Chapter 2 Primitive Types and Simple IO 20201122
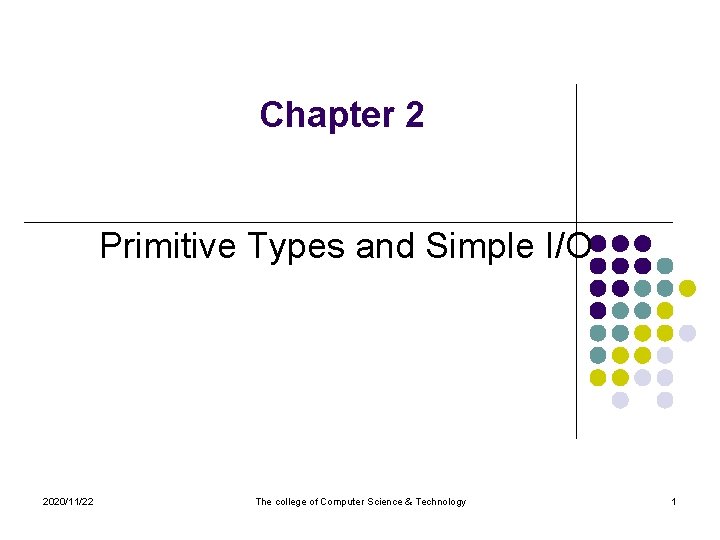
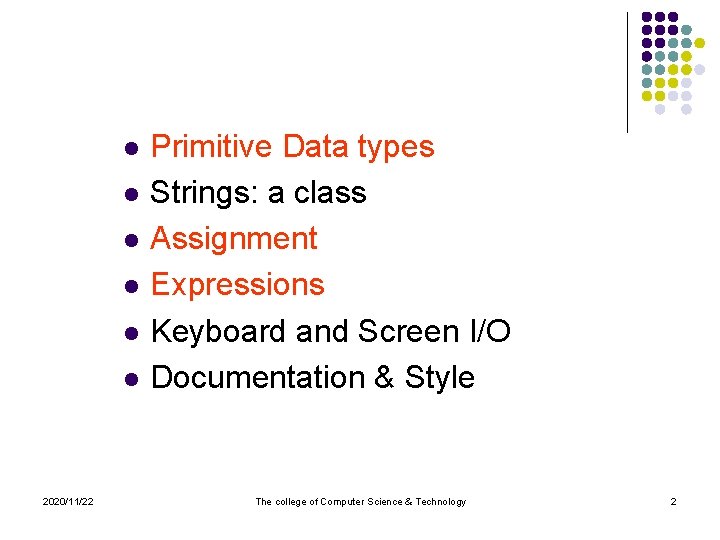
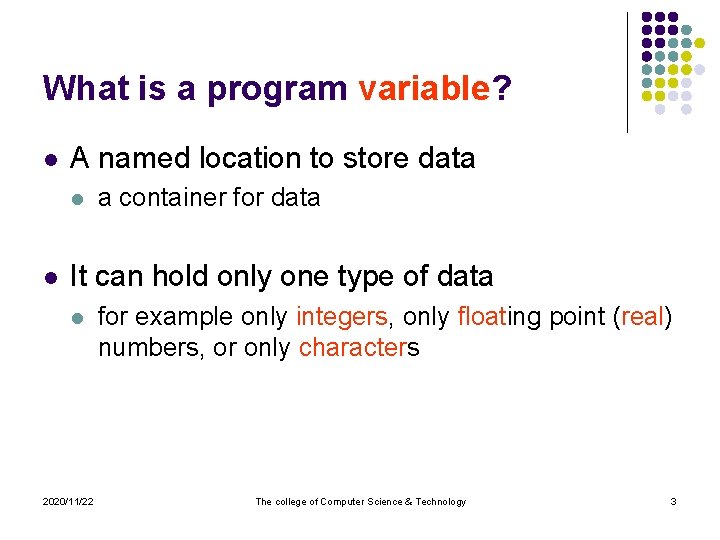
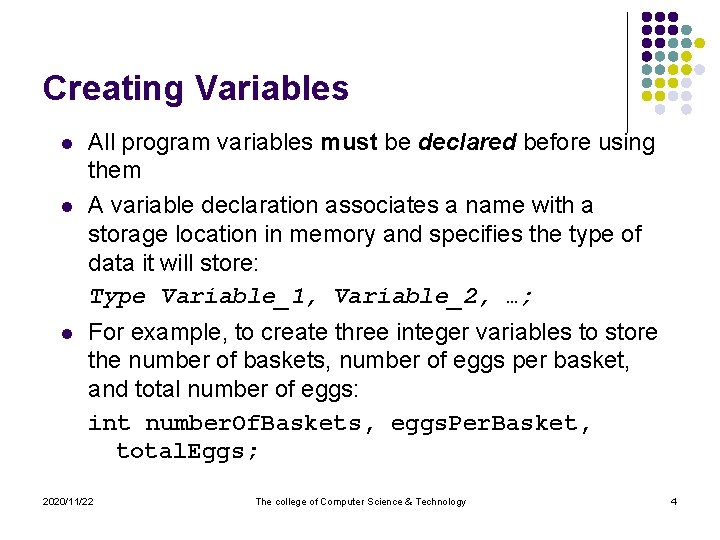
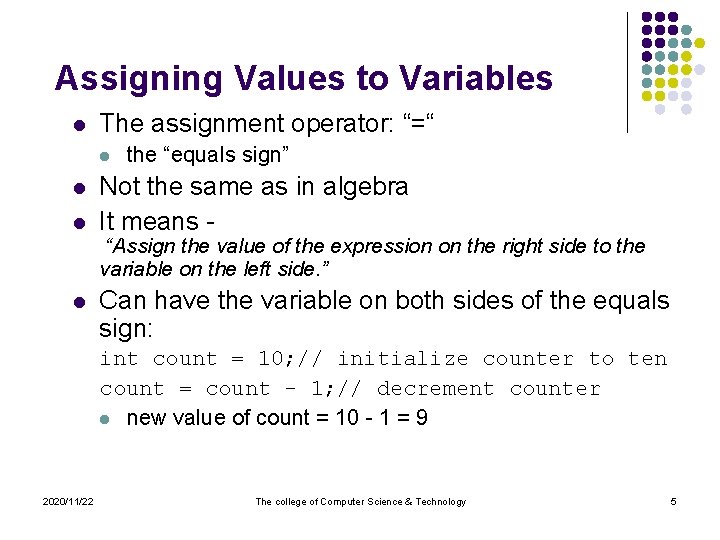
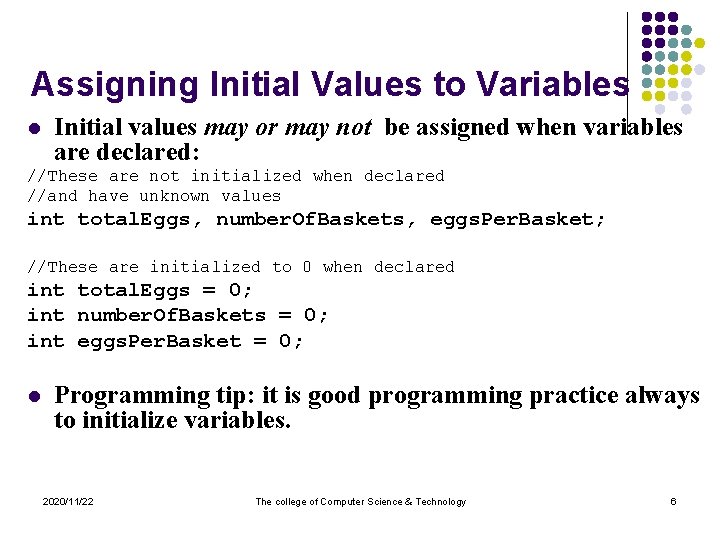
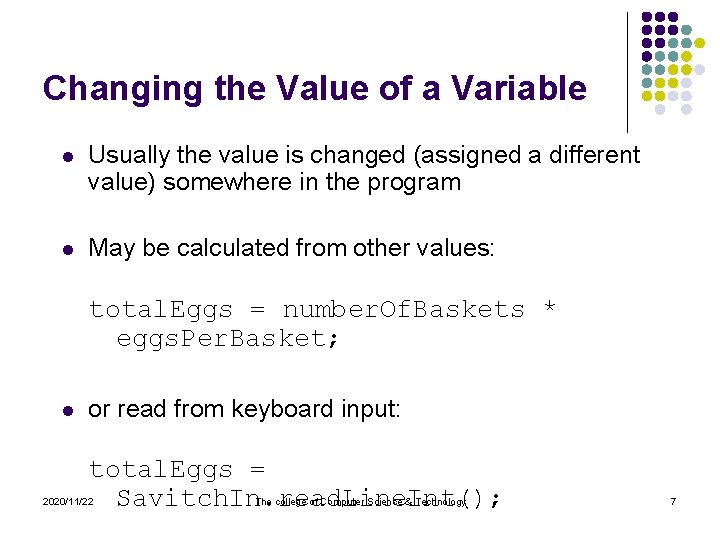
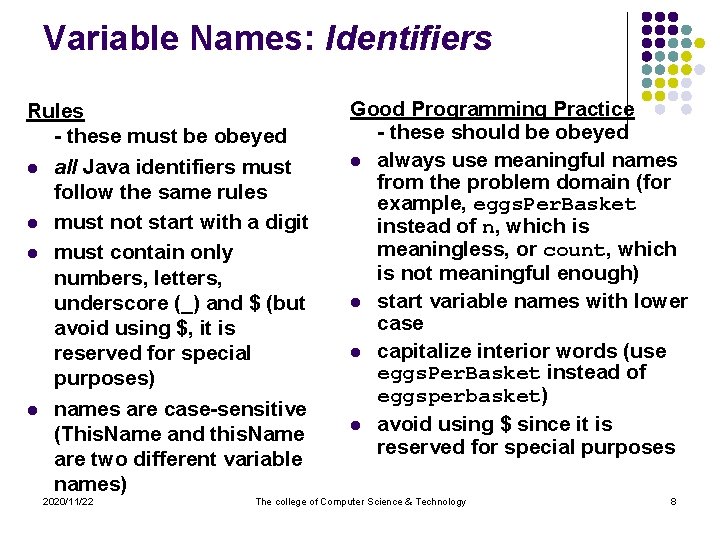
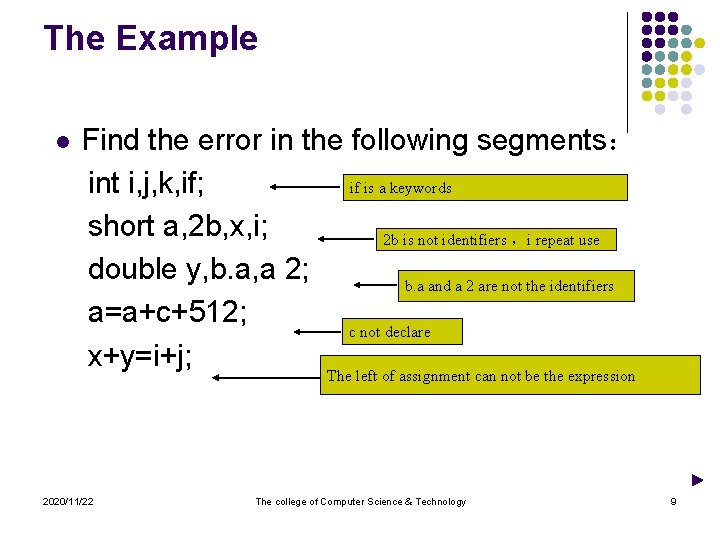
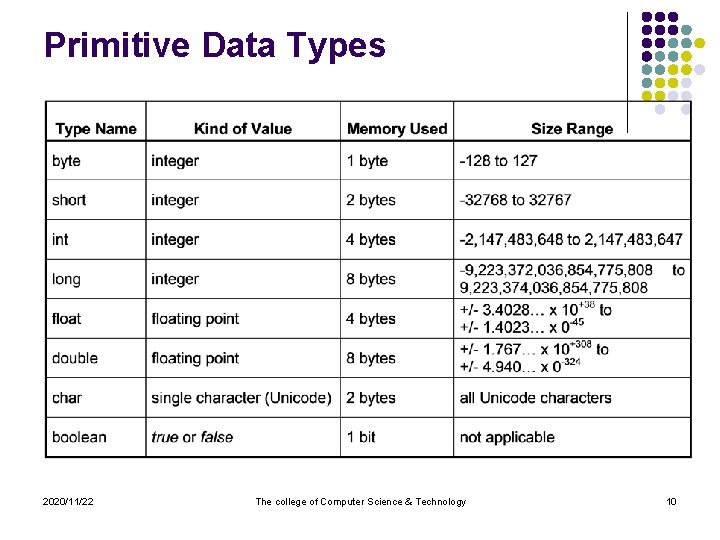
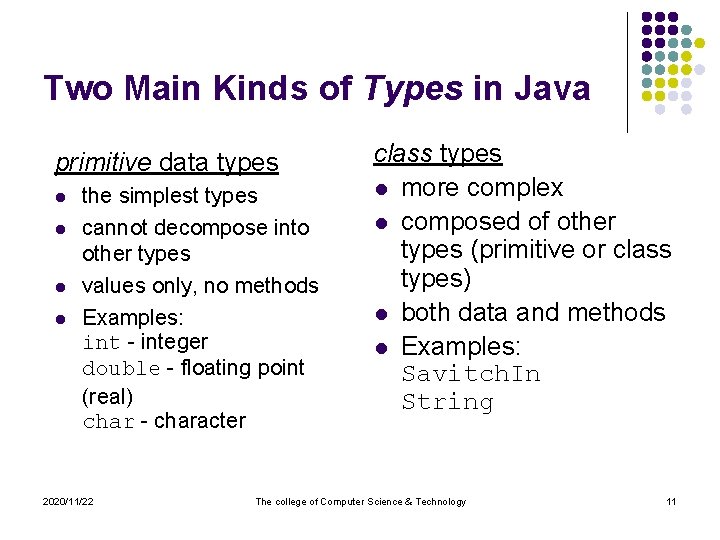
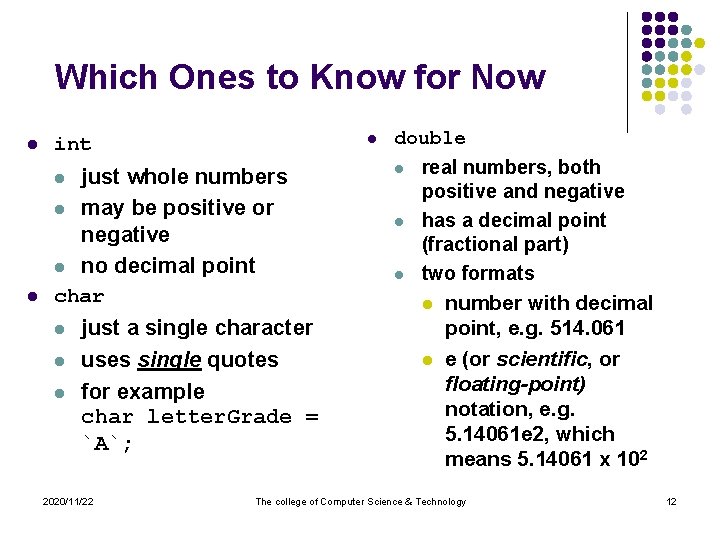
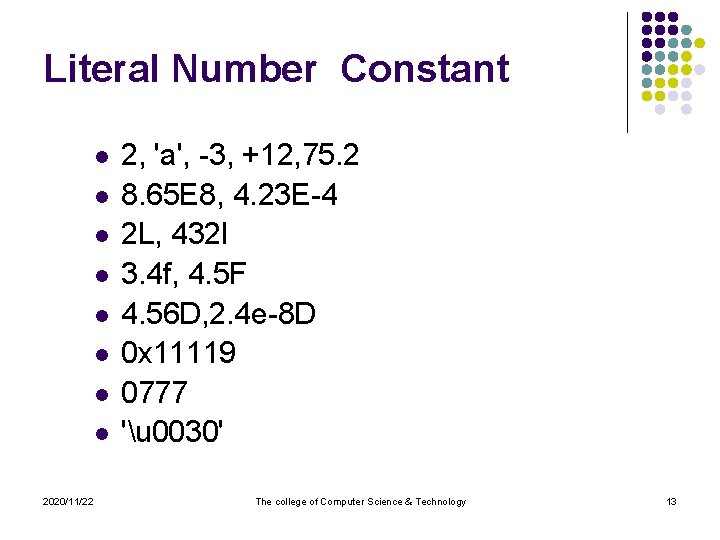
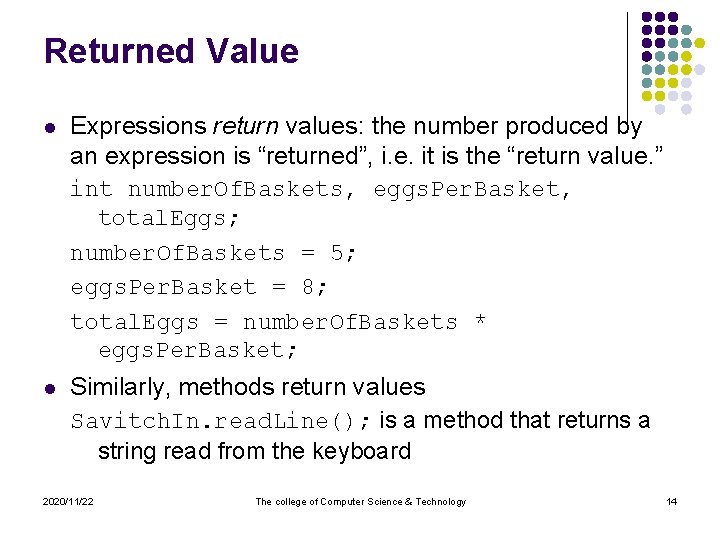
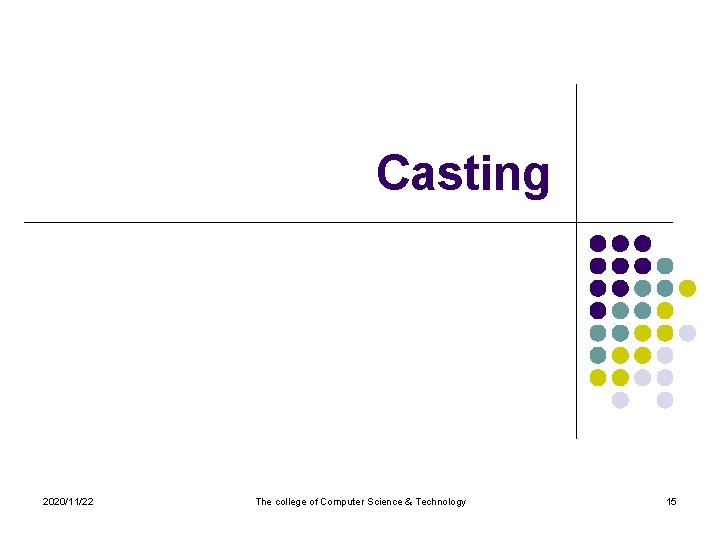
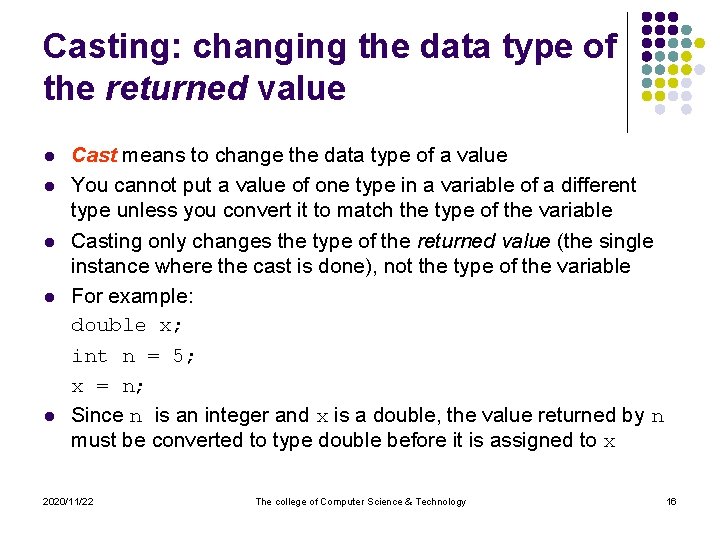
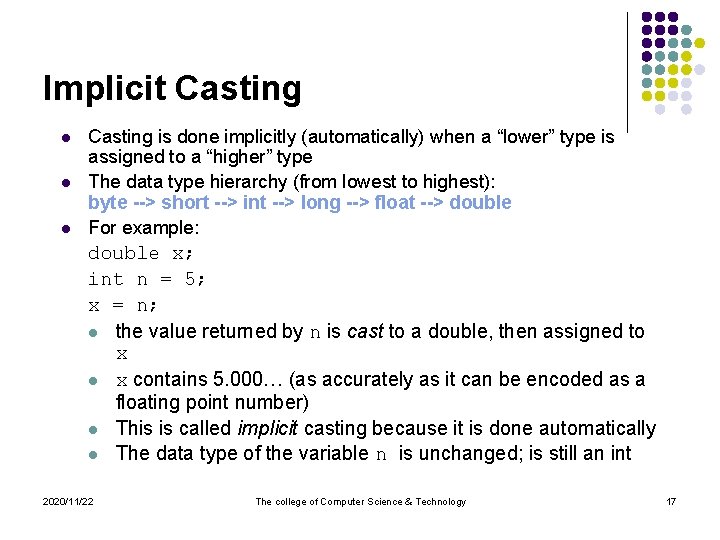
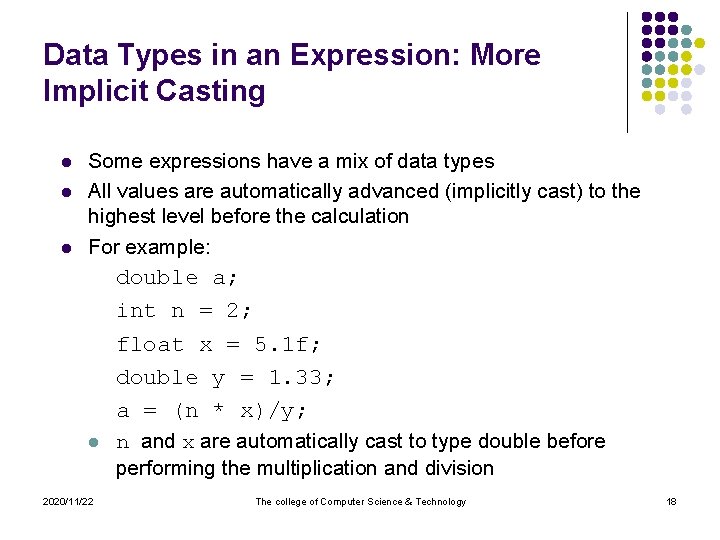
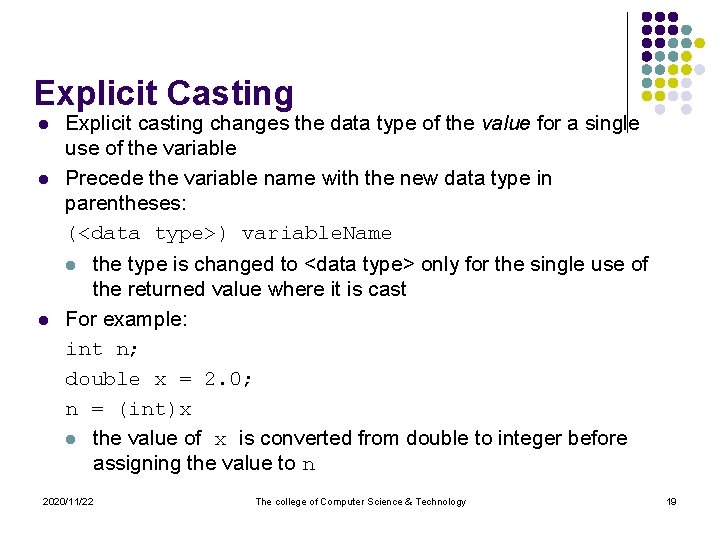
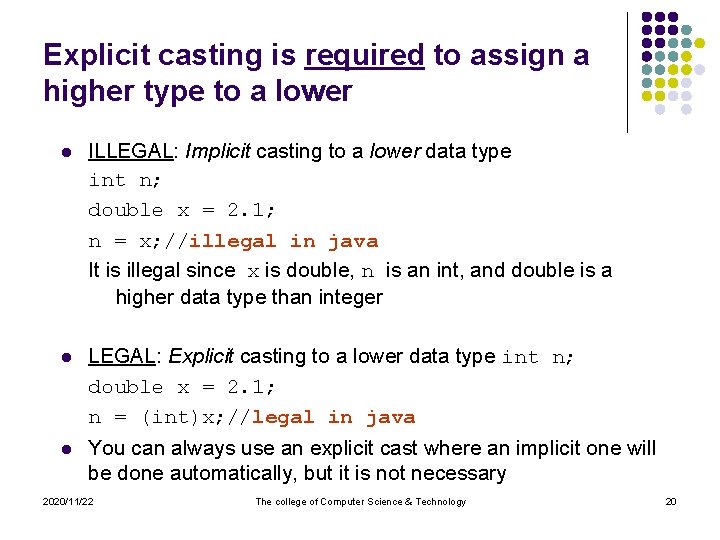
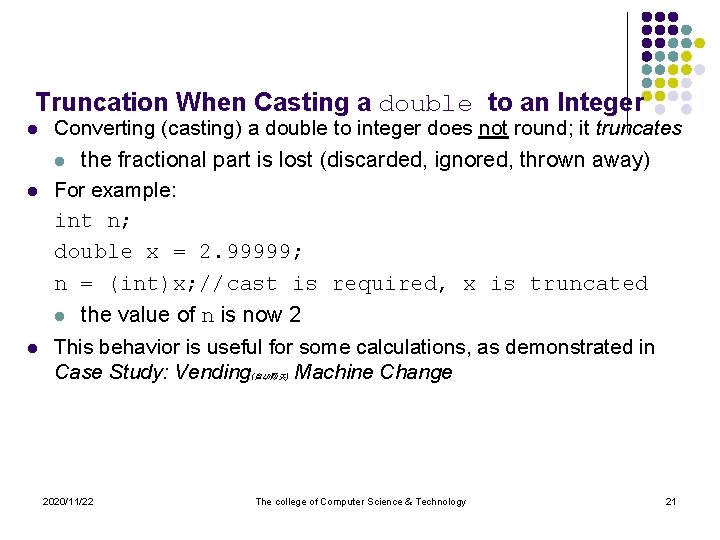
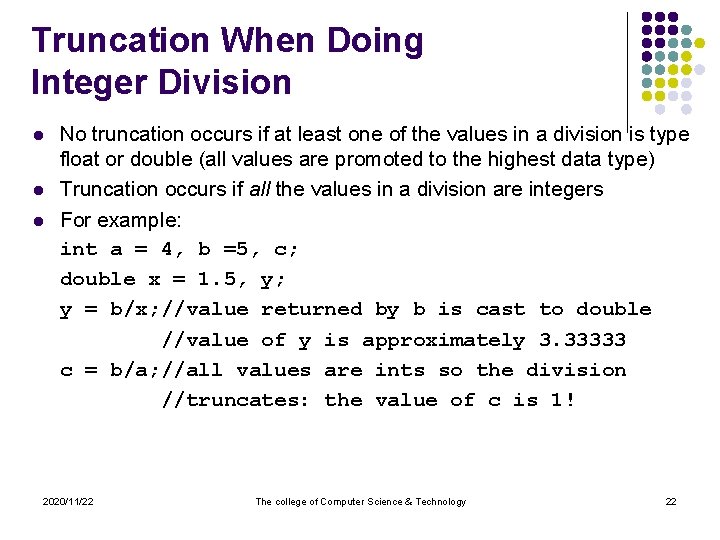
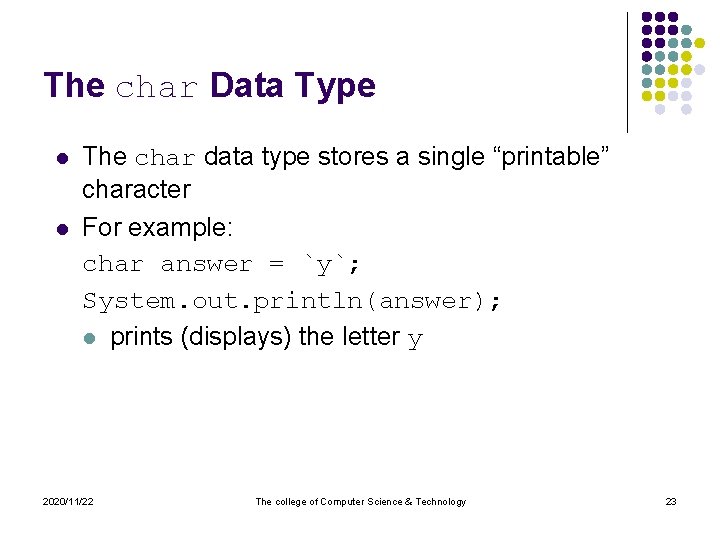
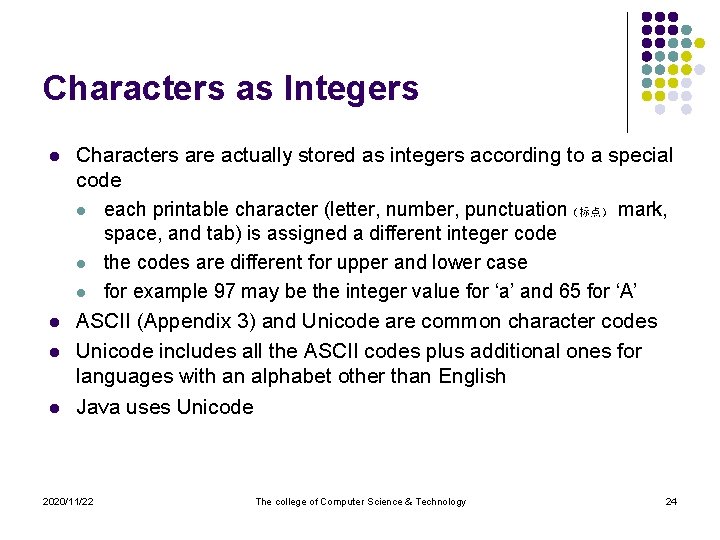
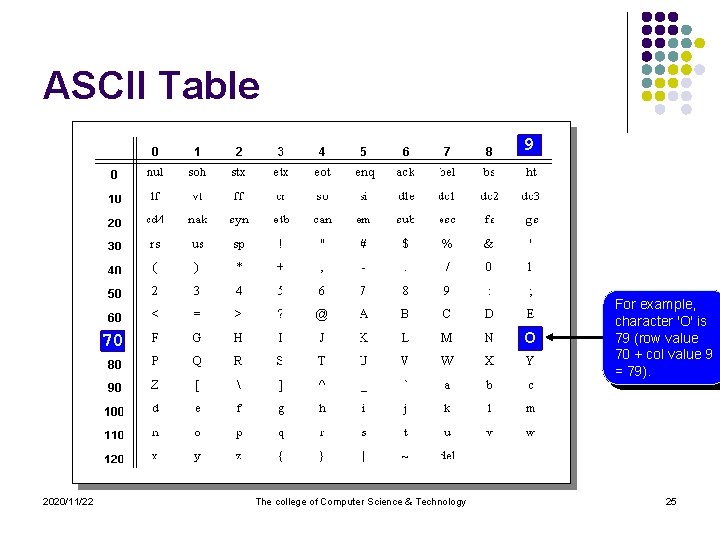
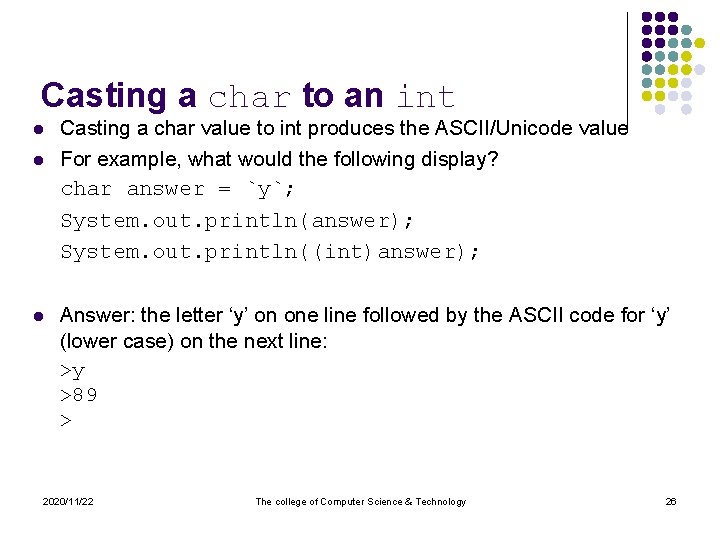
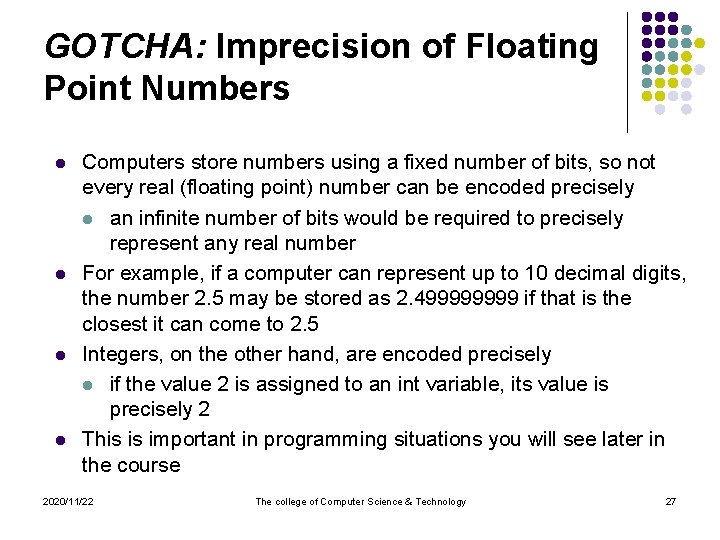
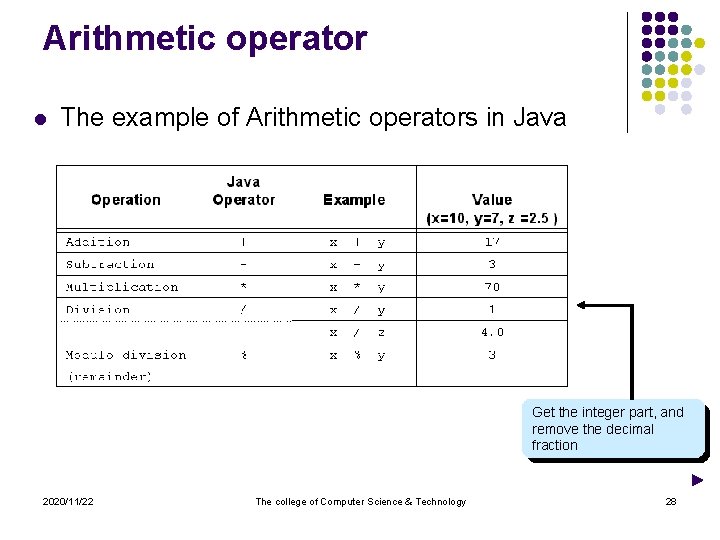
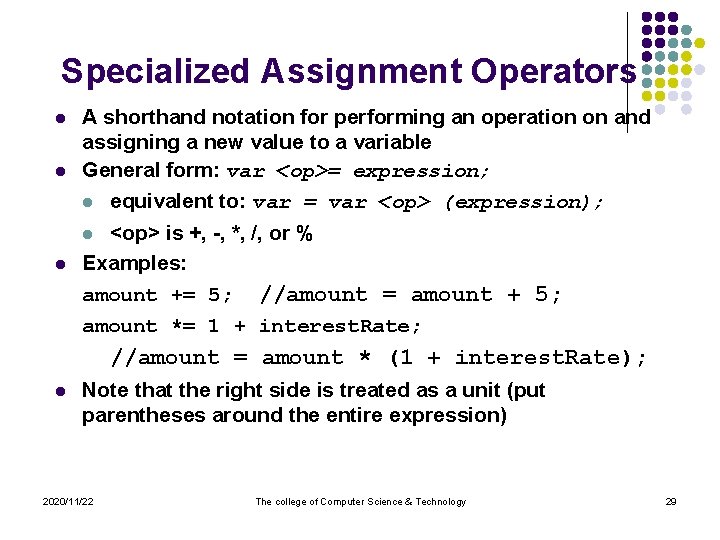
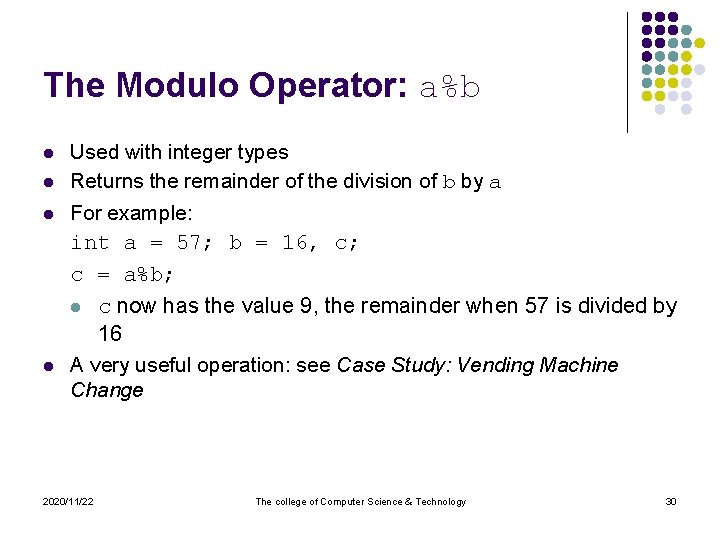
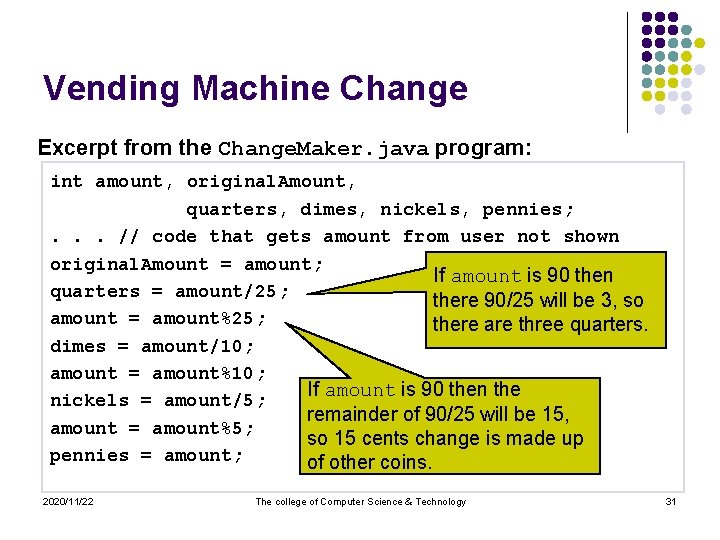
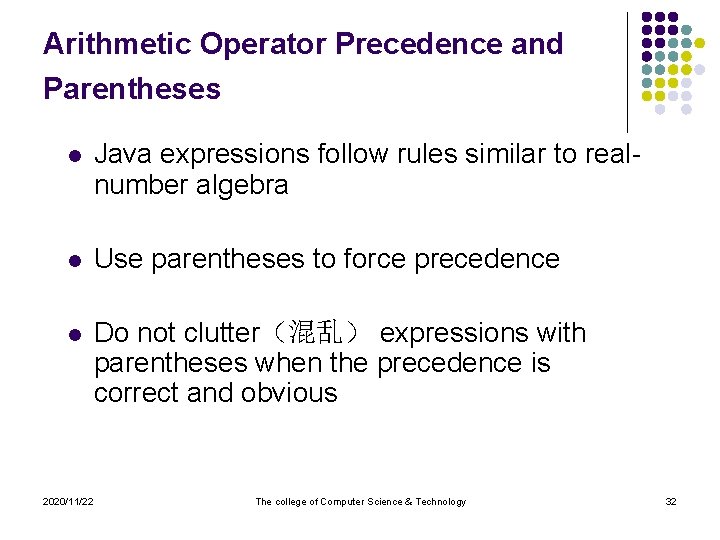
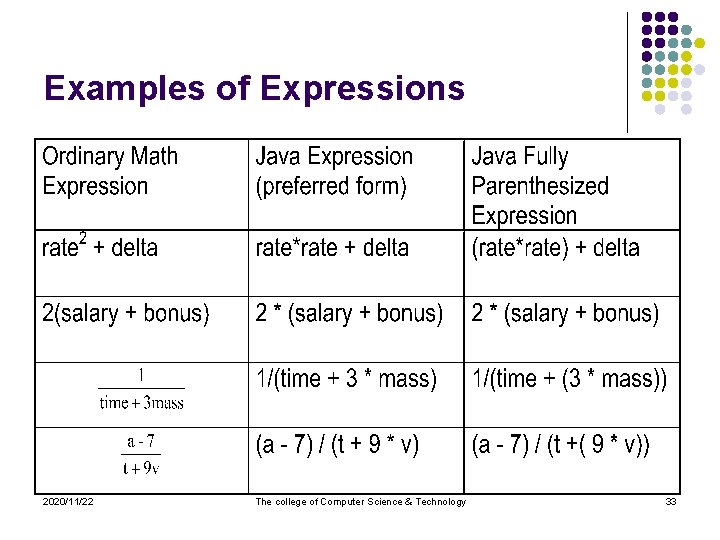
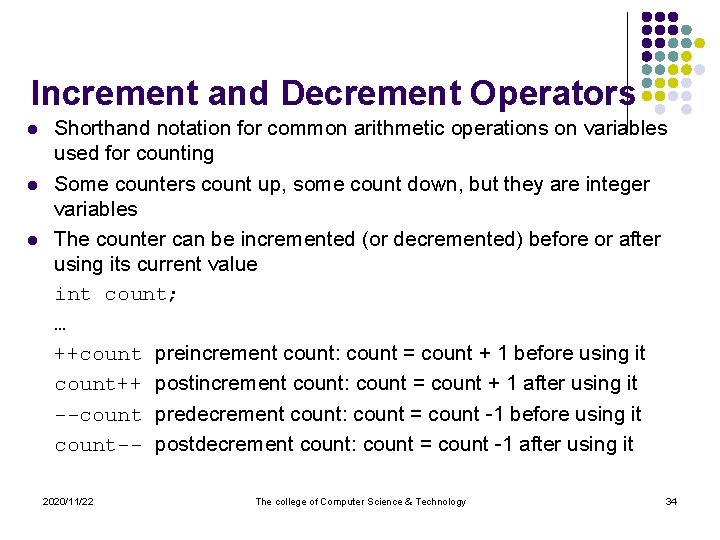
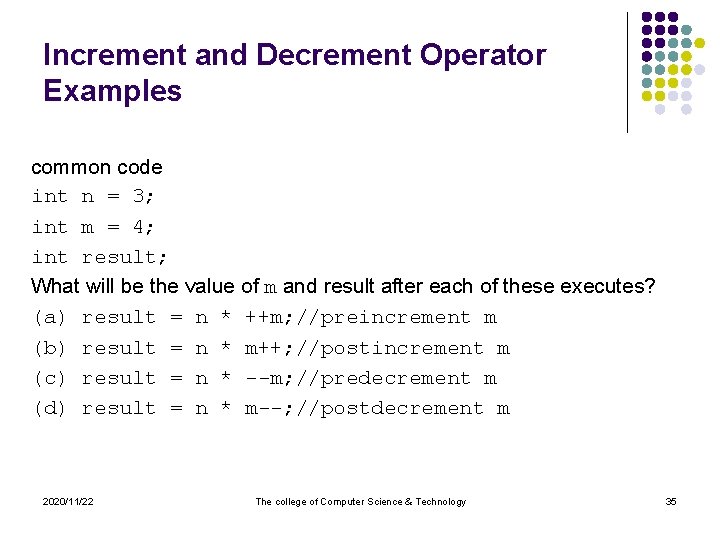
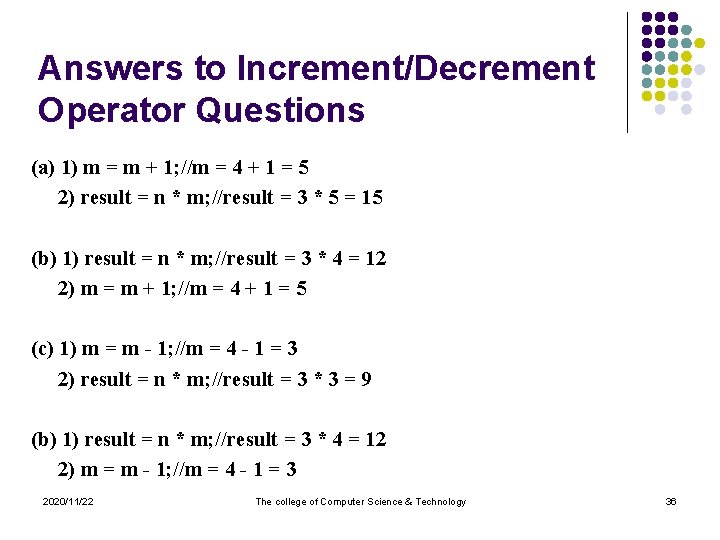
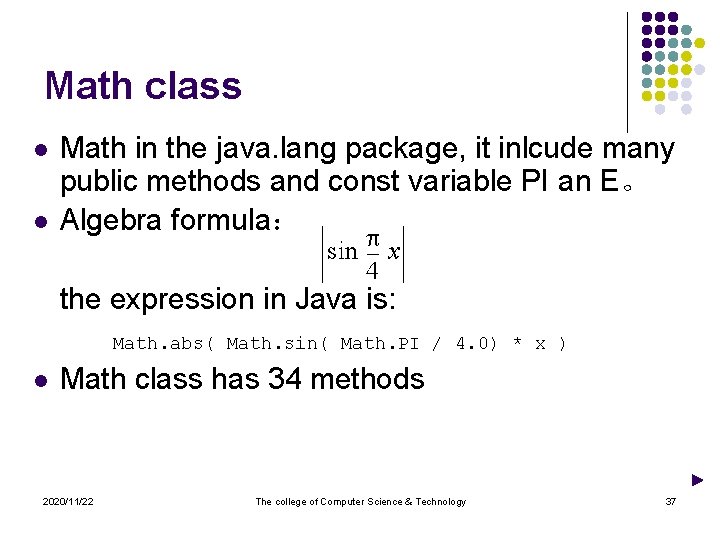
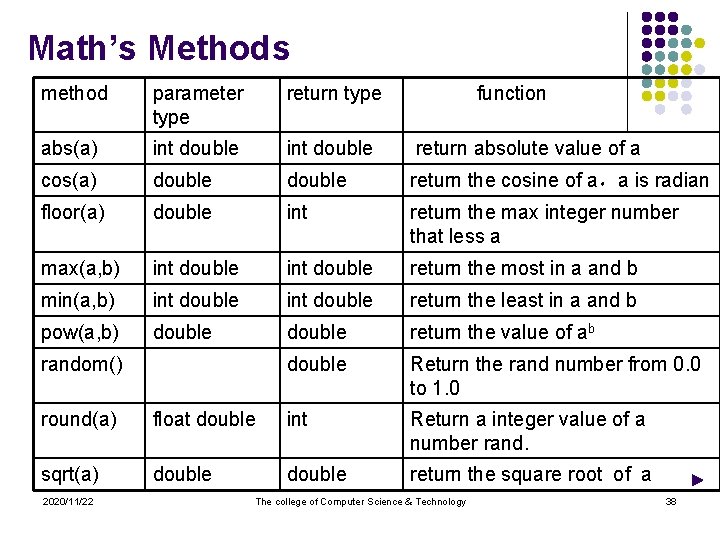
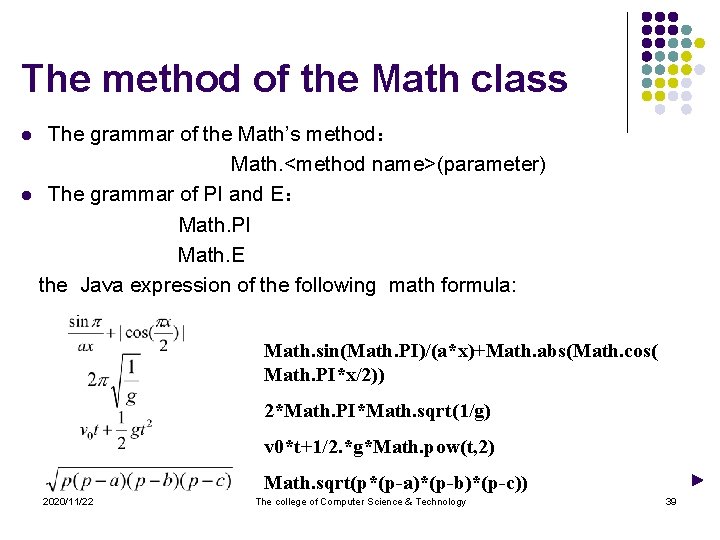
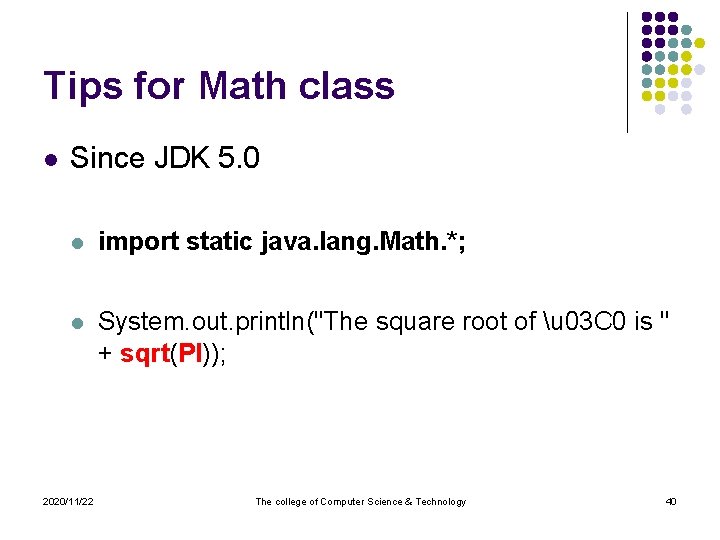
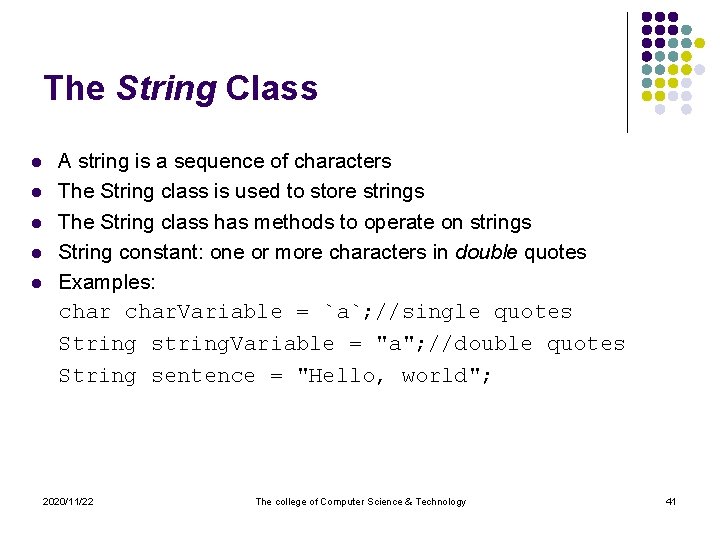
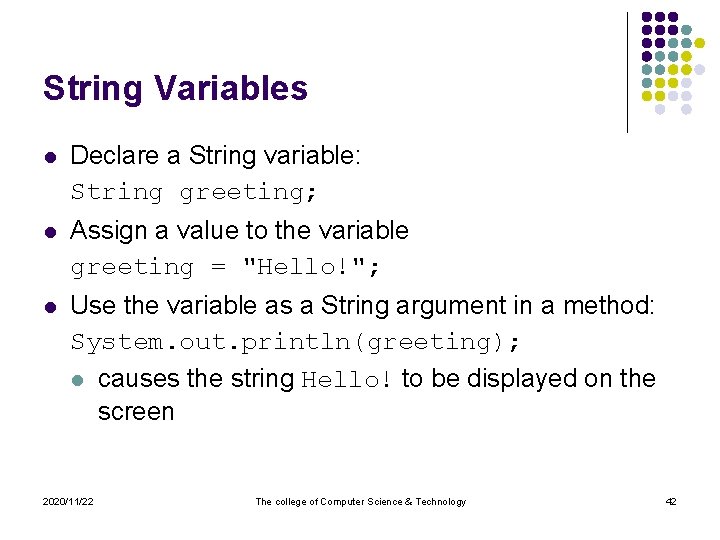
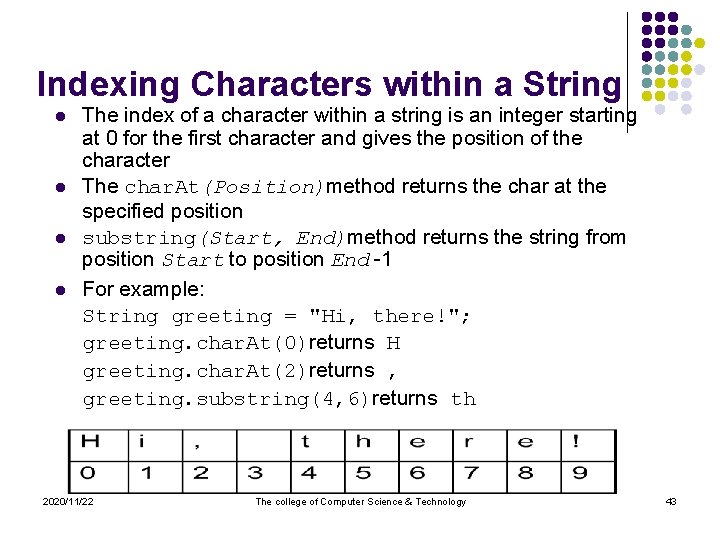
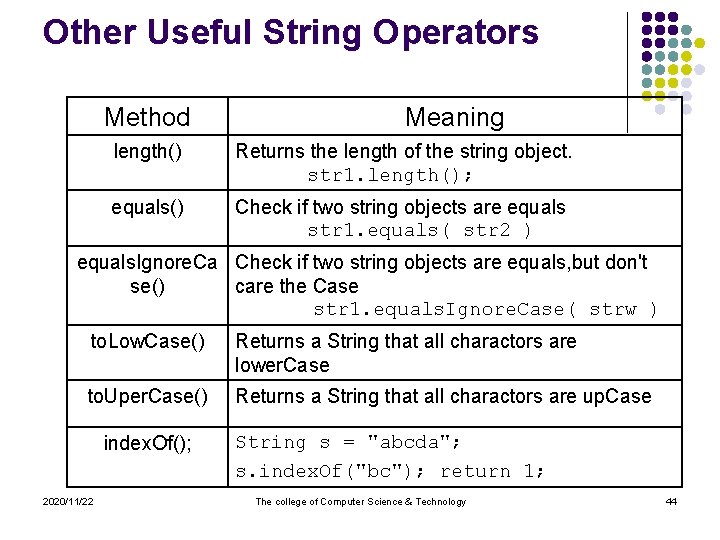
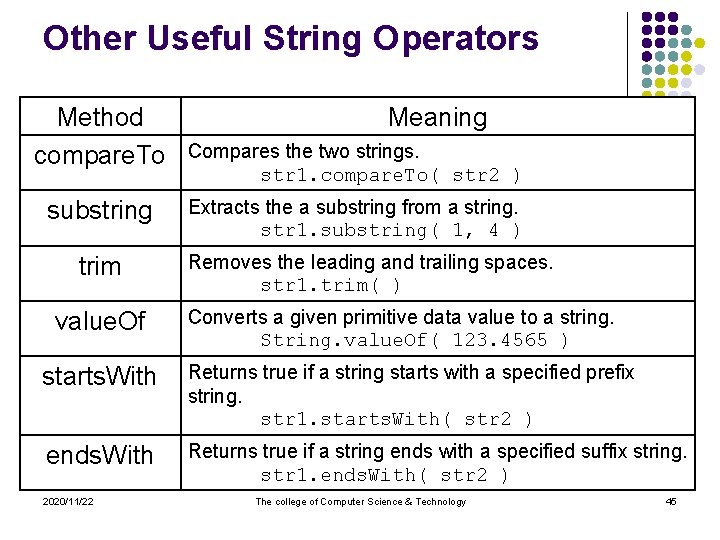
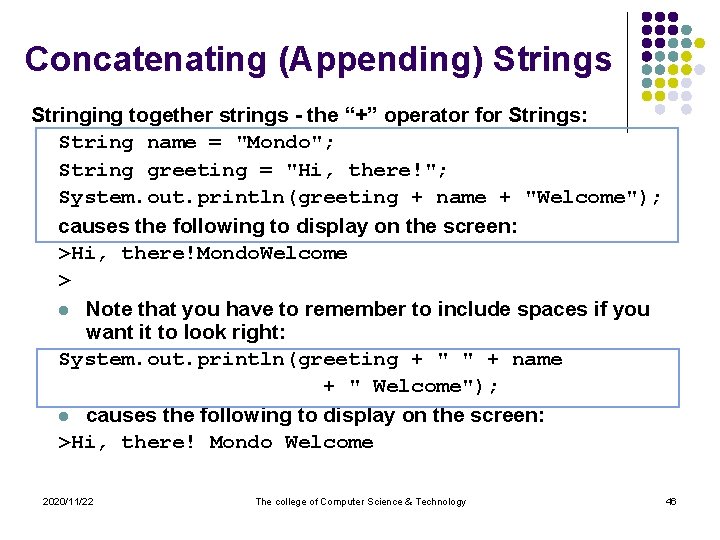
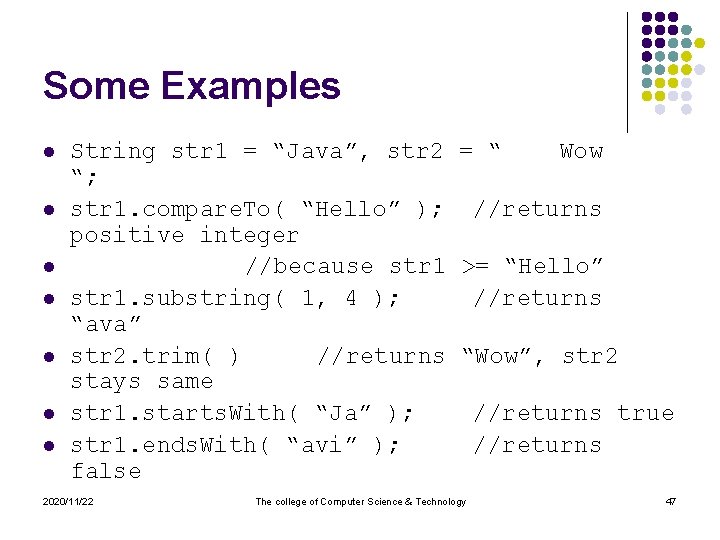
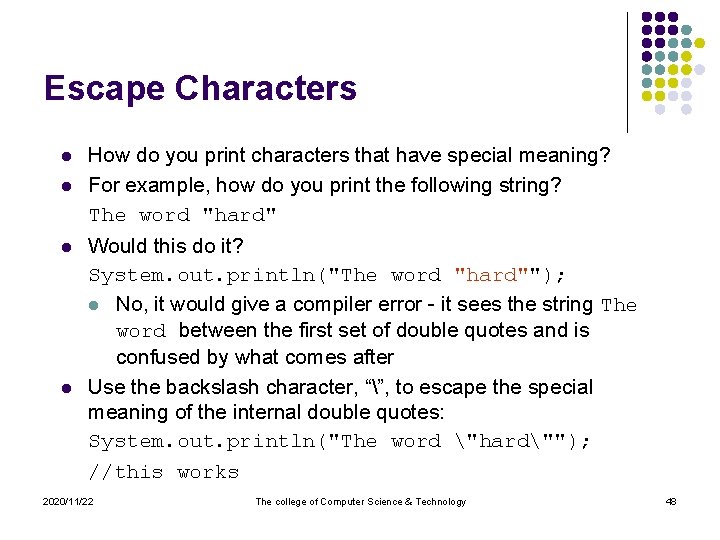
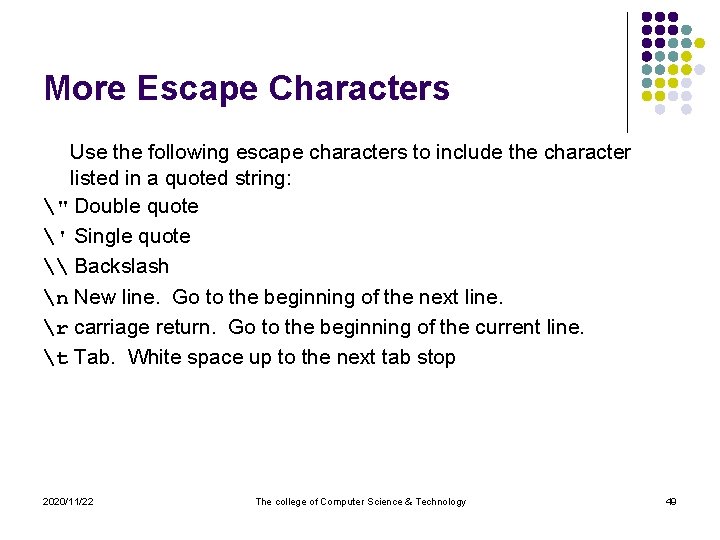
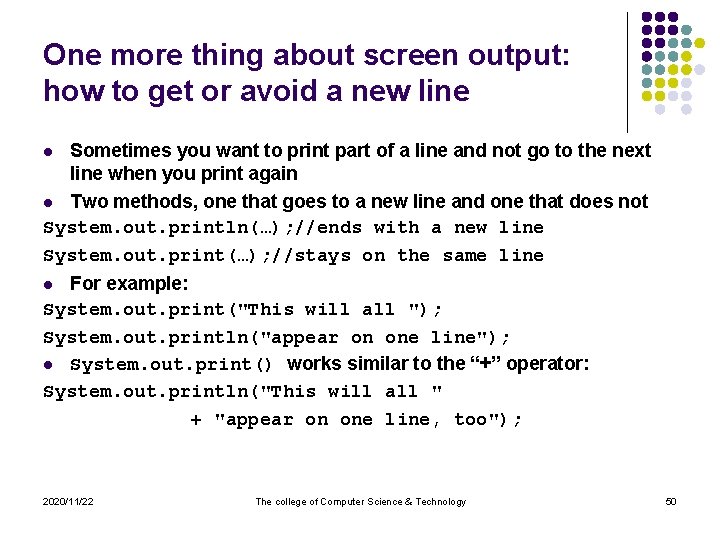
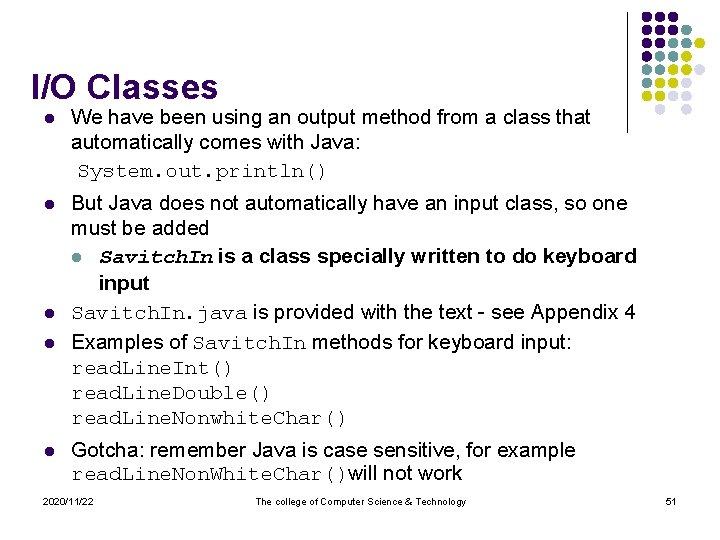
![I/O Classes import java. util. *; public class Input. Test{ public static void main(String[] I/O Classes import java. util. *; public class Input. Test{ public static void main(String[]](https://slidetodoc.com/presentation_image_h/87835e6389c3fef224397967370b5e16/image-52.jpg)
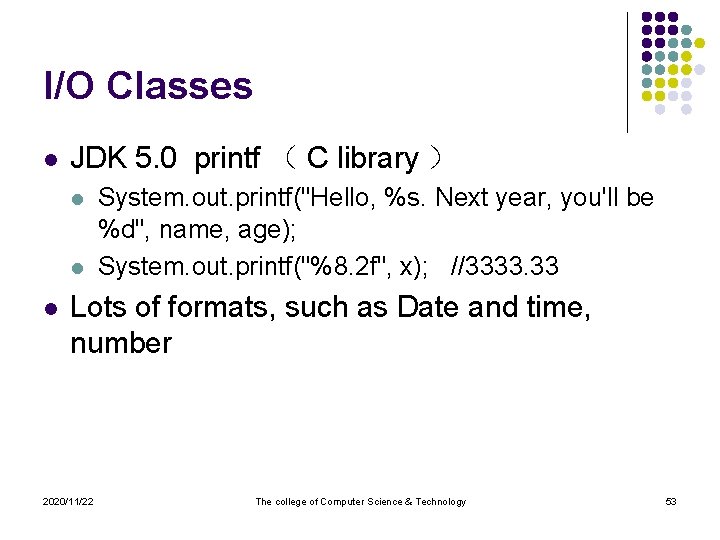
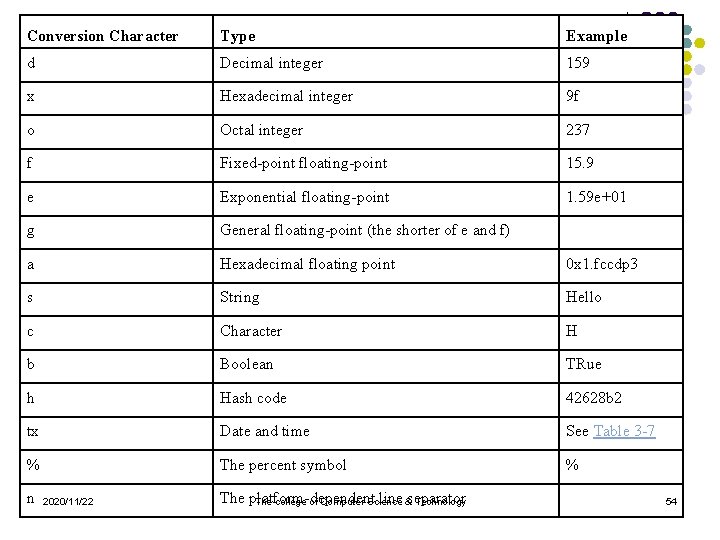
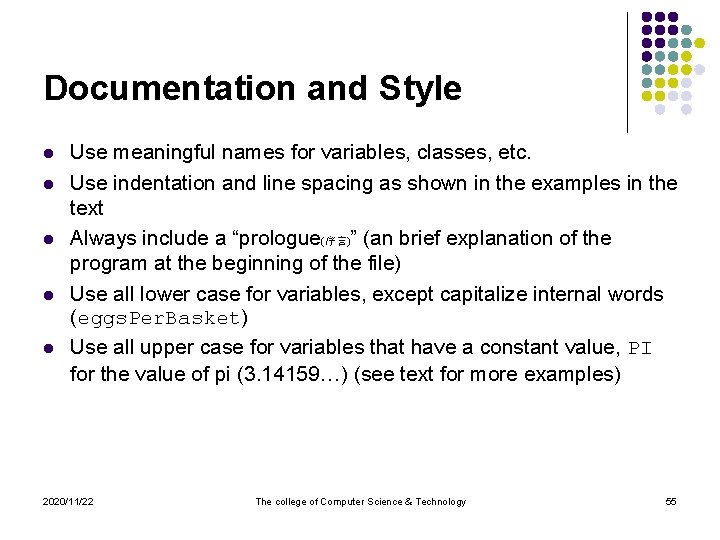
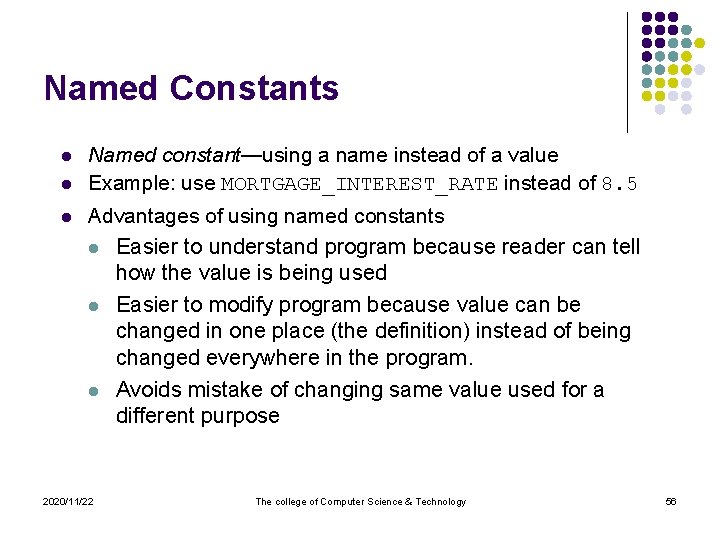
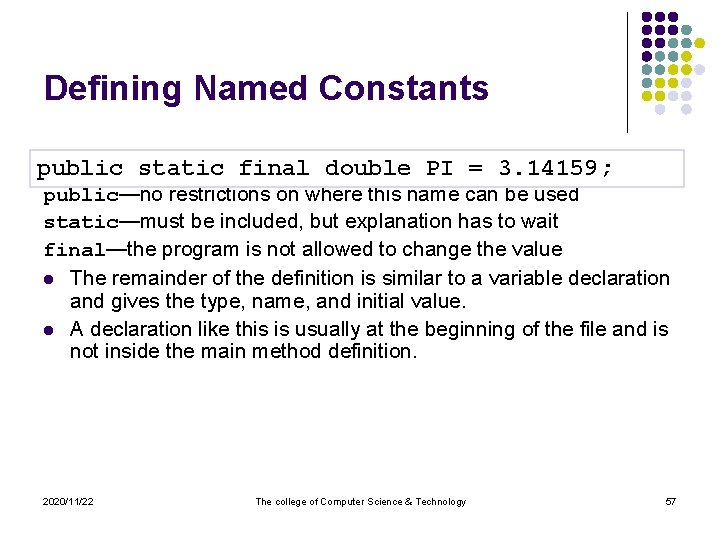
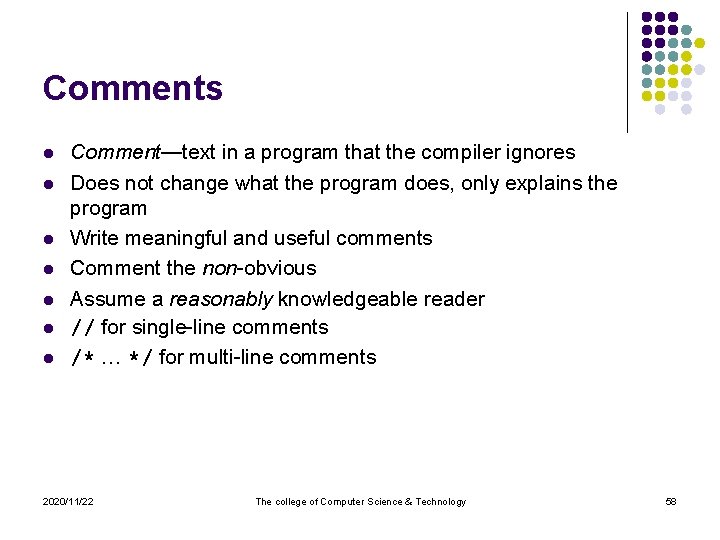
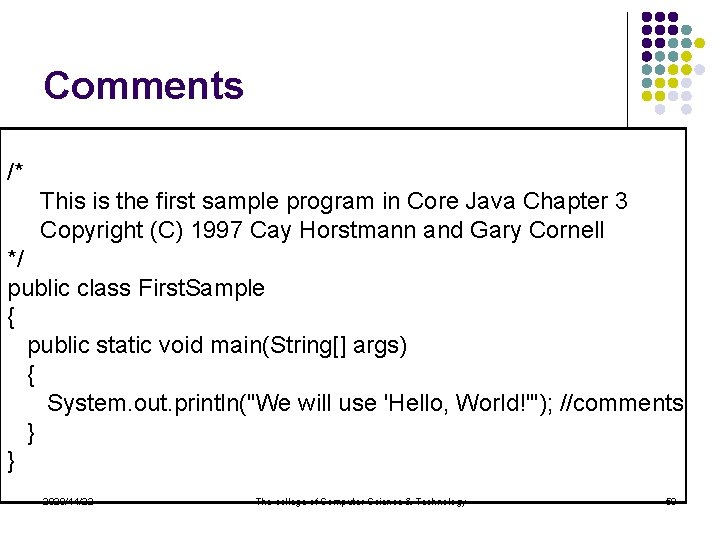
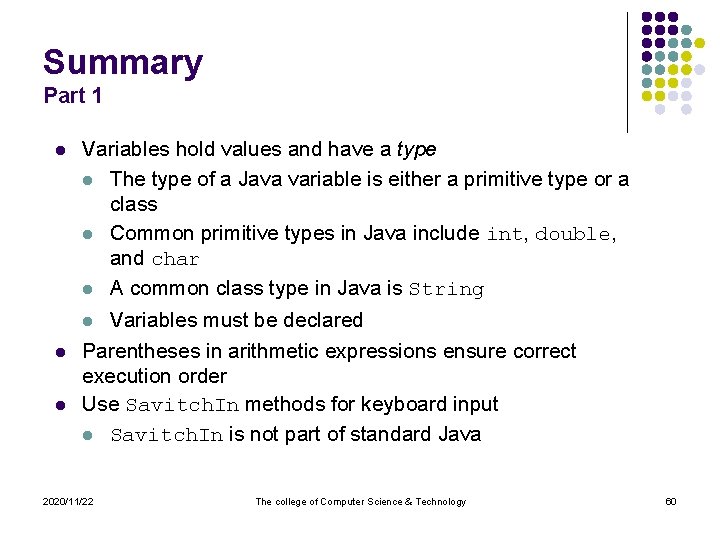
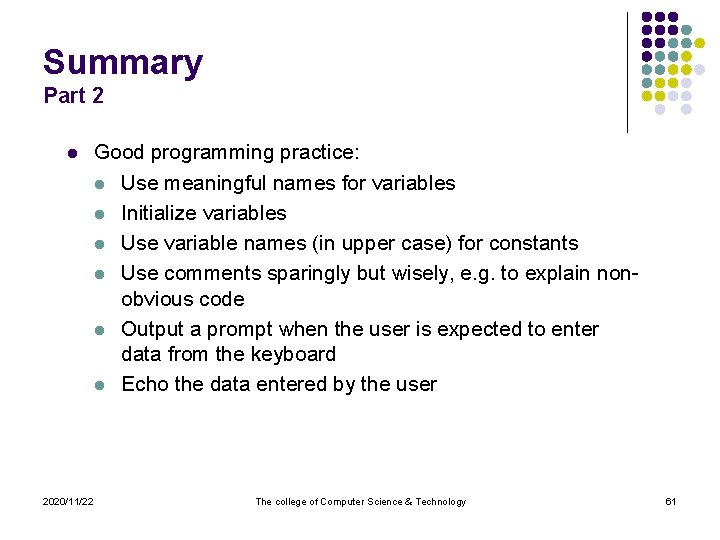
- Slides: 61
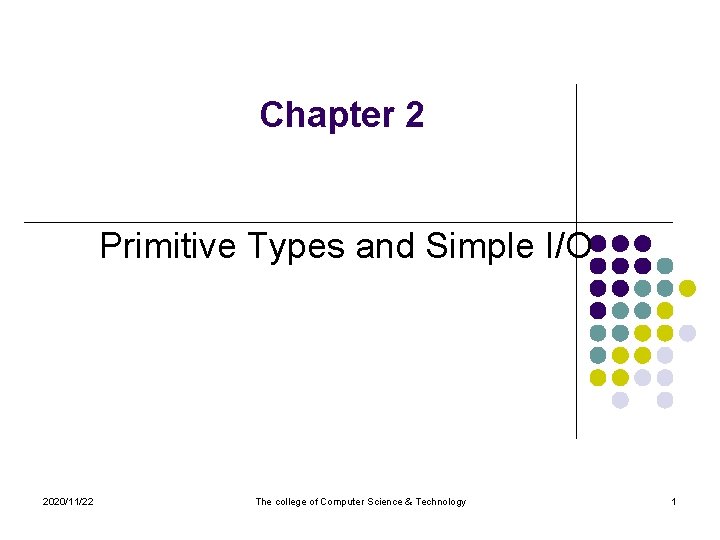
Chapter 2 Primitive Types and Simple I/O 2020/11/22 The college of Computer Science & Technology 1
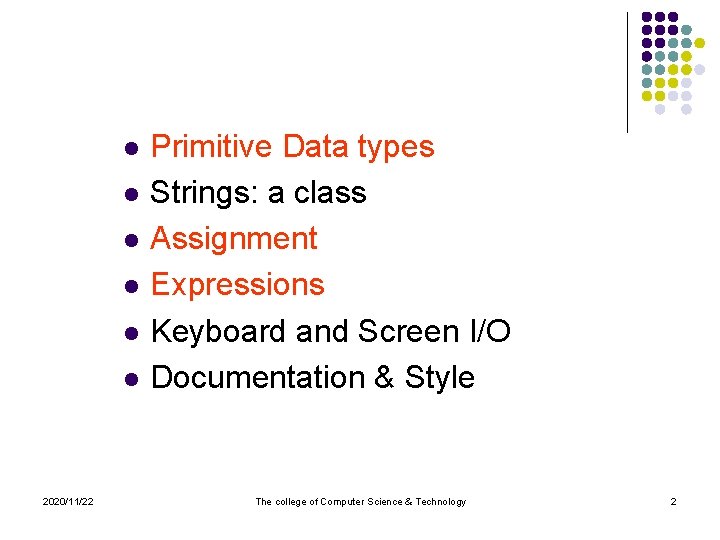
l l l 2020/11/22 Primitive Data types Strings: a class Assignment Expressions Keyboard and Screen I/O Documentation & Style The college of Computer Science & Technology 2
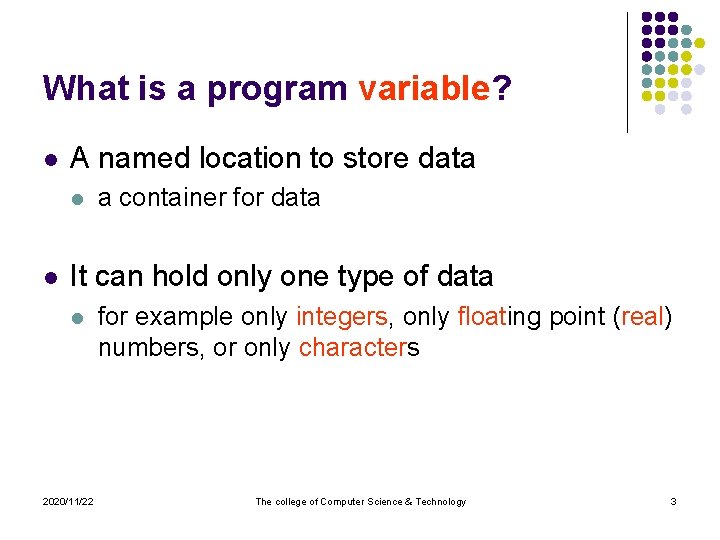
What is a program variable? l A named location to store data l l a container for data It can hold only one type of data l 2020/11/22 for example only integers, only floating point (real) numbers, or only characters The college of Computer Science & Technology 3
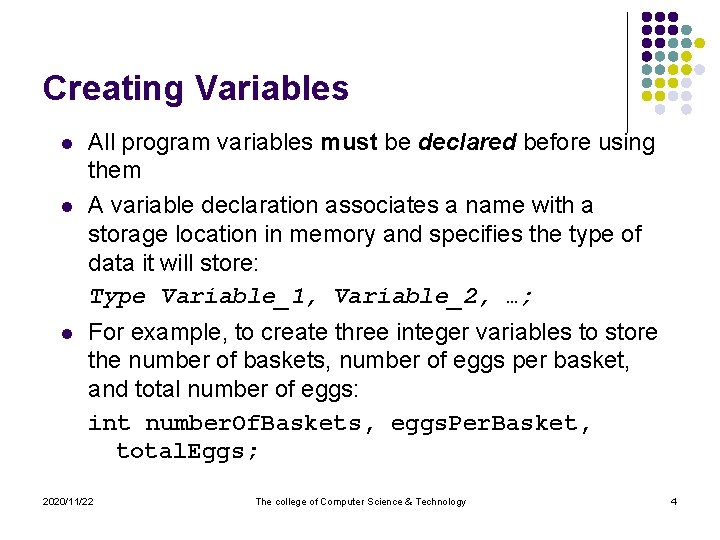
Creating Variables l l l All program variables must be declared before using them A variable declaration associates a name with a storage location in memory and specifies the type of data it will store: Type Variable_1, Variable_2, …; For example, to create three integer variables to store the number of baskets, number of eggs per basket, and total number of eggs: int number. Of. Baskets, eggs. Per. Basket, total. Eggs; 2020/11/22 The college of Computer Science & Technology 4
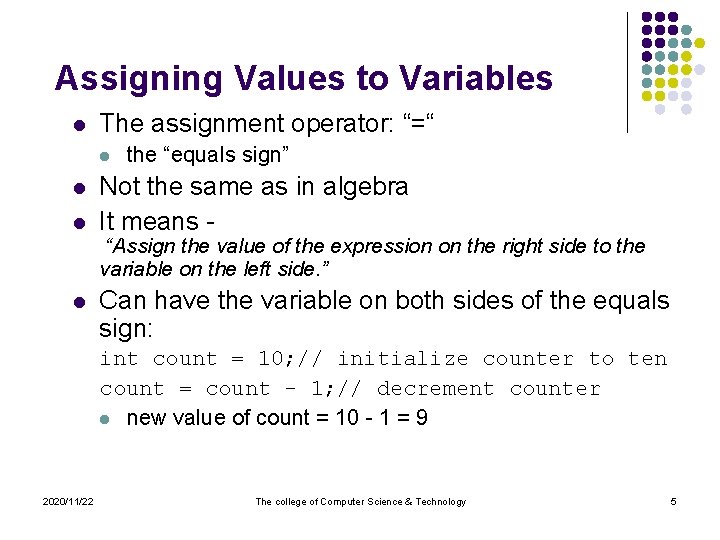
Assigning Values to Variables l The assignment operator: “=“ l l l the “equals sign” Not the same as in algebra It means - “Assign the value of the expression on the right side to the variable on the left side. ” l Can have the variable on both sides of the equals sign: int count = 10; // initialize counter to ten count = count - 1; // decrement counter l new value of count = 10 - 1 = 9 2020/11/22 The college of Computer Science & Technology 5
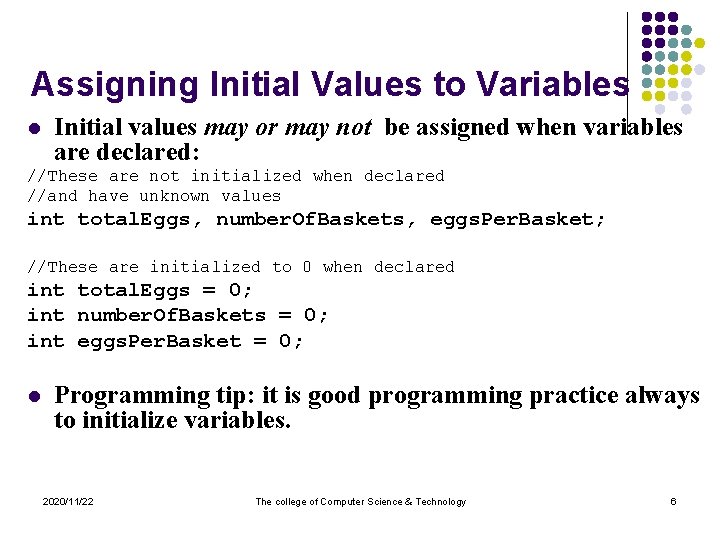
Assigning Initial Values to Variables l Initial values may or may not be assigned when variables are declared: //These are not initialized when declared //and have unknown values int total. Eggs, number. Of. Baskets, eggs. Per. Basket; //These are initialized to 0 when declared int total. Eggs = 0; int number. Of. Baskets = 0; int eggs. Per. Basket = 0; l Programming tip: it is good programming practice always to initialize variables. 2020/11/22 The college of Computer Science & Technology 6
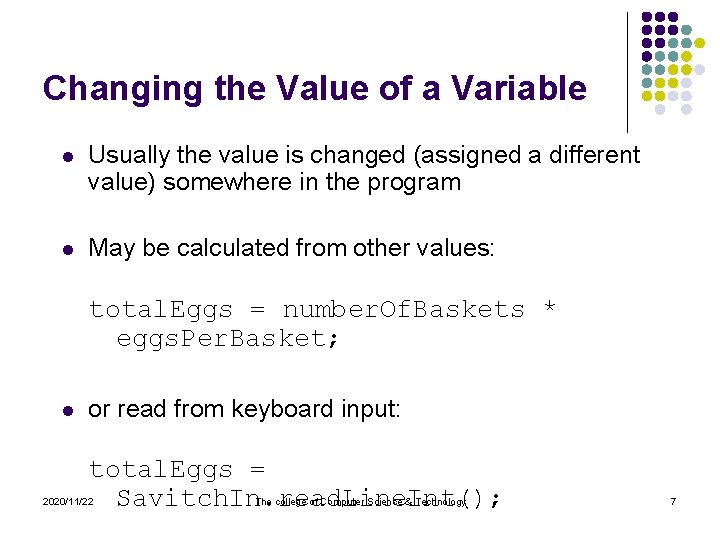
Changing the Value of a Variable l Usually the value is changed (assigned a different value) somewhere in the program l May be calculated from other values: total. Eggs = number. Of. Baskets * eggs. Per. Basket; l or read from keyboard input: total. Eggs = Savitch. In. read. Line. Int(); 2020/11/22 The college of Computer Science & Technology 7
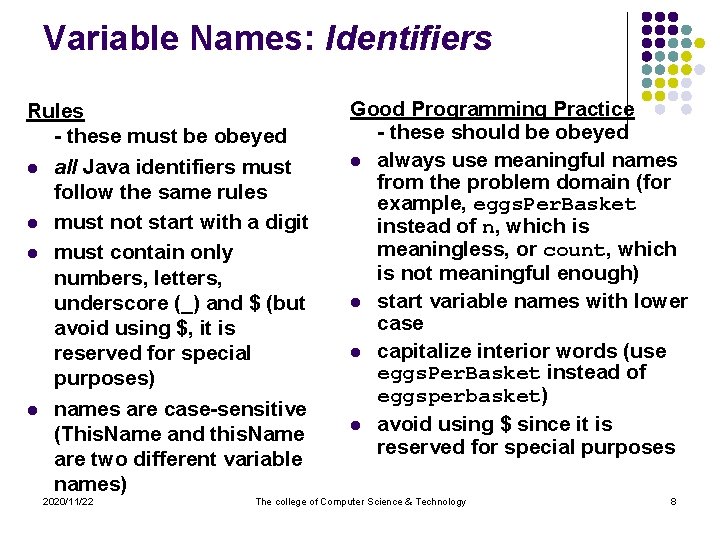
Variable Names: Identifiers Rules - these must be obeyed l all Java identifiers must follow the same rules l must not start with a digit l must contain only numbers, letters, underscore (_) and $ (but avoid using $, it is reserved for special purposes) l names are case-sensitive (This. Name and this. Name are two different variable names) 2020/11/22 Good Programming Practice - these should be obeyed l always use meaningful names from the problem domain (for example, eggs. Per. Basket instead of n, which is meaningless, or count, which is not meaningful enough) l start variable names with lower case l capitalize interior words (use eggs. Per. Basket instead of eggsperbasket) l avoid using $ since it is reserved for special purposes The college of Computer Science & Technology 8
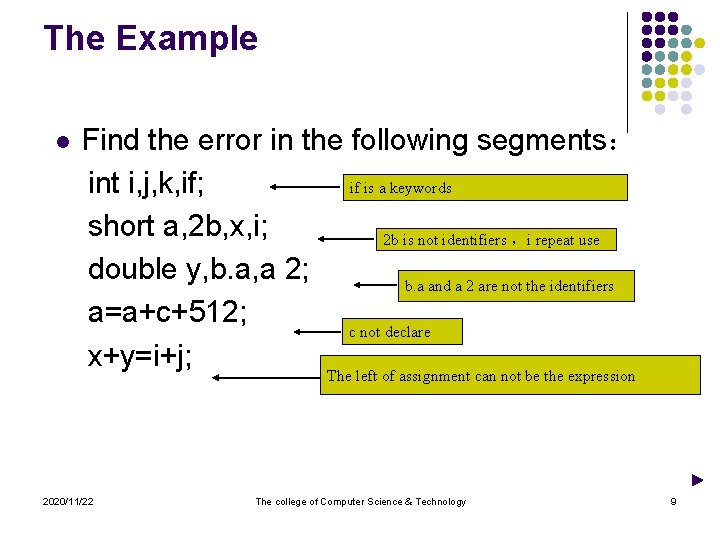
The Example l Find the error in the following segments: int i, j, k, if; if is a keywords short a, 2 b, x, i; 2 b is not identifiers ,i repeat use double y, b. a, a 2; b. a and a 2 are not the identifiers a=a+c+512; c not declare x+y=i+j; The left of assignment can not be the expression 2020/11/22 The college of Computer Science & Technology 9
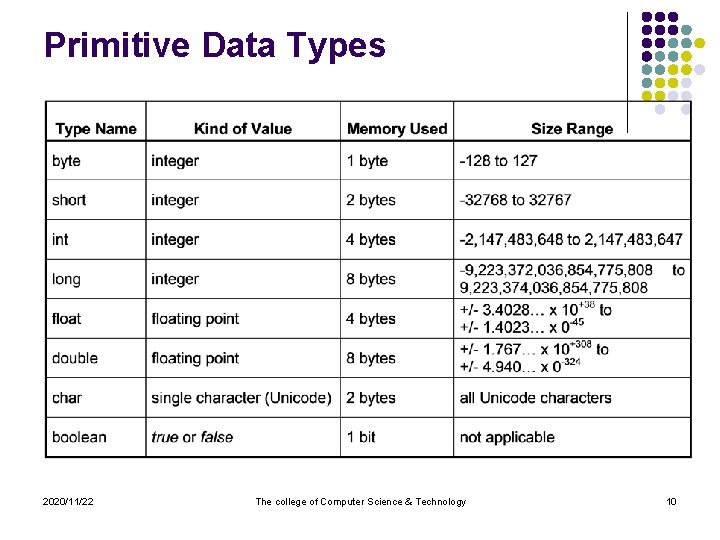
Primitive Data Types 2020/11/22 The college of Computer Science & Technology 10
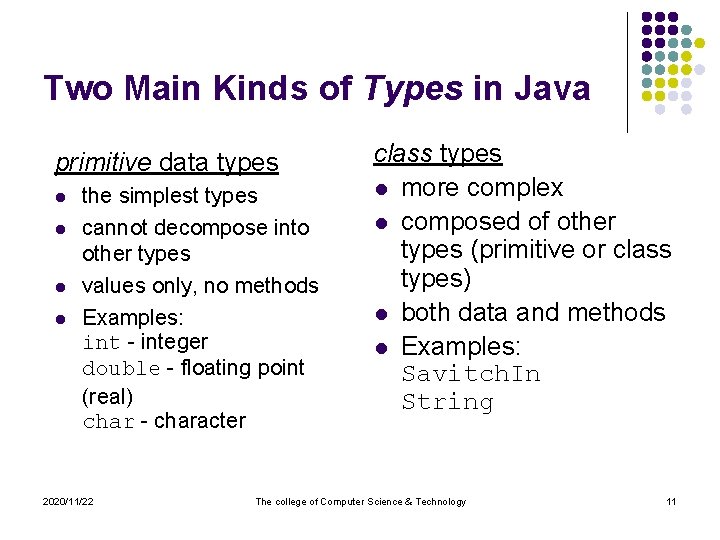
Two Main Kinds of Types in Java primitive data types l l the simplest types cannot decompose into other types values only, no methods Examples: int - integer double - floating point (real) char - character 2020/11/22 class types l more complex l composed of other types (primitive or class types) l both data and methods l Examples: Savitch. In String The college of Computer Science & Technology 11
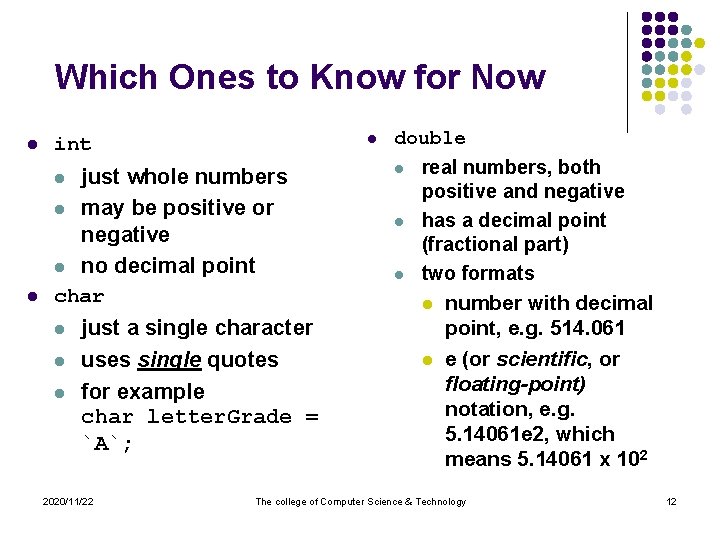
Which Ones to Know for Now l l int l just whole numbers l may be positive or negative l no decimal point char l l just a single character uses single quotes for example char letter. Grade = `A`; 2020/11/22 double l l l real numbers, both positive and negative has a decimal point (fractional part) two formats l l number with decimal point, e. g. 514. 061 e (or scientific, or floating-point) notation, e. g. 5. 14061 e 2, which means 5. 14061 x 102 The college of Computer Science & Technology 12
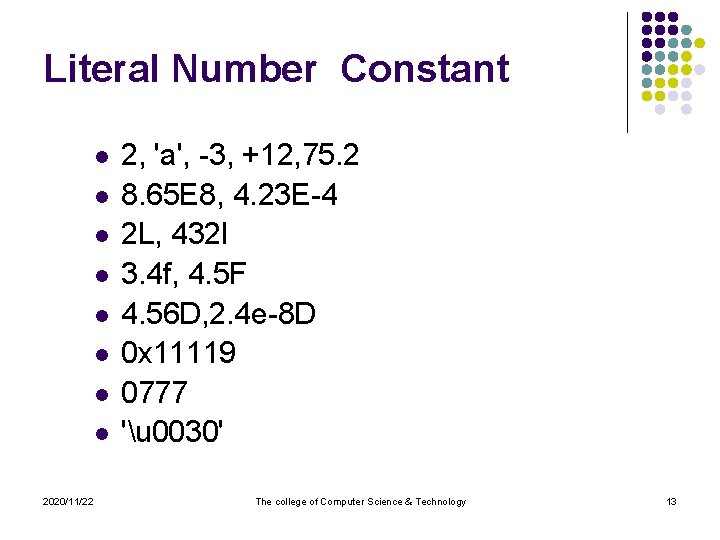
Literal Number Constant l l l l 2020/11/22 2, 'a', -3, +12, 75. 2 8. 65 E 8, 4. 23 E-4 2 L, 432 l 3. 4 f, 4. 5 F 4. 56 D, 2. 4 e-8 D 0 x 11119 0777 'u 0030' The college of Computer Science & Technology 13
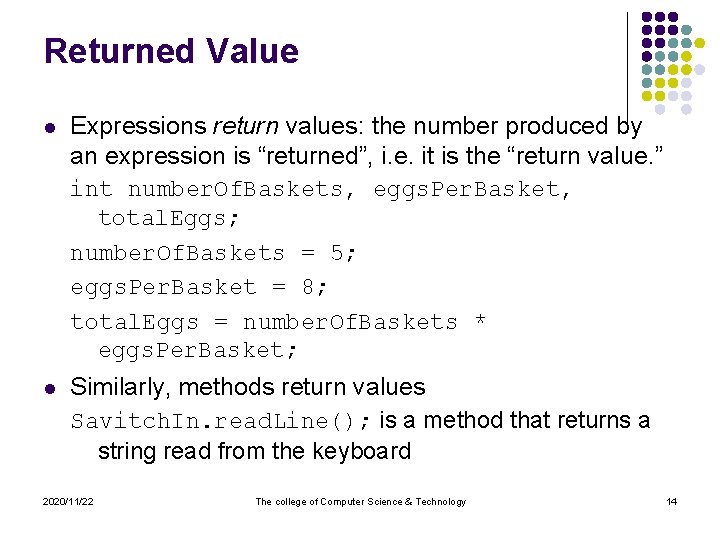
Returned Value l Expressions return values: the number produced by an expression is “returned”, i. e. it is the “return value. ” int number. Of. Baskets, eggs. Per. Basket, total. Eggs; number. Of. Baskets = 5; eggs. Per. Basket = 8; total. Eggs = number. Of. Baskets * eggs. Per. Basket; l Similarly, methods return values Savitch. In. read. Line(); is a method that returns a string read from the keyboard 2020/11/22 The college of Computer Science & Technology 14
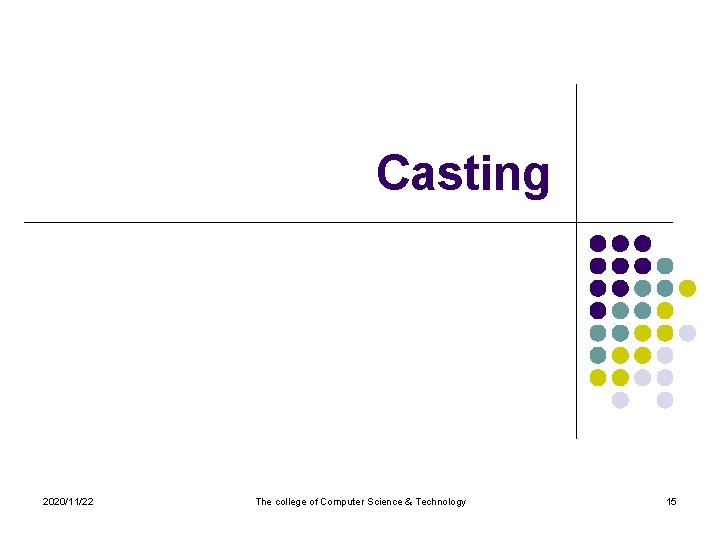
Casting 2020/11/22 The college of Computer Science & Technology 15
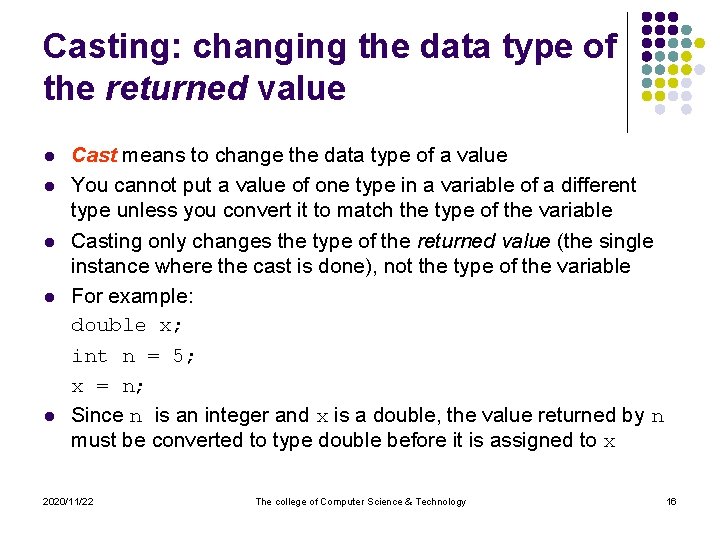
Casting: changing the data type of the returned value l l l Cast means to change the data type of a value You cannot put a value of one type in a variable of a different type unless you convert it to match the type of the variable Casting only changes the type of the returned value (the single instance where the cast is done), not the type of the variable For example: double x; int n = 5; x = n; Since n is an integer and x is a double, the value returned by n must be converted to type double before it is assigned to x 2020/11/22 The college of Computer Science & Technology 16
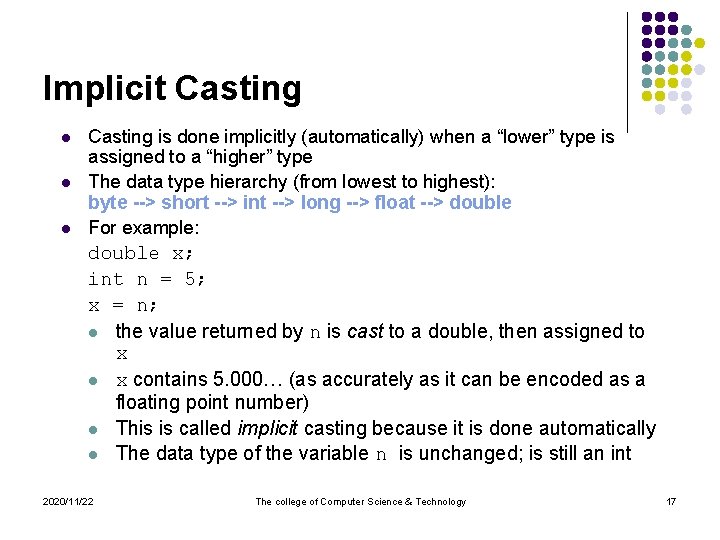
Implicit Casting l l l Casting is done implicitly (automatically) when a “lower” type is assigned to a “higher” type The data type hierarchy (from lowest to highest): byte --> short --> int --> long --> float --> double For example: double x; int n = 5; x = n; l the value returned by n is cast to a double, then assigned to x l x contains 5. 000… (as accurately as it can be encoded as a floating point number) l This is called implicit casting because it is done automatically l The data type of the variable n is unchanged; is still an int 2020/11/22 The college of Computer Science & Technology 17
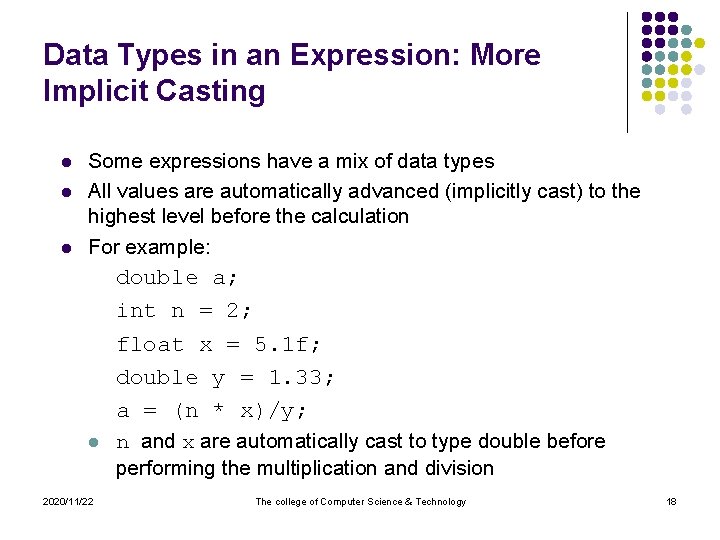
Data Types in an Expression: More Implicit Casting l l l Some expressions have a mix of data types All values are automatically advanced (implicitly cast) to the highest level before the calculation For example: double a; int n = 2; float x = 5. 1 f; double y = 1. 33; a = (n * x)/y; l 2020/11/22 n and x are automatically cast to type double before performing the multiplication and division The college of Computer Science & Technology 18
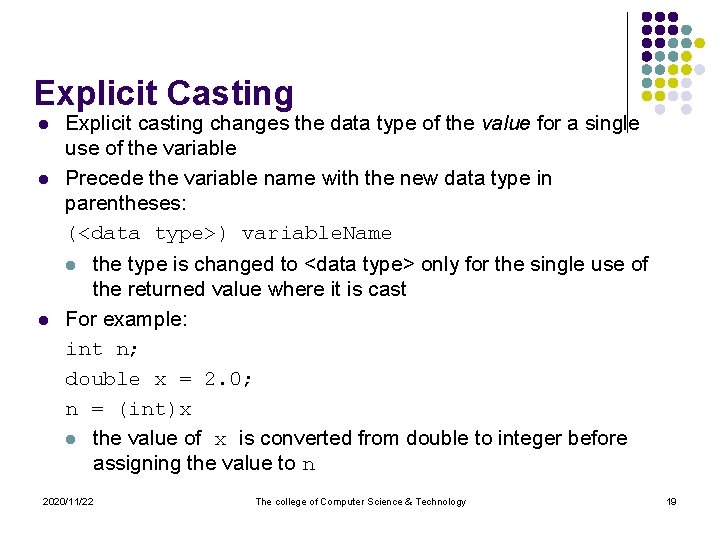
Explicit Casting l l Explicit casting changes the data type of the value for a single use of the variable Precede the variable name with the new data type in parentheses: (<data type>) variable. Name the type is changed to <data type> only for the single use of the returned value where it is cast For example: int n; double x = 2. 0; n = (int)x l the value of x is converted from double to integer before assigning the value to n l l 2020/11/22 The college of Computer Science & Technology 19
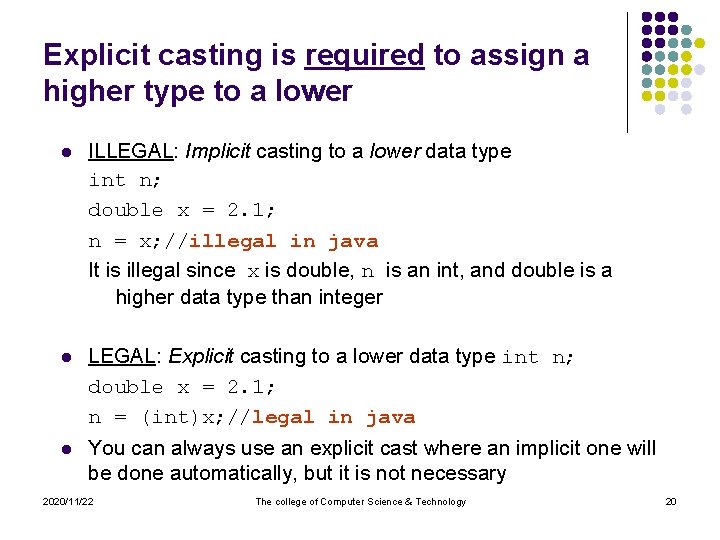
Explicit casting is required to assign a higher type to a lower l ILLEGAL: Implicit casting to a lower data type int n; double x = 2. 1; n = x; //illegal in java It is illegal since x is double, n is an int, and double is a higher data type than integer l LEGAL: Explicit casting to a lower data type int n; double x = 2. 1; n = (int)x; //legal in java l You can always use an explicit cast where an implicit one will be done automatically, but it is not necessary 2020/11/22 The college of Computer Science & Technology 20
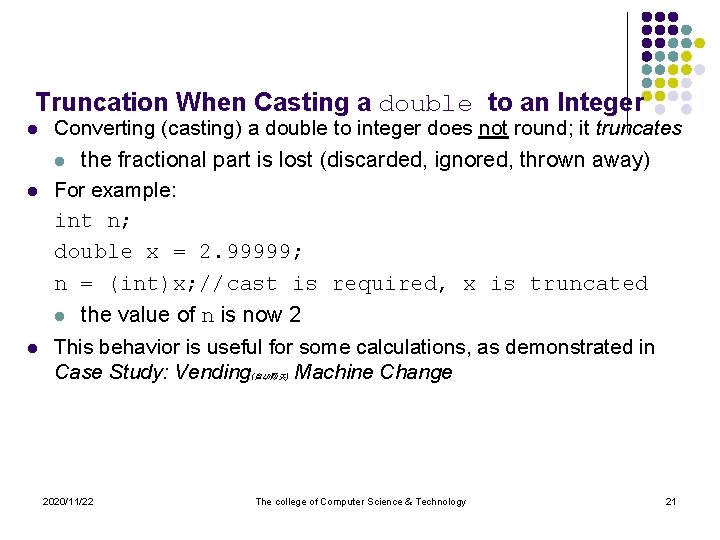
Truncation When Casting a double to an Integer l l l Converting (casting) a double to integer does not round; it truncates l the fractional part is lost (discarded, ignored, thrown away) For example: int n; double x = 2. 99999; n = (int)x; //cast is required, x is truncated l the value of n is now 2 This behavior is useful for some calculations, as demonstrated in Case Study: Vending Machine Change (自动贩卖) 2020/11/22 The college of Computer Science & Technology 21
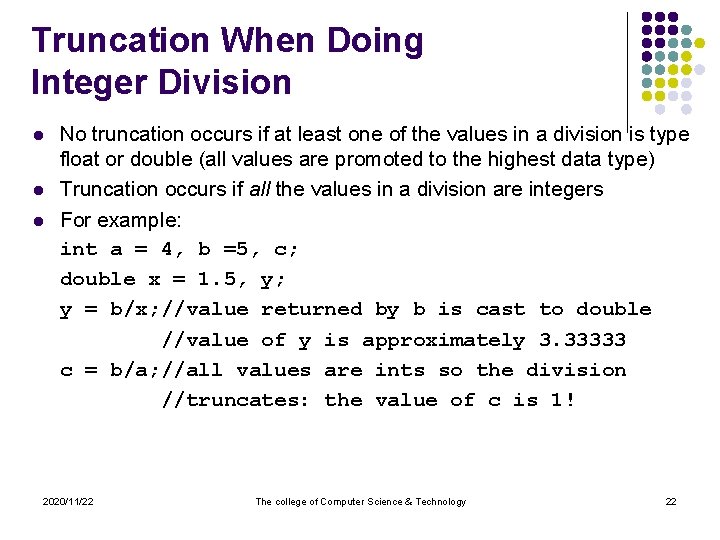
Truncation When Doing Integer Division l l l No truncation occurs if at least one of the values in a division is type float or double (all values are promoted to the highest data type) Truncation occurs if all the values in a division are integers For example: int a = 4, b =5, c; double x = 1. 5, y; y = b/x; //value returned by b is cast to double //value of y is approximately 3. 33333 c = b/a; //all values are ints so the division //truncates: the value of c is 1! 2020/11/22 The college of Computer Science & Technology 22
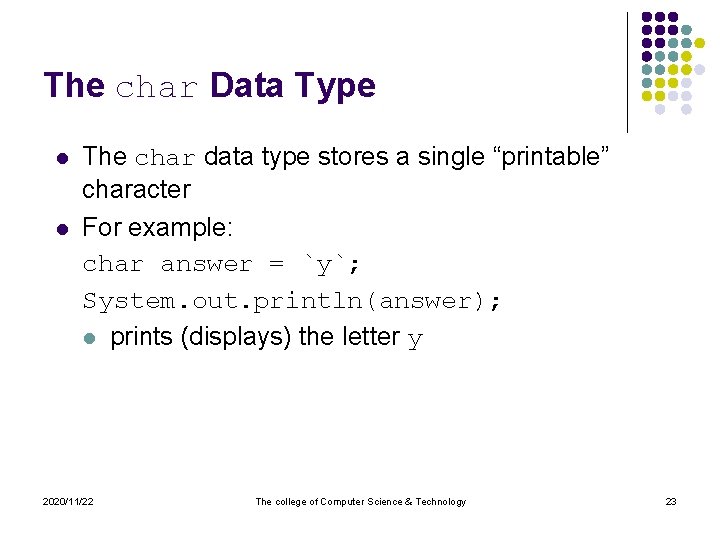
The char Data Type l l The char data type stores a single “printable” character For example: char answer = `y`; System. out. println(answer); l prints (displays) the letter y 2020/11/22 The college of Computer Science & Technology 23
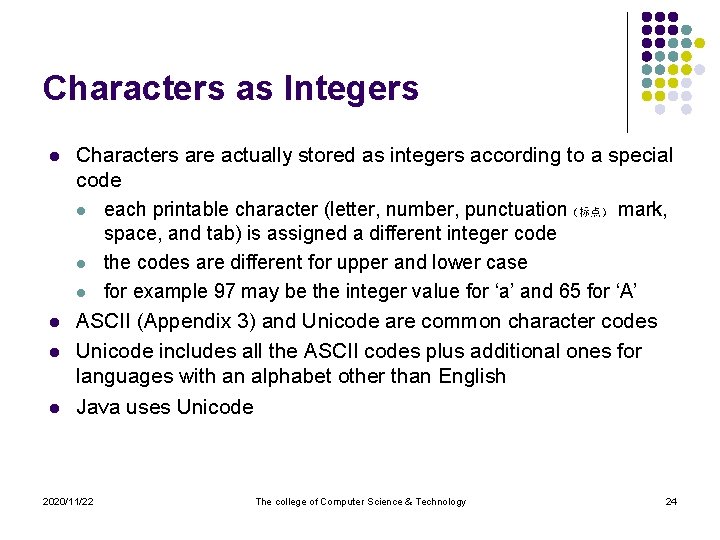
Characters as Integers l l Characters are actually stored as integers according to a special code l each printable character (letter, number, punctuation(标点) mark, space, and tab) is assigned a different integer code l the codes are different for upper and lower case l for example 97 may be the integer value for ‘a’ and 65 for ‘A’ ASCII (Appendix 3) and Unicode are common character codes Unicode includes all the ASCII codes plus additional ones for languages with an alphabet other than English Java uses Unicode 2020/11/22 The college of Computer Science & Technology 24
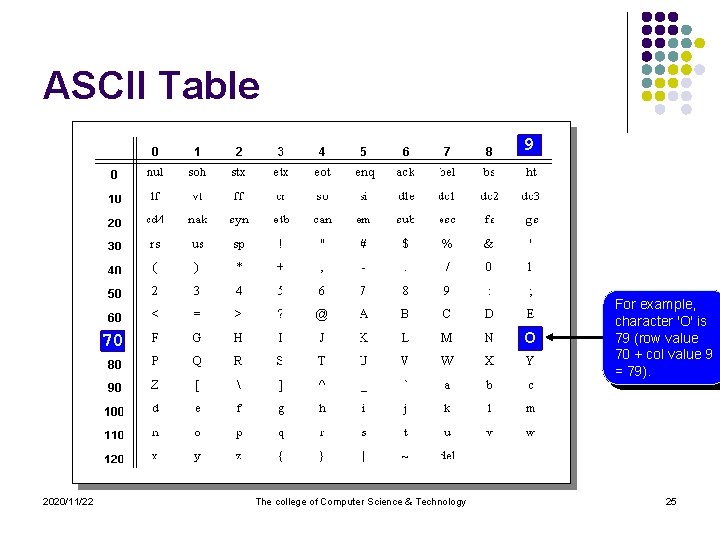
ASCII Table 9 O 70 2020/11/22 The college of Computer Science & Technology For example, character 'O' is 79 (row value 70 + col value 9 = 79). 25
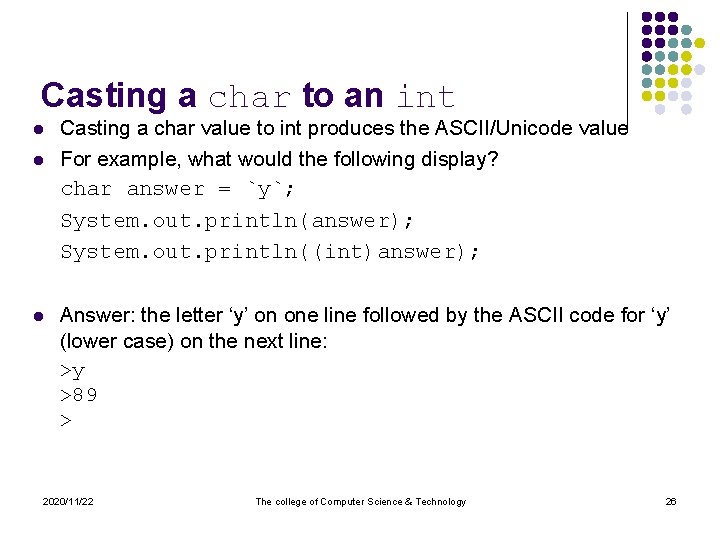
Casting a char to an int l l Casting a char value to int produces the ASCII/Unicode value For example, what would the following display? char answer = `y`; System. out. println(answer); System. out. println((int)answer); l Answer: the letter ‘y’ on one line followed by the ASCII code for ‘y’ (lower case) on the next line: >y >89 > 2020/11/22 The college of Computer Science & Technology 26
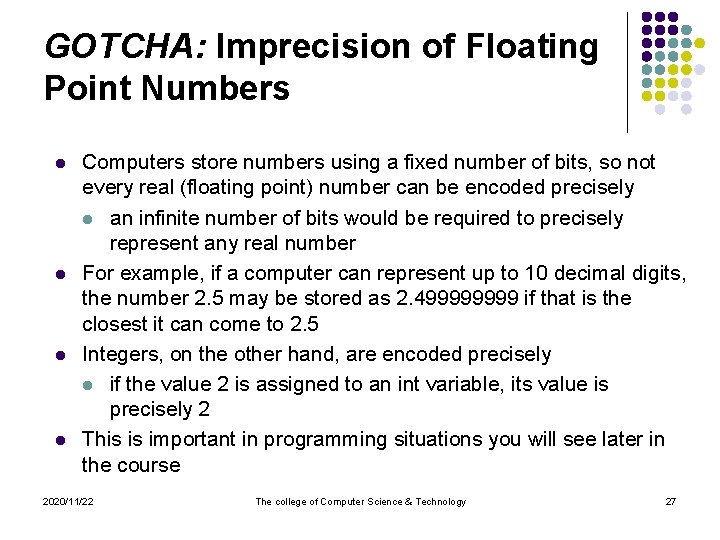
GOTCHA: Imprecision of Floating Point Numbers l l Computers store numbers using a fixed number of bits, so not every real (floating point) number can be encoded precisely l an infinite number of bits would be required to precisely represent any real number For example, if a computer can represent up to 10 decimal digits, the number 2. 5 may be stored as 2. 49999 if that is the closest it can come to 2. 5 Integers, on the other hand, are encoded precisely l if the value 2 is assigned to an int variable, its value is precisely 2 This is important in programming situations you will see later in the course 2020/11/22 The college of Computer Science & Technology 27
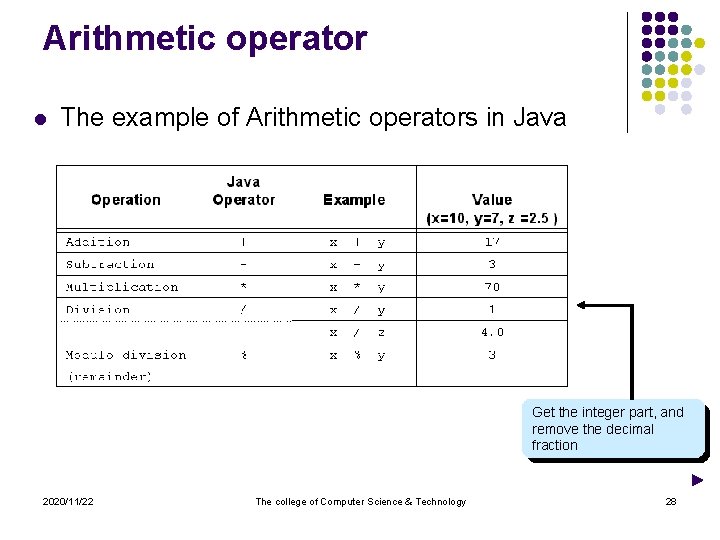
Arithmetic operator l The example of Arithmetic operators in Java Get the integer part, and remove the decimal fraction 2020/11/22 The college of Computer Science & Technology 28
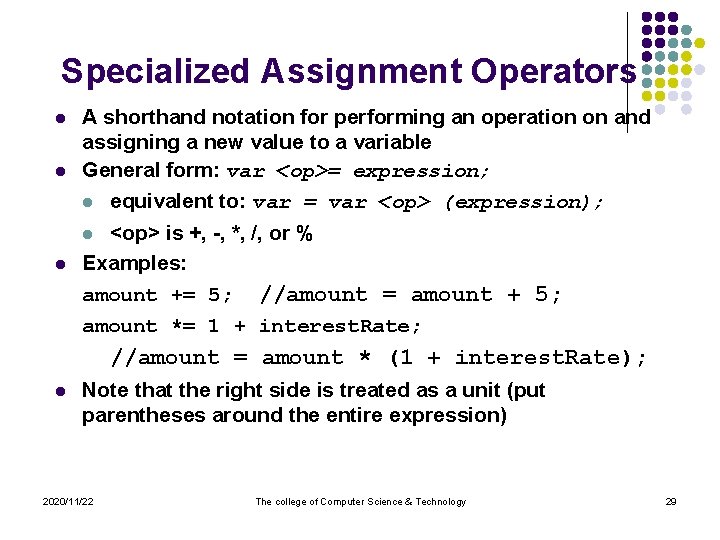
Specialized Assignment Operators l l A shorthand notation for performing an operation on and assigning a new value to a variable General form: var <op>= expression; l equivalent to: var = var <op> (expression); <op> is +, -, *, /, or % Examples: amount += 5; //amount = amount + 5; amount *= 1 + interest. Rate; l l //amount = amount * (1 + interest. Rate); l Note that the right side is treated as a unit (put parentheses around the entire expression) 2020/11/22 The college of Computer Science & Technology 29
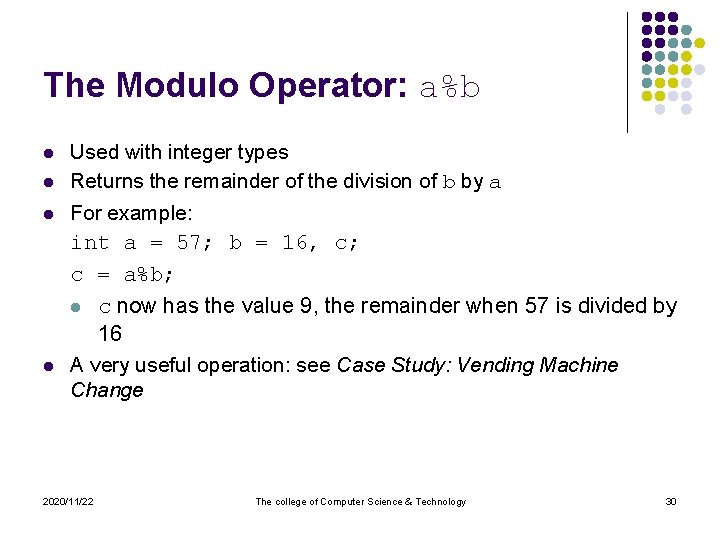
The Modulo Operator: a%b l l Used with integer types Returns the remainder of the division of b by a For example: int a = 57; b = 16, c; c = a%b; l c now has the value 9, the remainder when 57 is divided by 16 A very useful operation: see Case Study: Vending Machine Change 2020/11/22 The college of Computer Science & Technology 30
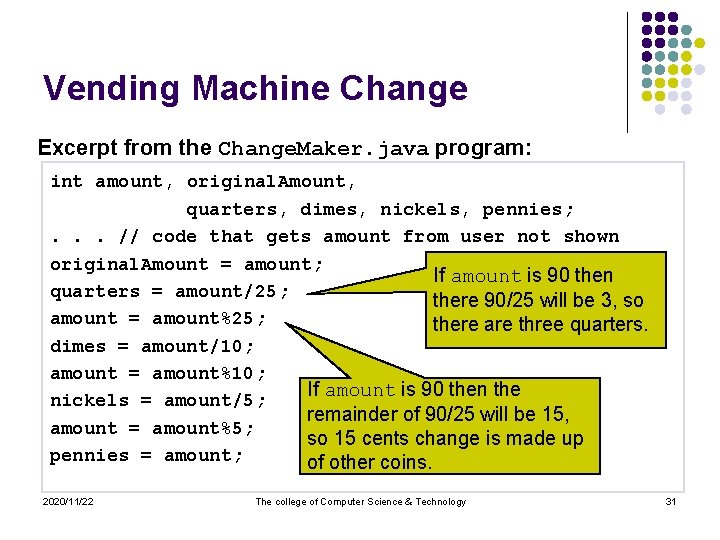
Vending Machine Change Excerpt from the Change. Maker. java program: int amount, original. Amount, quarters, dimes, nickels, pennies; . . . // code that gets amount from user not shown original. Amount = amount; If amount is 90 then quarters = amount/25; there 90/25 will be 3, so amount = amount%25; there are three quarters. dimes = amount/10; amount = amount%10; If amount is 90 then the nickels = amount/5; remainder of 90/25 will be 15, amount = amount%5; so 15 cents change is made up pennies = amount; of other coins. 2020/11/22 The college of Computer Science & Technology 31
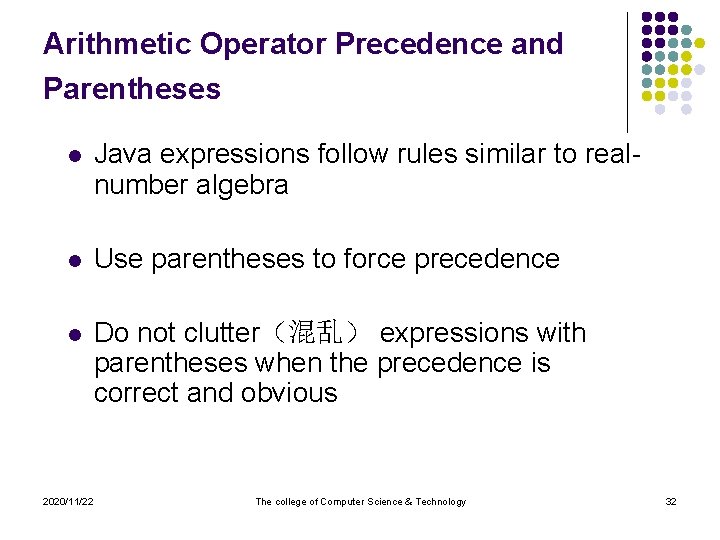
Arithmetic Operator Precedence and Parentheses l Java expressions follow rules similar to realnumber algebra l Use parentheses to force precedence l Do not clutter(混乱) expressions with parentheses when the precedence is correct and obvious 2020/11/22 The college of Computer Science & Technology 32
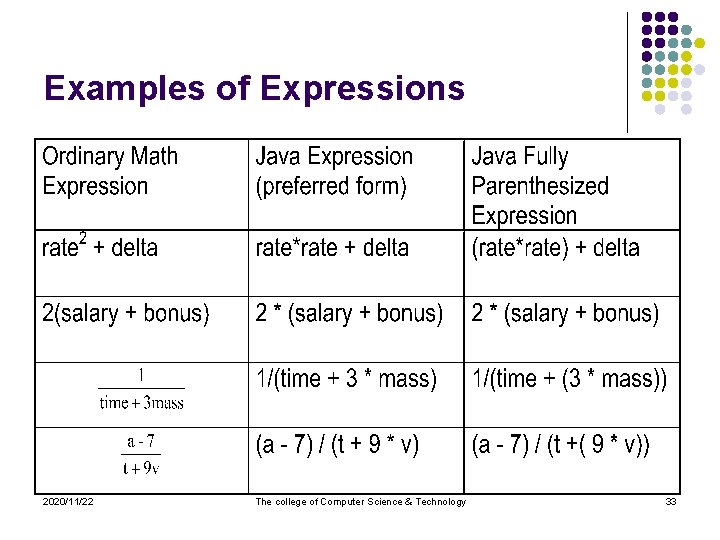
Examples of Expressions 2020/11/22 The college of Computer Science & Technology 33
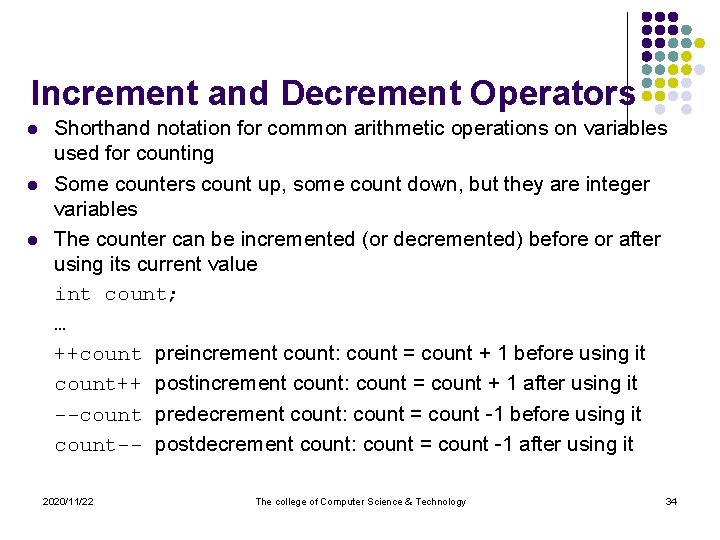
Increment and Decrement Operators l l l Shorthand notation for common arithmetic operations on variables used for counting Some counters count up, some count down, but they are integer variables The counter can be incremented (or decremented) before or after using its current value int count; … ++count preincrement count: count = count + 1 before using it count++ postincrement count: count = count + 1 after using it --count predecrement count: count = count -1 before using it count-- postdecrement count: count = count -1 after using it 2020/11/22 The college of Computer Science & Technology 34
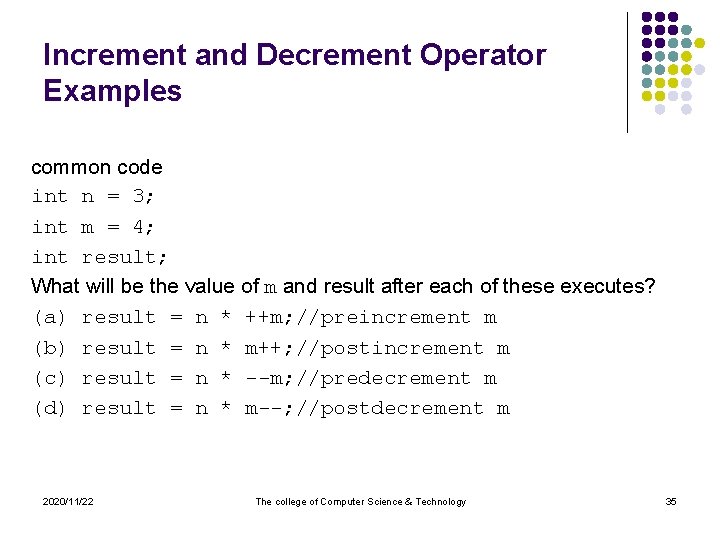
Increment and Decrement Operator Examples common code int n = 3; int m = 4; int result; What will be the value of m and result after each of these executes? (a) result = n * ++m; //preincrement m (b) result = n * m++; //postincrement m (c) result = n * --m; //predecrement m (d) result = n * m--; //postdecrement m 2020/11/22 The college of Computer Science & Technology 35
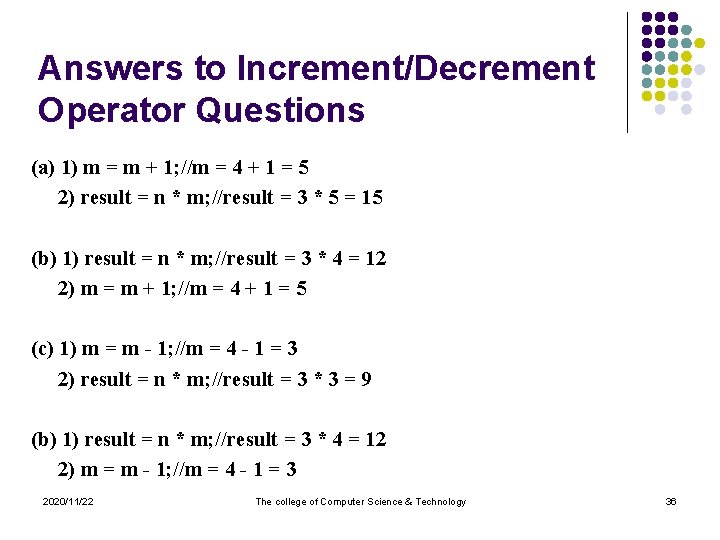
Answers to Increment/Decrement Operator Questions (a) 1) m = m + 1; //m = 4 + 1 = 5 2) result = n * m; //result = 3 * 5 = 15 (b) 1) result = n * m; //result = 3 * 4 = 12 2) m = m + 1; //m = 4 + 1 = 5 (c) 1) m = m - 1; //m = 4 - 1 = 3 2) result = n * m; //result = 3 * 3 = 9 (b) 1) result = n * m; //result = 3 * 4 = 12 2) m = m - 1; //m = 4 - 1 = 3 2020/11/22 The college of Computer Science & Technology 36
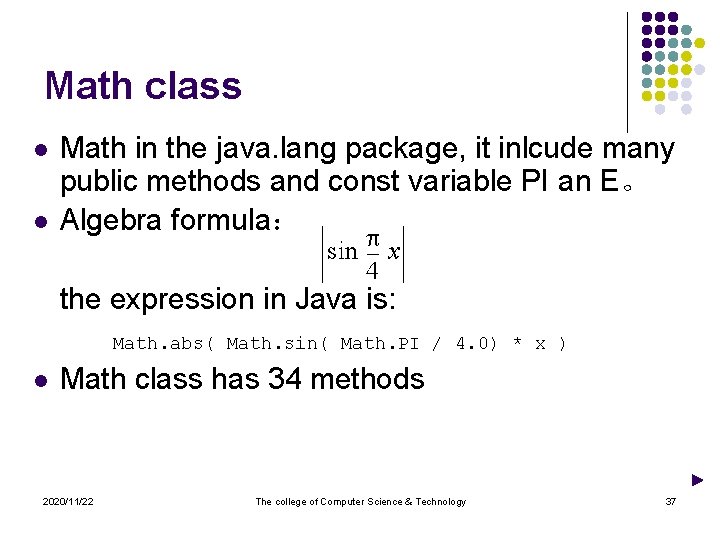
Math class l l Math in the java. lang package, it inlcude many public methods and const variable PI an E。 Algebra formula: the expression in Java is: Math. abs( Math. sin( Math. PI / 4. 0) * x ) l Math class has 34 methods 2020/11/22 The college of Computer Science & Technology 37
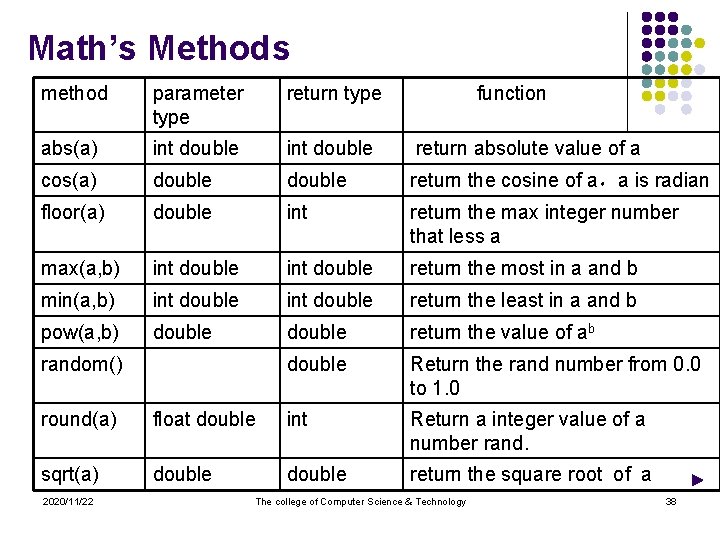
Math’s Methods method parameter type return type abs(a) int double return absolute value of a cos(a) double return the cosine of a,a is radian floor(a) double int return the max integer number that less a max(a, b) int double return the most in a and b min(a, b) int double return the least in a and b pow(a, b) double return the value of ab double Return the rand number from 0. 0 to 1. 0 random() function round(a) float double int Return a integer value of a number rand. sqrt(a) double return the square root of a 2020/11/22 The college of Computer Science & Technology 38
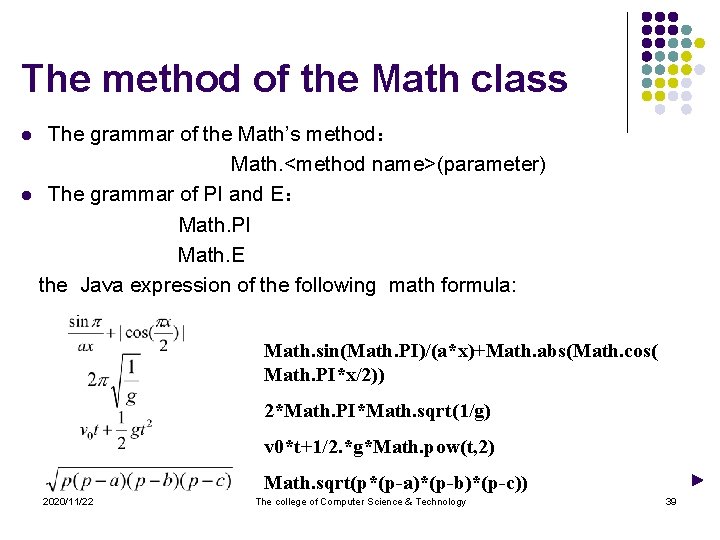
The method of the Math class The grammar of the Math’s method: Math. <method name>(parameter) l The grammar of PI and E: Math. PI Math. E the Java expression of the following math formula: l Math. sin(Math. PI)/(a*x)+Math. abs(Math. cos( Math. PI*x/2)) 2*Math. PI*Math. sqrt(1/g) v 0*t+1/2. *g*Math. pow(t, 2) Math. sqrt(p*(p-a)*(p-b)*(p-c)) 2020/11/22 The college of Computer Science & Technology 39
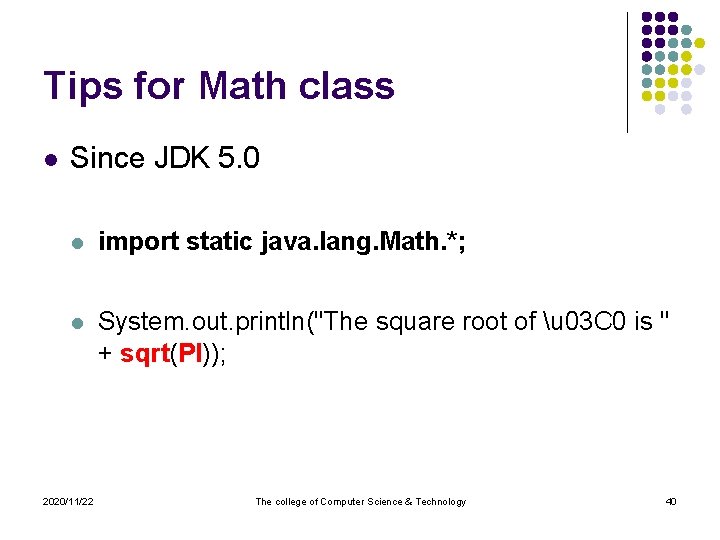
Tips for Math class l Since JDK 5. 0 l import static java. lang. Math. *; l System. out. println("The square root of u 03 C 0 is " + sqrt(PI)); 2020/11/22 The college of Computer Science & Technology 40
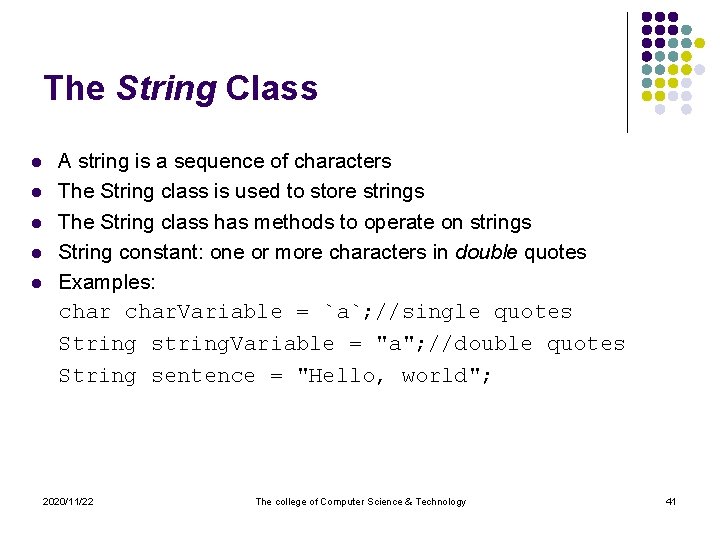
The String Class l l l A string is a sequence of characters The String class is used to store strings The String class has methods to operate on strings String constant: one or more characters in double quotes Examples: char. Variable = `a`; //single quotes String string. Variable = "a"; //double quotes String sentence = "Hello, world"; 2020/11/22 The college of Computer Science & Technology 41
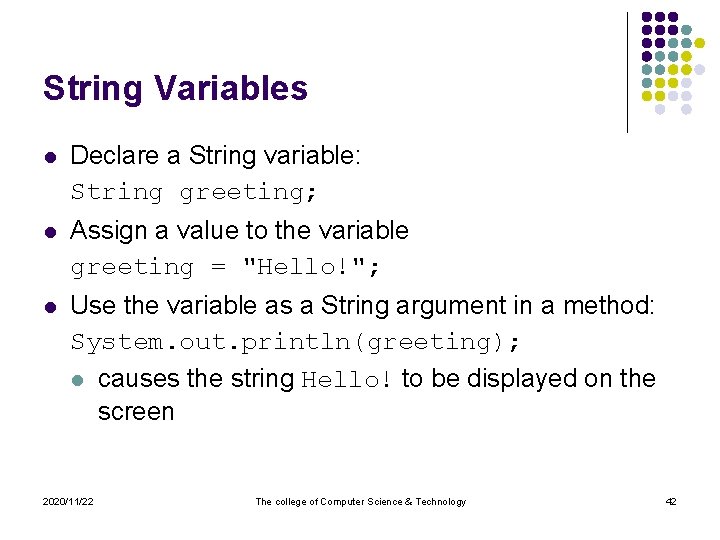
String Variables l Declare a String variable: String greeting; l Assign a value to the variable greeting = "Hello!"; l Use the variable as a String argument in a method: System. out. println(greeting); l causes the string Hello! to be displayed on the screen 2020/11/22 The college of Computer Science & Technology 42
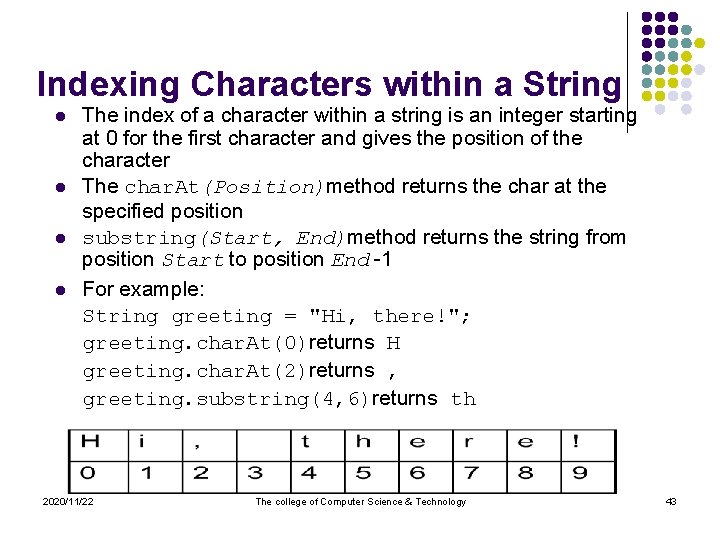
Indexing Characters within a String l l The index of a character within a string is an integer starting at 0 for the first character and gives the position of the character The char. At(Position)method returns the char at the specified position substring(Start, End)method returns the string from position Start to position End -1 For example: String greeting = "Hi, there!"; greeting. char. At(0)returns H greeting. char. At(2)returns , greeting. substring(4, 6)returns th 2020/11/22 The college of Computer Science & Technology 43
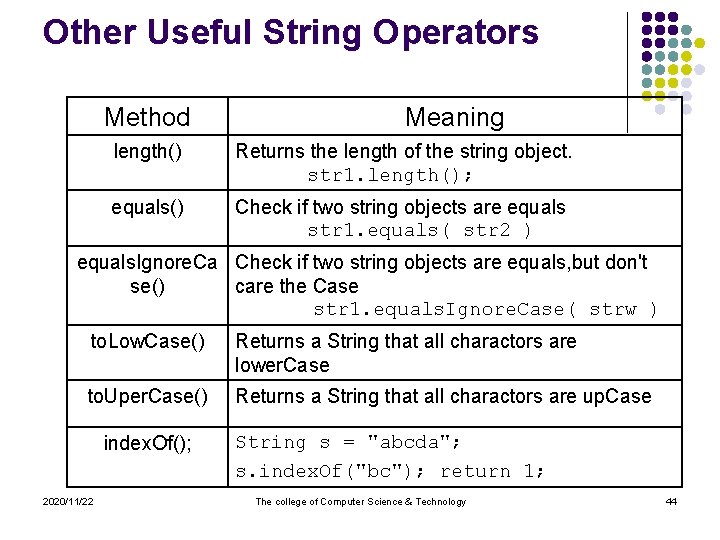
Other Useful String Operators Method Meaning length() Returns the length of the string object. str 1. length(); equals() Check if two string objects are equals str 1. equals( str 2 ) equals. Ignore. Ca Check if two string objects are equals, but don't se() care the Case str 1. equals. Ignore. Case( strw ) to. Low. Case() Returns a String that all charactors are lower. Case to. Uper. Case() Returns a String that all charactors are up. Case index. Of(); 2020/11/22 String s = "abcda"; s. index. Of("bc"); return 1; The college of Computer Science & Technology 44
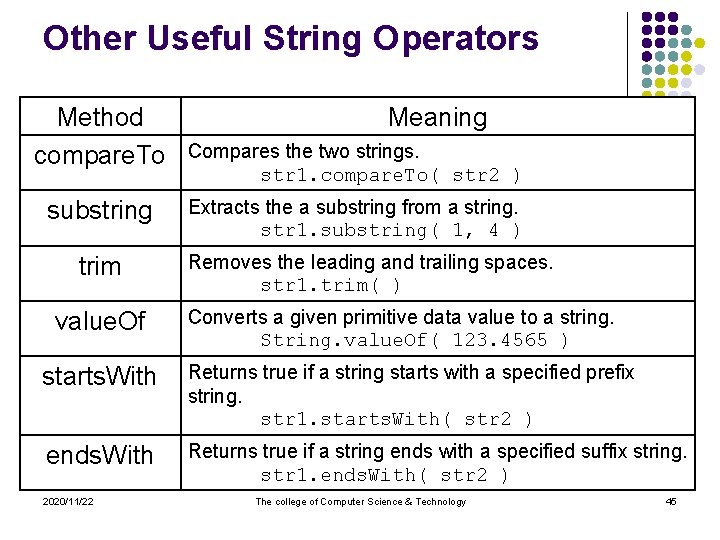
Other Useful String Operators Method compare. To substring trim value. Of Meaning Compares the two strings. str 1. compare. To( str 2 ) Extracts the a substring from a string. str 1. substring( 1, 4 ) Removes the leading and trailing spaces. str 1. trim( ) Converts a given primitive data value to a string. String. value. Of( 123. 4565 ) starts. With Returns true if a string starts with a specified prefix string. str 1. starts. With( str 2 ) ends. With Returns true if a string ends with a specified suffix string. str 1. ends. With( str 2 ) 2020/11/22 The college of Computer Science & Technology 45
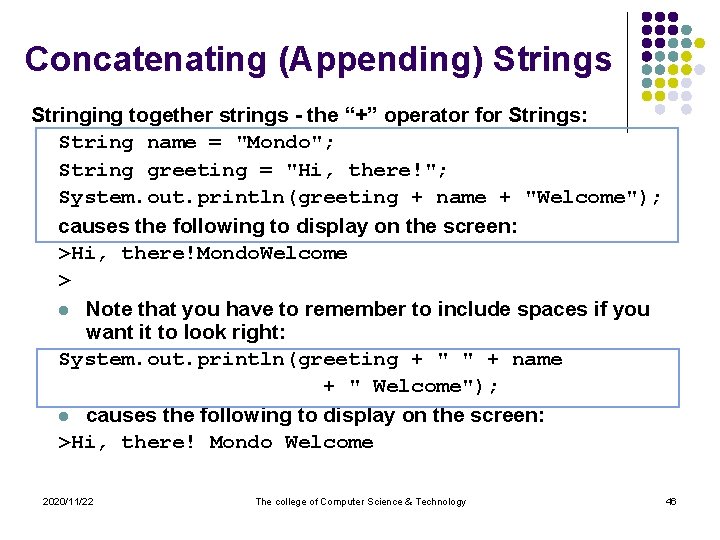
Concatenating (Appending) Strings Stringing together strings - the “+” operator for Strings: String name = "Mondo"; String greeting = "Hi, there!"; System. out. println(greeting + name + "Welcome"); causes the following to display on the screen: >Hi, there!Mondo. Welcome > l Note that you have to remember to include spaces if you want it to look right: System. out. println(greeting + " " + name + " Welcome"); l causes the following to display on the screen: >Hi, there! Mondo Welcome 2020/11/22 The college of Computer Science & Technology 46
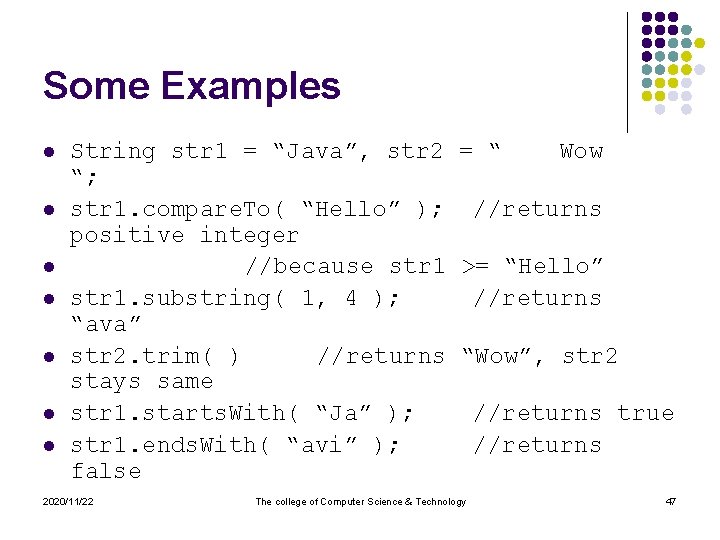
Some Examples l l l l String str 1 = “Java”, str 2 = “ Wow “; str 1. compare. To( “Hello” ); //returns positive integer //because str 1 >= “Hello” str 1. substring( 1, 4 ); //returns “ava” str 2. trim( ) //returns “Wow”, str 2 stays same str 1. starts. With( “Ja” ); //returns true str 1. ends. With( “avi” ); //returns false 2020/11/22 The college of Computer Science & Technology 47
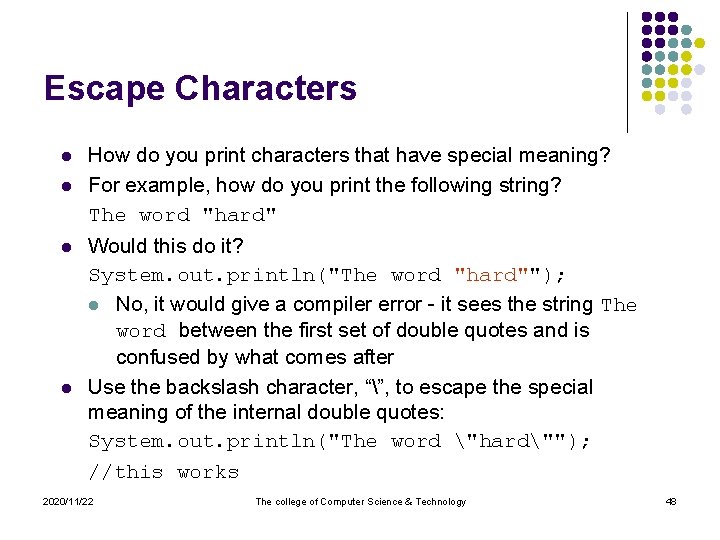
Escape Characters l l How do you print characters that have special meaning? For example, how do you print the following string? The word "hard" Would this do it? System. out. println("The word "hard""); l No, it would give a compiler error - it sees the string The word between the first set of double quotes and is confused by what comes after Use the backslash character, “”, to escape the special meaning of the internal double quotes: System. out. println("The word "hard""); //this works 2020/11/22 The college of Computer Science & Technology 48
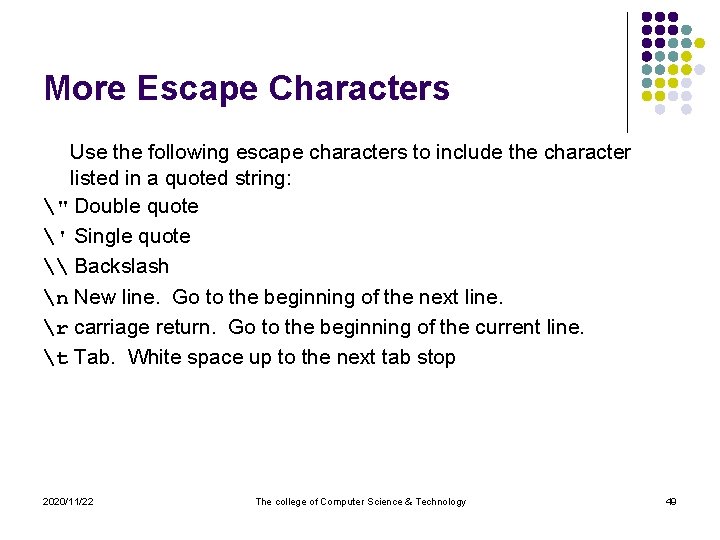
More Escape Characters Use the following escape characters to include the character listed in a quoted string: " Double quote ' Single quote \ Backslash n New line. Go to the beginning of the next line. r carriage return. Go to the beginning of the current line. t Tab. White space up to the next tab stop 2020/11/22 The college of Computer Science & Technology 49
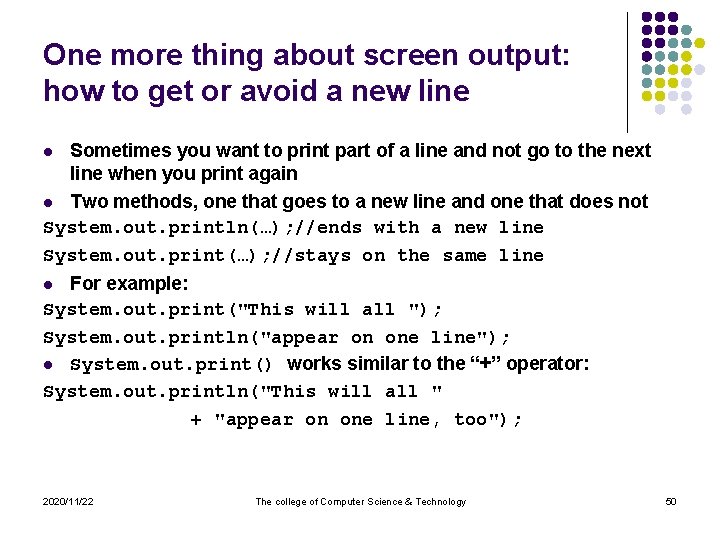
One more thing about screen output: how to get or avoid a new line Sometimes you want to print part of a line and not go to the next line when you print again l Two methods, one that goes to a new line and one that does not System. out. println(…); //ends with a new line System. out. print(…); //stays on the same line l For example: System. out. print("This will all "); System. out. println("appear on one line"); l System. out. print() works similar to the “+” operator: System. out. println("This will all " + "appear on one line, too"); l 2020/11/22 The college of Computer Science & Technology 50
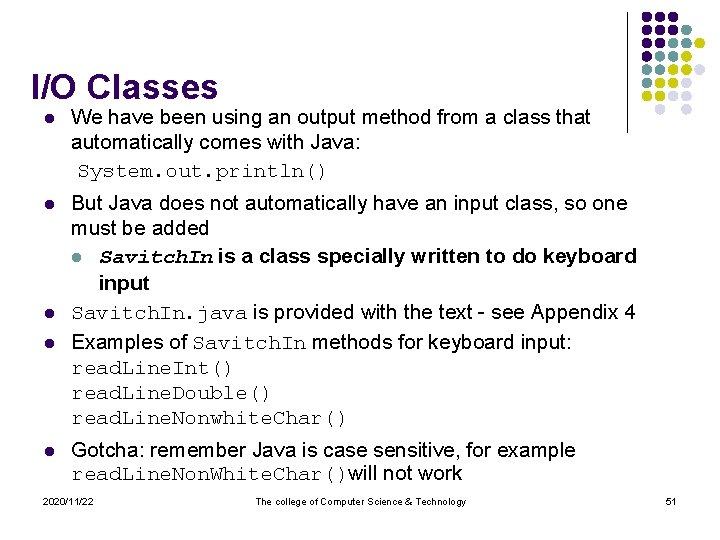
I/O Classes l We have been using an output method from a class that automatically comes with Java: System. out. println() l But Java does not automatically have an input class, so one must be added l Savitch. In is a class specially written to do keyboard input Savitch. In. java is provided with the text - see Appendix 4 Examples of Savitch. In methods for keyboard input: read. Line. Int() read. Line. Double() read. Line. Nonwhite. Char() l l l Gotcha: remember Java is case sensitive, for example read. Line. Non. White. Char()will not work 2020/11/22 The college of Computer Science & Technology 51
![IO Classes import java util public class Input Test public static void mainString I/O Classes import java. util. *; public class Input. Test{ public static void main(String[]](https://slidetodoc.com/presentation_image_h/87835e6389c3fef224397967370b5e16/image-52.jpg)
I/O Classes import java. util. *; public class Input. Test{ public static void main(String[] args) { Scanner in = new Scanner(System. in); System. out. print("What is your name? "); String name = in. next. Line(); // get second input System. out. print("How old are you? "); int age = in. next. Int(); // display output on console System. out. println("Hello, " + name + ". Next year, you'll be " + (age + 1)); } } 2020/11/22 The college of Computer Science & Technology 52
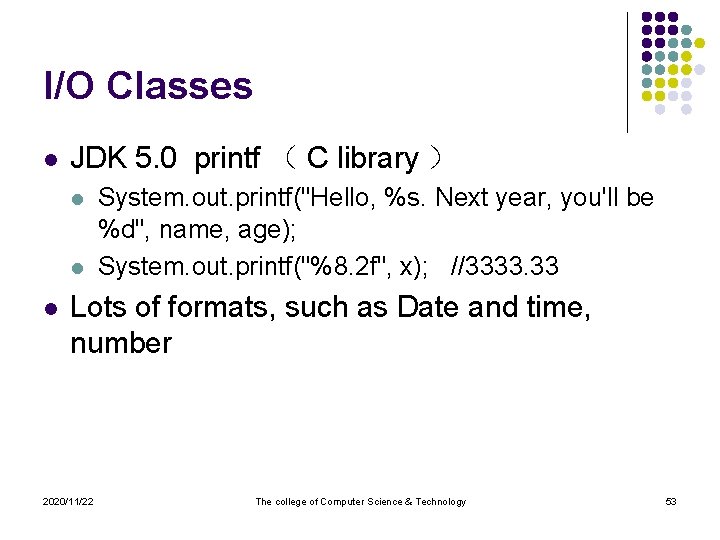
I/O Classes l JDK 5. 0 printf ( C library ) l l l System. out. printf("Hello, %s. Next year, you'll be %d", name, age); System. out. printf("%8. 2 f", x); //3333. 33 Lots of formats, such as Date and time, number 2020/11/22 The college of Computer Science & Technology 53
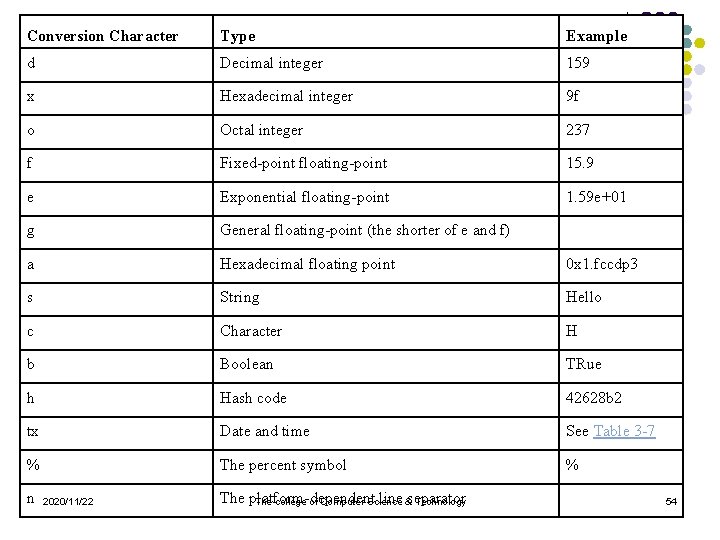
Conversion Character Type Example d Decimal integer 159 x Hexadecimal integer 9 f o Octal integer 237 f Fixed-point floating-point 15. 9 e Exponential floating-point 1. 59 e+01 g General floating-point (the shorter of e and f) a Hexadecimal floating point 0 x 1. fccdp 3 s String Hello c Character H b Boolean TRue h Hash code 42628 b 2 tx Date and time See Table 3 -7 % The percent symbol % The platform-dependent line separator The college of Computer Science & Technology n 2020/11/22 54
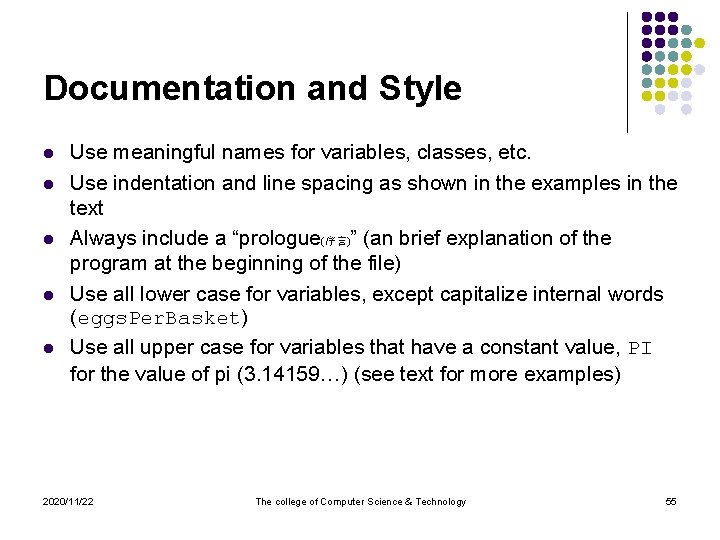
Documentation and Style l l l Use meaningful names for variables, classes, etc. Use indentation and line spacing as shown in the examples in the text Always include a “prologue(序言)” (an brief explanation of the program at the beginning of the file) Use all lower case for variables, except capitalize internal words (eggs. Per. Basket) Use all upper case for variables that have a constant value, PI for the value of pi (3. 14159…) (see text for more examples) 2020/11/22 The college of Computer Science & Technology 55
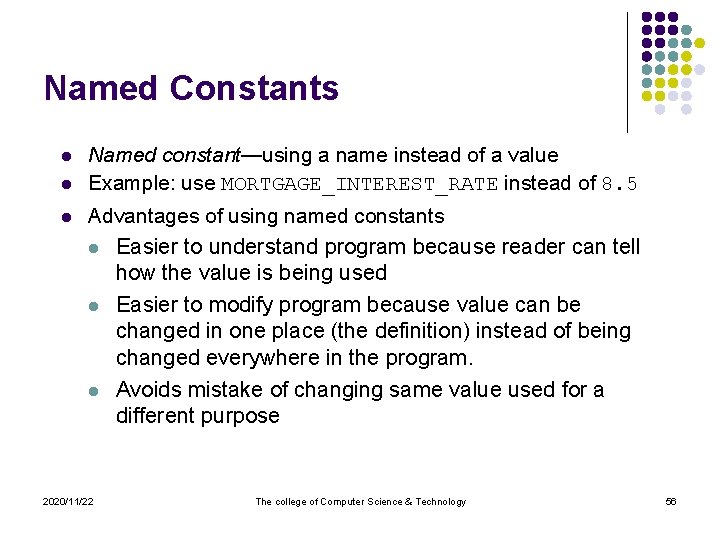
Named Constants l l l Named constant—using a name instead of a value Example: use MORTGAGE_INTEREST_RATE instead of 8. 5 Advantages of using named constants l Easier to understand program because reader can tell how the value is being used l l 2020/11/22 Easier to modify program because value can be changed in one place (the definition) instead of being changed everywhere in the program. Avoids mistake of changing same value used for a different purpose The college of Computer Science & Technology 56
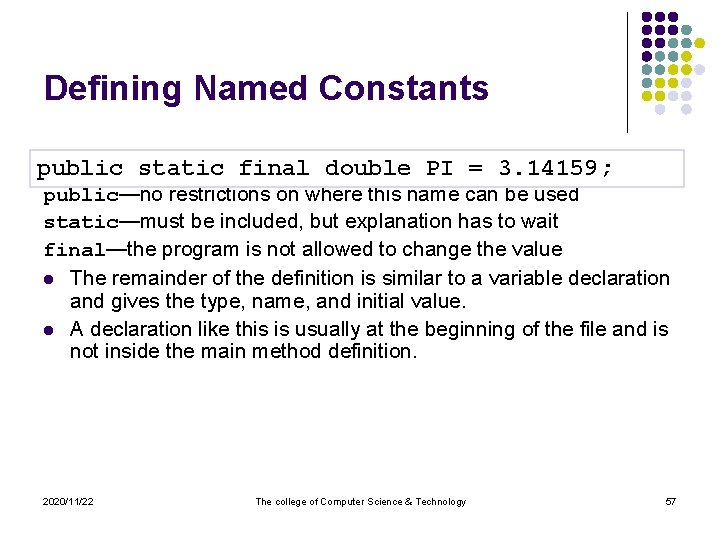
Defining Named Constants public static final double PI = 3. 14159; public—no restrictions on where this name can be used static—must be included, but explanation has to wait final—the program is not allowed to change the value l The remainder of the definition is similar to a variable declaration and gives the type, name, and initial value. l A declaration like this is usually at the beginning of the file and is not inside the main method definition. 2020/11/22 The college of Computer Science & Technology 57
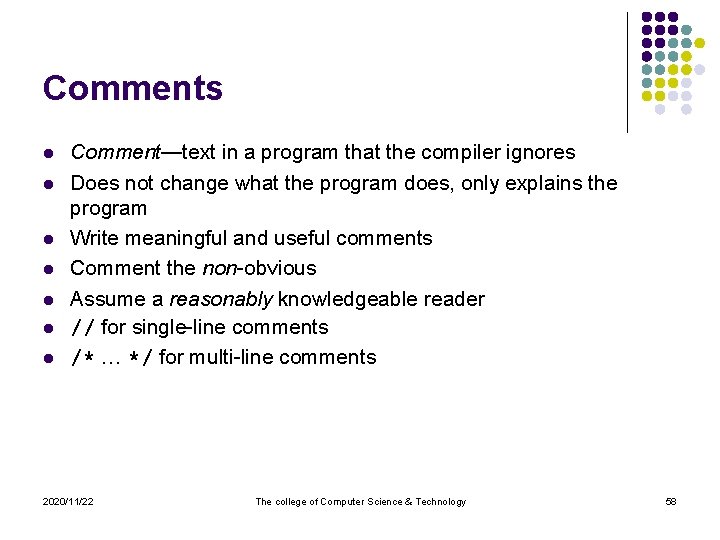
Comments l l l l Comment—text in a program that the compiler ignores Does not change what the program does, only explains the program Write meaningful and useful comments Comment the non-obvious Assume a reasonably knowledgeable reader // for single-line comments /* … */ for multi-line comments 2020/11/22 The college of Computer Science & Technology 58
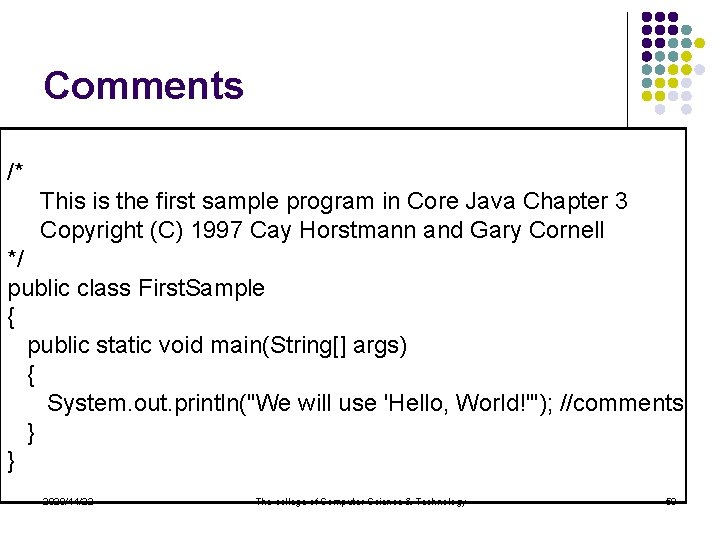
Comments /* This is the first sample program in Core Java Chapter 3 Copyright (C) 1997 Cay Horstmann and Gary Cornell */ public class First. Sample { public static void main(String[] args) { System. out. println("We will use 'Hello, World!'"); //comments } } 2020/11/22 The college of Computer Science & Technology 59
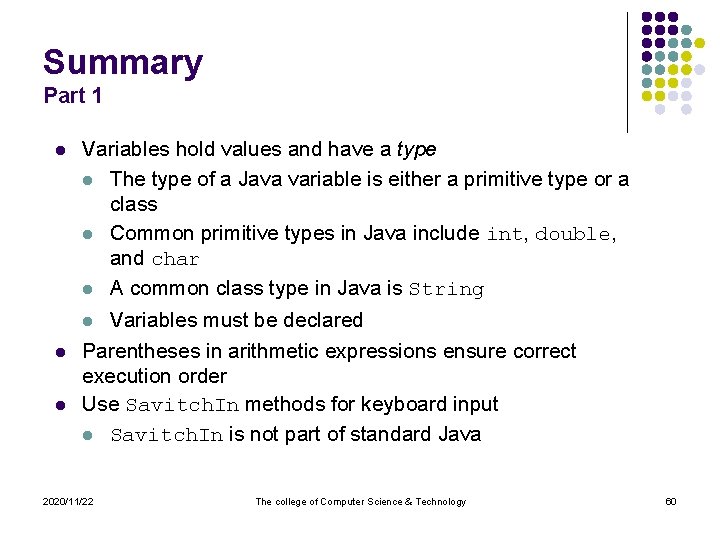
Summary Part 1 l Variables hold values and have a type l The type of a Java variable is either a primitive type or a class l Common primitive types in Java include int, double, and char l A common class type in Java is String Variables must be declared Parentheses in arithmetic expressions ensure correct execution order Use Savitch. In methods for keyboard input l Savitch. In is not part of standard Java l l l 2020/11/22 The college of Computer Science & Technology 60
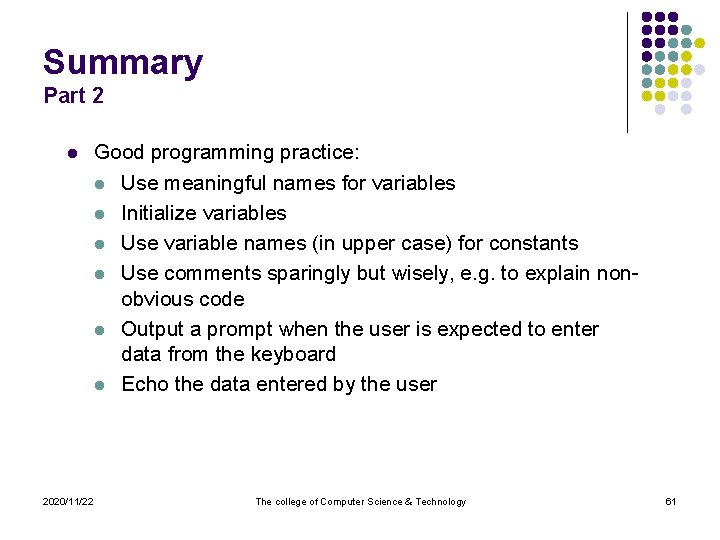
Summary Part 2 l 2020/11/22 Good programming practice: l Use meaningful names for variables l Initialize variables l Use variable names (in upper case) for constants l Use comments sparingly but wisely, e. g. to explain nonobvious code l Output a prompt when the user is expected to enter data from the keyboard l Echo the data entered by the user The college of Computer Science & Technology 61
Non primitive data structure
Data structure primitive and non primitive
Primitive vs reference types java
Unit 1 primitive types
Simple present simple past simple future
Primitive root in cryptography and network security
Semaphore provides a primitive yet powerful and flexible
Greek mythology study guide
Present simple past simple future simple exercise
Present simple future simple past simple
Present simple present continuous past simple future simple
Present continuous past continuous future continuous
Past present simple
Use of has been and have been
Simple present exemplos
Present simple present continuous 4 класс
Wigner-seitz primitive cell
Primitive coping mechanism
Parachute reflex
Political socialization definition
Pretrematic and post trematic nerves
Physical education in renaissance period
Dfd chapter 7
Infant reflexes chart
Primitive streak is formed from
Primitive genre cycle
What is primitive root
Difese primitive
Solid modeling in computer graphics
Hexagonal unit cell
Comment empiler des sphères
Fibrinolyse primitive
What are hyphae?
Primitive regular expressions
Primitive
Intraembryonic mesoderm
Psychosexual stages
Primitive
Non primitive data structure
Ankylo glossia
Primitive subsistence agriculture
Primitive testing 4
Primitive fire starting
Output primitive
Primitive instructions
Pragmatism in physical education
Dore's primitive speech acts
Notochord process
Vibration of crystals with monatomic basis
Where does the word "technology" comes from?
Planck's radiation law
Father of physical education in germany
Find primitive root of prime number
Primitive neuroectodermal tumor
Joseph lister
Progressive succession:
Primitive
Primitive neuroectodermal tumor
Primitive body cavity
First organic compound which was formed in primitive ocean
How do you write an input and output algorithm?
Counting primitive operations