Using Fang Engine The Fang Engine is created
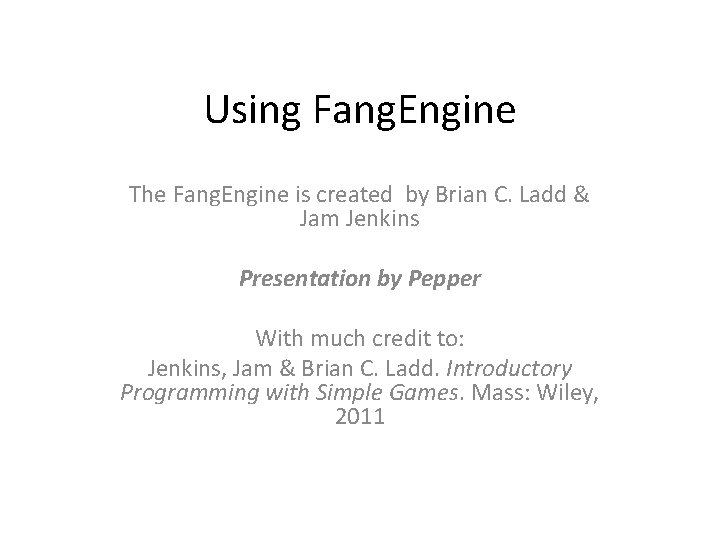
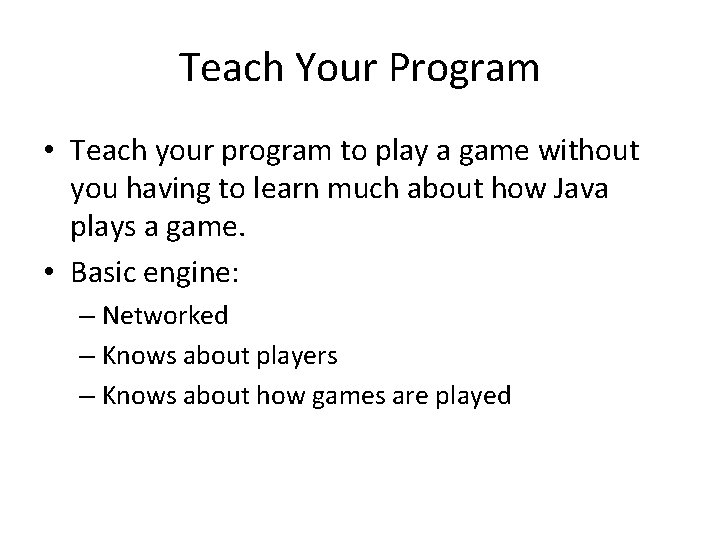
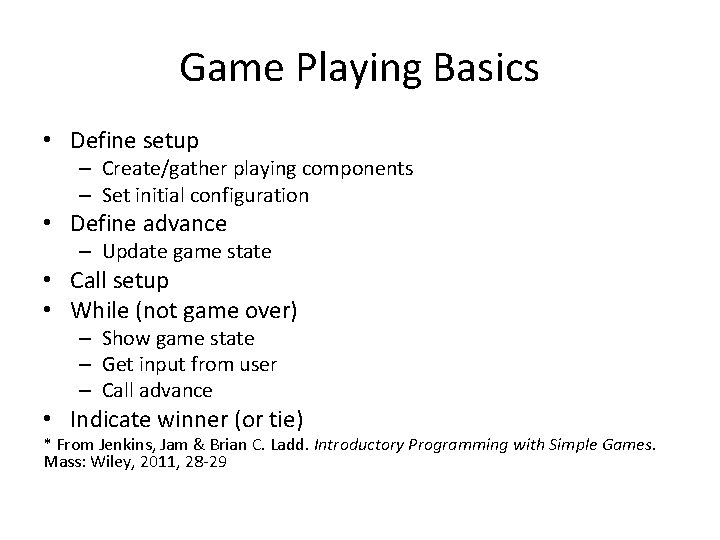
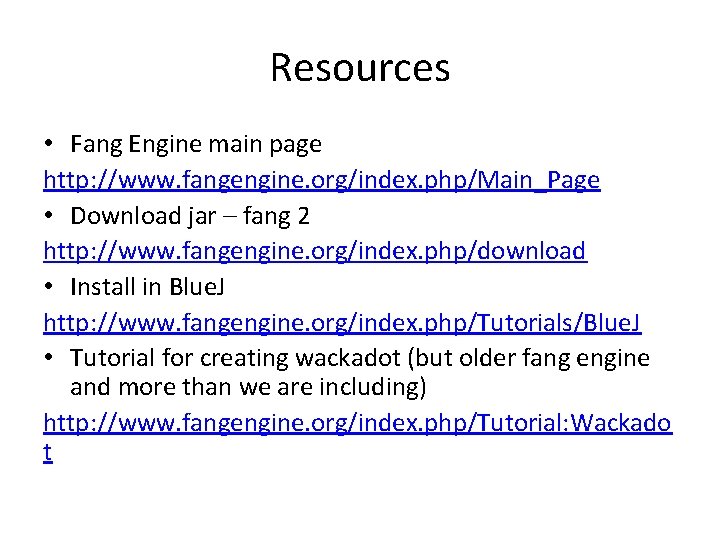
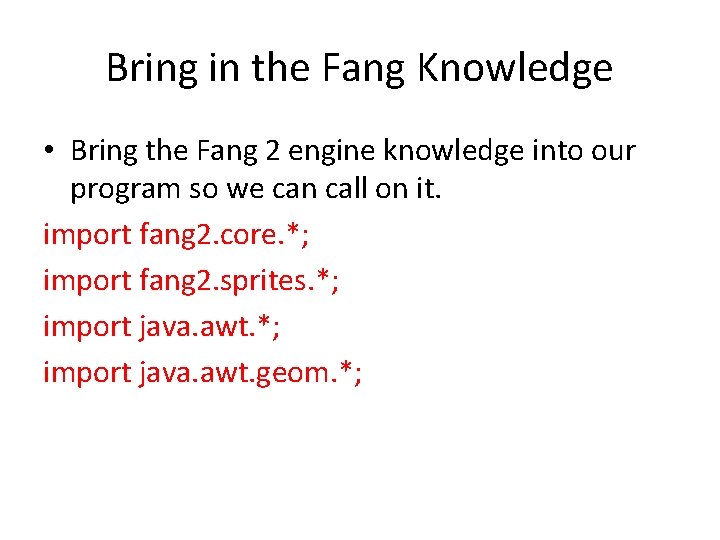
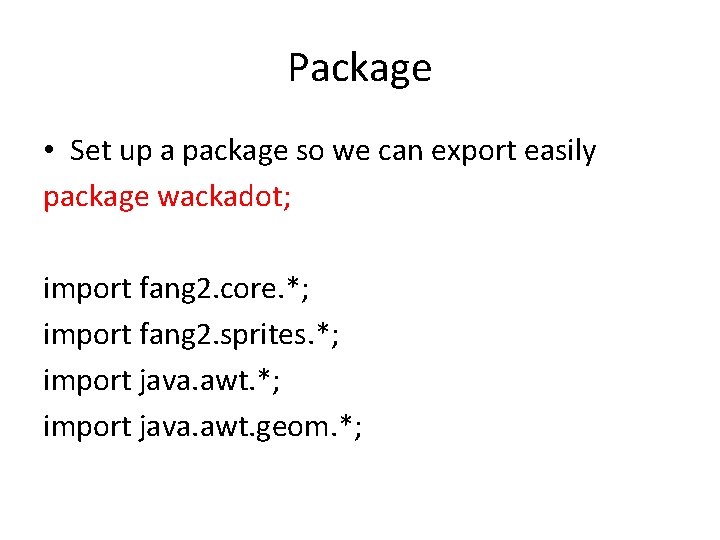
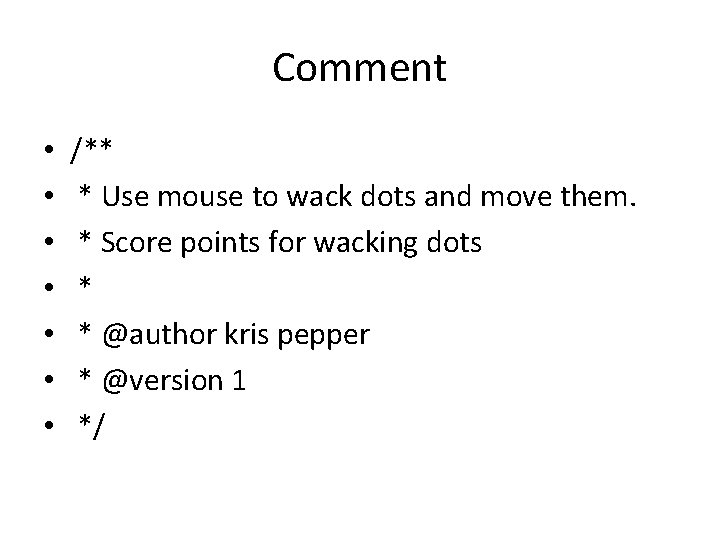
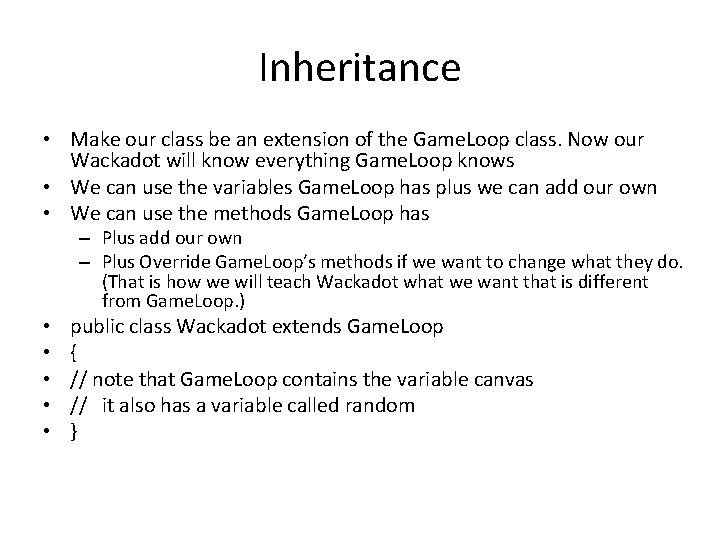
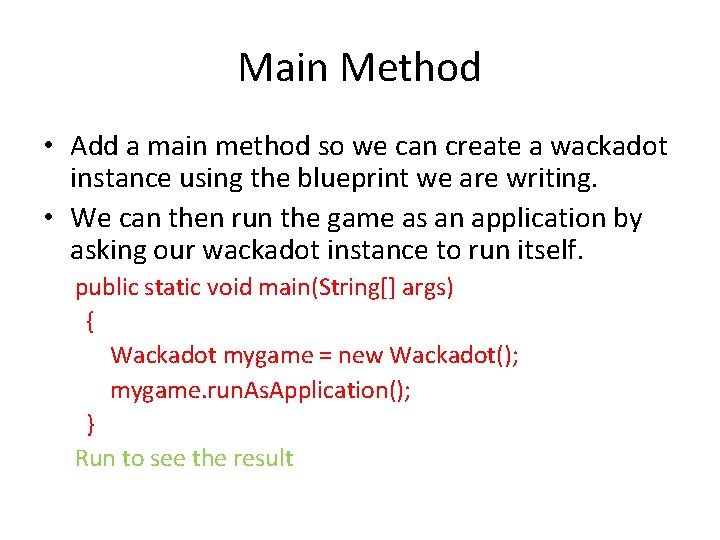
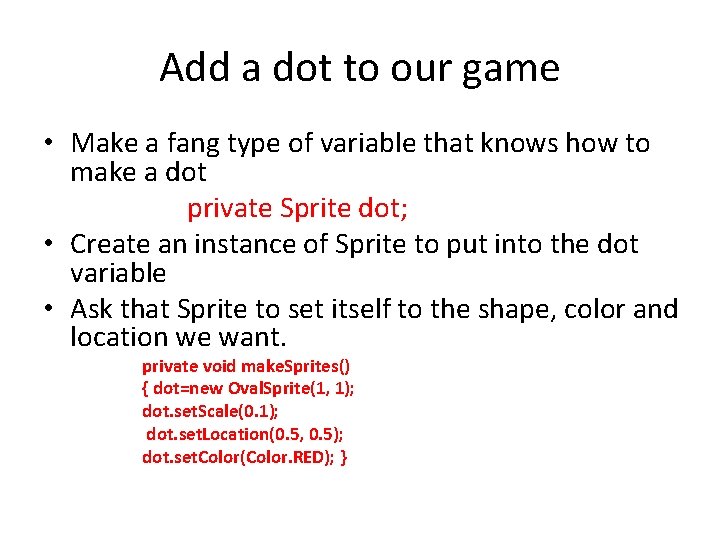
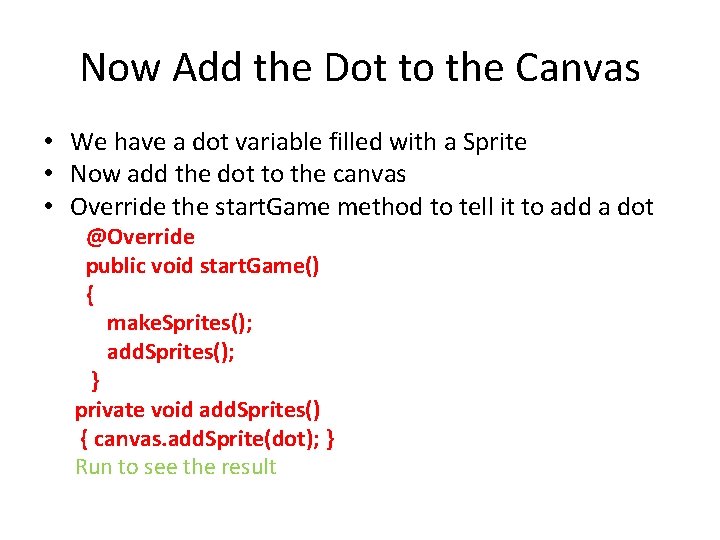
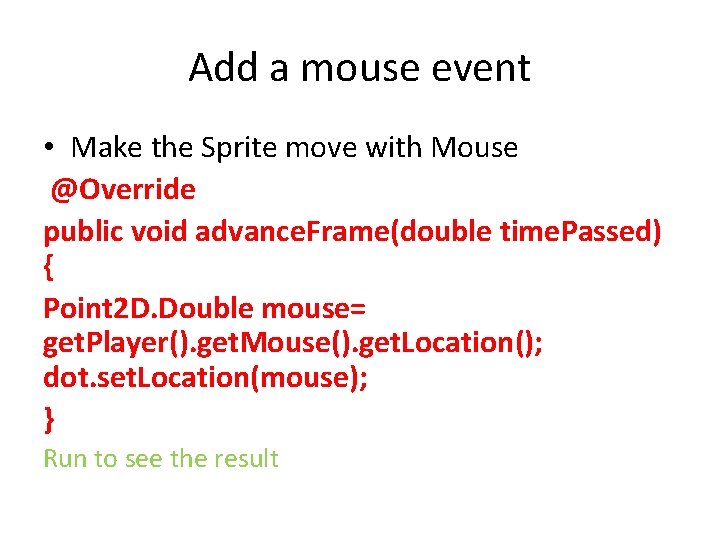
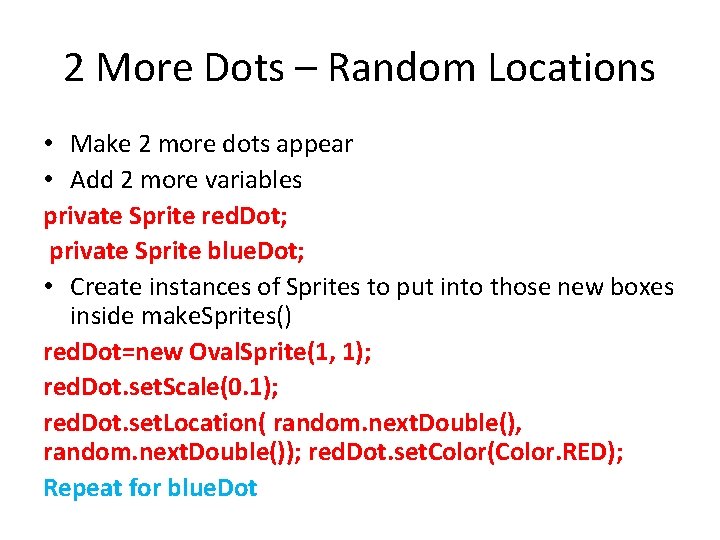
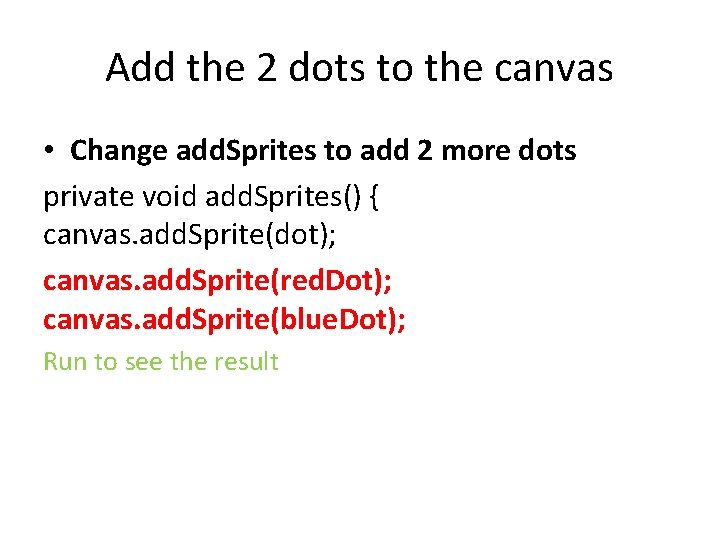
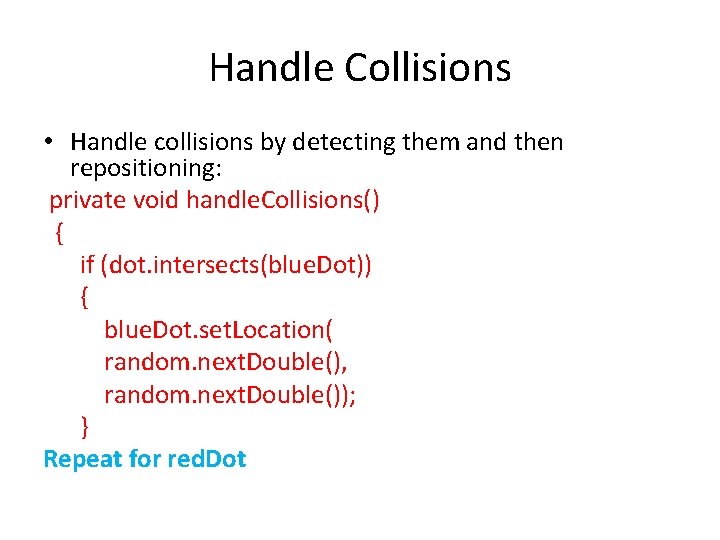
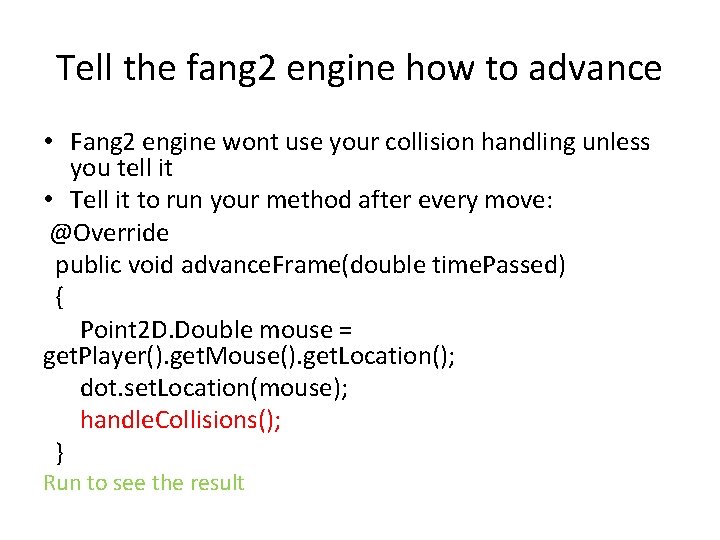
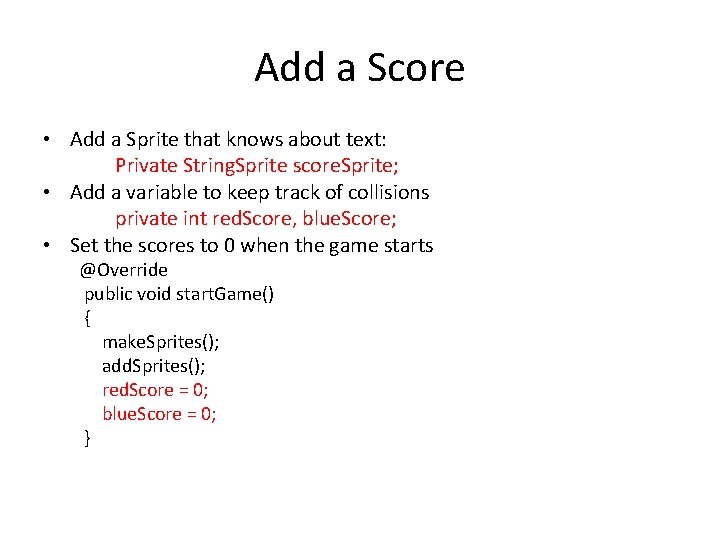
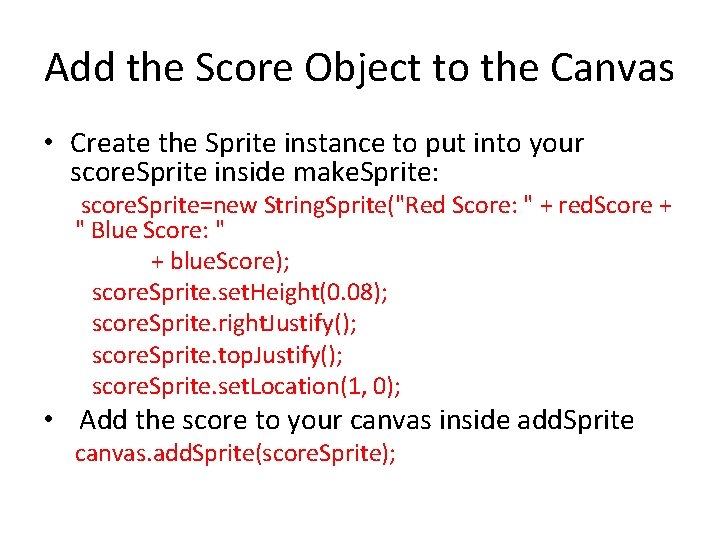
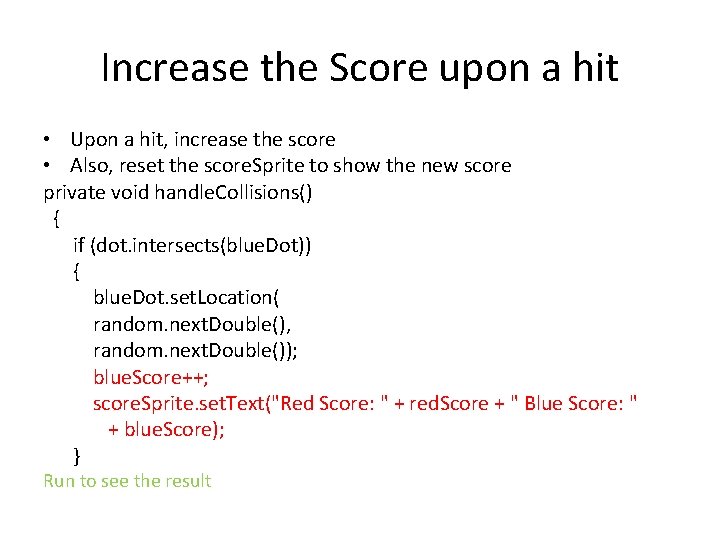
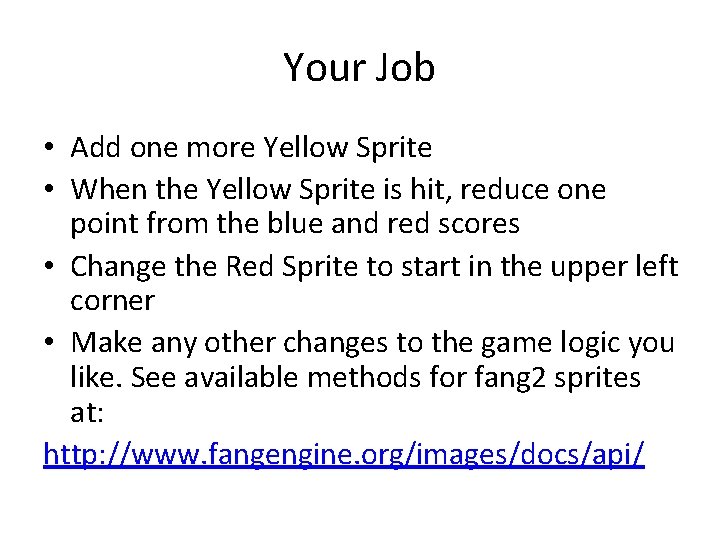
- Slides: 20
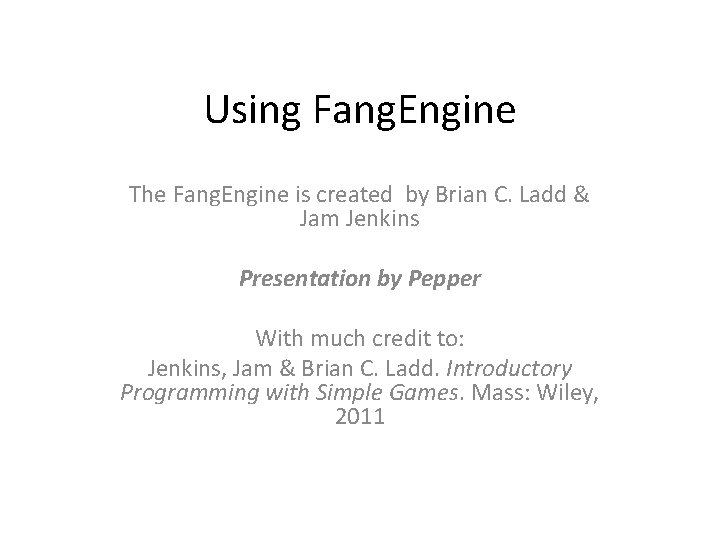
Using Fang. Engine The Fang. Engine is created by Brian C. Ladd & Jam Jenkins Presentation by Pepper With much credit to: Jenkins, Jam & Brian C. Ladd. Introductory Programming with Simple Games. Mass: Wiley, 2011
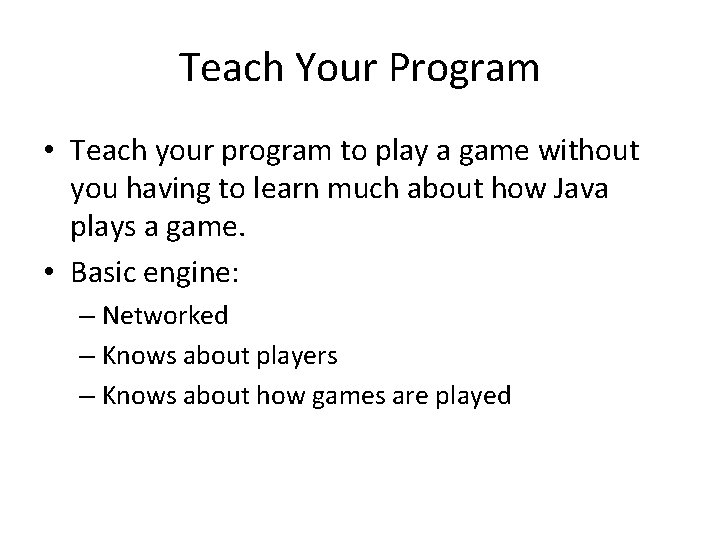
Teach Your Program • Teach your program to play a game without you having to learn much about how Java plays a game. • Basic engine: – Networked – Knows about players – Knows about how games are played
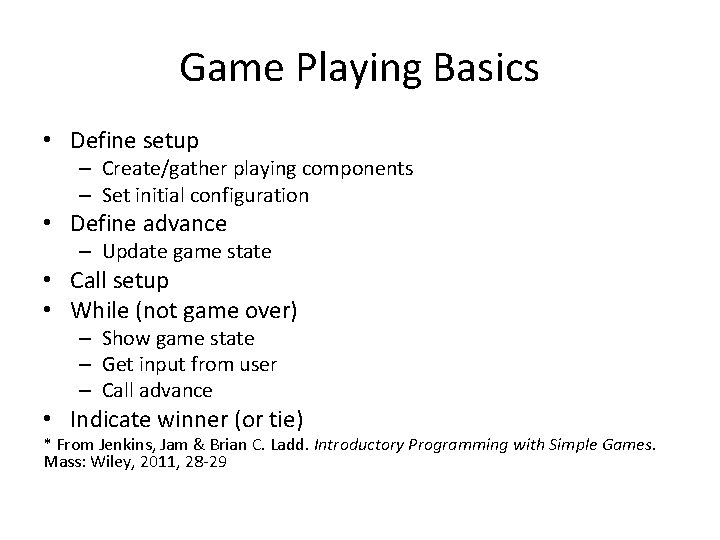
Game Playing Basics • Define setup – Create/gather playing components – Set initial configuration • Define advance – Update game state • Call setup • While (not game over) – Show game state – Get input from user – Call advance • Indicate winner (or tie) * From Jenkins, Jam & Brian C. Ladd. Introductory Programming with Simple Games. Mass: Wiley, 2011, 28 -29
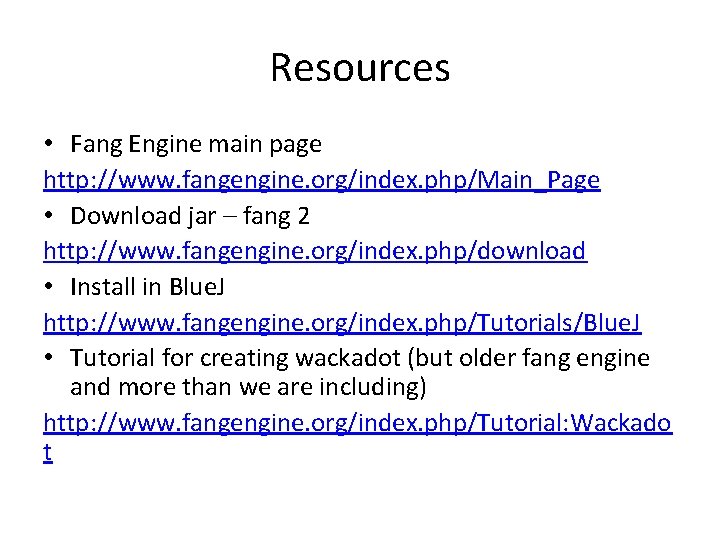
Resources • Fang Engine main page http: //www. fangengine. org/index. php/Main_Page • Download jar – fang 2 http: //www. fangengine. org/index. php/download • Install in Blue. J http: //www. fangengine. org/index. php/Tutorials/Blue. J • Tutorial for creating wackadot (but older fang engine and more than we are including) http: //www. fangengine. org/index. php/Tutorial: Wackado t
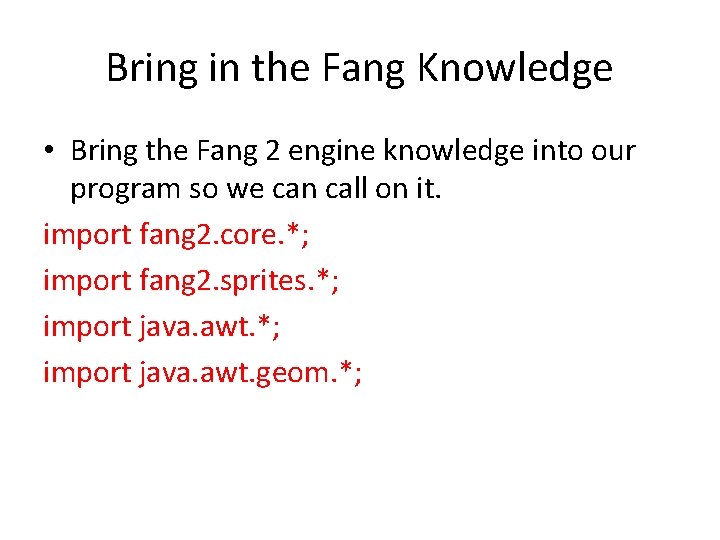
Bring in the Fang Knowledge • Bring the Fang 2 engine knowledge into our program so we can call on it. import fang 2. core. *; import fang 2. sprites. *; import java. awt. geom. *;
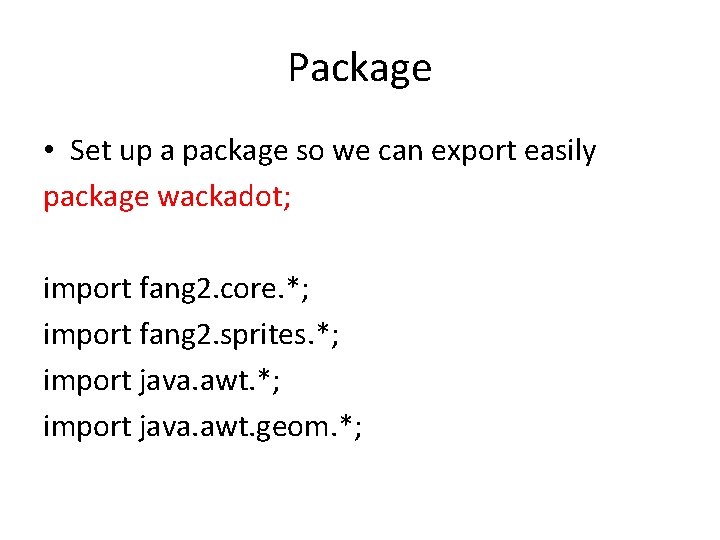
Package • Set up a package so we can export easily package wackadot; import fang 2. core. *; import fang 2. sprites. *; import java. awt. geom. *;
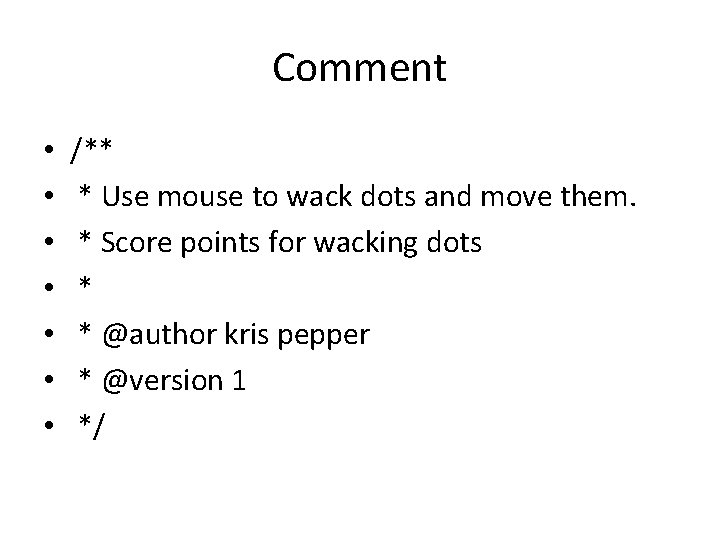
Comment • • /** * Use mouse to wack dots and move them. * Score points for wacking dots * * @author kris pepper * @version 1 */
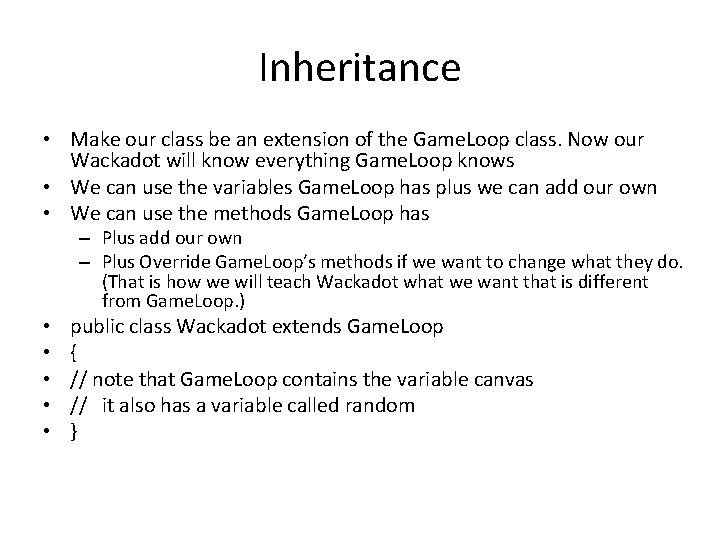
Inheritance • Make our class be an extension of the Game. Loop class. Now our Wackadot will know everything Game. Loop knows • We can use the variables Game. Loop has plus we can add our own • We can use the methods Game. Loop has – Plus add our own – Plus Override Game. Loop’s methods if we want to change what they do. (That is how we will teach Wackadot what we want that is different from Game. Loop. ) • • • public class Wackadot extends Game. Loop { // note that Game. Loop contains the variable canvas // it also has a variable called random }
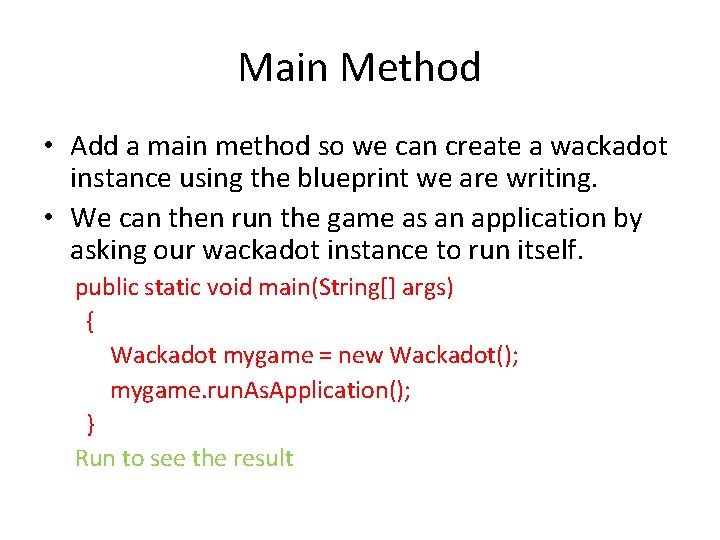
Main Method • Add a main method so we can create a wackadot instance using the blueprint we are writing. • We can then run the game as an application by asking our wackadot instance to run itself. public static void main(String[] args) { Wackadot mygame = new Wackadot(); mygame. run. As. Application(); } Run to see the result
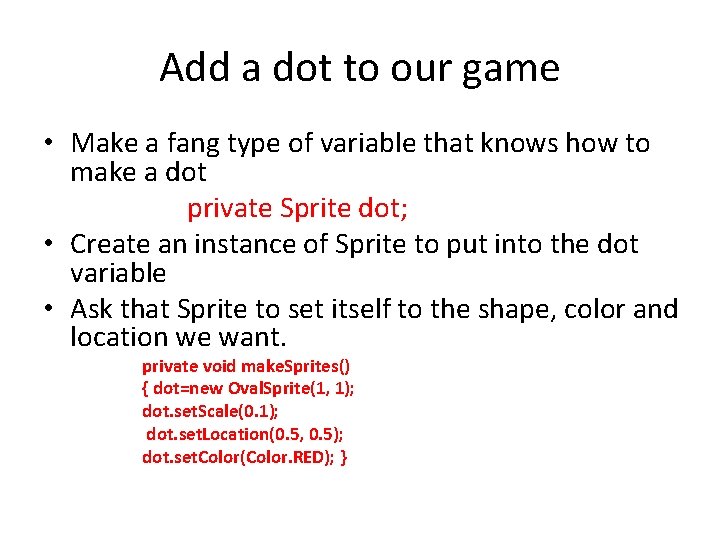
Add a dot to our game • Make a fang type of variable that knows how to make a dot private Sprite dot; • Create an instance of Sprite to put into the dot variable • Ask that Sprite to set itself to the shape, color and location we want. private void make. Sprites() { dot=new Oval. Sprite(1, 1); dot. set. Scale(0. 1); dot. set. Location(0. 5, 0. 5); dot. set. Color(Color. RED); }
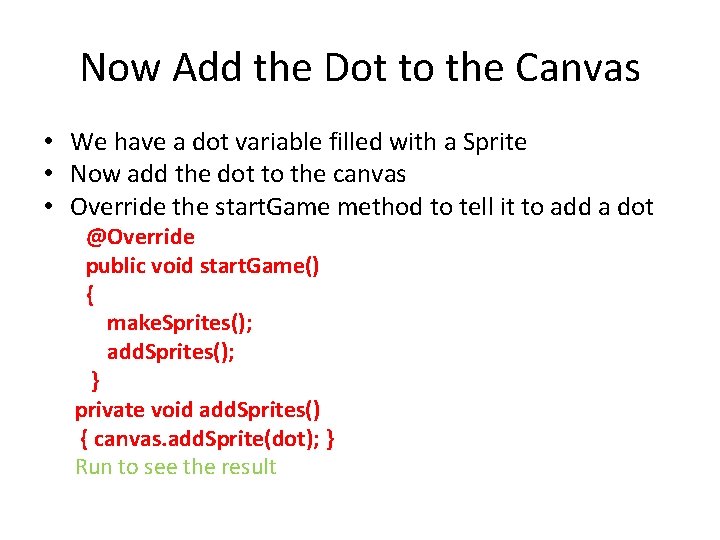
Now Add the Dot to the Canvas • We have a dot variable filled with a Sprite • Now add the dot to the canvas • Override the start. Game method to tell it to add a dot @Override public void start. Game() { make. Sprites(); add. Sprites(); } private void add. Sprites() { canvas. add. Sprite(dot); } Run to see the result
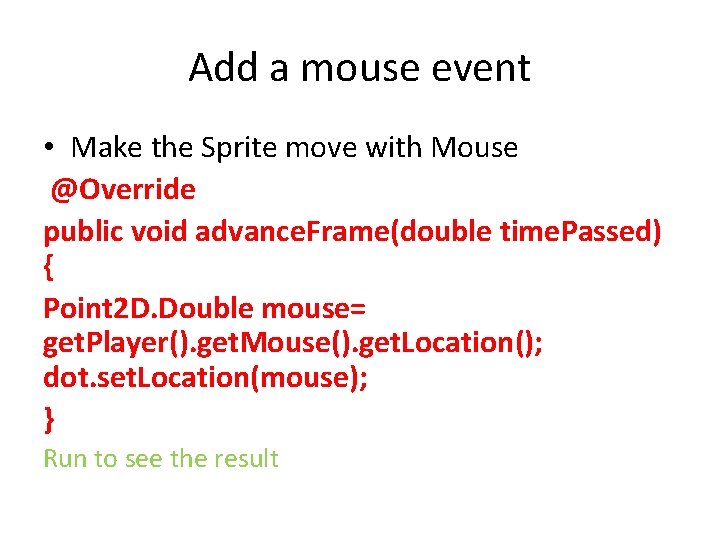
Add a mouse event • Make the Sprite move with Mouse @Override public void advance. Frame(double time. Passed) { Point 2 D. Double mouse= get. Player(). get. Mouse(). get. Location(); dot. set. Location(mouse); } Run to see the result
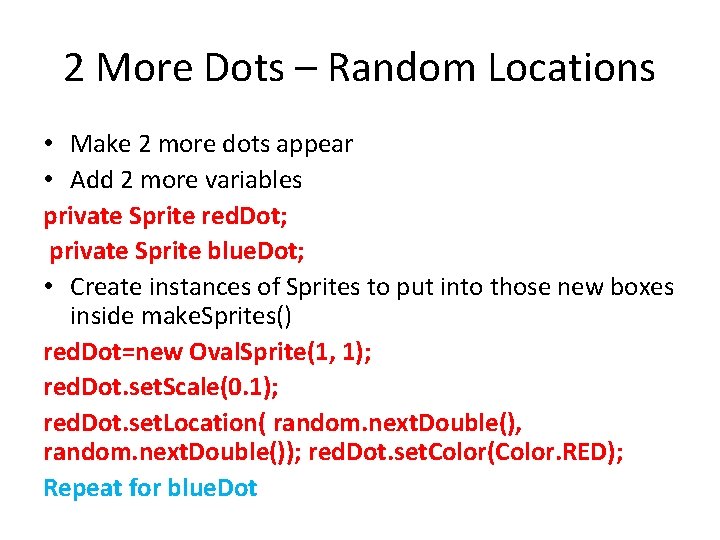
2 More Dots – Random Locations • Make 2 more dots appear • Add 2 more variables private Sprite red. Dot; private Sprite blue. Dot; • Create instances of Sprites to put into those new boxes inside make. Sprites() red. Dot=new Oval. Sprite(1, 1); red. Dot. set. Scale(0. 1); red. Dot. set. Location( random. next. Double(), random. next. Double()); red. Dot. set. Color(Color. RED); Repeat for blue. Dot
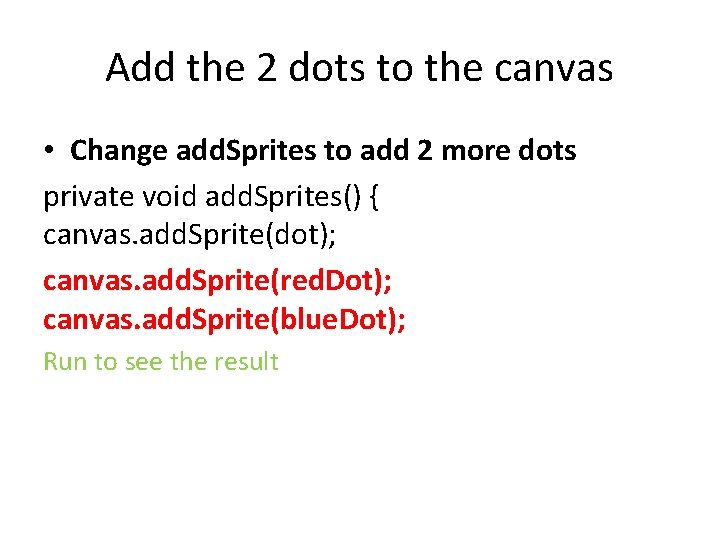
Add the 2 dots to the canvas • Change add. Sprites to add 2 more dots private void add. Sprites() { canvas. add. Sprite(dot); canvas. add. Sprite(red. Dot); canvas. add. Sprite(blue. Dot); Run to see the result
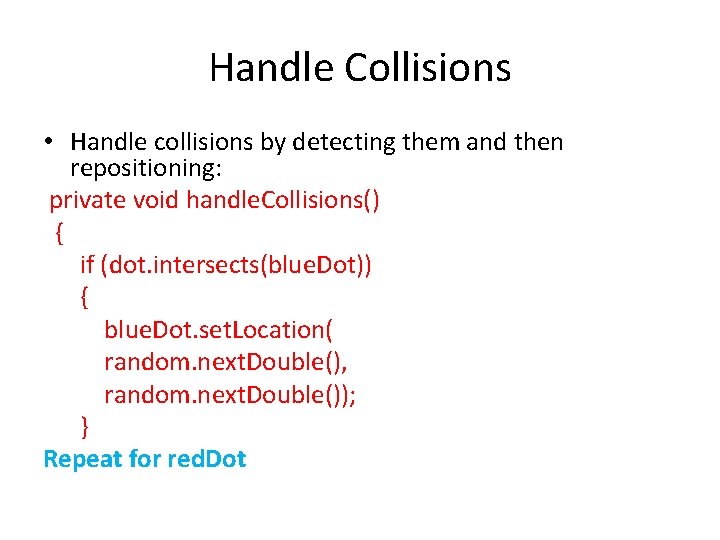
Handle Collisions • Handle collisions by detecting them and then repositioning: private void handle. Collisions() { if (dot. intersects(blue. Dot)) { blue. Dot. set. Location( random. next. Double(), random. next. Double()); } Repeat for red. Dot
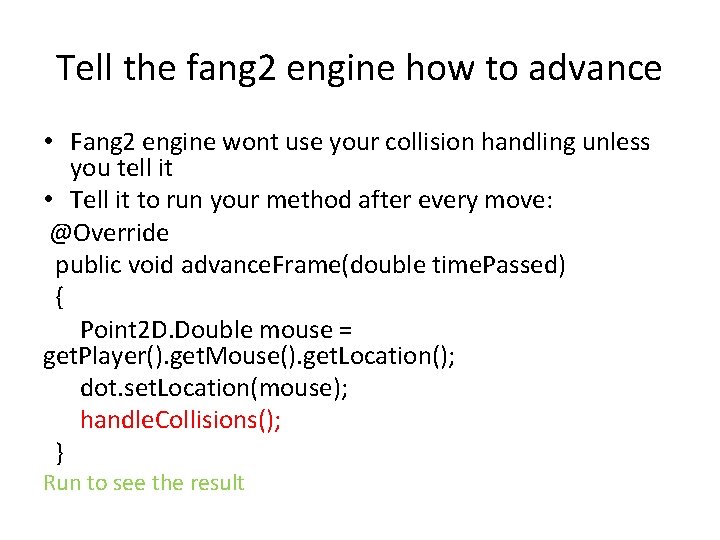
Tell the fang 2 engine how to advance • Fang 2 engine wont use your collision handling unless you tell it • Tell it to run your method after every move: @Override public void advance. Frame(double time. Passed) { Point 2 D. Double mouse = get. Player(). get. Mouse(). get. Location(); dot. set. Location(mouse); handle. Collisions(); } Run to see the result
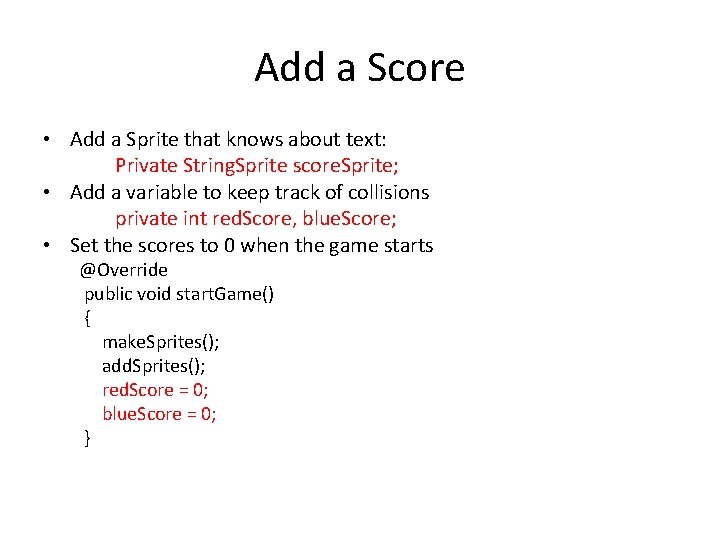
Add a Score • Add a Sprite that knows about text: Private String. Sprite score. Sprite; • Add a variable to keep track of collisions private int red. Score, blue. Score; • Set the scores to 0 when the game starts @Override public void start. Game() { make. Sprites(); add. Sprites(); red. Score = 0; blue. Score = 0; }
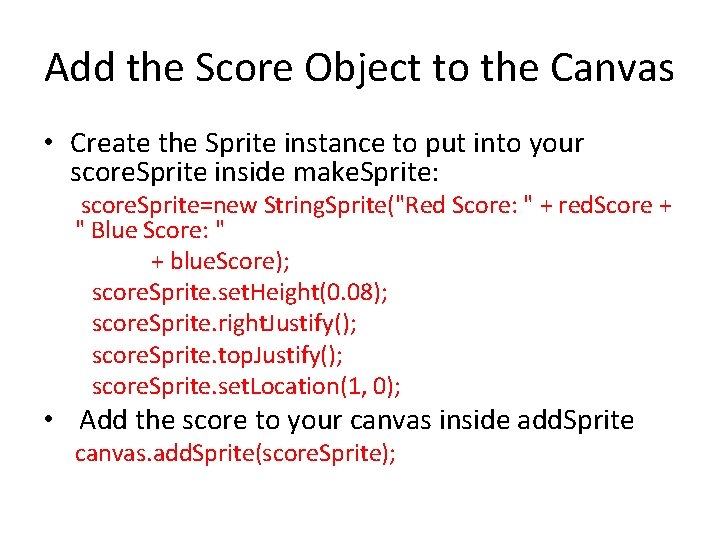
Add the Score Object to the Canvas • Create the Sprite instance to put into your score. Sprite inside make. Sprite: score. Sprite=new String. Sprite("Red Score: " + red. Score + " Blue Score: " + blue. Score); score. Sprite. set. Height(0. 08); score. Sprite. right. Justify(); score. Sprite. top. Justify(); score. Sprite. set. Location(1, 0); • Add the score to your canvas inside add. Sprite canvas. add. Sprite(score. Sprite);
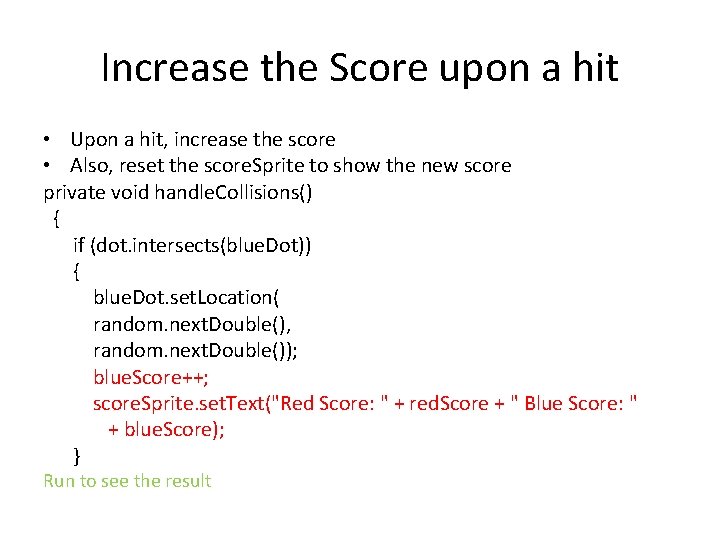
Increase the Score upon a hit • Upon a hit, increase the score • Also, reset the score. Sprite to show the new score private void handle. Collisions() { if (dot. intersects(blue. Dot)) { blue. Dot. set. Location( random. next. Double(), random. next. Double()); blue. Score++; score. Sprite. set. Text("Red Score: " + red. Score + " Blue Score: " + blue. Score); } Run to see the result
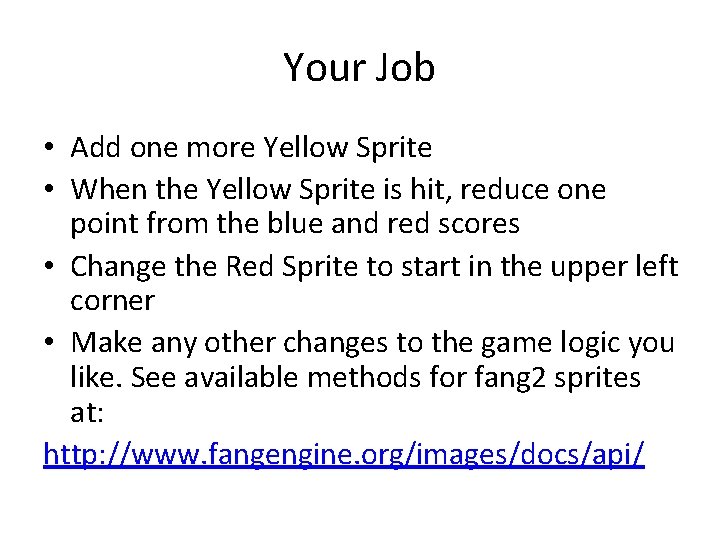
Your Job • Add one more Yellow Sprite • When the Yellow Sprite is hit, reduce one point from the blue and red scores • Change the Red Sprite to start in the upper left corner • Make any other changes to the game logic you like. See available methods for fang 2 sprites at: http: //www. fangengine. org/images/docs/api/