Lecture 7 3 STL Algorithms STL Algorithms n
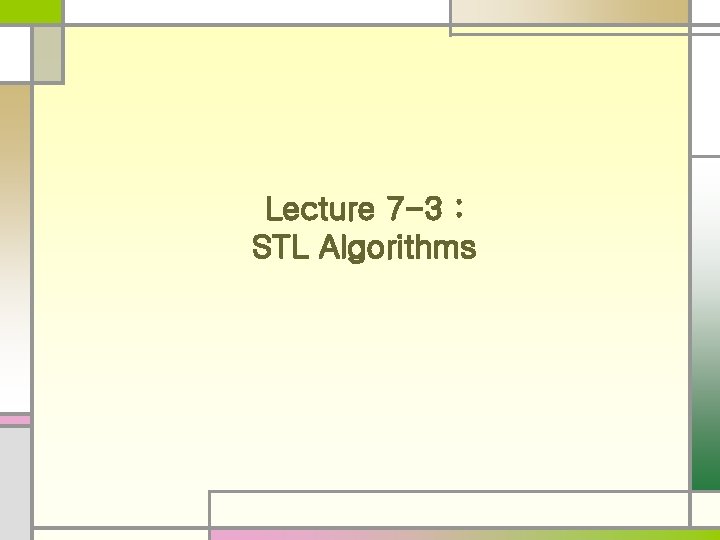
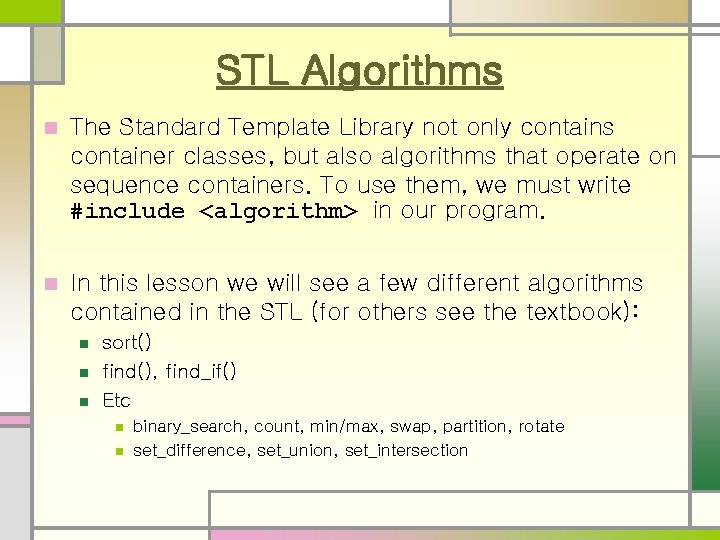
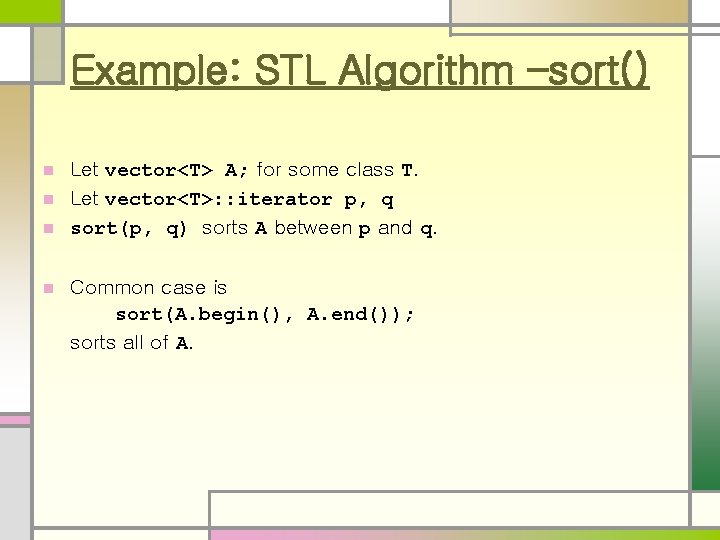
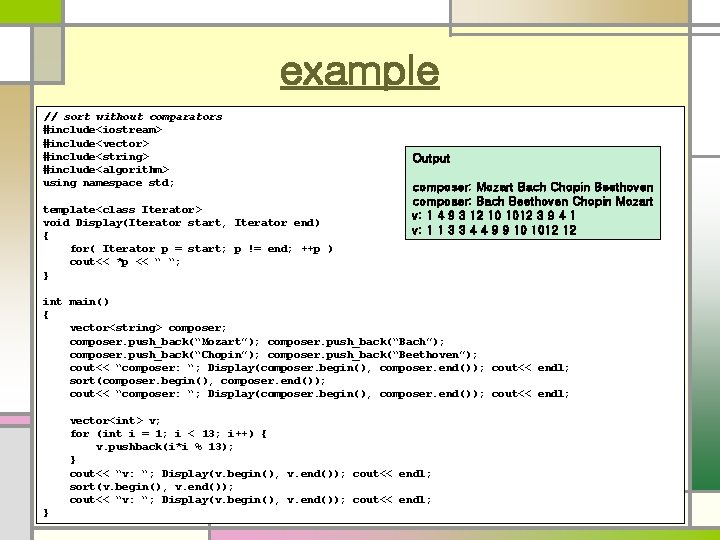
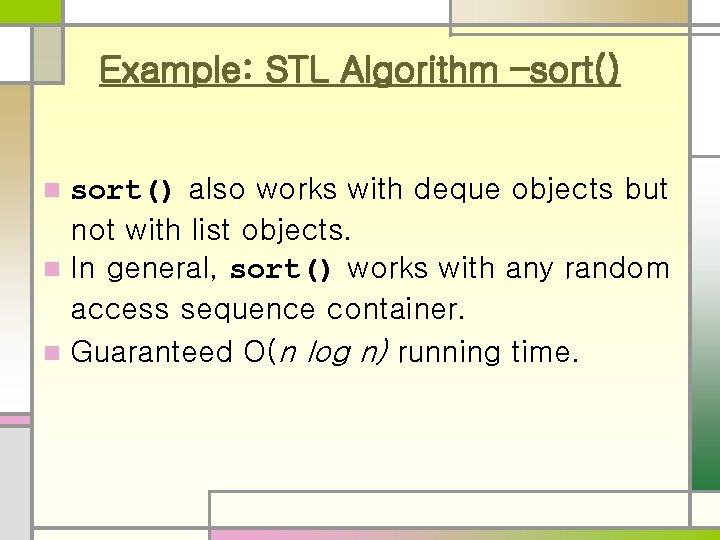
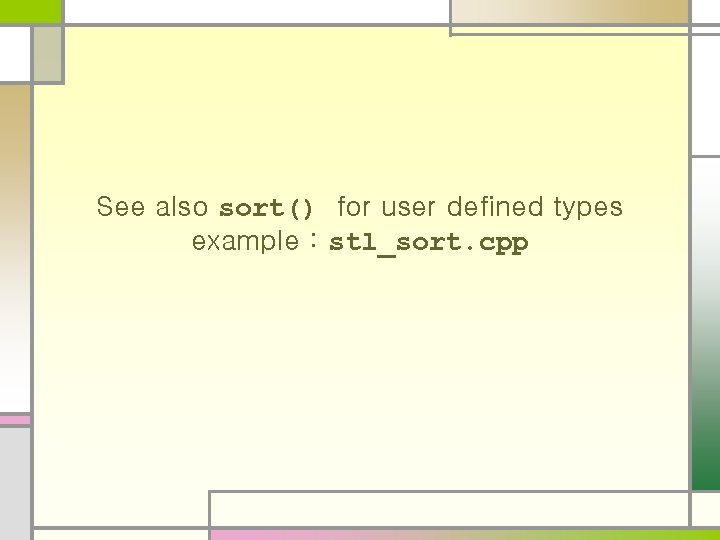
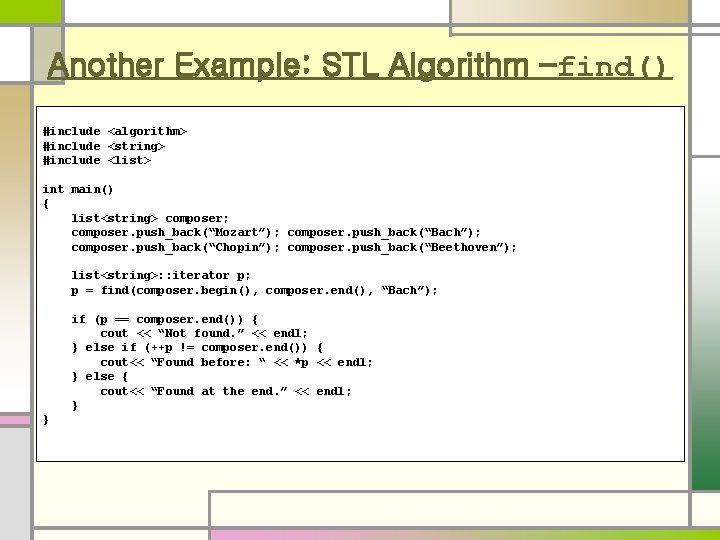
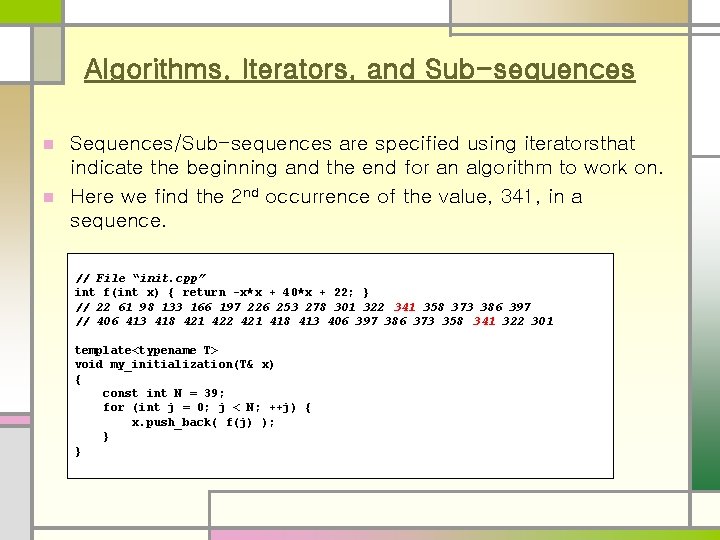
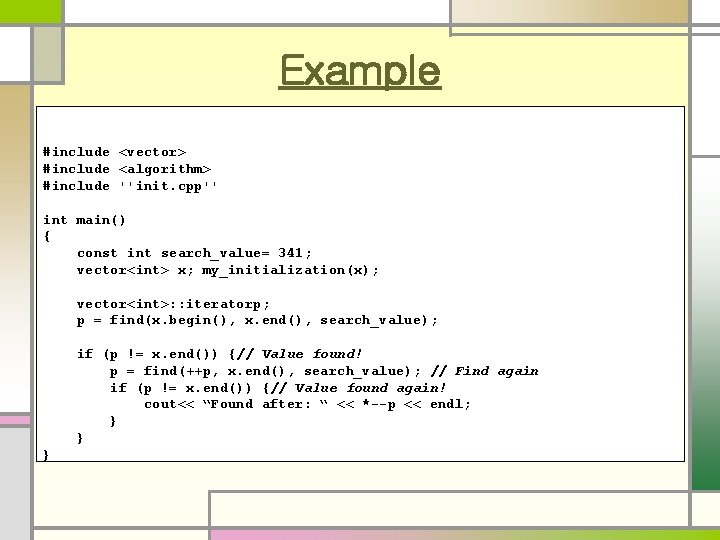
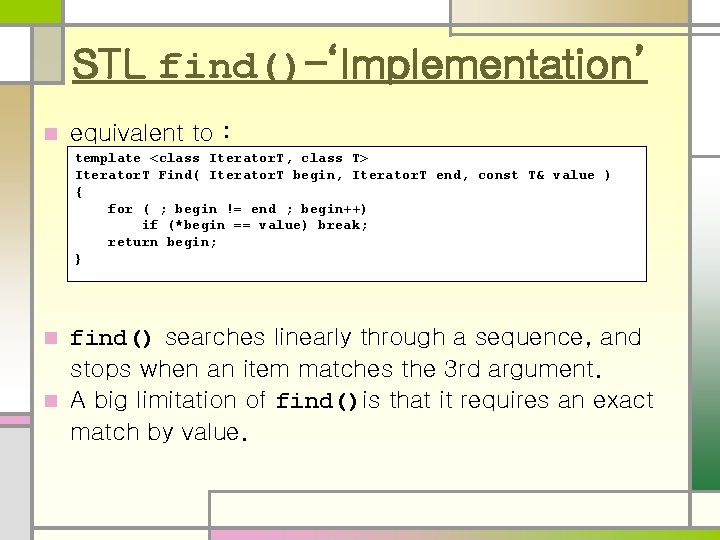
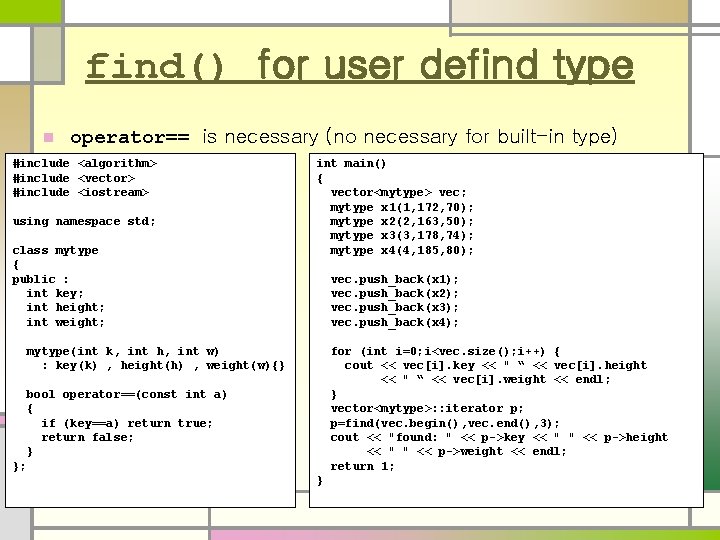
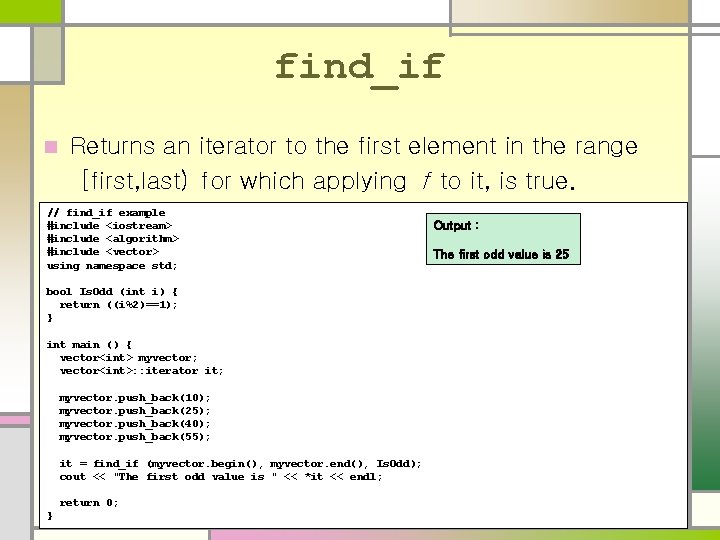
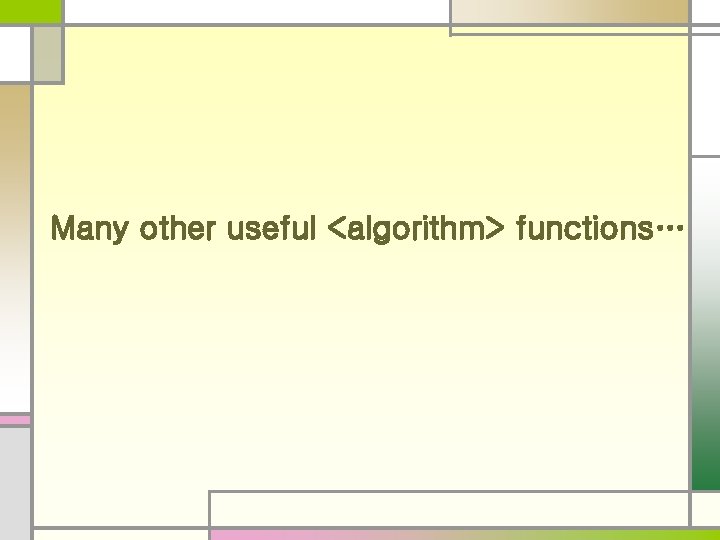
- Slides: 13
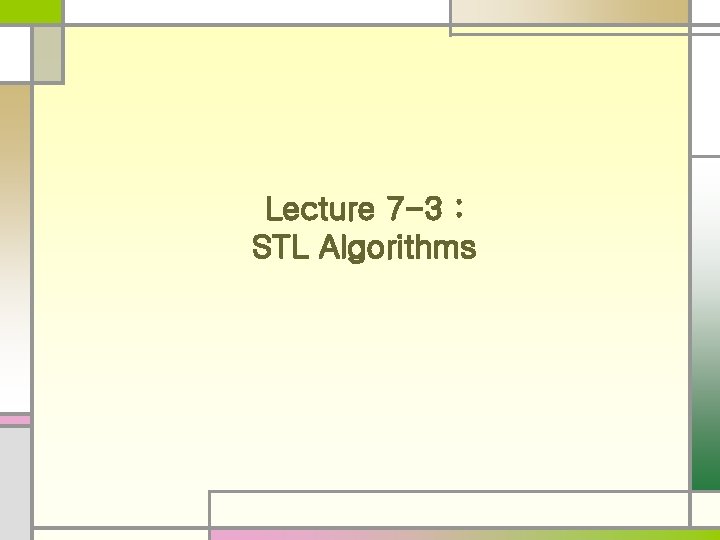
Lecture 7 -3 : STL Algorithms
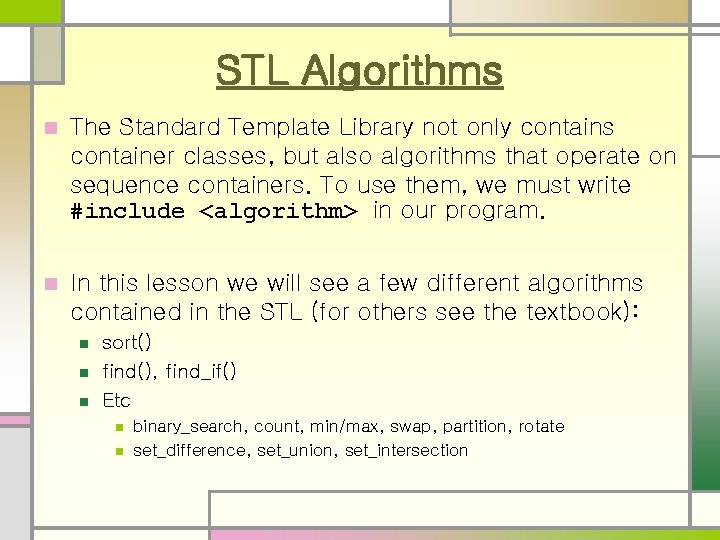
STL Algorithms n The Standard Template Library not only contains container classes, but also algorithms that operate on sequence containers. To use them, we must write #include <algorithm> in our program. n In this lesson we will see a few different algorithms contained in the STL (for others see the textbook): n n n sort() find(), find_if() Etc n n binary_search, count, min/max, swap, partition, rotate set_difference, set_union, set_intersection
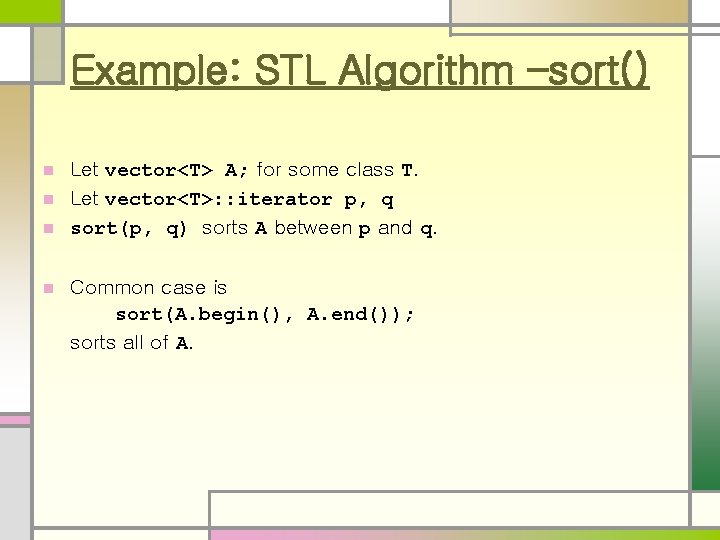
Example: STL Algorithm –sort() Let vector<T> A; for some class T. n Let vector<T>: : iterator p, q n sort(p, q) sorts A between p and q. n n Common case is sort(A. begin(), A. end()); sorts all of A.
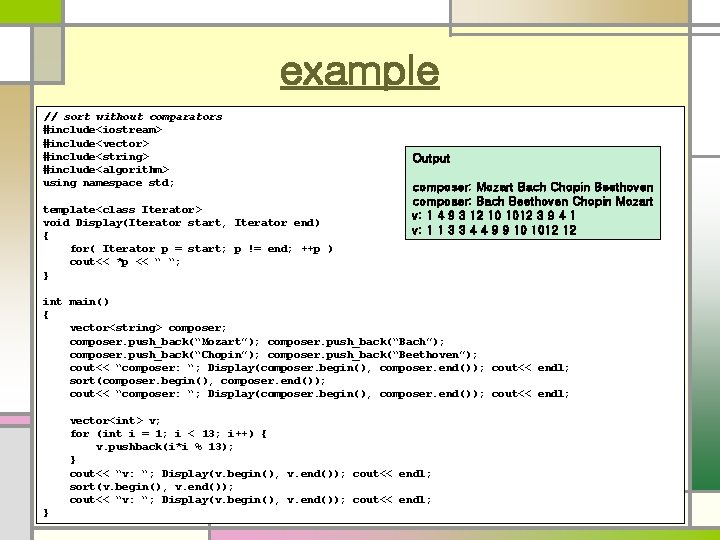
example // sort without comparators #include<iostream> #include<vector> #include<string> #include<algorithm> using namespace std; template<class Iterator> void Display(Iterator start, Iterator end) { for( Iterator p = start; p != end; ++p ) cout<< *p << “ “; } Output composer: Mozart Bach Chopin Beethoven composer: Bach Beethoven Chopin Mozart v: 1 4 9 3 12 10 1012 3 9 4 1 v: 1 1 3 3 4 4 9 9 10 1012 12 int main() { vector<string> composer; composer. push_back(“Mozart”); composer. push_back(“Bach”); composer. push_back(“Chopin”); composer. push_back(“Beethoven”); cout<< “composer: “; Display(composer. begin(), composer. end()); cout<< endl; sort(composer. begin(), composer. end()); cout<< “composer: “; Display(composer. begin(), composer. end()); cout<< endl; vector<int> v; for (int i = 1; i < 13; i++) { v. pushback(i*i % 13); } cout<< “v: “; Display(v. begin(), v. end()); cout<< endl; sort(v. begin(), v. end()); cout<< “v: “; Display(v. begin(), v. end()); cout<< endl; }
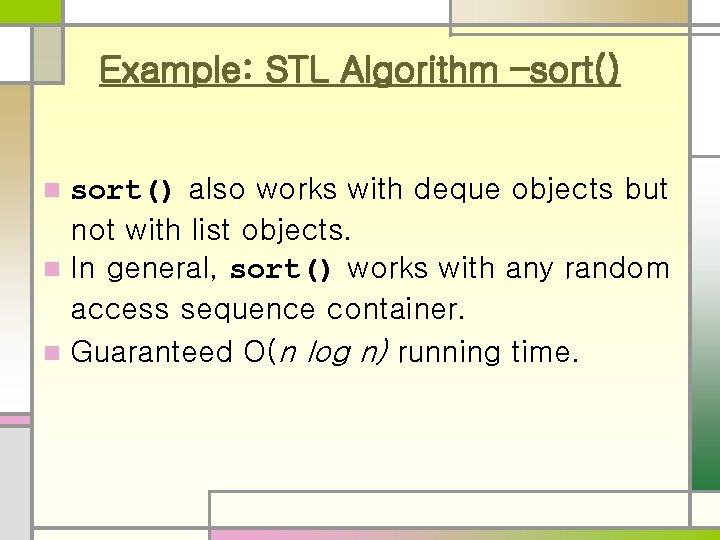
Example: STL Algorithm –sort() also works with deque objects but not with list objects. n In general, sort() works with any random access sequence container. n Guaranteed O(n log n) running time. n
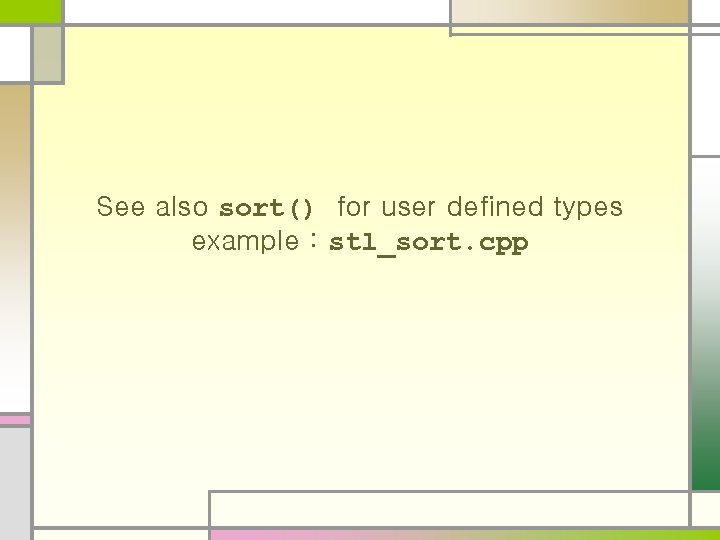
See also sort() for user defined types example : stl_sort. cpp
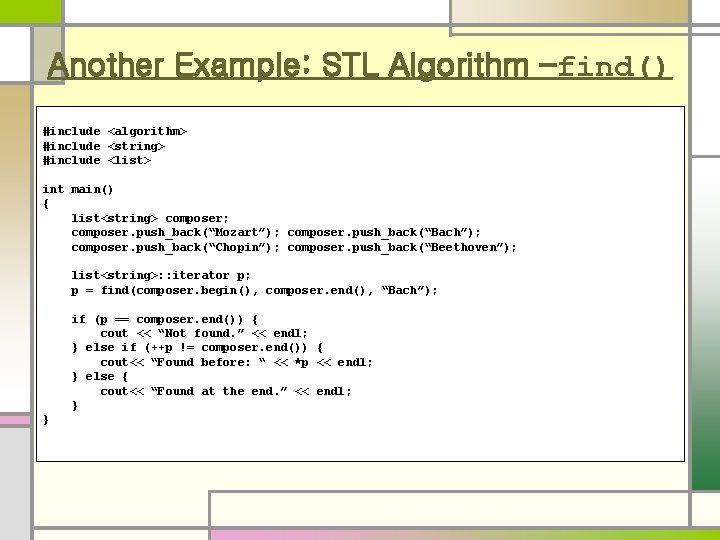
Another Example: STL Algorithm –find() #include <algorithm> #include <string> #include <list> int main() { list<string> composer; composer. push_back(“Mozart”); composer. push_back(“Bach”); composer. push_back(“Chopin”); composer. push_back(“Beethoven”); list<string>: : iterator p; p = find(composer. begin(), composer. end(), “Bach”); if (p == composer. end()) { cout << “Not found. ” << endl; } else if (++p != composer. end()) { cout<< “Found before: “ << *p << endl; } else { cout<< “Found at the end. ” << endl; } }
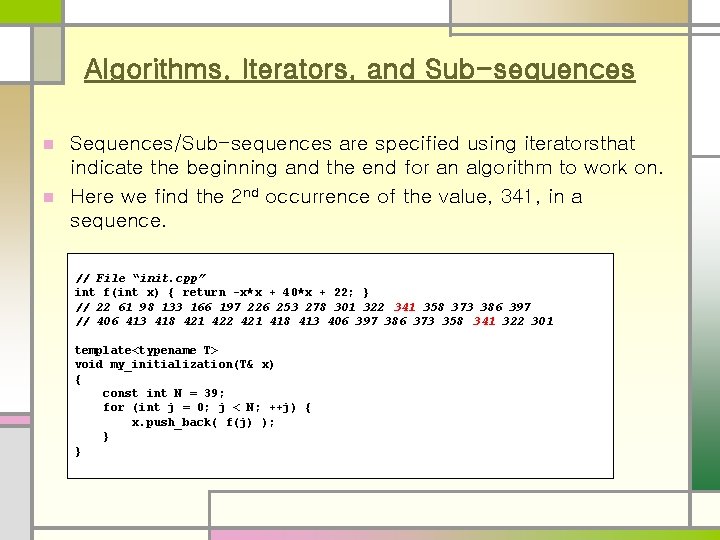
Algorithms, Iterators, and Sub-sequences Sequences/Sub-sequences are specified using iteratorsthat indicate the beginning and the end for an algorithm to work on. n Here we find the 2 nd occurrence of the value, 341, in a sequence. n // File “init. cpp” int f(int x) { return -x*x + 40*x + 22; } // 22 61 98 133 166 197 226 253 278 301 322 341 358 373 386 397 // 406 413 418 421 422 421 418 413 406 397 386 373 358 341 322 301 template<typename T> void my_initialization(T& x) { const int N = 39; for (int j = 0; j < N; ++j) { x. push_back( f(j) ); } }
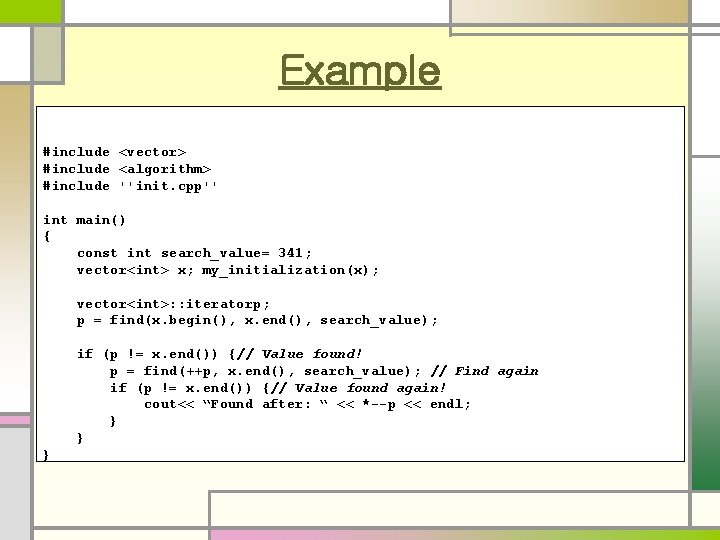
Example #include <vector> #include <algorithm> #include ''init. cpp'' int main() { const int search_value= 341; vector<int> x; my_initialization(x); vector<int>: : iteratorp; p = find(x. begin(), x. end(), search_value); if (p != x. end()) {// Value found! p = find(++p, x. end(), search_value); // Find again if (p != x. end()) {// Value found again! cout<< “Found after: “ << *--p << endl; } } }
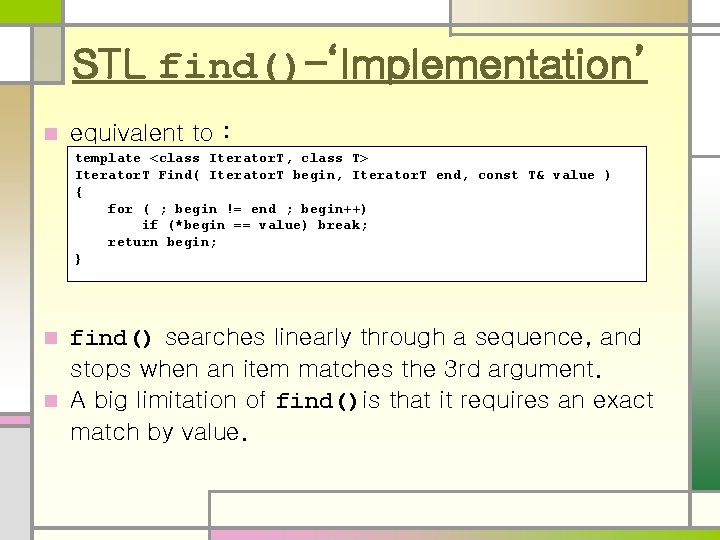
STL find()–‘Implementation’ n equivalent to : template <class Iterator. T, class T> Iterator. T Find( Iterator. T begin, Iterator. T end, const T& value ) { for ( ; begin != end ; begin++) if (*begin == value) break; return begin; } find() searches linearly through a sequence, and stops when an item matches the 3 rd argument. n A big limitation of find()is that it requires an exact match by value. n
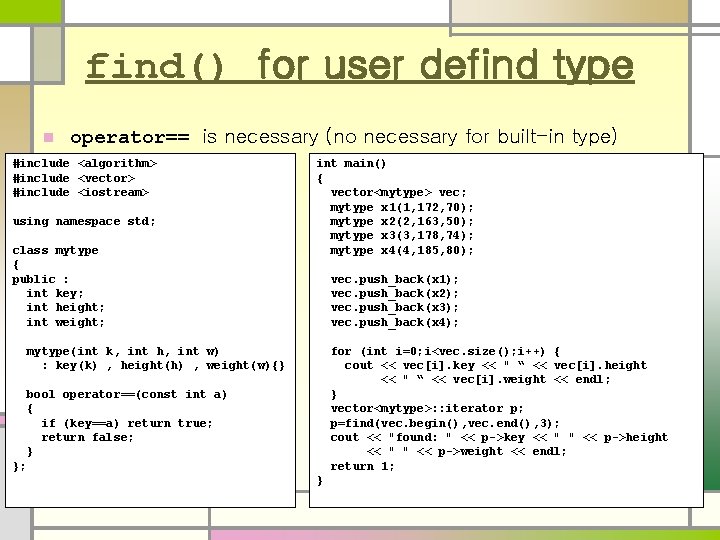
find() for user defind type n operator== is necessary (no necessary for built-in type) #include <algorithm> #include <vector> #include <iostream> using namespace std; class mytype { public : int key; int height; int weight; int main() { vector<mytype> vec; mytype x 1(1, 172, 70); mytype x 2(2, 163, 50); mytype x 3(3, 178, 74); mytype x 4(4, 185, 80); vec. push_back(x 1); vec. push_back(x 2); vec. push_back(x 3); vec. push_back(x 4); mytype(int k, int h, int w) : key(k) , height(h) , weight(w){} for (int i=0; i<vec. size(); i++) { cout << vec[i]. key << " “ << vec[i]. height << " “ << vec[i]. weight << endl; } vector<mytype>: : iterator p; p=find(vec. begin(), vec. end(), 3); cout << "found: " << p->key << " " << p->height << " " << p->weight << endl; return 1; bool operator==(const int a) { if (key==a) return true; return false; } }; }
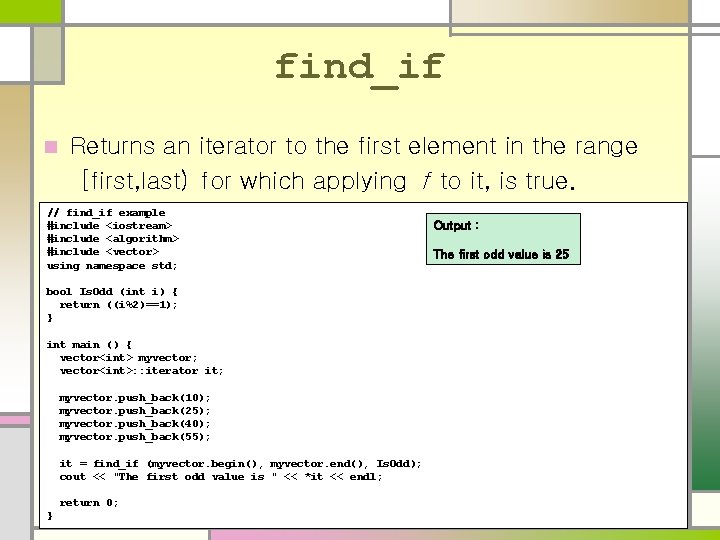
find_if n Returns an iterator to the first element in the range [first, last) for which applying f to it, is true. // find_if example #include <iostream> #include <algorithm> #include <vector> using namespace std; bool Is. Odd (int i) { return ((i%2)==1); } int main () { vector<int> myvector; vector<int>: : iterator it; myvector. push_back(10); myvector. push_back(25); myvector. push_back(40); myvector. push_back(55); it = find_if (myvector. begin(), myvector. end(), Is. Odd); cout << "The first odd value is " << *it << endl; return 0; } Output : The first odd value is 25
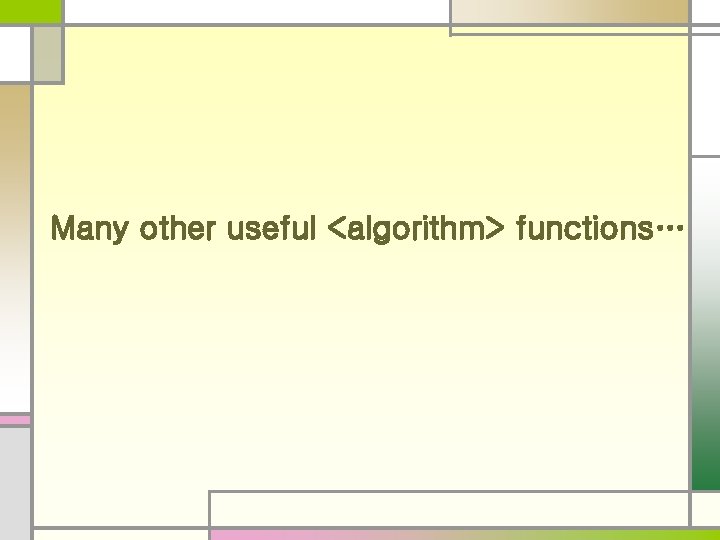
Many other useful <algorithm> functions…