Introduction to Flash Action Script 3 0 Events
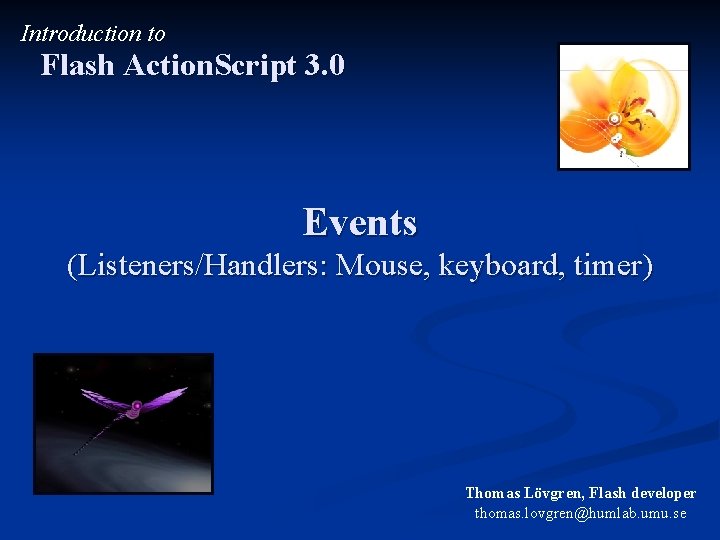
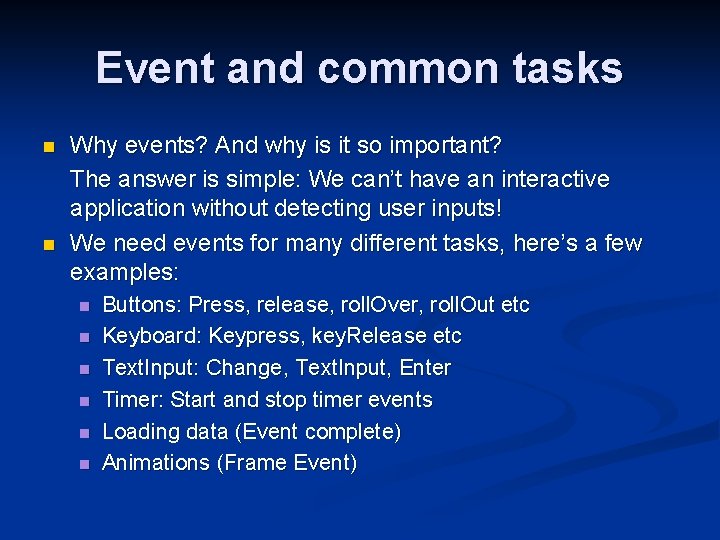
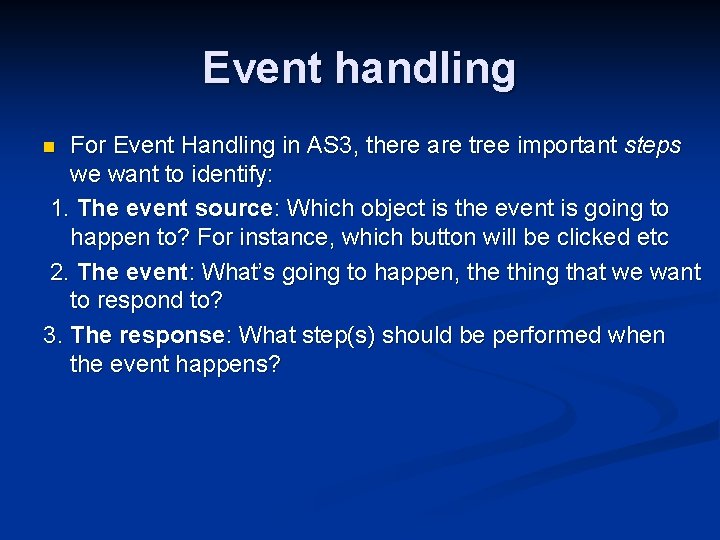
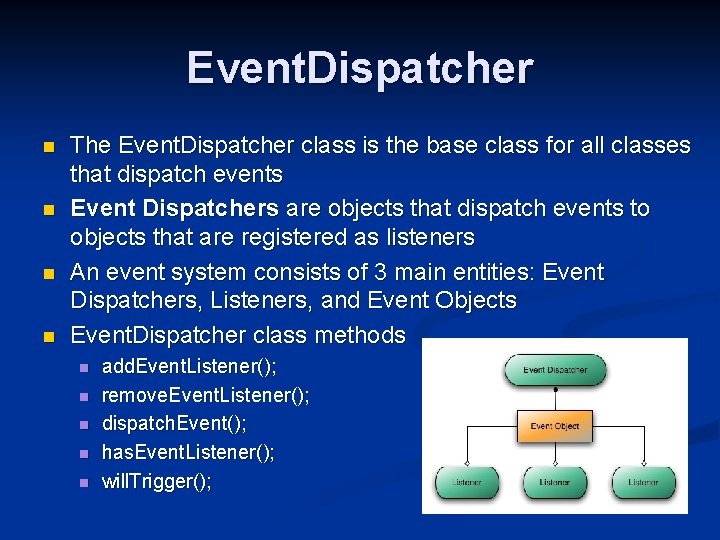
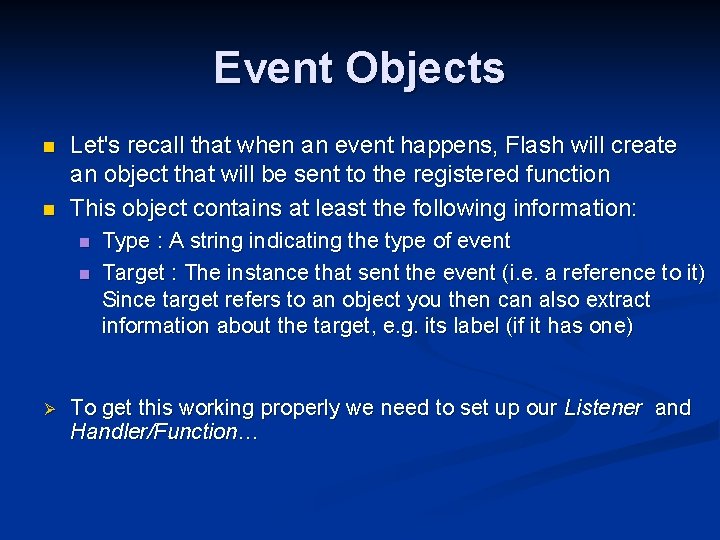
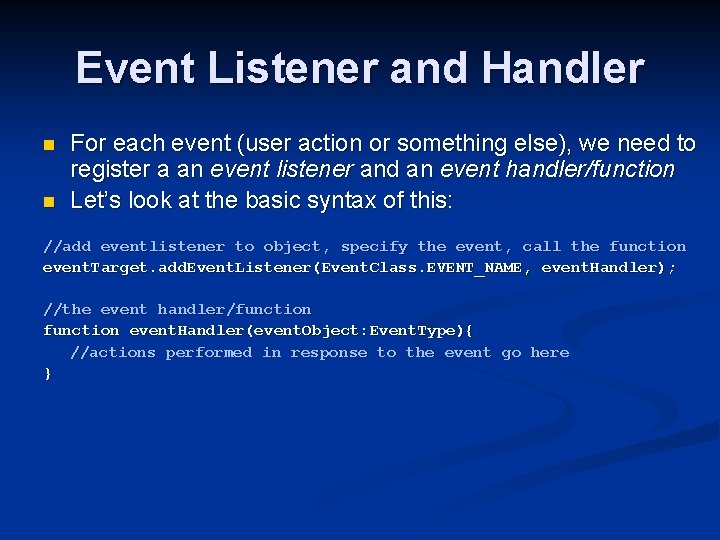
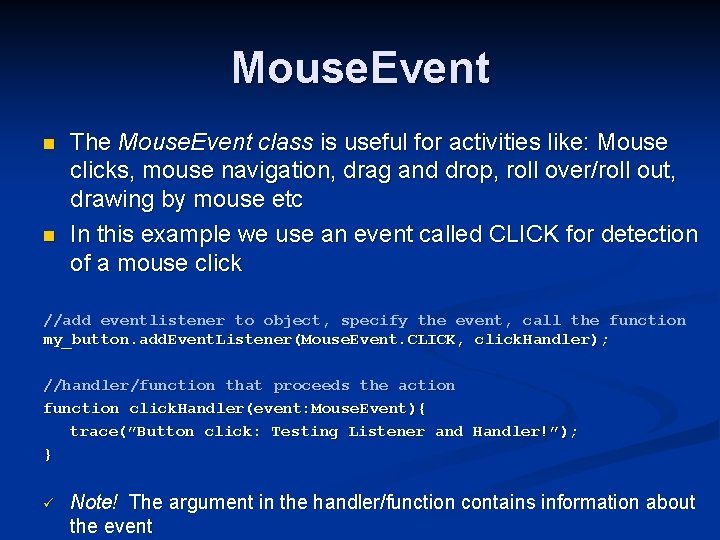
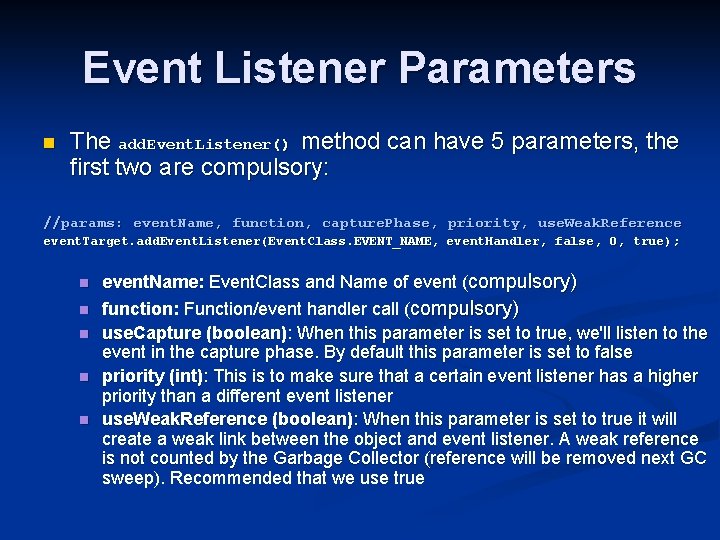
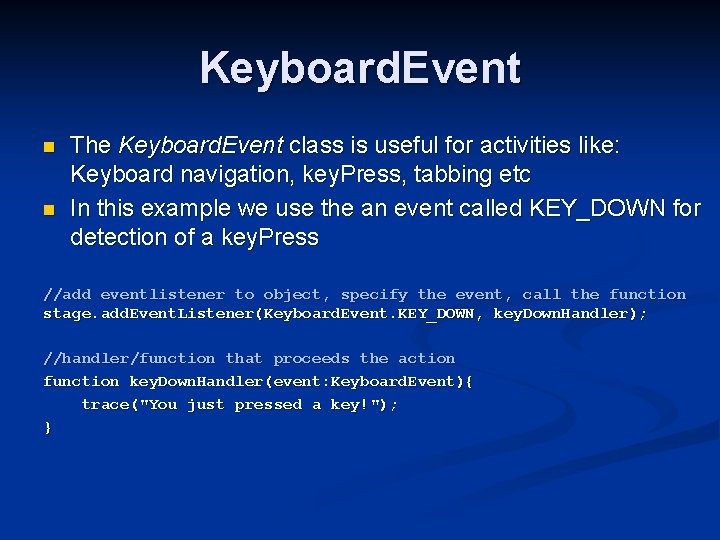
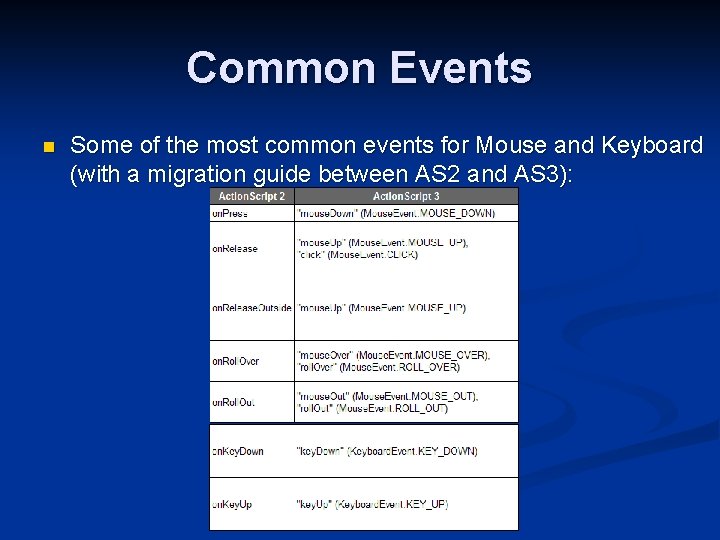
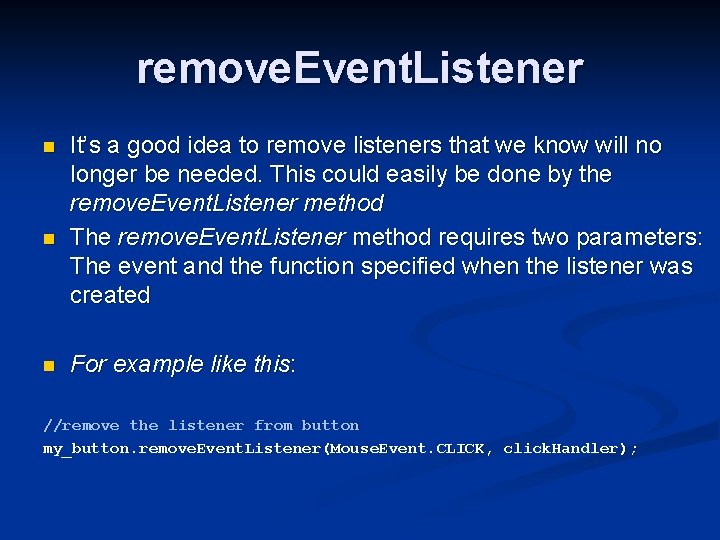
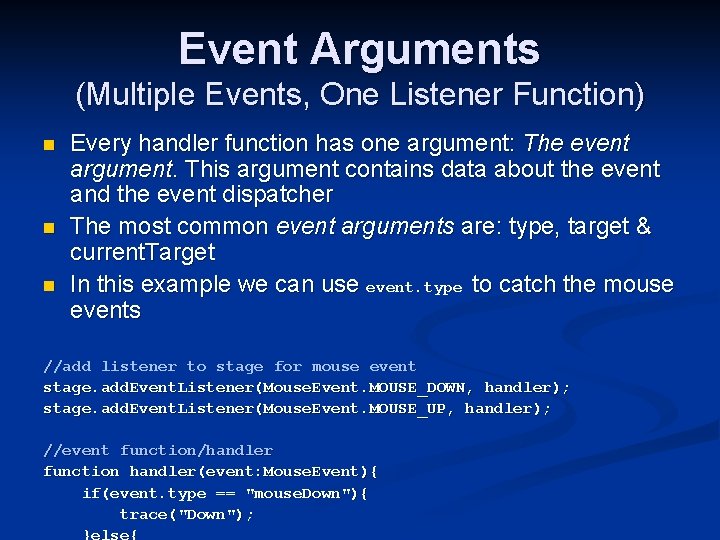
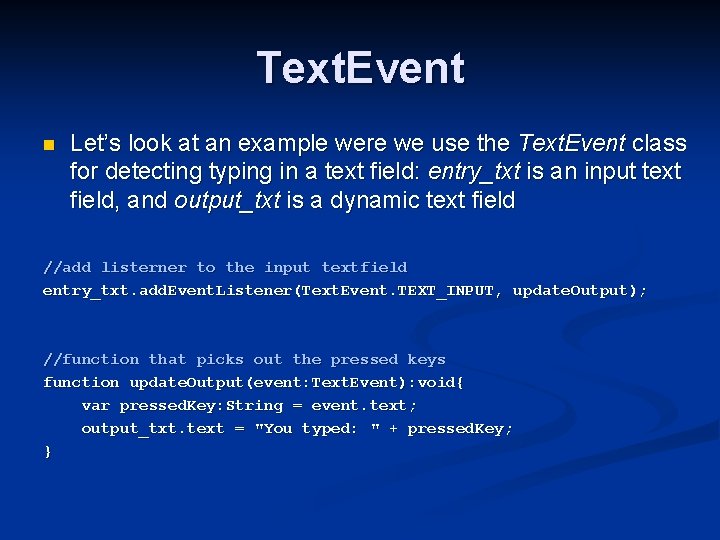
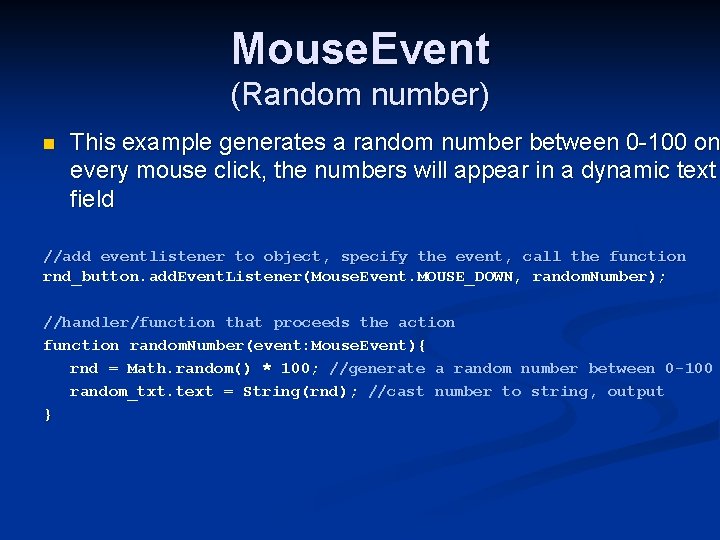
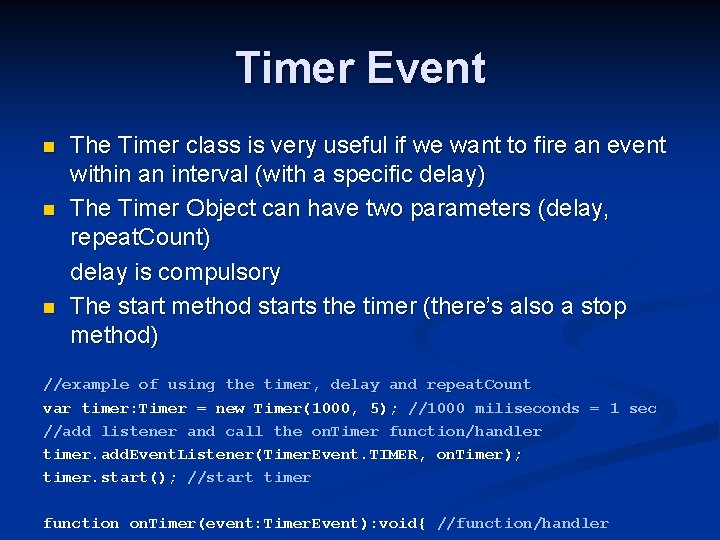
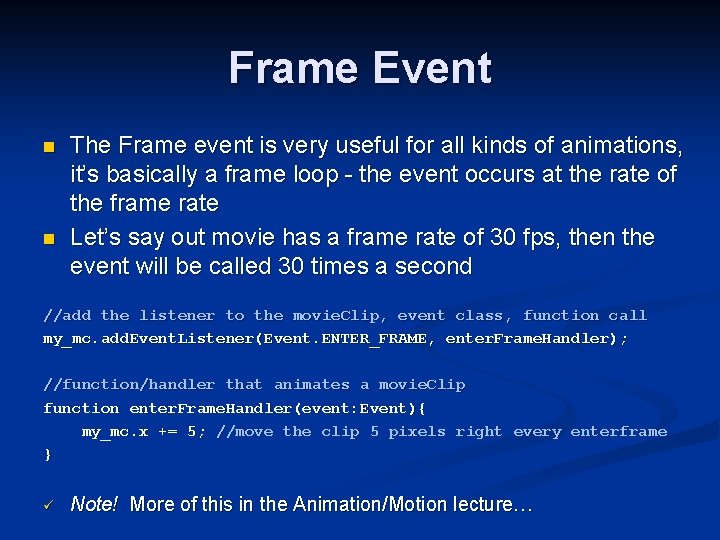
- Slides: 16
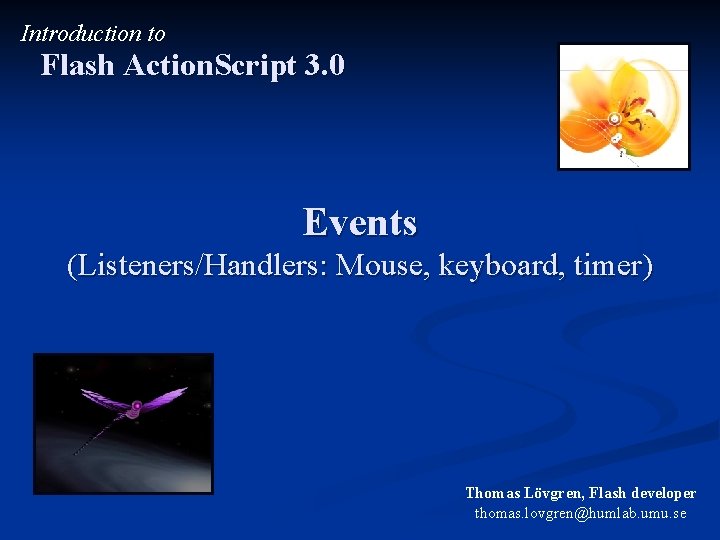
Introduction to Flash Action. Script 3. 0 Events (Listeners/Handlers: Mouse, keyboard, timer) Thomas Lövgren, Flash developer thomas. lovgren@humlab. umu. se
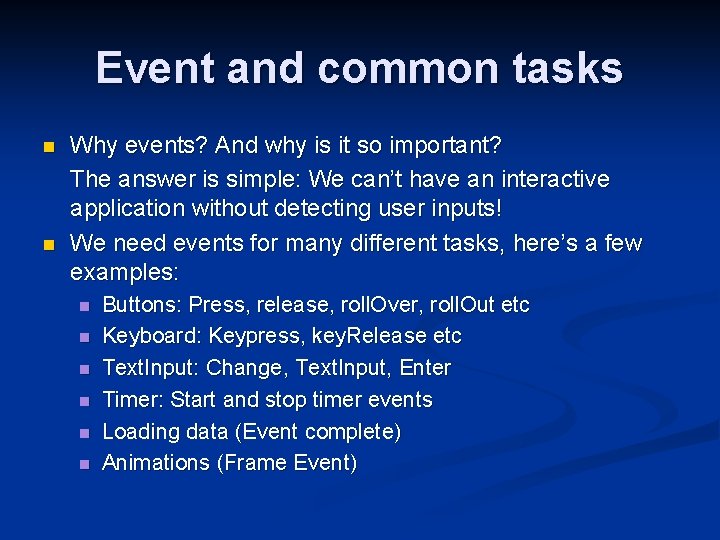
Event and common tasks n n Why events? And why is it so important? The answer is simple: We can’t have an interactive application without detecting user inputs! We need events for many different tasks, here’s a few examples: n n n Buttons: Press, release, roll. Over, roll. Out etc Keyboard: Keypress, key. Release etc Text. Input: Change, Text. Input, Enter Timer: Start and stop timer events Loading data (Event complete) Animations (Frame Event)
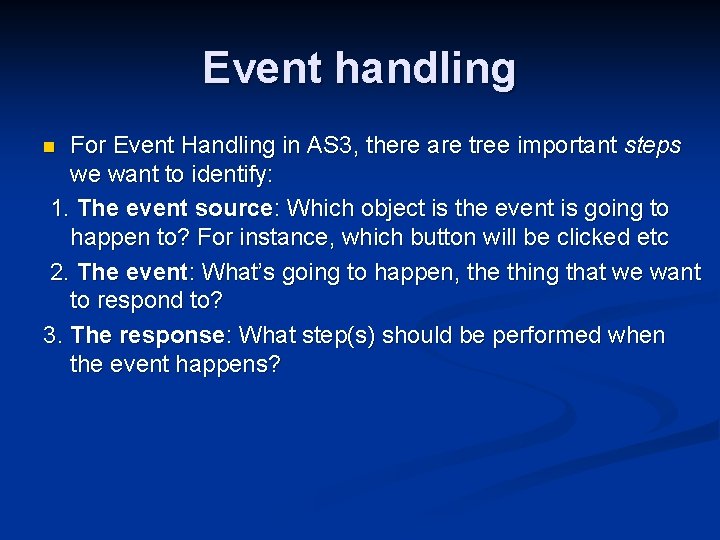
Event handling For Event Handling in AS 3, there are tree important steps we want to identify: 1. The event source: Which object is the event is going to happen to? For instance, which button will be clicked etc 2. The event: What’s going to happen, the thing that we want to respond to? 3. The response: What step(s) should be performed when the event happens? n
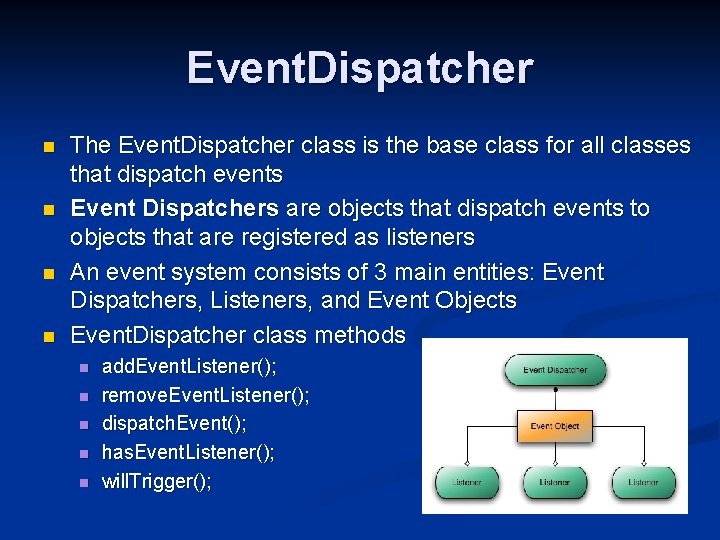
Event. Dispatcher n n The Event. Dispatcher class is the base class for all classes that dispatch events Event Dispatchers are objects that dispatch events to objects that are registered as listeners An event system consists of 3 main entities: Event Dispatchers, Listeners, and Event Objects Event. Dispatcher class methods n n n add. Event. Listener(); remove. Event. Listener(); dispatch. Event(); has. Event. Listener(); will. Trigger();
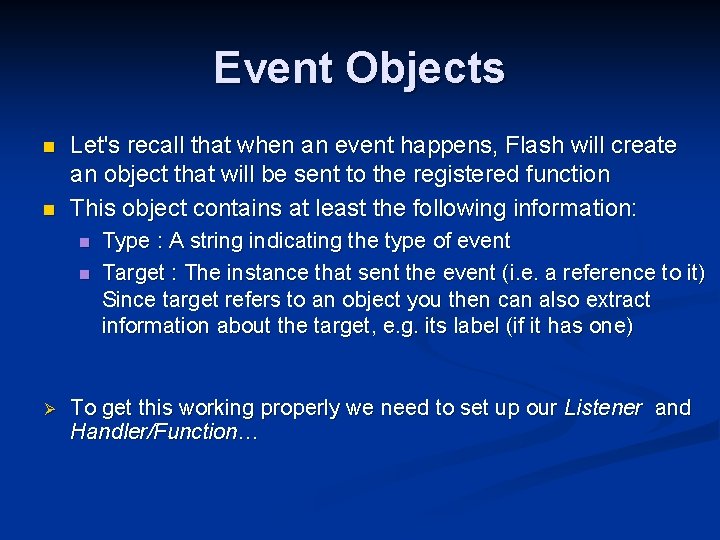
Event Objects n n Let's recall that when an event happens, Flash will create an object that will be sent to the registered function This object contains at least the following information: n n Ø Type : A string indicating the type of event Target : The instance that sent the event (i. e. a reference to it) Since target refers to an object you then can also extract information about the target, e. g. its label (if it has one) To get this working properly we need to set up our Listener and Handler/Function…
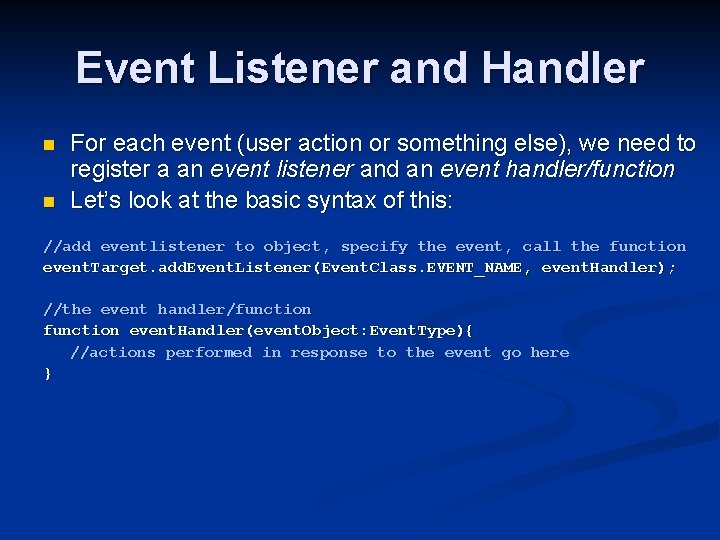
Event Listener and Handler n n For each event (user action or something else), we need to register a an event listener and an event handler/function Let’s look at the basic syntax of this: //add eventlistener to object, specify the event, call the function event. Target. add. Event. Listener(Event. Class. EVENT_NAME, event. Handler); //the event handler/function event. Handler(event. Object: Event. Type){ //actions performed in response to the event go here }
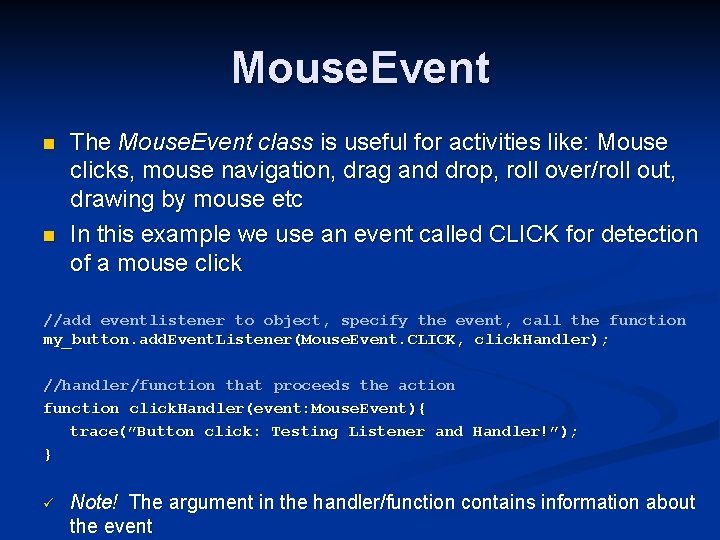
Mouse. Event n n The Mouse. Event class is useful for activities like: Mouse clicks, mouse navigation, drag and drop, roll over/roll out, drawing by mouse etc In this example we use an event called CLICK for detection of a mouse click //add eventlistener to object, specify the event, call the function my_button. add. Event. Listener(Mouse. Event. CLICK, click. Handler); //handler/function that proceeds the action function click. Handler(event: Mouse. Event){ trace(”Button click: Testing Listener and Handler!”); } ü Note! The argument in the handler/function contains information about the event
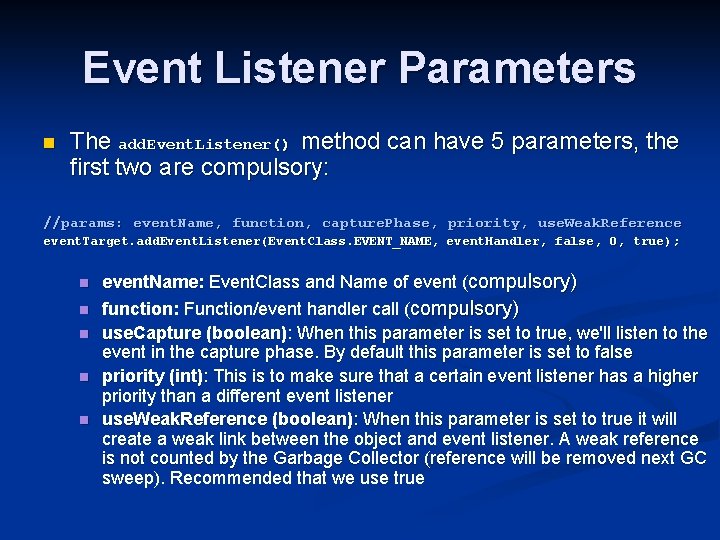
Event Listener Parameters n The add. Event. Listener() method can have 5 parameters, the first two are compulsory: //params: event. Name, function, capture. Phase, priority, use. Weak. Reference event. Target. add. Event. Listener(Event. Class. EVENT_NAME, event. Handler, false, 0, true); n n n event. Name: Event. Class and Name of event (compulsory) function: Function/event handler call (compulsory) use. Capture (boolean): When this parameter is set to true, we'll listen to the event in the capture phase. By default this parameter is set to false priority (int): This is to make sure that a certain event listener has a higher priority than a different event listener use. Weak. Reference (boolean): When this parameter is set to true it will create a weak link between the object and event listener. A weak reference is not counted by the Garbage Collector (reference will be removed next GC sweep). Recommended that we use true
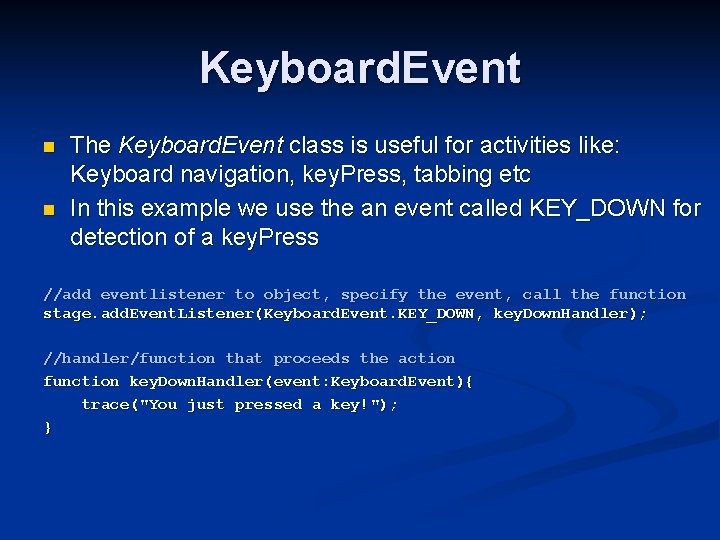
Keyboard. Event n n The Keyboard. Event class is useful for activities like: Keyboard navigation, key. Press, tabbing etc In this example we use the an event called KEY_DOWN for detection of a key. Press //add eventlistener to object, specify the event, call the function stage. add. Event. Listener(Keyboard. Event. KEY_DOWN, key. Down. Handler); //handler/function that proceeds the action function key. Down. Handler(event: Keyboard. Event){ trace("You just pressed a key!"); }
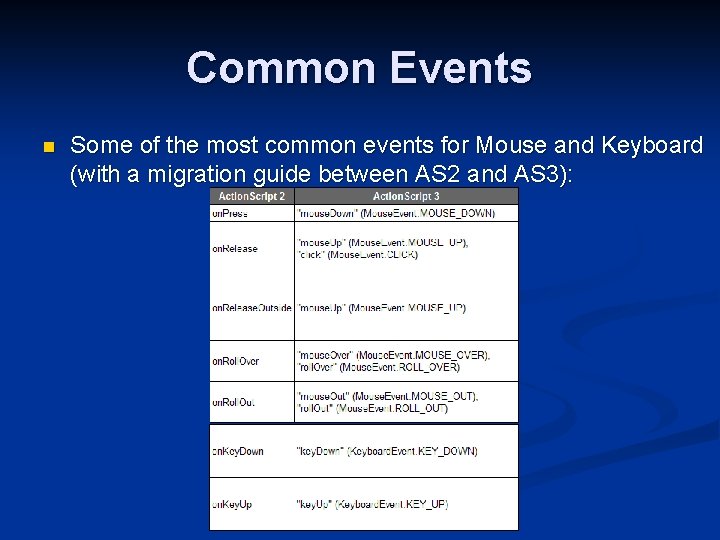
Common Events n Some of the most common events for Mouse and Keyboard (with a migration guide between AS 2 and AS 3):
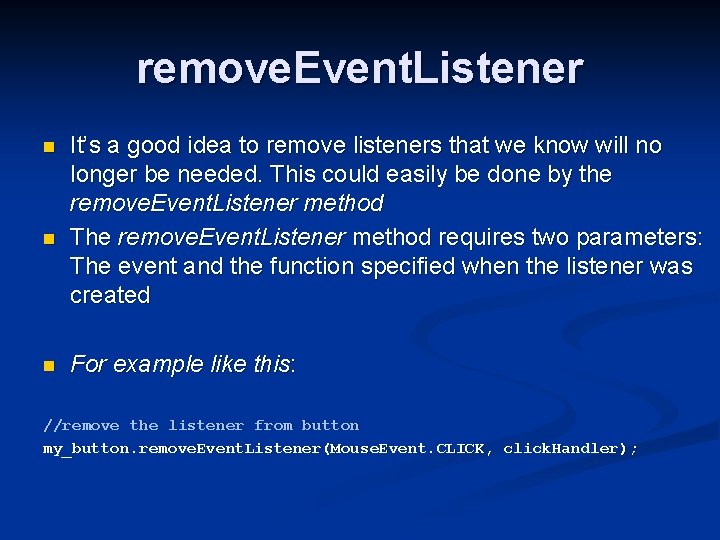
remove. Event. Listener n n n It’s a good idea to remove listeners that we know will no longer be needed. This could easily be done by the remove. Event. Listener method The remove. Event. Listener method requires two parameters: The event and the function specified when the listener was created For example like this: //remove the listener from button my_button. remove. Event. Listener(Mouse. Event. CLICK, click. Handler);
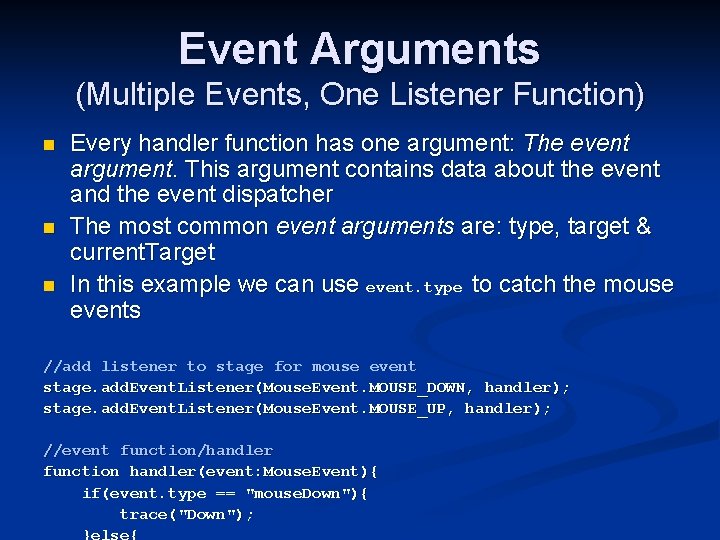
Event Arguments (Multiple Events, One Listener Function) n n n Every handler function has one argument: The event argument. This argument contains data about the event and the event dispatcher The most common event arguments are: type, target & current. Target In this example we can use event. type to catch the mouse events //add listener to stage for mouse event stage. add. Event. Listener(Mouse. Event. MOUSE_DOWN, handler); stage. add. Event. Listener(Mouse. Event. MOUSE_UP, handler); //event function/handler function handler(event: Mouse. Event){ if(event. type == "mouse. Down"){ trace("Down"); }else{
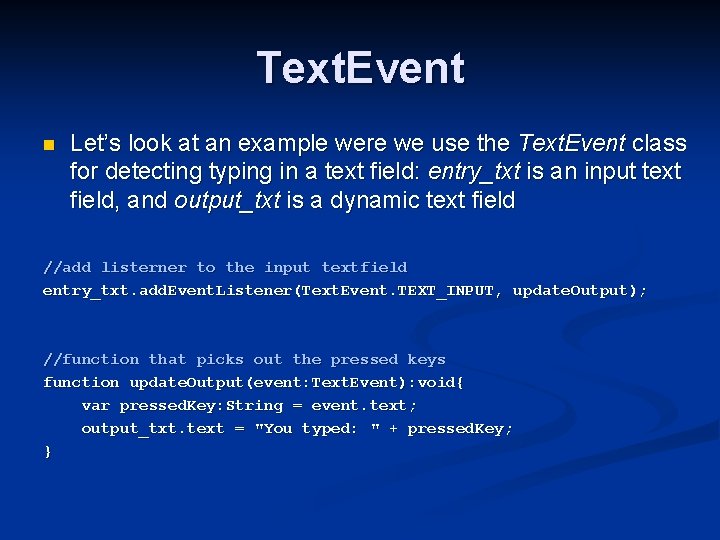
Text. Event n Let’s look at an example were we use the Text. Event class for detecting typing in a text field: entry_txt is an input text field, and output_txt is a dynamic text field //add listerner to the input textfield entry_txt. add. Event. Listener(Text. Event. TEXT_INPUT, update. Output); //function that picks out the pressed keys function update. Output(event: Text. Event): void{ var pressed. Key: String = event. text; output_txt. text = "You typed: " + pressed. Key; }
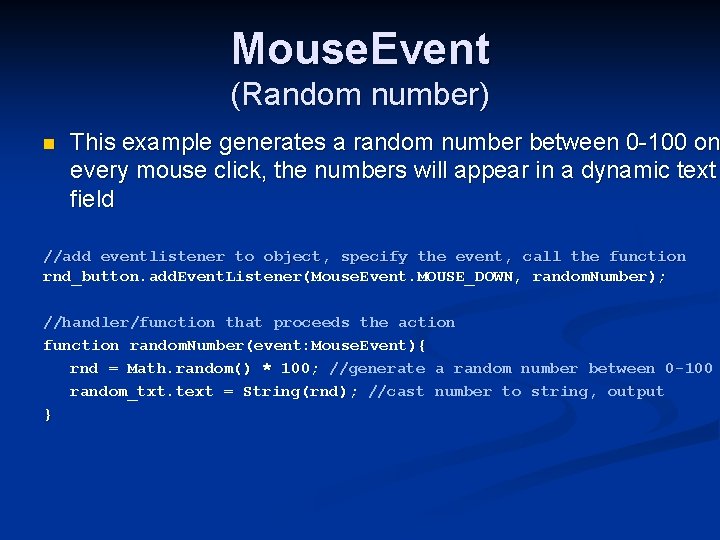
Mouse. Event (Random number) n This example generates a random number between 0 -100 on every mouse click, the numbers will appear in a dynamic text field //add eventlistener to object, specify the event, call the function rnd_button. add. Event. Listener(Mouse. Event. MOUSE_DOWN, random. Number); //handler/function that proceeds the action function random. Number(event: Mouse. Event){ rnd = Math. random() * 100; //generate a random number between 0 -100 random_txt. text = String(rnd); //cast number to string, output }
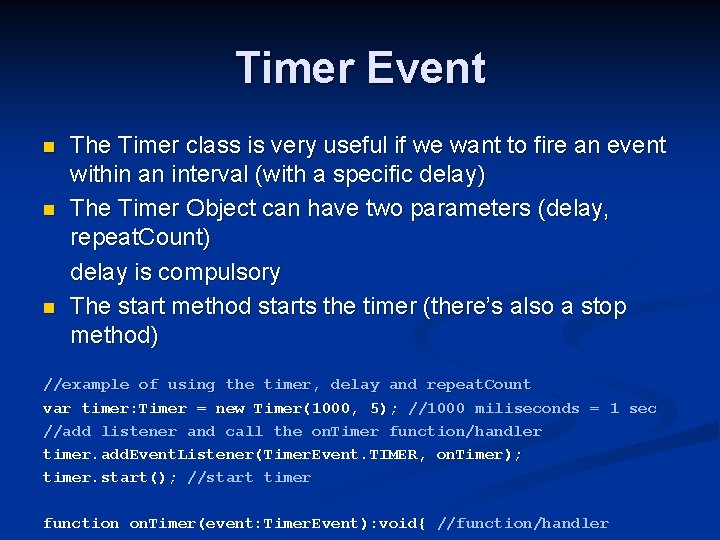
Timer Event n n n The Timer class is very useful if we want to fire an event within an interval (with a specific delay) The Timer Object can have two parameters (delay, repeat. Count) delay is compulsory The start method starts the timer (there’s also a stop method) //example of using the timer, delay and repeat. Count var timer: Timer = new Timer(1000, 5); //1000 miliseconds = 1 sec //add listener and call the on. Timer function/handler timer. add. Event. Listener(Timer. Event. TIMER, on. Timer); timer. start(); //start timer function on. Timer(event: Timer. Event): void{ //function/handler
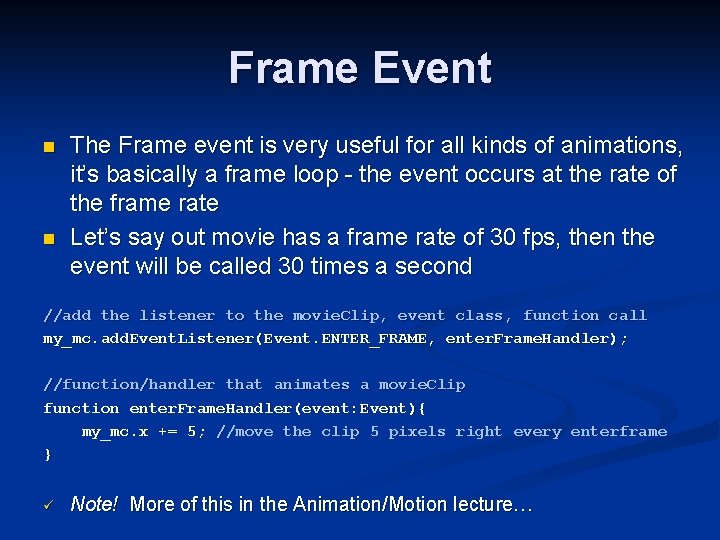
Frame Event n n The Frame event is very useful for all kinds of animations, it’s basically a frame loop - the event occurs at the rate of the frame rate Let’s say out movie has a frame rate of 30 fps, then the event will be called 30 times a second //add the listener to the movie. Clip, event class, function call my_mc. add. Event. Listener(Event. ENTER_FRAME, enter. Frame. Handler); //function/handler that animates a movie. Clip function enter. Frame. Handler(event: Event){ my_mc. x += 5; //move the clip 5 pixels right every enterframe } ü Note! More of this in the Animation/Motion lecture…