CHAPTER ONE Arithmetic operations decisions and looping Arithmetic
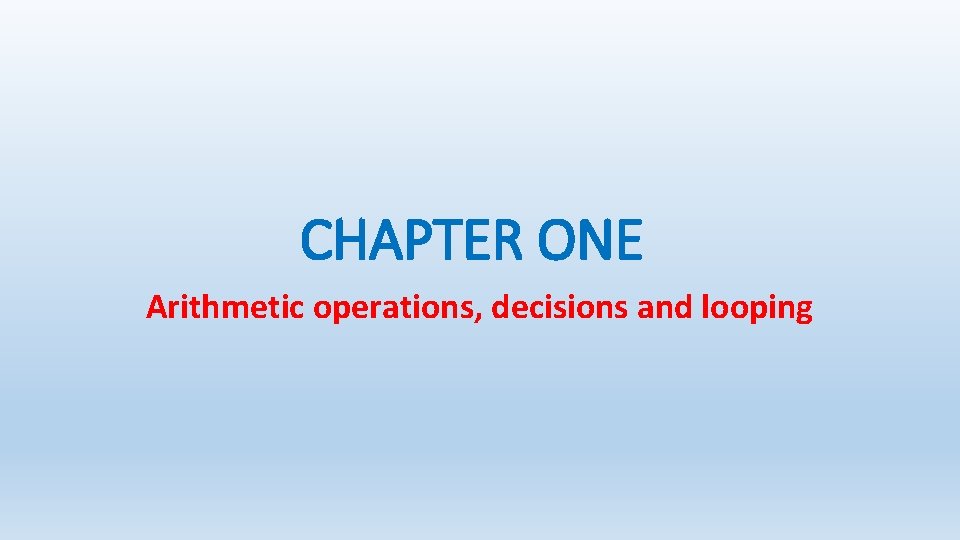
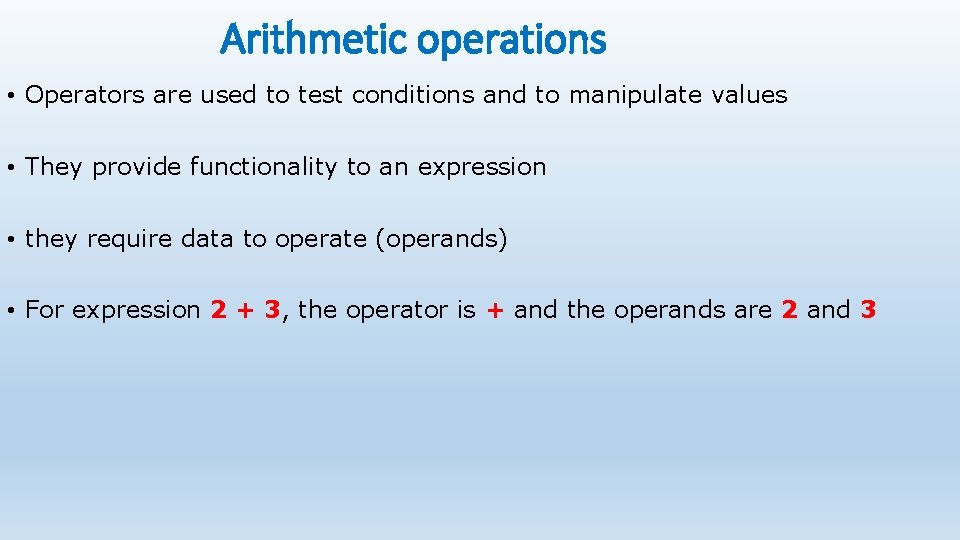
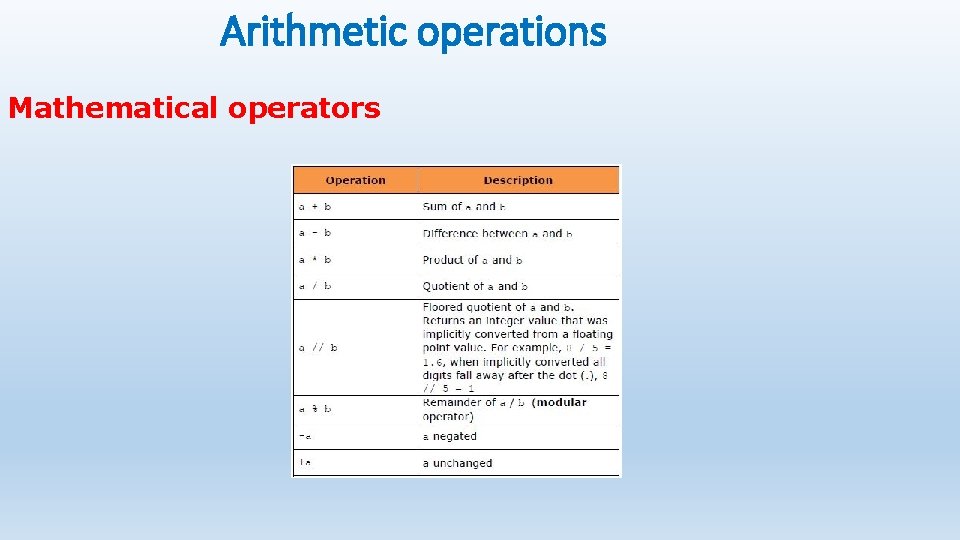
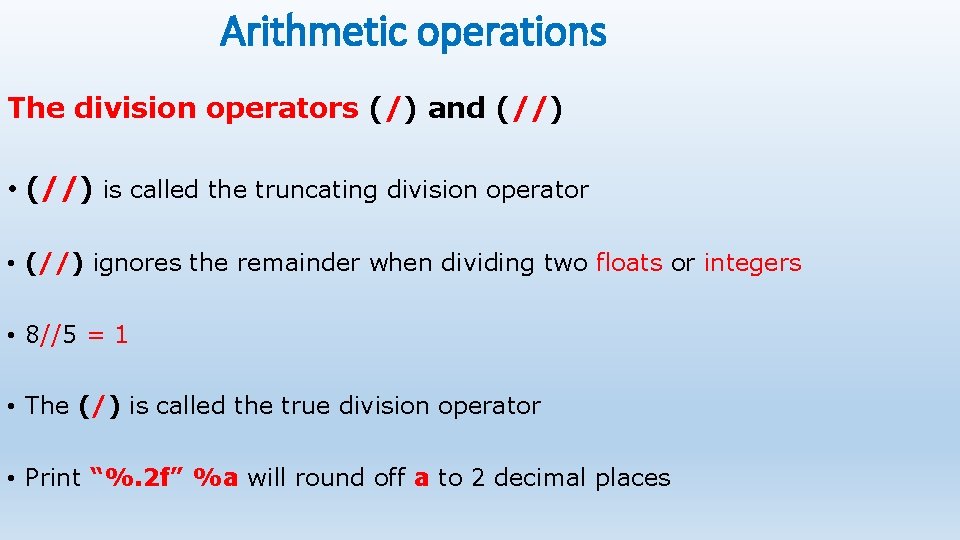
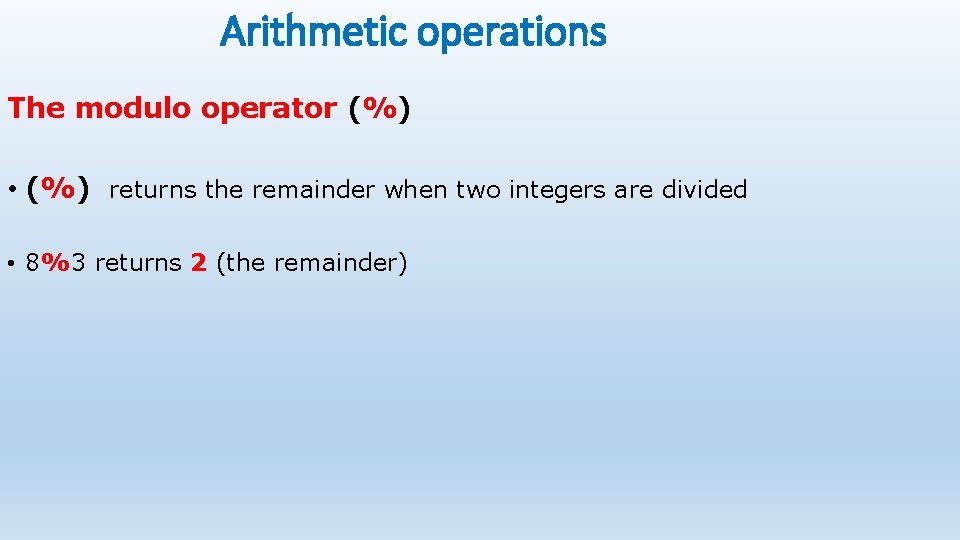
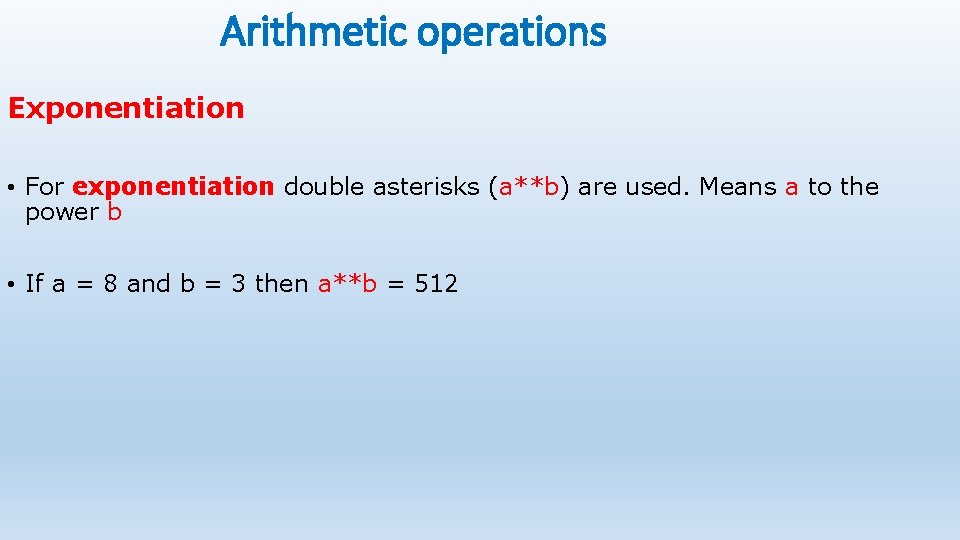
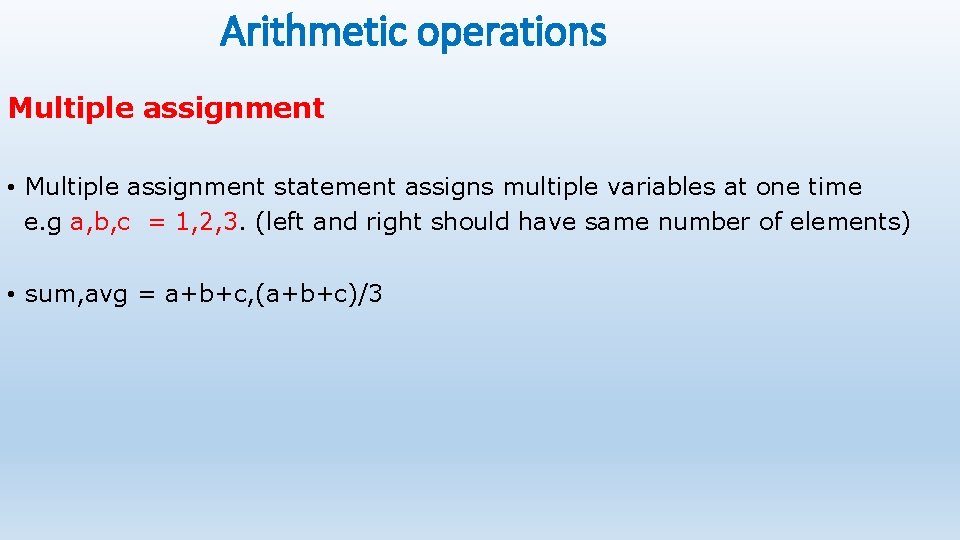
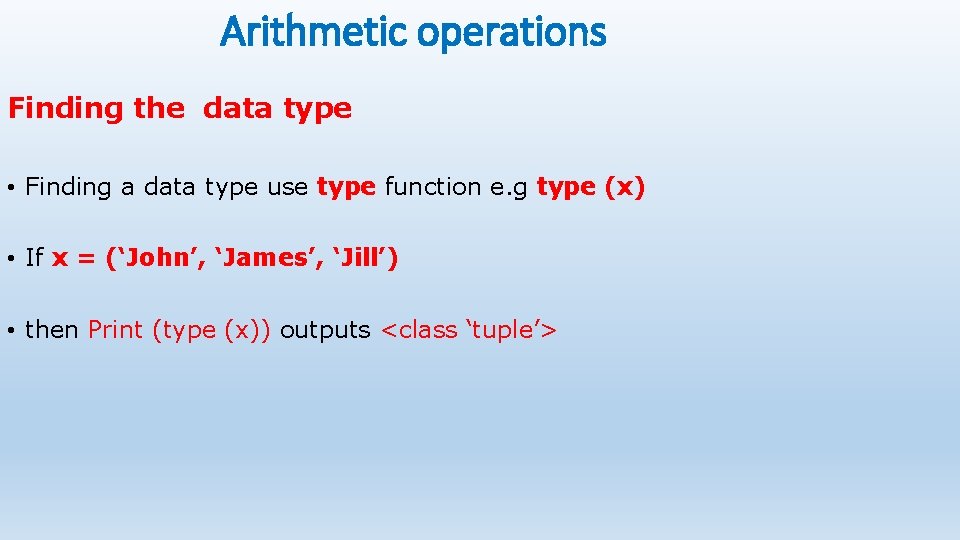
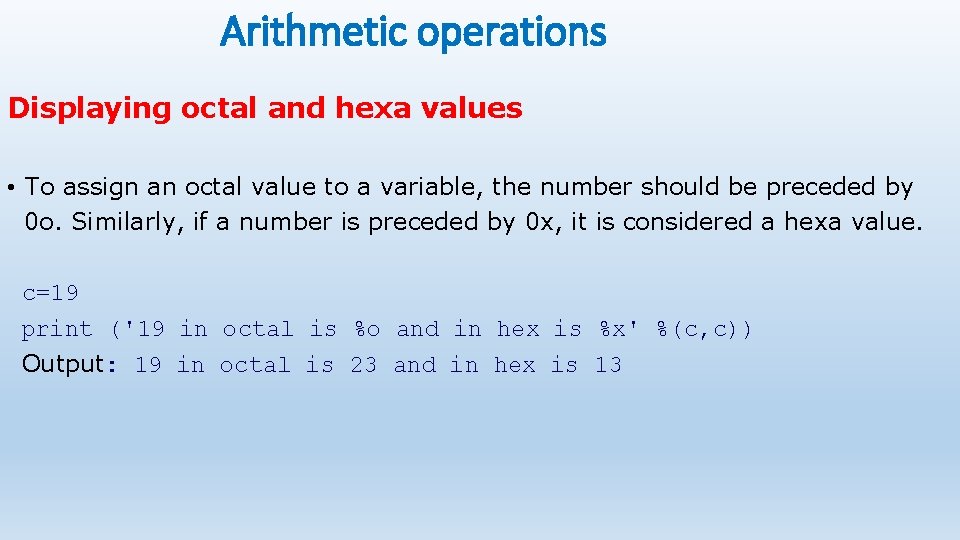
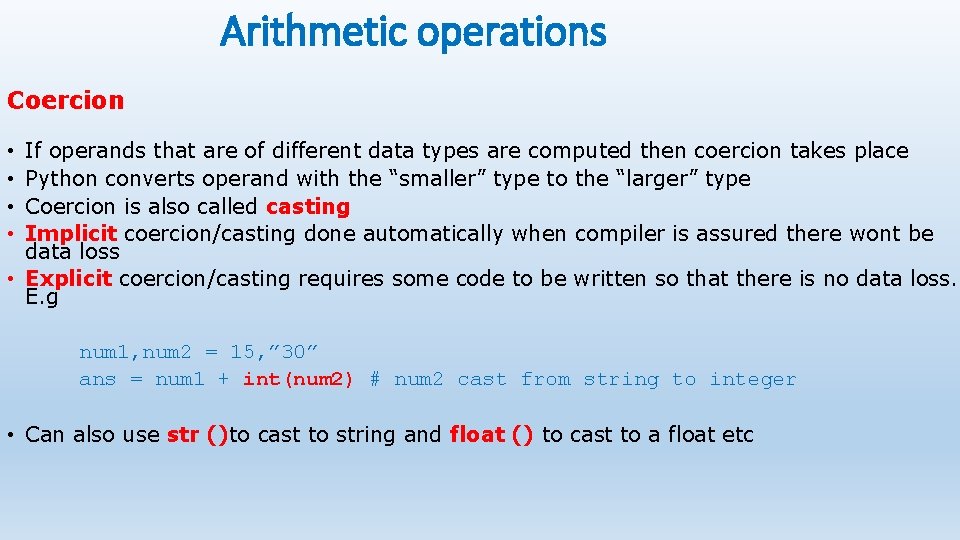
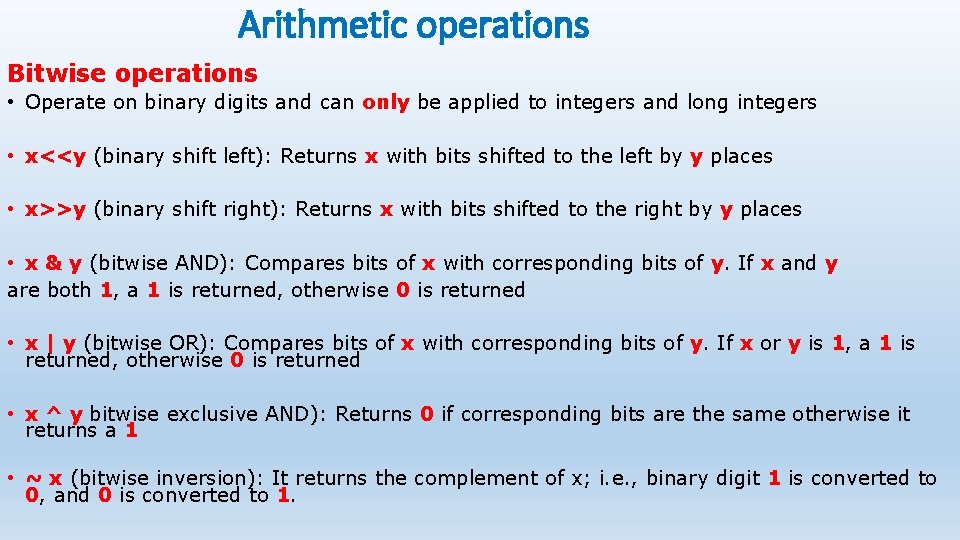
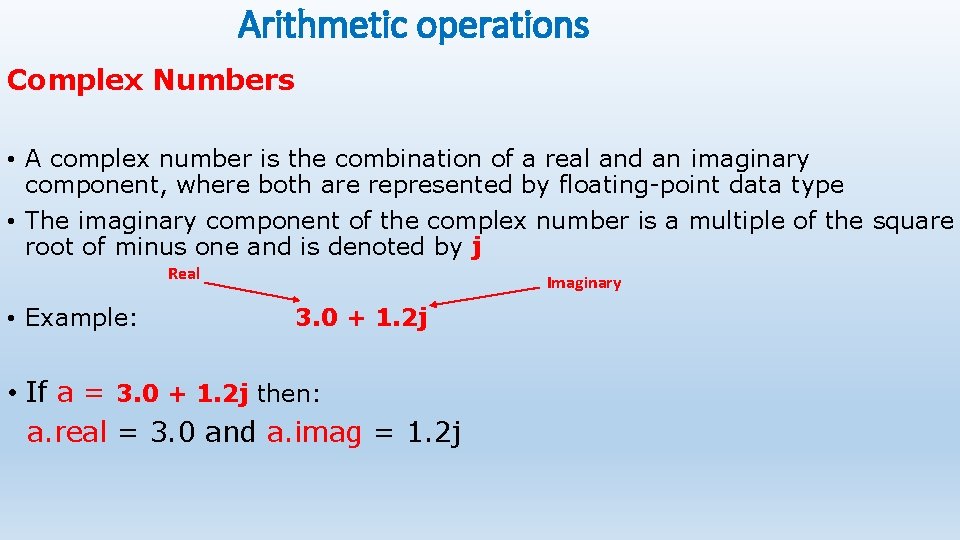
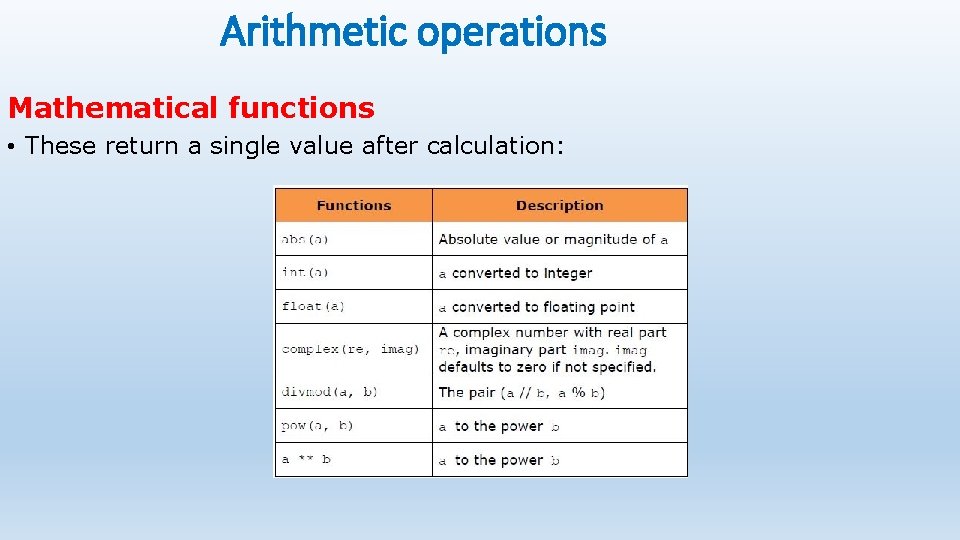
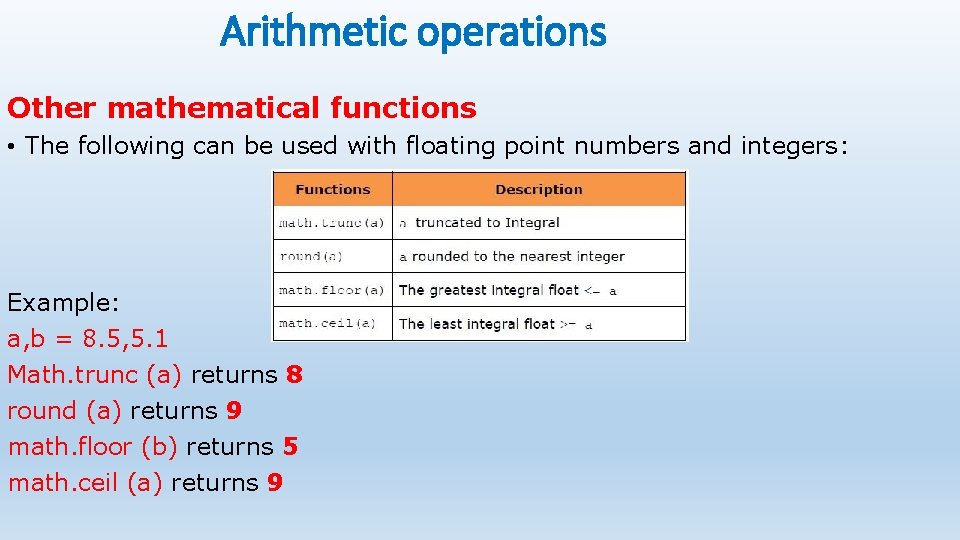
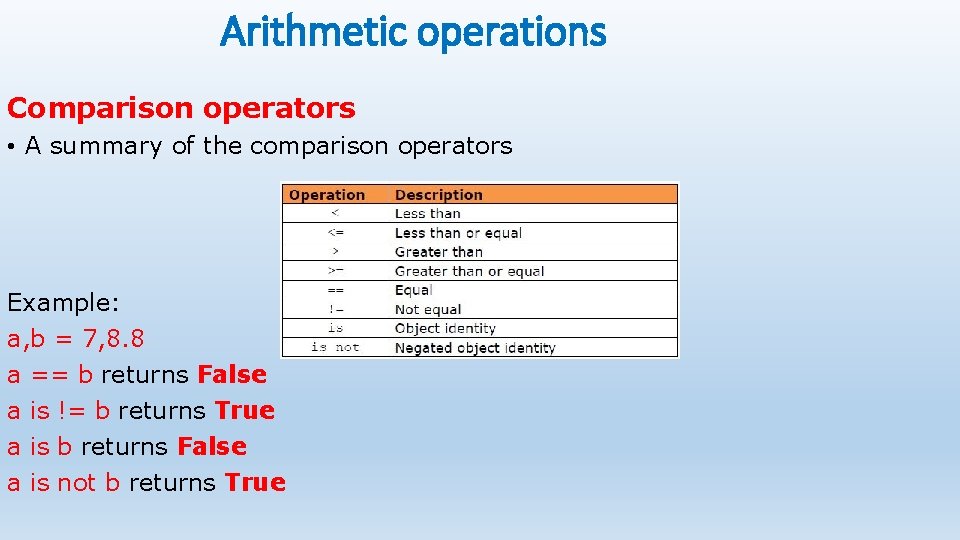
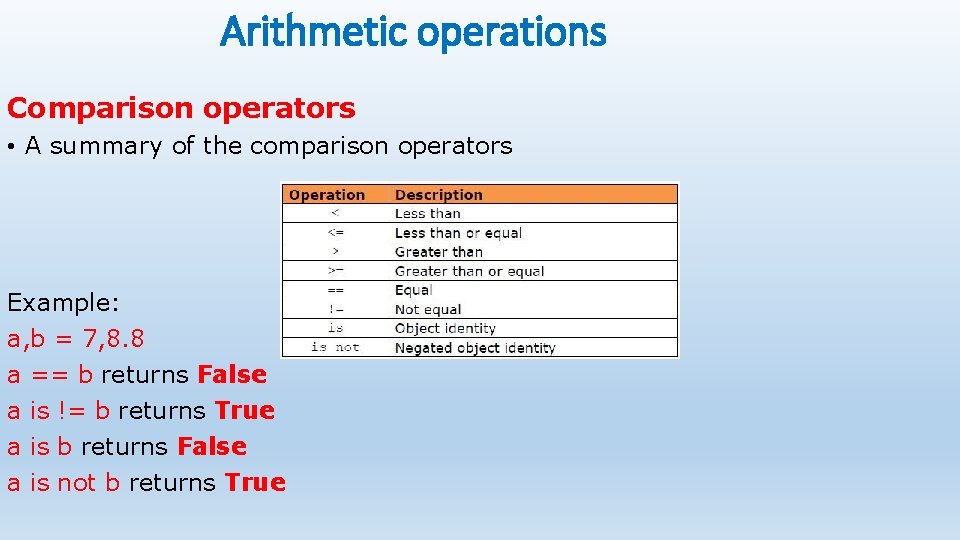
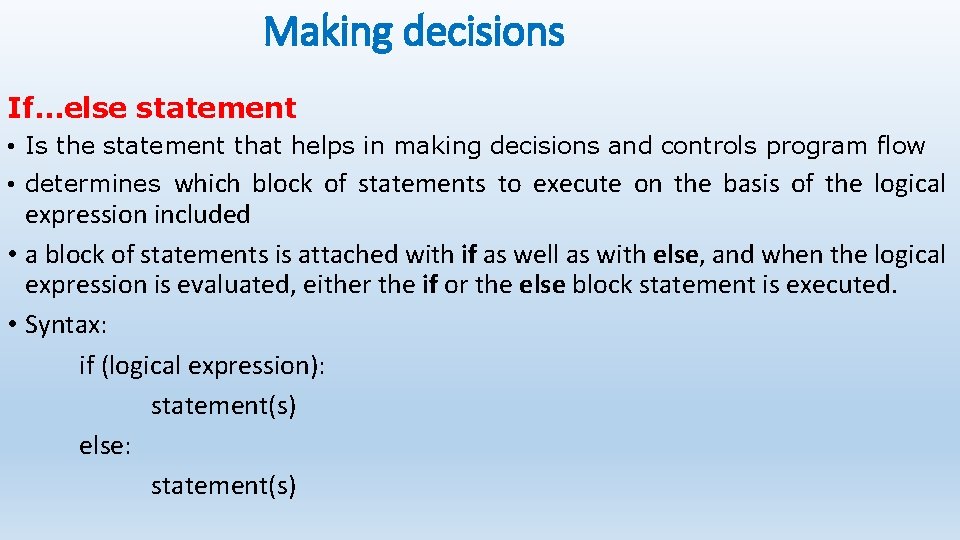
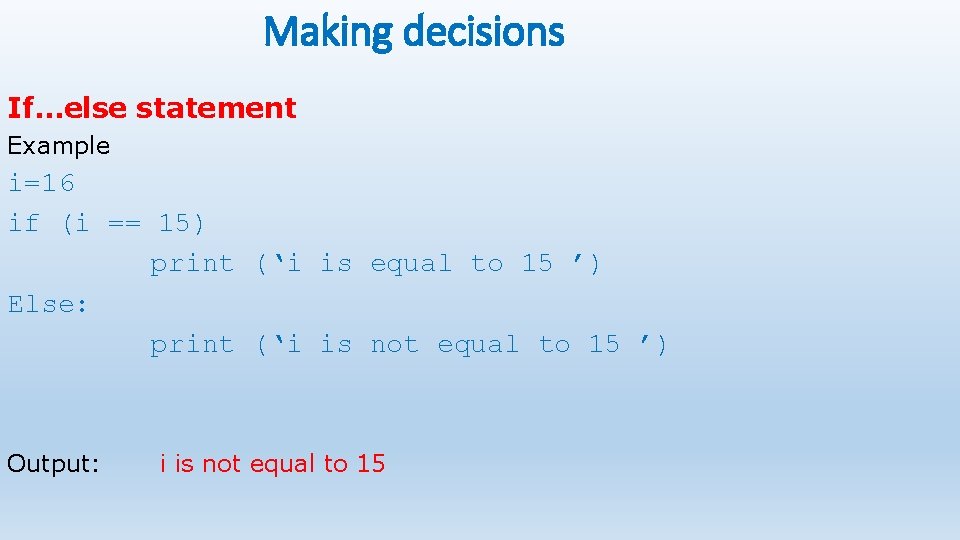
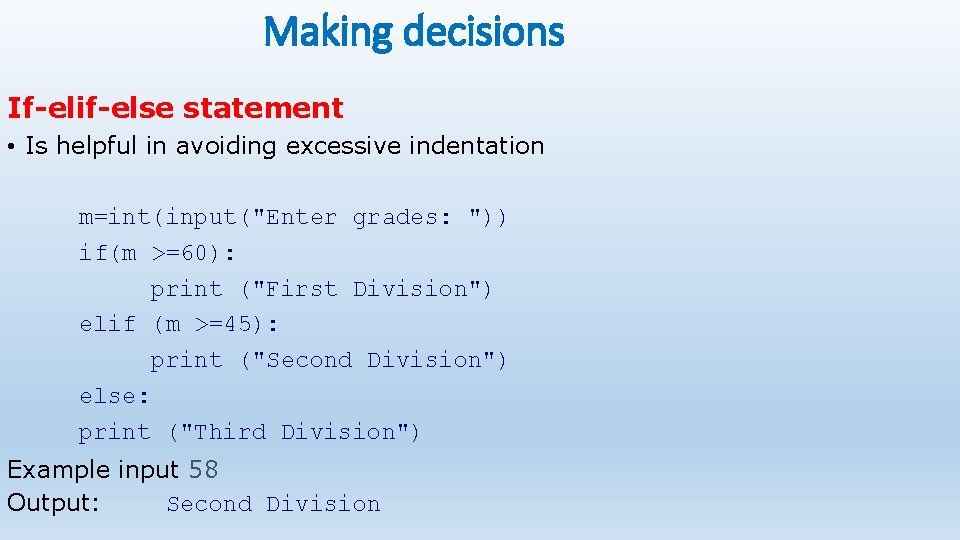
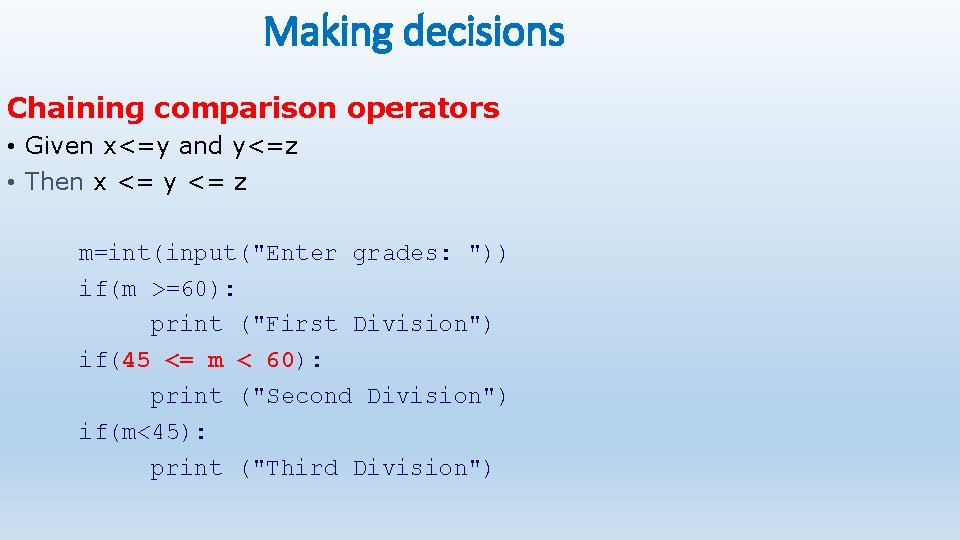
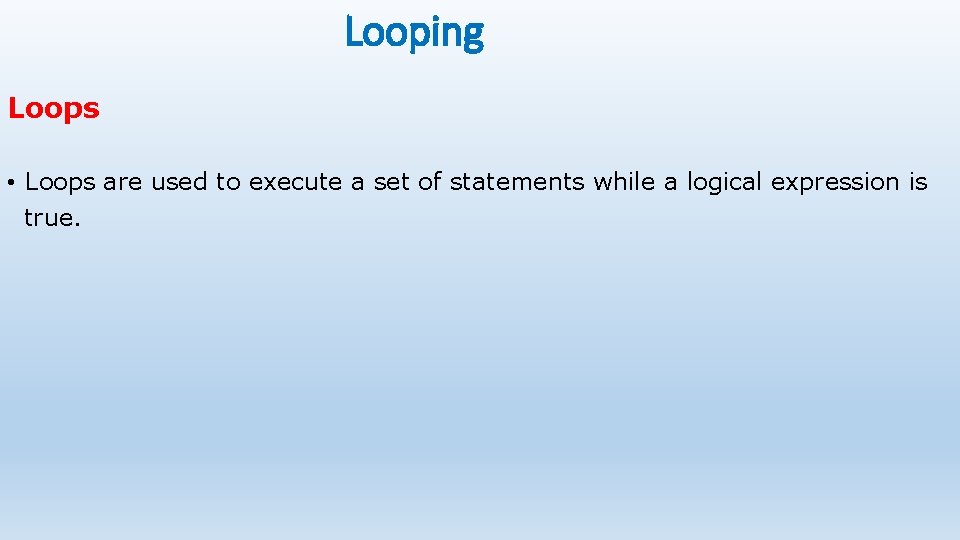
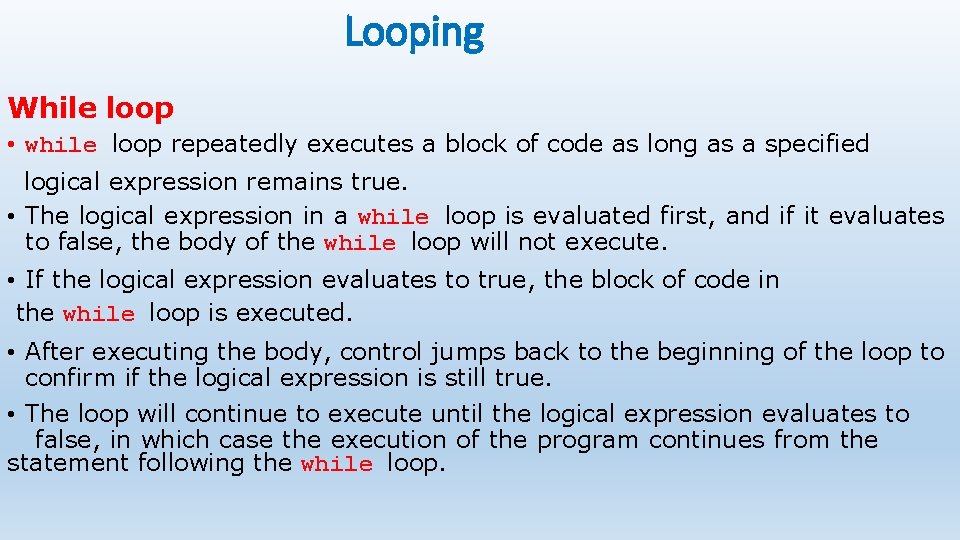
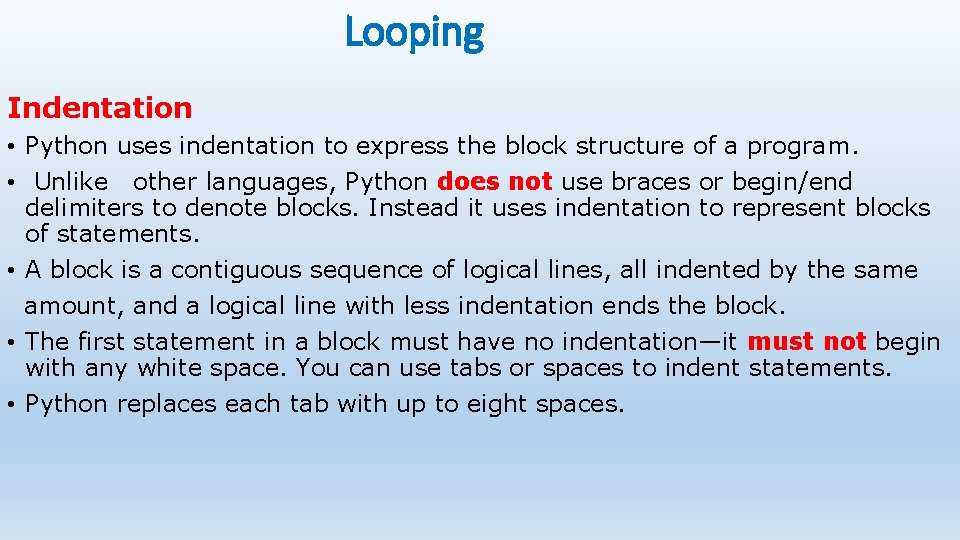
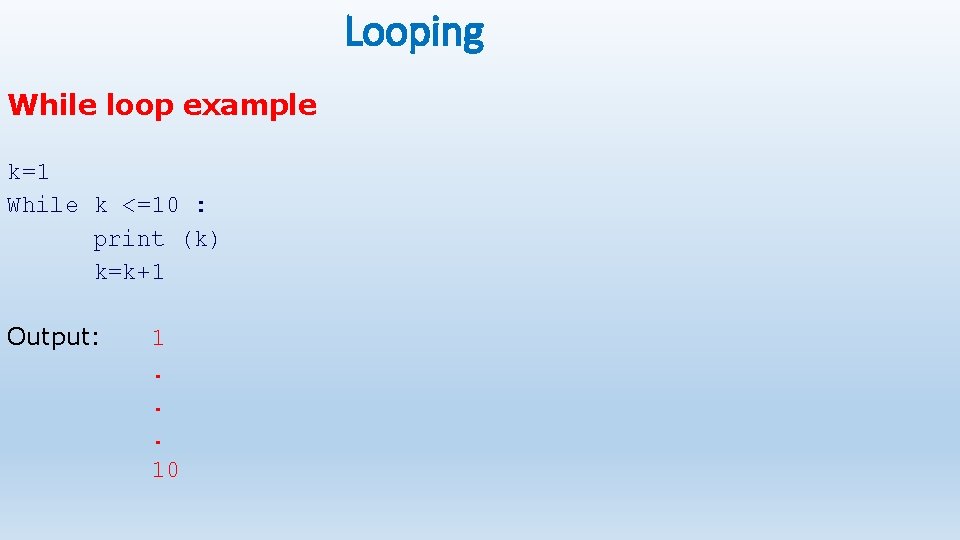
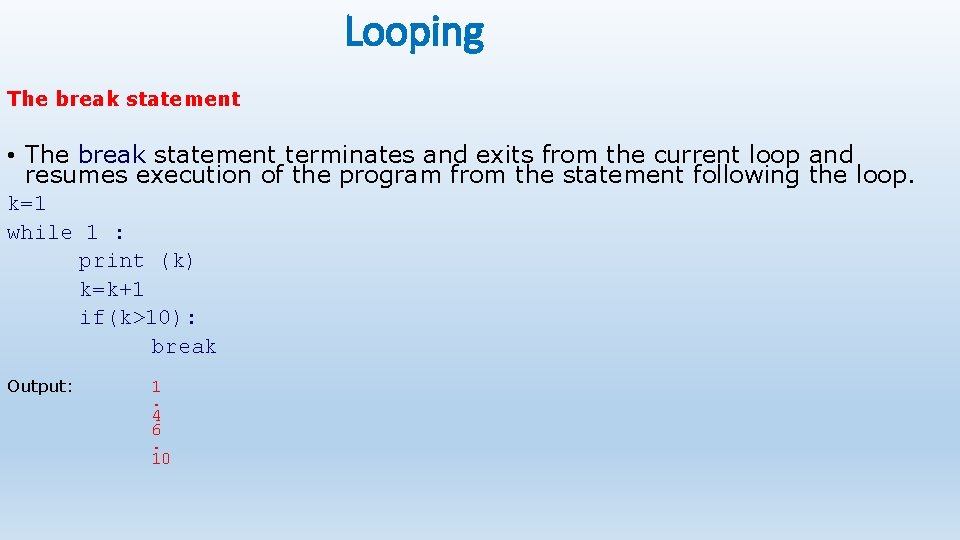
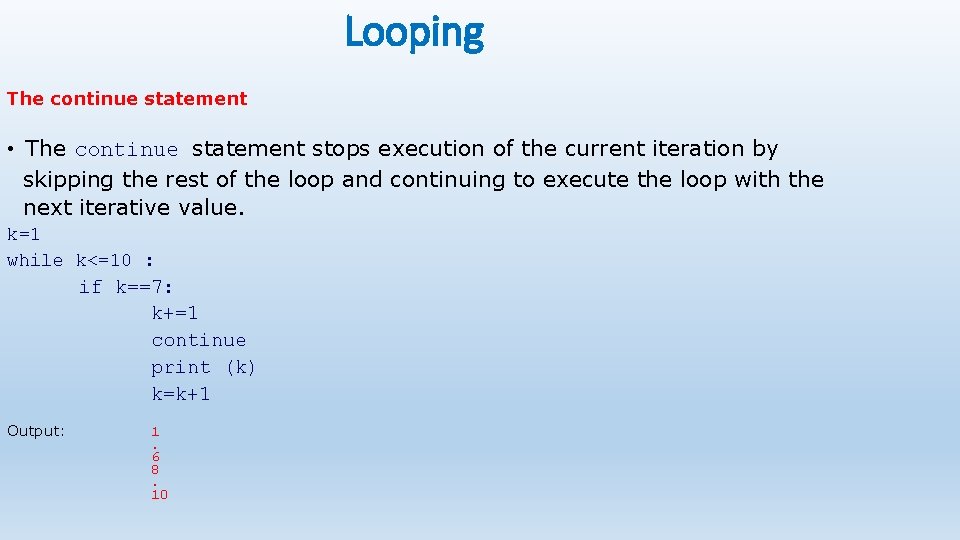
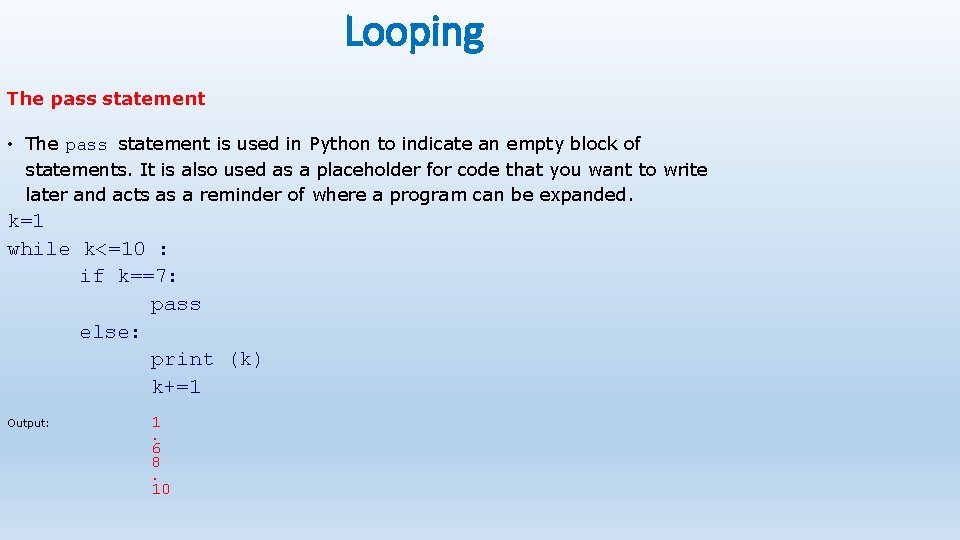
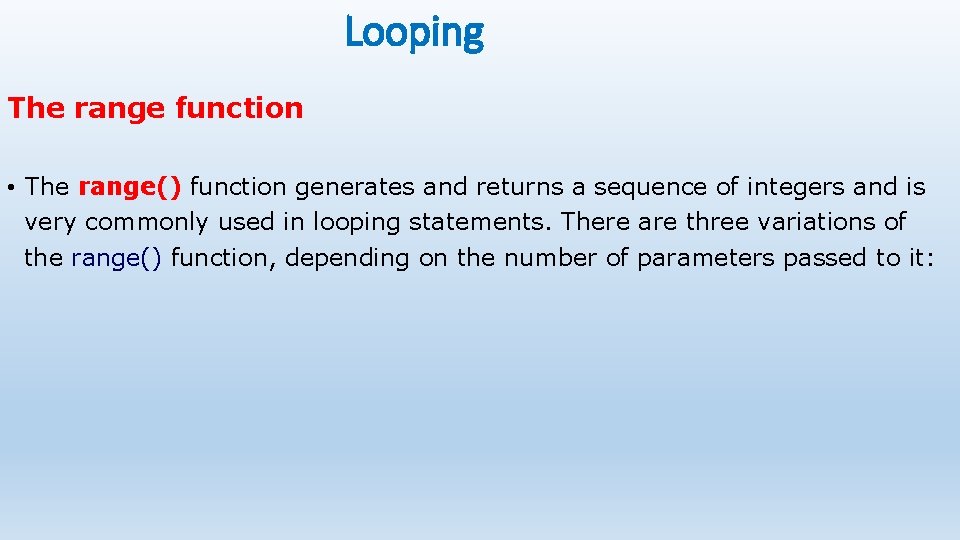
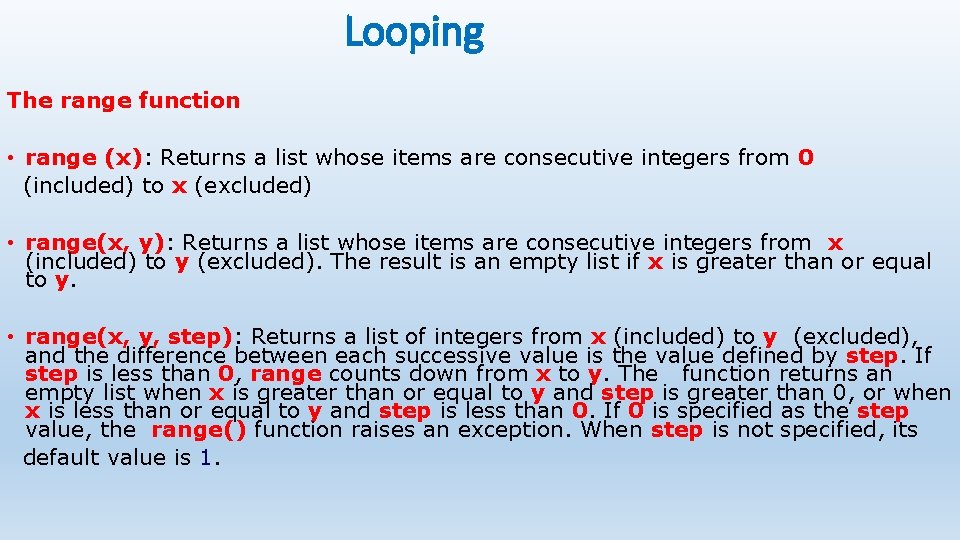
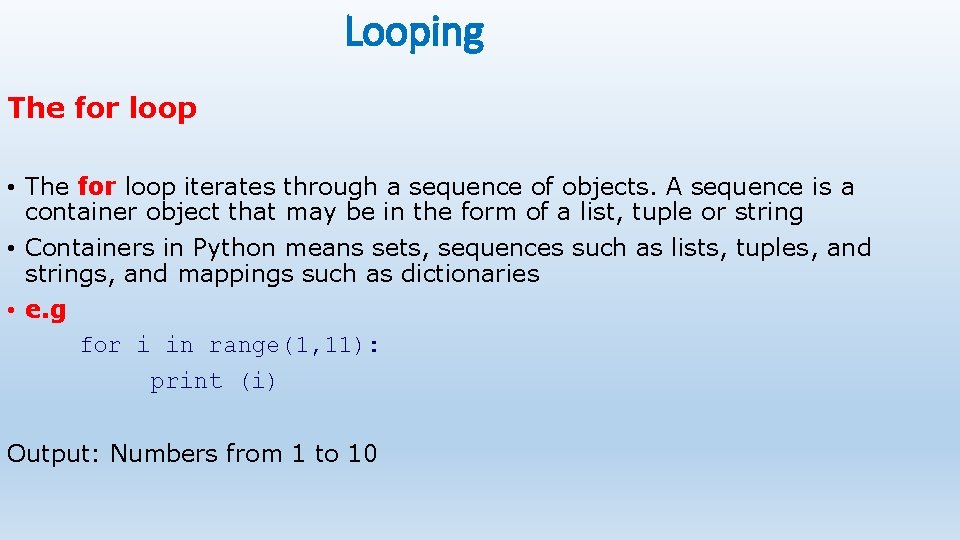
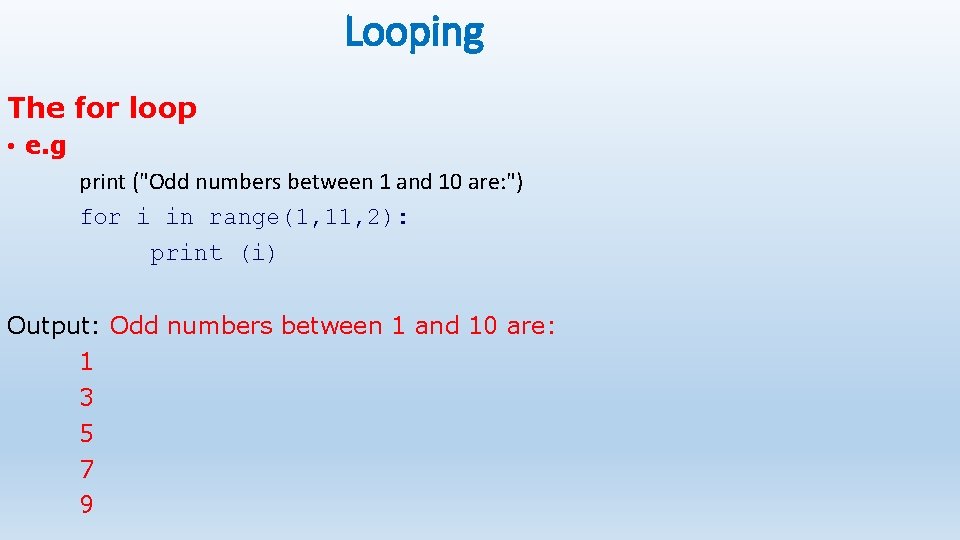
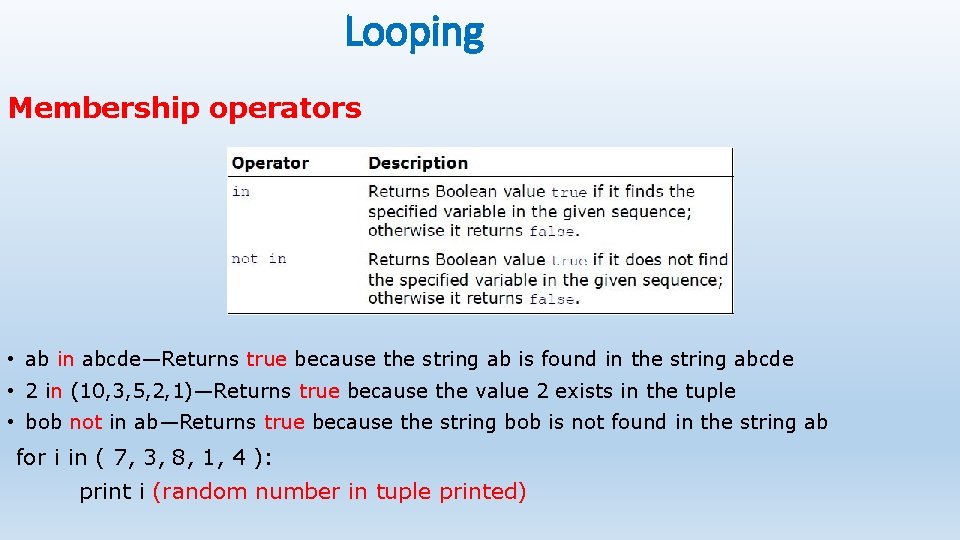
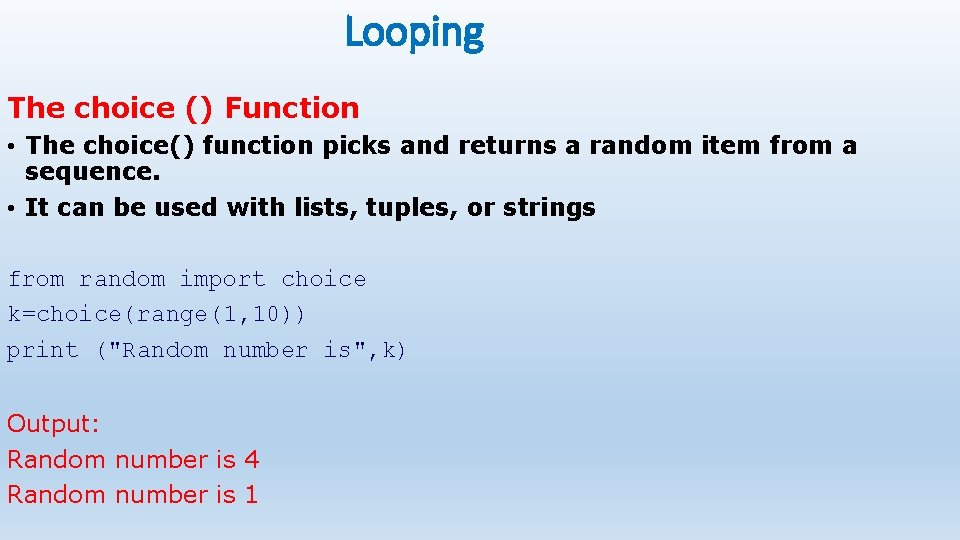
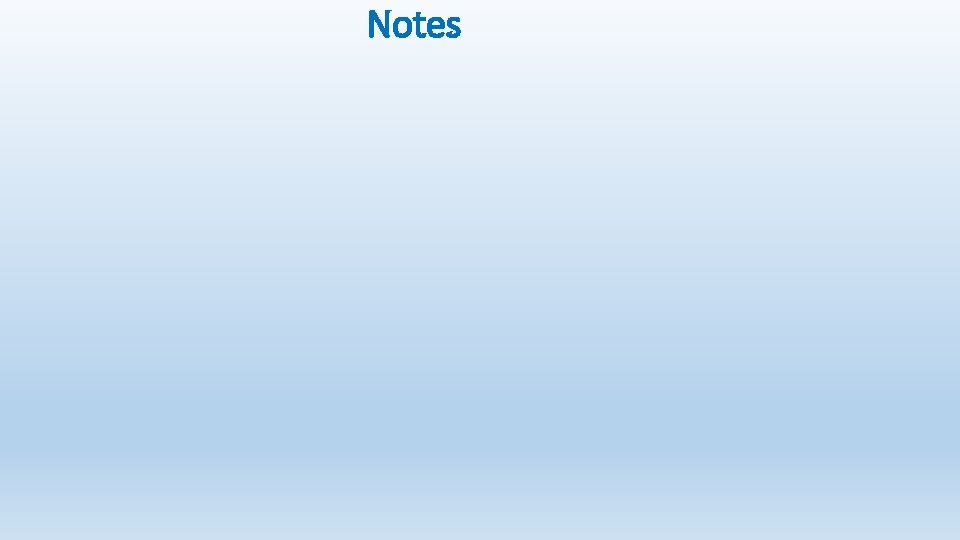
- Slides: 34
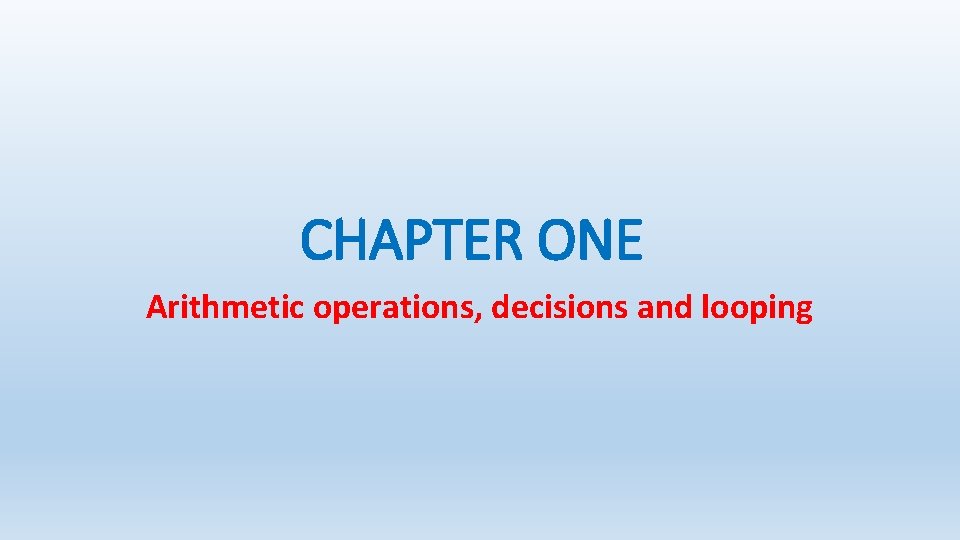
CHAPTER ONE Arithmetic operations, decisions and looping
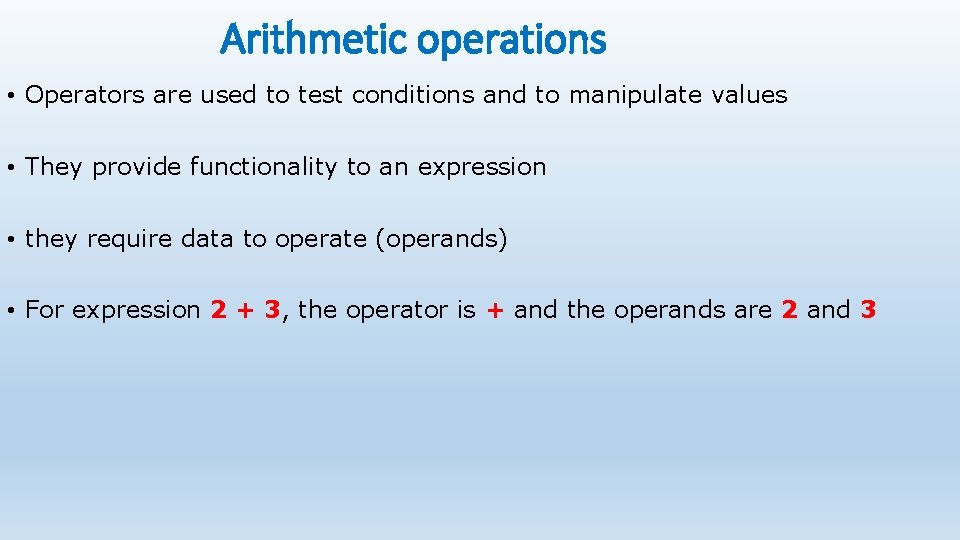
Arithmetic operations • Operators are used to test conditions and to manipulate values • They provide functionality to an expression • they require data to operate (operands) • For expression 2 + 3, the operator is + and the operands are 2 and 3
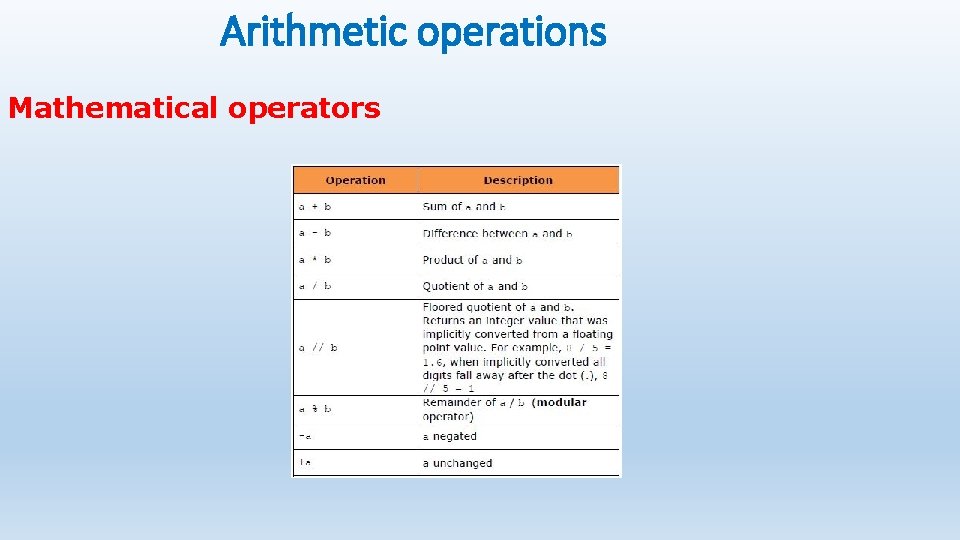
Arithmetic operations Mathematical operators
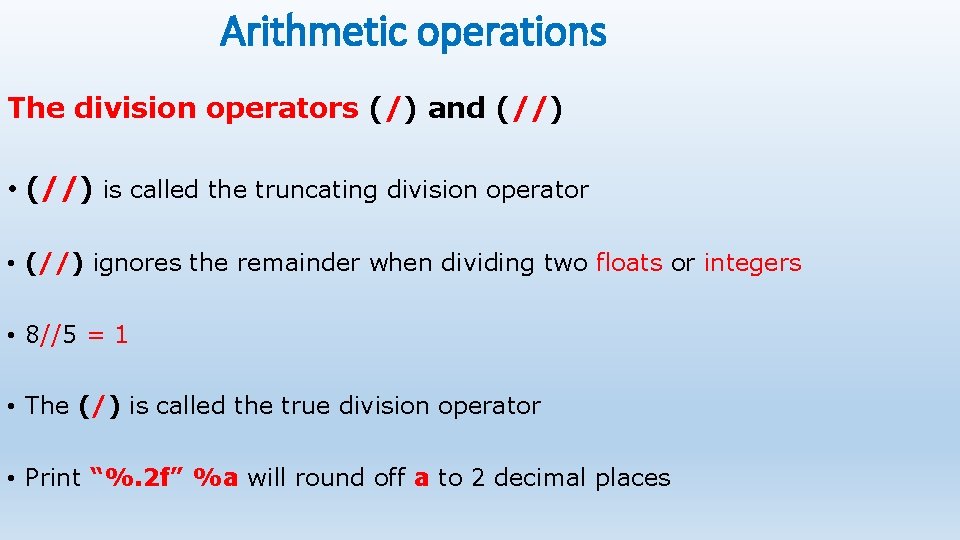
Arithmetic operations The division operators (/) and (//) • (//) is called the truncating division operator • (//) ignores the remainder when dividing two floats or integers • 8//5 = 1 • The (/) is called the true division operator • Print “%. 2 f” %a will round off a to 2 decimal places
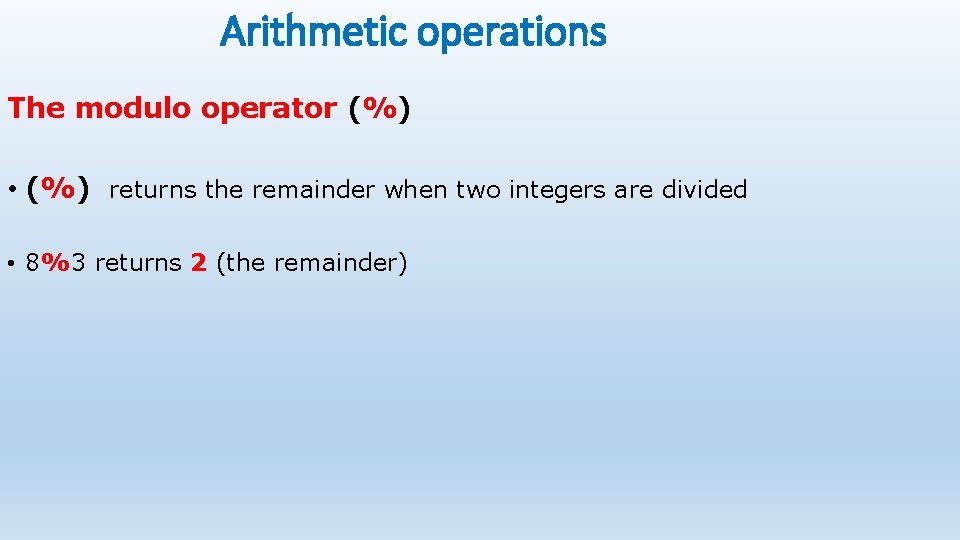
Arithmetic operations The modulo operator (%) • (%) returns the remainder when two integers are divided • 8%3 returns 2 (the remainder)
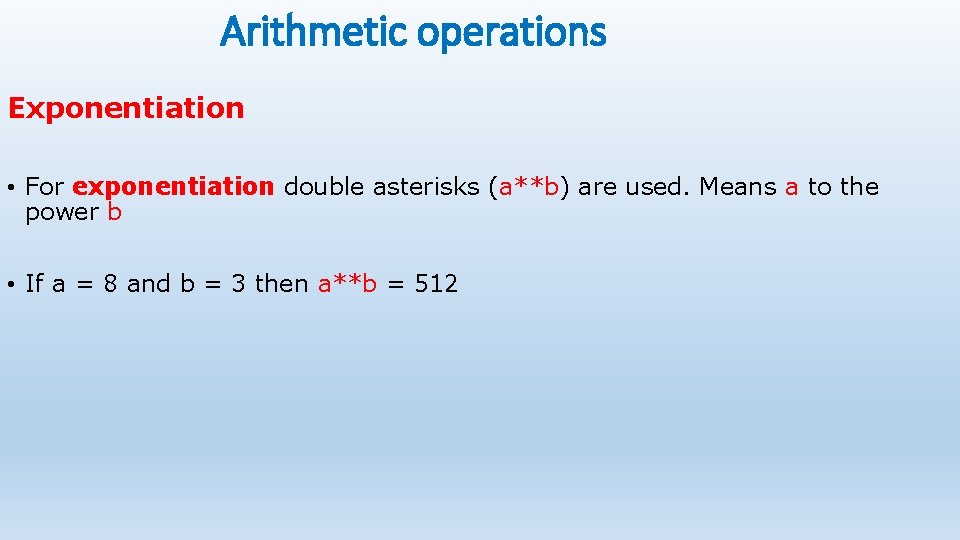
Arithmetic operations Exponentiation • For exponentiation double asterisks (a**b) are used. Means a to the power b • If a = 8 and b = 3 then a**b = 512
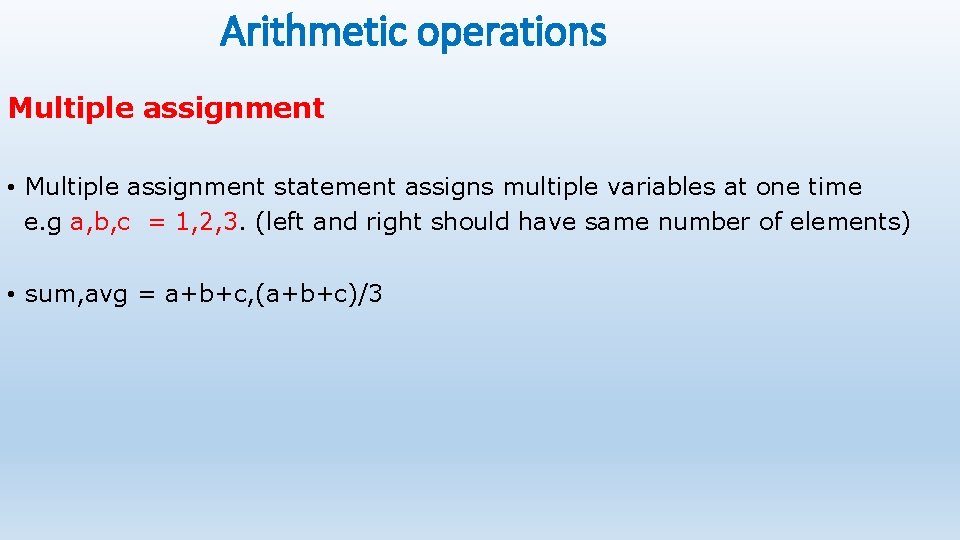
Arithmetic operations Multiple assignment • Multiple assignment statement assigns multiple variables at one time e. g a, b, c = 1, 2, 3. (left and right should have same number of elements) • sum, avg = a+b+c, (a+b+c)/3
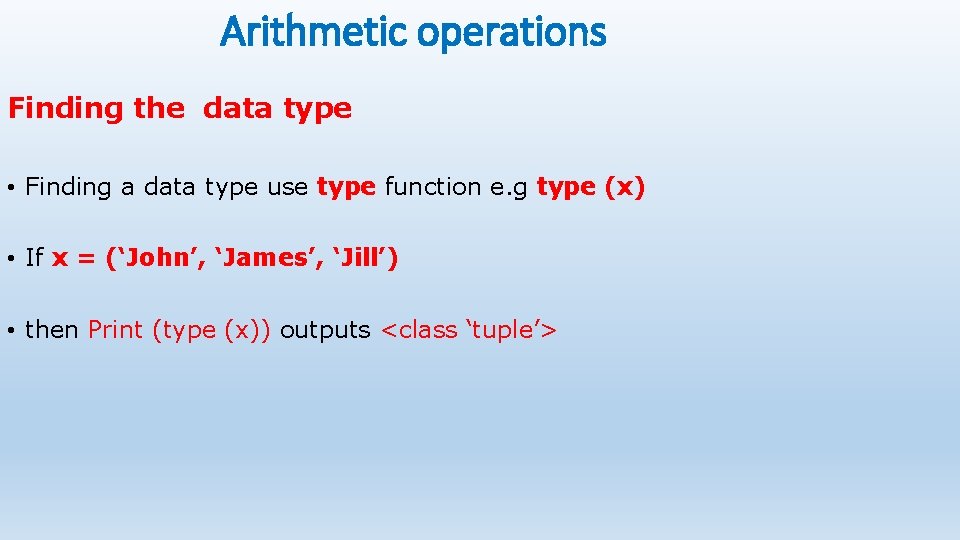
Arithmetic operations Finding the data type • Finding a data type use type function e. g type (x) • If x = (‘John’, ‘James’, ‘Jill’) • then Print (type (x)) outputs <class ‘tuple’>
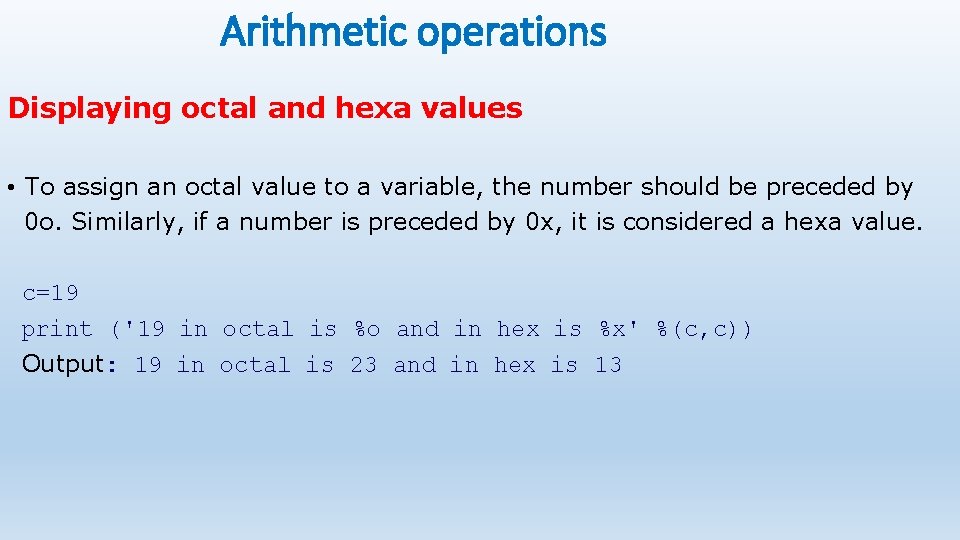
Arithmetic operations Displaying octal and hexa values • To assign an octal value to a variable, the number should be preceded by 0 o. Similarly, if a number is preceded by 0 x, it is considered a hexa value. c=19 print ('19 in octal is %o and in hex is %x' %(c, c)) Output: 19 in octal is 23 and in hex is 13
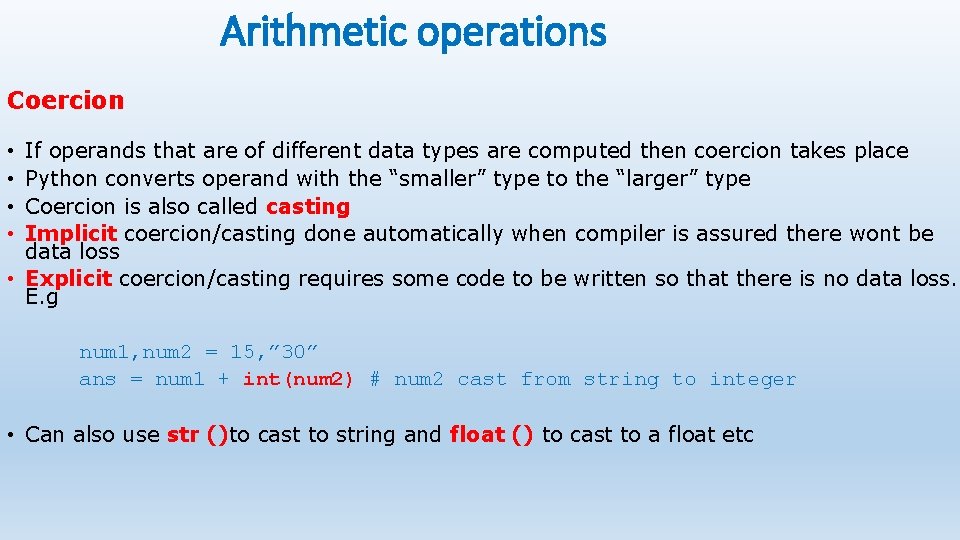
Arithmetic operations Coercion If operands that are of different data types are computed then coercion takes place Python converts operand with the “smaller” type to the “larger” type Coercion is also called casting Implicit coercion/casting done automatically when compiler is assured there wont be data loss • Explicit coercion/casting requires some code to be written so that there is no data loss. E. g • • num 1, num 2 = 15, ” 30” ans = num 1 + int(num 2) # num 2 cast from string to integer • Can also use str ()to cast to string and float () to cast to a float etc
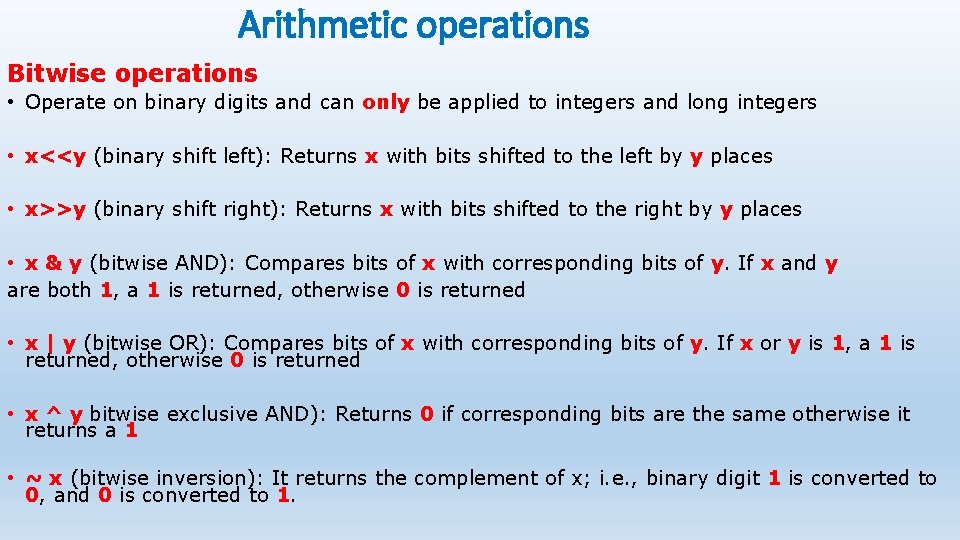
Arithmetic operations Bitwise operations • Operate on binary digits and can only be applied to integers and long integers • x<<y (binary shift left): Returns x with bits shifted to the left by y places • x>>y (binary shift right): Returns x with bits shifted to the right by y places • x & y (bitwise AND): Compares bits of x with corresponding bits of y. If x and y are both 1, a 1 is returned, otherwise 0 is returned • x | y (bitwise OR): Compares bits of x with corresponding bits of y. If x or y is 1, a 1 is returned, otherwise 0 is returned • x ^ y bitwise exclusive AND): Returns 0 if corresponding bits are the same otherwise it returns a 1 • ~ x (bitwise inversion): It returns the complement of x; i. e. , binary digit 1 is converted to 0, and 0 is converted to 1.
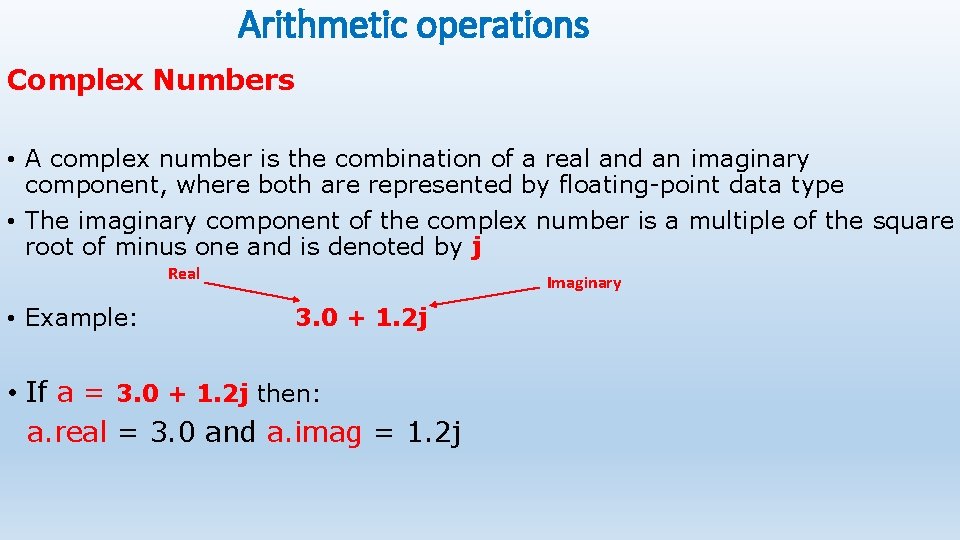
Arithmetic operations Complex Numbers • A complex number is the combination of a real and an imaginary component, where both are represented by floating-point data type • The imaginary component of the complex number is a multiple of the square root of minus one and is denoted by j Real • Example: Imaginary 3. 0 + 1. 2 j • If a = 3. 0 + 1. 2 j then: a. real = 3. 0 and a. imag = 1. 2 j
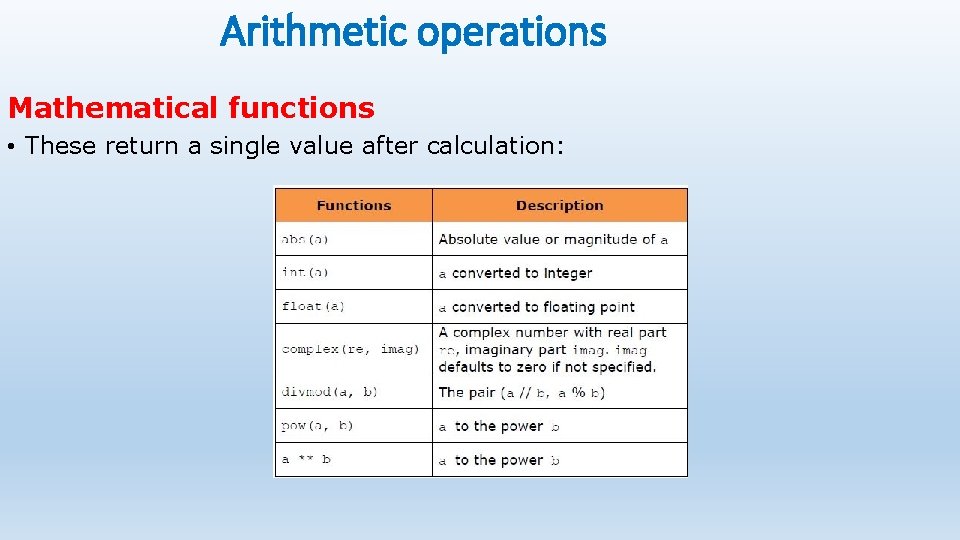
Arithmetic operations Mathematical functions • These return a single value after calculation:
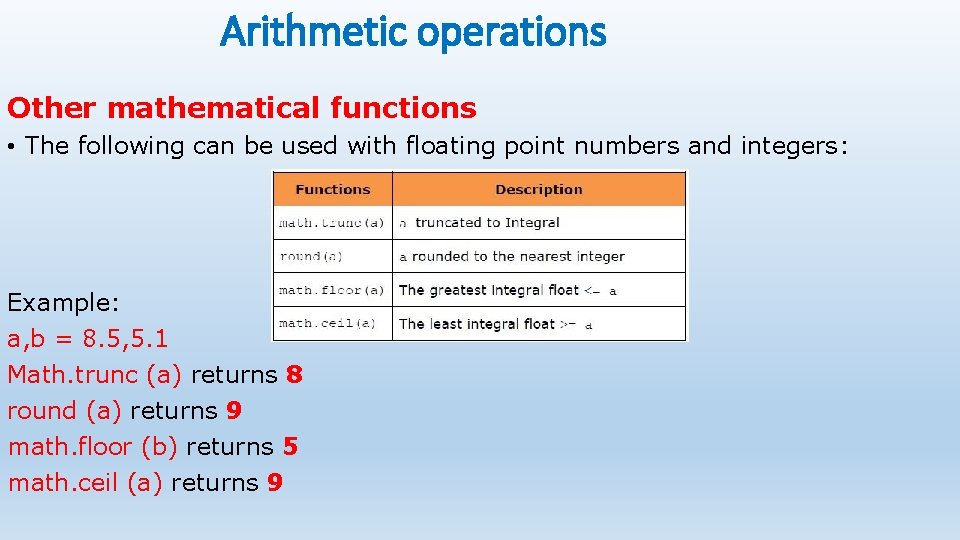
Arithmetic operations Other mathematical functions • The following can be used with floating point numbers and integers: Example: a, b = 8. 5, 5. 1 Math. trunc (a) returns 8 round (a) returns 9 math. floor (b) returns 5 math. ceil (a) returns 9
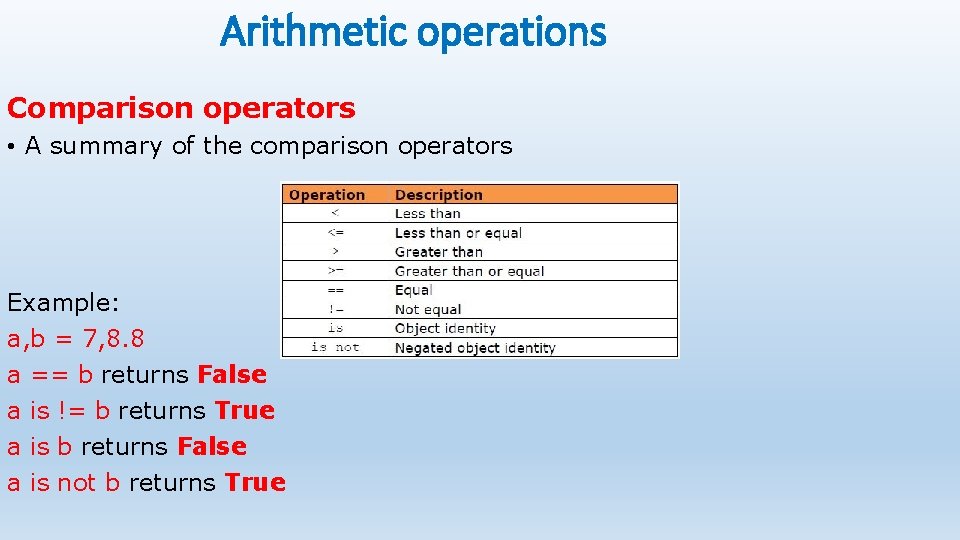
Arithmetic operations Comparison operators • A summary of the comparison operators Example: a, b = 7, 8. 8 a == b returns False a is != b returns True a is b returns False a is not b returns True
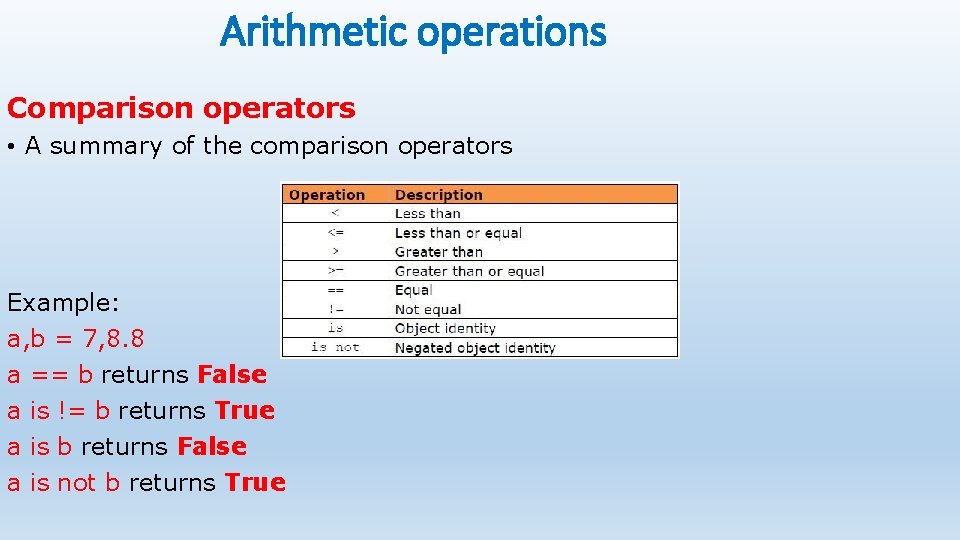
Arithmetic operations Comparison operators • A summary of the comparison operators Example: a, b = 7, 8. 8 a == b returns False a is != b returns True a is b returns False a is not b returns True
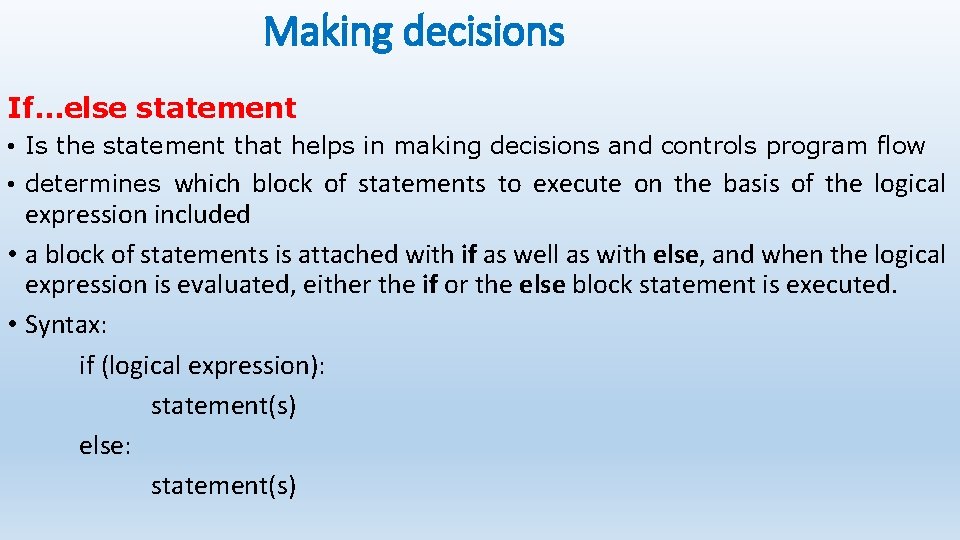
Making decisions If…else statement • Is the statement that helps in making decisions and controls program flow • determines which block of statements to execute on the basis of the logical expression included • a block of statements is attached with if as well as with else, and when the logical expression is evaluated, either the if or the else block statement is executed. • Syntax: if (logical expression): statement(s) else: statement(s)
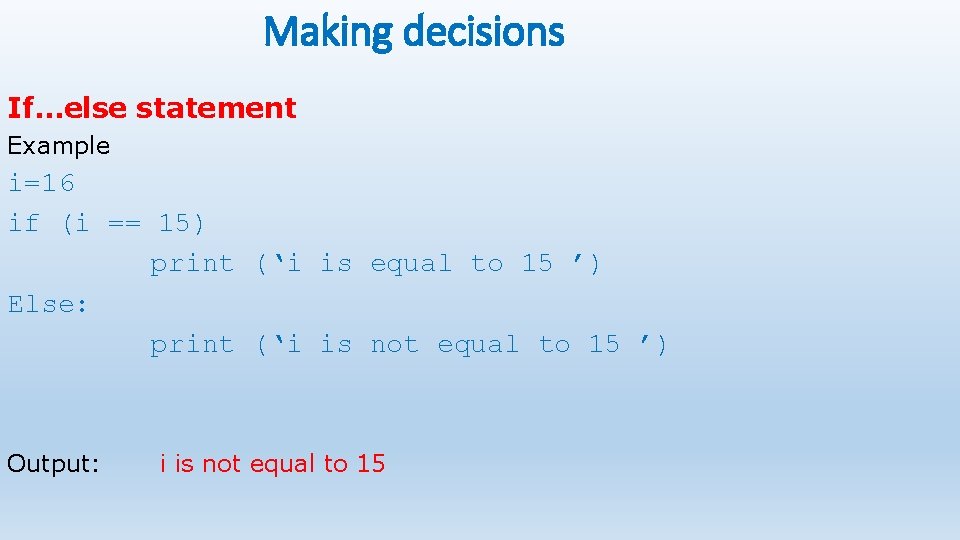
Making decisions If…else statement Example i=16 if (i == 15) print (‘i is equal to 15 ’) Else: print (‘i is not equal to 15 ’) Output: i is not equal to 15
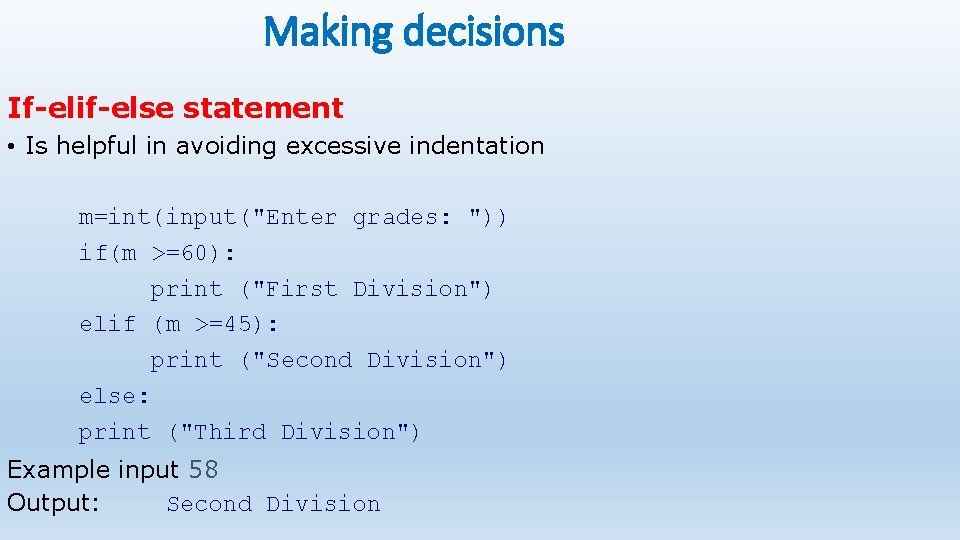
Making decisions If-elif-else statement • Is helpful in avoiding excessive indentation m=int(input("Enter grades: ")) if(m >=60): print ("First Division") elif (m >=45): print ("Second Division") else: print ("Third Division") Example input 58 Output: Second Division
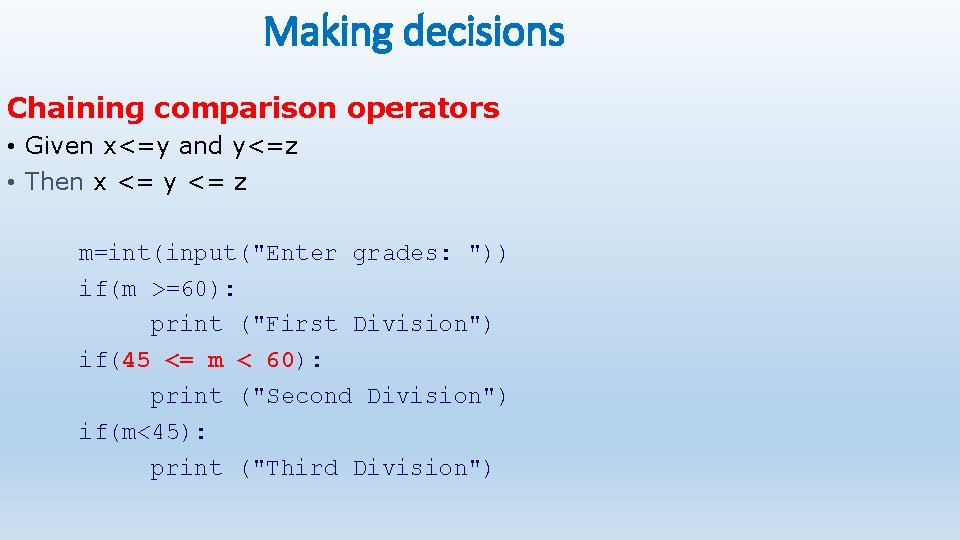
Making decisions Chaining comparison operators • Given x<=y and y<=z • Then x <= y <= z m=int(input("Enter grades: ")) if(m >=60): print ("First Division") if(45 <= m < 60): print ("Second Division") if(m<45): print ("Third Division")
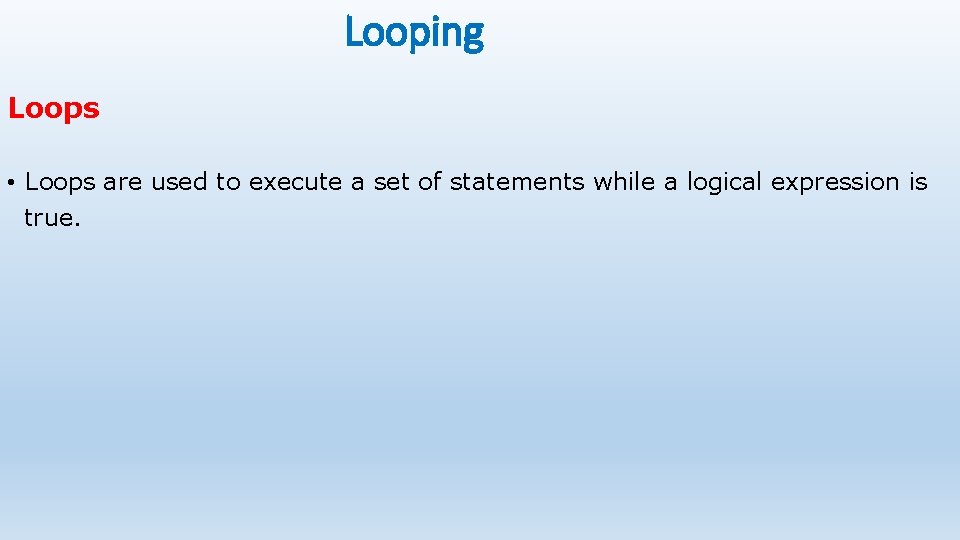
Looping Loops • Loops are used to execute a set of statements while a logical expression is true.
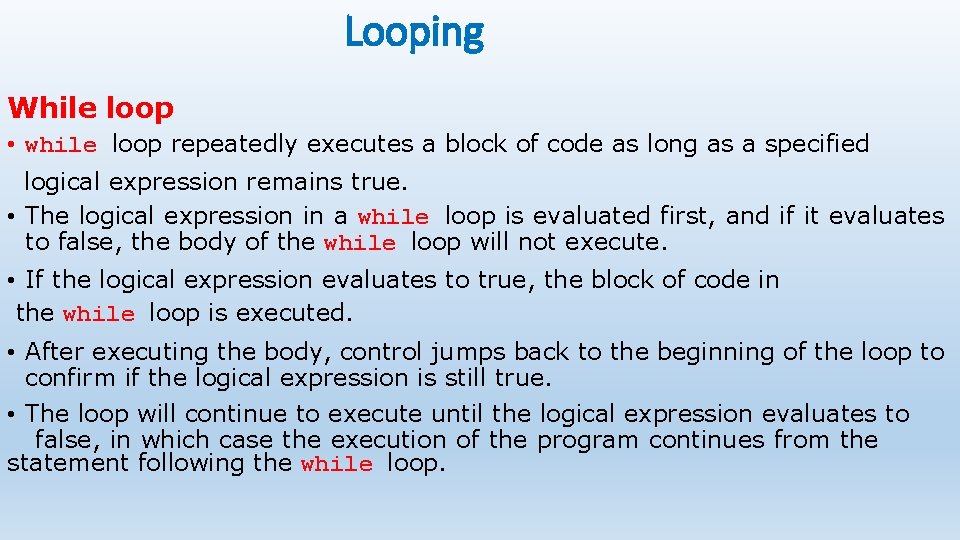
Looping While loop • while loop repeatedly executes a block of code as long as a specified logical expression remains true. • The logical expression in a while loop is evaluated first, and if it evaluates to false, the body of the while loop will not execute. • If the logical expression evaluates to true, the block of code in the while loop is executed. • After executing the body, control jumps back to the beginning of the loop to confirm if the logical expression is still true. • The loop will continue to execute until the logical expression evaluates to false, in which case the execution of the program continues from the statement following the while loop.
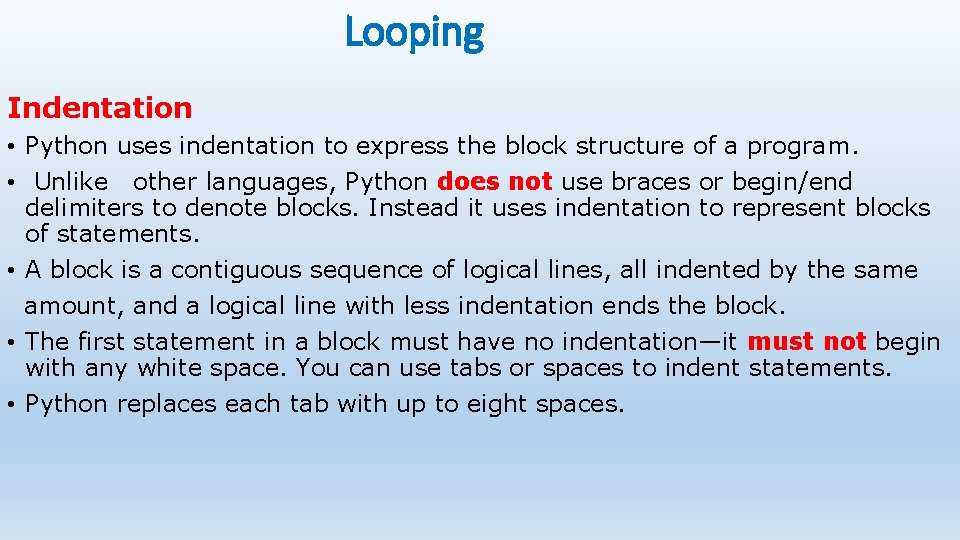
Looping Indentation • Python uses indentation to express the block structure of a program. • Unlike other languages, Python does not use braces or begin/end delimiters to denote blocks. Instead it uses indentation to represent blocks of statements. • A block is a contiguous sequence of logical lines, all indented by the same amount, and a logical line with less indentation ends the block. • The first statement in a block must have no indentation—it must not begin with any white space. You can use tabs or spaces to indent statements. • Python replaces each tab with up to eight spaces.
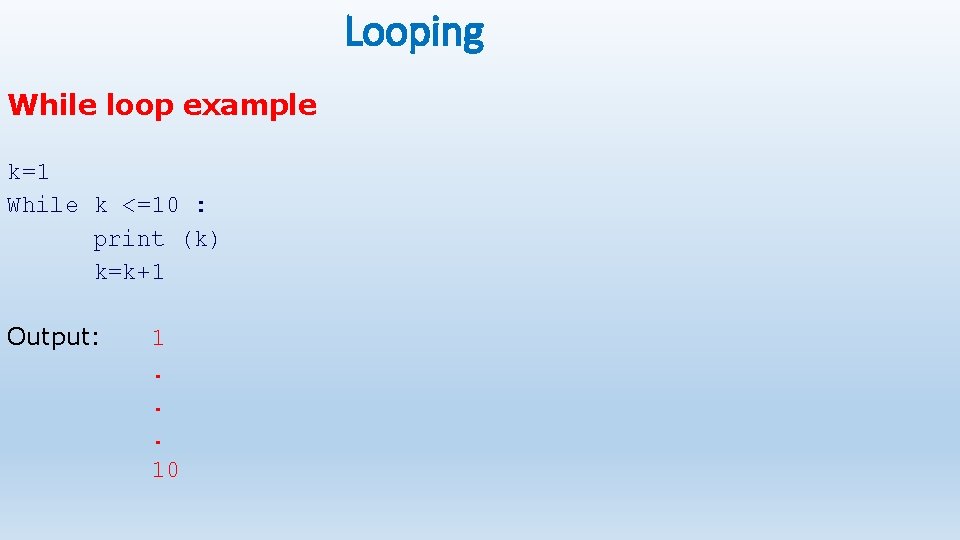
Looping While loop example k=1 While k <=10 : print (k) k=k+1 Output: 1. . . 10
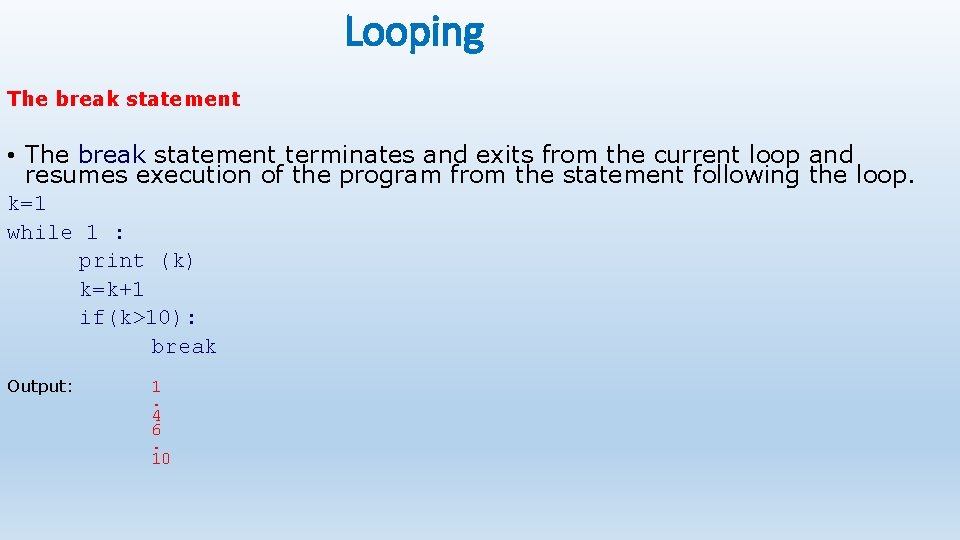
Looping The break statement • The break statement terminates and exits from the current loop and resumes execution of the program from the statement following the loop. k=1 while 1 : print (k) k=k+1 if(k>10): break Output: 1. 4 6. 10
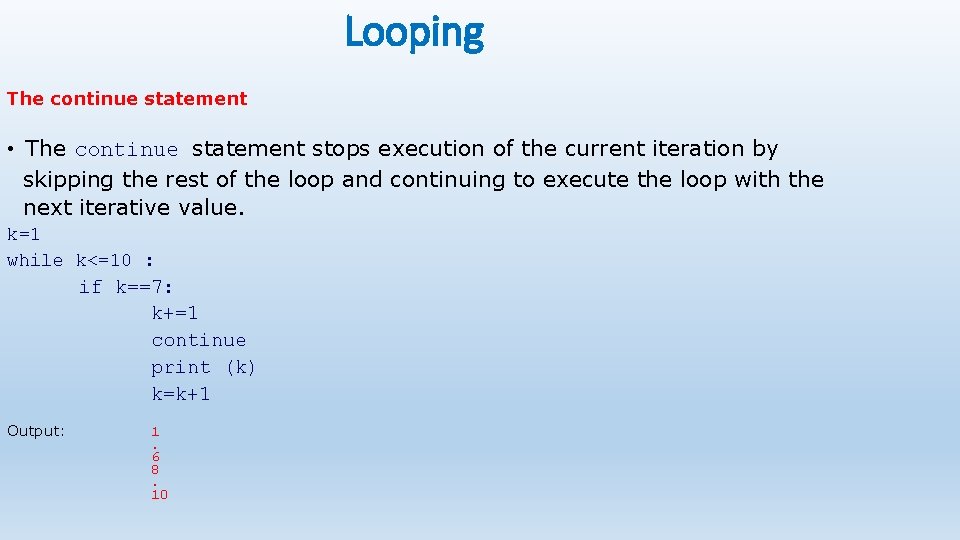
Looping The continue statement • The continue statement stops execution of the current iteration by skipping the rest of the loop and continuing to execute the loop with the next iterative value. k=1 while k<=10 : if k==7: k+=1 continue print (k) k=k+1 Output: 1. 6 8. 10
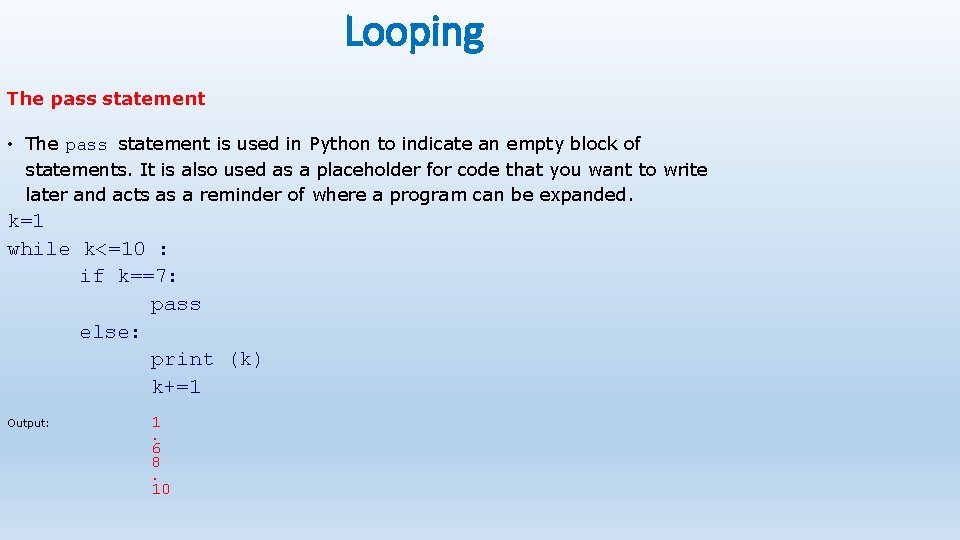
Looping The pass statement • The pass statement is used in Python to indicate an empty block of statements. It is also used as a placeholder for code that you want to write later and acts as a reminder of where a program can be expanded. k=1 while k<=10 : if k==7: pass else: print (k) k+=1 Output: 1. 6 8. 10
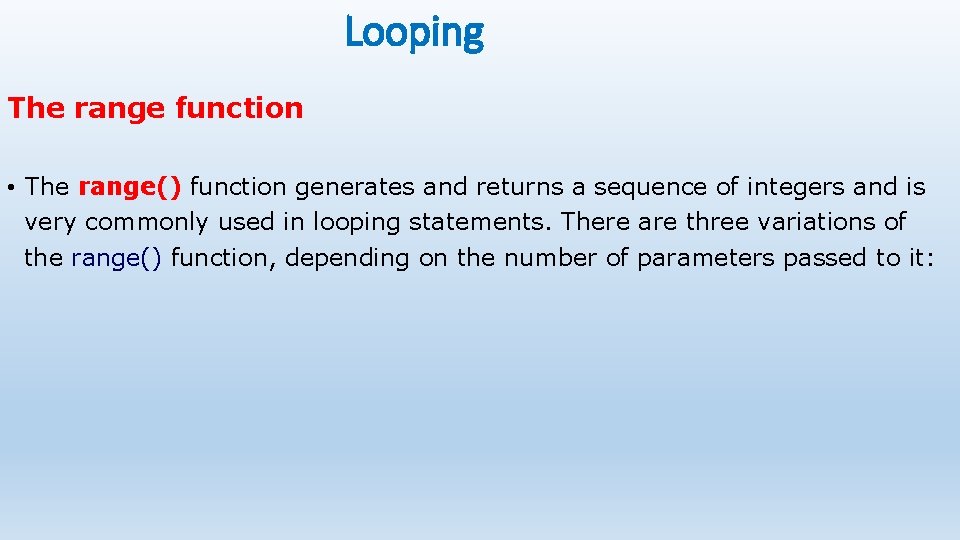
Looping The range function • The range() function generates and returns a sequence of integers and is very commonly used in looping statements. There are three variations of the range() function, depending on the number of parameters passed to it:
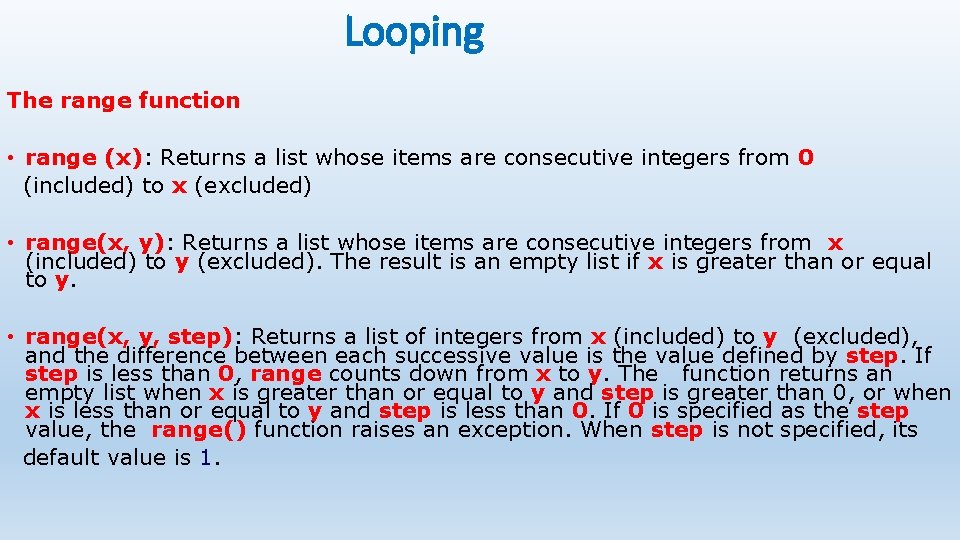
Looping The range function • range (x): Returns a list whose items are consecutive integers from 0 (included) to x (excluded) • range(x, y): Returns a list whose items are consecutive integers from x (included) to y (excluded). The result is an empty list if x is greater than or equal to y. • range(x, y, step): Returns a list of integers from x (included) to y (excluded), and the difference between each successive value is the value defined by step. If step is less than 0, range counts down from x to y. The function returns an empty list when x is greater than or equal to y and step is greater than 0, or when x is less than or equal to y and step is less than 0. If 0 is specified as the step value, the range() function raises an exception. When step is not specified, its default value is 1.
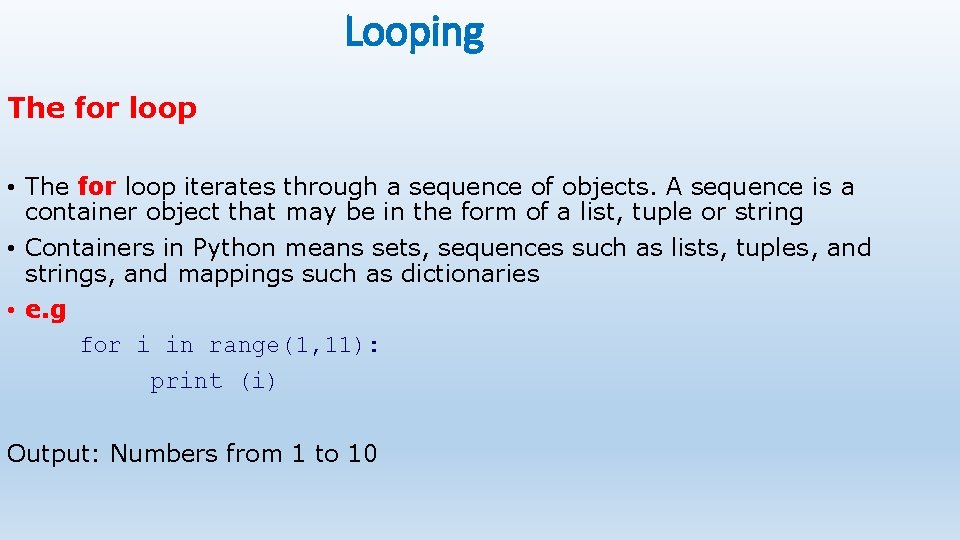
Looping The for loop • The for loop iterates through a sequence of objects. A sequence is a container object that may be in the form of a list, tuple or string • Containers in Python means sets, sequences such as lists, tuples, and strings, and mappings such as dictionaries • e. g for i in range(1, 11): print (i) Output: Numbers from 1 to 10
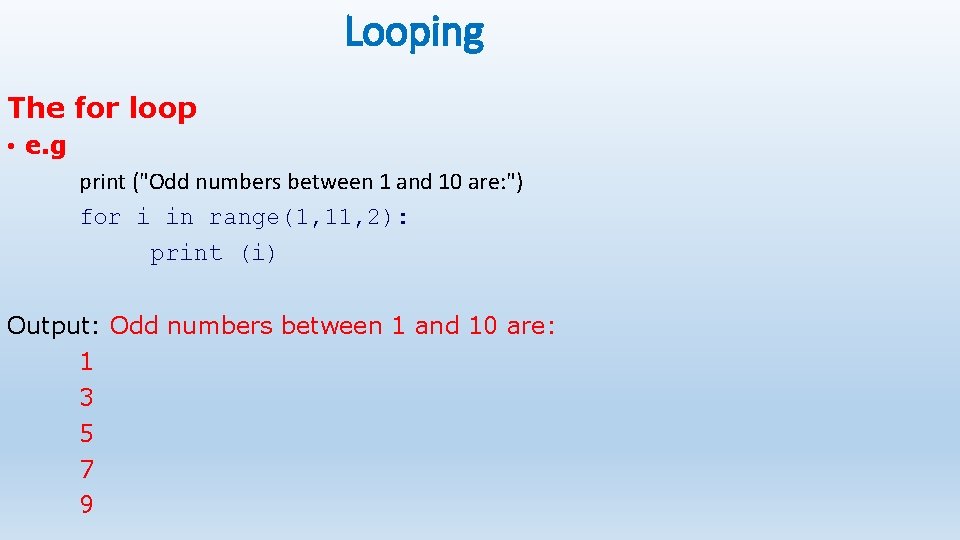
Looping The for loop • e. g print ("Odd numbers between 1 and 10 are: ") for i in range(1, 11, 2): print (i) Output: Odd numbers between 1 and 10 are: 1 3 5 7 9
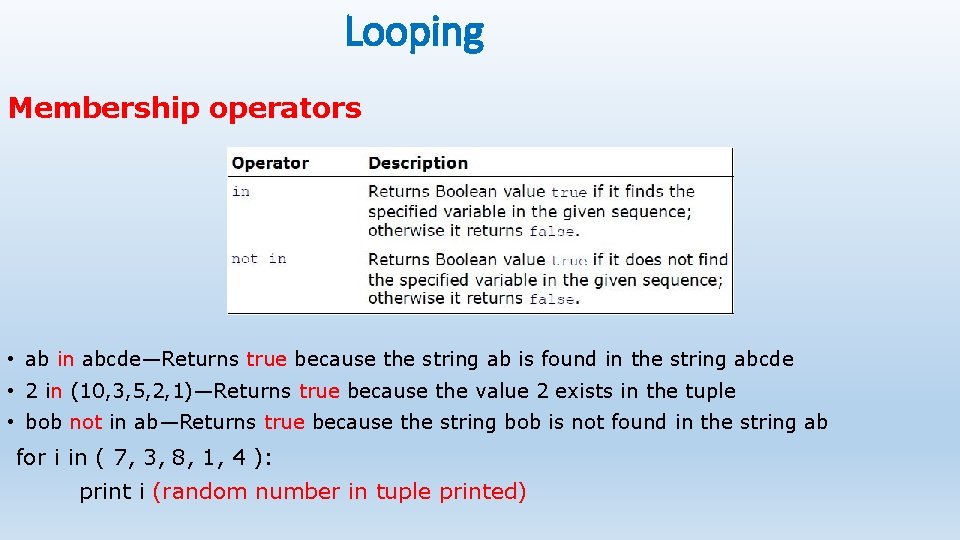
Looping Membership operators • ab in abcde—Returns true because the string ab is found in the string abcde • 2 in (10, 3, 5, 2, 1)—Returns true because the value 2 exists in the tuple • bob not in ab—Returns true because the string bob is not found in the string ab for i in ( 7, 3, 8, 1, 4 ): print i (random number in tuple printed)
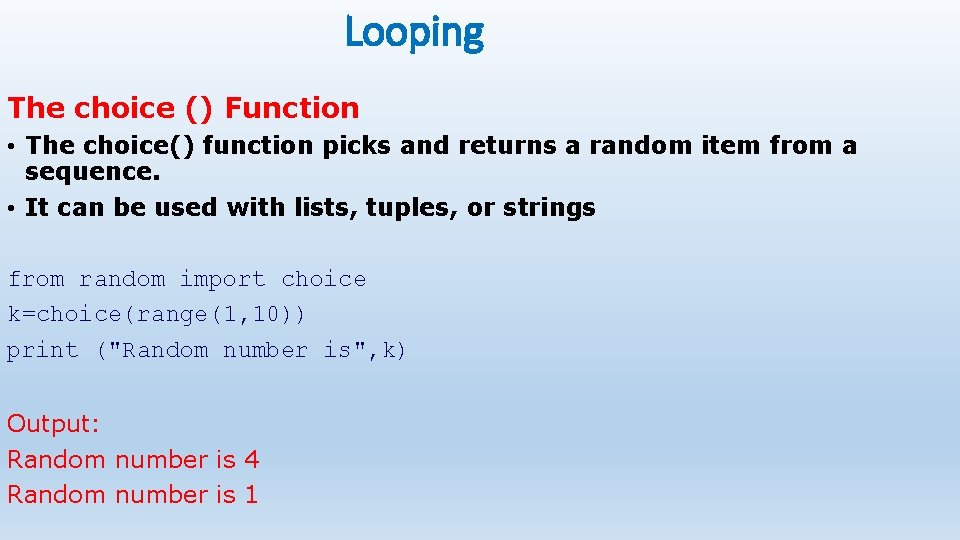
Looping The choice () Function • The choice() function picks and returns a random item from a sequence. • It can be used with lists, tuples, or strings from random import choice k=choice(range(1, 10)) print ("Random number is", k) Output: Random number is 4 Random number is 1
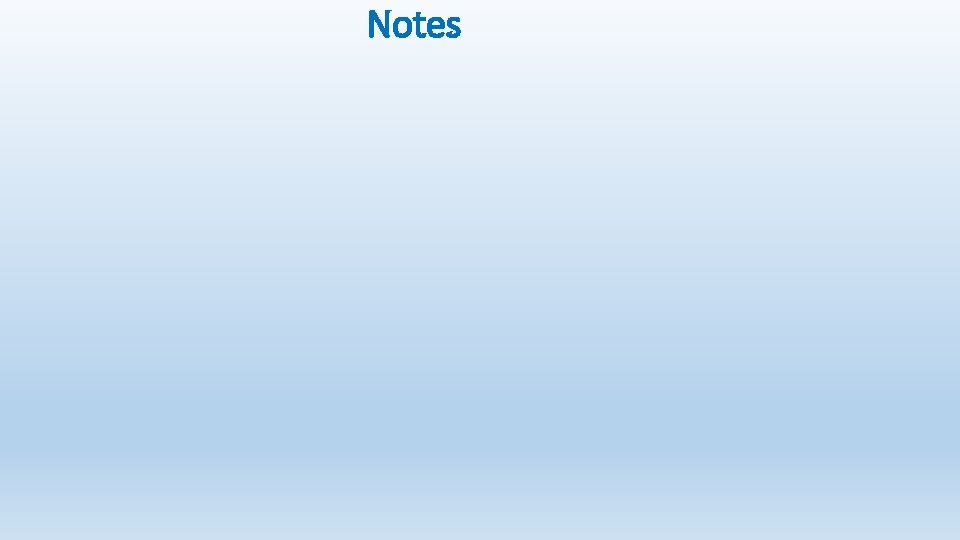
Notes
Meaning of poster making
Screening decisions and preference decisions
How would you use arithmetic sequence in making decisions
Layout decisions operations management
Operations and productivity
One face one voice one habit and two persons
Register transfer language
Arithmetic operationsarithmetic operations
Enhancement using arithmetic/logic operations
What does a prime polynomial look like
Floating point arithmetic examples
Counter-controlled
Looping and dangling in network diagram
What is counter and looping
Small basic turtle commands
One god one empire one religion
One one one little dogs run
One king one law one faith
Byzantine definition
Ford one plan
See one do one teach one
One price policy
See one do one teach one
Asean tourism strategic plan
Asean one vision one identity one community
Chapter 4 financial decisions and planning
Chapter 4 financial decisions and planning
Loan vocabulary
Chapter 1 economic decisions and systems answer key
Chapter 4 financial decisions and planning
Chapter 4 financial decisions and planning
Chapter 4 financial decisions and planning
Contoh loop
Program pengulangan pascal
What is looping in writing