Java Script Introduction Like CC and Java Java
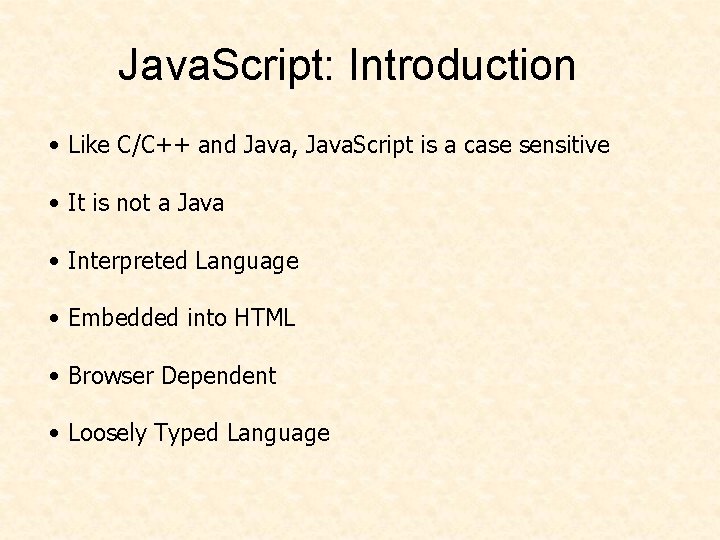
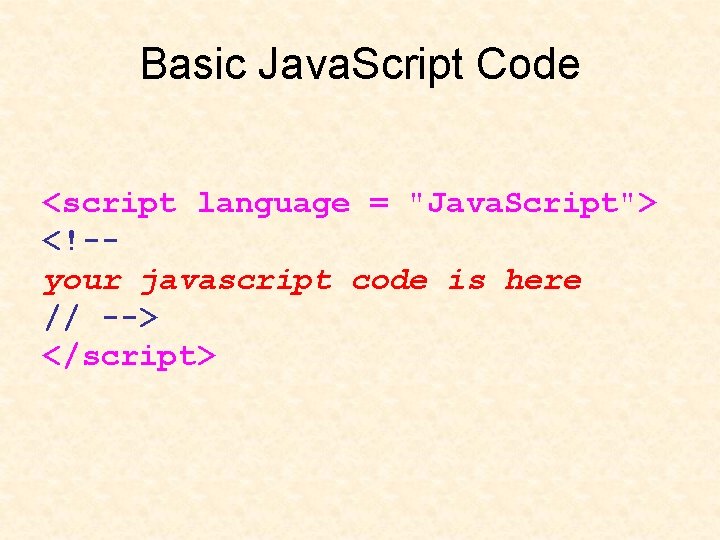
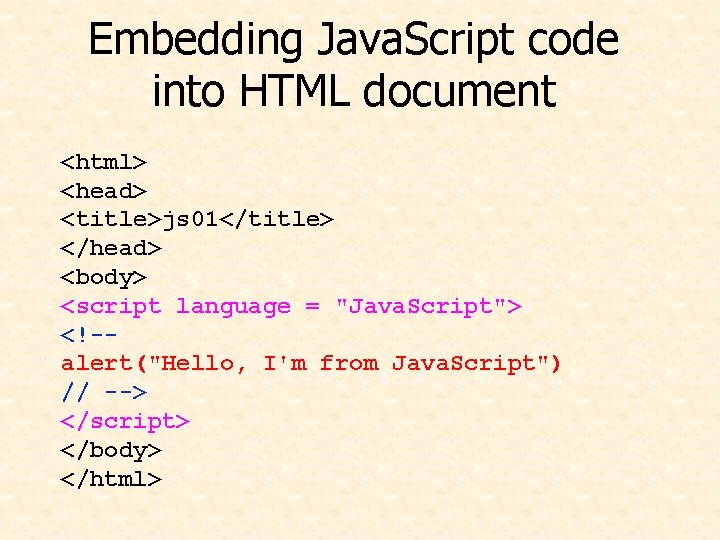
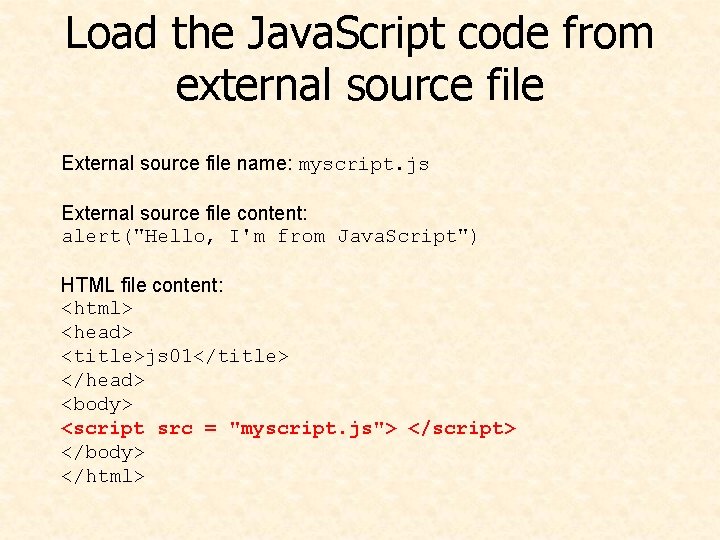
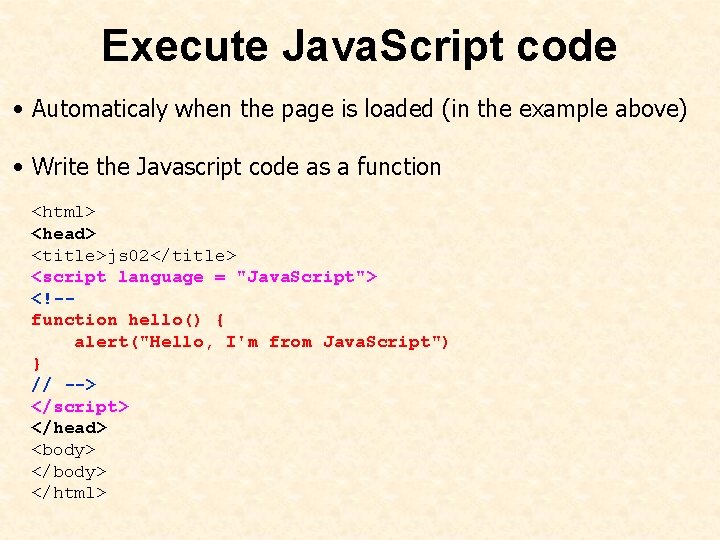
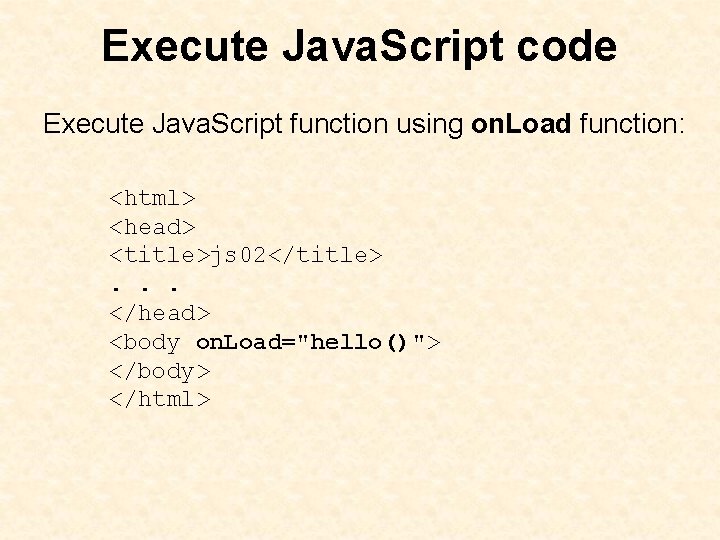
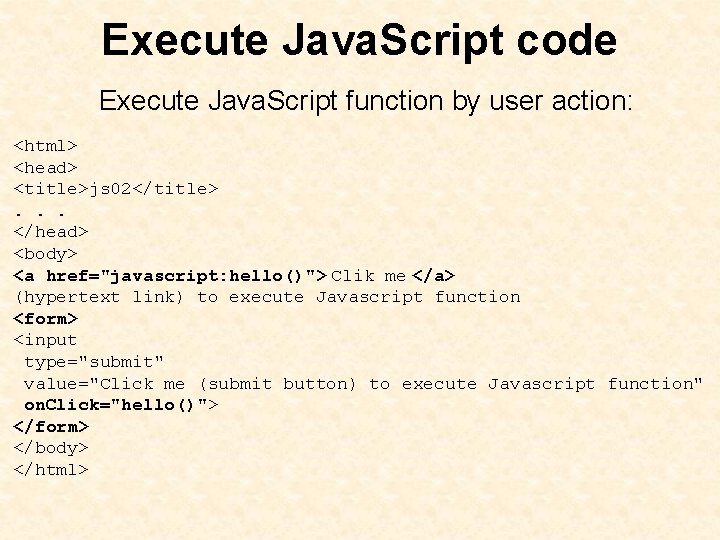
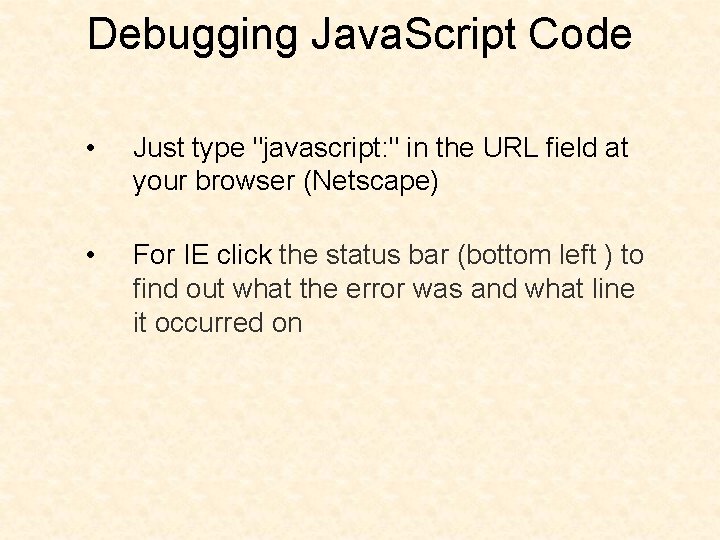
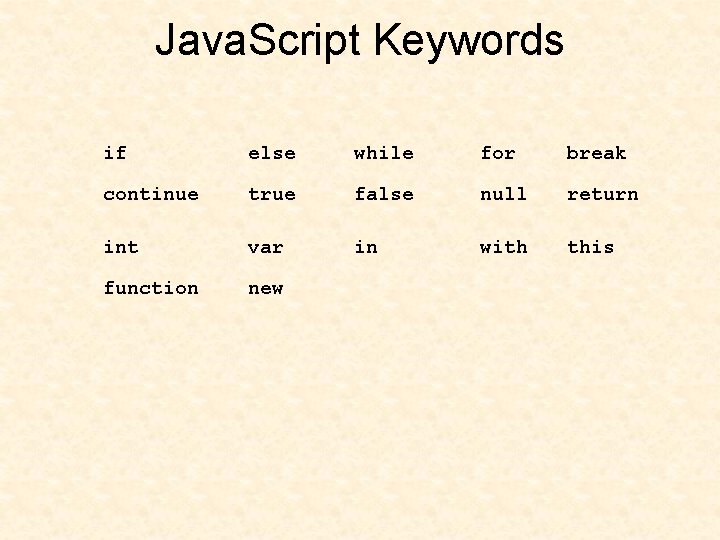
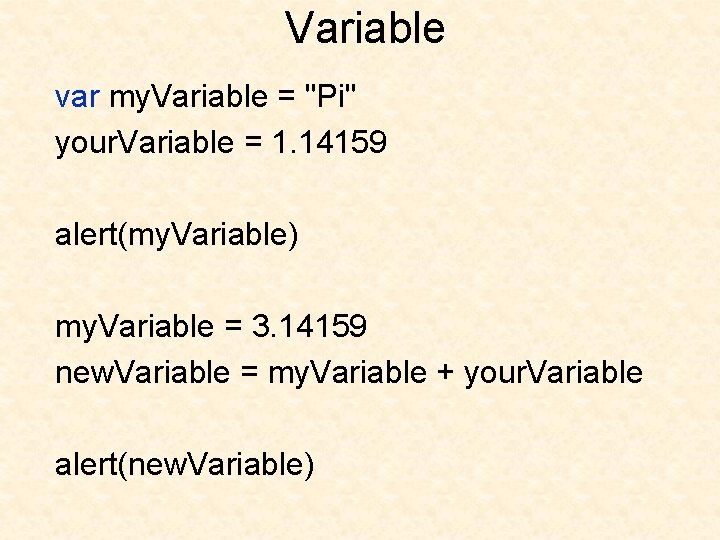
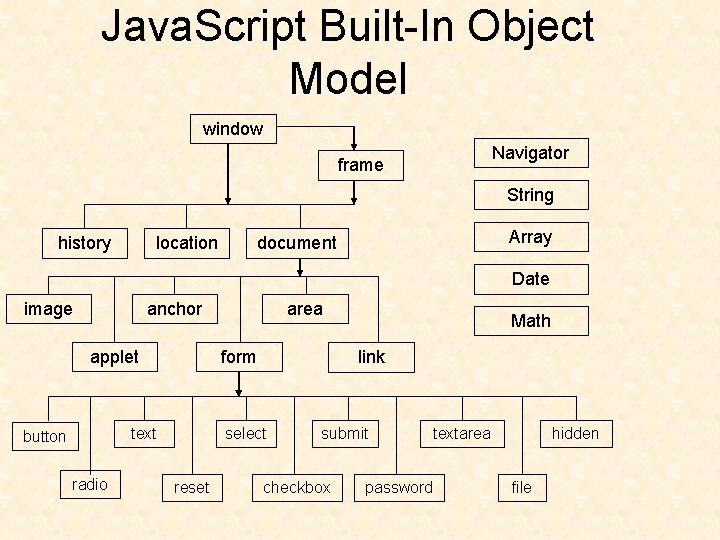
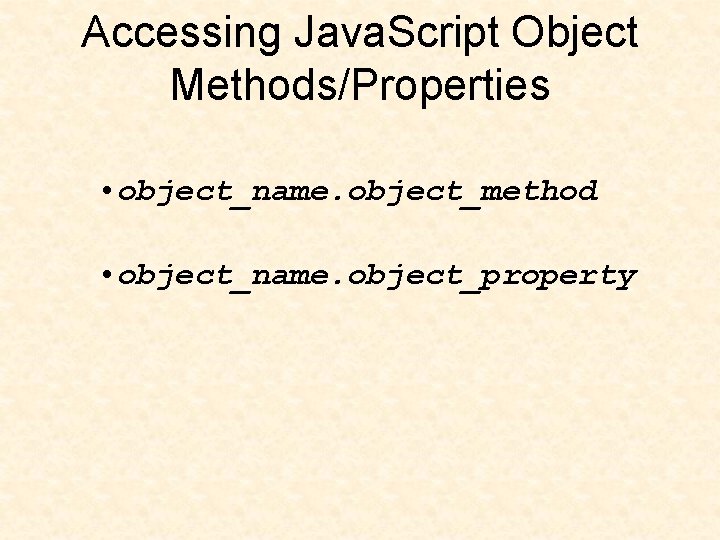
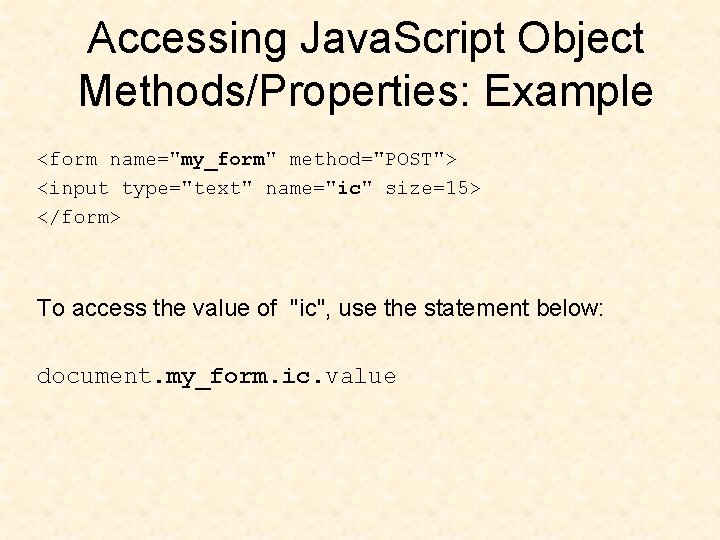
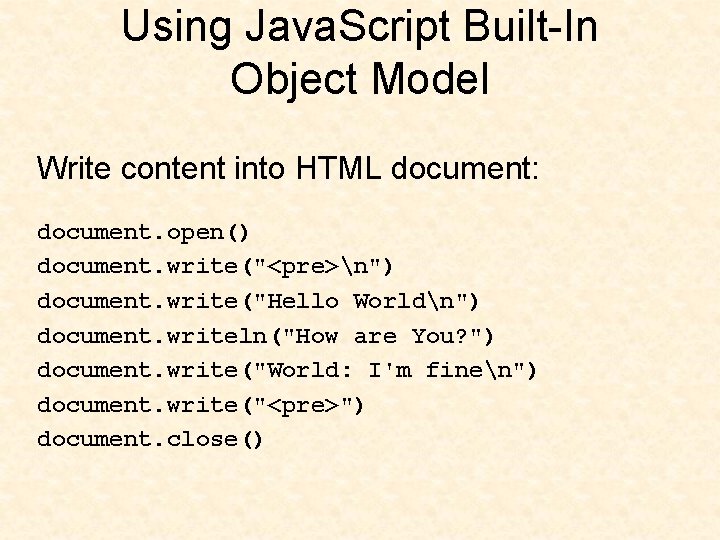
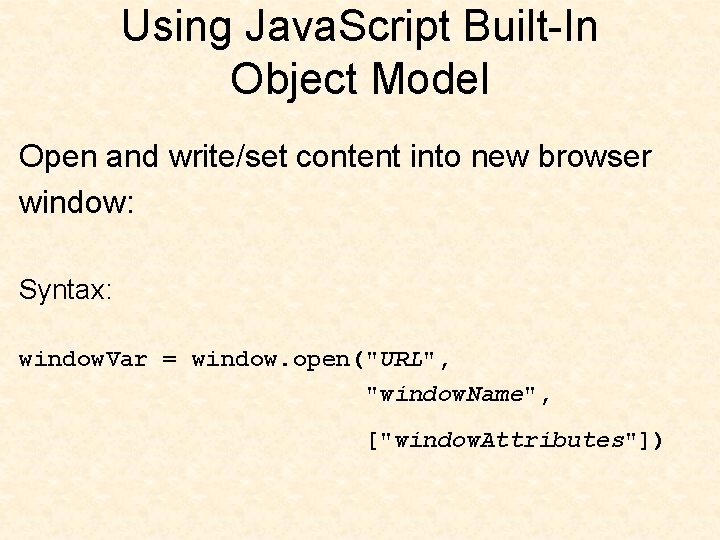
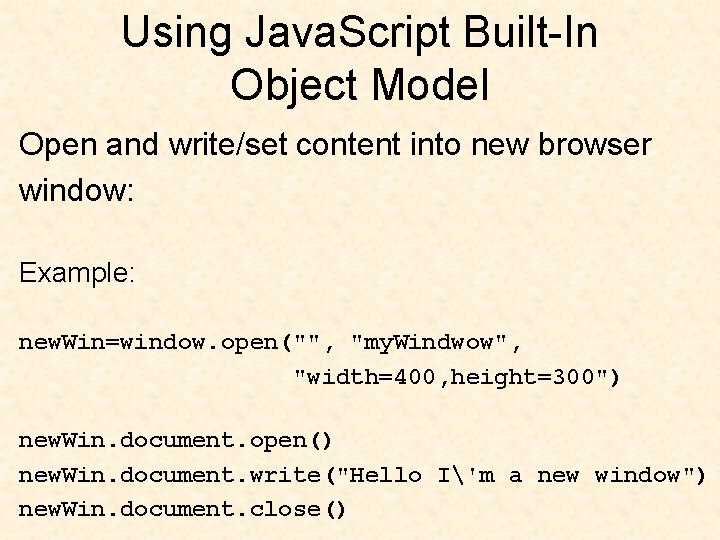
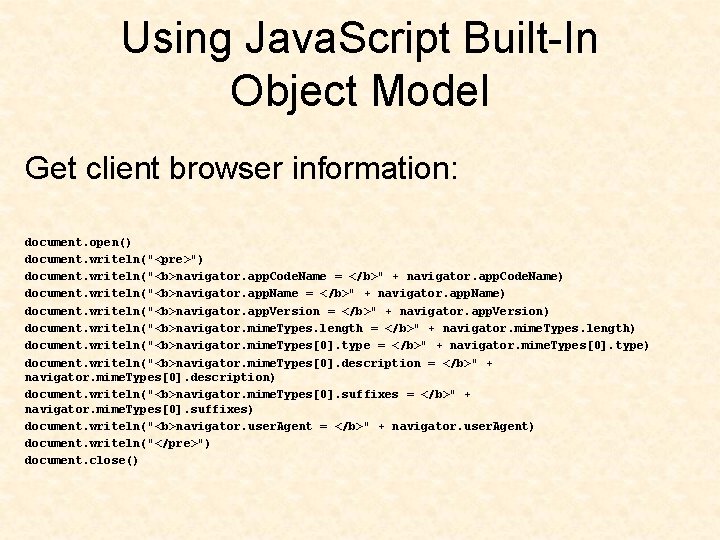
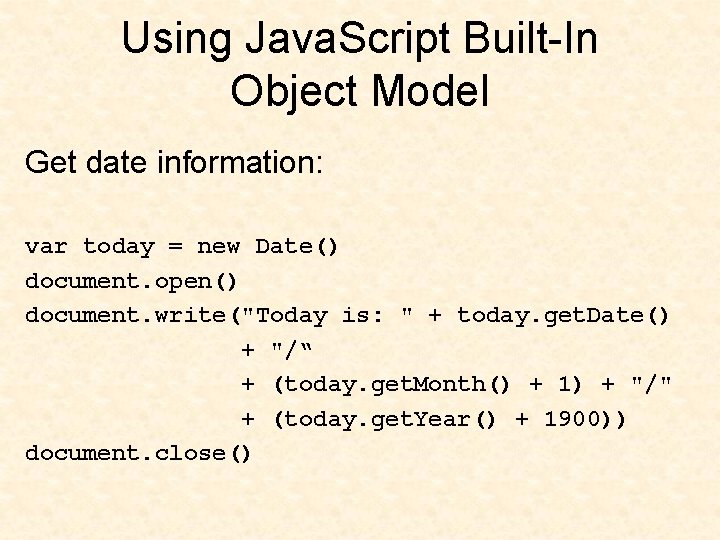
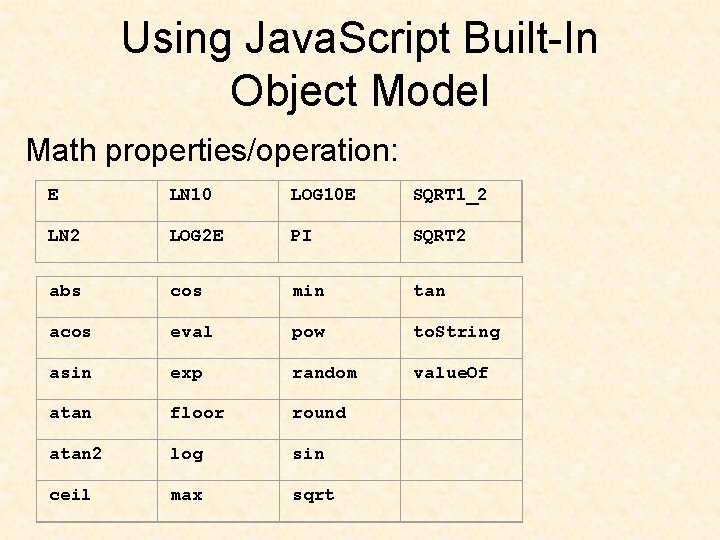
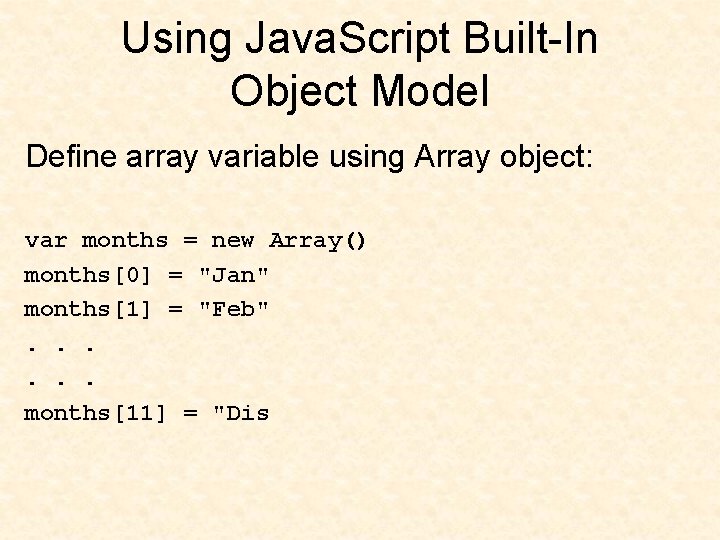
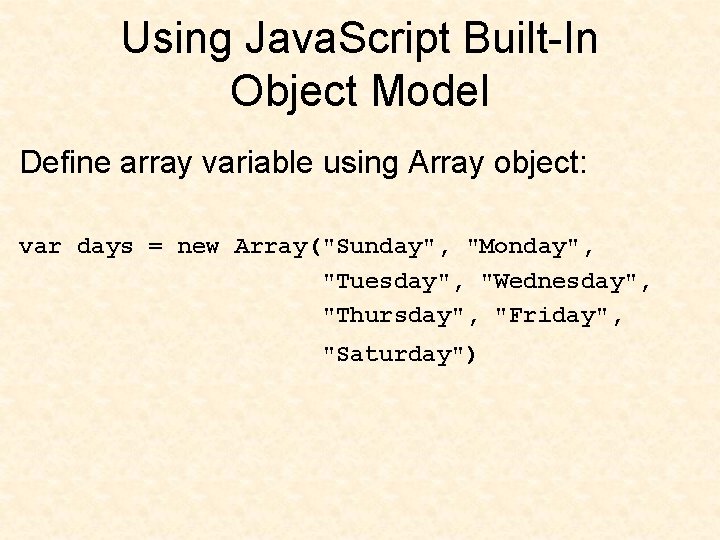
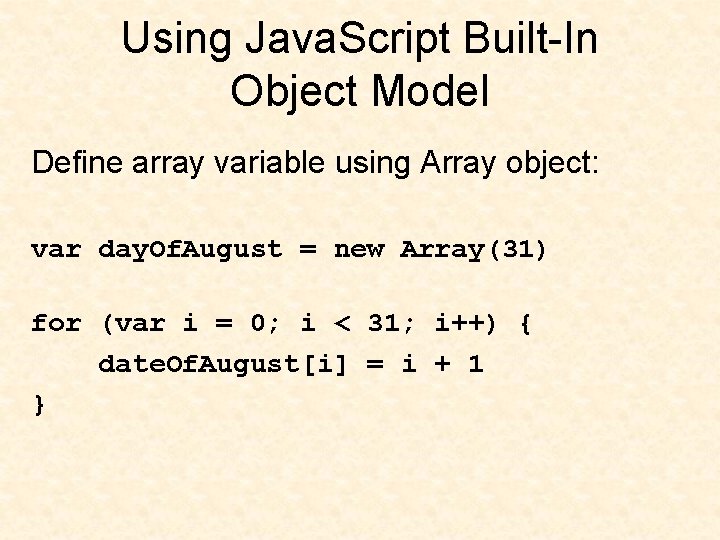
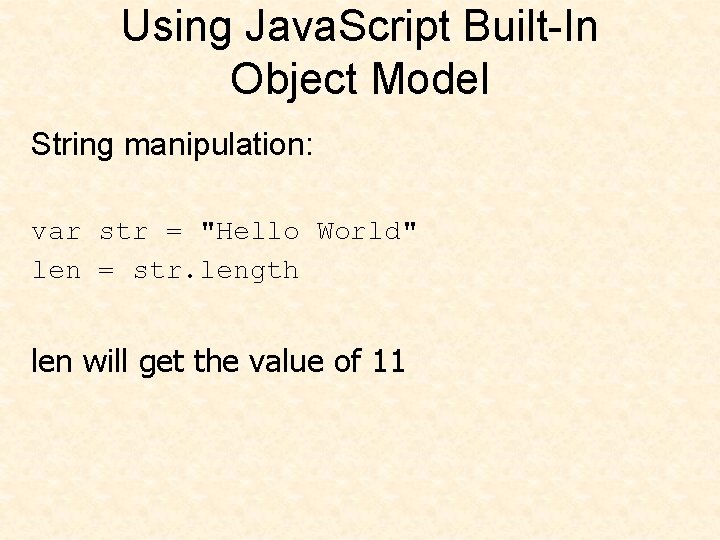
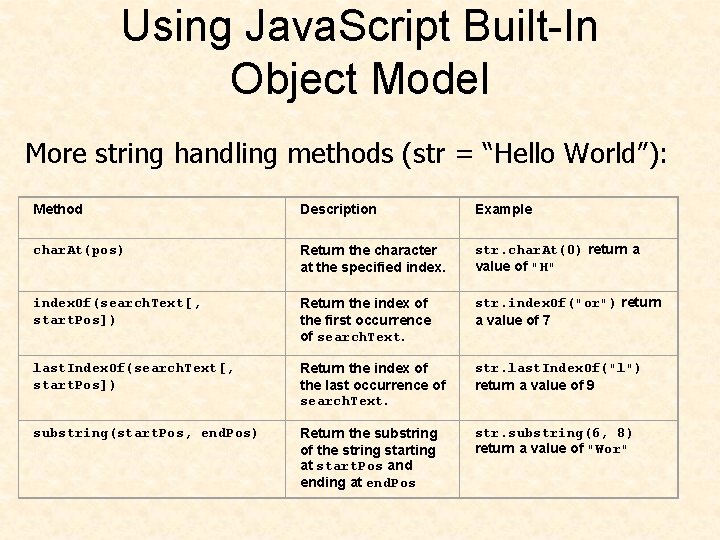
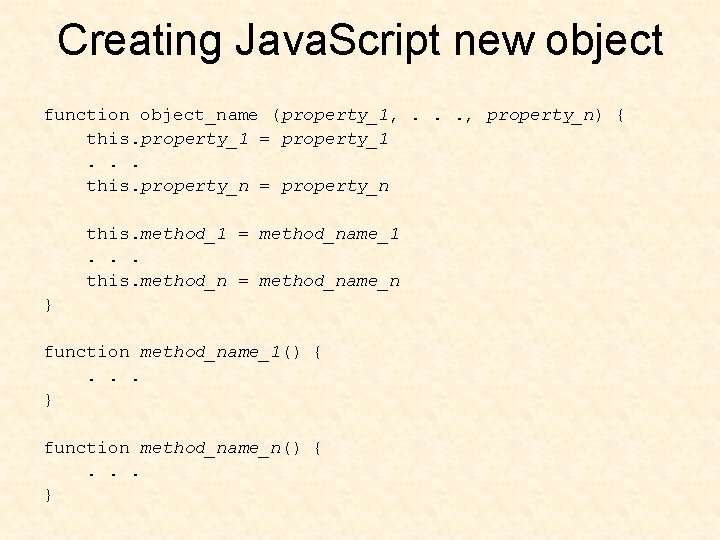
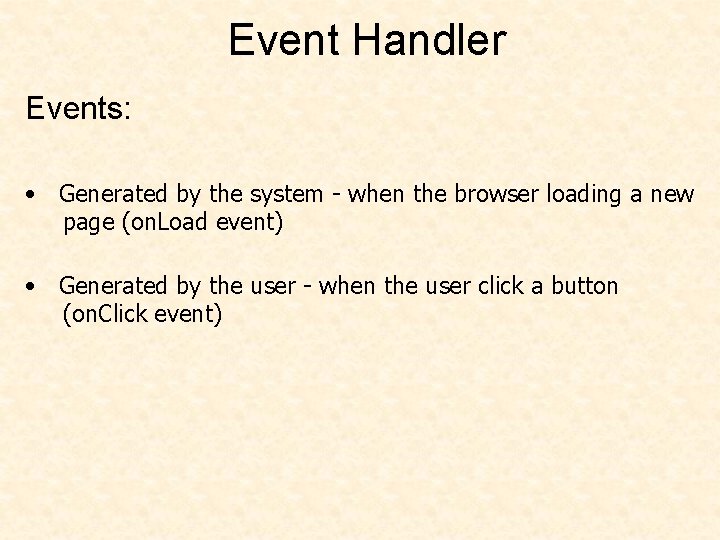
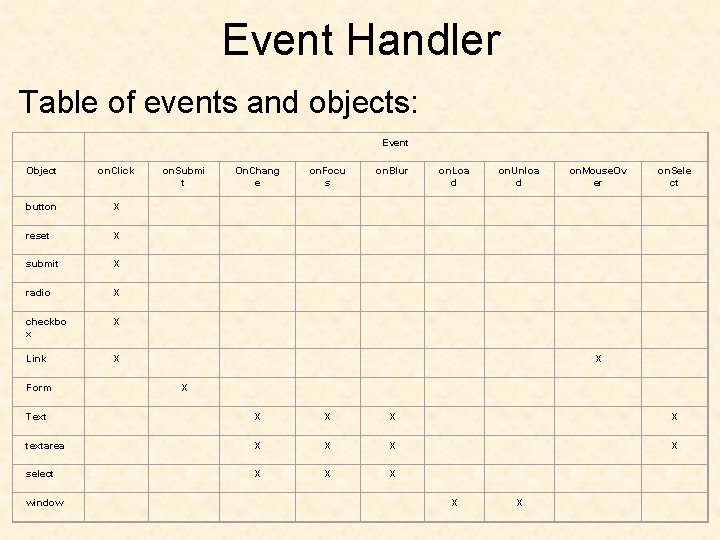
- Slides: 27
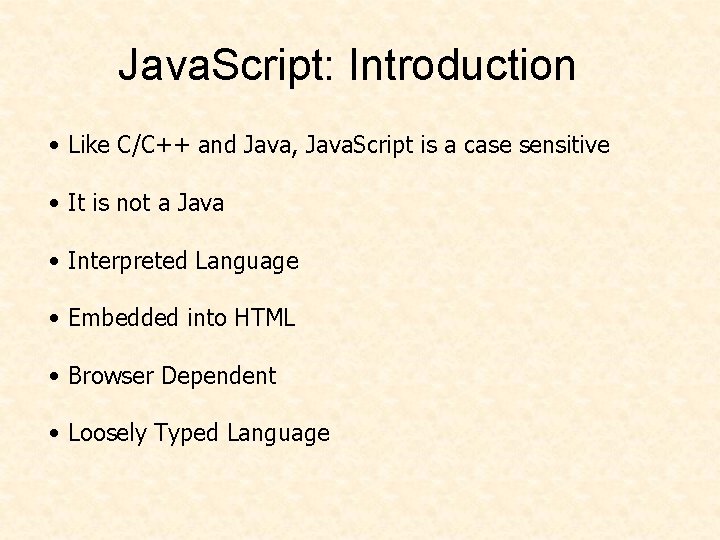
Java. Script: Introduction • Like C/C++ and Java, Java. Script is a case sensitive • It is not a Java • Interpreted Language • Embedded into HTML • Browser Dependent • Loosely Typed Language
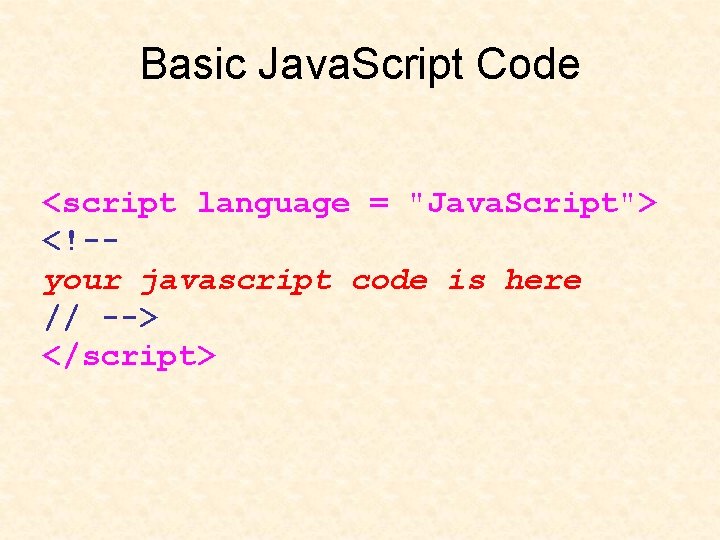
Basic Java. Script Code <script language = "Java. Script"> <!-your javascript code is here // --> </script>
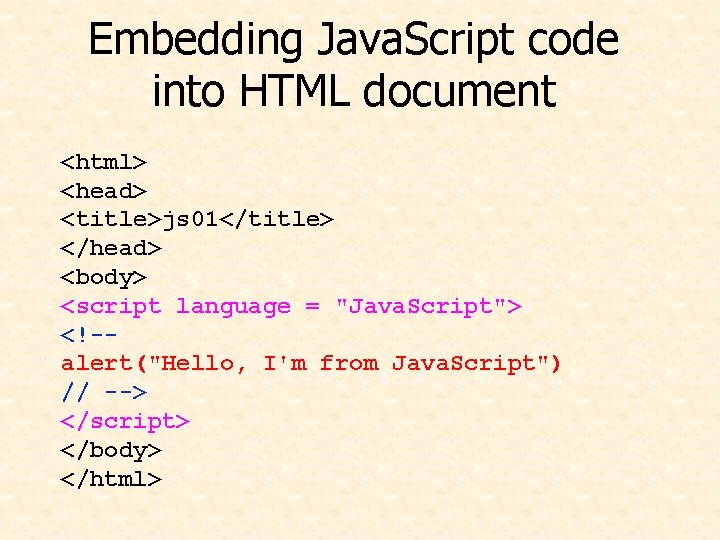
Embedding Java. Script code into HTML document <html> <head> <title>js 01</title> </head> <body> <script language = "Java. Script"> <!-alert("Hello, I'm from Java. Script") // --> </script> </body> </html>
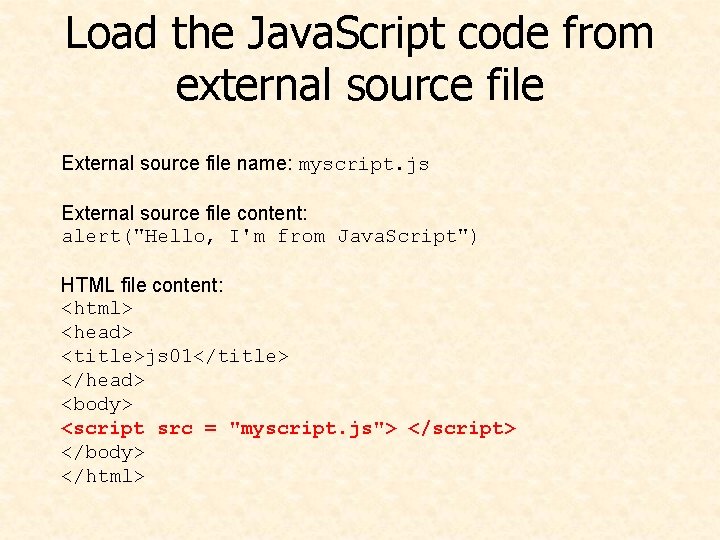
Load the Java. Script code from external source file External source file name: myscript. js External source file content: alert("Hello, I'm from Java. Script") HTML file content: <html> <head> <title>js 01</title> </head> <body> <script src = "myscript. js"> </script> </body> </html>
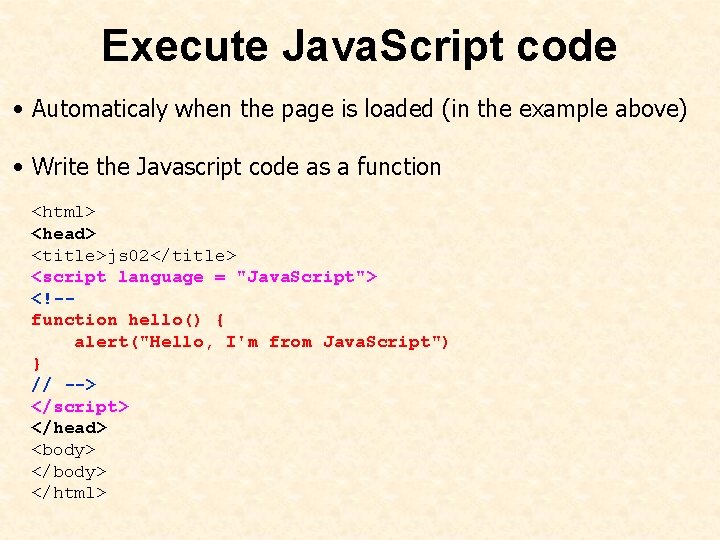
Execute Java. Script code • Automaticaly when the page is loaded (in the example above) • Write the Javascript code as a function <html> <head> <title>js 02</title> <script language = "Java. Script"> <!-function hello() { alert("Hello, I'm from Java. Script") } // --> </script> </head> <body> </html>
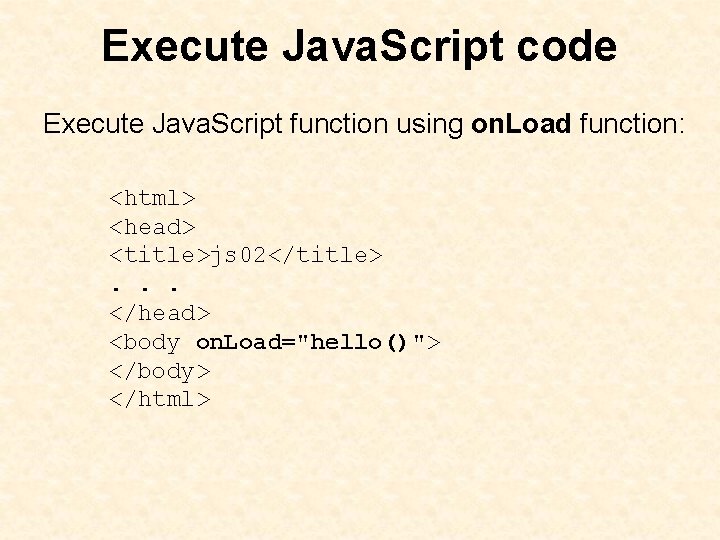
Execute Java. Script code Execute Java. Script function using on. Load function: <html> <head> <title>js 02</title>. . . </head> <body on. Load="hello()"> </body> </html>
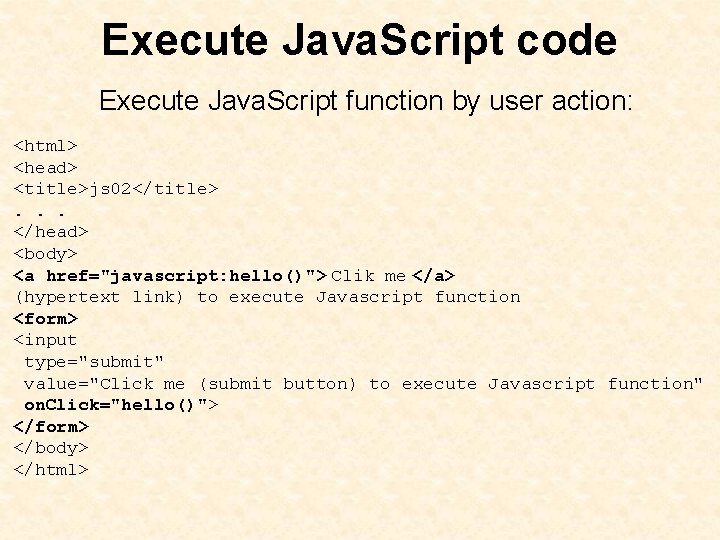
Execute Java. Script code Execute Java. Script function by user action: <html> <head> <title>js 02</title>. . . </head> <body> <a href="javascript: hello()"> Clik me </a> (hypertext link) to execute Javascript function <form> <input type="submit" value="Click me (submit button) to execute Javascript function" on. Click="hello()"> </form> </body> </html>
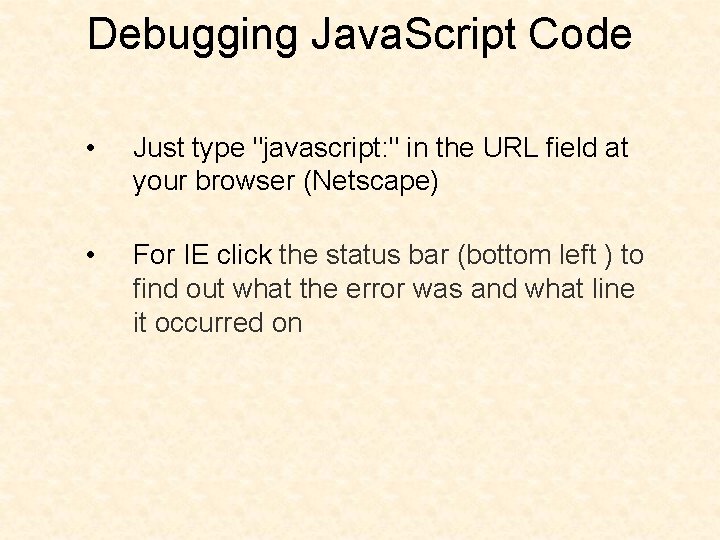
Debugging Java. Script Code • Just type "javascript: " in the URL field at your browser (Netscape) • For IE click the status bar (bottom left ) to find out what the error was and what line it occurred on
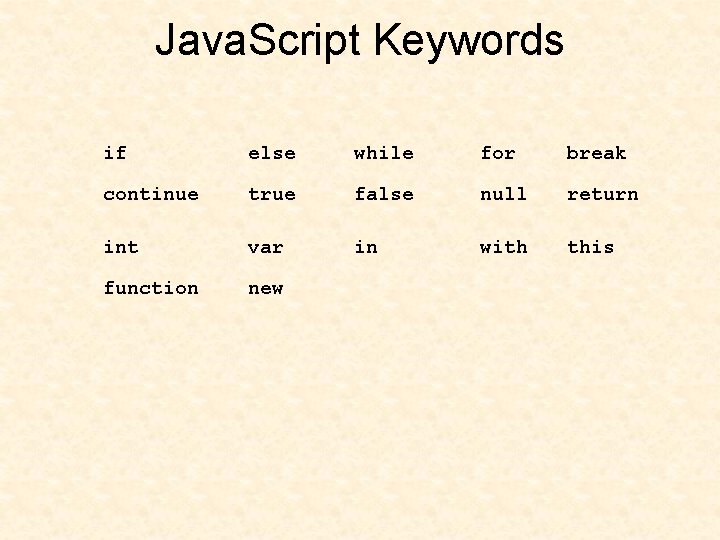
Java. Script Keywords if else while for break continue true false null return int var in with this function new
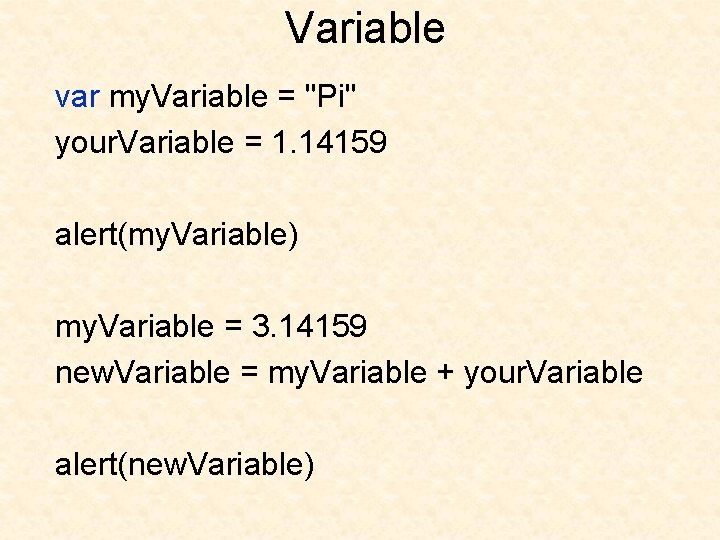
Variable var my. Variable = "Pi" your. Variable = 1. 14159 alert(my. Variable) my. Variable = 3. 14159 new. Variable = my. Variable + your. Variable alert(new. Variable)
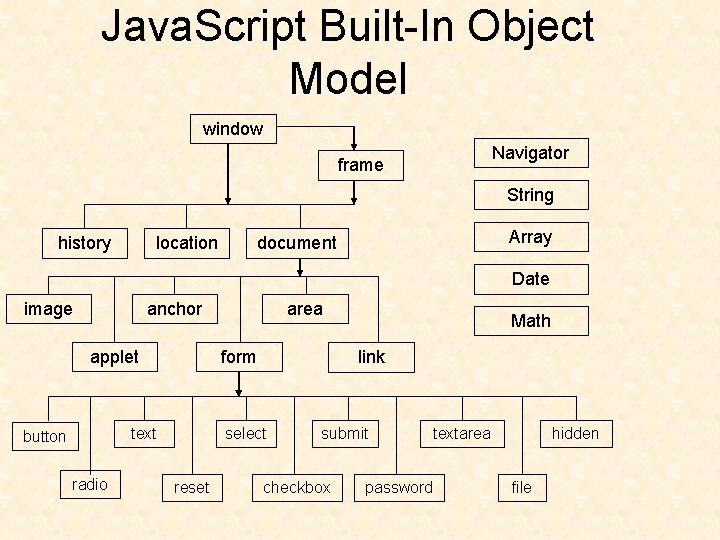
Java. Script Built-In Object Model window Navigator frame String history location Array document Date image anchor applet form text button radio area link select reset Math submit checkbox textarea password hidden file
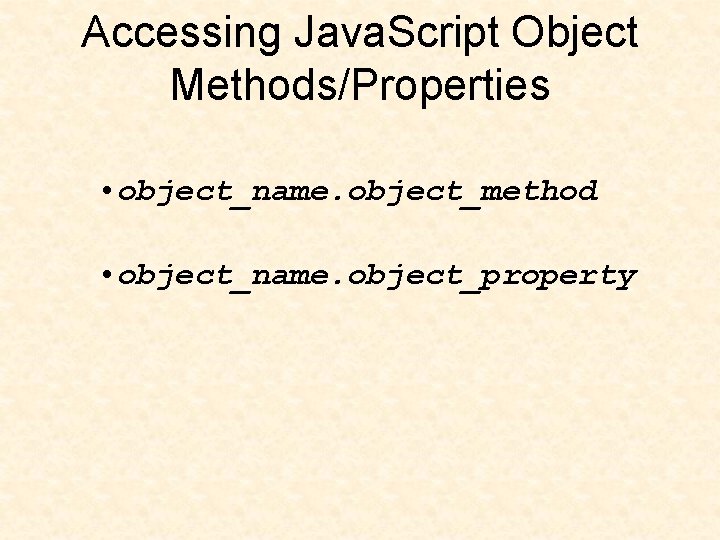
Accessing Java. Script Object Methods/Properties • object_name. object_method • object_name. object_property
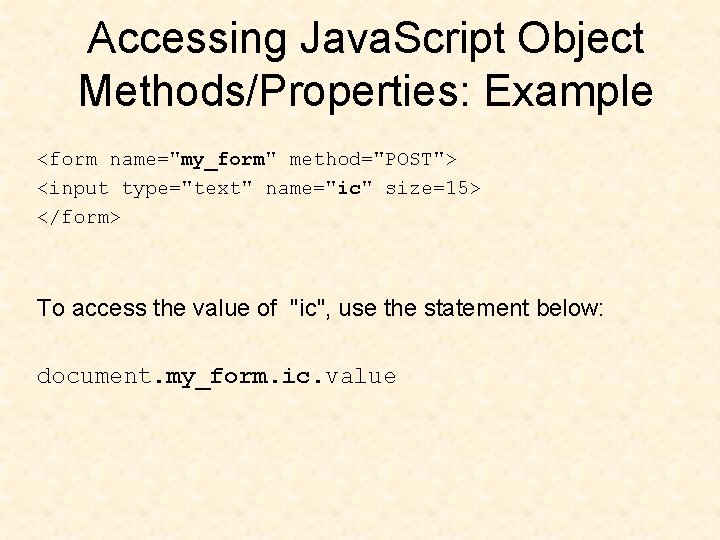
Accessing Java. Script Object Methods/Properties: Example <form name="my_form" method="POST"> <input type="text" name="ic" size=15> </form> To access the value of "ic", use the statement below: document. my_form. ic. value
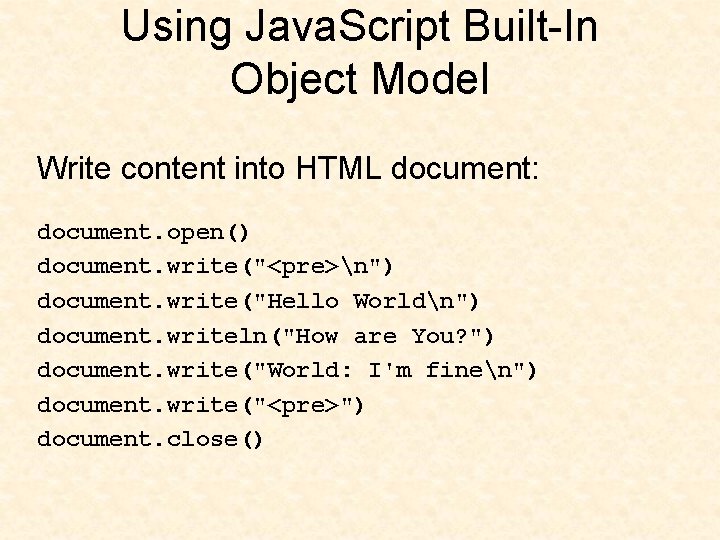
Using Java. Script Built-In Object Model Write content into HTML document: document. open() document. write("<pre>n") document. write("Hello Worldn") document. writeln("How are You? ") document. write("World: I'm finen") document. write("<pre>") document. close()
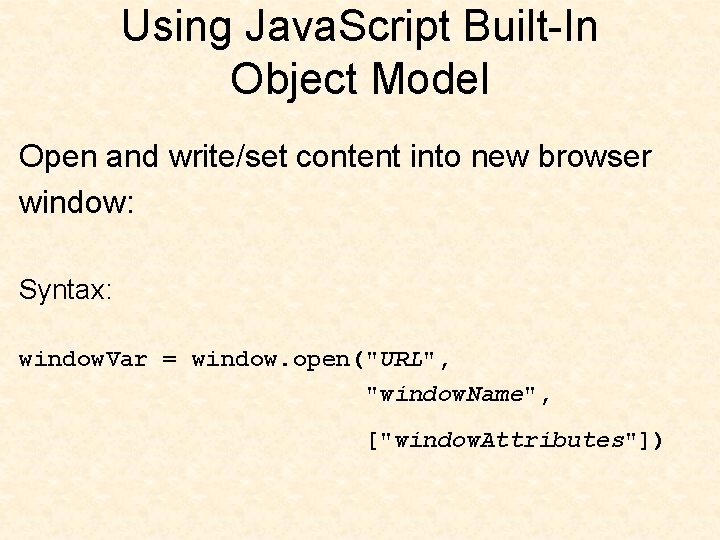
Using Java. Script Built-In Object Model Open and write/set content into new browser window: Syntax: window. Var = window. open("URL", "window. Name", ["window. Attributes"])
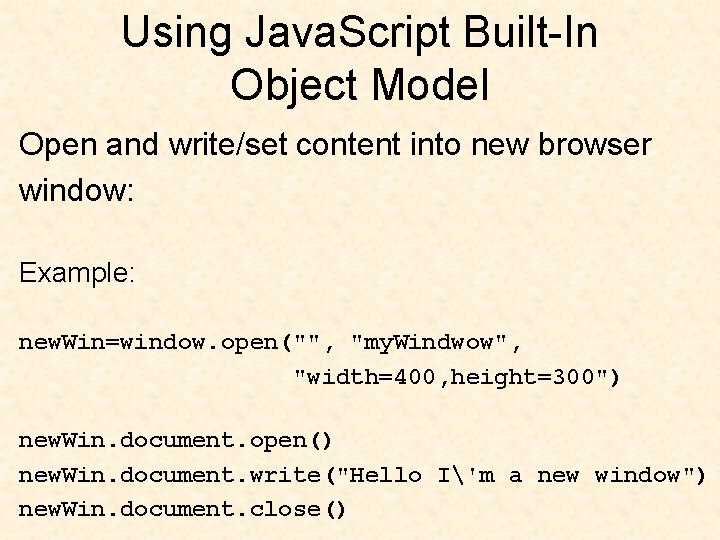
Using Java. Script Built-In Object Model Open and write/set content into new browser window: Example: new. Win=window. open("", "my. Windwow", "width=400, height=300") new. Win. document. open() new. Win. document. write("Hello I'm a new window") new. Win. document. close()
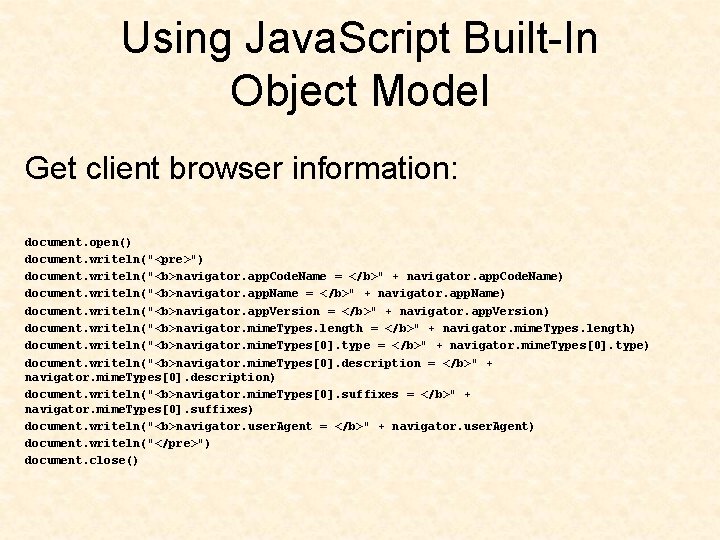
Using Java. Script Built-In Object Model Get client browser information: document. open() document. writeln("<pre>") document. writeln("<b>navigator. app. Code. Name = </b>" + navigator. app. Code. Name) document. writeln("<b>navigator. app. Name = </b>" + navigator. app. Name) document. writeln("<b>navigator. app. Version = </b>" + navigator. app. Version) document. writeln("<b>navigator. mime. Types. length = </b>" + navigator. mime. Types. length) document. writeln("<b>navigator. mime. Types[0]. type = </b>" + navigator. mime. Types[0]. type) document. writeln("<b>navigator. mime. Types[0]. description = </b>" + navigator. mime. Types[0]. description) document. writeln("<b>navigator. mime. Types[0]. suffixes = </b>" + navigator. mime. Types[0]. suffixes) document. writeln("<b>navigator. user. Agent = </b>" + navigator. user. Agent) document. writeln("</pre>") document. close()
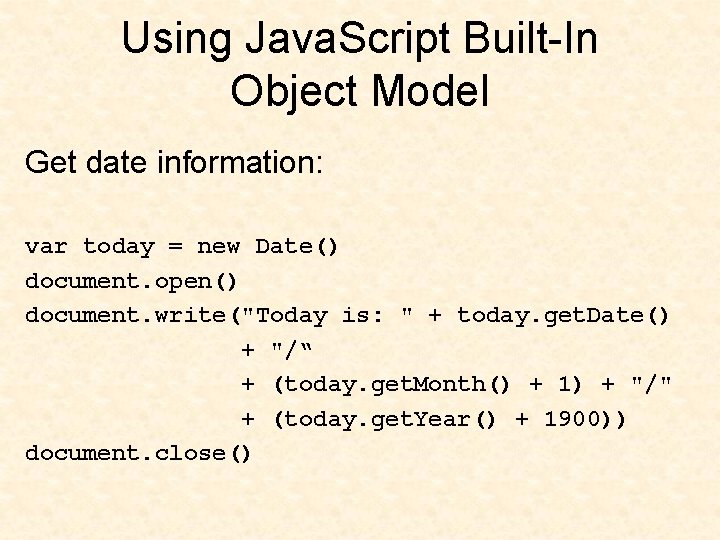
Using Java. Script Built-In Object Model Get date information: var today = new Date() document. open() document. write("Today is: " + today. get. Date() + "/“ + (today. get. Month() + 1) + "/" + (today. get. Year() + 1900)) document. close()
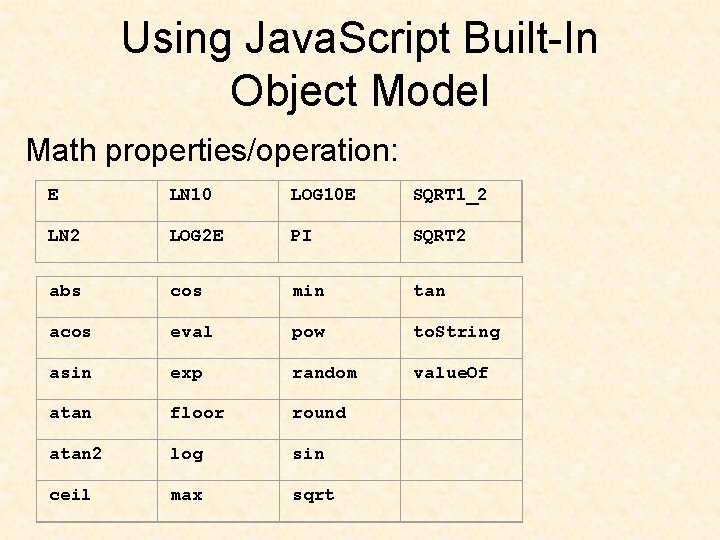
Using Java. Script Built-In Object Model Math properties/operation: E LN 10 LOG 10 E SQRT 1_2 LN 2 LOG 2 E PI SQRT 2 abs cos min tan acos eval pow to. String asin exp random value. Of atan floor round atan 2 log sin ceil max sqrt
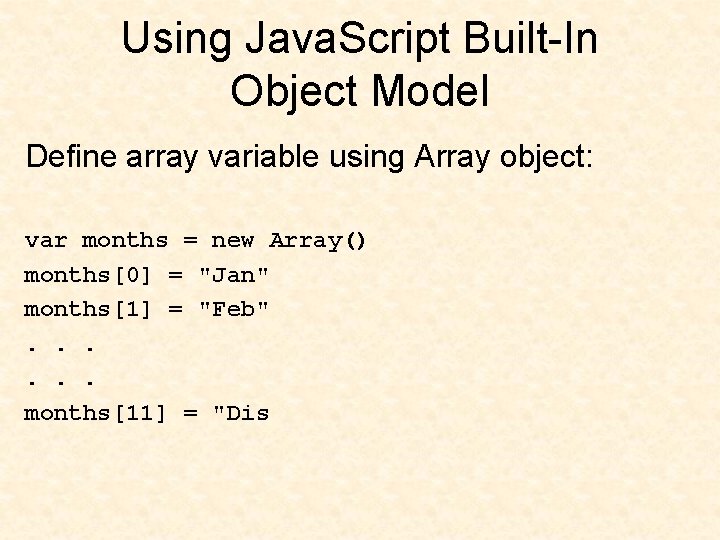
Using Java. Script Built-In Object Model Define array variable using Array object: var months = new Array() months[0] = "Jan" months[1] = "Feb". . . months[11] = "Dis
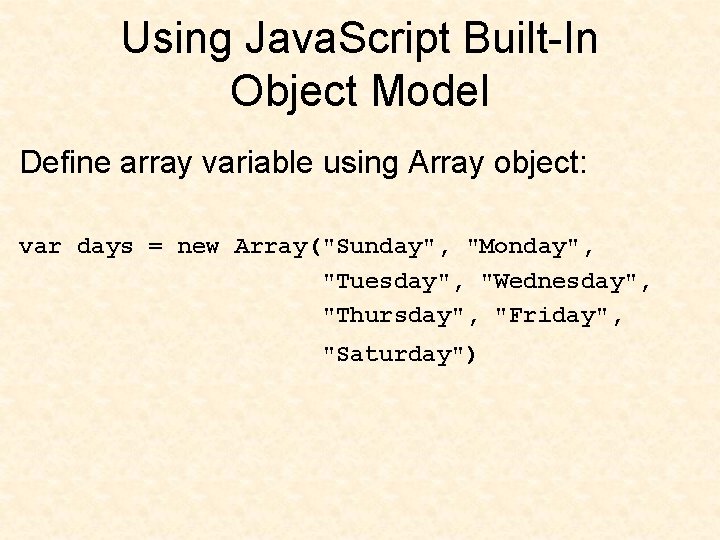
Using Java. Script Built-In Object Model Define array variable using Array object: var days = new Array("Sunday", "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday")
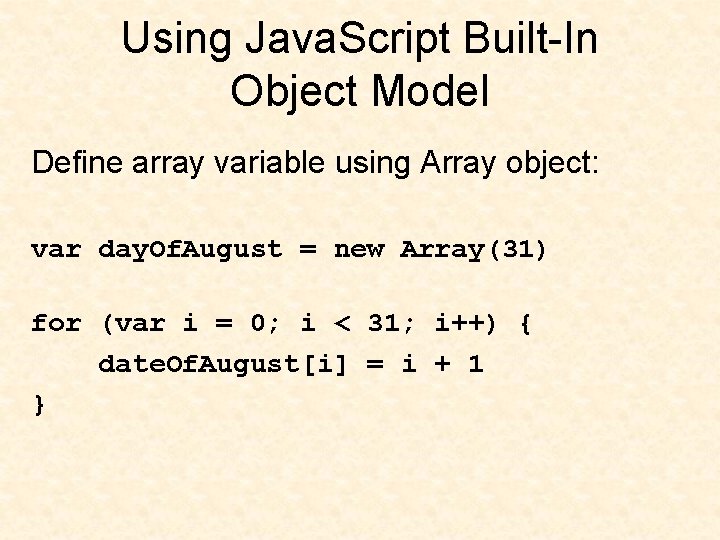
Using Java. Script Built-In Object Model Define array variable using Array object: var day. Of. August = new Array(31) for (var i = 0; i < 31; i++) { date. Of. August[i] = i + 1 }
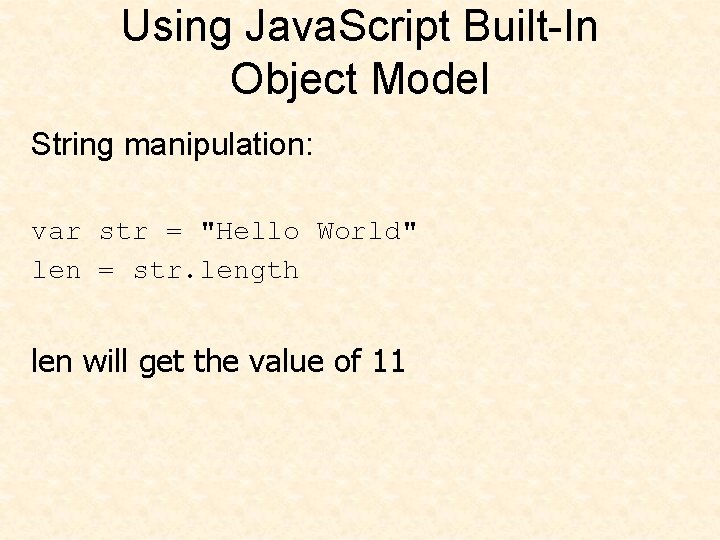
Using Java. Script Built-In Object Model String manipulation: var str = "Hello World" len = str. length len will get the value of 11
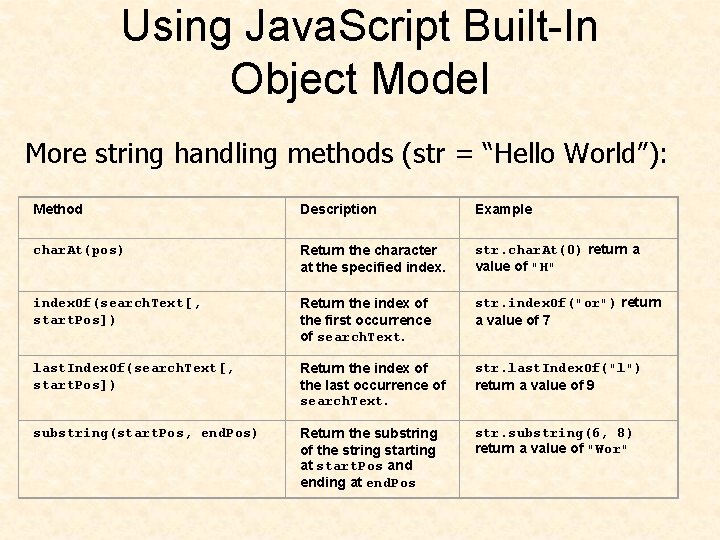
Using Java. Script Built-In Object Model More string handling methods (str = “Hello World”): Method Description Example char. At(pos) Return the character at the specified index. str. char. At(0) return a value of "H" index. Of(search. Text[, start. Pos]) Return the index of the first occurrence of search. Text. str. index. Of("or") return a value of 7 last. Index. Of(search. Text[, start. Pos]) Return the index of the last occurrence of search. Text. str. last. Index. Of("l") return a value of 9 substring(start. Pos, end. Pos) Return the substring of the string starting at start. Pos and ending at end. Pos str. substring(6, 8) return a value of "Wor"
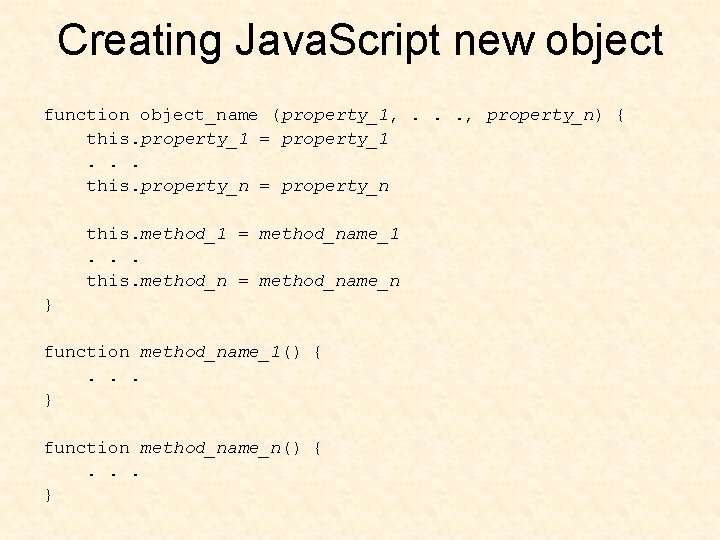
Creating Java. Script new object function object_name (property_1, . . . , property_n) { this. property_1 = property_1. . . this. property_n = property_n this. method_1 = method_name_1. . . this. method_n = method_name_n } function method_name_1() {. . . } function method_name_n() {. . . }
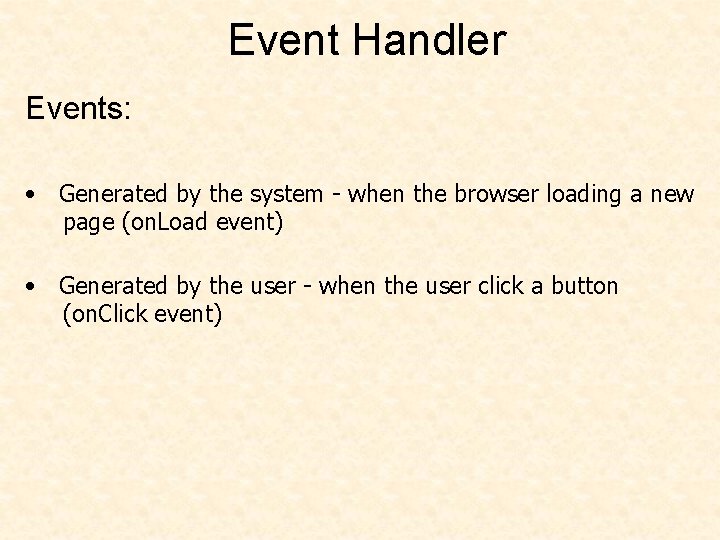
Event Handler Events: • Generated by the system - when the browser loading a new page (on. Load event) • Generated by the user - when the user click a button (on. Click event)
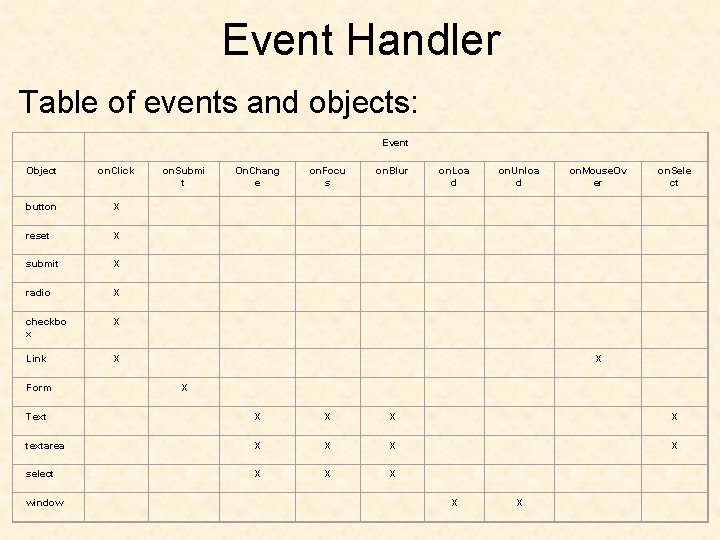
Event Handler Table of events and objects: Event Object on. Click button X reset X submit X radio X checkbo x X Link X Form on. Submi t On. Chang e on. Focu s on. Blur on. Loa d on. Unloa d on. Mouse. Ov er on. Sele ct X X Text X X textarea X X select X X X window X X
Event introduction script
Event introduction script
Thesis manuscript format
Moderator script for webinar sample
Script de java
Script
Java script wikipedia
Language
"java script"
"java script"
Java script course
Java script
"java script"
Hopper khan academy
Java script examples
.inside which html element do we put the javascript?
Java script email
Js ide
"java script"
Riad wahby
Java script classes
Would rather grammar rules
Remo ualberta
Which features of the sun look like huge cloudlike arches
Why does ethanol look like water but behave more like wood?
Like minds like mine
Who does he look like
What does he look like