Chapter 10 Using Menus and Validating Input Programming
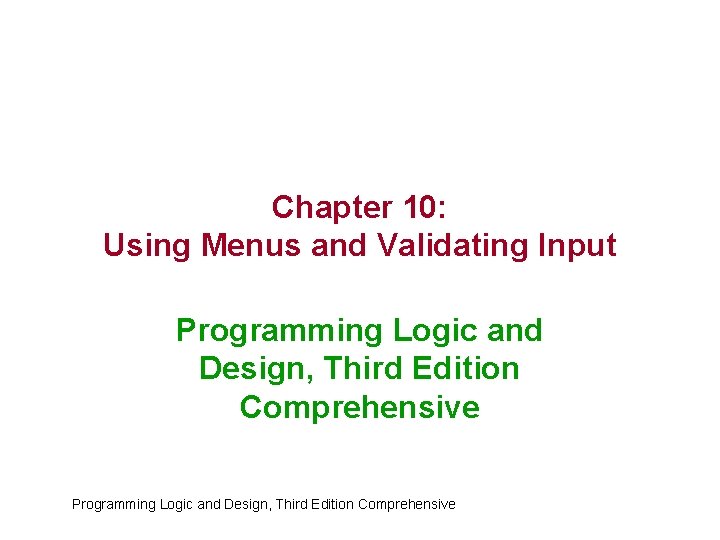
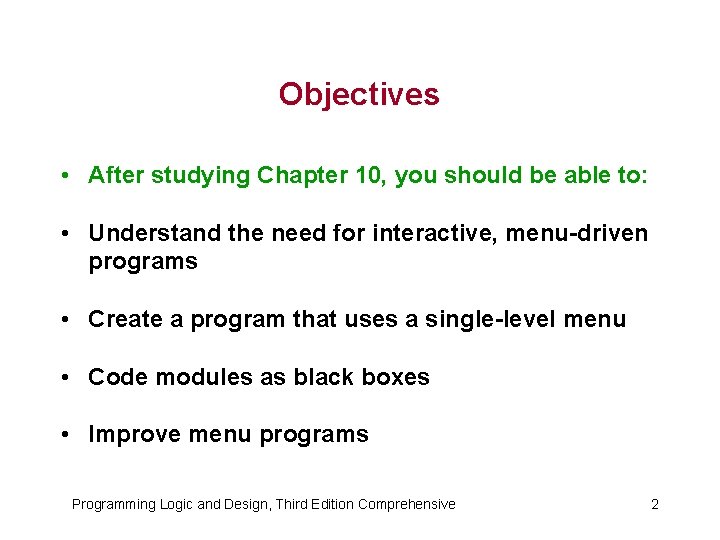
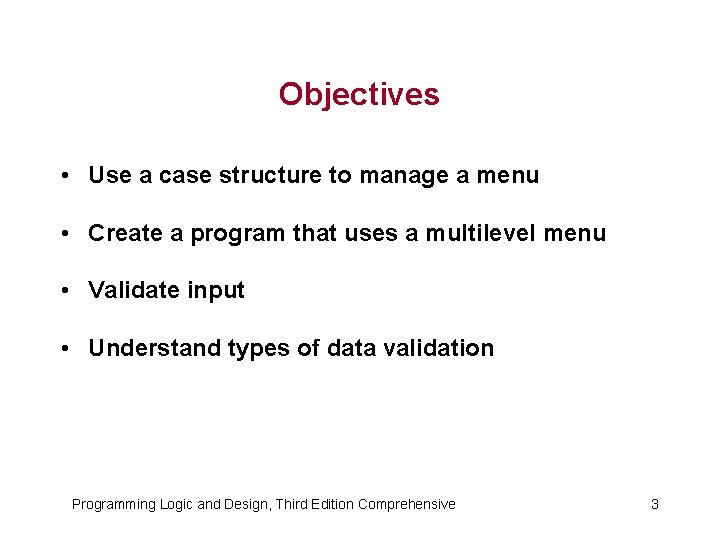
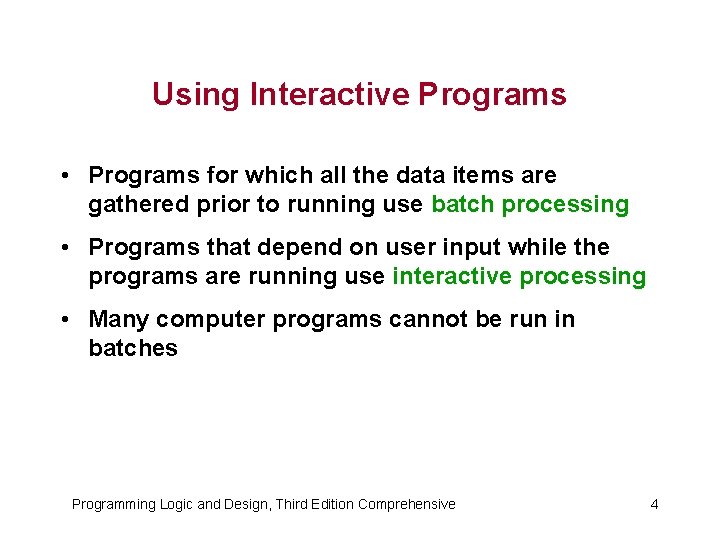
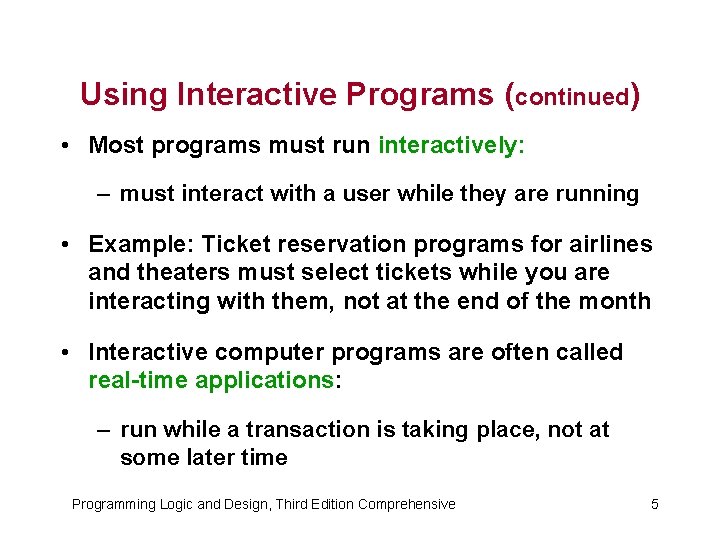
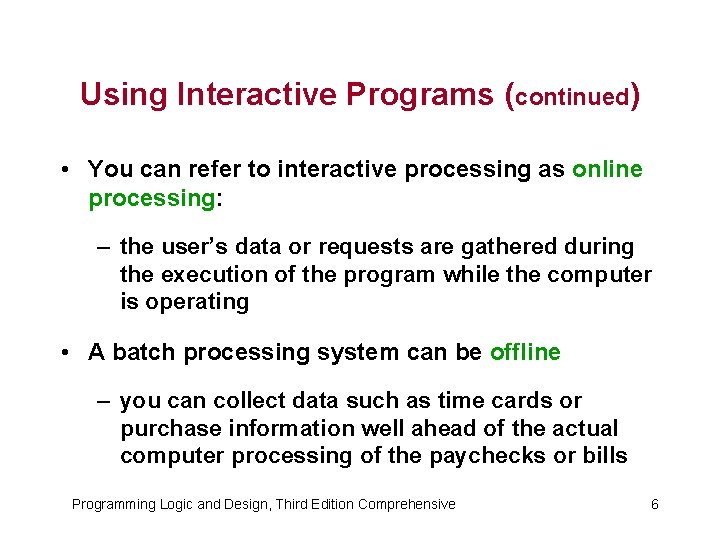
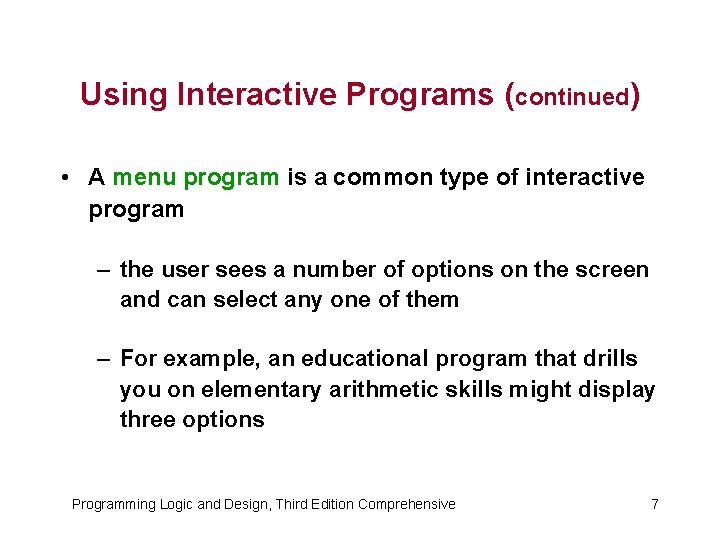
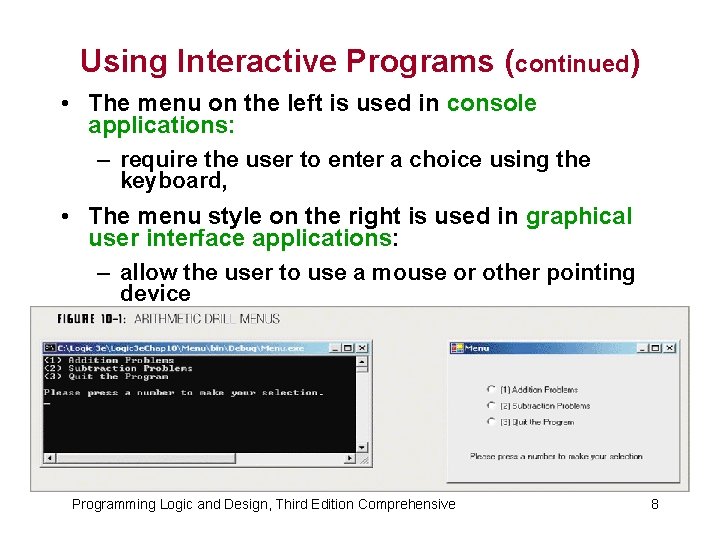
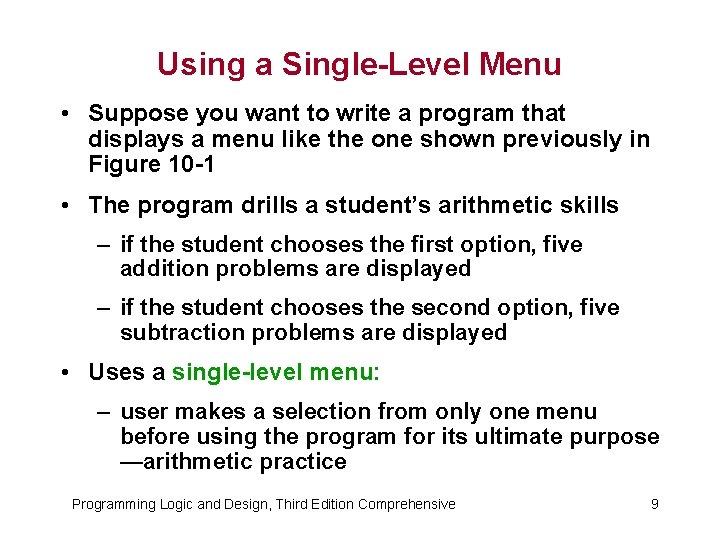
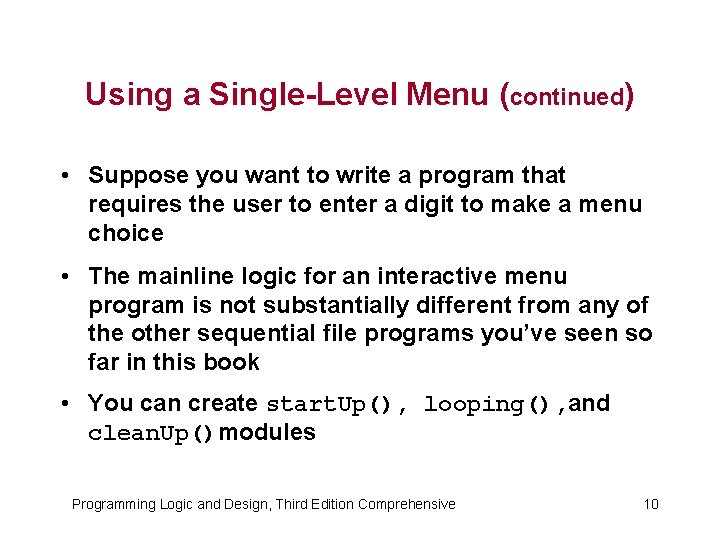
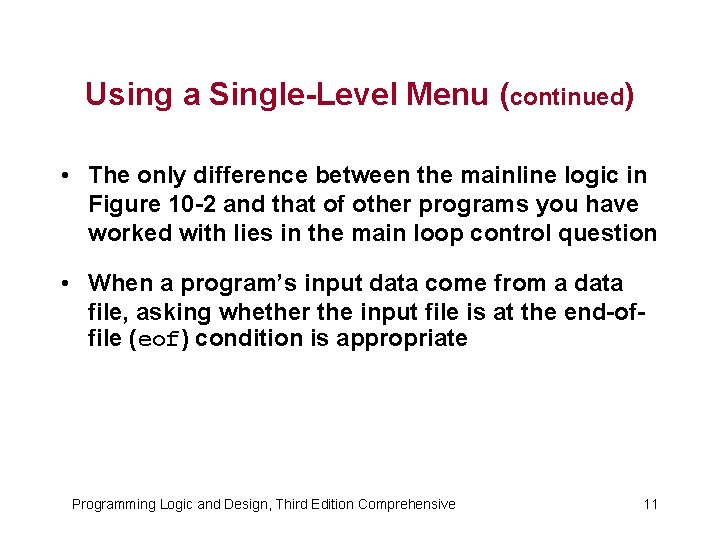
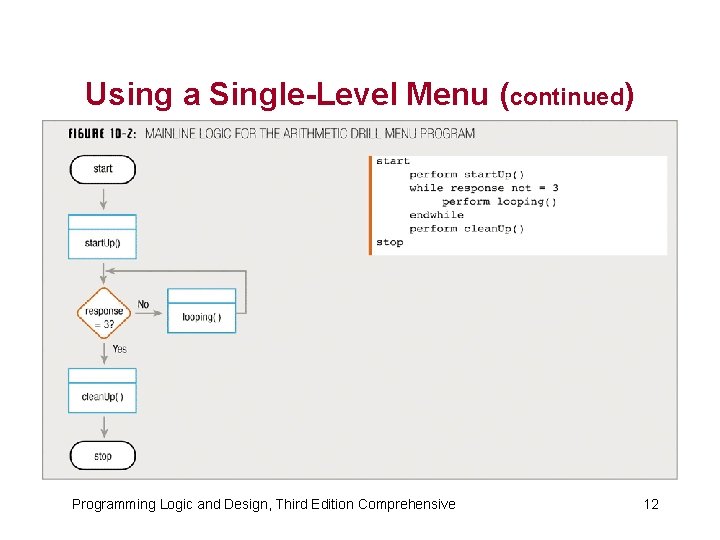
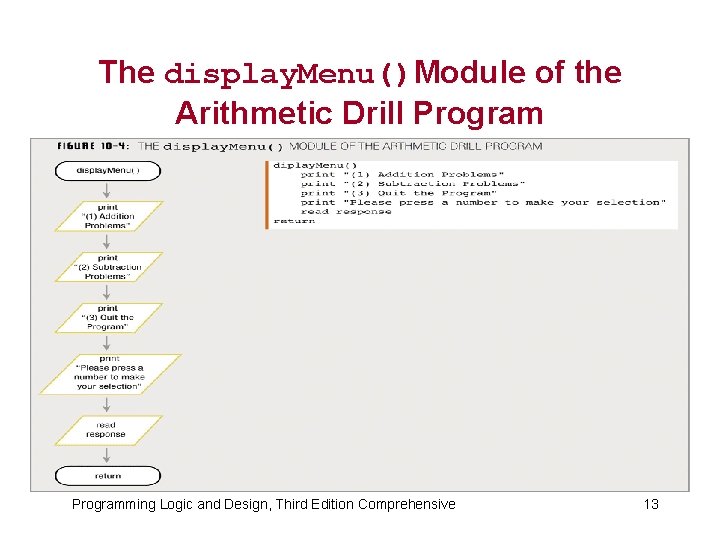
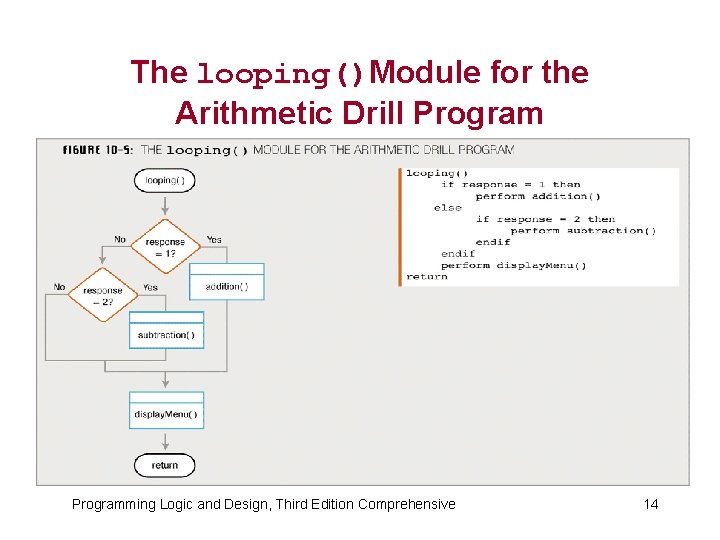
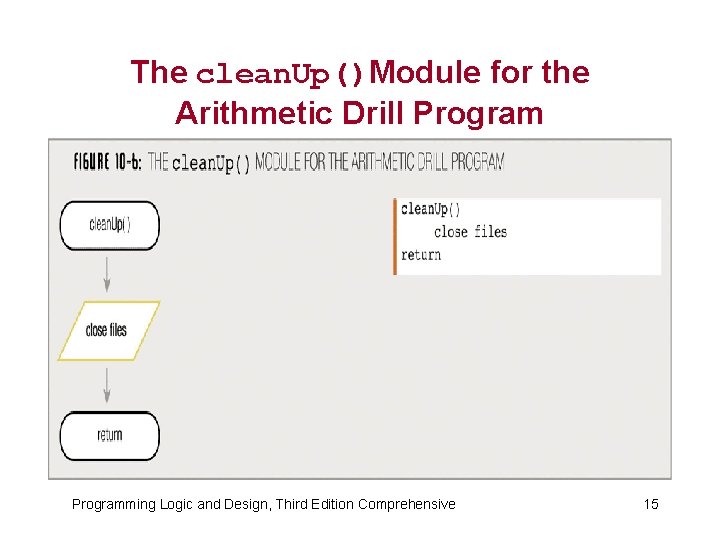
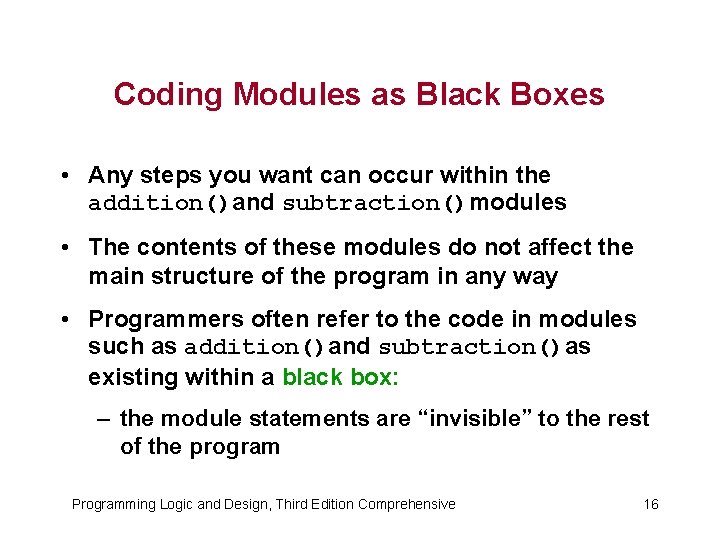
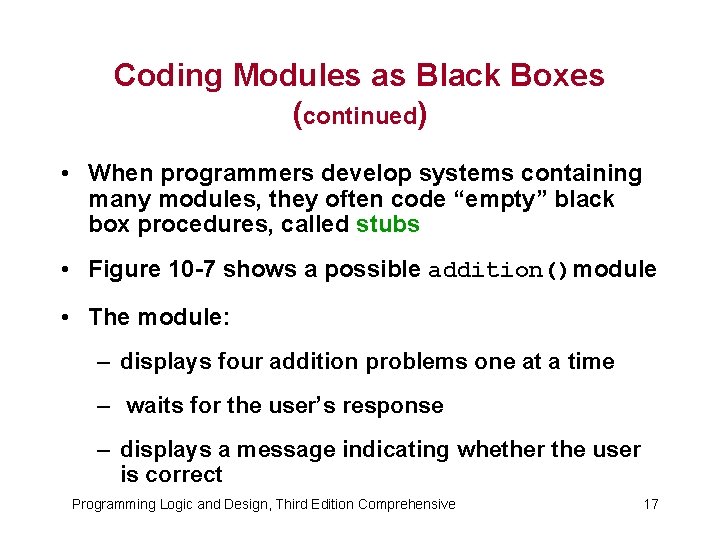
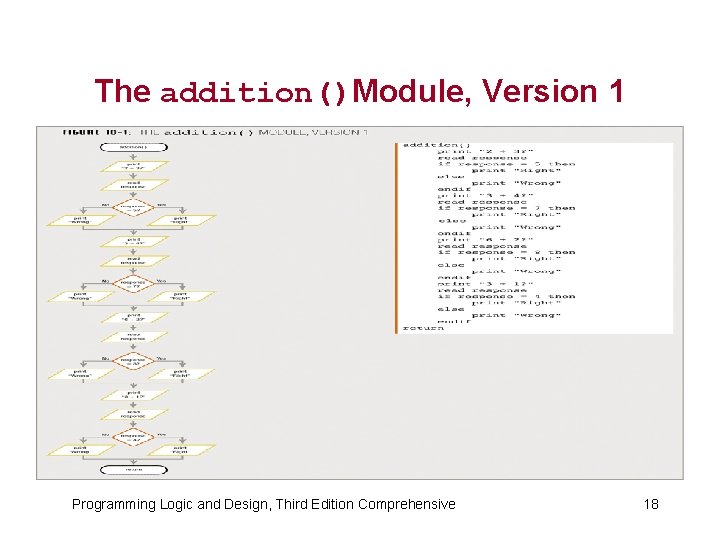
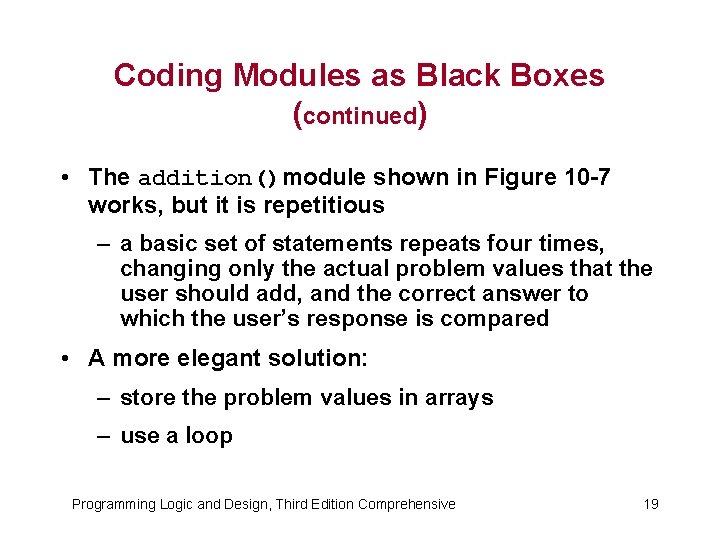
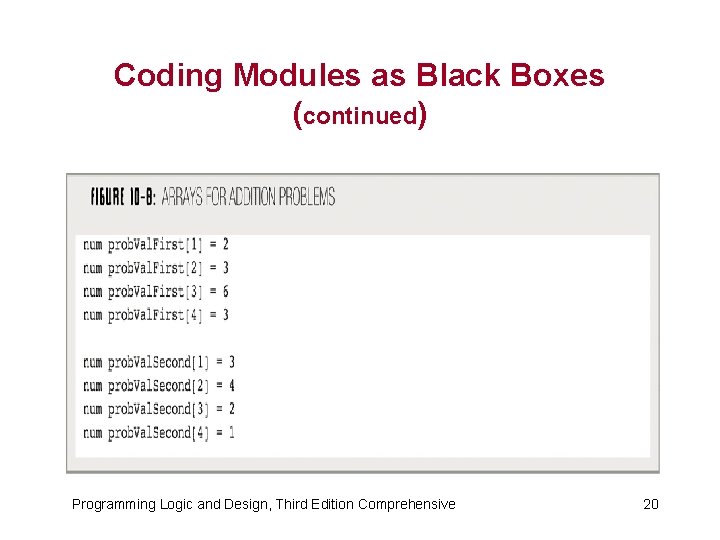
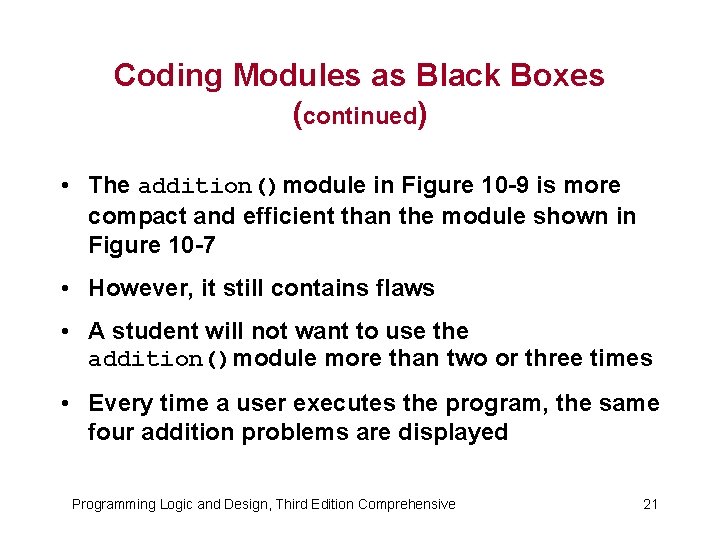
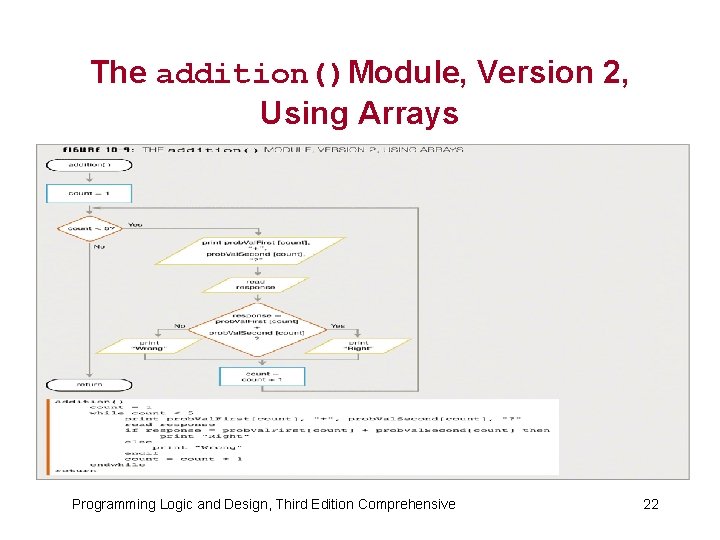
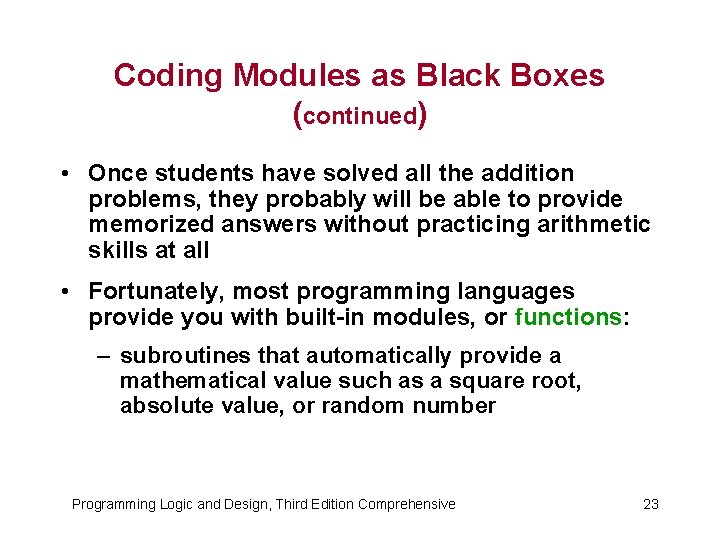
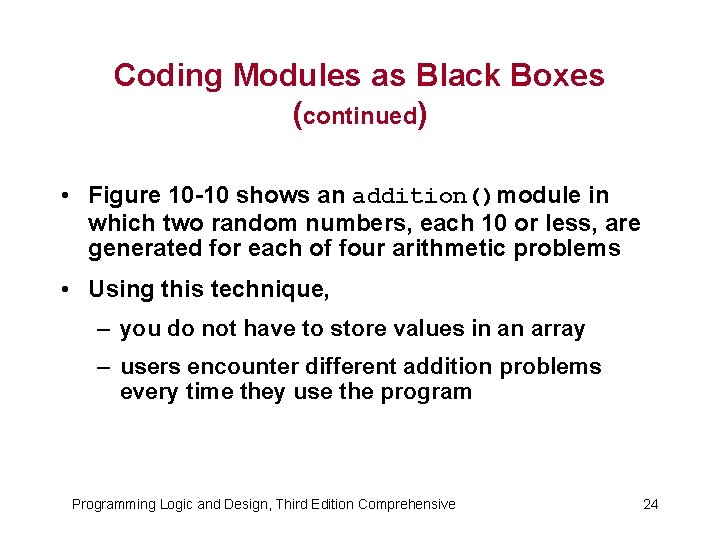
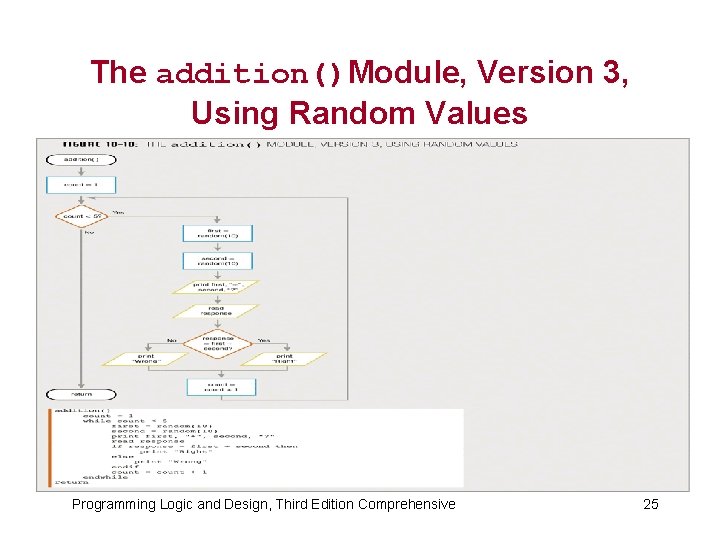
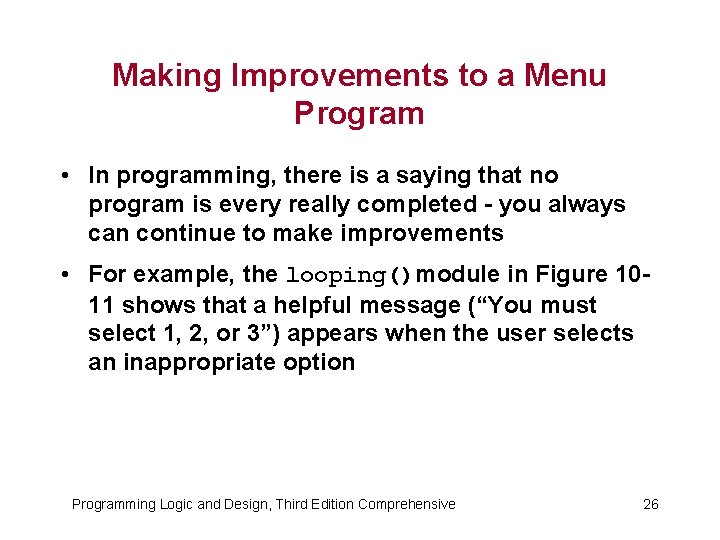
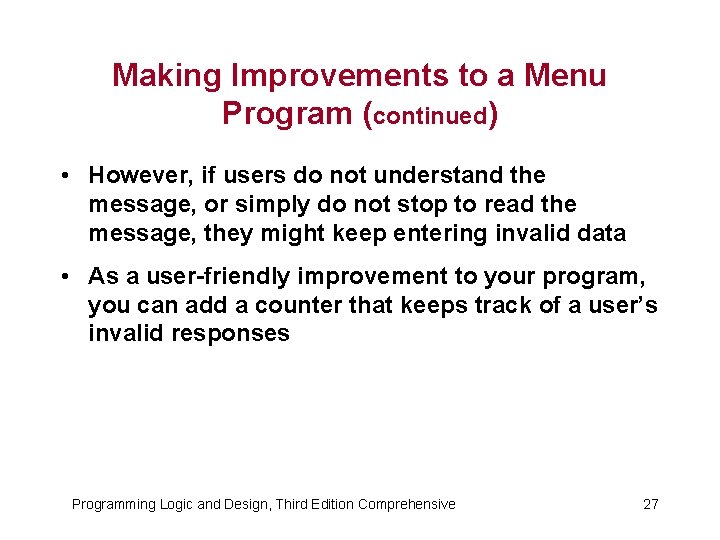
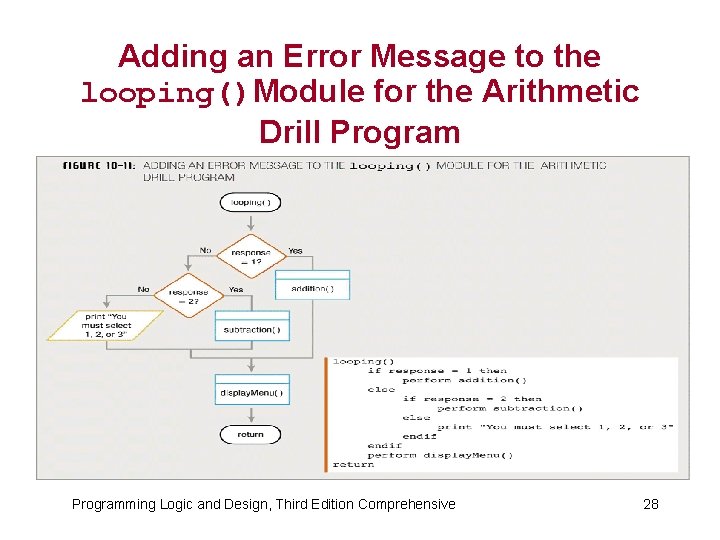
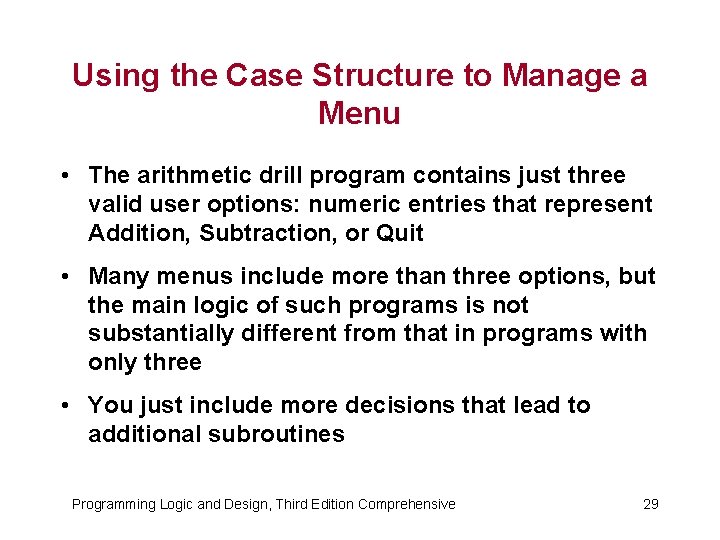
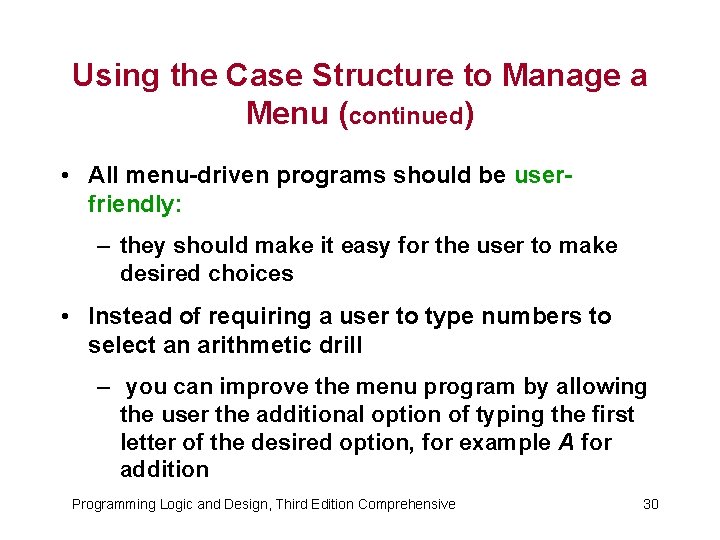
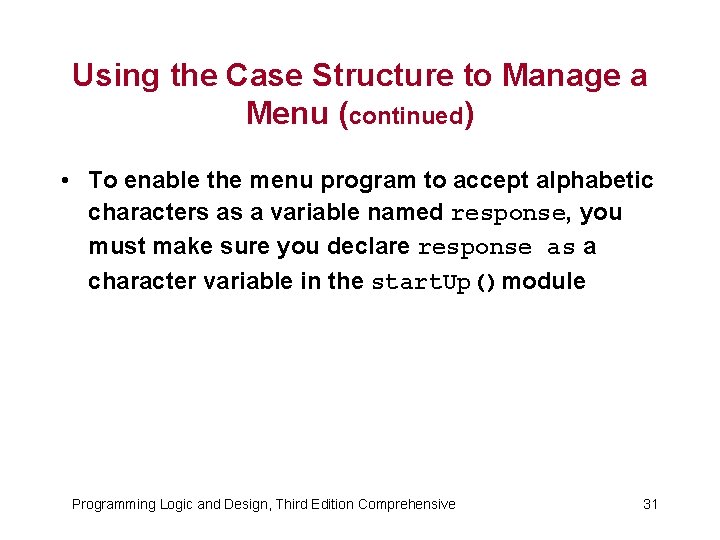
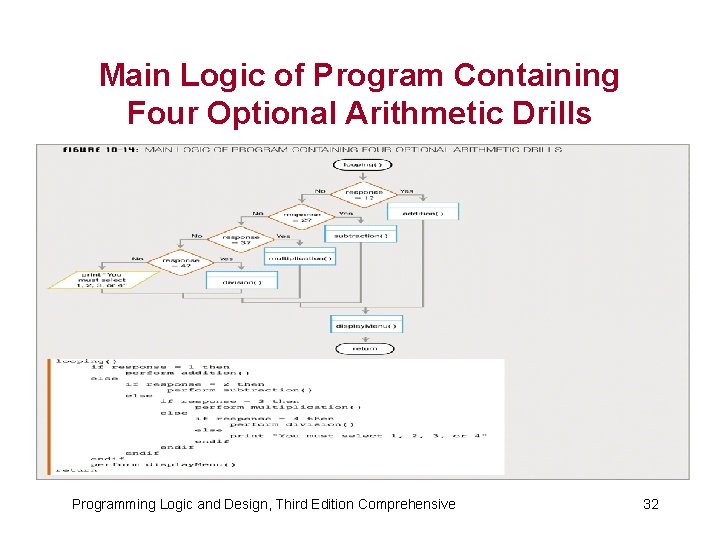
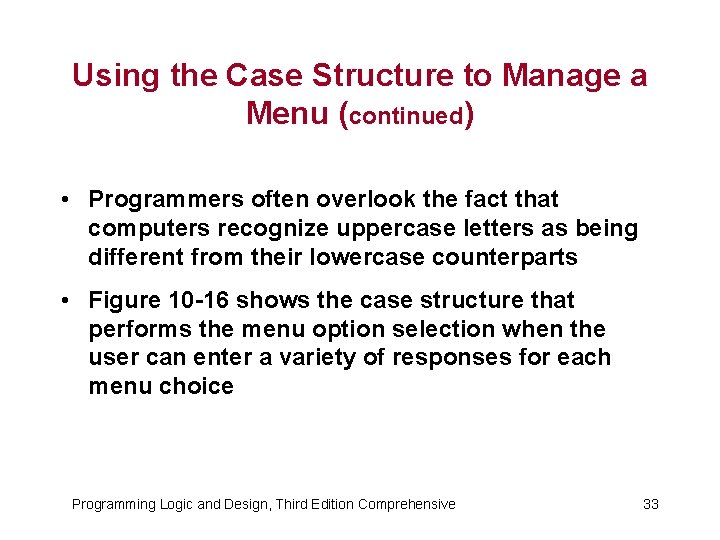
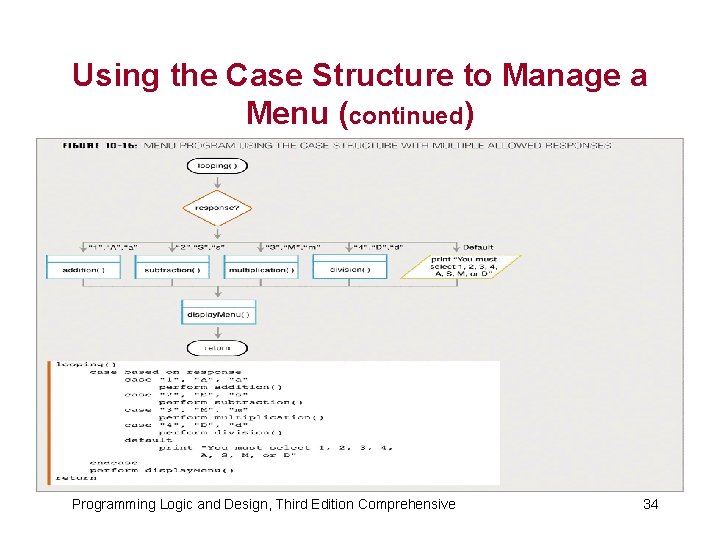
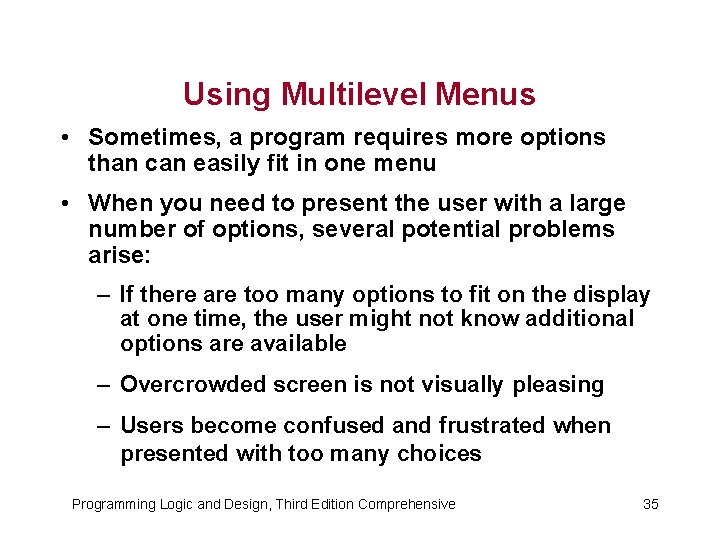
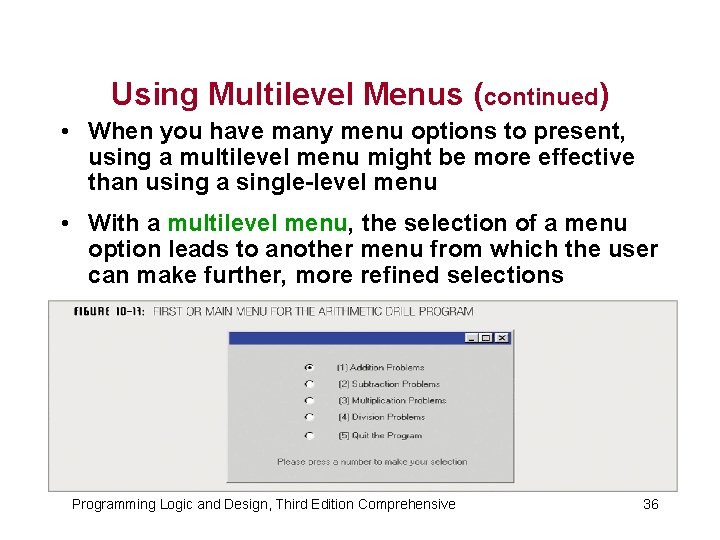
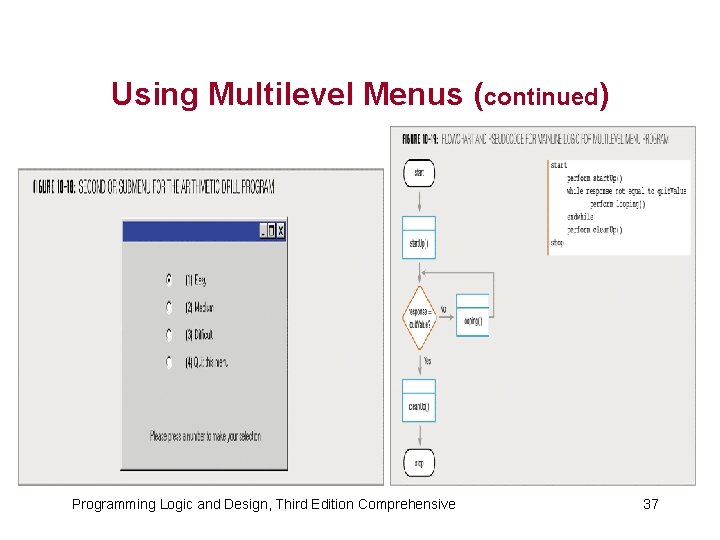
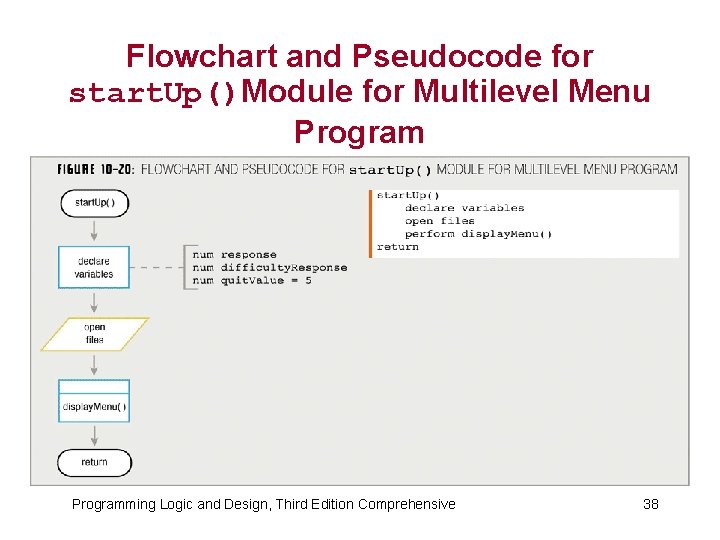
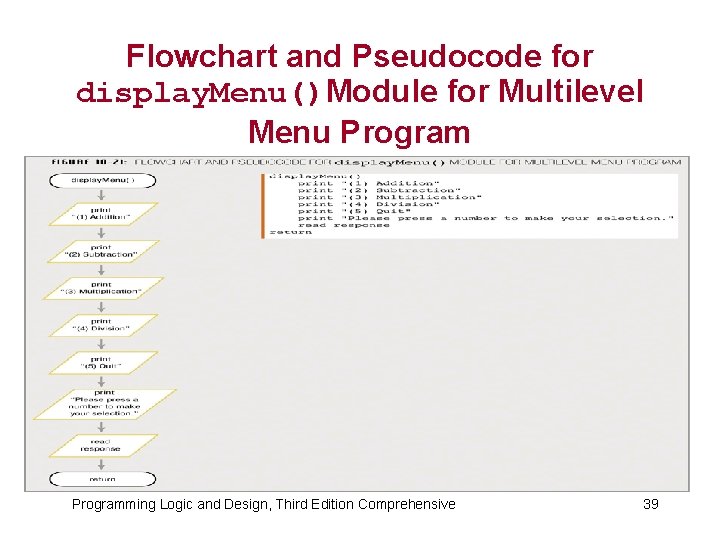
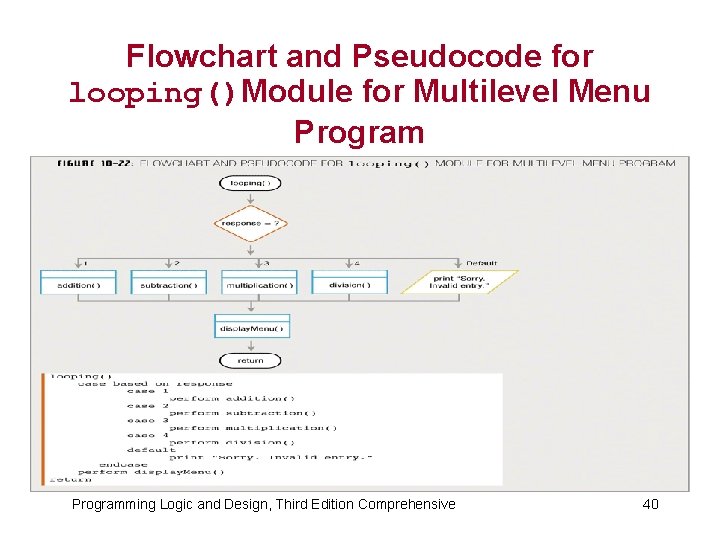
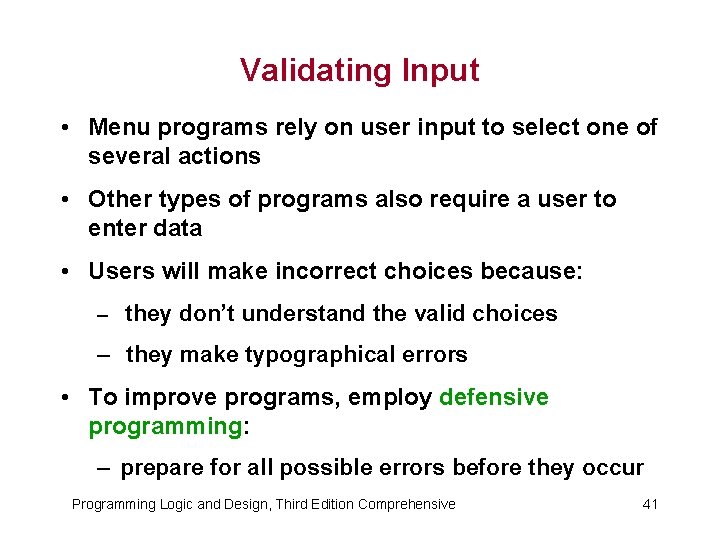
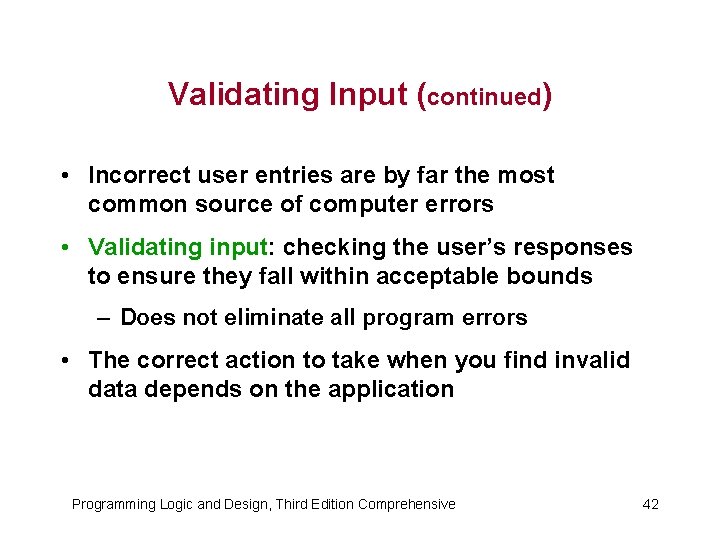
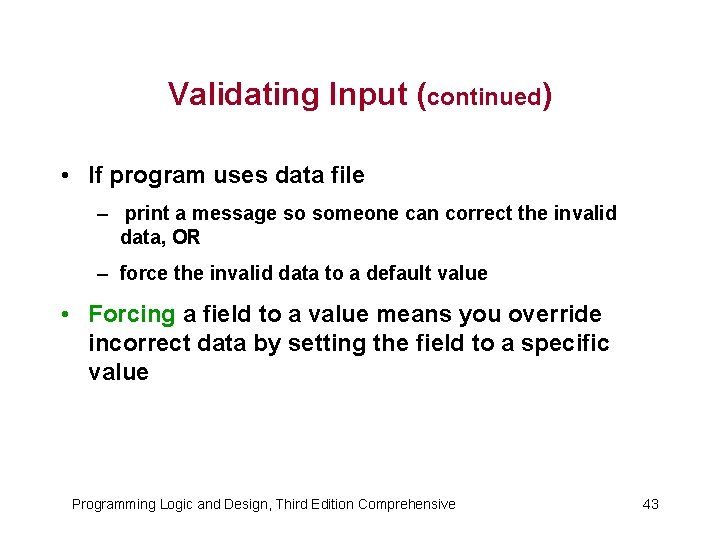
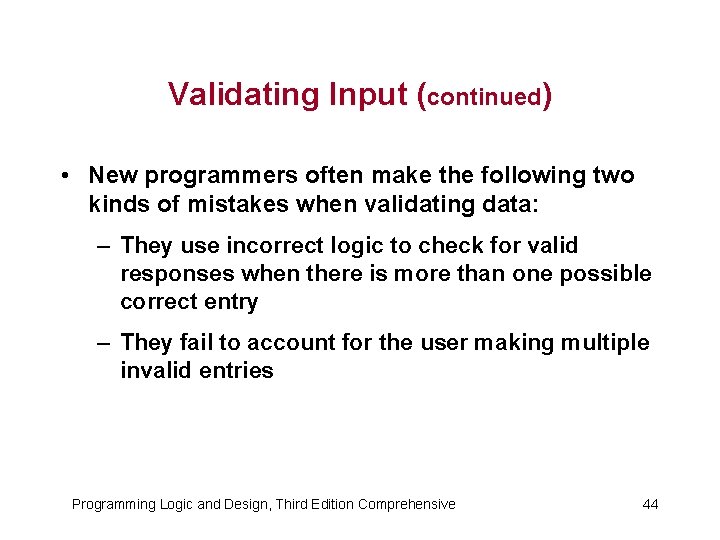
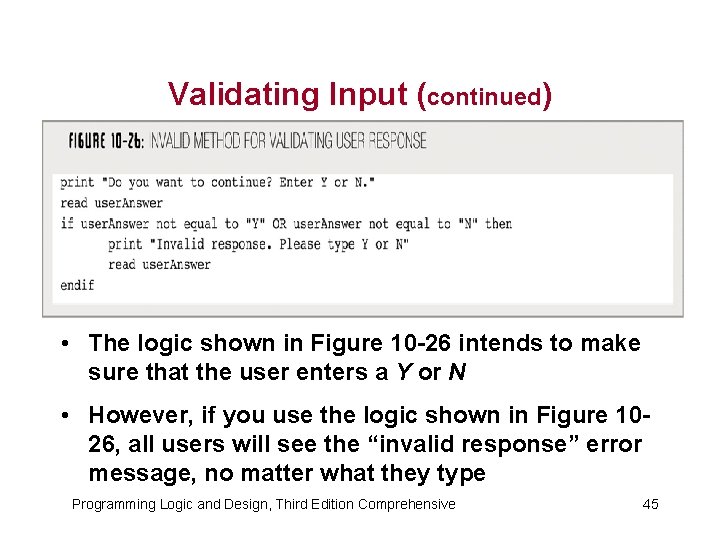
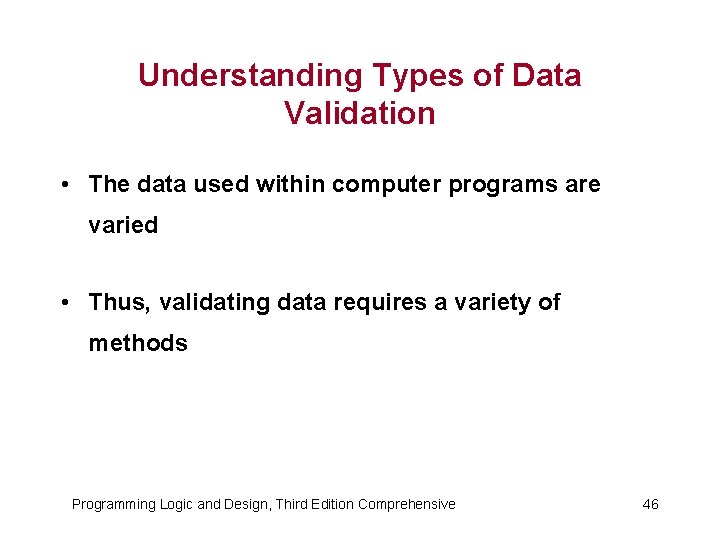
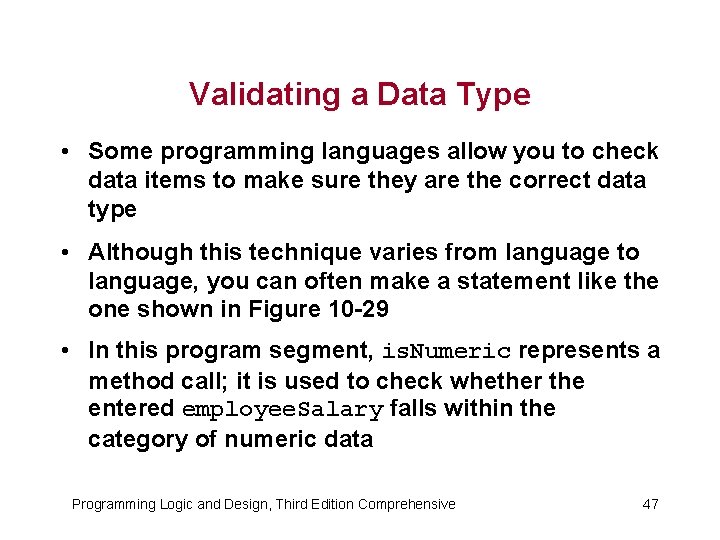
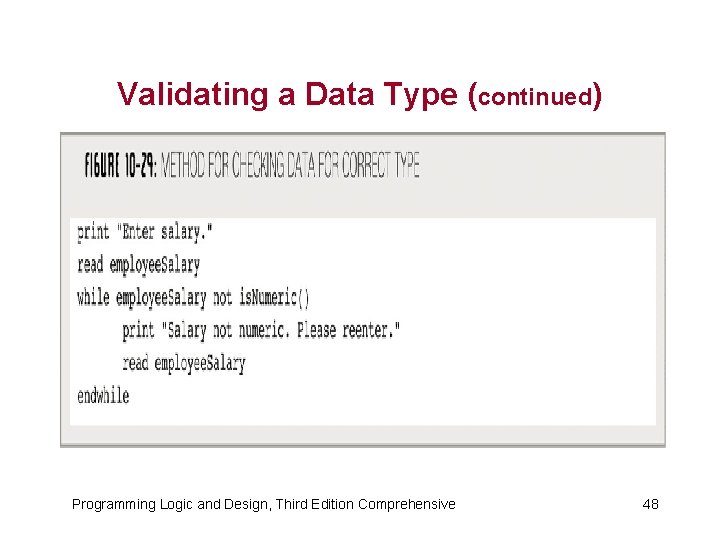
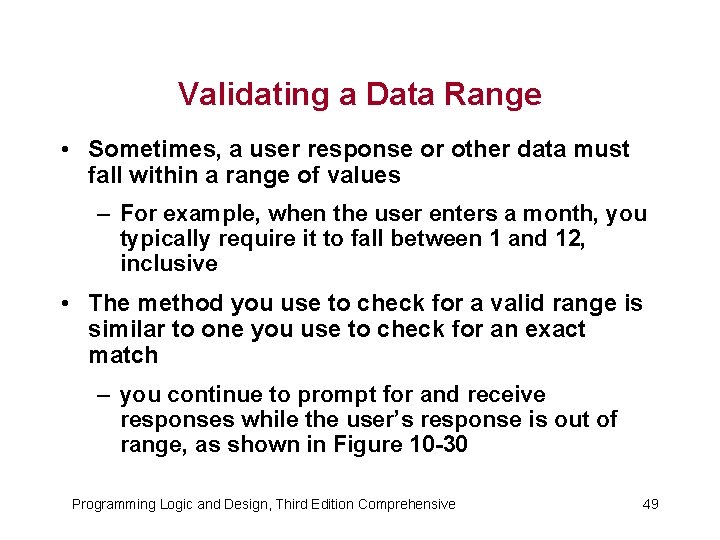
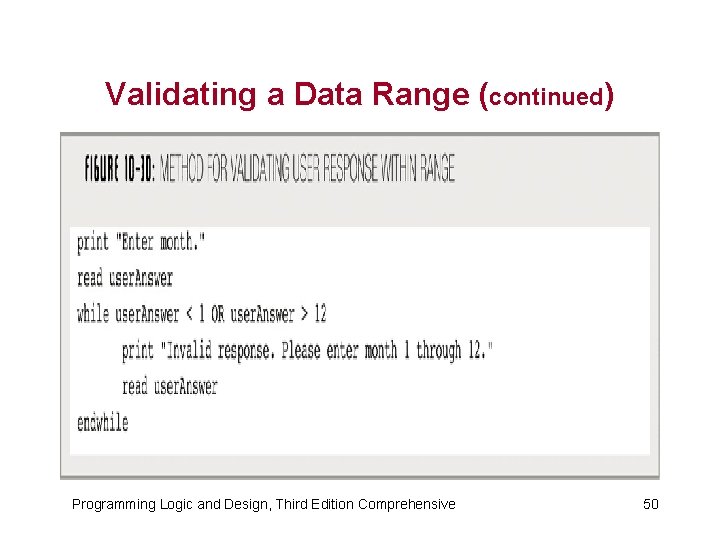
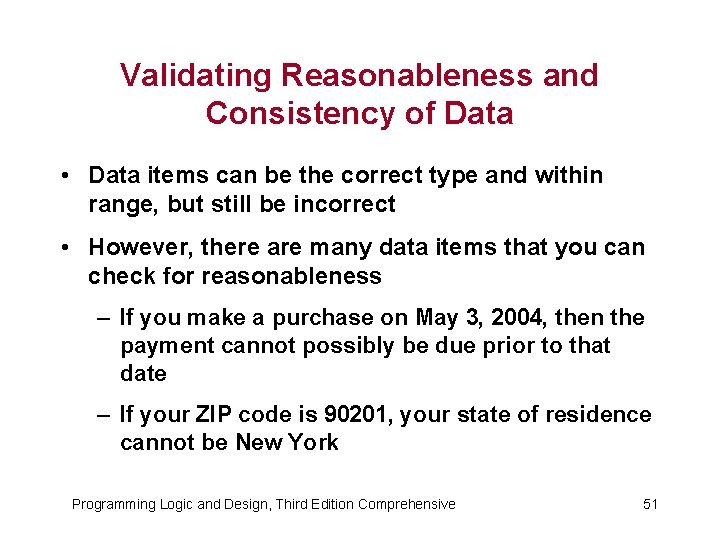
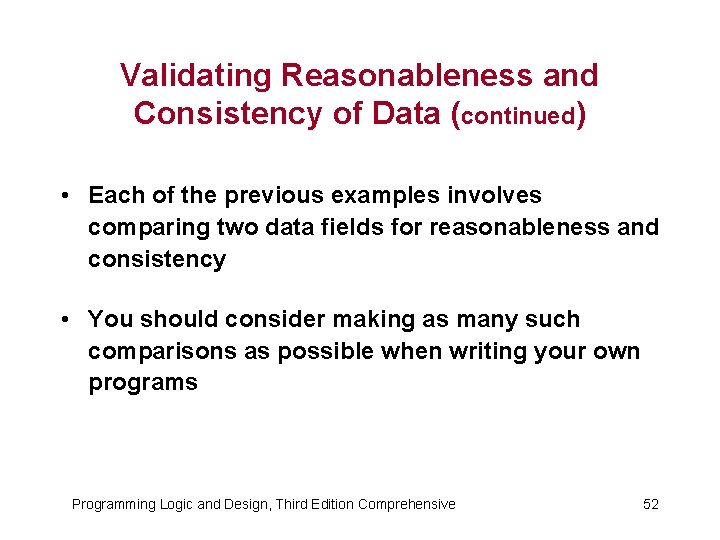
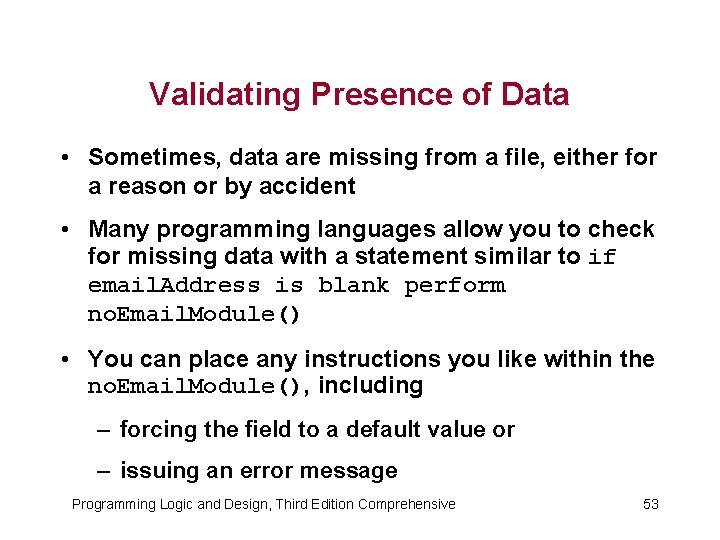
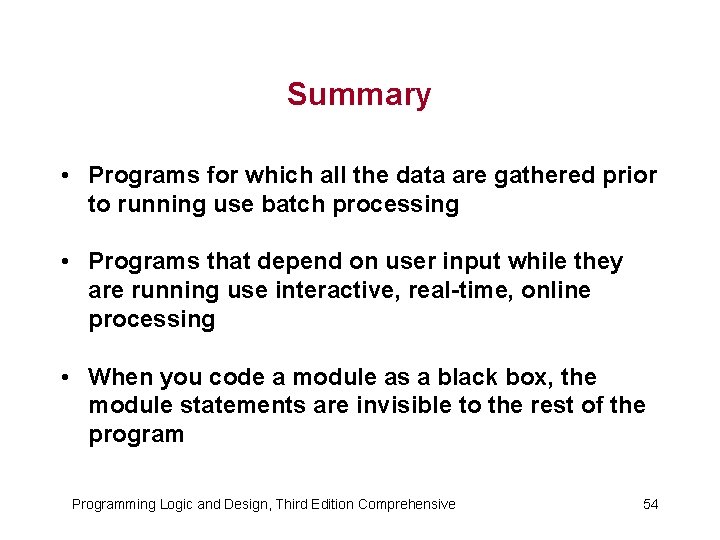
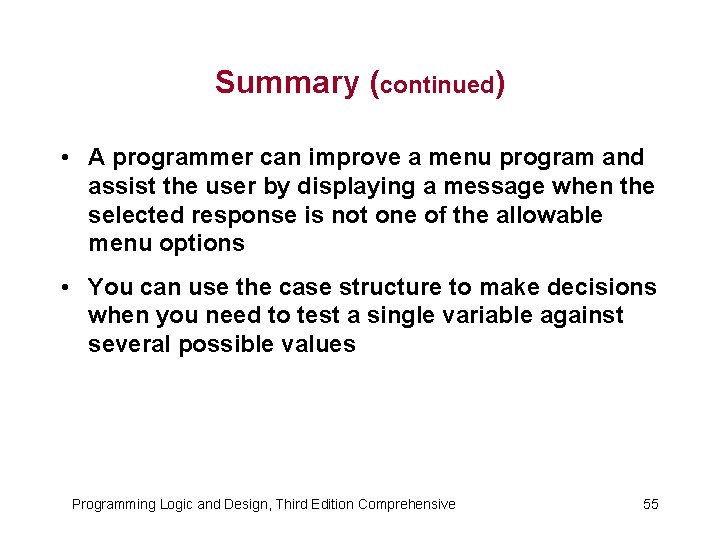
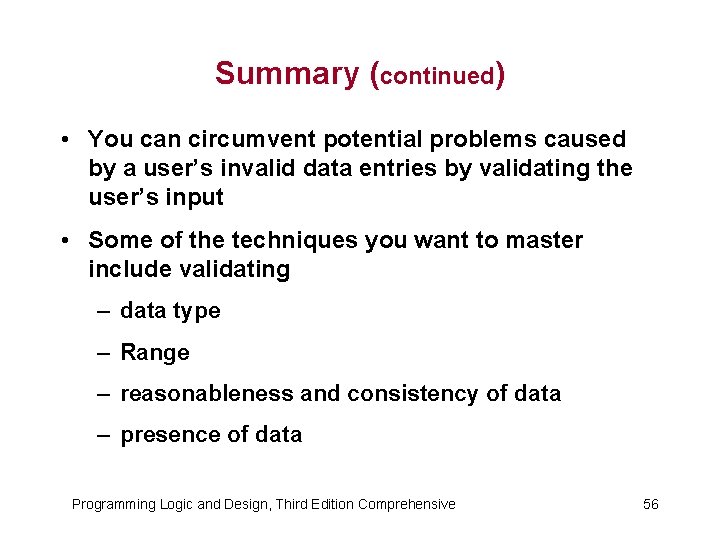
- Slides: 56
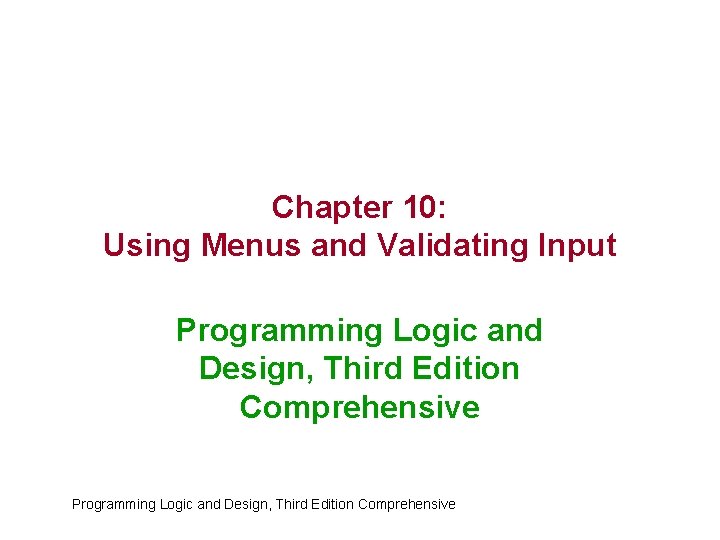
Chapter 10: Using Menus and Validating Input Programming Logic and Design, Third Edition Comprehensive
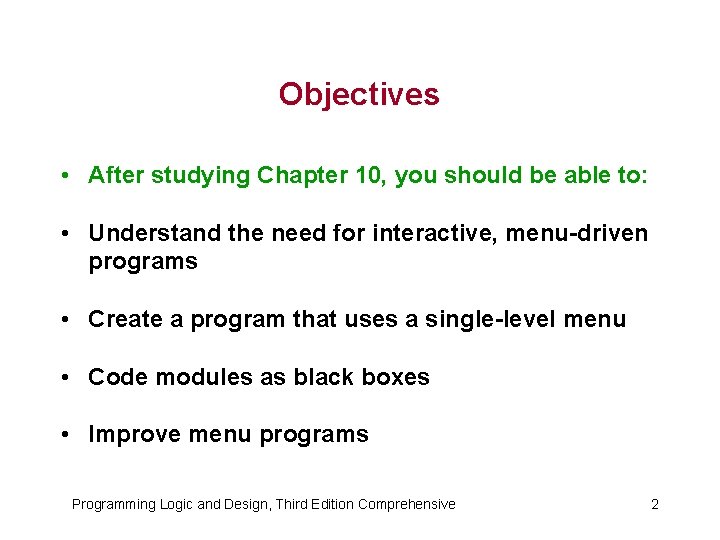
Objectives • After studying Chapter 10, you should be able to: • Understand the need for interactive, menu-driven programs • Create a program that uses a single-level menu • Code modules as black boxes • Improve menu programs Programming Logic and Design, Third Edition Comprehensive 2
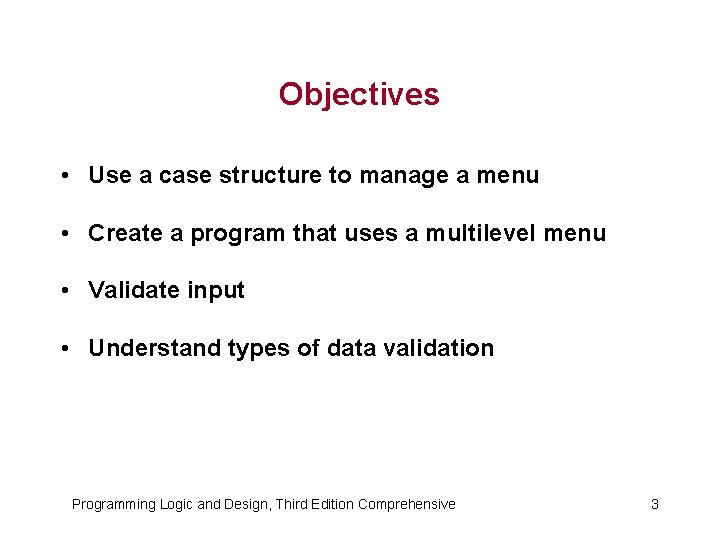
Objectives • Use a case structure to manage a menu • Create a program that uses a multilevel menu • Validate input • Understand types of data validation Programming Logic and Design, Third Edition Comprehensive 3
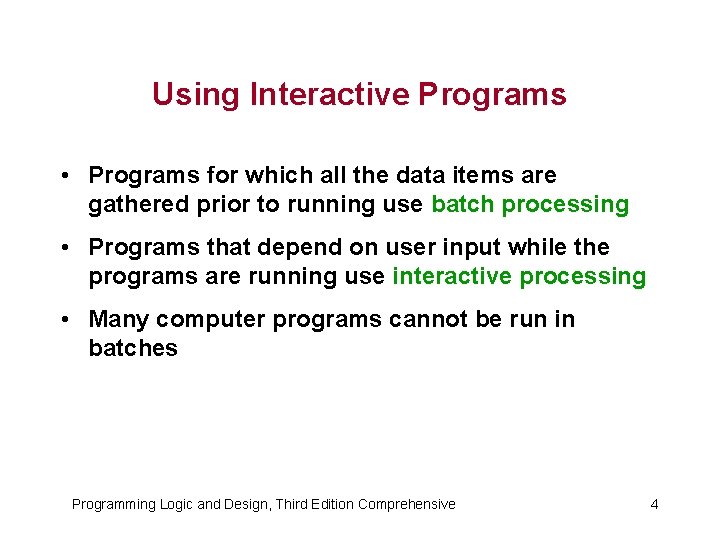
Using Interactive Programs • Programs for which all the data items are gathered prior to running use batch processing • Programs that depend on user input while the programs are running use interactive processing • Many computer programs cannot be run in batches Programming Logic and Design, Third Edition Comprehensive 4
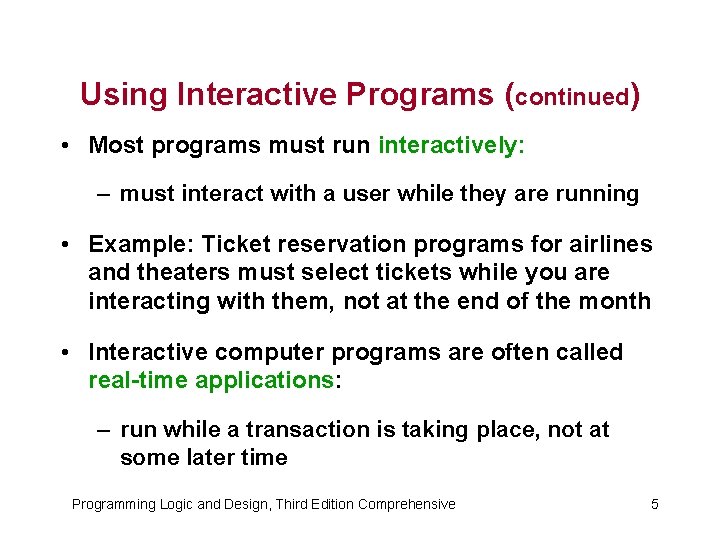
Using Interactive Programs (continued) • Most programs must run interactively: – must interact with a user while they are running • Example: Ticket reservation programs for airlines and theaters must select tickets while you are interacting with them, not at the end of the month • Interactive computer programs are often called real-time applications: – run while a transaction is taking place, not at some later time Programming Logic and Design, Third Edition Comprehensive 5
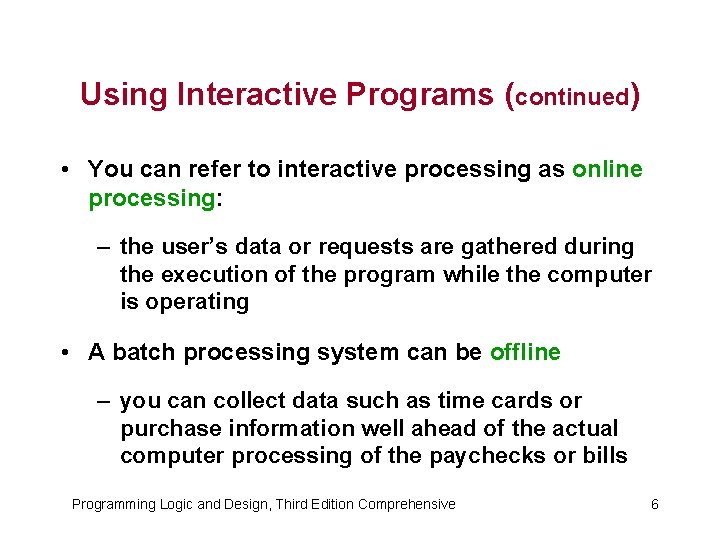
Using Interactive Programs (continued) • You can refer to interactive processing as online processing: – the user’s data or requests are gathered during the execution of the program while the computer is operating • A batch processing system can be offline – you can collect data such as time cards or purchase information well ahead of the actual computer processing of the paychecks or bills Programming Logic and Design, Third Edition Comprehensive 6
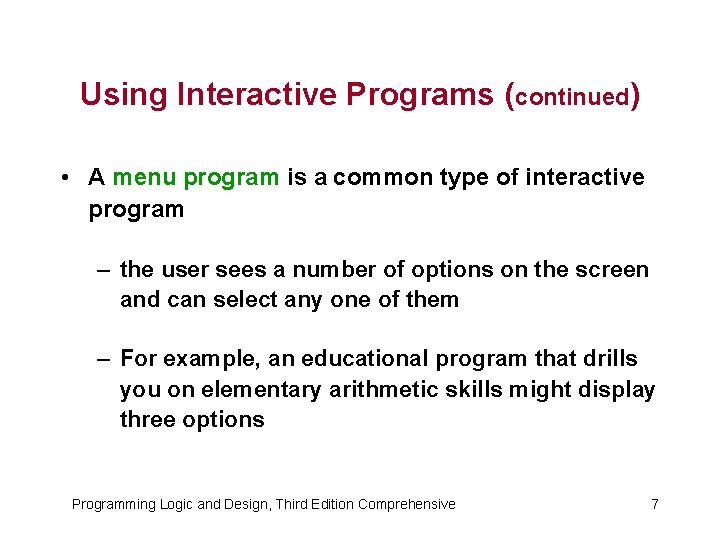
Using Interactive Programs (continued) • A menu program is a common type of interactive program – the user sees a number of options on the screen and can select any one of them – For example, an educational program that drills you on elementary arithmetic skills might display three options Programming Logic and Design, Third Edition Comprehensive 7
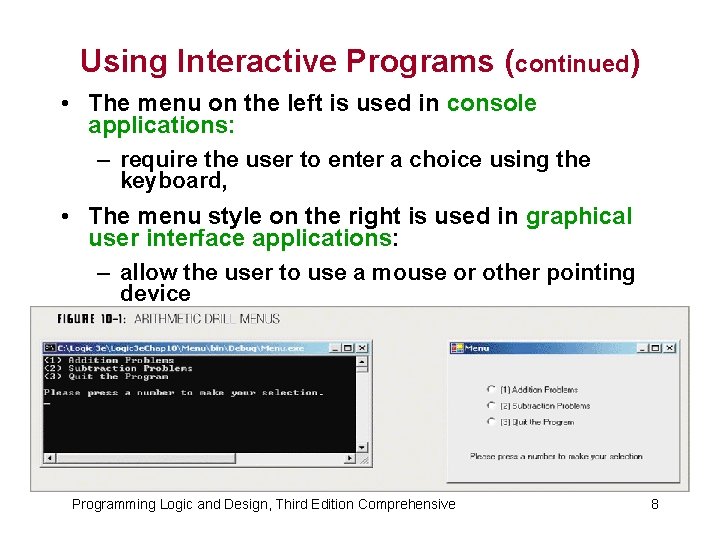
Using Interactive Programs (continued) • The menu on the left is used in console applications: – require the user to enter a choice using the keyboard, • The menu style on the right is used in graphical user interface applications: – allow the user to use a mouse or other pointing device Programming Logic and Design, Third Edition Comprehensive 8
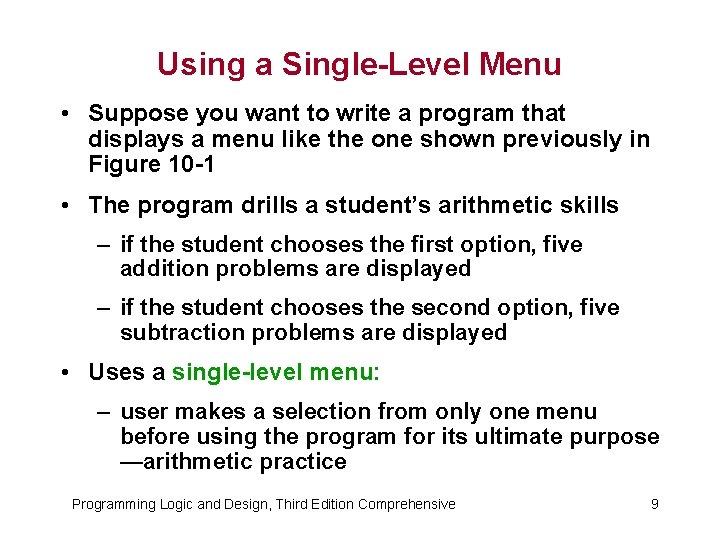
Using a Single-Level Menu • Suppose you want to write a program that displays a menu like the one shown previously in Figure 10 -1 • The program drills a student’s arithmetic skills – if the student chooses the first option, five addition problems are displayed – if the student chooses the second option, five subtraction problems are displayed • Uses a single-level menu: – user makes a selection from only one menu before using the program for its ultimate purpose —arithmetic practice Programming Logic and Design, Third Edition Comprehensive 9
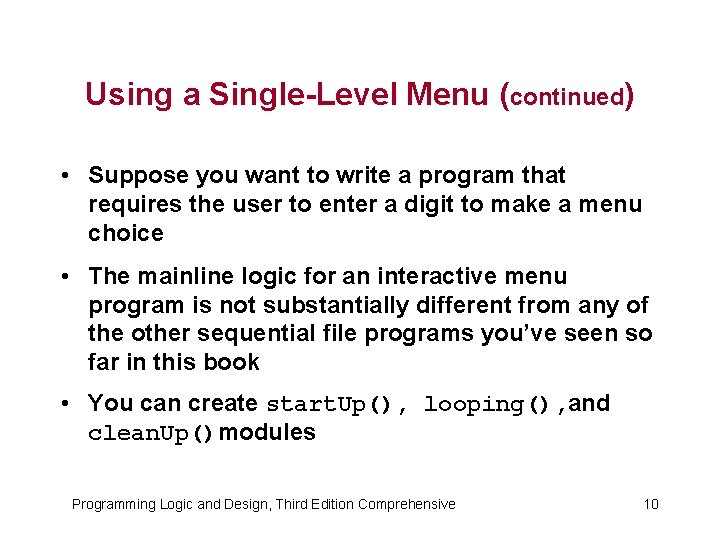
Using a Single-Level Menu (continued) • Suppose you want to write a program that requires the user to enter a digit to make a menu choice • The mainline logic for an interactive menu program is not substantially different from any of the other sequential file programs you’ve seen so far in this book • You can create start. Up(), looping(), and clean. Up()modules Programming Logic and Design, Third Edition Comprehensive 10
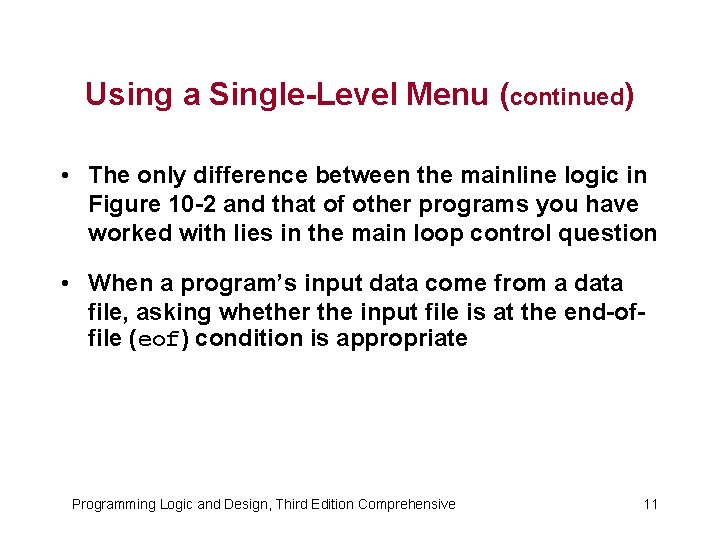
Using a Single-Level Menu (continued) • The only difference between the mainline logic in Figure 10 -2 and that of other programs you have worked with lies in the main loop control question • When a program’s input data come from a data file, asking whether the input file is at the end-offile (eof) condition is appropriate Programming Logic and Design, Third Edition Comprehensive 11
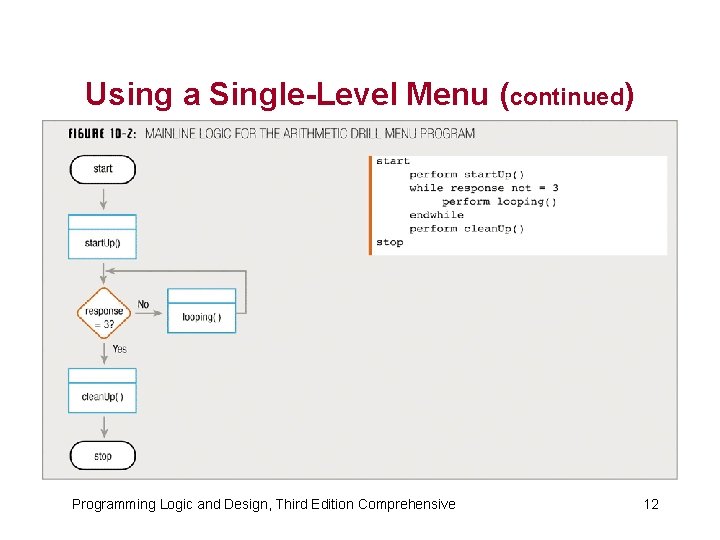
Using a Single-Level Menu (continued) Programming Logic and Design, Third Edition Comprehensive 12
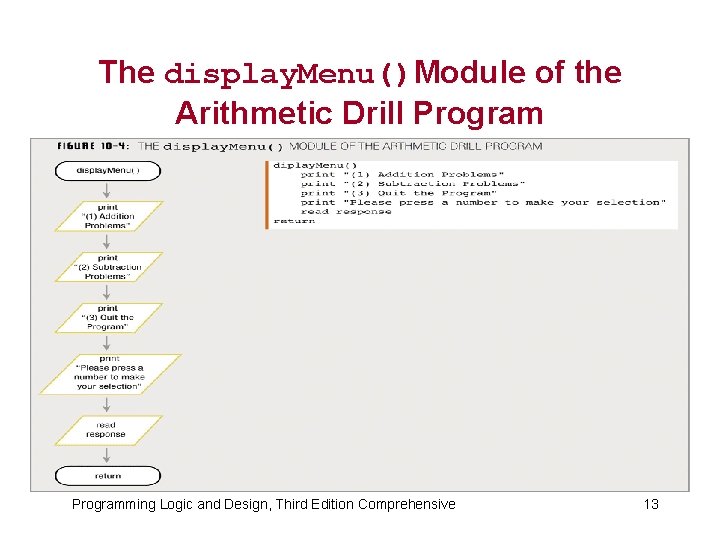
The display. Menu()Module of the Arithmetic Drill Programming Logic and Design, Third Edition Comprehensive 13
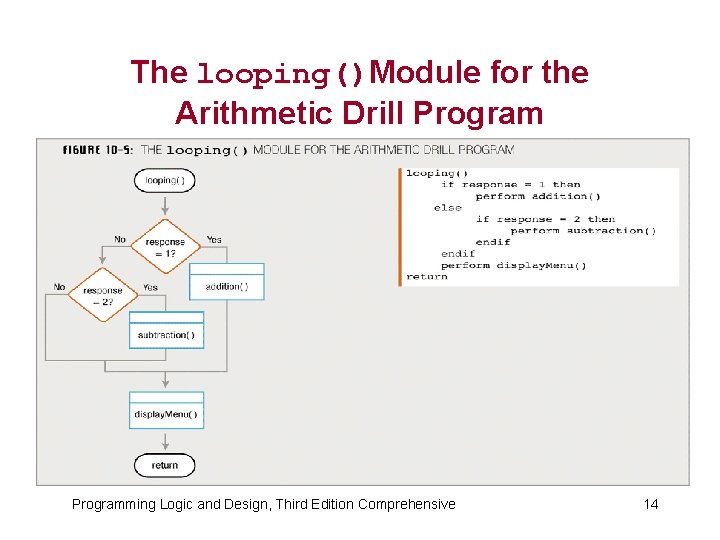
The looping()Module for the Arithmetic Drill Programming Logic and Design, Third Edition Comprehensive 14
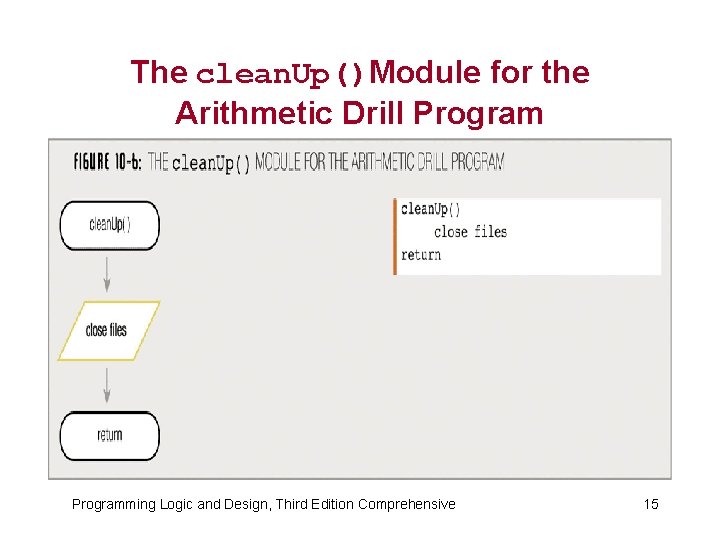
The clean. Up()Module for the Arithmetic Drill Programming Logic and Design, Third Edition Comprehensive 15
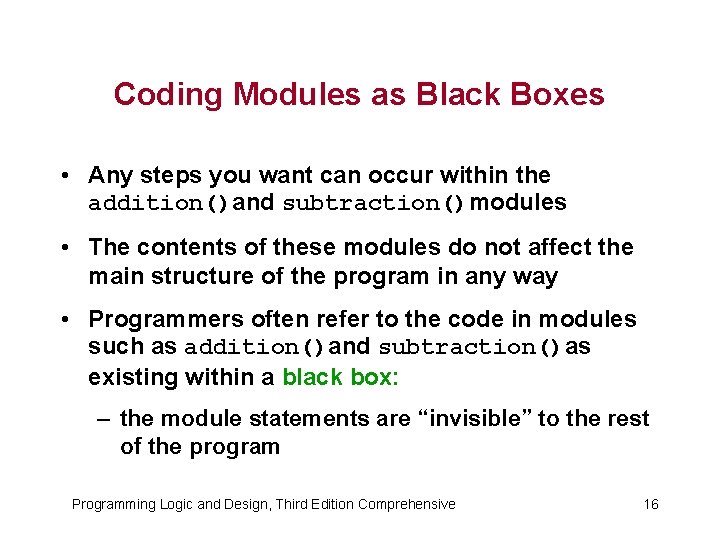
Coding Modules as Black Boxes • Any steps you want can occur within the addition()and subtraction()modules • The contents of these modules do not affect the main structure of the program in any way • Programmers often refer to the code in modules such as addition()and subtraction()as existing within a black box: – the module statements are “invisible” to the rest of the program Programming Logic and Design, Third Edition Comprehensive 16
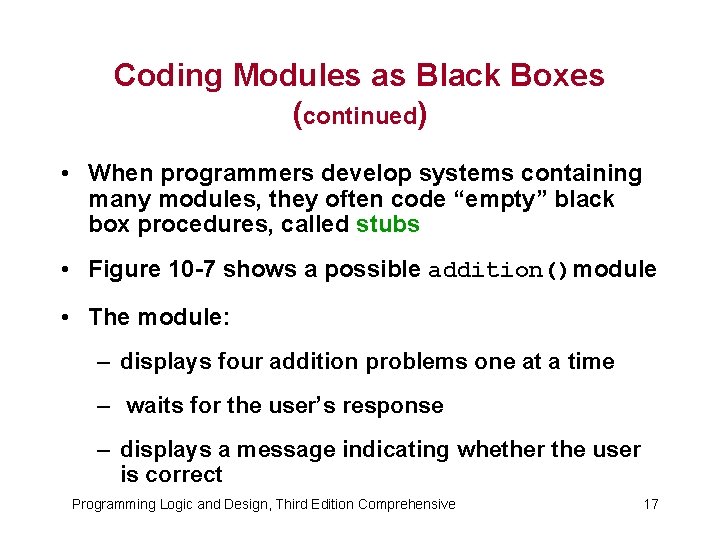
Coding Modules as Black Boxes (continued) • When programmers develop systems containing many modules, they often code “empty” black box procedures, called stubs • Figure 10 -7 shows a possible addition()module • The module: – displays four addition problems one at a time – waits for the user’s response – displays a message indicating whether the user is correct Programming Logic and Design, Third Edition Comprehensive 17
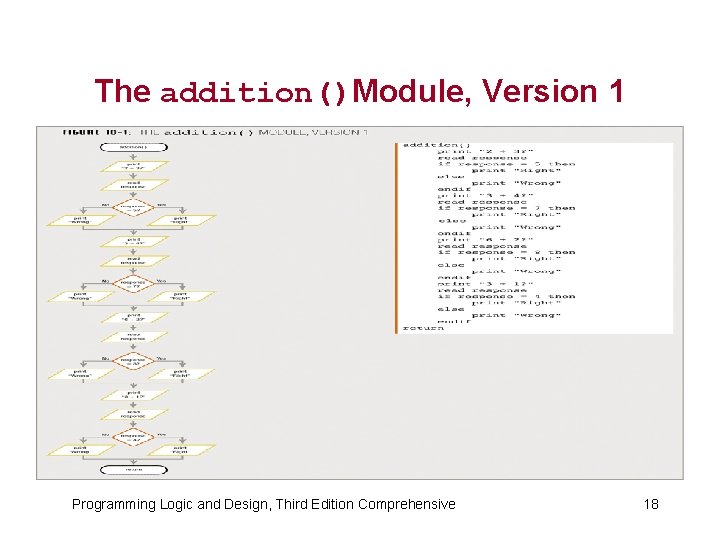
The addition()Module, Version 1 Programming Logic and Design, Third Edition Comprehensive 18
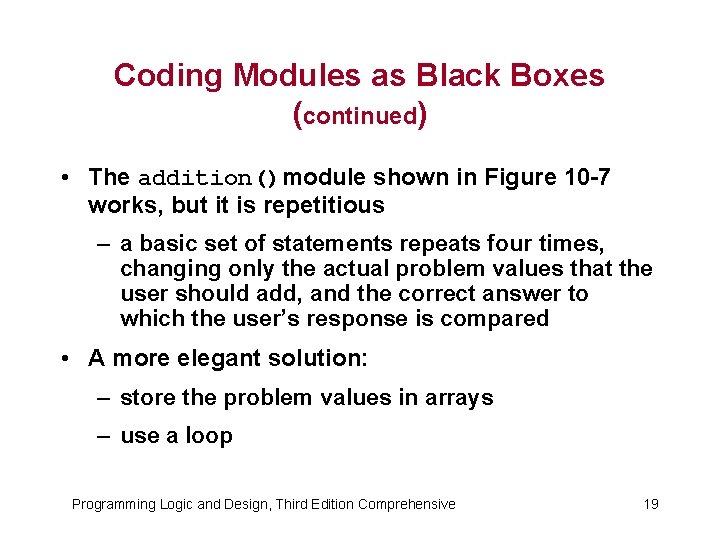
Coding Modules as Black Boxes (continued) • The addition()module shown in Figure 10 -7 works, but it is repetitious – a basic set of statements repeats four times, changing only the actual problem values that the user should add, and the correct answer to which the user’s response is compared • A more elegant solution: – store the problem values in arrays – use a loop Programming Logic and Design, Third Edition Comprehensive 19
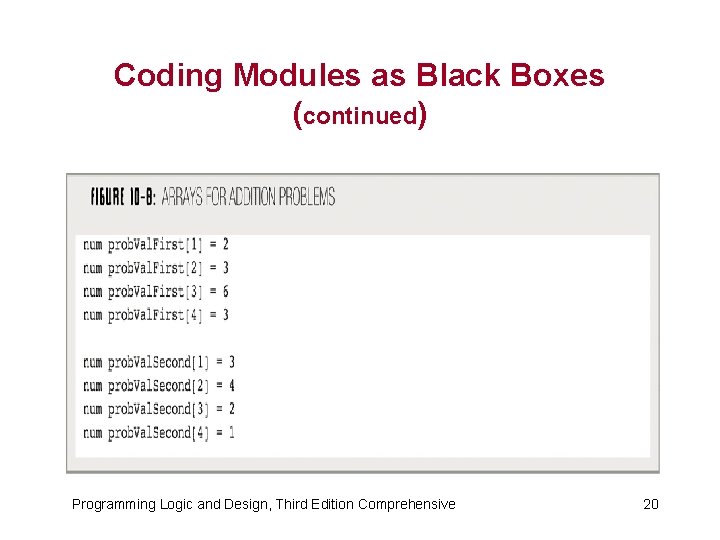
Coding Modules as Black Boxes (continued) Programming Logic and Design, Third Edition Comprehensive 20
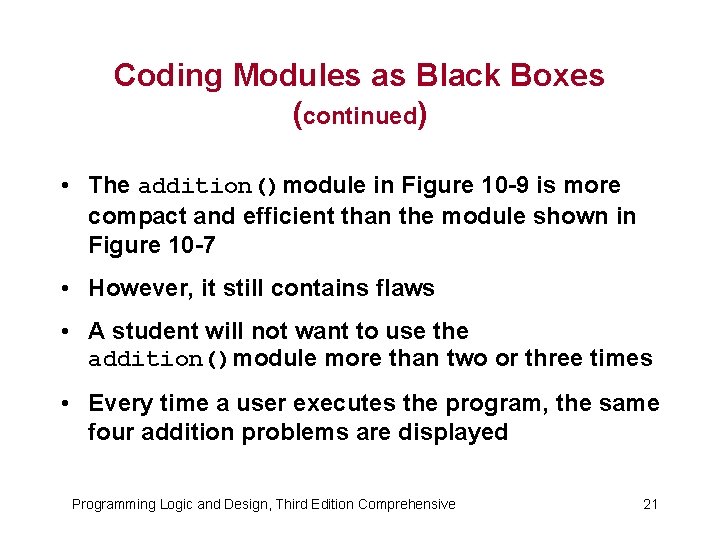
Coding Modules as Black Boxes (continued) • The addition()module in Figure 10 -9 is more compact and efficient than the module shown in Figure 10 -7 • However, it still contains flaws • A student will not want to use the addition()module more than two or three times • Every time a user executes the program, the same four addition problems are displayed Programming Logic and Design, Third Edition Comprehensive 21
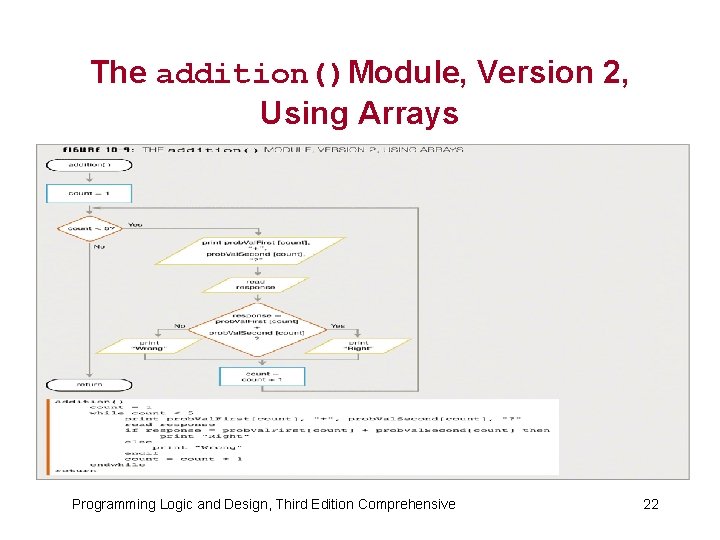
The addition()Module, Version 2, Using Arrays Programming Logic and Design, Third Edition Comprehensive 22
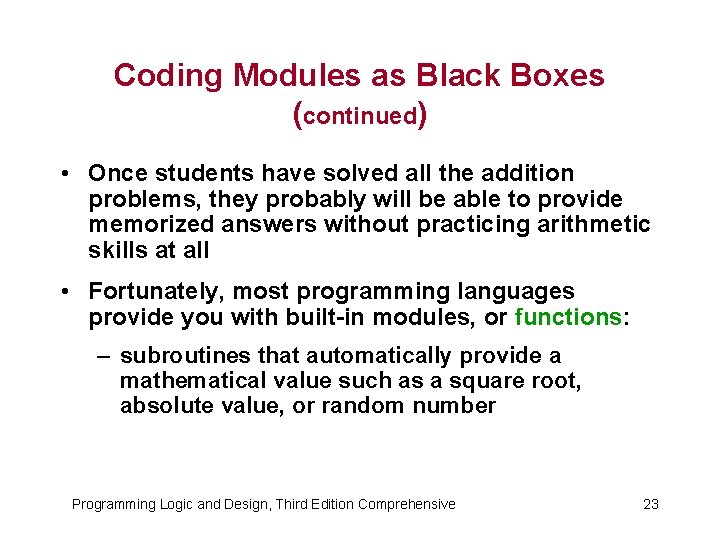
Coding Modules as Black Boxes (continued) • Once students have solved all the addition problems, they probably will be able to provide memorized answers without practicing arithmetic skills at all • Fortunately, most programming languages provide you with built-in modules, or functions: – subroutines that automatically provide a mathematical value such as a square root, absolute value, or random number Programming Logic and Design, Third Edition Comprehensive 23
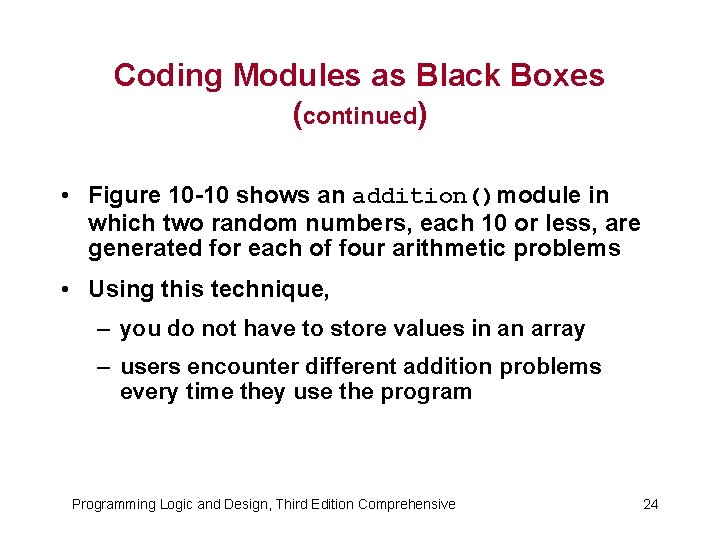
Coding Modules as Black Boxes (continued) • Figure 10 -10 shows an addition()module in which two random numbers, each 10 or less, are generated for each of four arithmetic problems • Using this technique, – you do not have to store values in an array – users encounter different addition problems every time they use the program Programming Logic and Design, Third Edition Comprehensive 24
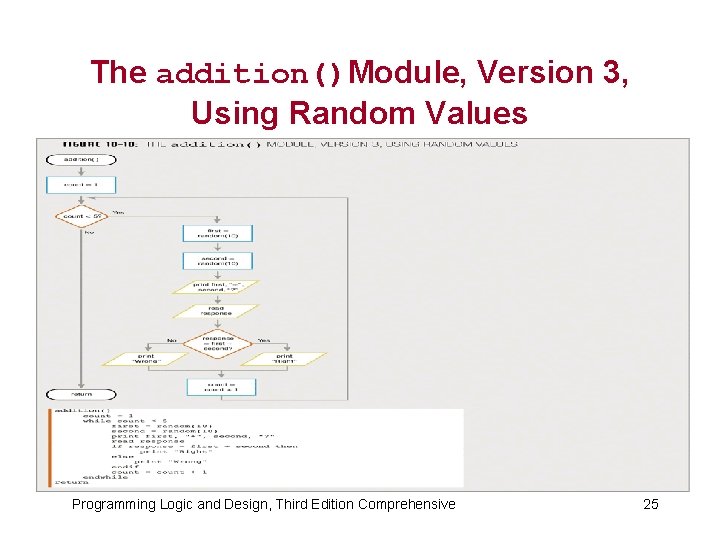
The addition()Module, Version 3, Using Random Values Programming Logic and Design, Third Edition Comprehensive 25
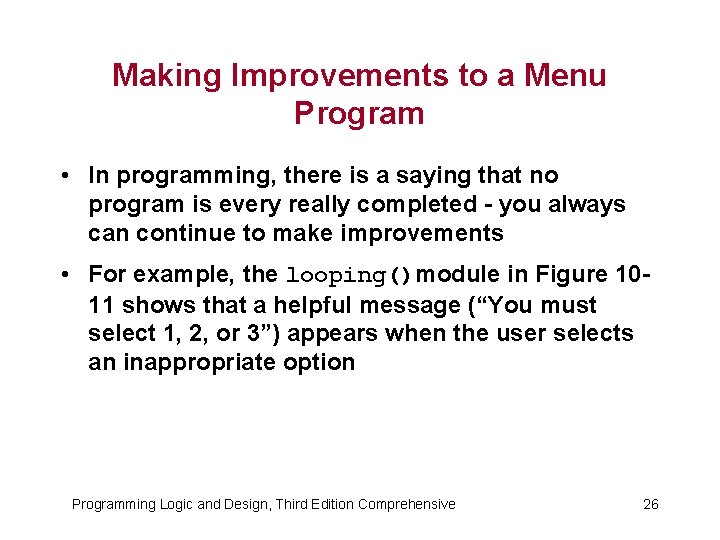
Making Improvements to a Menu Program • In programming, there is a saying that no program is every really completed - you always can continue to make improvements • For example, the looping()module in Figure 1011 shows that a helpful message (“You must select 1, 2, or 3”) appears when the user selects an inappropriate option Programming Logic and Design, Third Edition Comprehensive 26
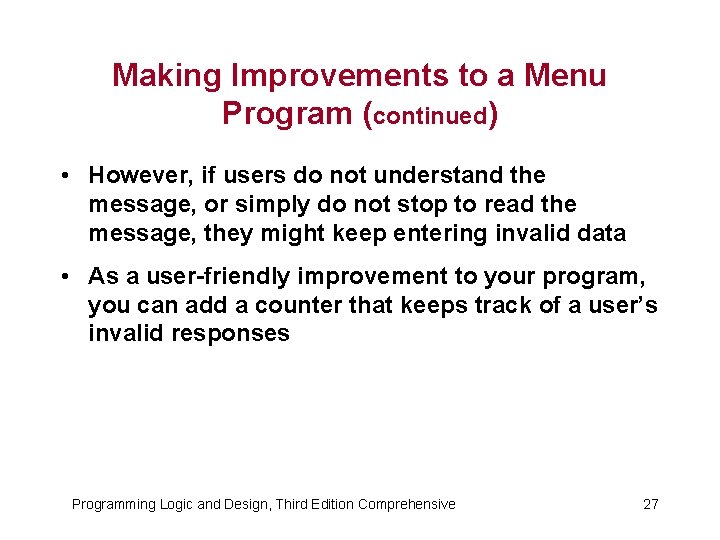
Making Improvements to a Menu Program (continued) • However, if users do not understand the message, or simply do not stop to read the message, they might keep entering invalid data • As a user-friendly improvement to your program, you can add a counter that keeps track of a user’s invalid responses Programming Logic and Design, Third Edition Comprehensive 27
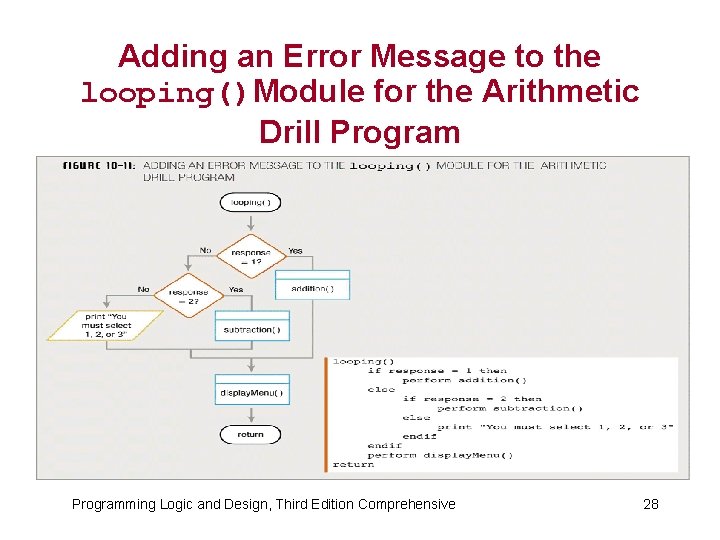
Adding an Error Message to the looping()Module for the Arithmetic Drill Programming Logic and Design, Third Edition Comprehensive 28
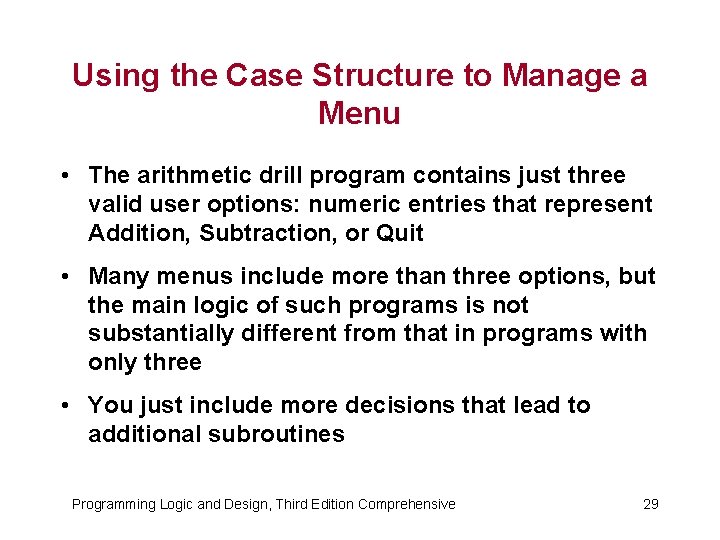
Using the Case Structure to Manage a Menu • The arithmetic drill program contains just three valid user options: numeric entries that represent Addition, Subtraction, or Quit • Many menus include more than three options, but the main logic of such programs is not substantially different from that in programs with only three • You just include more decisions that lead to additional subroutines Programming Logic and Design, Third Edition Comprehensive 29
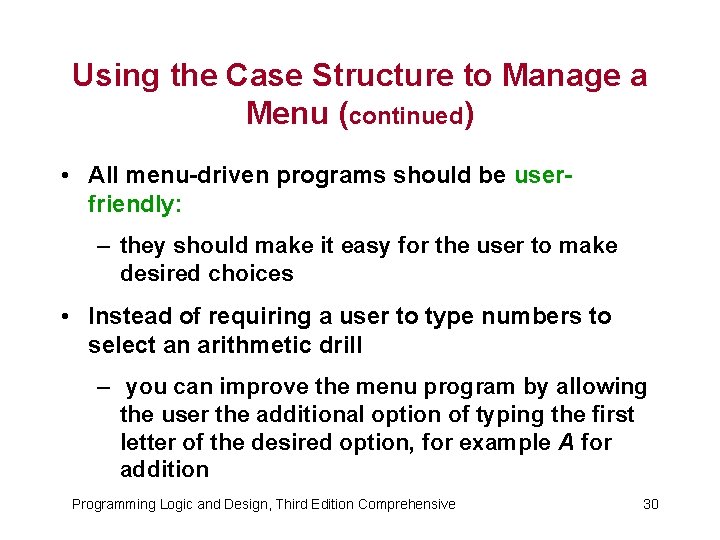
Using the Case Structure to Manage a Menu (continued) • All menu-driven programs should be userfriendly: – they should make it easy for the user to make desired choices • Instead of requiring a user to type numbers to select an arithmetic drill – you can improve the menu program by allowing the user the additional option of typing the first letter of the desired option, for example A for addition Programming Logic and Design, Third Edition Comprehensive 30
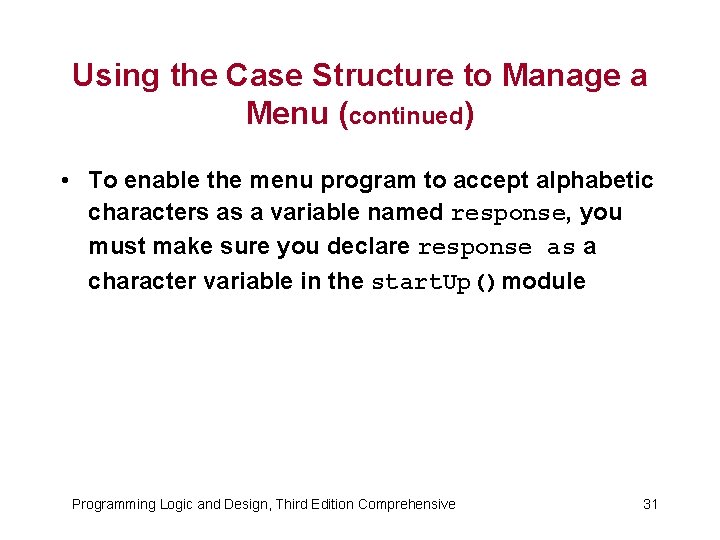
Using the Case Structure to Manage a Menu (continued) • To enable the menu program to accept alphabetic characters as a variable named response, you must make sure you declare response as a character variable in the start. Up()module Programming Logic and Design, Third Edition Comprehensive 31
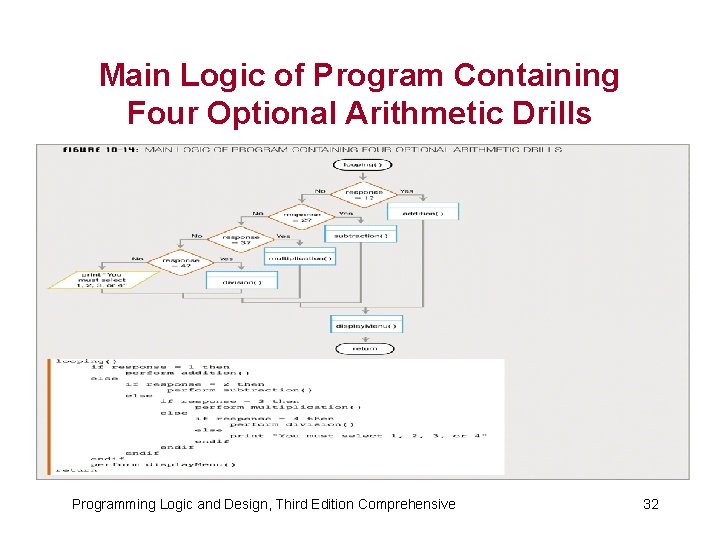
Main Logic of Program Containing Four Optional Arithmetic Drills Programming Logic and Design, Third Edition Comprehensive 32
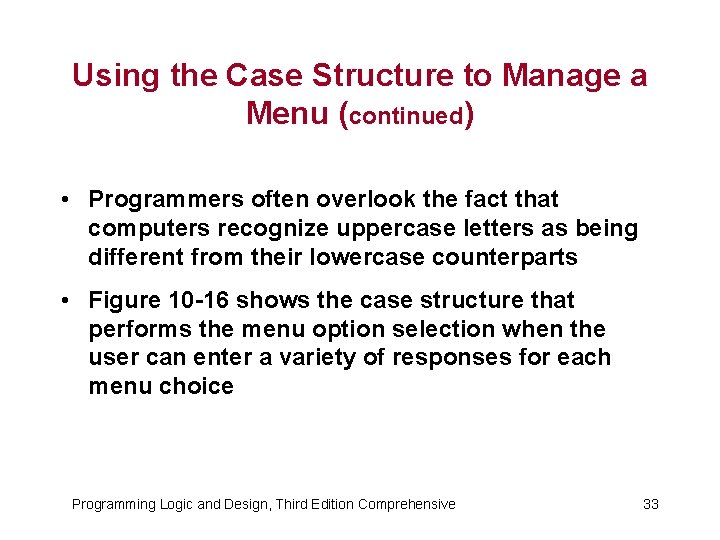
Using the Case Structure to Manage a Menu (continued) • Programmers often overlook the fact that computers recognize uppercase letters as being different from their lowercase counterparts • Figure 10 -16 shows the case structure that performs the menu option selection when the user can enter a variety of responses for each menu choice Programming Logic and Design, Third Edition Comprehensive 33
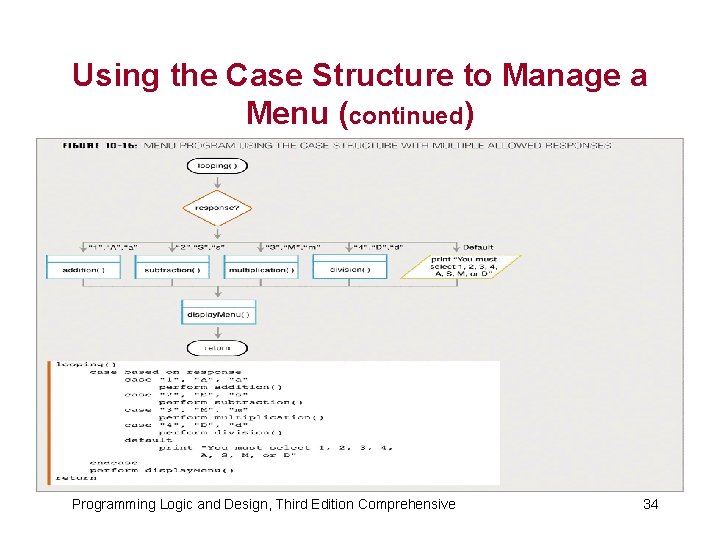
Using the Case Structure to Manage a Menu (continued) Programming Logic and Design, Third Edition Comprehensive 34
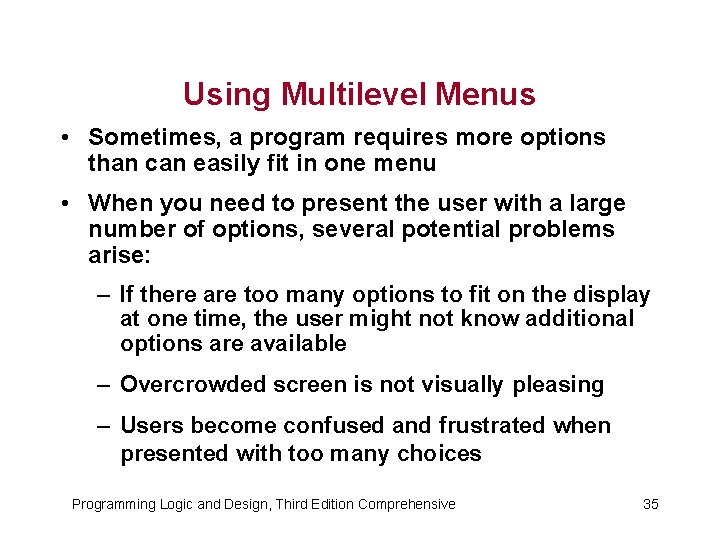
Using Multilevel Menus • Sometimes, a program requires more options than can easily fit in one menu • When you need to present the user with a large number of options, several potential problems arise: – If there are too many options to fit on the display at one time, the user might not know additional options are available – Overcrowded screen is not visually pleasing – Users become confused and frustrated when presented with too many choices Programming Logic and Design, Third Edition Comprehensive 35
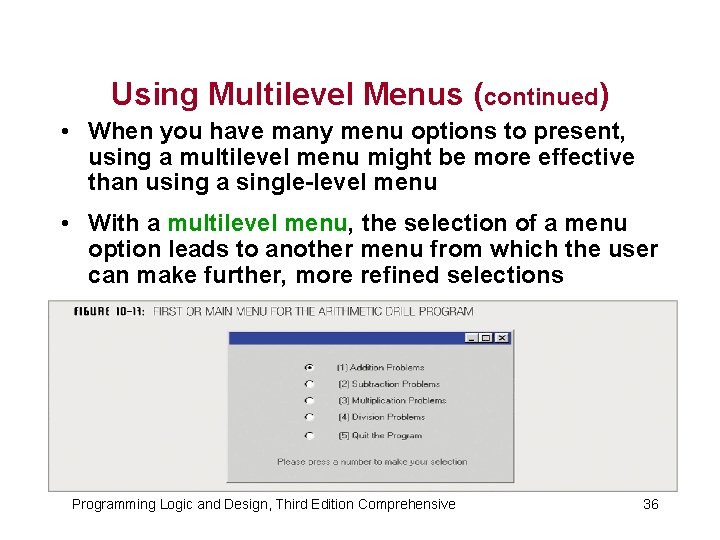
Using Multilevel Menus (continued) • When you have many menu options to present, using a multilevel menu might be more effective than using a single-level menu • With a multilevel menu, the selection of a menu option leads to another menu from which the user can make further, more refined selections Programming Logic and Design, Third Edition Comprehensive 36
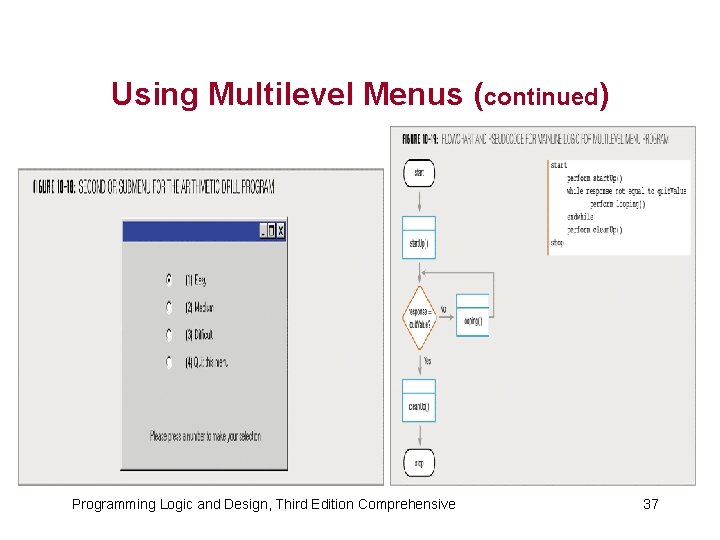
Using Multilevel Menus (continued) Programming Logic and Design, Third Edition Comprehensive 37
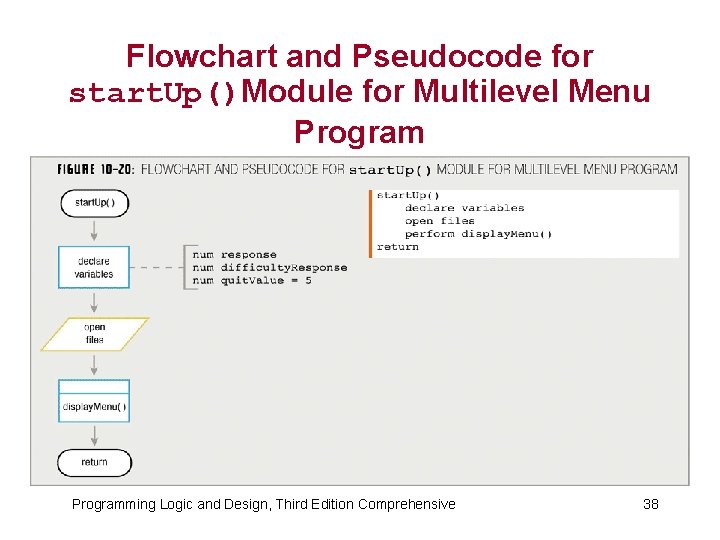
Flowchart and Pseudocode for start. Up()Module for Multilevel Menu Programming Logic and Design, Third Edition Comprehensive 38
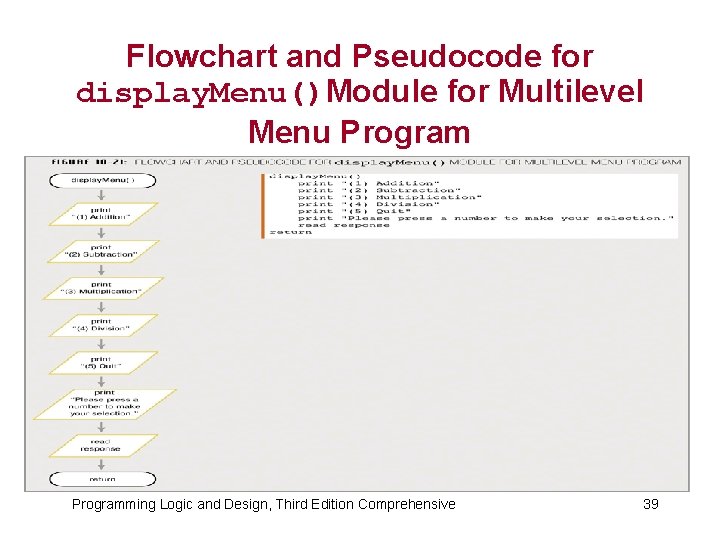
Flowchart and Pseudocode for display. Menu()Module for Multilevel Menu Programming Logic and Design, Third Edition Comprehensive 39
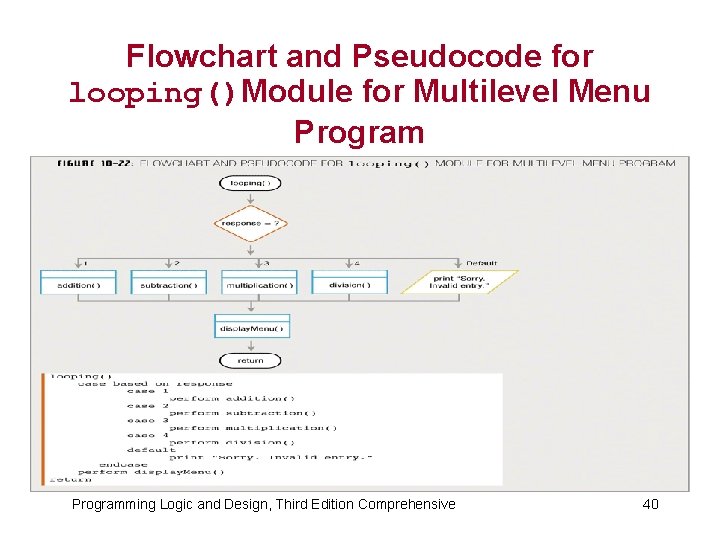
Flowchart and Pseudocode for looping()Module for Multilevel Menu Programming Logic and Design, Third Edition Comprehensive 40
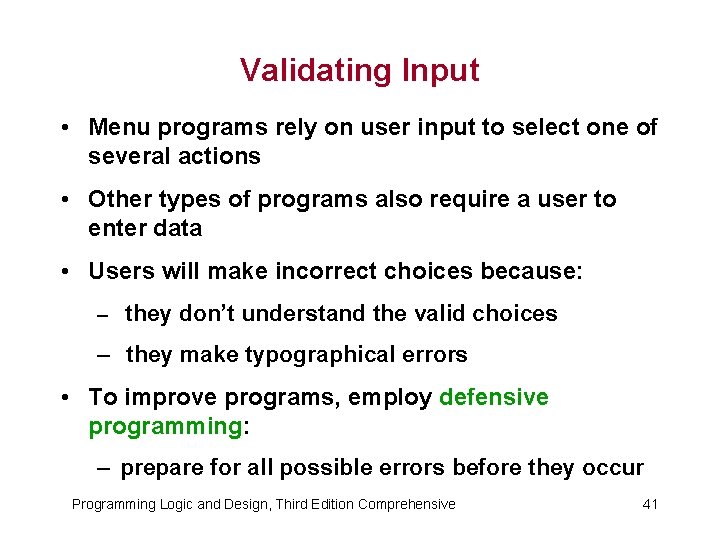
Validating Input • Menu programs rely on user input to select one of several actions • Other types of programs also require a user to enter data • Users will make incorrect choices because: – they don’t understand the valid choices – they make typographical errors • To improve programs, employ defensive programming: – prepare for all possible errors before they occur Programming Logic and Design, Third Edition Comprehensive 41
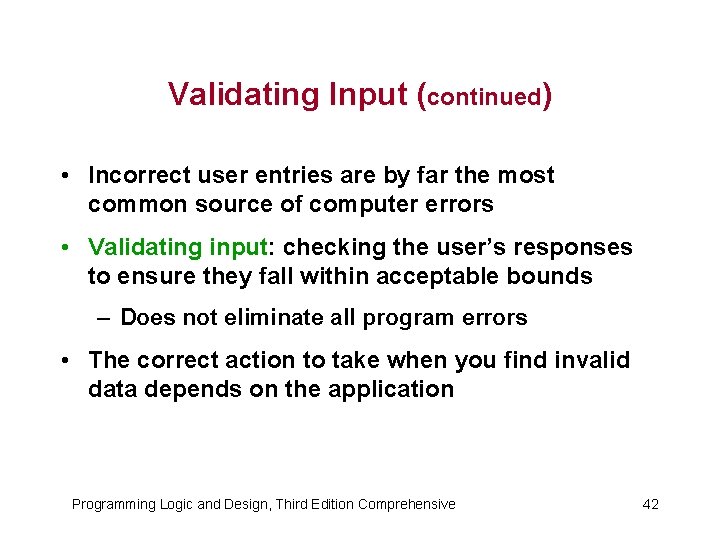
Validating Input (continued) • Incorrect user entries are by far the most common source of computer errors • Validating input: checking the user’s responses to ensure they fall within acceptable bounds – Does not eliminate all program errors • The correct action to take when you find invalid data depends on the application Programming Logic and Design, Third Edition Comprehensive 42
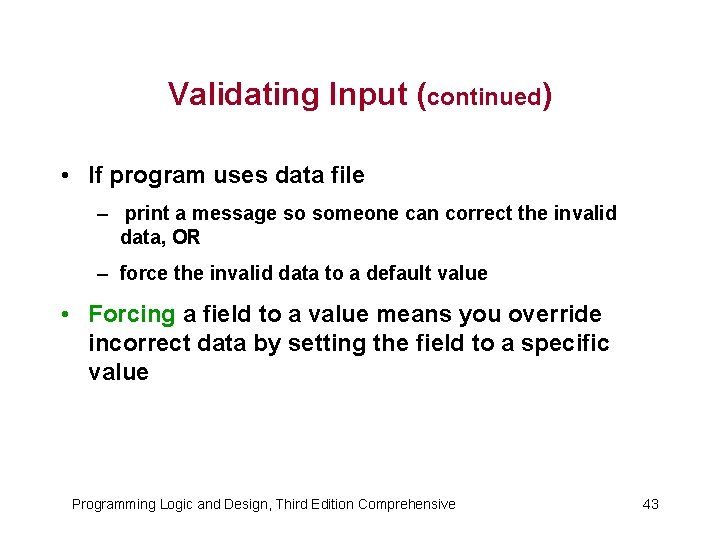
Validating Input (continued) • If program uses data file – print a message so someone can correct the invalid data, OR – force the invalid data to a default value • Forcing a field to a value means you override incorrect data by setting the field to a specific value Programming Logic and Design, Third Edition Comprehensive 43
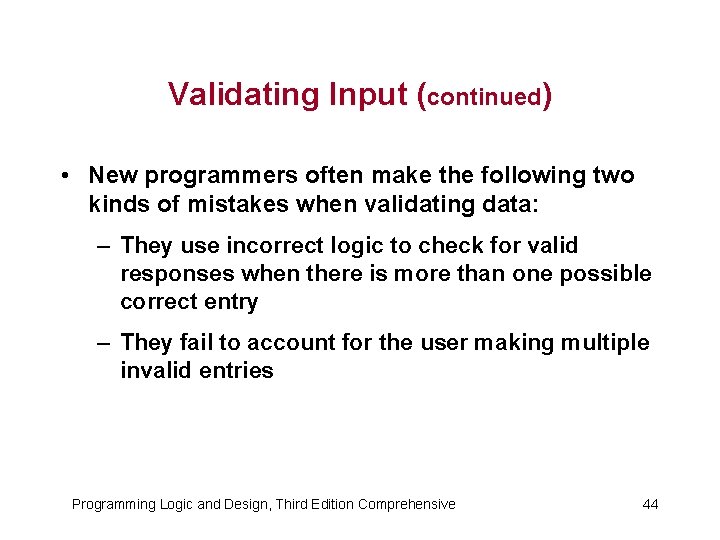
Validating Input (continued) • New programmers often make the following two kinds of mistakes when validating data: – They use incorrect logic to check for valid responses when there is more than one possible correct entry – They fail to account for the user making multiple invalid entries Programming Logic and Design, Third Edition Comprehensive 44
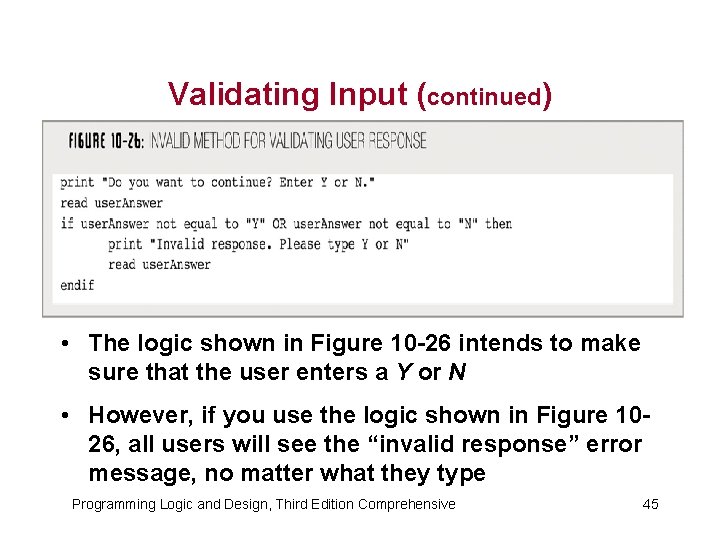
Validating Input (continued) • The logic shown in Figure 10 -26 intends to make sure that the user enters a Y or N • However, if you use the logic shown in Figure 1026, all users will see the “invalid response” error message, no matter what they type Programming Logic and Design, Third Edition Comprehensive 45
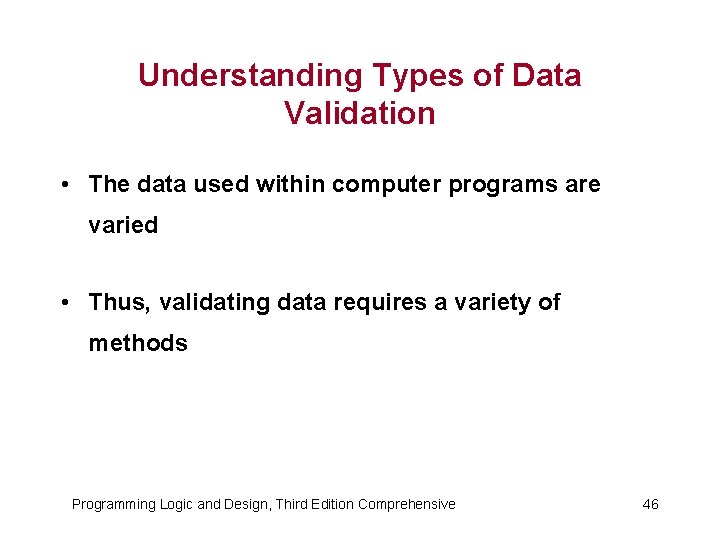
Understanding Types of Data Validation • The data used within computer programs are varied • Thus, validating data requires a variety of methods Programming Logic and Design, Third Edition Comprehensive 46
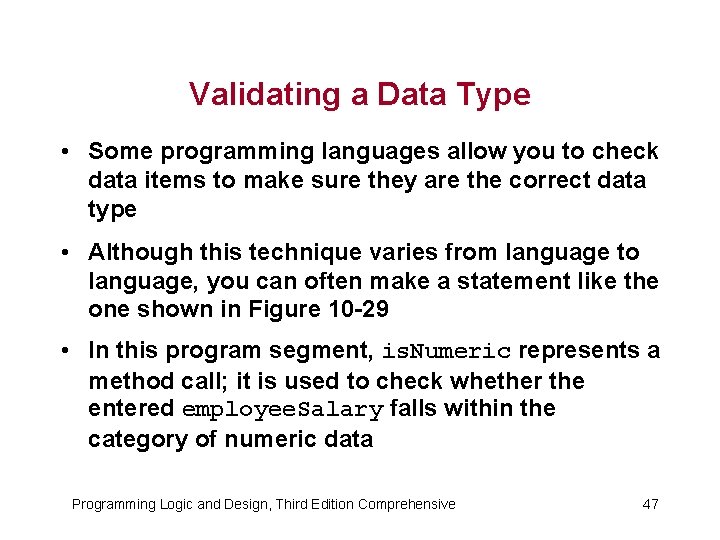
Validating a Data Type • Some programming languages allow you to check data items to make sure they are the correct data type • Although this technique varies from language to language, you can often make a statement like the one shown in Figure 10 -29 • In this program segment, is. Numeric represents a method call; it is used to check whether the entered employee. Salary falls within the category of numeric data Programming Logic and Design, Third Edition Comprehensive 47
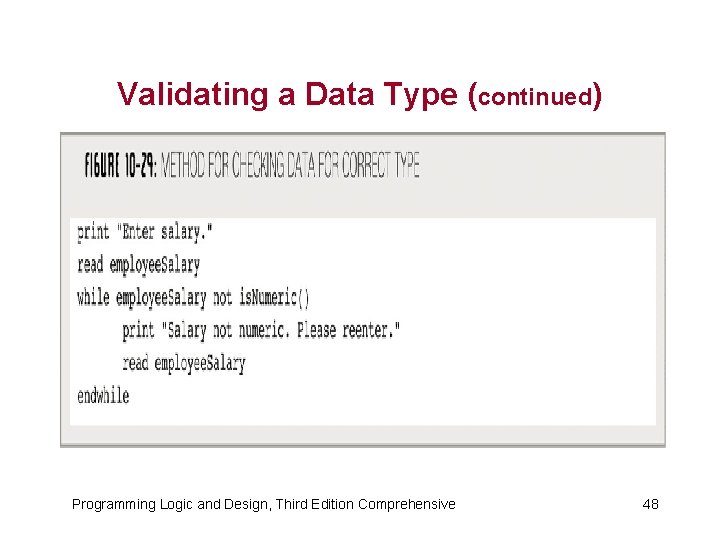
Validating a Data Type (continued) Programming Logic and Design, Third Edition Comprehensive 48
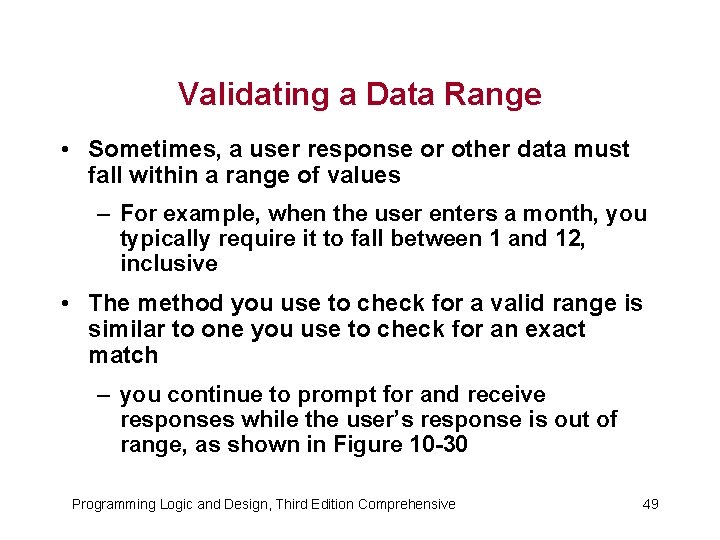
Validating a Data Range • Sometimes, a user response or other data must fall within a range of values – For example, when the user enters a month, you typically require it to fall between 1 and 12, inclusive • The method you use to check for a valid range is similar to one you use to check for an exact match – you continue to prompt for and receive responses while the user’s response is out of range, as shown in Figure 10 -30 Programming Logic and Design, Third Edition Comprehensive 49
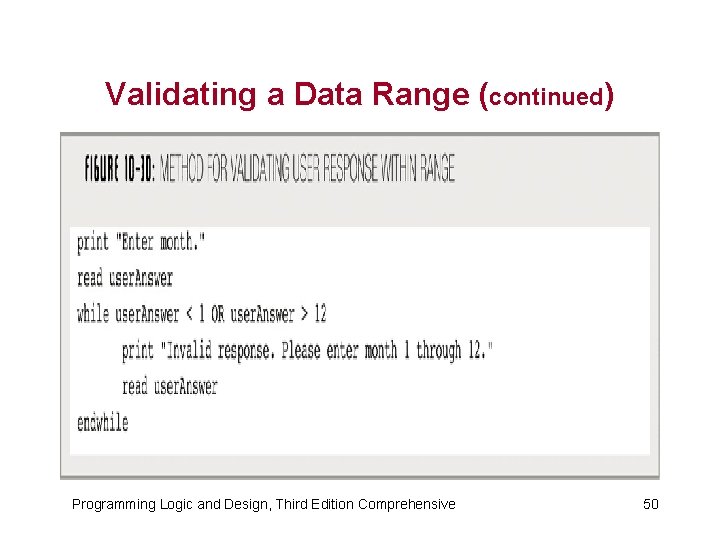
Validating a Data Range (continued) Programming Logic and Design, Third Edition Comprehensive 50
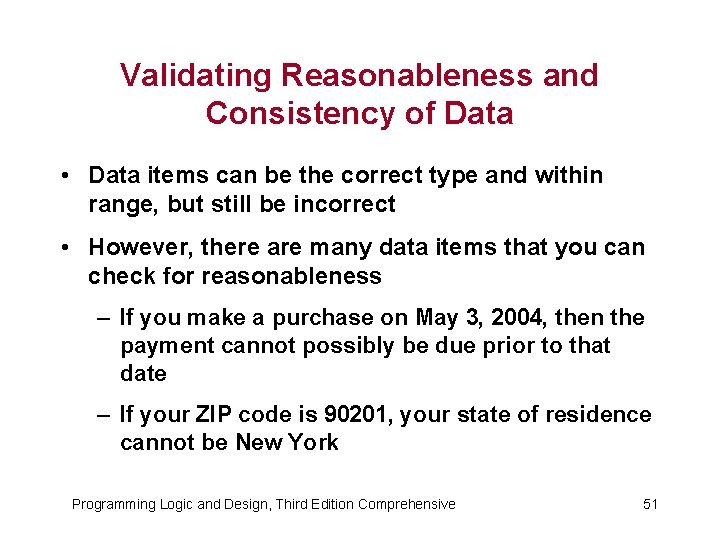
Validating Reasonableness and Consistency of Data • Data items can be the correct type and within range, but still be incorrect • However, there are many data items that you can check for reasonableness – If you make a purchase on May 3, 2004, then the payment cannot possibly be due prior to that date – If your ZIP code is 90201, your state of residence cannot be New York Programming Logic and Design, Third Edition Comprehensive 51
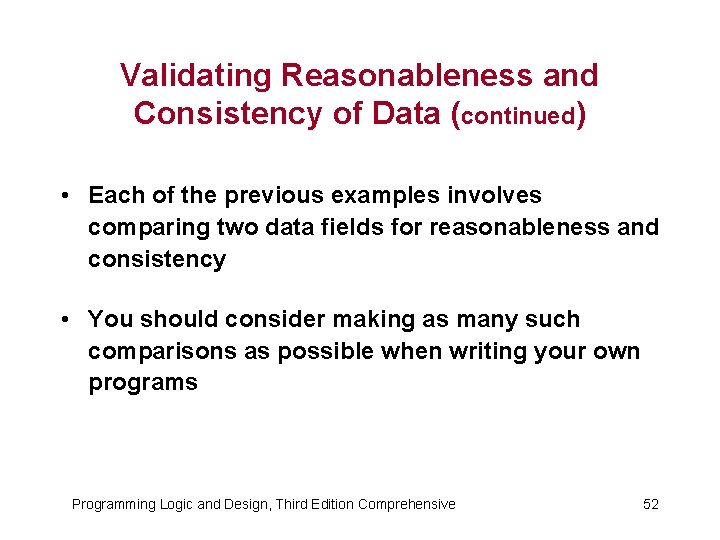
Validating Reasonableness and Consistency of Data (continued) • Each of the previous examples involves comparing two data fields for reasonableness and consistency • You should consider making as many such comparisons as possible when writing your own programs Programming Logic and Design, Third Edition Comprehensive 52
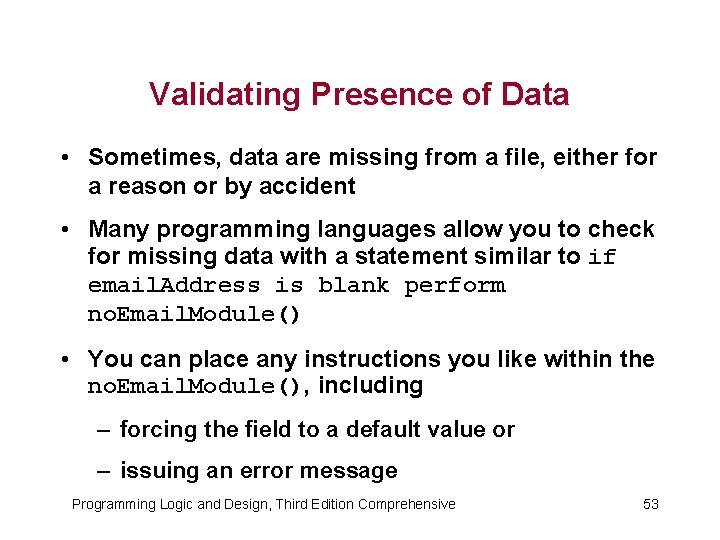
Validating Presence of Data • Sometimes, data are missing from a file, either for a reason or by accident • Many programming languages allow you to check for missing data with a statement similar to if email. Address is blank perform no. Email. Module() • You can place any instructions you like within the no. Email. Module(), including – forcing the field to a default value or – issuing an error message Programming Logic and Design, Third Edition Comprehensive 53
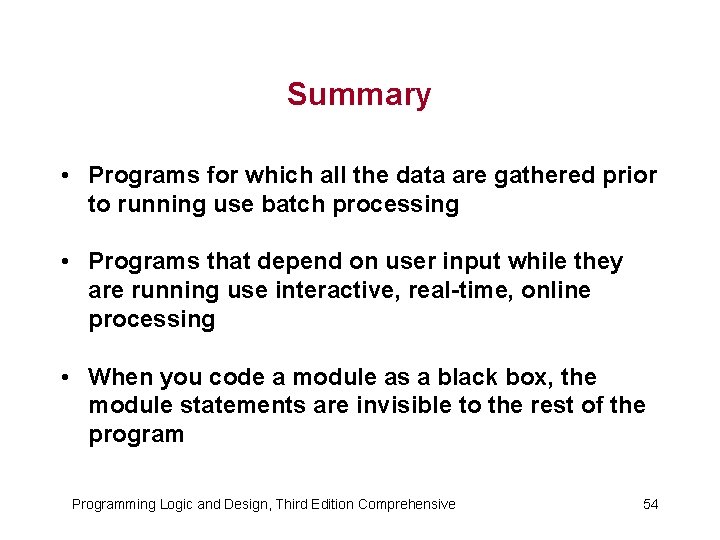
Summary • Programs for which all the data are gathered prior to running use batch processing • Programs that depend on user input while they are running use interactive, real-time, online processing • When you code a module as a black box, the module statements are invisible to the rest of the program Programming Logic and Design, Third Edition Comprehensive 54
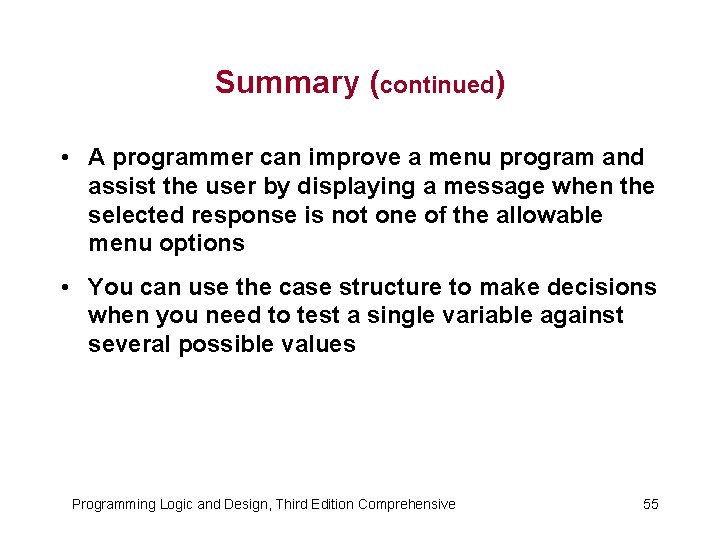
Summary (continued) • A programmer can improve a menu program and assist the user by displaying a message when the selected response is not one of the allowable menu options • You can use the case structure to make decisions when you need to test a single variable against several possible values Programming Logic and Design, Third Edition Comprehensive 55
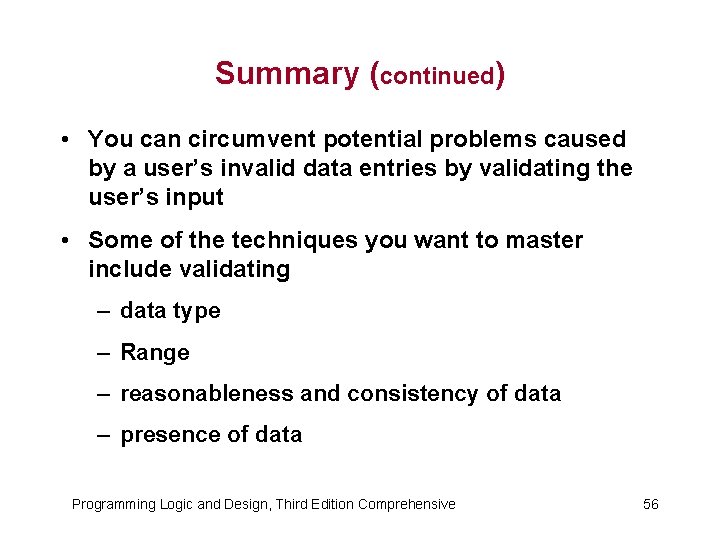
Summary (continued) • You can circumvent potential problems caused by a user’s invalid data entries by validating the user’s input • Some of the techniques you want to master include validating – data type – Range – reasonableness and consistency of data – presence of data Programming Logic and Design, Third Edition Comprehensive 56
Validating hypothesis
Menus recipes and cost management
Palettes and tear-off menus
Palettes and tear-off menus
Little windows of actions
Finely tuned input and roughly tuned input
A cyclical menu
Event trapping menus examples
Purpose of menu planning
Just lounge
Different types of menu
Ugr-m menus
Wjec hospitality and catering unit 2 examples
Cacfp sample menu
2 types of menu
Linear sequences dan multiple menus
What are the four types of menus
5 days of sample menus for pregnant women
Yang termasuk periferal input adalah
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
Windows 10 system programming, part 1
Linear vs integer programming
Perbedaan linear programming dan integer programming
Dynamic pass transistor
Ganged cmos
Assume that a firm produces output using one fixed input
Interrupt vs polling in microcontroller
Binomial coefficient using dynamic programming
Solving goal programming problems using simplex method
Apprenticeship learning using linear programming
System.collections.generics
Defrost using internal heat is accomplished using
Chapter 6 input and output
Barcode reader is input or output device
Chapter 2 input processing and output
Computer programming chapter 1
History of python
Chapter 1 introduction to computers and programming
C programming chapter 1
Chapter 15 using management and accounting information
Chapter 15 input output java
Python chapter 5
The zj row in a simplex table for maximization represents
Chapter 16 programming language
Chapter 16 programming language
Qm for windows
Computer programming chapter 1
Chapter 8 linear programming applications solutions
Chapter 2 elementary programming
Chapter 16 programming language
Form control in system analysis and design
Automatic input
Computer graphics
Chapter 11 section 4 using water wisely answer key
Chapter 11 section 4 using water wisely answer key
Chapter 23 using recipes student activity workbook answers