An Introduction to the Cg Shading Language Marco
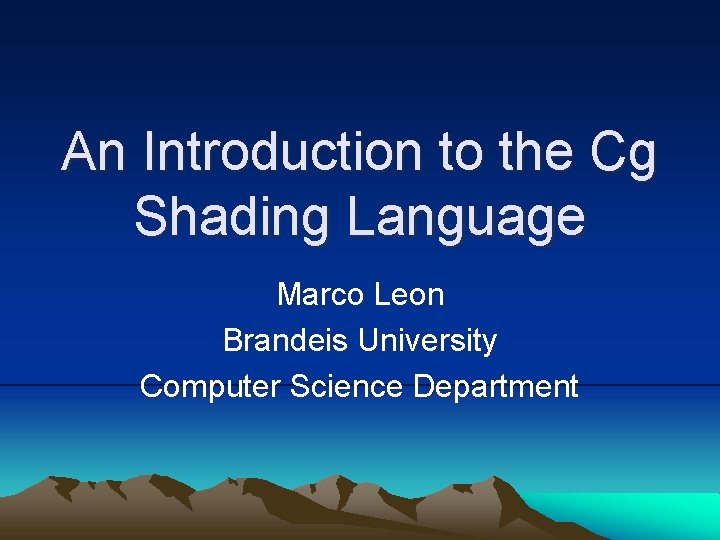
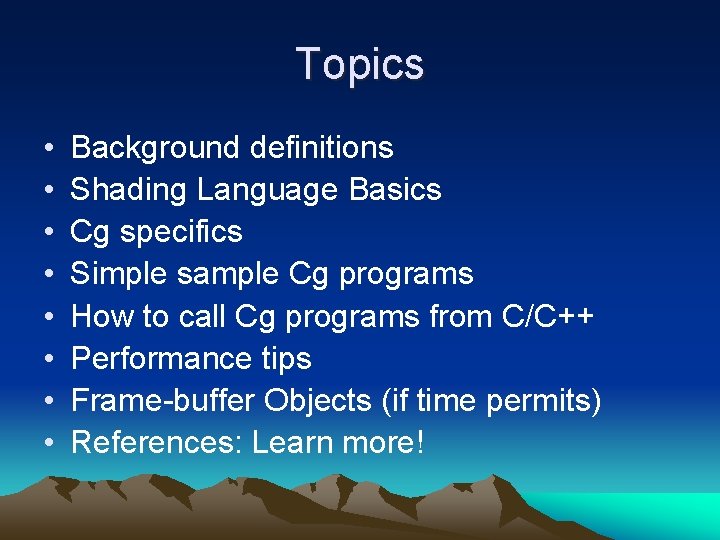
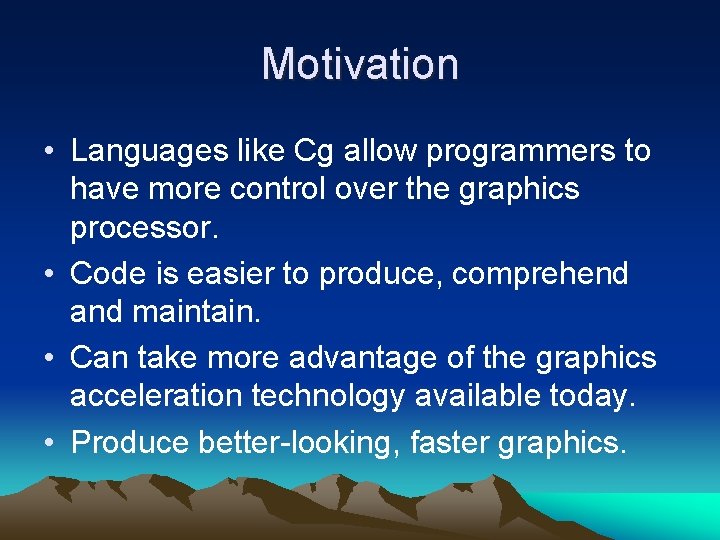
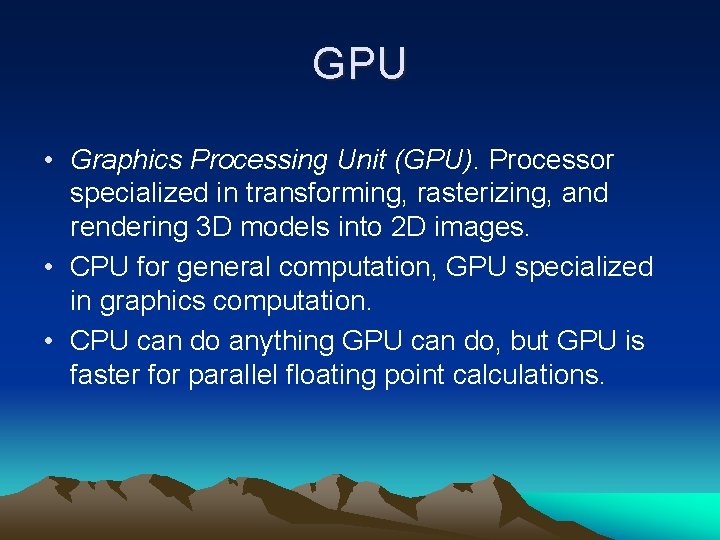
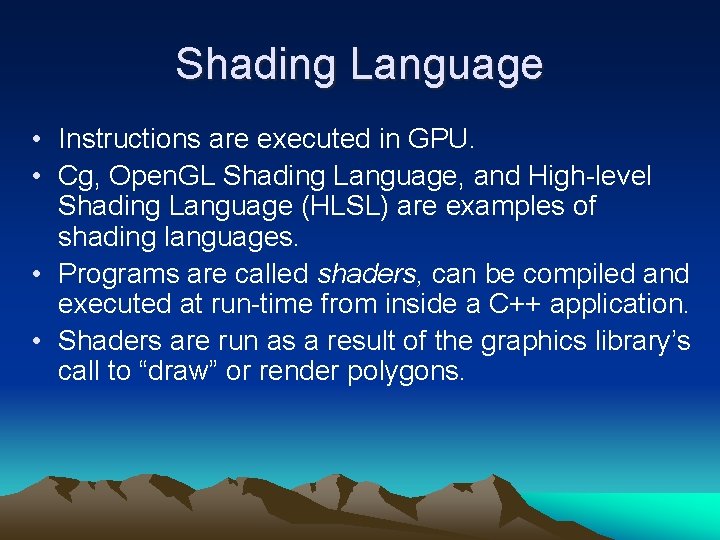
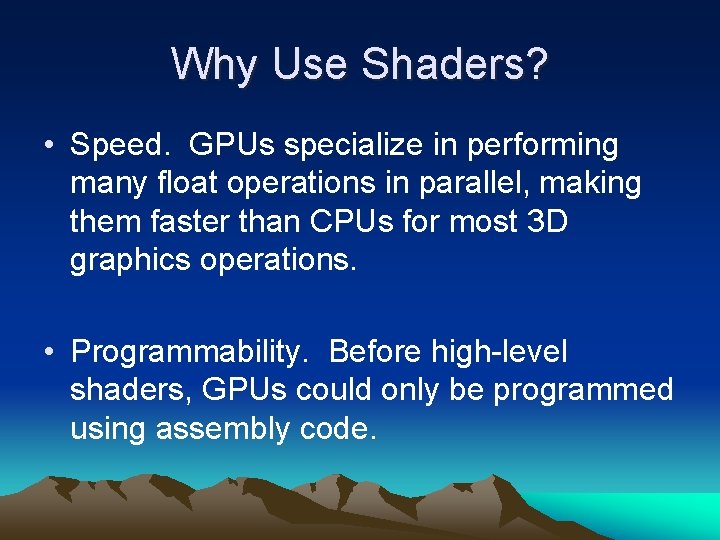
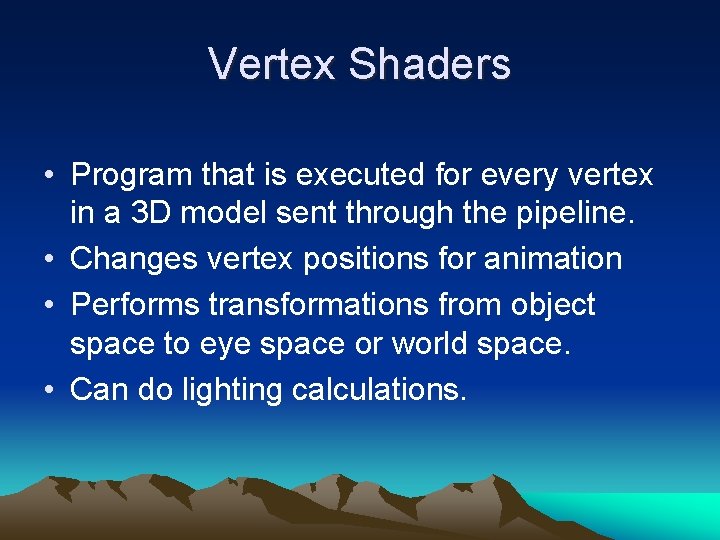
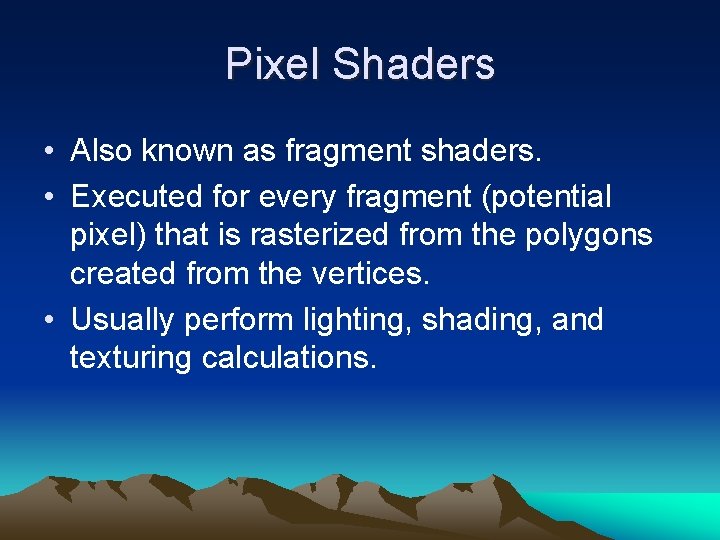
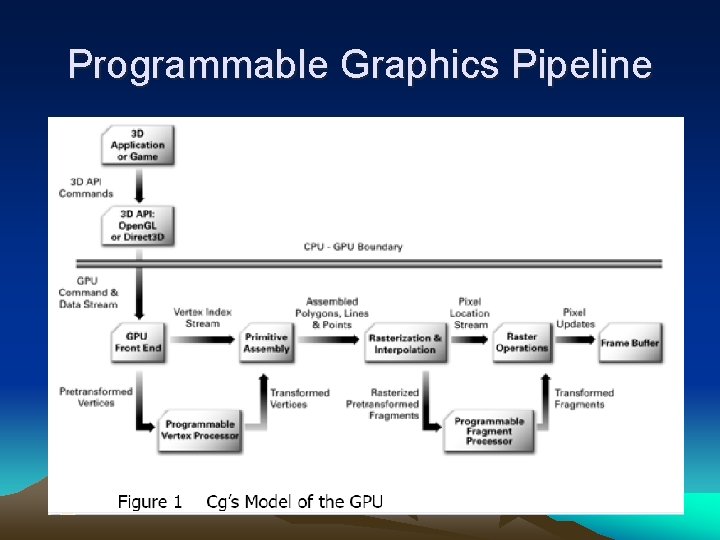
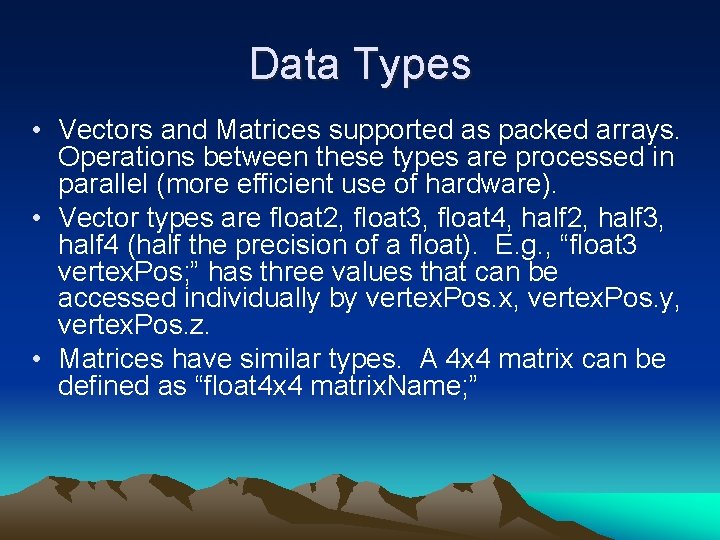
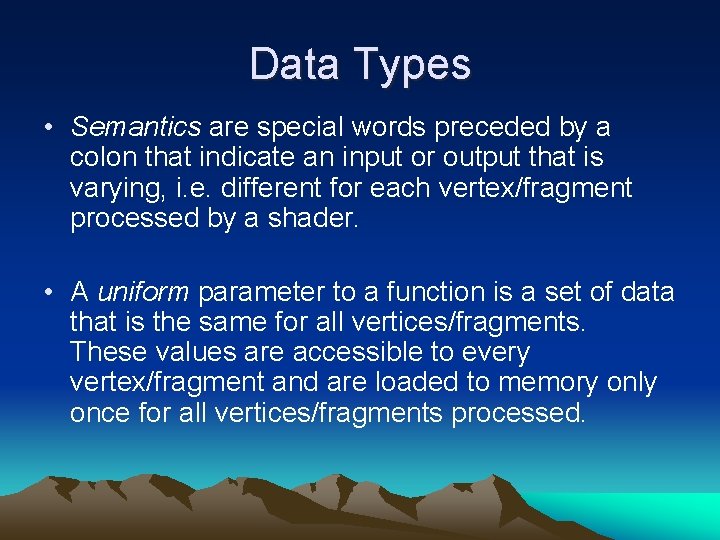
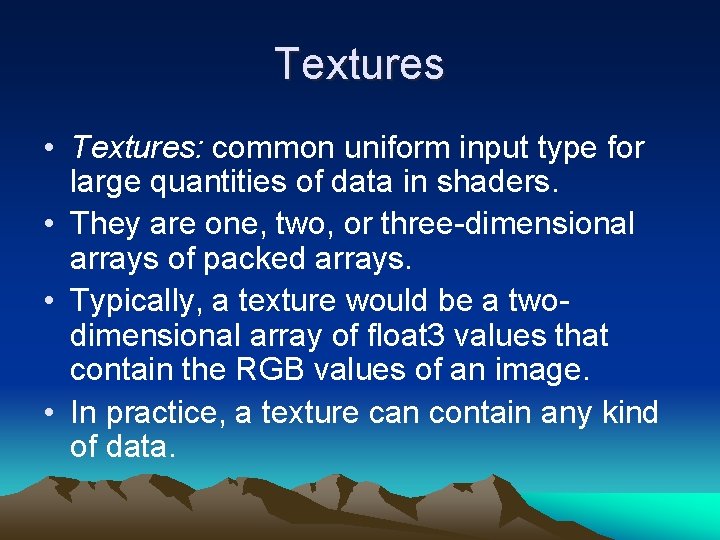
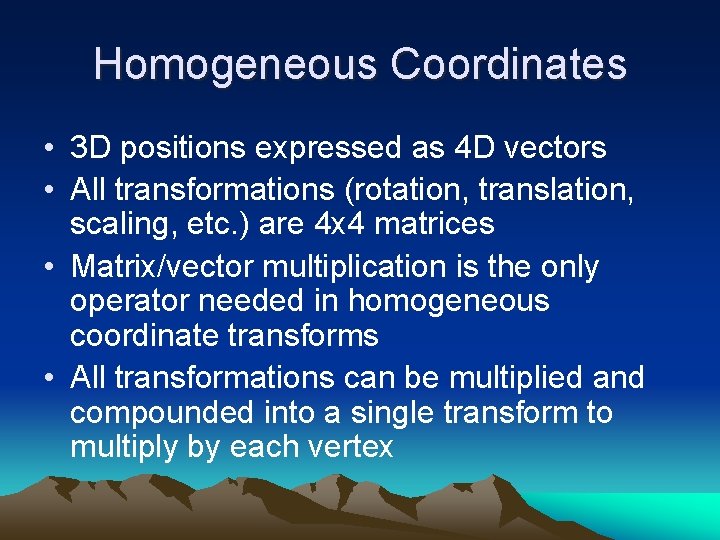
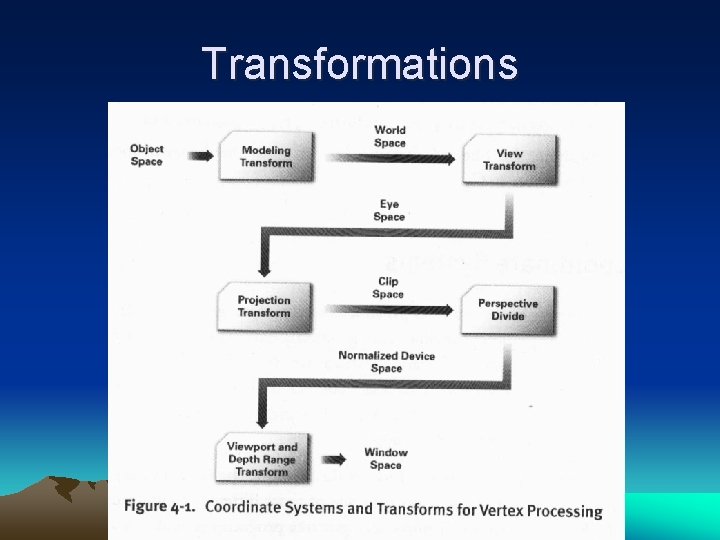
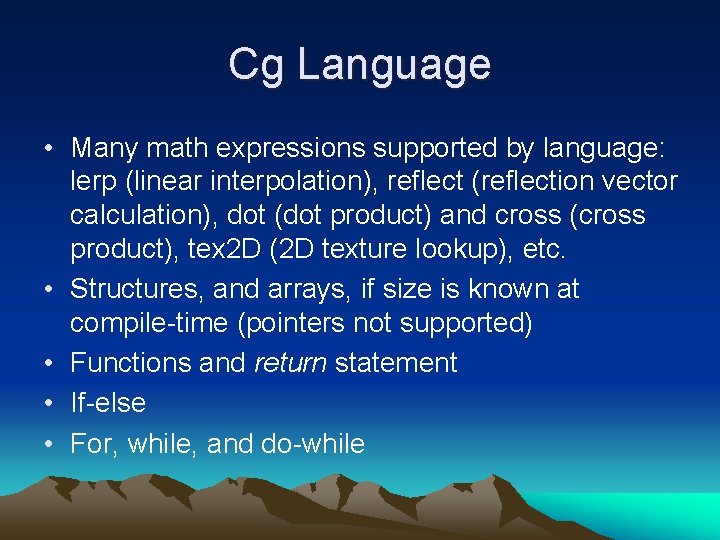
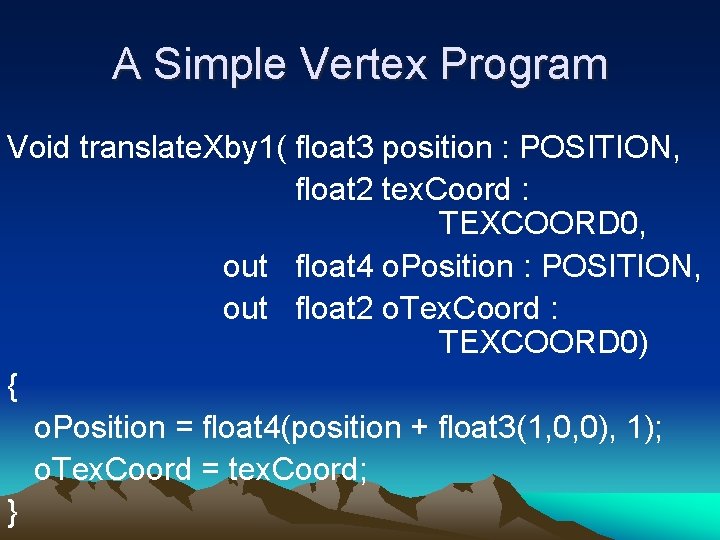
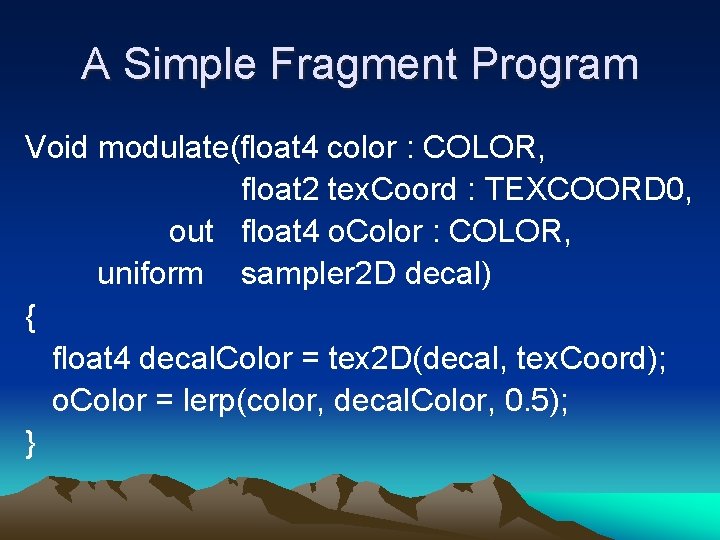
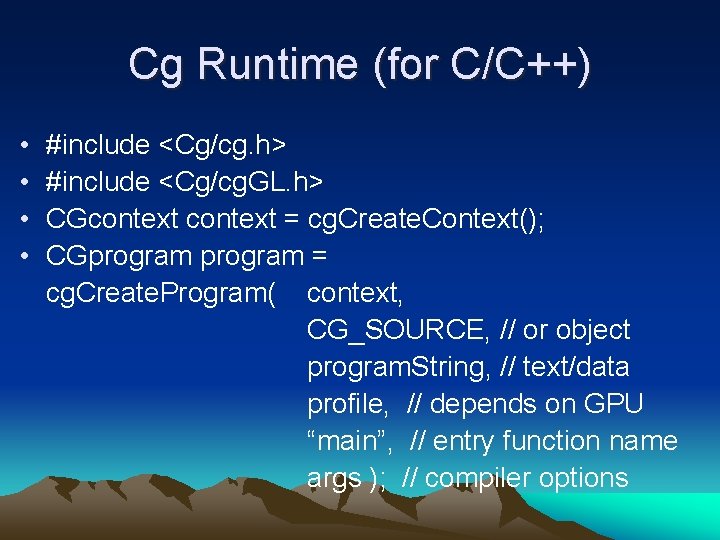
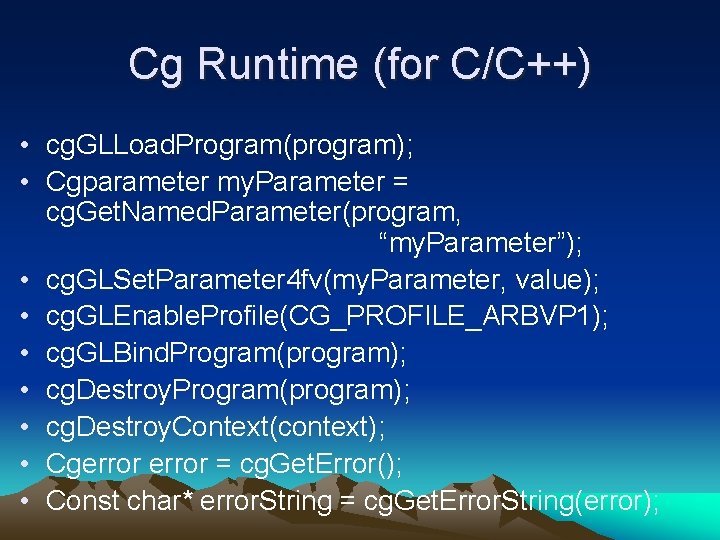
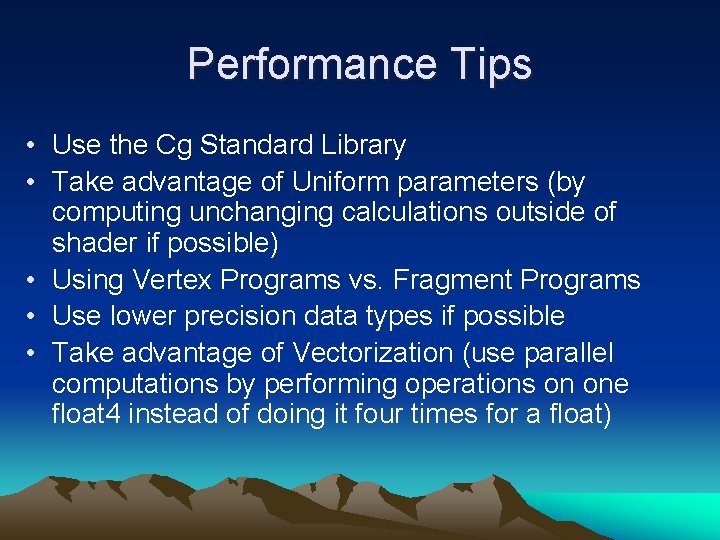
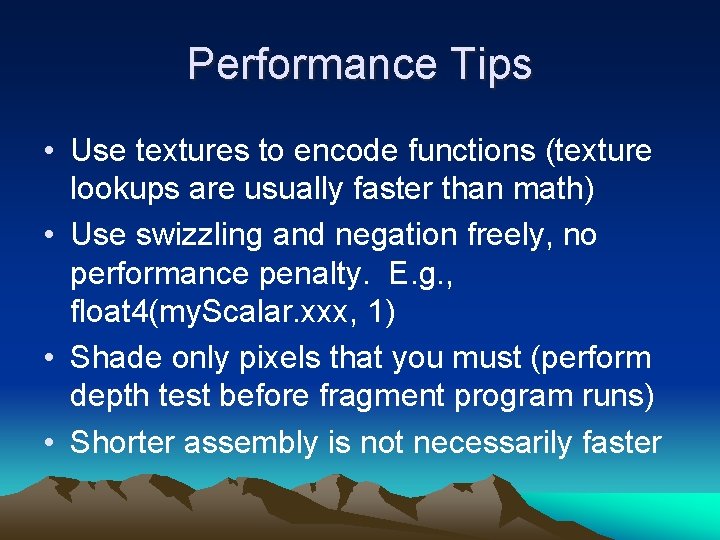
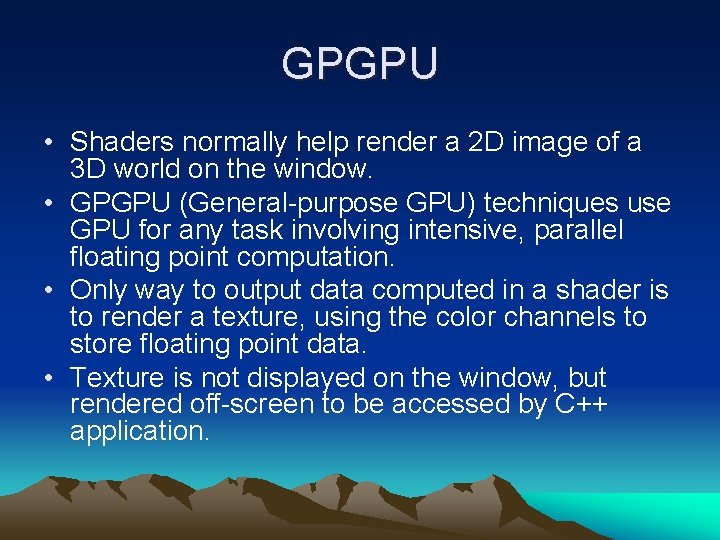
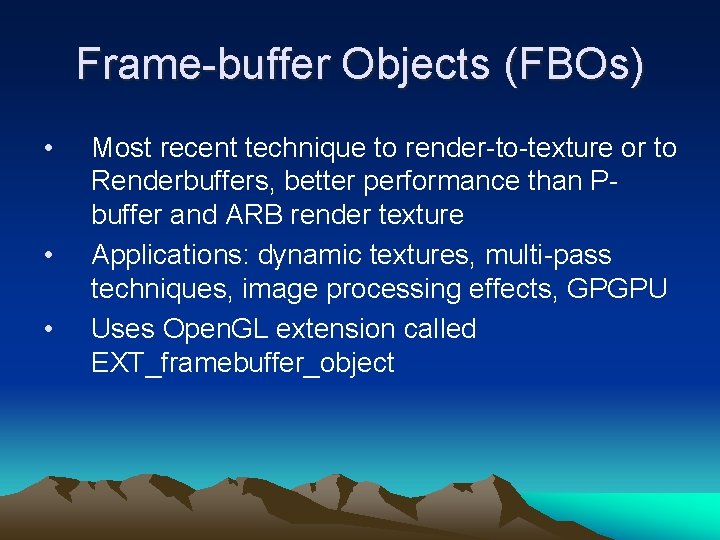
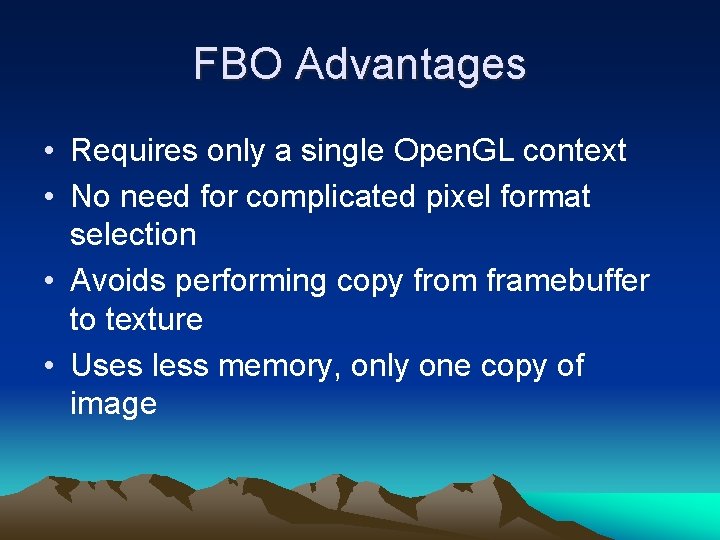
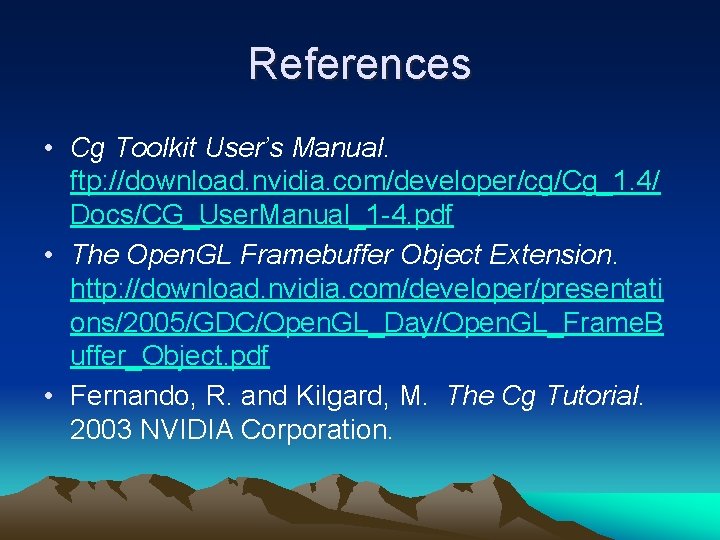
- Slides: 25
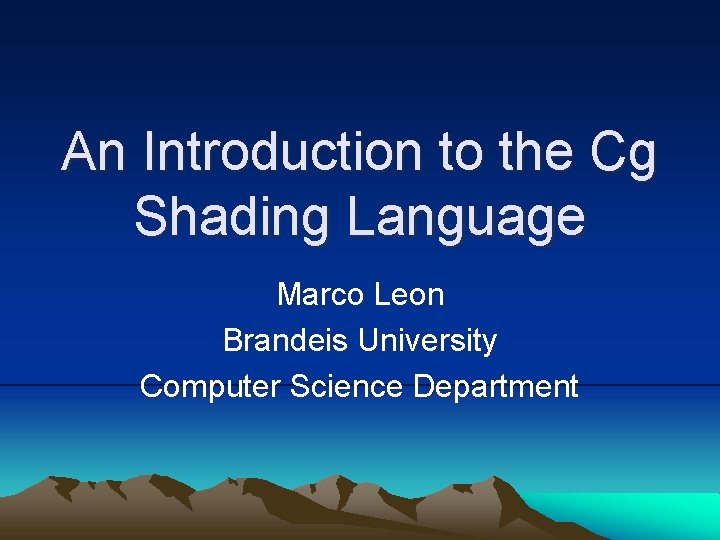
An Introduction to the Cg Shading Language Marco Leon Brandeis University Computer Science Department
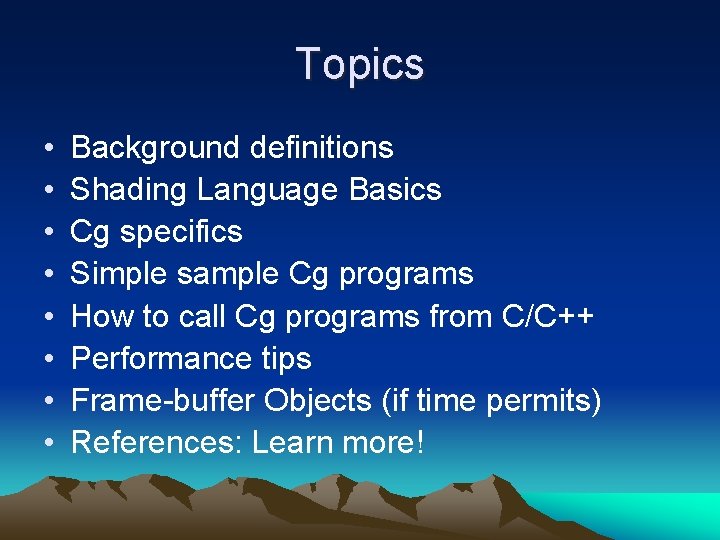
Topics • • Background definitions Shading Language Basics Cg specifics Simple sample Cg programs How to call Cg programs from C/C++ Performance tips Frame-buffer Objects (if time permits) References: Learn more!
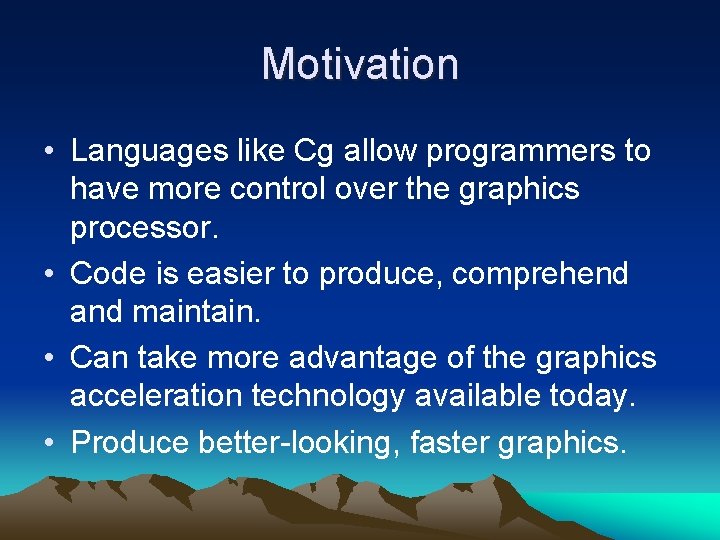
Motivation • Languages like Cg allow programmers to have more control over the graphics processor. • Code is easier to produce, comprehend and maintain. • Can take more advantage of the graphics acceleration technology available today. • Produce better-looking, faster graphics.
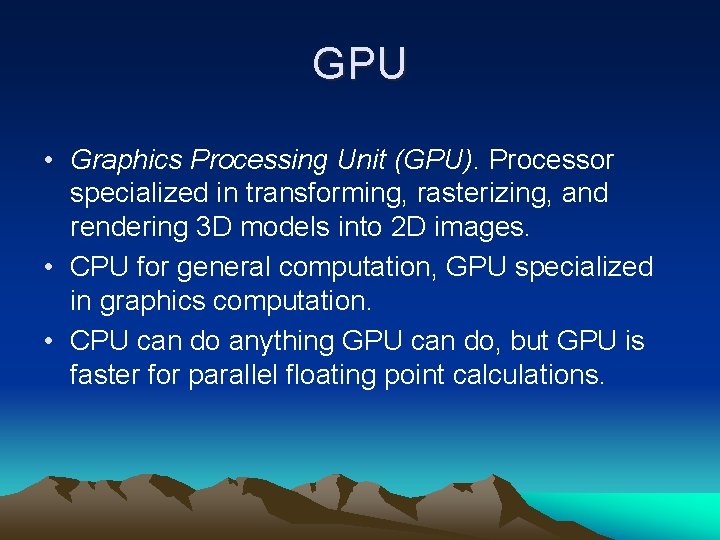
GPU • Graphics Processing Unit (GPU). Processor specialized in transforming, rasterizing, and rendering 3 D models into 2 D images. • CPU for general computation, GPU specialized in graphics computation. • CPU can do anything GPU can do, but GPU is faster for parallel floating point calculations.
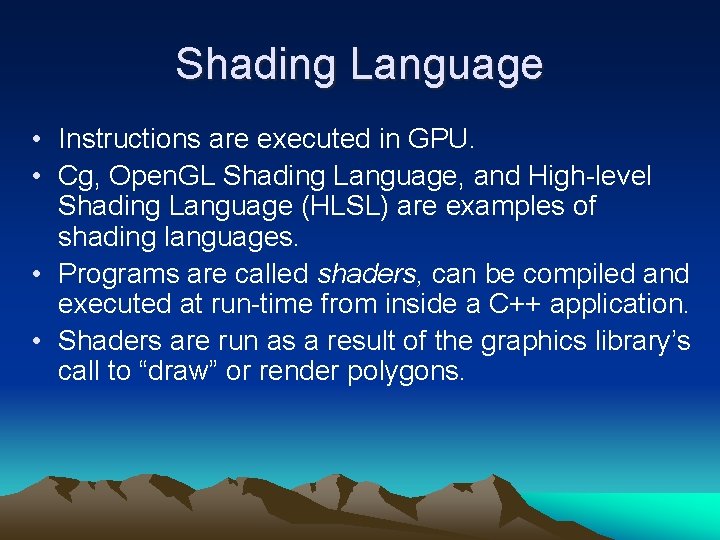
Shading Language • Instructions are executed in GPU. • Cg, Open. GL Shading Language, and High-level Shading Language (HLSL) are examples of shading languages. • Programs are called shaders, can be compiled and executed at run-time from inside a C++ application. • Shaders are run as a result of the graphics library’s call to “draw” or render polygons.
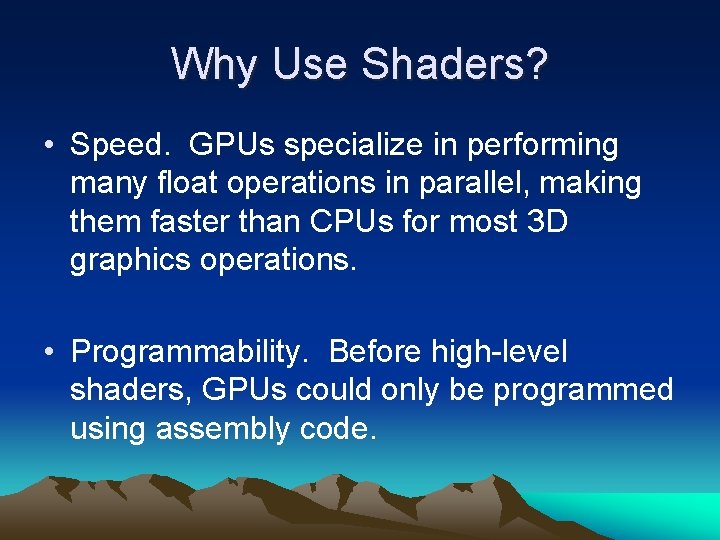
Why Use Shaders? • Speed. GPUs specialize in performing many float operations in parallel, making them faster than CPUs for most 3 D graphics operations. • Programmability. Before high-level shaders, GPUs could only be programmed using assembly code.
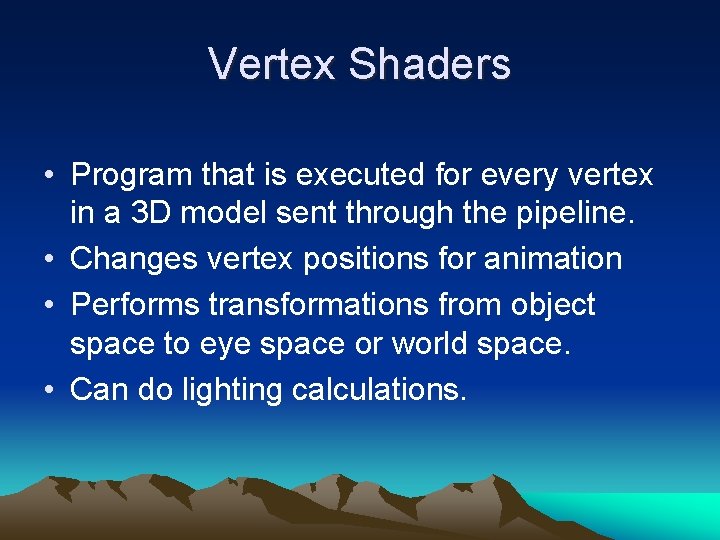
Vertex Shaders • Program that is executed for every vertex in a 3 D model sent through the pipeline. • Changes vertex positions for animation • Performs transformations from object space to eye space or world space. • Can do lighting calculations.
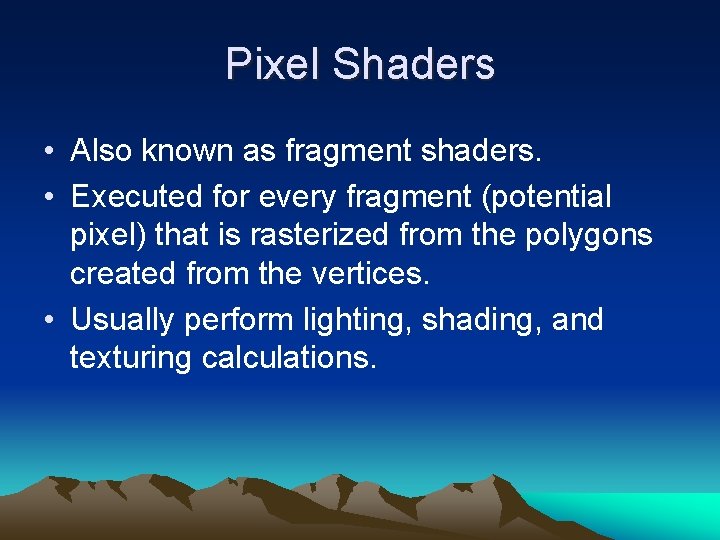
Pixel Shaders • Also known as fragment shaders. • Executed for every fragment (potential pixel) that is rasterized from the polygons created from the vertices. • Usually perform lighting, shading, and texturing calculations.
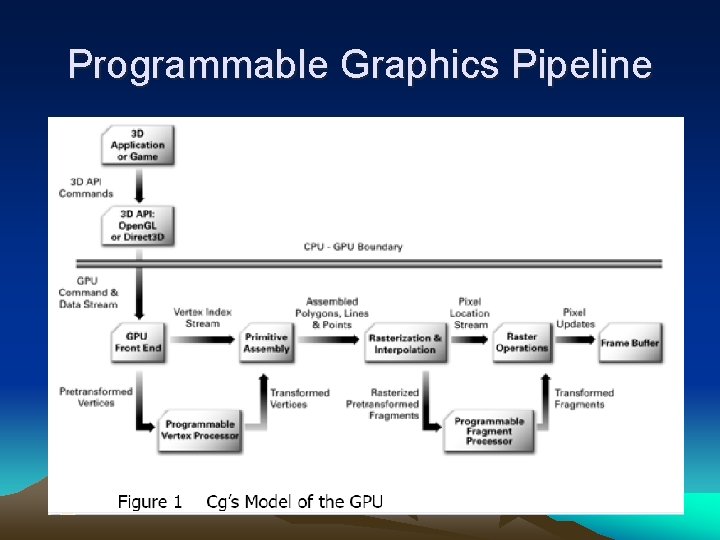
Programmable Graphics Pipeline
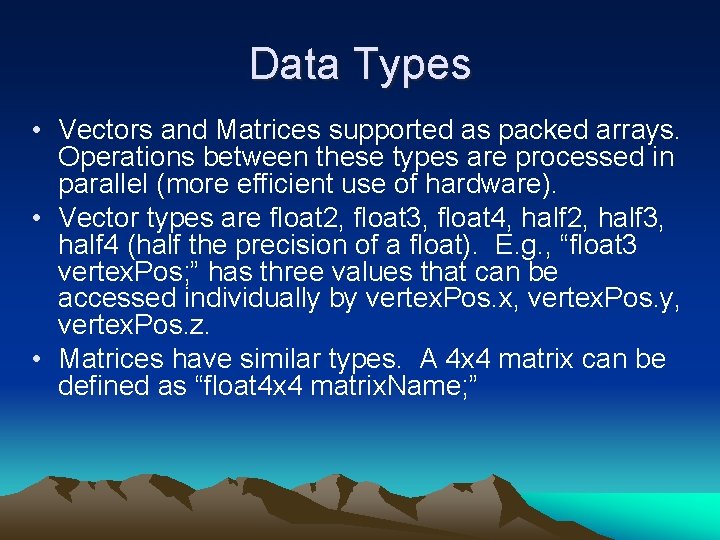
Data Types • Vectors and Matrices supported as packed arrays. Operations between these types are processed in parallel (more efficient use of hardware). • Vector types are float 2, float 3, float 4, half 2, half 3, half 4 (half the precision of a float). E. g. , “float 3 vertex. Pos; ” has three values that can be accessed individually by vertex. Pos. x, vertex. Pos. y, vertex. Pos. z. • Matrices have similar types. A 4 x 4 matrix can be defined as “float 4 x 4 matrix. Name; ”
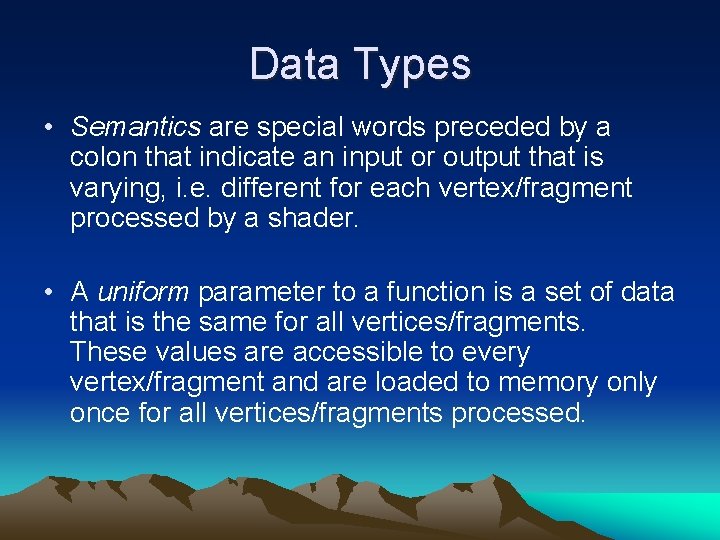
Data Types • Semantics are special words preceded by a colon that indicate an input or output that is varying, i. e. different for each vertex/fragment processed by a shader. • A uniform parameter to a function is a set of data that is the same for all vertices/fragments. These values are accessible to every vertex/fragment and are loaded to memory only once for all vertices/fragments processed.
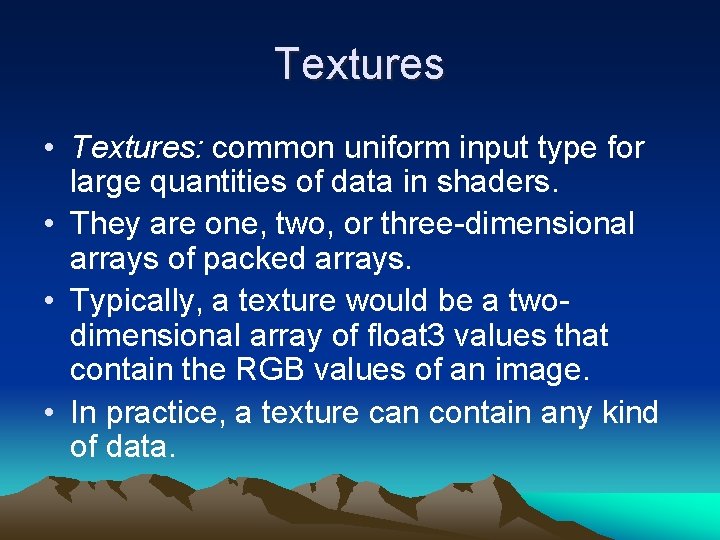
Textures • Textures: common uniform input type for large quantities of data in shaders. • They are one, two, or three-dimensional arrays of packed arrays. • Typically, a texture would be a twodimensional array of float 3 values that contain the RGB values of an image. • In practice, a texture can contain any kind of data.
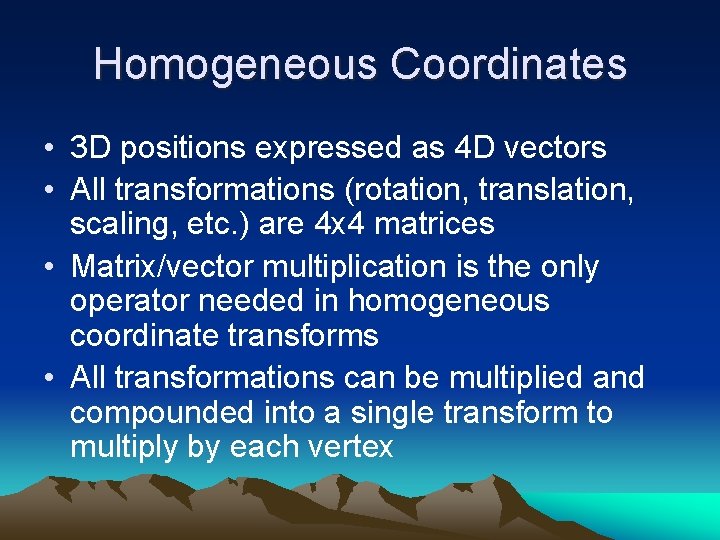
Homogeneous Coordinates • 3 D positions expressed as 4 D vectors • All transformations (rotation, translation, scaling, etc. ) are 4 x 4 matrices • Matrix/vector multiplication is the only operator needed in homogeneous coordinate transforms • All transformations can be multiplied and compounded into a single transform to multiply by each vertex
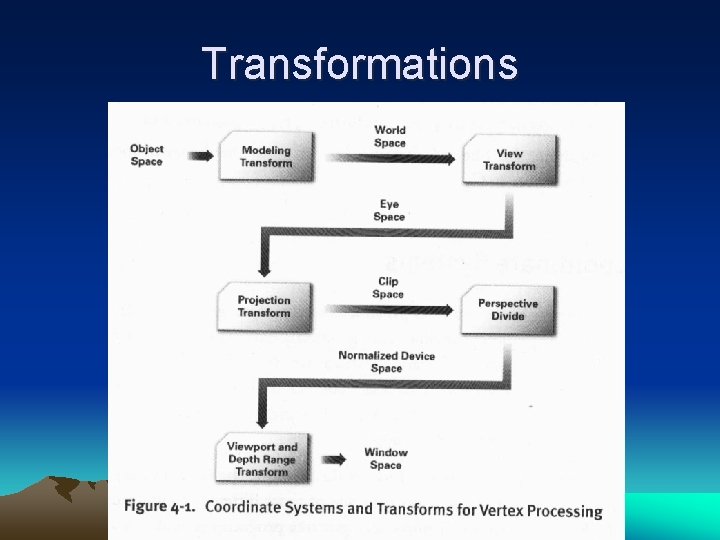
Transformations
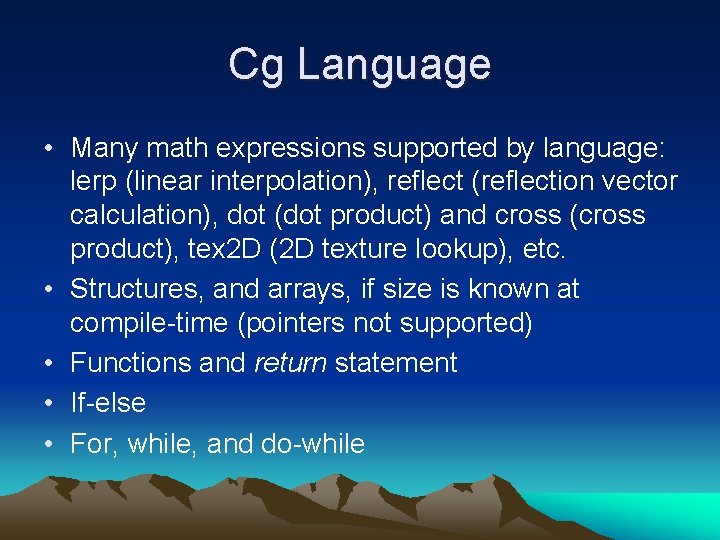
Cg Language • Many math expressions supported by language: lerp (linear interpolation), reflect (reflection vector calculation), dot (dot product) and cross (cross product), tex 2 D (2 D texture lookup), etc. • Structures, and arrays, if size is known at compile-time (pointers not supported) • Functions and return statement • If-else • For, while, and do-while
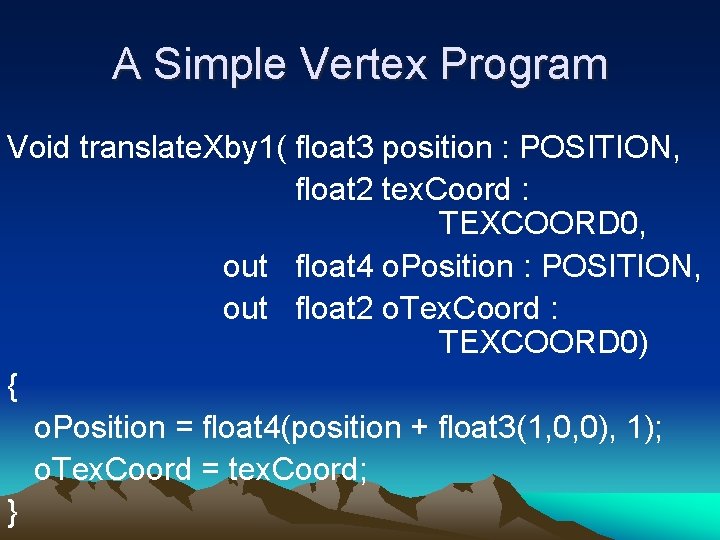
A Simple Vertex Program Void translate. Xby 1( float 3 position : POSITION, float 2 tex. Coord : TEXCOORD 0, out float 4 o. Position : POSITION, out float 2 o. Tex. Coord : TEXCOORD 0) { o. Position = float 4(position + float 3(1, 0, 0), 1); o. Tex. Coord = tex. Coord; }
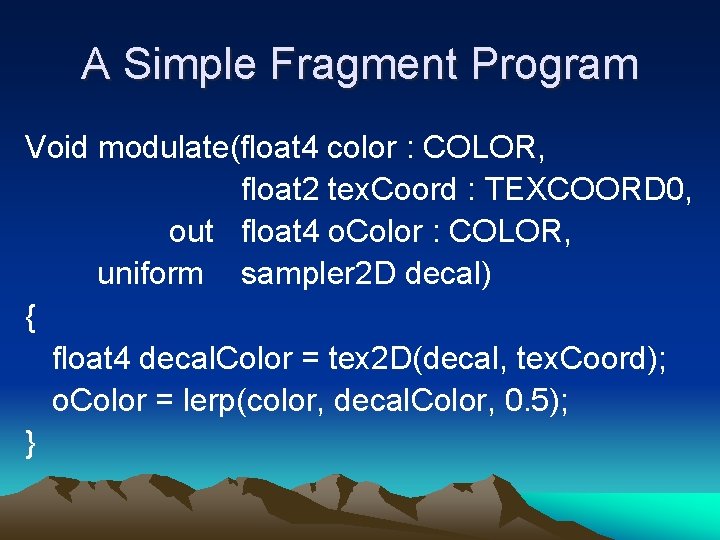
A Simple Fragment Program Void modulate(float 4 color : COLOR, float 2 tex. Coord : TEXCOORD 0, out float 4 o. Color : COLOR, uniform sampler 2 D decal) { float 4 decal. Color = tex 2 D(decal, tex. Coord); o. Color = lerp(color, decal. Color, 0. 5); }
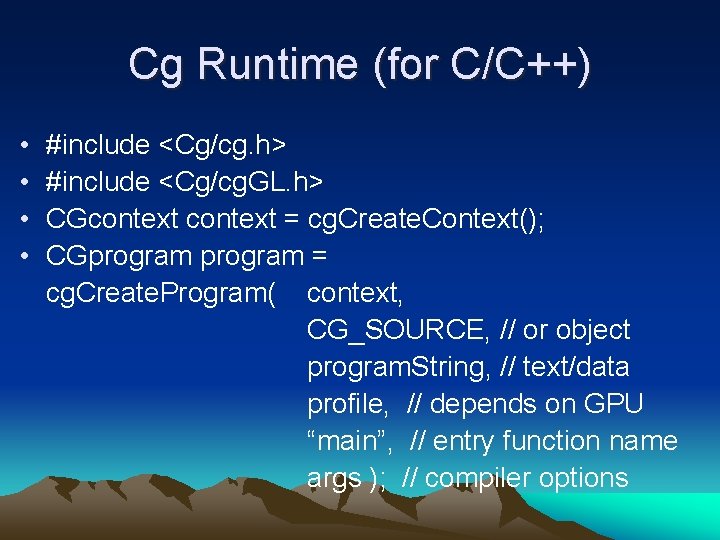
Cg Runtime (for C/C++) • • #include <Cg/cg. h> #include <Cg/cg. GL. h> CGcontext = cg. Create. Context(); CGprogram = cg. Create. Program( context, CG_SOURCE, // or object program. String, // text/data profile, // depends on GPU “main”, // entry function name args ); // compiler options
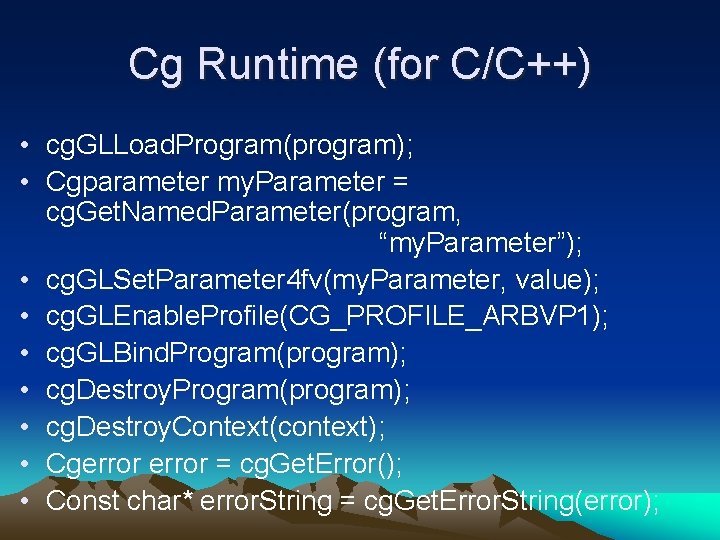
Cg Runtime (for C/C++) • cg. GLLoad. Program(program); • Cgparameter my. Parameter = cg. Get. Named. Parameter(program, “my. Parameter”); • cg. GLSet. Parameter 4 fv(my. Parameter, value); • cg. GLEnable. Profile(CG_PROFILE_ARBVP 1); • cg. GLBind. Program(program); • cg. Destroy. Context(context); • Cgerror = cg. Get. Error(); • Const char* error. String = cg. Get. Error. String(error);
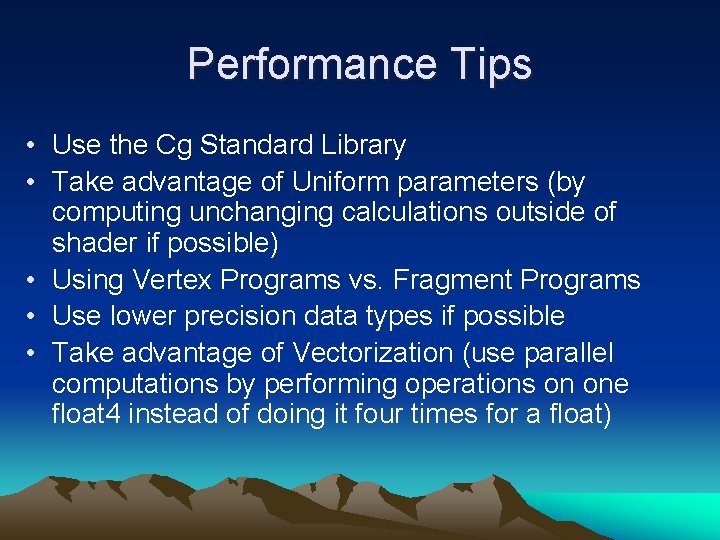
Performance Tips • Use the Cg Standard Library • Take advantage of Uniform parameters (by computing unchanging calculations outside of shader if possible) • Using Vertex Programs vs. Fragment Programs • Use lower precision data types if possible • Take advantage of Vectorization (use parallel computations by performing operations on one float 4 instead of doing it four times for a float)
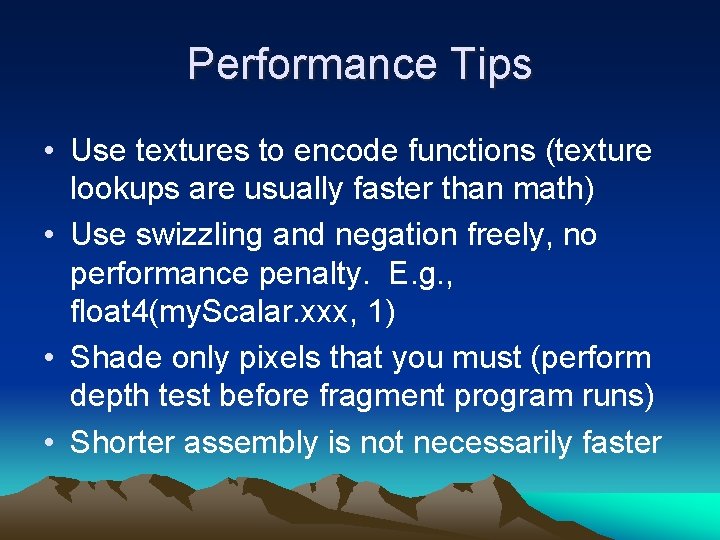
Performance Tips • Use textures to encode functions (texture lookups are usually faster than math) • Use swizzling and negation freely, no performance penalty. E. g. , float 4(my. Scalar. xxx, 1) • Shade only pixels that you must (perform depth test before fragment program runs) • Shorter assembly is not necessarily faster
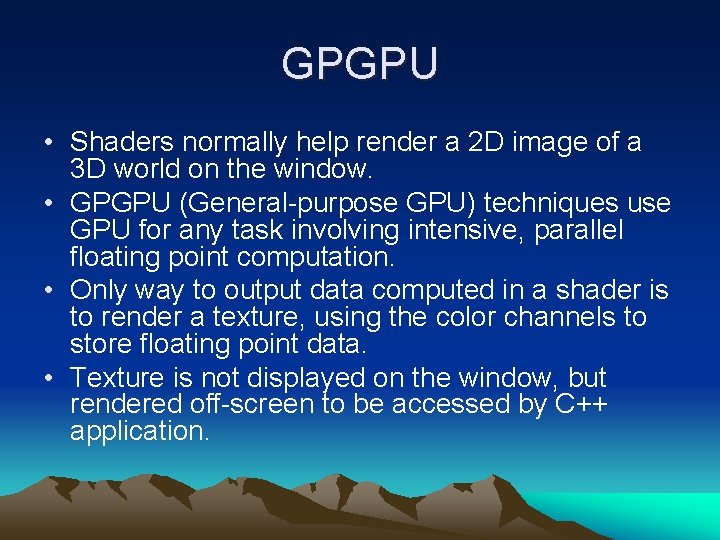
GPGPU • Shaders normally help render a 2 D image of a 3 D world on the window. • GPGPU (General-purpose GPU) techniques use GPU for any task involving intensive, parallel floating point computation. • Only way to output data computed in a shader is to render a texture, using the color channels to store floating point data. • Texture is not displayed on the window, but rendered off-screen to be accessed by C++ application.
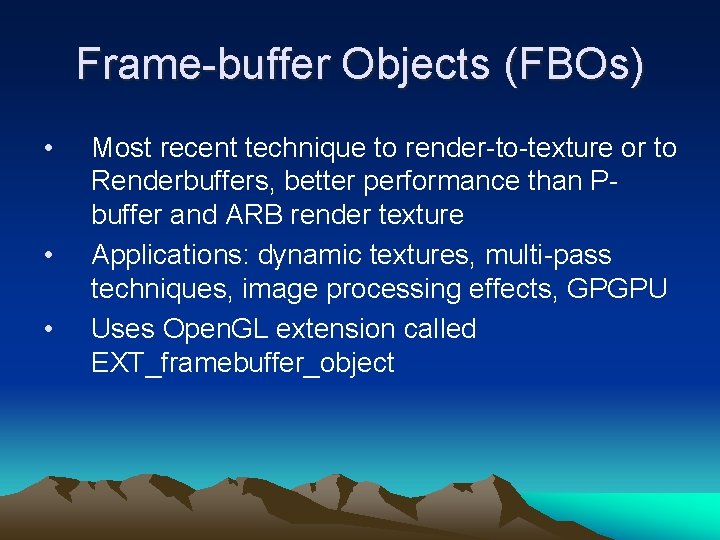
Frame-buffer Objects (FBOs) • • • Most recent technique to render-to-texture or to Renderbuffers, better performance than Pbuffer and ARB render texture Applications: dynamic textures, multi-pass techniques, image processing effects, GPGPU Uses Open. GL extension called EXT_framebuffer_object
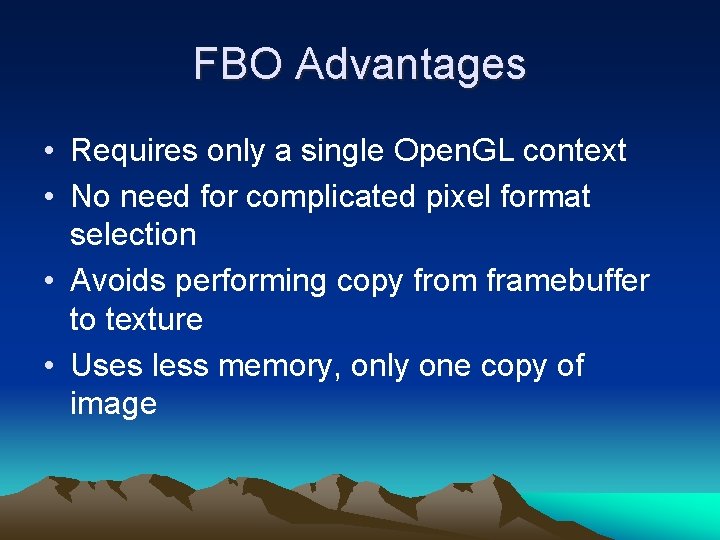
FBO Advantages • Requires only a single Open. GL context • No need for complicated pixel format selection • Avoids performing copy from framebuffer to texture • Uses less memory, only one copy of image
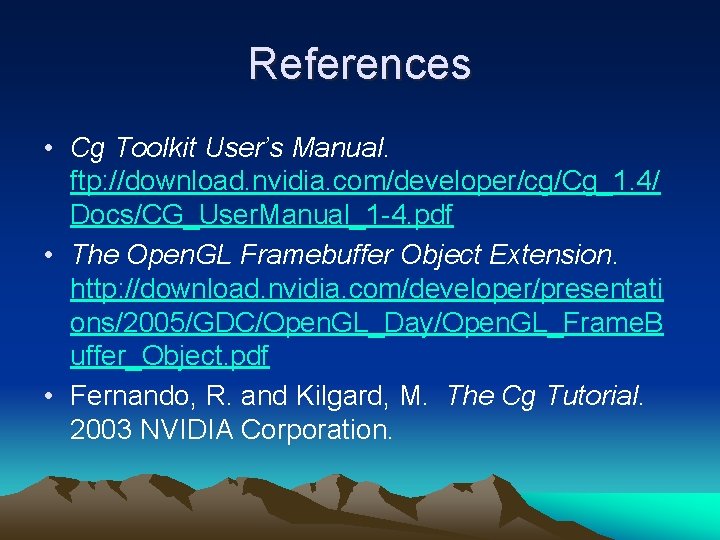
References • Cg Toolkit User’s Manual. ftp: //download. nvidia. com/developer/cg/Cg_1. 4/ Docs/CG_User. Manual_1 -4. pdf • The Open. GL Framebuffer Object Extension. http: //download. nvidia. com/developer/presentati ons/2005/GDC/Open. GL_Day/Open. GL_Frame. B uffer_Object. pdf • Fernando, R. and Kilgard, M. The Cg Tutorial. 2003 NVIDIA Corporation.
Phong shading vs gouraud shading
Slang shader
Cg shading language
Que contiene el marco contextual
Hình ảnh bộ gõ cơ thể búng tay
Bổ thể
Tỉ lệ cơ thể trẻ em
Voi kéo gỗ như thế nào
Thang điểm glasgow
Chúa sống lại
Kể tên các môn thể thao
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới
Công của trọng lực
Trời xanh đây là của chúng ta thể thơ
Mật thư anh em như thể tay chân
Phép trừ bù
độ dài liên kết
Các châu lục và đại dương trên thế giới
Thể thơ truyền thống
Quá trình desamine hóa có thể tạo ra
Một số thể thơ truyền thống
Bàn tay mà dây bẩn
Vẽ hình chiếu vuông góc của vật thể sau
Biện pháp chống mỏi cơ