Operations and Arithmetic Operations in C Have the
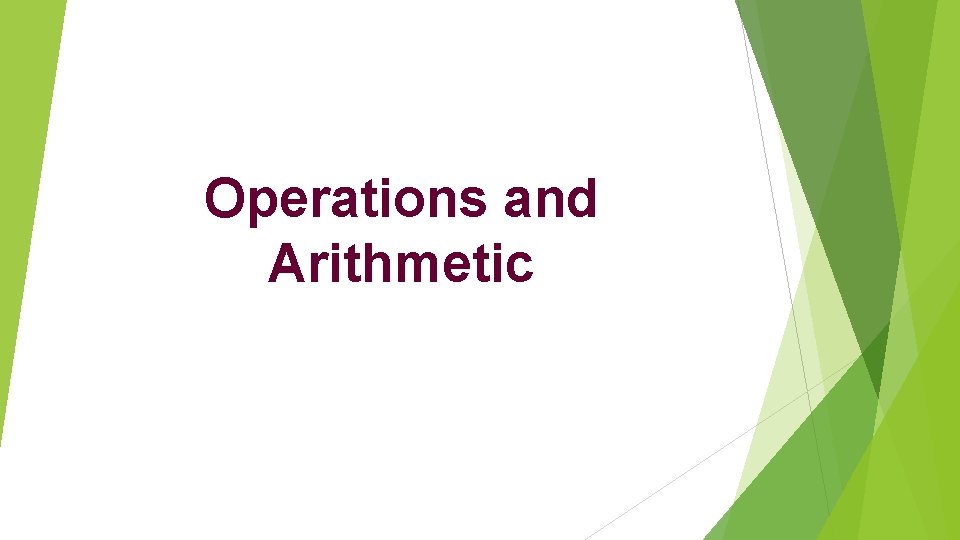
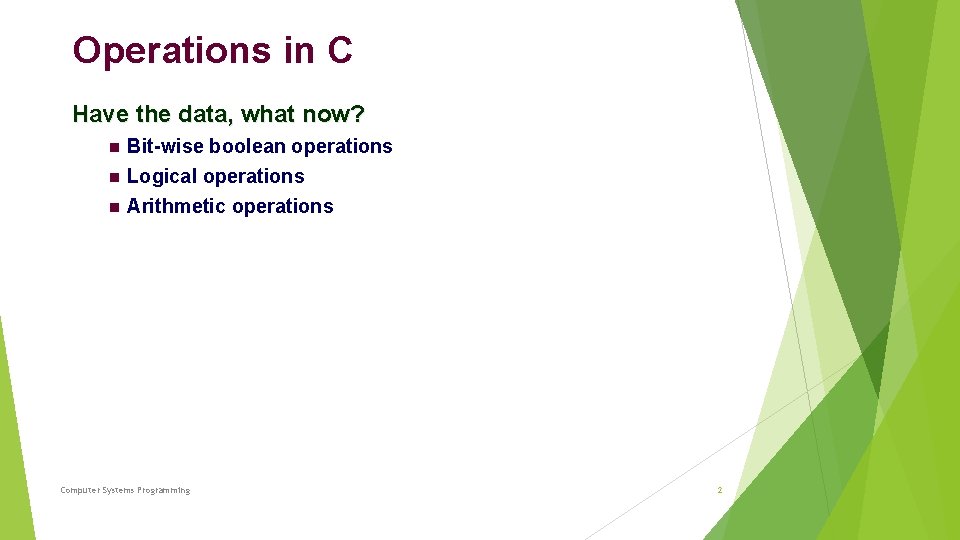
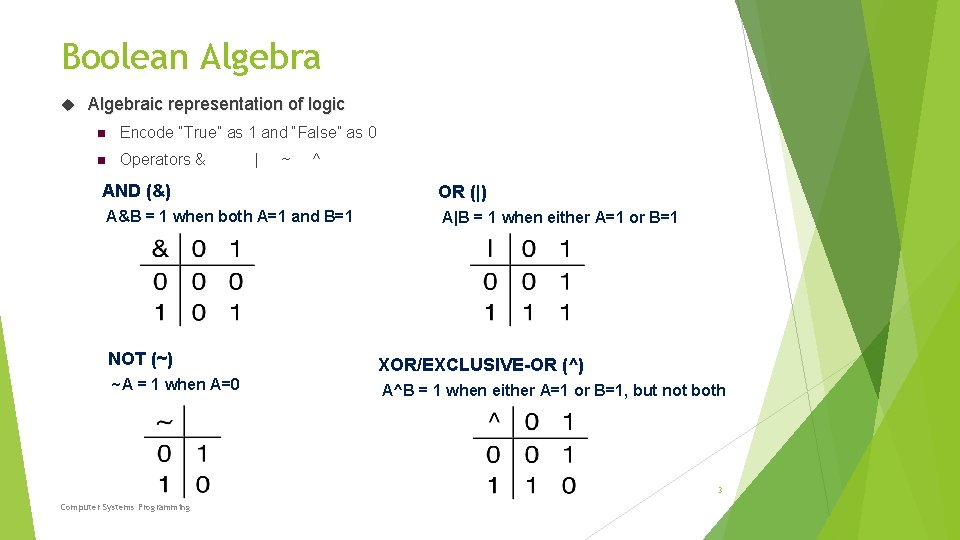
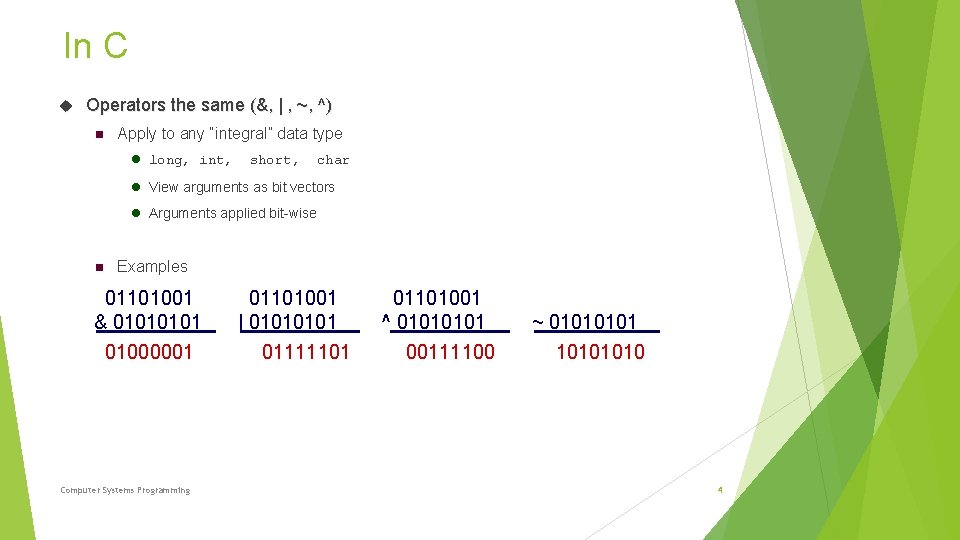
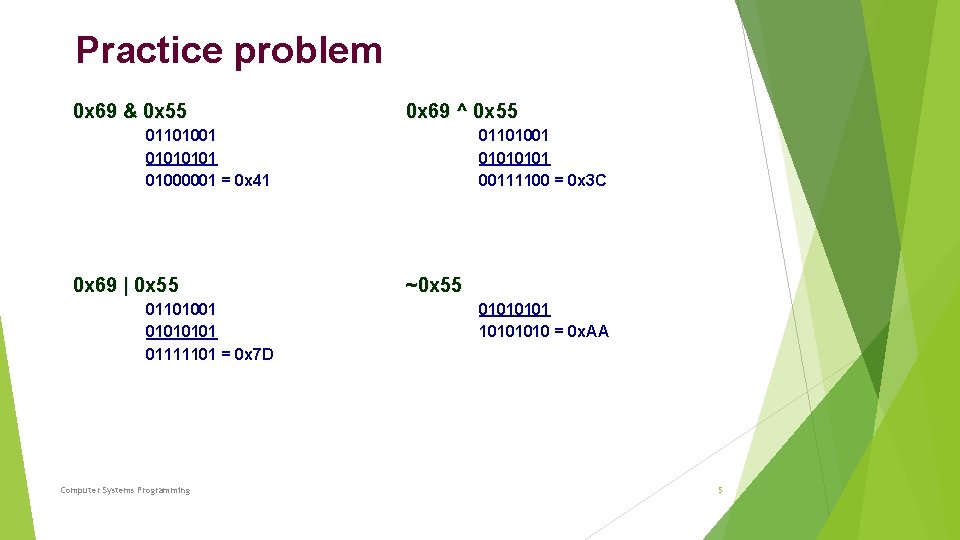
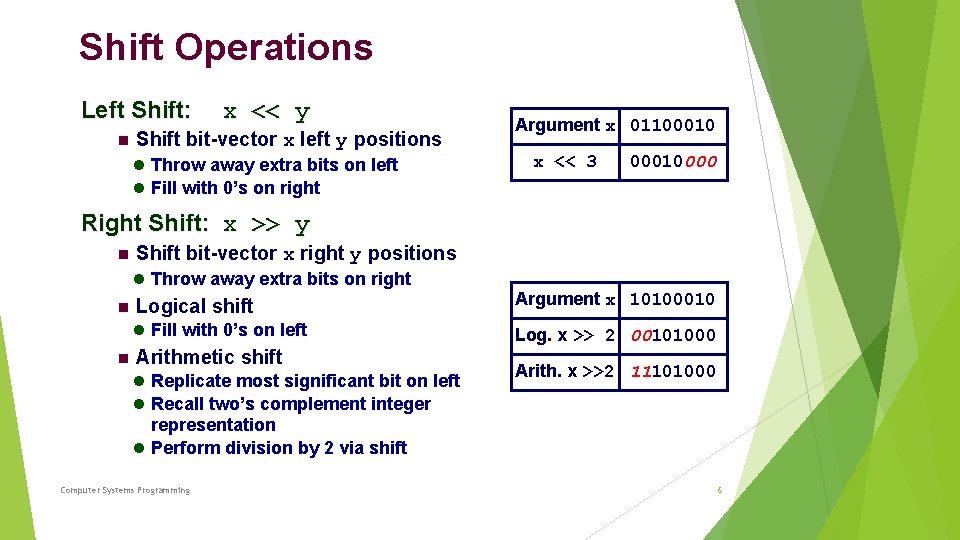
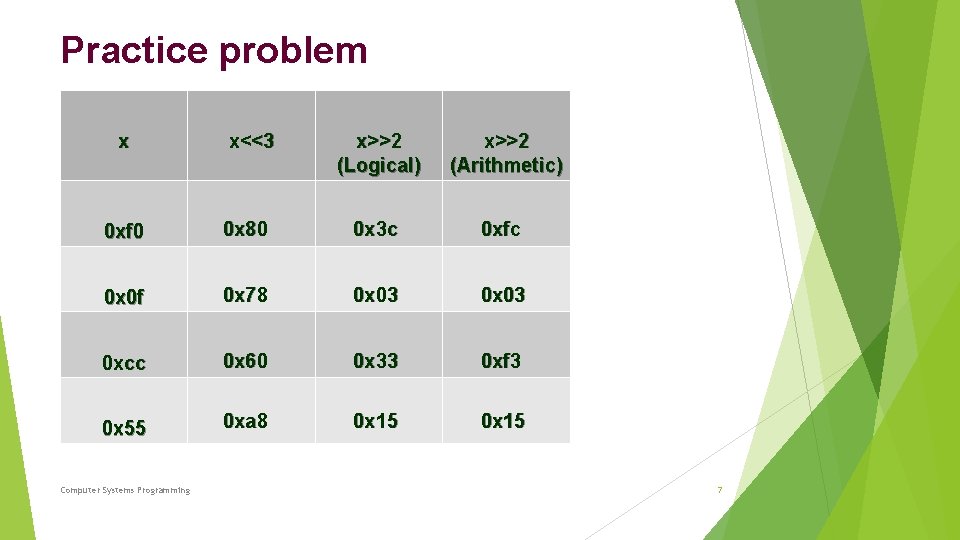
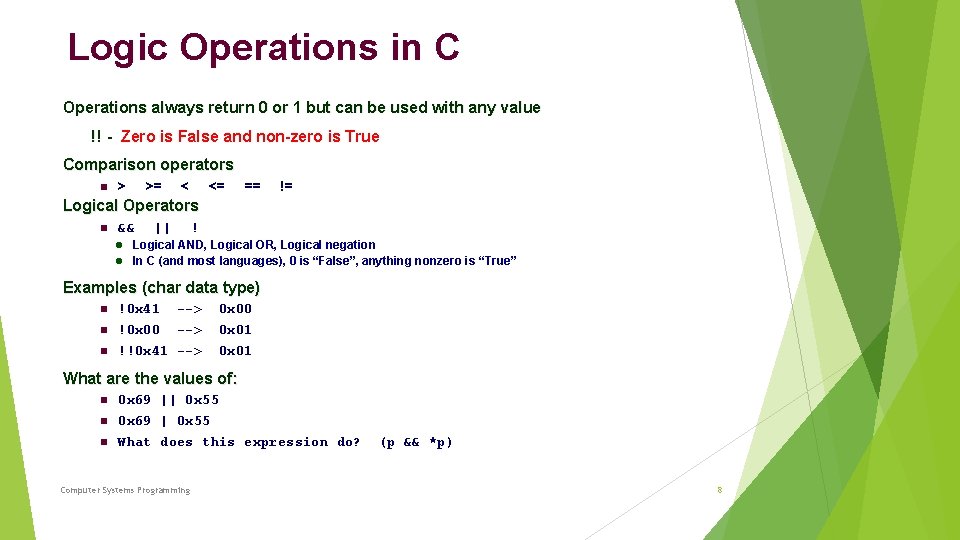
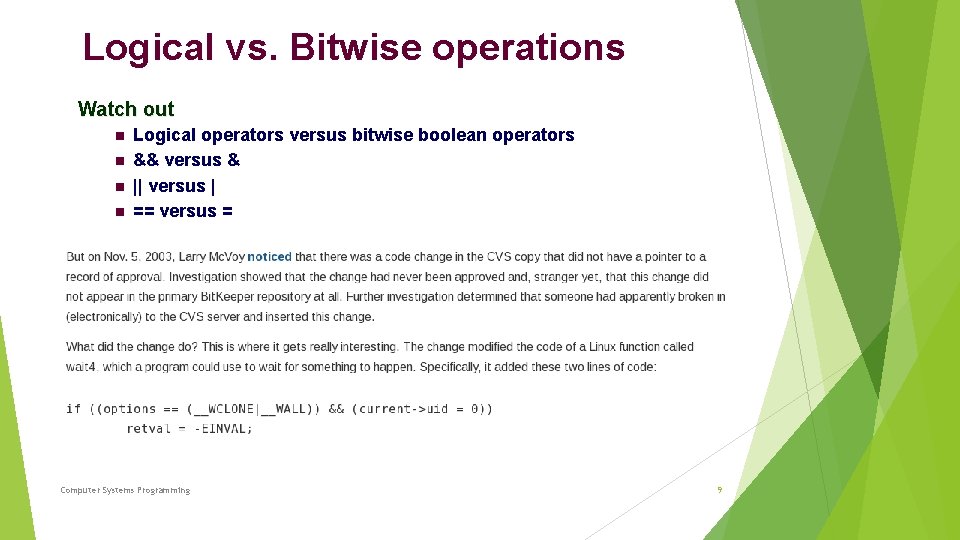
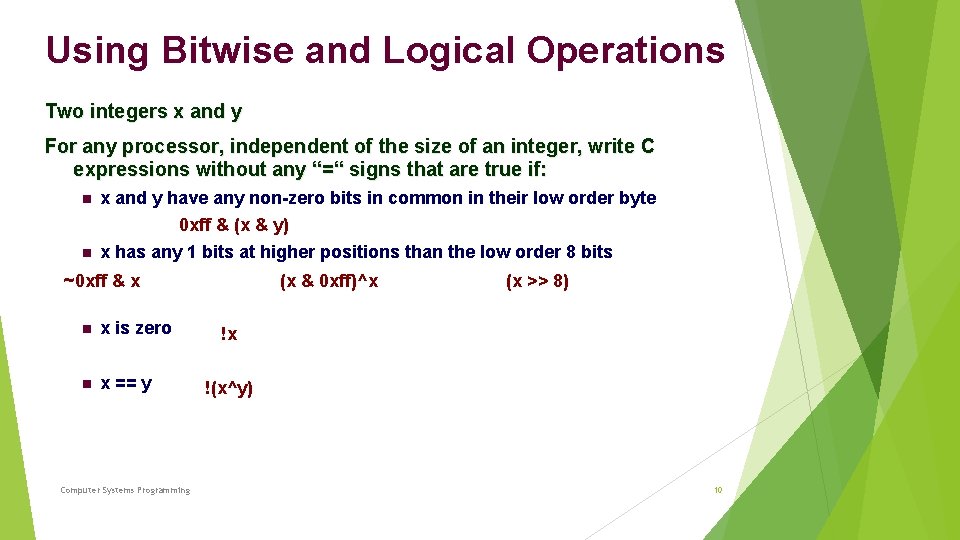
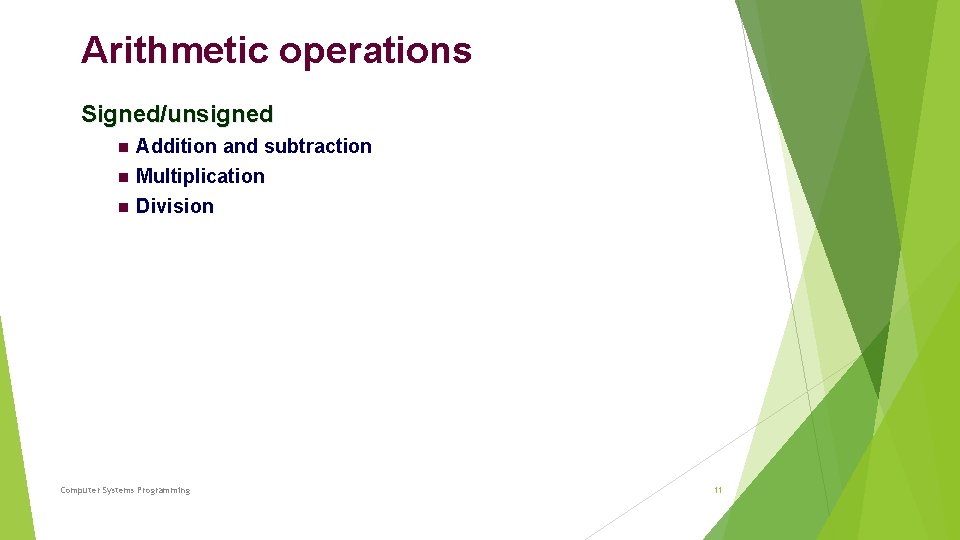
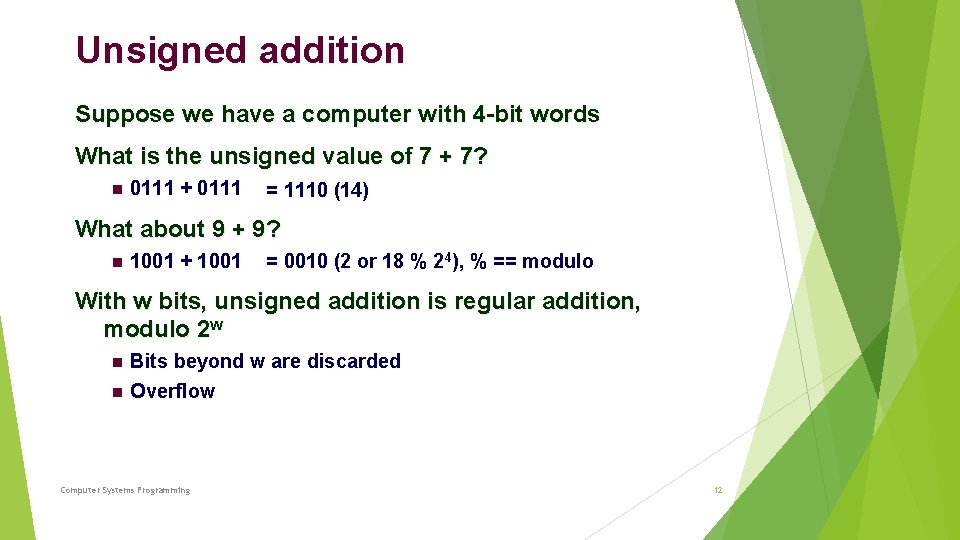
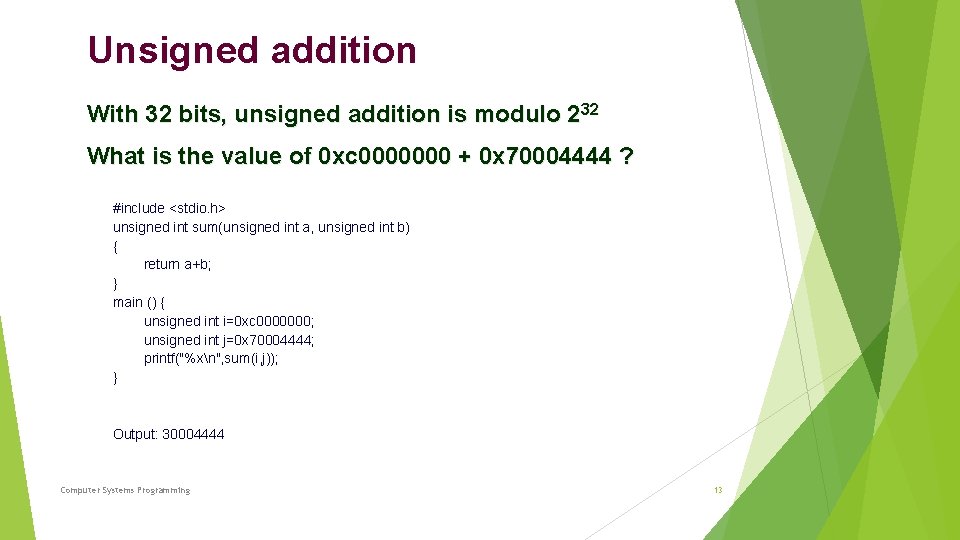
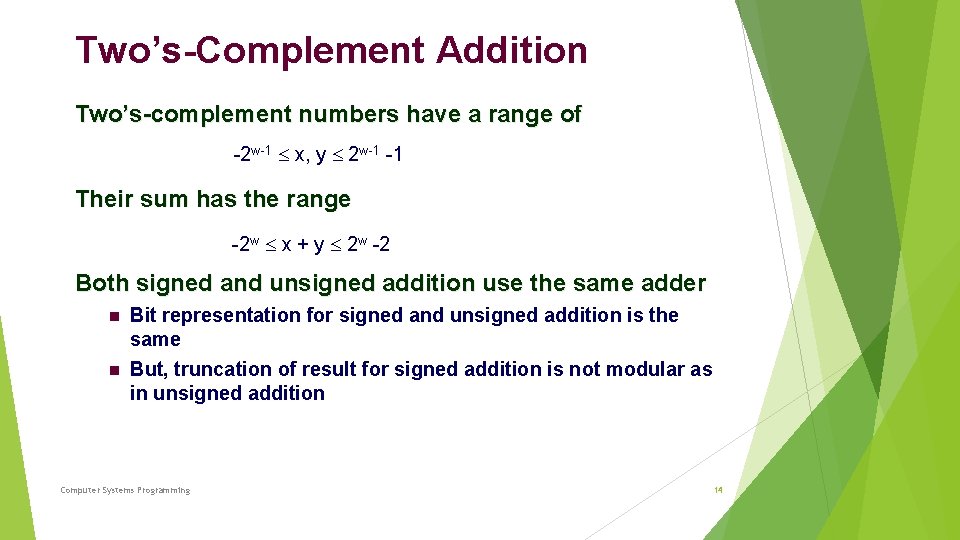
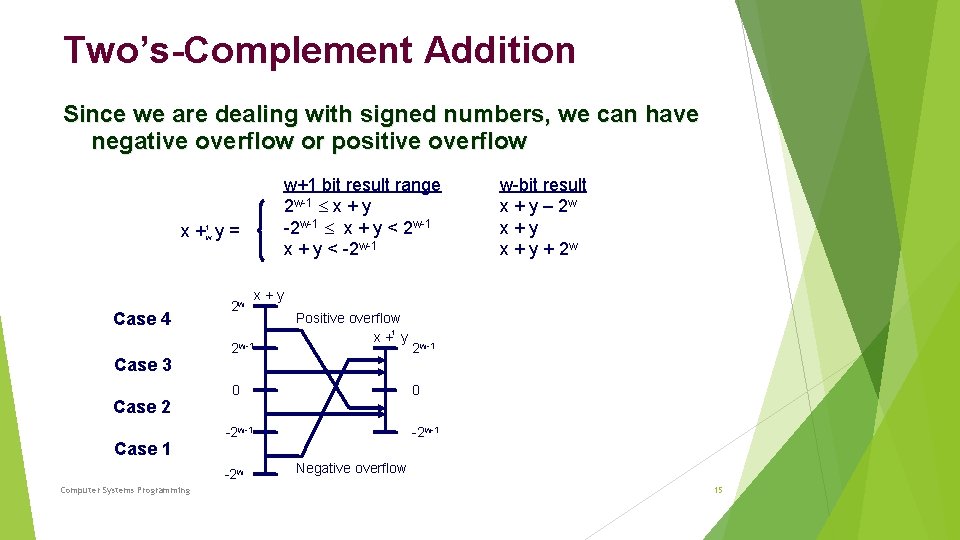
![Example (w=4) x y x+y t 4 x+ y Case 1 -8 [1000] -5 Example (w=4) x y x+y t 4 x+ y Case 1 -8 [1000] -5](https://slidetodoc.com/presentation_image_h2/733ab41c770db933081ed16fb521a27f/image-16.jpg)
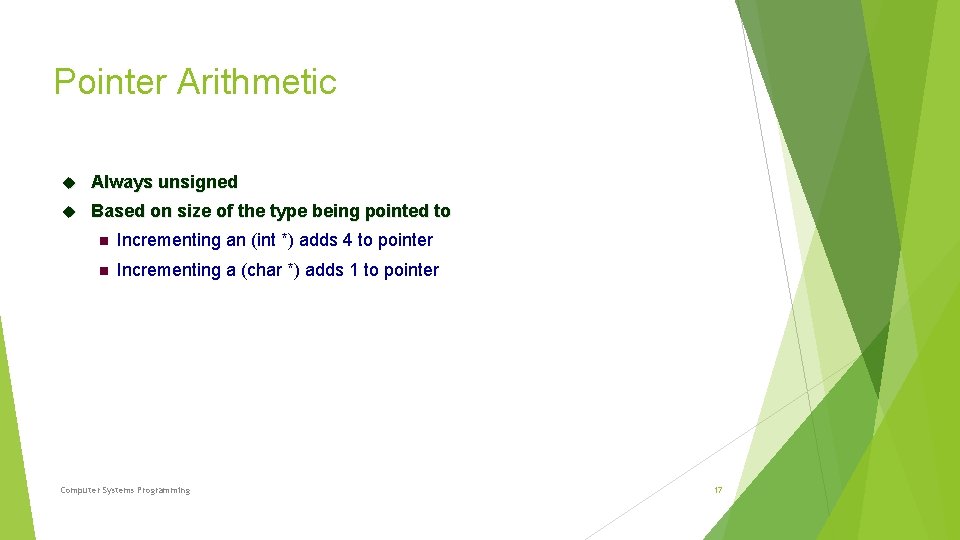
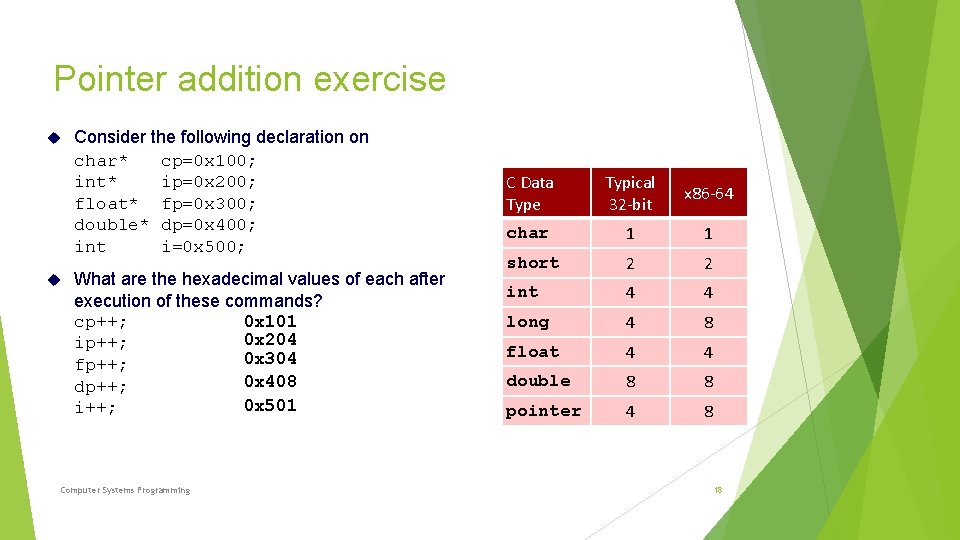
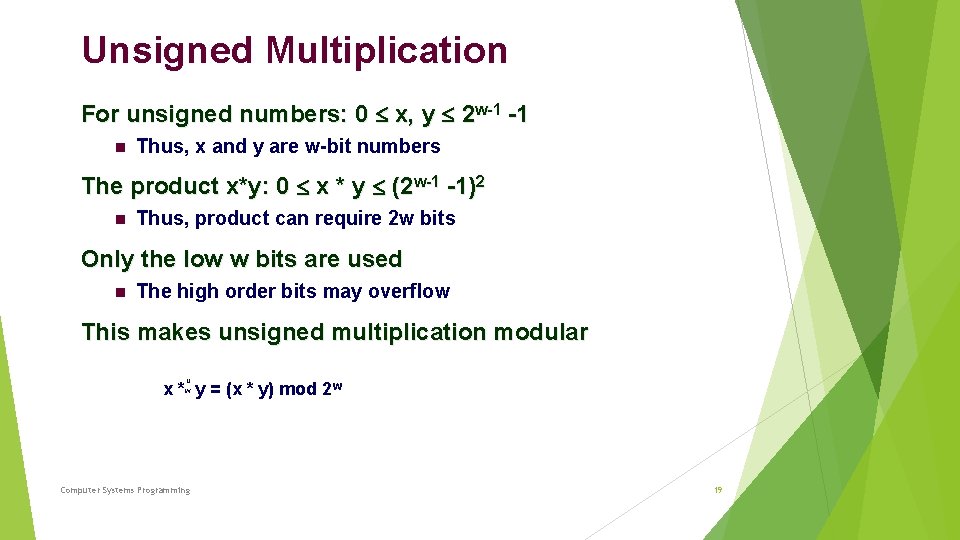
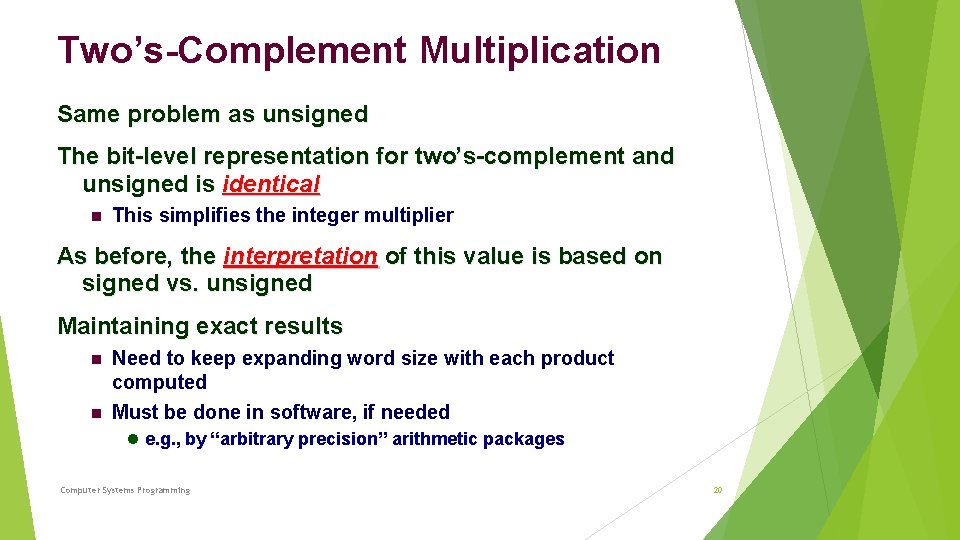
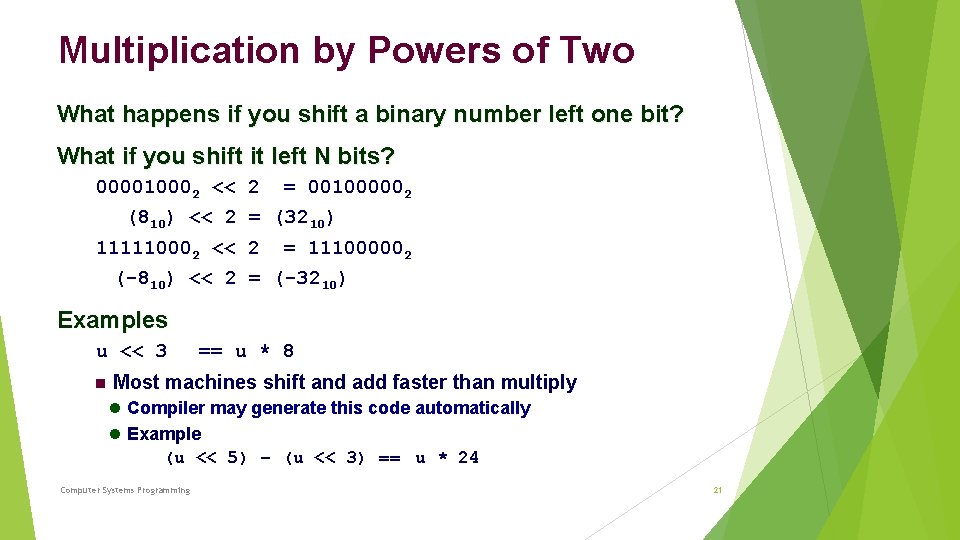
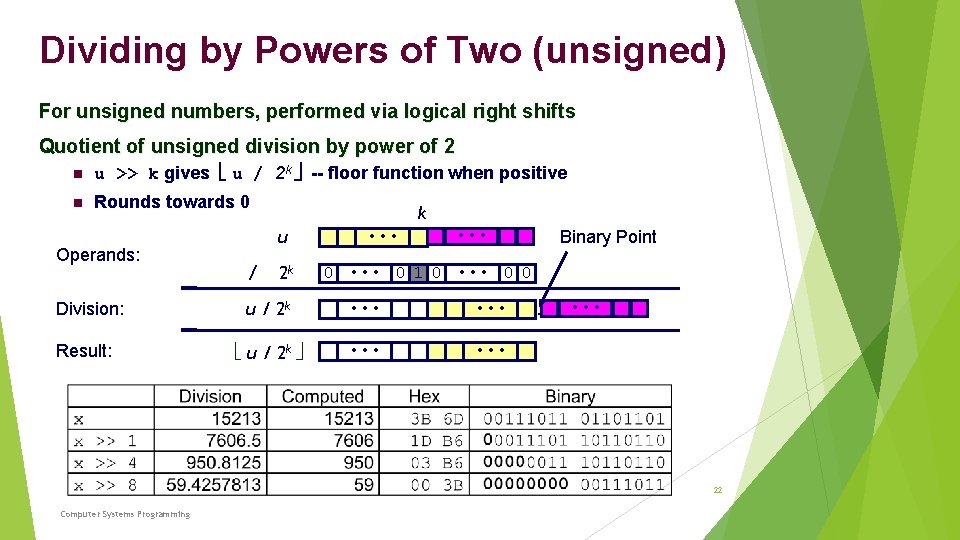
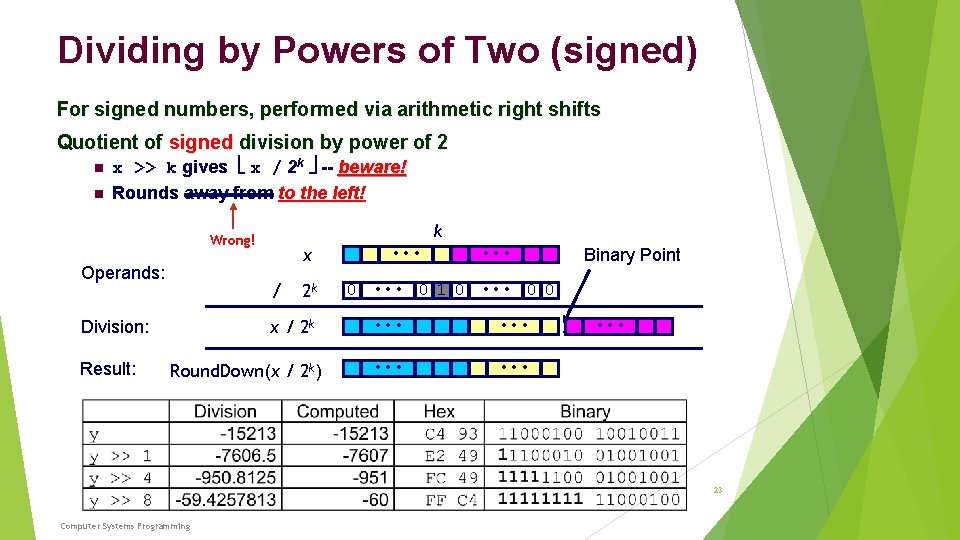
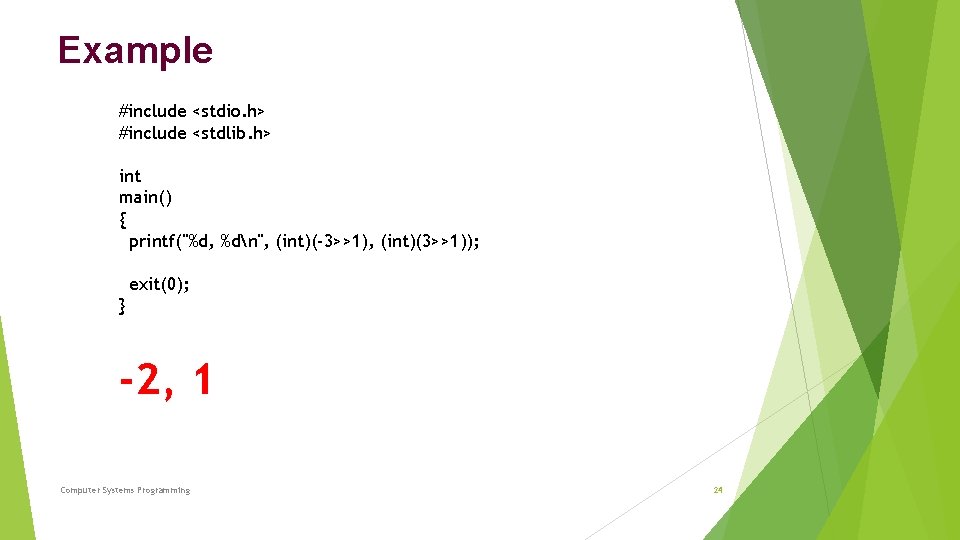
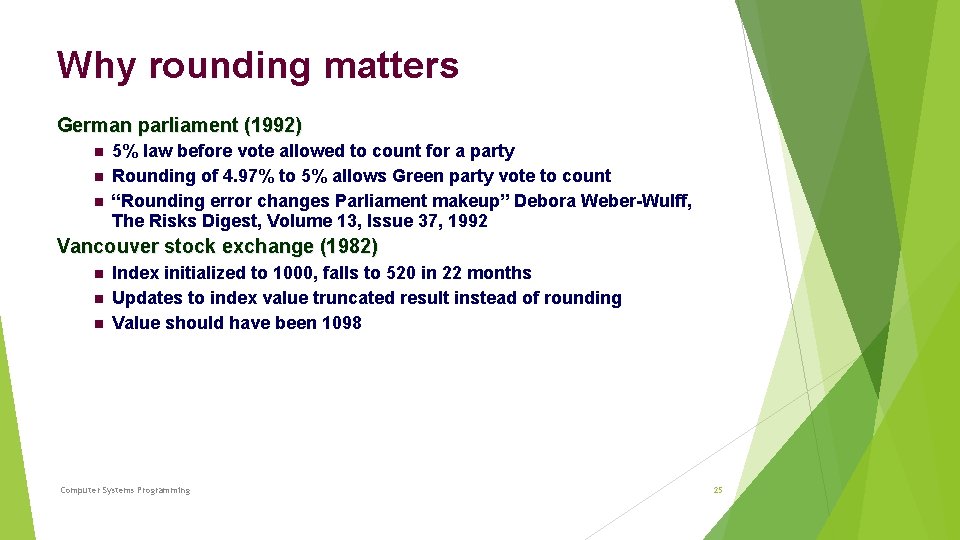
- Slides: 25
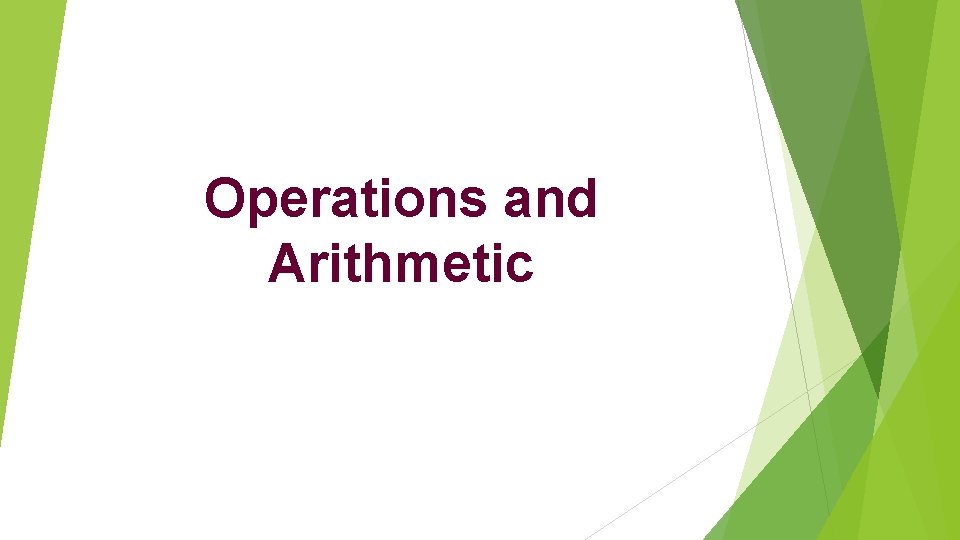
Operations and Arithmetic
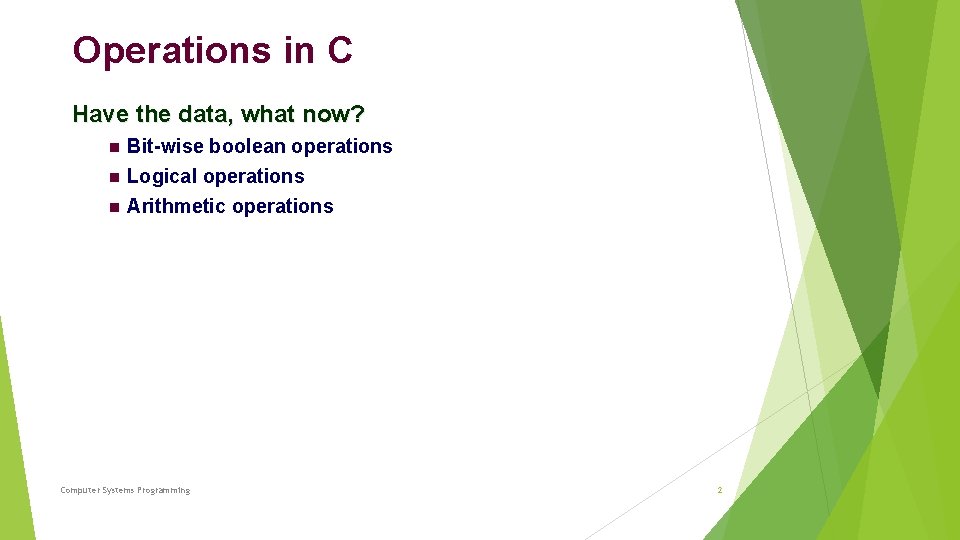
Operations in C Have the data, what now? Bit-wise boolean operations Logical operations Arithmetic operations Computer Systems Programming 2
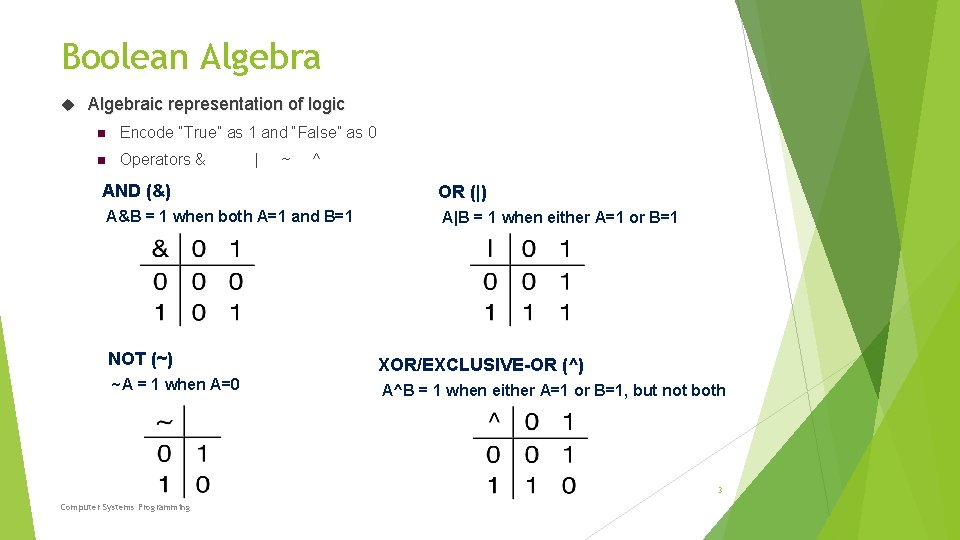
Boolean Algebraic representation of logic Encode “True” as 1 and “False” as 0 Operators & | ~ ^ AND (&) OR (|) A&B = 1 when both A=1 and B=1 A|B = 1 when either A=1 or B=1 NOT (~) XOR/EXCLUSIVE-OR (^) ~A = 1 when A=0 A^B = 1 when either A=1 or B=1, but not both 3 Computer Systems Programming
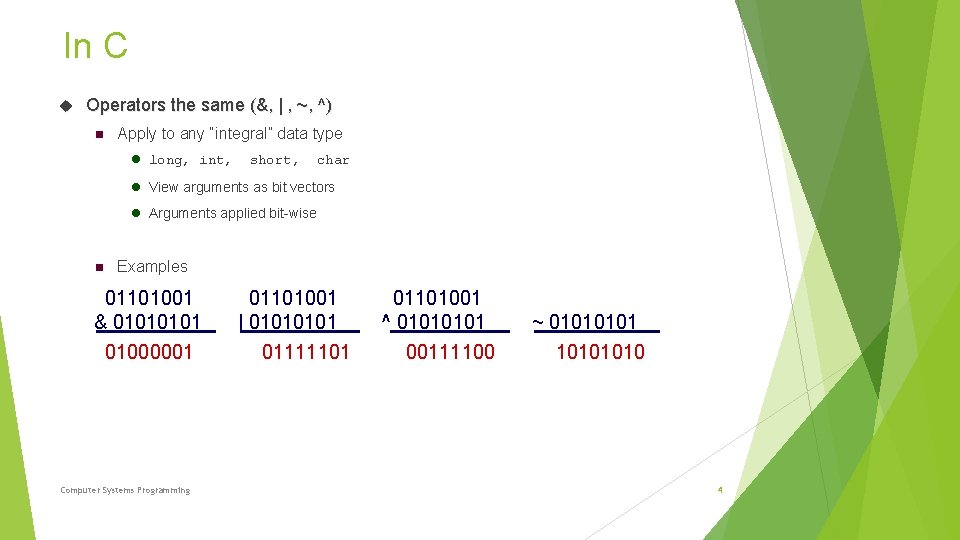
In C Operators the same (&, | , ~, ^) Apply to any “integral” data type long, int, short, char View arguments as bit vectors Arguments applied bit-wise Examples 01101001 & 0101 01000001 Computer Systems Programming 01101001 | 0101 01111101 01101001 ^ 0101 00111100 ~ 0101 10101010 4
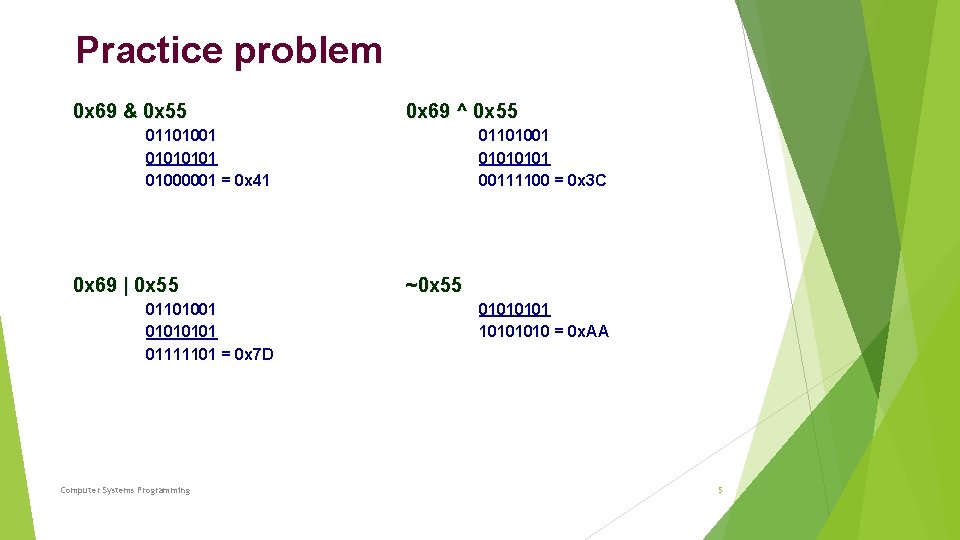
Practice problem 0 x 69 & 0 x 55 0 x 69 ^ 0 x 55 01101001 0101 01000001 = 0 x 41 0 x 69 | 0 x 55 01101001 0101 01111101 = 0 x 7 D Computer Systems Programming 01101001 0101 00111100 = 0 x 3 C ~0 x 55 0101 1010 = 0 x. AA 5
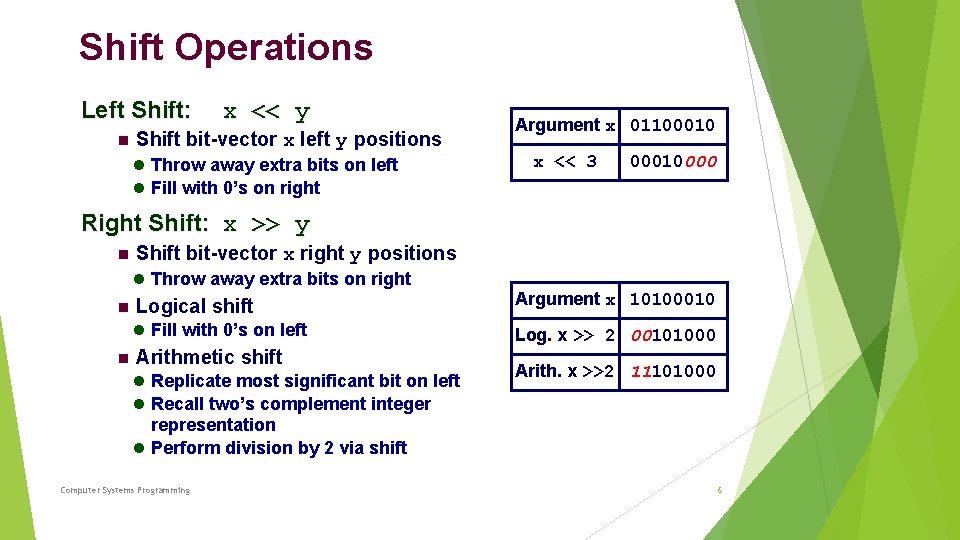
Shift Operations Left Shift: x << y Shift bit-vector x left y positions Throw away extra bits on left Fill with 0’s on right Argument x 01100010 x << 3 00010000 Right Shift: x >> y Shift bit-vector x right y positions Throw away extra bits on right Logical shift Argument x 10100010 Fill with 0’s on left Log. x >> 2 00101000 Arithmetic shift Replicate most significant bit on left Recall two’s complement integer Arith. x >>2 11101000 representation Perform division by 2 via shift Computer Systems Programming 6
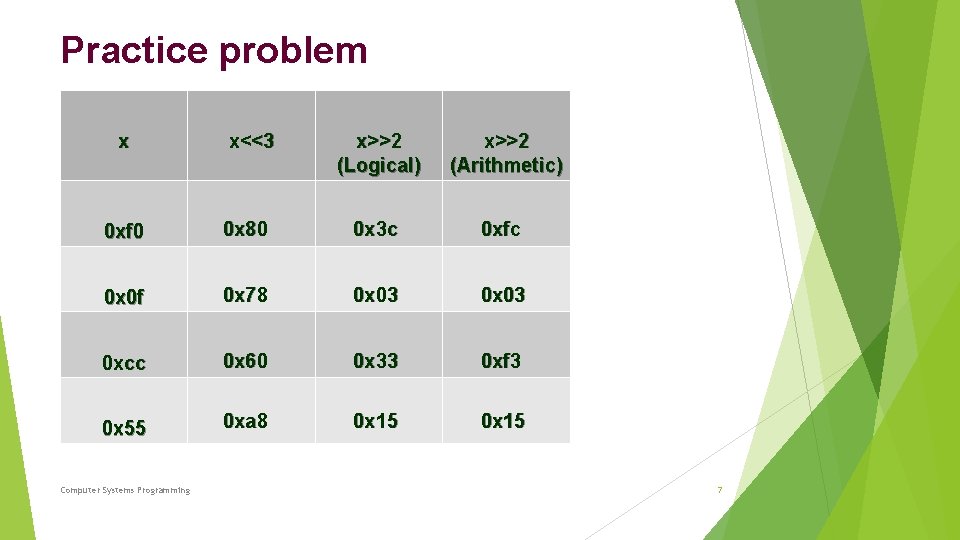
Practice problem x x<<3 x>>2 (Logical) x>>2 (Arithmetic) 0 xf 0 0 x 80 0 x 3 c 0 xfc 0 x 0 f 0 x 78 0 x 03 0 xcc 0 x 60 0 x 33 0 xf 3 0 x 55 0 xa 8 0 x 15 Computer Systems Programming 7
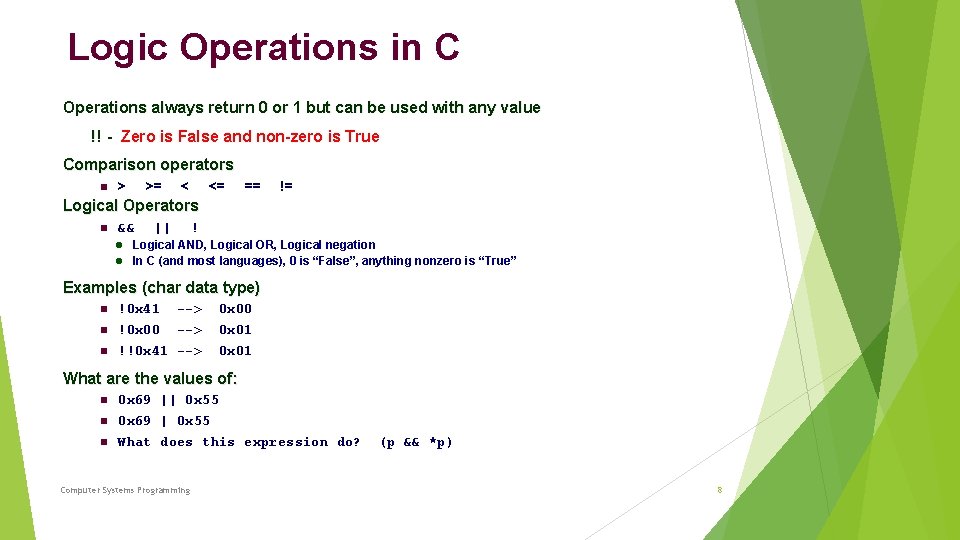
Logic Operations in C Operations always return 0 or 1 but can be used with any value !! - Zero is False and non-zero is True Comparison operators > >= < <= == != Logical Operators && || ! Logical AND, Logical OR, Logical negation In C (and most languages), 0 is “False”, anything nonzero is “True” Examples (char data type) !0 x 41 --> !0 x 00 --> !!0 x 41 --> 0 x 00 0 x 01 What are the values of: 0 x 69 || 0 x 55 0 x 69 | 0 x 55 What does this expression do? Computer Systems Programming (p && *p) 8
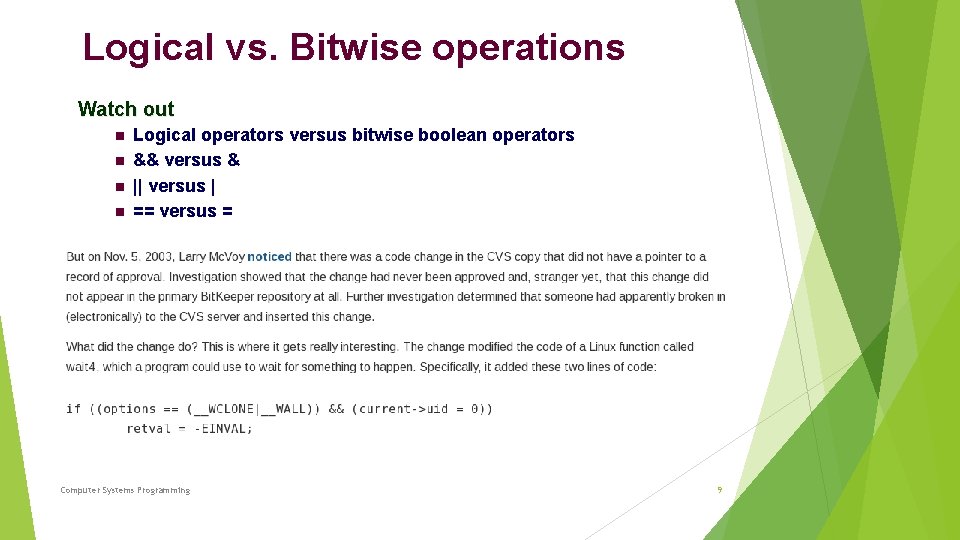
Logical vs. Bitwise operations Watch out Logical operators versus bitwise boolean operators && versus & || versus | == versus = Computer Systems Programming 9
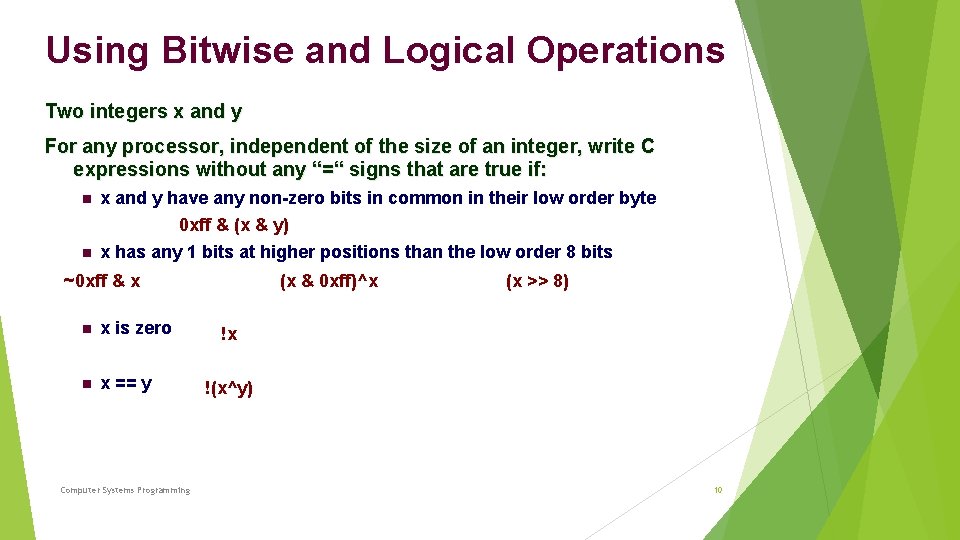
Using Bitwise and Logical Operations Two integers x and y For any processor, independent of the size of an integer, write C expressions without any “=“ signs that are true if: x and y have any non-zero bits in common in their low order byte 0 xff & (x & y) x has any 1 bits at higher positions than the low order 8 bits ~0 xff & x x is zero x == y Computer Systems Programming (x & 0 xff)^x (x >> 8) !x !(x^y) 10
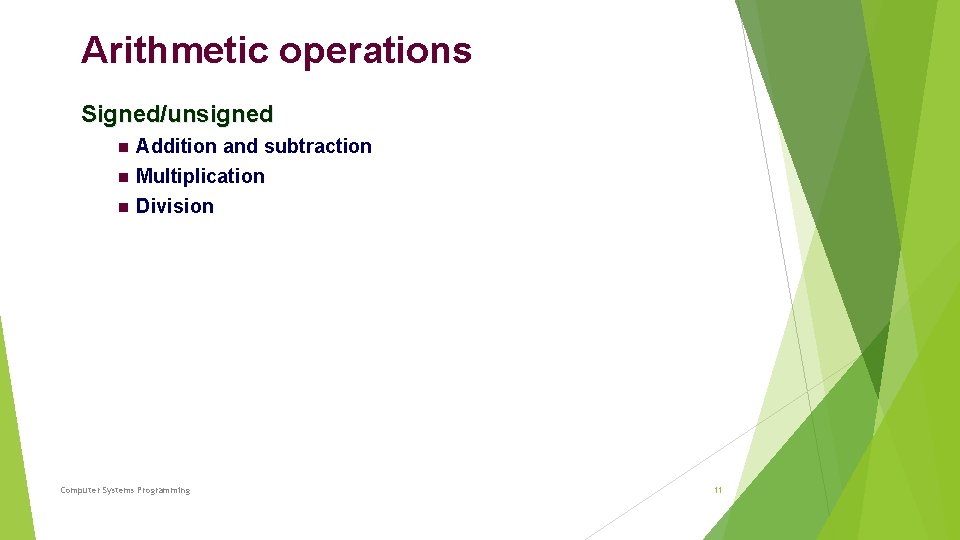
Arithmetic operations Signed/unsigned Addition and subtraction Multiplication Division Computer Systems Programming 11
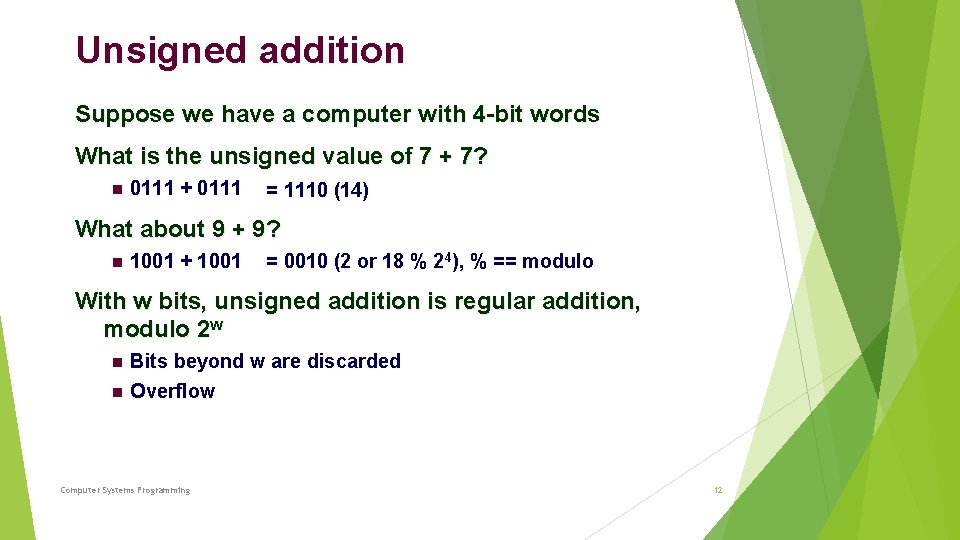
Unsigned addition Suppose we have a computer with 4 -bit words What is the unsigned value of 7 + 7? 0111 + 0111 = 1110 (14) What about 9 + 9? 1001 + 1001 = 0010 (2 or 18 % 24), % == modulo With w bits, unsigned addition is regular addition, modulo 2 w Bits beyond w are discarded Overflow Computer Systems Programming 12
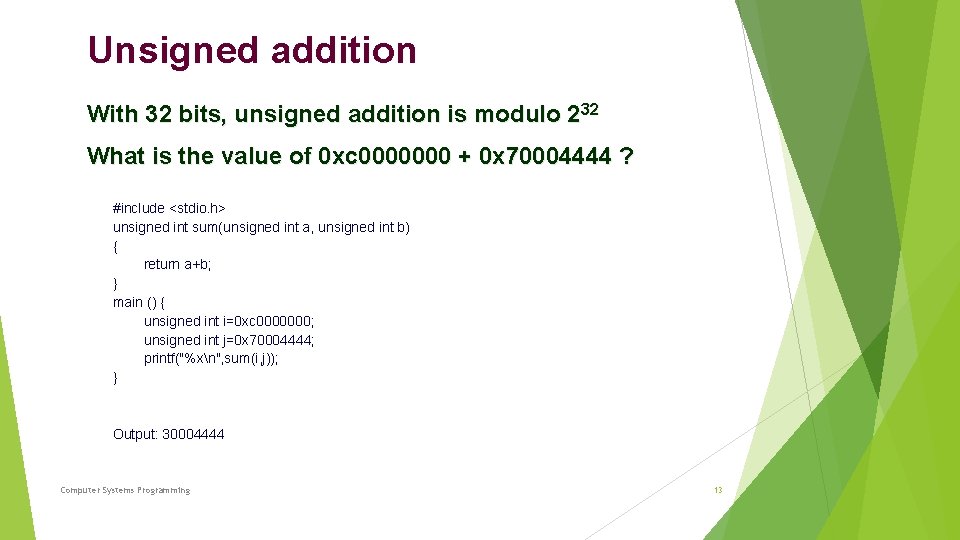
Unsigned addition With 32 bits, unsigned addition is modulo 232 What is the value of 0 xc 0000000 + 0 x 70004444 ? #include <stdio. h> unsigned int sum(unsigned int a, unsigned int b) { return a+b; } main () { unsigned int i=0 xc 0000000; unsigned int j=0 x 70004444; printf("%xn", sum(i, j)); } Output: 30004444 Computer Systems Programming 13
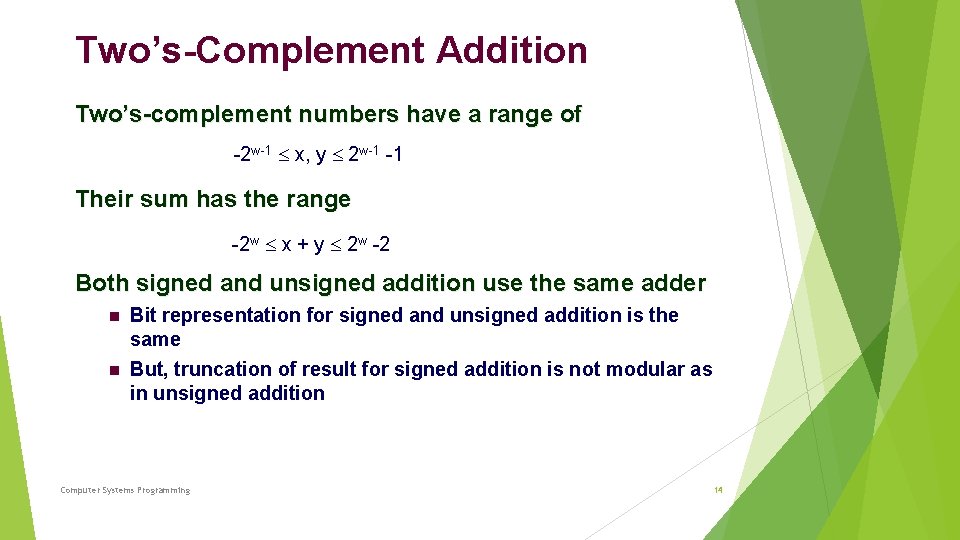
Two’s-Complement Addition Two’s-complement numbers have a range of -2 w-1 x, y 2 w-1 -1 Their sum has the range -2 w x + y 2 w -2 Both signed and unsigned addition use the same adder Bit representation for signed and unsigned addition is the same But, truncation of result for signed addition is not modular as in unsigned addition Computer Systems Programming 14
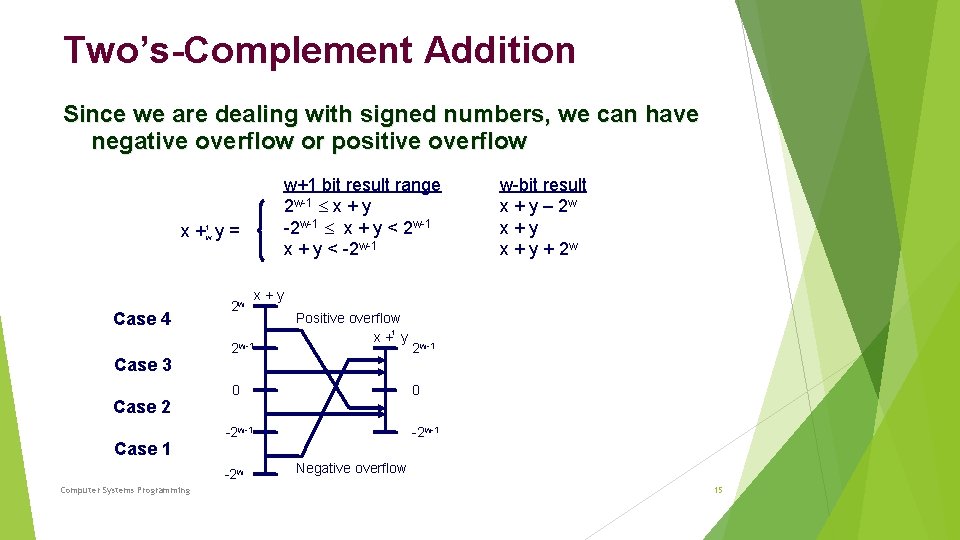
Two’s-Complement Addition Since we are dealing with signed numbers, we can have negative overflow or positive overflow x + tw y = Case 4 Case 3 Case 2 Case 1 2 w 2 w-1 w-bit result x + y – 2 w x+y x + y + 2 w x+y Positive overflow x +t y 0 2 w-1 0 -2 w-1 -2 w Computer Systems Programming w+1 bit result range 2 w-1 x + y -2 w-1 x + y < 2 w-1 x + y < -2 w-1 Negative overflow 15
![Example w4 x y xy t 4 x y Case 1 8 1000 5 Example (w=4) x y x+y t 4 x+ y Case 1 -8 [1000] -5](https://slidetodoc.com/presentation_image_h2/733ab41c770db933081ed16fb521a27f/image-16.jpg)
Example (w=4) x y x+y t 4 x+ y Case 1 -8 [1000] -5 [1011] -13 [10011] 3 [0011] -8 [1000] -16 [10000] 0 [0000] Case 1 -8 [1000] 5 [0101] -3 [1101] Case 2 2 [0010] 5 [0101] 7 [0111] Case 3 5 [0101] 10 [1010] -6 [1010] Case 4 x+ y= Computer Systems Programming x + y – 2 w, x + y + 2 w, 2 w-1 x + y (Case 4) -2 w-1 x + y < 2 w-1 (Case 2/3) x + y < -2 w-1 (Case 1) 16
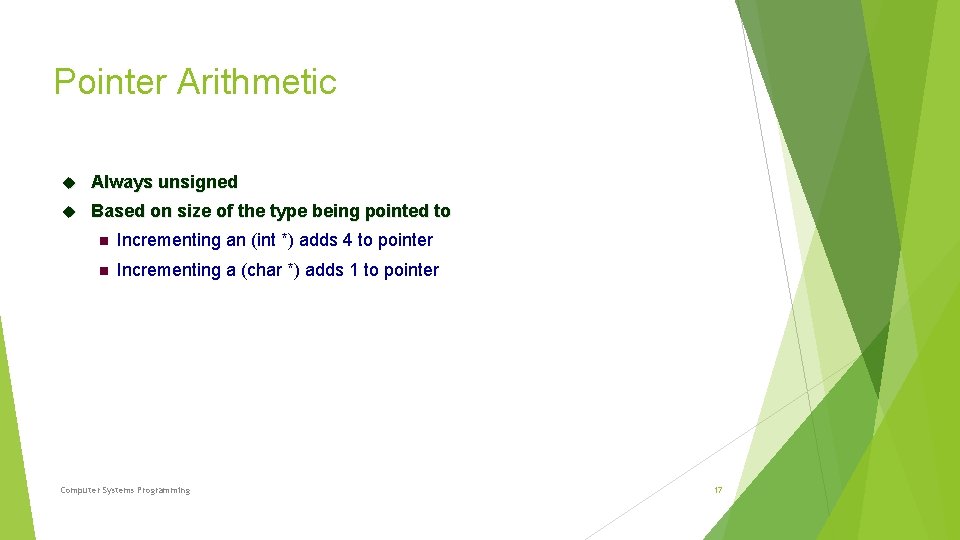
Pointer Arithmetic Always unsigned Based on size of the type being pointed to Incrementing an (int *) adds 4 to pointer Incrementing a (char *) adds 1 to pointer Computer Systems Programming 17
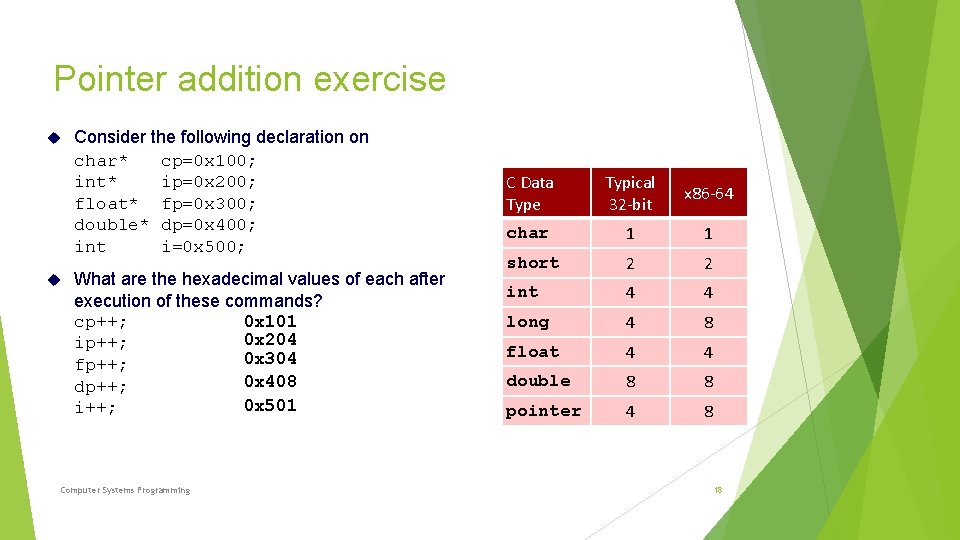
Pointer addition exercise Consider the following declaration on char* cp=0 x 100; int* ip=0 x 200; float* fp=0 x 300; double* dp=0 x 400; int i=0 x 500; What are the hexadecimal values of each after execution of these commands? 0 x 101 cp++; 0 x 204 ip++; 0 x 304 fp++; 0 x 408 dp++; 0 x 501 i++; Computer Systems Programming C Data Type Typical 32 -bit x 86 -64 char 1 1 short 2 2 int 4 4 long 4 8 float 4 4 double 8 8 pointer 4 8 18
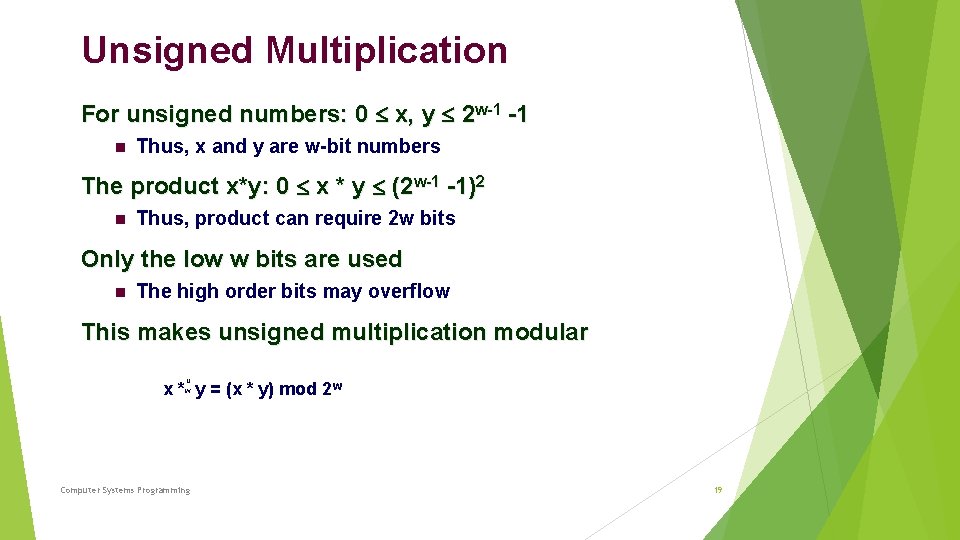
Unsigned Multiplication For unsigned numbers: 0 x, y 2 w-1 -1 Thus, x and y are w-bit numbers The product x*y: 0 x * y (2 w-1 -1)2 Thus, product can require 2 w bits Only the low w bits are used The high order bits may overflow This makes unsigned multiplication modular u x *w y = (x * y) mod 2 w Computer Systems Programming 19
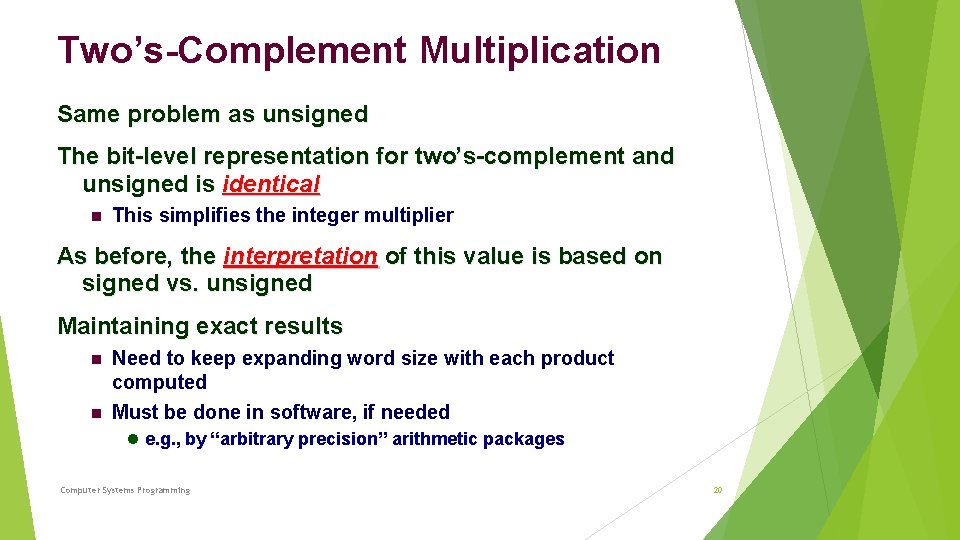
Two’s-Complement Multiplication Same problem as unsigned The bit-level representation for two’s-complement and unsigned is identical This simplifies the integer multiplier As before, the interpretation of this value is based on signed vs. unsigned Maintaining exact results Need to keep expanding word size with each product computed Must be done in software, if needed e. g. , by “arbitrary precision” arithmetic packages Computer Systems Programming 20
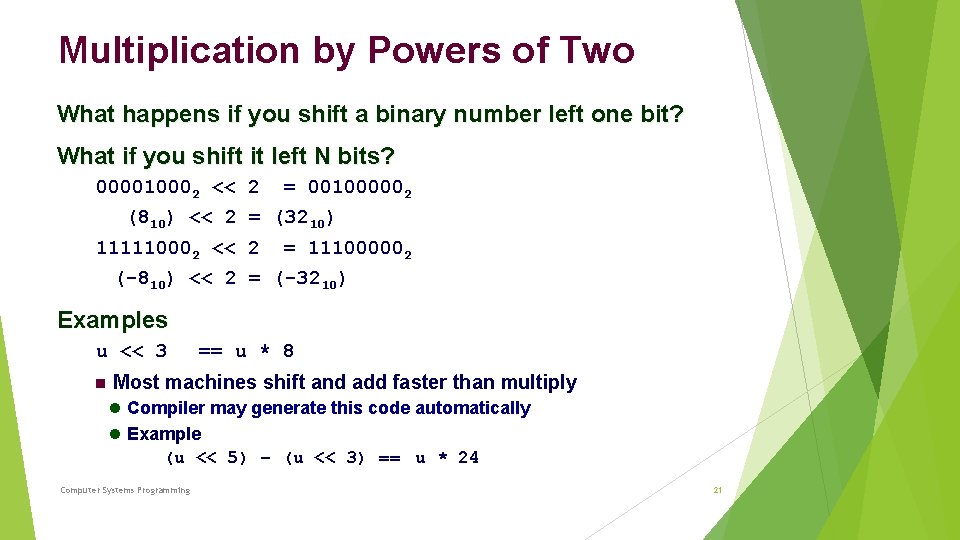
Multiplication by Powers of Two What happens if you shift a binary number left one bit? What if you shift it left N bits? 000010002 << 2 = 001000002 (810) << 2 = (3210) 111110002 << 2 = 111000002 (-810) << 2 = (-3210) Examples u << 3 == u * 8 Most machines shift and add faster than multiply Compiler may generate this code automatically Example (u << 5) – (u << 3) == u * 24 Computer Systems Programming 21
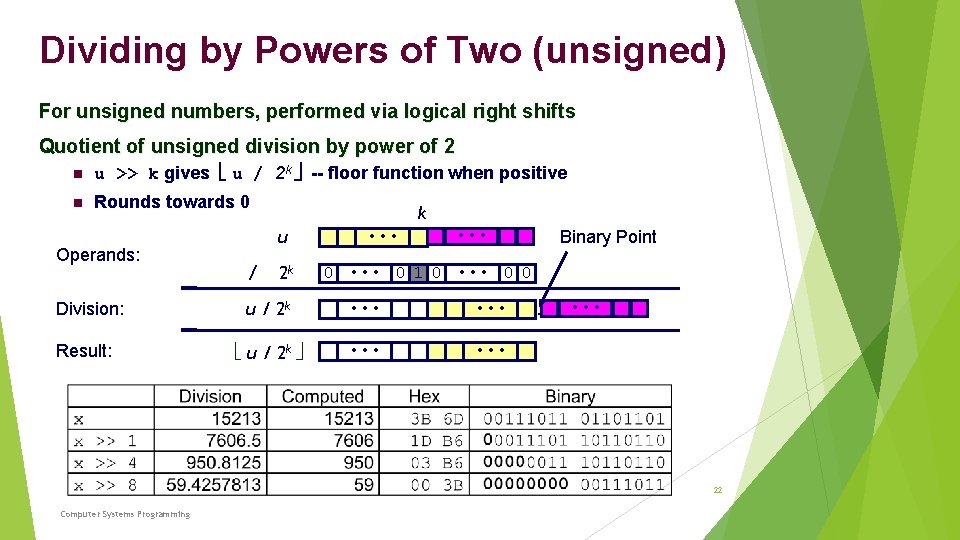
Dividing by Powers of Two (unsigned) For unsigned numbers, performed via logical right shifts Quotient of unsigned division by power of 2 u >> k gives u / 2 k -- floor function when positive Rounds towards 0 Operands: Division: Result: k u / 2 k • • • Binary Point 0 • • • 0 1 0 • • • 0 0 u / 2 k 0 • • • u / 2 k 0 • • • 22 Computer Systems Programming
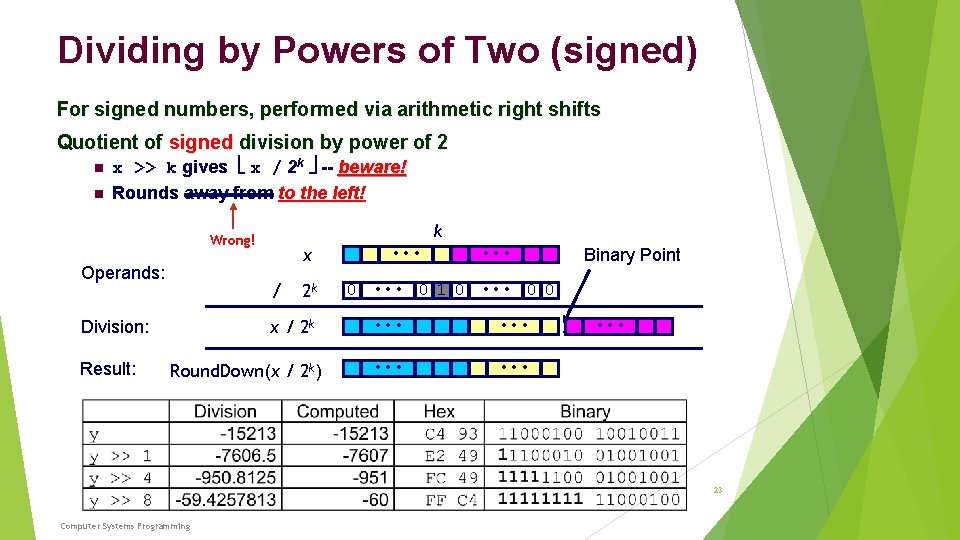
Dividing by Powers of Two (signed) For signed numbers, performed via arithmetic right shifts Quotient of signed division by power of 2 x >> k gives x / 2 k -- beware! Rounds away from to the left! k Wrong! Operands: / 2 k x / 2 k Division: Result: x Round. Down(x / 2 k) • • • Binary Point 0 • • • 0 1 0 • • • 0 0 0 • • • 23 Computer Systems Programming
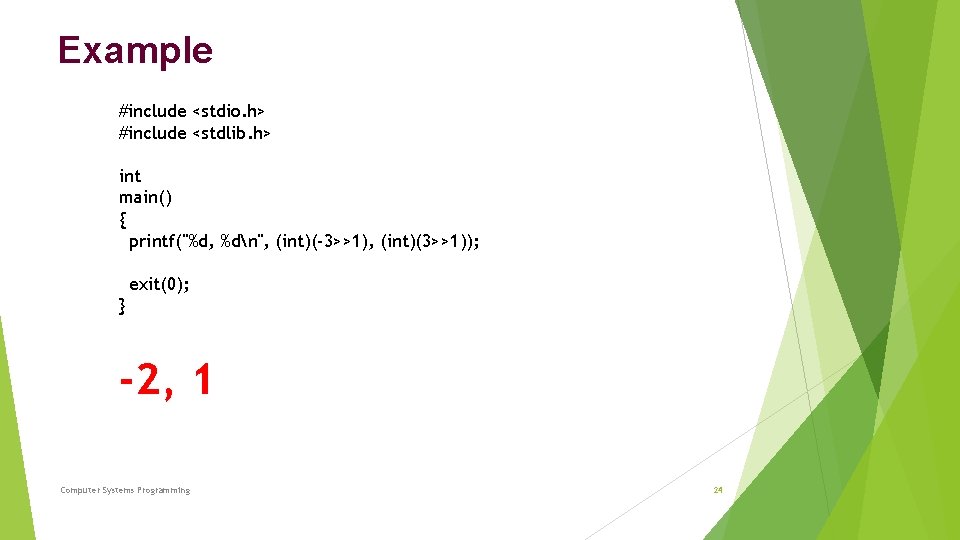
Example #include <stdio. h> #include <stdlib. h> int main() { printf("%d, %dn", (int)(-3>>1), (int)(3>>1)); exit(0); } -2, 1 Computer Systems Programming 24
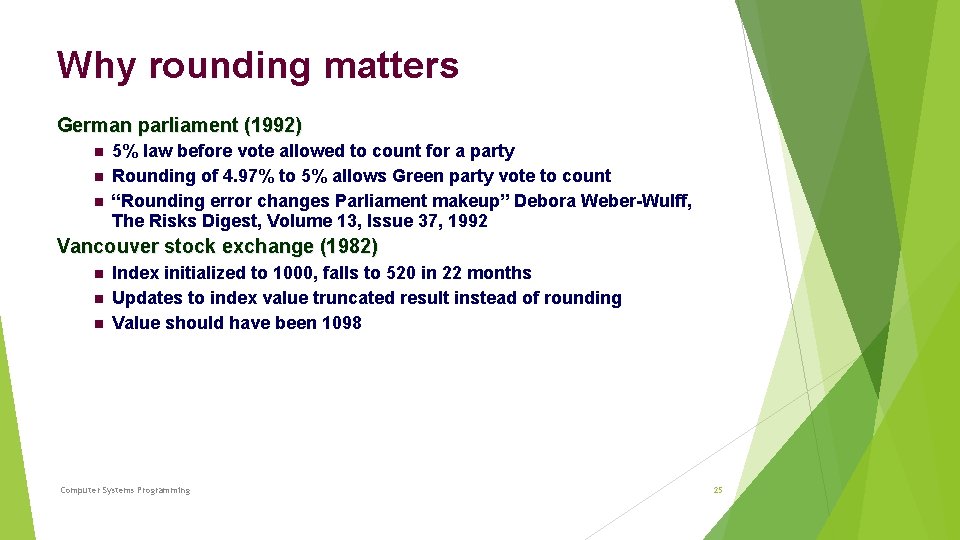
Why rounding matters German parliament (1992) 5% law before vote allowed to count for a party Rounding of 4. 97% to 5% allows Green party vote to count “Rounding error changes Parliament makeup” Debora Weber-Wulff, The Risks Digest, Volume 13, Issue 37, 1992 Vancouver stock exchange (1982) Index initialized to 1000, falls to 520 in 22 months Updates to index value truncated result instead of rounding Value should have been 1098 Computer Systems Programming 25
Define rtl
Arithmetic operationsarithmetic operations
Combining spatial enhancement methods
Arithmetic operations
Floating point
8 faces and 6 vertices
Hát kết hợp bộ gõ cơ thể
Bổ thể
Tỉ lệ cơ thể trẻ em
Voi kéo gỗ như thế nào
Thang điểm glasgow
Alleluia hat len nguoi oi
Môn thể thao bắt đầu bằng từ đua
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới
Cong thức tính động năng
Trời xanh đây là của chúng ta thể thơ
Cách giải mật thư tọa độ
Phép trừ bù
Phản ứng thế ankan
Các châu lục và đại dương trên thế giới
Thơ thất ngôn tứ tuyệt đường luật
Quá trình desamine hóa có thể tạo ra
Một số thể thơ truyền thống
Cái miệng bé xinh thế chỉ nói điều hay thôi