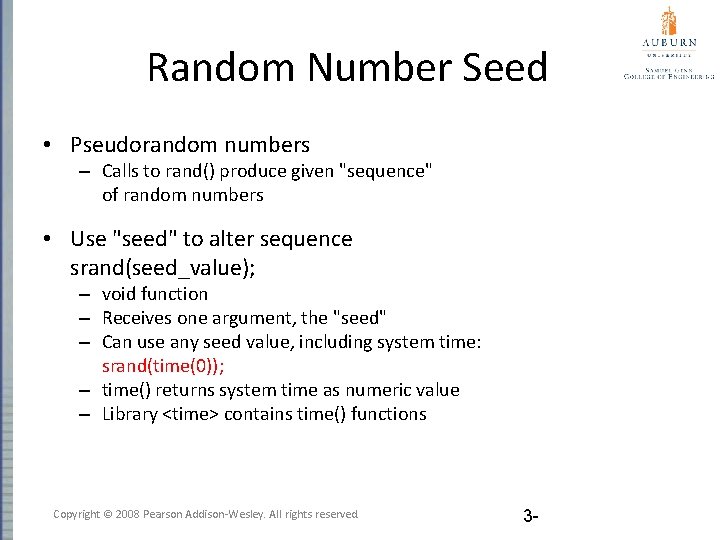
Random Number Seed • Pseudorandom numbers – Calls to rand() produce given "sequence" of random numbers • Use "seed" to alter sequence srand(seed_value); – void function – Receives one argument, the "seed" – Can use any seed value, including system time: srand(time(0)); – time() returns system time as numeric value – Library <time> contains time() functions Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 3 -
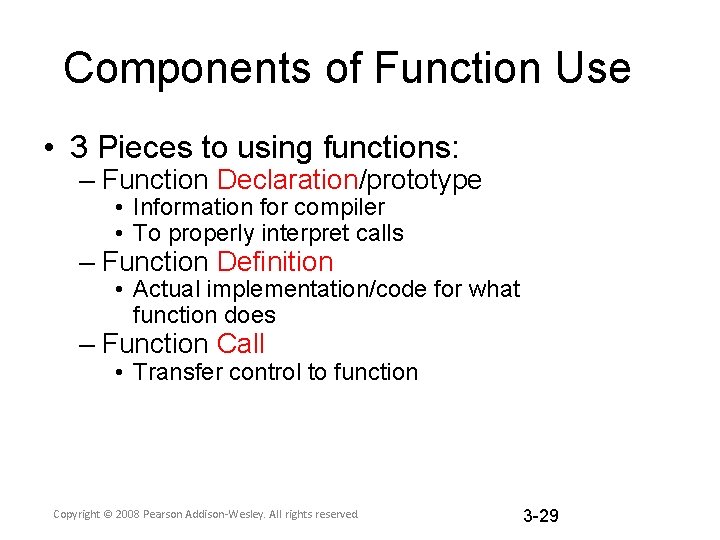
Components of Function Use • 3 Pieces to using functions: – Function Declaration/prototype • Information for compiler • To properly interpret calls – Function Definition • Actual implementation/code for what function does – Function Call • Transfer control to function Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 3 -29
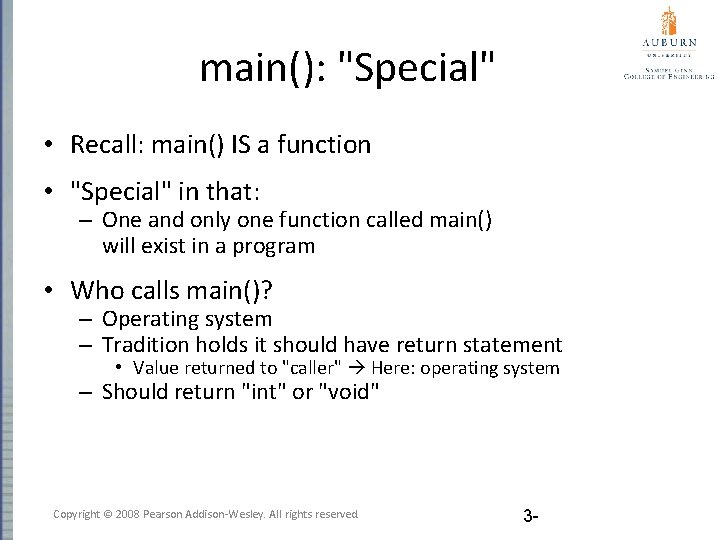
main(): "Special" • Recall: main() IS a function • "Special" in that: – One and only one function called main() will exist in a program • Who calls main()? – Operating system – Tradition holds it should have return statement • Value returned to "caller" Here: operating system – Should return "int" or "void" Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 3 -
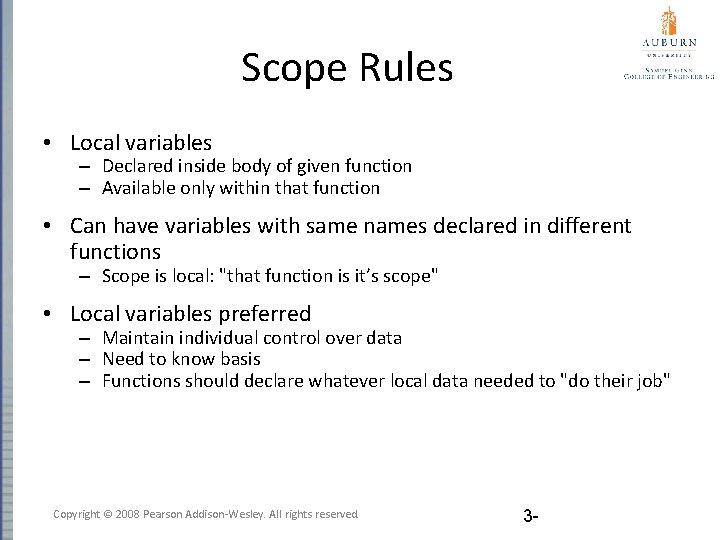
Scope Rules • Local variables – Declared inside body of given function – Available only within that function • Can have variables with same names declared in different functions – Scope is local: "that function is it’s scope" • Local variables preferred – Maintain individual control over data – Need to know basis – Functions should declare whatever local data needed to "do their job" Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 3 -
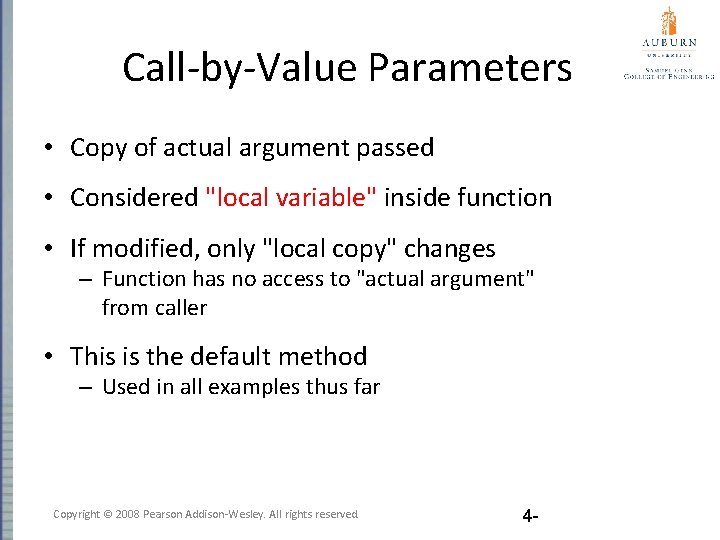
Call-by-Value Parameters • Copy of actual argument passed • Considered "local variable" inside function • If modified, only "local copy" changes – Function has no access to "actual argument" from caller • This is the default method – Used in all examples thus far Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 4 -
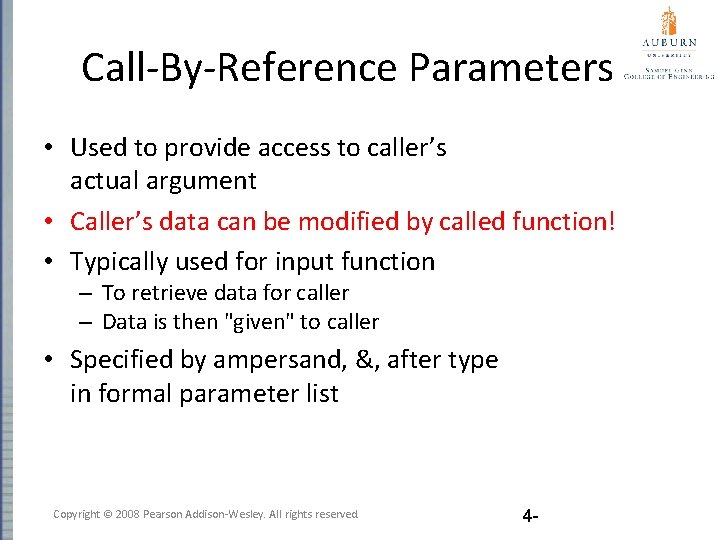
Call-By-Reference Parameters • Used to provide access to caller’s actual argument • Caller’s data can be modified by called function! • Typically used for input function – To retrieve data for caller – Data is then "given" to caller • Specified by ampersand, &, after type in formal parameter list Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 4 -
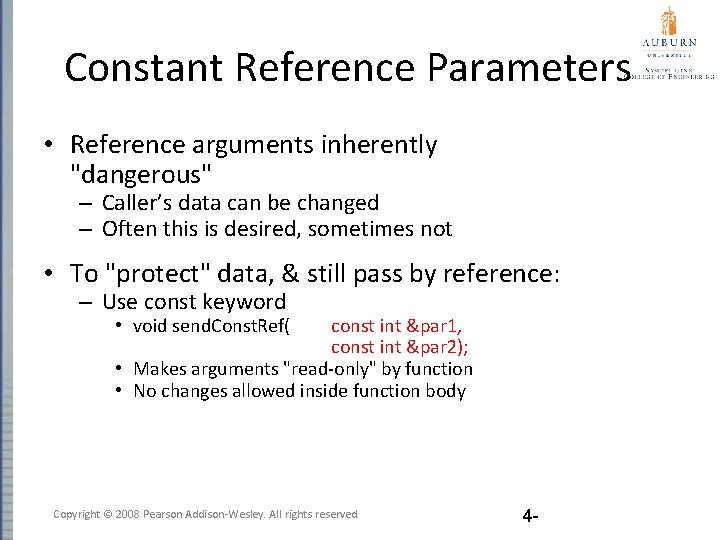
Constant Reference Parameters • Reference arguments inherently "dangerous" – Caller’s data can be changed – Often this is desired, sometimes not • To "protect" data, & still pass by reference: – Use const keyword • void send. Const. Ref( const int &par 1, const int &par 2); • Makes arguments "read-only" by function • No changes allowed inside function body Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 4 -
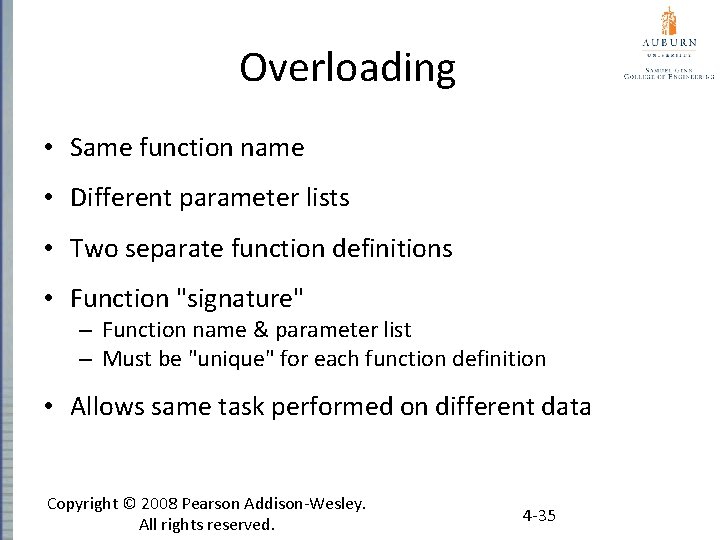
Overloading • Same function name • Different parameter lists • Two separate function definitions • Function "signature" – Function name & parameter list – Must be "unique" for each function definition • Allows same task performed on different data Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 4 -35
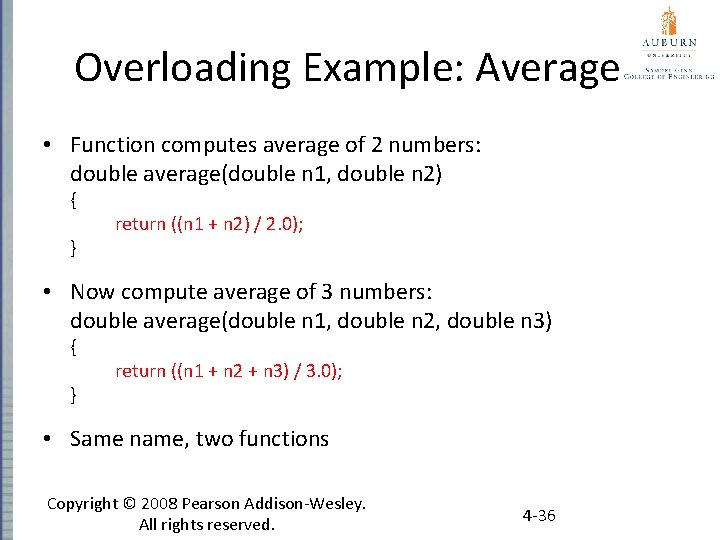
Overloading Example: Average • Function computes average of 2 numbers: double average(double n 1, double n 2) { } return ((n 1 + n 2) / 2. 0); • Now compute average of 3 numbers: double average(double n 1, double n 2, double n 3) { } return ((n 1 + n 2 + n 3) / 3. 0); • Same name, two functions Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 4 -36
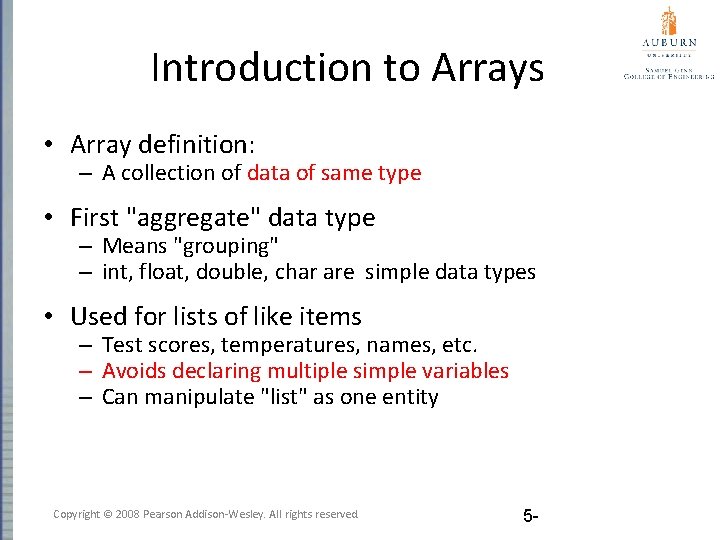
Introduction to Arrays • Array definition: – A collection of data of same type • First "aggregate" data type – Means "grouping" – int, float, double, char are simple data types • Used for lists of like items – Test scores, temperatures, names, etc. – Avoids declaring multiple simple variables – Can manipulate "list" as one entity Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 5 -
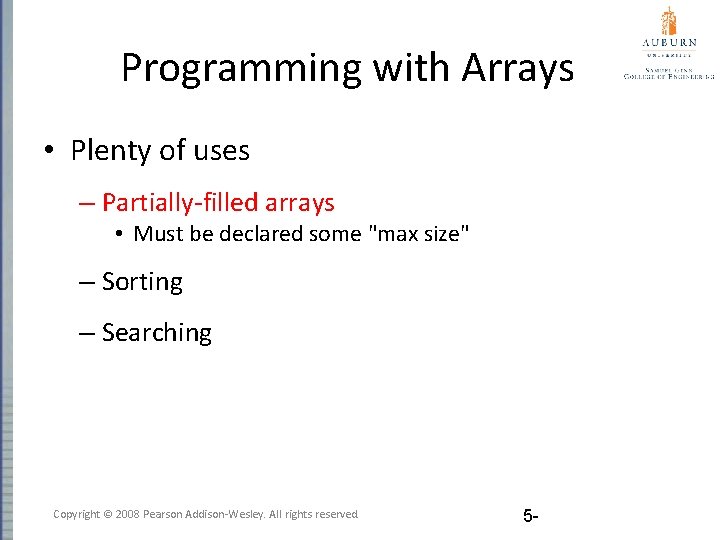
Programming with Arrays • Plenty of uses – Partially-filled arrays • Must be declared some "max size" – Sorting – Searching Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 5 -
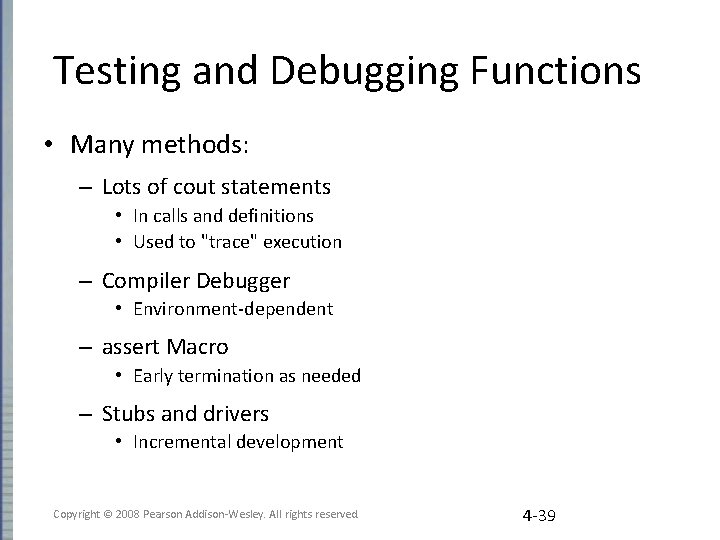
Testing and Debugging Functions • Many methods: – Lots of cout statements • In calls and definitions • Used to "trace" execution – Compiler Debugger • Environment-dependent – assert Macro • Early termination as needed – Stubs and drivers • Incremental development Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 4 -39
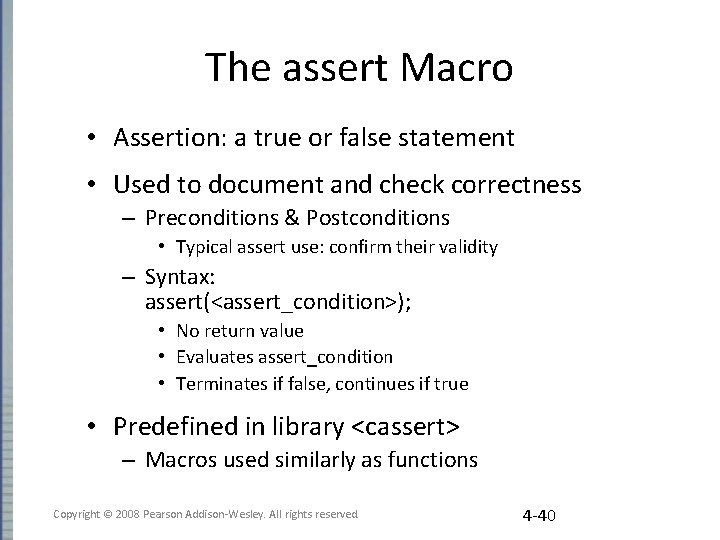
The assert Macro • Assertion: a true or false statement • Used to document and check correctness – Preconditions & Postconditions • Typical assert use: confirm their validity – Syntax: assert(<assert_condition>); • No return value • Evaluates assert_condition • Terminates if false, continues if true • Predefined in library <cassert> – Macros used similarly as functions Copyright © 2008 Pearson Addison-Wesley. All rights reserved. 4 -40
World history semester exam
Geometry midterm exam review
Algebra 2 midterm practice test
2710 19 43
2's complement
2710 gtip
Comp 248 midterm
Comp 248 midterm
Nlp midterm exam
Data mining exam
Features of html
English 1 midterm exam answers
Business law midterm exam
Research methodology midterm exam
English 9 midterm exam
Introduction to computer science midterm exam
Introduction to computer science midterm exam test
Ap english language and composition midterm exam
Grva midterm exam
Midterm exam format
American literature midterm exam
English 11 midterm exam
Database systems midterm exam
Apes midterm exam
What is a midterm
Exam midterm
Ap chemistry midterm
Algebra 1 midterm exam
Midterm exam announcement
Homework app
Operating system midterm exam
Xiao comp
Ap gov final review
Mnemonic for reticular formation
Whap midterm review
When backed by buying power wants become
Global 9 midterm review
Trig midterm review
Chemistry midterm review
Spanish 2 midterm review
Biology midterm review