SEQUENCES SORTING Sorting z Input unsorted sequence of
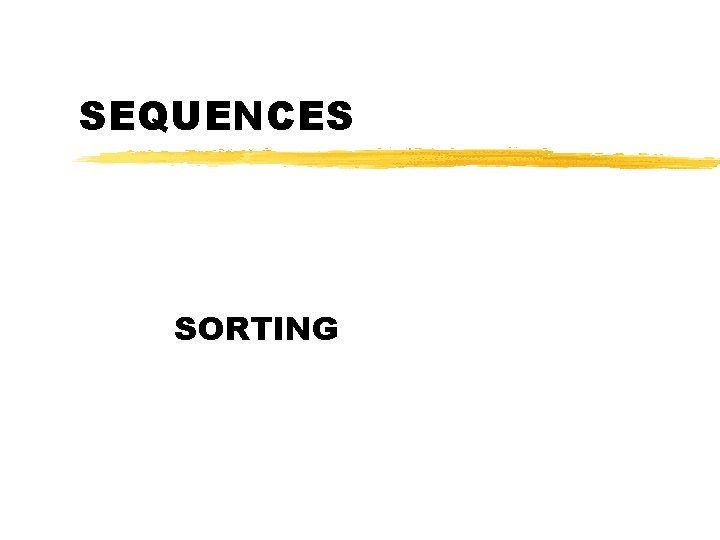
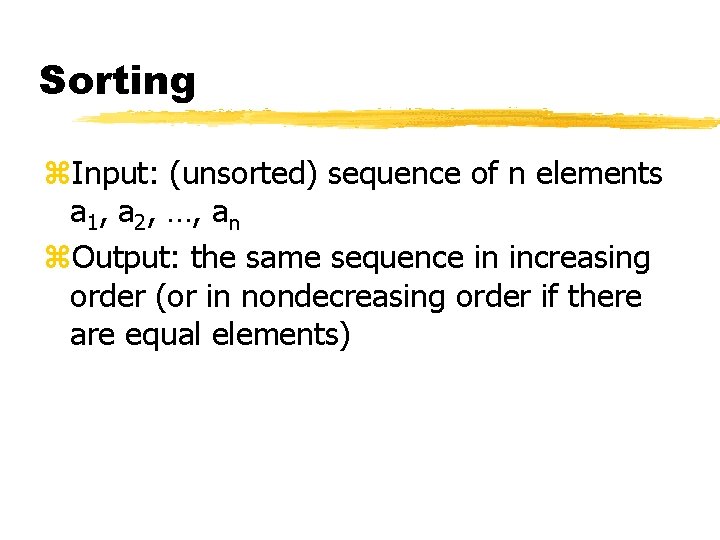
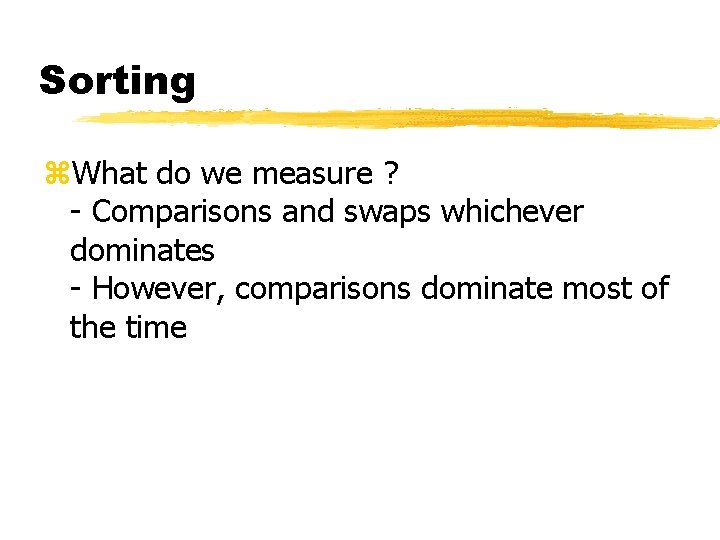
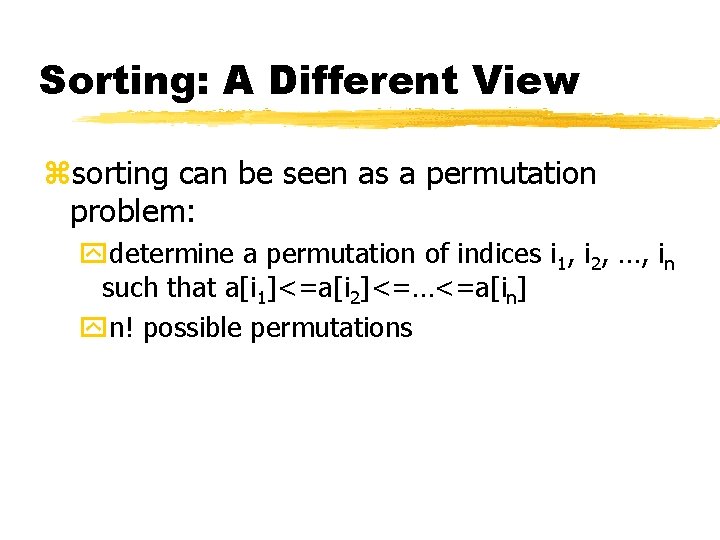
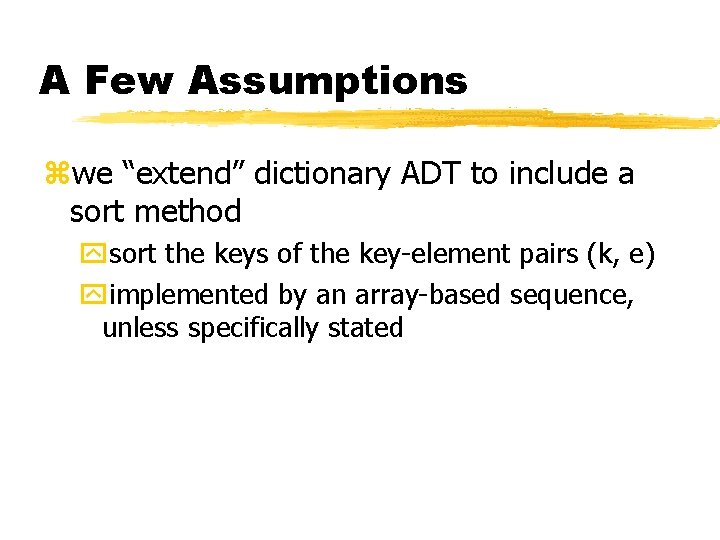
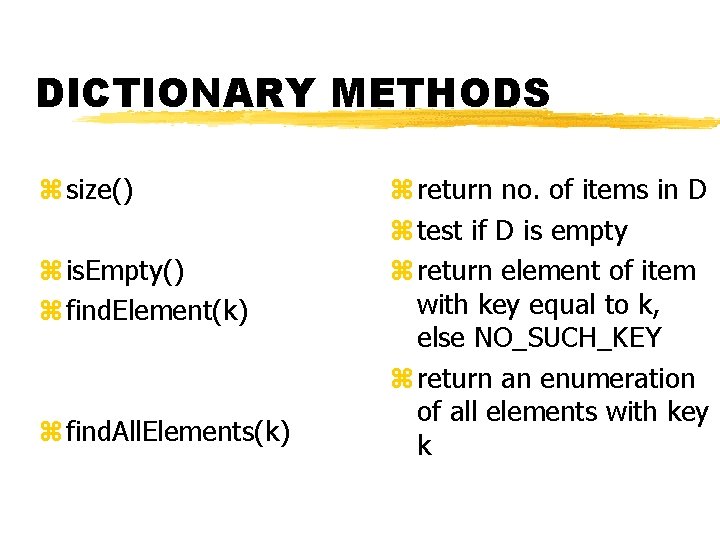
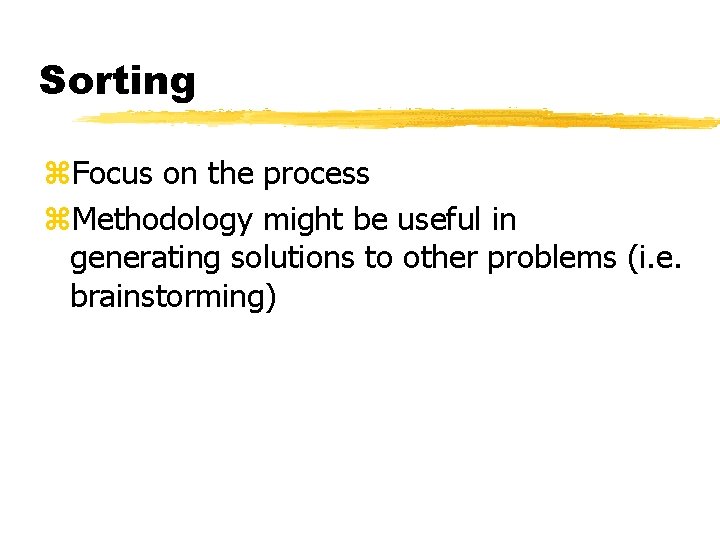
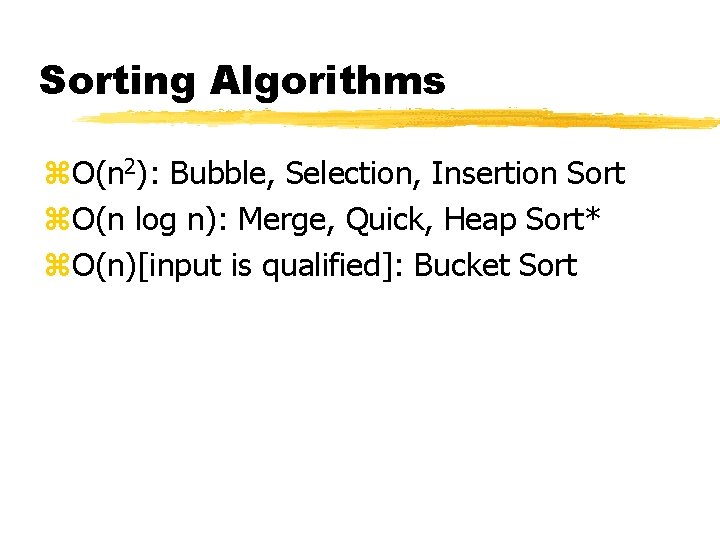
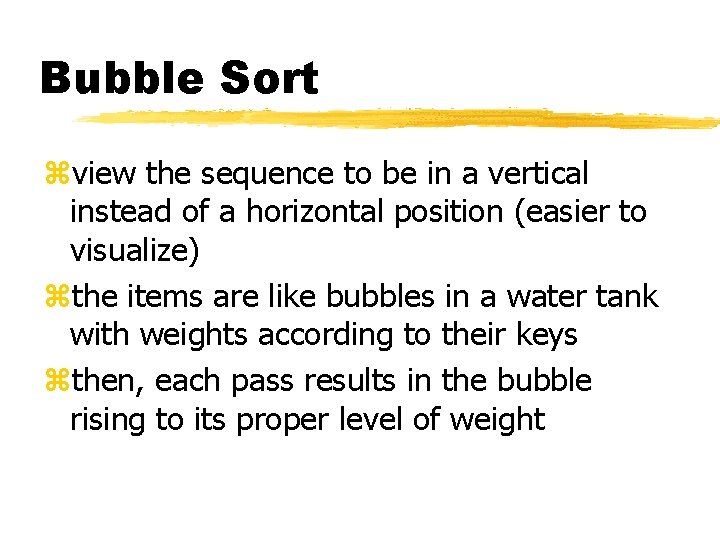
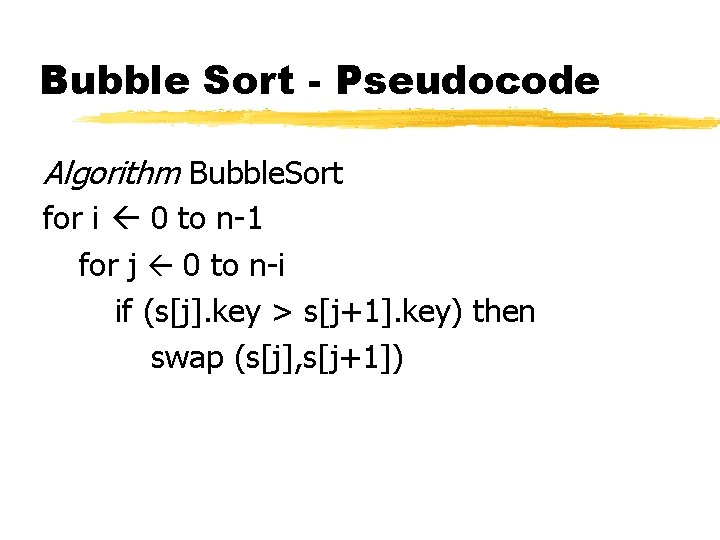
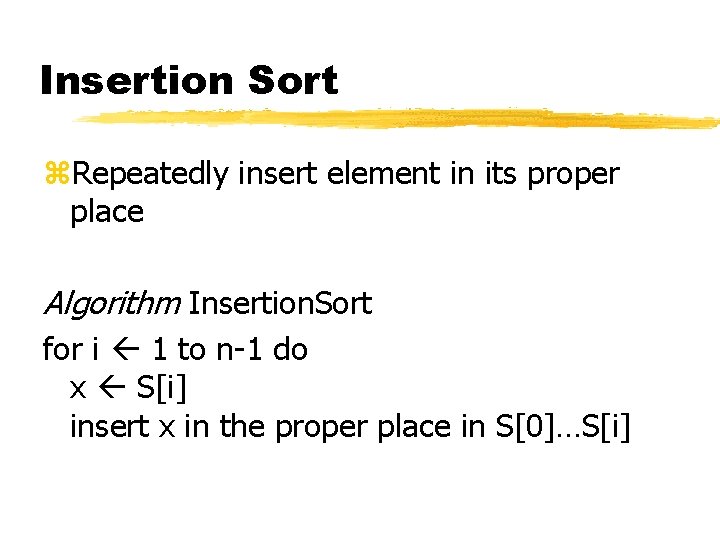
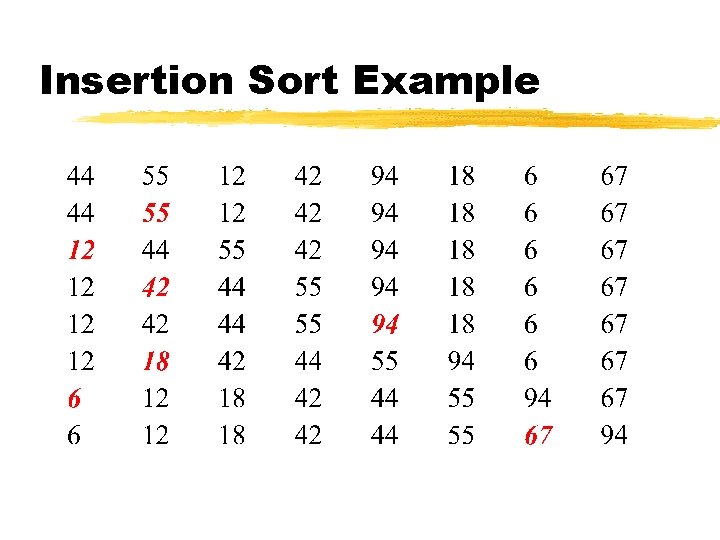
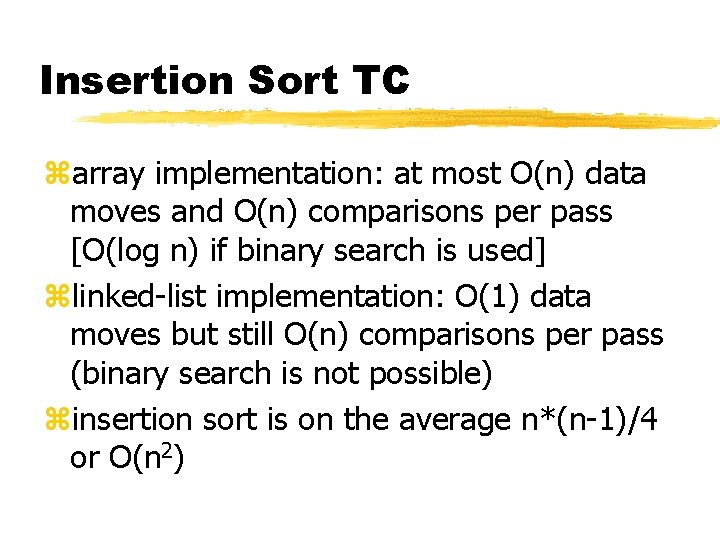
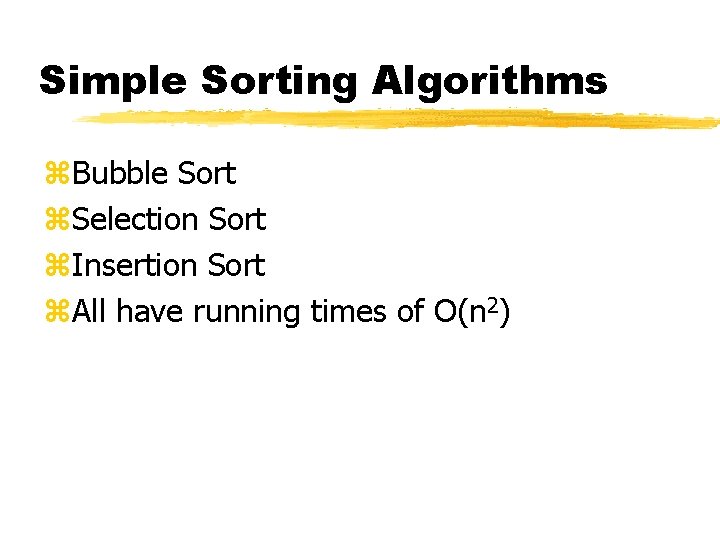
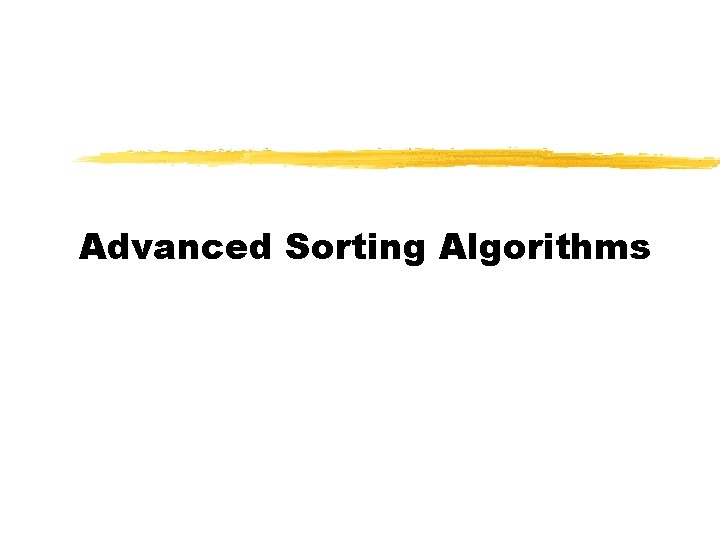
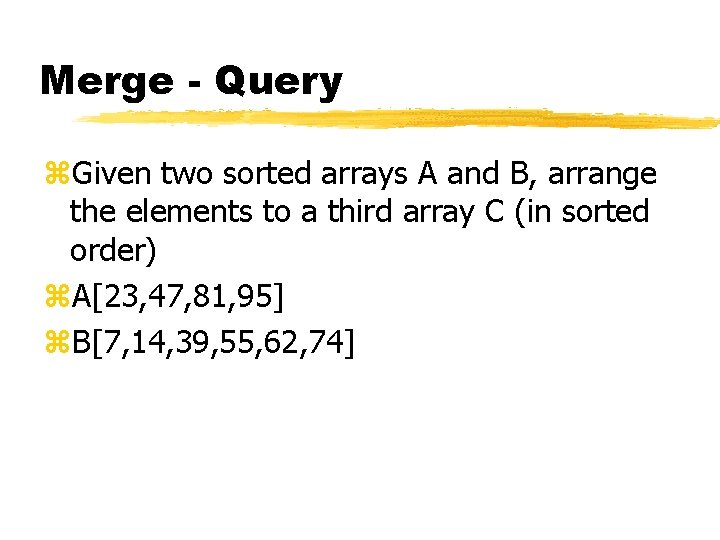
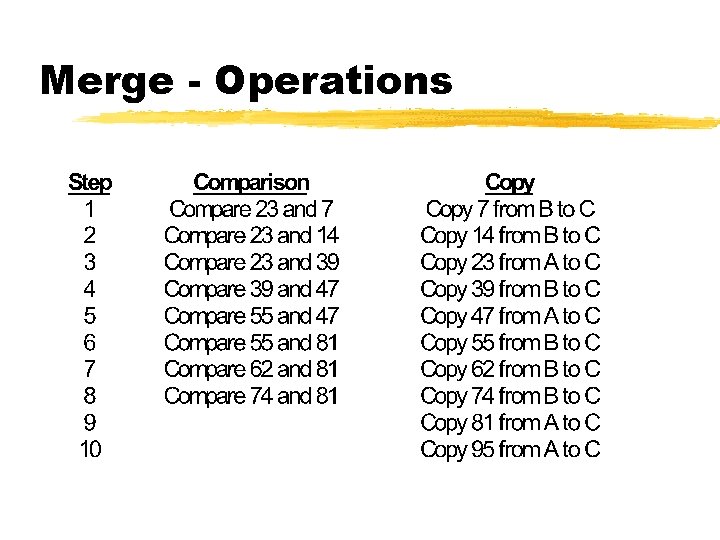
![Merged List z. A[23, 47, 81, 95] z. B[7, 14, 39, 55, 62, 74] Merged List z. A[23, 47, 81, 95] z. B[7, 14, 39, 55, 62, 74]](https://slidetodoc.com/presentation_image_h/0923c9967924211b845c80e7d8dc95f3/image-18.jpg)
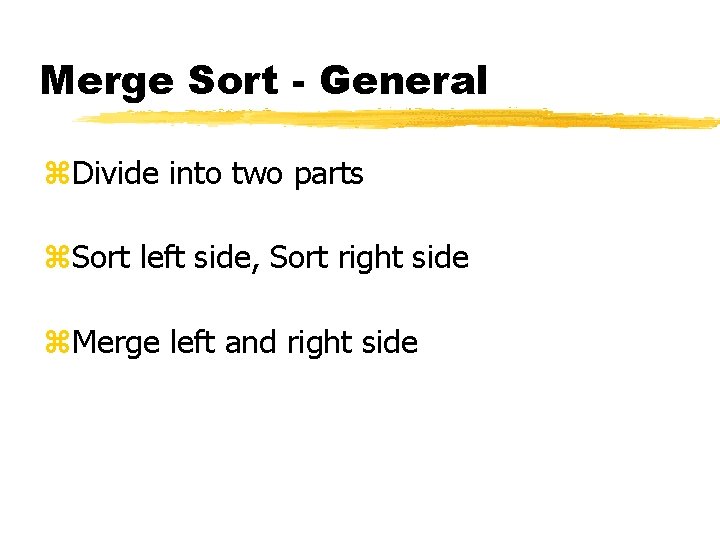
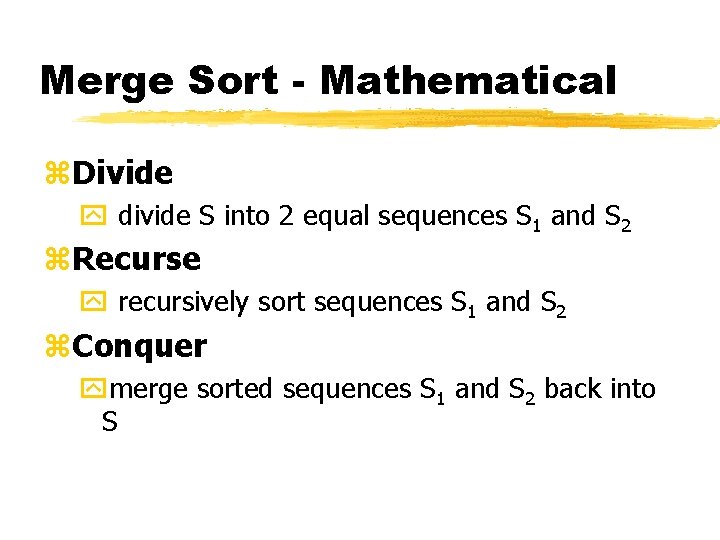
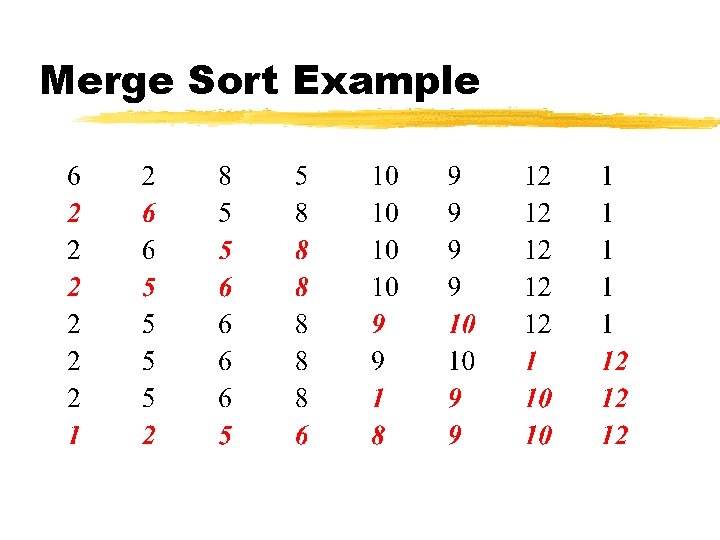
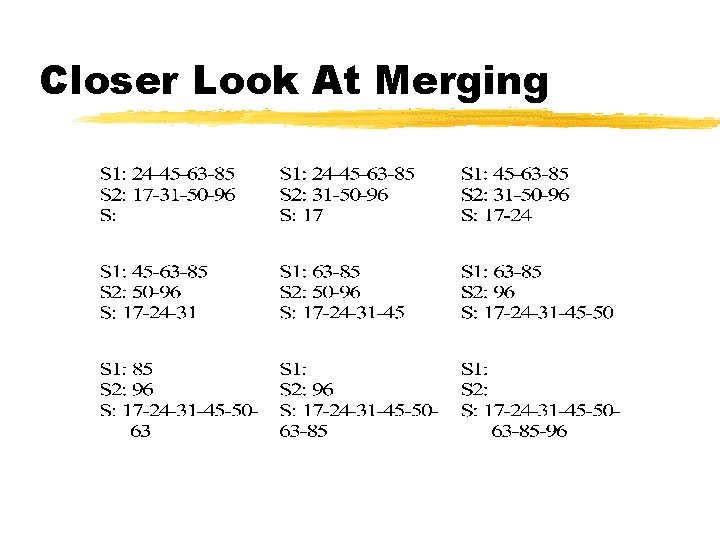
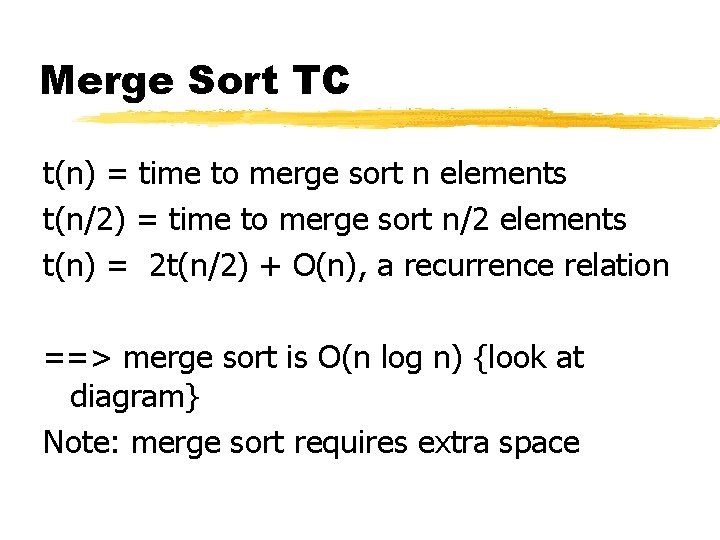
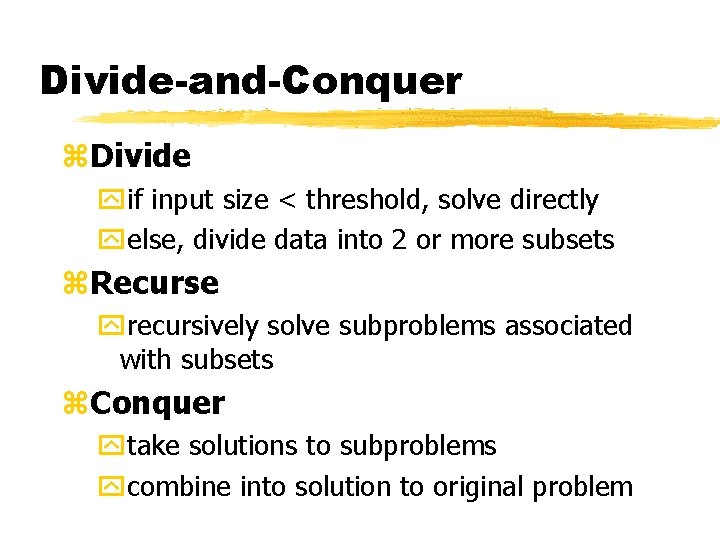
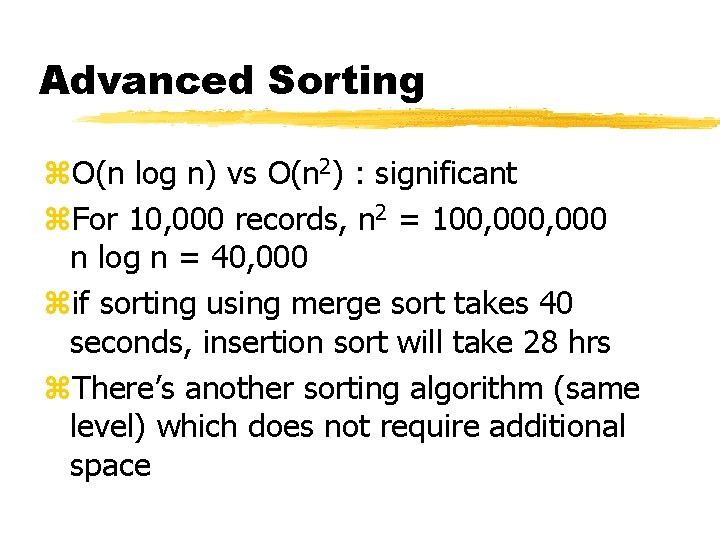
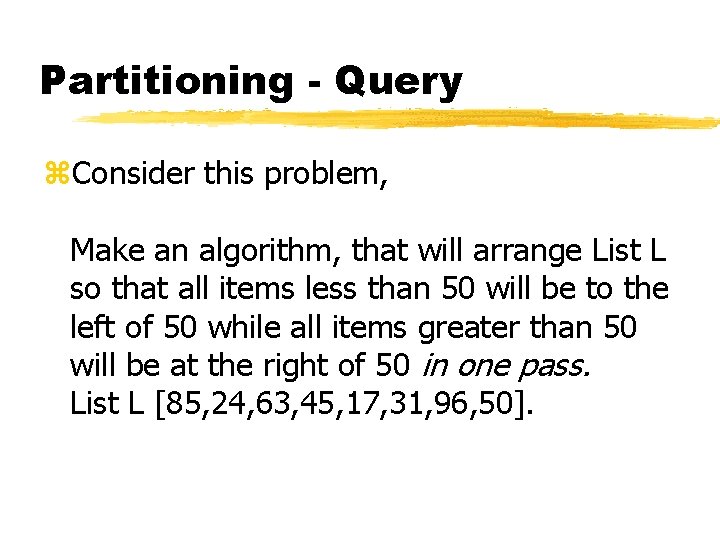
![Partitioning z Create pointer at both ends of the list z If a[0]<50, then Partitioning z Create pointer at both ends of the list z If a[0]<50, then](https://slidetodoc.com/presentation_image_h/0923c9967924211b845c80e7d8dc95f3/image-27.jpg)
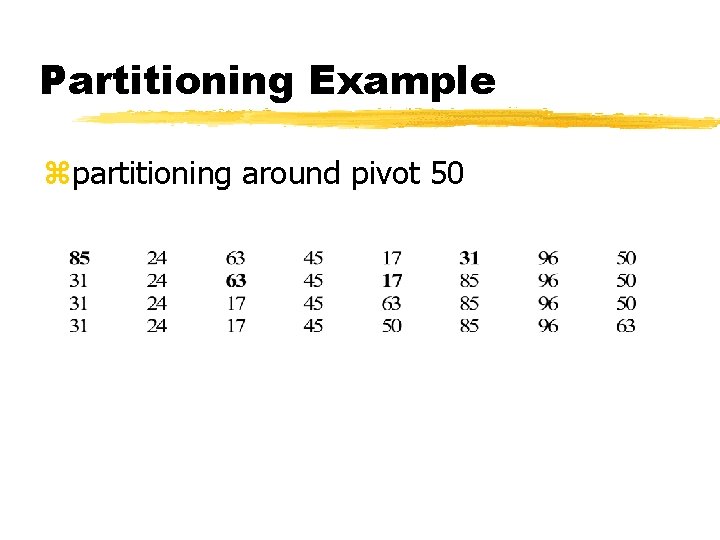
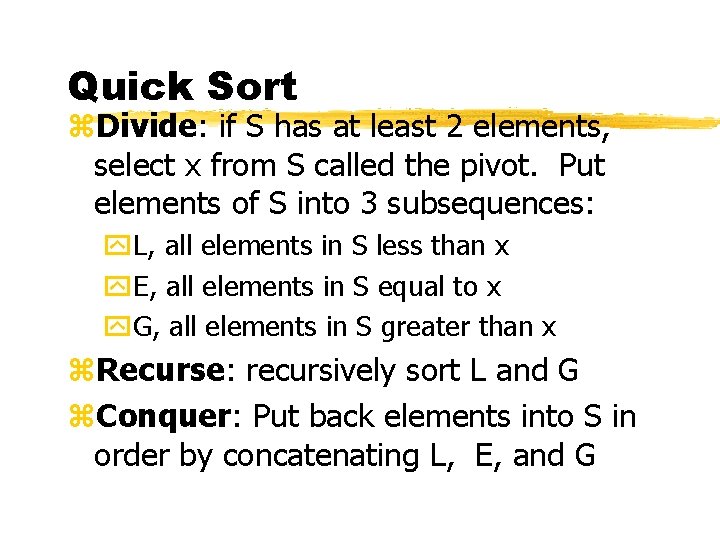
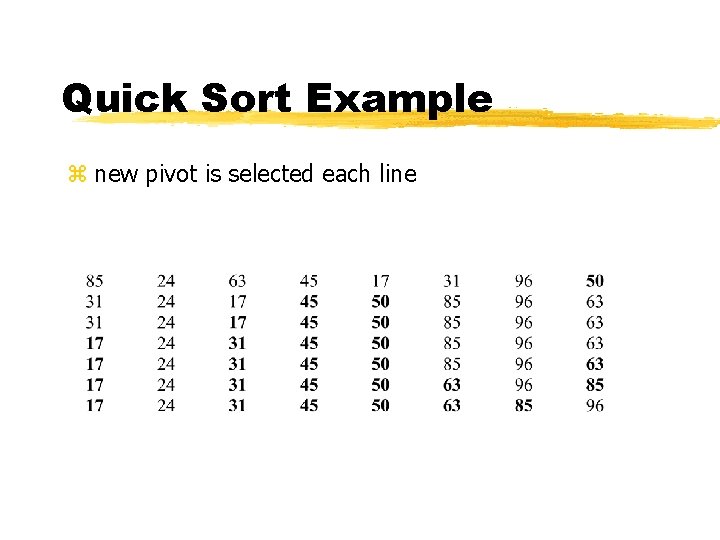
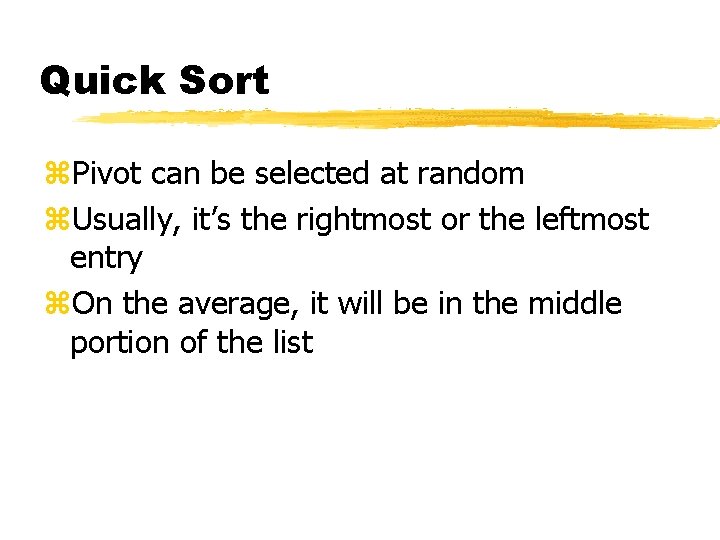
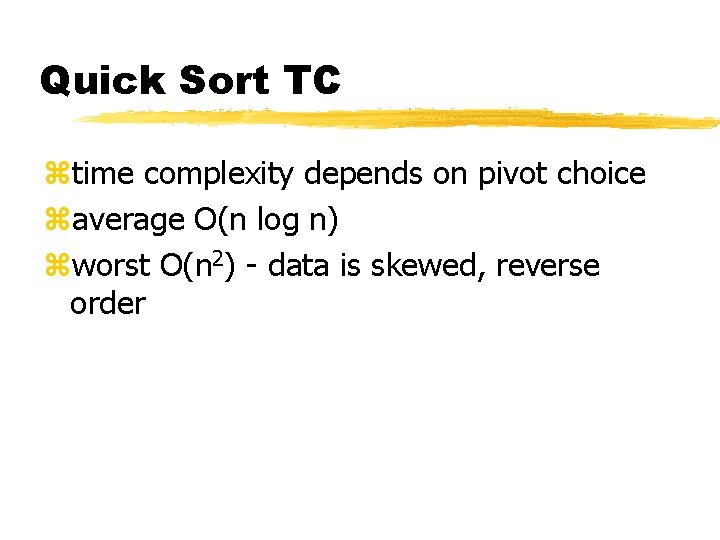
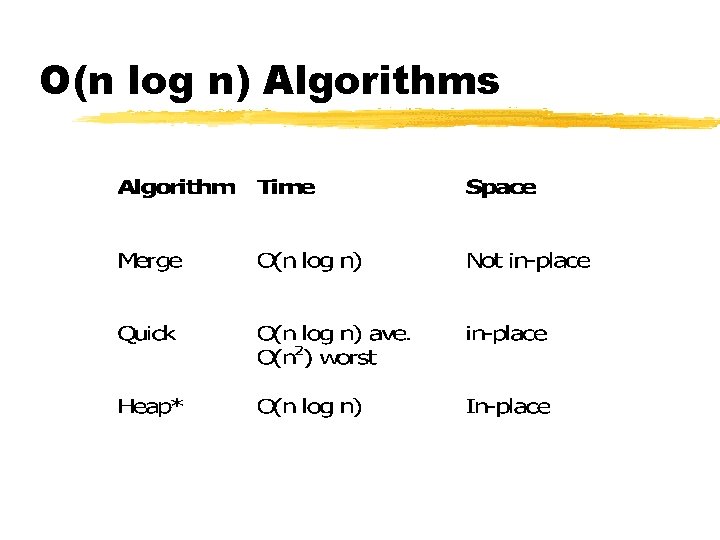
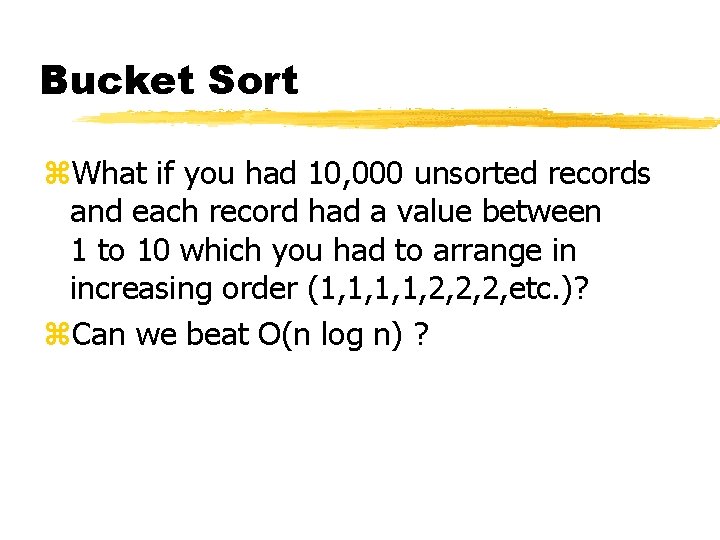
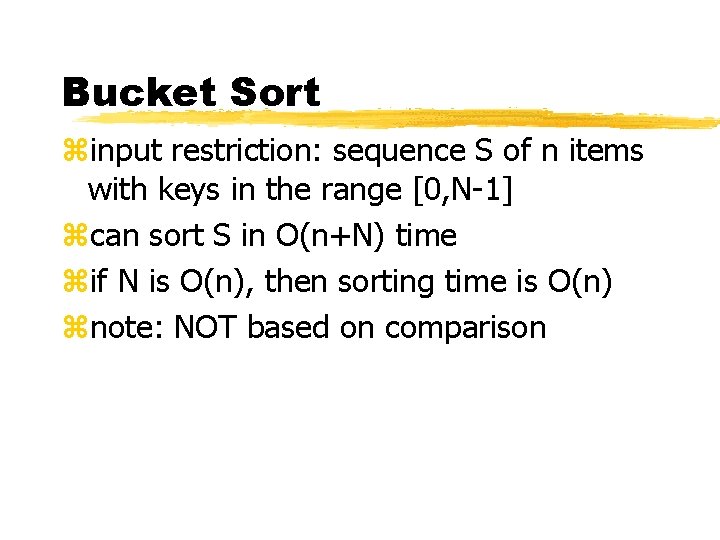
![Pseudocode Algorithm Bucket. Sort Input: Sequence S with integer keys in range [0, N-1] Pseudocode Algorithm Bucket. Sort Input: Sequence S with integer keys in range [0, N-1]](https://slidetodoc.com/presentation_image_h/0923c9967924211b845c80e7d8dc95f3/image-36.jpg)
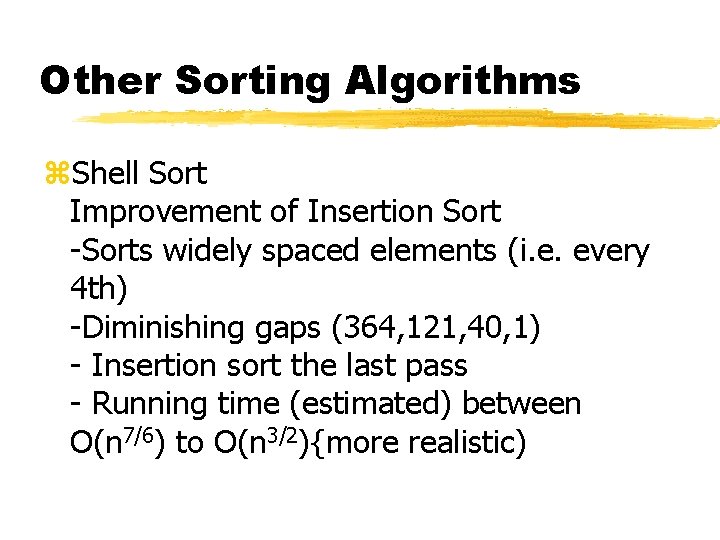
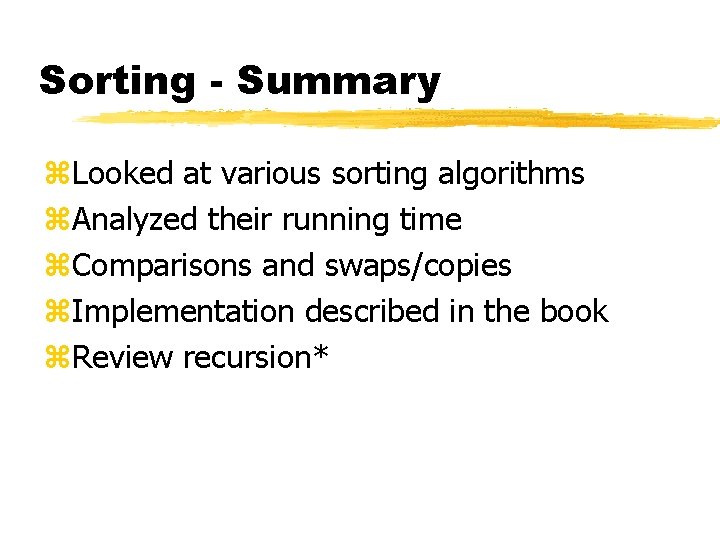
- Slides: 38
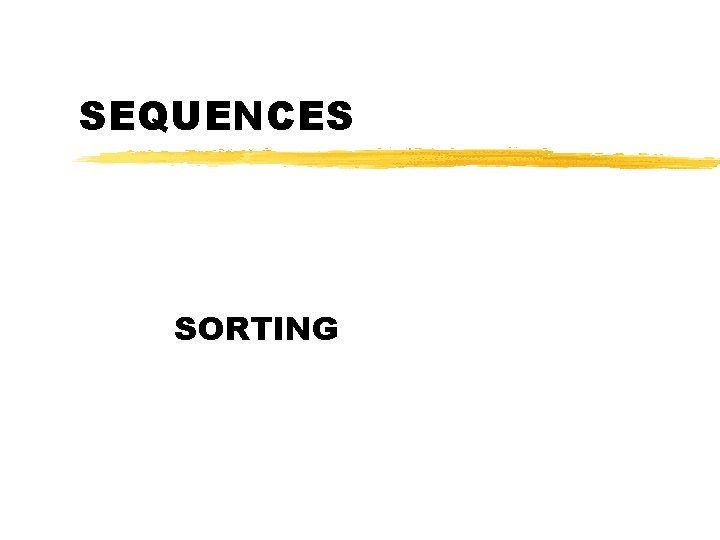
SEQUENCES SORTING
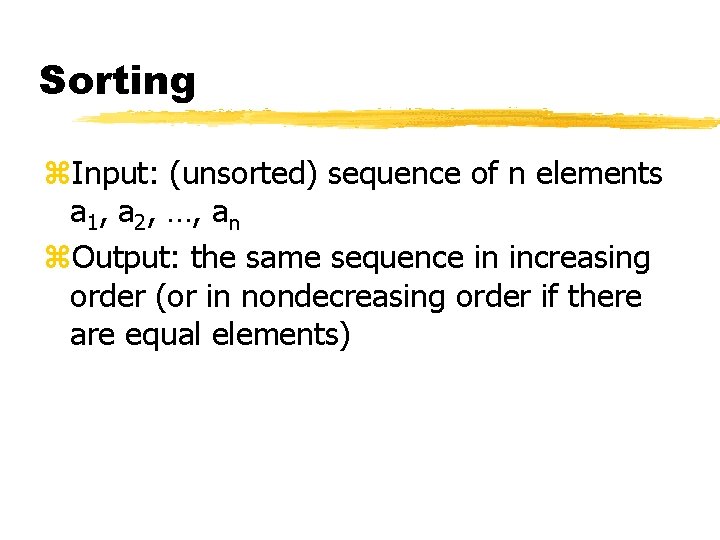
Sorting z. Input: (unsorted) sequence of n elements a 1, a 2, …, an z. Output: the same sequence in increasing order (or in nondecreasing order if there are equal elements)
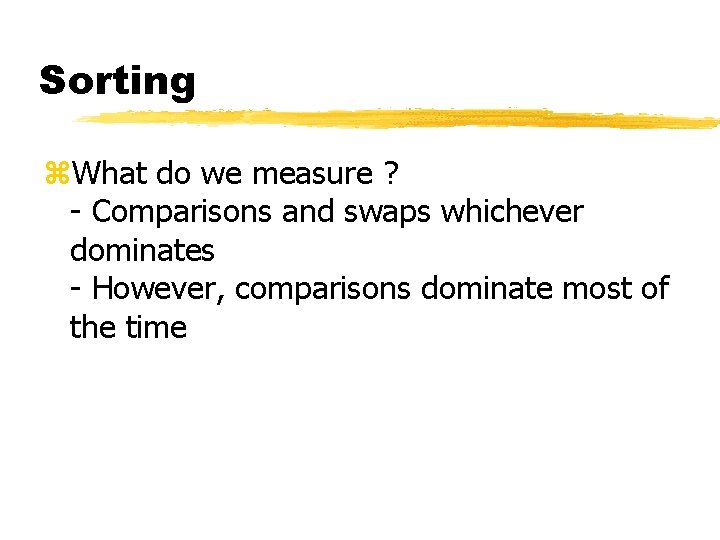
Sorting z. What do we measure ? - Comparisons and swaps whichever dominates - However, comparisons dominate most of the time
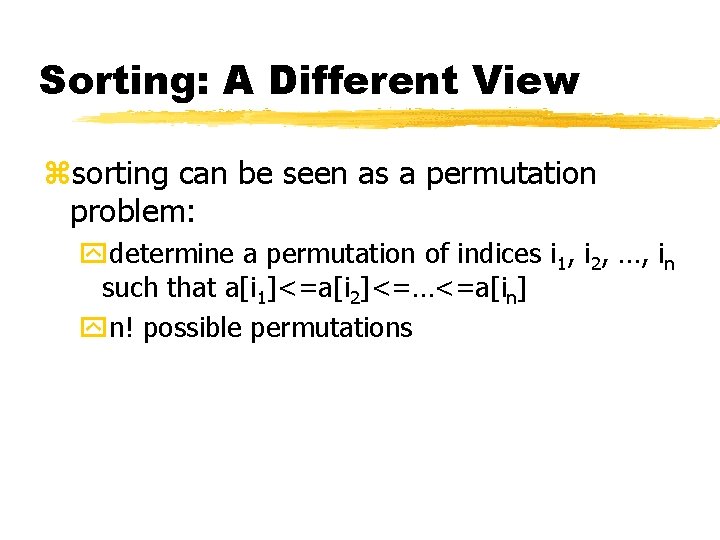
Sorting: A Different View zsorting can be seen as a permutation problem: ydetermine a permutation of indices i 1, i 2, …, in such that a[i 1]<=a[i 2]<=…<=a[in] yn! possible permutations
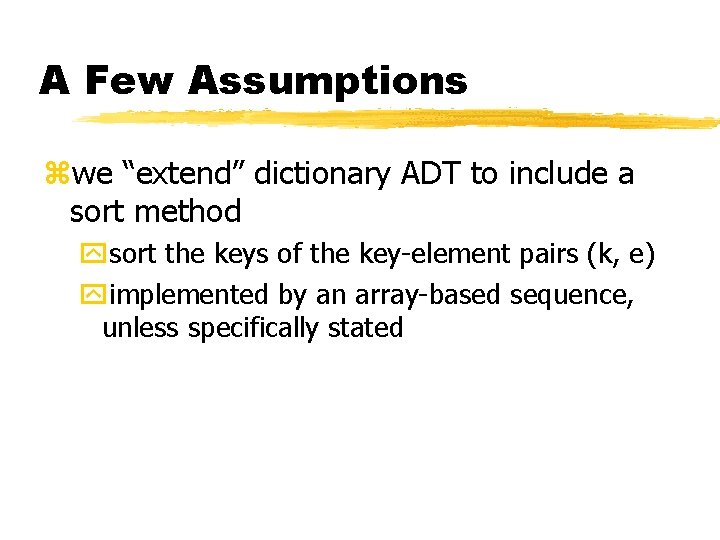
A Few Assumptions zwe “extend” dictionary ADT to include a sort method ysort the keys of the key-element pairs (k, e) yimplemented by an array-based sequence, unless specifically stated
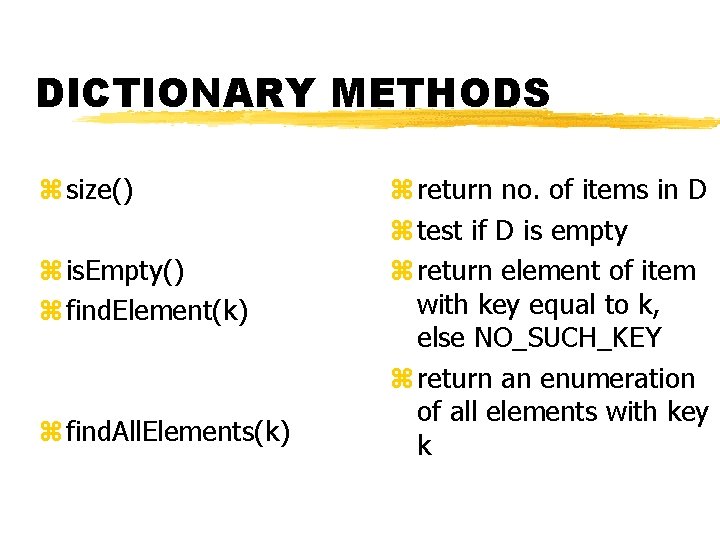
DICTIONARY METHODS z size() z is. Empty() z find. Element(k) z find. All. Elements(k) z return no. of items in D z test if D is empty z return element of item with key equal to k, else NO_SUCH_KEY z return an enumeration of all elements with key k
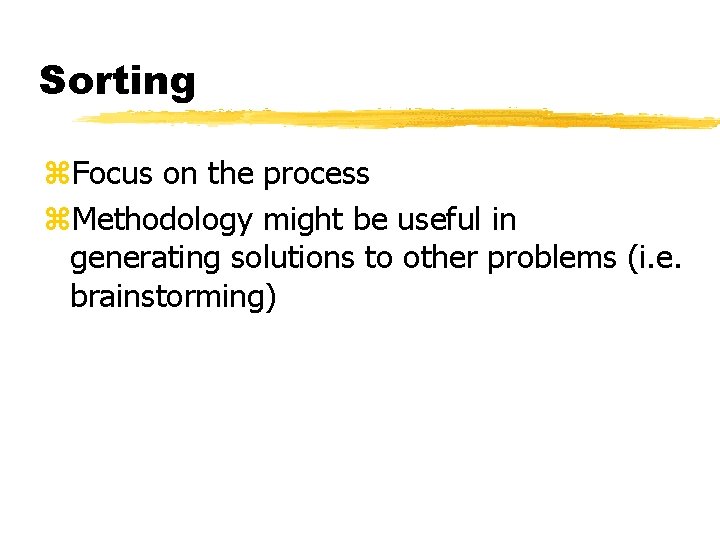
Sorting z. Focus on the process z. Methodology might be useful in generating solutions to other problems (i. e. brainstorming)
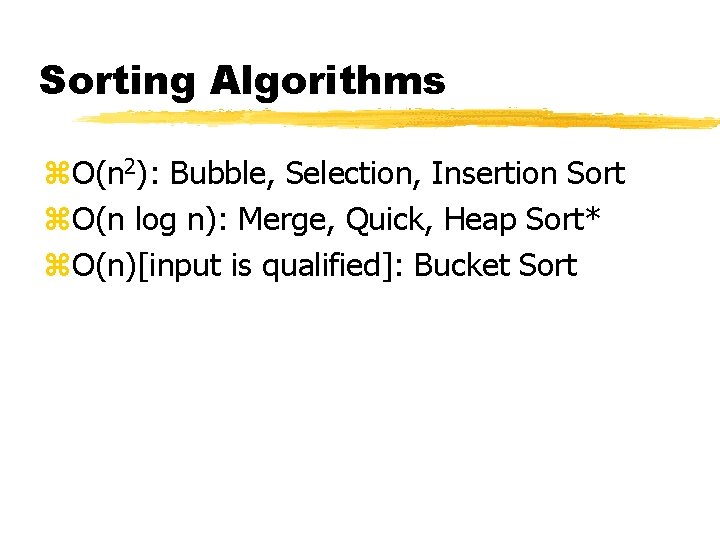
Sorting Algorithms z. O(n 2): Bubble, Selection, Insertion Sort z. O(n log n): Merge, Quick, Heap Sort* z. O(n)[input is qualified]: Bucket Sort
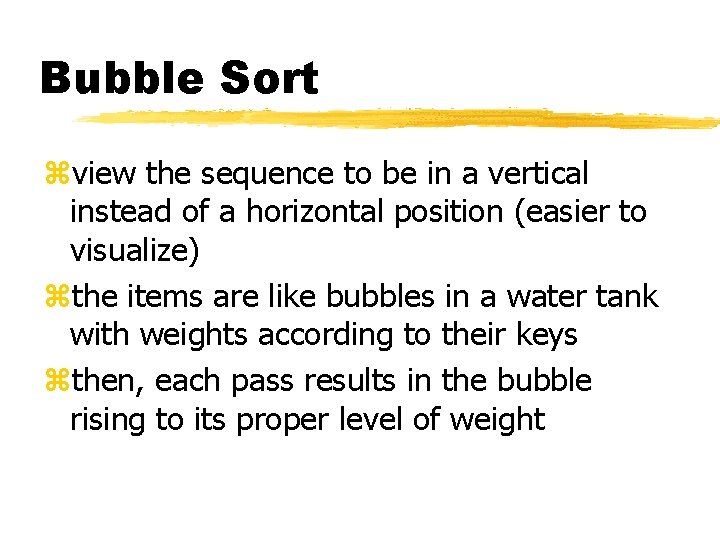
Bubble Sort zview the sequence to be in a vertical instead of a horizontal position (easier to visualize) zthe items are like bubbles in a water tank with weights according to their keys zthen, each pass results in the bubble rising to its proper level of weight
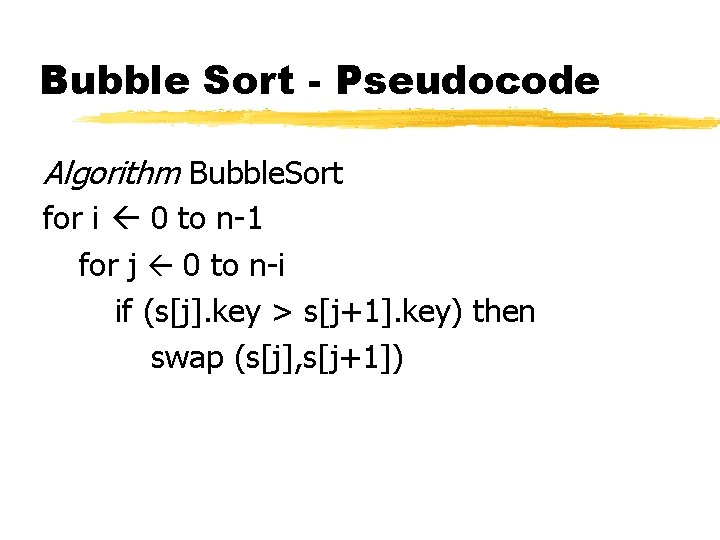
Bubble Sort - Pseudocode Algorithm Bubble. Sort for i 0 to n-1 for j 0 to n-i if (s[j]. key > s[j+1]. key) then swap (s[j], s[j+1])
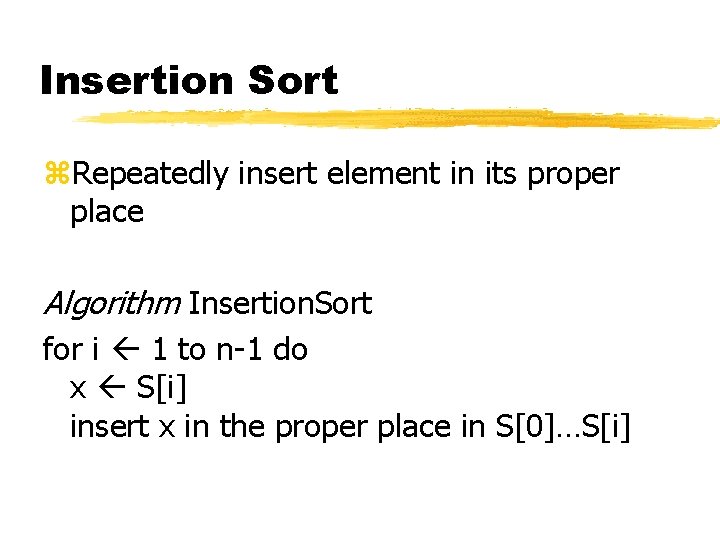
Insertion Sort z. Repeatedly insert element in its proper place Algorithm Insertion. Sort for i 1 to n-1 do x S[i] insert x in the proper place in S[0]…S[i]
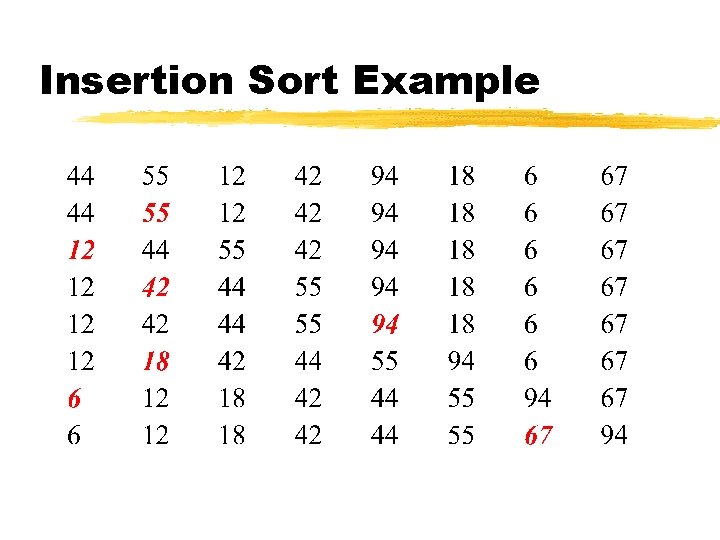
Insertion Sort Example
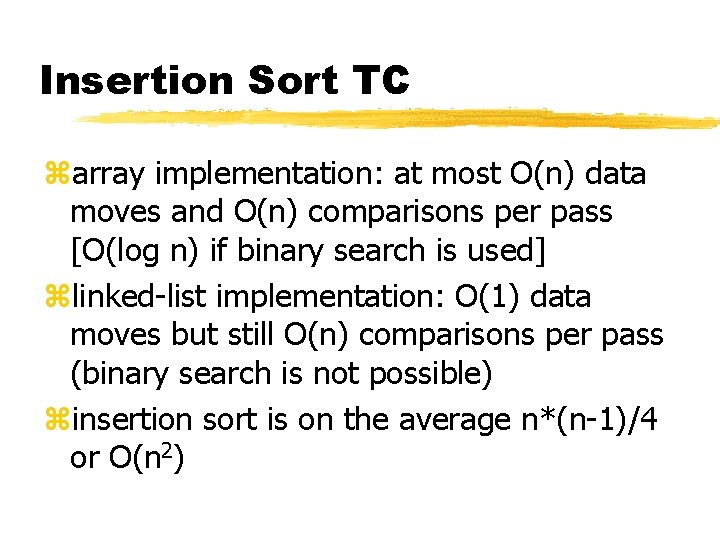
Insertion Sort TC zarray implementation: at most O(n) data moves and O(n) comparisons per pass [O(log n) if binary search is used] zlinked-list implementation: O(1) data moves but still O(n) comparisons per pass (binary search is not possible) zinsertion sort is on the average n*(n-1)/4 or O(n 2)
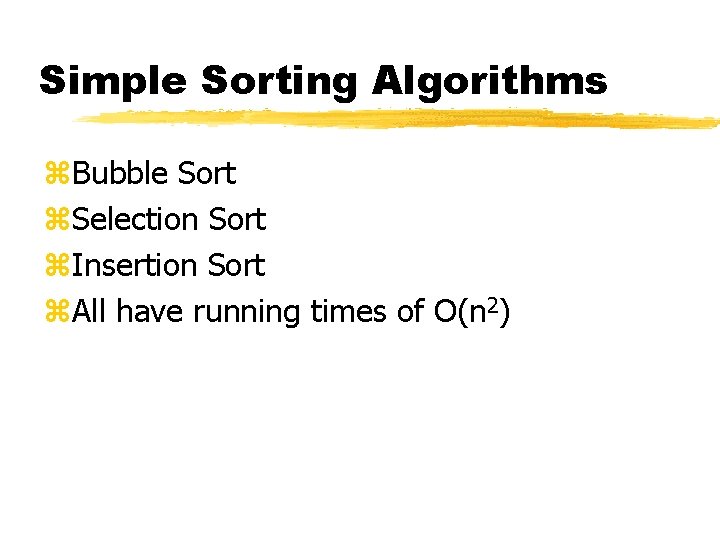
Simple Sorting Algorithms z. Bubble Sort z. Selection Sort z. Insertion Sort z. All have running times of O(n 2)
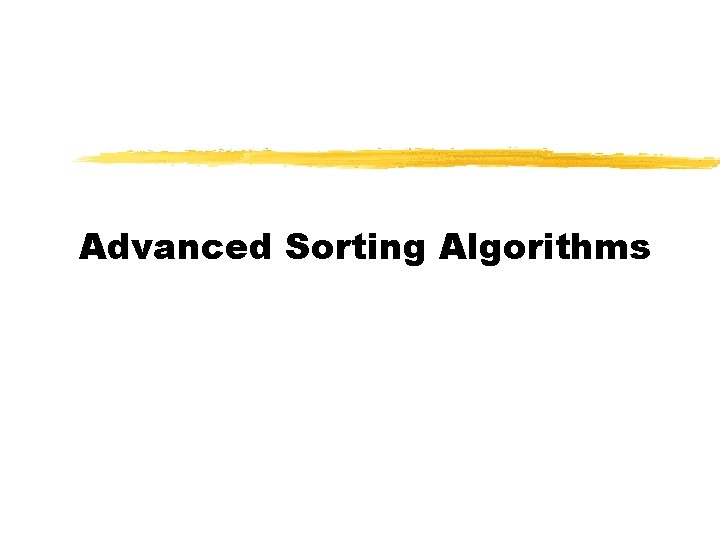
Advanced Sorting Algorithms
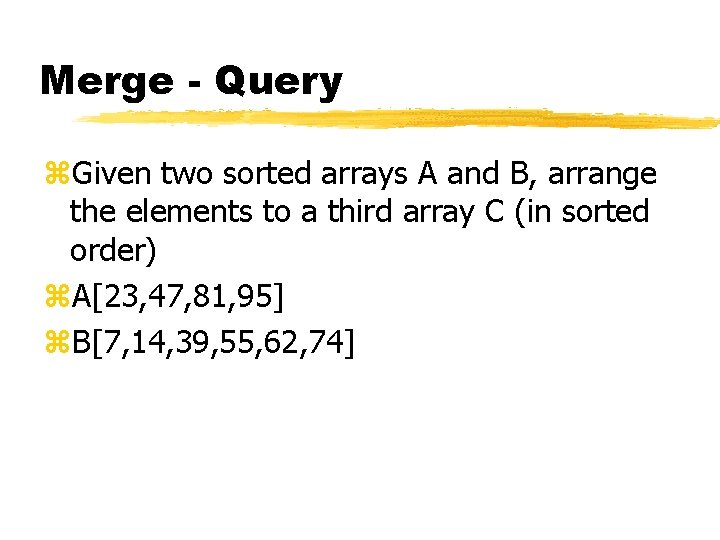
Merge - Query z. Given two sorted arrays A and B, arrange the elements to a third array C (in sorted order) z. A[23, 47, 81, 95] z. B[7, 14, 39, 55, 62, 74]
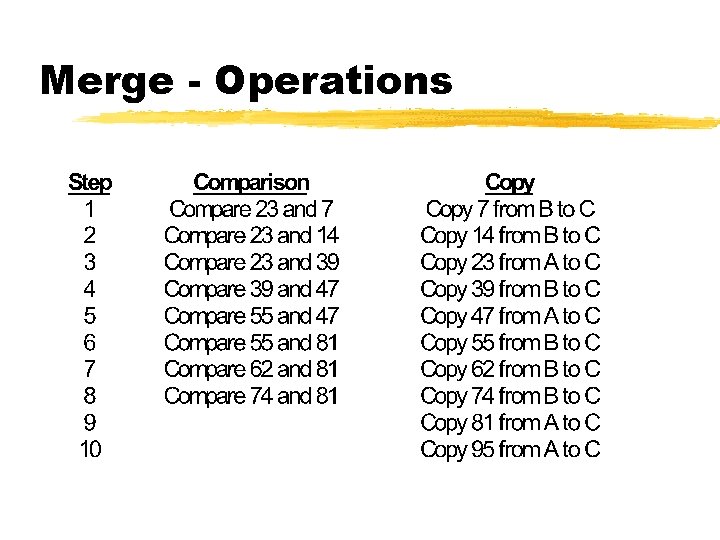
Merge - Operations
![Merged List z A23 47 81 95 z B7 14 39 55 62 74 Merged List z. A[23, 47, 81, 95] z. B[7, 14, 39, 55, 62, 74]](https://slidetodoc.com/presentation_image_h/0923c9967924211b845c80e7d8dc95f3/image-18.jpg)
Merged List z. A[23, 47, 81, 95] z. B[7, 14, 39, 55, 62, 74] z. C[7, 14, 23, 39, 47, 55, 62, 74, 81, 95]
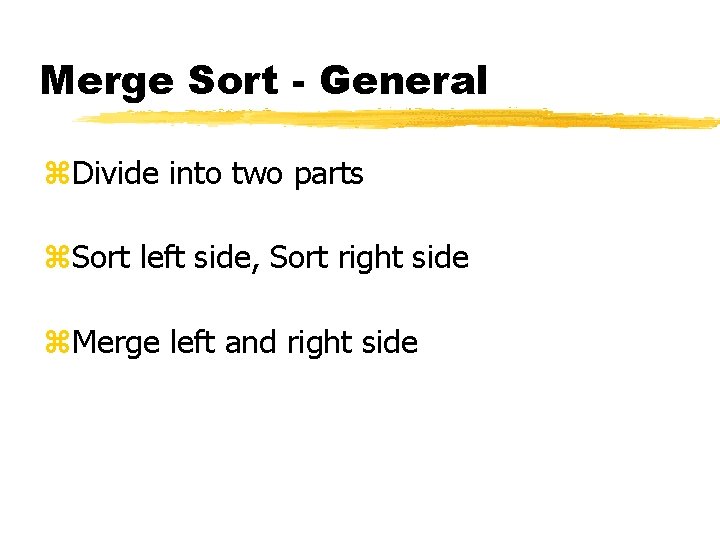
Merge Sort - General z. Divide into two parts z. Sort left side, Sort right side z. Merge left and right side
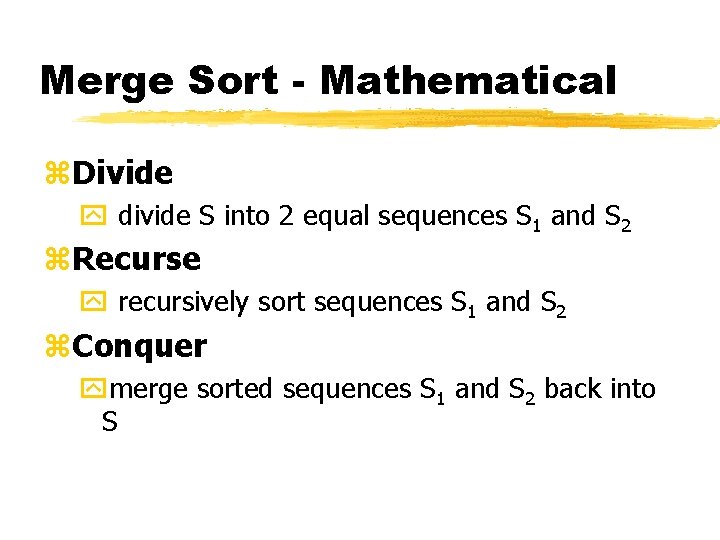
Merge Sort - Mathematical z. Divide y divide S into 2 equal sequences S 1 and S 2 z. Recurse y recursively sort sequences S 1 and S 2 z. Conquer ymerge sorted sequences S 1 and S 2 back into S
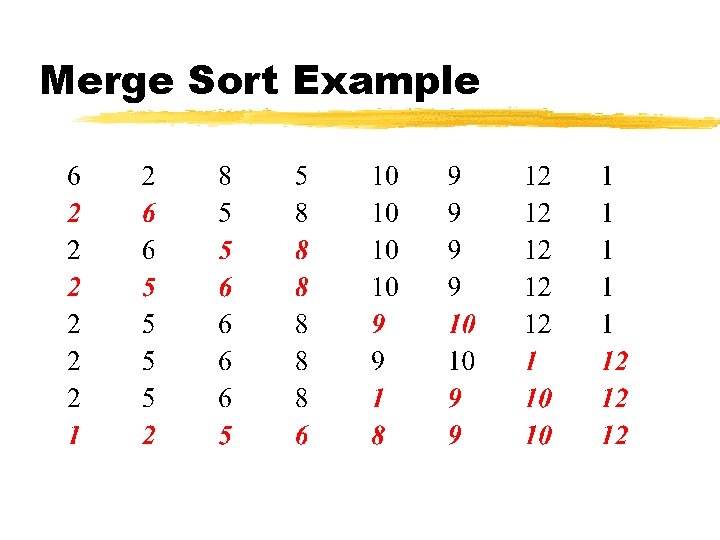
Merge Sort Example
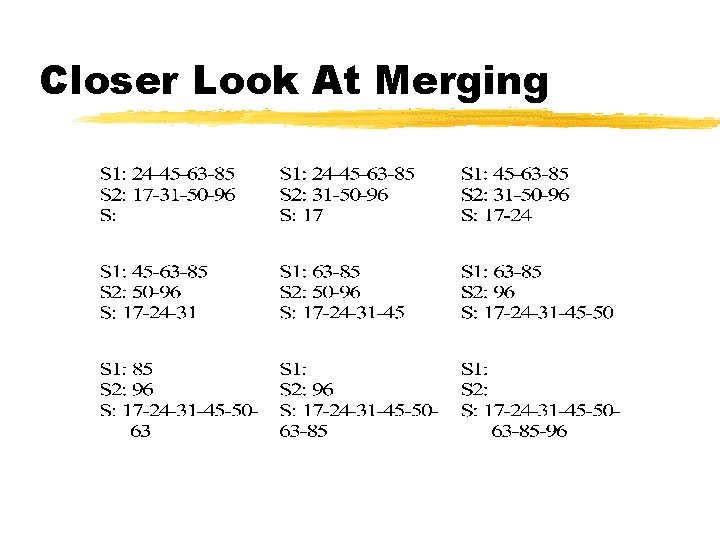
Closer Look At Merging
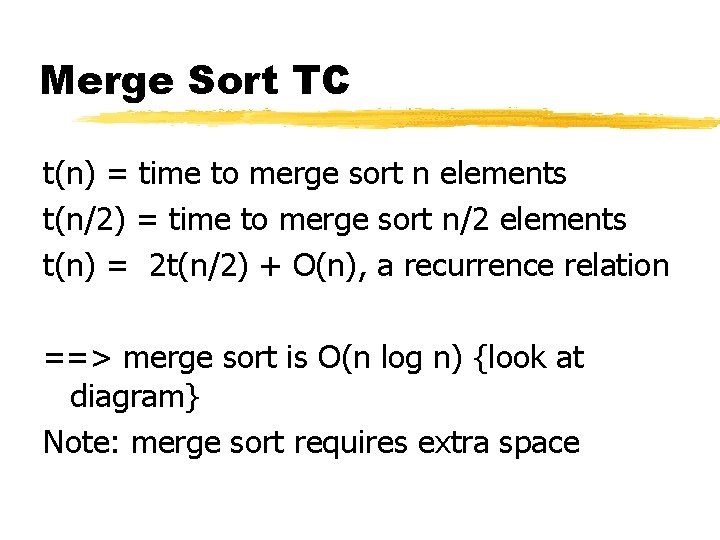
Merge Sort TC t(n) = time to merge sort n elements t(n/2) = time to merge sort n/2 elements t(n) = 2 t(n/2) + O(n), a recurrence relation ==> merge sort is O(n log n) {look at diagram} Note: merge sort requires extra space
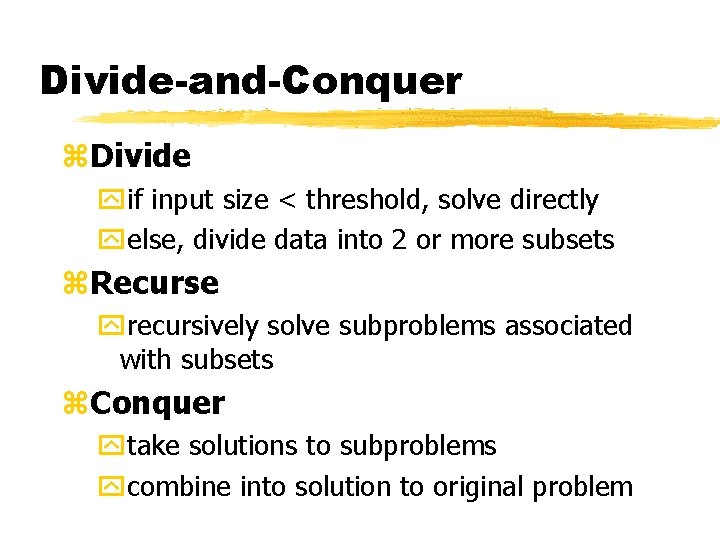
Divide-and-Conquer z. Divide yif input size < threshold, solve directly yelse, divide data into 2 or more subsets z. Recurse yrecursively solve subproblems associated with subsets z. Conquer ytake solutions to subproblems ycombine into solution to original problem
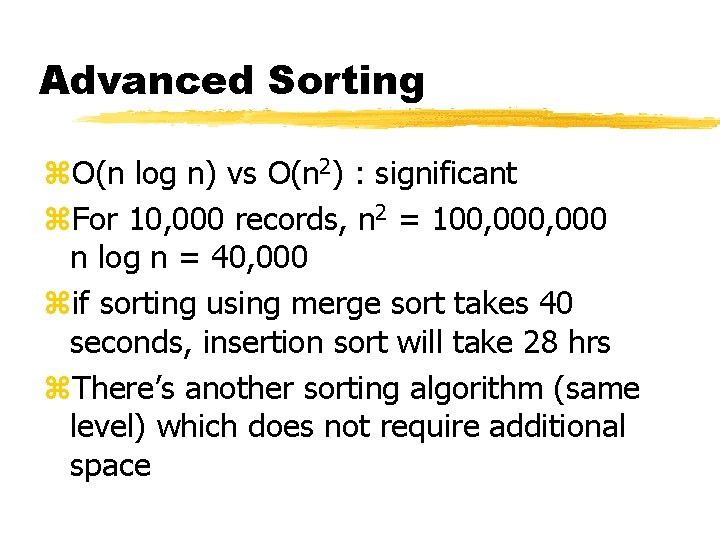
Advanced Sorting z. O(n log n) vs O(n 2) : significant z. For 10, 000 records, n 2 = 100, 000 n log n = 40, 000 zif sorting using merge sort takes 40 seconds, insertion sort will take 28 hrs z. There’s another sorting algorithm (same level) which does not require additional space
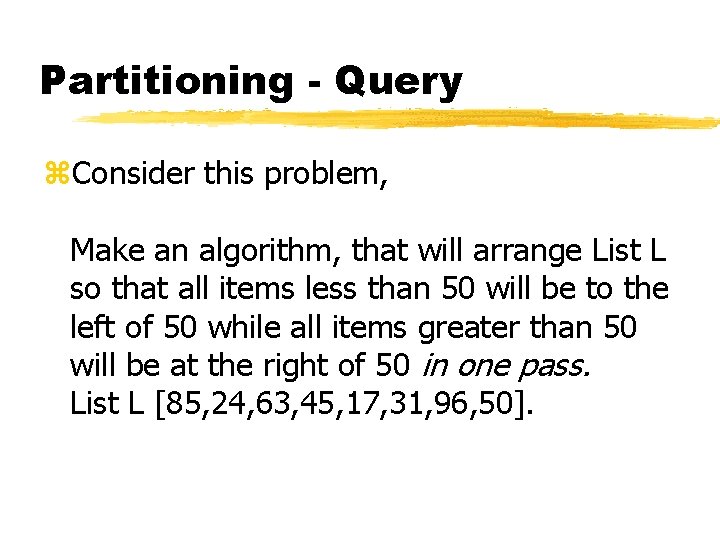
Partitioning - Query z. Consider this problem, Make an algorithm, that will arrange List L so that all items less than 50 will be to the left of 50 while all items greater than 50 will be at the right of 50 in one pass. List L [85, 24, 63, 45, 17, 31, 96, 50].
![Partitioning z Create pointer at both ends of the list z If a050 then Partitioning z Create pointer at both ends of the list z If a[0]<50, then](https://slidetodoc.com/presentation_image_h/0923c9967924211b845c80e7d8dc95f3/image-27.jpg)
Partitioning z Create pointer at both ends of the list z If a[0]<50, then it is in the right position, else (wrong position) stop and wait for the right side to find a wrong entry If a[n]>50 then it is in the right position else (wrong position) stop and wait for the right side to find a wrong entry If both wrong entries, swap
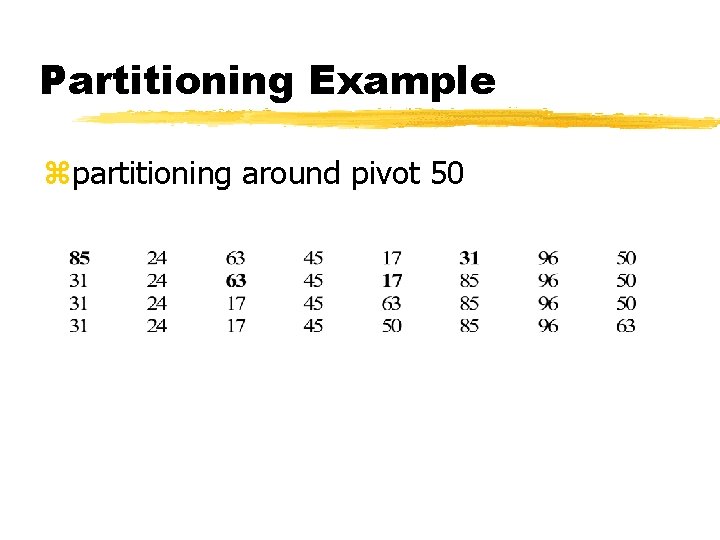
Partitioning Example zpartitioning around pivot 50
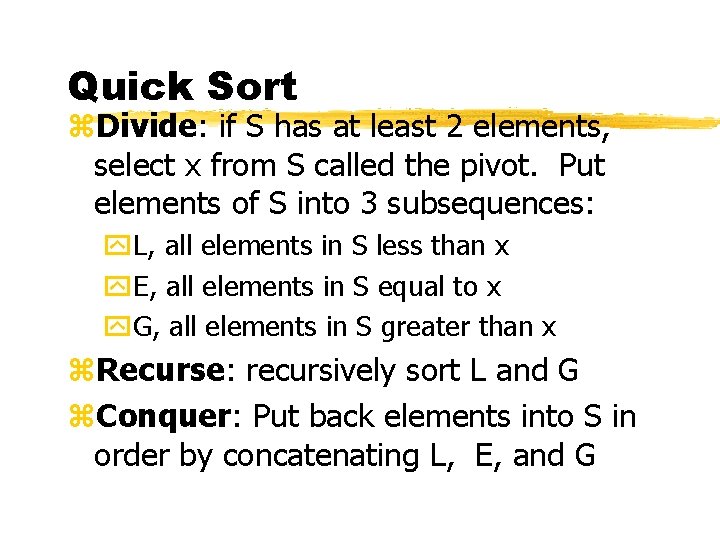
Quick Sort z. Divide: if S has at least 2 elements, select x from S called the pivot. Put elements of S into 3 subsequences: y. L, all elements in S less than x y. E, all elements in S equal to x y. G, all elements in S greater than x z. Recurse: recursively sort L and G z. Conquer: Put back elements into S in order by concatenating L, E, and G
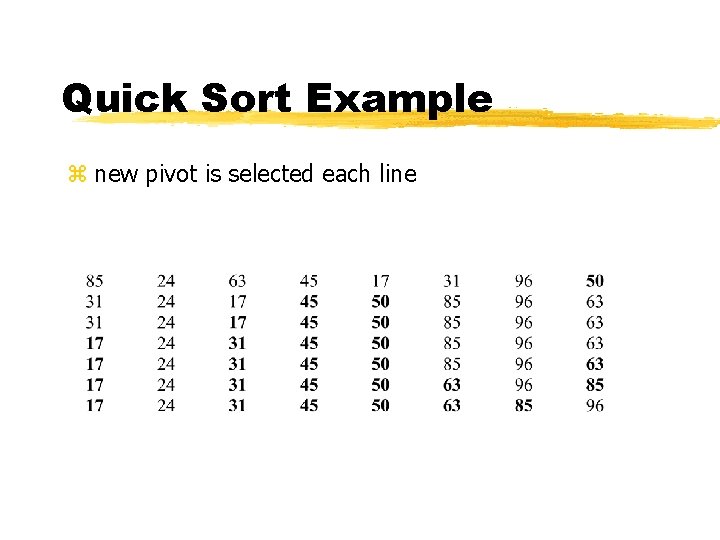
Quick Sort Example z new pivot is selected each line
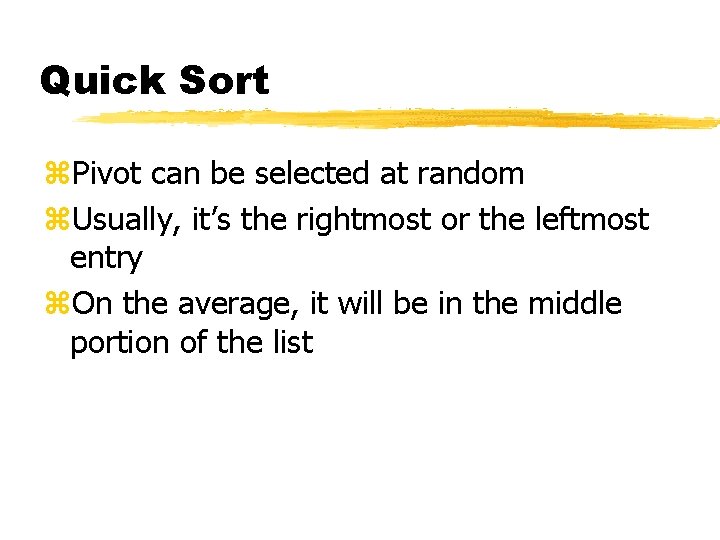
Quick Sort z. Pivot can be selected at random z. Usually, it’s the rightmost or the leftmost entry z. On the average, it will be in the middle portion of the list
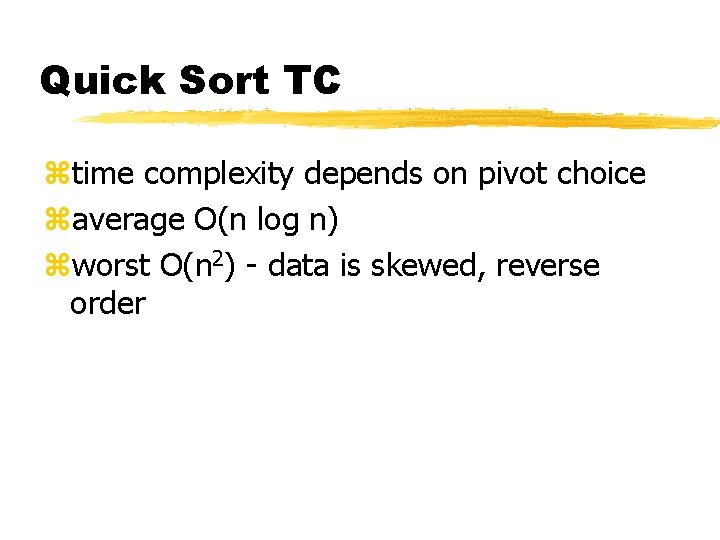
Quick Sort TC ztime complexity depends on pivot choice zaverage O(n log n) zworst O(n 2) - data is skewed, reverse order
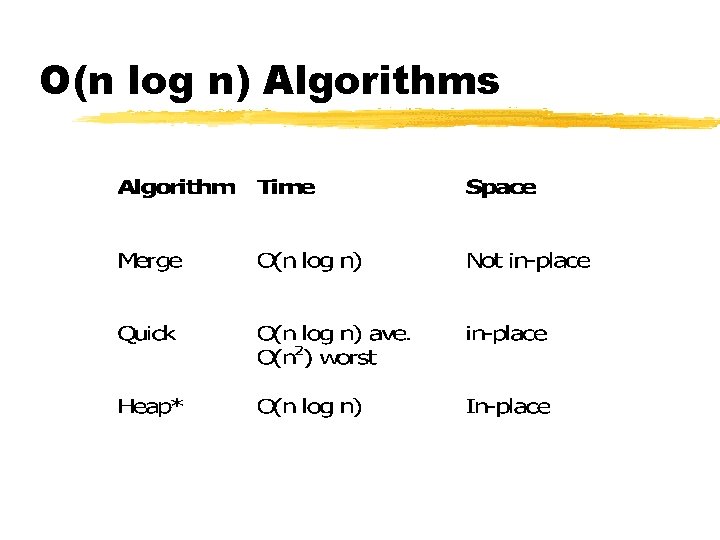
O(n log n) Algorithms
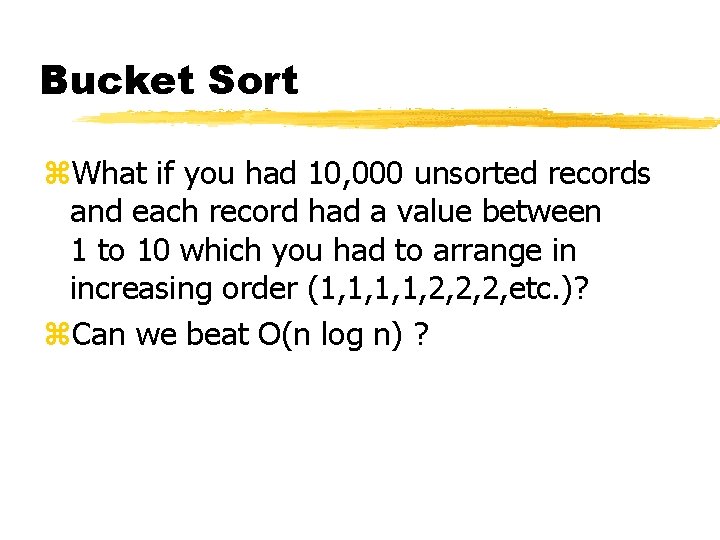
Bucket Sort z. What if you had 10, 000 unsorted records and each record had a value between 1 to 10 which you had to arrange in increasing order (1, 1, 2, 2, 2, etc. )? z. Can we beat O(n log n) ?
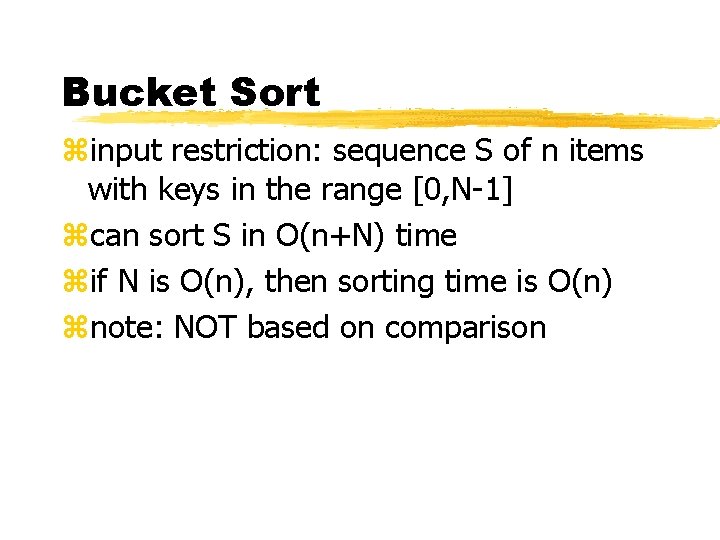
Bucket Sort zinput restriction: sequence S of n items with keys in the range [0, N-1] zcan sort S in O(n+N) time zif N is O(n), then sorting time is O(n) znote: NOT based on comparison
![Pseudocode Algorithm Bucket Sort Input Sequence S with integer keys in range 0 N1 Pseudocode Algorithm Bucket. Sort Input: Sequence S with integer keys in range [0, N-1]](https://slidetodoc.com/presentation_image_h/0923c9967924211b845c80e7d8dc95f3/image-36.jpg)
Pseudocode Algorithm Bucket. Sort Input: Sequence S with integer keys in range [0, N-1] Output: S sorted let B=array of N initially empty sequences for each item x in S do k=key of x remove x from S and insert at end of sequence B[k] for i 0 to N-1 do for each item x in sequence B[i] do remove x from B[i] and insert at end of S
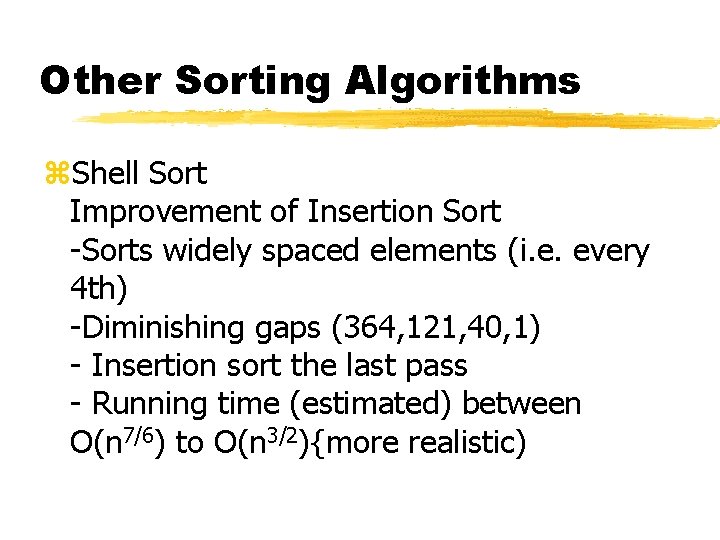
Other Sorting Algorithms z. Shell Sort Improvement of Insertion Sort -Sorts widely spaced elements (i. e. every 4 th) -Diminishing gaps (364, 121, 40, 1) - Insertion sort the last pass - Running time (estimated) between O(n 7/6) to O(n 3/2){more realistic)
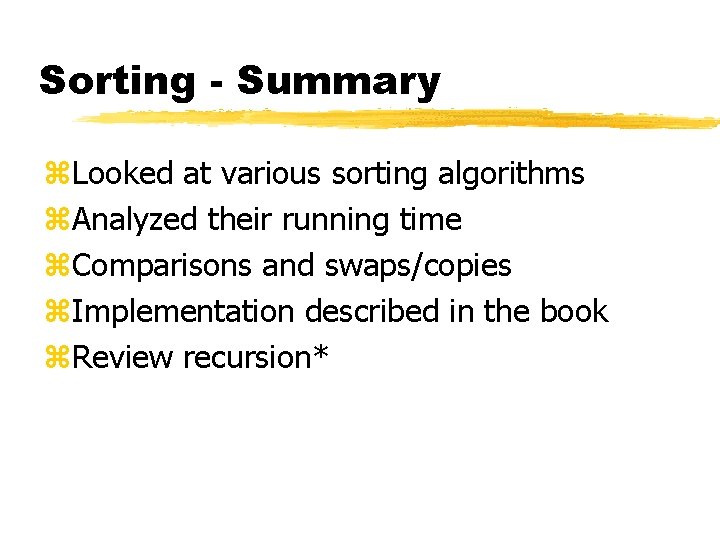
Sorting - Summary z. Looked at various sorting algorithms z. Analyzed their running time z. Comparisons and swaps/copies z. Implementation described in the book z. Review recursion*
Internal and external sort
A low hill is composed of unsorted sediments
Unsorted angular rough surface cobbles
What are the two main types of glaciers
Artem bityutskiy
Roughly-tuned input definition
Yang termasuk dalam perangkat peripheral input adalah ...
Convolutional sequence to sequence learning.
Amino acid nucleotide
What is selection in pseudocode
Difference between finite and infinite series
Restriction enzymes
Diatonic sequence
Math 20-1 sequences and series
Patterns and sequences module 4
Screenwriting 8 sequences
Sum of gp formula
P2ot
9-1 geometric sequences
Linear sequences dan multiple menus
Dna sequences
Unit 10 sequences and series homework 1 answers
Geometric sequence equation
Alignement multiple de séquences
10-2 arithmetic sequences and series
Arithmetic and geometric sequences and series
Write variable expressions for arithmetic sequences
Limits of sequences
Nth term of a sequence
Rock sequences
Modeling with arithmetic sequences
9 + 1 = 10
Recursive and explicit formula
Example of geometric sequence
Concatenation discrete math
Introducution
Formulas for geometric sequences
Geometric sequences formula
Power of reading teaching sequences