Chapter 5 Writing Classes Content Identifying classes and
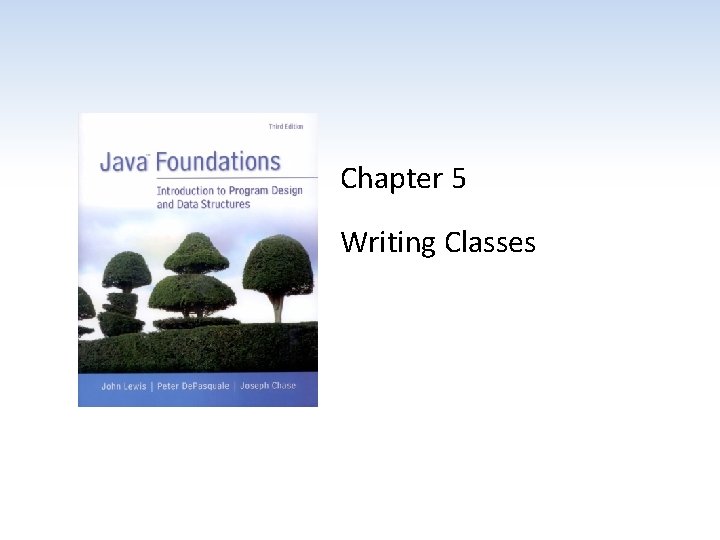
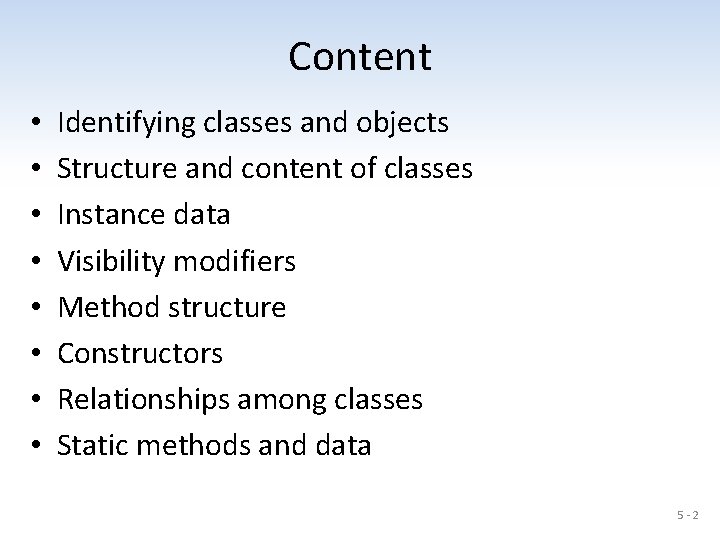
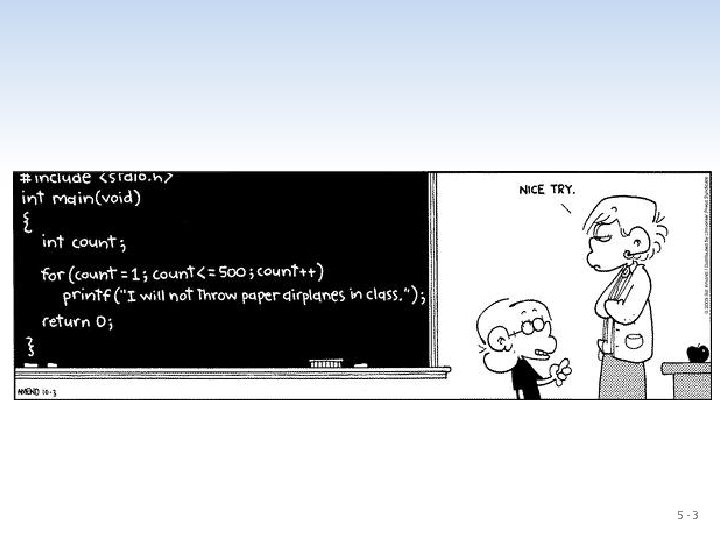
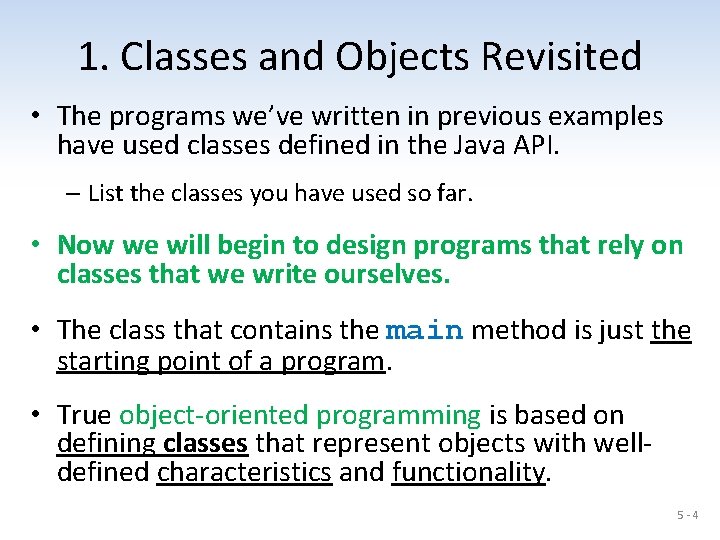
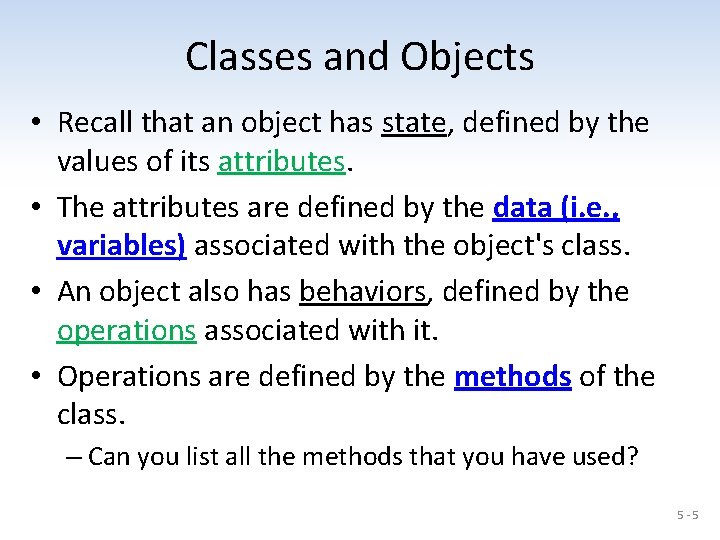
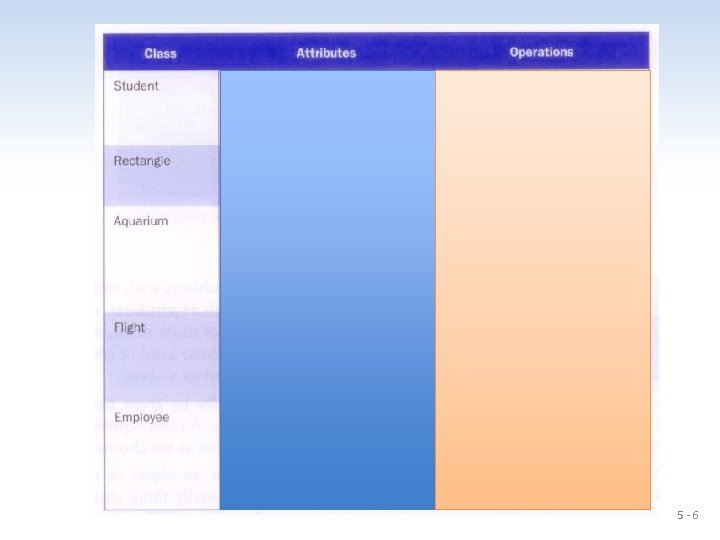
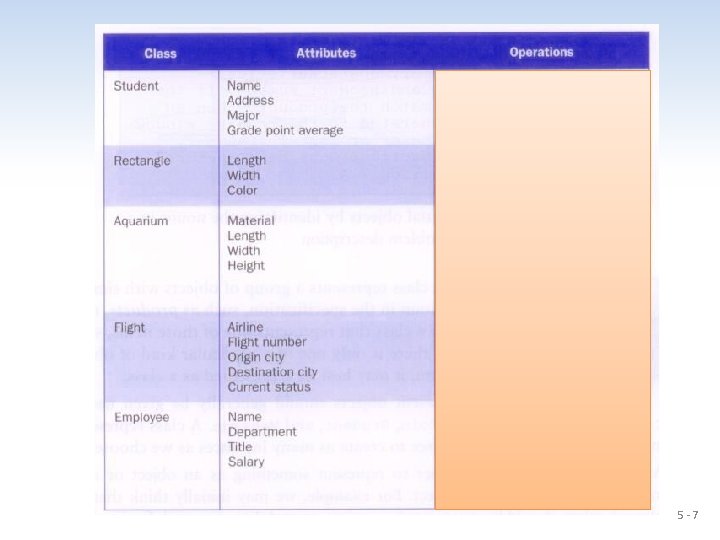
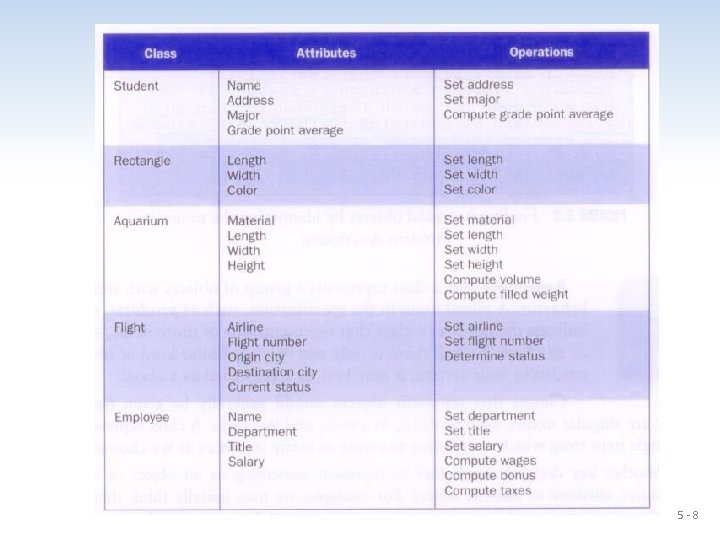
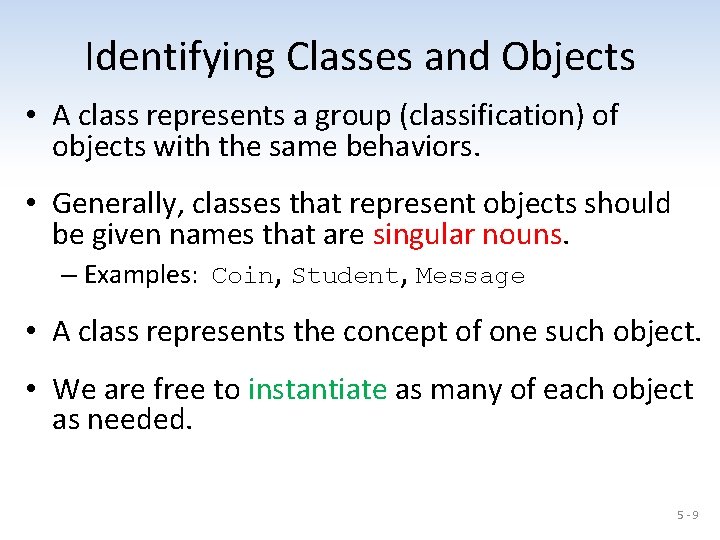
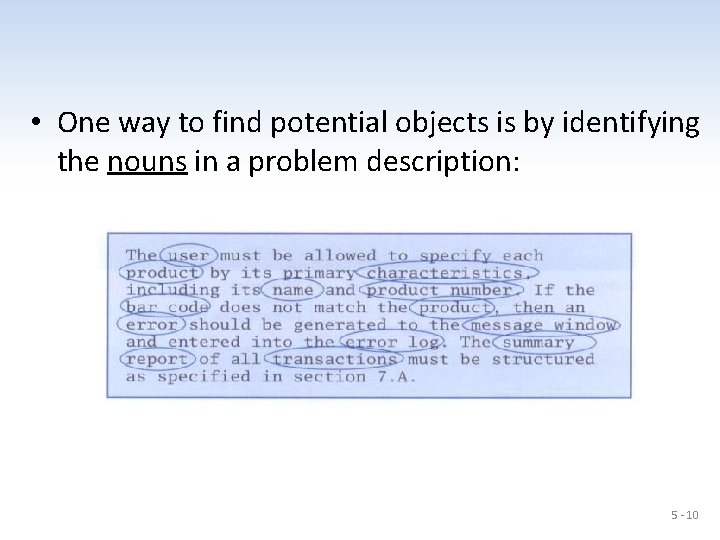
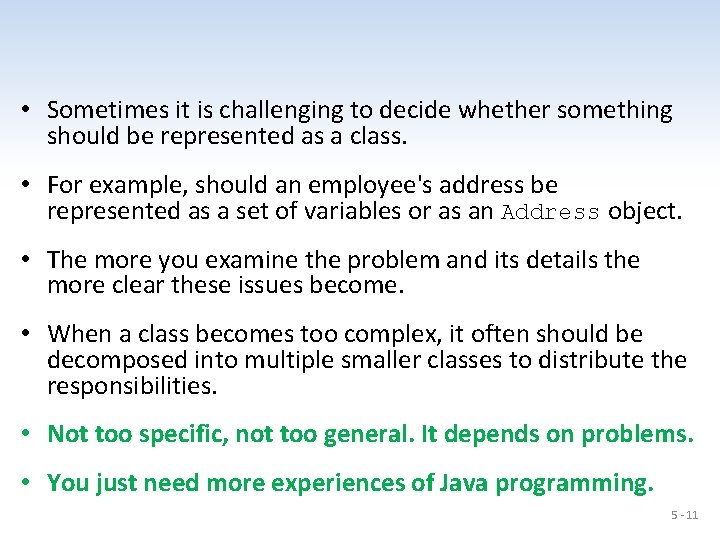
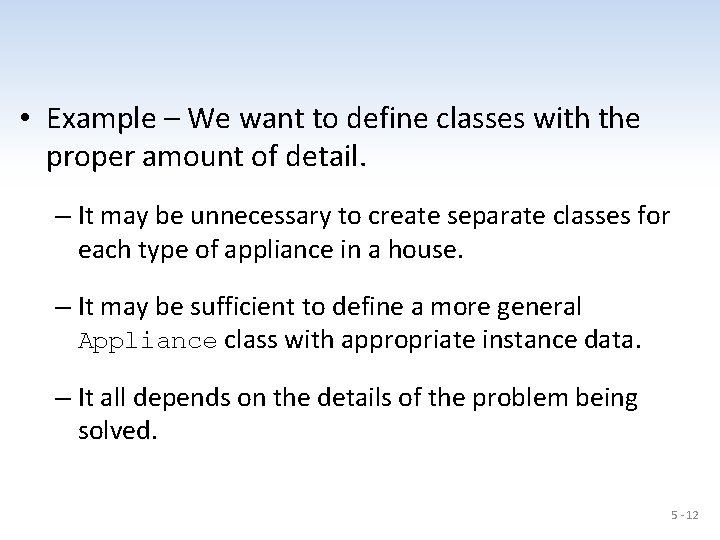
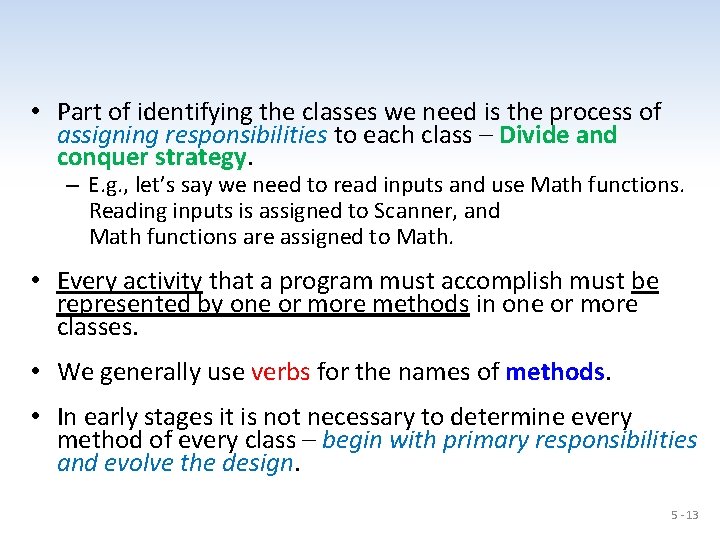
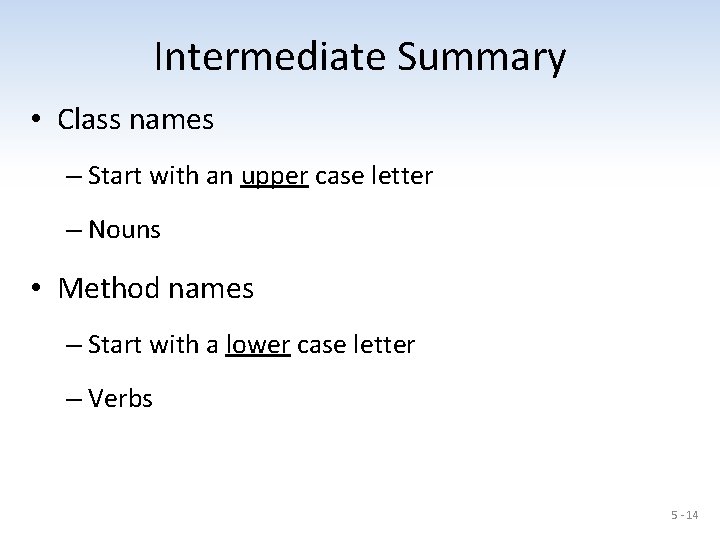
![2. Anatomy of a Class • [Q] What do the classes in your previous 2. Anatomy of a Class • [Q] What do the classes in your previous](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-15.jpg)
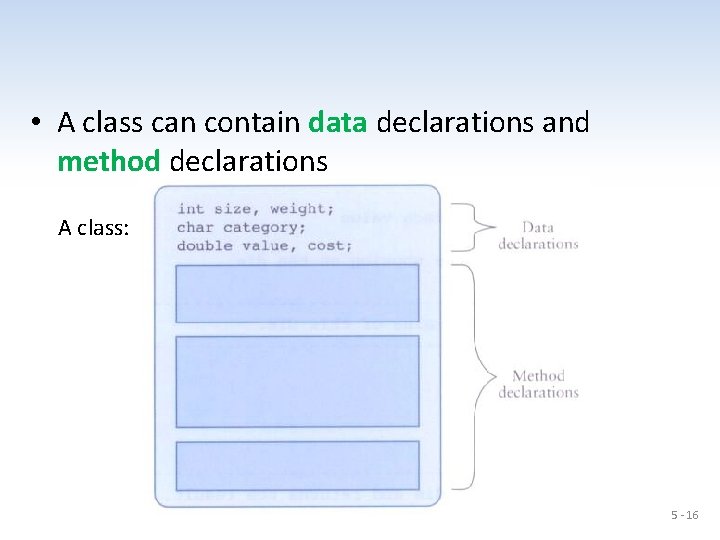
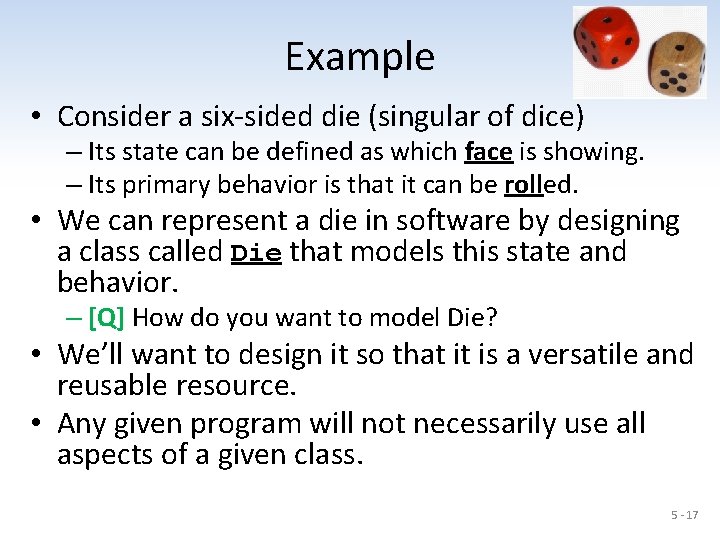
![public class Snake. Eyes // Game of craps { public static void main(String[] args) public class Snake. Eyes // Game of craps { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-18.jpg)
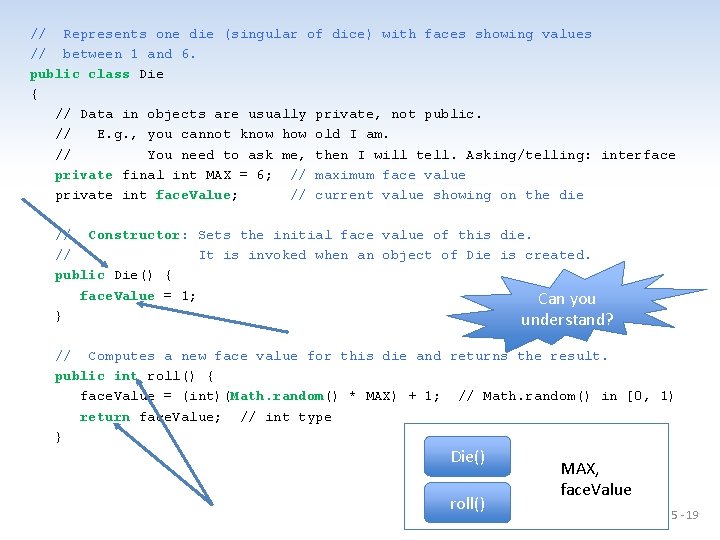
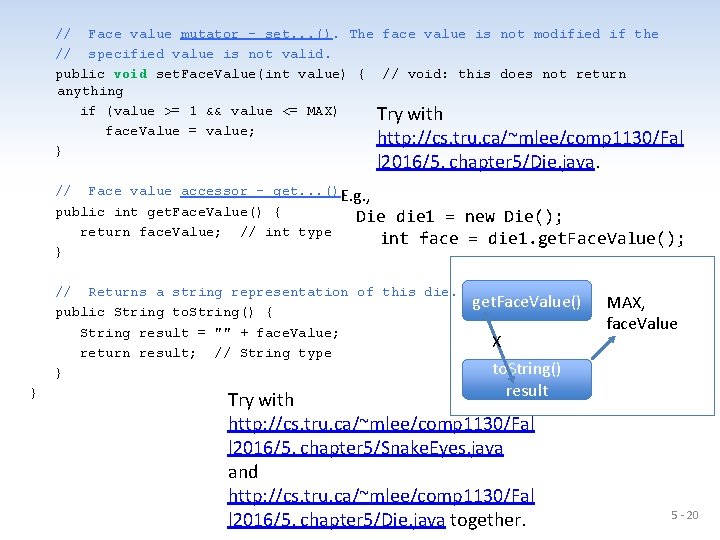
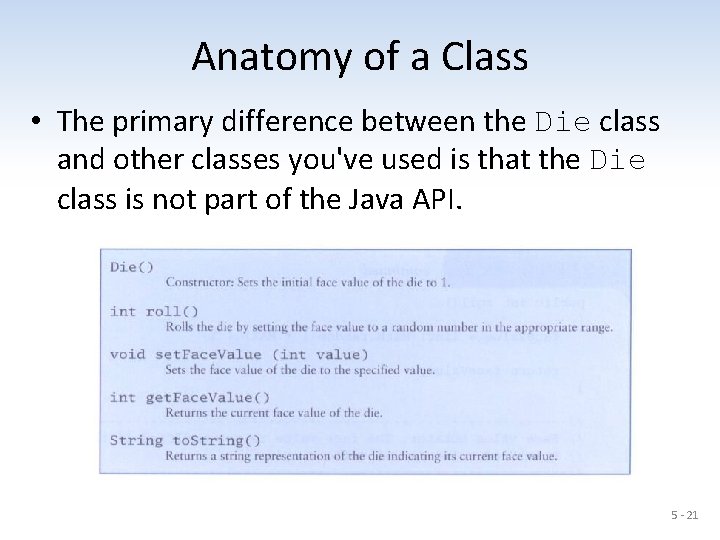
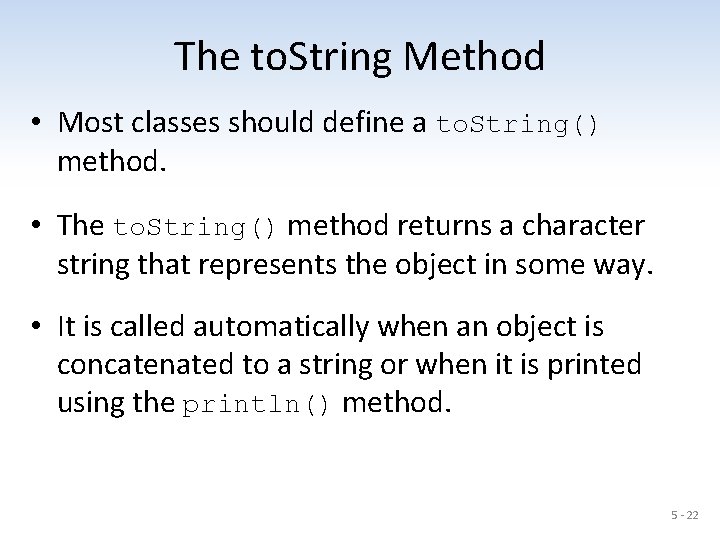
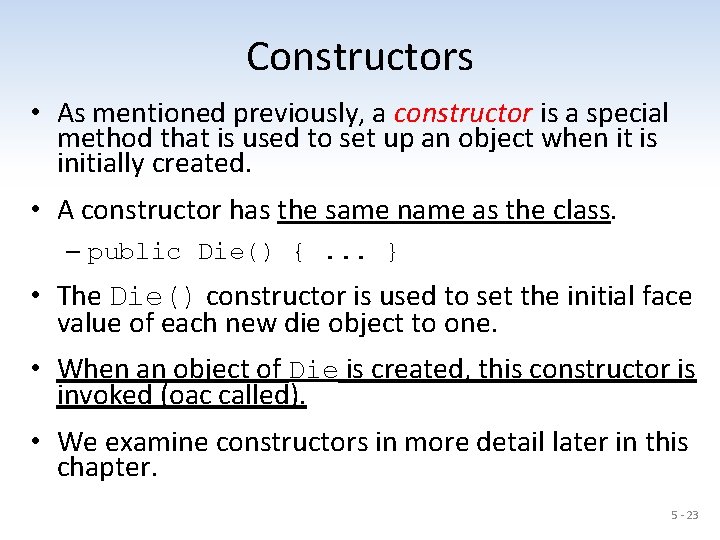
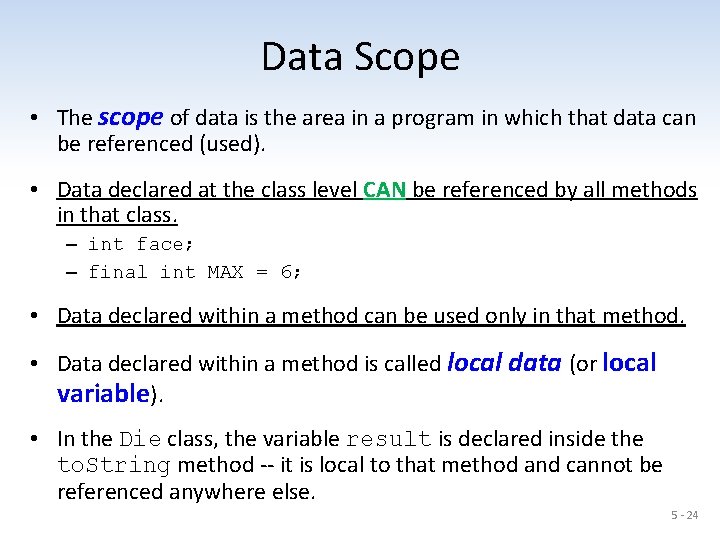
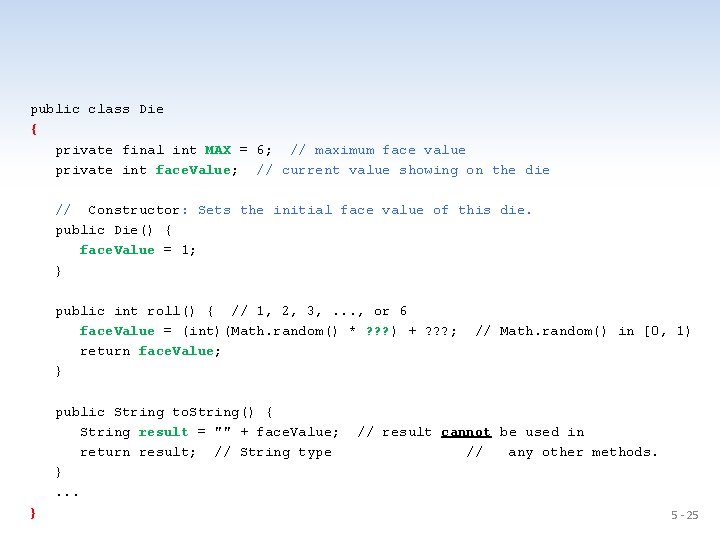
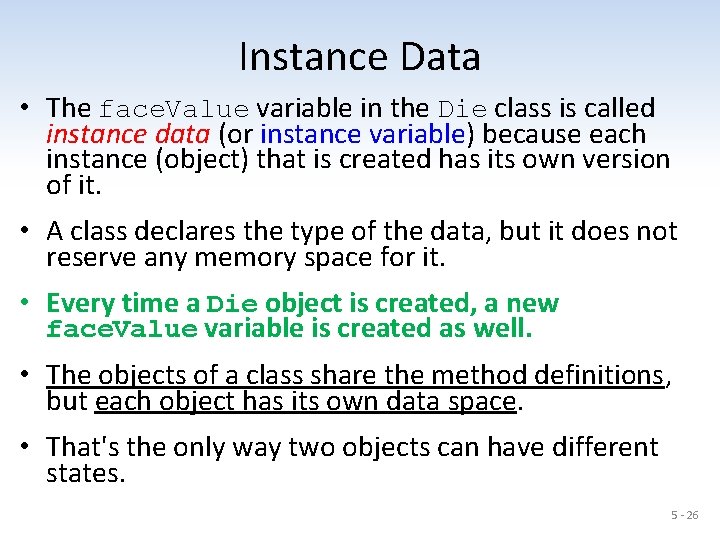
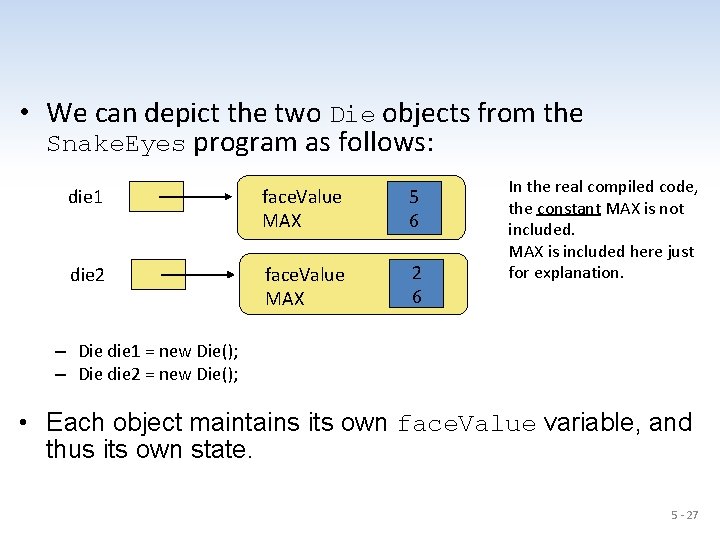
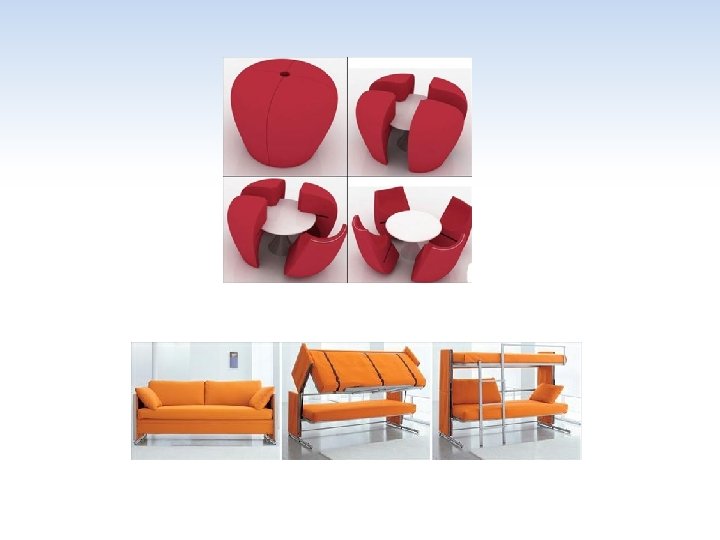
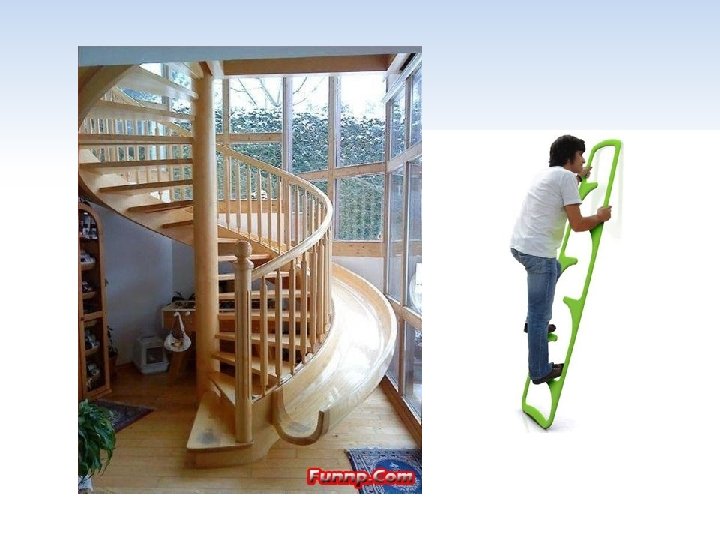
![UML Diagrams • [Q] Any good idea to describe/model a class before you start UML Diagrams • [Q] Any good idea to describe/model a class before you start](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-30.jpg)
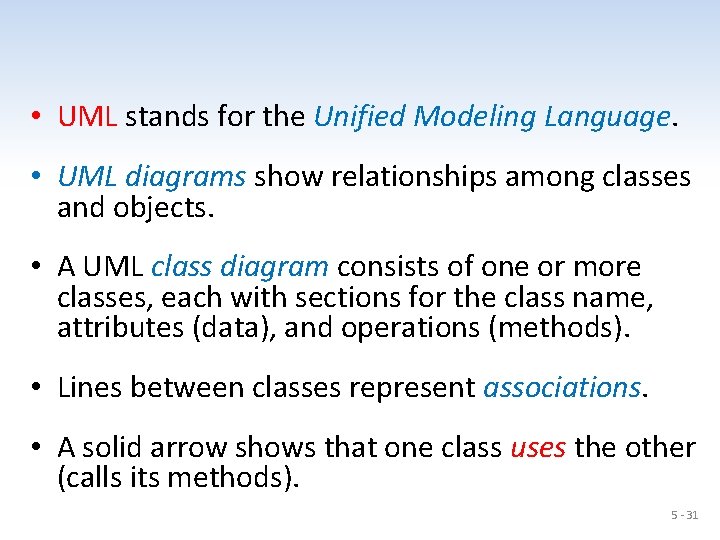
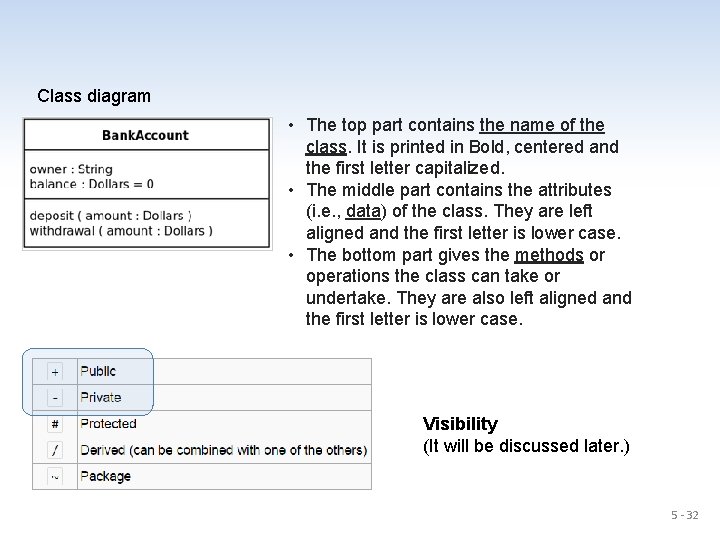
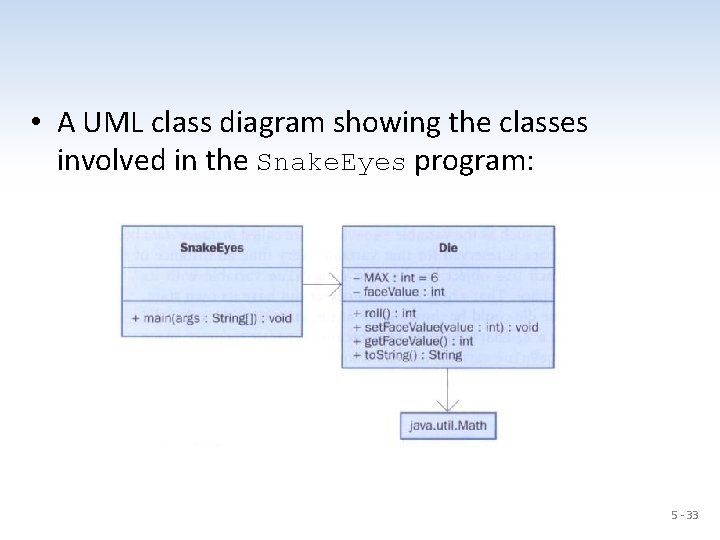
![public class Snake. Eyes { public static void main(String[] args) { final int ROLLS public class Snake. Eyes { public static void main(String[] args) { final int ROLLS](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-34.jpg)
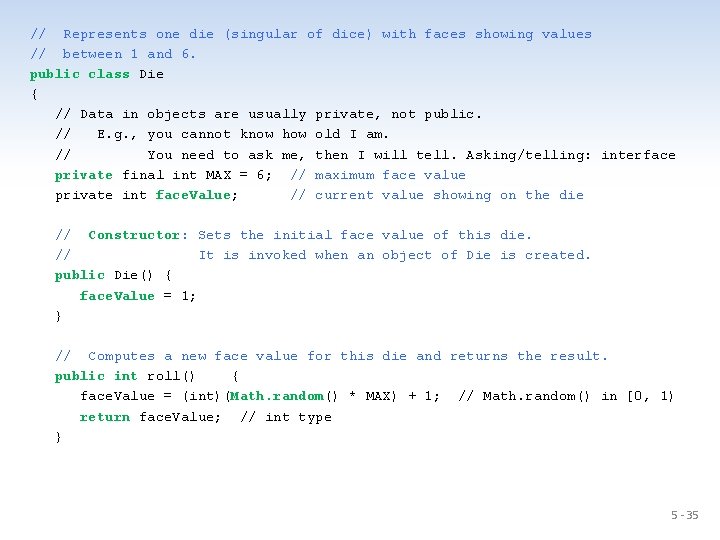
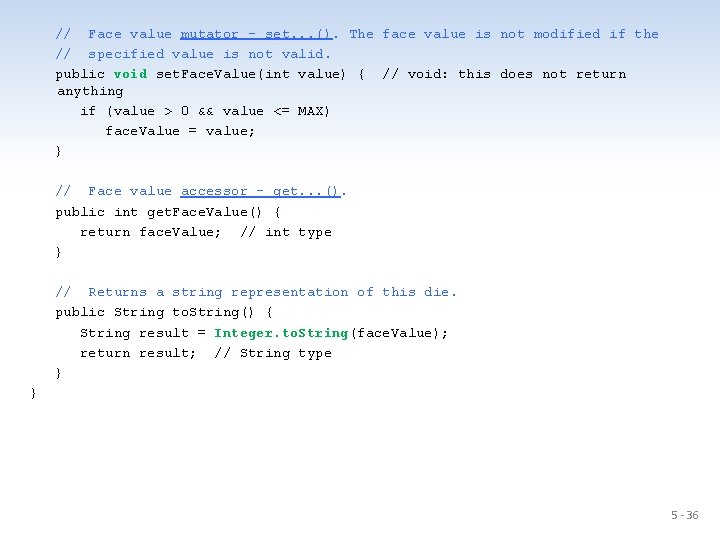
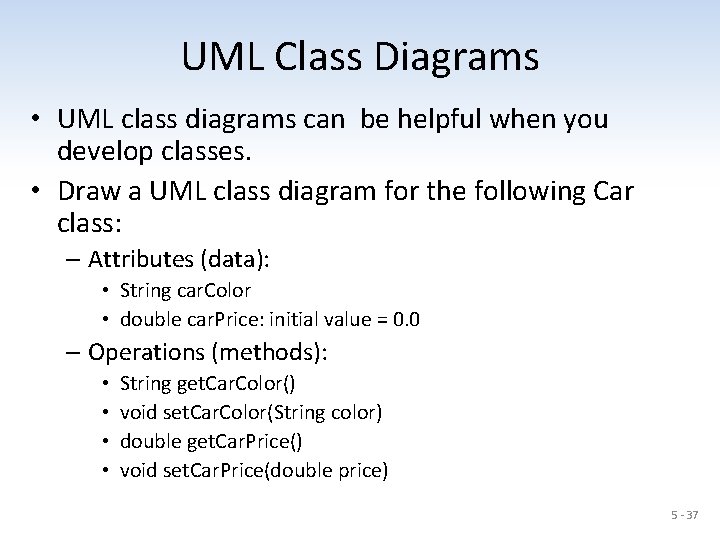
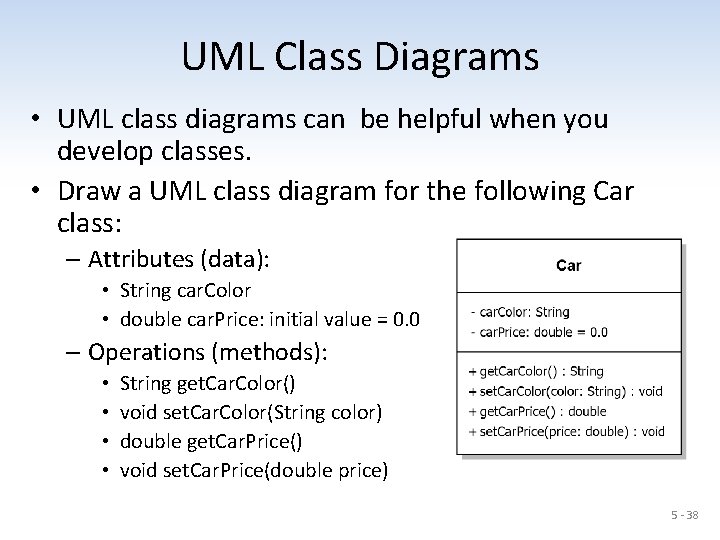
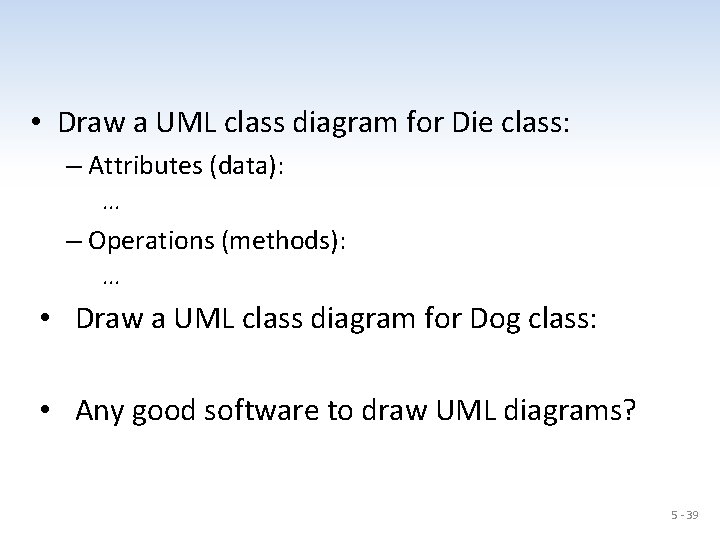
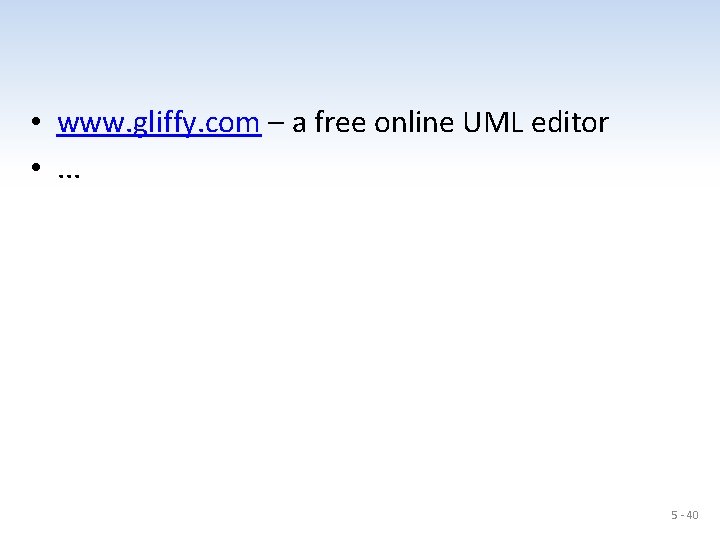
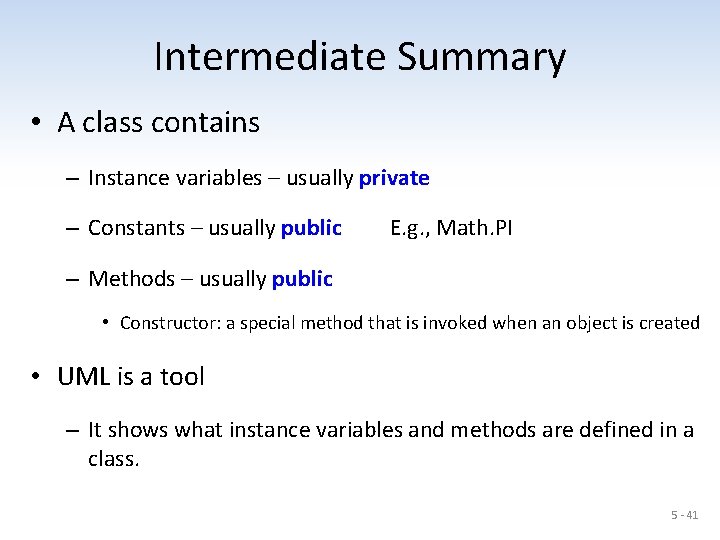
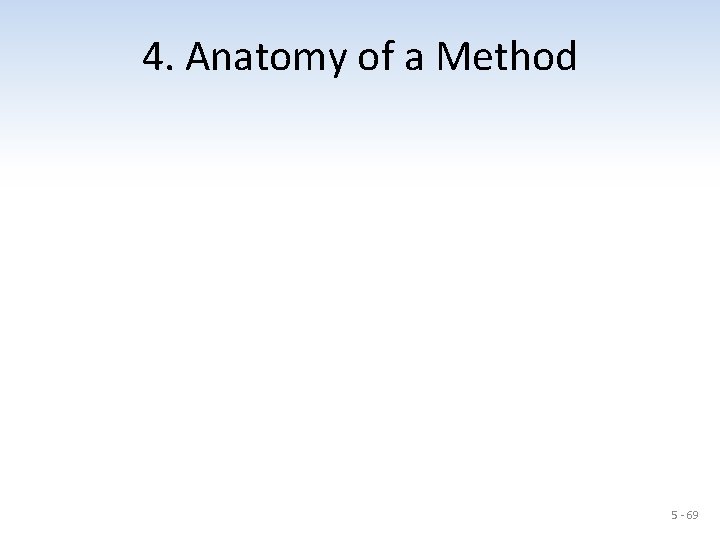
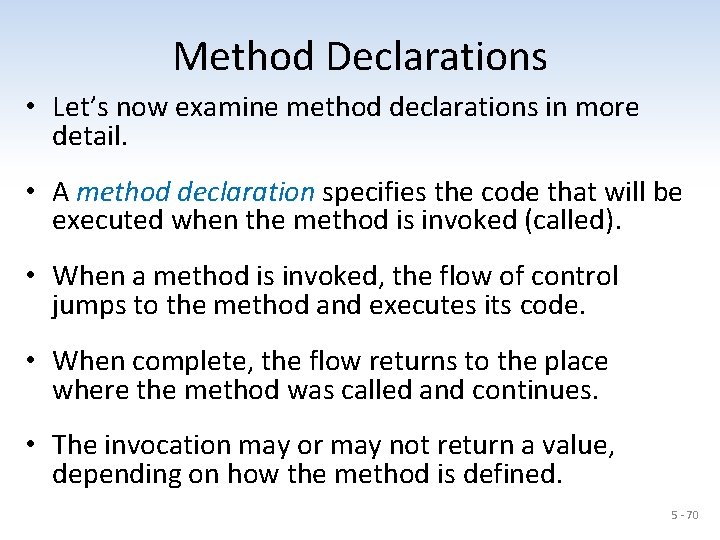
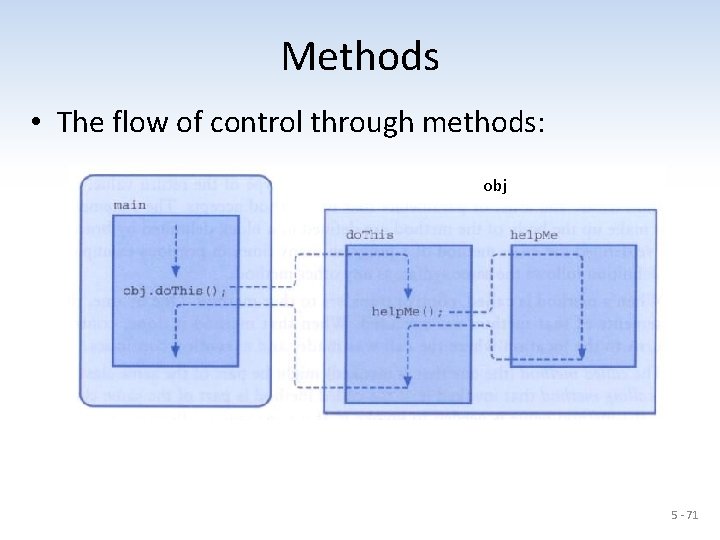
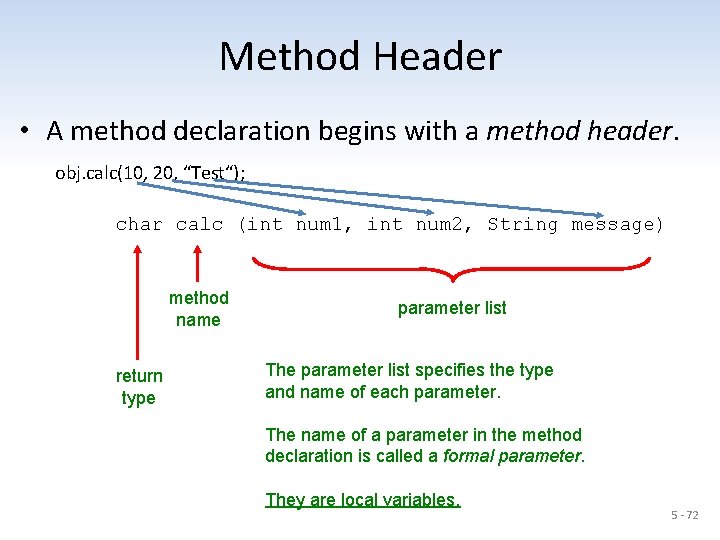
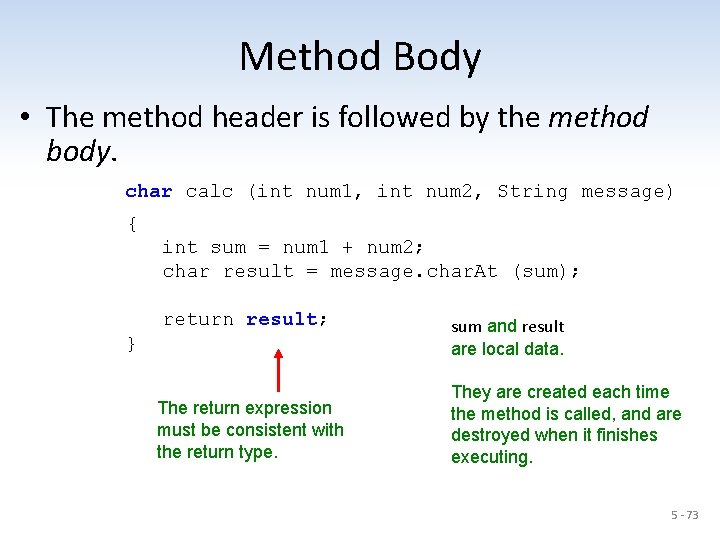
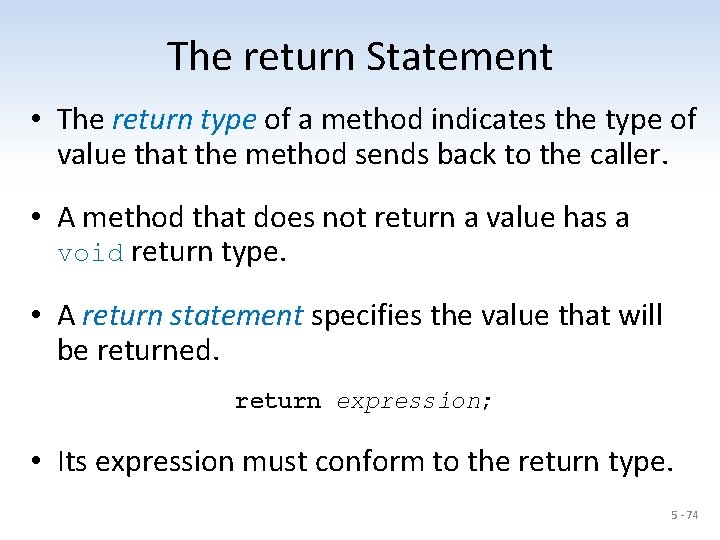
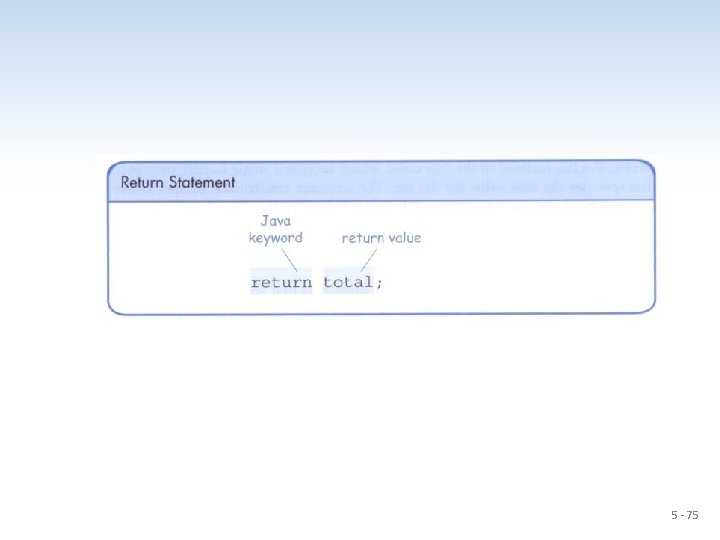
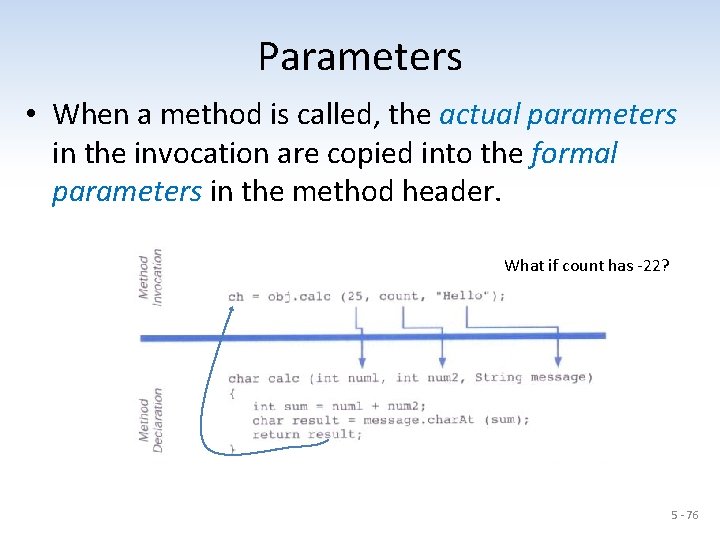
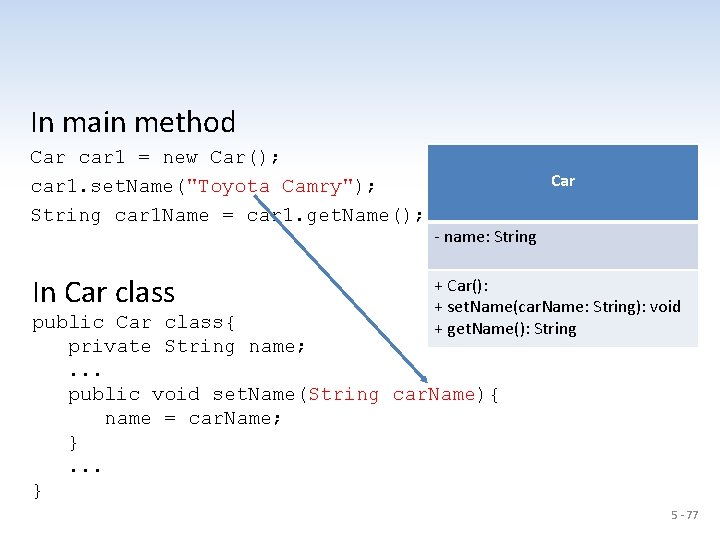
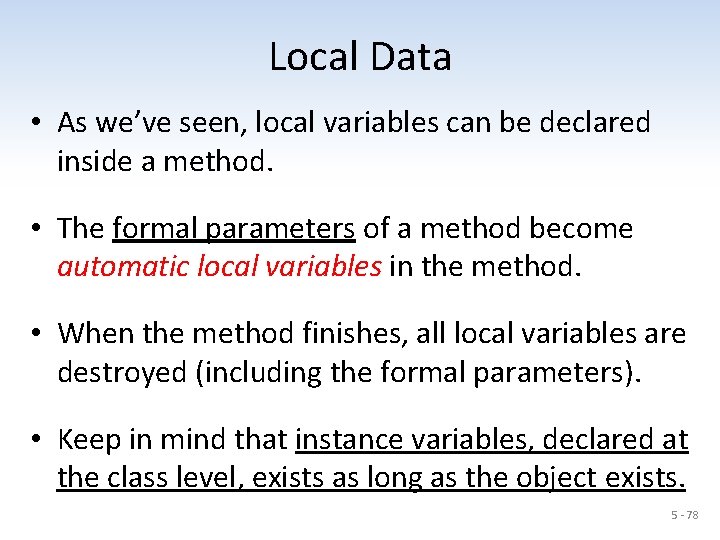
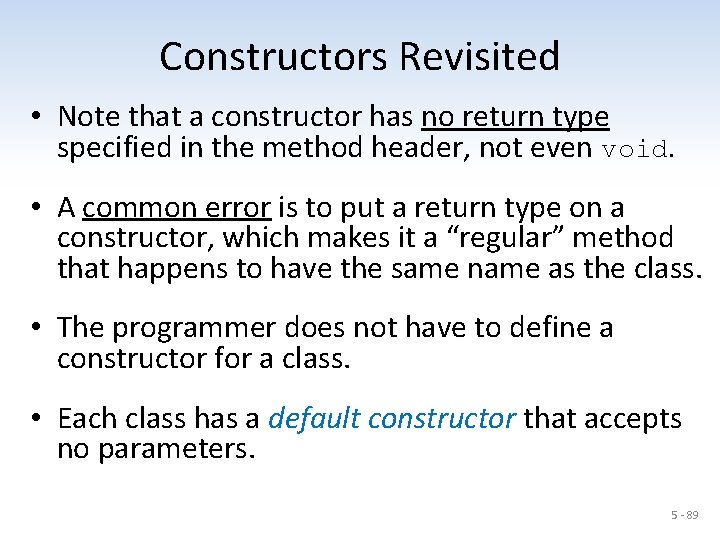
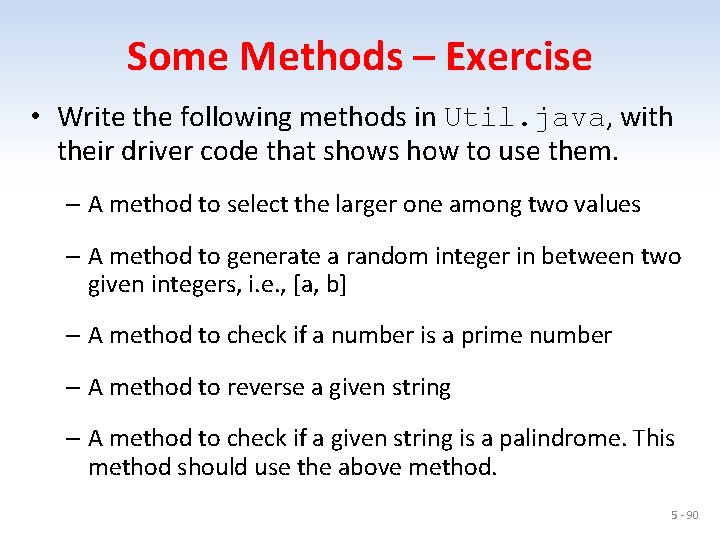
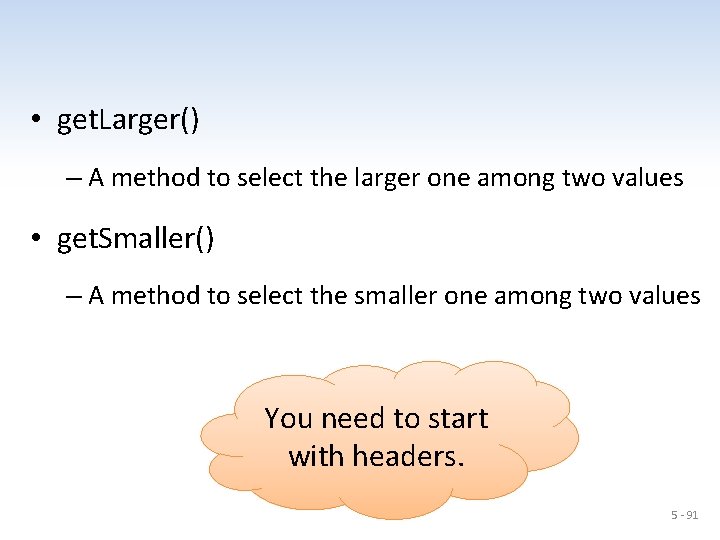
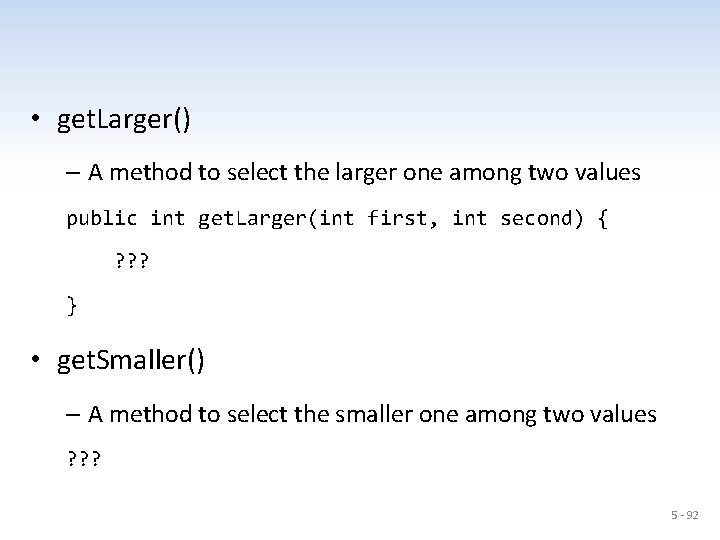
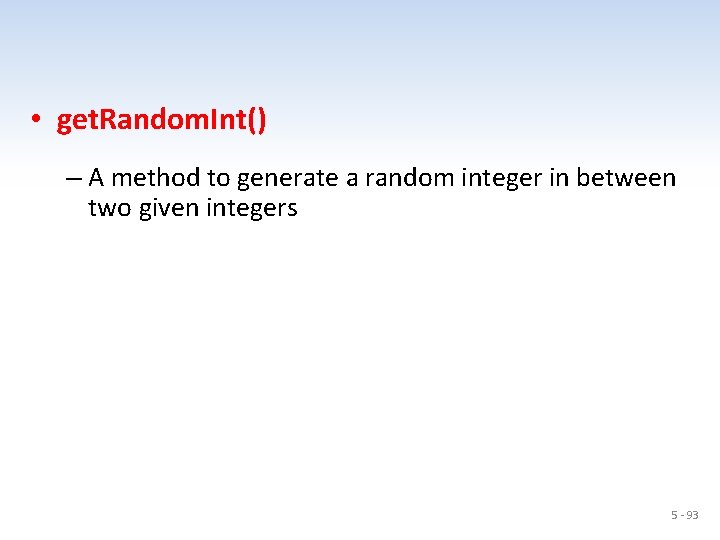
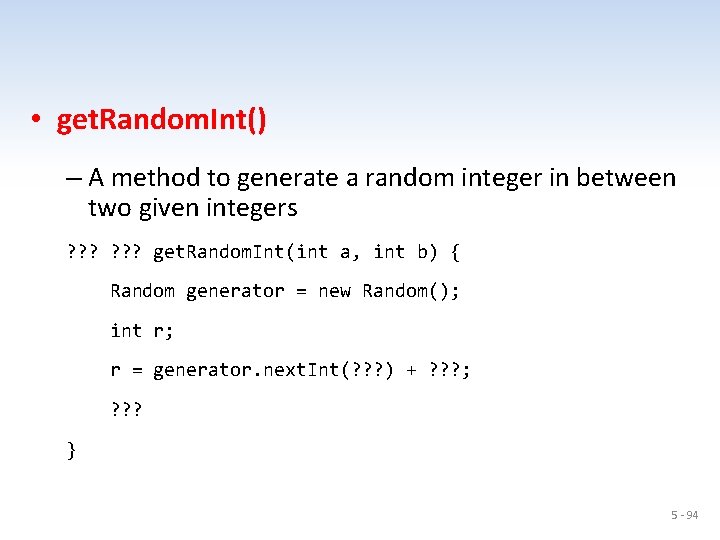
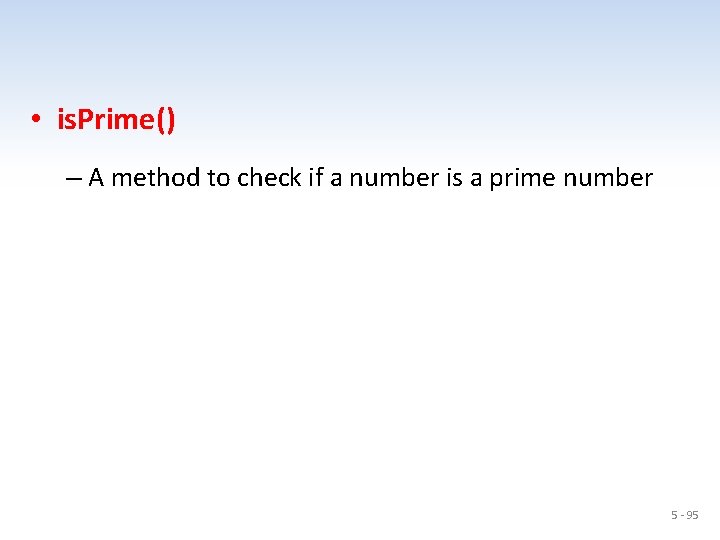
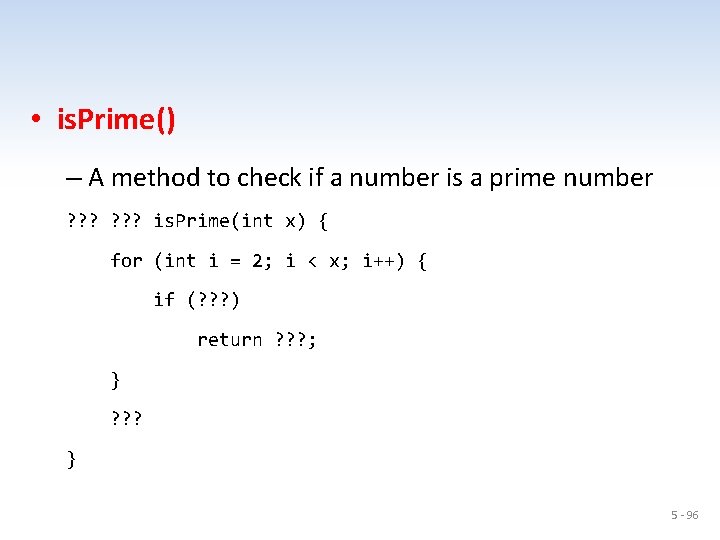
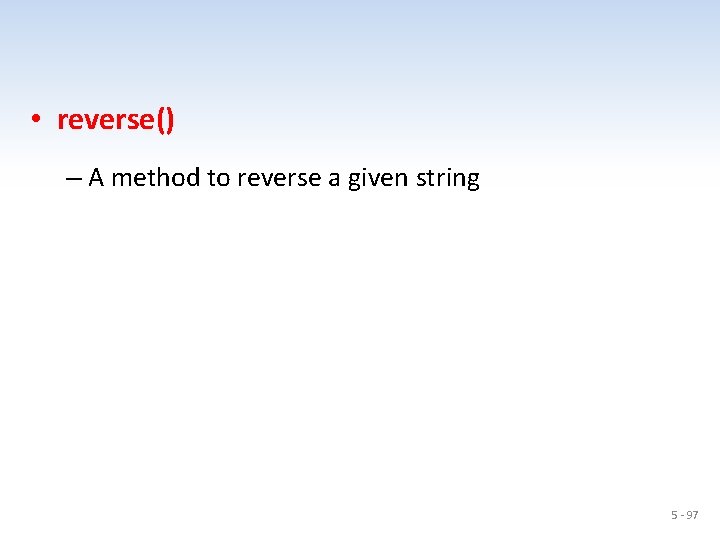
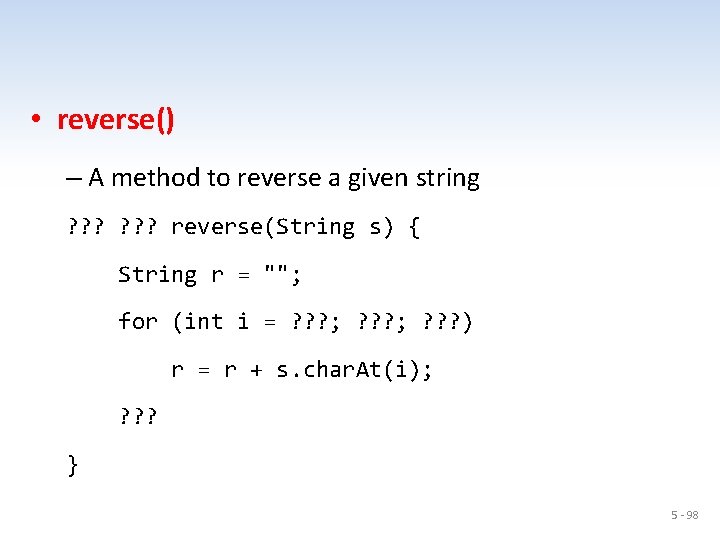
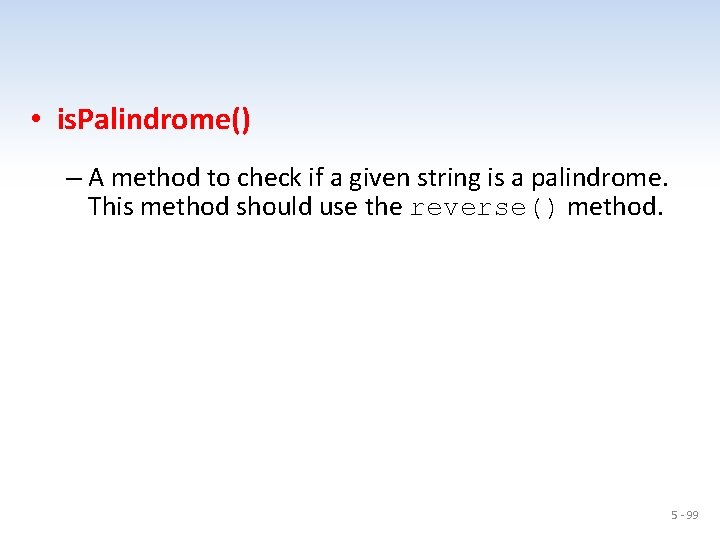
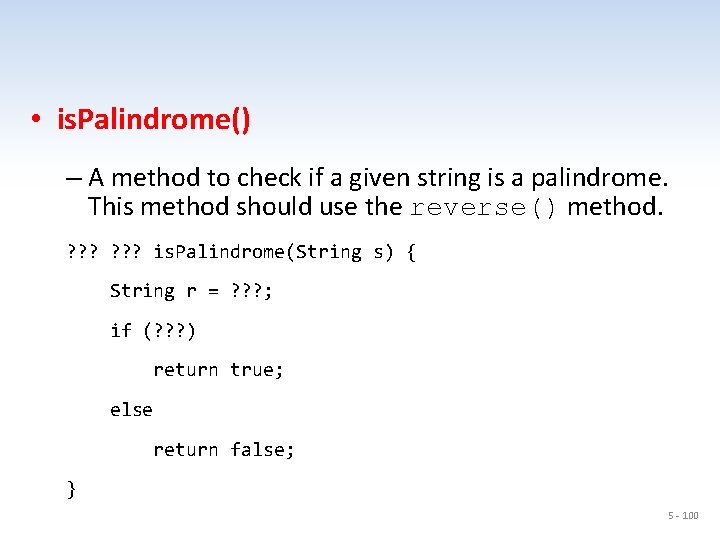
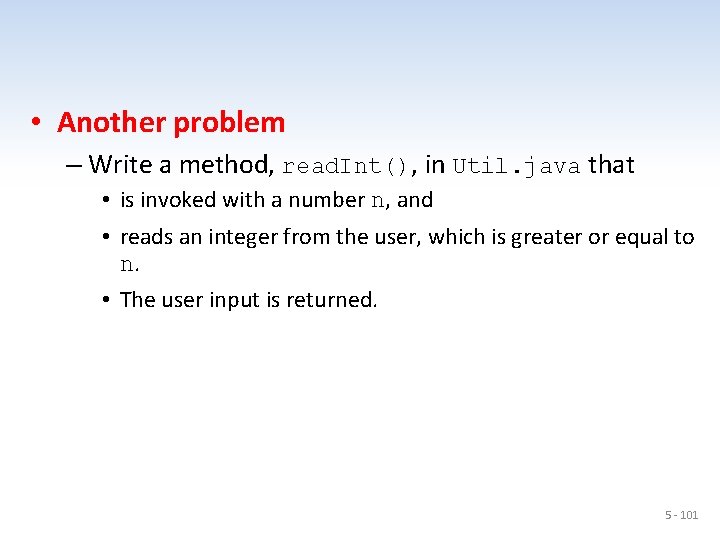
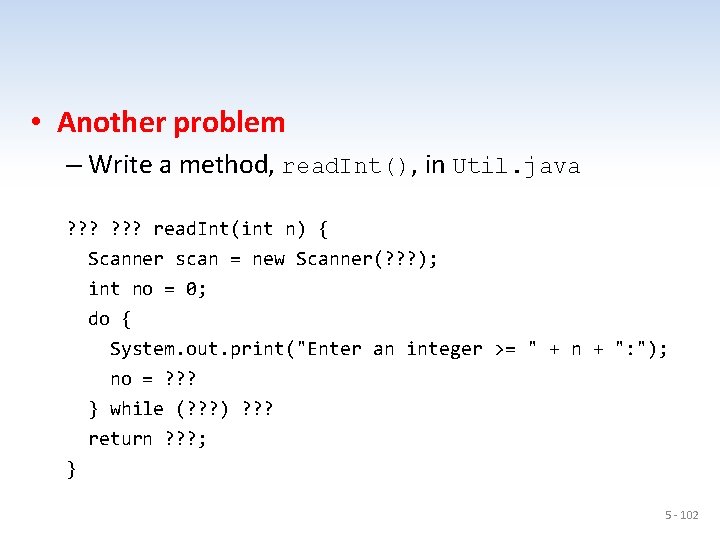
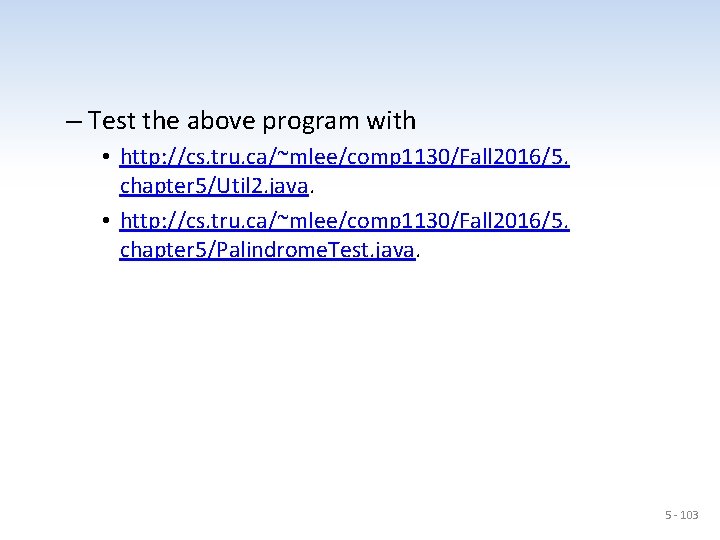
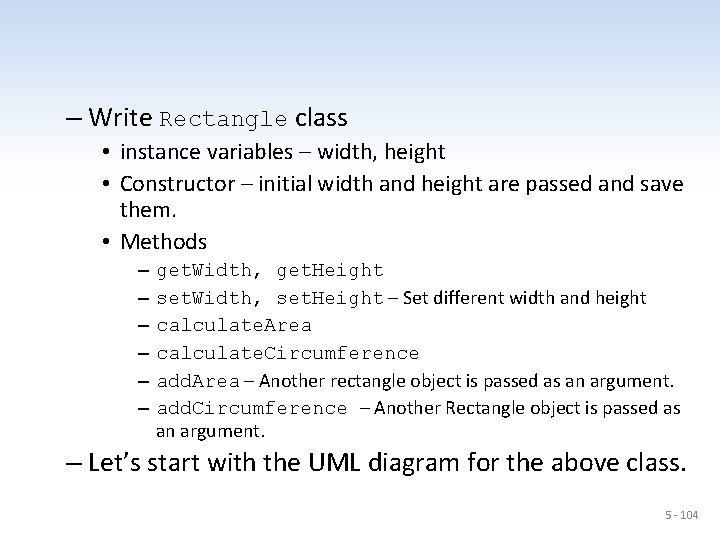
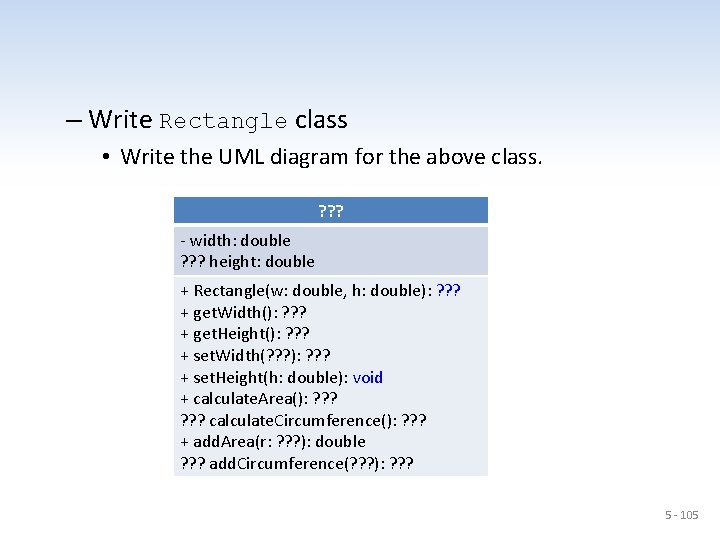
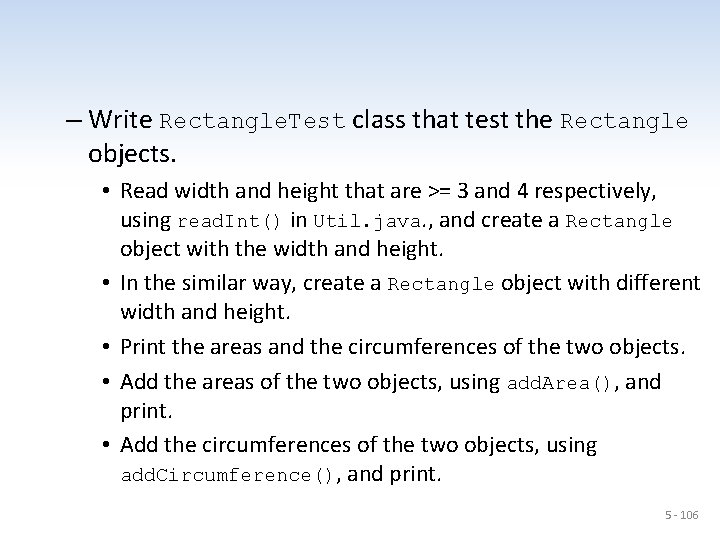
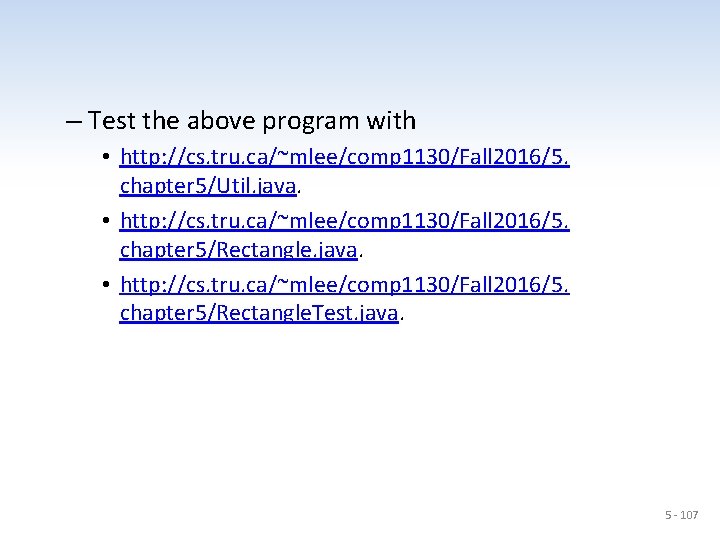
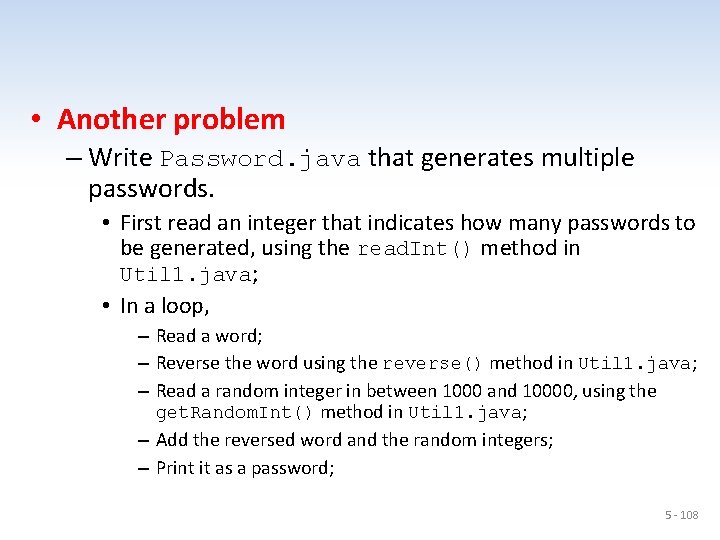
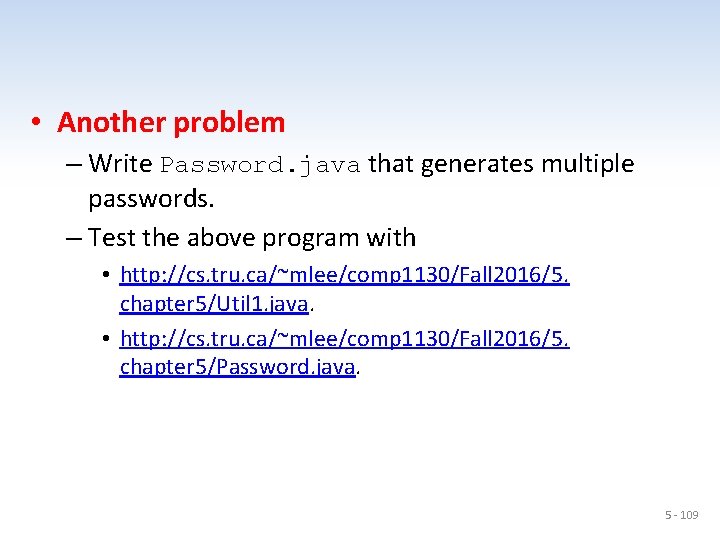
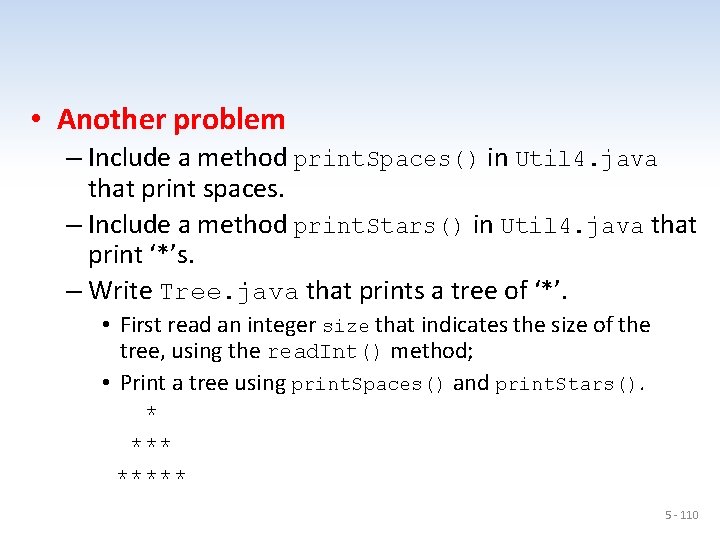
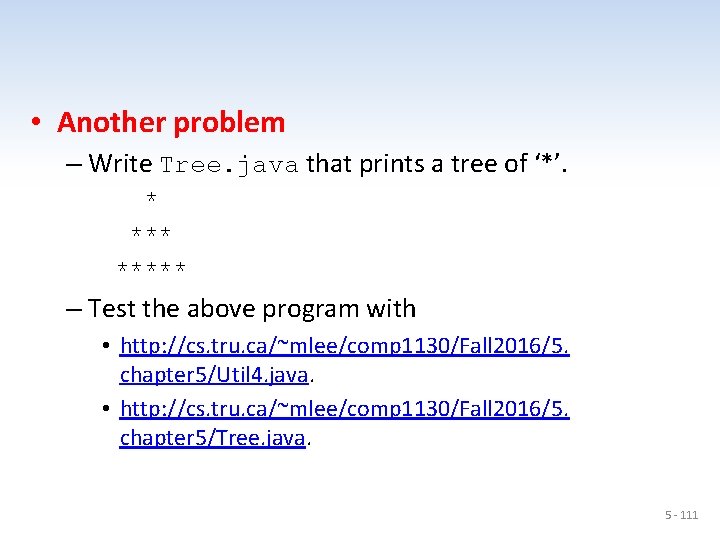
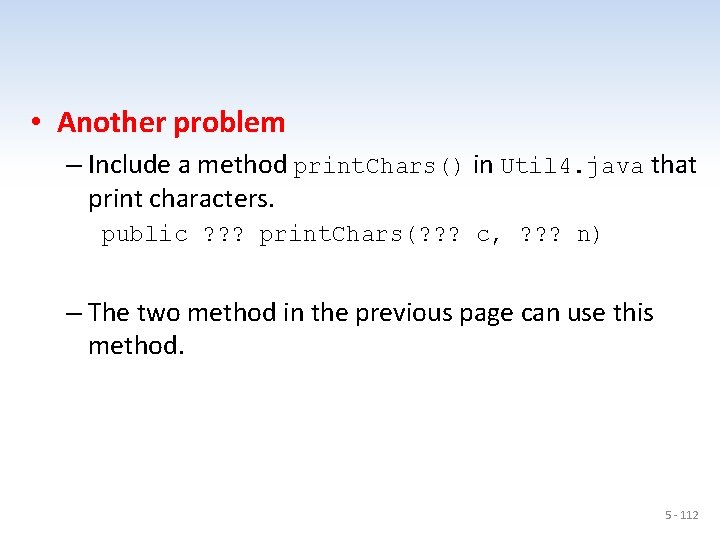
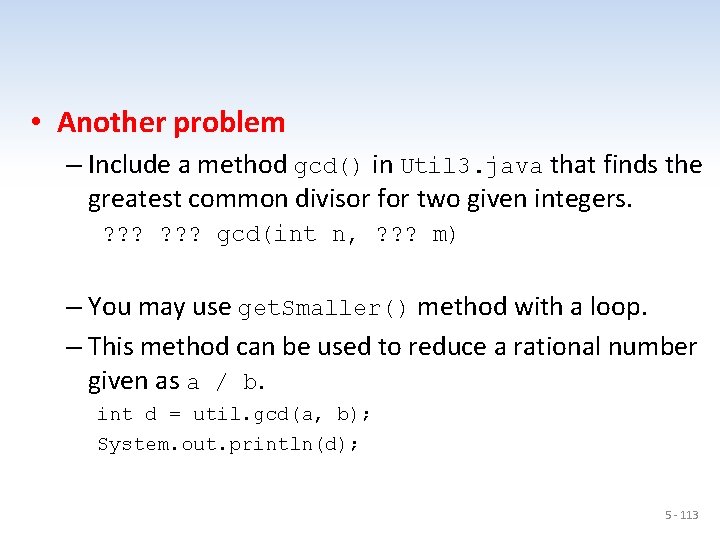
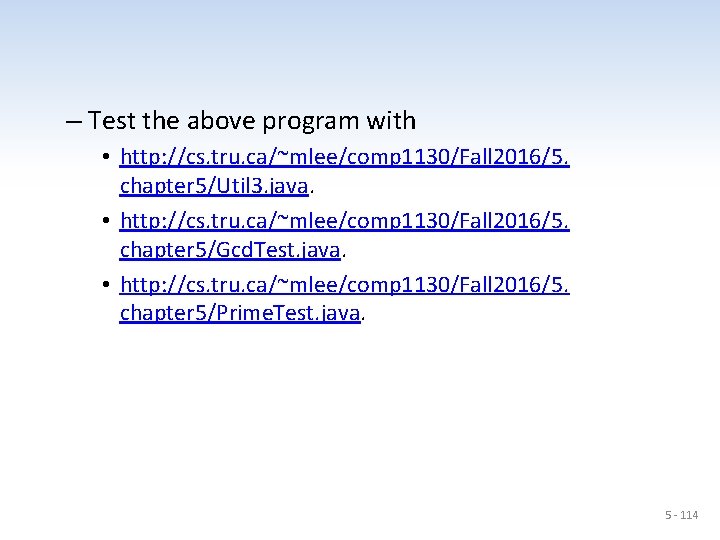
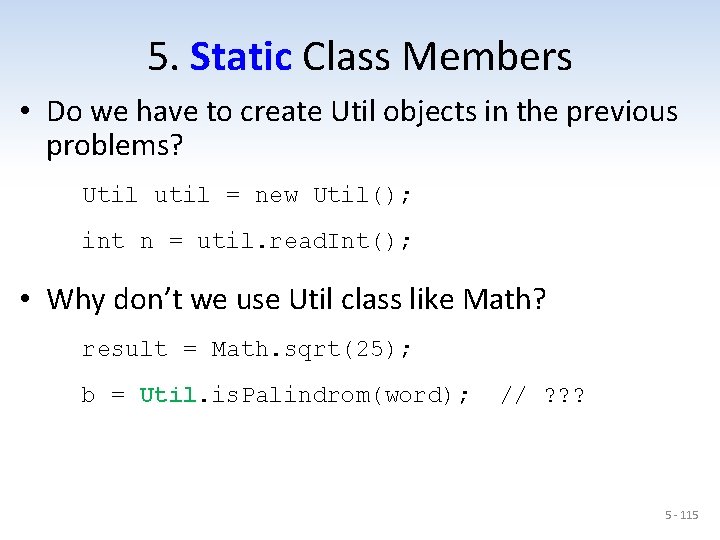
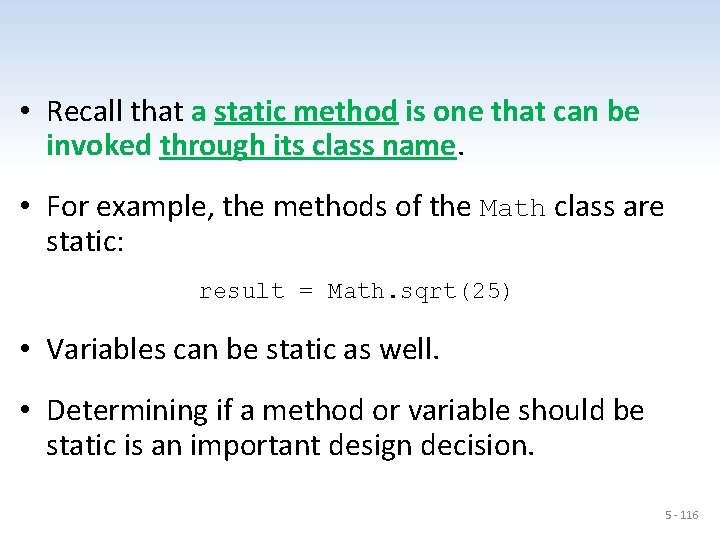
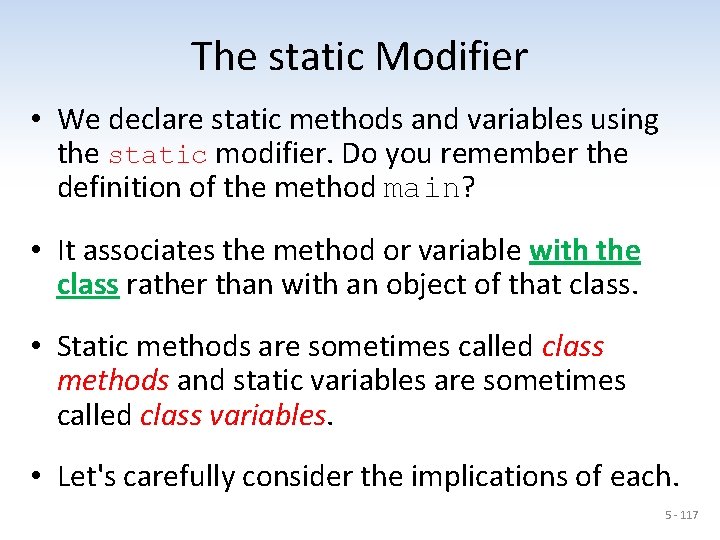
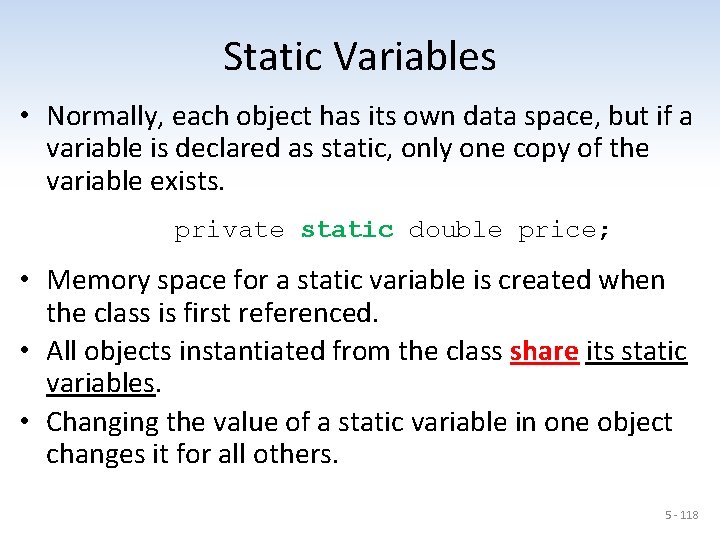
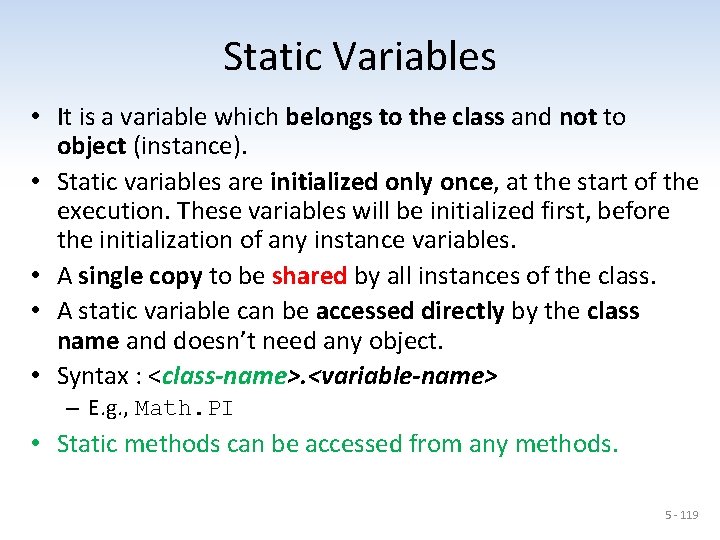
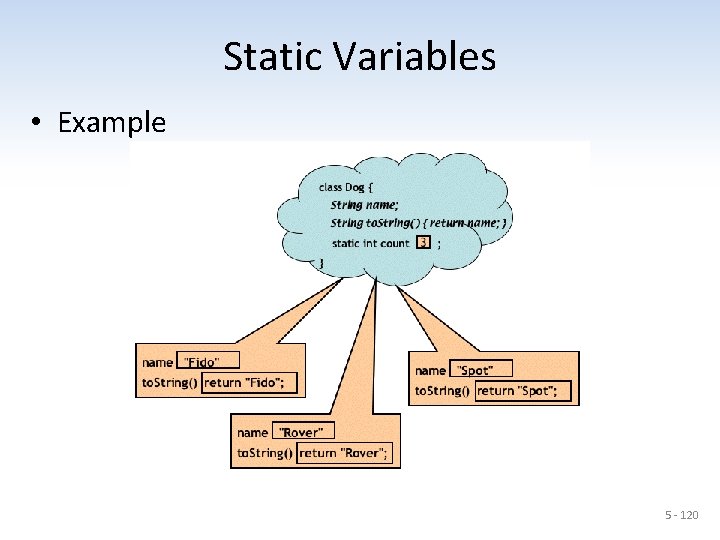
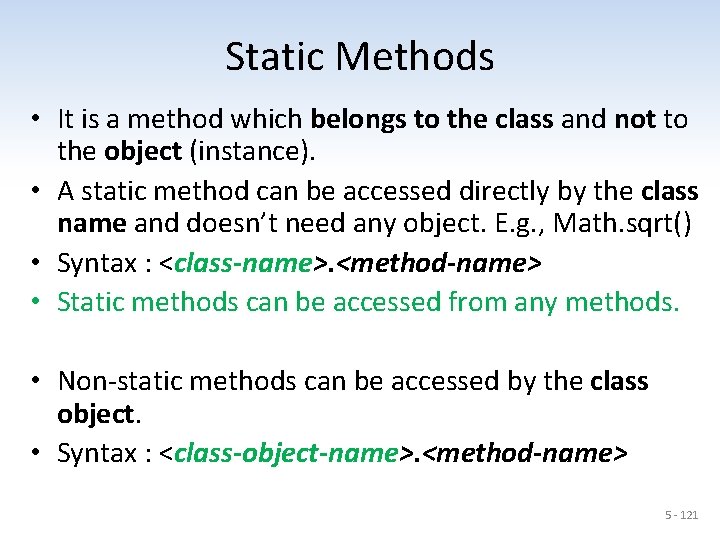
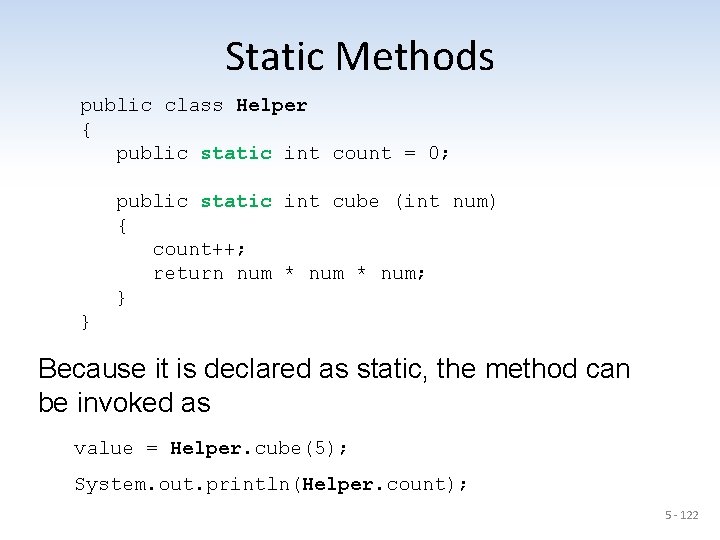
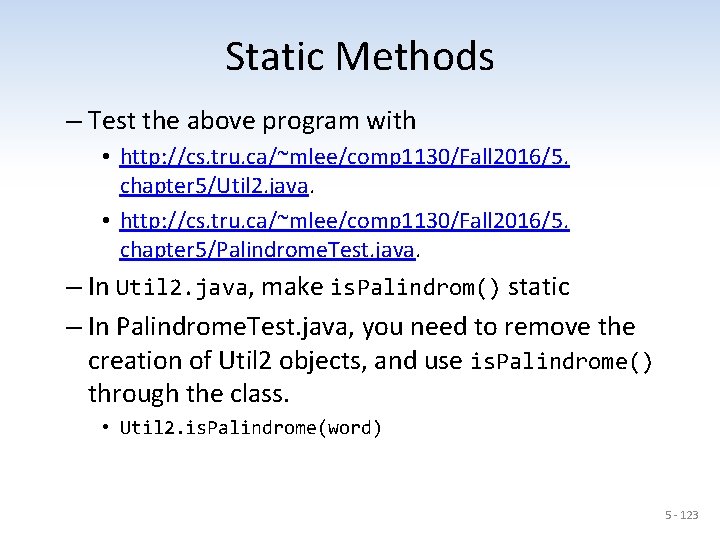
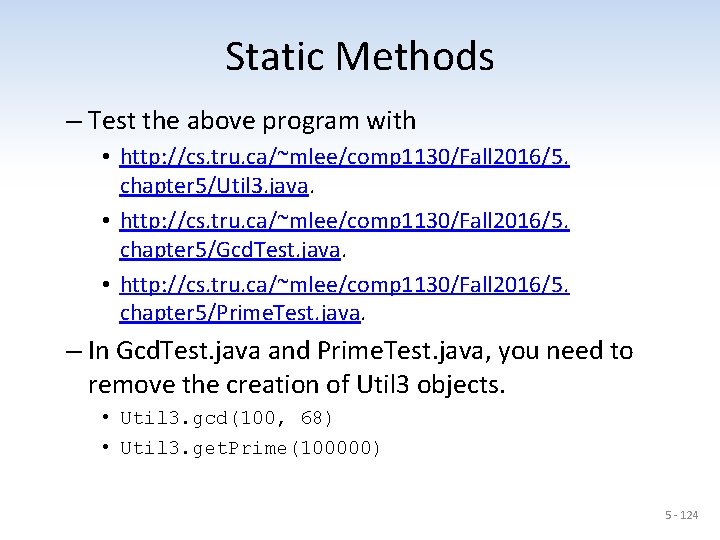
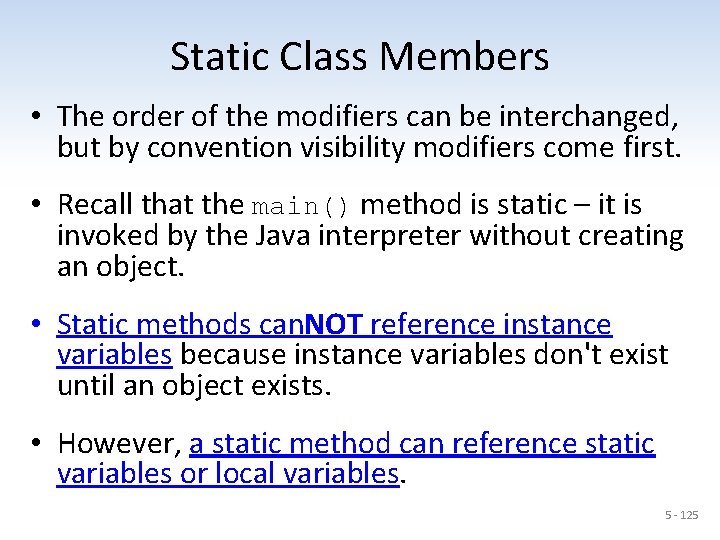
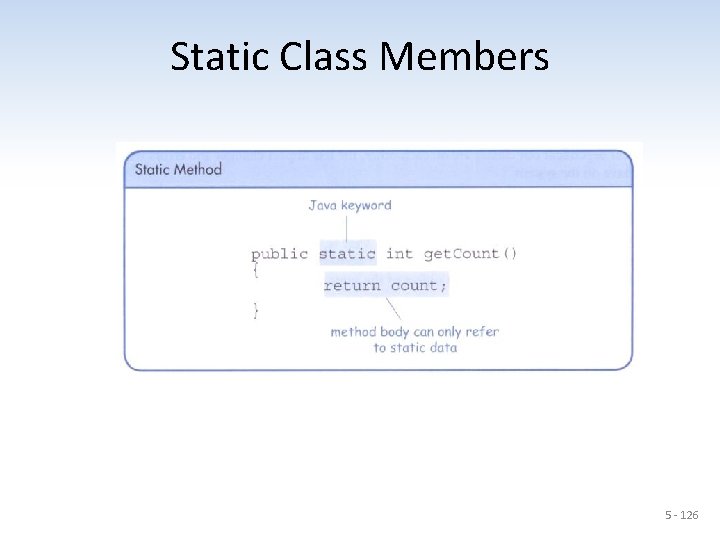
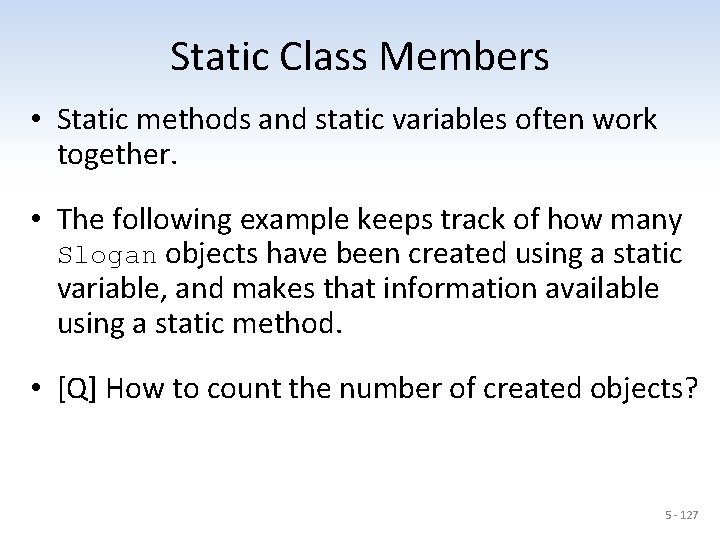
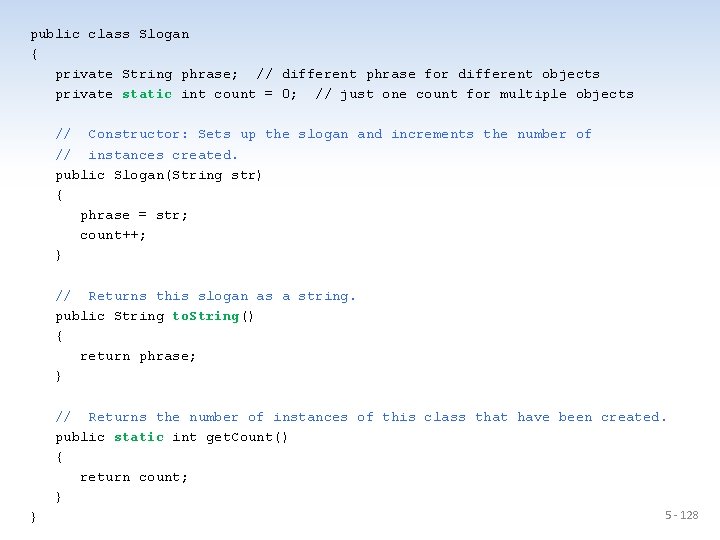
![public class Slogan. Counter { public static void main(String[] args) { Slogan obj; obj public class Slogan. Counter { public static void main(String[] args) { Slogan obj; obj](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-92.jpg)
![public class Slogan. Counter { public static void main(String[] args) { Slogan obj; obj public class Slogan. Counter { public static void main(String[] args) { Slogan obj; obj](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-93.jpg)
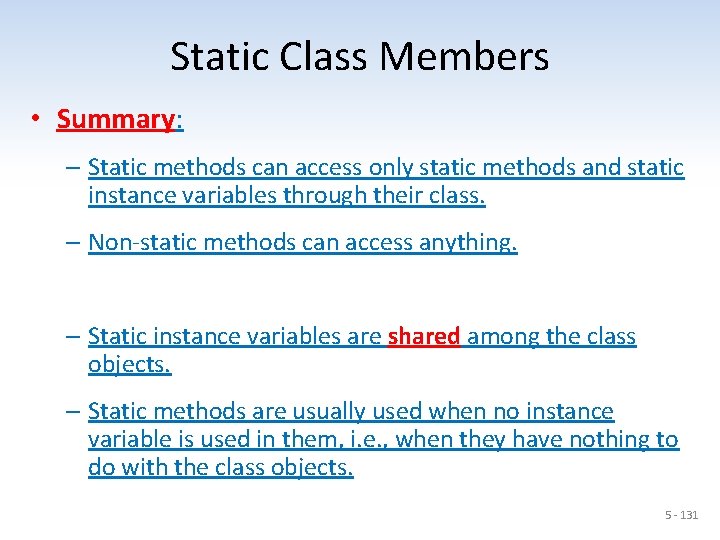
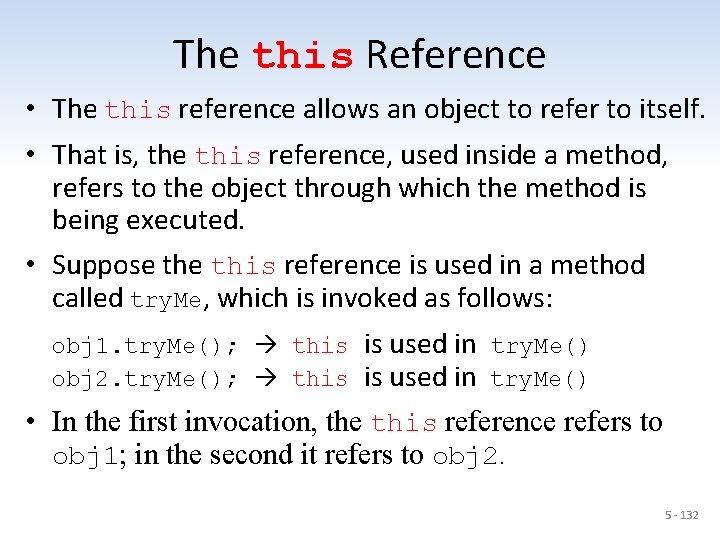
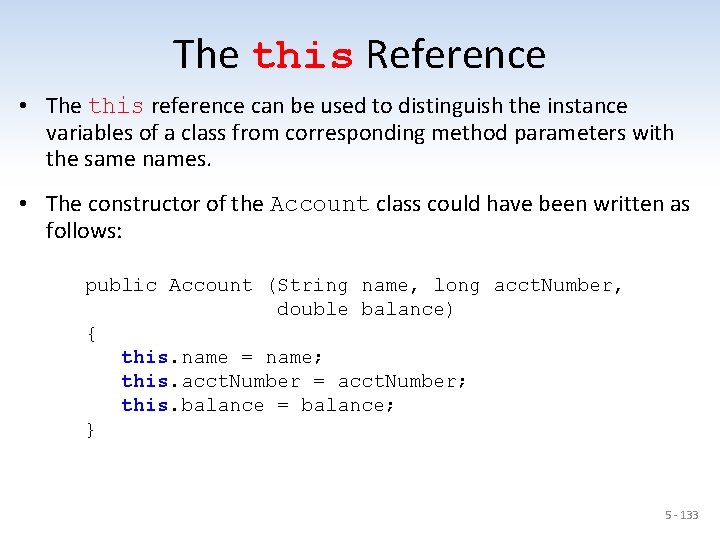
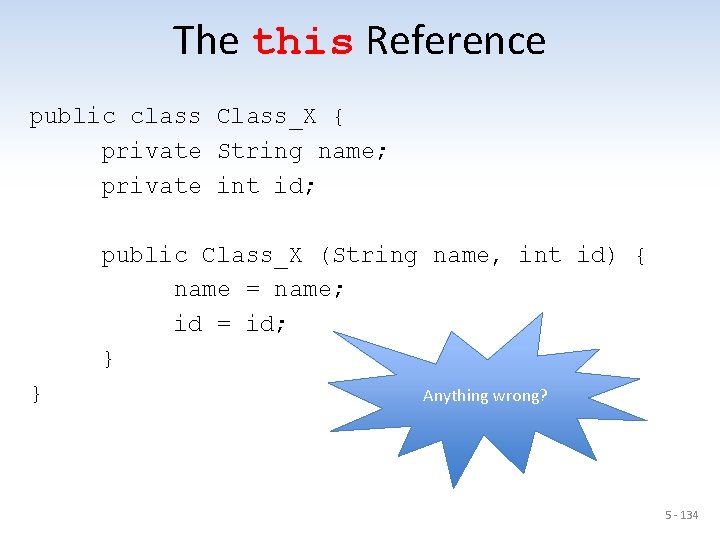
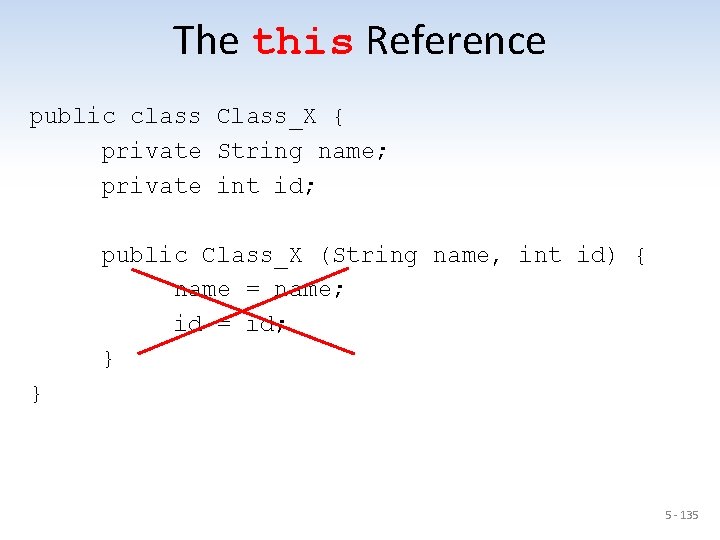
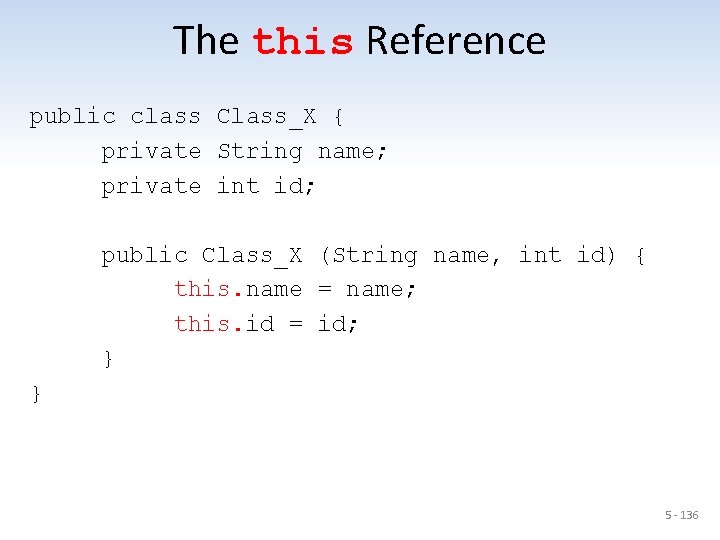
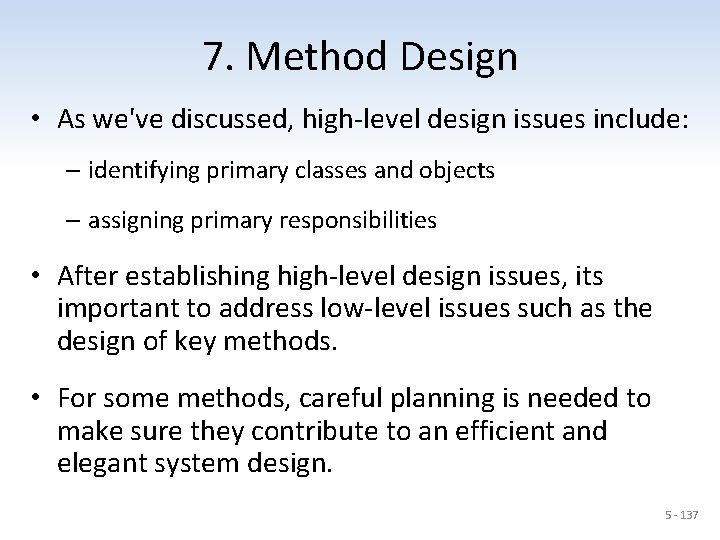
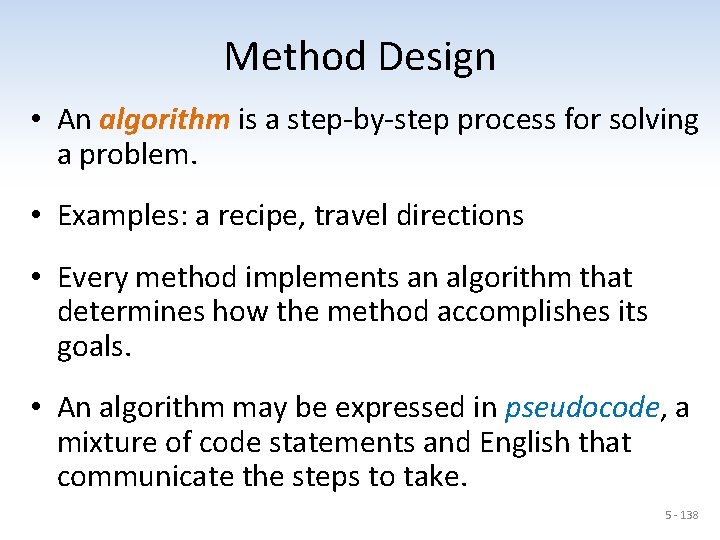
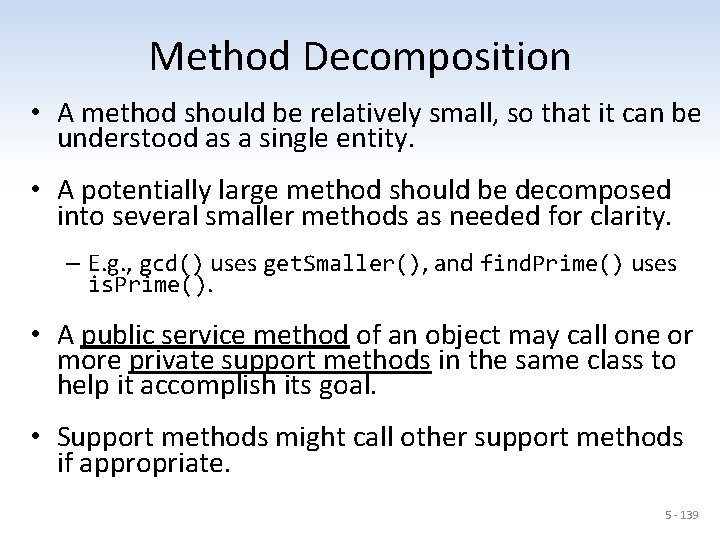
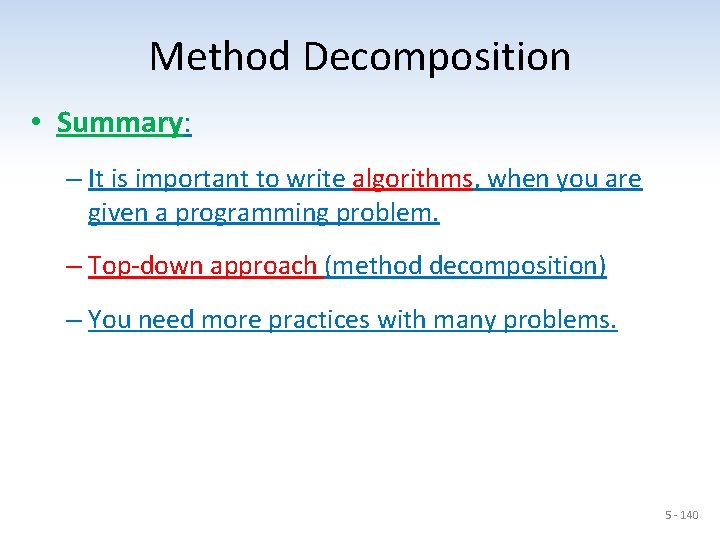
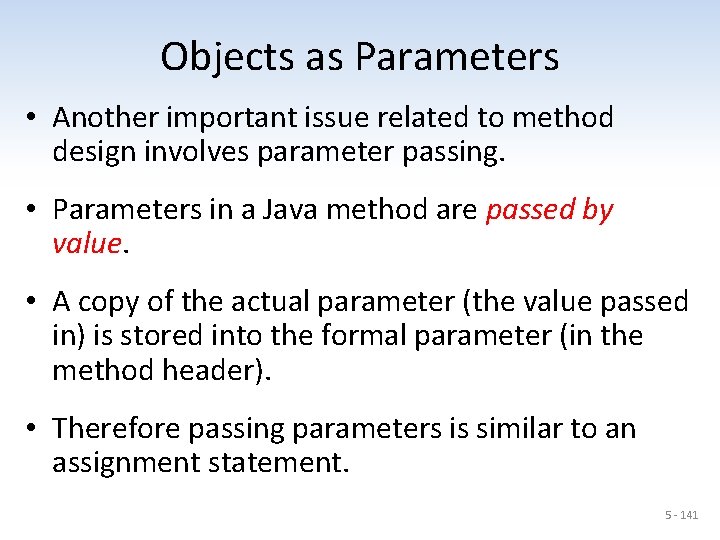
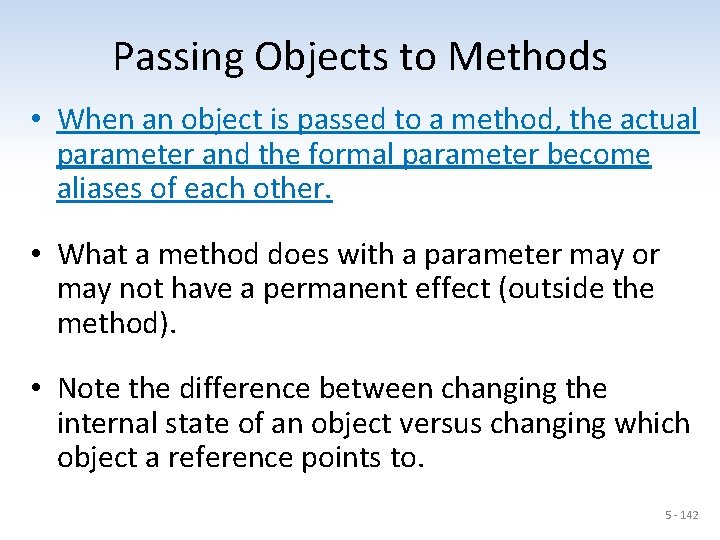
![public class Parameter. Tester { public static void main(String[] args) { Parameter. Modifier modifier public class Parameter. Tester { public static void main(String[] args) { Parameter. Modifier modifier](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-106.jpg)
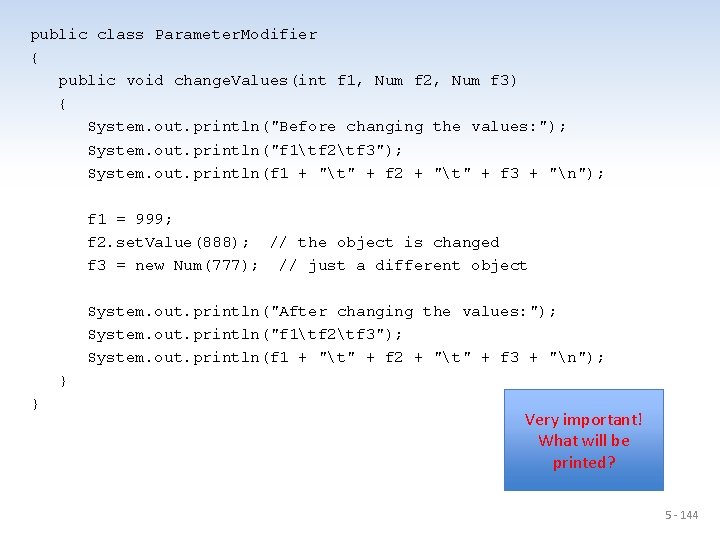
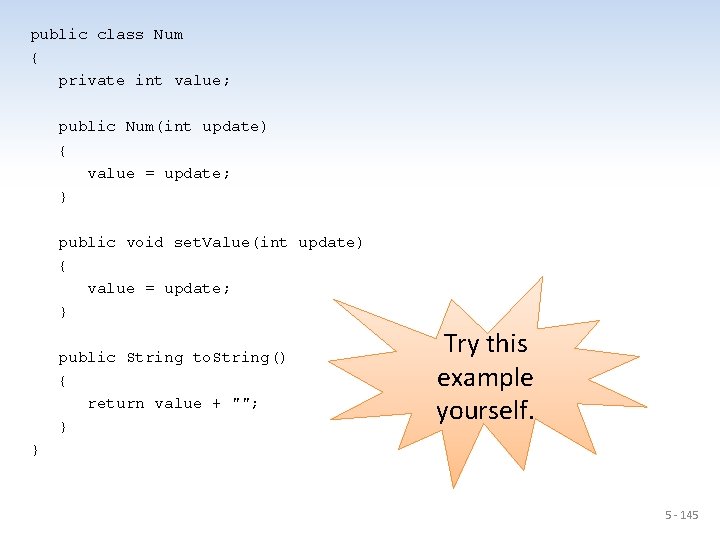
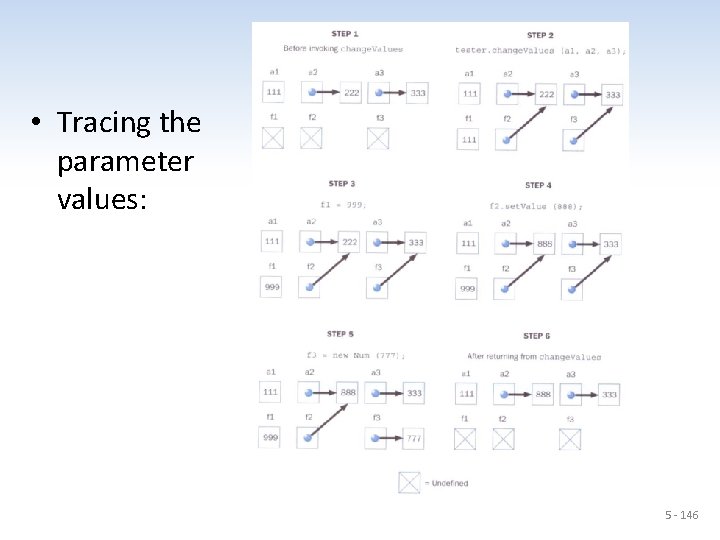
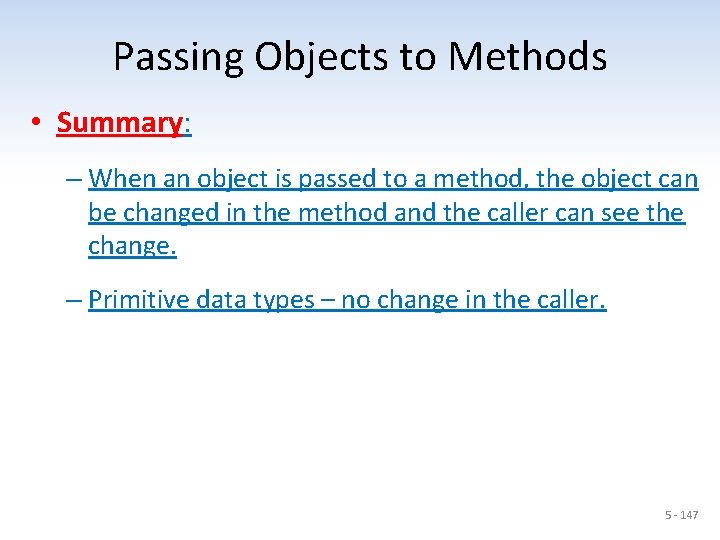
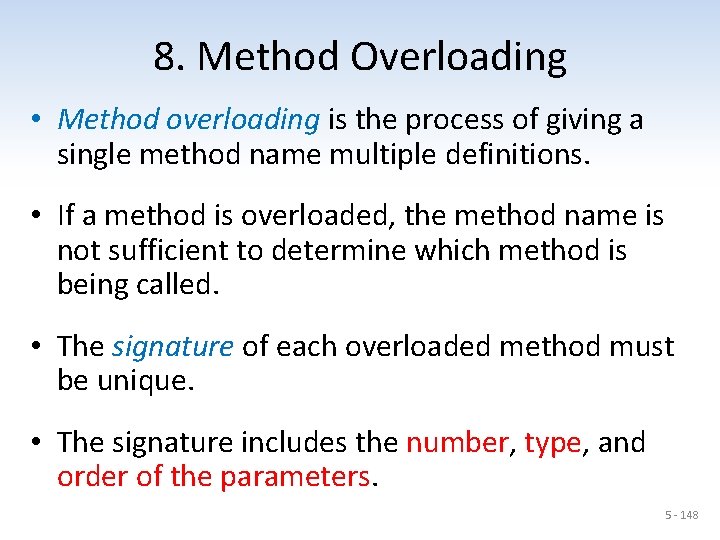
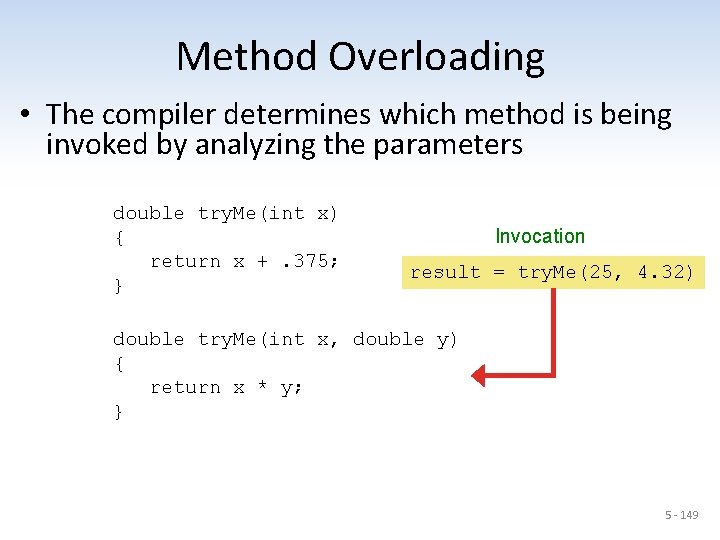
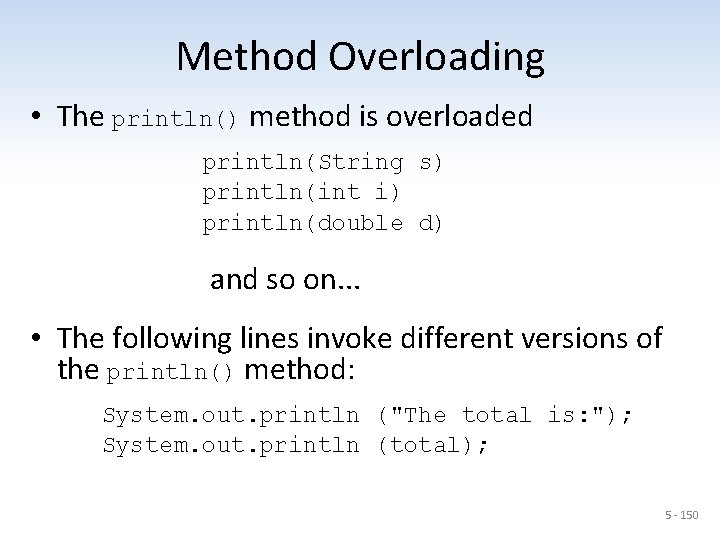
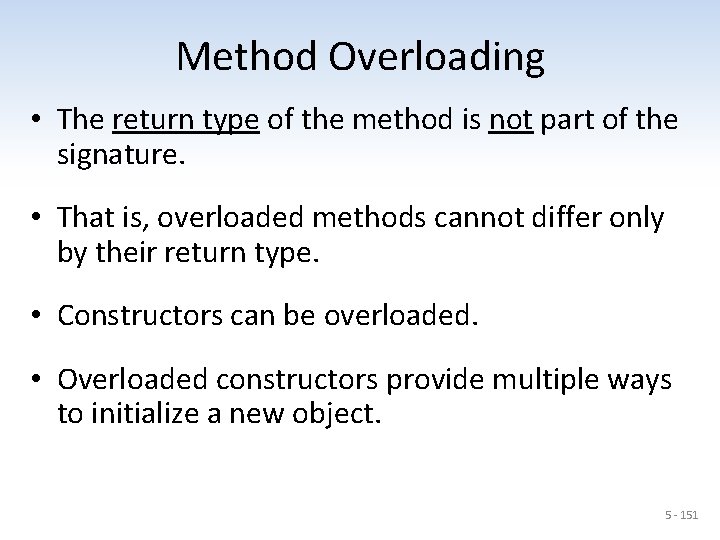
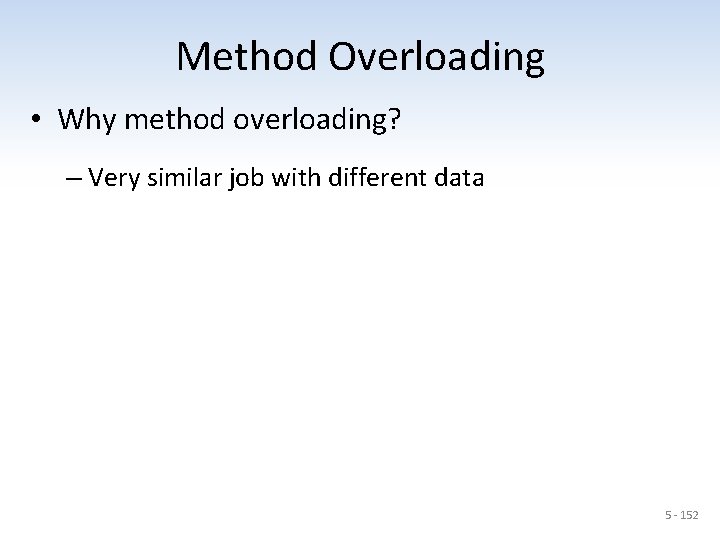
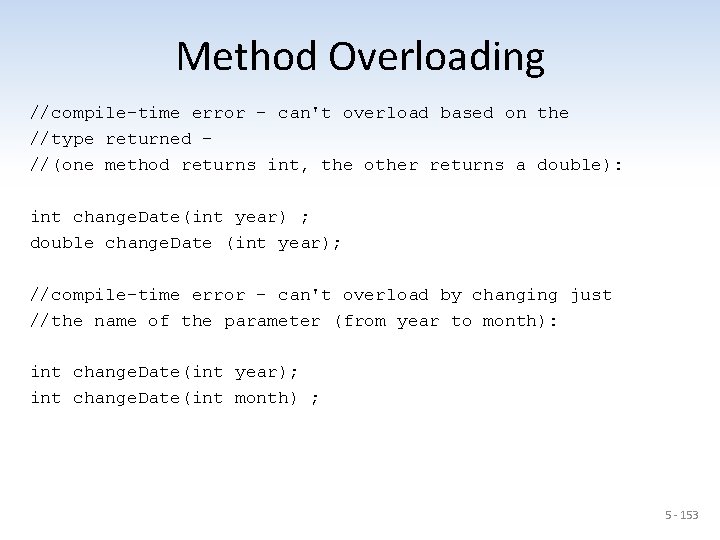
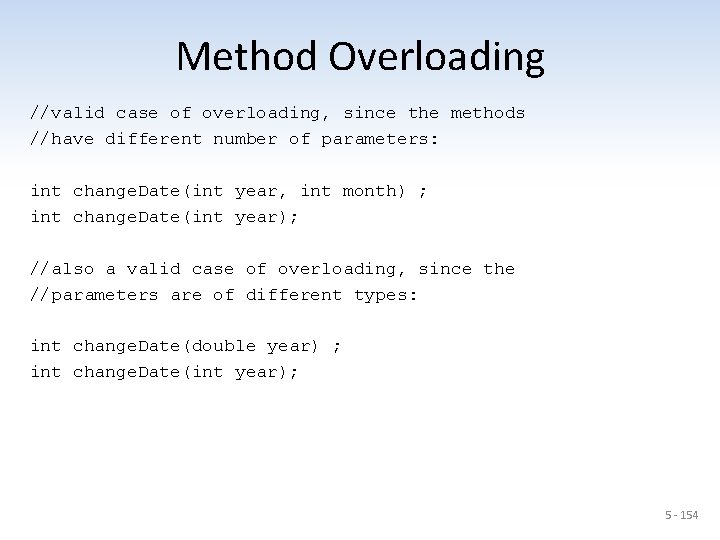
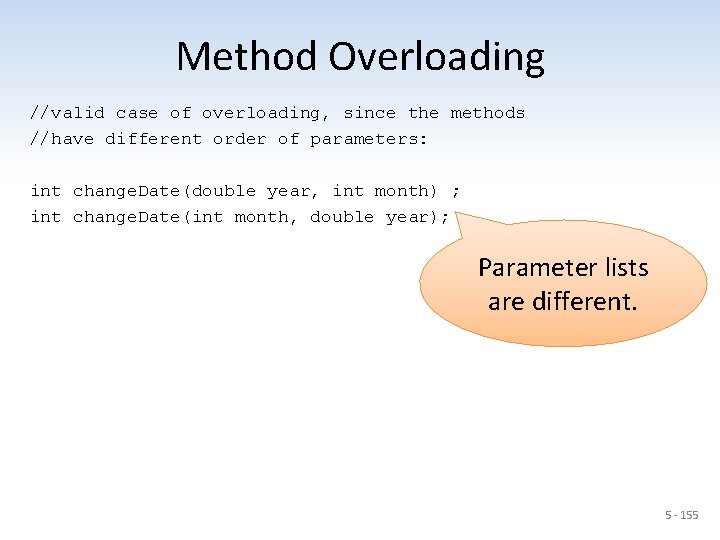
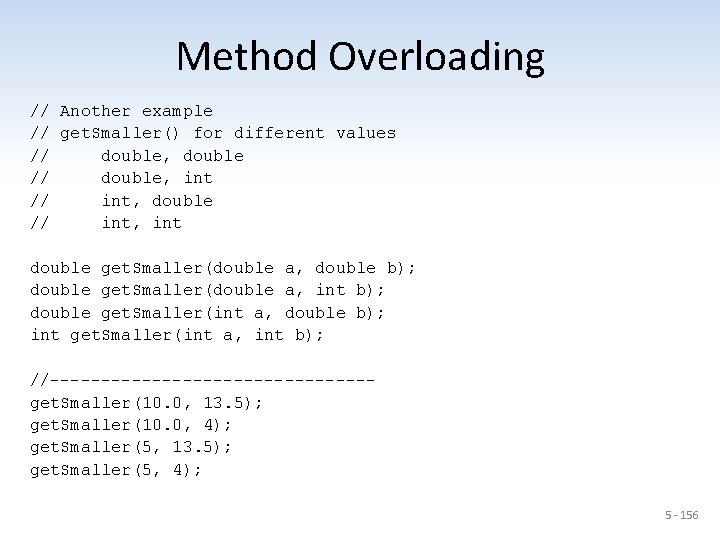
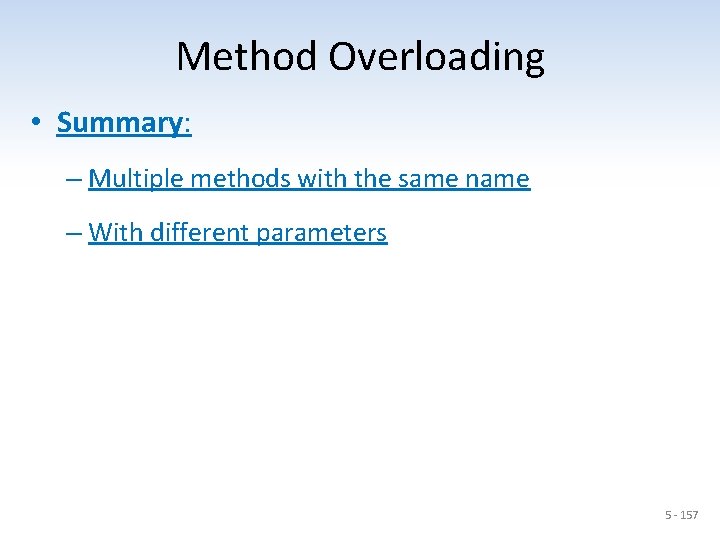
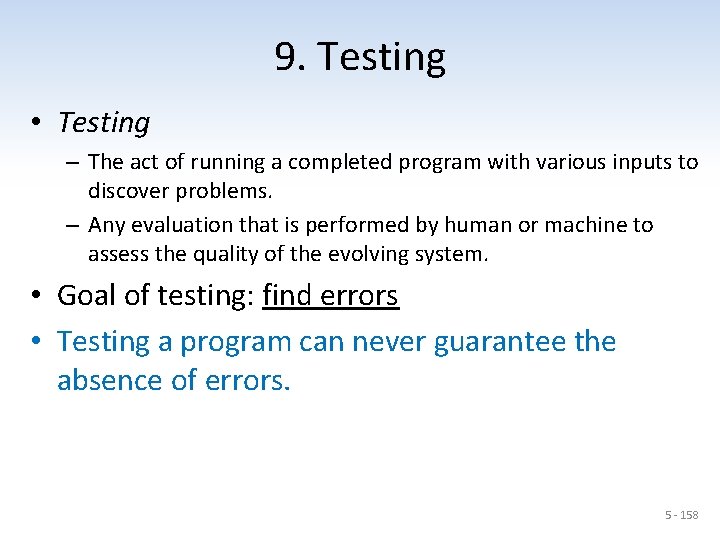
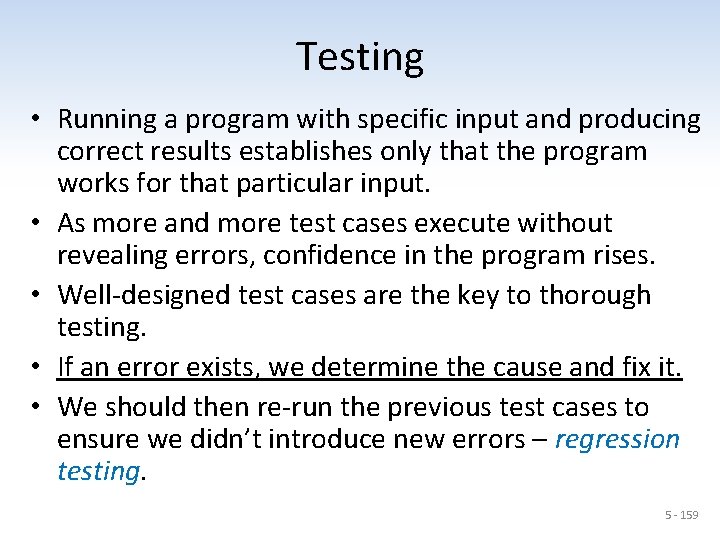
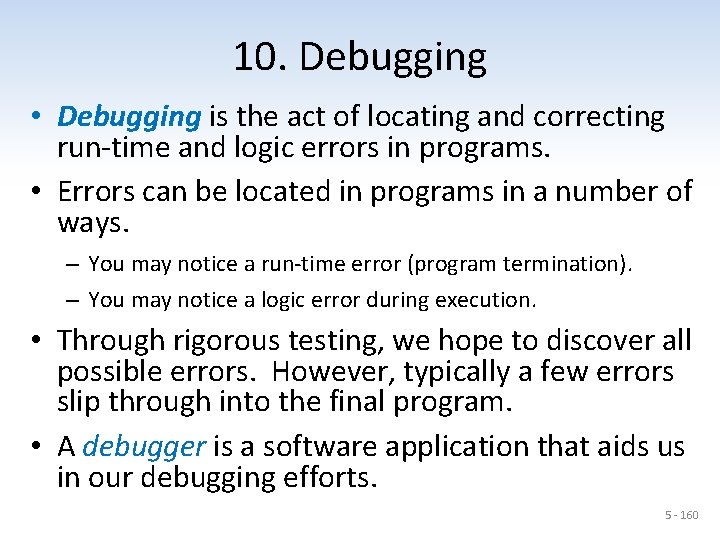
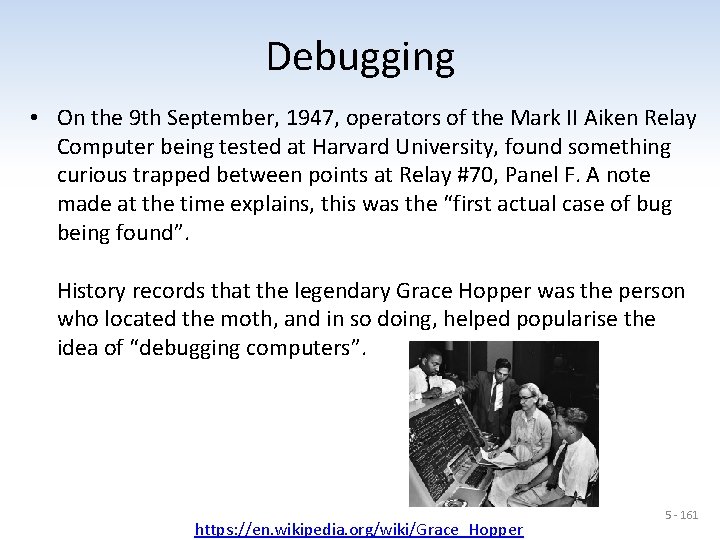
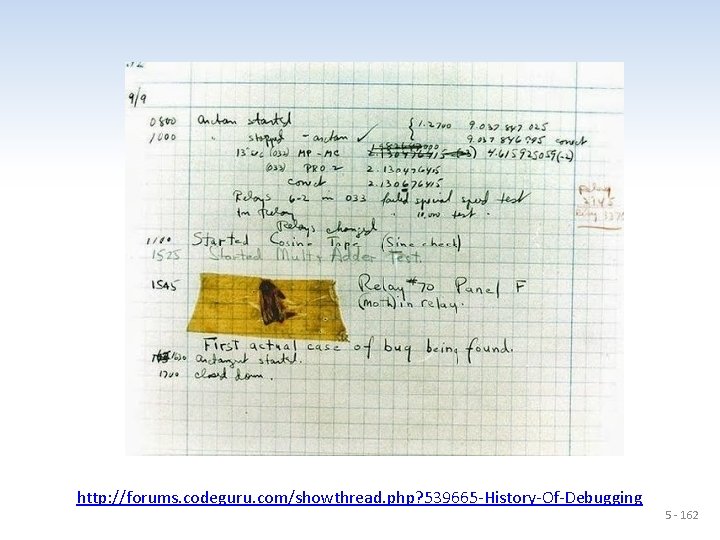
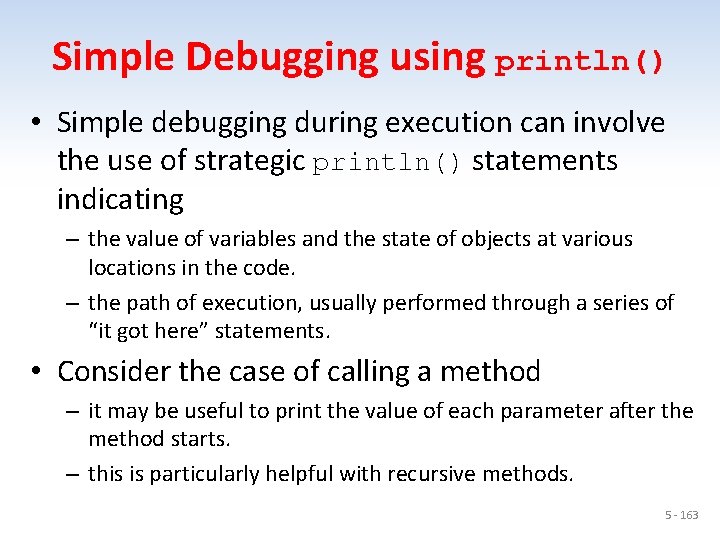
- Slides: 126
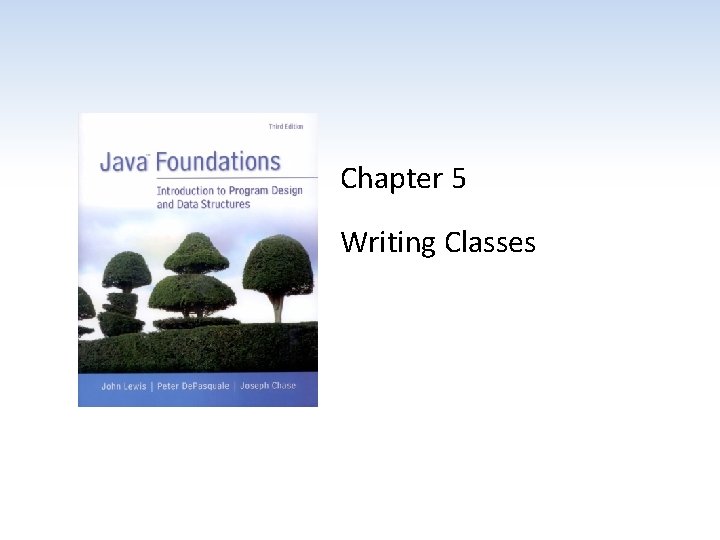
Chapter 5 Writing Classes
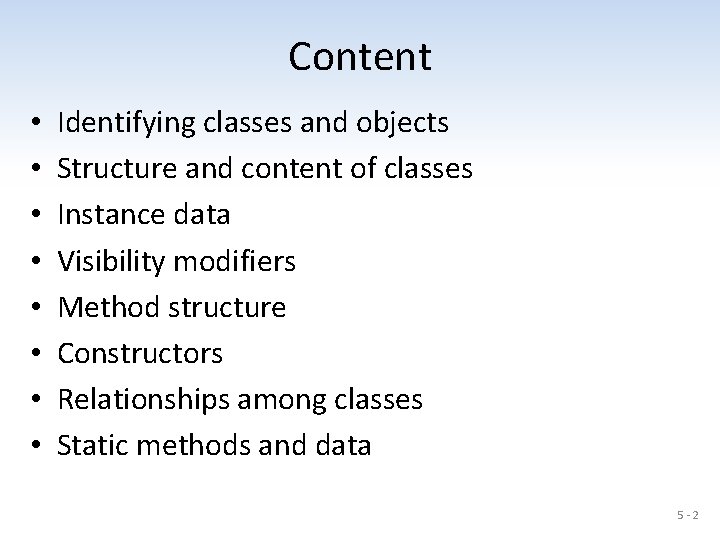
Content • • Identifying classes and objects Structure and content of classes Instance data Visibility modifiers Method structure Constructors Relationships among classes Static methods and data 5 -2
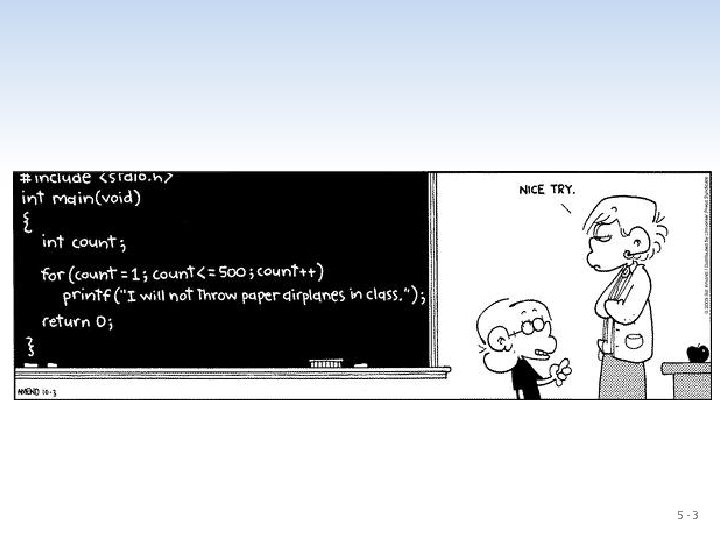
5 -3
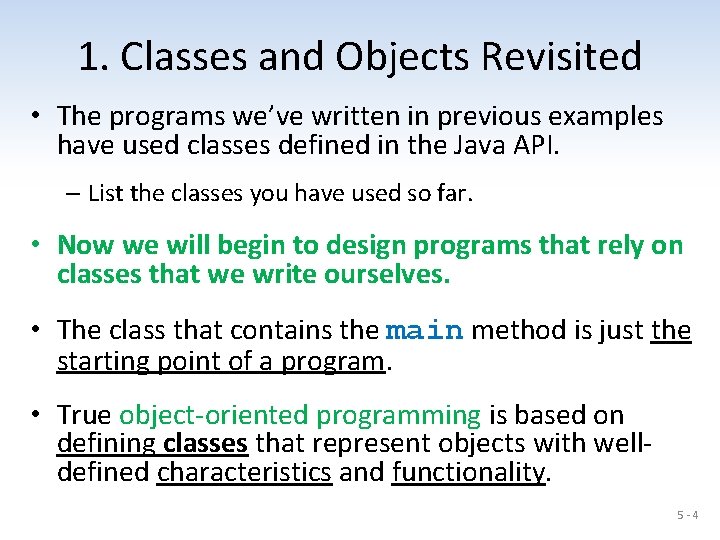
1. Classes and Objects Revisited • The programs we’ve written in previous examples have used classes defined in the Java API. – List the classes you have used so far. • Now we will begin to design programs that rely on classes that we write ourselves. • The class that contains the main method is just the starting point of a program. • True object-oriented programming is based on defining classes that represent objects with welldefined characteristics and functionality. 5 -4
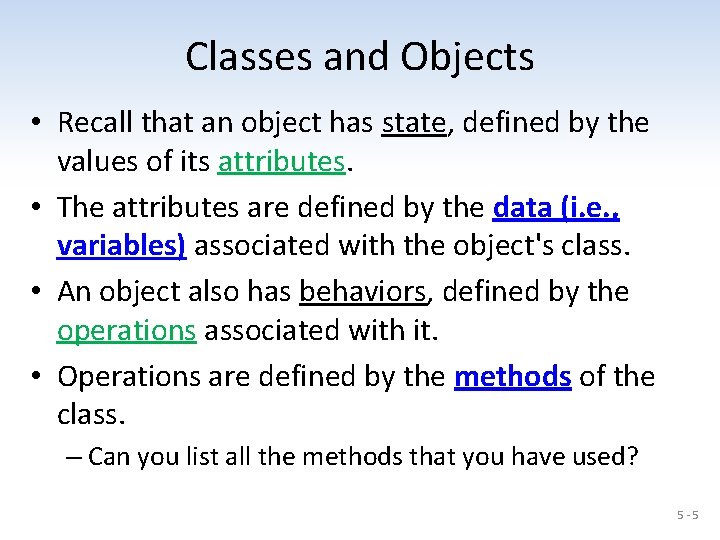
Classes and Objects • Recall that an object has state, defined by the values of its attributes. • The attributes are defined by the data (i. e. , variables) associated with the object's class. • An object also has behaviors, defined by the operations associated with it. • Operations are defined by the methods of the class. – Can you list all the methods that you have used? 5 -5
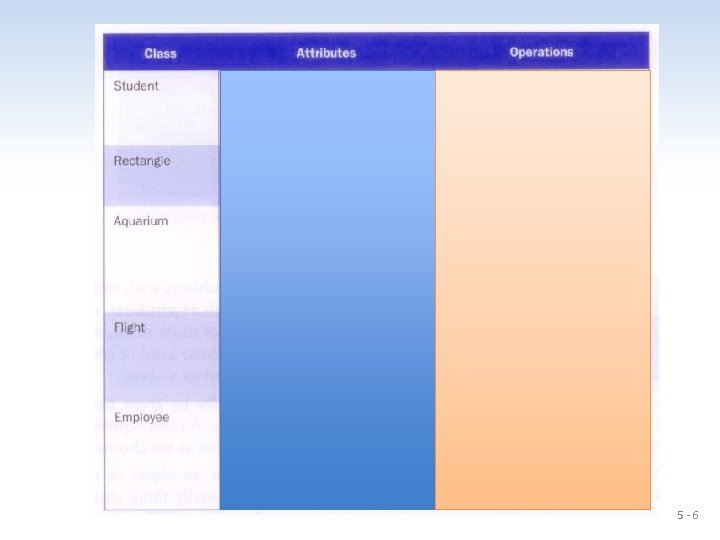
5 -6
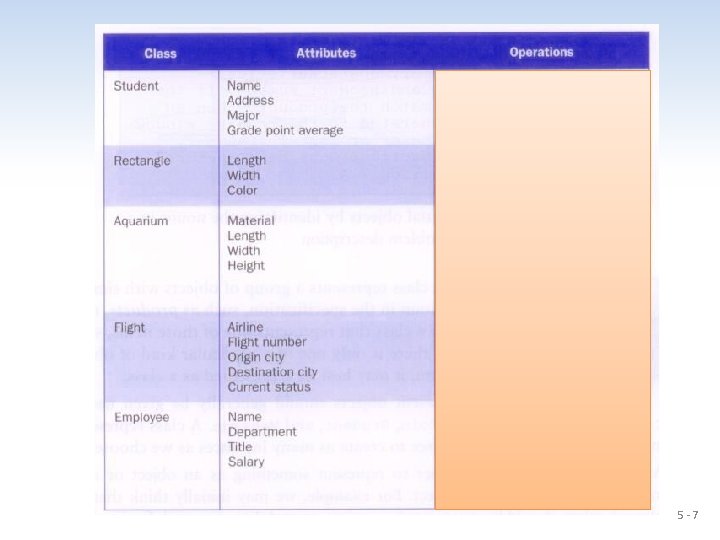
5 -7
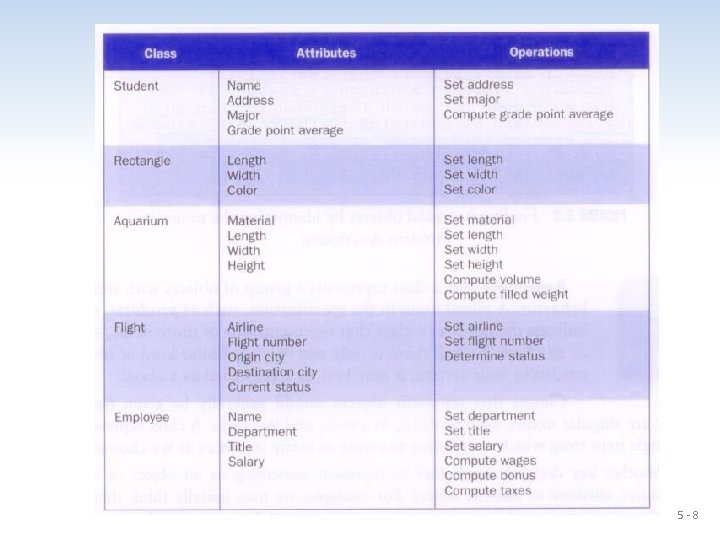
5 -8
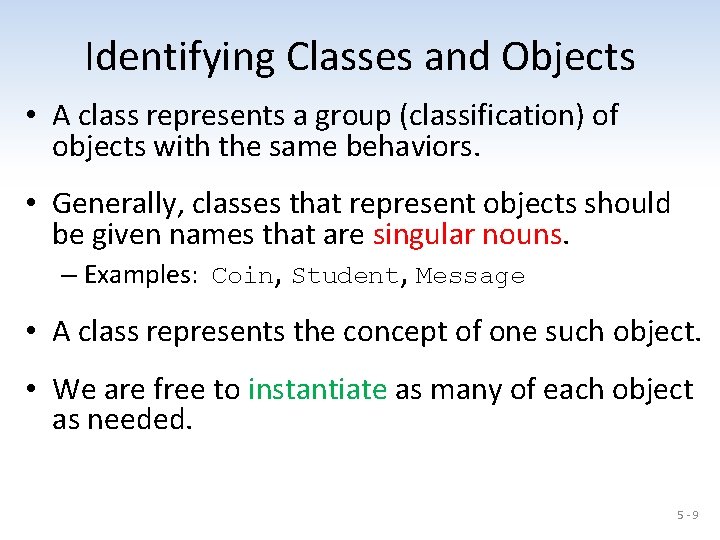
Identifying Classes and Objects • A class represents a group (classification) of objects with the same behaviors. • Generally, classes that represent objects should be given names that are singular nouns. – Examples: Coin, Student, Message • A class represents the concept of one such object. • We are free to instantiate as many of each object as needed. 5 -9
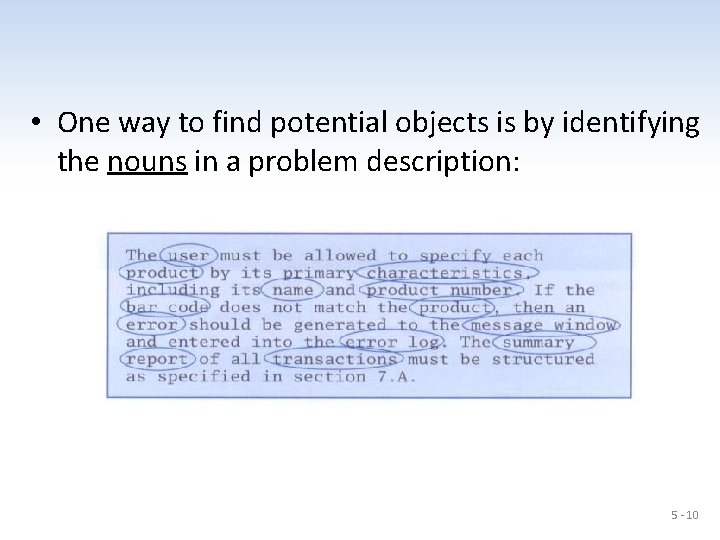
• One way to find potential objects is by identifying the nouns in a problem description: 5 - 10
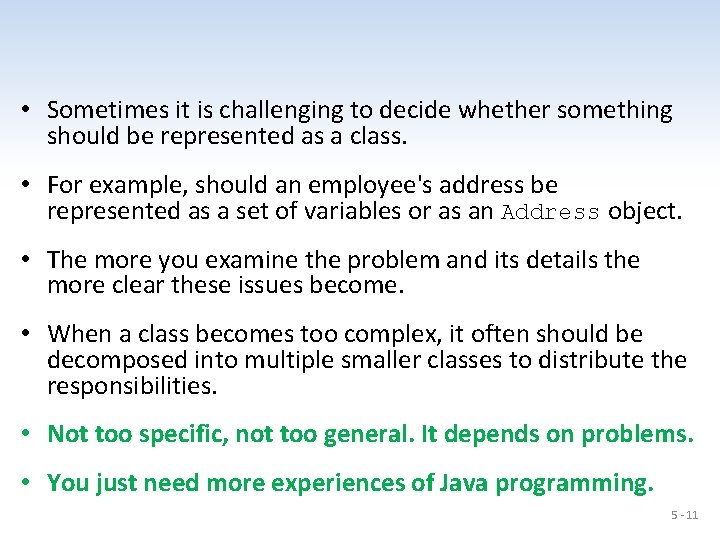
• Sometimes it is challenging to decide whether something should be represented as a class. • For example, should an employee's address be represented as a set of variables or as an Address object. • The more you examine the problem and its details the more clear these issues become. • When a class becomes too complex, it often should be decomposed into multiple smaller classes to distribute the responsibilities. • Not too specific, not too general. It depends on problems. • You just need more experiences of Java programming. 5 - 11
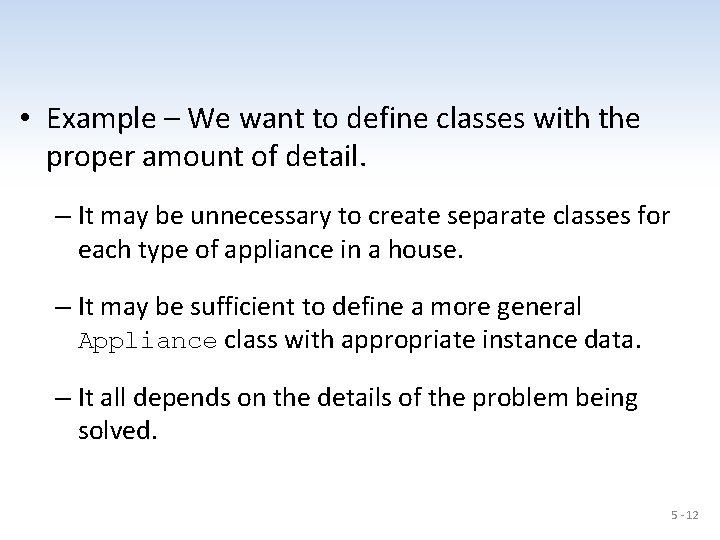
• Example – We want to define classes with the proper amount of detail. – It may be unnecessary to create separate classes for each type of appliance in a house. – It may be sufficient to define a more general Appliance class with appropriate instance data. – It all depends on the details of the problem being solved. 5 - 12
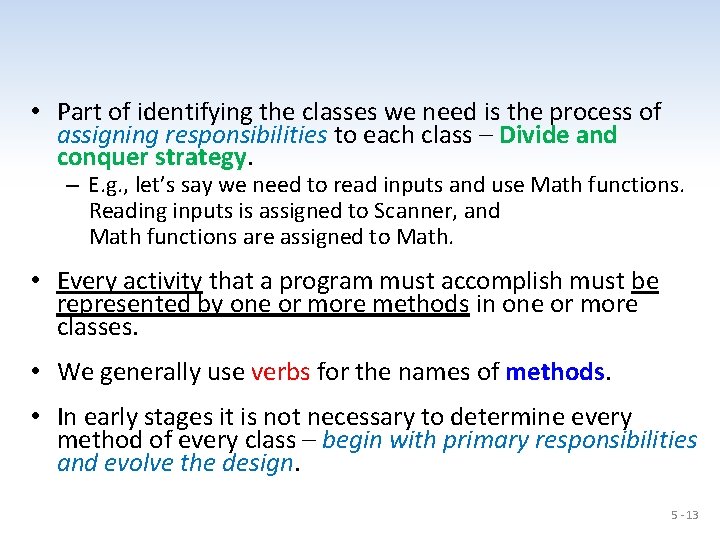
• Part of identifying the classes we need is the process of assigning responsibilities to each class – Divide and conquer strategy. – E. g. , let’s say we need to read inputs and use Math functions. Reading inputs is assigned to Scanner, and Math functions are assigned to Math. • Every activity that a program must accomplish must be represented by one or more methods in one or more classes. • We generally use verbs for the names of methods. • In early stages it is not necessary to determine every method of every class – begin with primary responsibilities and evolve the design. 5 - 13
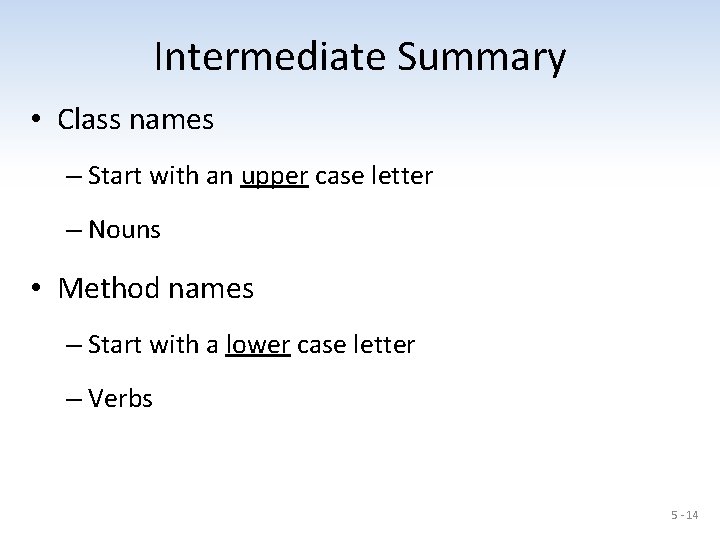
Intermediate Summary • Class names – Start with an upper case letter – Nouns • Method names – Start with a lower case letter – Verbs 5 - 14
![2 Anatomy of a Class Q What do the classes in your previous 2. Anatomy of a Class • [Q] What do the classes in your previous](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-15.jpg)
2. Anatomy of a Class • [Q] What do the classes in your previous programs include? . . . public class Calculator { public static void main(String[] args) {. . . } } 5 - 15
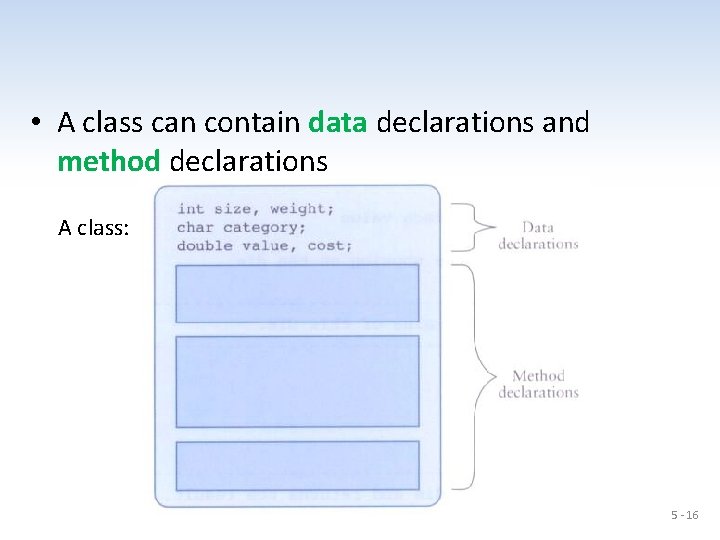
• A class can contain data declarations and method declarations A class: 5 - 16
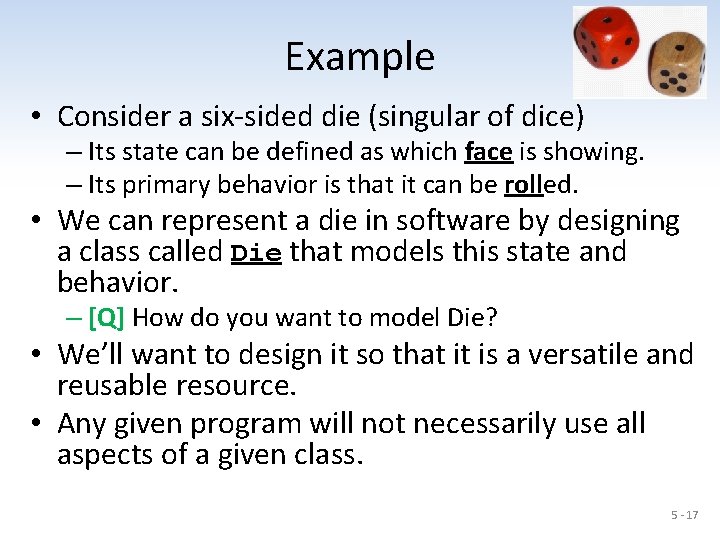
Example • Consider a six-sided die (singular of dice) – Its state can be defined as which face is showing. – Its primary behavior is that it can be rolled. • We can represent a die in software by designing a class called Die that models this state and behavior. – [Q] How do you want to model Die? • We’ll want to design it so that it is a versatile and reusable resource. • Any given program will not necessarily use all aspects of a given class. 5 - 17
![public class Snake Eyes Game of craps public static void mainString args public class Snake. Eyes // Game of craps { public static void main(String[] args)](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-18.jpg)
public class Snake. Eyes // Game of craps { public static void main(String[] args) { final int ROLLS = 500; int num 1, num 2, count = 0; Die die 1 = new Die(); Die die 2 = new Die(); Try with http: //cs. tru. ca/~mlee/comp 1130/Fal l 2016/5. chapter 5/Snake. Eyes. java. Any error? // What is the class Die? for (int roll=0; roll < ROLLS; roll++) { num 1 = die 1. roll(); // What is roll()? num 2 = die 2. roll(); if (num 1 == 1 && num 2 == 1) count++; This class has only main(). // check for snake eyes } System. out. println("Number of rolls: " + ROLLS); System. out. println("Number of snake eyes: " + count); System. out. println("Ratio: " + (double)count / ROLLS); } } 5 - 18
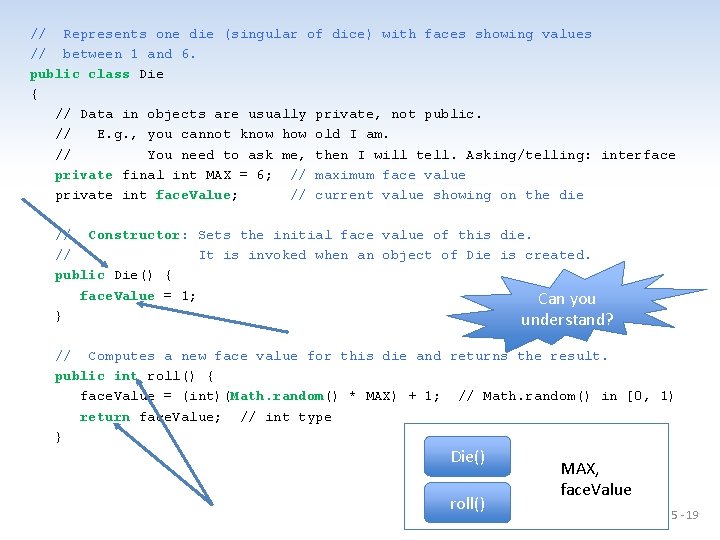
// Represents one die (singular of dice) with faces showing values // between 1 and 6. public class Die { // Data in objects are usually private, not public. // E. g. , you cannot know how old I am. // You need to ask me, then I will tell. Asking/telling: interface private final int MAX = 6; // maximum face value private int face. Value; // current value showing on the die // Constructor: Sets the initial face value of this die. // It is invoked when an object of Die is created. public Die() { face. Value = 1; Can you } understand? // Computes a new face value for this die and returns the result. public int roll() { face. Value = (int)(Math. random() * MAX) + 1; // Math. random() in [0, 1) return face. Value; // int type } Die() roll() MAX, face. Value 5 - 19
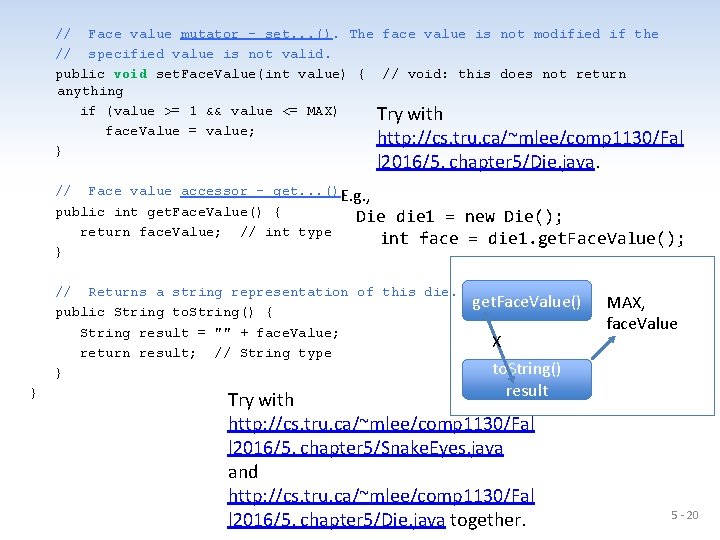
// Face value mutator – set. . . (). The face value is not modified if the // specified value is not valid. public void set. Face. Value(int value) { // void: this does not return anything if (value >= 1 && value <= MAX) Try with face. Value = value; http: //cs. tru. ca/~mlee/comp 1130/Fal } l 2016/5. chapter 5/Die. java. // Face value accessor – get. . . (). E. g. , public int get. Face. Value() { Die die 1 = return face. Value; // int type int face } // Returns a string representation of this die. public String to. String() { String result = "" + face. Value; return result; // String type } } new Die(); = die 1. get. Face. Value(); get. Face. Value() X MAX, face. Value to. String() result Try with http: //cs. tru. ca/~mlee/comp 1130/Fal l 2016/5. chapter 5/Snake. Eyes. java and http: //cs. tru. ca/~mlee/comp 1130/Fal l 2016/5. chapter 5/Die. java together. 5 - 20
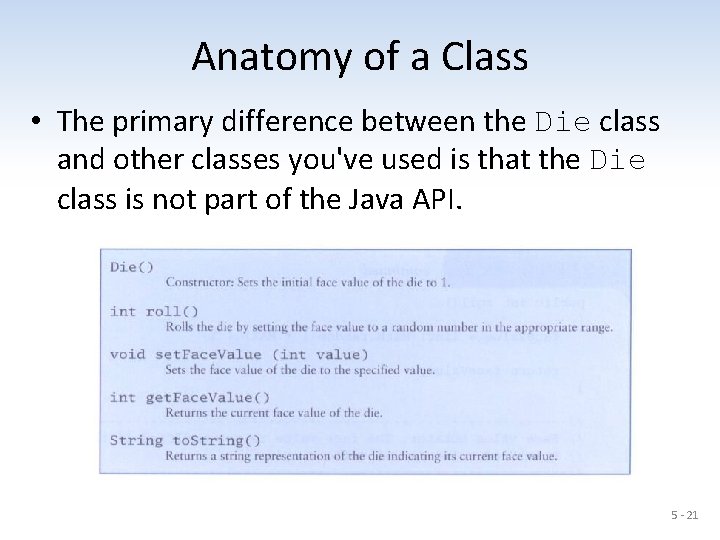
Anatomy of a Class • The primary difference between the Die class and other classes you've used is that the Die class is not part of the Java API. 5 - 21
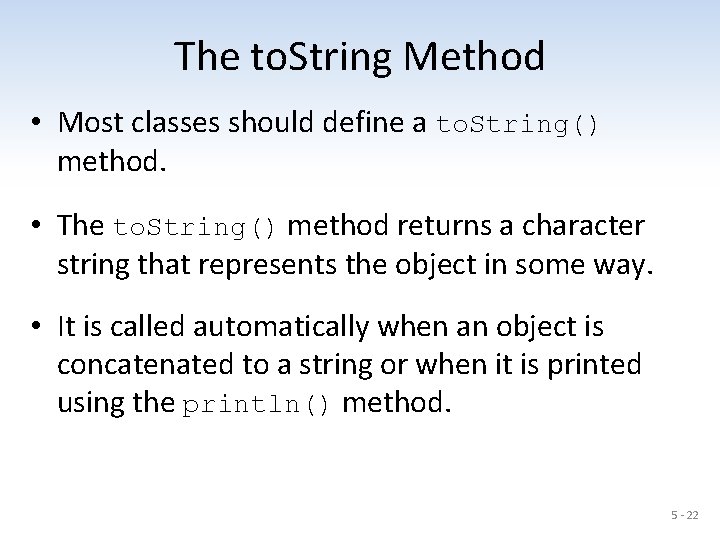
The to. String Method • Most classes should define a to. String() method. • The to. String() method returns a character string that represents the object in some way. • It is called automatically when an object is concatenated to a string or when it is printed using the println() method. 5 - 22
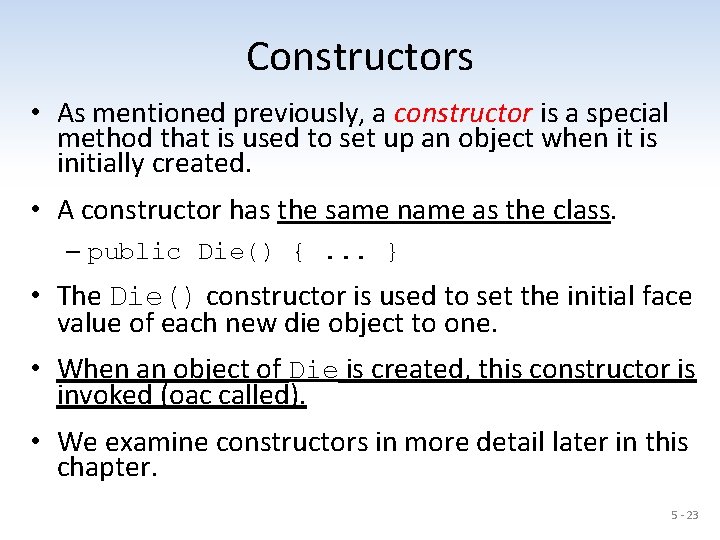
Constructors • As mentioned previously, a constructor is a special method that is used to set up an object when it is initially created. • A constructor has the same name as the class. – public Die() {. . . } • The Die() constructor is used to set the initial face value of each new die object to one. • When an object of Die is created, this constructor is invoked (oac called). • We examine constructors in more detail later in this chapter. 5 - 23
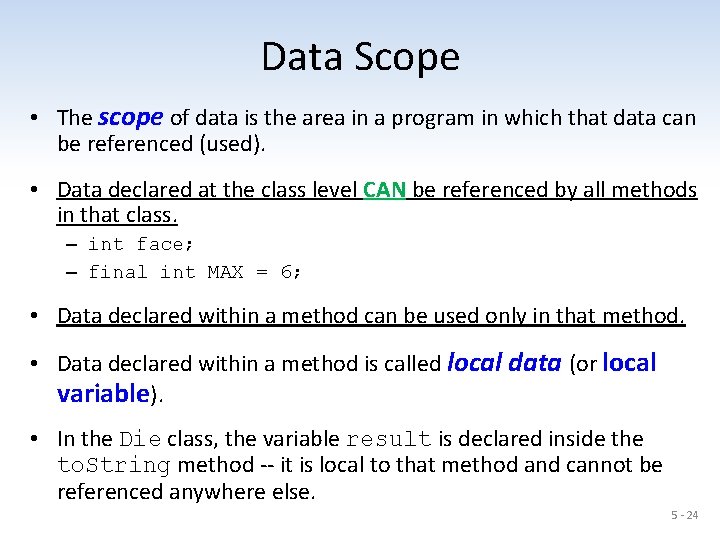
Data Scope • The scope of data is the area in a program in which that data can be referenced (used). • Data declared at the class level CAN be referenced by all methods in that class. – int face; – final int MAX = 6; • Data declared within a method can be used only in that method. • Data declared within a method is called local data (or local variable). • In the Die class, the variable result is declared inside the to. String method -- it is local to that method and cannot be referenced anywhere else. 5 - 24
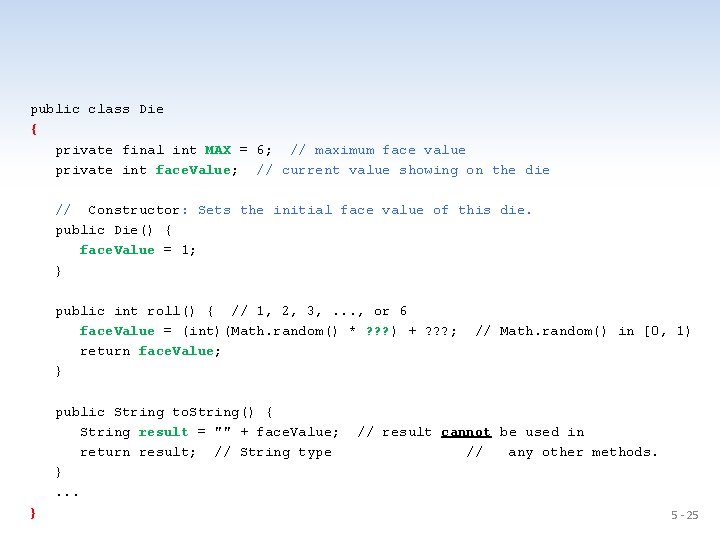
public class Die { private final int MAX = 6; // maximum face value private int face. Value; // current value showing on the die // Constructor: Sets the initial face value of this die. public Die() { face. Value = 1; } public int roll() { // 1, 2, 3, . . . , or 6 face. Value = (int)(Math. random() * ? ? ? ) + ? ? ? ; return face. Value; } public String to. String() { String result = "" + face. Value; return result; // String type }. . . } // Math. random() in [0, 1) // result cannot be used in // any other methods. 5 - 25
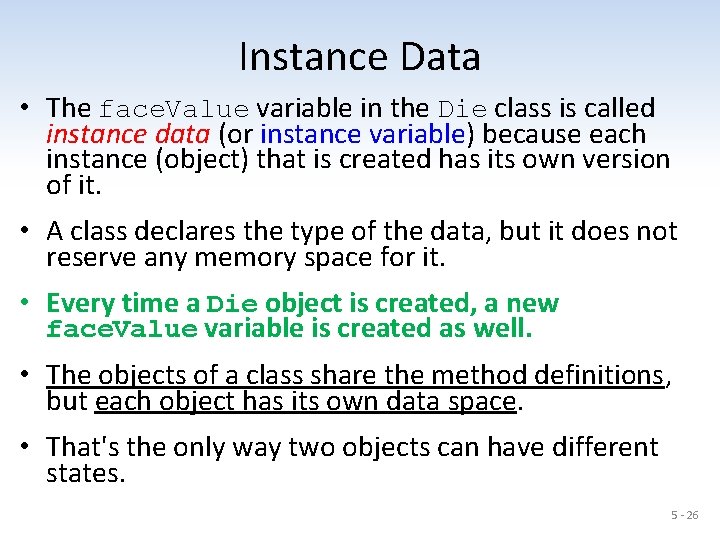
Instance Data • The face. Value variable in the Die class is called instance data (or instance variable) because each instance (object) that is created has its own version of it. • A class declares the type of the data, but it does not reserve any memory space for it. • Every time a Die object is created, a new face. Value variable is created as well. • The objects of a class share the method definitions, but each object has its own data space. • That's the only way two objects can have different states. 5 - 26
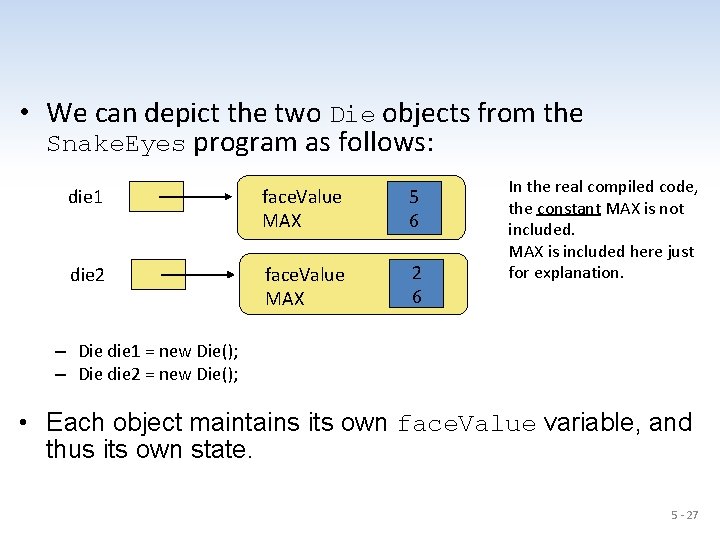
• We can depict the two Die objects from the Snake. Eyes program as follows: die 1 face. Value MAX 5 6 die 2 face. Value MAX 2 6 In the real compiled code, the constant MAX is not included. MAX is included here just for explanation. – Die die 1 = new Die(); – Die die 2 = new Die(); • Each object maintains its own face. Value variable, and thus its own state. 5 - 27
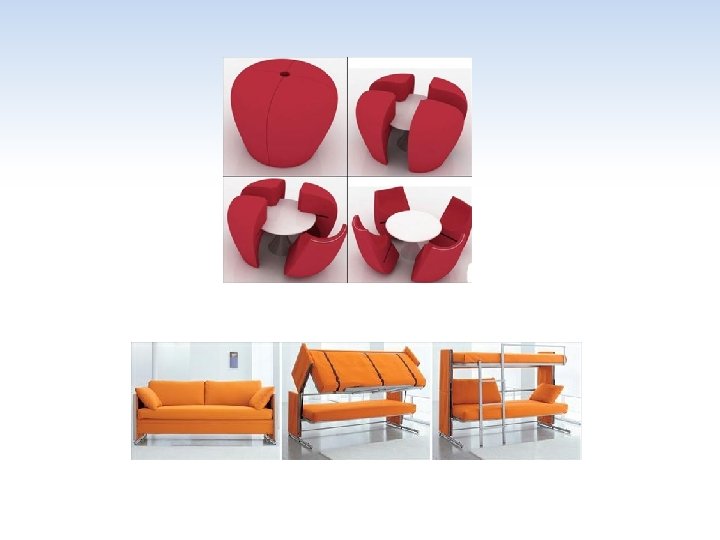
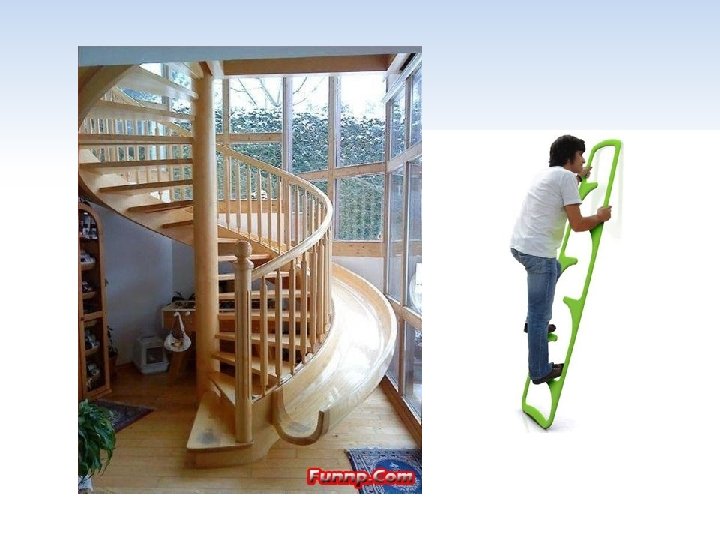
![UML Diagrams Q Any good idea to describemodel a class before you start UML Diagrams • [Q] Any good idea to describe/model a class before you start](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-30.jpg)
UML Diagrams • [Q] Any good idea to describe/model a class before you start coding? • [Q] What do you need to decide? – Instance variables (i. e. , data) with their purposes – Methods with their responsibilities 5 - 30
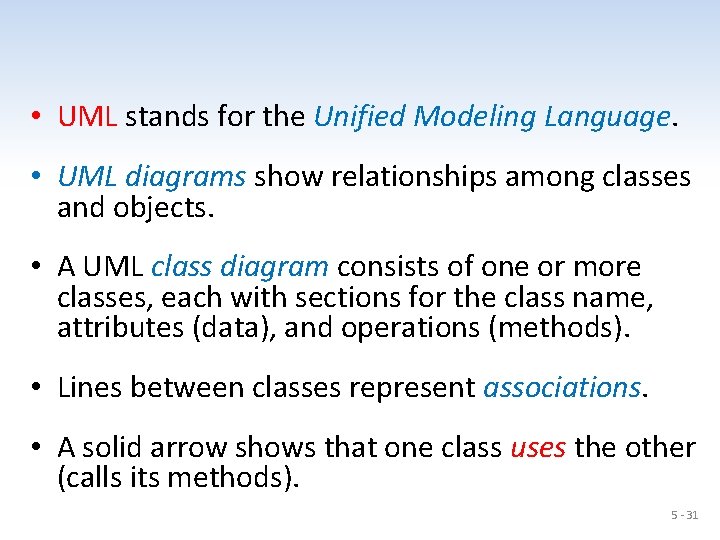
• UML stands for the Unified Modeling Language. • UML diagrams show relationships among classes and objects. • A UML class diagram consists of one or more classes, each with sections for the class name, attributes (data), and operations (methods). • Lines between classes represent associations. • A solid arrow shows that one class uses the other (calls its methods). 5 - 31
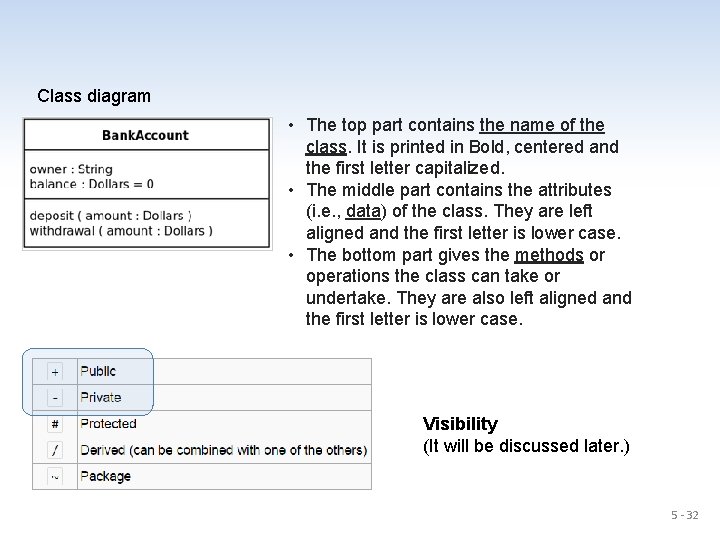
Class diagram • The top part contains the name of the class. It is printed in Bold, centered and the first letter capitalized. • The middle part contains the attributes (i. e. , data) of the class. They are left aligned and the first letter is lower case. • The bottom part gives the methods or operations the class can take or undertake. They are also left aligned and the first letter is lower case. Visibility (It will be discussed later. ) 5 - 32
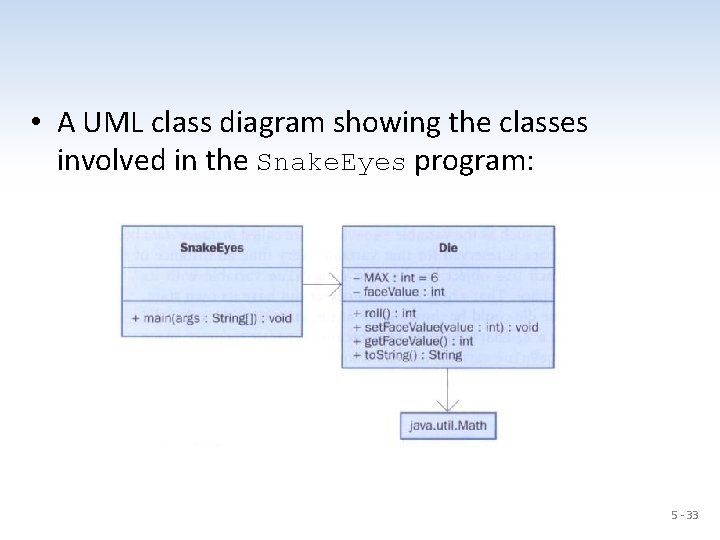
• A UML class diagram showing the classes involved in the Snake. Eyes program: 5 - 33
![public class Snake Eyes public static void mainString args final int ROLLS public class Snake. Eyes { public static void main(String[] args) { final int ROLLS](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-34.jpg)
public class Snake. Eyes { public static void main(String[] args) { final int ROLLS = 500; int num 1, num 2, count = 0; Die die 1 = new Die(); Die die 2 = new Die(); // What is Die here? for (int roll=1; roll <= ROLLS; roll++) { num 1 = die 1. roll(); // What is roll()? num 2 = die 2. roll(); if (num 1 == 1 && num 2 == 1) count++; // check for snake eyes } System. out. println("Number of rolls: " + ROLLS); System. out. println("Number of snake eyes: " + count); System. out. println("Ratio: " + (double)count / ROLLS); } } 5 - 34
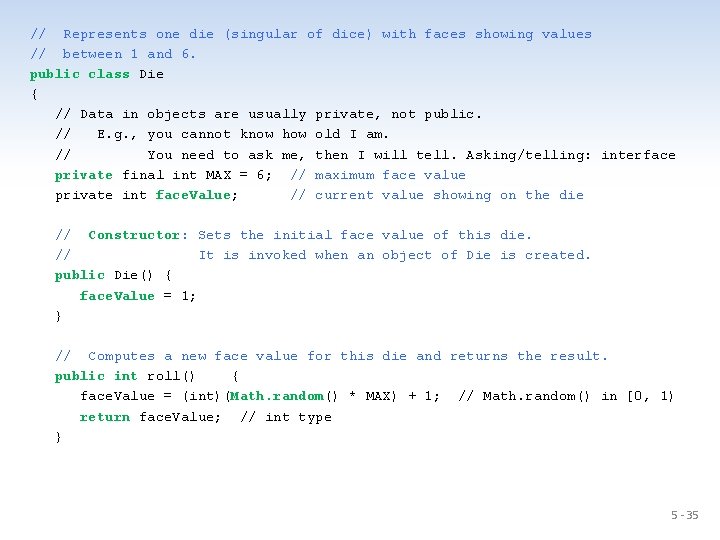
// Represents one die (singular of dice) with faces showing values // between 1 and 6. public class Die { // Data in objects are usually private, not public. // E. g. , you cannot know how old I am. // You need to ask me, then I will tell. Asking/telling: interface private final int MAX = 6; // maximum face value private int face. Value; // current value showing on the die // Constructor: Sets the initial face value of this die. // It is invoked when an object of Die is created. public Die() { face. Value = 1; } // Computes a new face value for this die and returns the result. public int roll() { face. Value = (int)(Math. random() * MAX) + 1; // Math. random() in [0, 1) return face. Value; // int type } 5 - 35
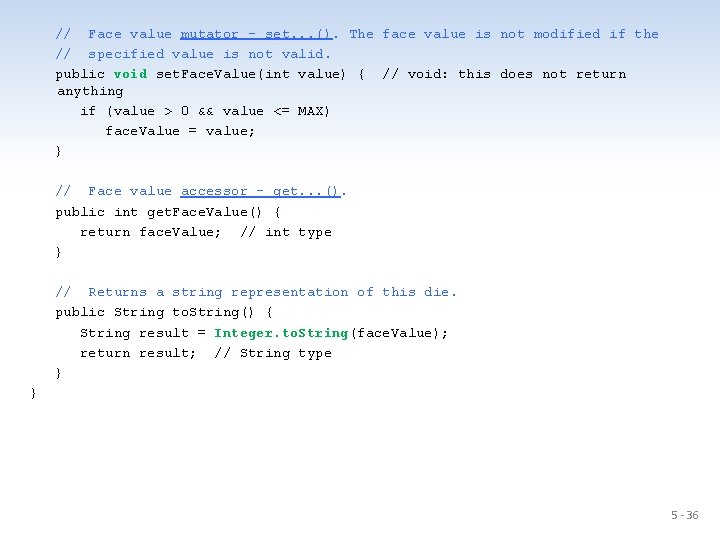
// Face value mutator – set. . . (). The face value is not modified if the // specified value is not valid. public void set. Face. Value(int value) { // void: this does not return anything if (value > 0 && value <= MAX) face. Value = value; } // Face value accessor – get. . . (). public int get. Face. Value() { return face. Value; // int type } // Returns a string representation of this die. public String to. String() { String result = Integer. to. String(face. Value); return result; // String type } } 5 - 36
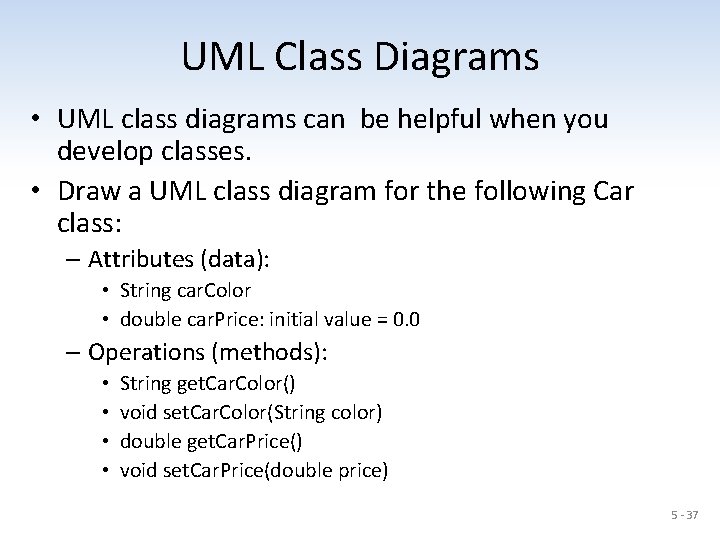
UML Class Diagrams • UML class diagrams can be helpful when you develop classes. • Draw a UML class diagram for the following Car class: – Attributes (data): • String car. Color • double car. Price: initial value = 0. 0 – Operations (methods): • • String get. Car. Color() void set. Car. Color(String color) double get. Car. Price() void set. Car. Price(double price) 5 - 37
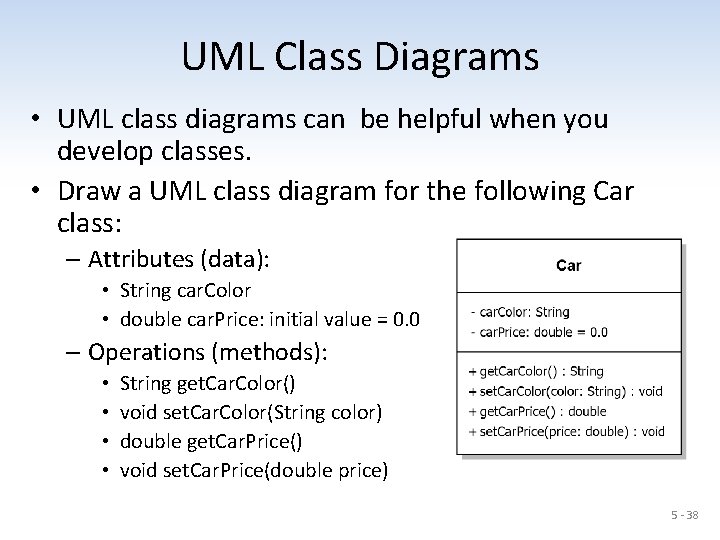
UML Class Diagrams • UML class diagrams can be helpful when you develop classes. • Draw a UML class diagram for the following Car class: – Attributes (data): • String car. Color • double car. Price: initial value = 0. 0 – Operations (methods): • • String get. Car. Color() void set. Car. Color(String color) double get. Car. Price() void set. Car. Price(double price) 5 - 38
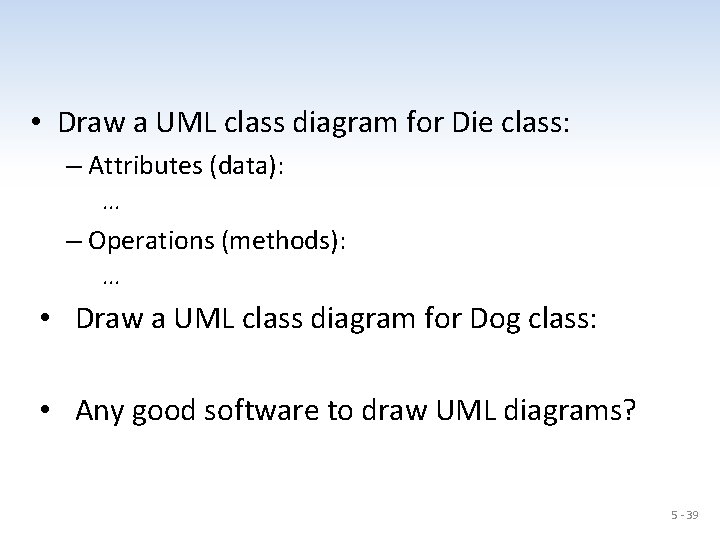
• Draw a UML class diagram for Die class: – Attributes (data): . . . – Operations (methods): . . . • Draw a UML class diagram for Dog class: • Any good software to draw UML diagrams? 5 - 39
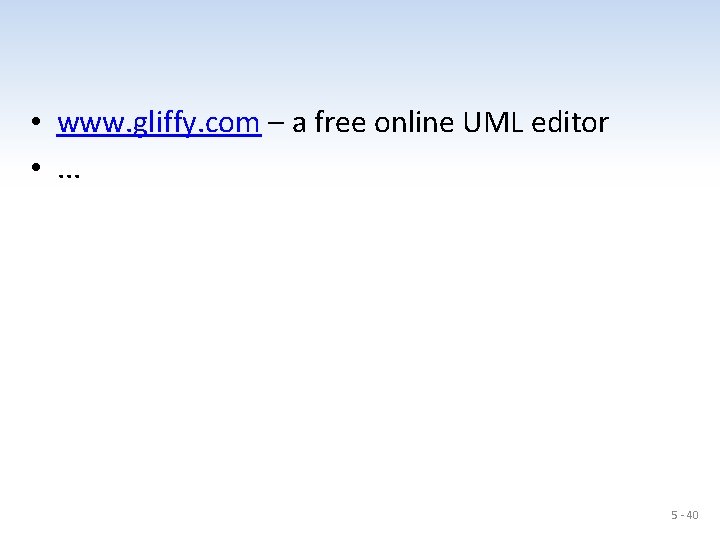
• www. gliffy. com – a free online UML editor • . . . 5 - 40
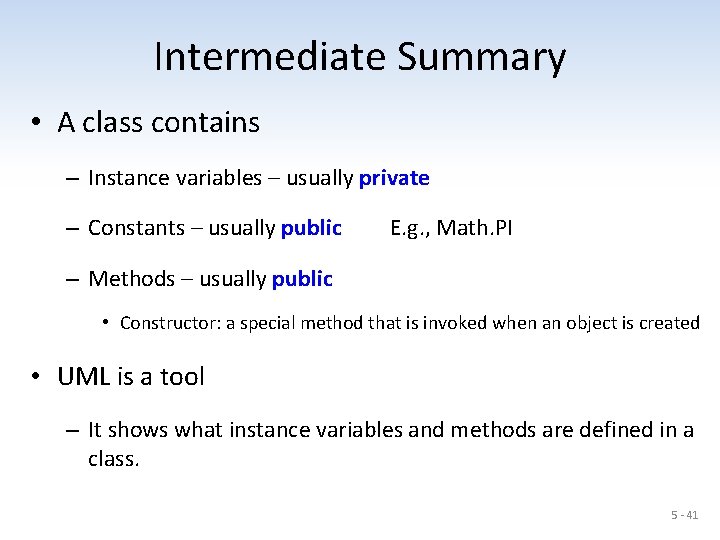
Intermediate Summary • A class contains – Instance variables – usually private – Constants – usually public E. g. , Math. PI – Methods – usually public • Constructor: a special method that is invoked when an object is created • UML is a tool – It shows what instance variables and methods are defined in a class. 5 - 41
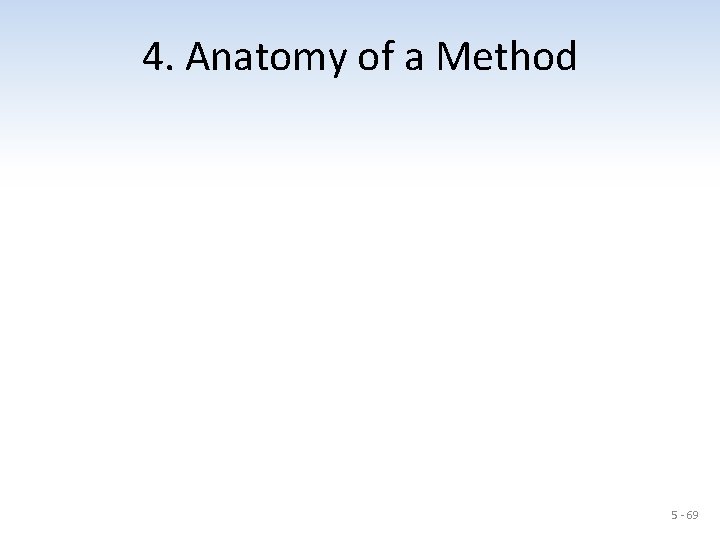
4. Anatomy of a Method 5 - 69
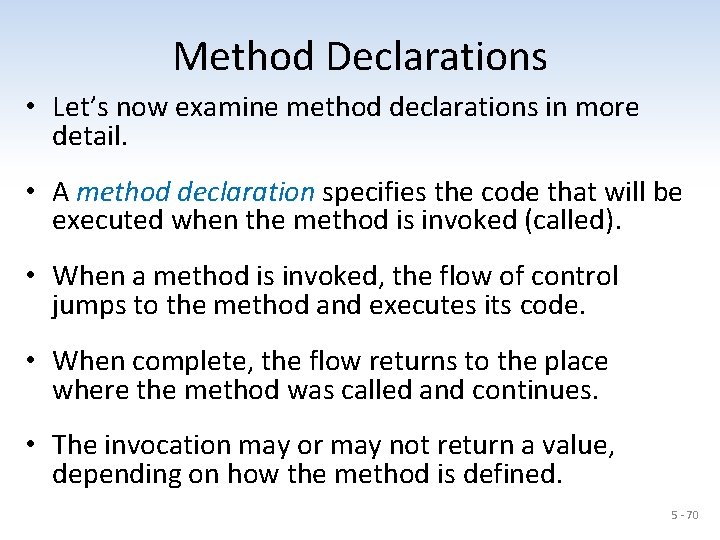
Method Declarations • Let’s now examine method declarations in more detail. • A method declaration specifies the code that will be executed when the method is invoked (called). • When a method is invoked, the flow of control jumps to the method and executes its code. • When complete, the flow returns to the place where the method was called and continues. • The invocation may or may not return a value, depending on how the method is defined. 5 - 70
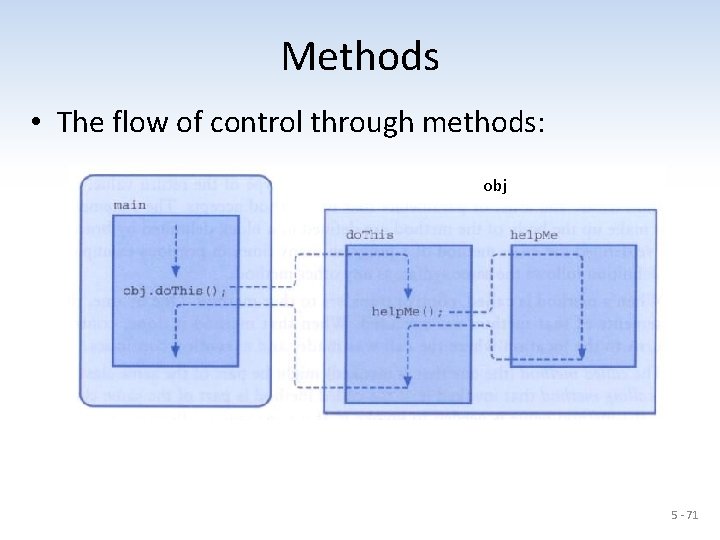
Methods • The flow of control through methods: obj 5 - 71
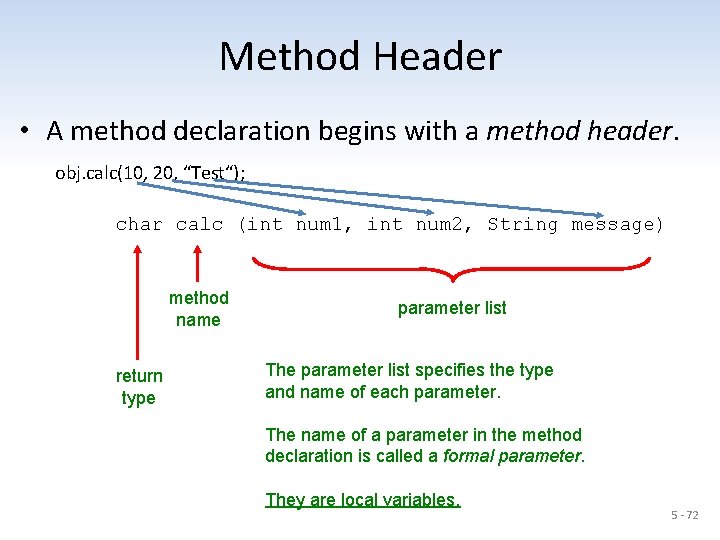
Method Header • A method declaration begins with a method header. obj. calc(10, 20, “Test“); char calc (int num 1, int num 2, String message) method name return type parameter list The parameter list specifies the type and name of each parameter. The name of a parameter in the method declaration is called a formal parameter. They are local variables. 5 - 72
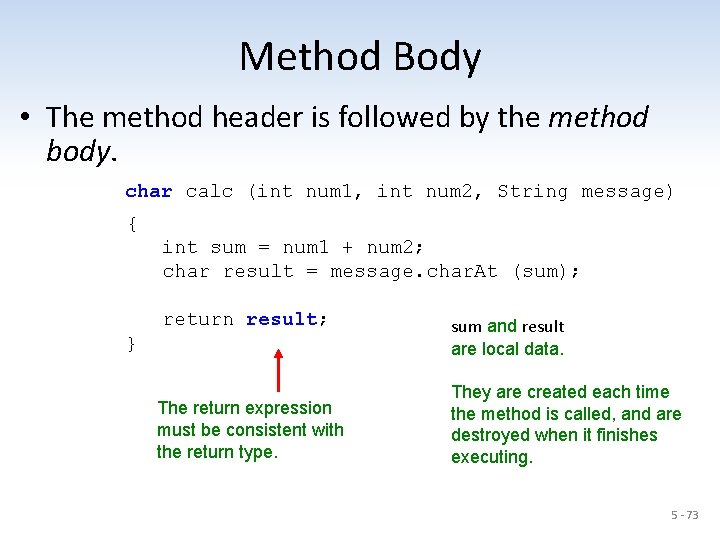
Method Body • The method header is followed by the method body. char calc (int num 1, int num 2, String message) { int sum = num 1 + num 2; char result = message. char. At (sum); return result; } The return expression must be consistent with the return type. sum and result are local data. They are created each time the method is called, and are destroyed when it finishes executing. 5 - 73
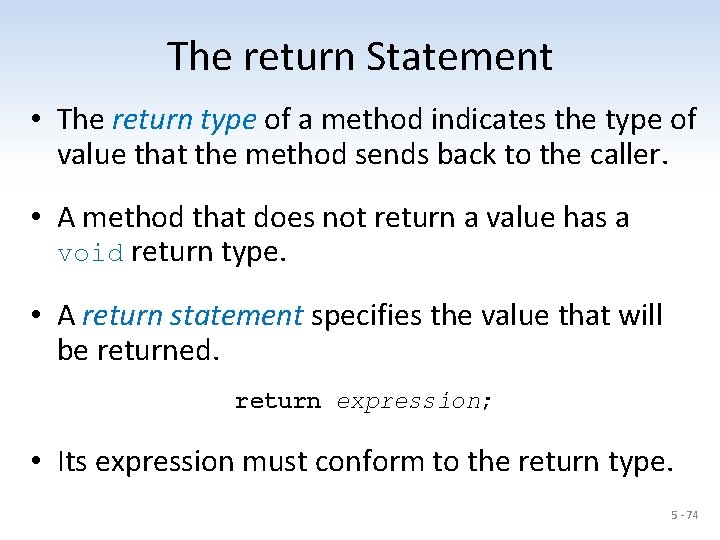
The return Statement • The return type of a method indicates the type of value that the method sends back to the caller. • A method that does not return a value has a void return type. • A return statement specifies the value that will be returned. return expression; • Its expression must conform to the return type. 5 - 74
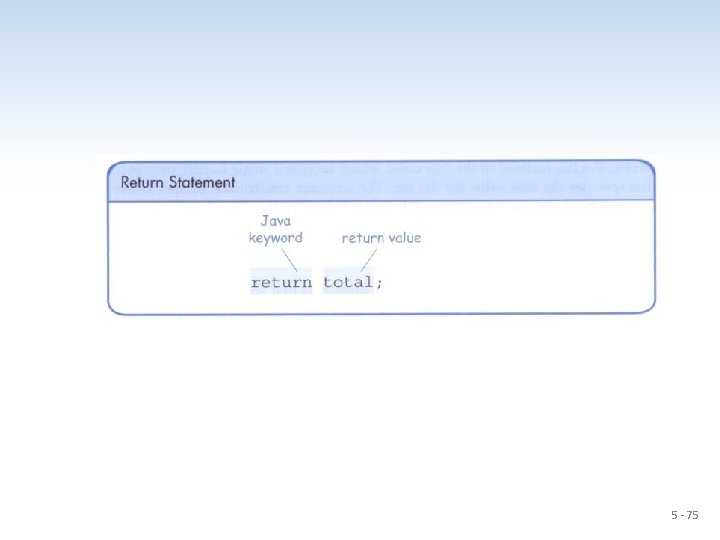
5 - 75
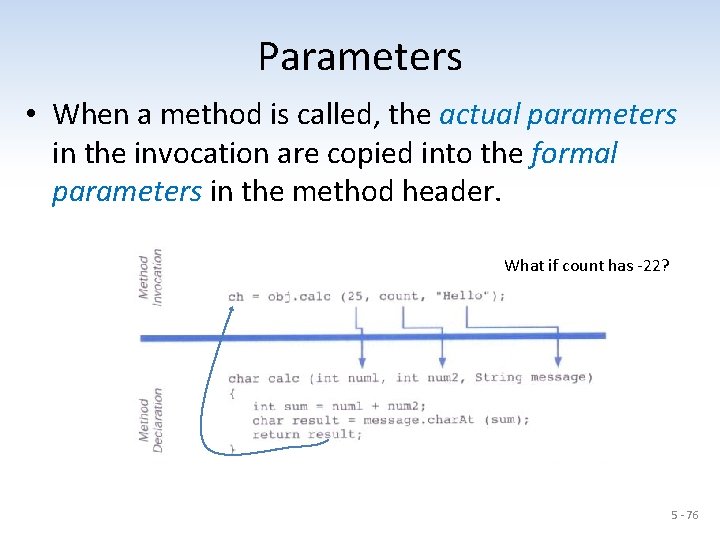
Parameters • When a method is called, the actual parameters in the invocation are copied into the formal parameters in the method header. What if count has -22? 5 - 76
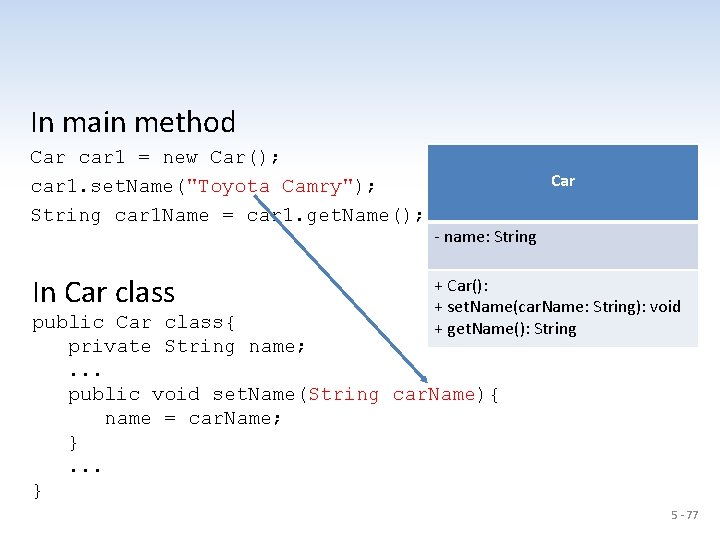
In main method Car car 1 = new Car(); car 1. set. Name("Toyota Camry"); String car 1 Name = car 1. get. Name(); In Car class Car - name: String + Car(): + set. Name(car. Name: String): void + get. Name(): String public Car class{ private String name; . . . public void set. Name(String car. Name){ name = car. Name; }. . . } 5 - 77
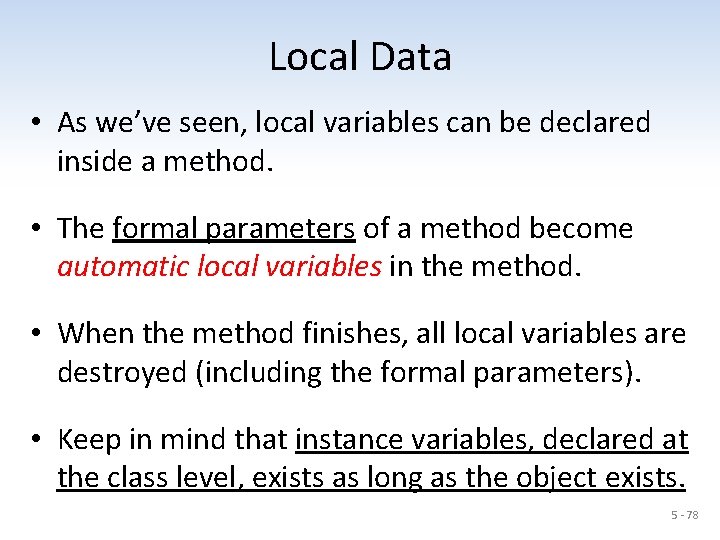
Local Data • As we’ve seen, local variables can be declared inside a method. • The formal parameters of a method become automatic local variables in the method. • When the method finishes, all local variables are destroyed (including the formal parameters). • Keep in mind that instance variables, declared at the class level, exists as long as the object exists. 5 - 78
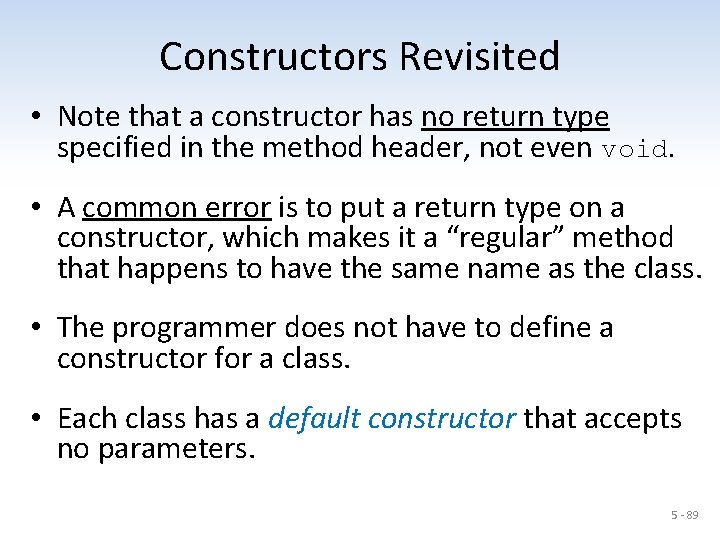
Constructors Revisited • Note that a constructor has no return type specified in the method header, not even void. • A common error is to put a return type on a constructor, which makes it a “regular” method that happens to have the same name as the class. • The programmer does not have to define a constructor for a class. • Each class has a default constructor that accepts no parameters. 5 - 89
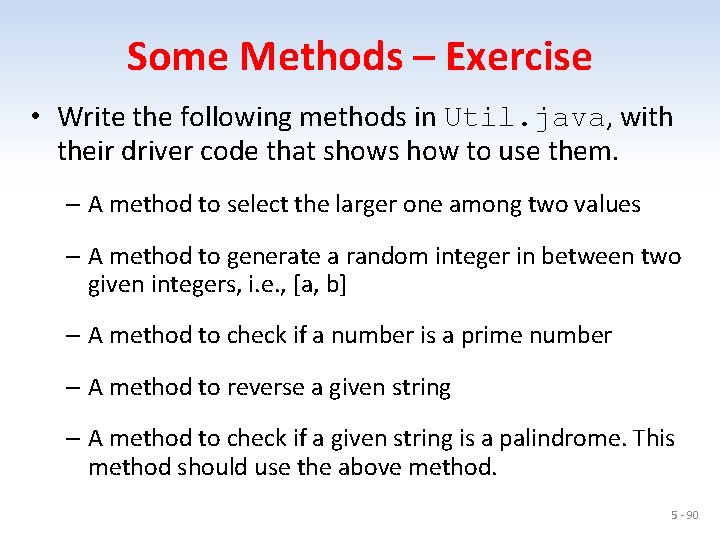
Some Methods – Exercise • Write the following methods in Util. java, with their driver code that shows how to use them. – A method to select the larger one among two values – A method to generate a random integer in between two given integers, i. e. , [a, b] – A method to check if a number is a prime number – A method to reverse a given string – A method to check if a given string is a palindrome. This method should use the above method. 5 - 90
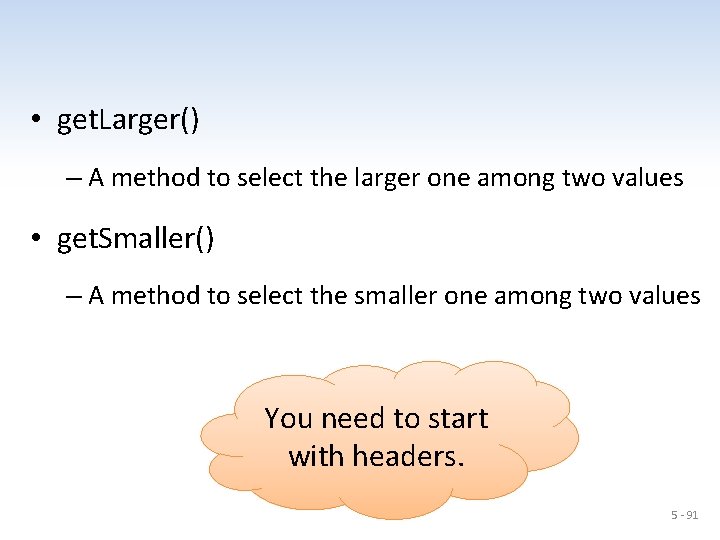
• get. Larger() – A method to select the larger one among two values • get. Smaller() – A method to select the smaller one among two values You need to start with headers. 5 - 91
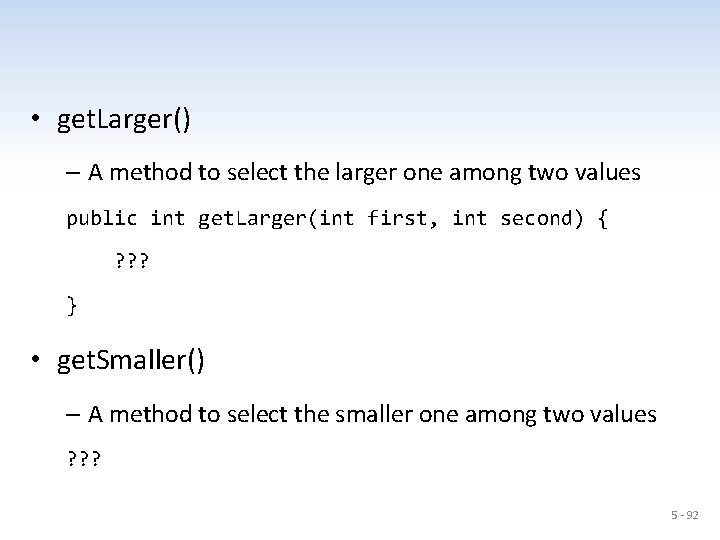
• get. Larger() – A method to select the larger one among two values public int get. Larger(int first, int second) { ? ? ? } • get. Smaller() – A method to select the smaller one among two values ? ? ? 5 - 92
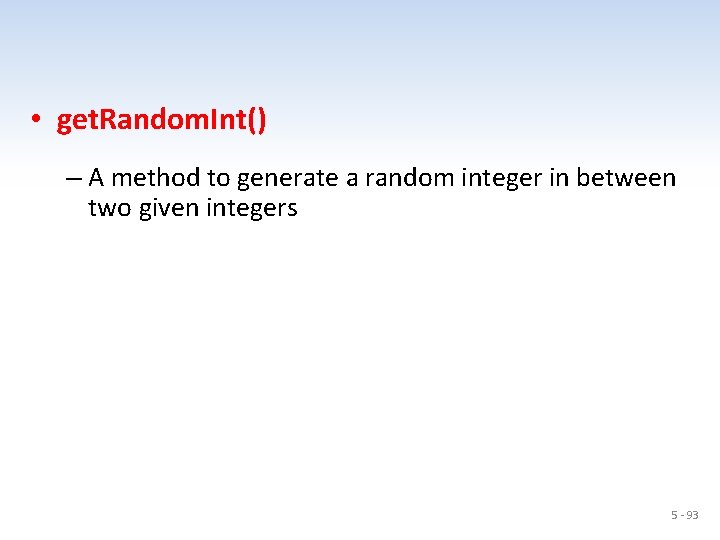
• get. Random. Int() – A method to generate a random integer in between two given integers 5 - 93
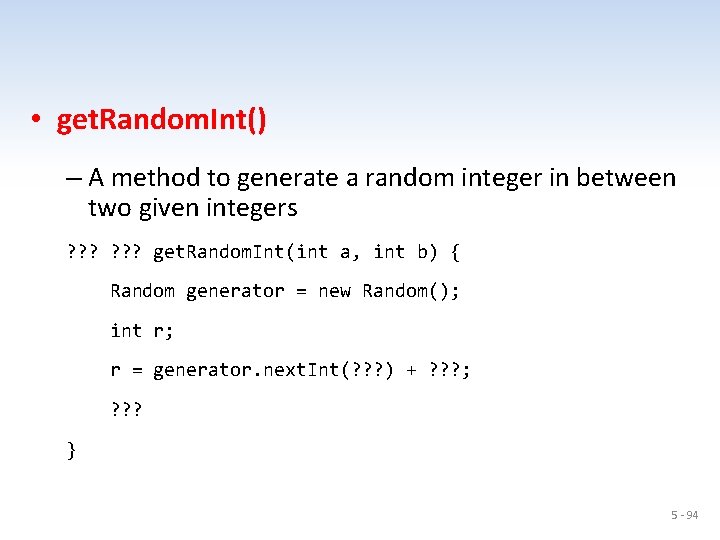
• get. Random. Int() – A method to generate a random integer in between two given integers ? ? ? get. Random. Int(int a, int b) { Random generator = new Random(); int r; r = generator. next. Int(? ? ? ) + ? ? ? ; ? ? ? } 5 - 94
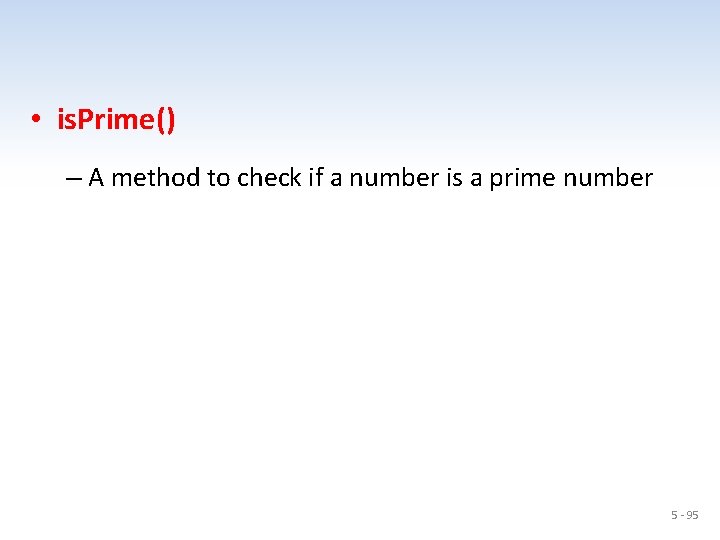
• is. Prime() – A method to check if a number is a prime number 5 - 95
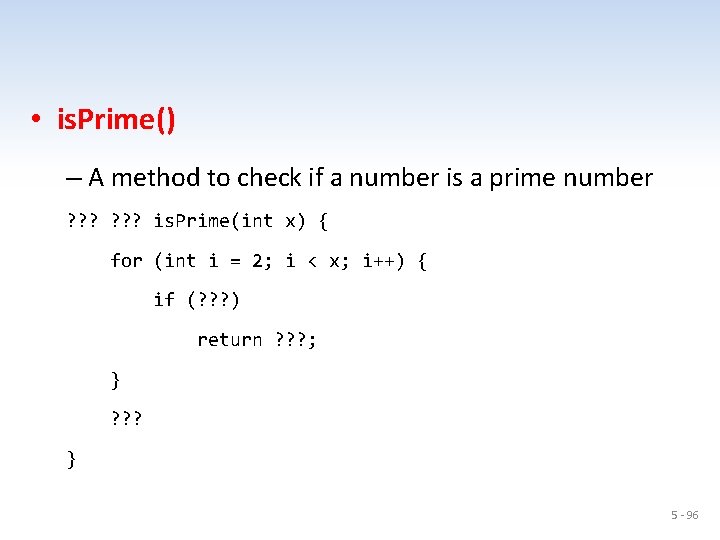
• is. Prime() – A method to check if a number is a prime number ? ? ? is. Prime(int x) { for (int i = 2; i < x; i++) { if (? ? ? ) return ? ? ? ; } ? ? ? } 5 - 96
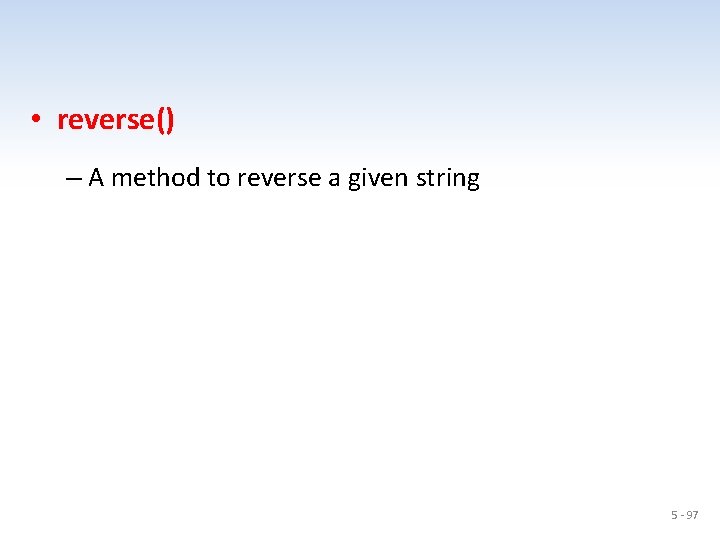
• reverse() – A method to reverse a given string 5 - 97
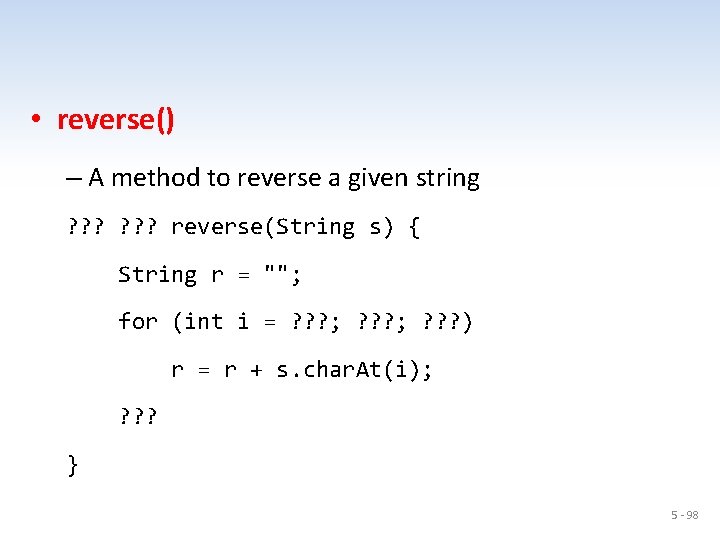
• reverse() – A method to reverse a given string ? ? ? reverse(String s) { String r = ""; for (int i = ? ? ? ; ? ? ? ) r = r + s. char. At(i); ? ? ? } 5 - 98
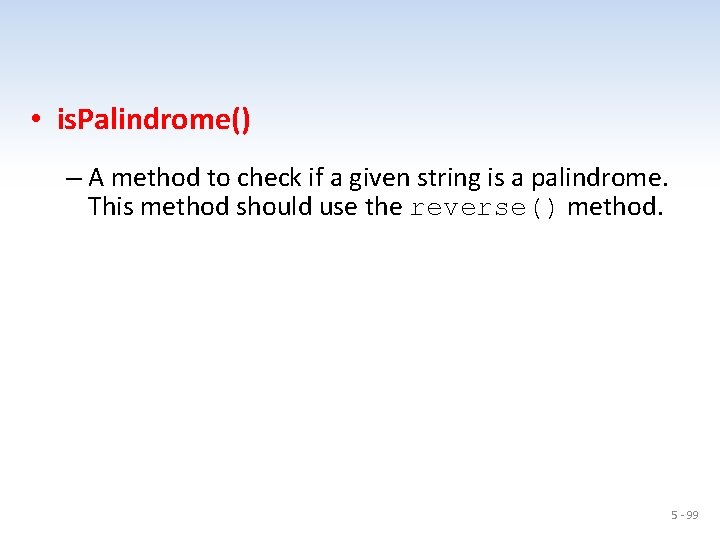
• is. Palindrome() – A method to check if a given string is a palindrome. This method should use the reverse() method. 5 - 99
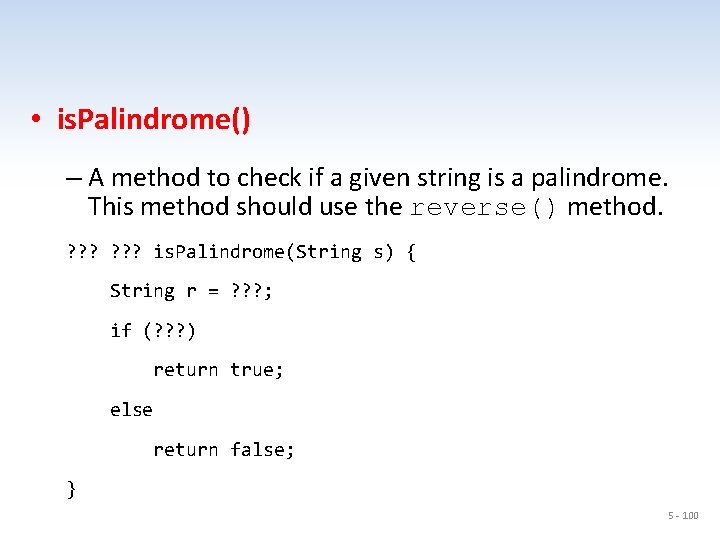
• is. Palindrome() – A method to check if a given string is a palindrome. This method should use the reverse() method. ? ? ? is. Palindrome(String s) { String r = ? ? ? ; if (? ? ? ) return true; else return false; } 5 - 100
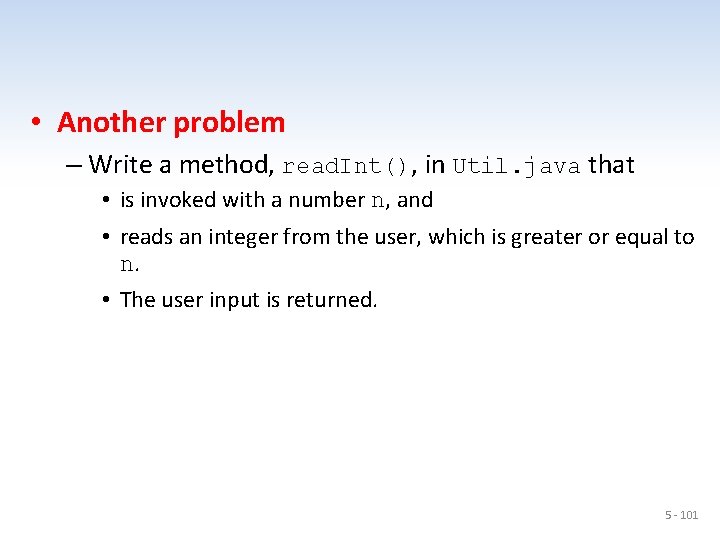
• Another problem – Write a method, read. Int(), in Util. java that • is invoked with a number n, and • reads an integer from the user, which is greater or equal to n. • The user input is returned. 5 - 101
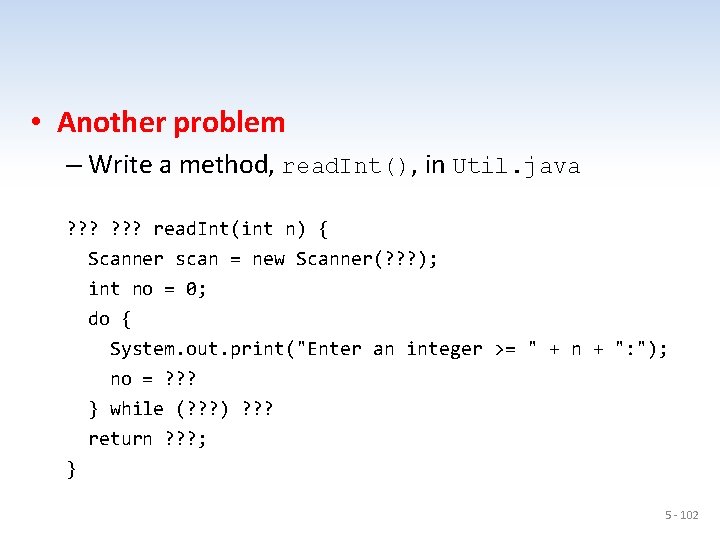
• Another problem – Write a method, read. Int(), in Util. java ? ? ? read. Int(int n) { Scanner scan = new Scanner(? ? ? ); int no = 0; do { System. out. print("Enter an integer >= " + n + ": "); no = ? ? ? } while (? ? ? ) ? ? ? return ? ? ? ; } 5 - 102
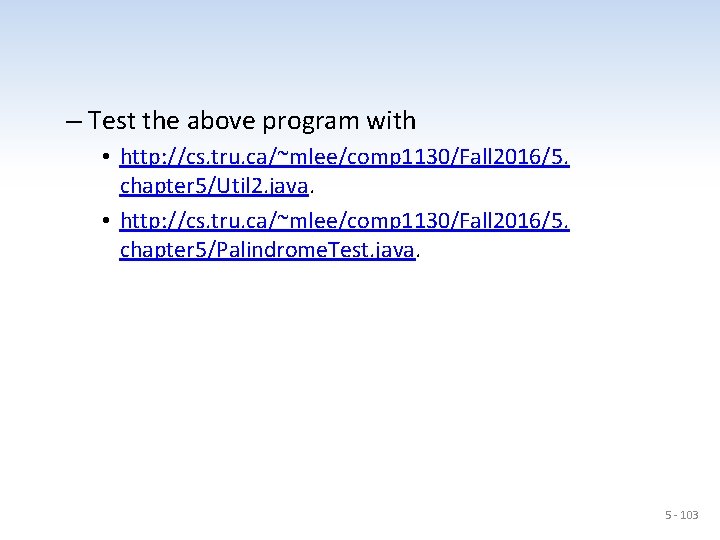
– Test the above program with • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Util 2. java. • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Palindrome. Test. java. 5 - 103
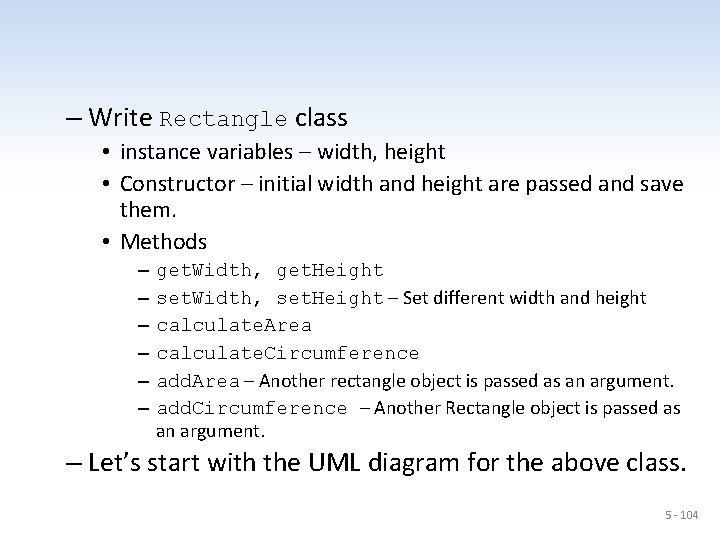
– Write Rectangle class • instance variables – width, height • Constructor – initial width and height are passed and save them. • Methods – – – get. Width, get. Height set. Width, set. Height – Set different width and height calculate. Area calculate. Circumference add. Area – Another rectangle object is passed as an argument. add. Circumference – Another Rectangle object is passed as an argument. – Let’s start with the UML diagram for the above class. 5 - 104
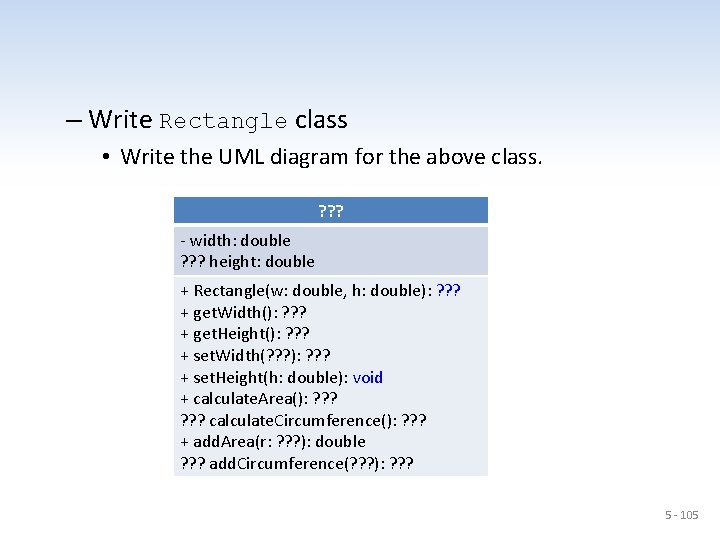
– Write Rectangle class • Write the UML diagram for the above class. ? ? ? - width: double ? ? ? height: double + Rectangle(w: double, h: double): ? ? ? + get. Width(): ? ? ? + get. Height(): ? ? ? + set. Width(? ? ? ): ? ? ? + set. Height(h: double): void + calculate. Area(): ? ? ? calculate. Circumference(): ? ? ? + add. Area(r: ? ? ? ): double ? ? ? add. Circumference(? ? ? ): ? ? ? 5 - 105
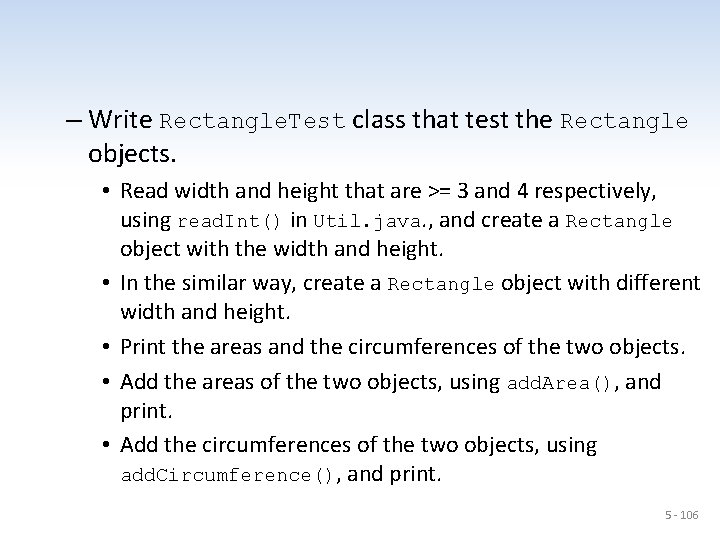
– Write Rectangle. Test class that test the Rectangle objects. • Read width and height that are >= 3 and 4 respectively, using read. Int() in Util. java. , and create a Rectangle object with the width and height. • In the similar way, create a Rectangle object with different width and height. • Print the areas and the circumferences of the two objects. • Add the areas of the two objects, using add. Area(), and print. • Add the circumferences of the two objects, using add. Circumference(), and print. 5 - 106
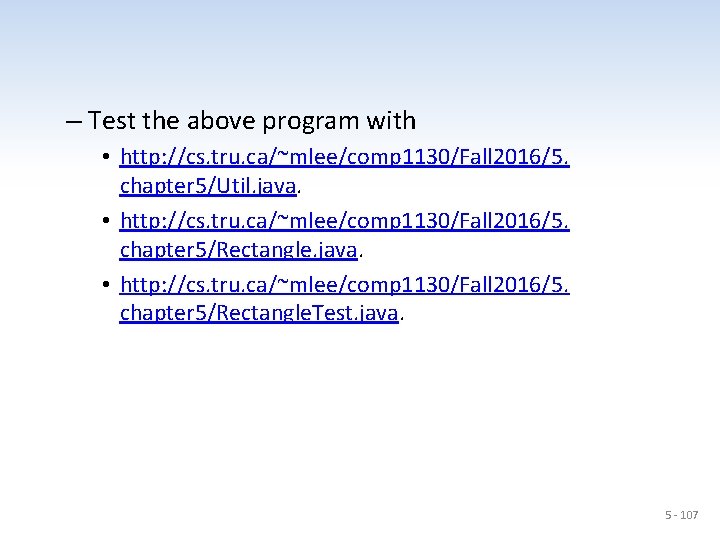
– Test the above program with • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Util. java. • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Rectangle. Test. java. 5 - 107
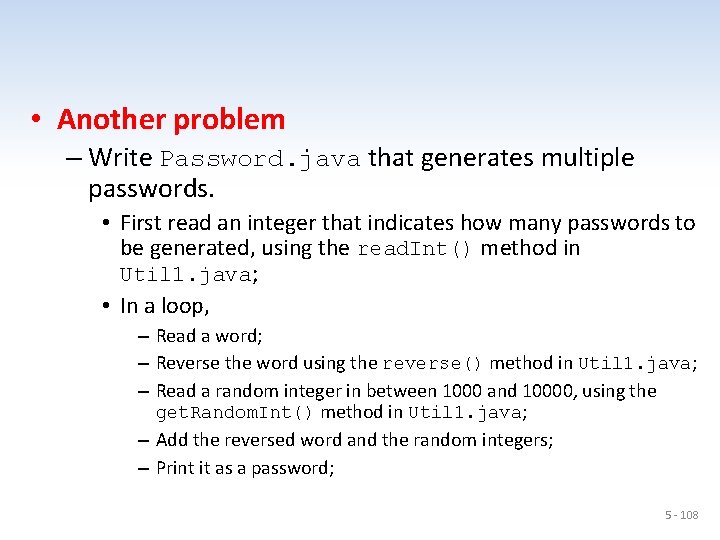
• Another problem – Write Password. java that generates multiple passwords. • First read an integer that indicates how many passwords to be generated, using the read. Int() method in Util 1. java; • In a loop, – Read a word; – Reverse the word using the reverse() method in Util 1. java; – Read a random integer in between 1000 and 10000, using the get. Random. Int() method in Util 1. java; – Add the reversed word and the random integers; – Print it as a password; 5 - 108
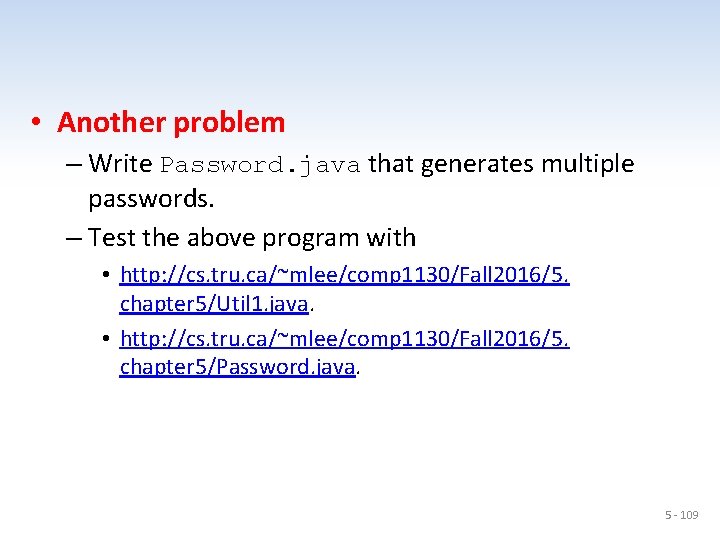
• Another problem – Write Password. java that generates multiple passwords. – Test the above program with • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Util 1. java. • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Password. java. 5 - 109
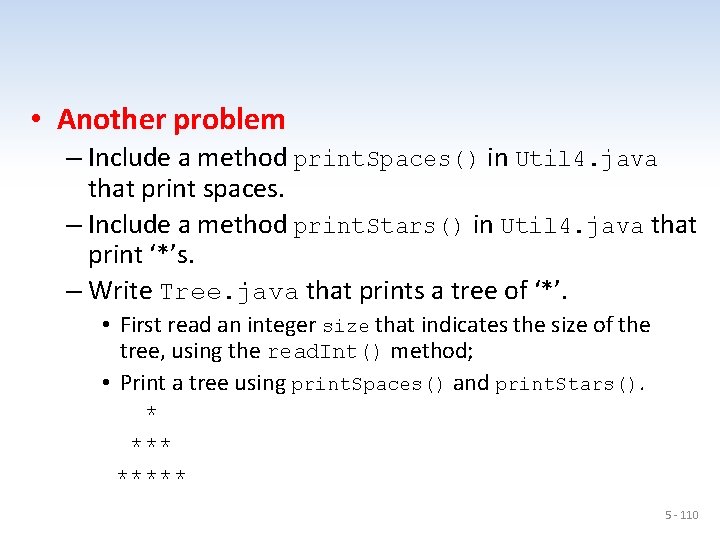
• Another problem – Include a method print. Spaces() in Util 4. java that print spaces. – Include a method print. Stars() in Util 4. java that print ‘*’s. – Write Tree. java that prints a tree of ‘*’. • First read an integer size that indicates the size of the tree, using the read. Int() method; • Print a tree using print. Spaces() and print. Stars(). * ***** 5 - 110
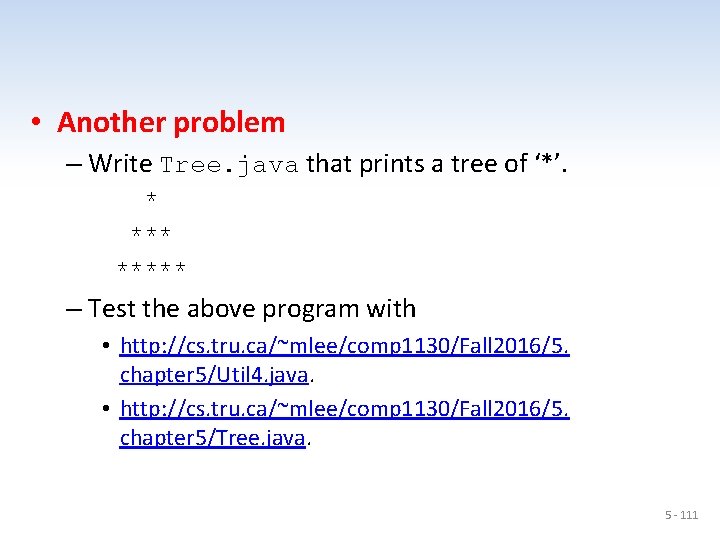
• Another problem – Write Tree. java that prints a tree of ‘*’. * ***** – Test the above program with • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Util 4. java. • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Tree. java. 5 - 111
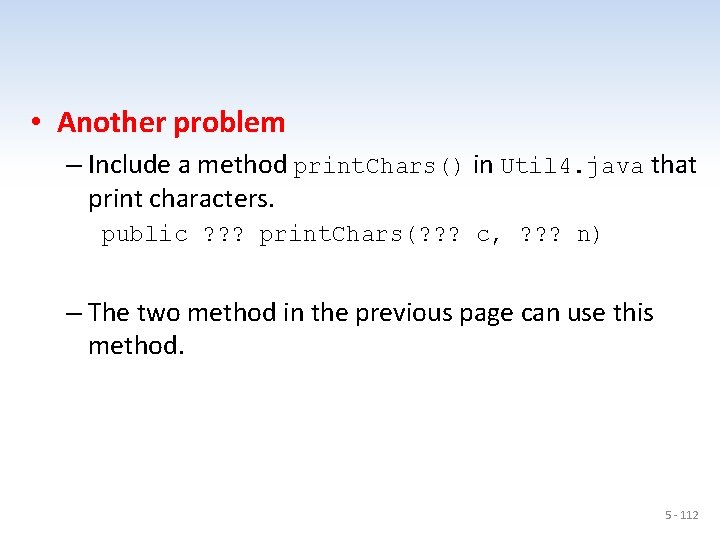
• Another problem – Include a method print. Chars() in Util 4. java that print characters. public ? ? ? print. Chars(? ? ? c, ? ? ? n) – The two method in the previous page can use this method. 5 - 112
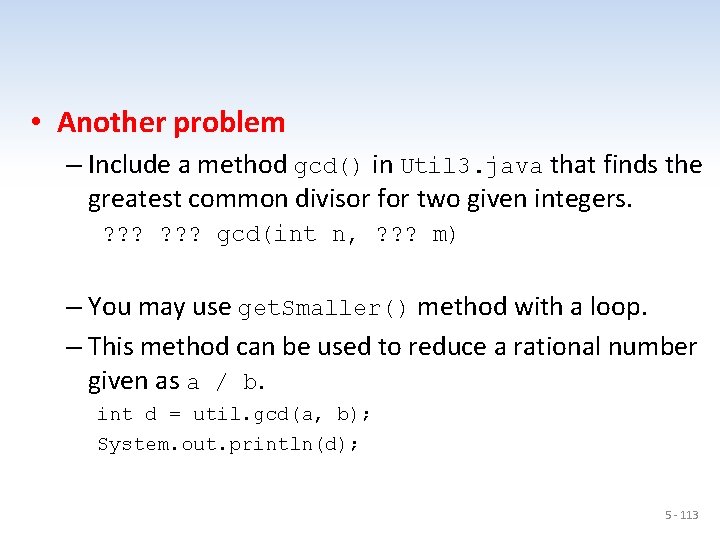
• Another problem – Include a method gcd() in Util 3. java that finds the greatest common divisor for two given integers. ? ? ? gcd(int n, ? ? ? m) – You may use get. Smaller() method with a loop. – This method can be used to reduce a rational number given as a / b. int d = util. gcd(a, b); System. out. println(d); 5 - 113
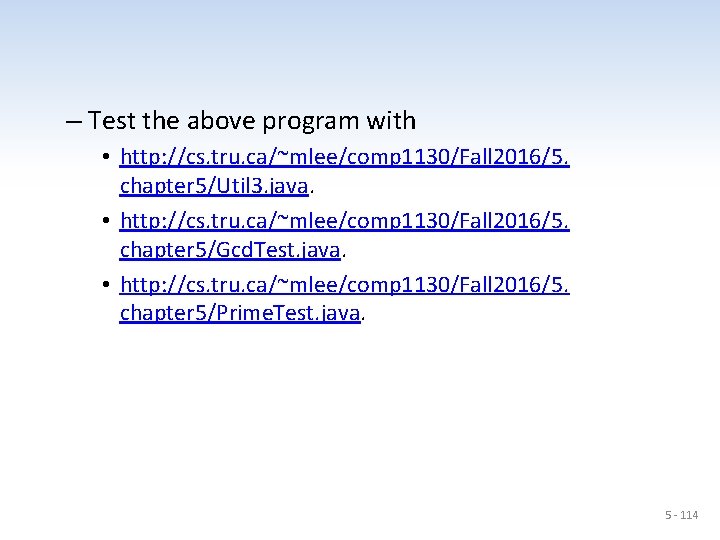
– Test the above program with • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Util 3. java. • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Gcd. Test. java. • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Prime. Test. java. 5 - 114
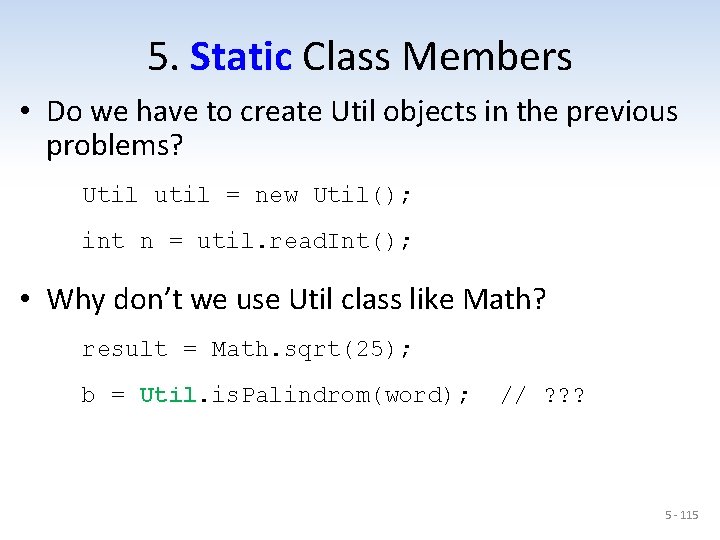
5. Static Class Members • Do we have to create Util objects in the previous problems? Util util = new Util(); int n = util. read. Int(); • Why don’t we use Util class like Math? result = Math. sqrt(25); b = Util. is. Palindrom(word); // ? ? ? 5 - 115
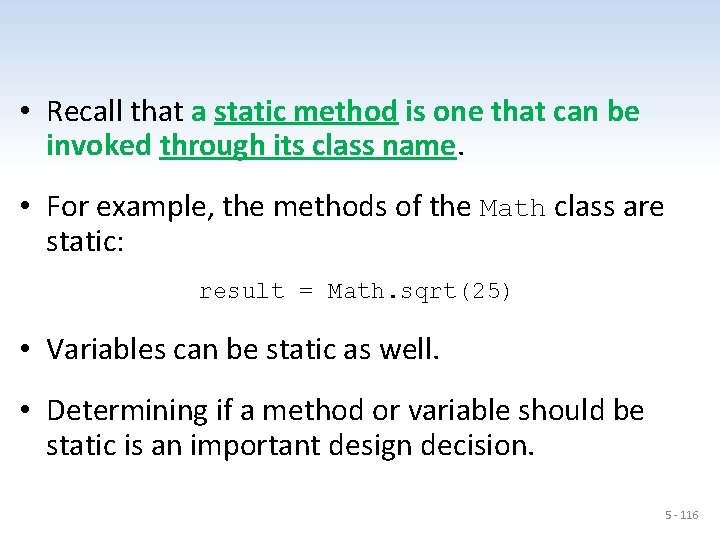
• Recall that a static method is one that can be invoked through its class name. • For example, the methods of the Math class are static: result = Math. sqrt(25) • Variables can be static as well. • Determining if a method or variable should be static is an important design decision. 5 - 116
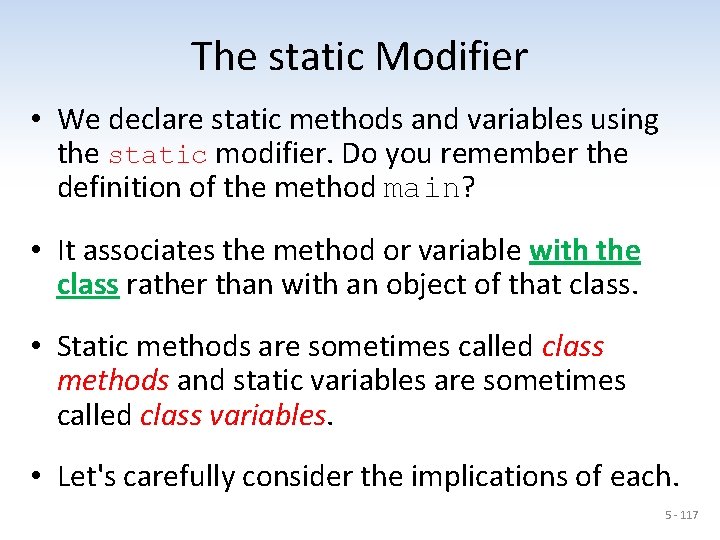
The static Modifier • We declare static methods and variables using the static modifier. Do you remember the definition of the method main? • It associates the method or variable with the class rather than with an object of that class. • Static methods are sometimes called class methods and static variables are sometimes called class variables. • Let's carefully consider the implications of each. 5 - 117
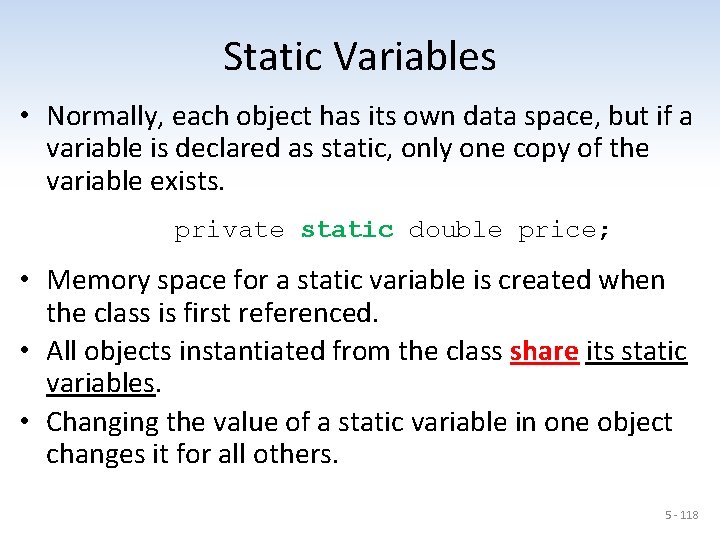
Static Variables • Normally, each object has its own data space, but if a variable is declared as static, only one copy of the variable exists. private static double price; • Memory space for a static variable is created when the class is first referenced. • All objects instantiated from the class share its static variables. • Changing the value of a static variable in one object changes it for all others. 5 - 118
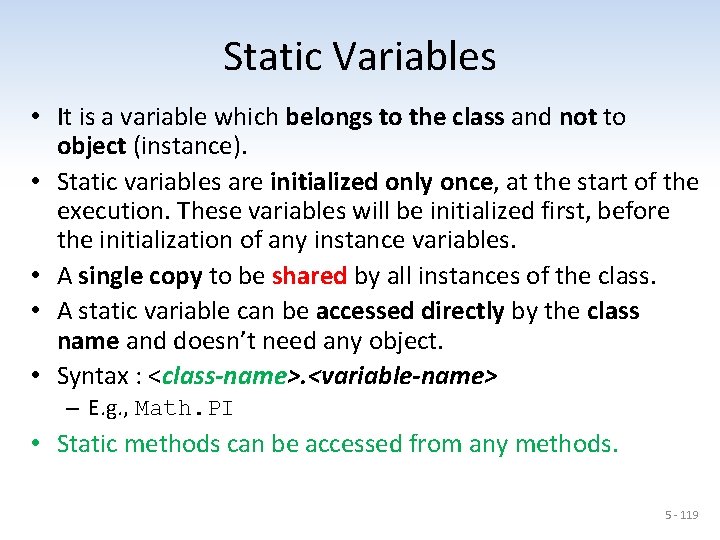
Static Variables • It is a variable which belongs to the class and not to object (instance). • Static variables are initialized only once, at the start of the execution. These variables will be initialized first, before the initialization of any instance variables. • A single copy to be shared by all instances of the class. • A static variable can be accessed directly by the class name and doesn’t need any object. • Syntax : <class-name>. <variable-name> – E. g. , Math. PI • Static methods can be accessed from any methods. 5 - 119
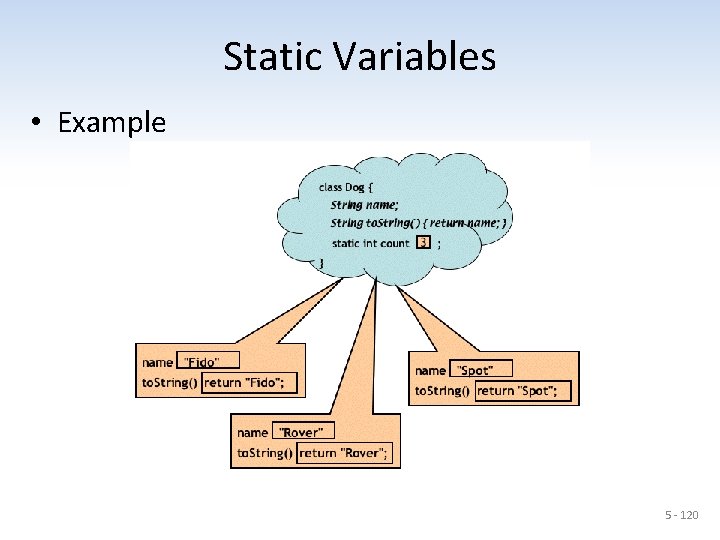
Static Variables • Example 5 - 120
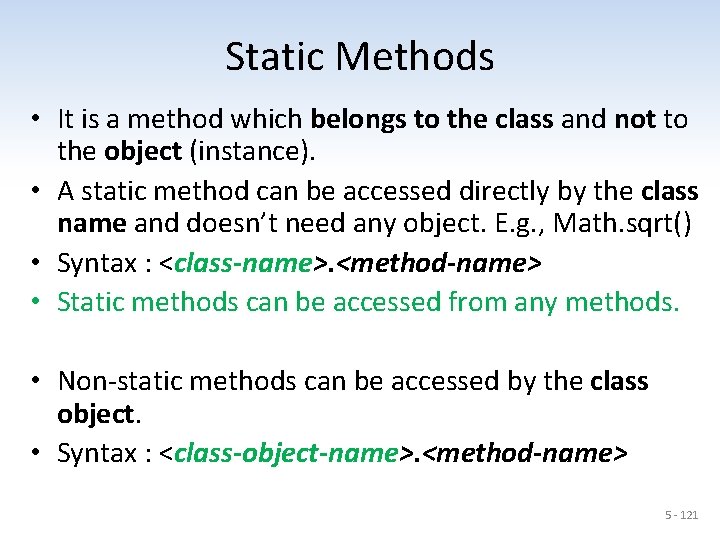
Static Methods • It is a method which belongs to the class and not to the object (instance). • A static method can be accessed directly by the class name and doesn’t need any object. E. g. , Math. sqrt() • Syntax : <class-name>. <method-name> • Static methods can be accessed from any methods. • Non-static methods can be accessed by the class object. • Syntax : <class-object-name>. <method-name> 5 - 121
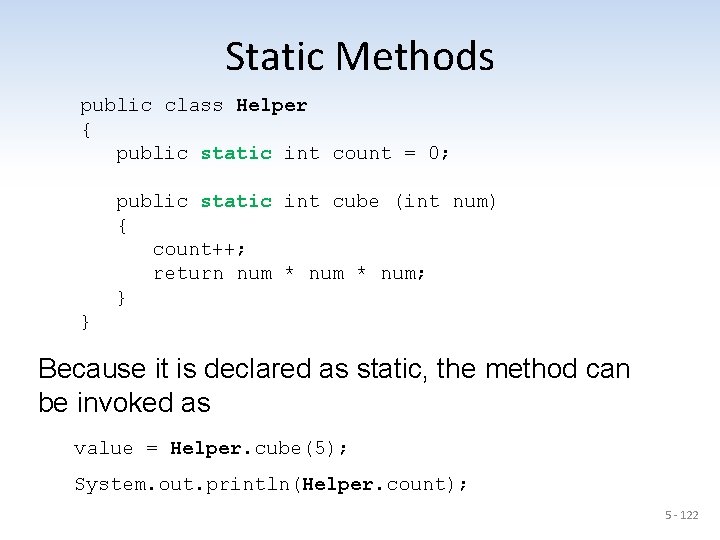
Static Methods public class Helper { public static int count = 0; public static int cube (int num) { count++; return num * num; } } Because it is declared as static, the method can be invoked as value = Helper. cube(5); System. out. println(Helper. count); 5 - 122
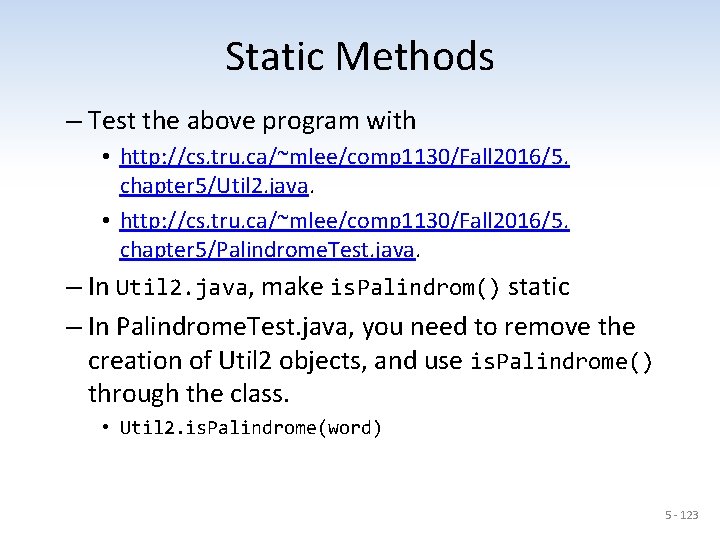
Static Methods – Test the above program with • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Util 2. java. • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Palindrome. Test. java. – In Util 2. java, make is. Palindrom() static – In Palindrome. Test. java, you need to remove the creation of Util 2 objects, and use is. Palindrome() through the class. • Util 2. is. Palindrome(word) 5 - 123
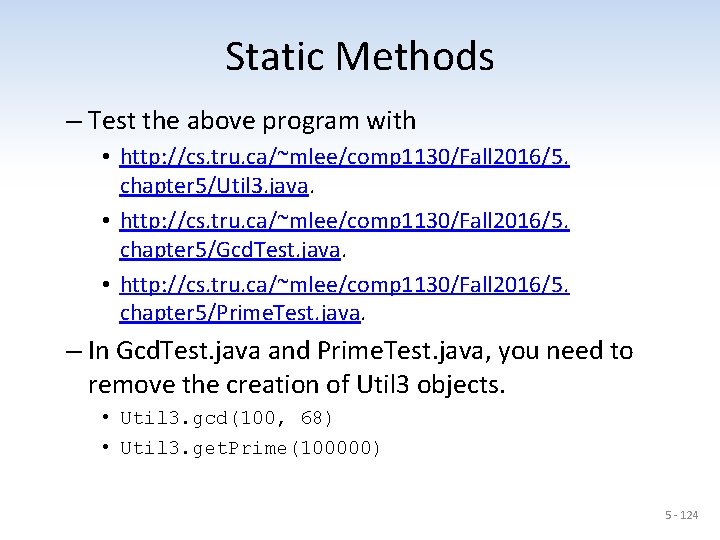
Static Methods – Test the above program with • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Util 3. java. • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Gcd. Test. java. • http: //cs. tru. ca/~mlee/comp 1130/Fall 2016/5. chapter 5/Prime. Test. java. – In Gcd. Test. java and Prime. Test. java, you need to remove the creation of Util 3 objects. • Util 3. gcd(100, 68) • Util 3. get. Prime(100000) 5 - 124
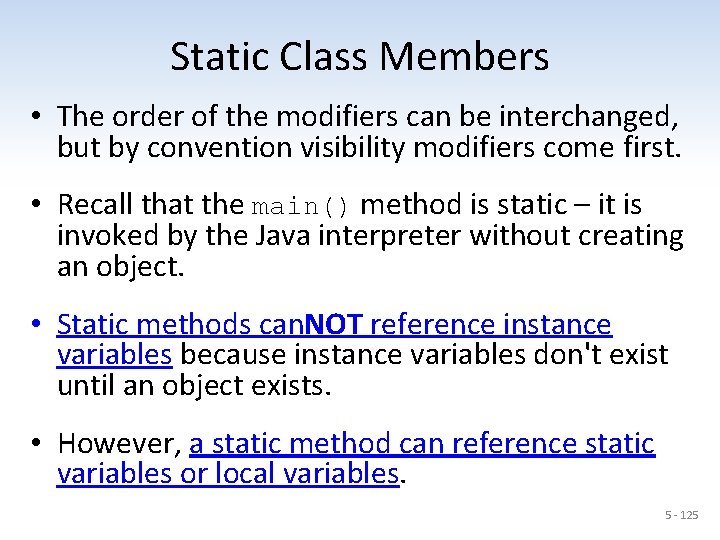
Static Class Members • The order of the modifiers can be interchanged, but by convention visibility modifiers come first. • Recall that the main() method is static – it is invoked by the Java interpreter without creating an object. • Static methods can. NOT reference instance variables because instance variables don't exist until an object exists. • However, a static method can reference static variables or local variables. 5 - 125
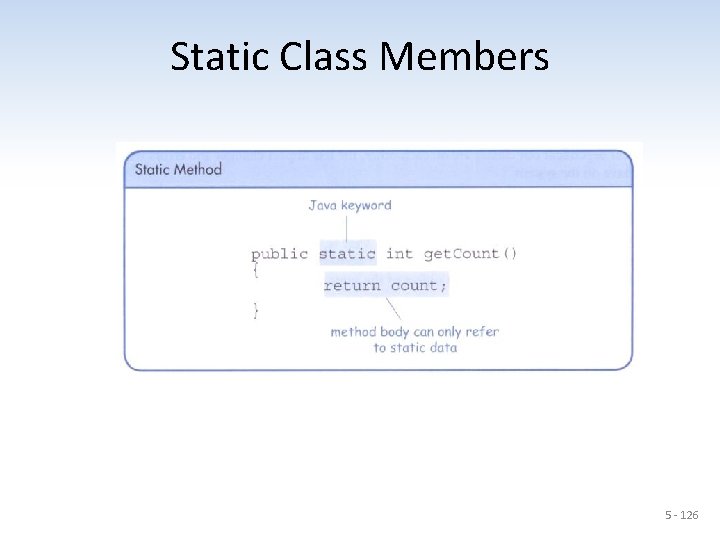
Static Class Members 5 - 126
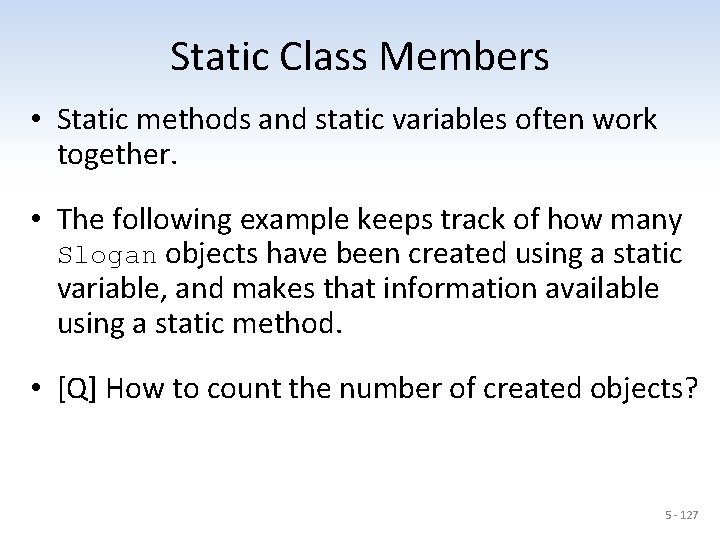
Static Class Members • Static methods and static variables often work together. • The following example keeps track of how many Slogan objects have been created using a static variable, and makes that information available using a static method. • [Q] How to count the number of created objects? 5 - 127
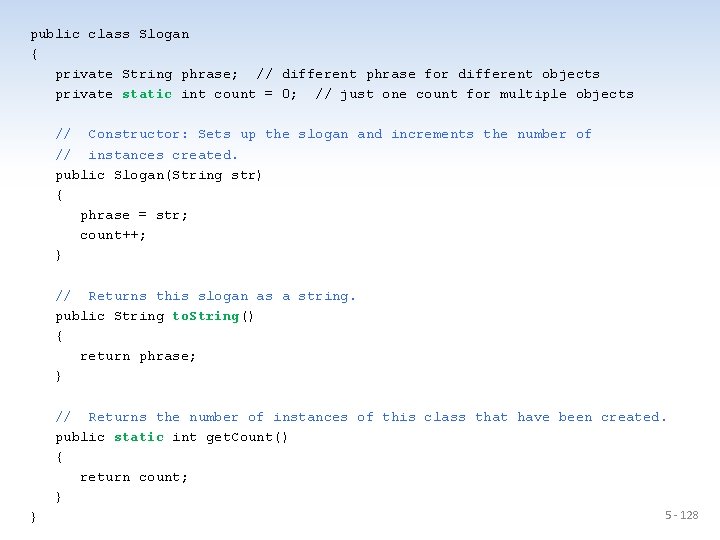
public class Slogan { private String phrase; // different phrase for different objects private static int count = 0; // just one count for multiple objects // Constructor: Sets up the slogan and increments the number of // instances created. public Slogan(String str) { phrase = str; count++; } // Returns this slogan as a string. public String to. String() { return phrase; } // Returns the number of instances of this class that have been created. public static int get. Count() { return count; } } 5 - 128
![public class Slogan Counter public static void mainString args Slogan obj obj public class Slogan. Counter { public static void main(String[] args) { Slogan obj; obj](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-92.jpg)
public class Slogan. Counter { public static void main(String[] args) { Slogan obj; obj = new Slogan("Remember the Alamo. "); System. out. println(obj); // ? ? ? to. String() obj = new Slogan("Don't Worry. Be Happy. "); System. out. println(obj); obj = new Slogan("Live Free or Die. "); System. out. println(obj); obj = new Slogan("Talk is Cheap. "); System. out. println(obj); obj = new Slogan("Write Once, Run Anywhere. "); System. out. println(obj); System. out. println("Slogans created: " + Slogan. get. Count()); System. out. println("Slogans created: " + obj. get. Count()); // ? ? ? } } 5 - 129
![public class Slogan Counter public static void mainString args Slogan obj obj public class Slogan. Counter { public static void main(String[] args) { Slogan obj; obj](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-93.jpg)
public class Slogan. Counter { public static void main(String[] args) { Slogan obj; obj = new Slogan("Remember the Alamo. "); System. out. println(obj); // ? ? ? to. String() obj = new Slogan("Don't Worry. Be Happy. "); System. out. println(obj); obj = new Slogan("Live Free or Die. "); System. out. println(obj); obj = new Slogan("Talk is Cheap. "); System. out. println(obj); obj = new Slogan("Write Once, Run Anywhere. "); System. out. println(obj); System. out. println("Slogans created: " + Slogan. get. Count()); System. out. println("Slogans created: " + obj. get. Count()); // Possible, // but good practice? } } 5 - 130
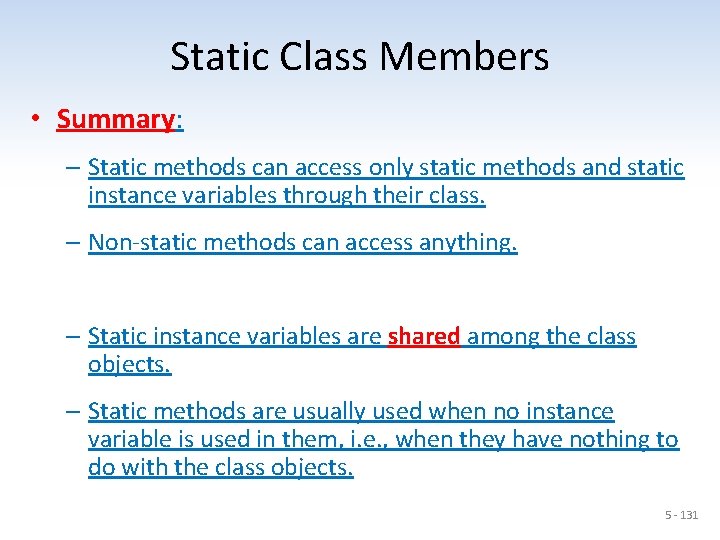
Static Class Members • Summary: – Static methods can access only static methods and static instance variables through their class. – Non-static methods can access anything. – Static instance variables are shared among the class objects. – Static methods are usually used when no instance variable is used in them, i. e. , when they have nothing to do with the class objects. 5 - 131
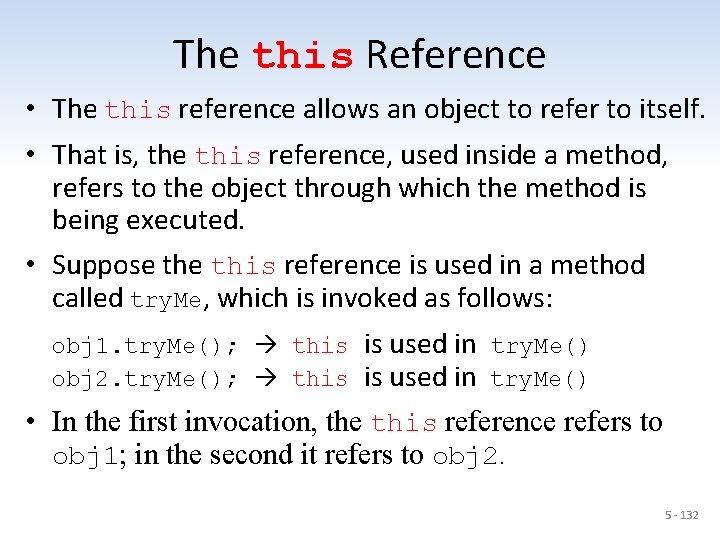
The this Reference • The this reference allows an object to refer to itself. • That is, the this reference, used inside a method, refers to the object through which the method is being executed. • Suppose this reference is used in a method called try. Me, which is invoked as follows: obj 1. try. Me(); this is used in try. Me() obj 2. try. Me(); this is used in try. Me() • In the first invocation, the this reference refers to obj 1; in the second it refers to obj 2. 5 - 132
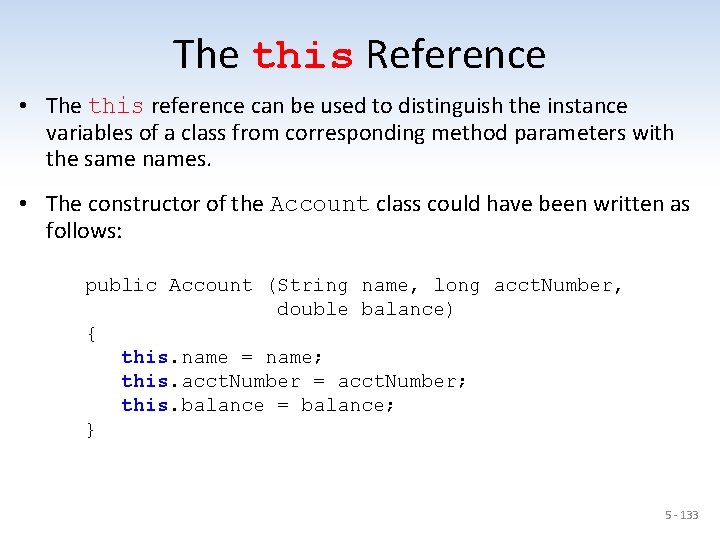
The this Reference • The this reference can be used to distinguish the instance variables of a class from corresponding method parameters with the same names. • The constructor of the Account class could have been written as follows: public Account (String name, long acct. Number, double balance) { this. name = name; this. acct. Number = acct. Number; this. balance = balance; } 5 - 133
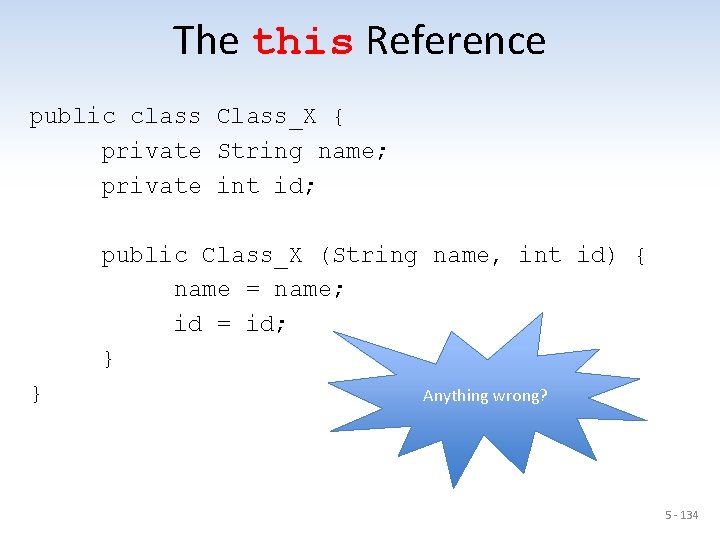
The this Reference public class Class_X { private String name; private int id; public Class_X (String name, int id) { name = name; id = id; } } Anything wrong? 5 - 134
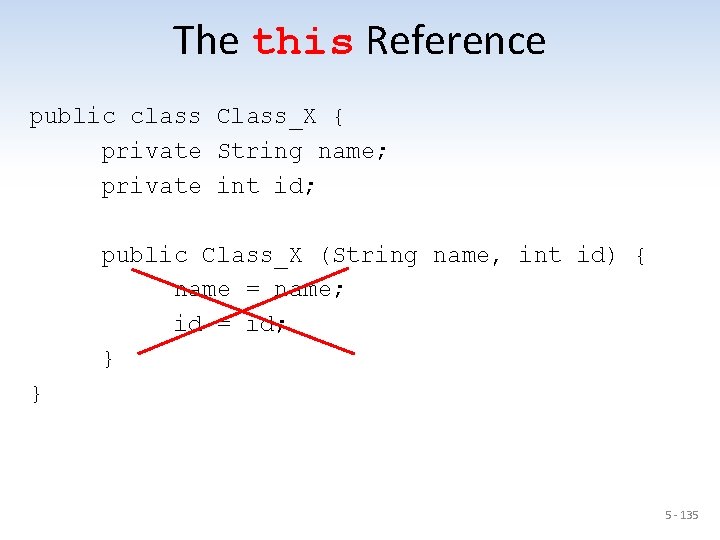
The this Reference public class Class_X { private String name; private int id; public Class_X (String name, int id) { name = name; id = id; } } 5 - 135
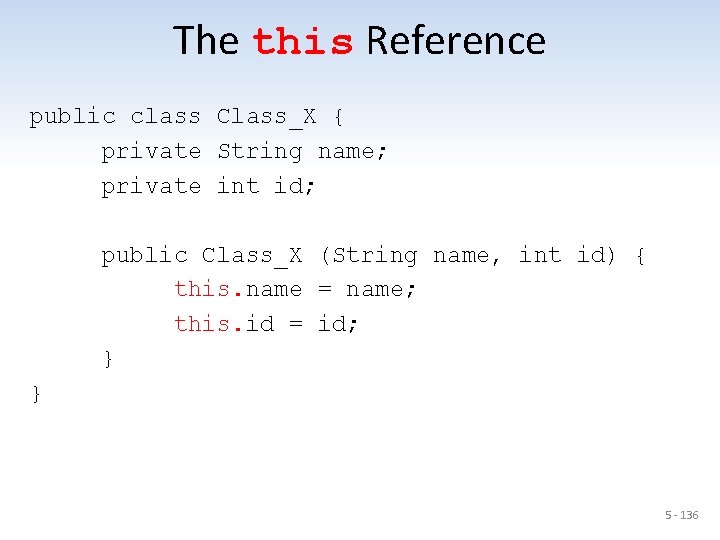
The this Reference public class Class_X { private String name; private int id; public Class_X (String name, int id) { this. name = name; this. id = id; } } 5 - 136
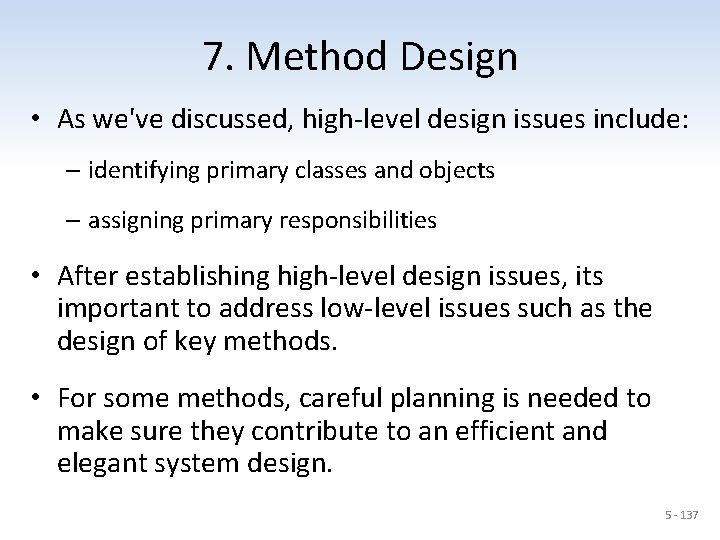
7. Method Design • As we've discussed, high-level design issues include: – identifying primary classes and objects – assigning primary responsibilities • After establishing high-level design issues, its important to address low-level issues such as the design of key methods. • For some methods, careful planning is needed to make sure they contribute to an efficient and elegant system design. 5 - 137
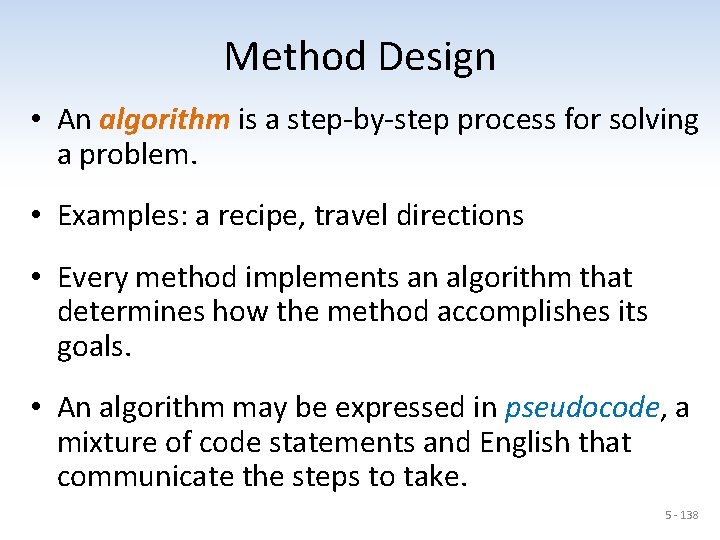
Method Design • An algorithm is a step-by-step process for solving a problem. • Examples: a recipe, travel directions • Every method implements an algorithm that determines how the method accomplishes its goals. • An algorithm may be expressed in pseudocode, a mixture of code statements and English that communicate the steps to take. 5 - 138
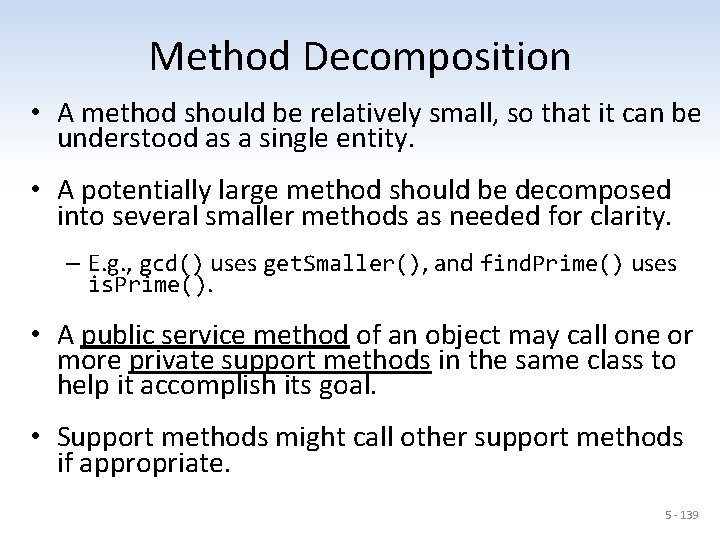
Method Decomposition • A method should be relatively small, so that it can be understood as a single entity. • A potentially large method should be decomposed into several smaller methods as needed for clarity. – E. g. , gcd() uses get. Smaller(), and find. Prime() uses is. Prime(). • A public service method of an object may call one or more private support methods in the same class to help it accomplish its goal. • Support methods might call other support methods if appropriate. 5 - 139
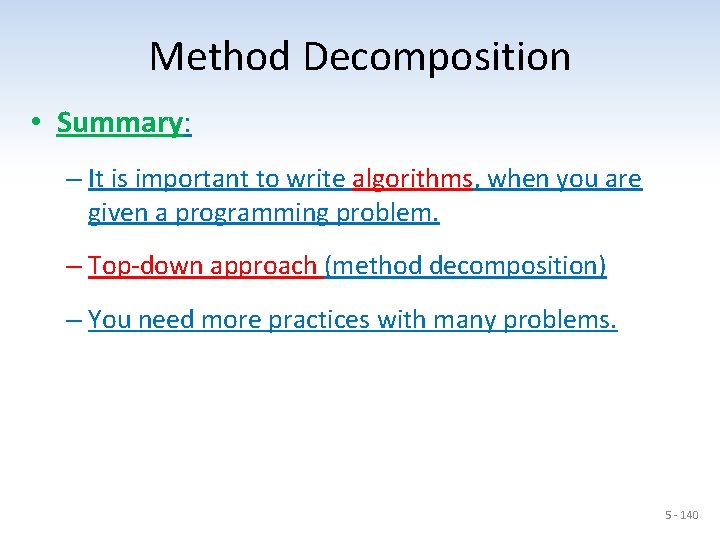
Method Decomposition • Summary: – It is important to write algorithms, when you are given a programming problem. – Top-down approach (method decomposition) – You need more practices with many problems. 5 - 140
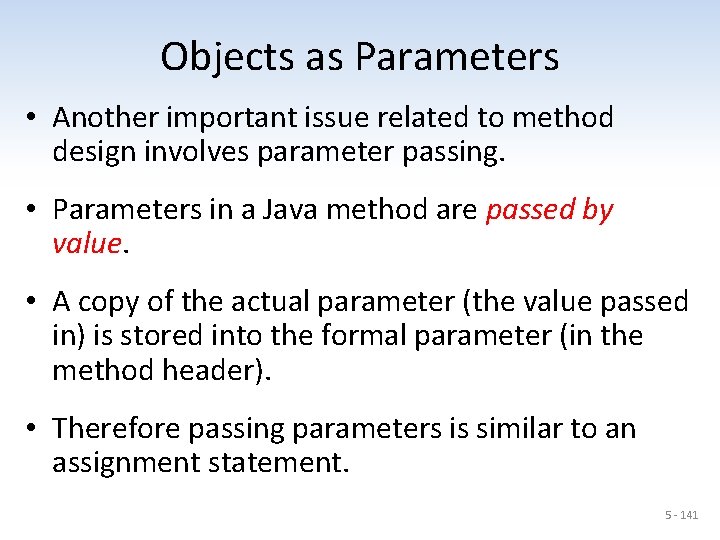
Objects as Parameters • Another important issue related to method design involves parameter passing. • Parameters in a Java method are passed by value. • A copy of the actual parameter (the value passed in) is stored into the formal parameter (in the method header). • Therefore passing parameters is similar to an assignment statement. 5 - 141
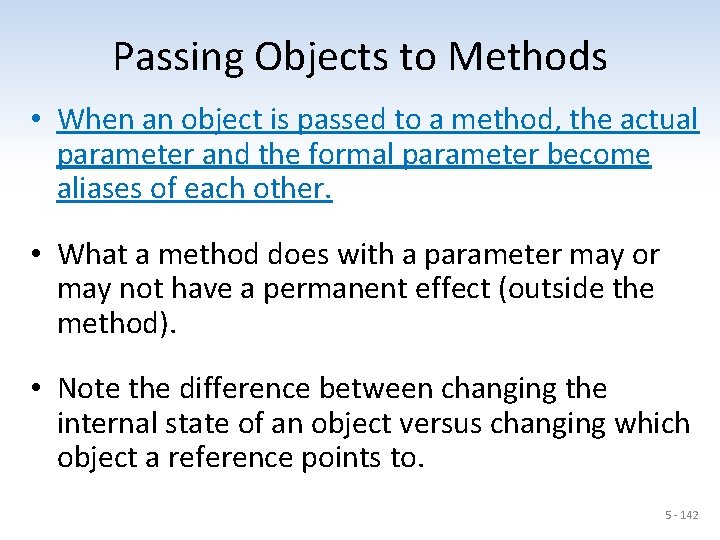
Passing Objects to Methods • When an object is passed to a method, the actual parameter and the formal parameter become aliases of each other. • What a method does with a parameter may or may not have a permanent effect (outside the method). • Note the difference between changing the internal state of an object versus changing which object a reference points to. 5 - 142
![public class Parameter Tester public static void mainString args Parameter Modifier modifier public class Parameter. Tester { public static void main(String[] args) { Parameter. Modifier modifier](https://slidetodoc.com/presentation_image_h2/30e837dee7bf3607d4cac41eb353bd5f/image-106.jpg)
public class Parameter. Tester { public static void main(String[] args) { Parameter. Modifier modifier = new Parameter. Modifier(); int a 1 = 111; Num a 2 = new Num(222); Num a 3 = new Num(333); System. out. println("Before calling change. Values: "); System. out. println("a 1ta 2ta 3"); System. out. println(a 1 + "t" + a 2 + "t" + a 3 + "n"); modifier. change. Values(a 1, a 2, a 3); Very important! System. out. println("After calling change. Values: "); System. out. println("a 1ta 2ta 3"); System. out. println(a 1 + "t" + a 2 + "t" + a 3 + "n"); } } 5 - 143
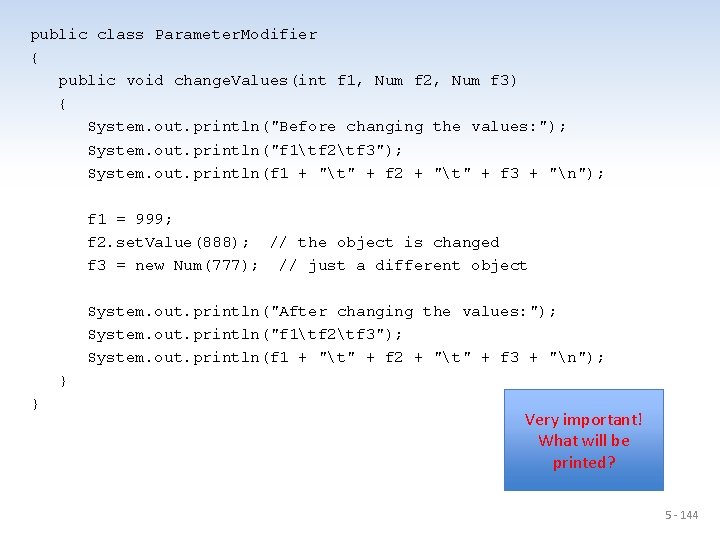
public class Parameter. Modifier { public void change. Values(int f 1, Num f 2, Num f 3) { System. out. println("Before changing the values: "); System. out. println("f 1tf 2tf 3"); System. out. println(f 1 + "t" + f 2 + "t" + f 3 + "n"); f 1 = 999; f 2. set. Value(888); // the object is changed f 3 = new Num(777); // just a different object System. out. println("After changing the values: "); System. out. println("f 1tf 2tf 3"); System. out. println(f 1 + "t" + f 2 + "t" + f 3 + "n"); } } Very important! What will be printed? 5 - 144
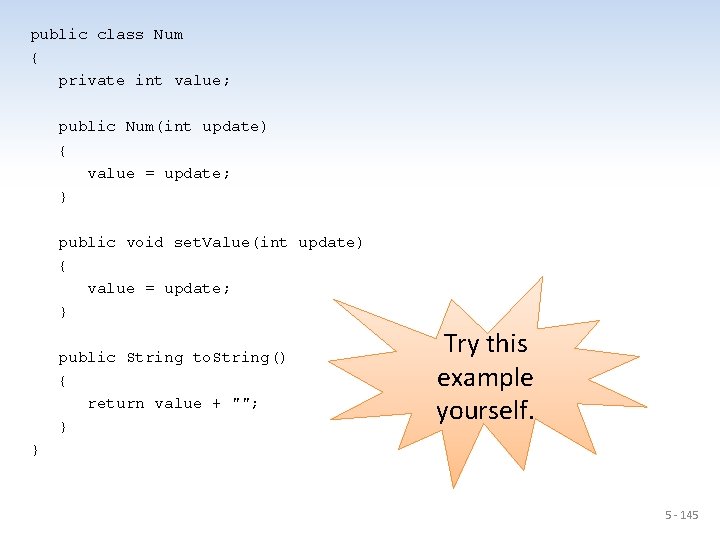
public class Num { private int value; public Num(int update) { value = update; } public void set. Value(int update) { value = update; } public String to. String() { return value + ""; } Try this example yourself. } 5 - 145
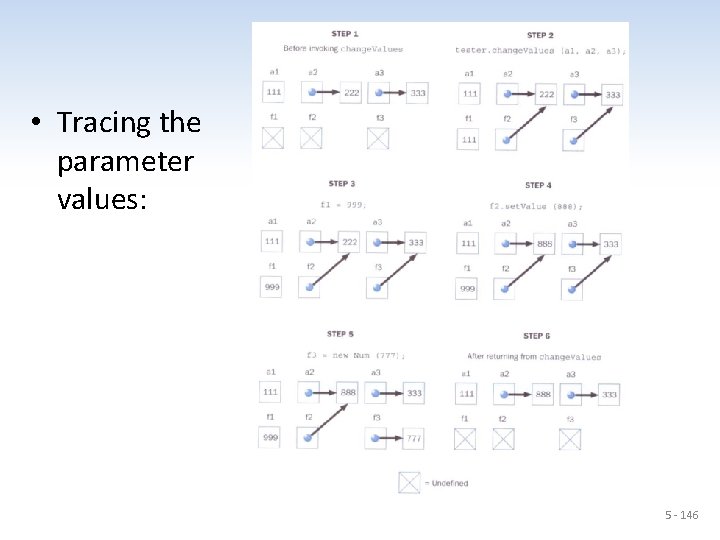
xxx • Tracing the parameter values: 5 - 146
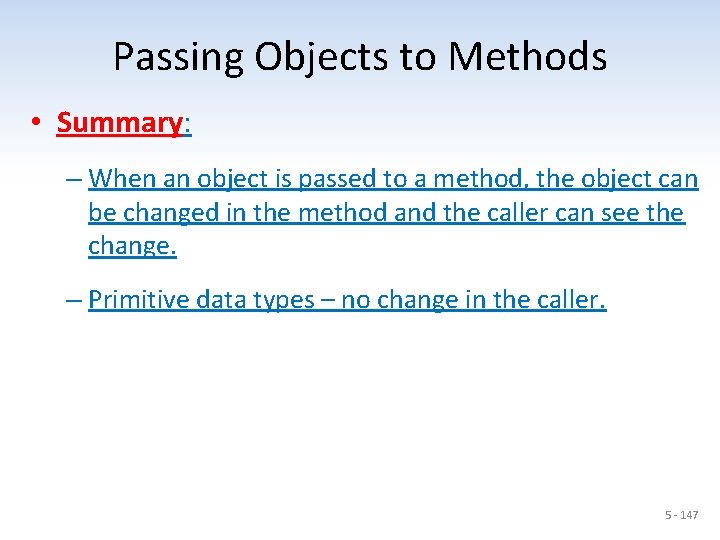
Passing Objects to Methods • Summary: – When an object is passed to a method, the object can be changed in the method and the caller can see the change. – Primitive data types – no change in the caller. 5 - 147
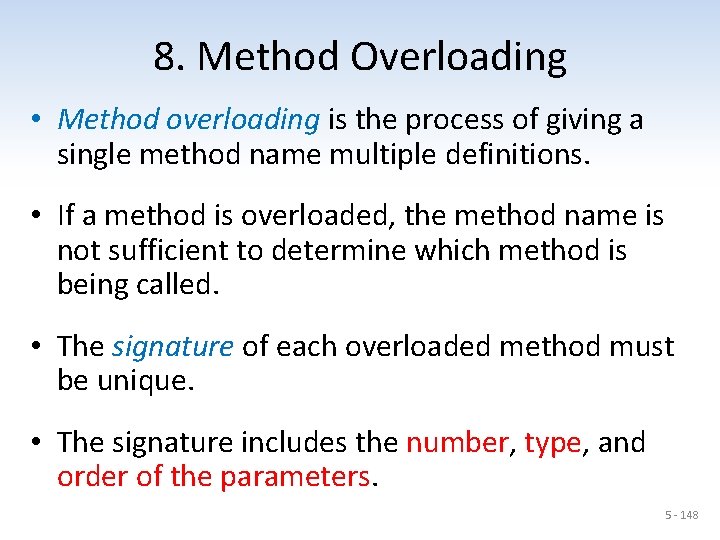
8. Method Overloading • Method overloading is the process of giving a single method name multiple definitions. • If a method is overloaded, the method name is not sufficient to determine which method is being called. • The signature of each overloaded method must be unique. • The signature includes the number, type, and order of the parameters. 5 - 148
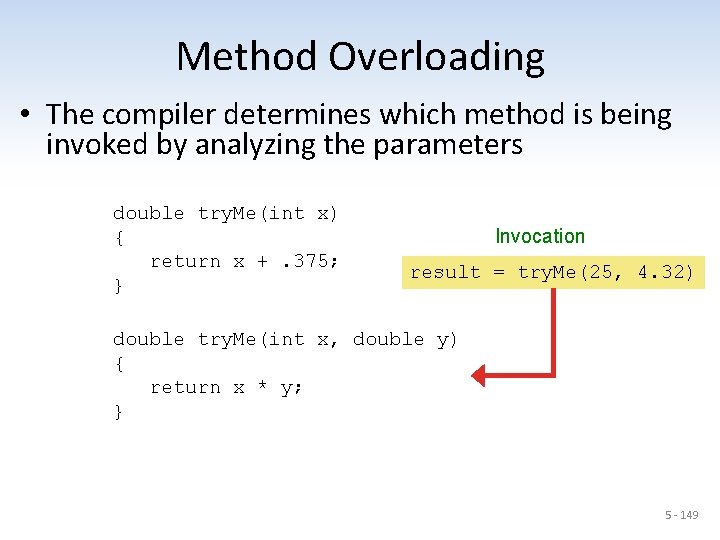
Method Overloading • The compiler determines which method is being invoked by analyzing the parameters double try. Me(int x) { return x +. 375; } Invocation result = try. Me(25, 4. 32) double try. Me(int x, double y) { return x * y; } 5 - 149
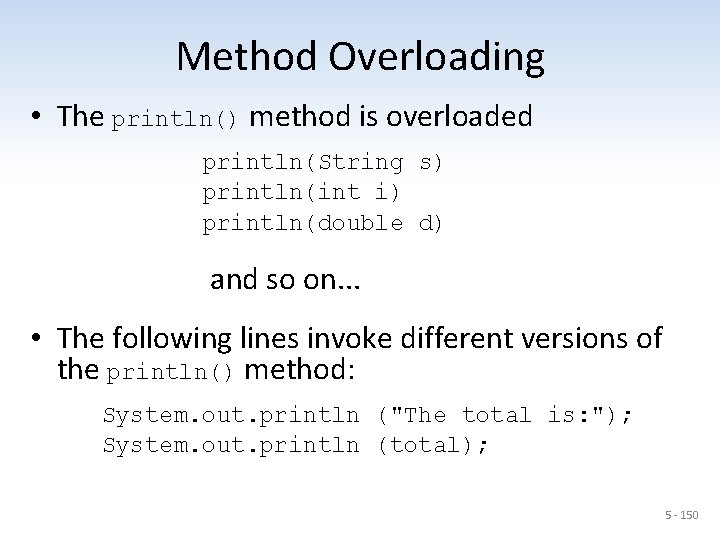
Method Overloading • The println() method is overloaded println(String s) println(int i) println(double d) and so on. . . • The following lines invoke different versions of the println() method: System. out. println ("The total is: "); System. out. println (total); 5 - 150
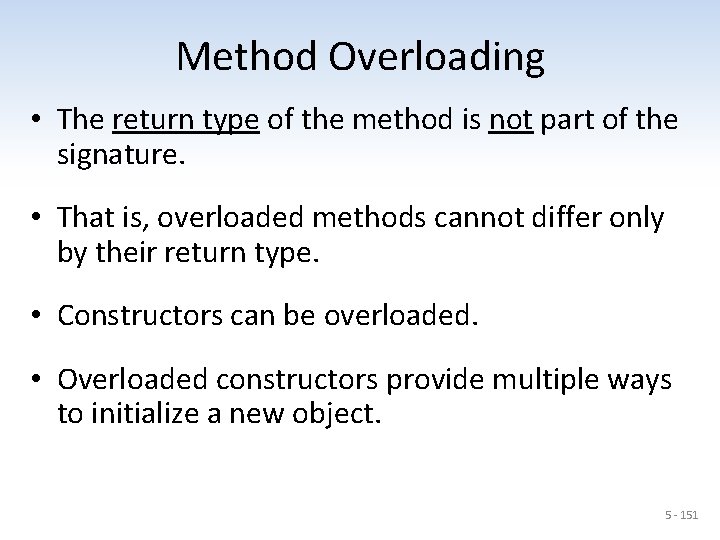
Method Overloading • The return type of the method is not part of the signature. • That is, overloaded methods cannot differ only by their return type. • Constructors can be overloaded. • Overloaded constructors provide multiple ways to initialize a new object. 5 - 151
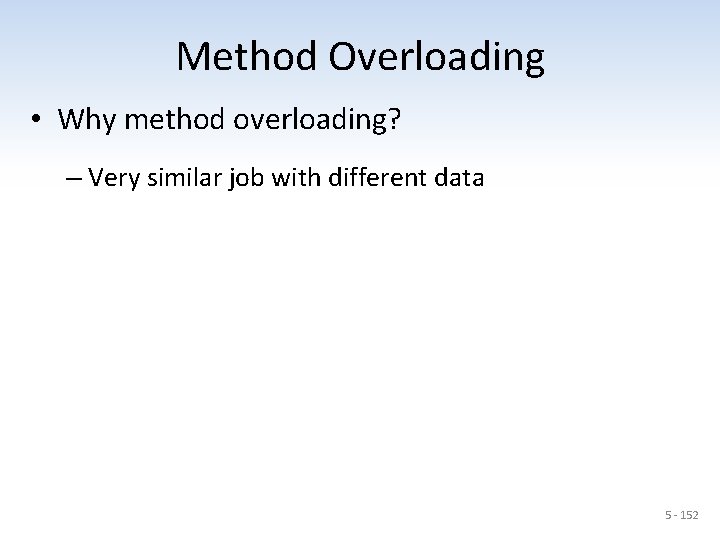
Method Overloading • Why method overloading? – Very similar job with different data 5 - 152
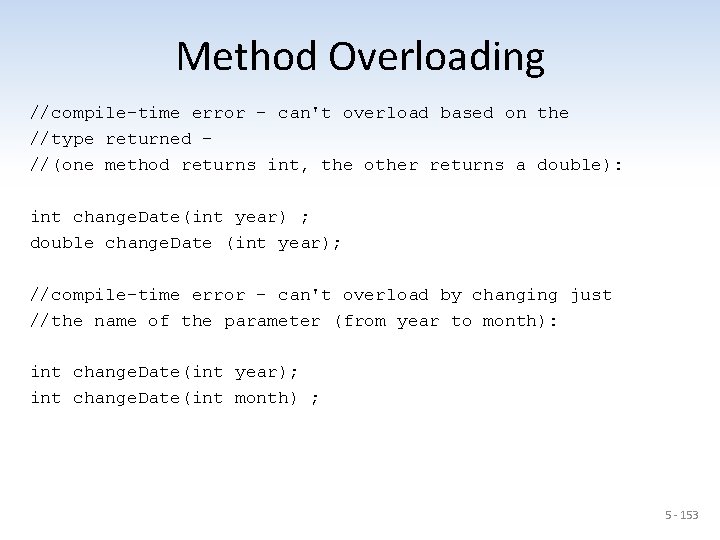
Method Overloading //compile-time error - can't overload based on the //type returned //(one method returns int, the other returns a double): int change. Date(int year) ; double change. Date (int year); //compile-time error - can't overload by changing just //the name of the parameter (from year to month): int change. Date(int year); int change. Date(int month) ; 5 - 153
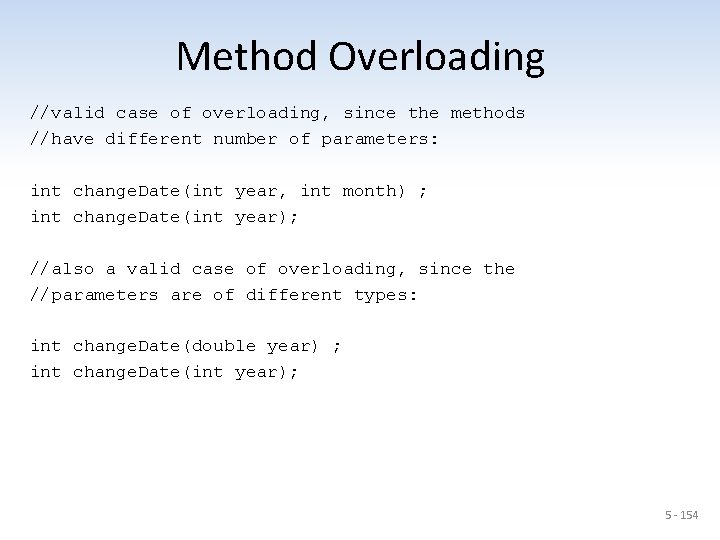
Method Overloading //valid case of overloading, since the methods //have different number of parameters: int change. Date(int year, int month) ; int change. Date(int year); //also a valid case of overloading, since the //parameters are of different types: int change. Date(double year) ; int change. Date(int year); 5 - 154
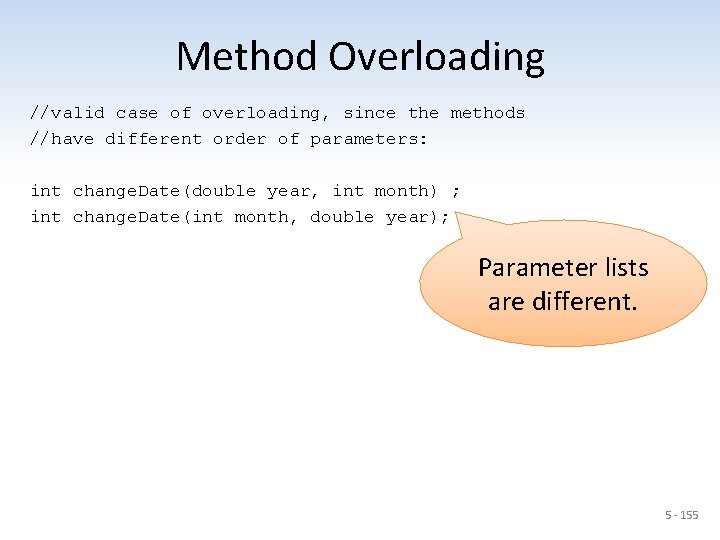
Method Overloading //valid case of overloading, since the methods //have different order of parameters: int change. Date(double year, int month) ; int change. Date(int month, double year); Parameter lists are different. 5 - 155
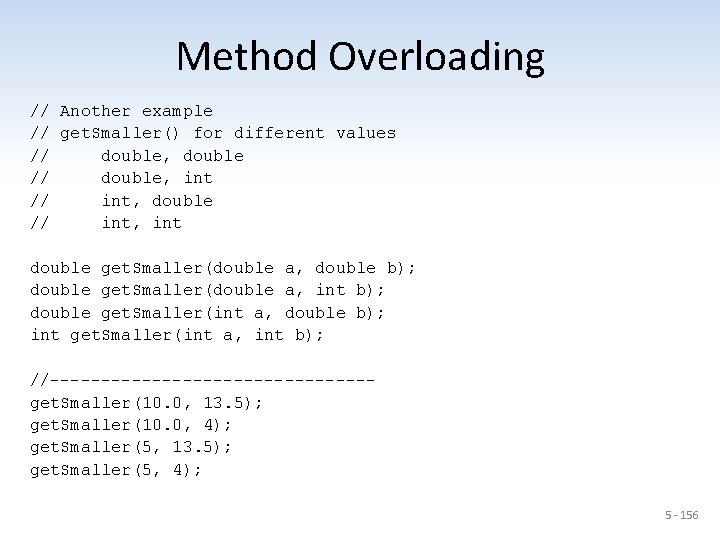
Method Overloading // Another example // get. Smaller() for different values // double, double // double, int // int, double // int, int double get. Smaller(double a, double b); double get. Smaller(double a, int b); double get. Smaller(int a, double b); int get. Smaller(int a, int b); //----------------get. Smaller(10. 0, 13. 5); get. Smaller(10. 0, 4); get. Smaller(5, 13. 5); get. Smaller(5, 4); 5 - 156
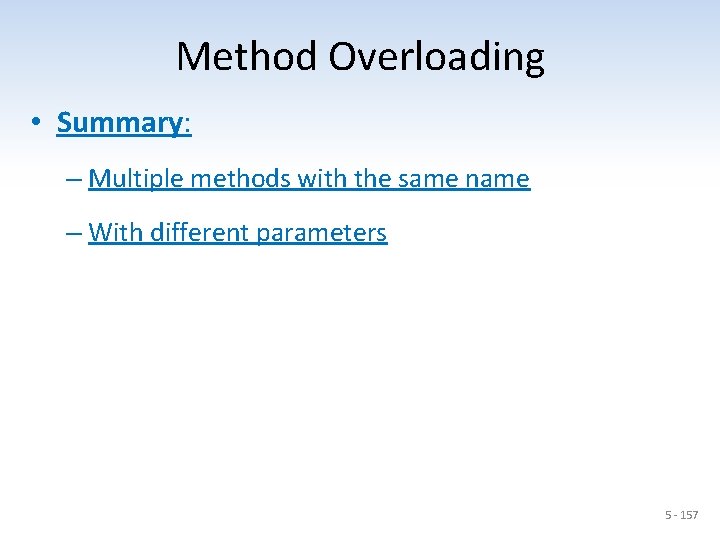
Method Overloading • Summary: – Multiple methods with the same name – With different parameters 5 - 157
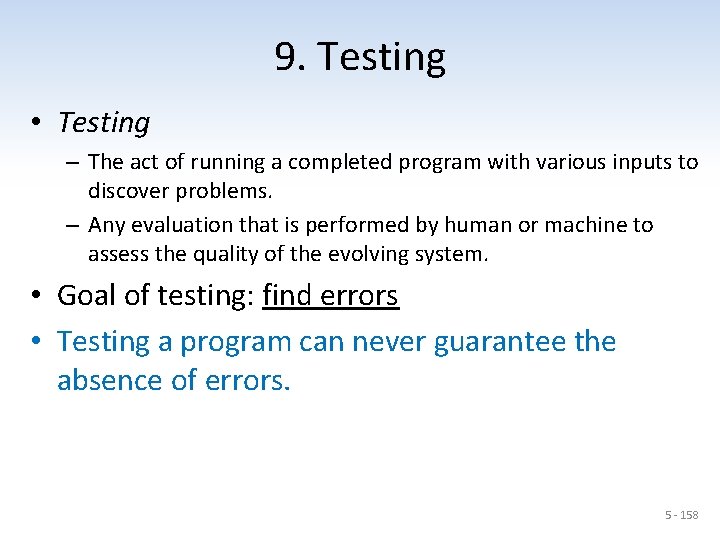
9. Testing • Testing – The act of running a completed program with various inputs to discover problems. – Any evaluation that is performed by human or machine to assess the quality of the evolving system. • Goal of testing: find errors • Testing a program can never guarantee the absence of errors. 5 - 158
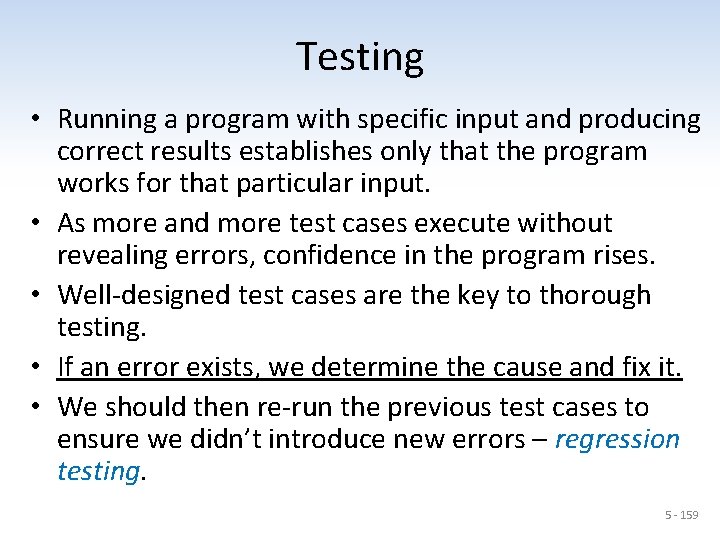
Testing • Running a program with specific input and producing correct results establishes only that the program works for that particular input. • As more and more test cases execute without revealing errors, confidence in the program rises. • Well-designed test cases are the key to thorough testing. • If an error exists, we determine the cause and fix it. • We should then re-run the previous test cases to ensure we didn’t introduce new errors – regression testing. 5 - 159
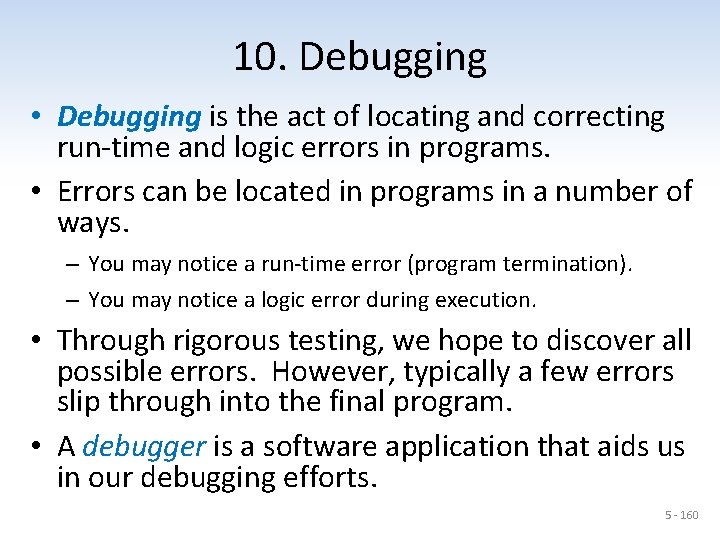
10. Debugging • Debugging is the act of locating and correcting run-time and logic errors in programs. • Errors can be located in programs in a number of ways. – You may notice a run-time error (program termination). – You may notice a logic error during execution. • Through rigorous testing, we hope to discover all possible errors. However, typically a few errors slip through into the final program. • A debugger is a software application that aids us in our debugging efforts. 5 - 160
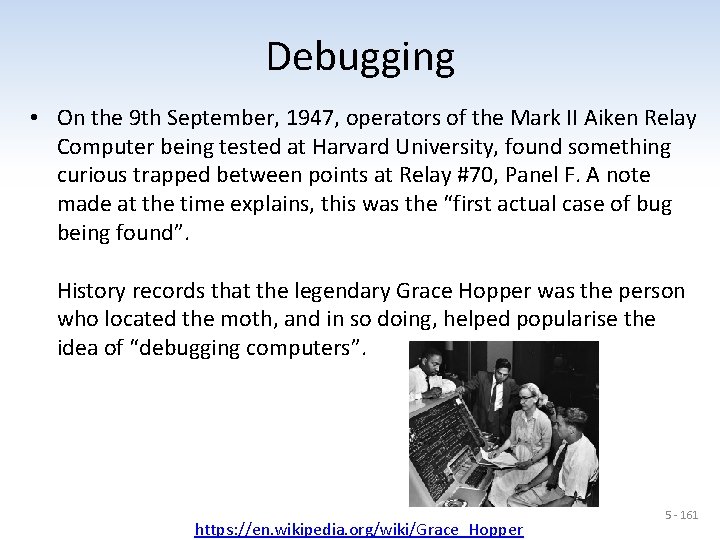
Debugging • On the 9 th September, 1947, operators of the Mark II Aiken Relay Computer being tested at Harvard University, found something curious trapped between points at Relay #70, Panel F. A note made at the time explains, this was the “first actual case of bug being found”. History records that the legendary Grace Hopper was the person who located the moth, and in so doing, helped popularise the idea of “debugging computers”. https: //en. wikipedia. org/wiki/Grace_Hopper 5 - 161
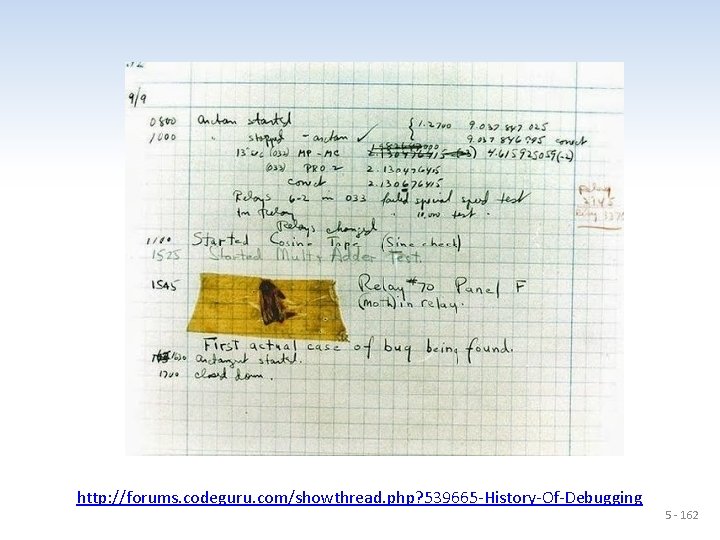
http: //forums. codeguru. com/showthread. php? 539665 -History-Of-Debugging 5 - 162
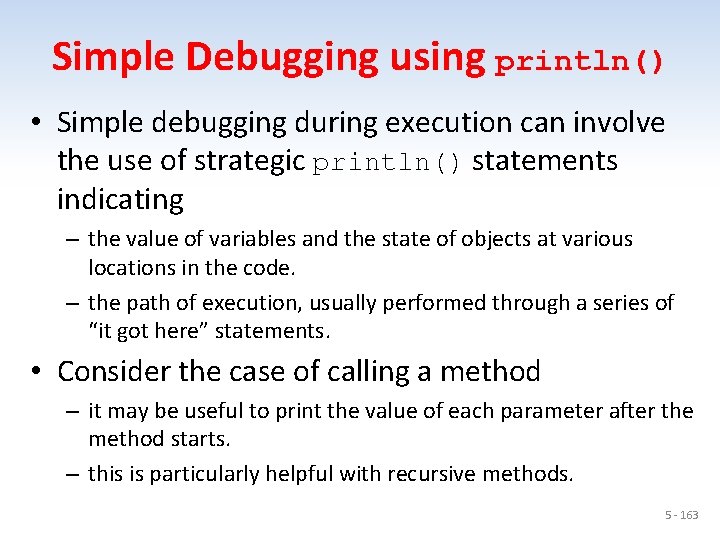
Simple Debugging using println() • Simple debugging during execution can involve the use of strategic println() statements indicating – the value of variables and the state of objects at various locations in the code. – the path of execution, usually performed through a series of “it got here” statements. • Consider the case of calling a method – it may be useful to print the value of each parameter after the method starts. – this is particularly helpful with recursive methods. 5 - 163