COMP 2710 Software Construction Homework 2 Design and
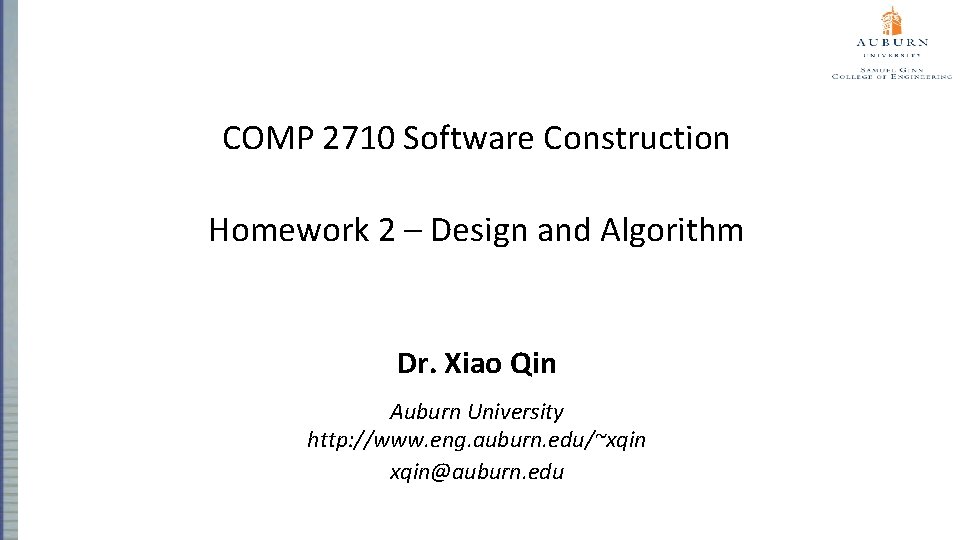
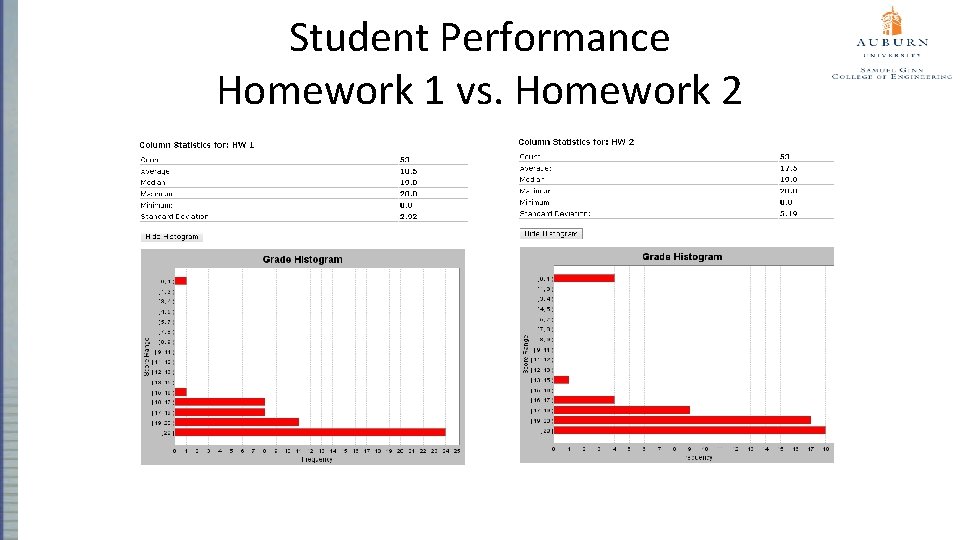
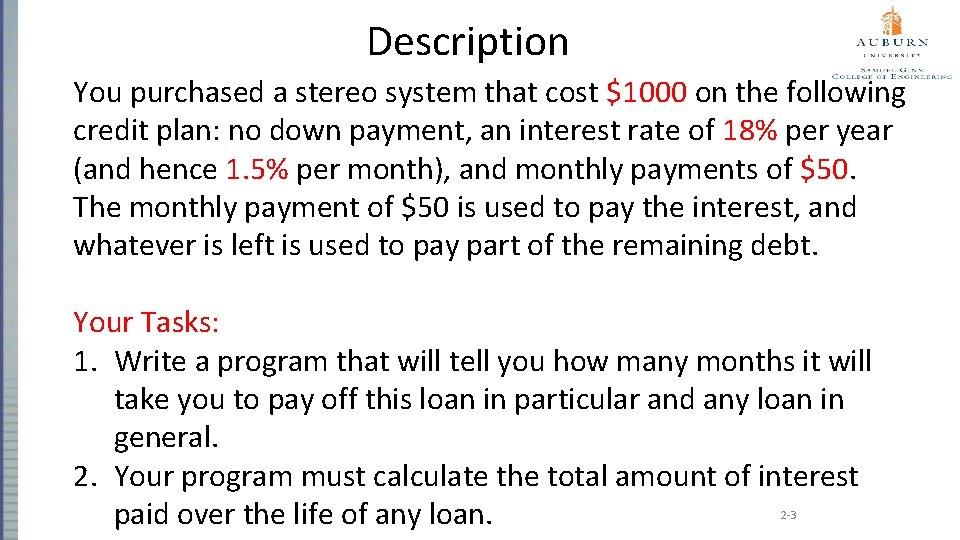
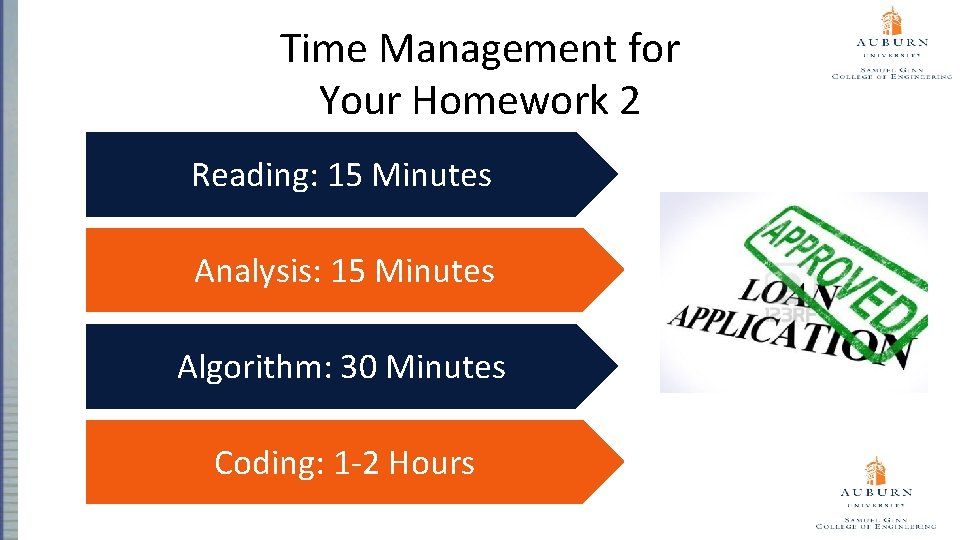
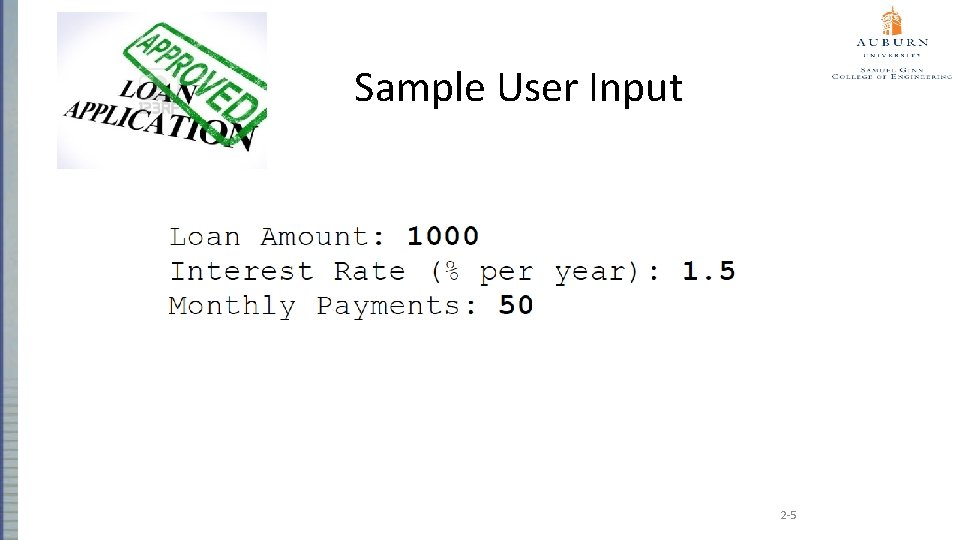
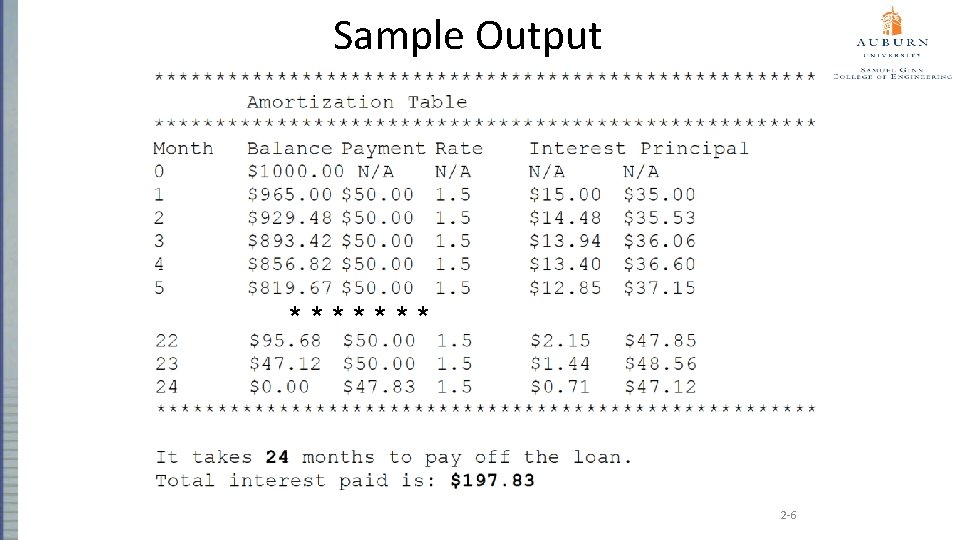
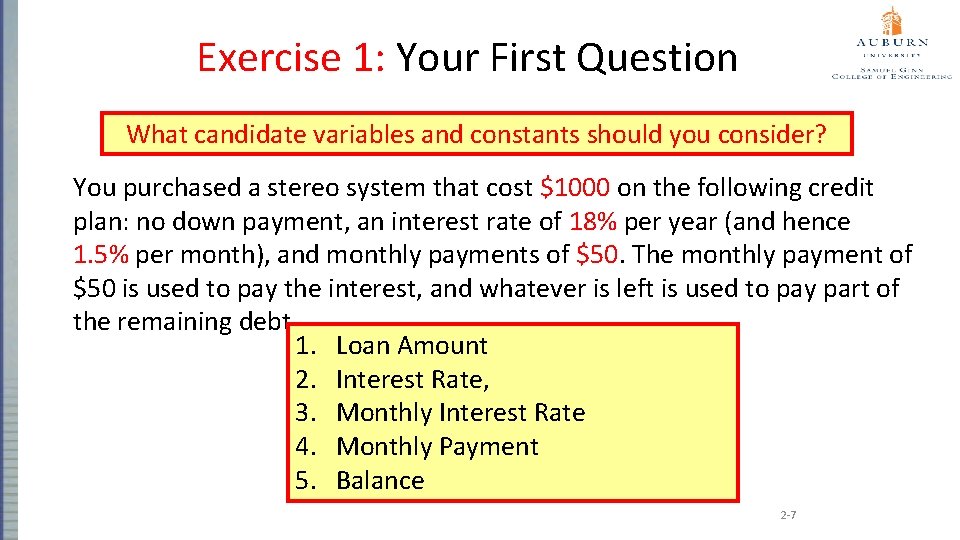
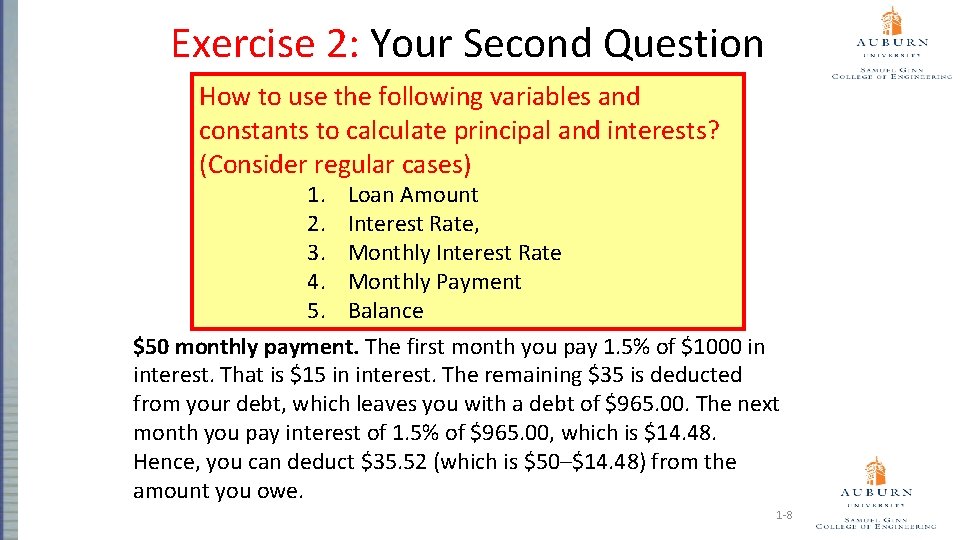
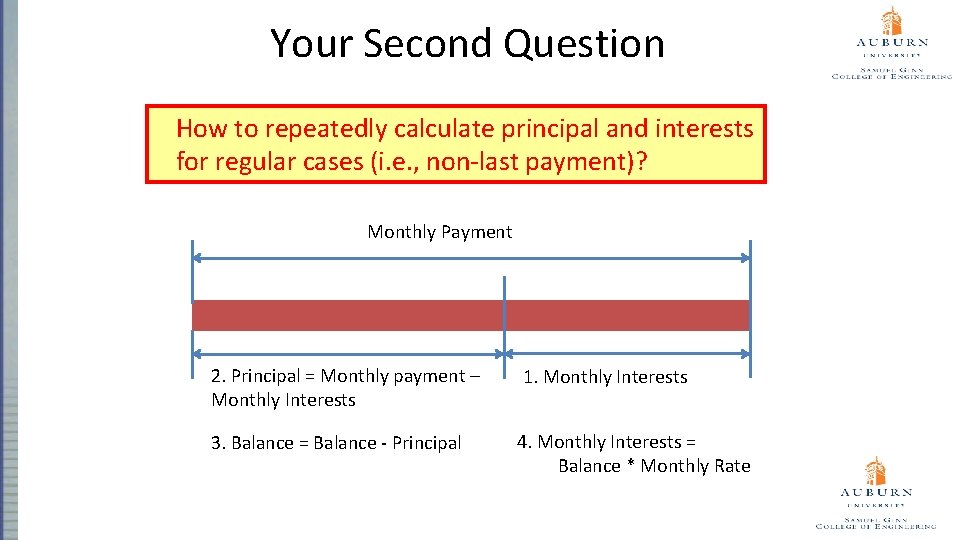
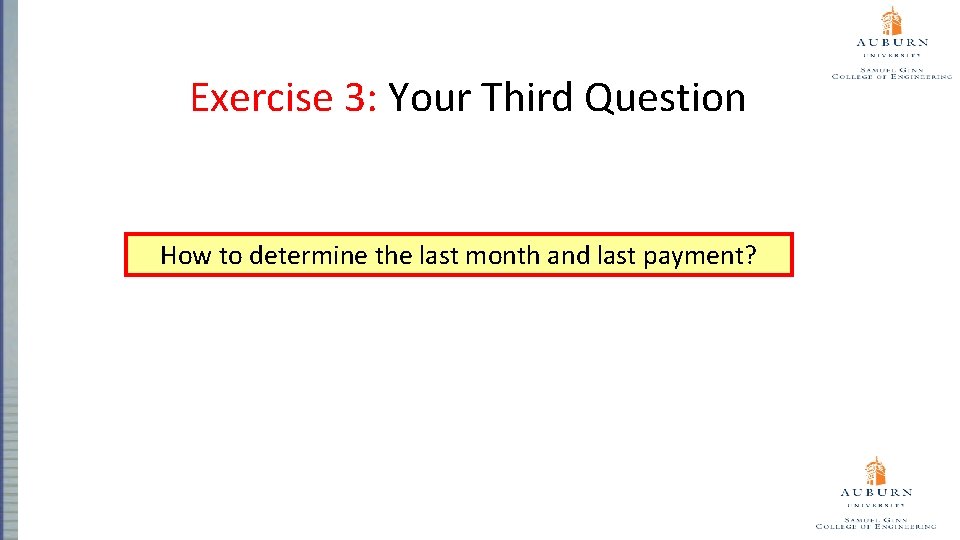
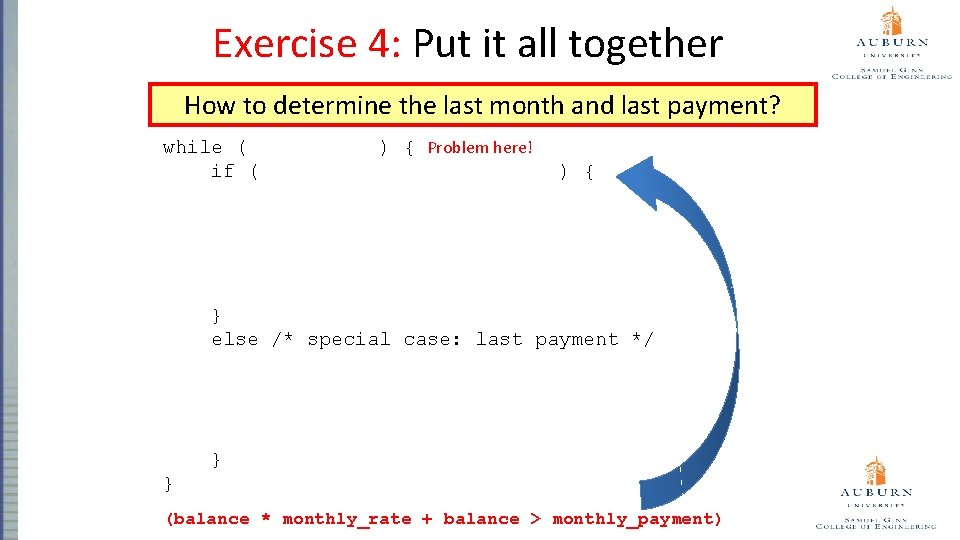
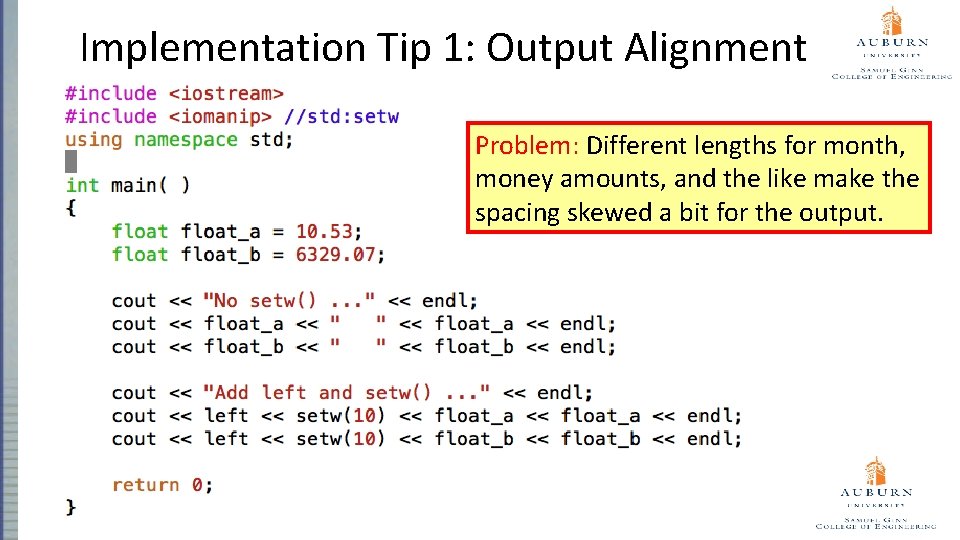
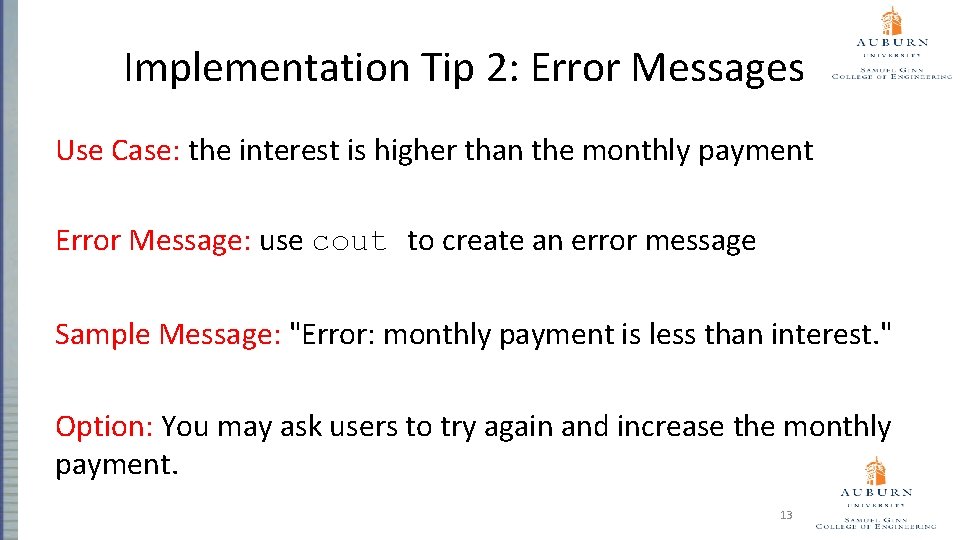
- Slides: 13
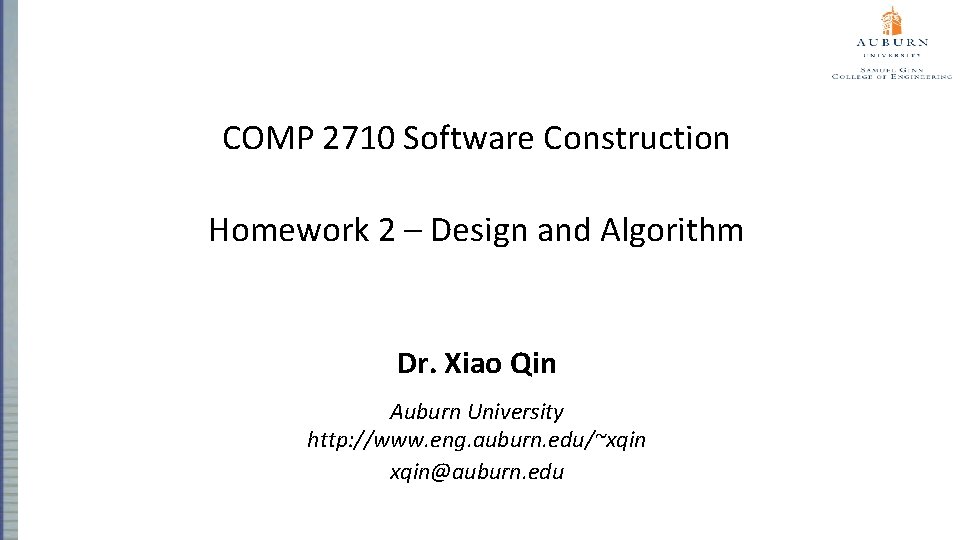
COMP 2710 Software Construction Homework 2 – Design and Algorithm Dr. Xiao Qin Auburn University http: //www. eng. auburn. edu/~xqin@auburn. edu
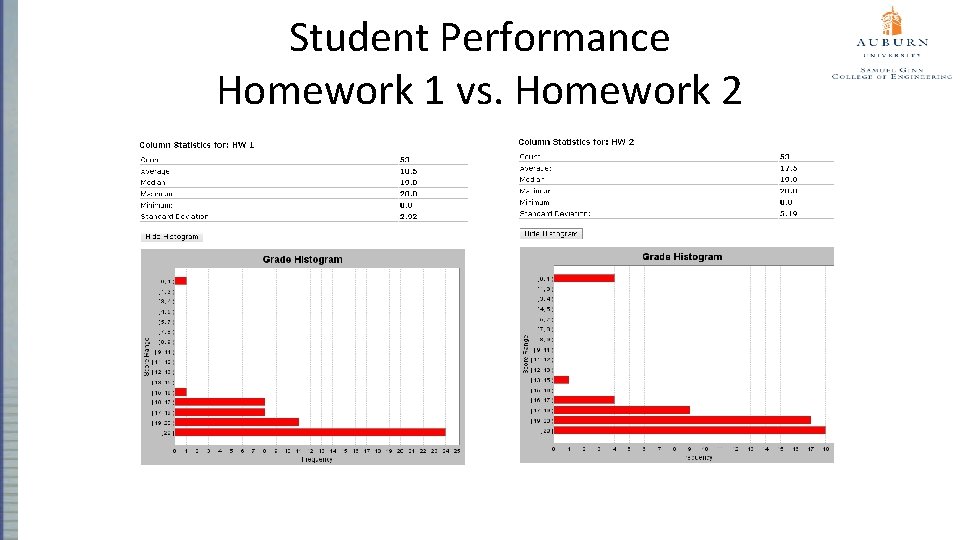
Student Performance Homework 1 vs. Homework 2
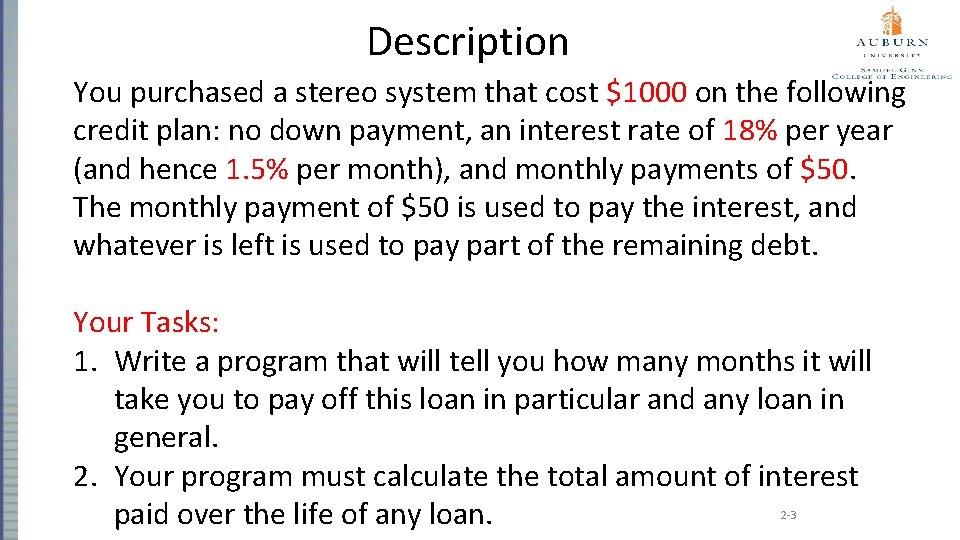
Description You purchased a stereo system that cost $1000 on the following credit plan: no down payment, an interest rate of 18% per year (and hence 1. 5% per month), and monthly payments of $50. The monthly payment of $50 is used to pay the interest, and whatever is left is used to pay part of the remaining debt. Your Tasks: 1. Write a program that will tell you how many months it will take you to pay off this loan in particular and any loan in general. 2. Your program must calculate the total amount of interest paid over the life of any loan. 2 -3
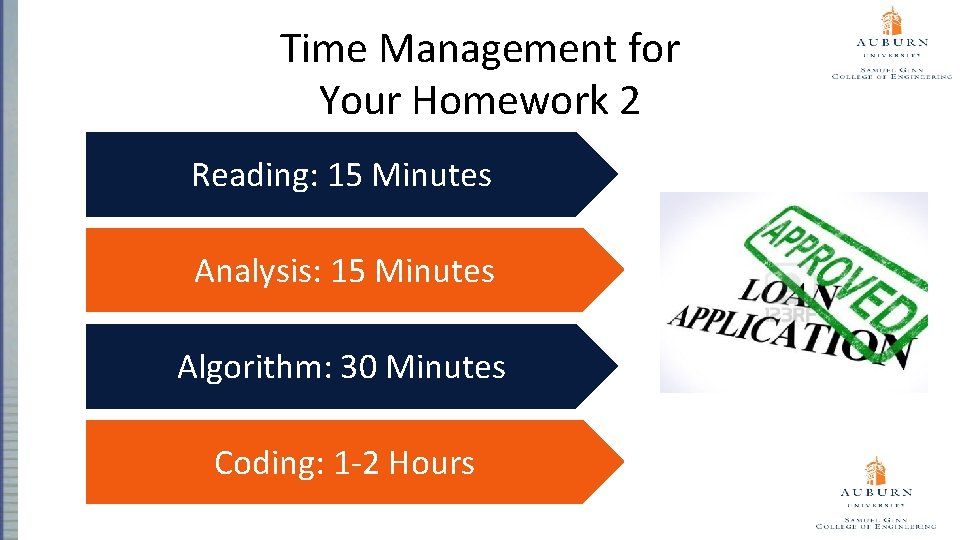
Time Management for Your Homework 2 Reading: 15 Minutes Analysis: 15 Minutes Algorithm: 30 Minutes Coding: 1 -2 Hours
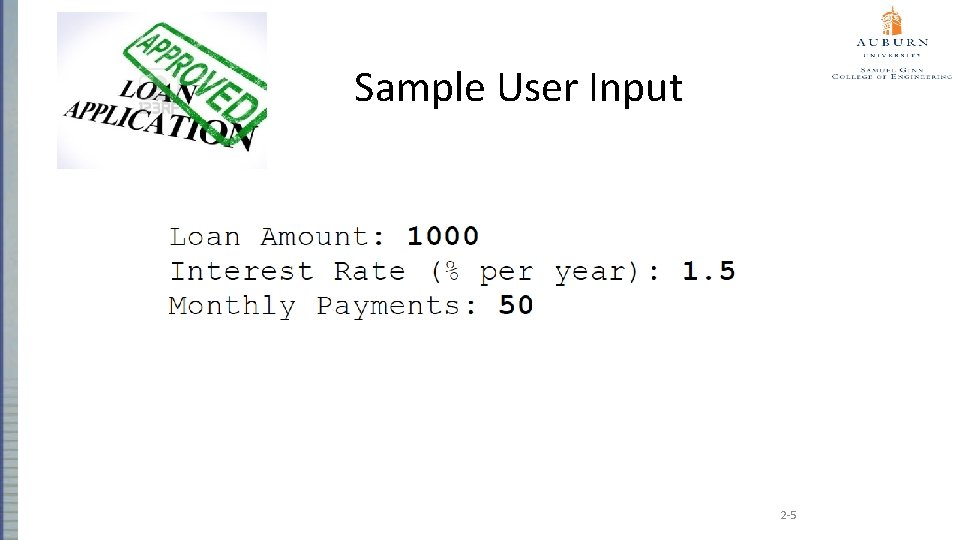
Sample User Input 2 -5
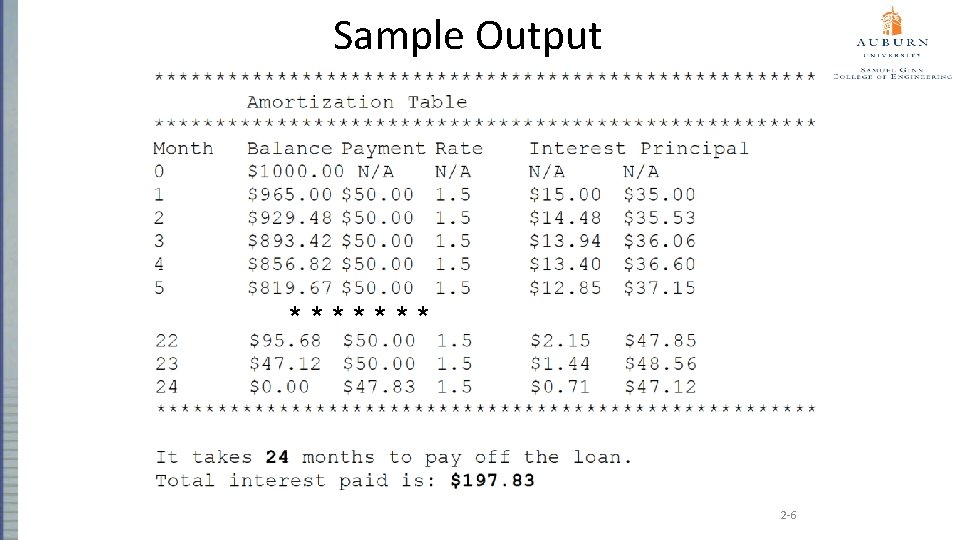
Sample Output ******* 2 -6
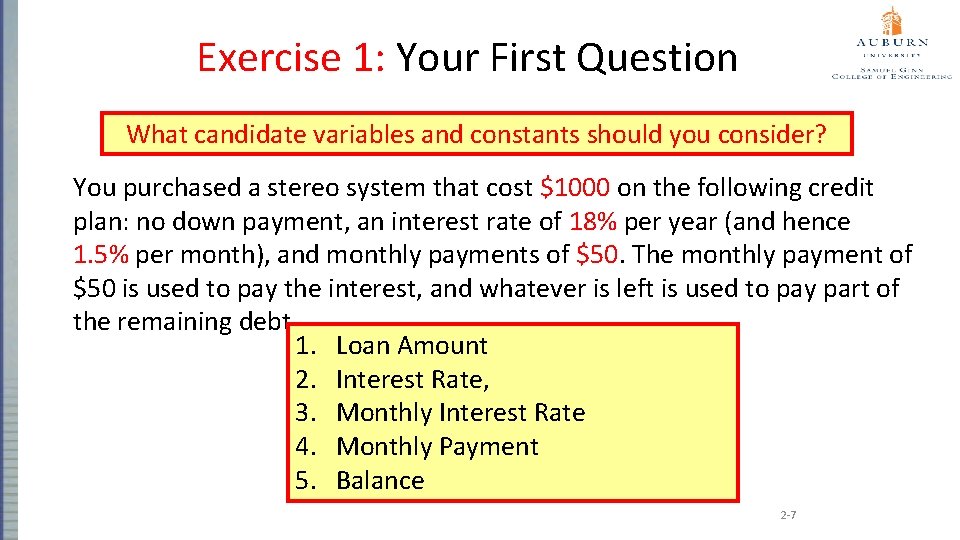
Exercise 1: Your First Question What candidate variables and constants should you consider? You purchased a stereo system that cost $1000 on the following credit plan: no down payment, an interest rate of 18% per year (and hence 1. 5% per month), and monthly payments of $50. The monthly payment of $50 is used to pay the interest, and whatever is left is used to pay part of the remaining debt. 1. Loan Amount 2. Interest Rate, 3. Monthly Interest Rate 4. Monthly Payment 5. Balance 2 -7
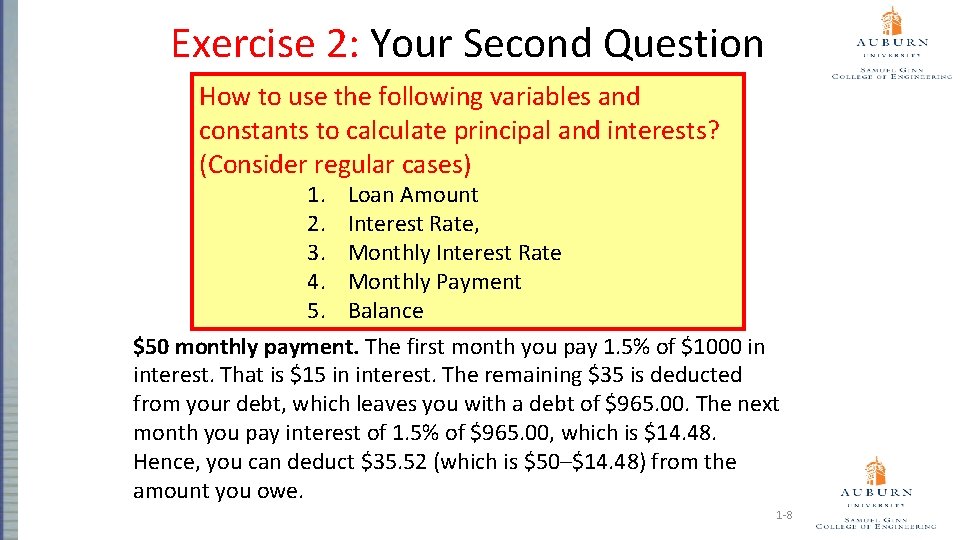
Exercise 2: Your Second Question How to use the following variables and constants to calculate principal and interests? (Consider regular cases) 1. Loan Amount 2. Interest Rate, 3. Monthly Interest Rate 4. Monthly Payment 5. Balance $50 monthly payment. The first month you pay 1. 5% of $1000 in interest. That is $15 in interest. The remaining $35 is deducted from your debt, which leaves you with a debt of $965. 00. The next month you pay interest of 1. 5% of $965. 00, which is $14. 48. Hence, you can deduct $35. 52 (which is $50–$14. 48) from the amount you owe. 1 -8
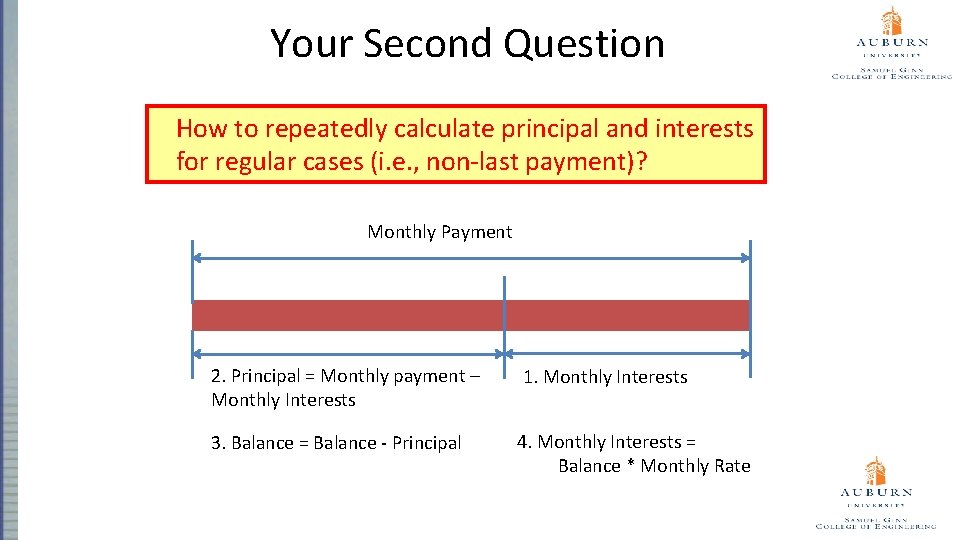
Your Second Question How to repeatedly calculate principal and interests for regular cases (i. e. , non-last payment)? Monthly Payment 2. Principal = Monthly payment – Monthly Interests 1. Monthly Interests 3. Balance = Balance - Principal 4. Monthly Interests = Balance * Monthly Rate
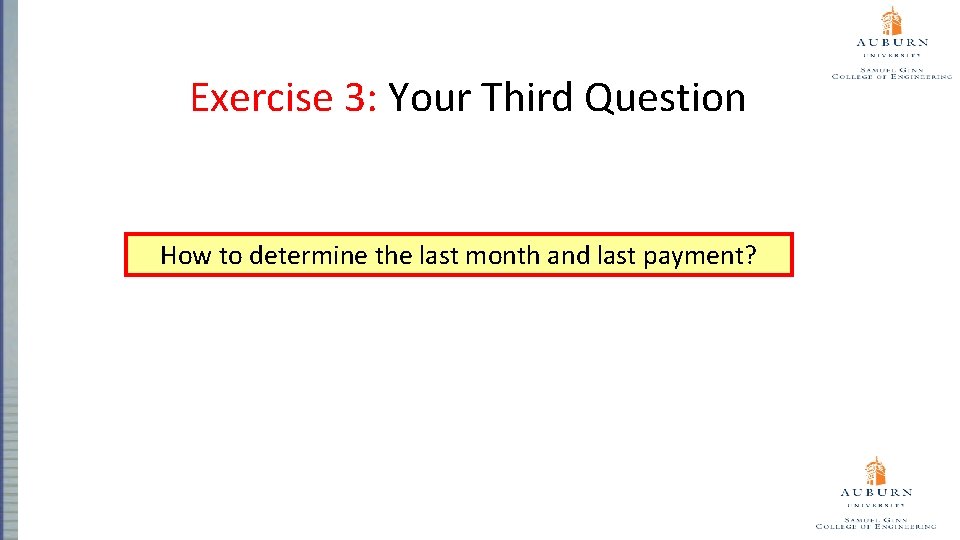
Exercise 3: Your Third Question How to determine the last month and last payment?
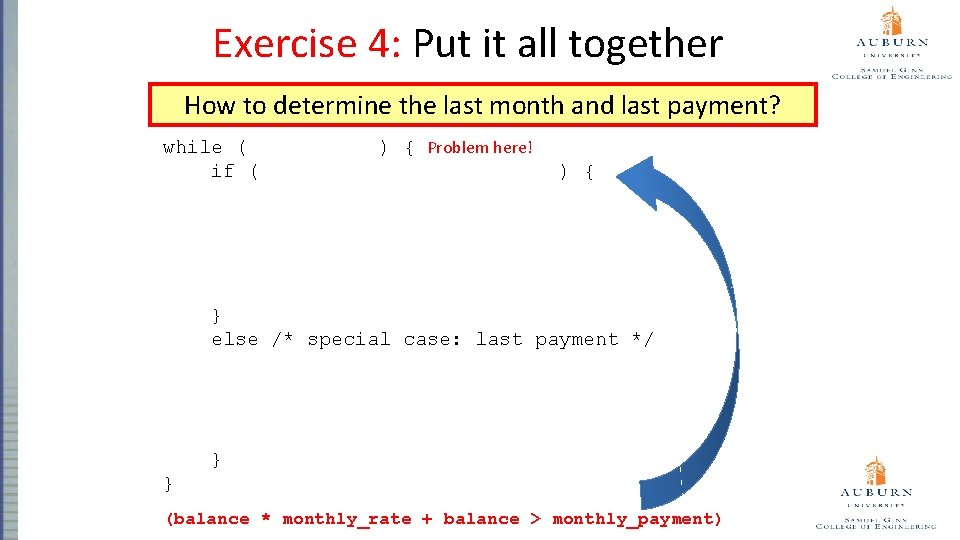
Exercise 4: Put it all together How to determine the last month and last payment? while (balance > 0) { Problem here! if (balance > monthly_payment) { /* regular case */ monthly_interest = _______; total_interest = _______; principal = _______; balance = ____; } else /* special case: last payment */ monthly_interest = _______; total_interest = _______; last_payment = monthly_interest + balance; balance = 0; } } (balance * monthly_rate + balance > monthly_payment)
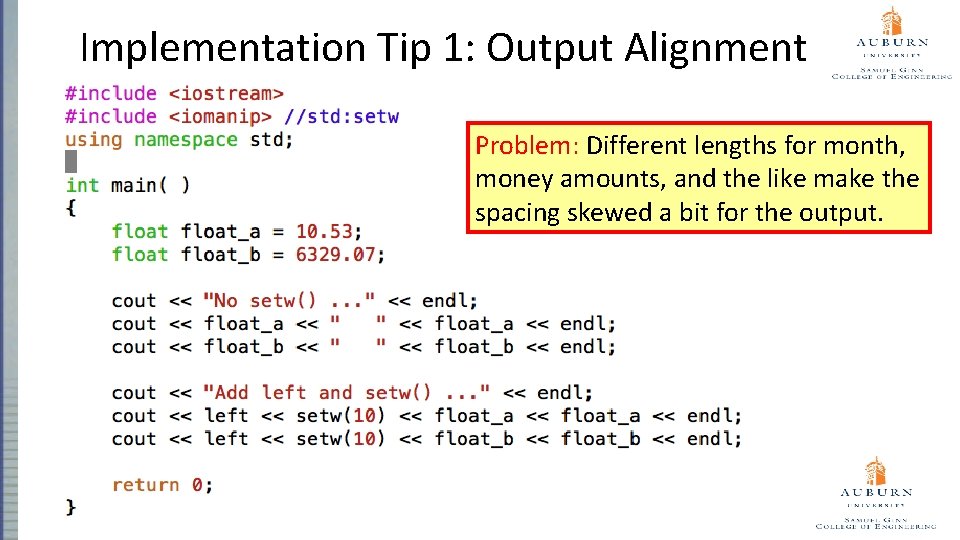
Implementation Tip 1: Output Alignment Problem: Different lengths for month, money amounts, and the like make the spacing skewed a bit for the output.
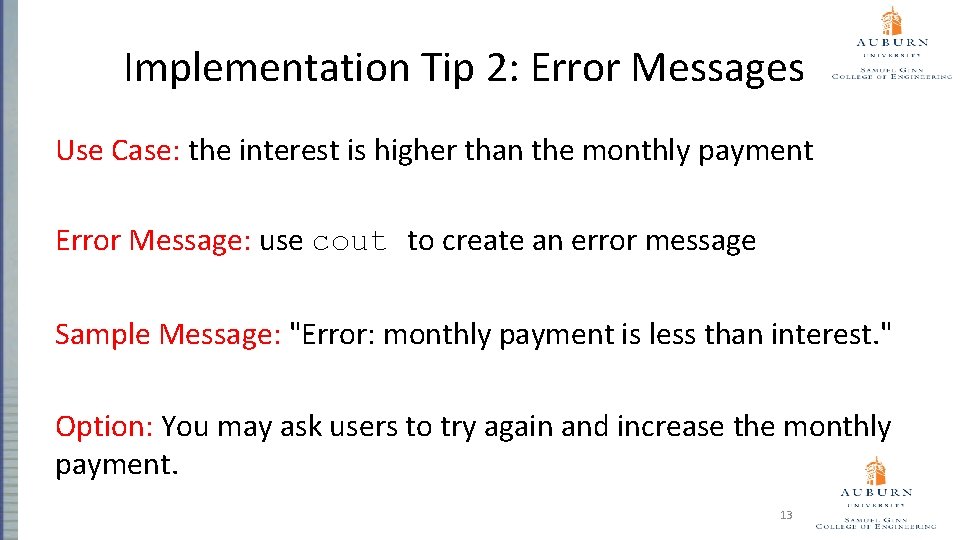
Implementation Tip 2: Error Messages Use Case: the interest is higher than the monthly payment Error Message: use cout to create an error message Sample Message: "Error: monthly payment is less than interest. " Option: You may ask users to try again and increase the monthly payment. 13