http www csie nctu edu twtsaiwncpp 03generic STL
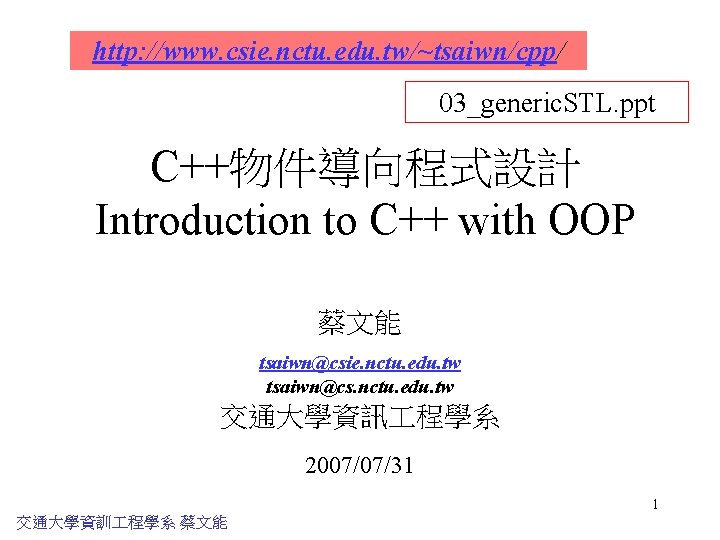
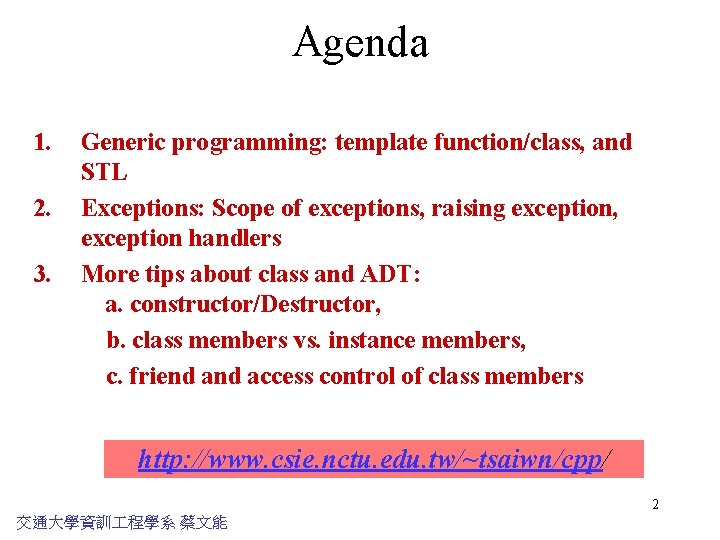
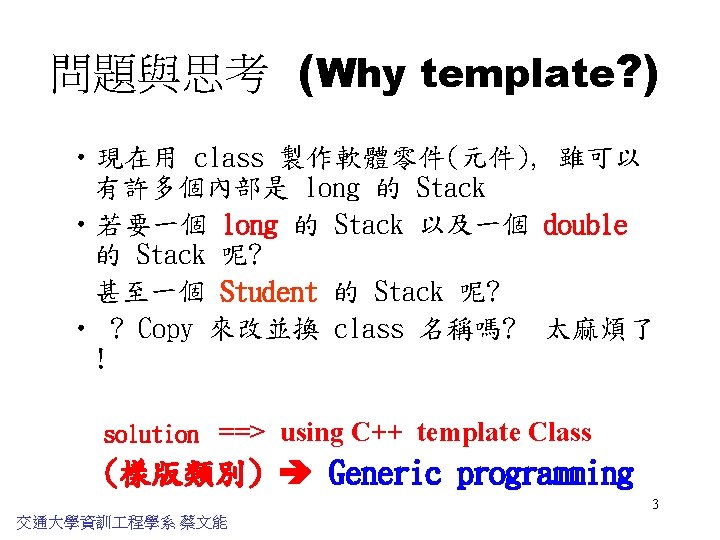
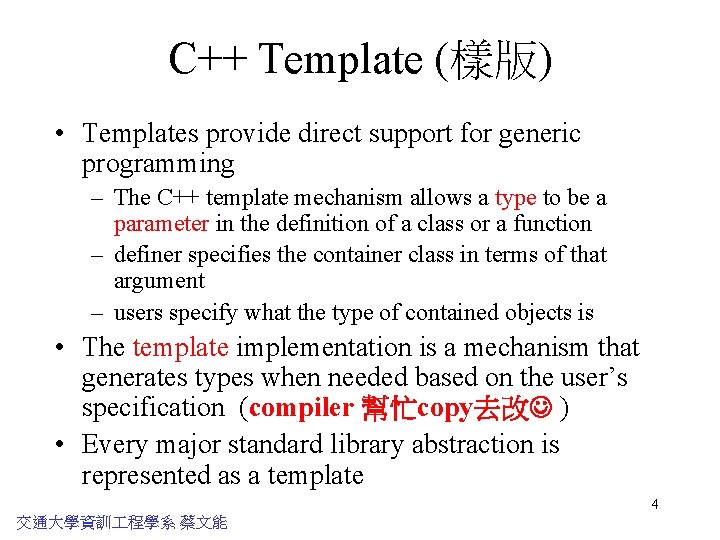
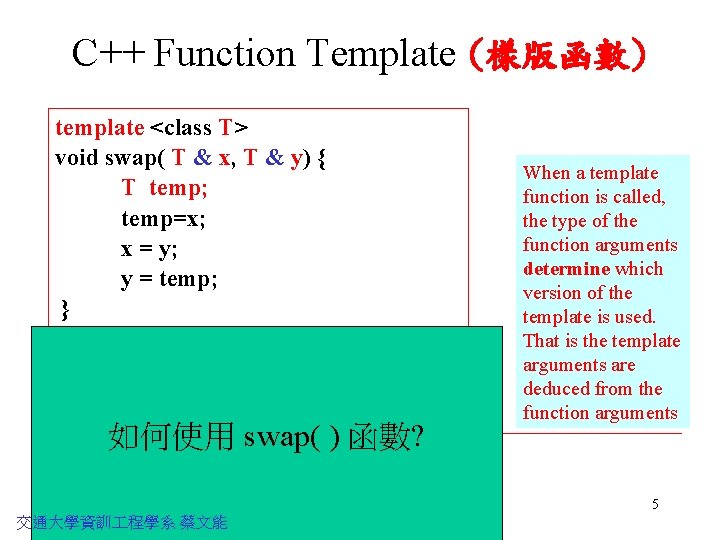
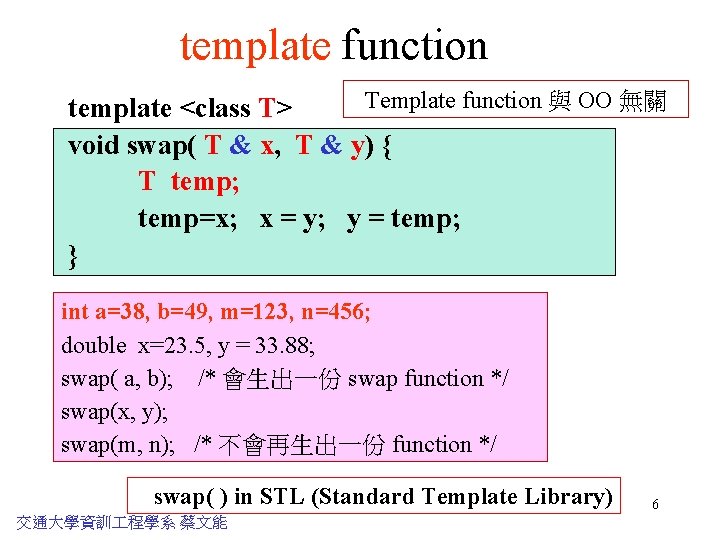
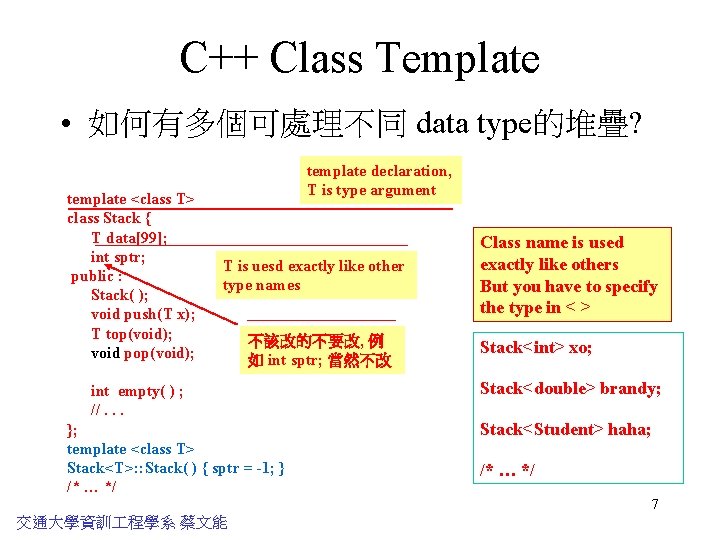
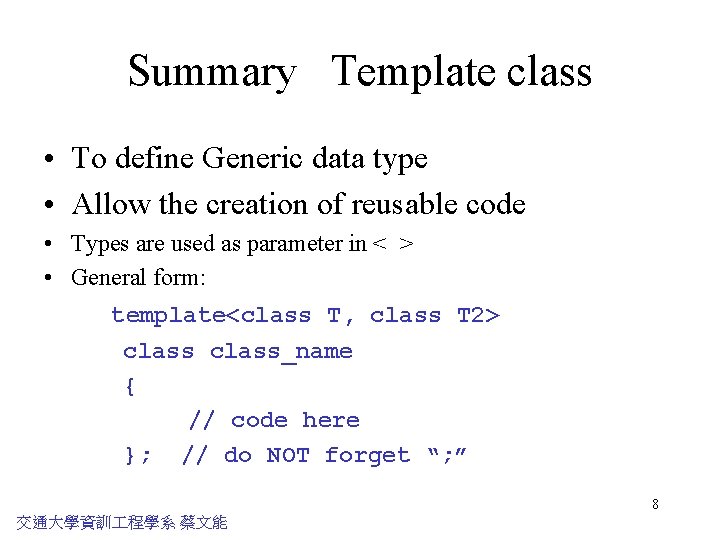
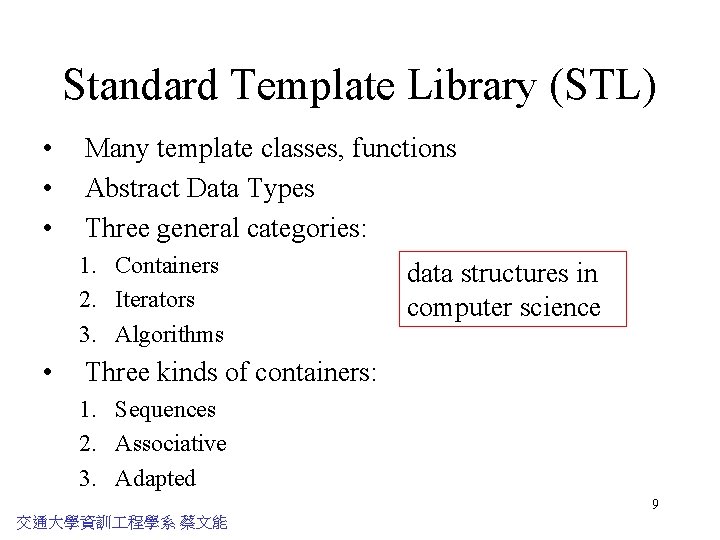
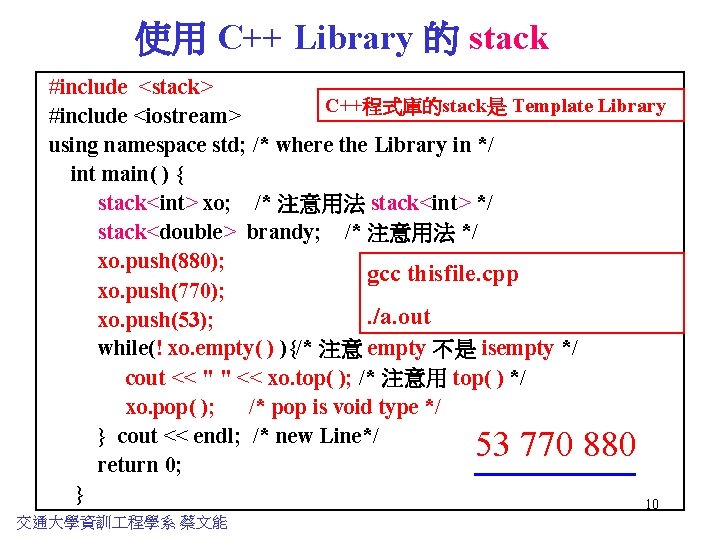
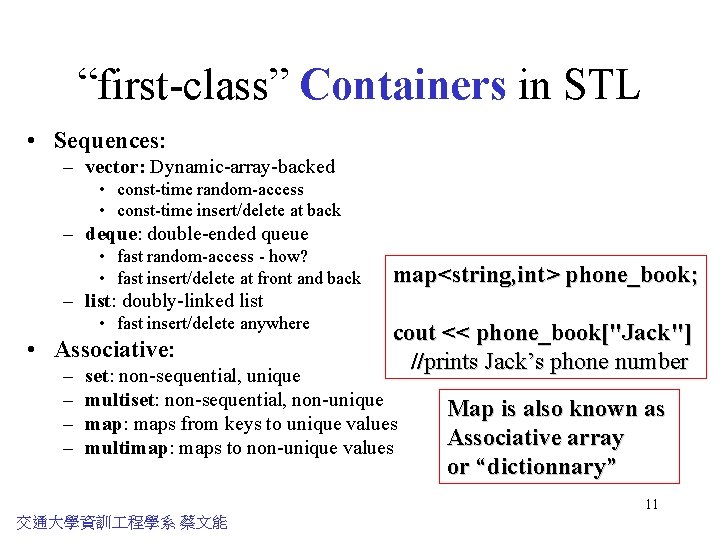
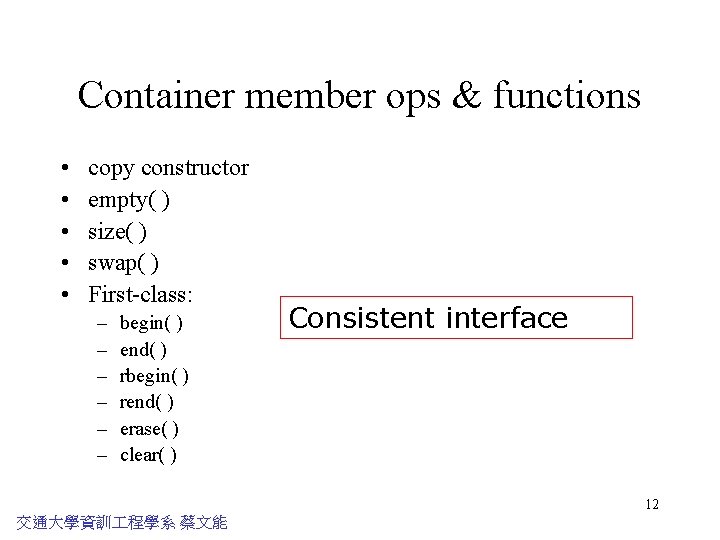
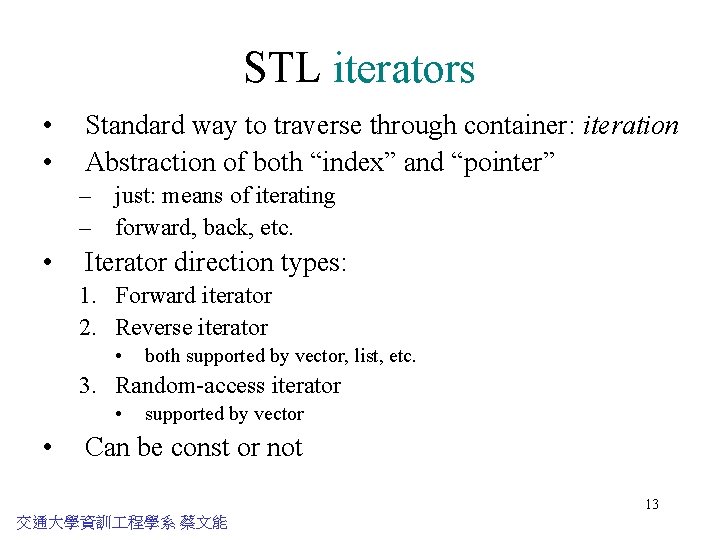
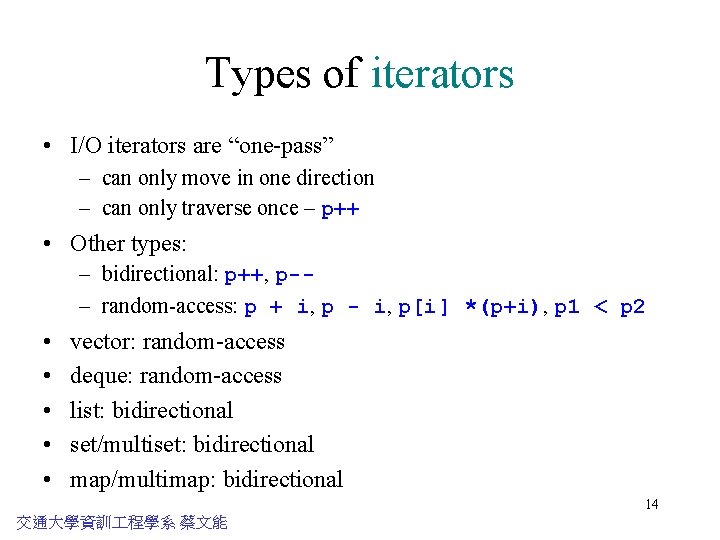
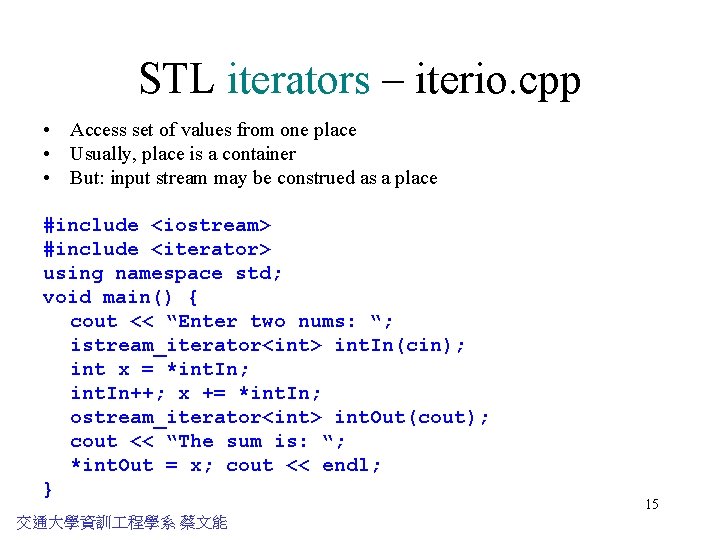
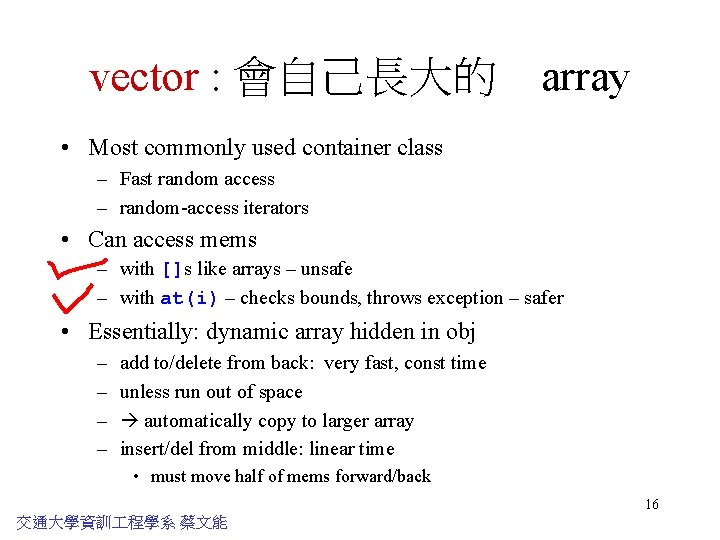
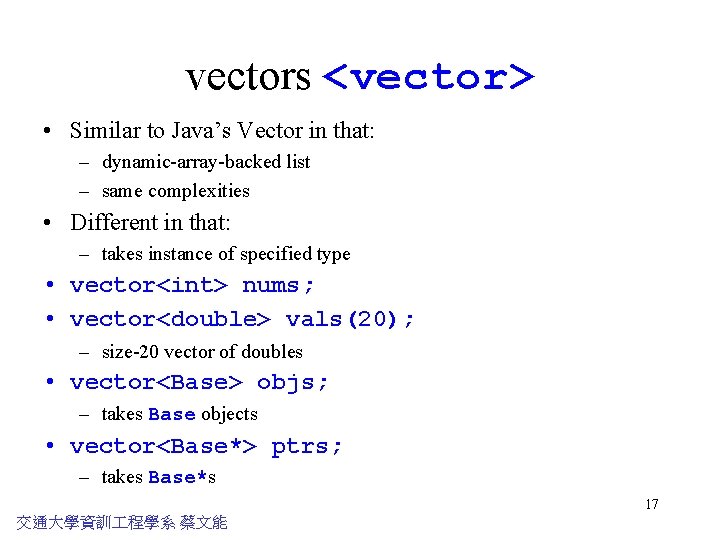
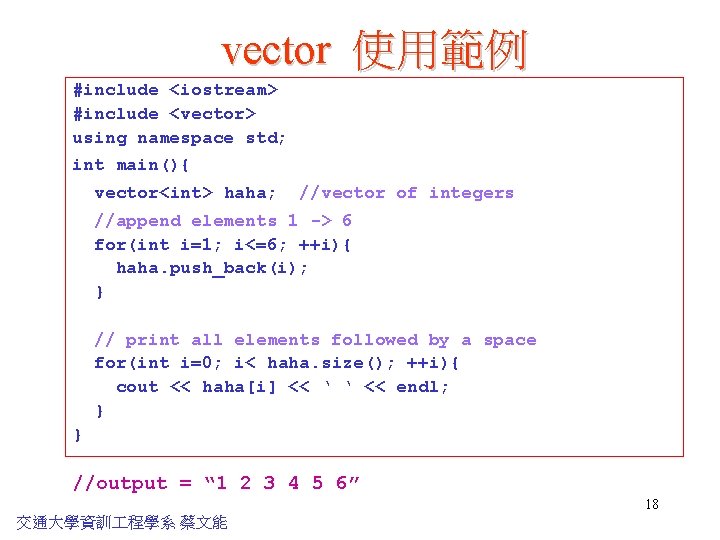
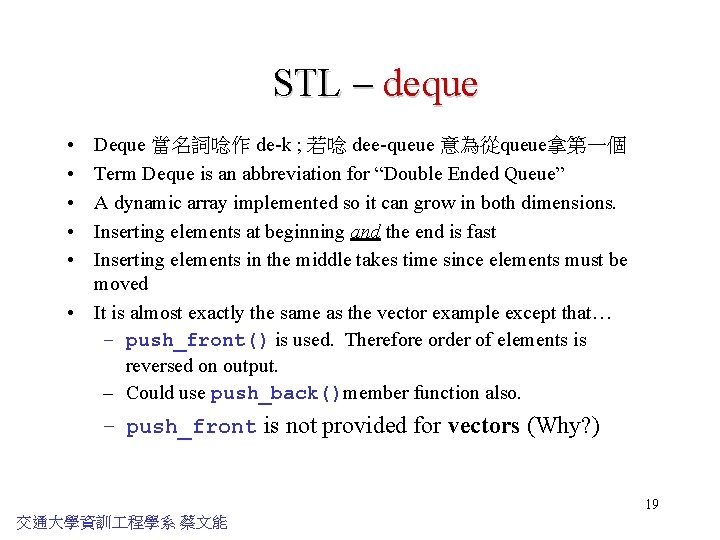
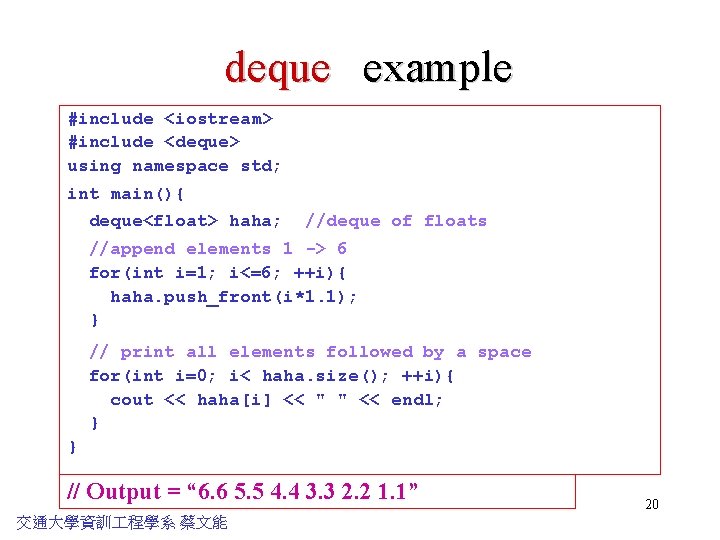
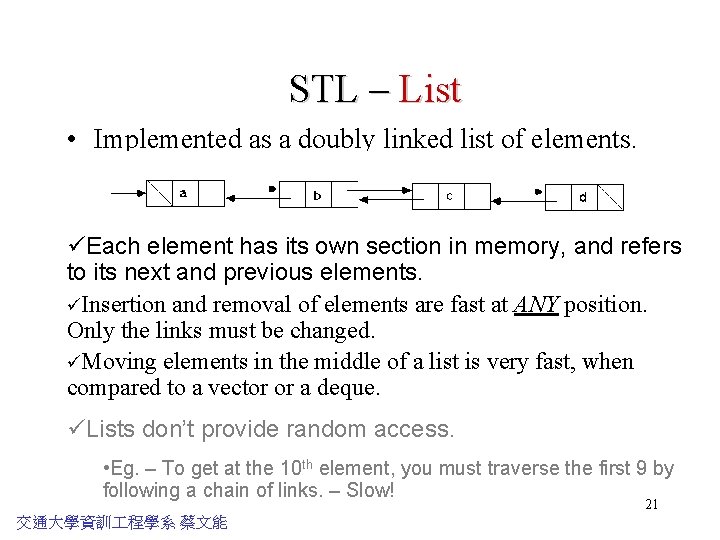
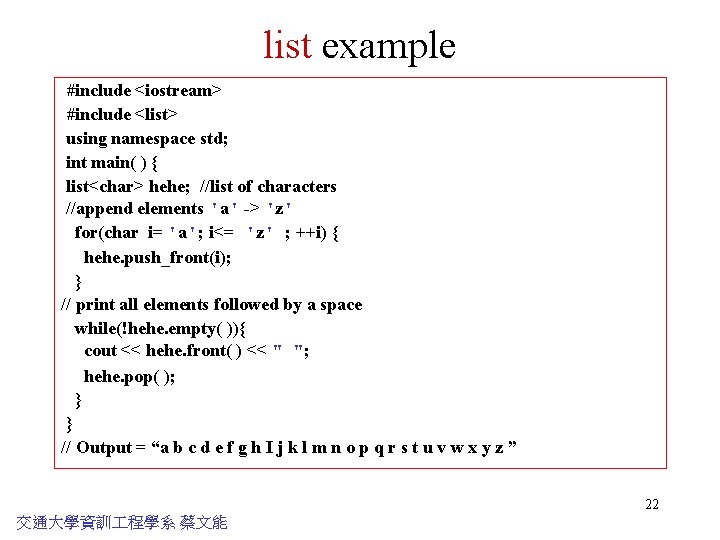
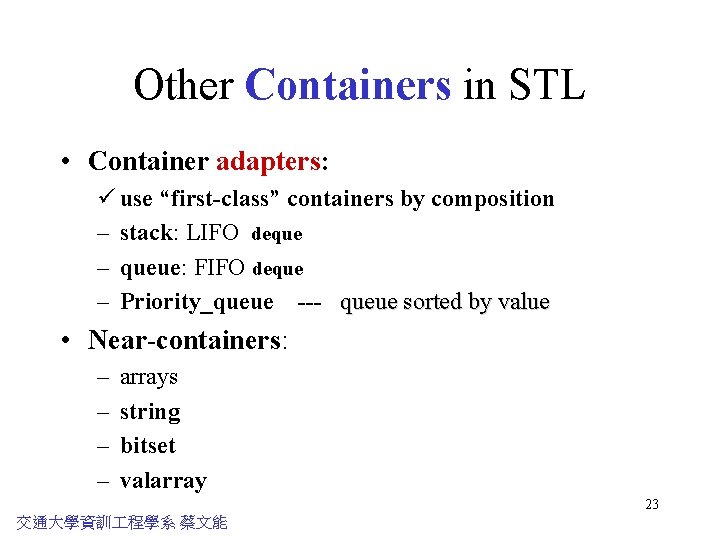
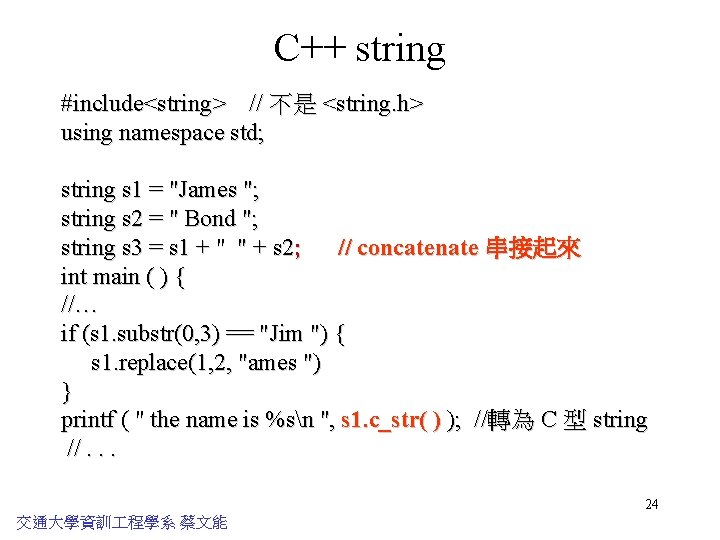
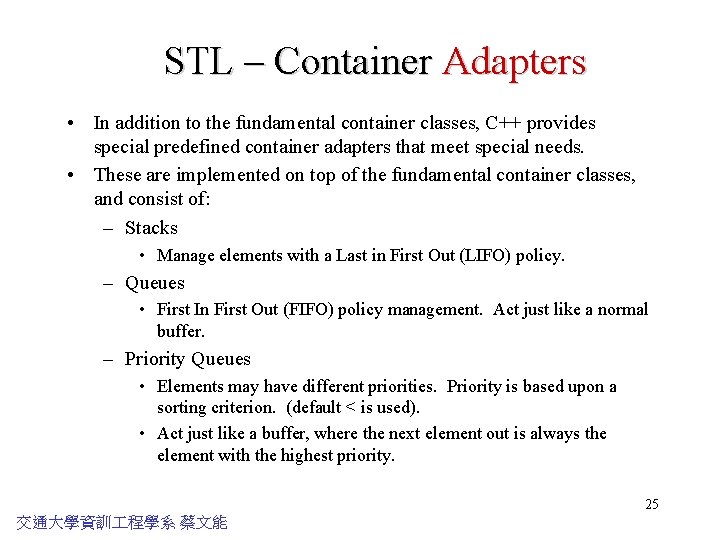
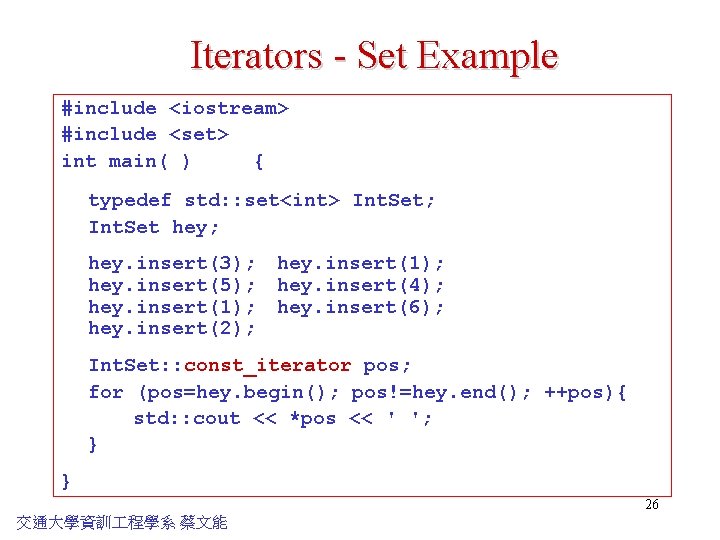
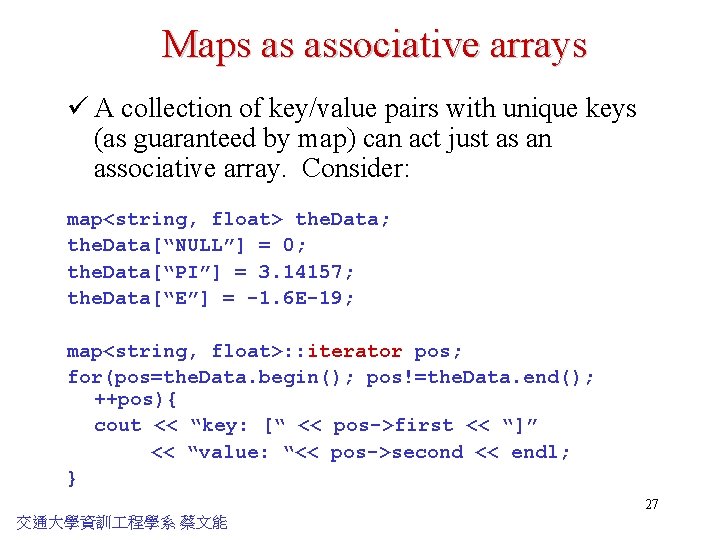
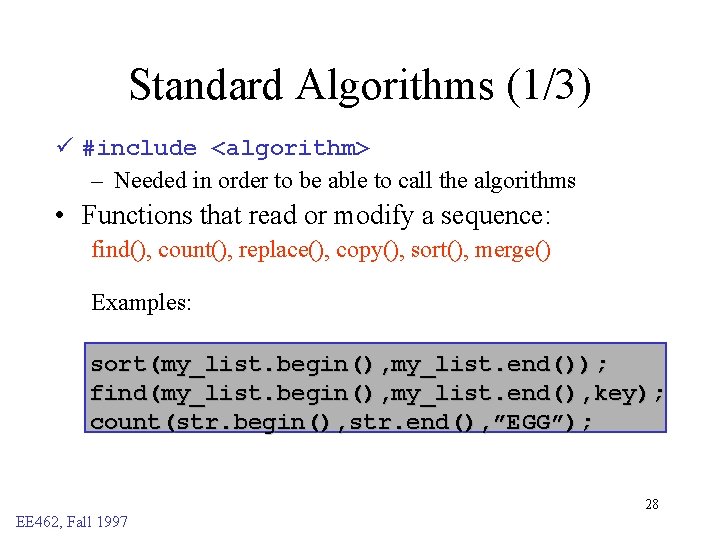
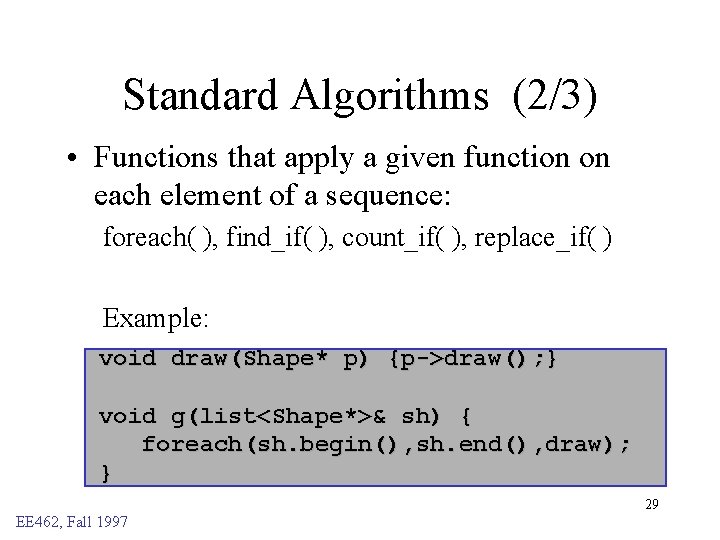
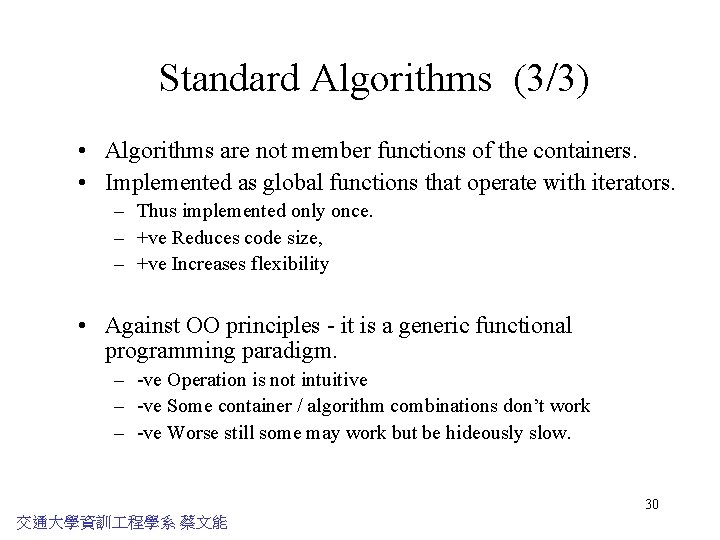
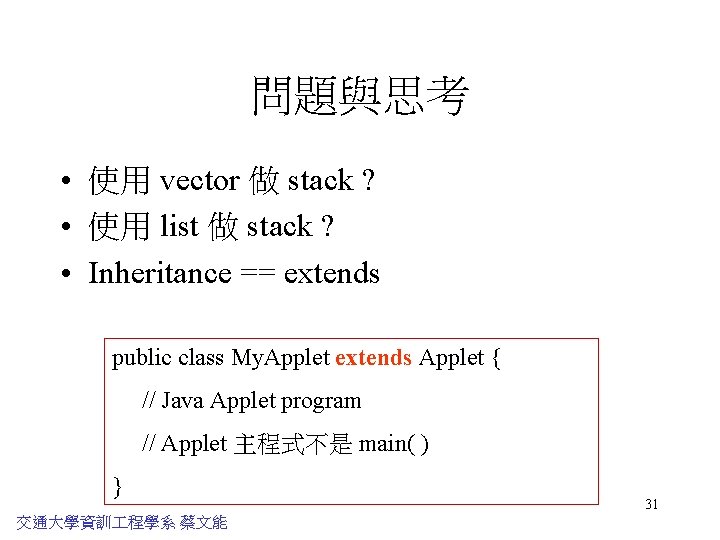
![用 vector 做出 stack (1/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mystk 3. h 用 vector 做出 stack (1/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mystk 3. h](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-32.jpg)
![用 vector 做出 stack (2/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mymain 3. cpp 用 vector 做出 stack (2/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mymain 3. cpp](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-33.jpg)
![用 list 做出 stack (1/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mystk 5. h 用 list 做出 stack (1/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mystk 5. h](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-34.jpg)
![用 list 做出 stack (2/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mymain 5. cpp 用 list 做出 stack (2/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mymain 5. cpp](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-35.jpg)
![把 list 擴充成 stack (1/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mystk 6. h 把 list 擴充成 stack (1/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mystk 6. h](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-36.jpg)
![把 list 擴充成 stack (2/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mymain 6. cpp 把 list 擴充成 stack (2/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mymain 6. cpp](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-37.jpg)
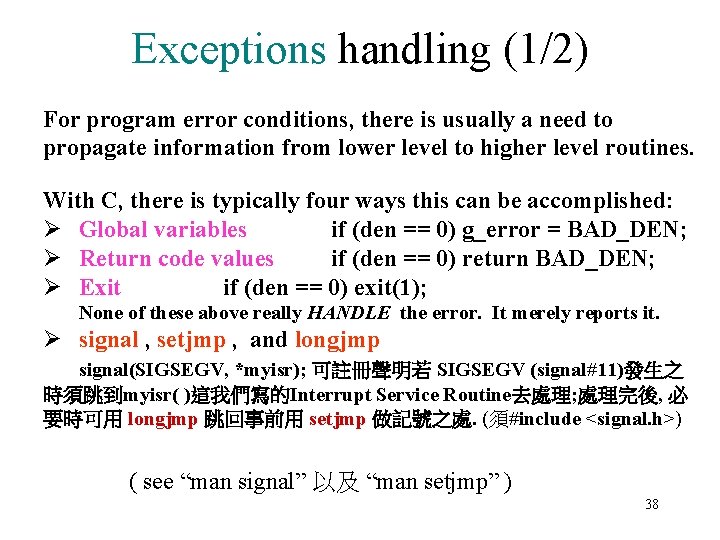
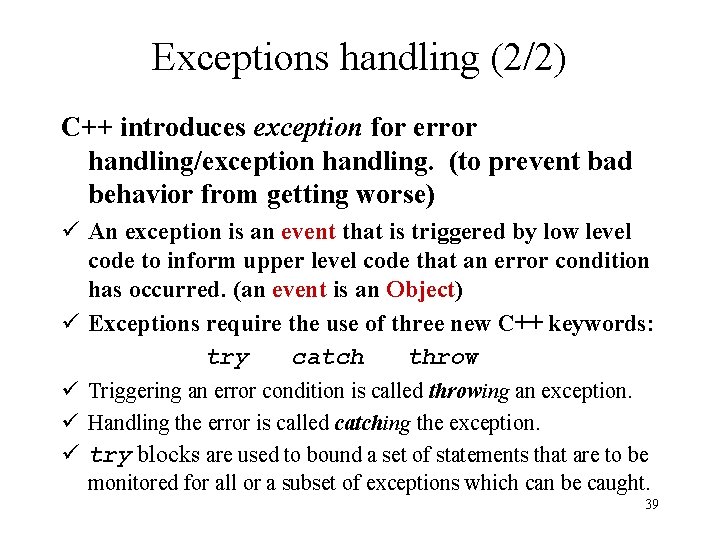
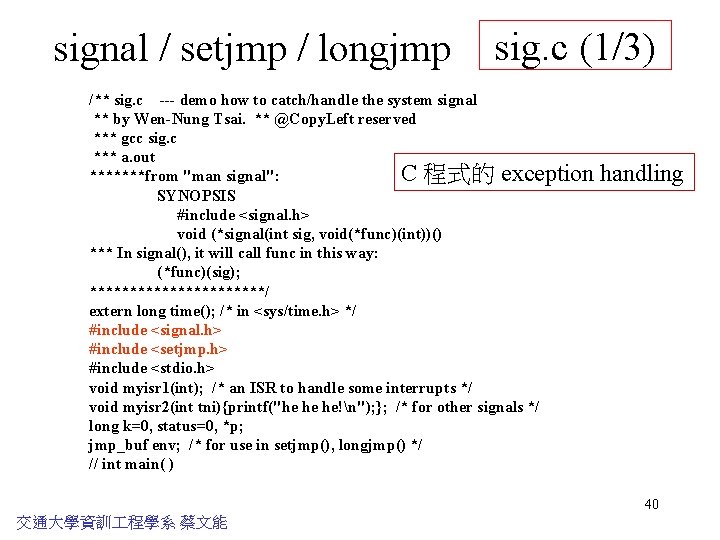
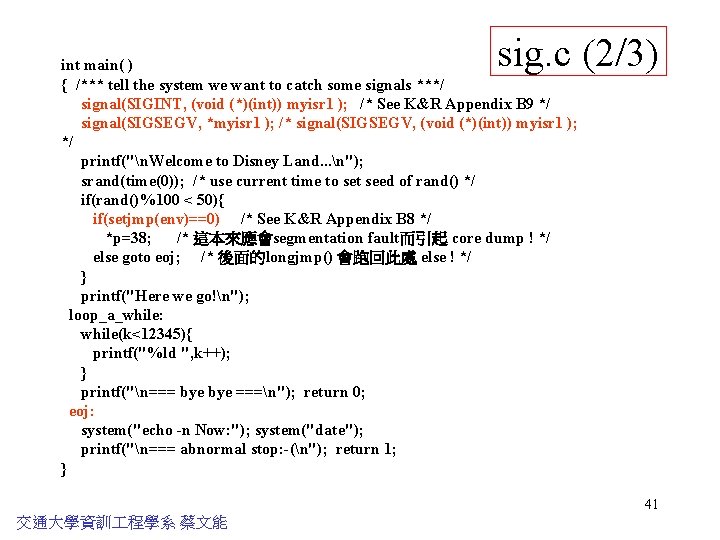
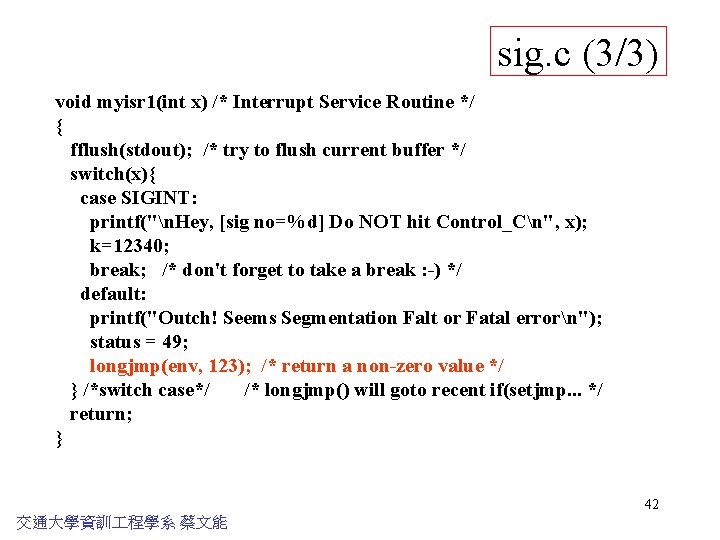
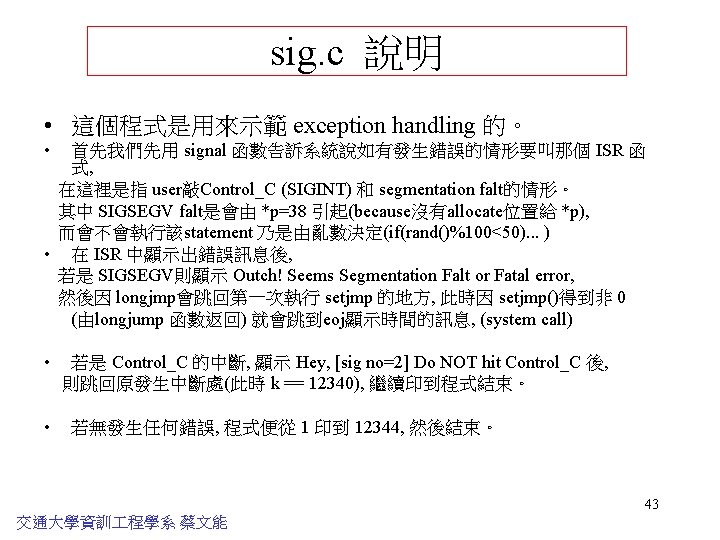
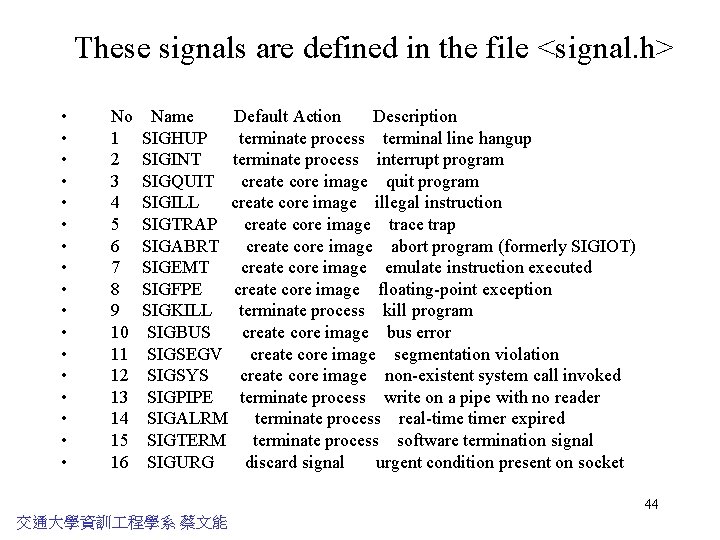
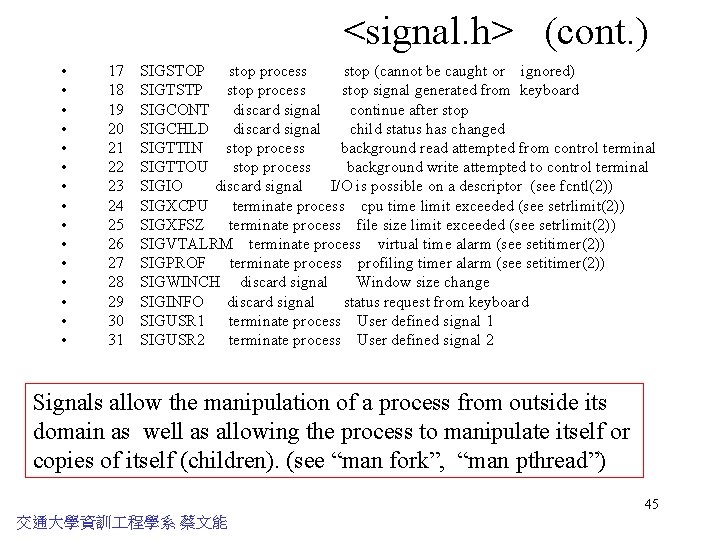
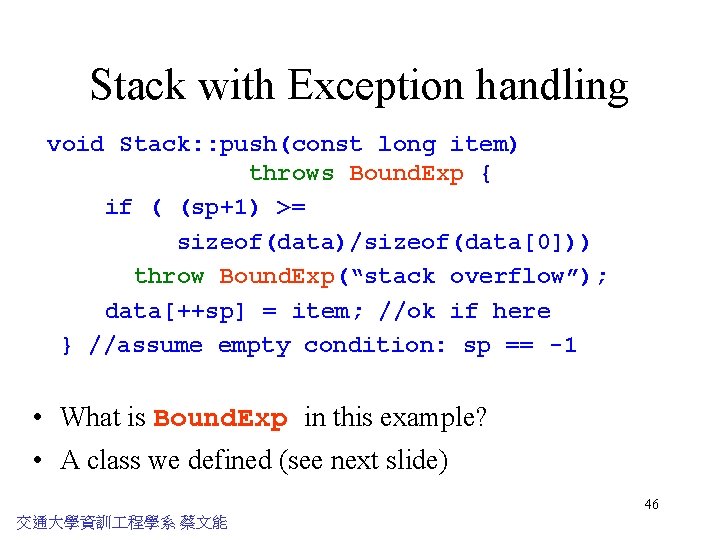
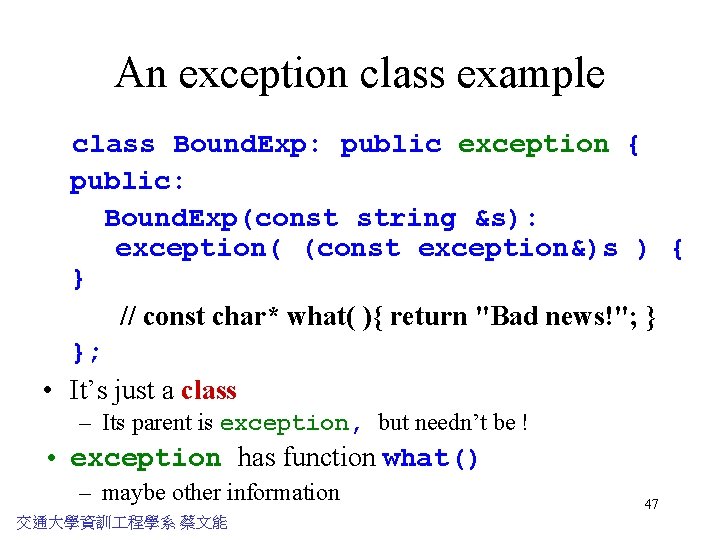
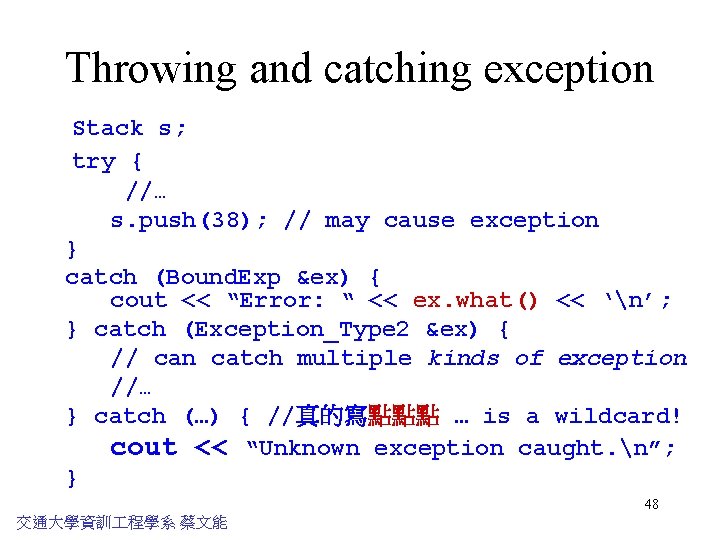
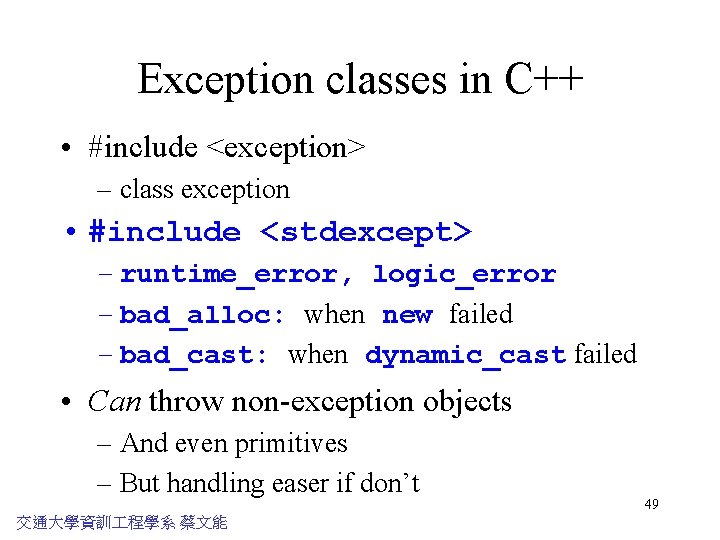
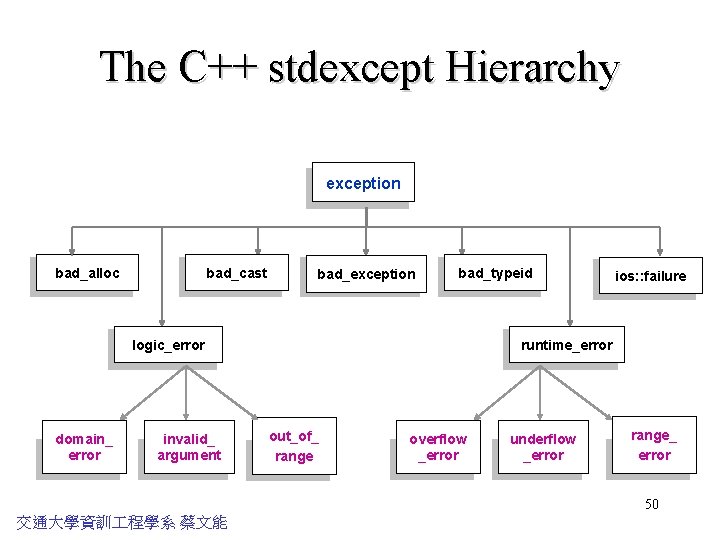
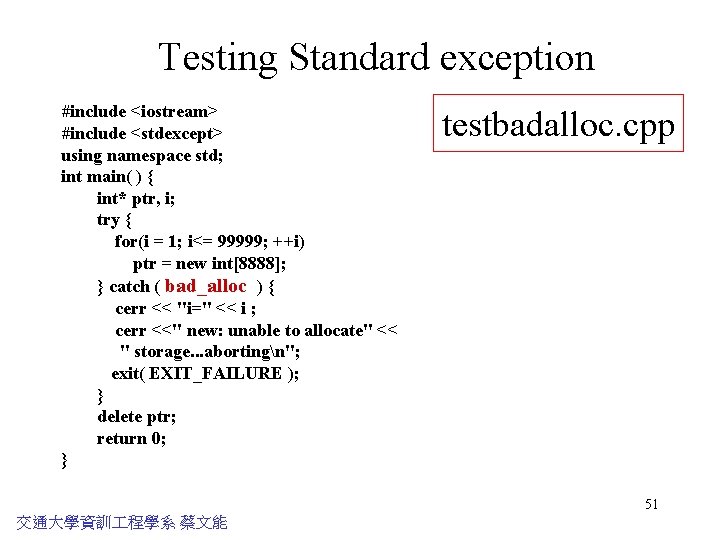
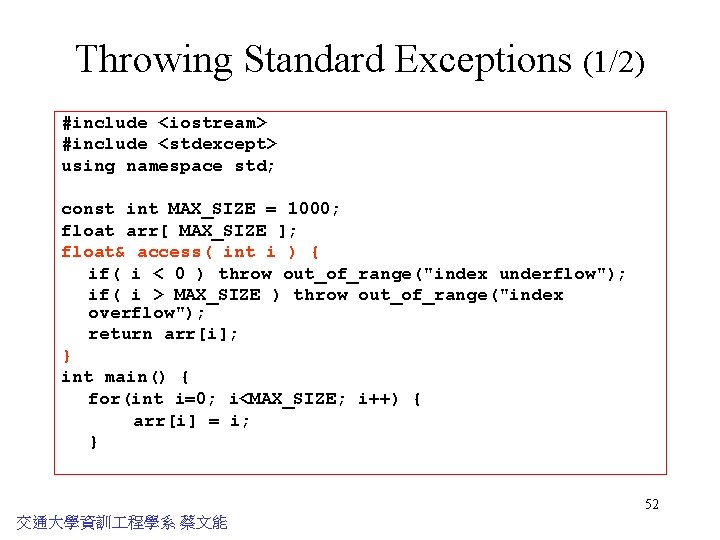
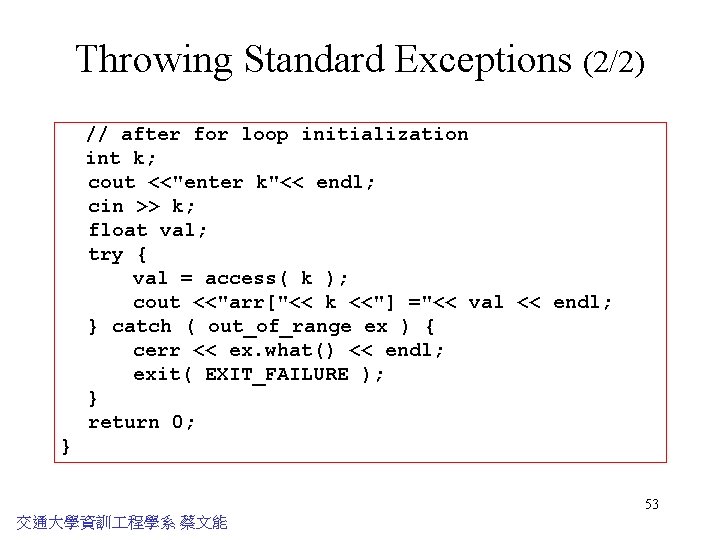
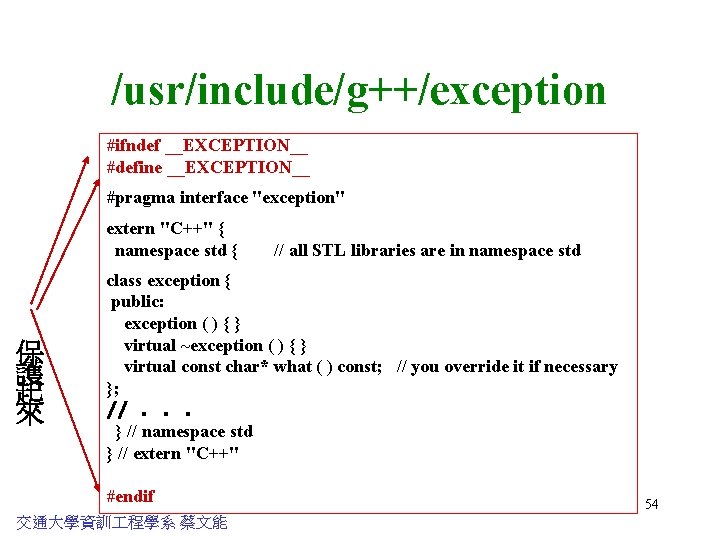
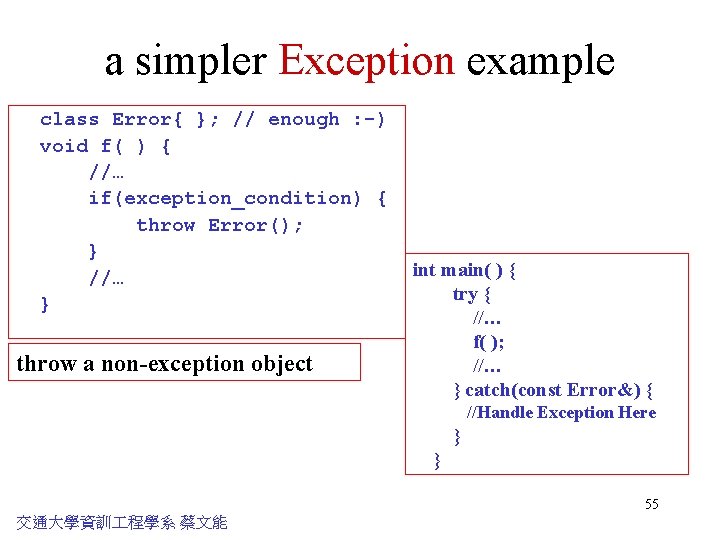
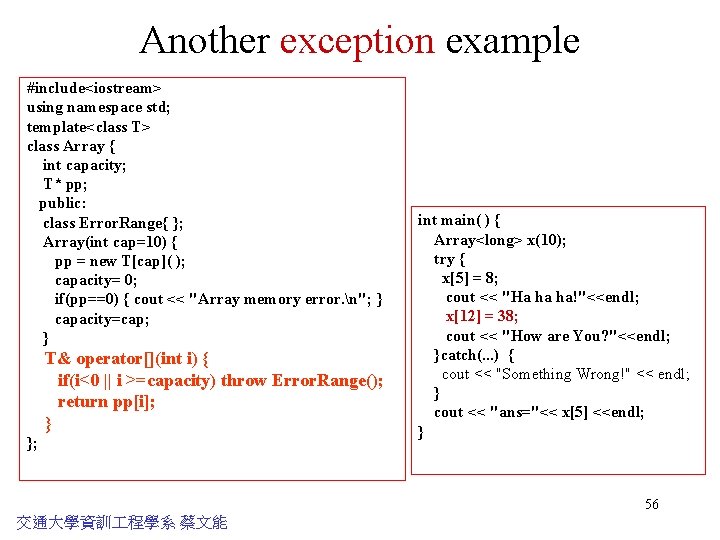
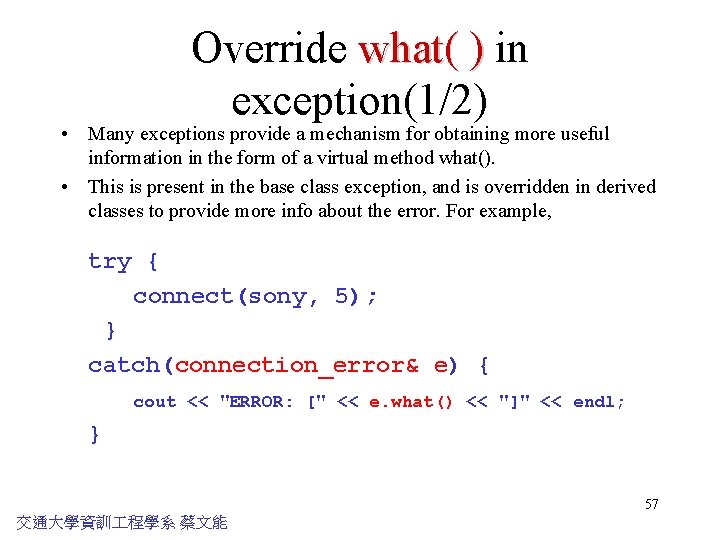
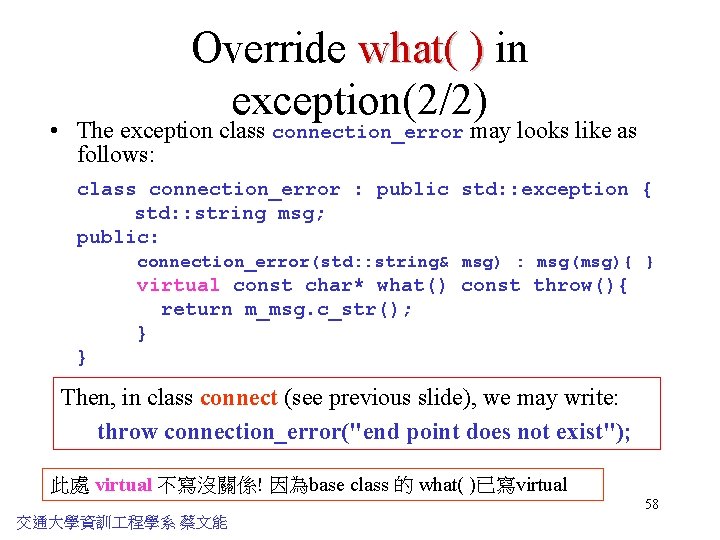
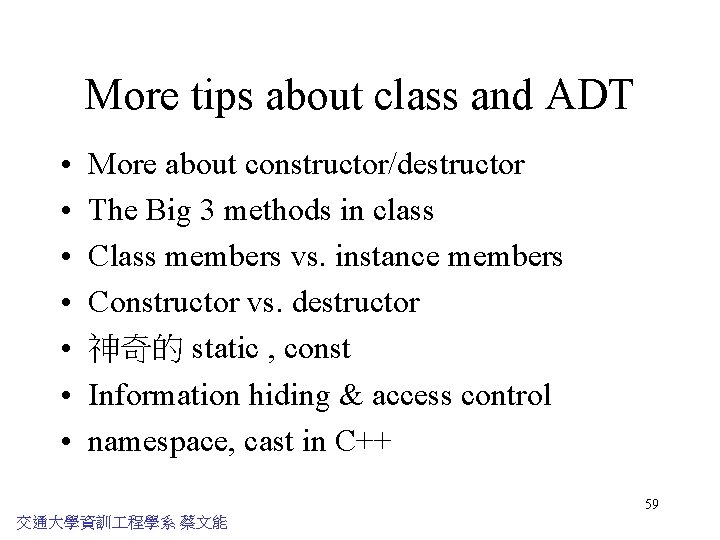
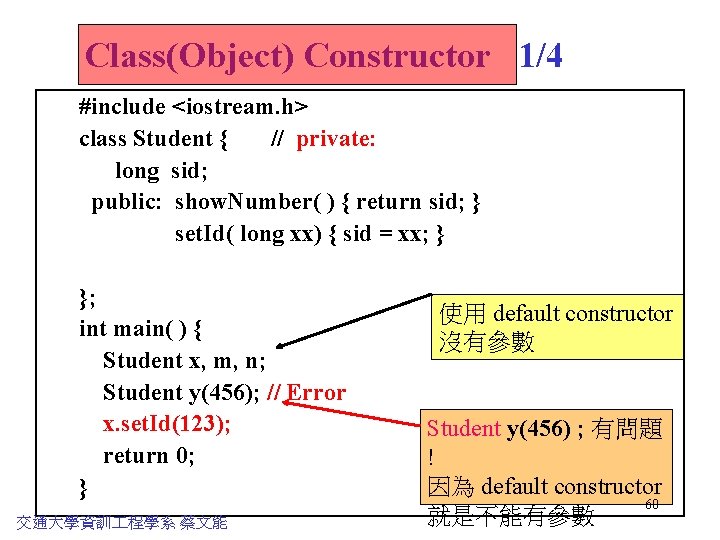
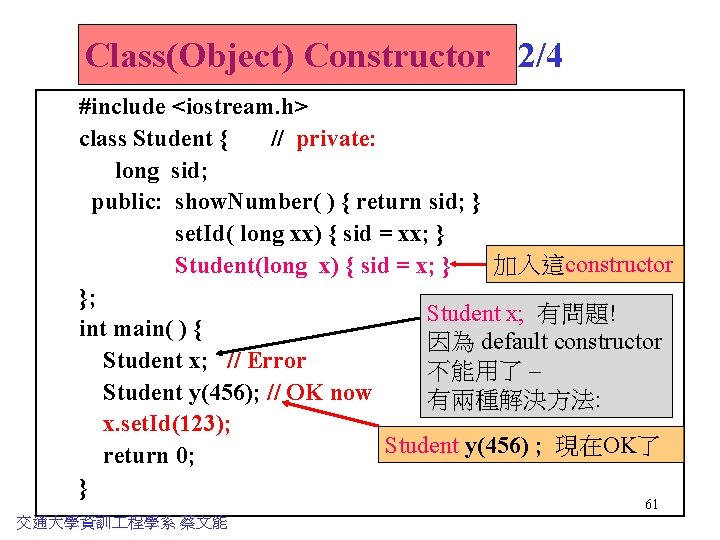
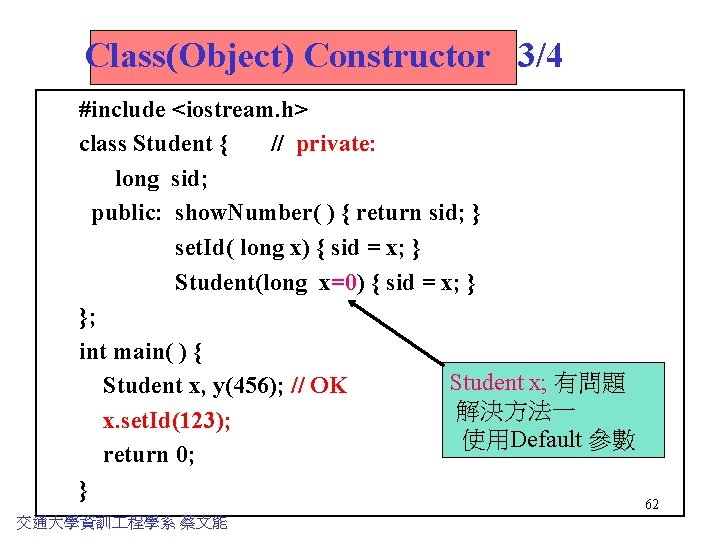
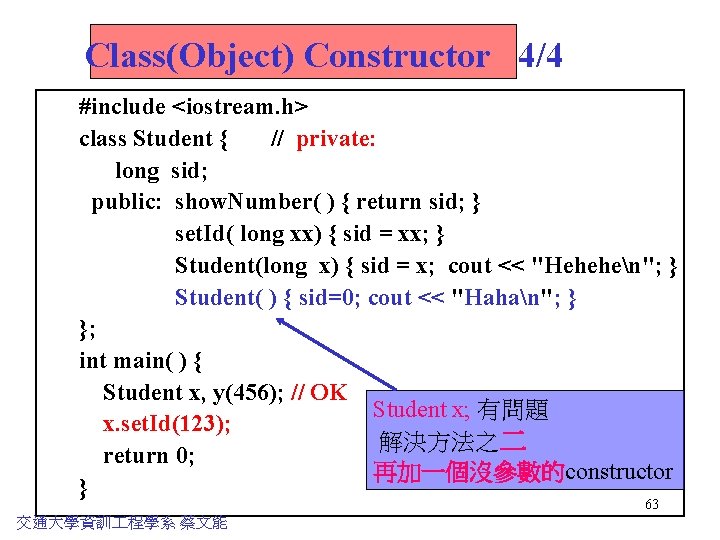
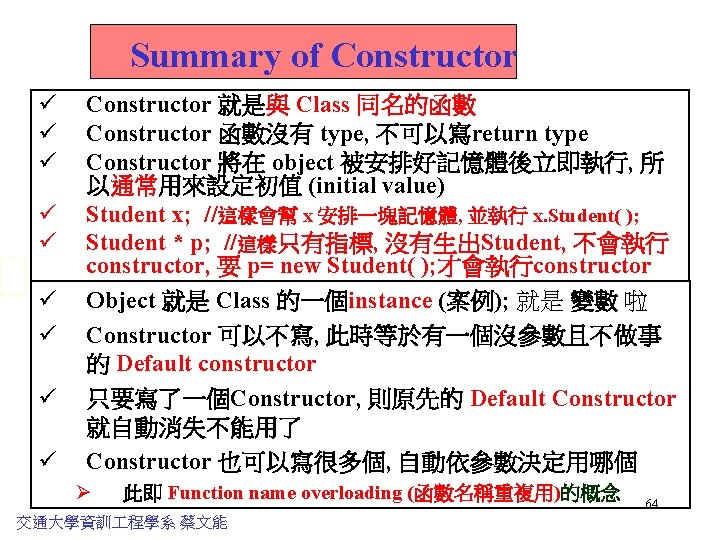
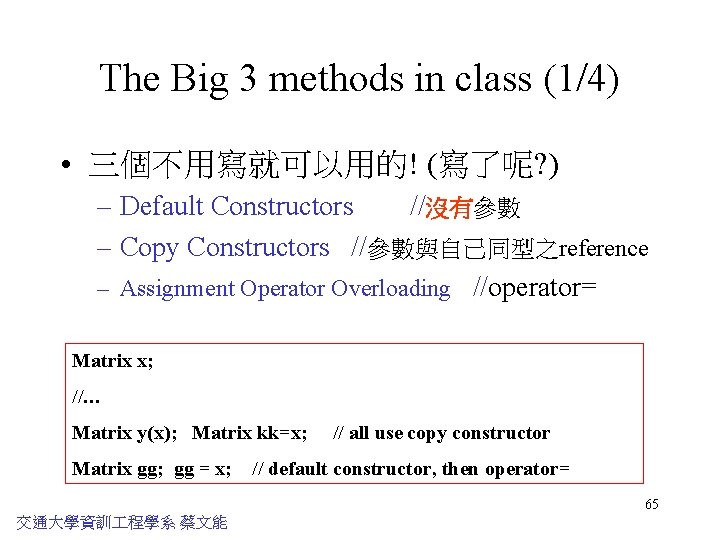
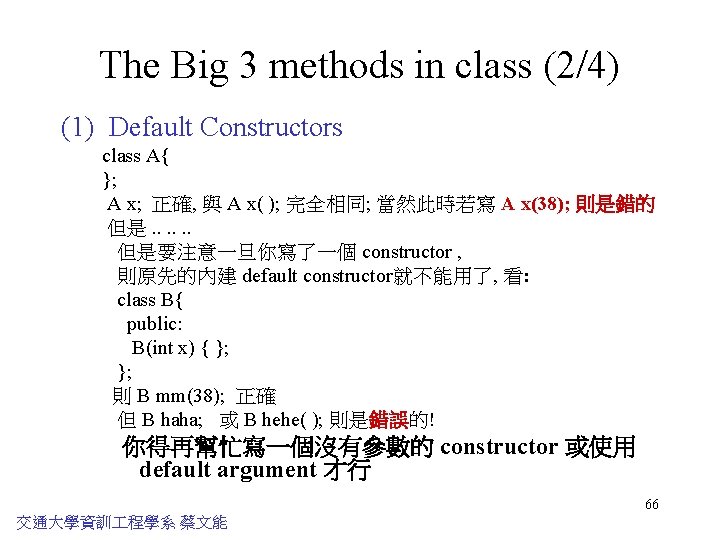
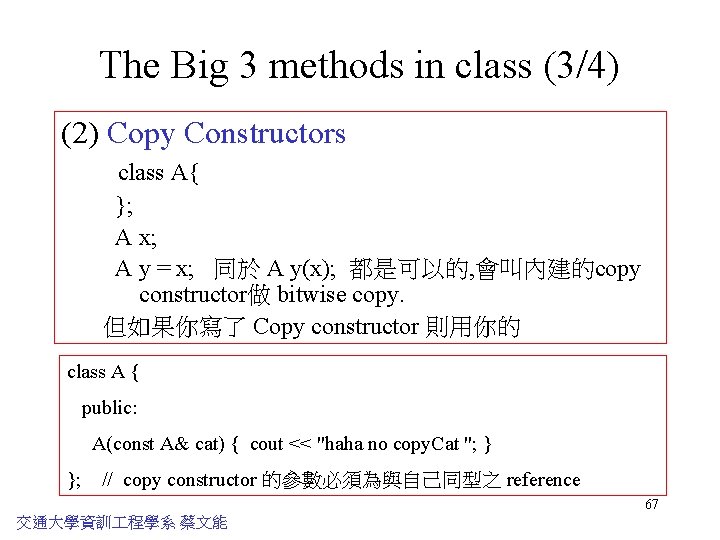
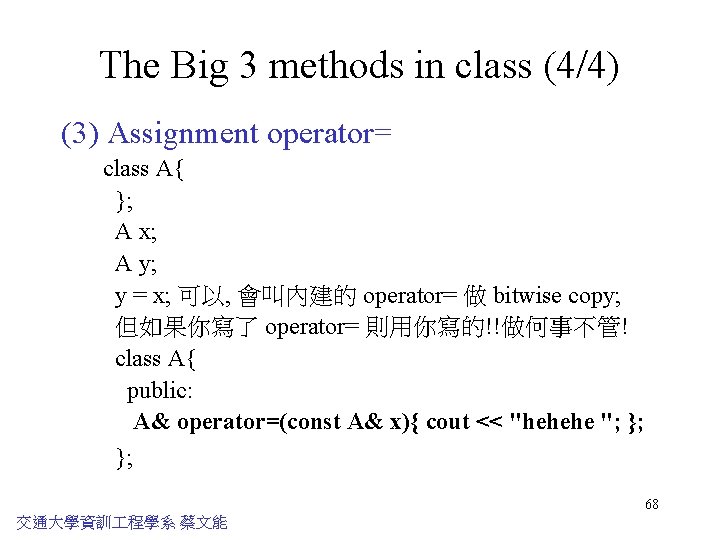
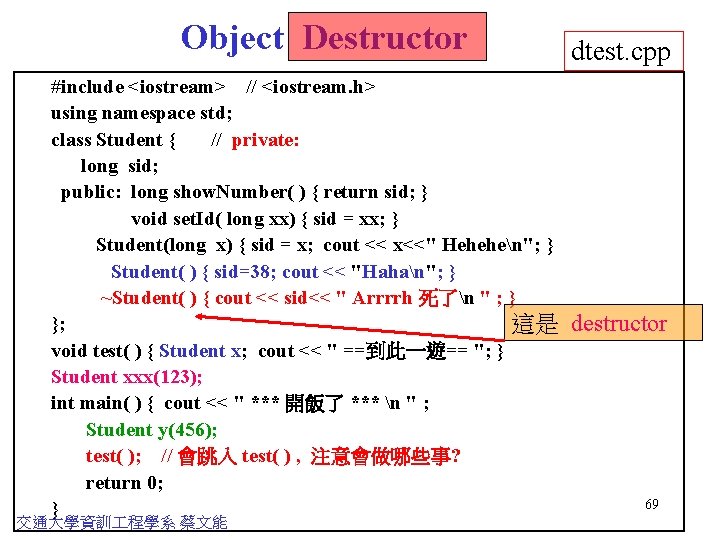
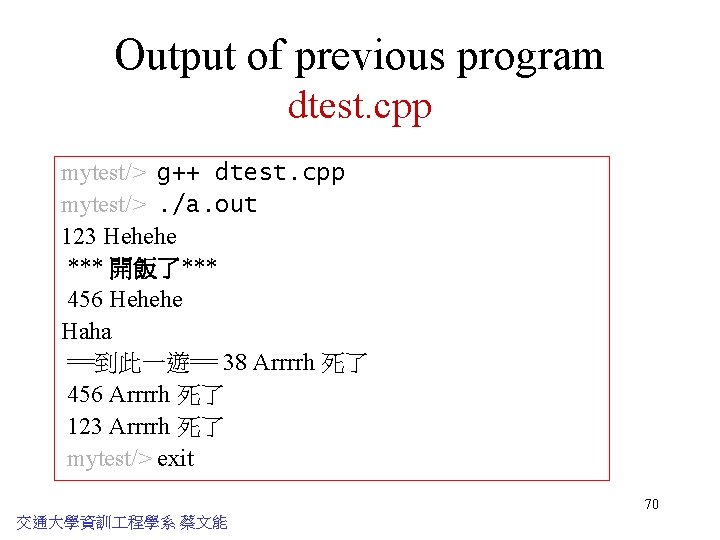
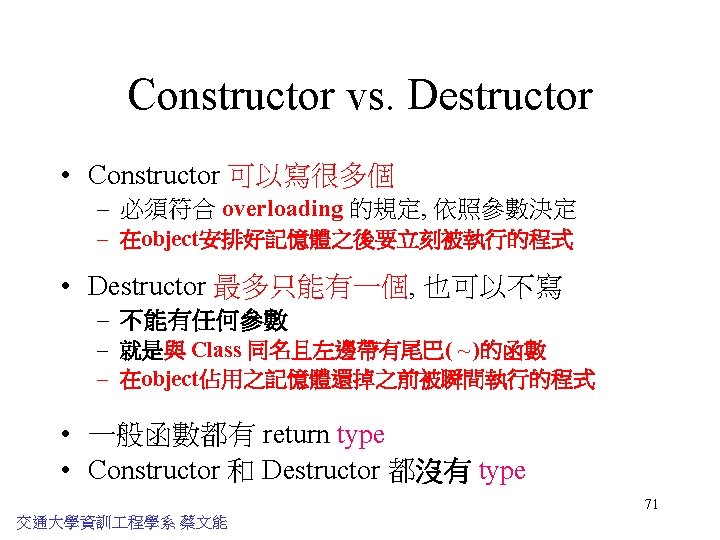
![神奇的 “static” ü On a global variable or a function static long my. Data[38]; 神奇的 “static” ü On a global variable or a function static long my. Data[38];](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-72.jpg)
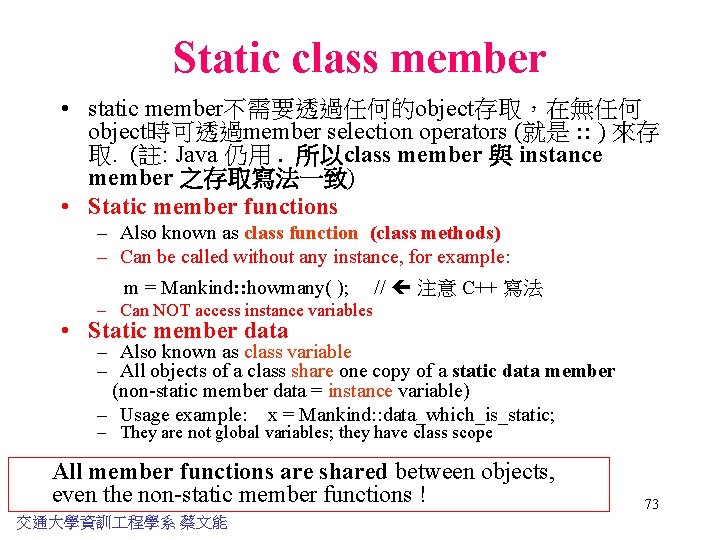
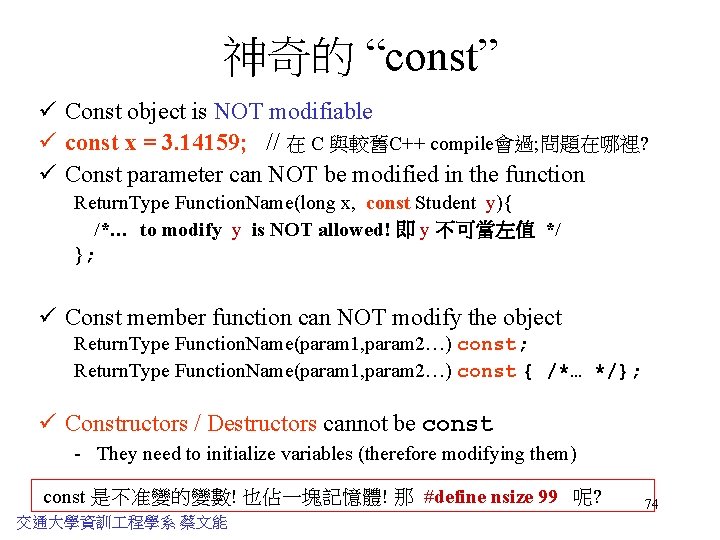
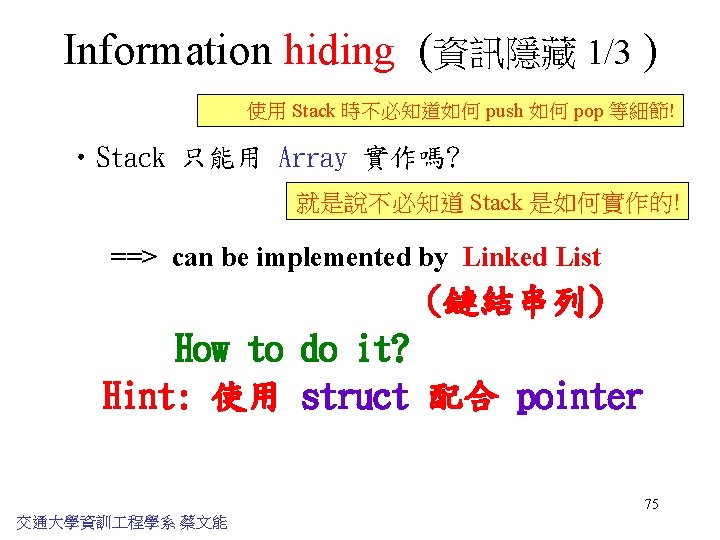
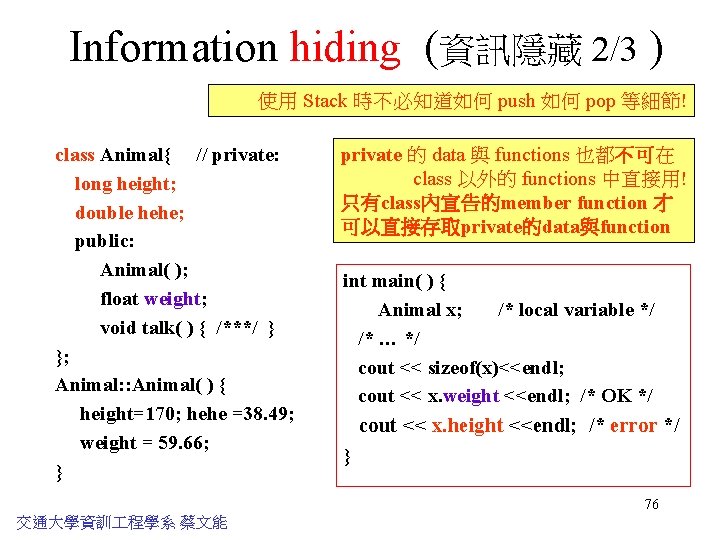
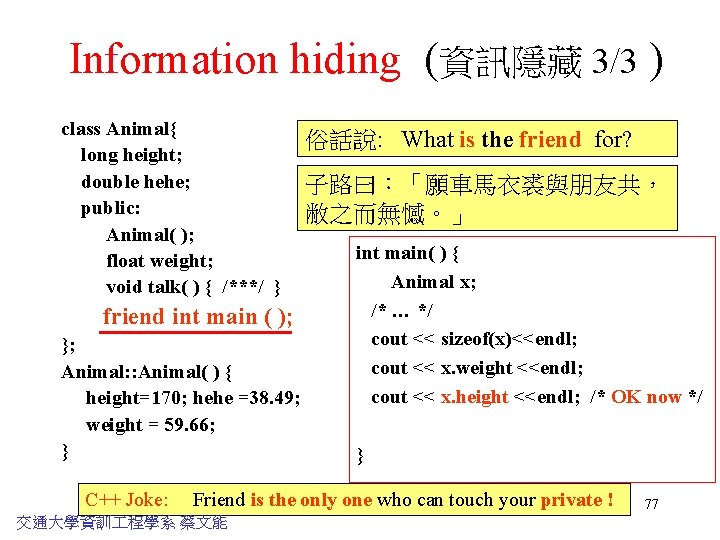
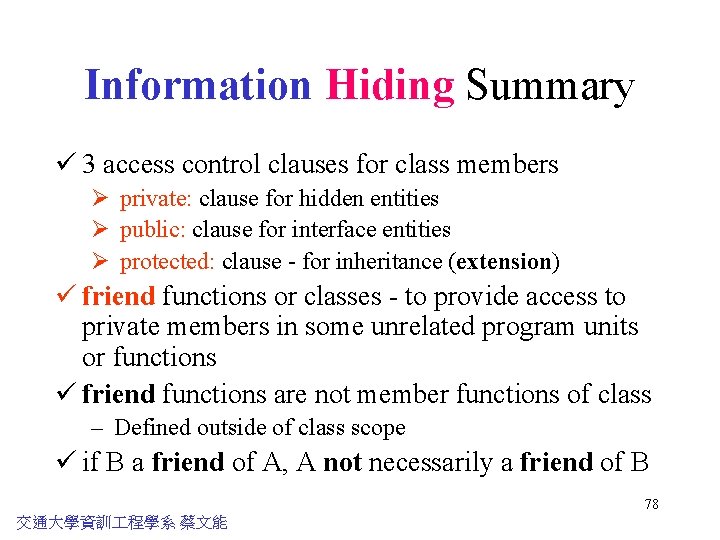
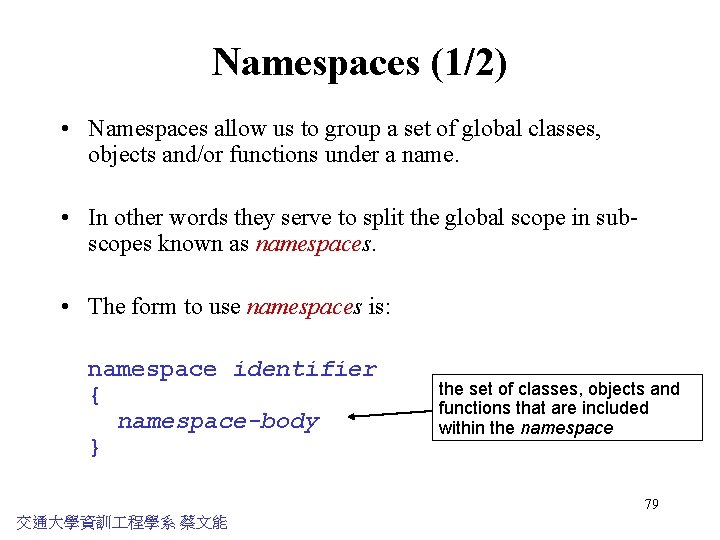
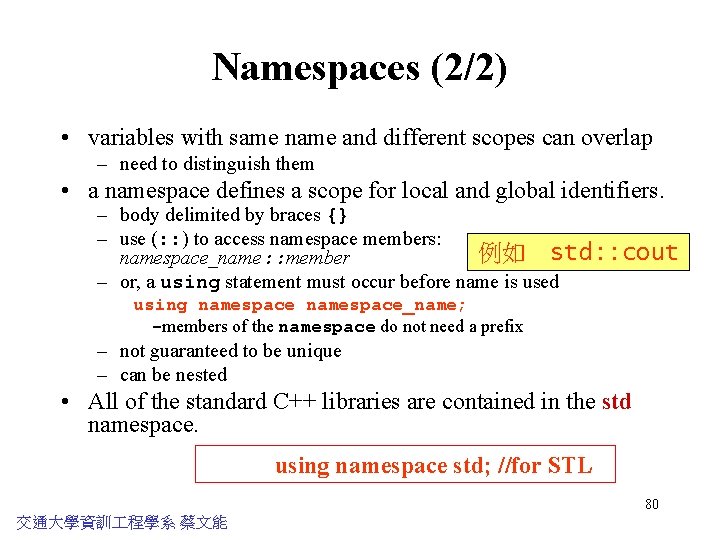
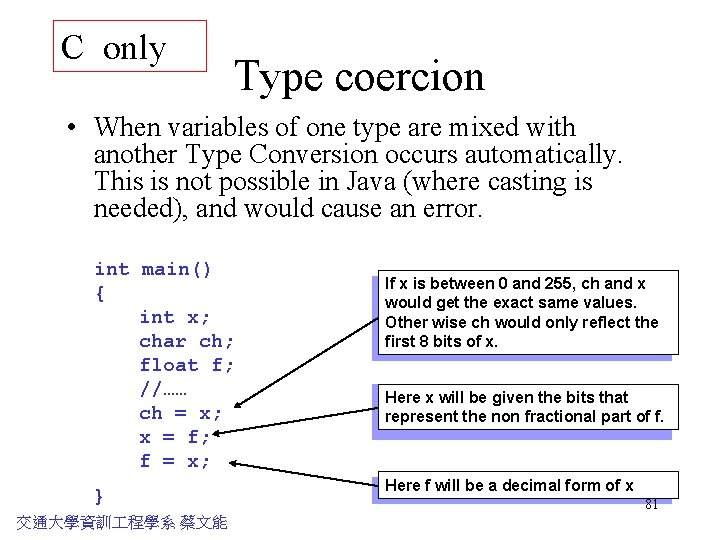
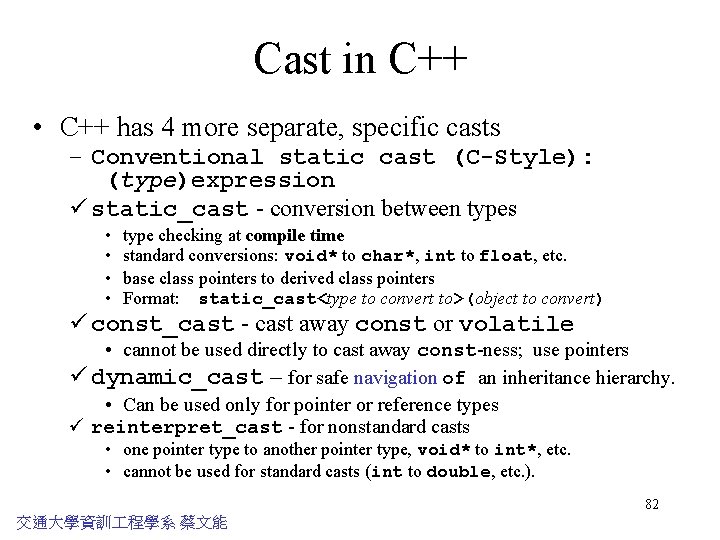
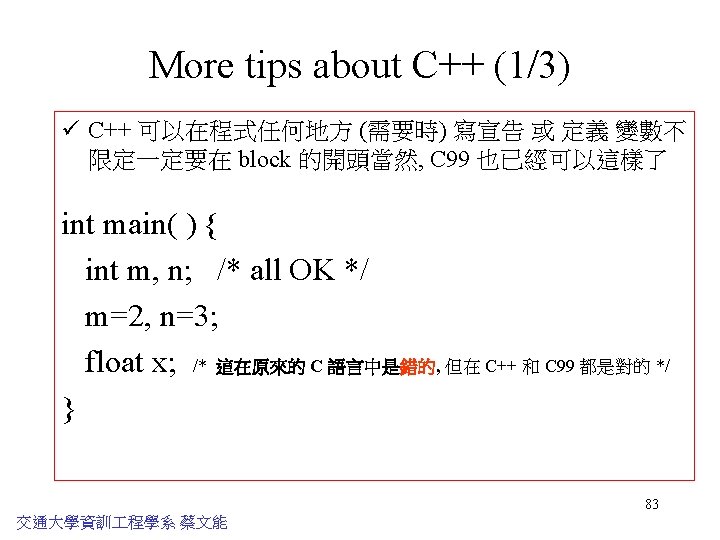
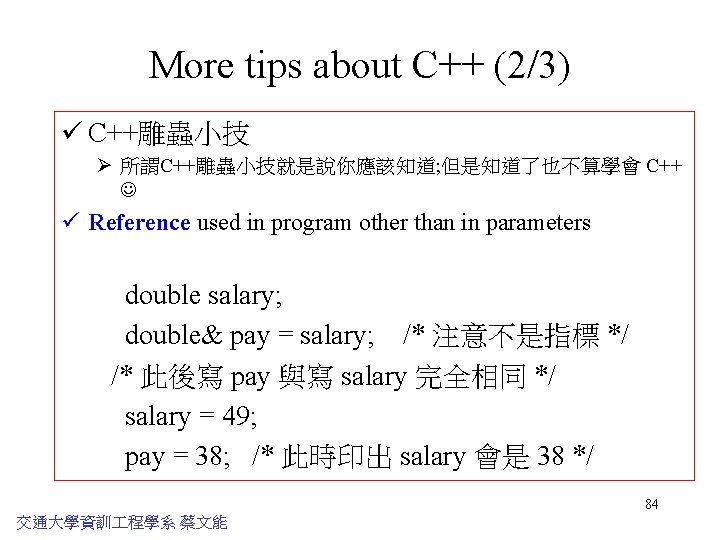
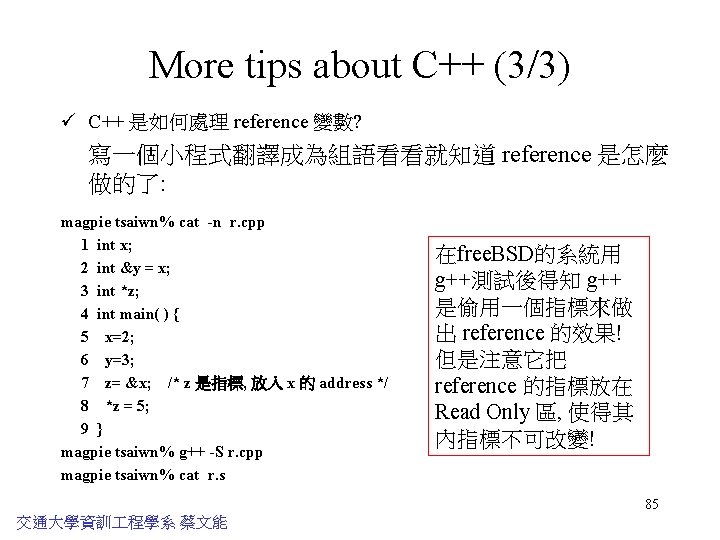
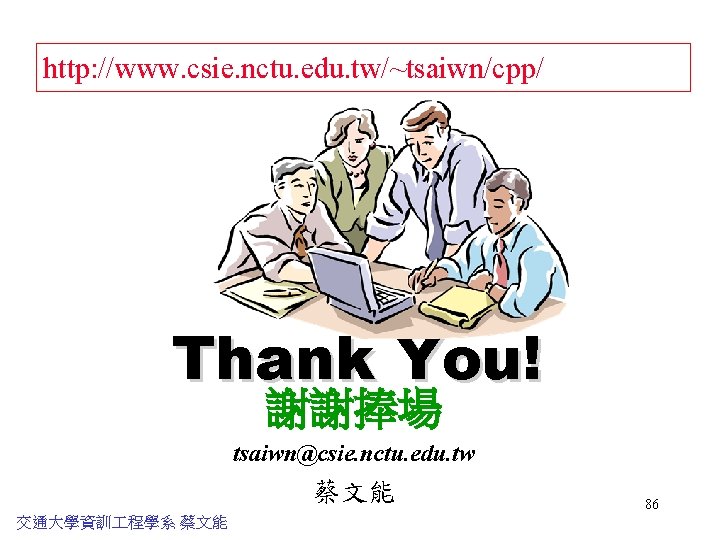
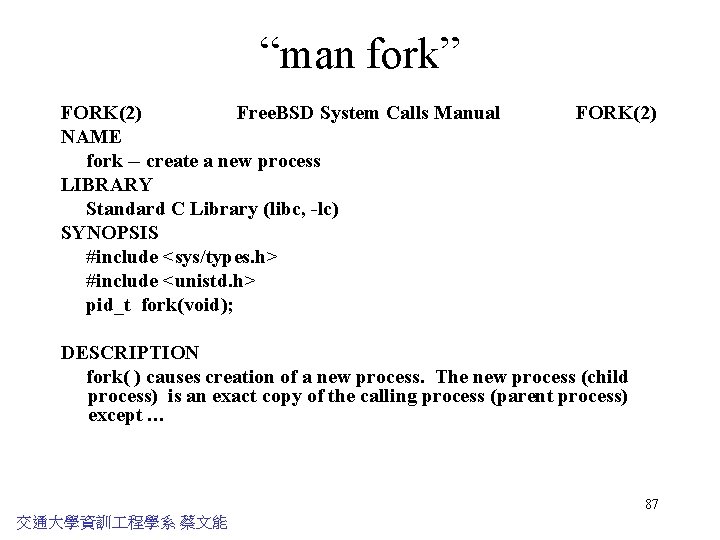
![fork( ) example switch(pid=fork( ) ) { case -1: syslog("Fork failed"); free(nchunk); close(null); close(fd[0]); fork( ) example switch(pid=fork( ) ) { case -1: syslog("Fork failed"); free(nchunk); close(null); close(fd[0]);](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-88.jpg)
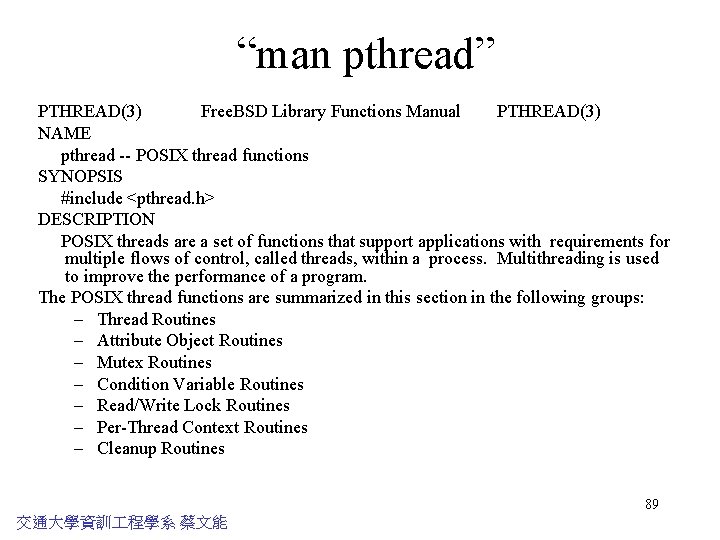
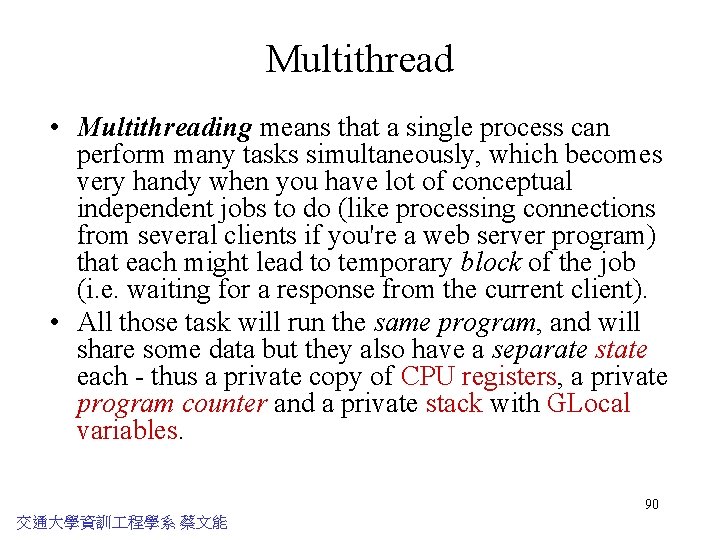
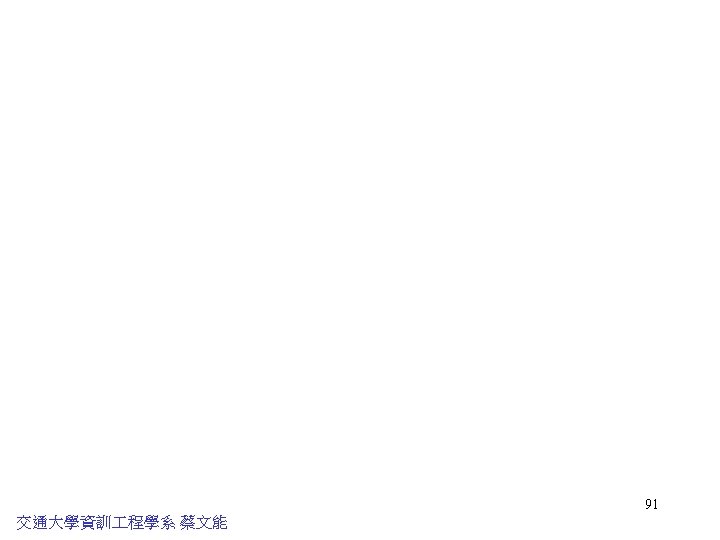
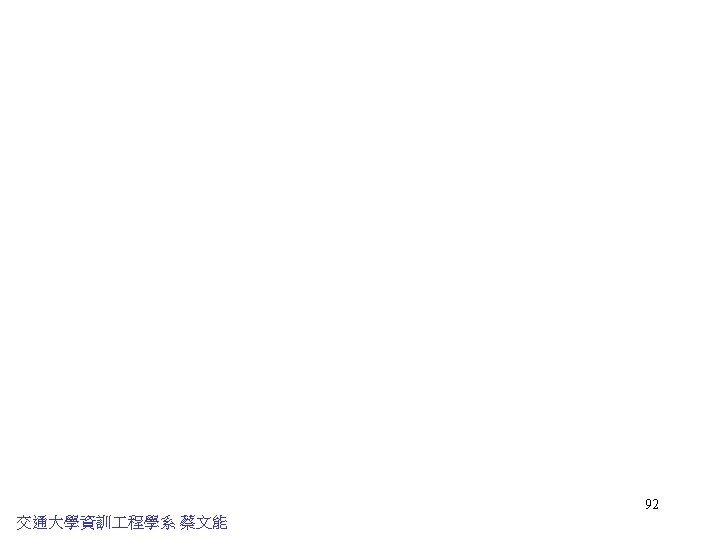
- Slides: 92
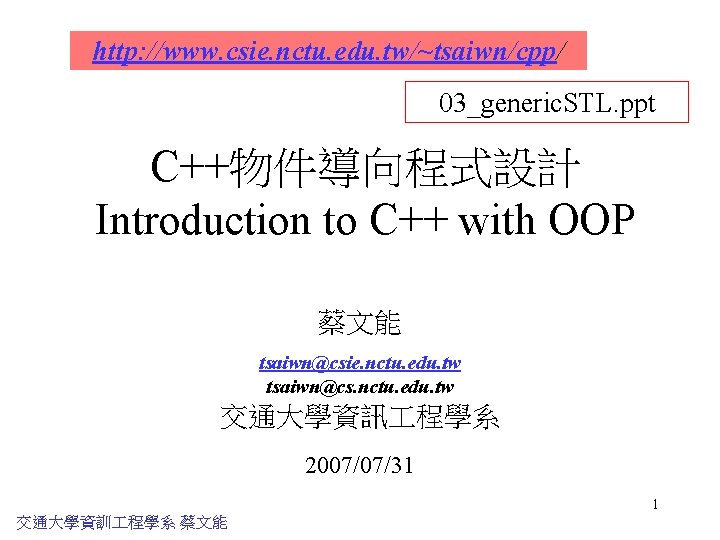
http: //www. csie. nctu. edu. tw/~tsaiwn/cpp/ 03_generic. STL. ppt C++物件導向程式設計 Introduction to C++ with OOP 蔡文能 tsaiwn@csie. nctu. edu. tw tsaiwn@cs. nctu. edu. tw 交通大學資訊 程學系 2007/07/31 1 交通大學資訓 程學系 蔡文能
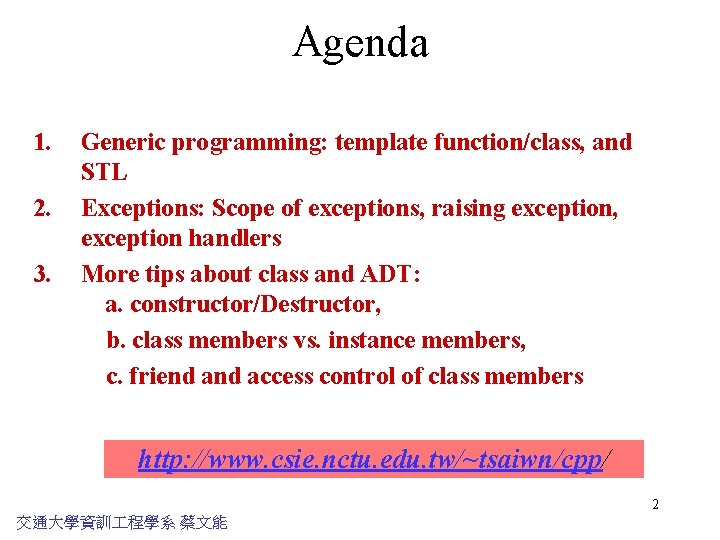
Agenda 1. 2. 3. Generic programming: template function/class, and STL Exceptions: Scope of exceptions, raising exception, exception handlers More tips about class and ADT: a. constructor/Destructor, b. class members vs. instance members, c. friend access control of class members http: //www. csie. nctu. edu. tw/~tsaiwn/cpp/ 2 交通大學資訓 程學系 蔡文能
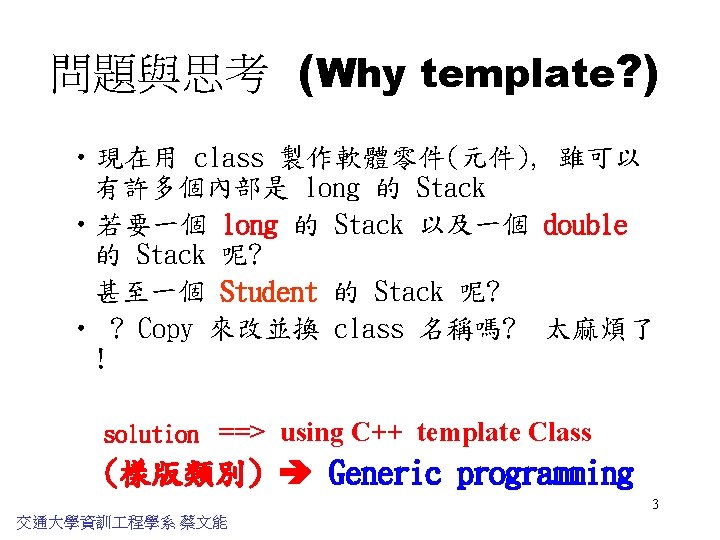
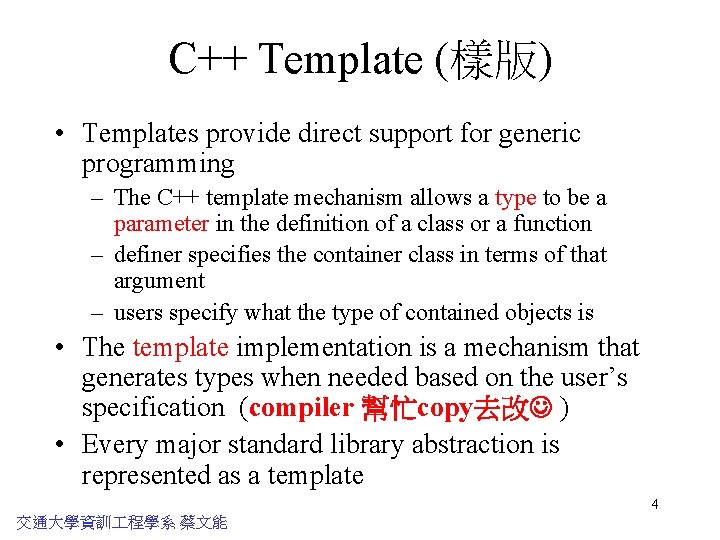
C++ Template (樣版) • Templates provide direct support for generic programming – The C++ template mechanism allows a type to be a parameter in the definition of a class or a function – definer specifies the container class in terms of that argument – users specify what the type of contained objects is • The template implementation is a mechanism that generates types when needed based on the user’s specification (compiler 幫忙copy去改 ) • Every major standard library abstraction is represented as a template 4 交通大學資訓 程學系 蔡文能
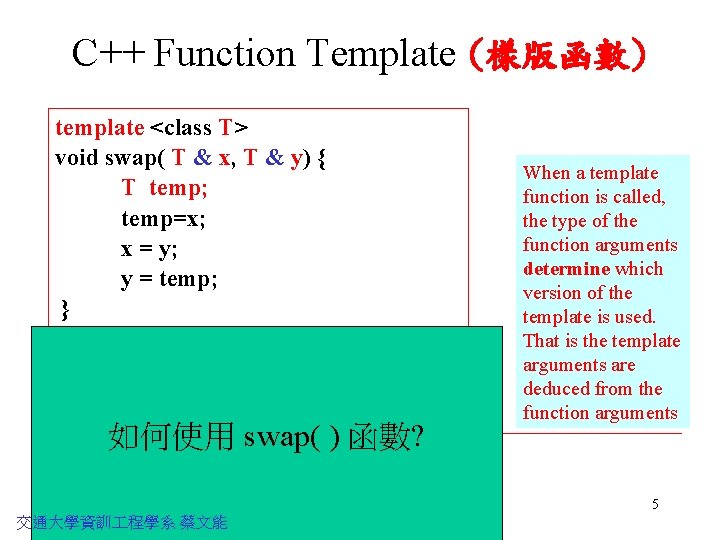
C++ Function Template (樣版函數) template <class T> void swap( T & x, T & y) { T temp; temp=x; x = y; y = temp; } int main( ) { int m=38, n=49; double x=12. 34, y=567. 135; swap(x, y); 如何使用 swap( ) 函數? swap(m, n); /** … **/ 交通大學資訓 程學系 蔡文能 } When a template function is called, the type of the function arguments determine which version of the template is used. That is the template arguments are deduced from the function arguments 5
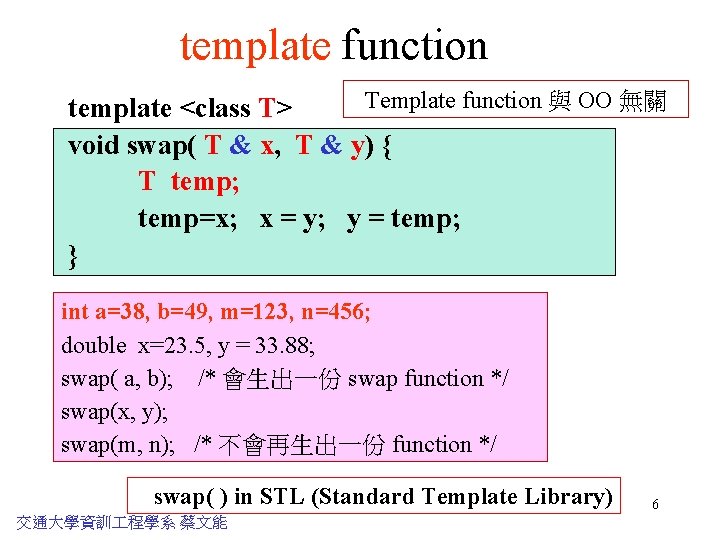
template function Template function 與 OO 無關 template <class T> void swap( T & x, T & y) { T temp; temp=x; x = y; y = temp; } int a=38, b=49, m=123, n=456; double x=23. 5, y = 33. 88; swap( a, b); /* 會生出一份 swap function */ swap(x, y); swap(m, n); /* 不會再生出一份 function */ swap( ) in STL (Standard Template Library) 交通大學資訓 程學系 蔡文能 6
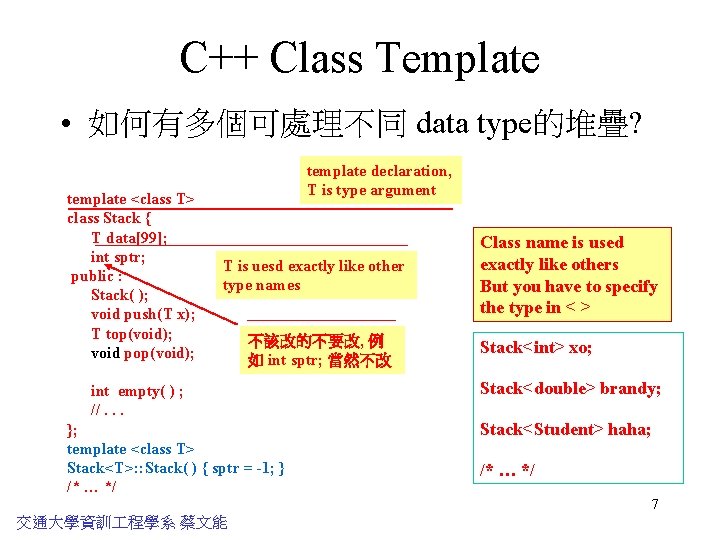
C++ Class Template • 如何有多個可處理不同 data type的堆疊? template <class T> class Stack { T data[99]; int sptr; public : Stack( ); void push(T x); T top(void); void pop(void); template declaration, T is type argument T is uesd exactly like other type names 不該改的不要改, 例 如 int sptr; 當然不改 int empty( ) ; //. . . }; template <class T> Stack<T>: : Stack( ) { sptr = -1; } /* … */ Class name is used exactly like others But you have to specify the type in < > Stack<int> xo; Stack<double> brandy; Stack<Student> haha; /* … */ 7 交通大學資訓 程學系 蔡文能
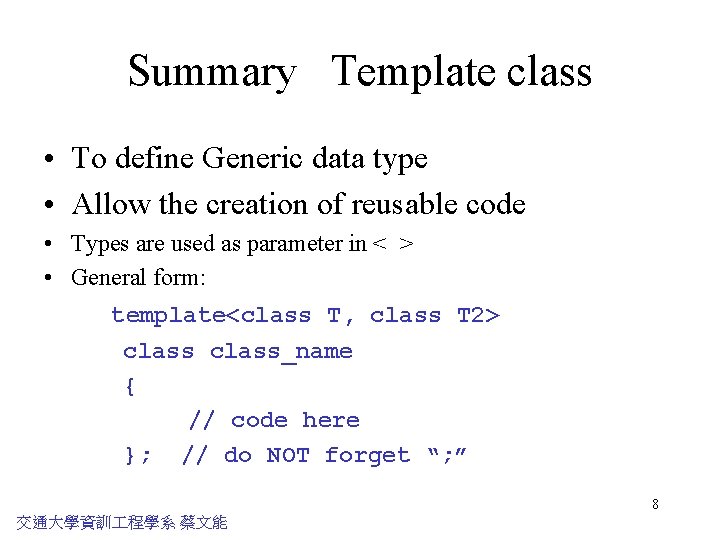
Summary Template class • To define Generic data type • Allow the creation of reusable code • Types are used as parameter in < > • General form: template<class T, class T 2> class_name { // code here }; // do NOT forget “; ” 8 交通大學資訓 程學系 蔡文能
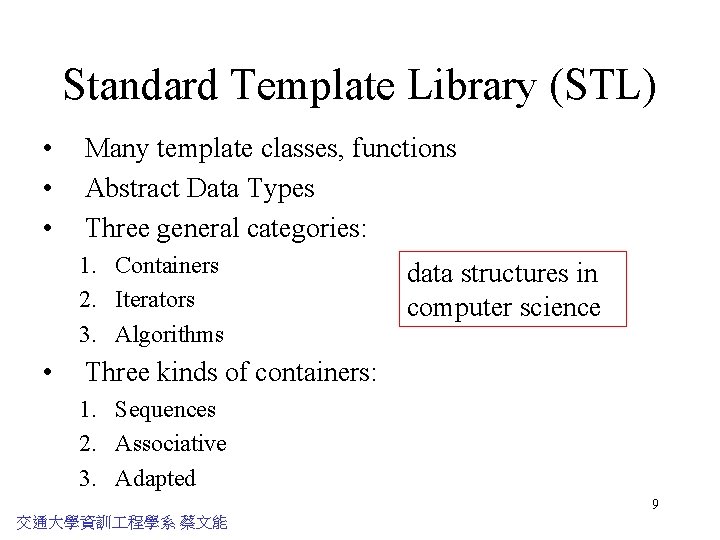
Standard Template Library (STL) • • • Many template classes, functions Abstract Data Types Three general categories: 1. Containers 2. Iterators 3. Algorithms • data structures in computer science Three kinds of containers: 1. Sequences 2. Associative 3. Adapted 9 交通大學資訓 程學系 蔡文能
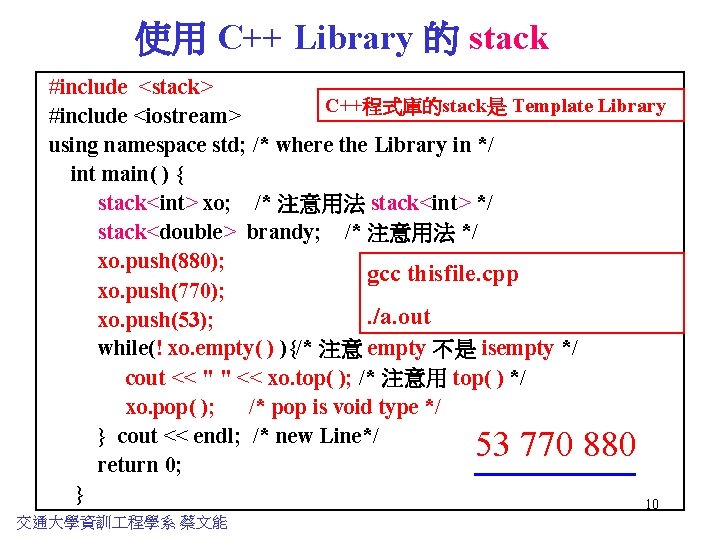
使用 C++ Library 的 stack #include <stack> C++程式庫的stack是 Template Library #include <iostream> using namespace std; /* where the Library in */ int main( ) { stack<int> xo; /* 注意用法 stack<int> */ stack<double> brandy; /* 注意用法 */ xo. push(880); gcc thisfile. cpp xo. push(770); . /a. out xo. push(53); while(! xo. empty( ) ){/* 注意 empty 不是 isempty */ cout << " " << xo. top( ); /* 注意用 top( ) */ xo. pop( ); /* pop is void type */ } cout << endl; /* new Line*/ return 0; } 10 53 770 880 交通大學資訓 程學系 蔡文能
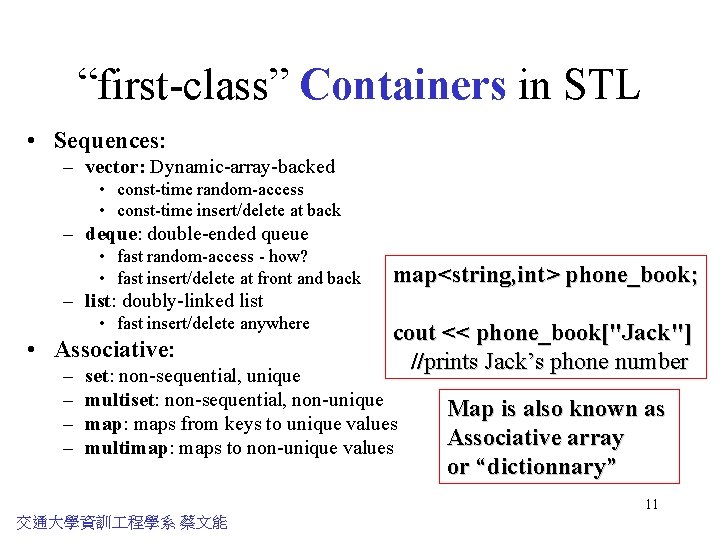
“first-class” Containers in STL • Sequences: – vector: Dynamic-array-backed • const-time random-access • const-time insert/delete at back – deque: double-ended queue • fast random-access - how? • fast insert/delete at front and back map<string, int> phone_book; – list: doubly-linked list • fast insert/delete anywhere • Associative: – – cout << phone_book["Jack"] //prints Jack’s phone number set: non-sequential, unique multiset: non-sequential, non-unique map: maps from keys to unique values multimap: maps to non-unique values Map is also known as Associative array or “dictionnary” 11 交通大學資訓 程學系 蔡文能
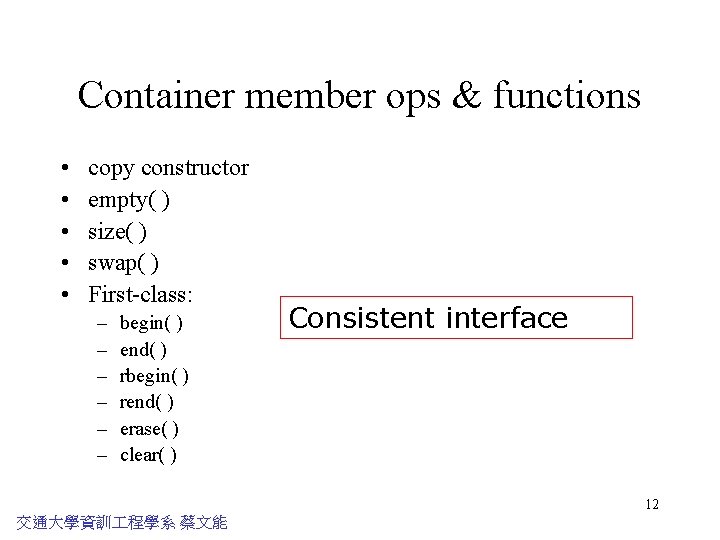
Container member ops & functions • • • copy constructor empty( ) size( ) swap( ) First-class: – – – begin( ) end( ) rbegin( ) rend( ) erase( ) clear( ) Consistent interface 12 交通大學資訓 程學系 蔡文能
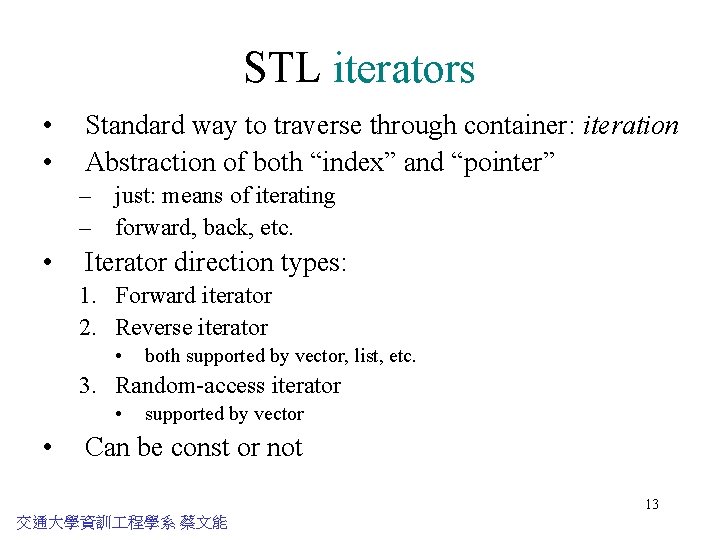
STL iterators • • Standard way to traverse through container: iteration Abstraction of both “index” and “pointer” – just: means of iterating – forward, back, etc. • Iterator direction types: 1. Forward iterator 2. Reverse iterator • both supported by vector, list, etc. 3. Random-access iterator • • supported by vector Can be const or not 13 交通大學資訓 程學系 蔡文能
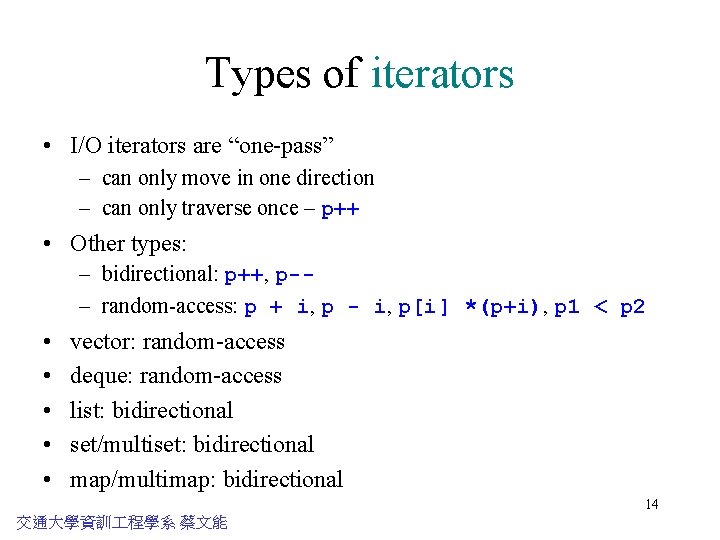
Types of iterators • I/O iterators are “one-pass” – can only move in one direction – can only traverse once – p++ • Other types: – bidirectional: p++, p-– random-access: p + i, p - i, p[i] *(p+i), p 1 < p 2 • • • vector: random-access deque: random-access list: bidirectional set/multiset: bidirectional map/multimap: bidirectional 14 交通大學資訓 程學系 蔡文能
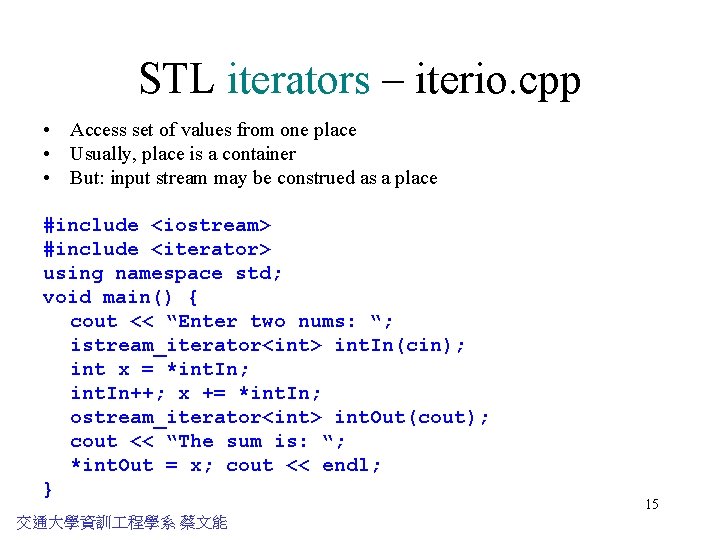
STL iterators – iterio. cpp • Access set of values from one place • Usually, place is a container • But: input stream may be construed as a place #include <iostream> #include <iterator> using namespace std; void main() { cout << “Enter two nums: “; istream_iterator<int> int. In(cin); int x = *int. In; int. In++; x += *int. In; ostream_iterator<int> int. Out(cout); cout << “The sum is: “; *int. Out = x; cout << endl; } 交通大學資訓 程學系 蔡文能 15
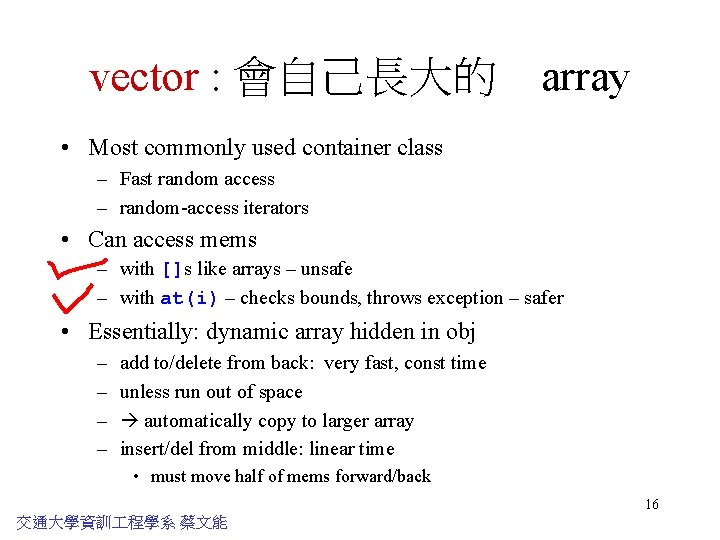
vector : 會自己長大的 array • Most commonly used container class – Fast random access – random-access iterators • Can access mems – with []s like arrays – unsafe – with at(i) – checks bounds, throws exception – safer • Essentially: dynamic array hidden in obj – – add to/delete from back: very fast, const time unless run out of space automatically copy to larger array insert/del from middle: linear time • must move half of mems forward/back 16 交通大學資訓 程學系 蔡文能
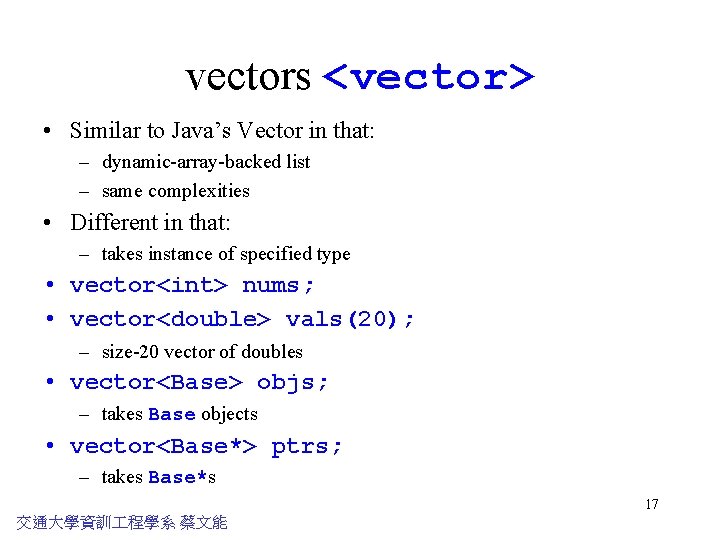
vectors <vector> • Similar to Java’s Vector in that: – dynamic-array-backed list – same complexities • Different in that: – takes instance of specified type • vector<int> nums; • vector<double> vals(20); – size-20 vector of doubles • vector<Base> objs; – takes Base objects • vector<Base*> ptrs; – takes Base*s 17 交通大學資訓 程學系 蔡文能
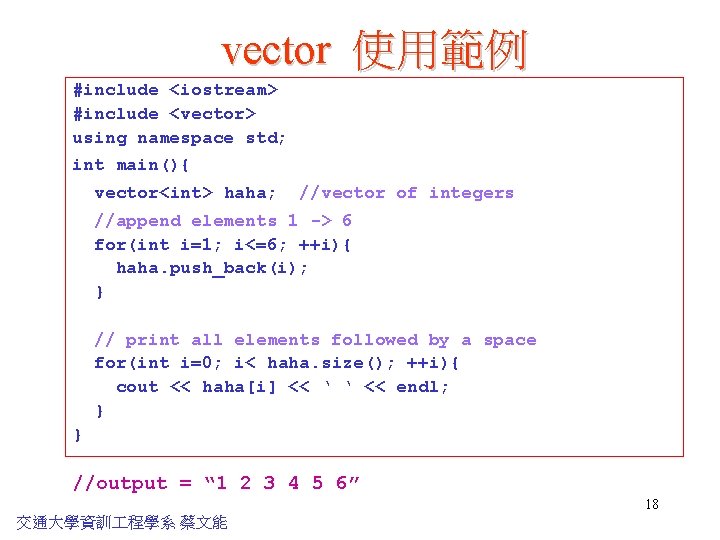
vector 使用範例 #include <iostream> #include <vector> using namespace std; int main(){ vector<int> haha; //vector of integers //append elements 1 -> 6 for(int i=1; i<=6; ++i){ haha. push_back(i); } // print all elements followed by a space for(int i=0; i< haha. size(); ++i){ cout << haha[i] << ‘ ‘ << endl; } } //output = “ 1 2 3 4 5 6” 18 交通大學資訓 程學系 蔡文能
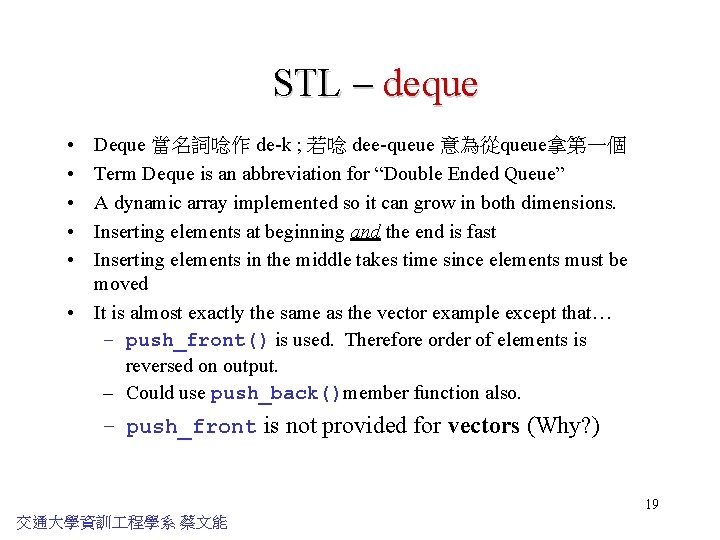
STL – deque • • • Deque 當名詞唸作 de-k ; 若唸 dee-queue 意為從queue拿第一個 Term Deque is an abbreviation for “Double Ended Queue” A dynamic array implemented so it can grow in both dimensions. Inserting elements at beginning and the end is fast Inserting elements in the middle takes time since elements must be moved • It is almost exactly the same as the vector example except that… – push_front() is used. Therefore order of elements is reversed on output. – Could use push_back()member function also. – push_front is not provided for vectors (Why? ) 19 交通大學資訓 程學系 蔡文能
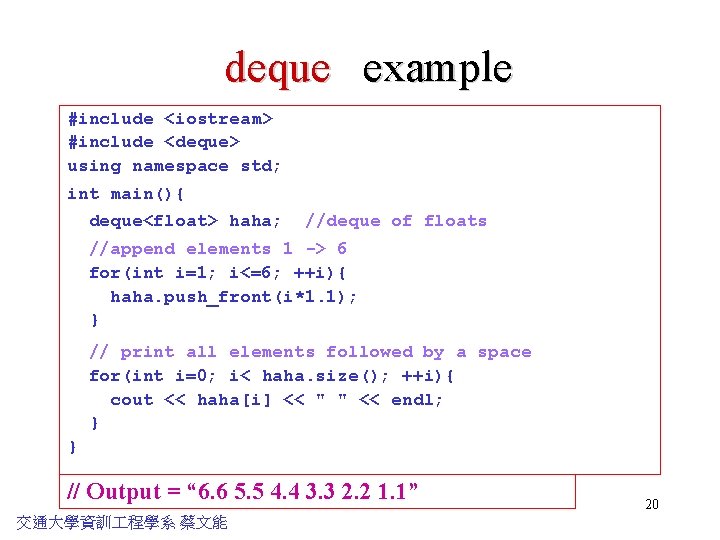
deque example #include <iostream> #include <deque> using namespace std; int main(){ deque<float> haha; //deque of floats //append elements 1 -> 6 for(int i=1; i<=6; ++i){ haha. push_front(i*1. 1); } // print all elements followed by a space for(int i=0; i< haha. size(); ++i){ cout << haha[i] << " " << endl; } } // Output = “ 6. 6 5. 5 4. 4 3. 3 2. 2 1. 1” 交通大學資訓 程學系 蔡文能 20
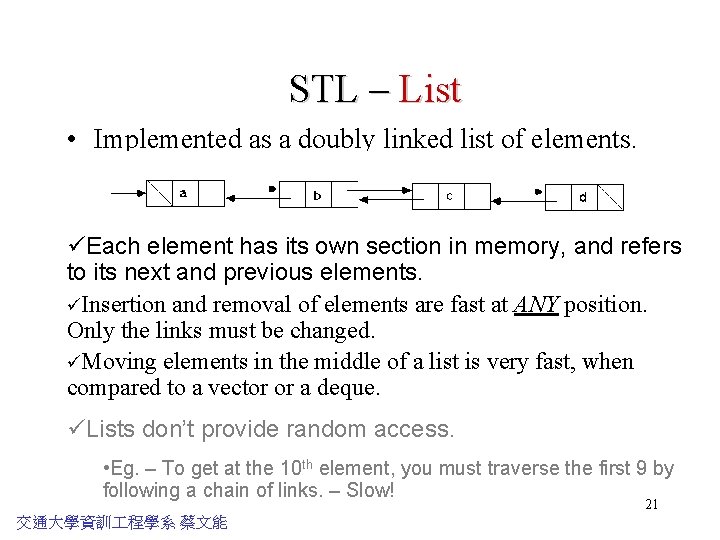
STL – List • Implemented as a doubly linked list of elements. üEach element has its own section in memory, and refers to its next and previous elements. üInsertion and removal of elements are fast at ANY position. Only the links must be changed. üMoving elements in the middle of a list is very fast, when compared to a vector or a deque. üLists don’t provide random access. • Eg. – To get at the 10 th element, you must traverse the first 9 by following a chain of links. – Slow! 21 交通大學資訓 程學系 蔡文能
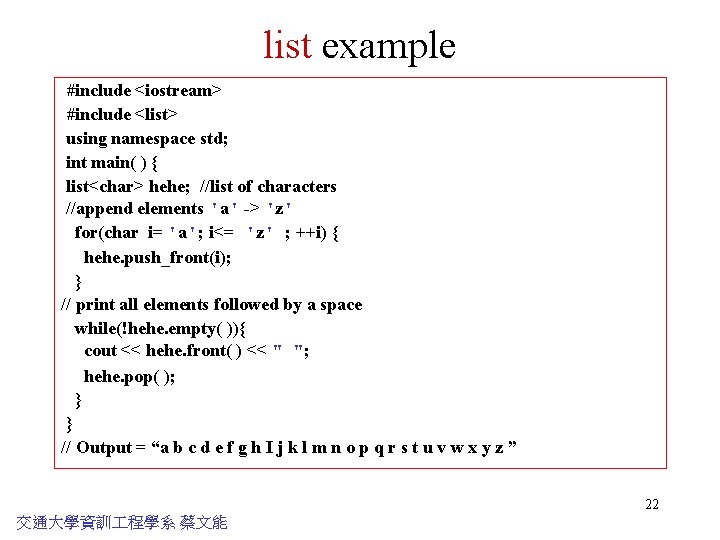
list example #include <iostream> #include <list> using namespace std; int main( ) { list<char> hehe; //list of characters //append elements 'a' -> 'z' for(char i= 'a'; i<= 'z' ; ++i) { hehe. push_front(i); } // print all elements followed by a space while(!hehe. empty( )){ cout << hehe. front( ) << " "; hehe. pop( ); } } // Output = “a b c d e f g h I j k l m n o p q r s t u v w x y z ” 22 交通大學資訓 程學系 蔡文能
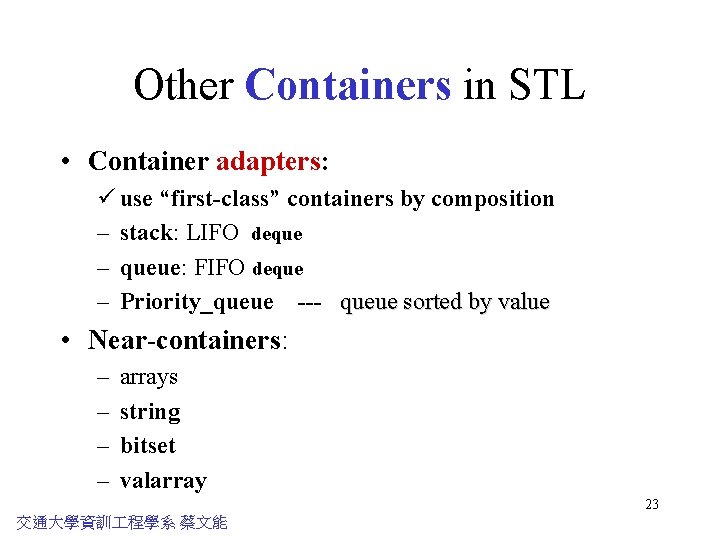
Other Containers in STL • Container adapters: ü use “first-class” containers by composition – stack: LIFO deque – queue: FIFO deque – Priority_queue --- queue sorted by value • Near-containers: – – arrays string bitset valarray 23 交通大學資訓 程學系 蔡文能
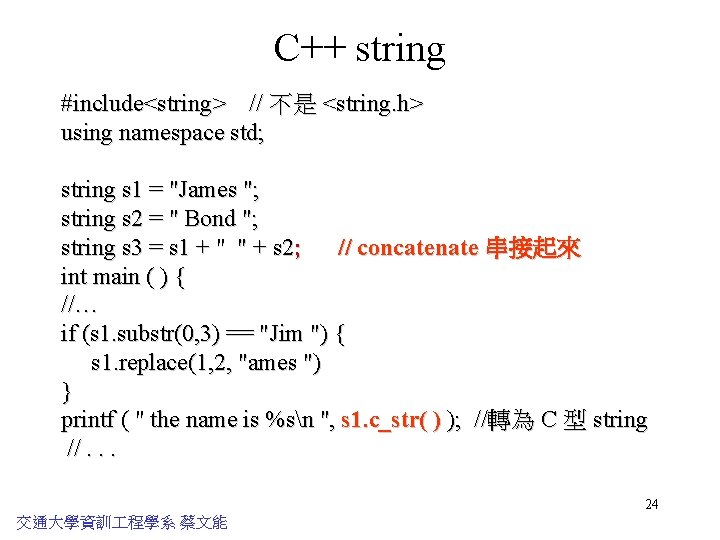
C++ string #include<string> // 不是 <string. h> using namespace std; string s 1 = "James "; string s 2 = " Bond "; string s 3 = s 1 + " " + s 2; // concatenate 串接起來 int main ( ) { //… if (s 1. substr(0, 3) == "Jim ") { s 1. replace(1, 2, "ames ") } printf ( " the name is %sn ", s 1. c_str( ) ); //轉為 C 型 string //. . . 24 交通大學資訓 程學系 蔡文能
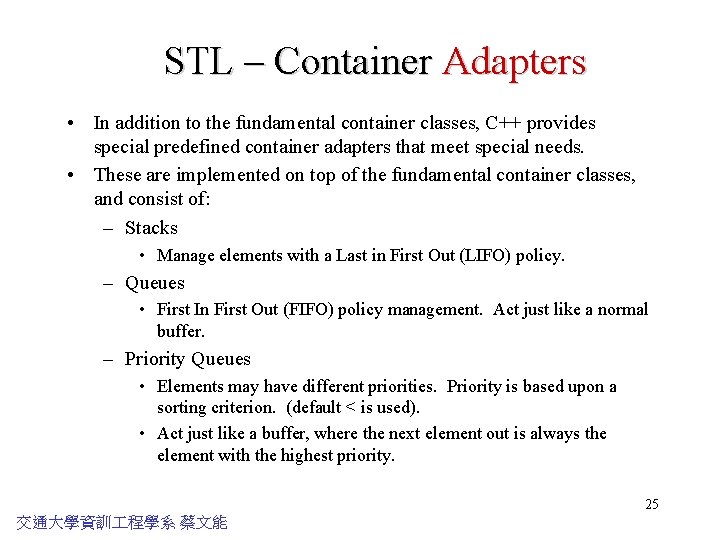
STL – Container Adapters • In addition to the fundamental container classes, C++ provides special predefined container adapters that meet special needs. • These are implemented on top of the fundamental container classes, and consist of: – Stacks • Manage elements with a Last in First Out (LIFO) policy. – Queues • First In First Out (FIFO) policy management. Act just like a normal buffer. – Priority Queues • Elements may have different priorities. Priority is based upon a sorting criterion. (default < is used). • Act just like a buffer, where the next element out is always the element with the highest priority. 25 交通大學資訓 程學系 蔡文能
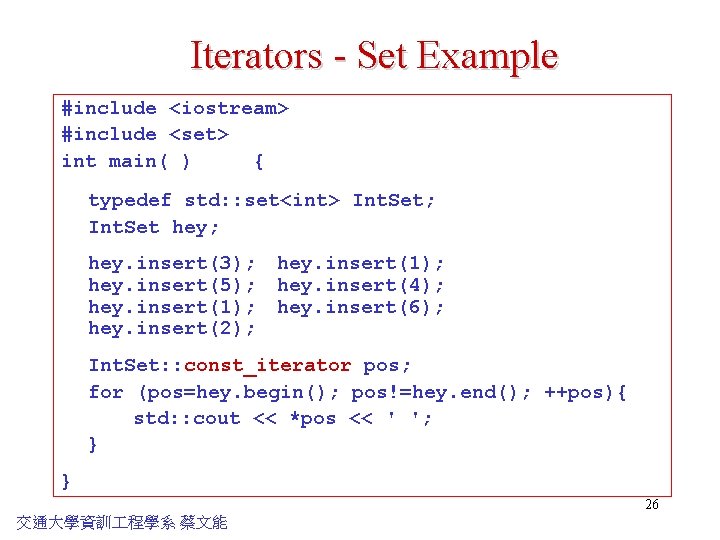
Iterators - Set Example #include <iostream> #include <set> int main( ) { typedef std: : set<int> Int. Set; Int. Set hey; hey. insert(3); hey. insert(1); hey. insert(5); hey. insert(4); hey. insert(1); hey. insert(6); hey. insert(2); Int. Set: : const_iterator pos; for (pos=hey. begin(); pos!=hey. end(); ++pos){ std: : cout << *pos << ' '; } 26 交通大學資訓 程學系 蔡文能
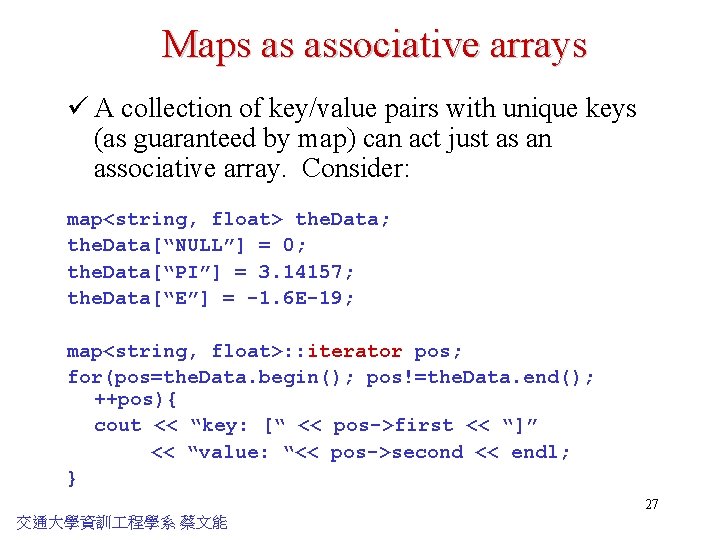
Maps as associative arrays ü A collection of key/value pairs with unique keys (as guaranteed by map) can act just as an associative array. Consider: map<string, float> the. Data; the. Data[“NULL”] = 0; the. Data[“PI”] = 3. 14157; the. Data[“E”] = -1. 6 E-19; map<string, float>: : iterator pos; for(pos=the. Data. begin(); pos!=the. Data. end(); ++pos){ cout << “key: [“ << pos->first << “]” << “value: “<< pos->second << endl; } 27 交通大學資訓 程學系 蔡文能
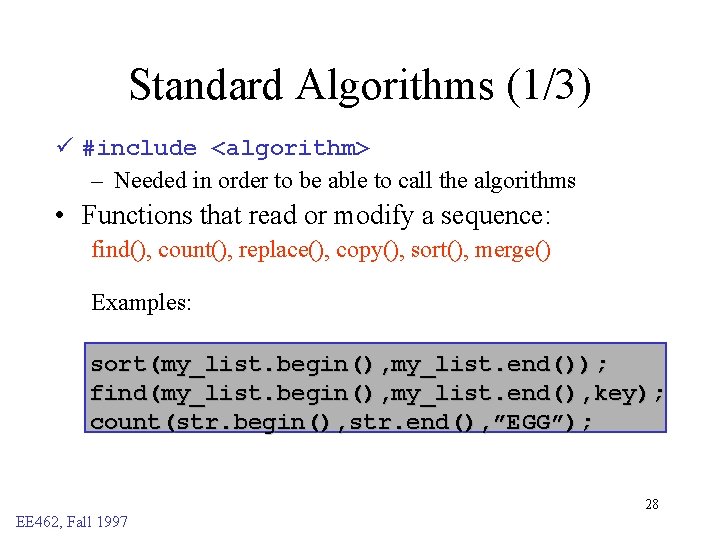
Standard Algorithms (1/3) ü #include <algorithm> – Needed in order to be able to call the algorithms • Functions that read or modify a sequence: find(), count(), replace(), copy(), sort(), merge() Examples: sort(my_list. begin(), my_list. end()); find(my_list. begin(), my_list. end(), key); count(str. begin(), str. end(), ”EGG”); 28 EE 462, Fall 1997
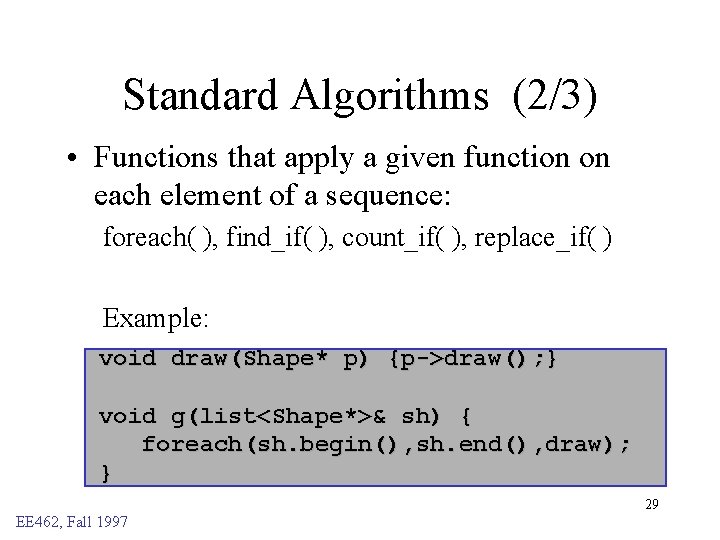
Standard Algorithms (2/3) • Functions that apply a given function on each element of a sequence: foreach( ), find_if( ), count_if( ), replace_if( ) Example: void draw(Shape* p) {p->draw(); } void g(list<Shape*>& sh) { foreach(sh. begin(), sh. end(), draw); } 29 EE 462, Fall 1997
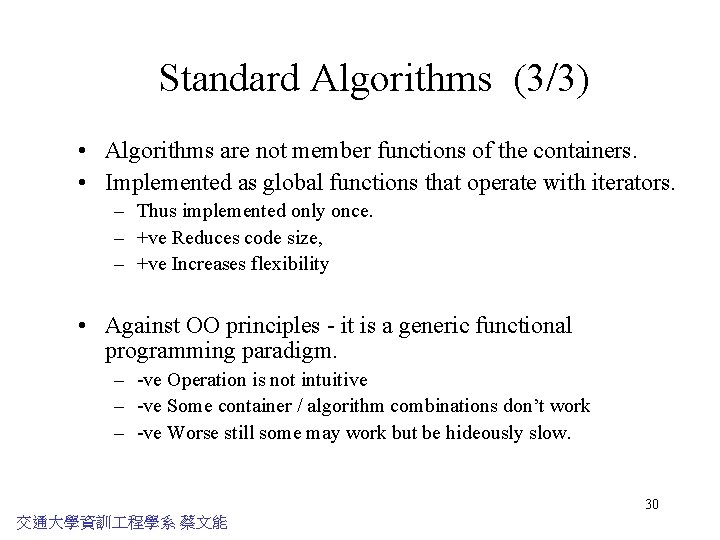
Standard Algorithms (3/3) • Algorithms are not member functions of the containers. • Implemented as global functions that operate with iterators. – Thus implemented only once. – +ve Reduces code size, – +ve Increases flexibility • Against OO principles - it is a generic functional programming paradigm. – -ve Operation is not intuitive – -ve Some container / algorithm combinations don’t work – -ve Worse still some may work but be hideously slow. 30 交通大學資訓 程學系 蔡文能
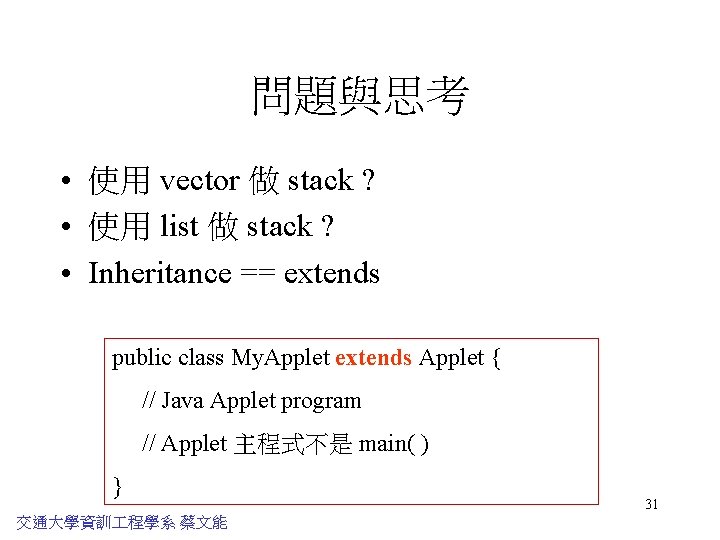
問題與思考 • 使用 vector 做 stack ? • 使用 list 做 stack ? • Inheritance == extends public class My. Applet extends Applet { // Java Applet program // Applet 主程式不是 main( ) } 交通大學資訓 程學系 蔡文能 31
![用 vector 做出 stack 12 tsaiwnccbsd 3 vector STK cat n mystk 3 h 用 vector 做出 stack (1/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mystk 3. h](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-32.jpg)
用 vector 做出 stack (1/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mystk 3. h 1 #include <vector> 2 using namespace std; 3 template <class T> 4 class My. Stack { 5 vector <T> x; 6 public: 7 void push(T y) { 8 x. push_back(y); 9 } Stack contains 10 T top( ) { 11 return x. back( ); 12 } 13 void pop( ) { 14 x. pop_back( ); 15 } 16 bool empty( ) { return x. begin() == x. end(); } 17 }; a vector 32 交通大學資訓 程學系 蔡文能
![用 vector 做出 stack 22 tsaiwnccbsd 3 vector STK cat n mymain 3 cpp 用 vector 做出 stack (2/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mymain 3. cpp](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-33.jpg)
用 vector 做出 stack (2/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mymain 3. cpp 1 // mymain 3. cpp; g++ mymain 3. cpp ; . /a. out 2 using namespace std; 3 #include "mystk 3. h" 4 //注意以下是 1999之後的 C++ 新寫法, 舊法使用 <iostream. h> 5 #include<iostream> 6 int main( ) { 7 My. Stack <int> x; // 注意這! 8 x. push(880); 9 x. push(770); 10 x. push(53); Stack contains a vector 11 while(!x. empty( ) ) { 12 cout << x. top(); x. pop(); 13 } 14 cout << endl; 15 } [tsaiwn@ccbsd 3] vector. STK> g++ mymain 3. cpp [tsaiwn@ccbsd 3] vector. STK>. /a. out 53770880 33 交通大學資訓 程學系 蔡文能
![用 list 做出 stack 12 tsaiwnccbsd 3 vector STK cat n mystk 5 h 用 list 做出 stack (1/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mystk 5. h](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-34.jpg)
用 list 做出 stack (1/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mystk 5. h 1 #include <list> 2 using namespace std; 3 template <class T> 4 class My. Stack { 5 list <T> x; 6 public: 7 void push(const T& y) { 8 x. push_front(y); 9 } Stack 10 T top( ) { 11 return x. front( ); 12 } 13 void pop( ) { 14 x. pop_front( ); 15 } 16 bool empty( ) { return x. begin() == x. end(); } 17 }; contains a list 34 交通大學資訓 程學系 蔡文能
![用 list 做出 stack 22 tsaiwnccbsd 3 vector STK cat n mymain 5 cpp 用 list 做出 stack (2/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mymain 5. cpp](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-35.jpg)
用 list 做出 stack (2/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mymain 5. cpp 1 // mymain 5. cpp; g++ mymain 5. cpp ; . /a. out 2 using namespace std; 3 #include "mystk 5. h" 4 //注意以下是 1999之後的 C++ 新寫法, 舊法使用 <iostream. h> 5 #include<iostream> 6 int main( ){ 7 My. Stack <int> x; // 注意這! 8 x. push(880); 9 x. push(770); Stack contains a 10 x. push(53); 11 while(!x. empty()){ 12 cout << x. top(); x. pop(); 13 } 14 cout << endl; 15 } [tsaiwn@ccbsd 3] vector. STK> g++ mymain 5. cpp [tsaiwn@ccbsd 3] vector. STK>. /a. out 53770880 交通大學資訓 程學系 蔡文能 list 35
![把 list 擴充成 stack 12 tsaiwnccbsd 3 vector STK cat n mystk 6 h 把 list 擴充成 stack (1/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mystk 6. h](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-36.jpg)
把 list 擴充成 stack (1/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mystk 6. h 1 #include <list> 2 using namespace std; Stack extends 3 template <class T> 4 class My. Stack: list<T> { 5 public: 6 void push(const T& y) { 7 My. Stack<T>: : push_front(y); 8 } 9 T top( ) { 10 return My. Stack<T>: : front( ); 11 } 12 void pop( ) { 13 My. Stack<T>: : pop_front( ); 14 } 15 bool empty( ) { 16 return My. Stack<T>: : begin() == My. Stack<T>: : end( ); 17 } 18 }; // class list 36 交通大學資訓 程學系 蔡文能
![把 list 擴充成 stack 22 tsaiwnccbsd 3 vector STK cat n mymain 6 cpp 把 list 擴充成 stack (2/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mymain 6. cpp](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-37.jpg)
把 list 擴充成 stack (2/2) [tsaiwn@ccbsd 3] vector. STK> cat -n mymain 6. cpp 1 // mymain 6. cpp; g++ mymain 6. cpp ; . /a. out 2 using namespace std; 3 #include "mystk 6. h" 4 //注意以下是 1999之後的 C++ 新寫法, 舊法使用 <iostream. h> 5 #include<iostream> 6 int main( ){ 7 My. Stack <int> x; // 注意這! 8 x. push(880); 9 x. push(770); Stack extends list 10 x. push(53); 11 while(!x. empty()){ 12 cout << x. top(); x. pop(); 13 } 14 cout << endl; 15 } [tsaiwn@ccbsd 3] vector. STK> g++ mymain 6. cpp [tsaiwn@ccbsd 3] vector. STK>. /a. out 53770880 交通大學資訓 程學系 蔡文能 37
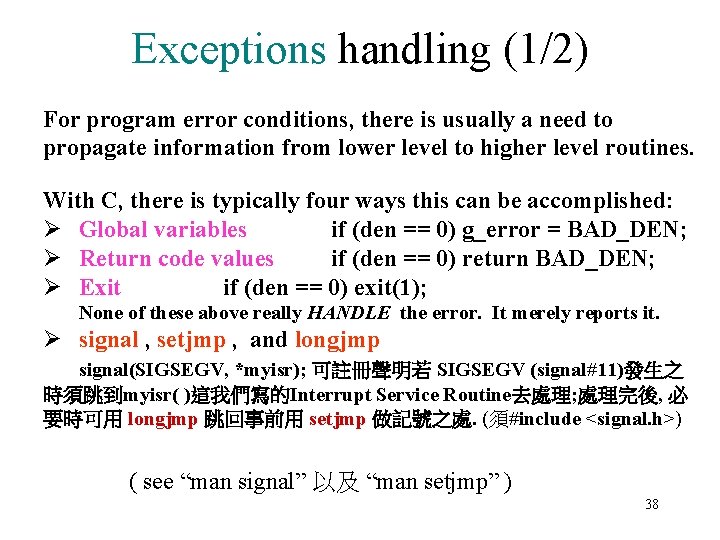
Exceptions handling (1/2) For program error conditions, there is usually a need to propagate information from lower level to higher level routines. With C, there is typically four ways this can be accomplished: Ø Global variables if (den == 0) g_error = BAD_DEN; Ø Return code values if (den == 0) return BAD_DEN; Ø Exit if (den == 0) exit(1); None of these above really HANDLE the error. It merely reports it. Ø signal , setjmp , and longjmp signal(SIGSEGV, *myisr); 可註冊聲明若 SIGSEGV (signal#11)發生之 時須跳到myisr( )這我們寫的Interrupt Service Routine去處理; 處理完後, 必 要時可用 longjmp 跳回事前用 setjmp 做記號之處. (須#include <signal. h>) ( see “man signal” 以及 “man setjmp” ) 38
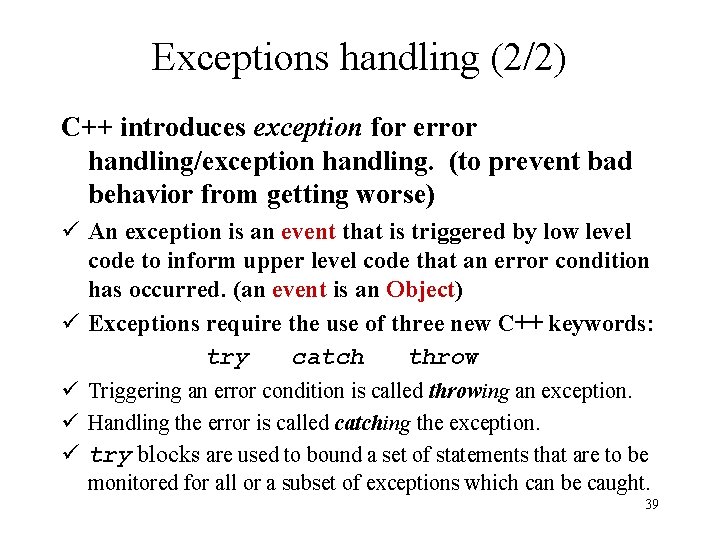
Exceptions handling (2/2) C++ introduces exception for error handling/exception handling. (to prevent bad behavior from getting worse) ü An exception is an event that is triggered by low level code to inform upper level code that an error condition has occurred. (an event is an Object) ü Exceptions require the use of three new C++ keywords: try catch throw ü Triggering an error condition is called throwing an exception. ü Handling the error is called catching the exception. ü try blocks are used to bound a set of statements that are to be monitored for all or a subset of exceptions which can be caught. 39
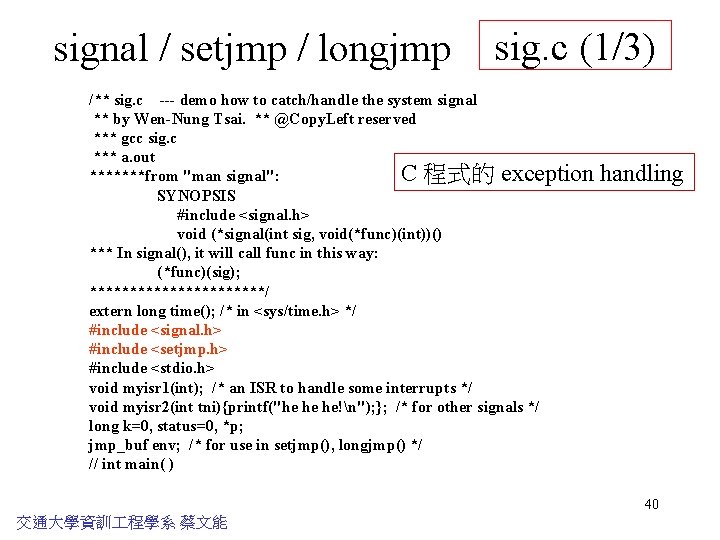
signal / setjmp / longjmp sig. c (1/3) /** sig. c --- demo how to catch/handle the system signal ** by Wen-Nung Tsai. ** @Copy. Left reserved *** gcc sig. c *** a. out C 程式的 exception *******from "man signal": SYNOPSIS #include <signal. h> void (*signal(int sig, void(*func)(int))() *** In signal(), it will call func in this way: (*func)(sig); ***********/ extern long time(); /* in <sys/time. h> */ #include <signal. h> #include <setjmp. h> #include <stdio. h> void myisr 1(int); /* an ISR to handle some interrupts */ void myisr 2(int tni){printf("he he he!n"); }; /* for other signals */ long k=0, status=0, *p; jmp_buf env; /* for use in setjmp(), longjmp() */ // int main( ) handling 40 交通大學資訓 程學系 蔡文能
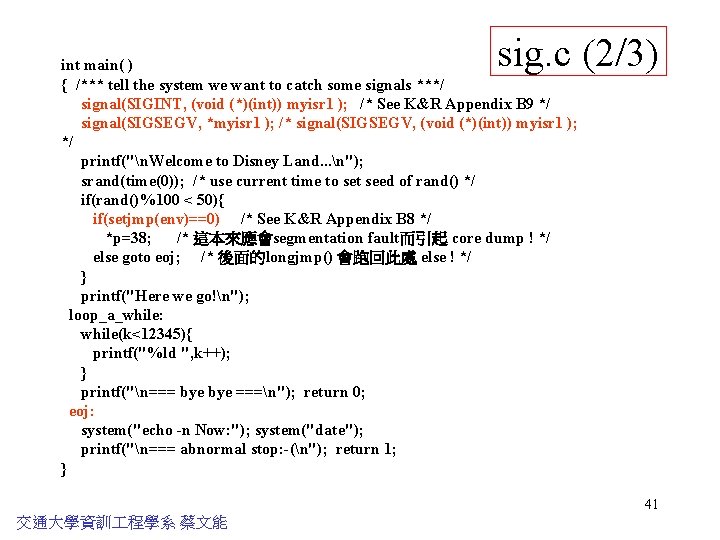
sig. c (2/3) int main( ) { /*** tell the system we want to catch some signals ***/ signal(SIGINT, (void (*)(int)) myisr 1 ); /* See K&R Appendix B 9 */ signal(SIGSEGV, *myisr 1 ); /* signal(SIGSEGV, (void (*)(int)) myisr 1 ); */ printf("n. Welcome to Disney Land. . . n"); srand(time(0)); /* use current time to set seed of rand() */ if(rand()%100 < 50){ if(setjmp(env)==0) /* See K&R Appendix B 8 */ *p=38; /* 這本來應會segmentation fault而引起 core dump ! */ else goto eoj; /* 後面的longjmp() 會跑回此處 else ! */ } printf("Here we go!n"); loop_a_while: while(k<12345){ printf("%ld ", k++); } printf("n=== bye ===n"); return 0; eoj: system("echo -n Now: "); system("date"); printf("n=== abnormal stop: -(n"); return 1; } 41 交通大學資訓 程學系 蔡文能
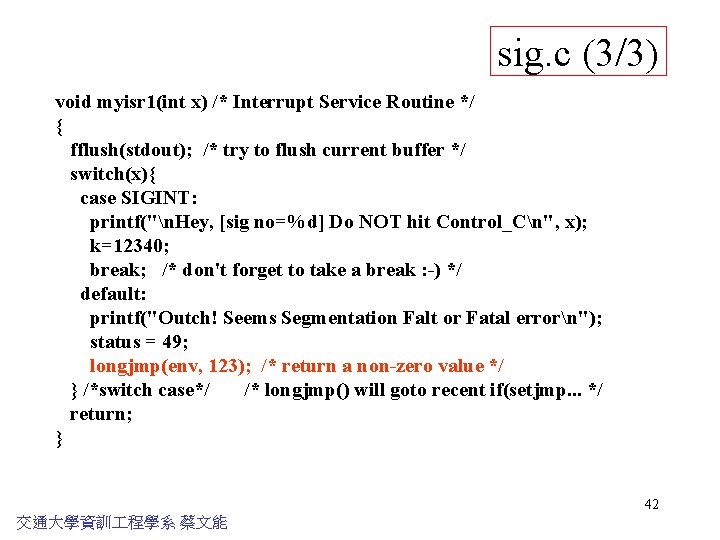
sig. c (3/3) void myisr 1(int x) /* Interrupt Service Routine */ { fflush(stdout); /* try to flush current buffer */ switch(x){ case SIGINT: printf("n. Hey, [sig no=%d] Do NOT hit Control_Cn", x); k=12340; break; /* don't forget to take a break : -) */ default: printf("Outch! Seems Segmentation Falt or Fatal errorn"); status = 49; longjmp(env, 123); /* return a non-zero value */ } /*switch case*/ /* longjmp() will goto recent if(setjmp. . . */ return; } 42 交通大學資訓 程學系 蔡文能
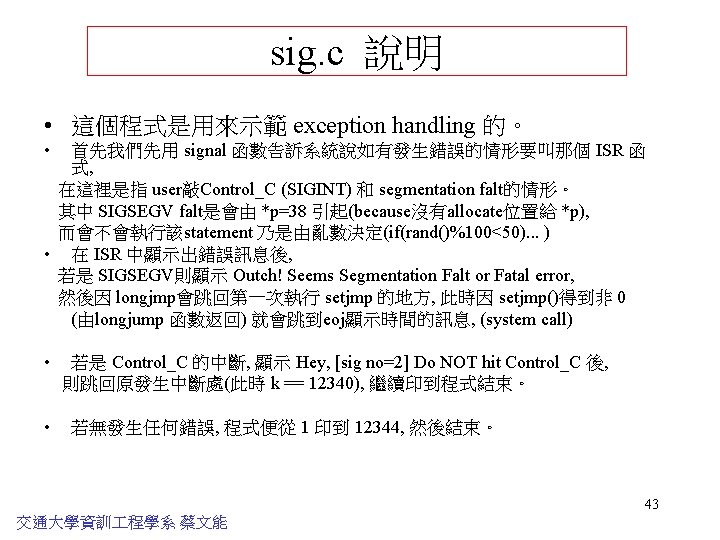
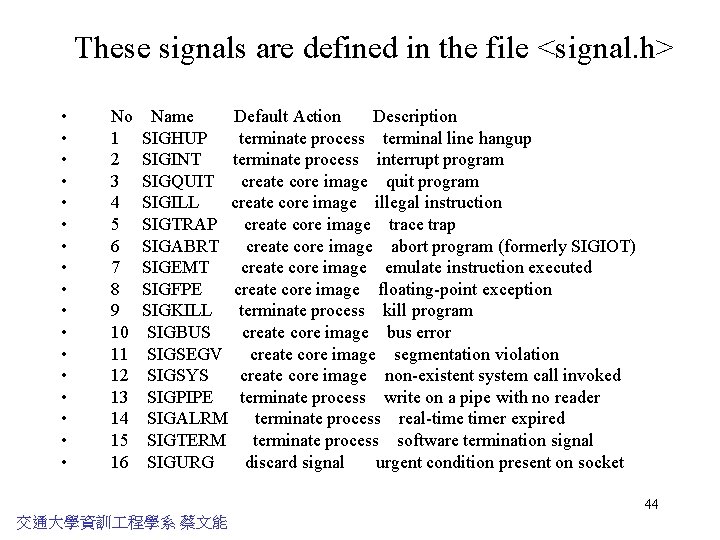
These signals are defined in the file <signal. h> • • • • • No 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 Name Default Action Description SIGHUP terminate process terminal line hangup SIGINT terminate process interrupt program SIGQUIT create core image quit program SIGILL create core image illegal instruction SIGTRAP create core image trace trap SIGABRT create core image abort program (formerly SIGIOT) SIGEMT create core image emulate instruction executed SIGFPE create core image floating-point exception SIGKILL terminate process kill program SIGBUS create core image bus error SIGSEGV create core image segmentation violation SIGSYS create core image non-existent system call invoked SIGPIPE terminate process write on a pipe with no reader SIGALRM terminate process real-timer expired SIGTERM terminate process software termination signal SIGURG discard signal urgent condition present on socket 44 交通大學資訓 程學系 蔡文能
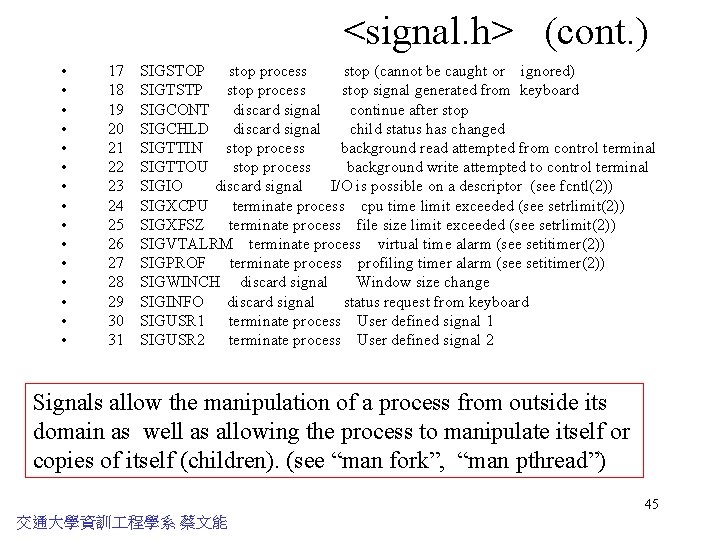
<signal. h> (cont. ) • • • • 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 SIGSTOP stop process stop (cannot be caught or ignored) SIGTSTP stop process stop signal generated from keyboard SIGCONT discard signal continue after stop SIGCHLD discard signal child status has changed SIGTTIN stop process background read attempted from control terminal SIGTTOU stop process background write attempted to control terminal SIGIO discard signal I/O is possible on a descriptor (see fcntl(2)) SIGXCPU terminate process cpu time limit exceeded (see setrlimit(2)) SIGXFSZ terminate process file size limit exceeded (see setrlimit(2)) SIGVTALRM terminate process virtual time alarm (see setitimer(2)) SIGPROF terminate process profiling timer alarm (see setitimer(2)) SIGWINCH discard signal Window size change SIGINFO discard signal status request from keyboard SIGUSR 1 terminate process User defined signal 1 SIGUSR 2 terminate process User defined signal 2 Signals allow the manipulation of a process from outside its domain as well as allowing the process to manipulate itself or copies of itself (children). (see “man fork”, “man pthread”) 45 交通大學資訓 程學系 蔡文能
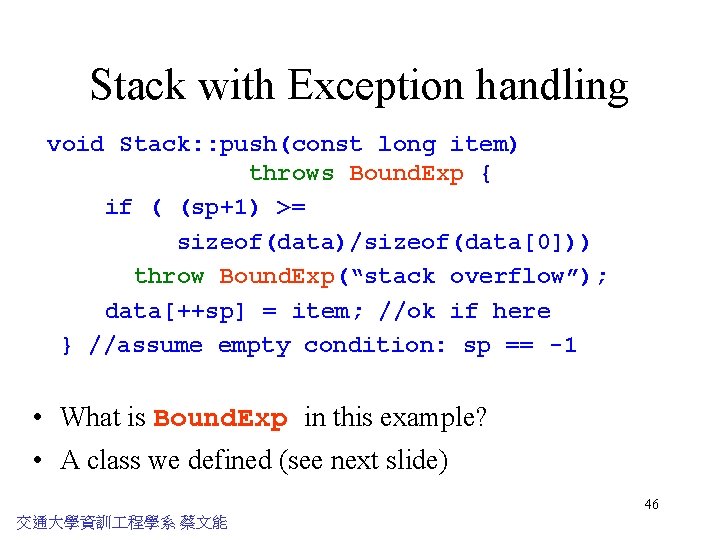
Stack with Exception handling void Stack: : push(const long item) throws Bound. Exp { if ( (sp+1) >= sizeof(data)/sizeof(data[0])) throw Bound. Exp(“stack overflow”); data[++sp] = item; //ok if here } //assume empty condition: sp == -1 • What is Bound. Exp in this example? • A class we defined (see next slide) 46 交通大學資訓 程學系 蔡文能
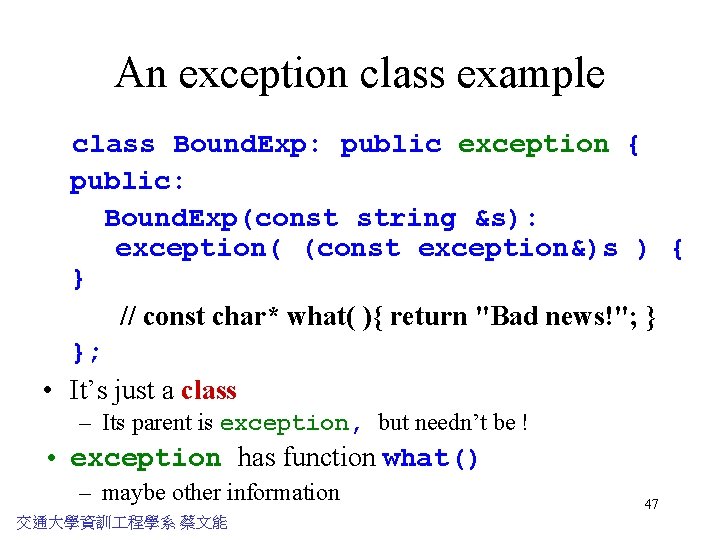
An exception class example class Bound. Exp: public exception { public: Bound. Exp(const string &s): exception( (const exception&)s ) { } // const char* what( ){ return "Bad news!"; } }; • It’s just a class – Its parent is exception, but needn’t be ! • exception has function what() – maybe other information 交通大學資訓 程學系 蔡文能 47
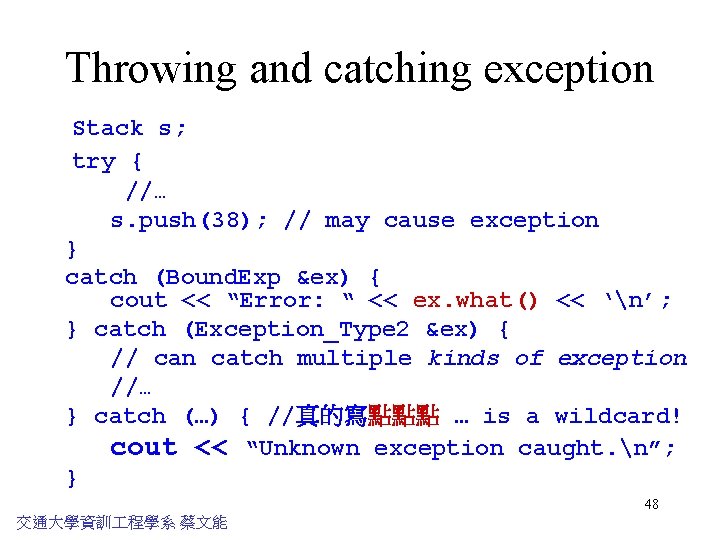
Throwing and catching exception Stack s; try { //… s. push(38); // may cause exception } catch (Bound. Exp &ex) { cout << “Error: “ << ex. what() << ‘n’; } catch (Exception_Type 2 &ex) { // can catch multiple kinds of exception //… } catch (…) { //真的寫點點點 … is a wildcard! cout << “Unknown exception caught. n”; } 48 交通大學資訓 程學系 蔡文能
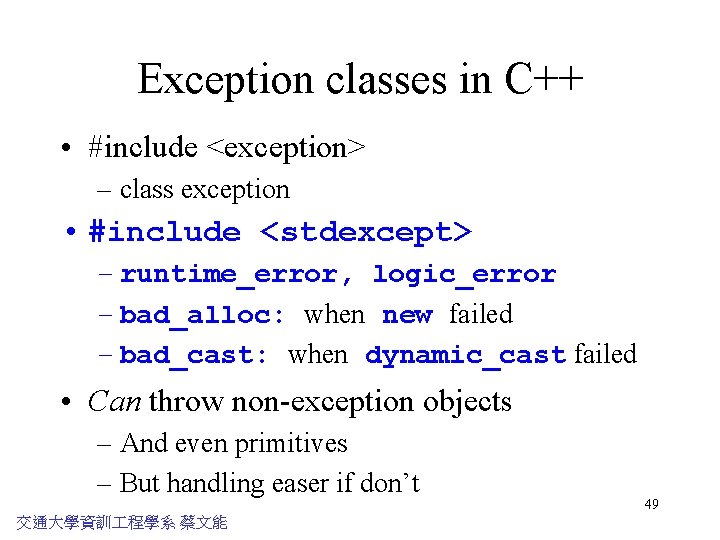
Exception classes in C++ • #include <exception> – class exception • #include <stdexcept> – runtime_error, logic_error – bad_alloc: when new failed – bad_cast: when dynamic_cast failed • Can throw non-exception objects – And even primitives – But handling easer if don’t 交通大學資訓 程學系 蔡文能 49
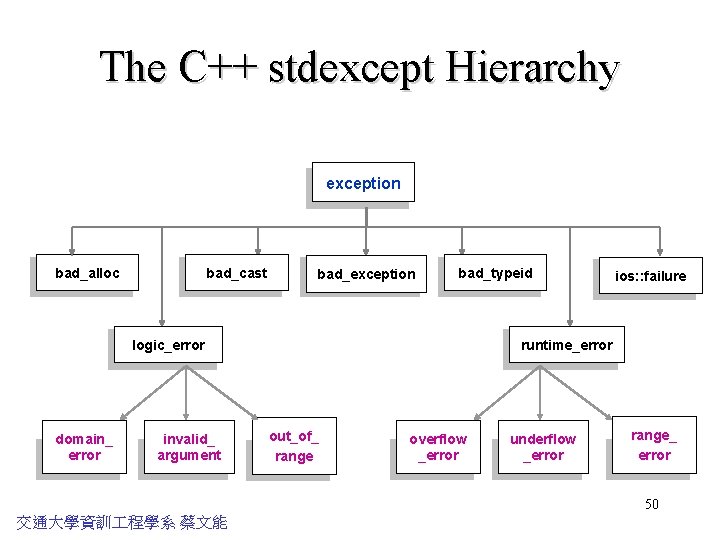
The C++ stdexcept Hierarchy exception bad_alloc bad_cast bad_exception bad_typeid logic_error domain_ error invalid_ argument ios: : failure runtime_error out_of_ range overflow _error underflow _error range_ error 50 交通大學資訓 程學系 蔡文能
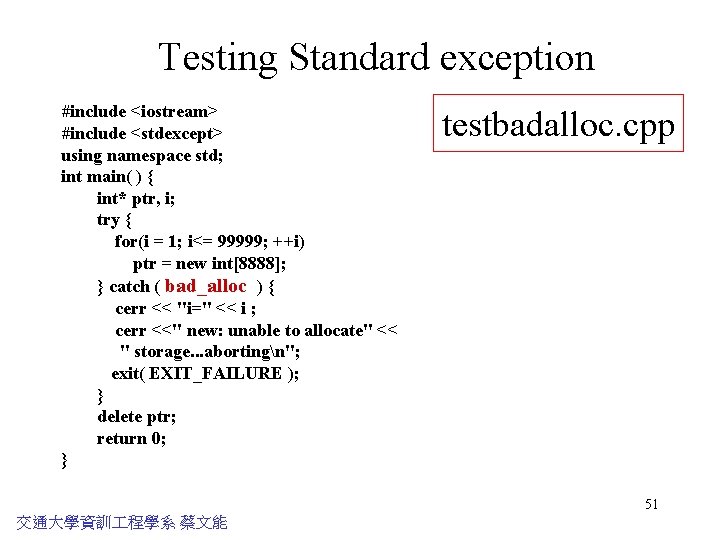
Testing Standard exception #include <iostream> #include <stdexcept> using namespace std; int main( ) { int* ptr, i; try { for(i = 1; i<= 99999; ++i) ptr = new int[8888]; } catch ( bad_alloc ) { cerr << "i=" << i ; cerr <<" new: unable to allocate" << " storage. . . abortingn"; exit( EXIT_FAILURE ); } delete ptr; return 0; } testbadalloc. cpp 51 交通大學資訓 程學系 蔡文能
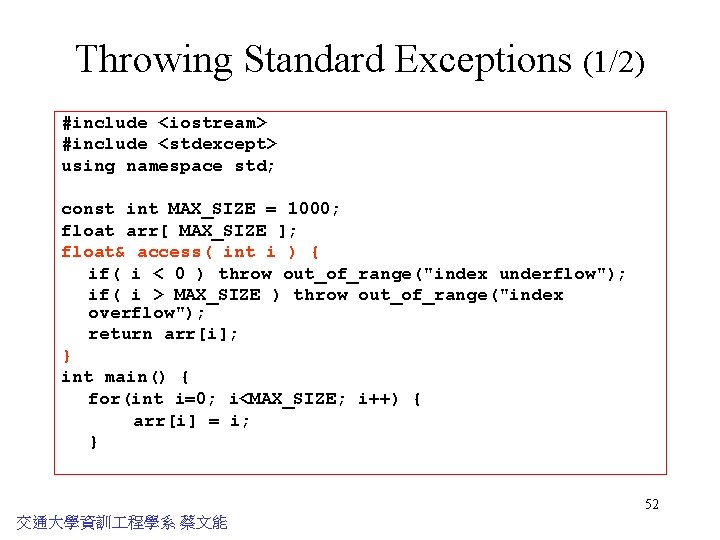
Throwing Standard Exceptions (1/2) #include <iostream> #include <stdexcept> using namespace std; const int MAX_SIZE = 1000; float arr[ MAX_SIZE ]; float& access( int i ) { if( i < 0 ) throw out_of_range("index underflow"); if( i > MAX_SIZE ) throw out_of_range("index overflow"); return arr[i]; } int main() { for(int i=0; i<MAX_SIZE; i++) { arr[i] = i; } 52 交通大學資訓 程學系 蔡文能
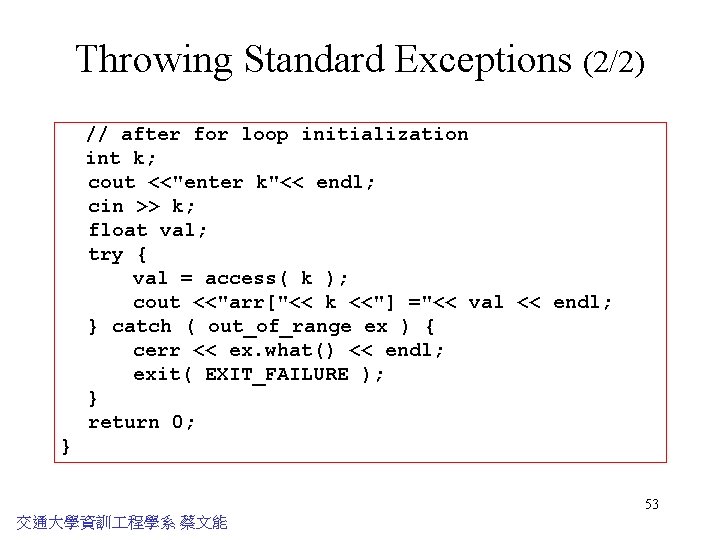
Throwing Standard Exceptions (2/2) // after for loop initialization int k; cout <<"enter k"<< endl; cin >> k; float val; try { val = access( k ); cout <<"arr["<< k <<"] ="<< val << endl; } catch ( out_of_range ex ) { cerr << ex. what() << endl; exit( EXIT_FAILURE ); } return 0; } 53 交通大學資訓 程學系 蔡文能
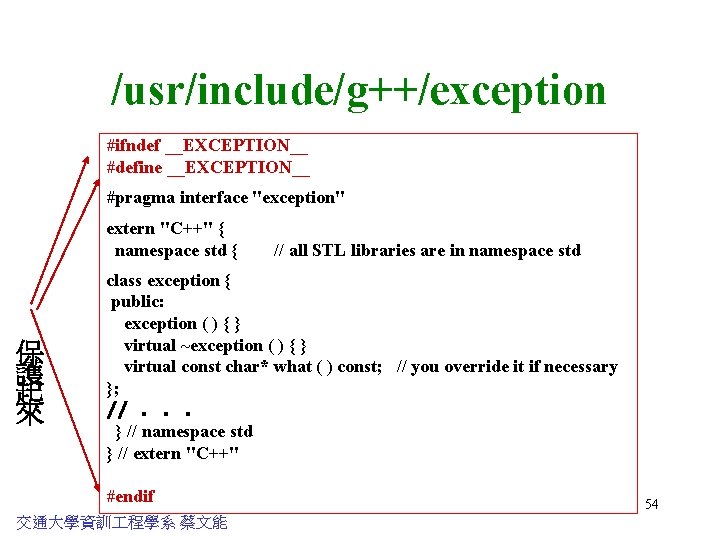
/usr/include/g++/exception #ifndef __EXCEPTION__ #define __EXCEPTION__ #pragma interface "exception" extern "C++" { namespace std { 保 護 起 來 // all STL libraries are in namespace std class exception { public: exception ( ) { } virtual ~exception ( ) { } virtual const char* what ( ) const; // you override it if necessary }; //. . . } // namespace std } // extern "C++" #endif 交通大學資訓 程學系 蔡文能 54
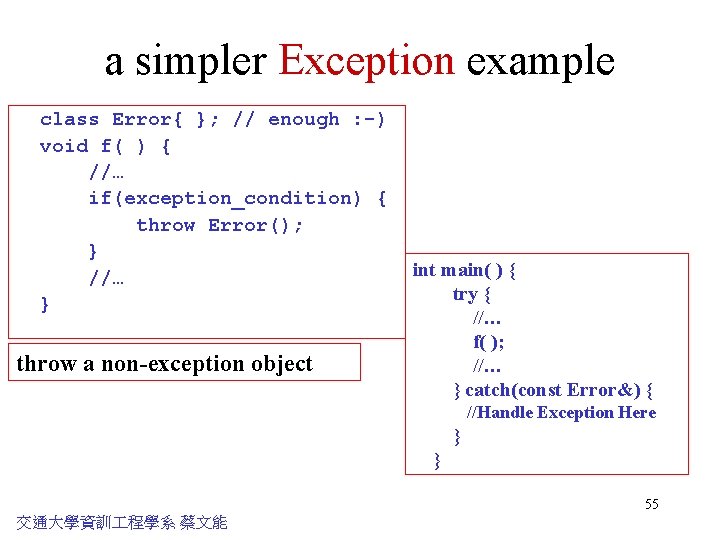
a simpler Exception example class Error{ }; // enough : -) void f( ) { //… if(exception_condition) { throw Error(); } //… } throw a non-exception object int main( ) { try { //… f( ); //… } catch(const Error&) { //Handle Exception Here } } 55 交通大學資訓 程學系 蔡文能
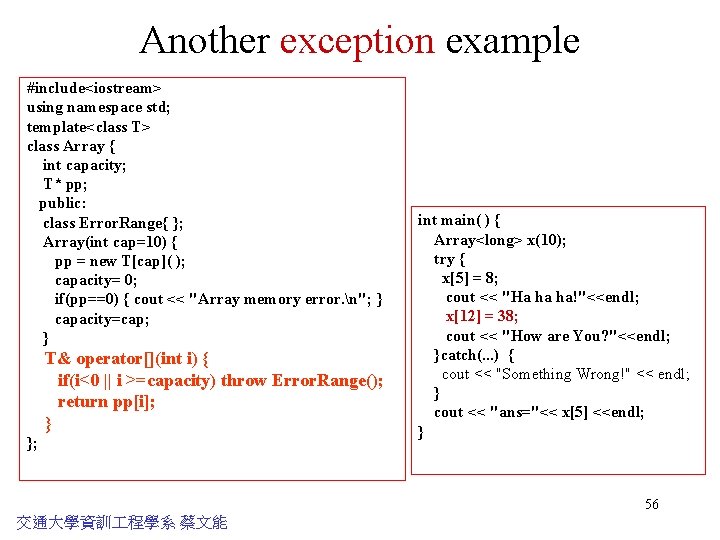
Another exception example #include<iostream> using namespace std; template<class T> class Array { int capacity; T* pp; public: class Error. Range{ }; Array(int cap=10) { pp = new T[cap]( ); capacity= 0; if(pp==0) { cout << "Array memory error. n"; } capacity=cap; } T& operator[](int i) { if(i<0 || i >=capacity) throw Error. Range(); return pp[i]; } }; int main( ) { Array<long> x(10); try { x[5] = 8; cout << "Ha ha ha!"<<endl; x[12] = 38; cout << "How are You? "<<endl; }catch(. . . ) { cout << "Something Wrong!" << endl; } cout << "ans="<< x[5] <<endl; } 56 交通大學資訓 程學系 蔡文能
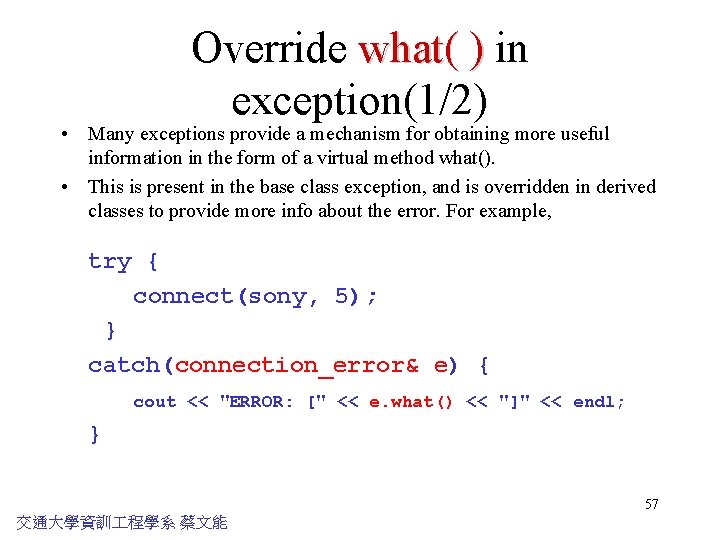
Override what( ) in exception(1/2) • Many exceptions provide a mechanism for obtaining more useful information in the form of a virtual method what(). • This is present in the base class exception, and is overridden in derived classes to provide more info about the error. For example, try { connect(sony, 5); } catch(connection_error& e) { cout << "ERROR: [" << e. what() << "]" << endl; } 57 交通大學資訓 程學系 蔡文能
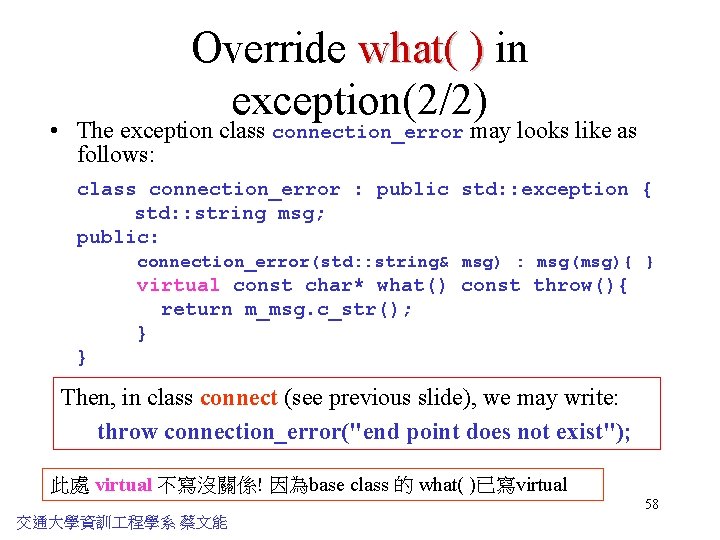
Override what( ) in exception(2/2) • The exception class connection_error may looks like as follows: class connection_error : public std: : exception { std: : string msg; public: connection_error(std: : string& msg) : msg(msg){ } virtual const char* what() const throw(){ return m_msg. c_str(); } } Then, in class connect (see previous slide), we may write: throw connection_error("end point does not exist"); 此處 virtual 不寫沒關係! 因為base class 的 what( )已寫virtual 58 交通大學資訓 程學系 蔡文能
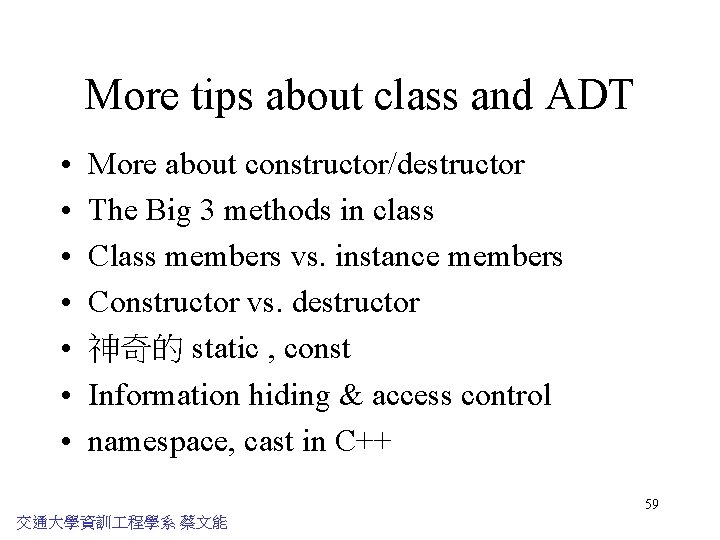
More tips about class and ADT • • More about constructor/destructor The Big 3 methods in class Class members vs. instance members Constructor vs. destructor 神奇的 static , const Information hiding & access control namespace, cast in C++ 59 交通大學資訓 程學系 蔡文能
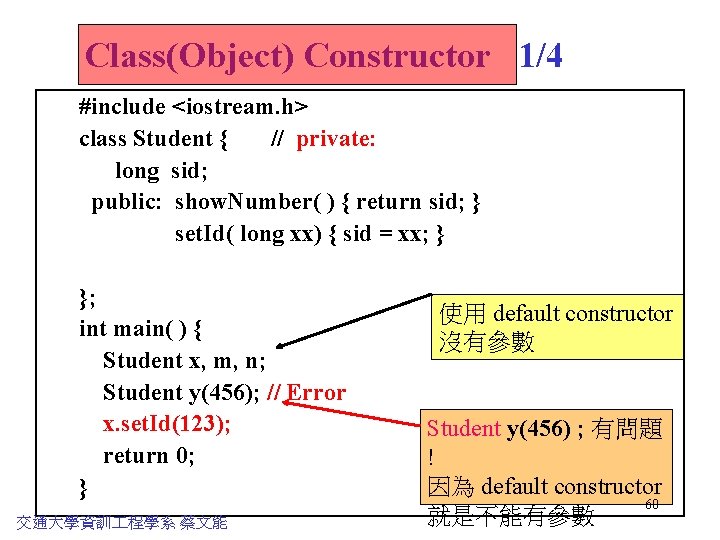
Class(Object) Constructor 1/4 #include <iostream. h> class Student { // private: long sid; public: show. Number( ) { return sid; } set. Id( long xx) { sid = xx; } }; int main( ) { Student x, m, n; Student y(456); // Error x. set. Id(123); return 0; } 交通大學資訓 程學系 蔡文能 使用 default constructor 沒有參數 Student y(456) ; 有問題 ! 因為 default constructor 60 就是不能有參數
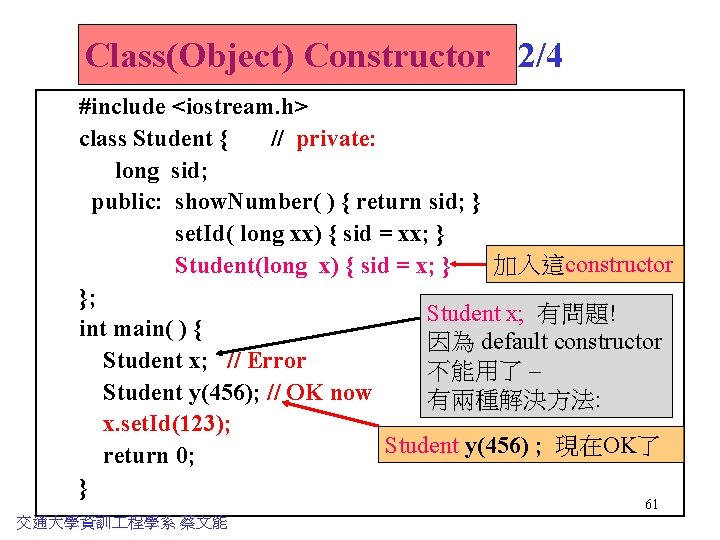
Class(Object) Constructor 2/4 #include <iostream. h> class Student { // private: long sid; public: show. Number( ) { return sid; } set. Id( long xx) { sid = xx; } 加入這constructor Student(long x) { sid = x; } }; Student x; 有問題! int main( ) { 因為 default constructor Student x; // Error 不能用了 – Student y(456); // OK now 有兩種解決方法: x. set. Id(123); Student y(456) ; 現在OK了 return 0; } 61 交通大學資訓 程學系 蔡文能
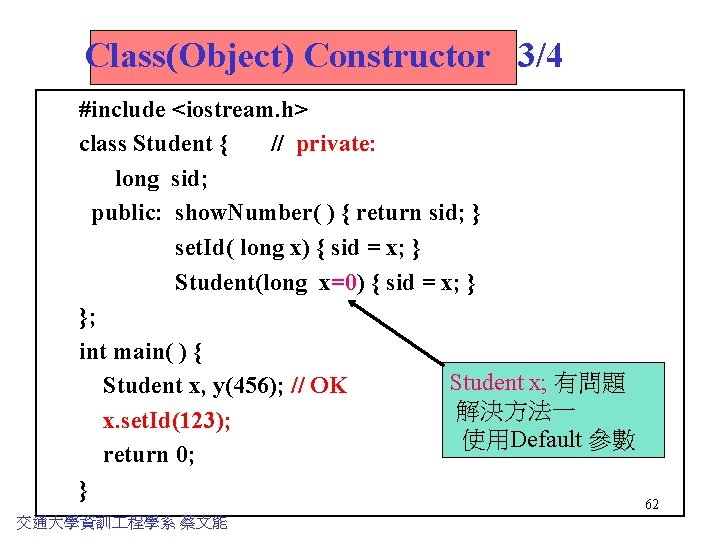
Class(Object) Constructor 3/4 #include <iostream. h> class Student { // private: long sid; public: show. Number( ) { return sid; } set. Id( long x) { sid = x; } Student(long x=0) { sid = x; } }; int main( ) { Student x; 有問題 Student x, y(456); // OK 解決方法一 x. set. Id(123); 使用Default 參數 return 0; } 交通大學資訓 程學系 蔡文能 62
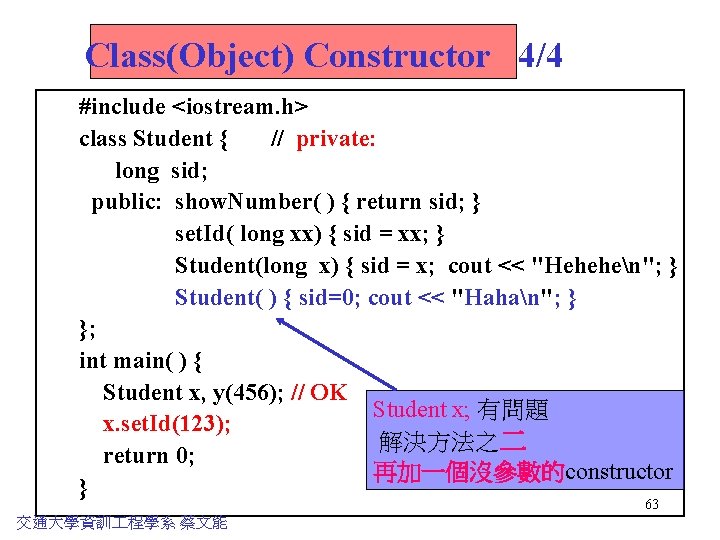
Class(Object) Constructor 4/4 #include <iostream. h> class Student { // private: long sid; public: show. Number( ) { return sid; } set. Id( long xx) { sid = xx; } Student(long x) { sid = x; cout << "Hehehen"; } Student( ) { sid=0; cout << "Hahan"; } }; int main( ) { Student x, y(456); // OK Student x; 有問題 x. set. Id(123); 解決方法之二 return 0; 再加一個沒參數的constructor } 63 交通大學資訓 程學系 蔡文能
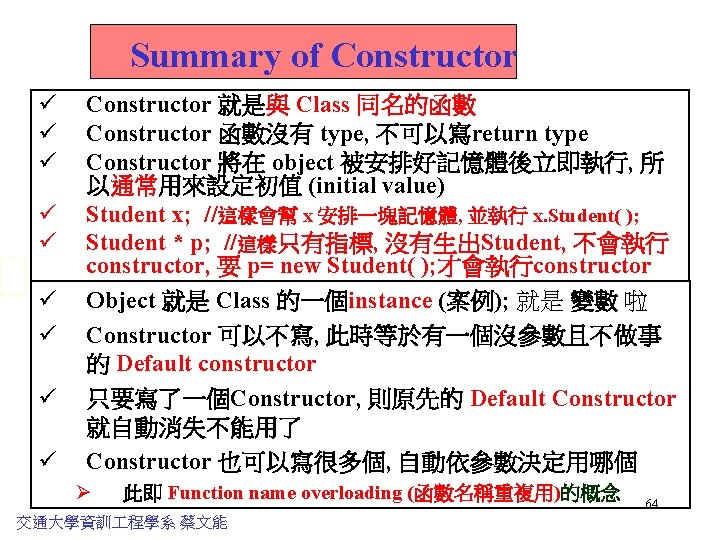
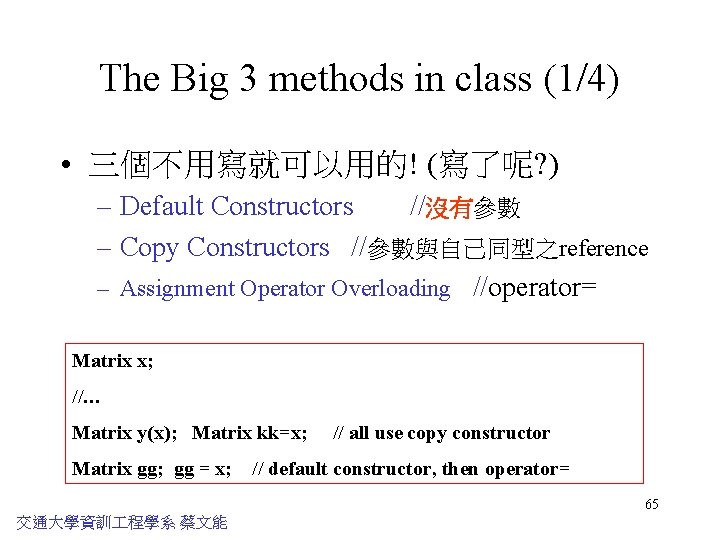
The Big 3 methods in class (1/4) • 三個不用寫就可以用的! (寫了呢? ) – Default Constructors //沒有參數 – Copy Constructors //參數與自己同型之reference – Assignment Operator Overloading //operator= Matrix x; //… Matrix y(x); Matrix kk=x; Matrix gg; gg = x; // all use copy constructor // default constructor, then operator= 65 交通大學資訓 程學系 蔡文能
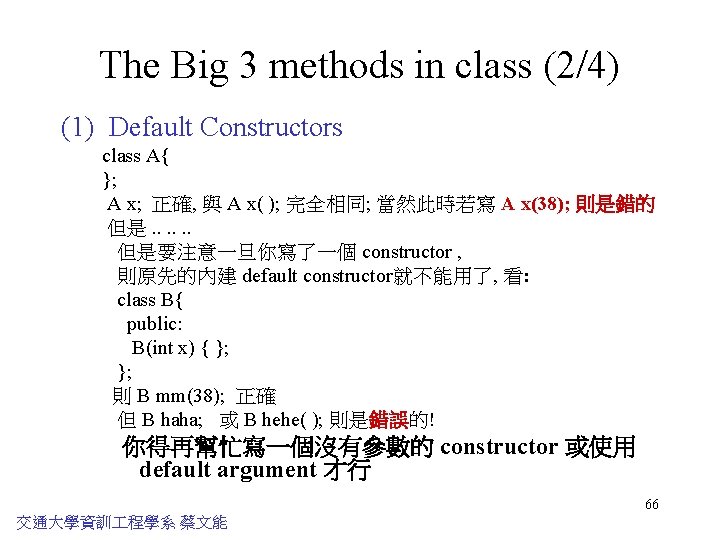
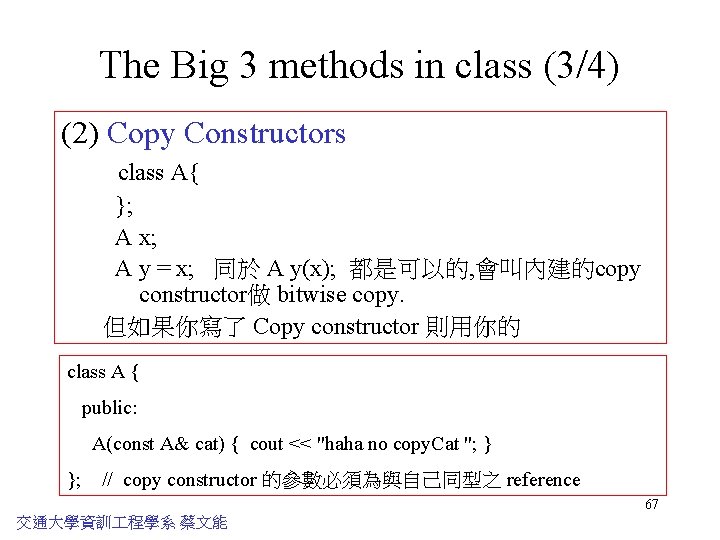
The Big 3 methods in class (3/4) (2) Copy Constructors class A{ }; A x; A y = x; 同於 A y(x); 都是可以的, 會叫內建的copy constructor做 bitwise copy. 但如果你寫了 Copy constructor 則用你的 class A { public: A(const A& cat) { cout << "haha no copy. Cat "; } }; // copy constructor 的參數必須為與自己同型之 reference 67 交通大學資訓 程學系 蔡文能
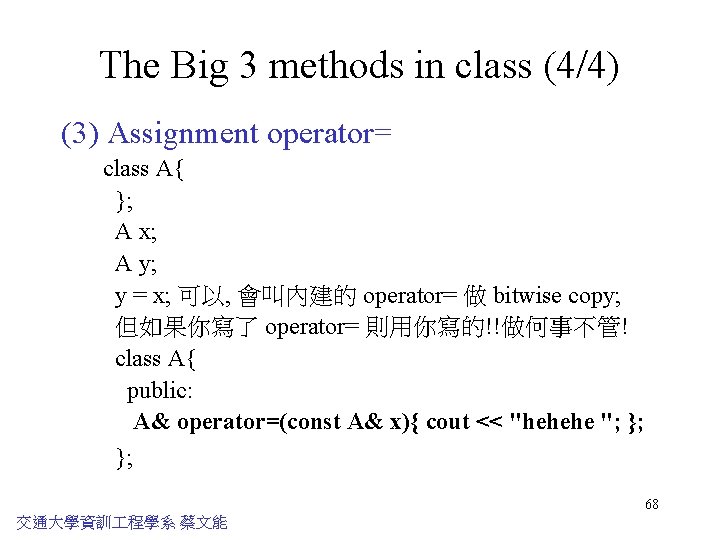
The Big 3 methods in class (4/4) (3) Assignment operator= class A{ }; A x; A y; y = x; 可以, 會叫內建的 operator= 做 bitwise copy; 但如果你寫了 operator= 則用你寫的!!做何事不管! class A{ public: A& operator=(const A& x){ cout << "hehehe "; }; }; 68 交通大學資訓 程學系 蔡文能
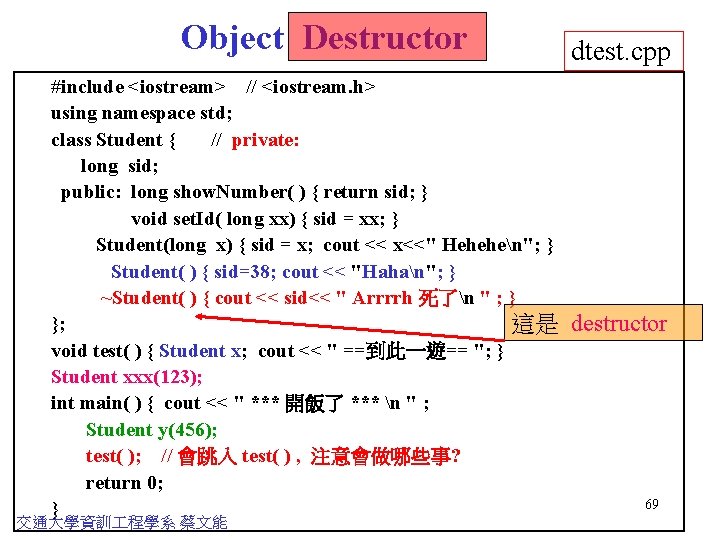
Object Destructor #include <iostream> // <iostream. h> using namespace std; class Student { // private: long sid; public: long show. Number( ) { return sid; } void set. Id( long xx) { sid = xx; } Student(long x) { sid = x; cout << x<<" Hehehen"; } Student( ) { sid=38; cout << "Hahan"; } ~Student( ) { cout << sid<< " Arrrrh 死了n " ; } }; 這是 void test( ) { Student x; cout << " ==到此一遊== "; } Student xxx(123); int main( ) { cout << " *** 開飯了 *** n " ; Student y(456); test( ); // 會跳入 test( ) , 注意會做哪些事? return 0; } 交通大學資訓 程學系 蔡文能 dtest. cpp destructor 69
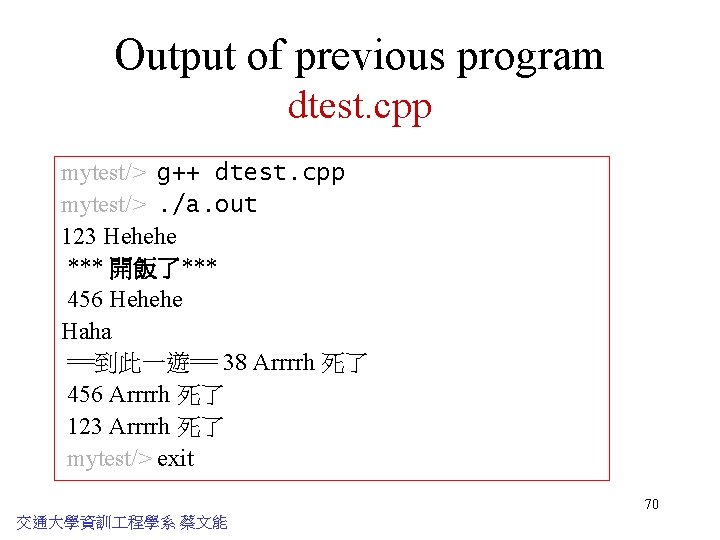
Output of previous program dtest. cpp mytest/> g++ dtest. cpp mytest/>. /a. out 123 Hehehe *** 開飯了*** 456 Hehehe Haha ==到此一遊== 38 Arrrrh 死了 456 Arrrrh 死了 123 Arrrrh 死了 mytest/> exit 70 交通大學資訓 程學系 蔡文能
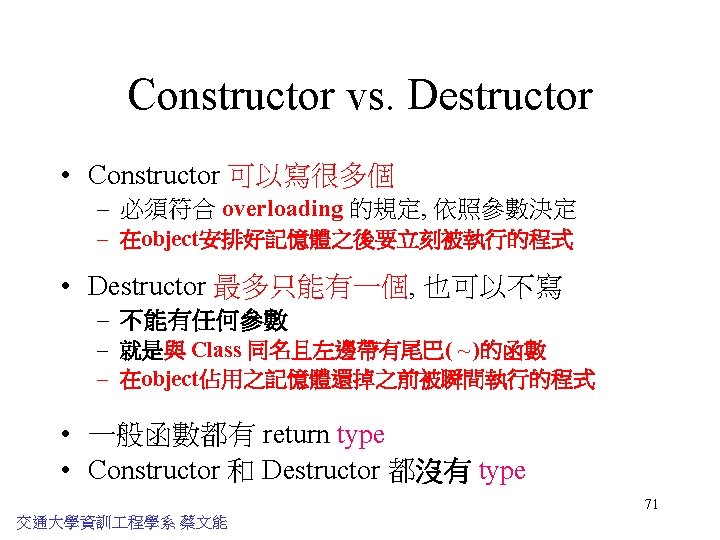
![神奇的 static ü On a global variable or a function static long my Data38 神奇的 “static” ü On a global variable or a function static long my. Data[38];](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-72.jpg)
神奇的 “static” ü On a global variable or a function static long my. Data[38]; //information hiding static void my. Function(float); – Tells the linker not to export the variable or function. – Makes the identifier “file scope, ” as the linker will not use it fulfill dependencies from other files. ü On a local variable in a function void some. Func(void) { static int array[4000]; } Places the variable off the stack. This has the side-effect that it retains it value across calls. It is often used when a variable is too large to be put on the stack. (auto變數則在 stack) ü On a class member data or member function (next slide) 72 交通大學資訓 程學系 蔡文能
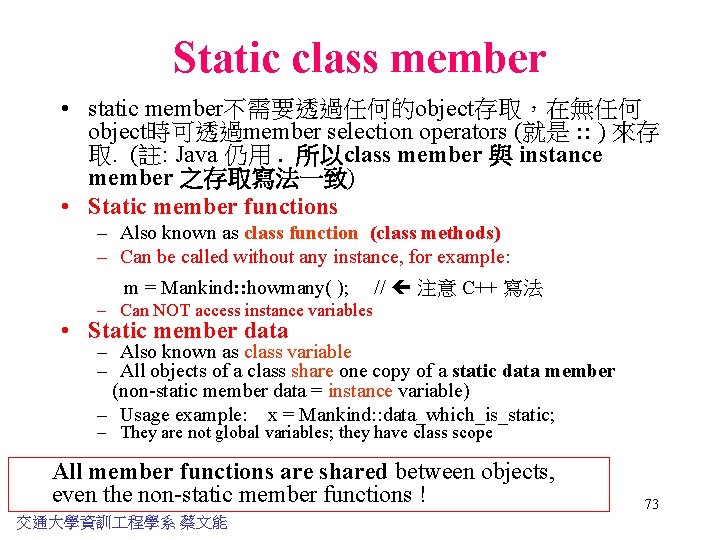
Static class member • static member不需要透過任何的object存取,在無任何 object時可透過member selection operators (就是 : : ) 來存 取. (註: Java 仍用. 所以class member 與 instance member 之存取寫法一致) • Static member functions – Also known as class function (class methods) – Can be called without any instance, for example: m = Mankind: : howmany( ); // 注意 C++ 寫法 – Can NOT access instance variables • Static member data – Also known as class variable – All objects of a class share one copy of a static data member (non-static member data = instance variable) – Usage example: x = Mankind: : data_which_is_static; – They are not global variables; they have class scope All member functions are shared between objects, even the non-static member functions ! 交通大學資訓 程學系 蔡文能 73
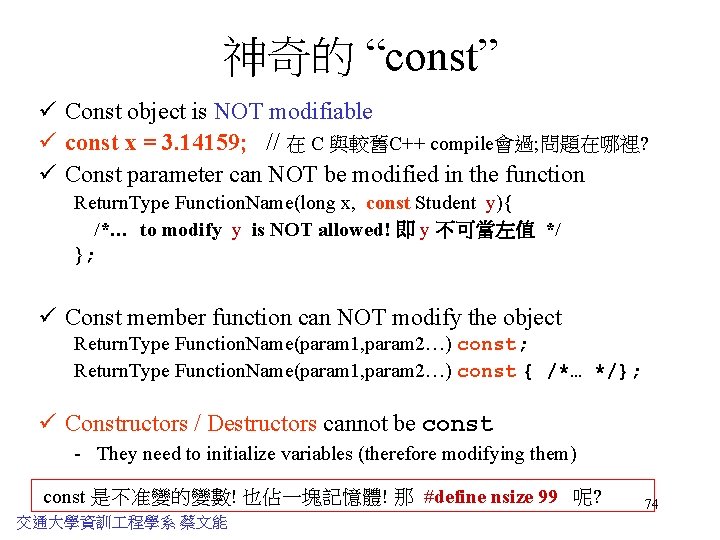
神奇的 “const” ü Const object is NOT modifiable ü const x = 3. 14159; // 在 C 與較舊C++ compile會過; 問題在哪裡? ü Const parameter can NOT be modified in the function Return. Type Function. Name(long x, const Student y){ /*… to modify y is NOT allowed! 即 y 不可當左值 */ }; ü Const member function can NOT modify the object Return. Type Function. Name(param 1, param 2…) const; Return. Type Function. Name(param 1, param 2…) const { /*… */}; ü Constructors / Destructors cannot be const - They need to initialize variables (therefore modifying them) const 是不准變的變數! 也佔一塊記憶體! 那 #define nsize 99 呢? 交通大學資訓 程學系 蔡文能 74
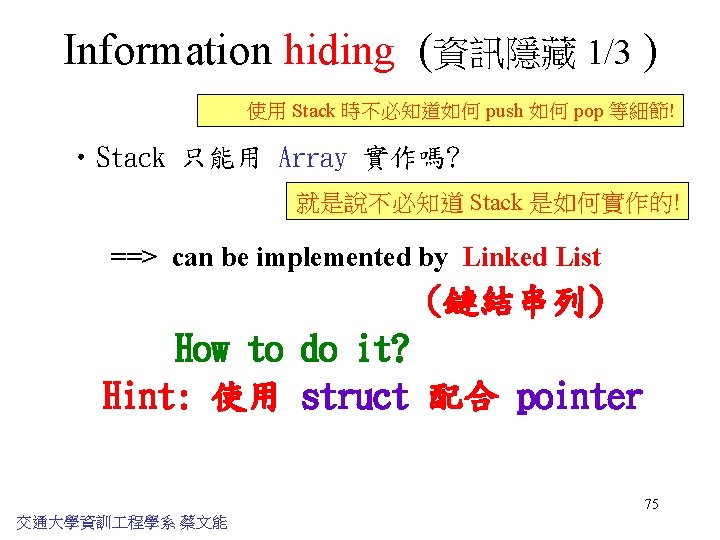
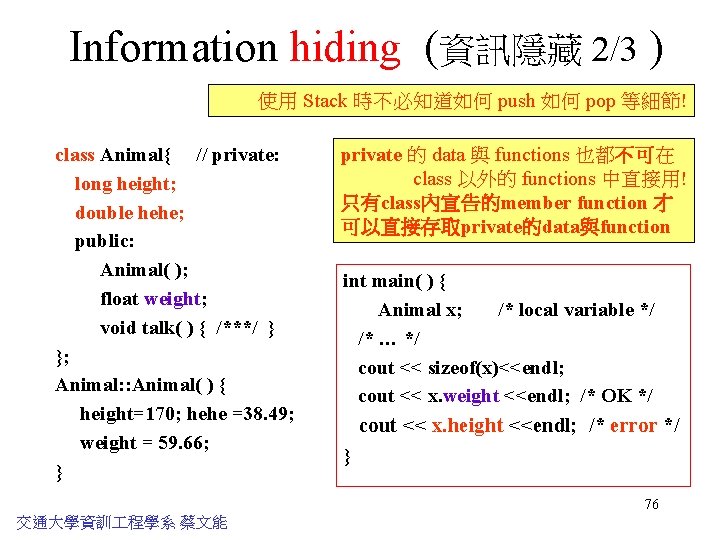
Information hiding (資訊隱藏 2/3 ) 使用 Stack 時不必知道如何 push 如何 pop 等細節! class Animal{ // private: long height; double hehe; public: Animal( ); float weight; void talk( ) { /***/ } }; Animal: : Animal( ) { height=170; hehe =38. 49; weight = 59. 66; } private 的 data 與 functions 也都不可在 class 以外的 functions 中直接用! 只有class內宣告的member function 才 可以直接存取private的data與function int main( ) { Animal x; /* local variable */ /* … */ cout << sizeof(x)<<endl; cout << x. weight <<endl; /* OK */ cout << x. height <<endl; /* error */ } 76 交通大學資訓 程學系 蔡文能
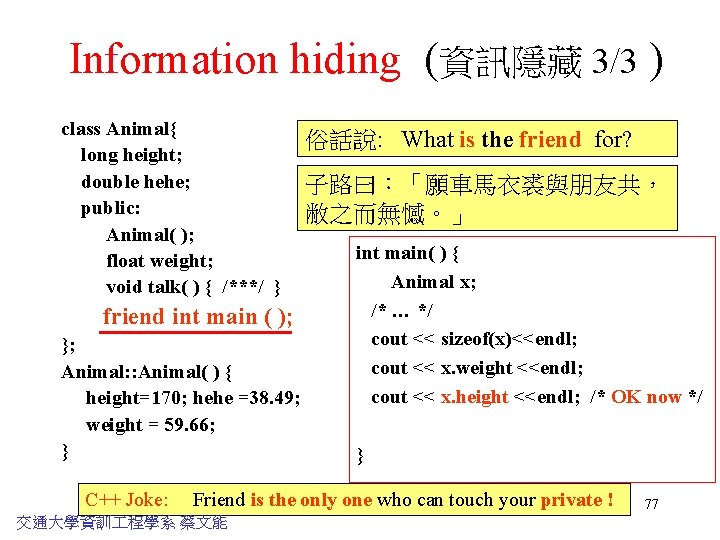
Information hiding (資訊隱藏 3/3 ) class Animal{ long height; double hehe; public: Animal( ); float weight; void talk( ) { /***/ } friend int main ( ); }; Animal: : Animal( ) { height=170; hehe =38. 49; weight = 59. 66; } C++ Joke: 俗話說: What is the friend for? 子路曰:「願車馬衣裘與朋友共, 敝之而無憾。」 int main( ) { Animal x; /* … */ cout << sizeof(x)<<endl; cout << x. weight <<endl; cout << x. height <<endl; /* OK now */ } Friend is the only one who can touch your private ! 交通大學資訓 程學系 蔡文能 77
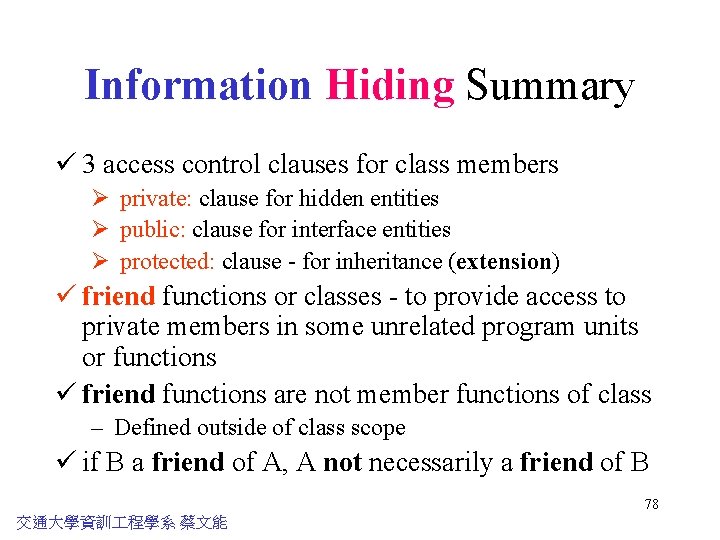
Information Hiding Summary ü 3 access control clauses for class members Ø private: clause for hidden entities Ø public: clause for interface entities Ø protected: clause - for inheritance (extension) ü friend functions or classes - to provide access to private members in some unrelated program units or functions ü friend functions are not member functions of class – Defined outside of class scope ü if B a friend of A, A not necessarily a friend of B 78 交通大學資訓 程學系 蔡文能
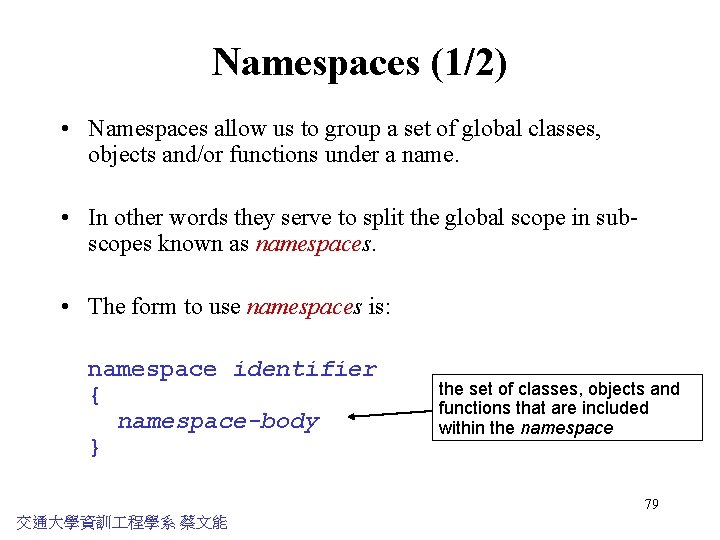
Namespaces (1/2) • Namespaces allow us to group a set of global classes, objects and/or functions under a name. • In other words they serve to split the global scope in subscopes known as namespaces. • The form to use namespaces is: namespace identifier { namespace-body } the set of classes, objects and functions that are included within the namespace 79 交通大學資訓 程學系 蔡文能
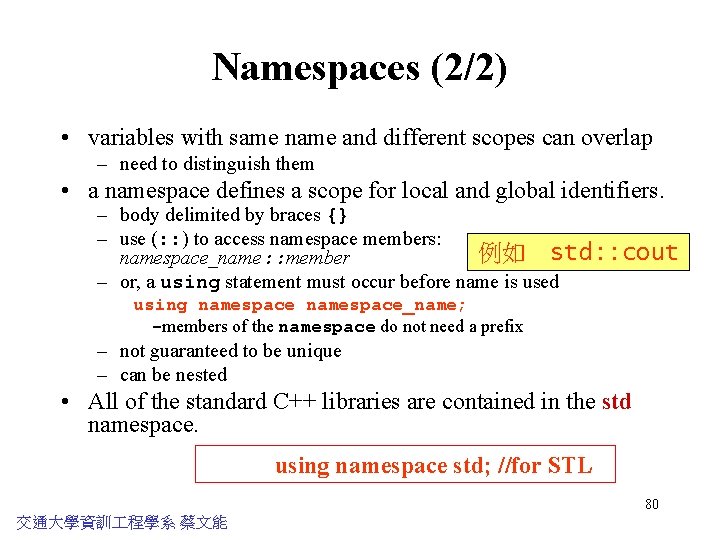
Namespaces (2/2) • variables with same name and different scopes can overlap – need to distinguish them • a namespace defines a scope for local and global identifiers. – body delimited by braces {} – use (: : ) to access namespace members: 例如 std: : cout namespace_name: : member – or, a using statement must occur before name is used using namespace_name; -members of the namespace do not need a prefix – not guaranteed to be unique – can be nested • All of the standard C++ libraries are contained in the std namespace. using namespace std; //for STL 80 交通大學資訓 程學系 蔡文能
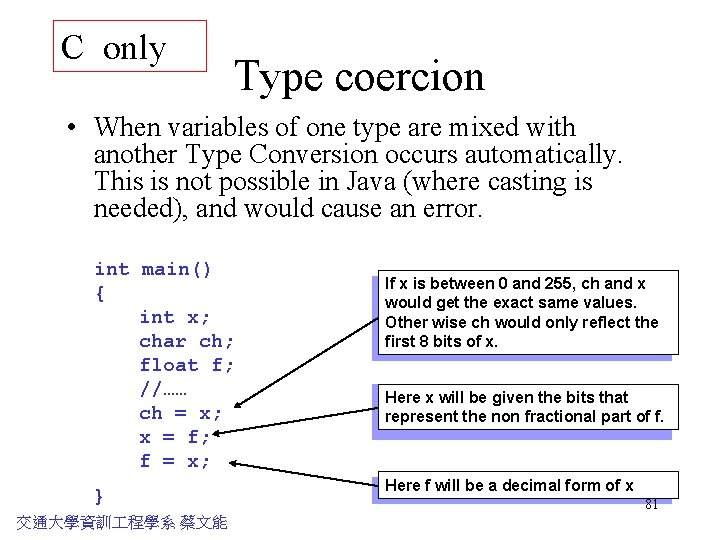
C only Type coercion • When variables of one type are mixed with another Type Conversion occurs automatically. This is not possible in Java (where casting is needed), and would cause an error. int main() { int x; char ch; float f; //…… ch = x; x = f; f = x; } 交通大學資訓 程學系 蔡文能 If x is between 0 and 255, ch and x would get the exact same values. Other wise ch would only reflect the first 8 bits of x. Here x will be given the bits that represent the non fractional part of f. Here f will be a decimal form of x 81
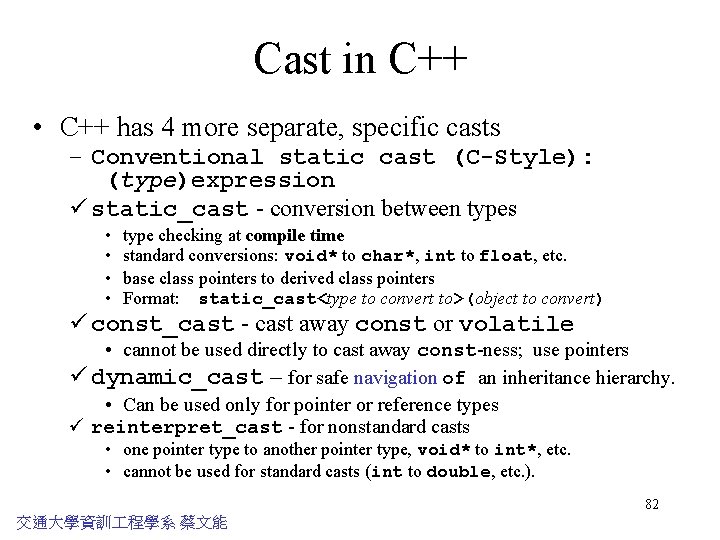
Cast in C++ • C++ has 4 more separate, specific casts – Conventional static cast (C-Style): (type)expression ü static_cast - conversion between types • • type checking at compile time standard conversions: void* to char*, int to float, etc. base class pointers to derived class pointers Format: static_cast<type to convert to>(object to convert) ü const_cast - cast away const or volatile • cannot be used directly to cast away const-ness; use pointers ü dynamic_cast – for safe navigation of an inheritance hierarchy. • Can be used only for pointer or reference types ü reinterpret_cast - for nonstandard casts • one pointer type to another pointer type, void* to int*, etc. • cannot be used for standard casts (int to double, etc. ). 82 交通大學資訓 程學系 蔡文能
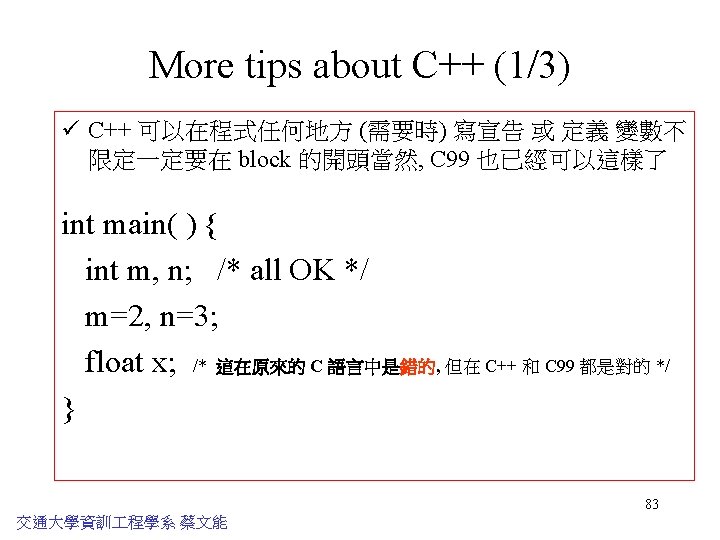
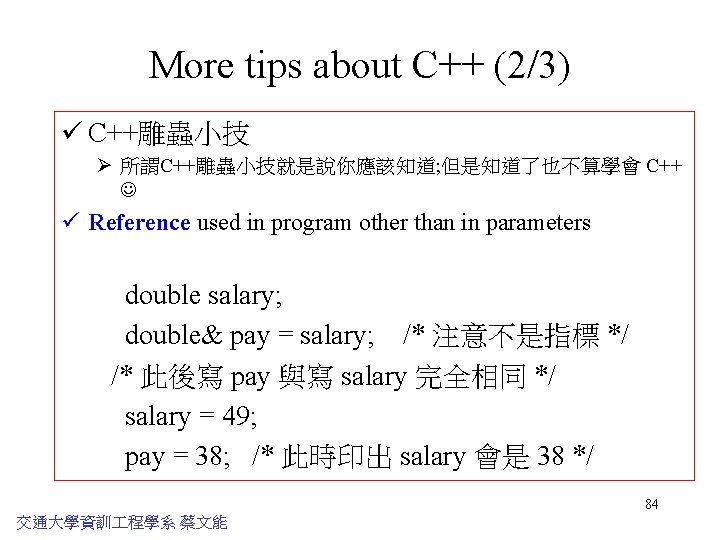
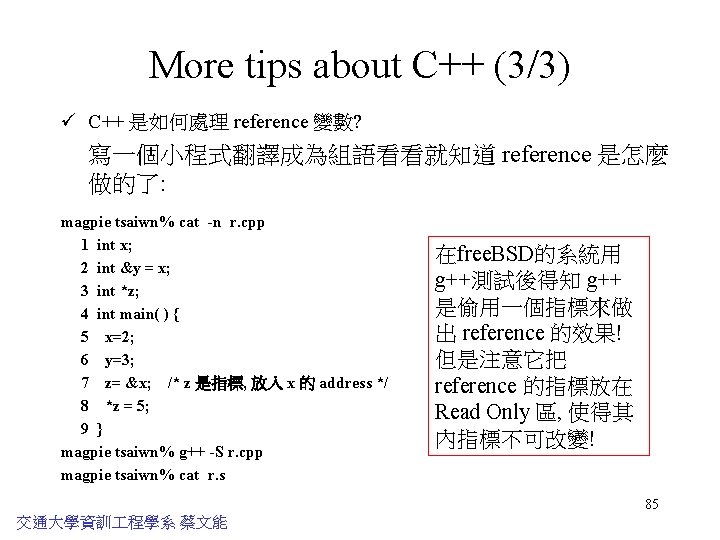
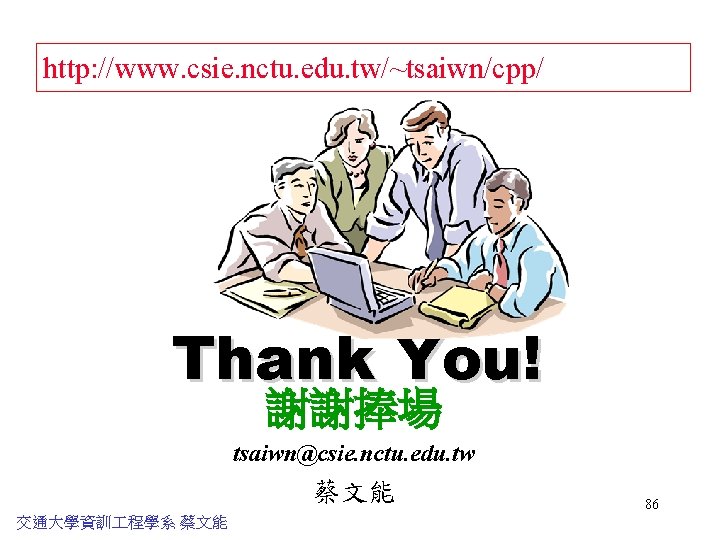
http: //www. csie. nctu. edu. tw/~tsaiwn/cpp/ Thank You! 謝謝捧場 tsaiwn@csie. nctu. edu. tw 蔡文能 交通大學資訓 程學系 蔡文能 86
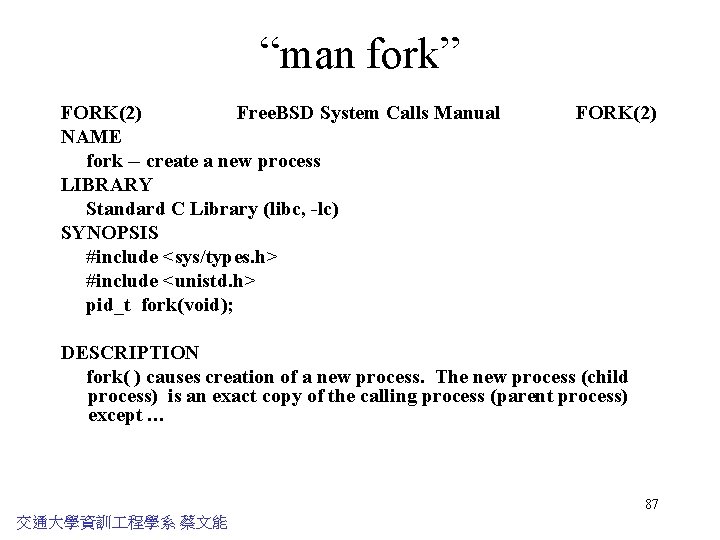
“man fork” FORK(2) Free. BSD System Calls Manual NAME fork -- create a new process LIBRARY Standard C Library (libc, -lc) SYNOPSIS #include <sys/types. h> #include <unistd. h> pid_t fork(void); FORK(2) DESCRIPTION fork( ) causes creation of a new process. The new process (child process) is an exact copy of the calling process (parent process) except … 87 交通大學資訓 程學系 蔡文能
![fork example switchpidfork case 1 syslogFork failed freenchunk closenull closefd0 fork( ) example switch(pid=fork( ) ) { case -1: syslog("Fork failed"); free(nchunk); close(null); close(fd[0]);](https://slidetodoc.com/presentation_image_h/85d256c0a41ee43f24cefa965d565a1a/image-88.jpg)
fork( ) example switch(pid=fork( ) ) { case -1: syslog("Fork failed"); free(nchunk); close(null); close(fd[0]); close(fd[1]); return NULL; case 0: /* child */ signal(SIG_TSTP, SIG_IGN); /* Stop TSTP */ close(fd[0]); close(0); close(1); close(2); dup 2(null, 0); dup 2(fd[1], 1); dup 2(null, 2); exec("/usr/bin/ps", "ax"); exit(0); /* Should never get here */ default: /* original parent process */ close(fd[1]); close(0); break; /* … */ 88 交通大學資訓 程學系 蔡文能
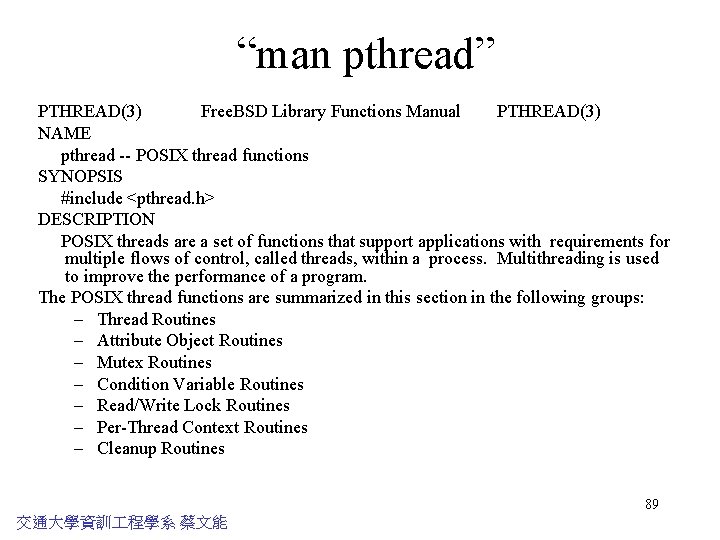
“man pthread” PTHREAD(3) Free. BSD Library Functions Manual PTHREAD(3) NAME pthread -- POSIX thread functions SYNOPSIS #include <pthread. h> DESCRIPTION POSIX threads are a set of functions that support applications with requirements for multiple flows of control, called threads, within a process. Multithreading is used to improve the performance of a program. The POSIX thread functions are summarized in this section in the following groups: – Thread Routines – Attribute Object Routines – Mutex Routines – Condition Variable Routines – Read/Write Lock Routines – Per-Thread Context Routines – Cleanup Routines 89 交通大學資訓 程學系 蔡文能
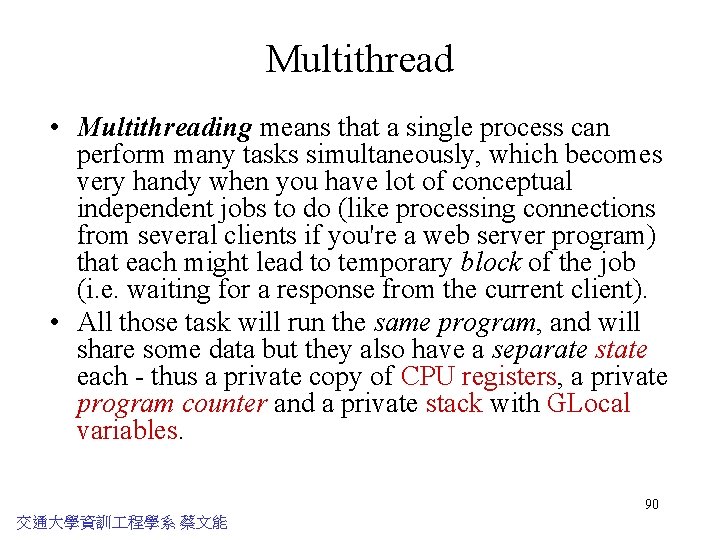
Multithread • Multithreading means that a single process can perform many tasks simultaneously, which becomes very handy when you have lot of conceptual independent jobs to do (like processing connections from several clients if you're a web server program) that each might lead to temporary block of the job (i. e. waiting for a response from the current client). • All those task will run the same program, and will share some data but they also have a separate state each - thus a private copy of CPU registers, a private program counter and a private stack with GLocal variables. 90 交通大學資訓 程學系 蔡文能
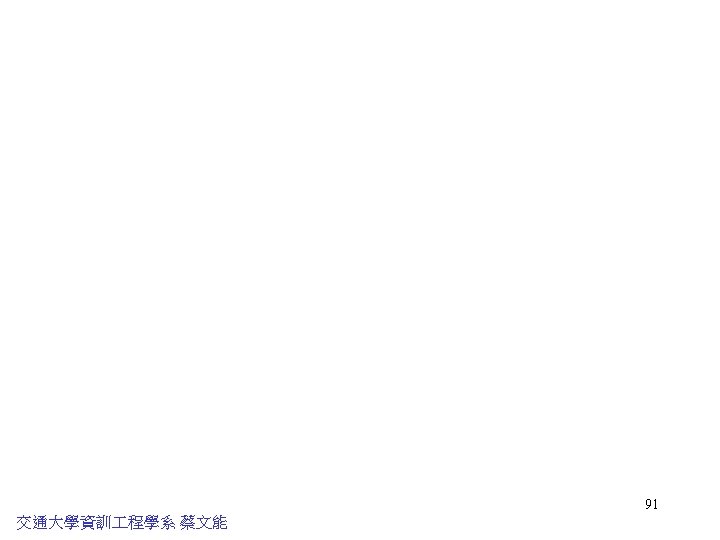
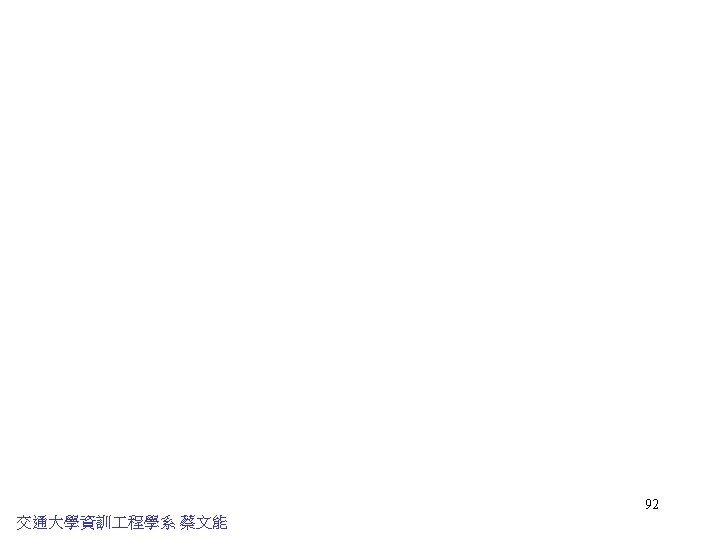
Nctu csie
Irssi screen
Csie.nctu.edu.tw login
Transport postfix
Tw-network
Nctu vpn
Webmail nctu
Ok nctu
Nctu electrophysics
Wire tun vpn
Nctu domain
Ok nctu
Nctu mail
Nfc nctu
Nctu pulse secure
Postfix transport map
Nctu network programming github
Csie workstation
Bill fenner
Cgu csie
Ncnu csie
Ntu csie vpn
Ccu csie
Cmlab
Ncue csie
Poker bet challenge
Ntu sslvpn
Edu.sharif.edu
Stl biblioteka
Stl components are grouped under
Stl stands for in c++
Stl bgb
Terminale stl spcl systèmes et procédés
Queue stl
Stl link st leonards
Prigent stl
Cs 247
Biblioteka stl
Rocinante stl
What wind is forecast for stl at 12 000 feet
Sandefjordskolen stl+
Biblioteka stl
Stl uren
Introduction to stl
Obj formato
Libreria stl
Projet stl exemple
Stl c++
The only cloud type forecast in taf reports is
Stepanov stl
Std sort
Generic programming and the stl
Recristallisation aspirine
Drsr.sk elektronická komunikácia
Parallel stl
From statsmodels.tsa.seasonal import stl
Reduce polygons
Thư viện stl
Stl biblioteka
Rapid prototyping data formats
C++ functor
Teachertech.rice.edu
Http://numericalmethods.eng.usf.edu
Http://weather.uwyo.edu/upperair/sounding.html
Http //www.phys.hawaii.edu/ teb/optics/java/slitdiffr/
Http://learn.genetics.utah.edu/
Http //www.scratch.mit.edu
002
Http://www.colorado.edu/physics/phet
Dendro.cnre.vt.edu photosynthesis chloroplast
Assessform login
Https //scratch.mit.edu online
Http //2learner.hcmup.edu.vn
Http://weather.uwyo.edu/upperair/sounding.html
Http://numericalmethods.eng.usf.edu
Sir isaac newton
Numericalmethods.eng.usf.edu
Http://learn.genetics.utah.edu/content/basics/
Http corpus byu edu coca
Gestalt leveling
Http://teachertech.rice.edu/participants/louviere/newton/
Http.//scratch.mit.edu
Dlib.nyu.edu/aco/
Wave optics topics
Http://learn.genetics.utah.edu/content/addiction/
Scratch.mit.e
Reshuege math
Naplan online test administration handbook for teachers
Evolution.berkeley.edu
Egif umich
Green flag
Https://scratch.mit.edu/
Sinhvien.hufi.edu.vm