Introduction to Computing Using Python Execution Control Structures
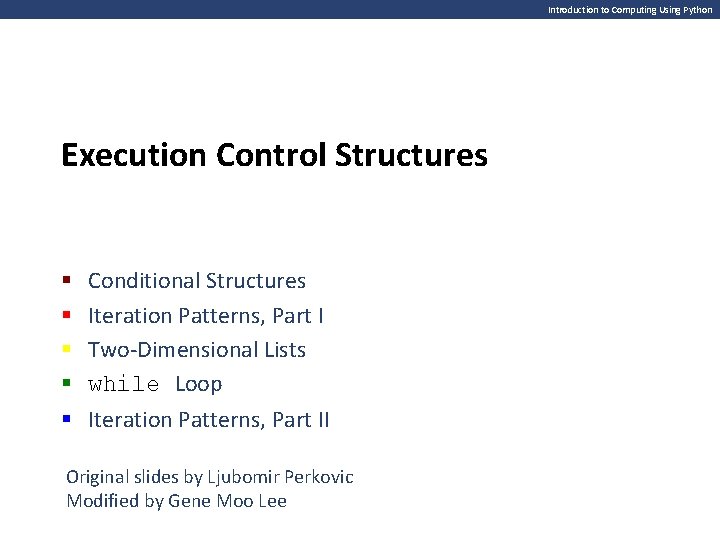
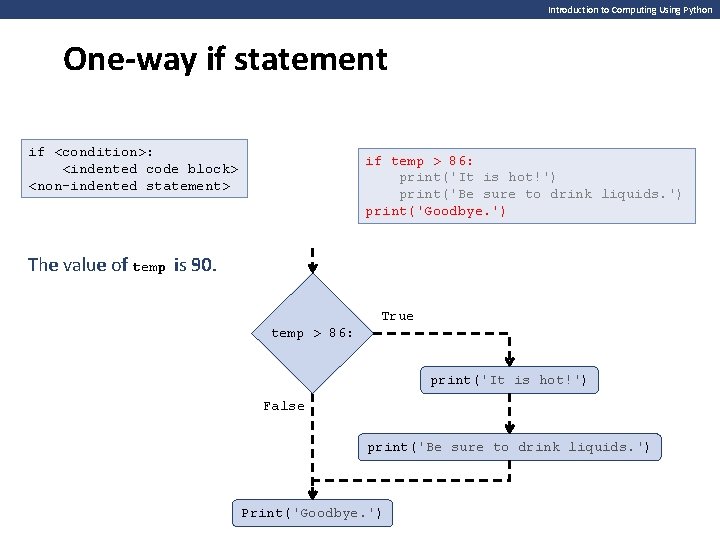
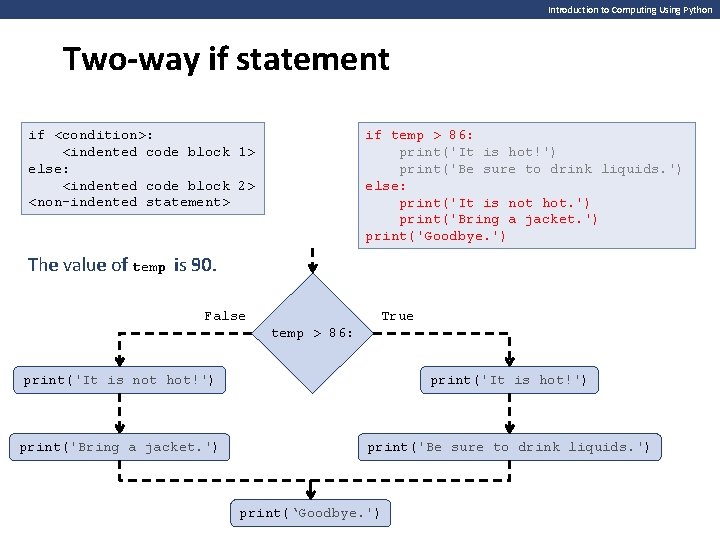
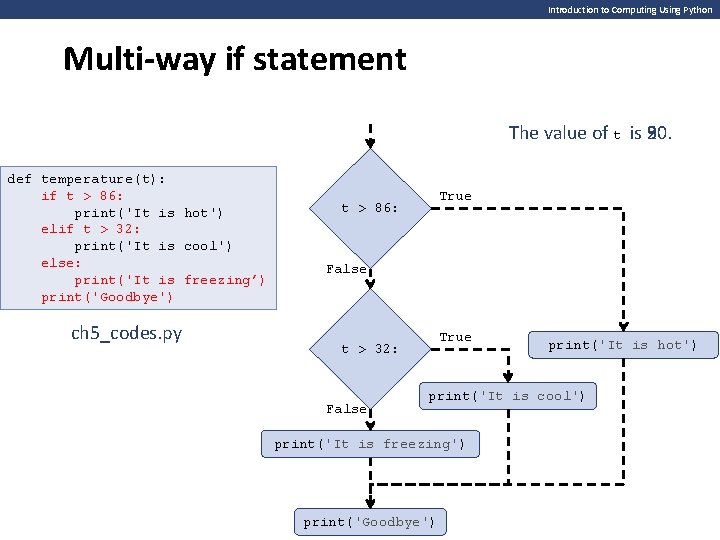
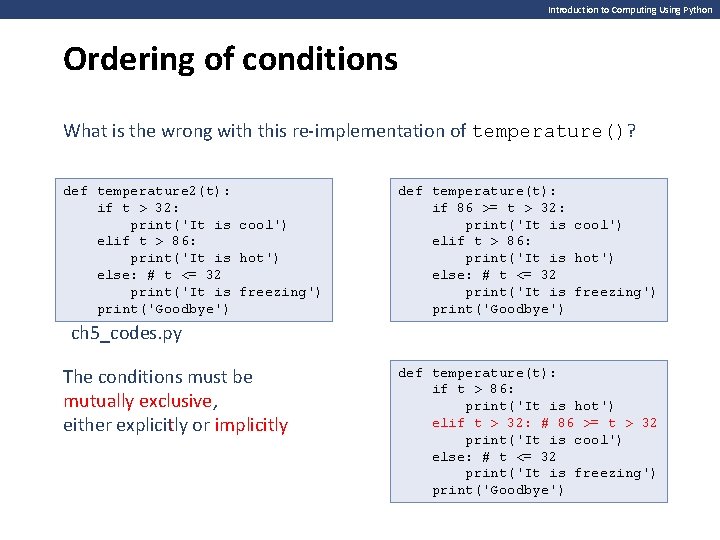
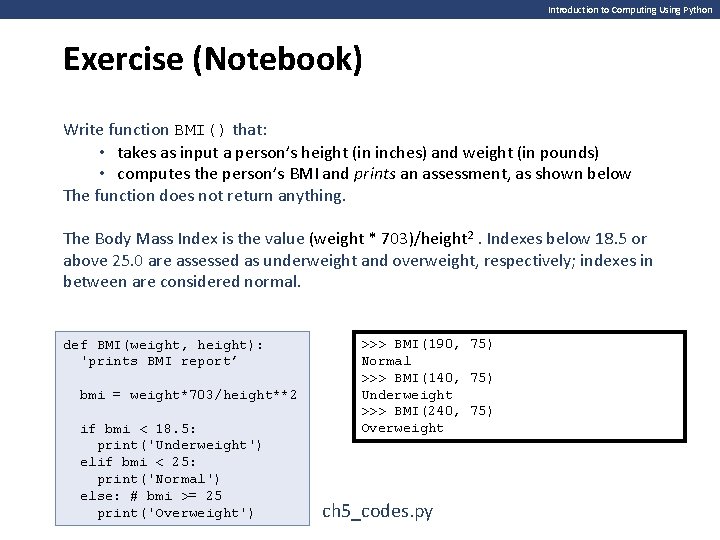
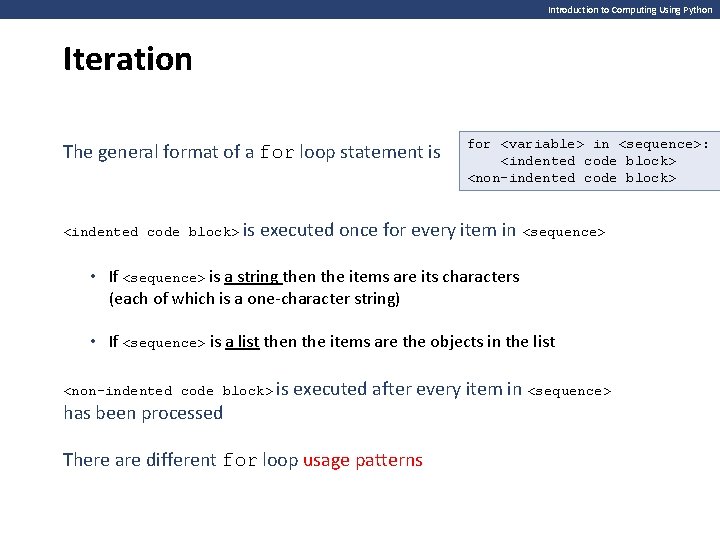
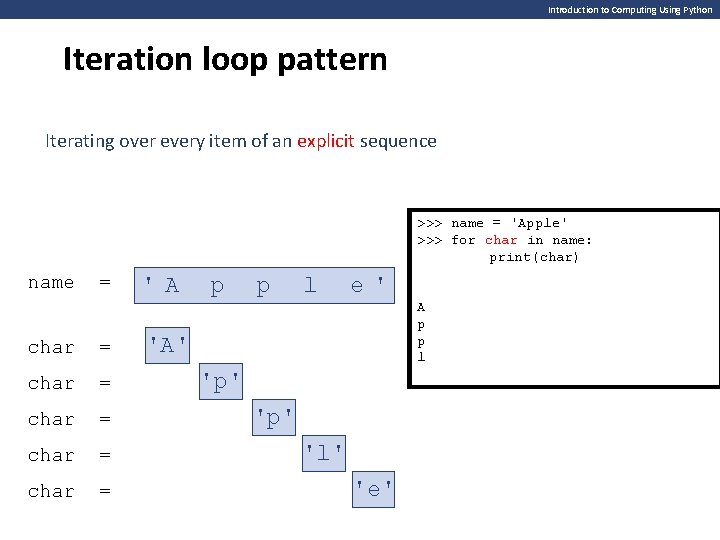
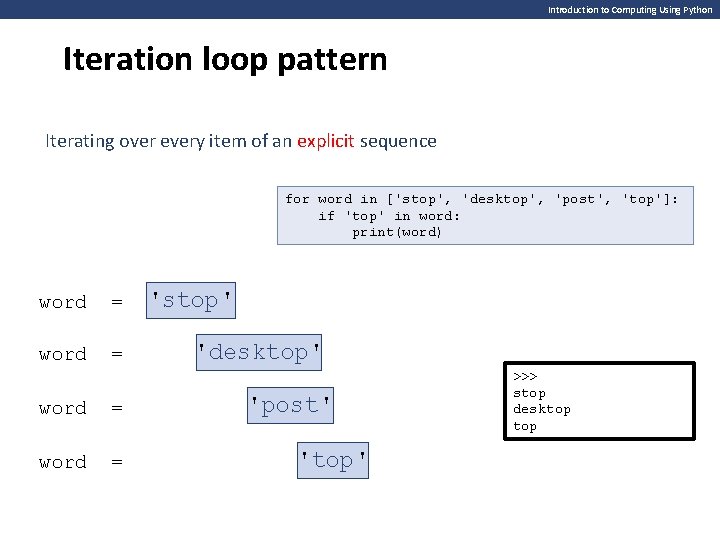
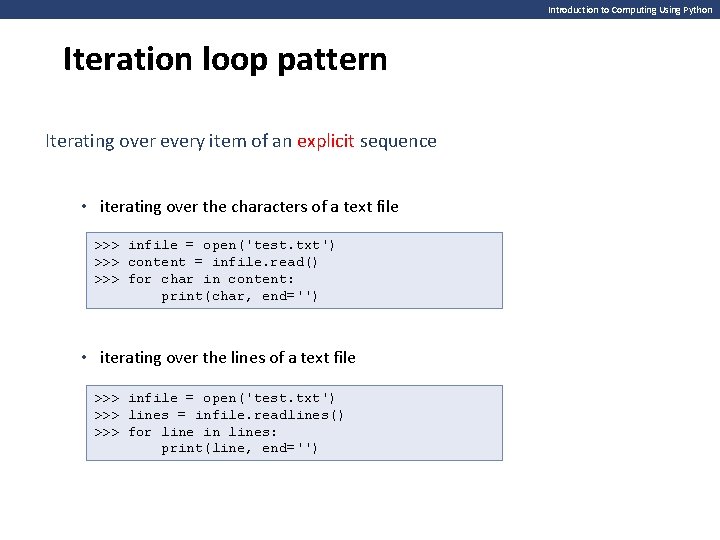
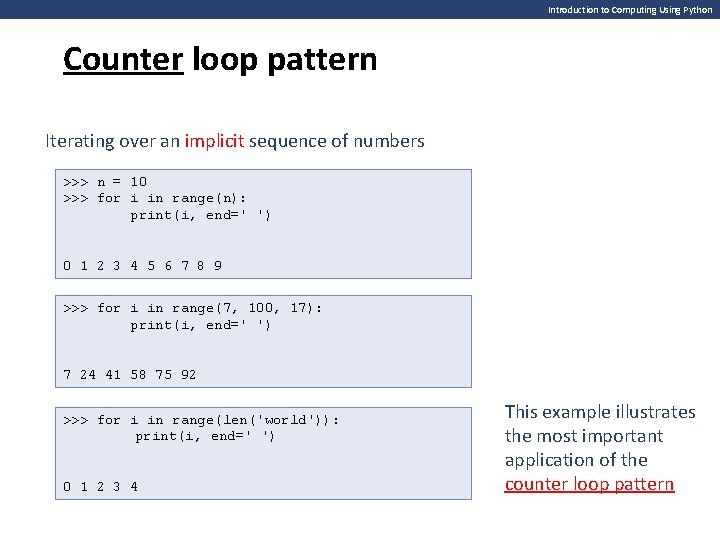
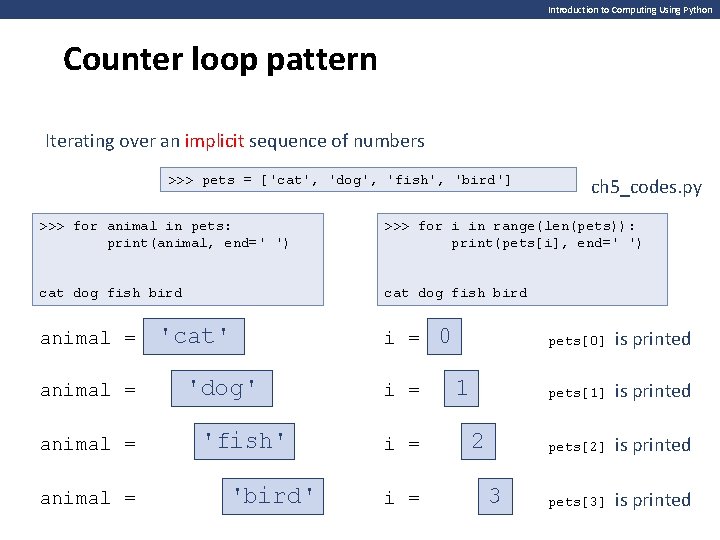
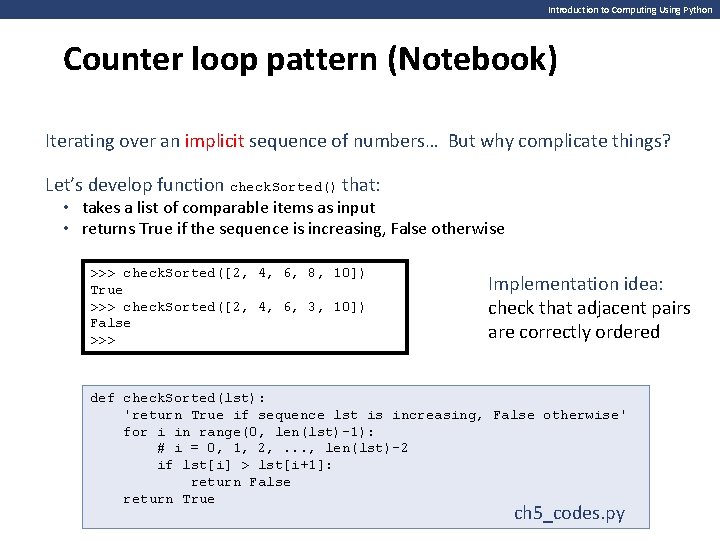
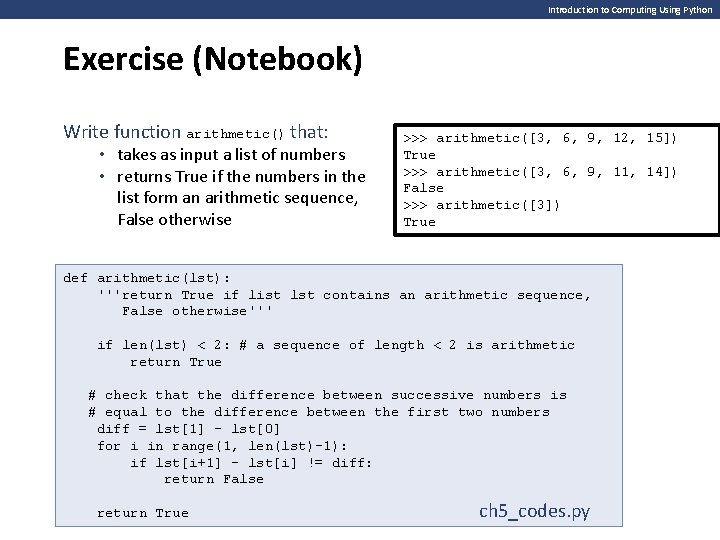
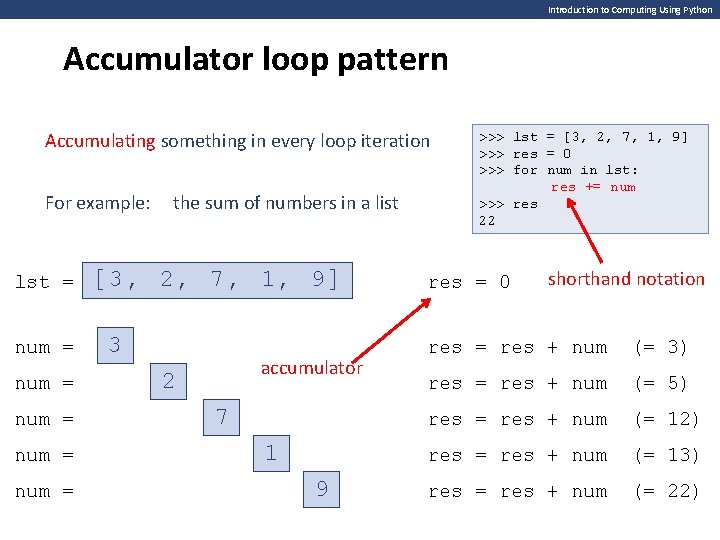
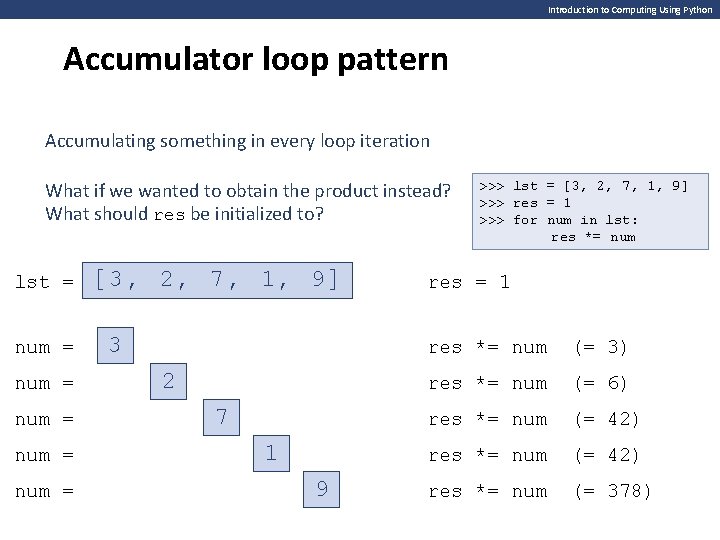
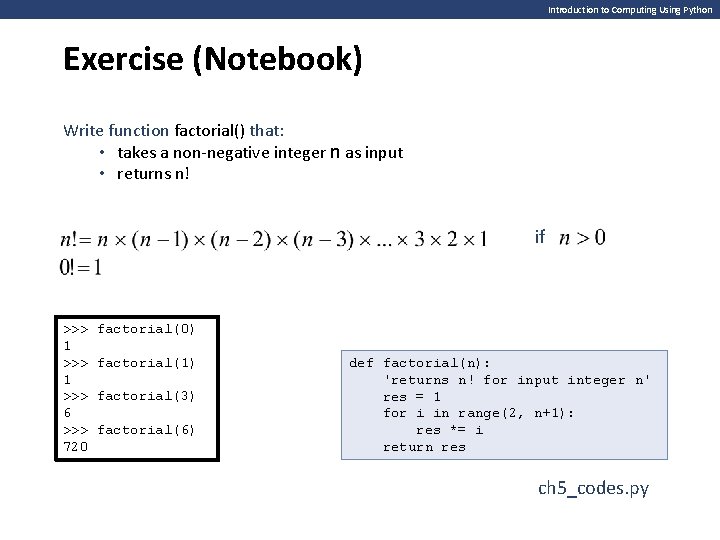
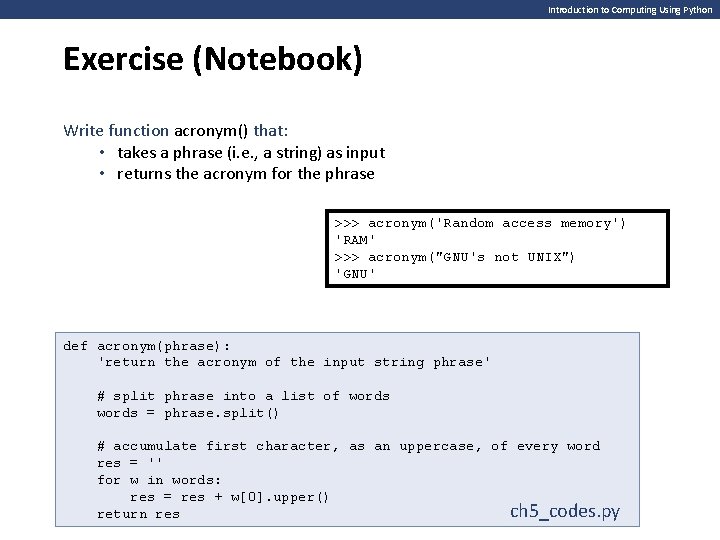
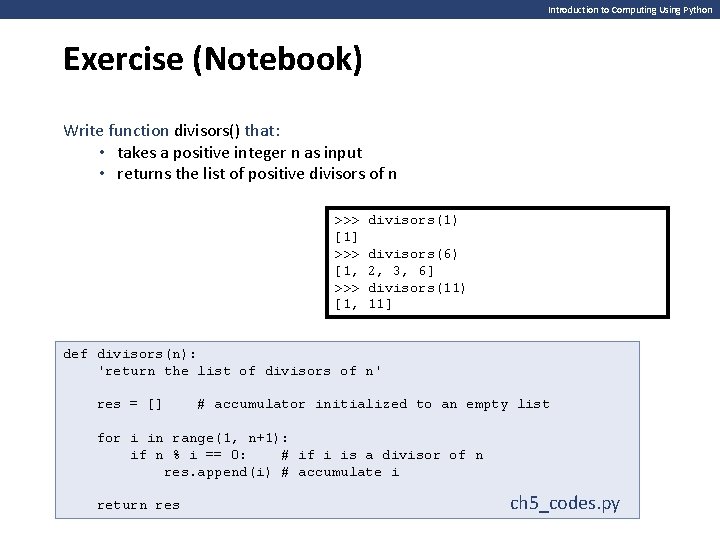
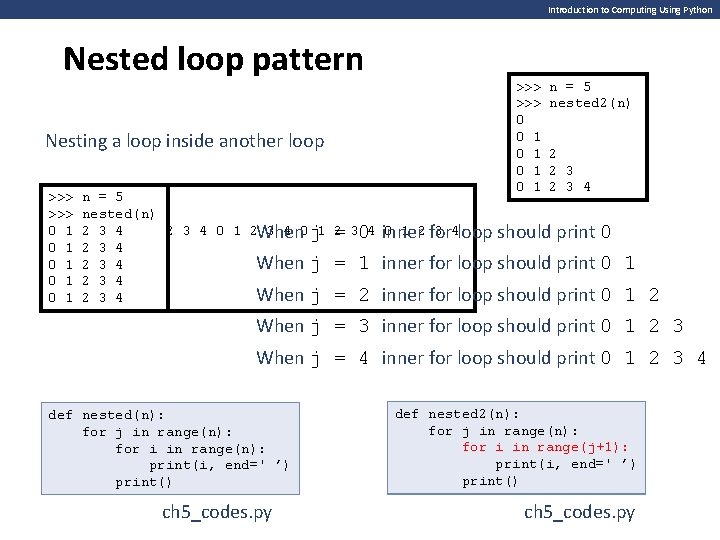
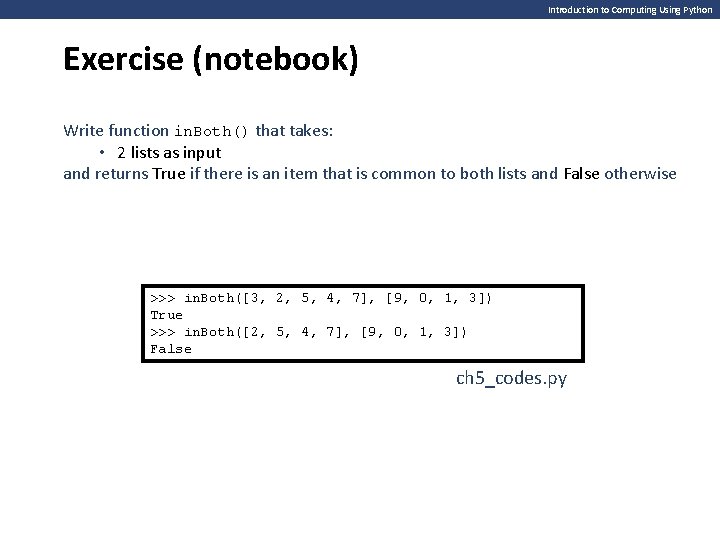
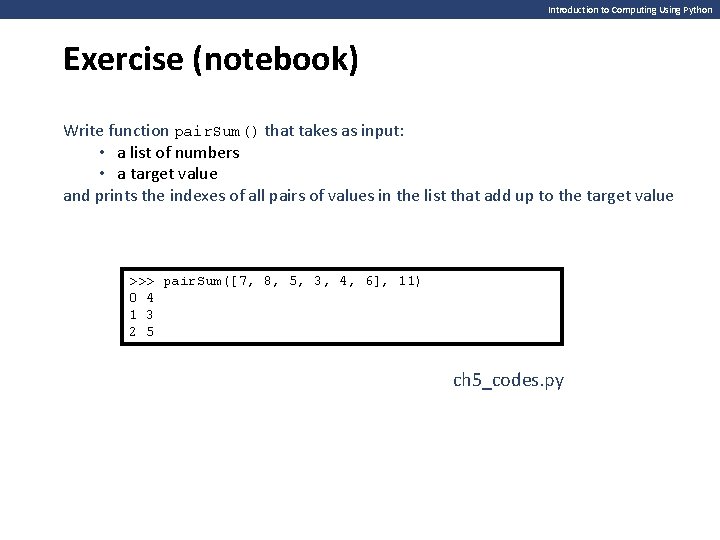
![Introduction to Computing Using Python Two-dimensional lists The list [3, 5, 7, 9] can Introduction to Computing Using Python Two-dimensional lists The list [3, 5, 7, 9] can](https://slidetodoc.com/presentation_image_h/982bc1419ebf4c3572a638644c5eabe7/image-23.jpg)
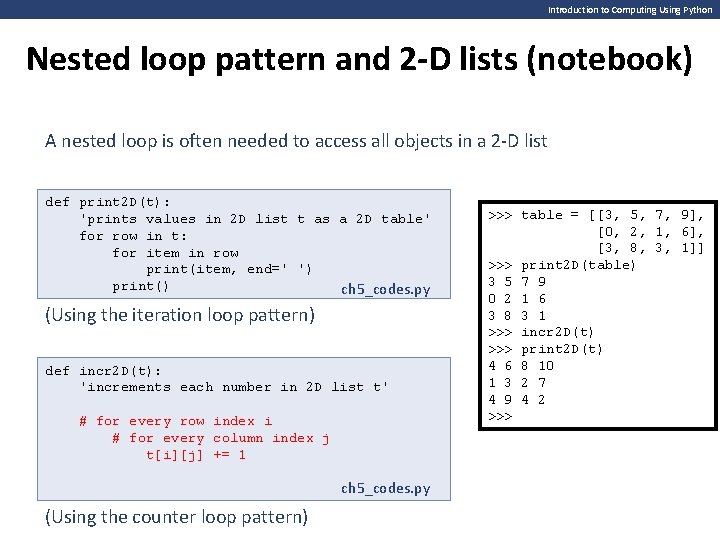
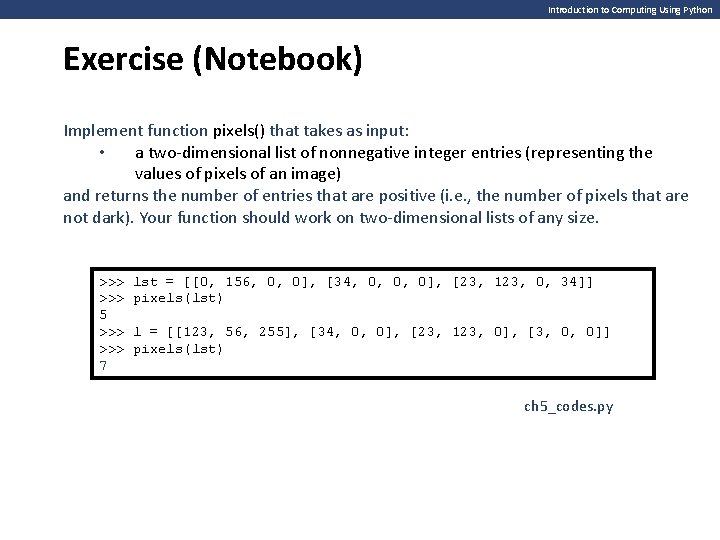
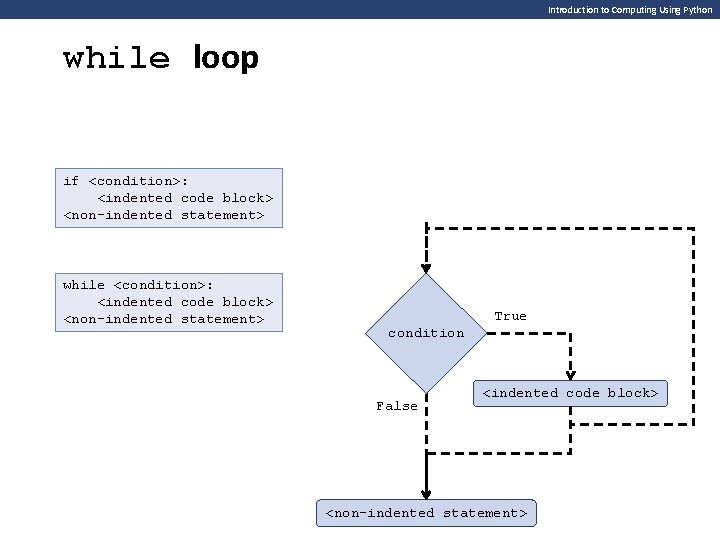
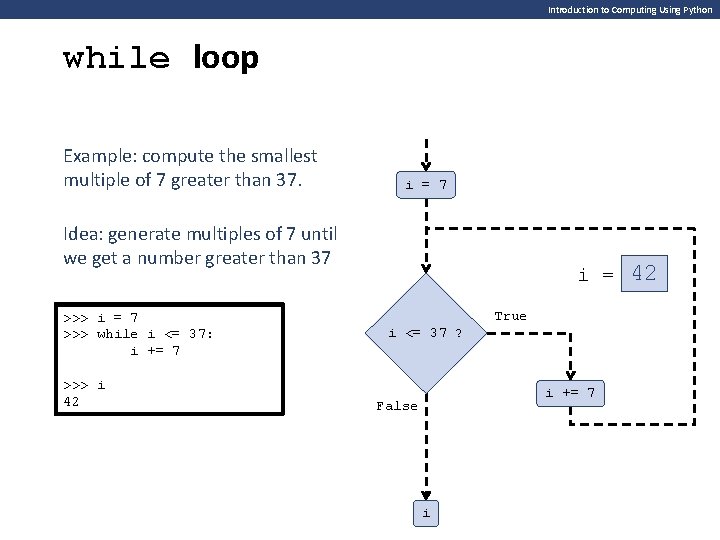
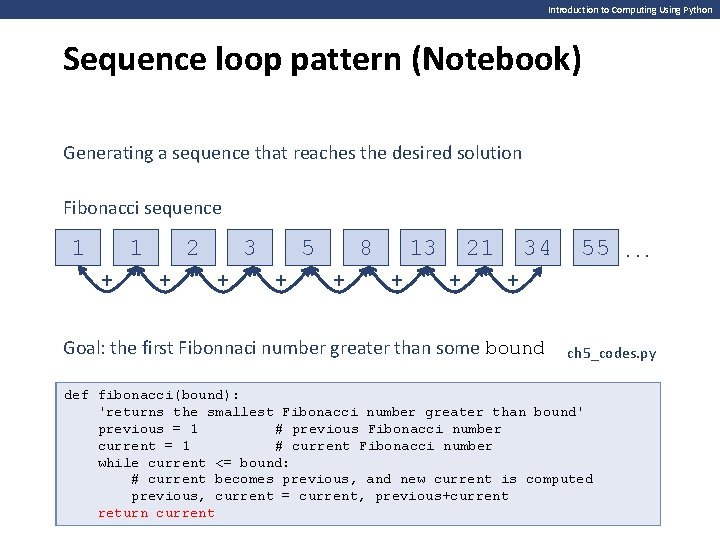
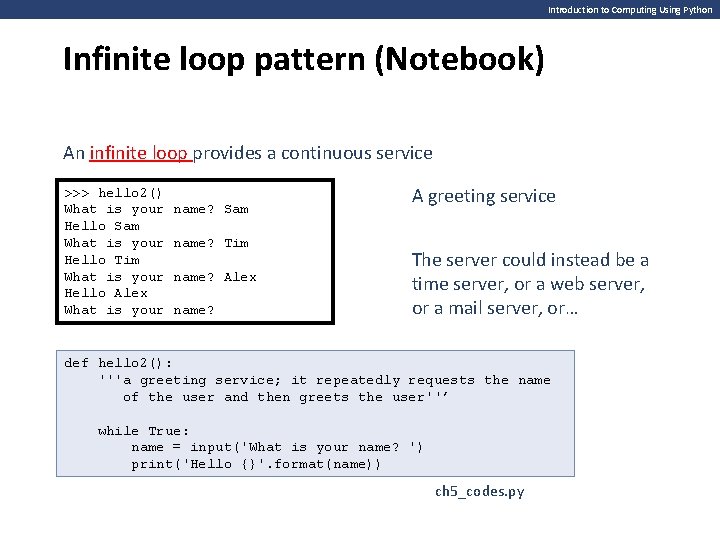
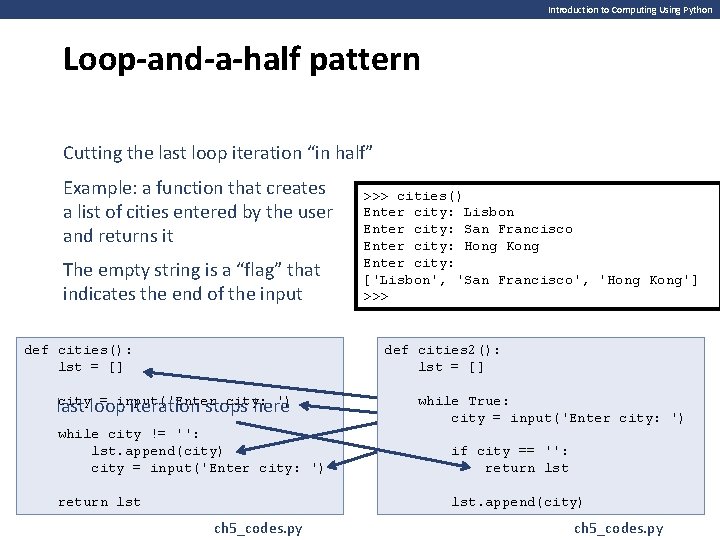
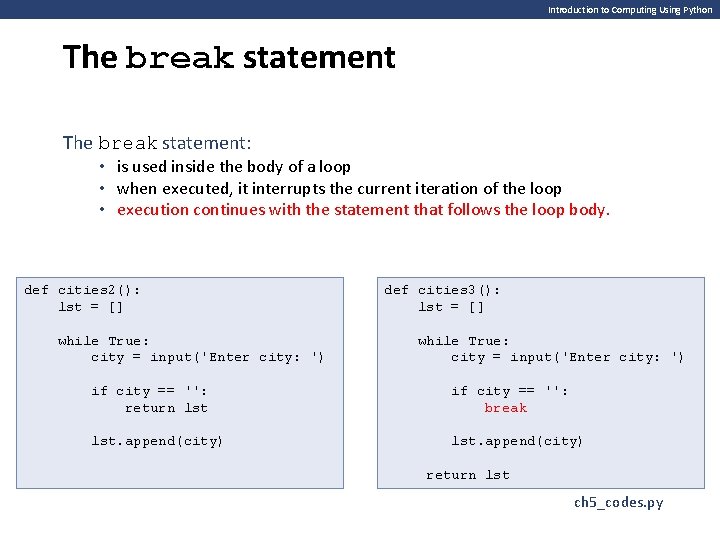
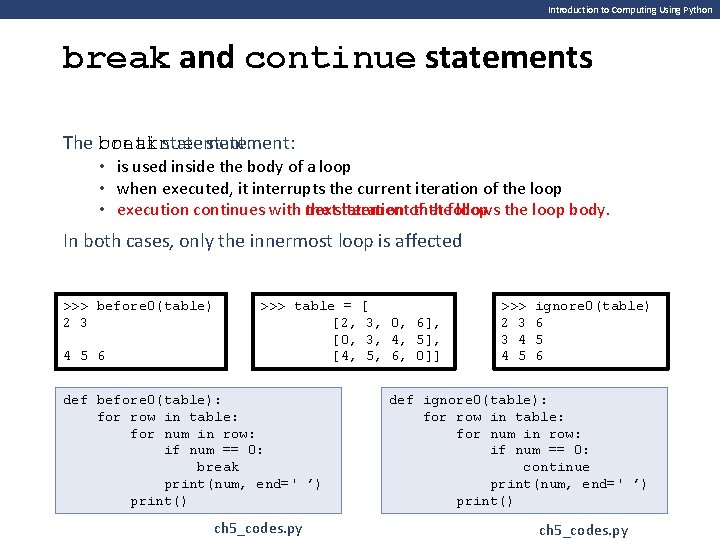
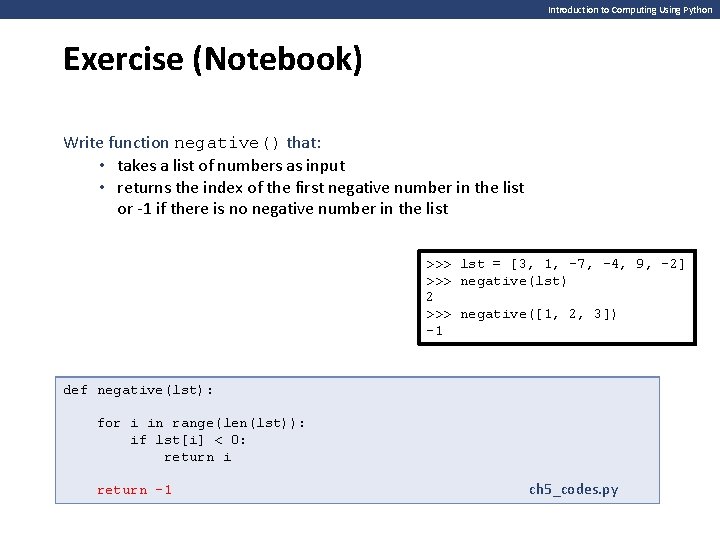
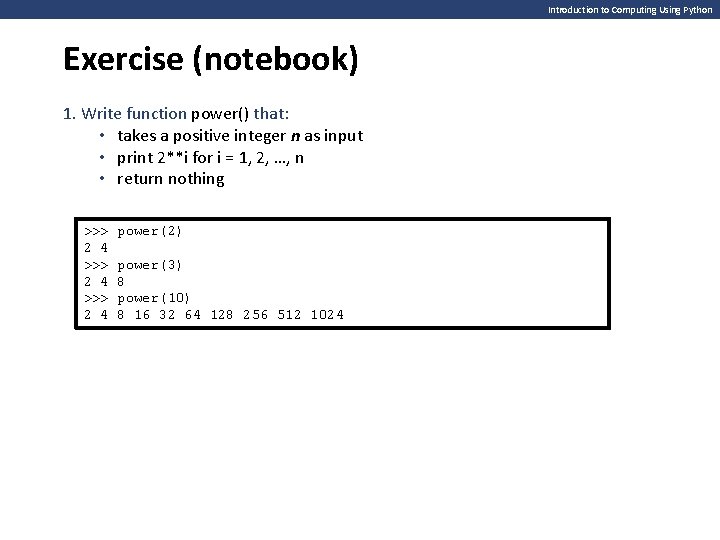
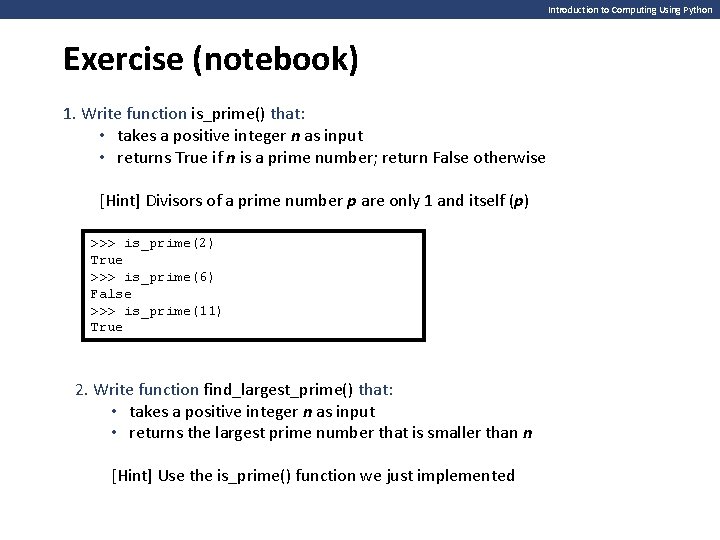
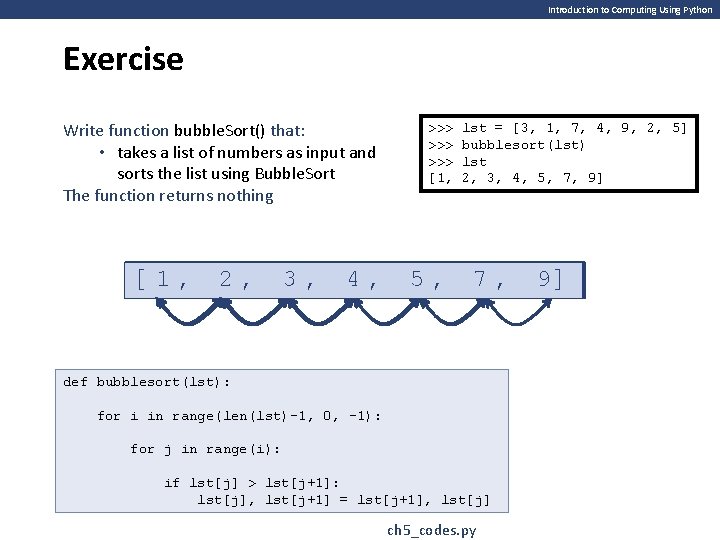
- Slides: 36
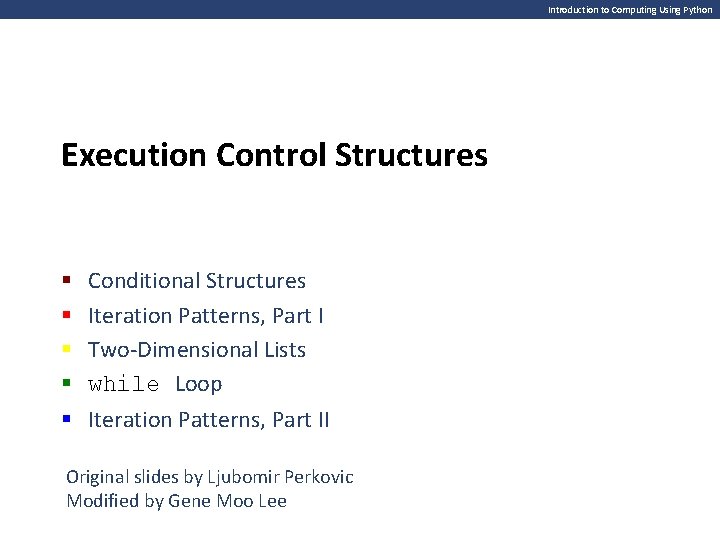
Introduction to Computing Using Python Execution Control Structures § § § Conditional Structures Iteration Patterns, Part I Two-Dimensional Lists while Loop Iteration Patterns, Part II Original slides by Ljubomir Perkovic Modified by Gene Moo Lee
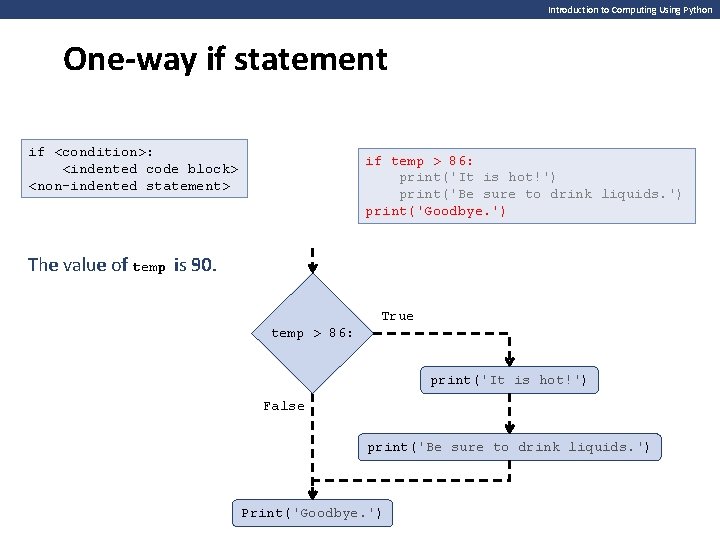
Introduction to Computing Using Python One-way if statement if <condition>: <indented code block> <non-indented statement> if temp > 86: print('It is hot!') print('Be sure to drink liquids. ') print('Goodbye. ') The value of temp is 50. 90. True temp > 86: print('It is hot!') False print('Be sure to drink liquids. ') Print('Goodbye. ')
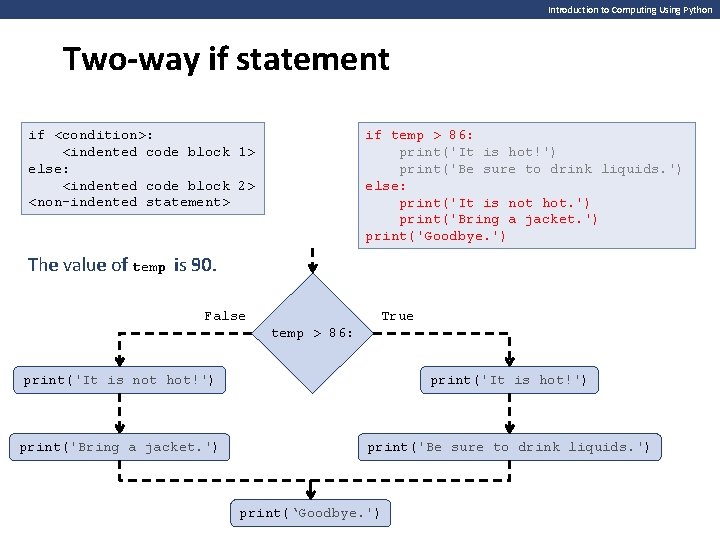
Introduction to Computing Using Python Two-way if statement if <condition>: <indented code block 1> else: <indented code block 2> <non-indented statement> if temp > 86: print('It is hot!') print('Be sure to drink liquids. ') else: print('It is not hot. ') print('Bring a jacket. ') print('Goodbye. ') The value of temp is 50. 90. False True temp > 86: print('It is not hot!') print('It is hot!') print('Bring a jacket. ') print('Be sure to drink liquids. ') print(‘Goodbye. ')
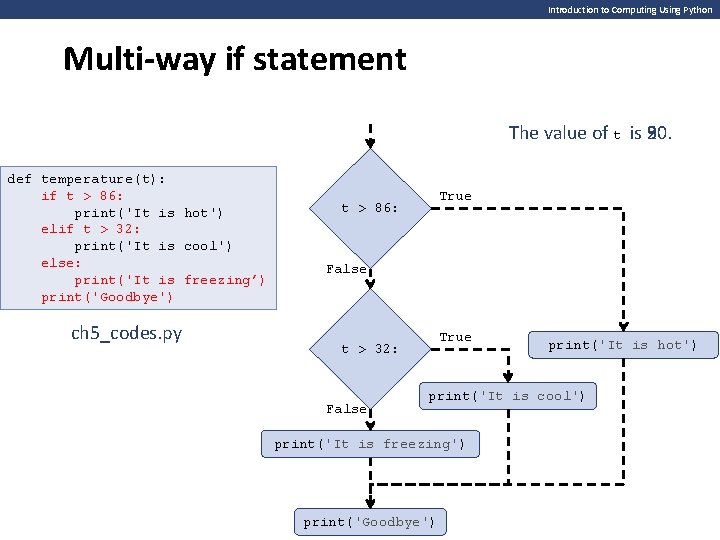
Introduction to Computing Using Python Multi-way if statement The value of t is 20. 90. 50. def temperature(t): if t > 86: print('It is hot') elif t > 32: print('It is cool') else: print('It is freezing’) print('Goodbye') ch 5_codes. py True t > 86: False True t > 32: False print('It is hot') print('It is cool') print('It is freezing') print('Goodbye')
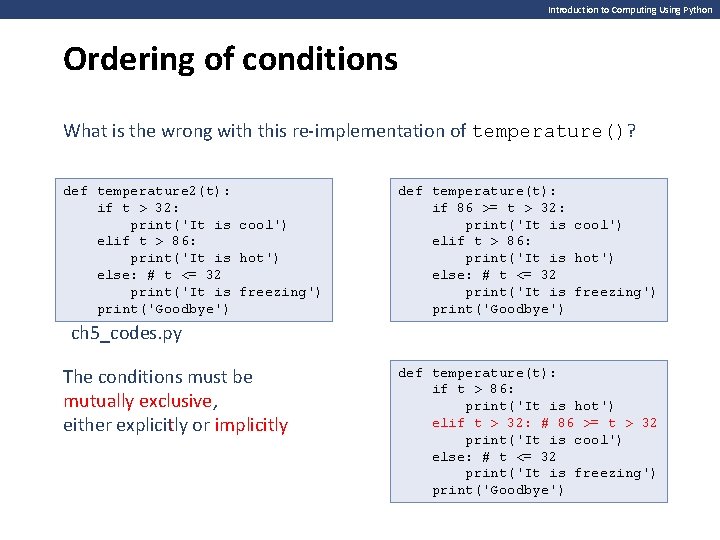
Introduction to Computing Using Python Ordering of conditions What is the wrong with this re-implementation of temperature()? def temperature 2(t): if t > 32: print('It is cool') elif t > 86: print('It is hot') else: # t <= 32 print('It is freezing') print('Goodbye') def temperature(t): if 86 >= t > 32: print('It is cool') elif t > 86: print('It is hot') else: # t <= 32 print('It is freezing') print('Goodbye') ch 5_codes. py The conditions must be mutually exclusive, either explicitly or implicitly def temperature(t): if t > 86: print('It is hot') elif t > 32: # 86 >= t > 32 print('It is cool') else: # t <= 32 print('It is freezing') print('Goodbye')
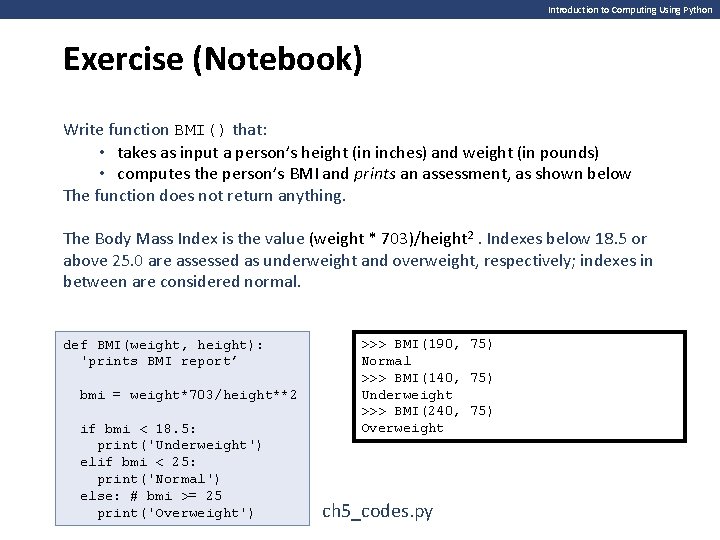
Introduction to Computing Using Python Exercise (Notebook) Write function BMI() that: • takes as input a person’s height (in inches) and weight (in pounds) • computes the person’s BMI and prints an assessment, as shown below The function does not return anything. The Body Mass Index is the value (weight * 703)/height 2. Indexes below 18. 5 or above 25. 0 are assessed as underweight and overweight, respectively; indexes in between are considered normal. def BMI(weight, height): 'prints BMI report’ bmi = weight*703/height**2 if bmi < 18. 5: print('Underweight') elif bmi < 25: print('Normal') else: # bmi >= 25 print('Overweight') >>> BMI(190, 75) Normal >>> BMI(140, 75) Underweight >>> BMI(240, 75) Overweight ch 5_codes. py
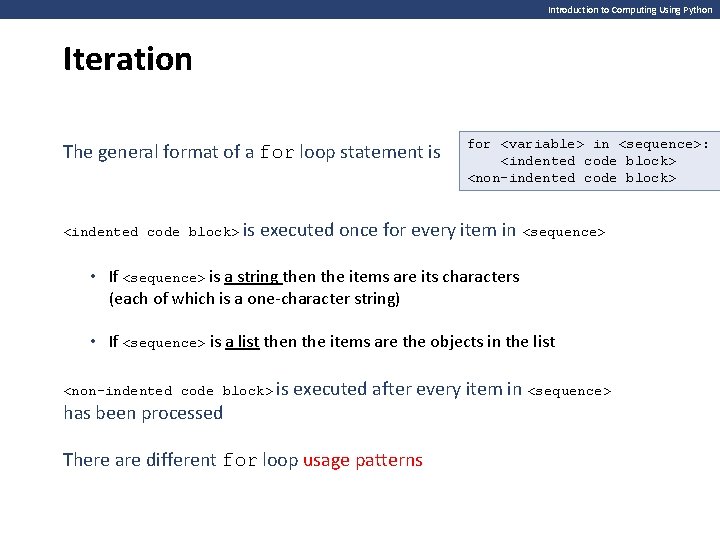
Introduction to Computing Using Python Iteration The general format of a for loop statement is <indented code block> is for <variable> in <sequence>: <indented code block> <non-indented code block> executed once for every item in <sequence> • If <sequence> is a string then the items are its characters (each of which is a one-character string) • If <sequence> is a list then the items are the objects in the list <non-indented code block> is has been processed executed after every item in <sequence> There are different for loop usage patterns
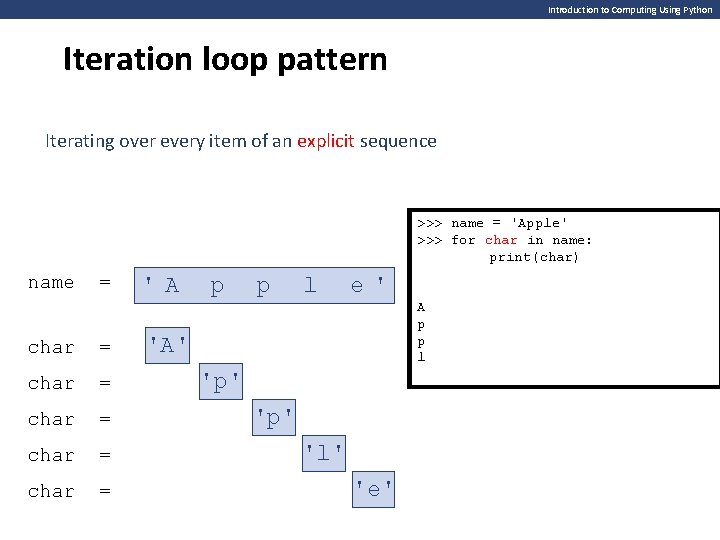
Introduction to Computing Using Python Iteration loop pattern Iterating over every item of an explicit sequence >>> name = 'Apple' >>> for char in name: print(char) name = char = char = 'A p p l e' A p p l e 'A' 'p' 'l' 'e'
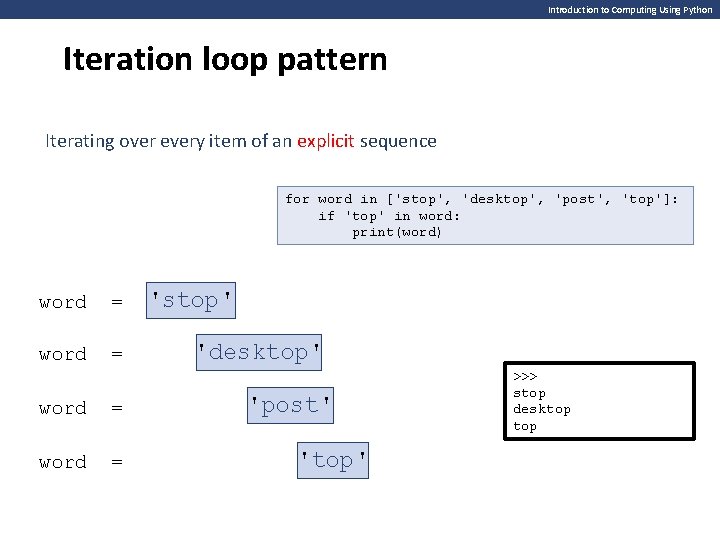
Introduction to Computing Using Python Iteration loop pattern Iterating over every item of an explicit sequence for word in ['stop', 'desktop', 'post', 'top']: if 'top' in word: print(word) word = 'stop' 'desktop' 'post' 'top' >>> stop desktop
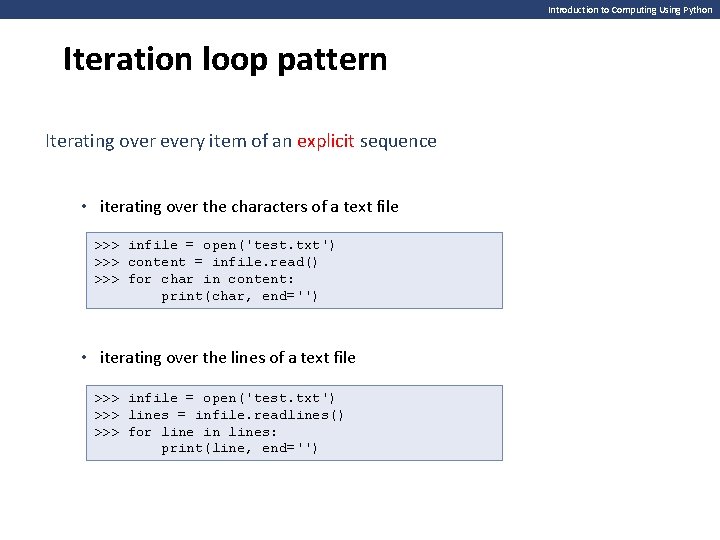
Introduction to Computing Using Python Iteration loop pattern Iterating over every item of an explicit sequence • iterating over the characters of a text file >>> infile = open('test. txt') >>> content = infile. read() >>> for char in content: print(char, end='') • iterating over the lines of a text file >>> infile = open('test. txt') >>> lines = infile. readlines() >>> for line in lines: print(line, end='')
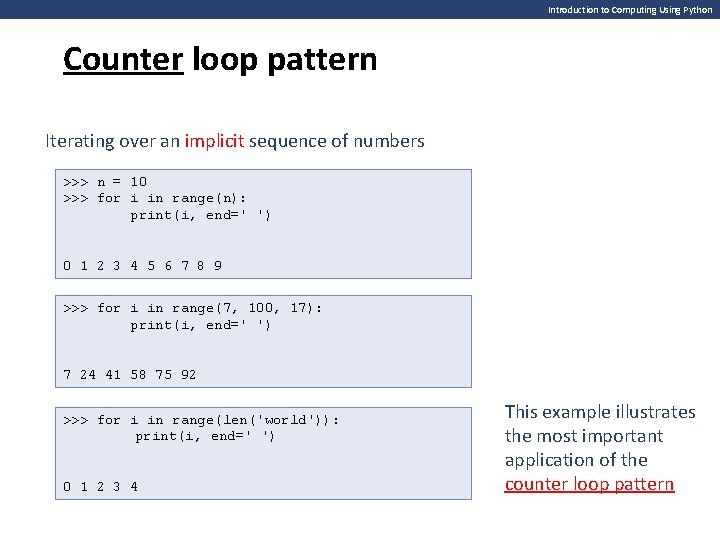
Introduction to Computing Using Python Counter loop pattern Iterating over an implicit sequence of numbers >>> n = 10 >>> for i in range(n): print(i, end=' ') 0 1 2 3 4 5 6 7 8 9 >>> for i in range(7, 100, 17): print(i, end=' ') 7 24 41 58 75 92 >>> for i in range(len('world')): print(i, end=' ') 0 1 2 3 4 This example illustrates the most important application of the counter loop pattern
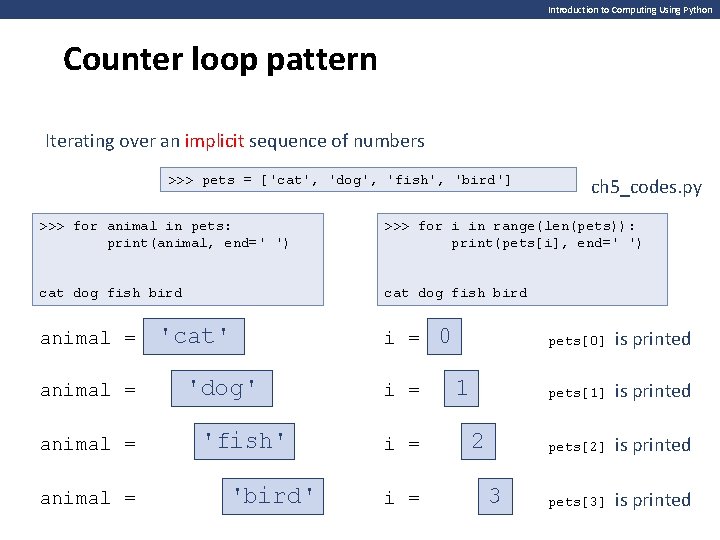
Introduction to Computing Using Python Counter loop pattern Iterating over an implicit sequence of numbers >>> pets = ['cat', 'dog', 'fish', 'bird'] ch 5_codes. py >>> for animal in pets: print(animal, end=' ') >>> for i in range(len(pets)): print(pets[i], end=' ') cat dog fish bird animal = 'cat' 'dog' 'fish' 'bird' i = i = 0 1 2 3 pets[0] is printed pets[1] is printed pets[2] is printed pets[3] is printed
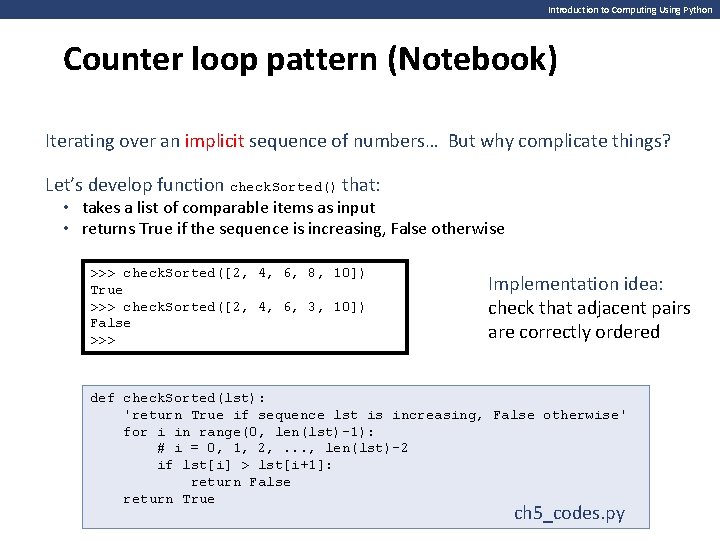
Introduction to Computing Using Python Counter loop pattern (Notebook) Iterating over an implicit sequence of numbers… But why complicate things? Let’s develop function check. Sorted() that: • takes a list of comparable items as input • returns True if the sequence is increasing, False otherwise >>> check. Sorted([2, 4, 6, 8, 10]) True >>> check. Sorted([2, 4, 6, 3, 10]) False >>> Implementation idea: check that adjacent pairs are correctly ordered def check. Sorted(lst): 'return True if sequence lst is increasing, False otherwise' for num in lst: for i in range(len(lst)-1): for i in range(0, len(lst)-1): for i in range(len(lst)): # compare num with next number in list # i = 0, 1, 2, . . . , len(lst)-2 # compare lst[i] with lst[i+1] if lst[i] > lst[i+1]: if lst[i] <= lst[i+1]: return False # correctly ordered, continue on return True else: # all adjacent pairs are correctly ordered, return true # incorrectly ordered, return false ch 5_codes. py
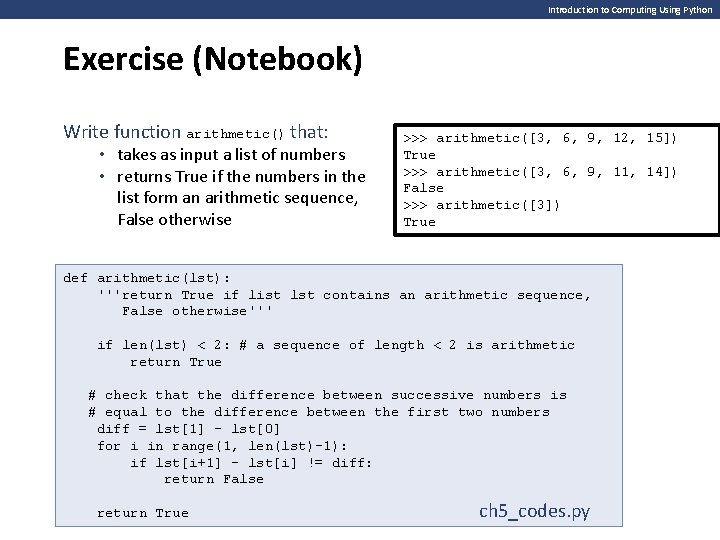
Introduction to Computing Using Python Exercise (Notebook) Write function arithmetic() that: • takes as input a list of numbers • returns True if the numbers in the list form an arithmetic sequence, False otherwise >>> arithmetic([3, 6, 9, 12, 15]) True >>> arithmetic([3, 6, 9, 11, 14]) False >>> arithmetic([3]) True def arithmetic(lst): '''return True if list lst contains an arithmetic sequence, if len(lst) < 2: False otherwise''' return True if len(lst) < 2: # a sequence of length < 2 is arithmetic diff = lst[1] - lst[0] return True for i in range(1, len(lst)-1): if lst[i+1] - lst[i] != diff: # check that the difference between successive numbers is return False # equal to the difference between the first two numbers diff = lst[1] - lst[0] return True for i in range(1, len(lst)-1): if lst[i+1] - lst[i] != diff: return False return True ch 5_codes. py
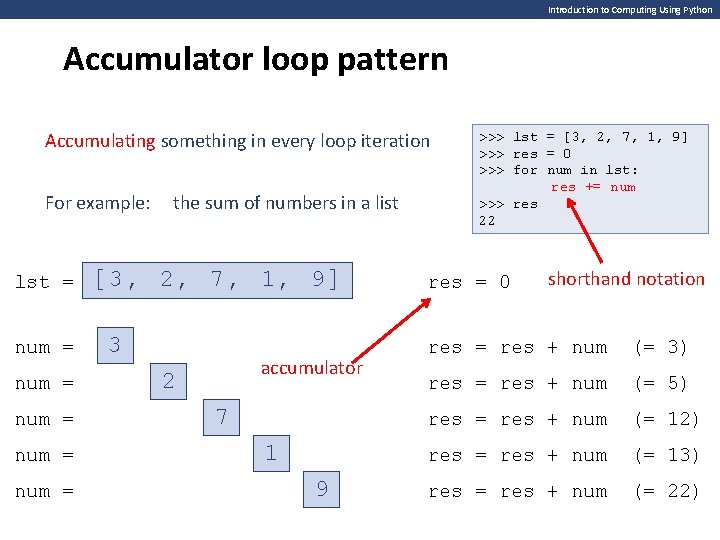
Introduction to Computing Using Python Accumulator loop pattern Accumulating something in every loop iteration For example: lst = num = num = the sum of numbers in a list [ 3 , 2 , 7 , 1 , 9] 3 accumulator 2 7 >>> lst = [3, 2, 7, 1, 9] >>> res = 0 >>> for num in lst: res = res + num res += num >>> res 22 res = 0 shorthand notation res = res + num (= 3) res = res + num (= 5) res = res + num (= 12) 1 res = res + num (= 13) 9 res = res + num (= 22)
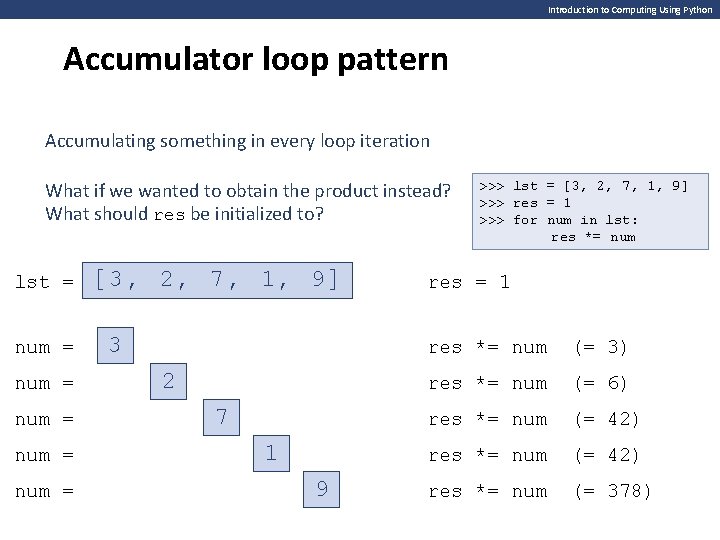
Introduction to Computing Using Python Accumulator loop pattern Accumulating something in every loop iteration What if we wanted to obtain the product instead? What should res be initialized to? lst = num = num = [ 3 , 2 , 7 , 1 , 9] 3 >>> lst = [3, 2, 7, 1, 9] >>> res = 1 >>> for num in lst: res *= num res = 1 res *= num (= 3) 2 res *= num (= 6) 7 res *= num (= 42) 1 res *= num (= 42) 9 res *= num (= 378)
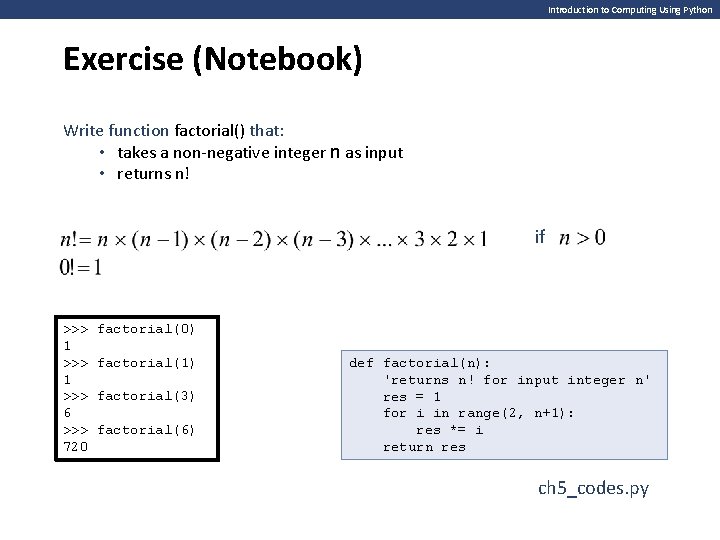
Introduction to Computing Using Python Exercise (Notebook) Write function factorial() that: • takes a non-negative integer n as input • returns n! if >>> factorial(0) 1 >>> factorial(1) 1 >>> factorial(3) 6 >>> factorial(6) 720 def factorial(n): 'returns n! for input integer n' res = 1 for i in range(2, n+1): res *= i return res ch 5_codes. py
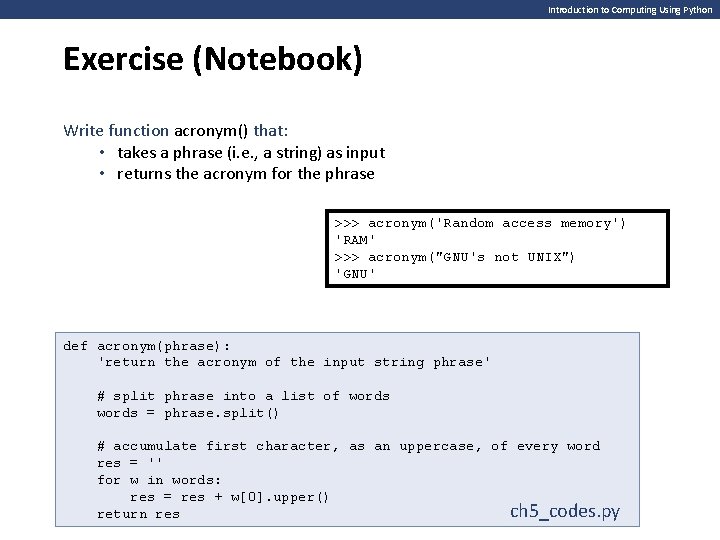
Introduction to Computing Using Python Exercise (Notebook) Write function acronym() that: • takes a phrase (i. e. , a string) as input • returns the acronym for the phrase >>> acronym('Random access memory') 'RAM' >>> acronym("GNU's not UNIX") 'GNU' def acronym(phrase): 'return the acronym of the input string phrase' # split phrase into a list of words = phrase. split() # accumulate first character, as an uppercase, of every word res = '' for w in words: res = res + w[0]. upper() ch 5_codes. py return res
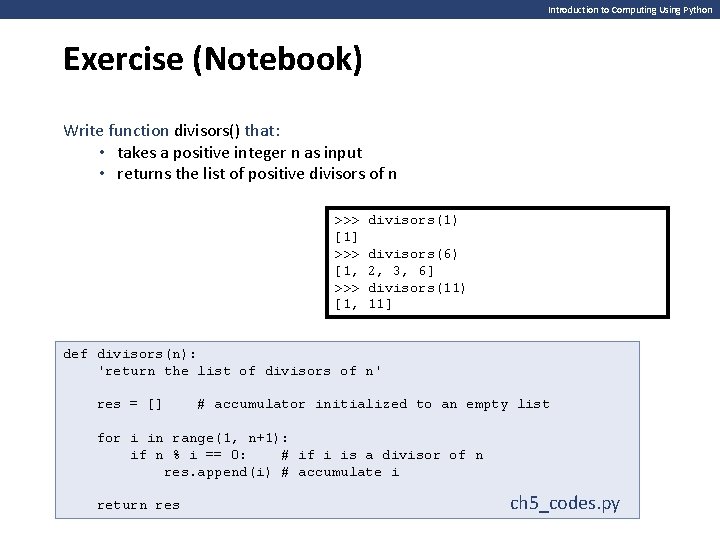
Introduction to Computing Using Python Exercise (Notebook) Write function divisors() that: • takes a positive integer n as input • returns the list of positive divisors of n >>> divisors(1) [1] >>> divisors(6) [1, 2, 3, 6] >>> divisors(11) [1, 11] def divisors(n): 'return the list of divisors of n' res = [] # accumulator initialized to an empty list for i in range(1, n+1): if n % i == 0: # if i is a divisor of n res. append(i) # accumulate i return res ch 5_codes. py
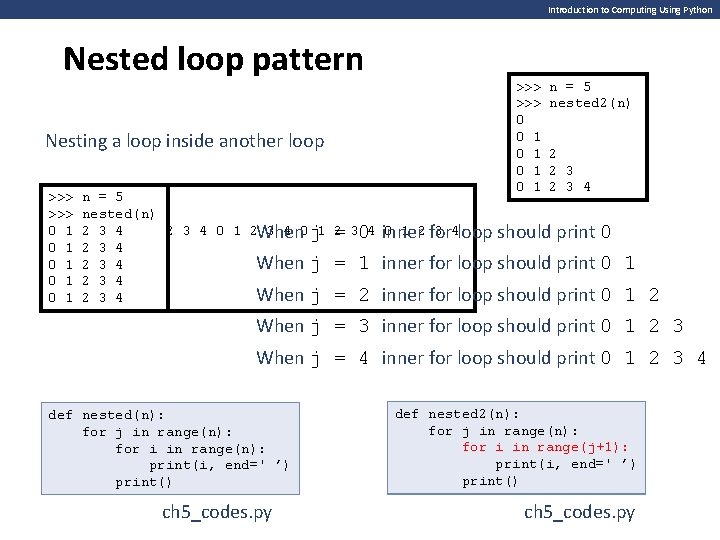
Introduction to Computing Using Python Nested loop pattern Nesting a loop inside another loop >>> n = 5 >>> nested(n) 0 1 2 3 4 0 1 2 3 4 When j = 0 inner for loop >>> 0 1 2 3 4 When j = 1 inner for loop 0 1 2 3 4 When j = 2 inner for loop 0 1 2 3 4 >>> n = 5 >>> nested 2(n) 0 0 1 2 3 4 should print 0 1 2 When j = 3 inner for loop should print 0 1 2 3 When j = 4 inner for loop should print 0 1 2 3 4 def nested(n): for i in range(n): for j in range(n): print(i, end=' ') for i in range(n): print(i, end=' ') print(i, end=' ’) print() ch 5_codes. py def nested 2(n): for j in range(n): for i in range(j+1): for i in range(n): print(i, end=' ’) print() ch 5_codes. py
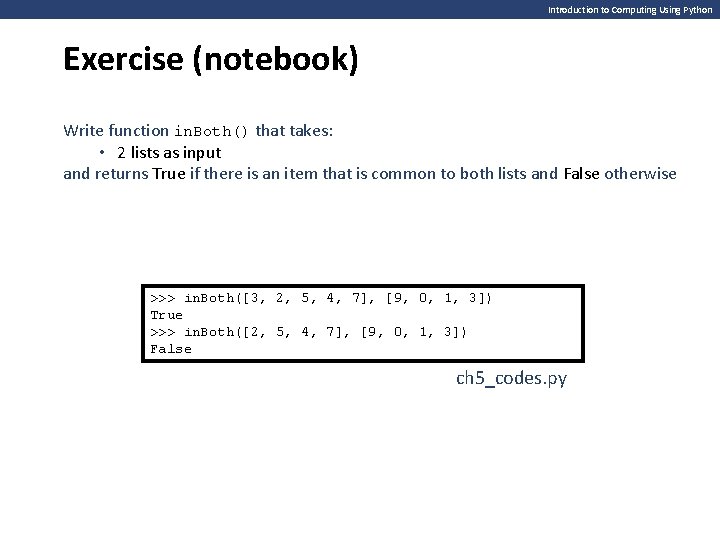
Introduction to Computing Using Python Exercise (notebook) Write function in. Both() that takes: • 2 lists as input and returns True if there is an item that is common to both lists and False otherwise >>> in. Both([3, 2, 5, 4, 7], [9, 0, 1, 3]) True >>> in. Both([2, 5, 4, 7], [9, 0, 1, 3]) False ch 5_codes. py
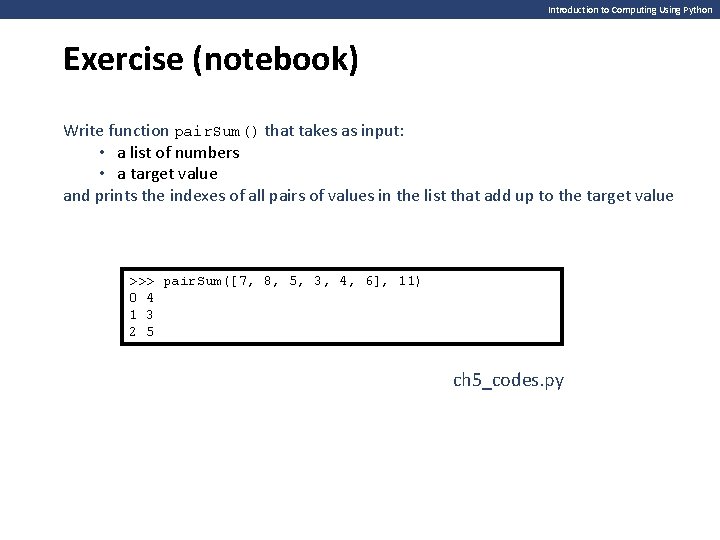
Introduction to Computing Using Python Exercise (notebook) Write function pair. Sum() that takes as input: • a list of numbers • a target value and prints the indexes of all pairs of values in the list that add up to the target value >>> pair. Sum([7, 8, 5, 3, 4, 6], 11) 0 4 1 3 2 5 ch 5_codes. py
![Introduction to Computing Using Python Twodimensional lists The list 3 5 7 9 can Introduction to Computing Using Python Two-dimensional lists The list [3, 5, 7, 9] can](https://slidetodoc.com/presentation_image_h/982bc1419ebf4c3572a638644c5eabe7/image-23.jpg)
Introduction to Computing Using Python Two-dimensional lists The list [3, 5, 7, 9] can be viewed as a 1 -D table [3, 5, 7, 9] = How to represent a 2 -D table? 3 5 7 9 0 1 2 3 [3, 5, 7, 9] = [ [3, 5, 7, 9] [0, 2, 1, 6] = [0, 2, 1, 6] 0 3 5 7 9 1 0 2 1 6 [3, 8, 3, 1] ] [3, 8, 3, 1] = 2 3 8 3 1 A 2 -D table is just a list of rows (i. e. , 1 -D tables) >>> lst = [[3, 5, 7, 9], [0, 2, 1, 6], [3, 8, 3, 1]] >>> lst >>> [[3, 5, 7, 9], [0, 2, 1, 6], [3, 8, 3, 1]] >>> lst[0] >>> [3, 5, 7, 9] >>> lst[1] >>> [0, 2, 1, 6] >>> lst[2] >>> [3, 8, 3, 1] >>> lst[0][0] >>> 3 >>> lst[1][2] >>> 1 >>> lst[2][0] 3 >>>
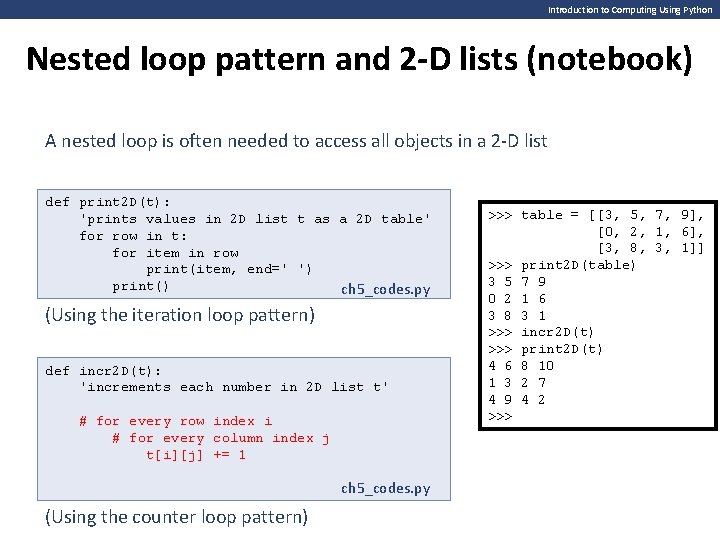
Introduction to Computing Using Python Nested loop pattern and 2 -D lists (notebook) A nested loop is often needed to access all objects in a 2 -D list def print 2 D(t): 'prints values in 2 D list t as a 2 D table' # for every row of t for row in t: # for every object in the row for item in row # print object print(item, end=' ') print() ch 5_codes. py (Using the iteration loop pattern) def incr 2 D(t): 'increments each number in 2 D list t' nrows = len(t) # nrows = number of rows in t # for every row index i ncols = len(t[0]) # ncols = number of columns in t # for every column index j t[i][j] += 1 for i in range(nrows): for j in range(ncols): t[i][j] += 1 ch 5_codes. py (Using the counter loop pattern) >>> table = [[3, 5, 7, 9], [0, 2, 1, 6], [3, 8, 3, 1]] >>> print 2 D(table) 3 5 7 9 0 2 1 6 3 8 3 1 >>> incr 2 D(t) >>> print 2 D(t) 4 6 8 10 1 3 2 7 4 9 4 2 >>>
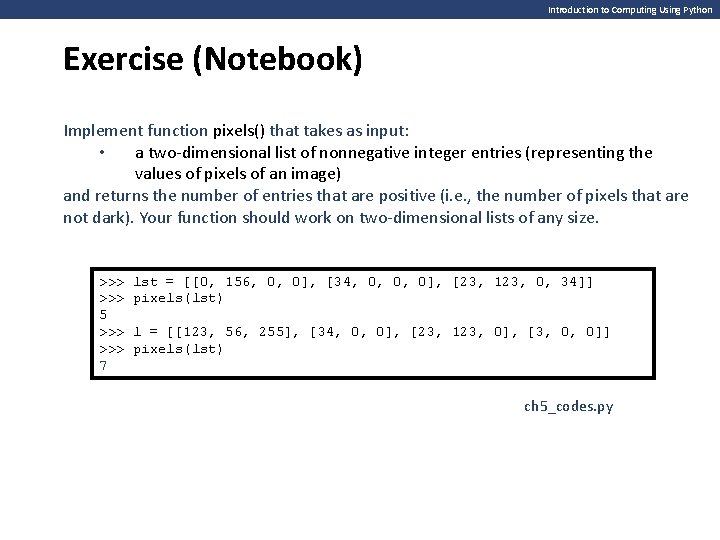
Introduction to Computing Using Python Exercise (Notebook) Implement function pixels() that takes as input: • a two-dimensional list of nonnegative integer entries (representing the values of pixels of an image) and returns the number of entries that are positive (i. e. , the number of pixels that are not dark). Your function should work on two-dimensional lists of any size. >>> lst = [[0, 156, 0, 0], [34, 0, 0, 0], [23, 123, 0, 34]] >>> pixels(lst) 5 >>> l = [[123, 56, 255], [34, 0, 0], [23, 123, 0], [3, 0, 0]] >>> pixels(lst) 7 ch 5_codes. py
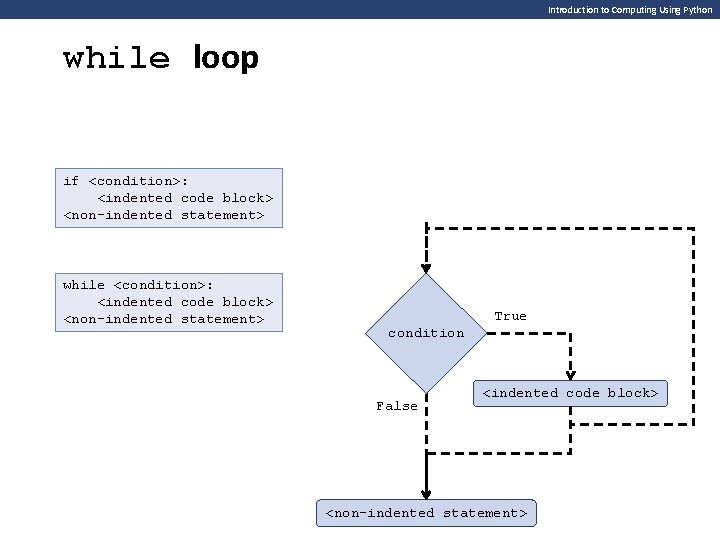
Introduction to Computing Using Python while loop if <condition>: <indented code block> <non-indented statement> while <condition>: <indented code block> <non-indented statement> True condition False <indented code block> <non-indented statement>
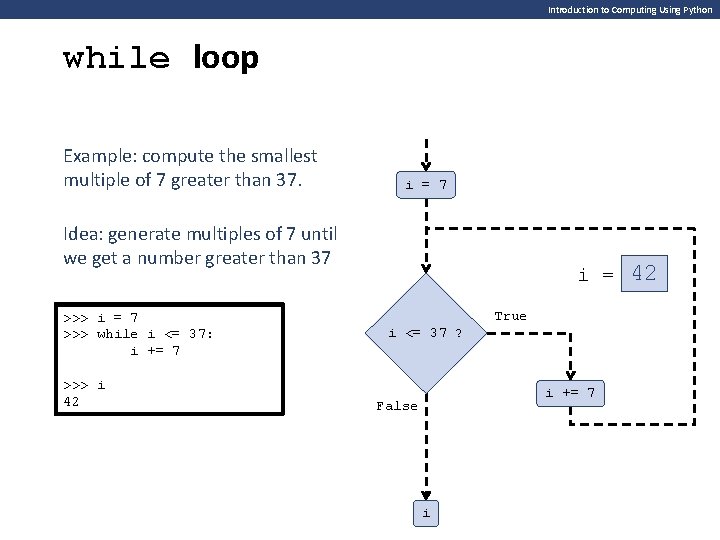
Introduction to Computing Using Python while loop Example: compute the smallest multiple of 7 greater than 37. i = 7 Idea: generate multiples of 7 until we get a number greater than 37 >>> i = 7 >>> while i <= 37: i += 7 >>> i 42 i = True i <= 37 ? i += 7 False i 21 14 35 28 42 7
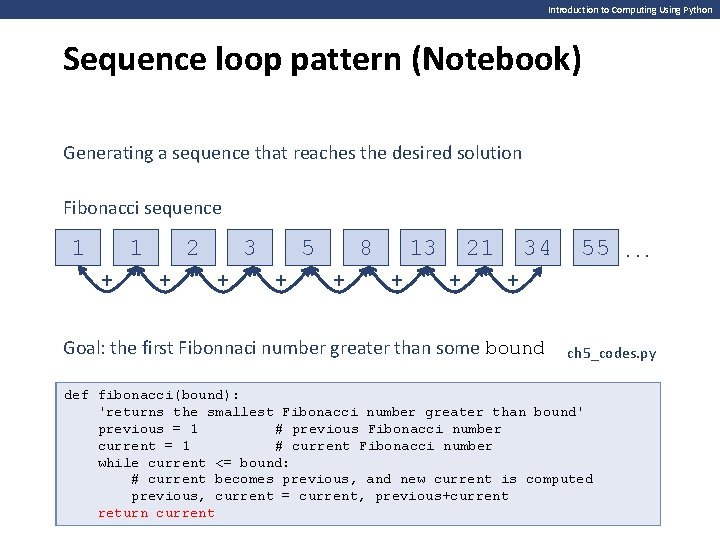
Introduction to Computing Using Python Sequence loop pattern (Notebook) Generating a sequence that reaches the desired solution Fibonacci sequence 1 1 + 2 + 3 + 5 + 8 + 13 + 21 + 34 55. . . + Goal: the first Fibonnaci number greater than some bound ch 5_codes. py def fibonacci(bound): 'returns the smallest Fibonacci number greater than bound' 'returns the smallest Fibonacci number greater than bound’ previous = 1 # previous Fibonacci number current = 1 # current Fibonacci number while current <= bound: # not done yet, make current be next Fibonacci number # current becomes previous, and new current is computed previous, current = current, previous+current return current
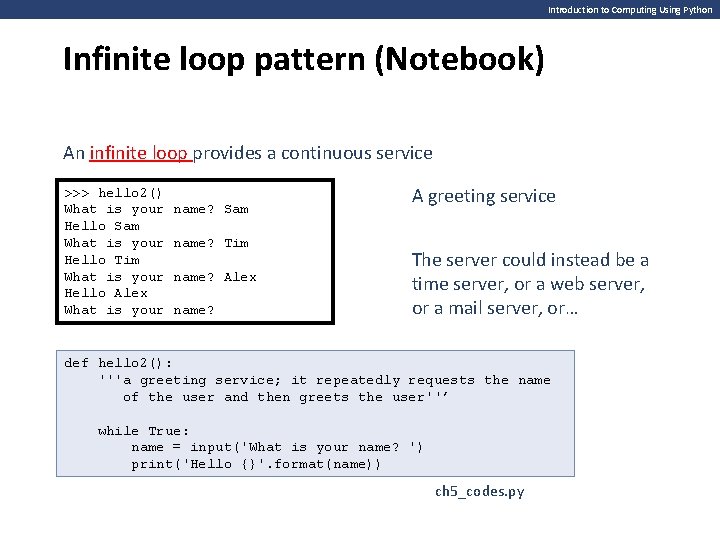
Introduction to Computing Using Python Infinite loop pattern (Notebook) An infinite loop provides a continuous service >>> hello 2() What is your name? Sam Hello Sam What is your name? Tim Hello Tim What is your name? Alex Hello Alex What is your name? A greeting service The server could instead be a time server, or a web server, or a mail server, or… def hello 2(): '''a greeting service; it repeatedly requests the name of the user and then greets the user''’ while True: name = input('What is your name? ') print('Hello {}'. format(name)) ch 5_codes. py
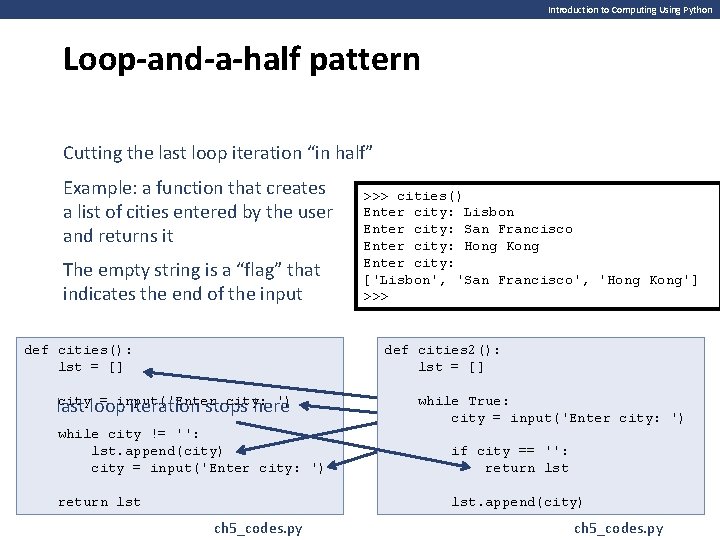
Introduction to Computing Using Python Loop-and-a-half pattern Cutting the last loop iteration “in half” Example: a function that creates a list of cities entered by the user and returns it The empty string is a “flag” that indicates the end of the input def cities(): lst = [] city = input('Enter city: ') last loop iteration stops here >>> cities() Enter city: Lisbon Enter city: San Francisco Enter city: Hong Kong Enter city: ['Lisbon', 'San Francisco', 'Hong Kong'] >>> def cities 2(): lst = [] while True: # repeat: accumulator awkward andpattern not quite # ask user to enter city = input('Enter city: ') intuitive while city != '': lst. append(city) city = input('Enter city: ') if city == '': # if user entered flag # then return lst # append city to lst lst. append(city) ch 5_codes. py
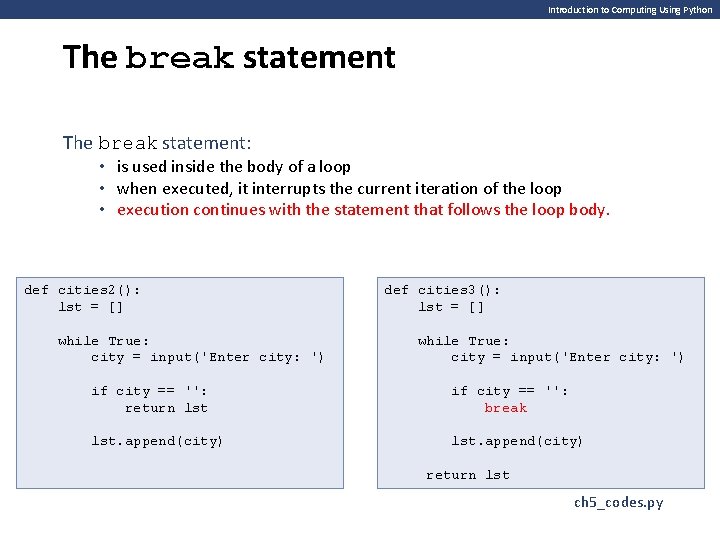
Introduction to Computing Using Python The break statement: • is used inside the body of a loop • when executed, it interrupts the current iteration of the loop • execution continues with the statement that follows the loop body. def cities 2(): lst = [] def cities 3(): lst = [] while True: city = input('Enter city: ') if city == '': return lst if city == '': break lst. append(city) return lst ch 5_codes. py
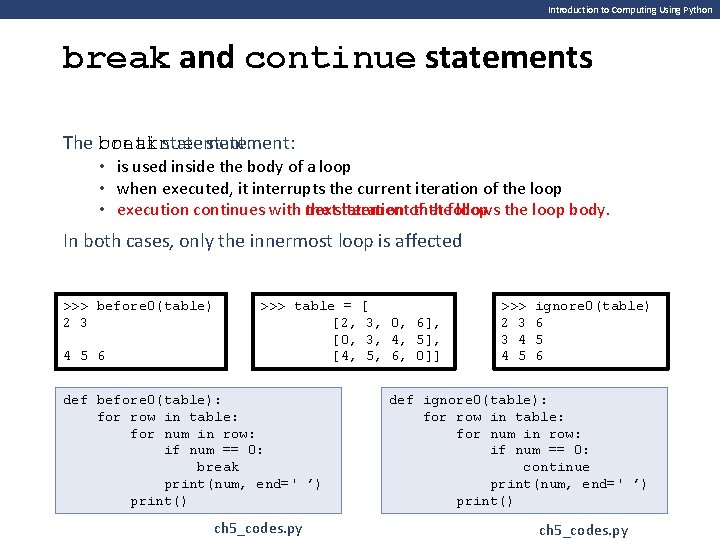
Introduction to Computing Using Python break and continue statements The continue statement: break statement: • is used inside the body of a loop • when executed, it interrupts the current iteration of the loop • execution continues with next the statement iteration of that thefollows loop the loop body. In both cases, only the innermost loop is affected >>> before 0(table) 2 3 4 5 6 >>> table = [ [2, 3, 0, 6], [0, 3, 4, 5], [4, 5, 6, 0]] def before 0(table): for row in table: for num in row: if num == 0: break print(num, end=' ’) print() ch 5_codes. py >>> ignore 0(table) 2 3 6 3 4 5 6 def ignore 0(table): for row in table: for num in row: if num == 0: continue print(num, end=' ’) print() ch 5_codes. py
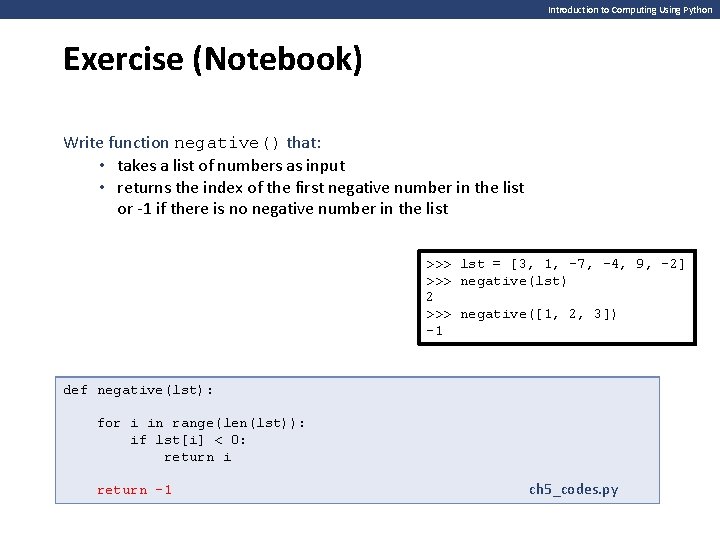
Introduction to Computing Using Python Exercise (Notebook) Write function negative() that: • takes a list of numbers as input • returns the index of the first negative number in the list or -1 if there is no negative number in the list >>> lst = [3, 1, -7, -4, 9, -2] >>> negative(lst) 2 >>> negative([1, 2, 3]) -1 def greater(lst): def negative(lst): # iterate through list lst and for i in range(len(lst)): # using counter loop pattern for i in range(len(lst)): # compare each number with 0 if lst[i] < 0: # number at index i is first return i # Which loop pattern shoud we use? # negative number, so return i # if for loop completes execution, lst contains no negative number ch 5_codes. py return -1
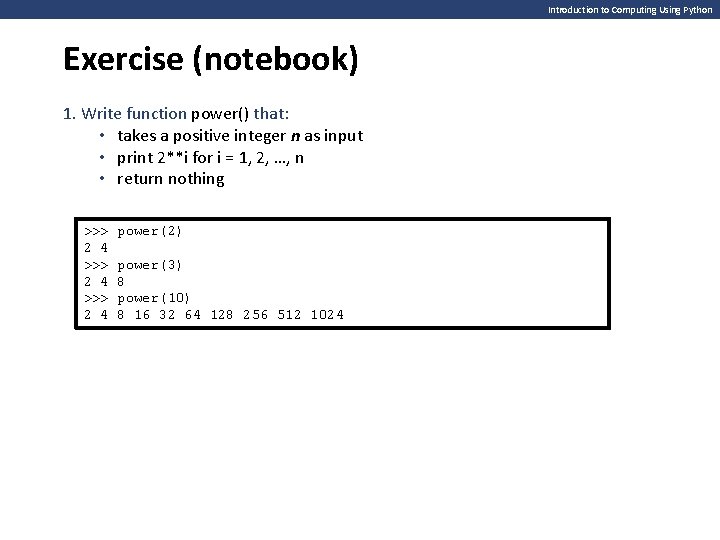
Introduction to Computing Using Python Exercise (notebook) 1. Write function power() that: • takes a positive integer n as input • print 2**i for i = 1, 2, …, n • return nothing >>> power(2) 2 4 >>> power(3) 2 4 8 >>> power(10) 2 4 8 16 32 64 128 256 512 1024
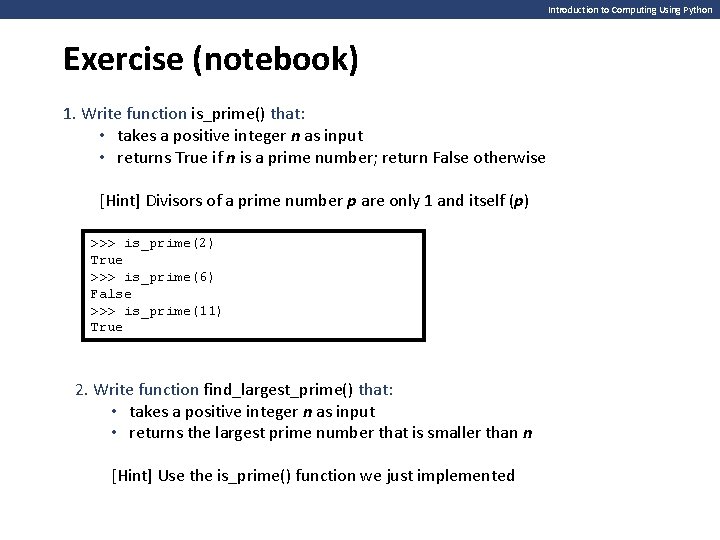
Introduction to Computing Using Python Exercise (notebook) 1. Write function is_prime() that: • takes a positive integer n as input • returns True if n is a prime number; return False otherwise [Hint] Divisors of a prime number p are only 1 and itself (p) >>> is_prime(2) True >>> is_prime(6) False >>> is_prime(11) True 2. Write function find_largest_prime() that: • takes a positive integer n as input • returns the largest prime number that is smaller than n [Hint] Use the is_prime() function we just implemented
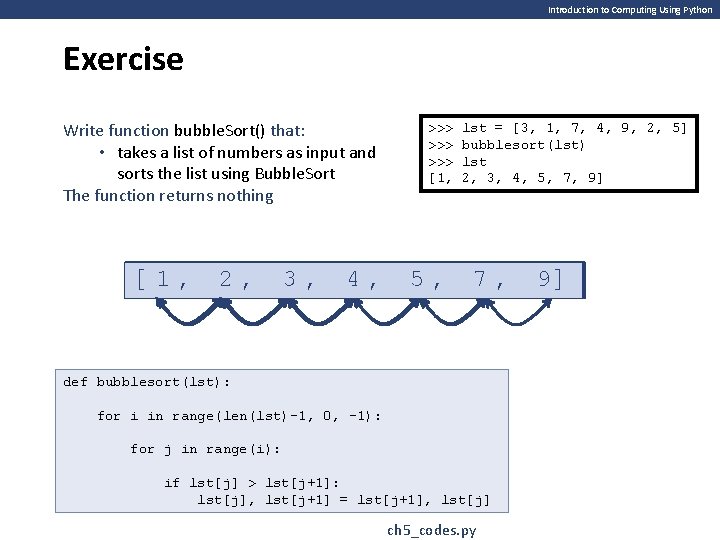
Introduction to Computing Using Python Exercise Write function bubble. Sort() that: • takes a list of numbers as input and sorts the list using Bubble. Sort The function returns nothing >>> lst = [3, 1, 7, 4, 9, 2, 5] >>> bubblesort(lst) >>> lst [1, 2, 3, 4, 5, 7, 9] 4 7 2 2 5 [[ 13 , , 231 , , 32 7 , , 4 4 , , 5 9 7 , , 7 2 , , 9 9 5] ] def bubblesort(lst): for i in range(len(lst)-1, 0, -1): # i = len(last)-1, len(lst)-2, …, 1 for j in range(i): if lst[j] > lst[j+1]: # number whose final position should be i # bubbles up to position i lst[j], lst[j+1] = lst[j+1], lst[j] ch 5_codes. py
Homologous structure
Tools and mechanisms for virtualization
Data structures for parallel computing
Conventional computing and intelligent computing
Decision structures and boolean logic
Decision structures in python
Advanced data structures in python
Fundamentals of python data structures
Numerical computing with python
Python ide for scientific computing
Stacks in data structures
Data structures using java
Data structures using java
Representation of polynomial using linked list
Python desktop automation
Sentiment analysis (with spark)
Eight puzzle problem in python
Polynomial linked list c
Hardware and control structures
Statement level control structures
Control structures in php
C++ control structures
Eof controlled while loop c++
Types of control structures
If else in 8086
Statement level control structures
Control structures in php
Iteration control structures
Which is not a repetition control structure in java?
Iteration control structures
Entity life history
Algorithm control structures
Control structure in visual basic
Media access control in mobile computing
Feedback control of computing systems
End user computing controls sox
Python comparators