UNIT VI Introduction to STL Components of STL
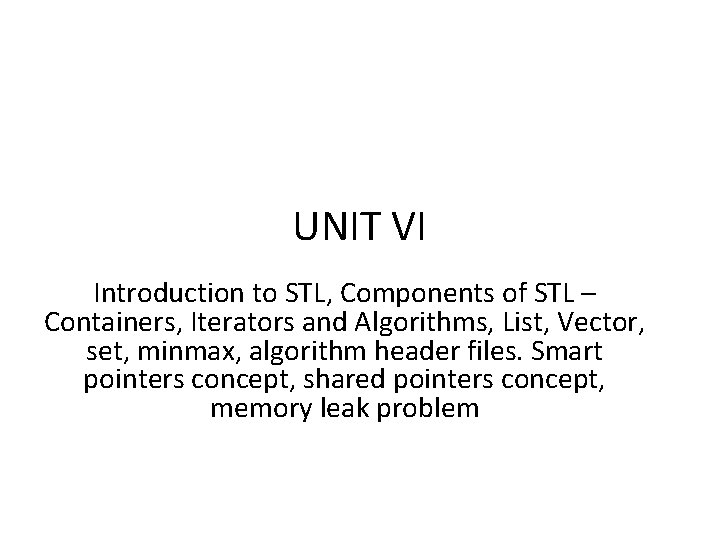
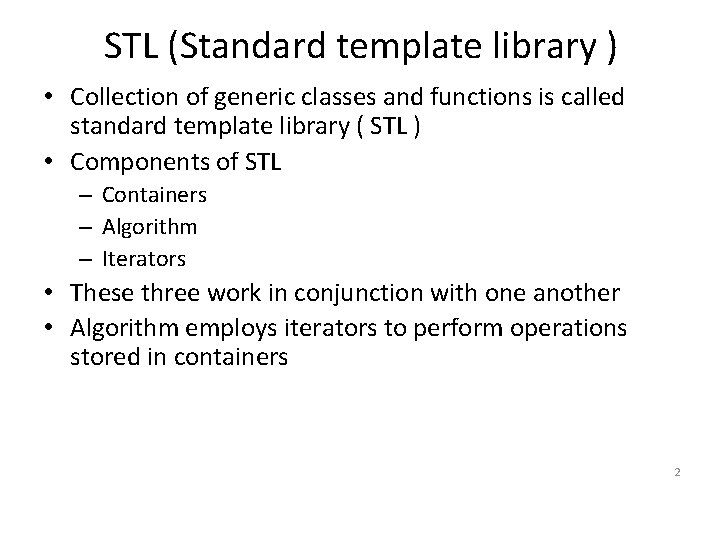
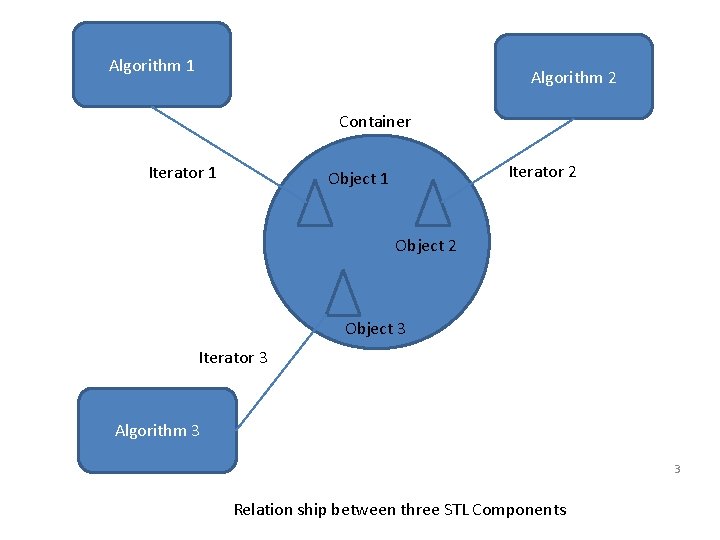
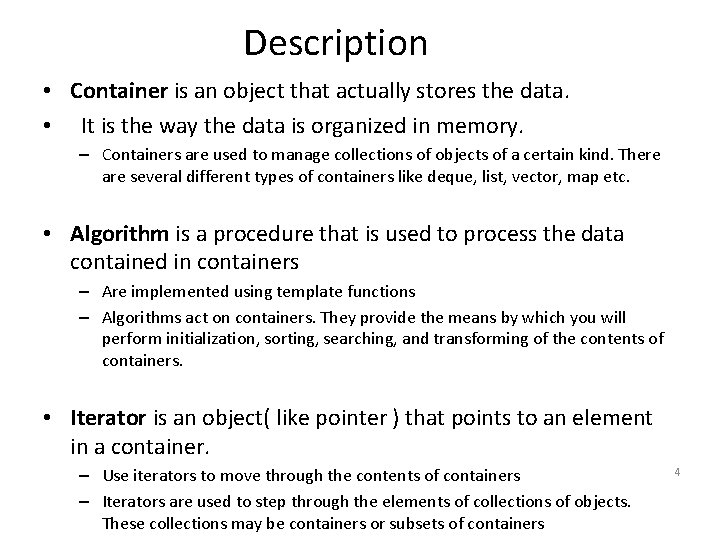
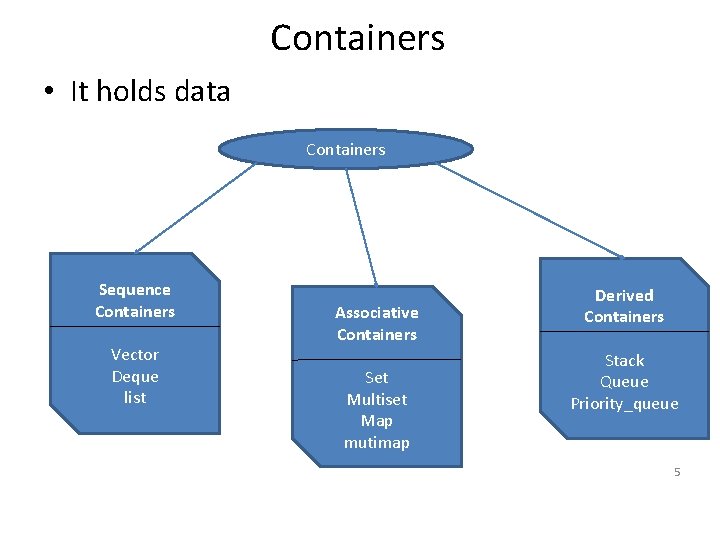
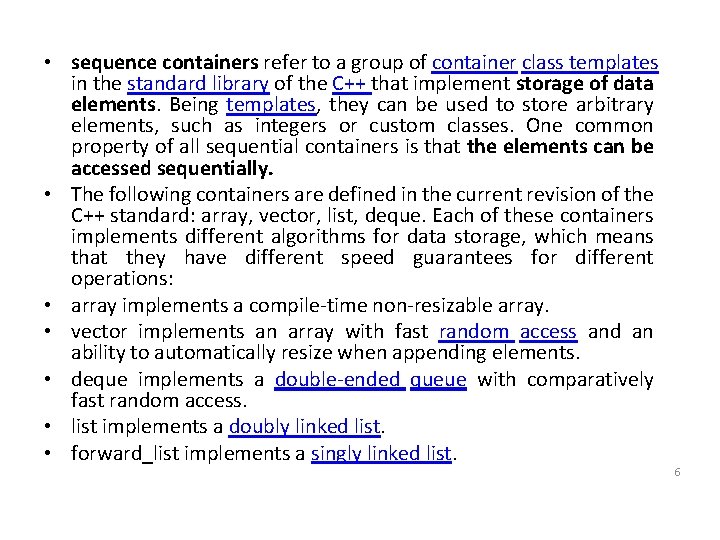
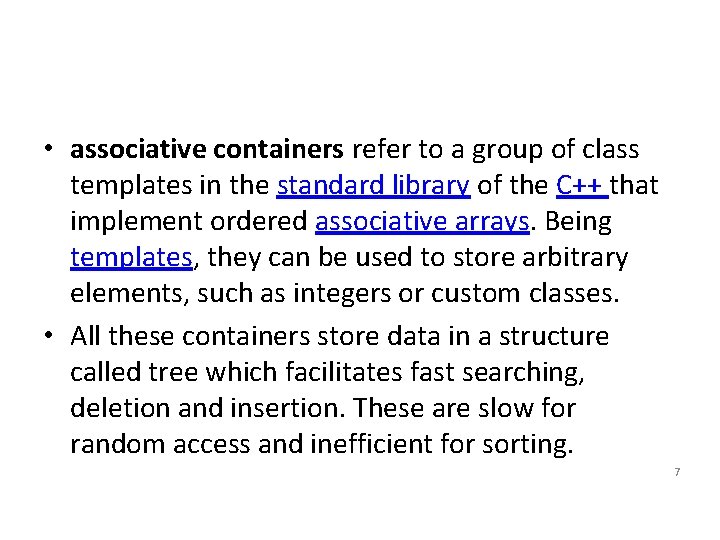
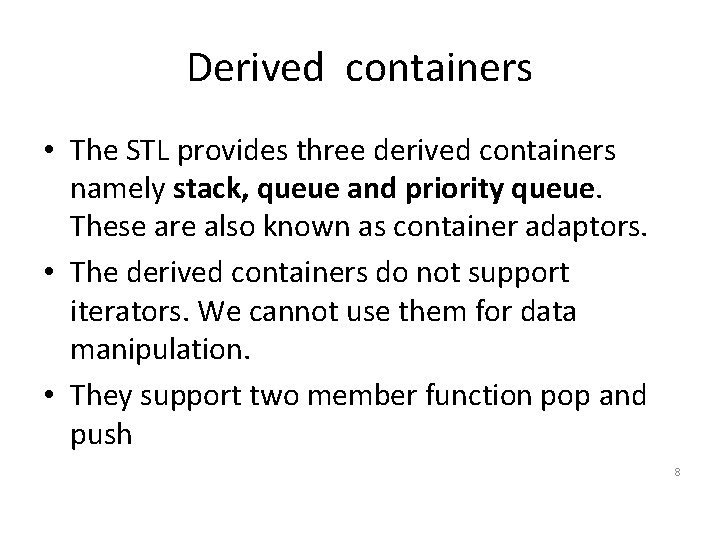
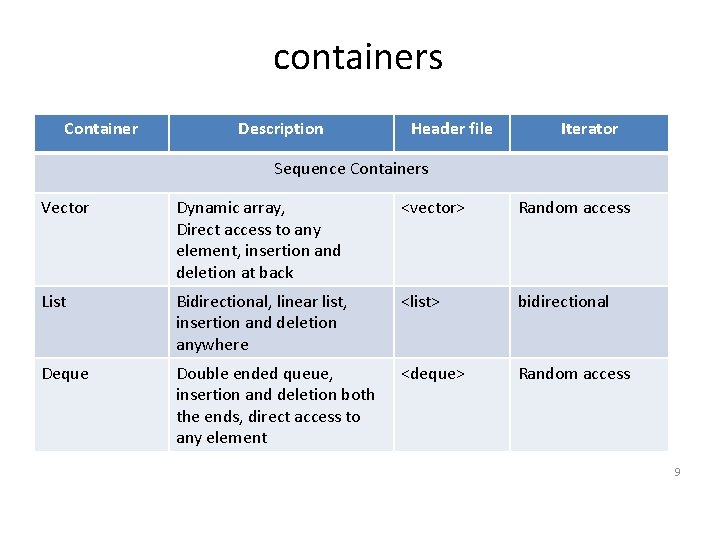
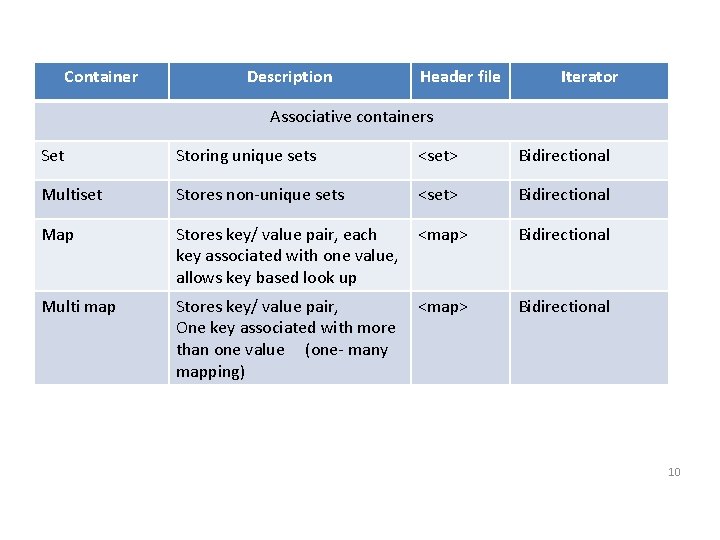
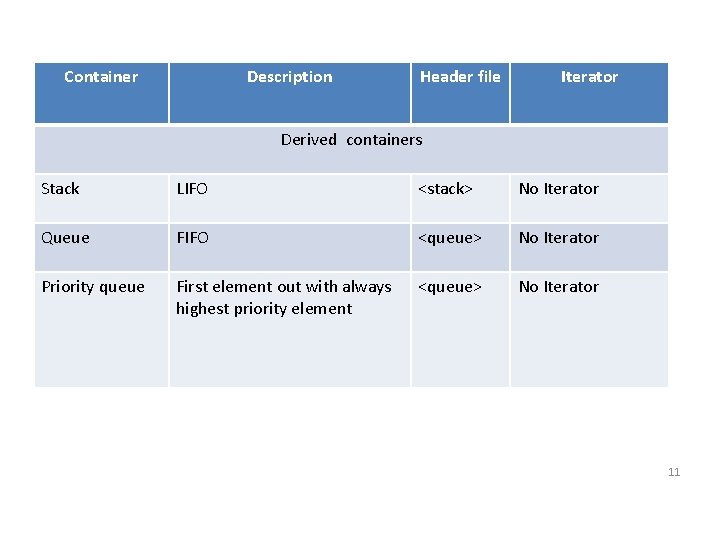
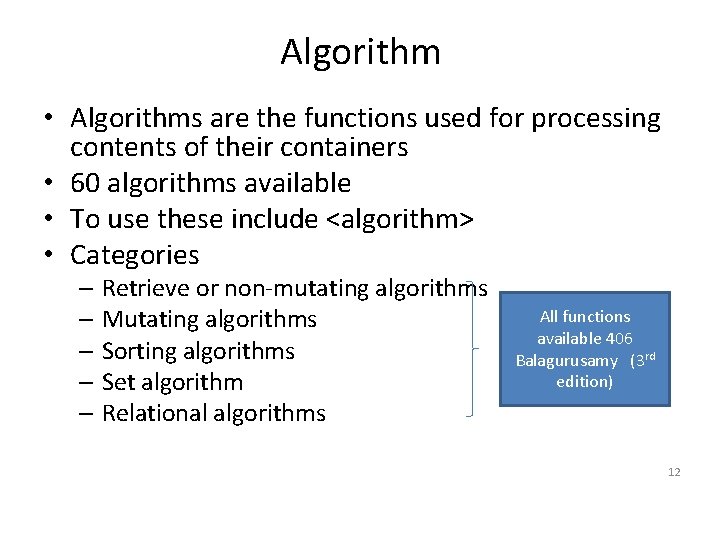
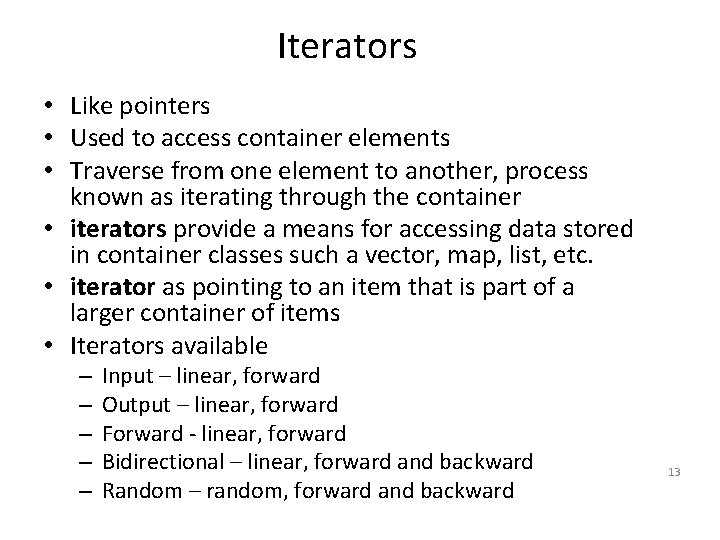
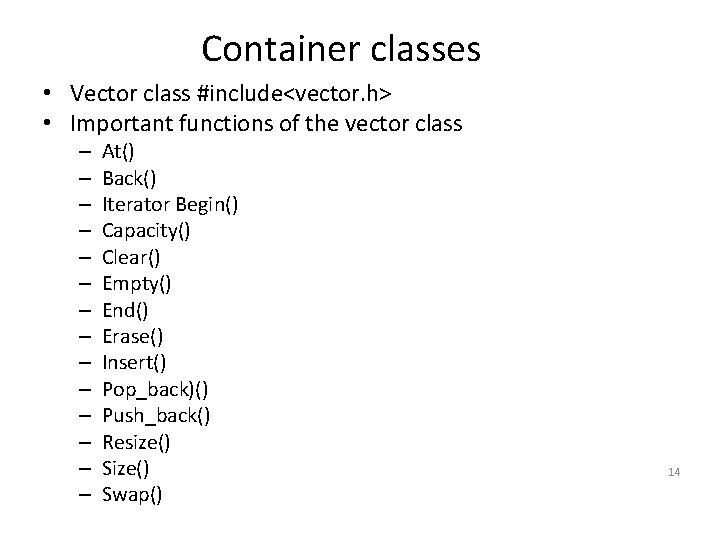
![Vectors class demo program Void display(vector<int> &v) { for(i =0 to v. size()) cout<<v[i] Vectors class demo program Void display(vector<int> &v) { for(i =0 to v. size()) cout<<v[i]](https://slidetodoc.com/presentation_image/1d7b9204b1c620ff26b8613f916f8c50/image-15.jpg)
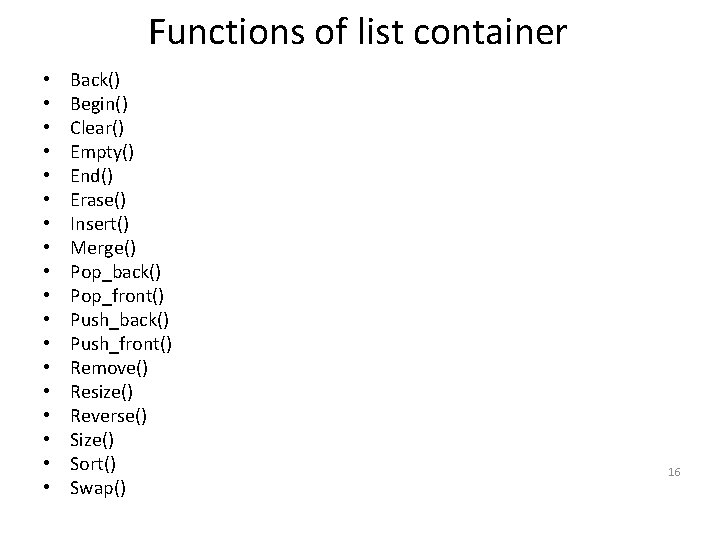
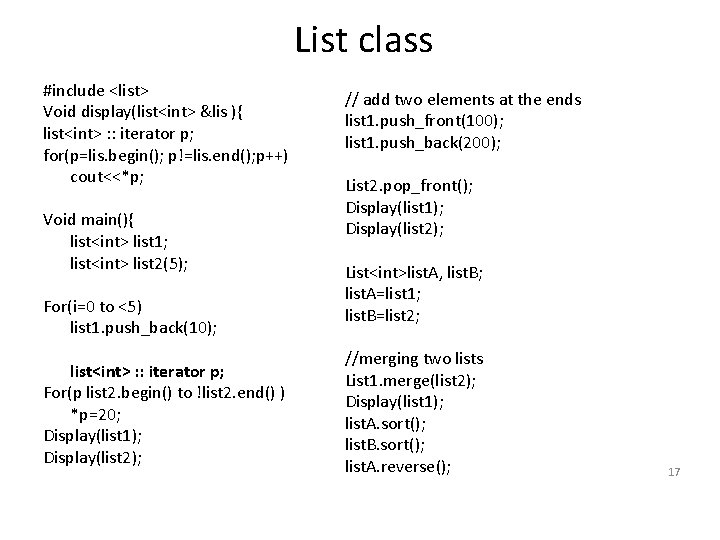
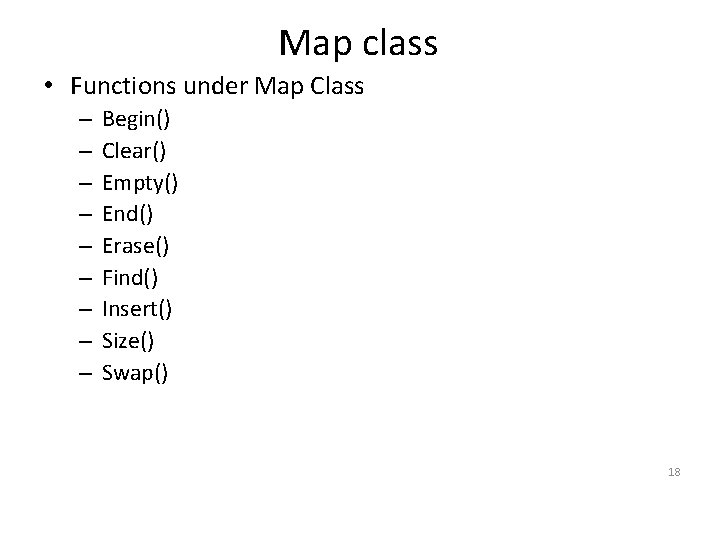
- Slides: 18
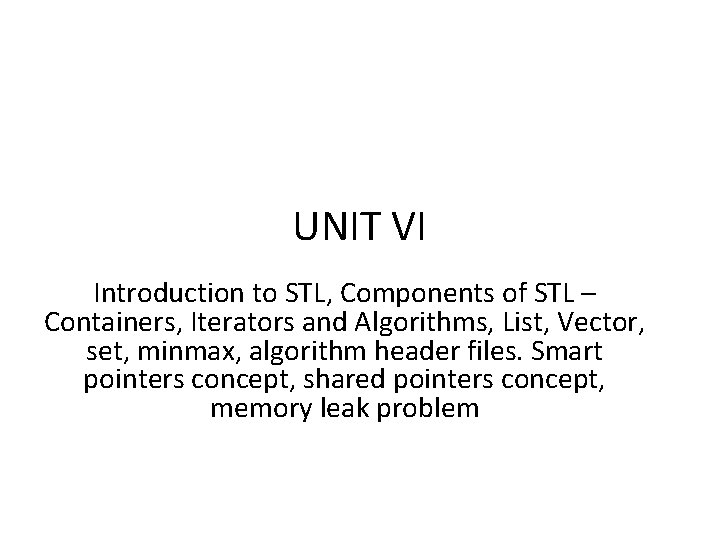
UNIT VI Introduction to STL, Components of STL – Containers, Iterators and Algorithms, List, Vector, set, minmax, algorithm header files. Smart pointers concept, shared pointers concept, memory leak problem
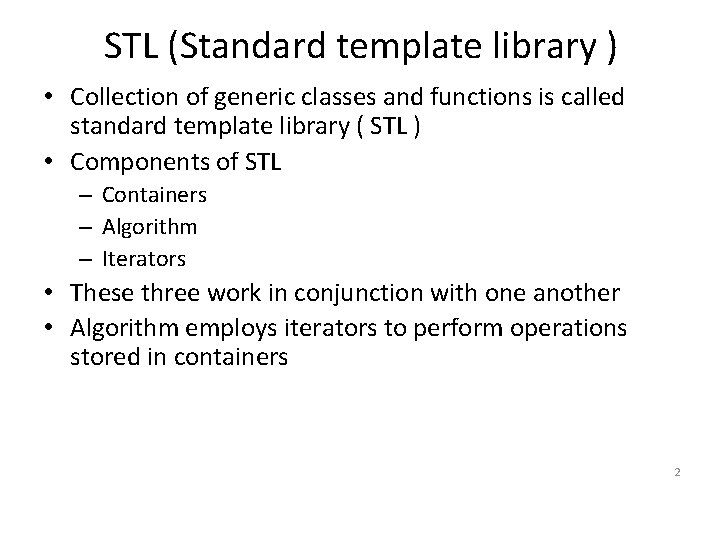
STL (Standard template library ) • Collection of generic classes and functions is called standard template library ( STL ) • Components of STL – Containers – Algorithm – Iterators • These three work in conjunction with one another • Algorithm employs iterators to perform operations stored in containers 2
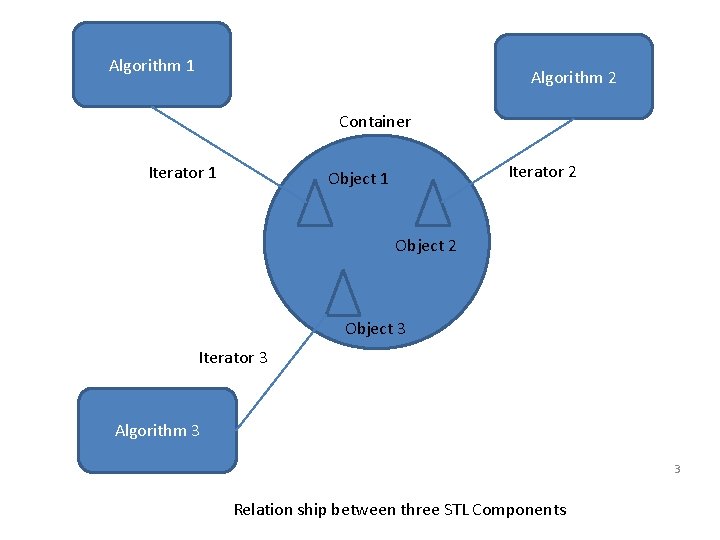
Algorithm 1 Algorithm 2 Container Iterator 1 Iterator 2 Object 1 Object 2 Object 3 Iterator 3 Algorithm 3 3 Relation ship between three STL Components
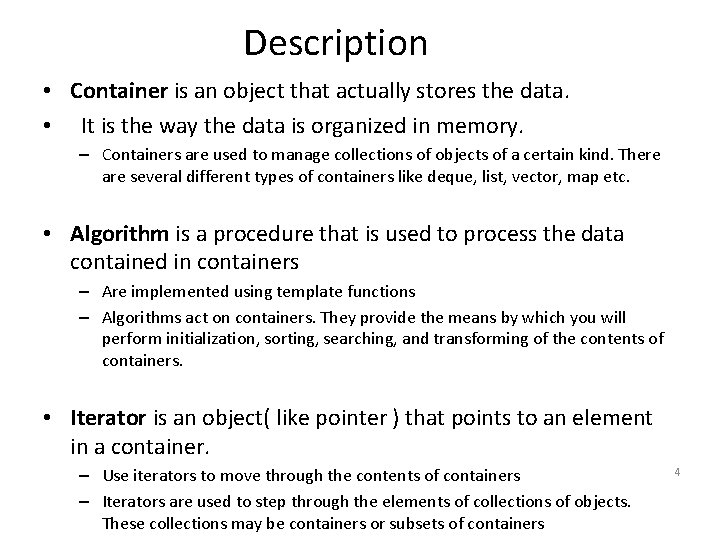
Description • Container is an object that actually stores the data. • It is the way the data is organized in memory. – Containers are used to manage collections of objects of a certain kind. There are several different types of containers like deque, list, vector, map etc. • Algorithm is a procedure that is used to process the data contained in containers – Are implemented using template functions – Algorithms act on containers. They provide the means by which you will perform initialization, sorting, searching, and transforming of the contents of containers. • Iterator is an object( like pointer ) that points to an element in a container. – Use iterators to move through the contents of containers – Iterators are used to step through the elements of collections of objects. These collections may be containers or subsets of containers 4
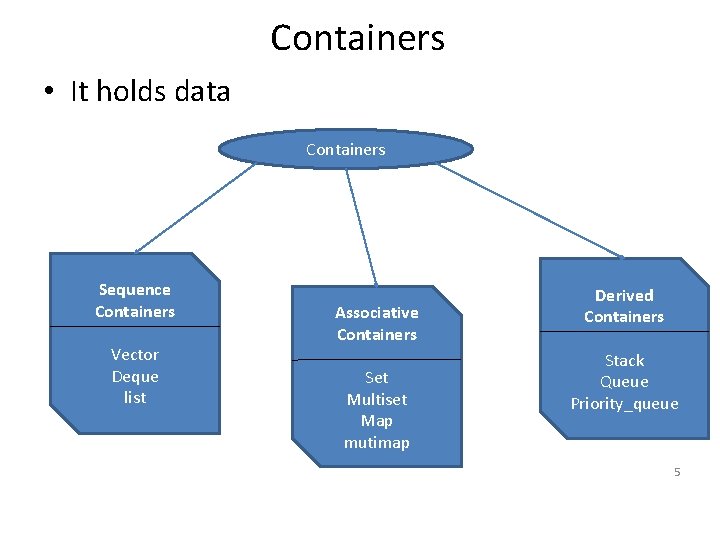
Containers • It holds data Containers Sequence Containers Vector Deque list Associative Containers Set Multiset Map mutimap Derived Containers Stack Queue Priority_queue 5
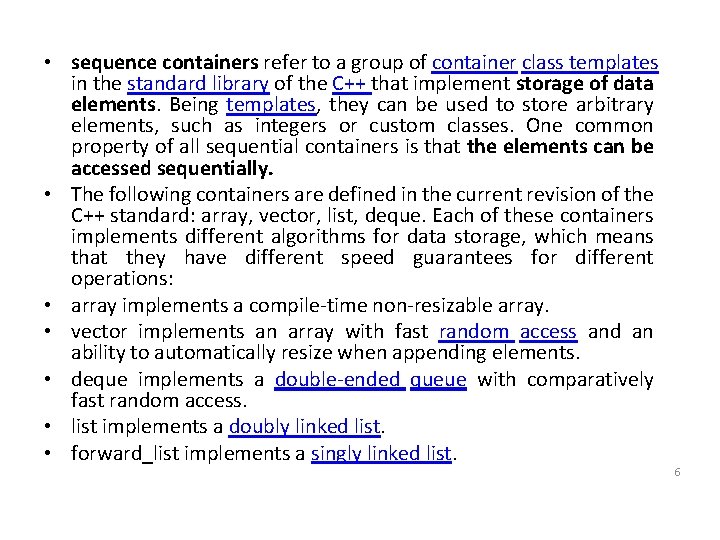
• sequence containers refer to a group of container class templates in the standard library of the C++ that implement storage of data elements. Being templates, they can be used to store arbitrary elements, such as integers or custom classes. One common property of all sequential containers is that the elements can be accessed sequentially. • The following containers are defined in the current revision of the C++ standard: array, vector, list, deque. Each of these containers implements different algorithms for data storage, which means that they have different speed guarantees for different operations: • array implements a compile-time non-resizable array. • vector implements an array with fast random access and an ability to automatically resize when appending elements. • deque implements a double-ended queue with comparatively fast random access. • list implements a doubly linked list. • forward_list implements a singly linked list. 6
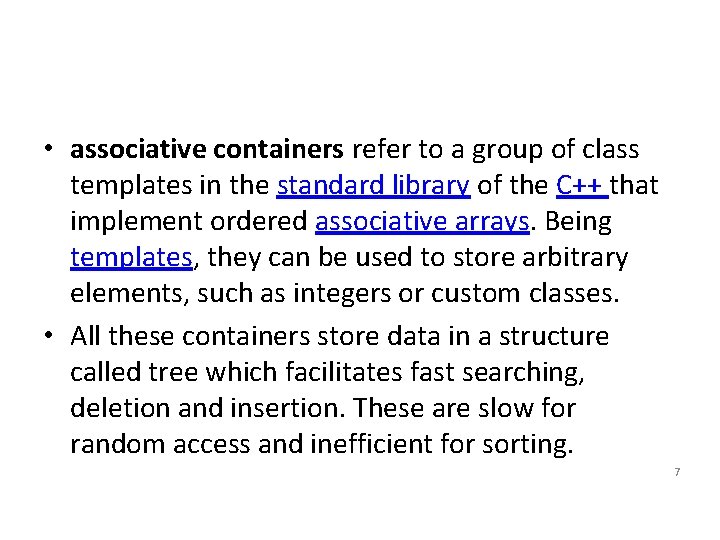
• associative containers refer to a group of class templates in the standard library of the C++ that implement ordered associative arrays. Being templates, they can be used to store arbitrary elements, such as integers or custom classes. • All these containers store data in a structure called tree which facilitates fast searching, deletion and insertion. These are slow for random access and inefficient for sorting. 7
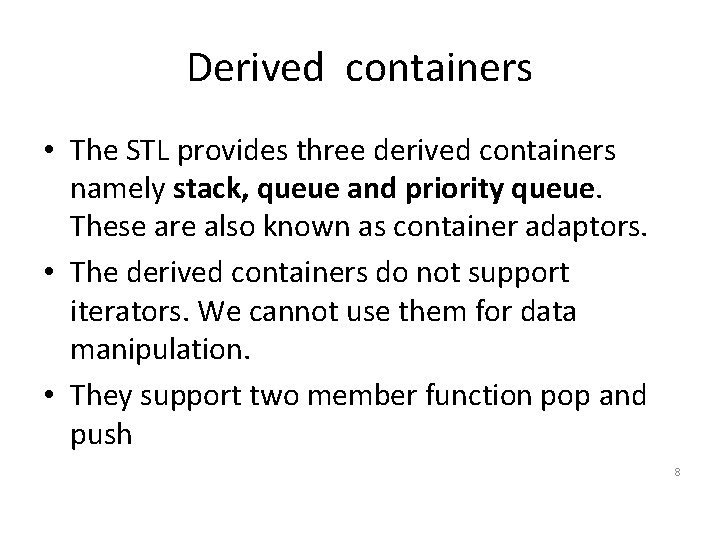
Derived containers • The STL provides three derived containers namely stack, queue and priority queue. These are also known as container adaptors. • The derived containers do not support iterators. We cannot use them for data manipulation. • They support two member function pop and push 8
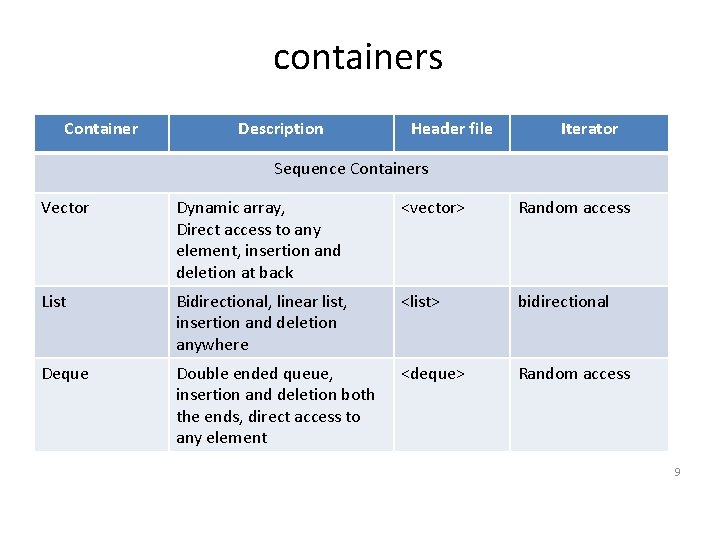
containers Container Description Header file Iterator Sequence Containers Vector Dynamic array, Direct access to any element, insertion and deletion at back <vector> Random access List Bidirectional, linear list, insertion and deletion anywhere <list> bidirectional Deque Double ended queue, insertion and deletion both the ends, direct access to any element <deque> Random access 9
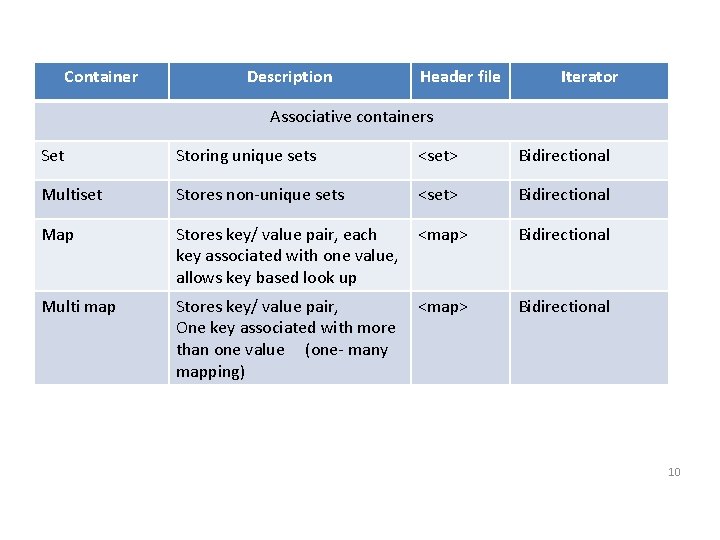
Container Description Header file Iterator Associative containers Set Storing unique sets <set> Bidirectional Multiset Stores non-unique sets <set> Bidirectional Map Stores key/ value pair, each key associated with one value, allows key based look up <map> Bidirectional Multi map Stores key/ value pair, One key associated with more than one value (one- many mapping) <map> Bidirectional 10
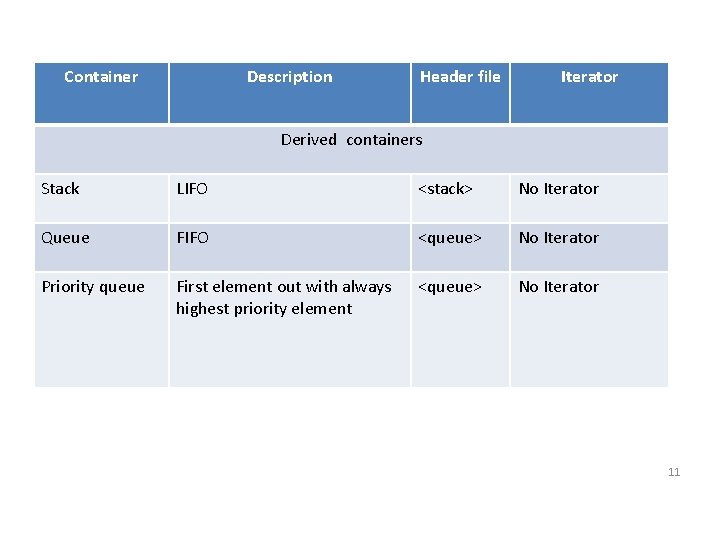
Container Description Header file Iterator Derived containers Stack LIFO <stack> No Iterator Queue FIFO <queue> No Iterator Priority queue First element out with always highest priority element <queue> No Iterator 11
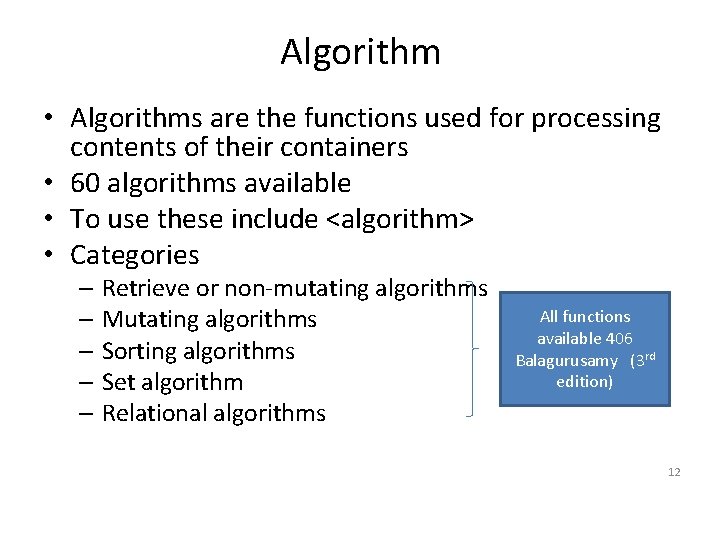
Algorithm • Algorithms are the functions used for processing contents of their containers • 60 algorithms available • To use these include <algorithm> • Categories – Retrieve or non-mutating algorithms – Mutating algorithms – Sorting algorithms – Set algorithm – Relational algorithms All functions available 406 Balagurusamy (3 rd edition) 12
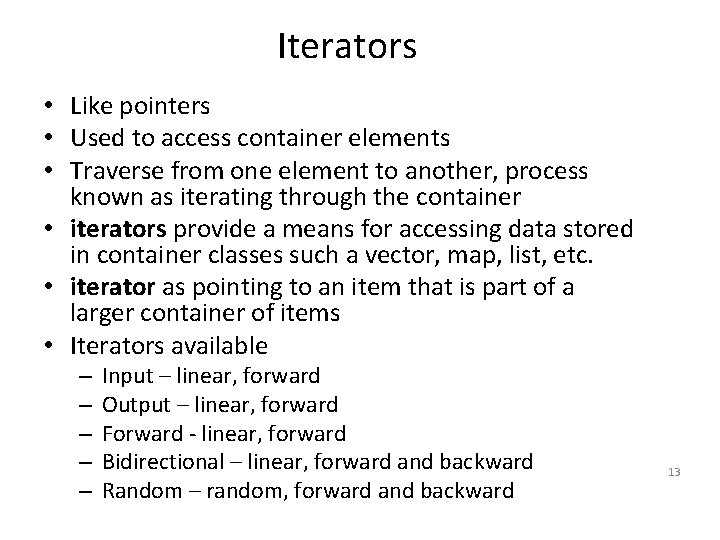
Iterators • Like pointers • Used to access container elements • Traverse from one element to another, process known as iterating through the container • iterators provide a means for accessing data stored in container classes such a vector, map, list, etc. • iterator as pointing to an item that is part of a larger container of items • Iterators available – – – Input – linear, forward Output – linear, forward Forward - linear, forward Bidirectional – linear, forward and backward Random – random, forward and backward 13
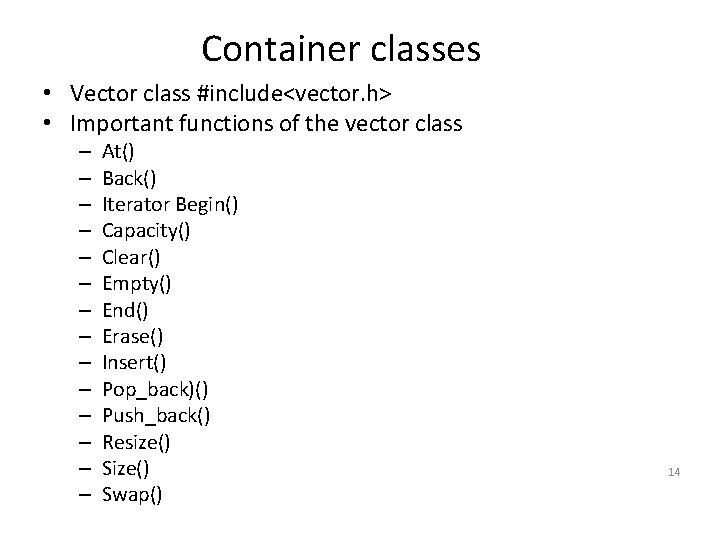
Container classes • Vector class #include<vector. h> • Important functions of the vector class – – – – At() Back() Iterator Begin() Capacity() Clear() Empty() End() Erase() Insert() Pop_back)() Push_back() Resize() Swap() 14
![Vectors class demo program Void displayvectorint v fori 0 to v size coutvi Vectors class demo program Void display(vector<int> &v) { for(i =0 to v. size()) cout<<v[i]](https://slidetodoc.com/presentation_image/1d7b9204b1c620ff26b8613f916f8c50/image-15.jpg)
Vectors class demo program Void display(vector<int> &v) { for(i =0 to v. size()) cout<<v[i] } int main(){ vector<int> v; int x; for(i= 0 to <5){ cin>>x; v. push_back(x); } v. size(); Display(v); // add one more elements v. push_back(10. 5); //call size() and display() // using iterators vector <int> : : iterator itr = v. begin(); itr = itr + 3; // itr points to 4 th element v. insert(itr, 9); // call display() //delete 4 th element v. erase(v. begin()+3); // call display() } 15
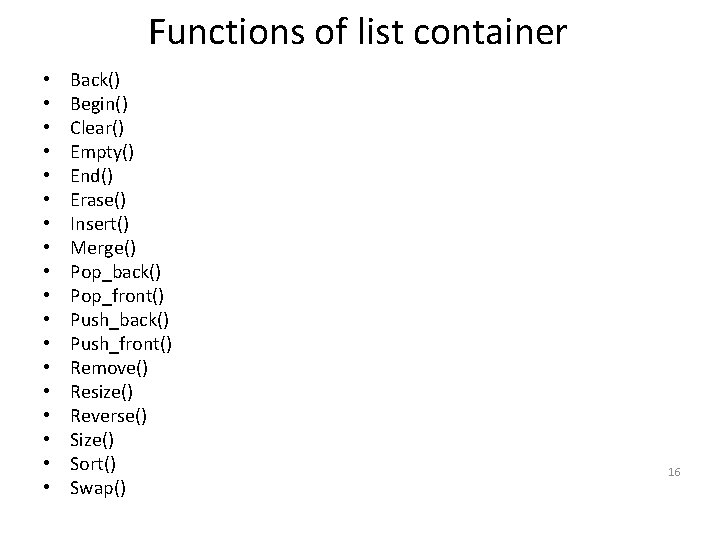
Functions of list container • • • • • Back() Begin() Clear() Empty() End() Erase() Insert() Merge() Pop_back() Pop_front() Push_back() Push_front() Remove() Resize() Reverse() Size() Sort() Swap() 16
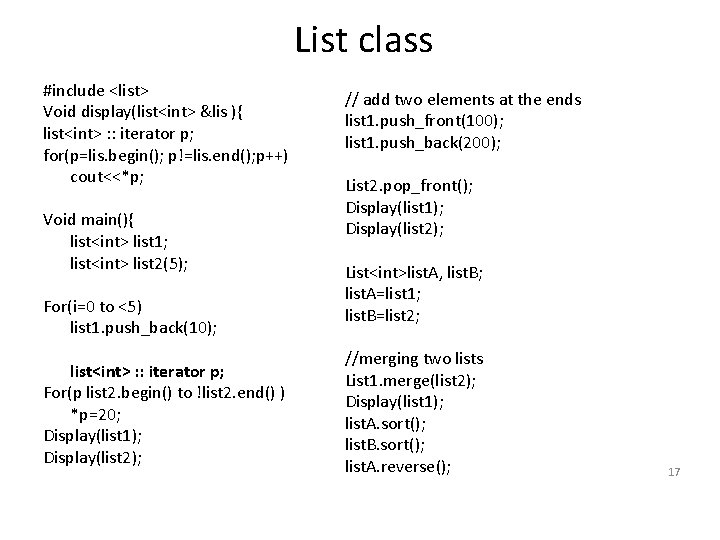
List class #include <list> Void display(list<int> &lis ){ list<int> : : iterator p; for(p=lis. begin(); p!=lis. end(); p++) cout<<*p; Void main(){ list<int> list 1; list<int> list 2(5); For(i=0 to <5) list 1. push_back(10); list<int> : : iterator p; For(p list 2. begin() to !list 2. end() ) *p=20; Display(list 1); Display(list 2); // add two elements at the ends list 1. push_front(100); list 1. push_back(200); List 2. pop_front(); Display(list 1); Display(list 2); List<int>list. A, list. B; list. A=list 1; list. B=list 2; //merging two lists List 1. merge(list 2); Display(list 1); list. A. sort(); list. B. sort(); list. A. reverse(); 17
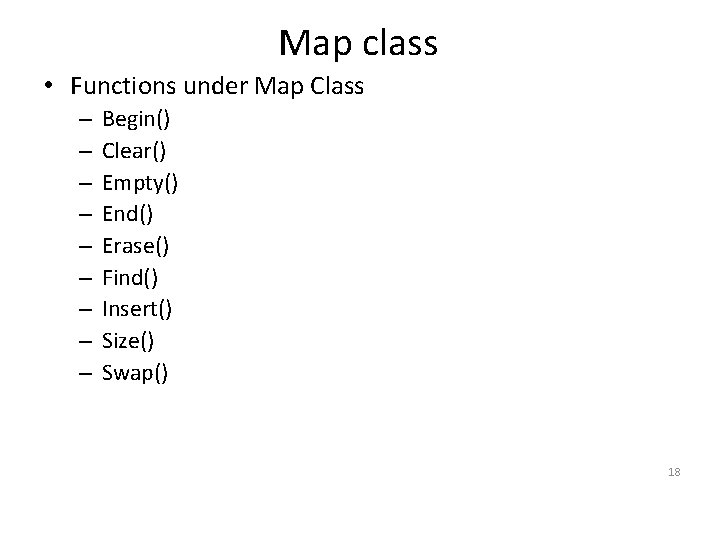
Map class • Functions under Map Class – – – – – Begin() Clear() Empty() End() Erase() Find() Insert() Size() Swap() 18
Stl components are grouped under
Introduction to stl
Unit 6 review questions
Compare stl and slc file format
Kontenery stl
Generic programming and the stl
C++ functor vs function pointer
Cs 247
Projet stl exemple
From statsmodels.tsa.seasonal import stl
Terminale stl spcl systèmes et procédés
Library stl
Std sort
Stl biblioteka
Prigent stl
Libreria stl
Parallel stl
Stl stands for
Sandefjordskolen stl+