INTRODUCTION TO C STL STL CLASSES Container Classes
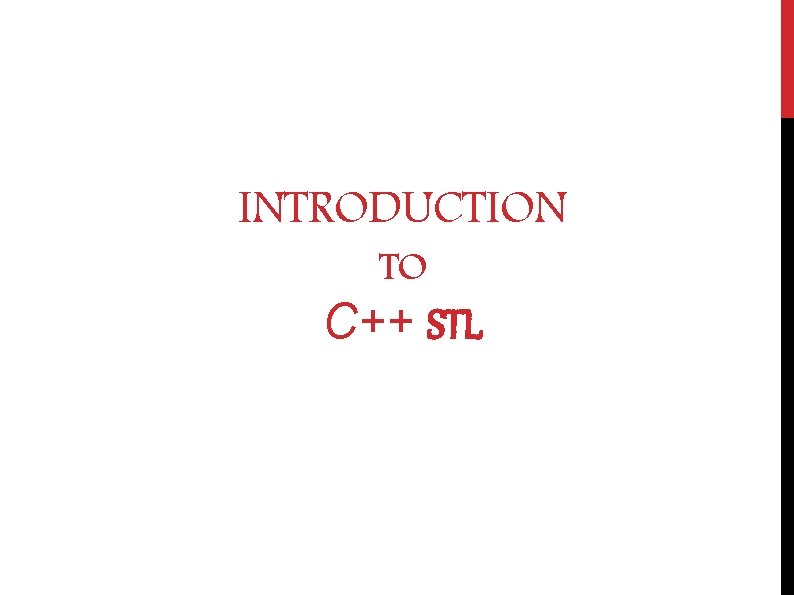
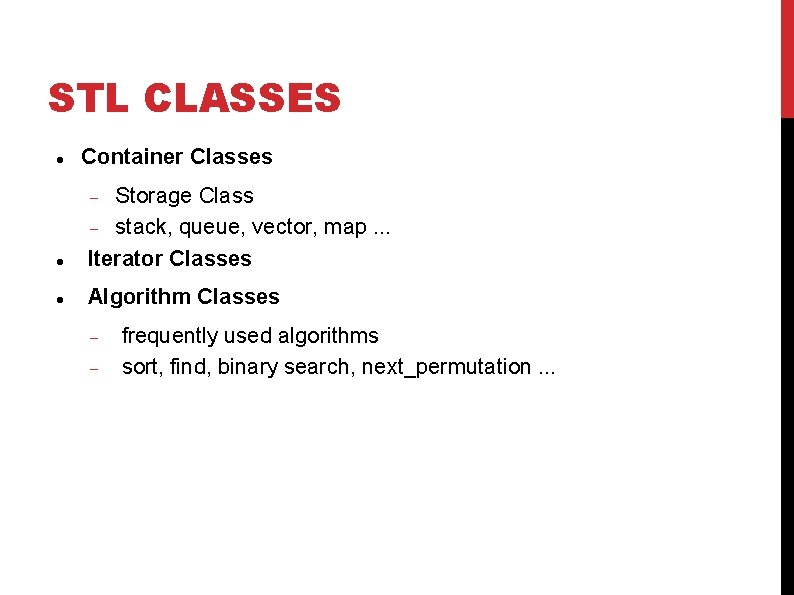
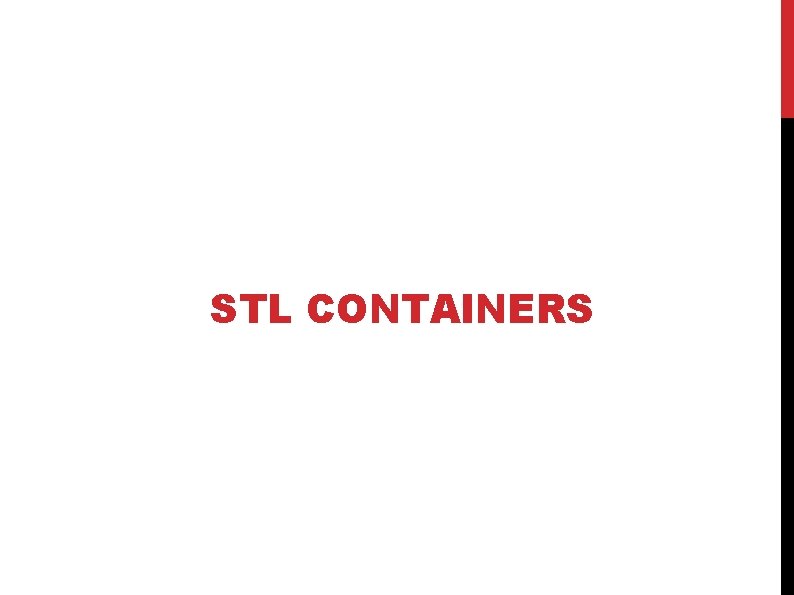
![VECTOR random access ([] operator) like an array add / remove an element in VECTOR random access ([] operator) like an array add / remove an element in](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-4.jpg)
![VECTOR OPERATIONS #include <vector> int main() { vector<int> v; v. push_back(10); cout<<v[0]<<endl; /* Output: VECTOR OPERATIONS #include <vector> int main() { vector<int> v; v. push_back(10); cout<<v[0]<<endl; /* Output:](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-5.jpg)
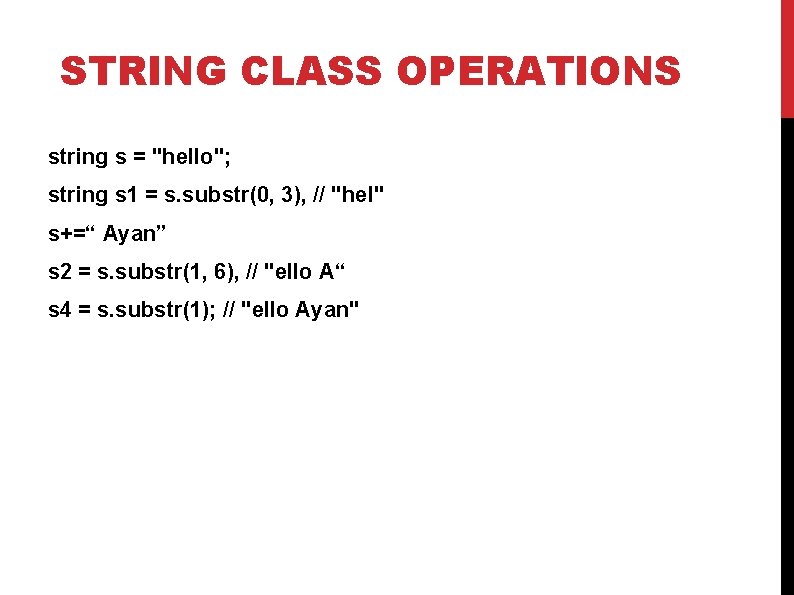
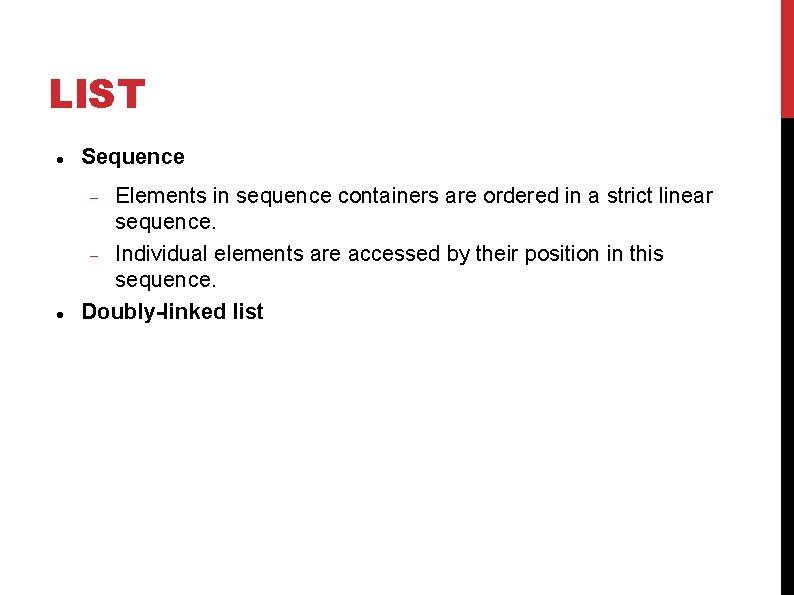
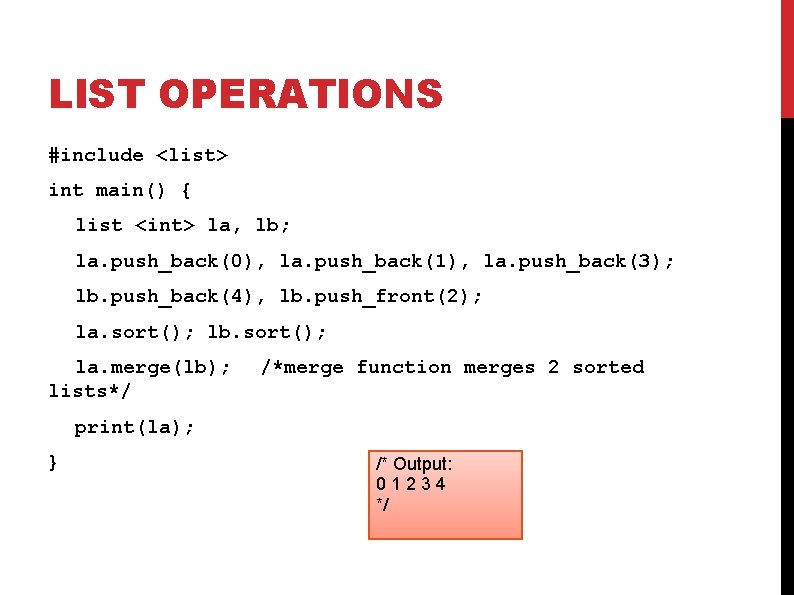
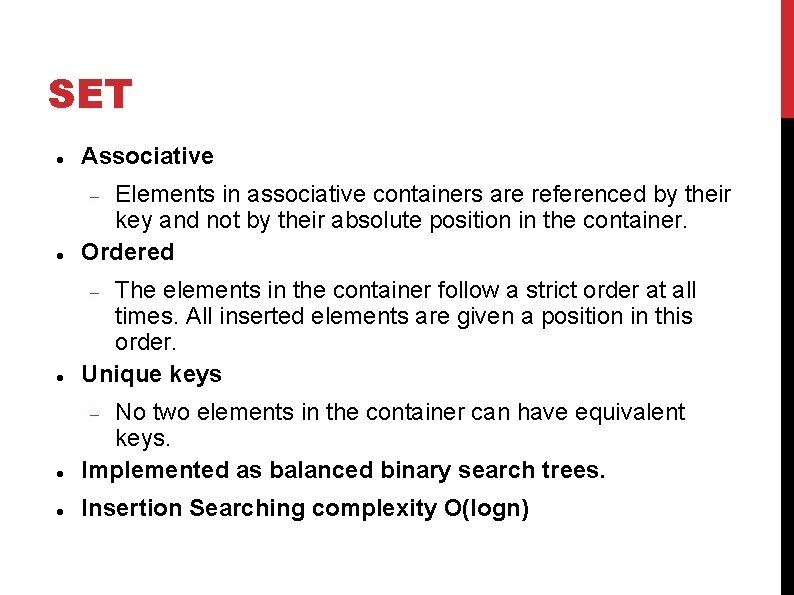
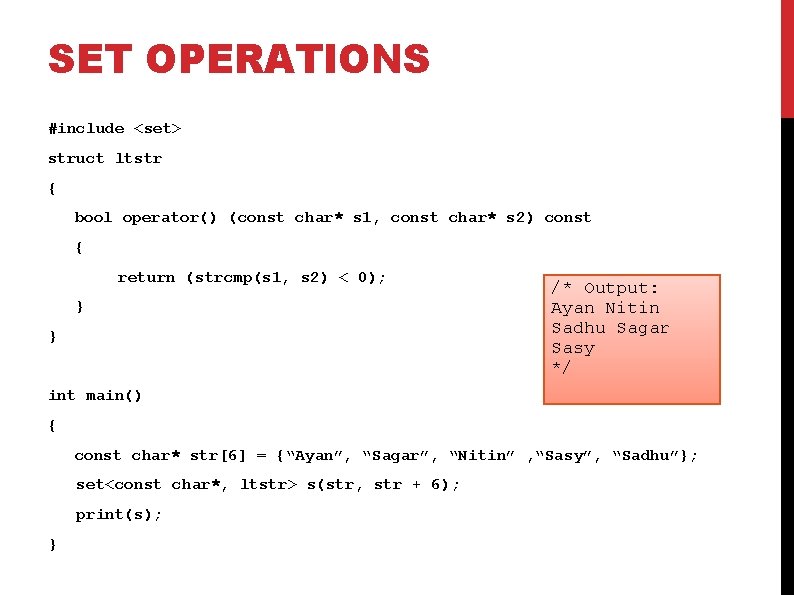
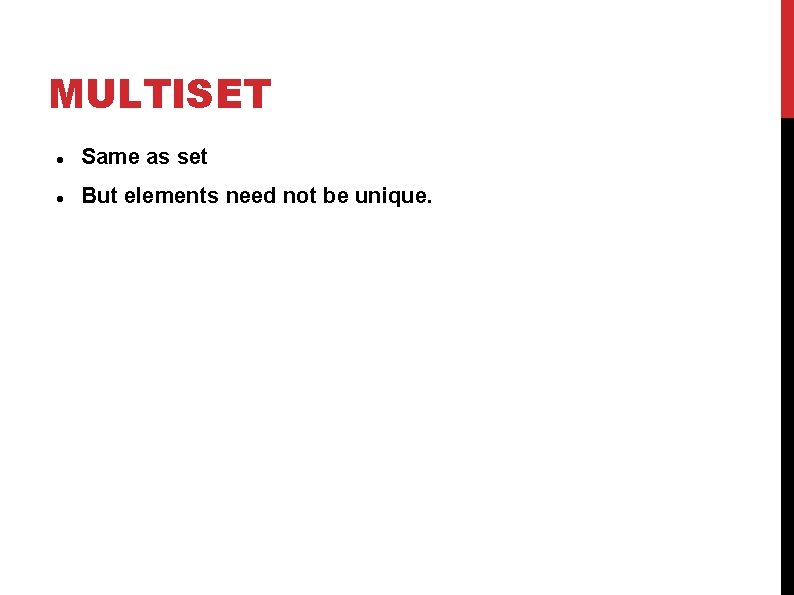
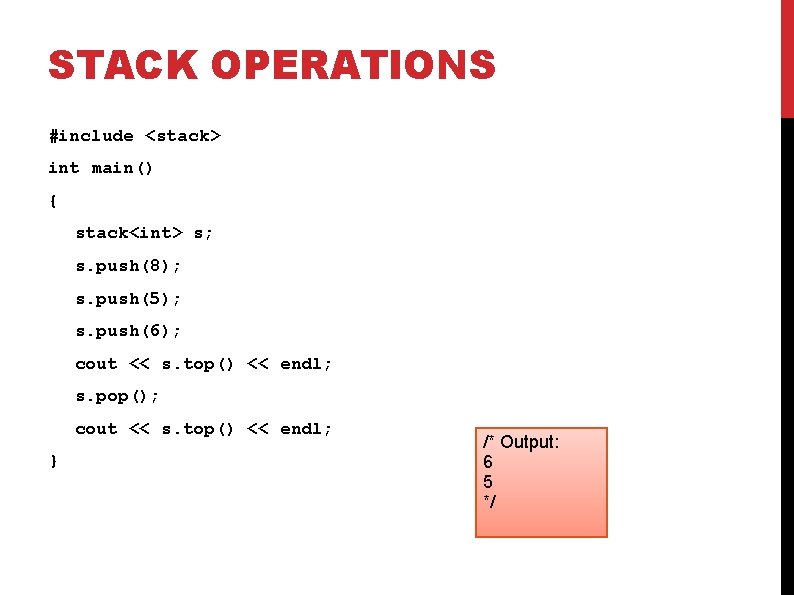
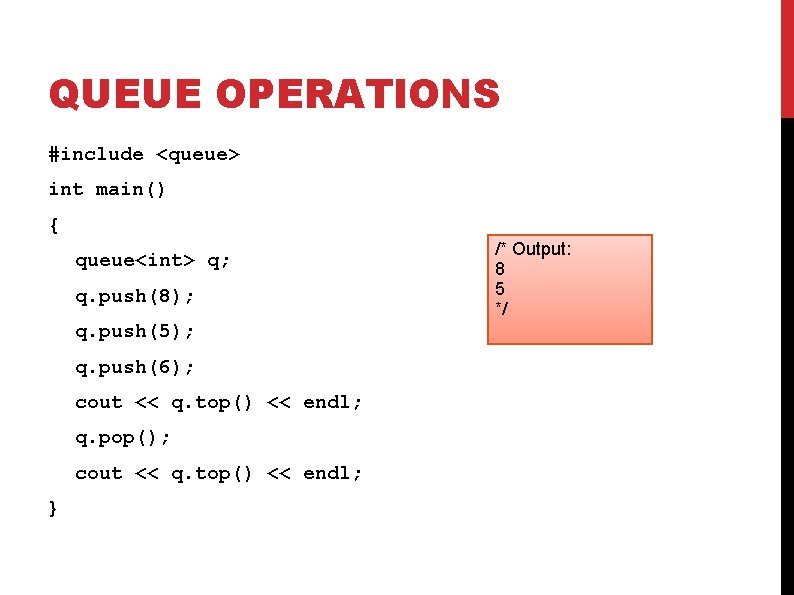
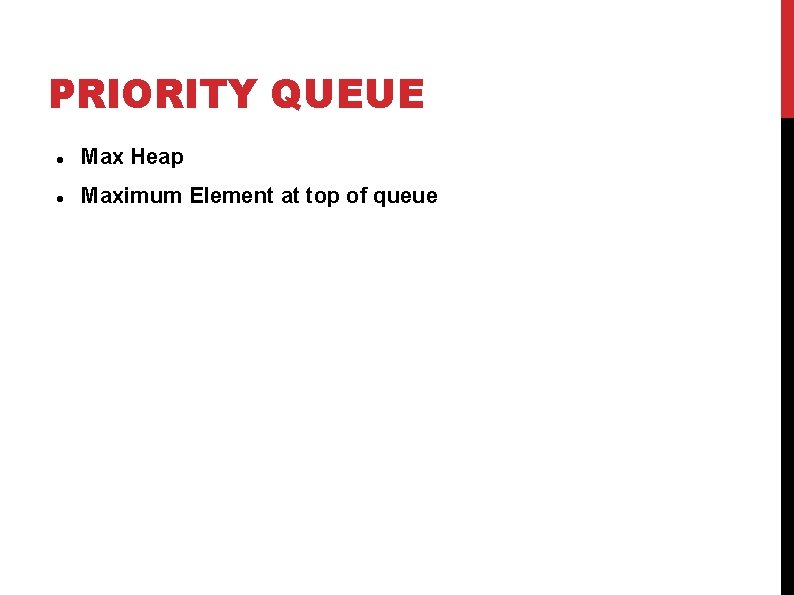
![PRIORITY_QUEUE OPERATIONS #include <queue> int main() { priority_queue< int > pq; int ary[6] = PRIORITY_QUEUE OPERATIONS #include <queue> int main() { priority_queue< int > pq; int ary[6] =](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-15.jpg)
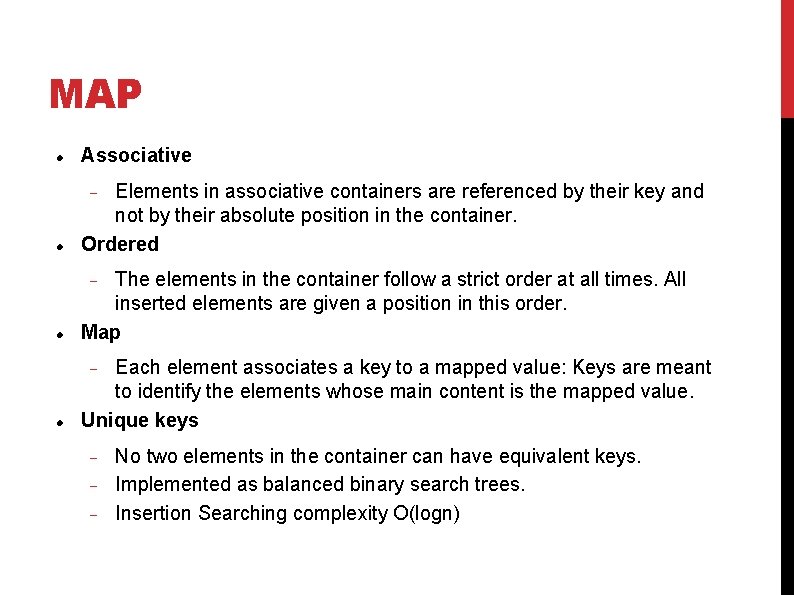
![MAP OPERATIONS #include <map> int main() { map<string, int> grade; grade[“Mark”] = 95; grade[“Edward”] MAP OPERATIONS #include <map> int main() { map<string, int> grade; grade[“Mark”] = 95; grade[“Edward”]](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-17.jpg)
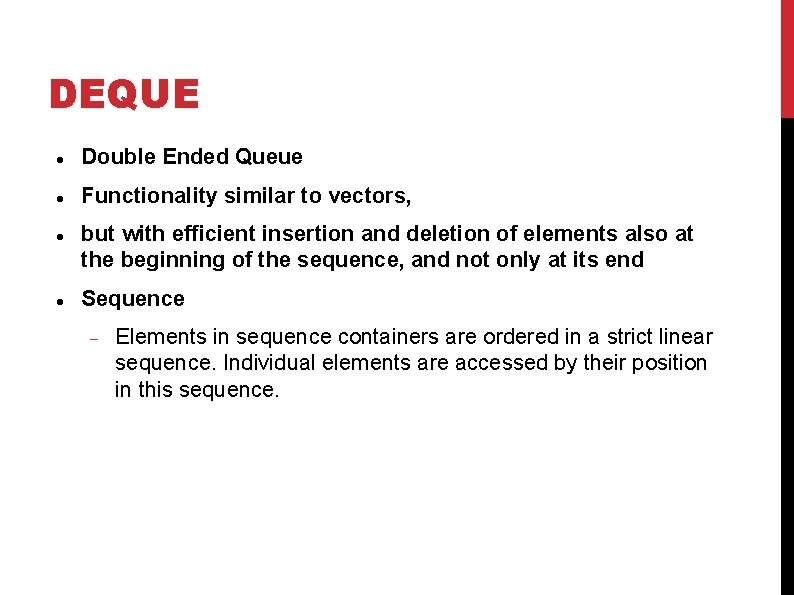
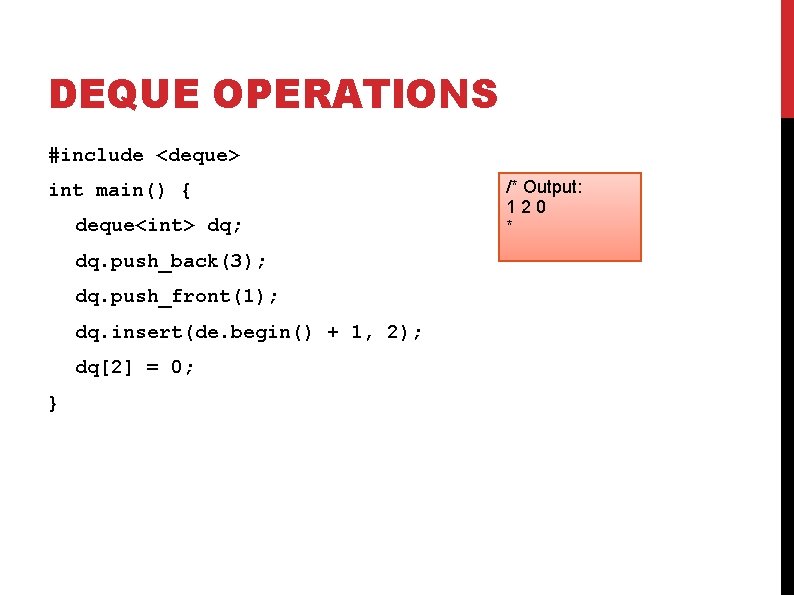
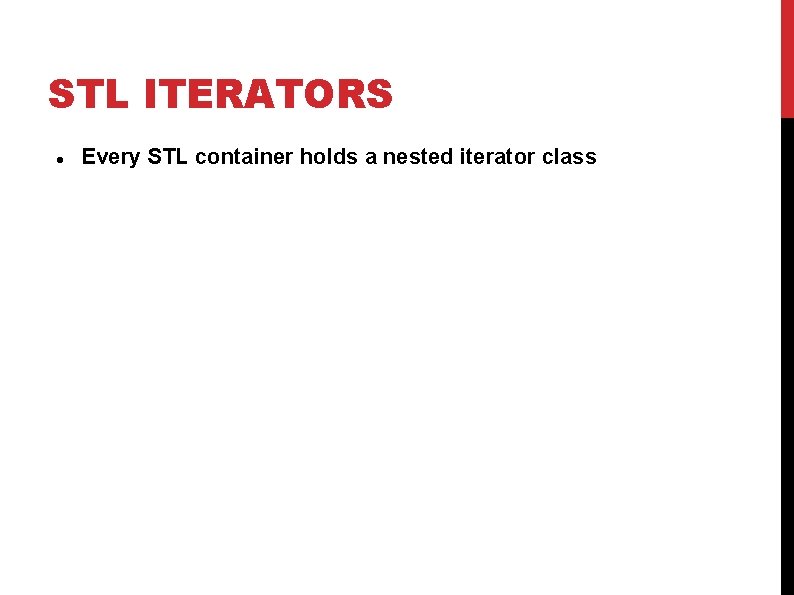
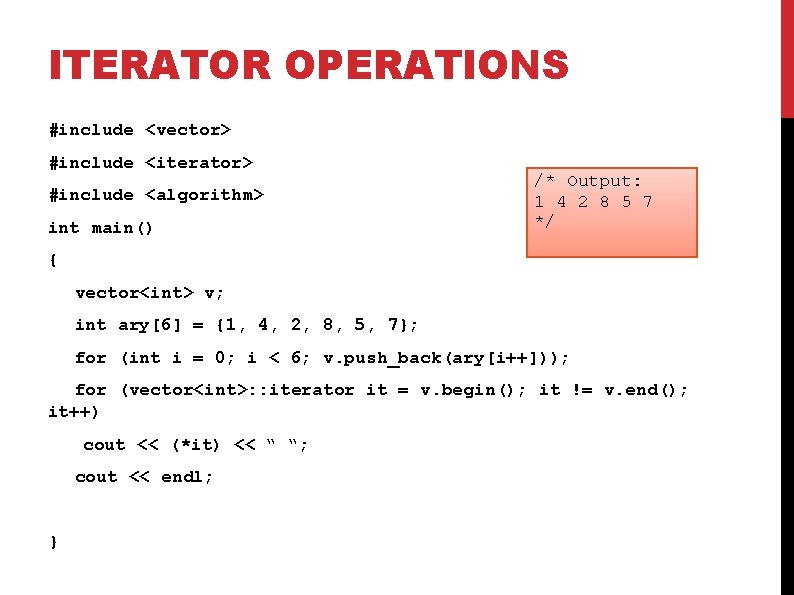
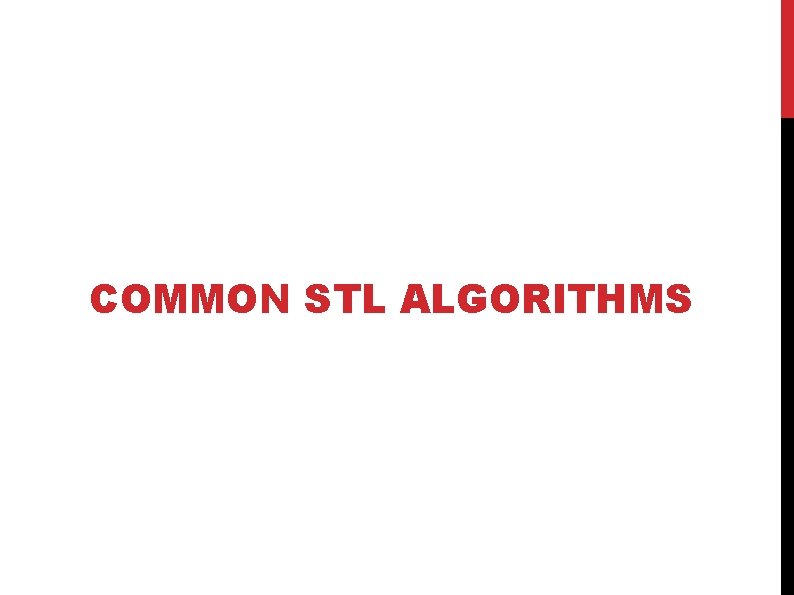
![FIND int main () { int myints[] = { 10, 20, 30 , 40 FIND int main () { int myints[] = { 10, 20, 30 , 40](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-23.jpg)
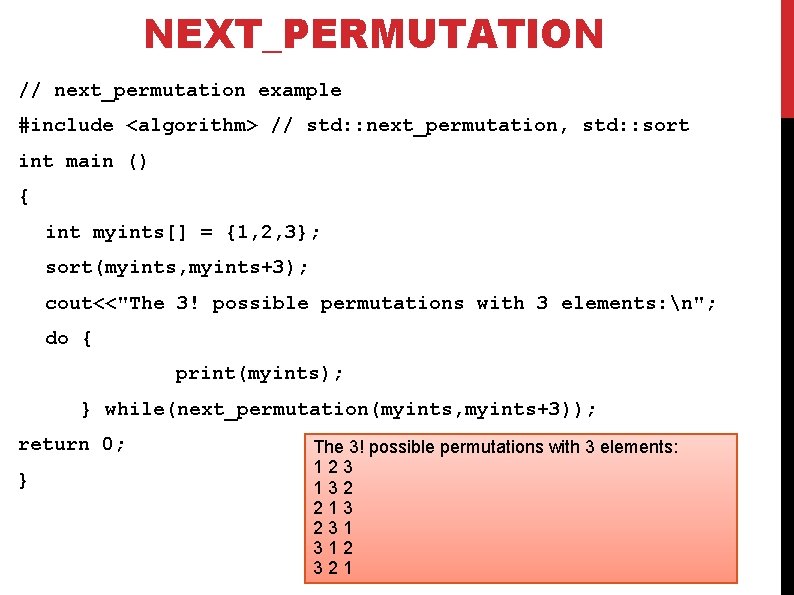
![SORT() #include<algorithm> int main() { int ar[5]={3, 2, 4, 1, 5}; vector<int> v; for(int SORT() #include<algorithm> int main() { int ar[5]={3, 2, 4, 1, 5}; vector<int> v; for(int](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-25.jpg)
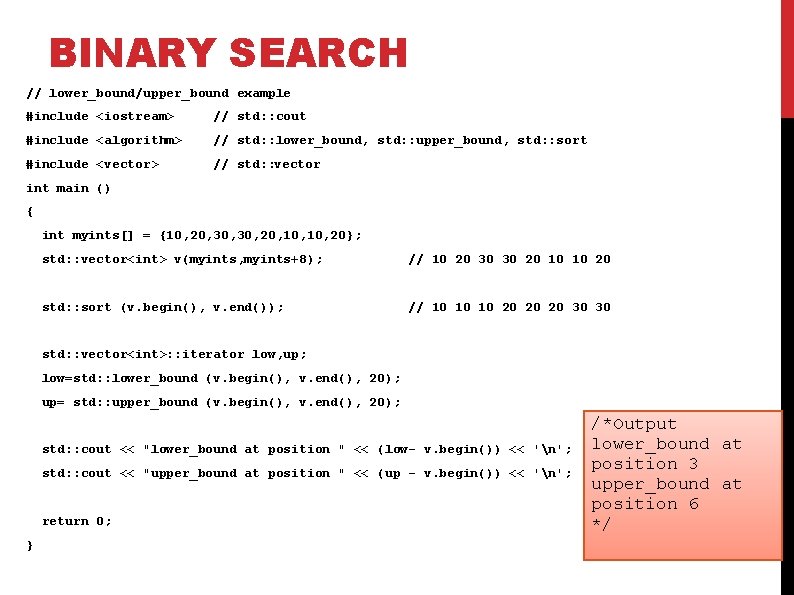
![A SMALL EXAMPLE vector<pair<int, int> > v(5); for(int i=0; i<5; i++) v[i]=make_pair(5 -i, i); A SMALL EXAMPLE vector<pair<int, int> > v(5); for(int i=0; i<5; i++) v[i]=make_pair(5 -i, i);](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-27.jpg)
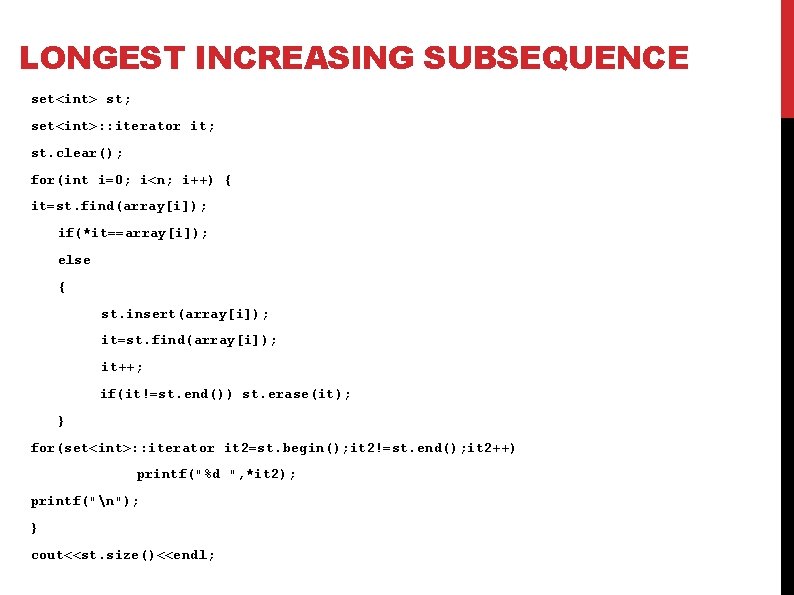
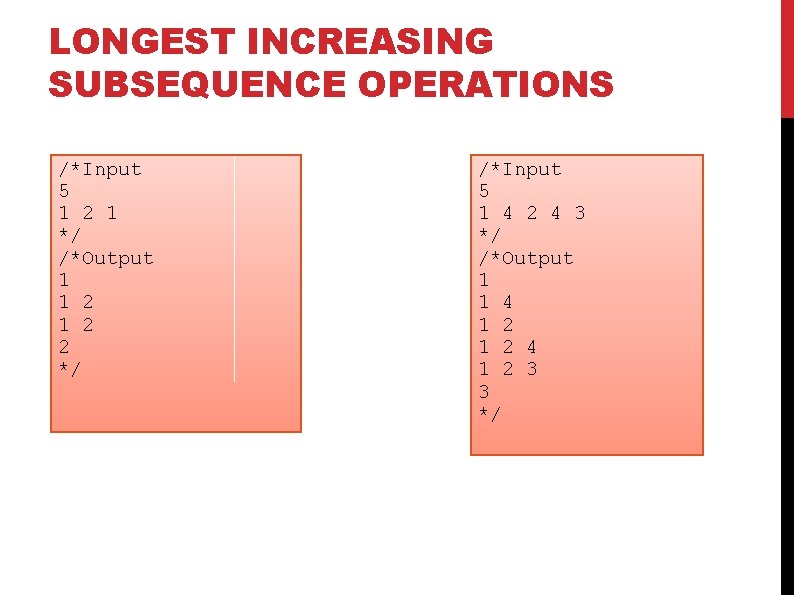
- Slides: 29
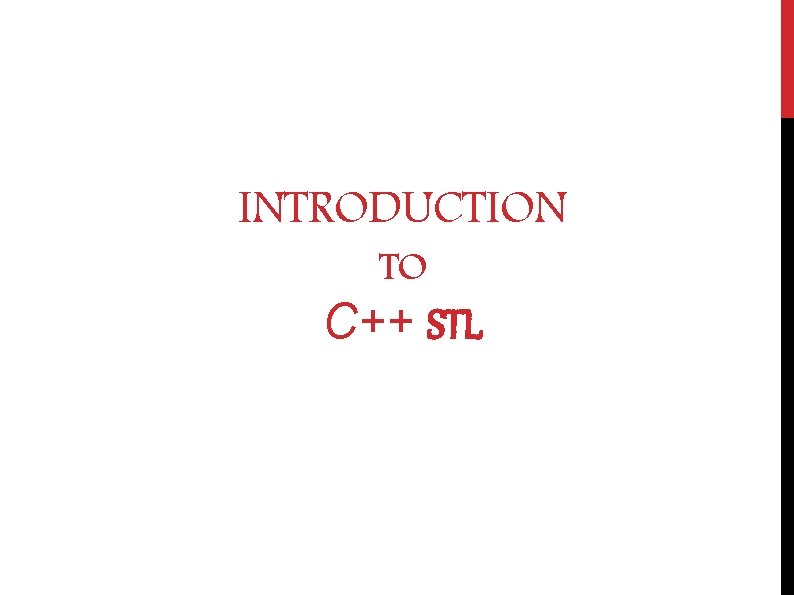
INTRODUCTION TO C++ STL
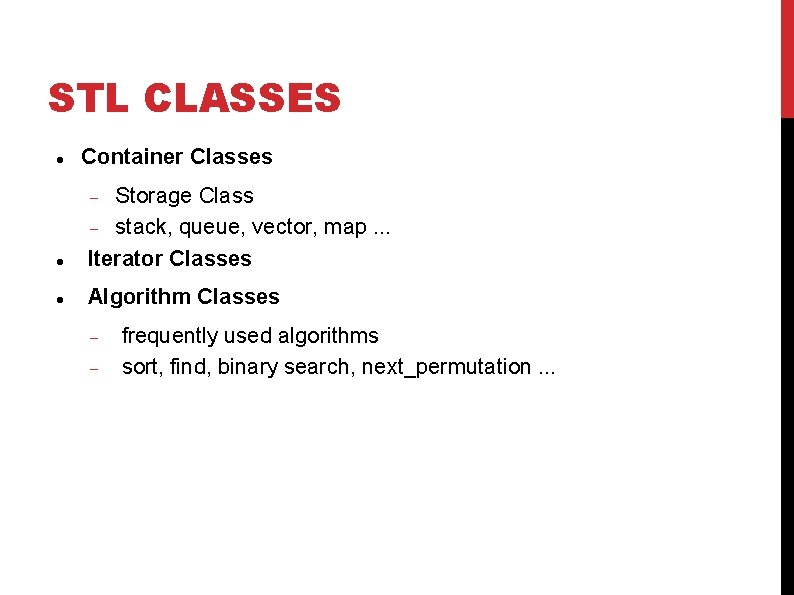
STL CLASSES Container Classes Storage Class stack, queue, vector, map. . . Iterator Classes Algorithm Classes frequently used algorithms sort, find, binary search, next_permutation. . .
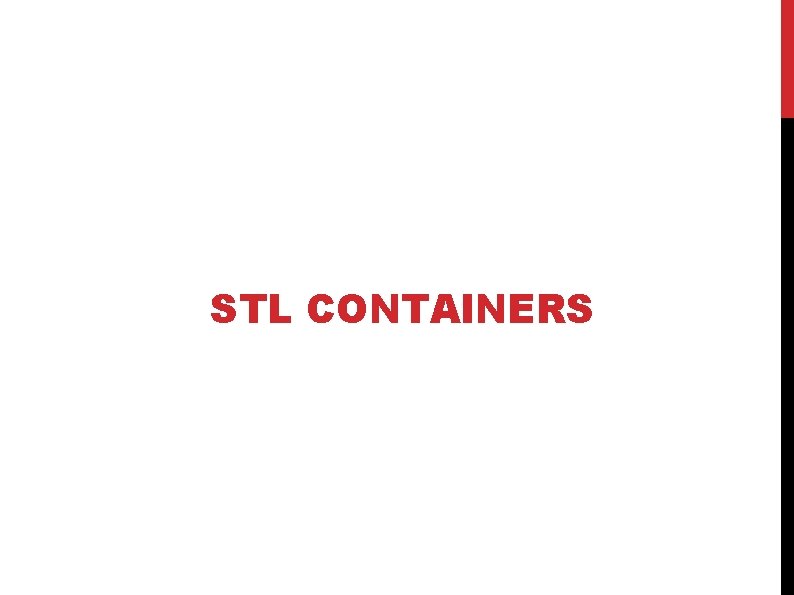
STL CONTAINERS
![VECTOR random access operator like an array add remove an element in VECTOR random access ([] operator) like an array add / remove an element in](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-4.jpg)
VECTOR random access ([] operator) like an array add / remove an element in constant time at the last of vector add / remove an element in linear time in the middle of vector automatic memory management needn’t specify quantity of elements
![VECTOR OPERATIONS include vector int main vectorint v v pushback10 coutv0endl Output VECTOR OPERATIONS #include <vector> int main() { vector<int> v; v. push_back(10); cout<<v[0]<<endl; /* Output:](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-5.jpg)
VECTOR OPERATIONS #include <vector> int main() { vector<int> v; v. push_back(10); cout<<v[0]<<endl; /* Output: 10 10 20 22 10 12 */ v. push_back(20); cout<<v[0]<<” “<<v[1]<<endl; cout << v. size() << “ “ << v. capacity() << endl; v. pop_back(); print(v); cout << v. size() << “ “ << v. capacity() << endl; }
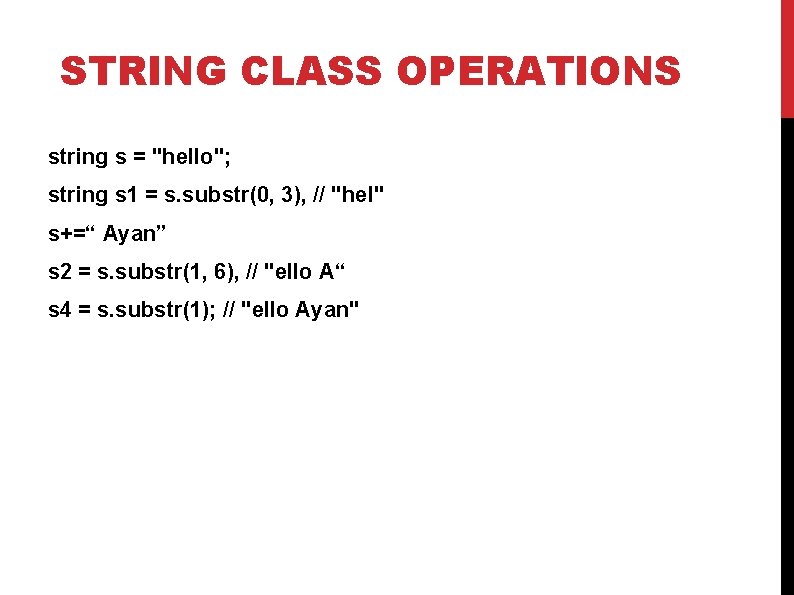
STRING CLASS OPERATIONS string s = "hello"; string s 1 = s. substr(0, 3), // "hel" s+=“ Ayan” s 2 = s. substr(1, 6), // "ello A“ s 4 = s. substr(1); // "ello Ayan"
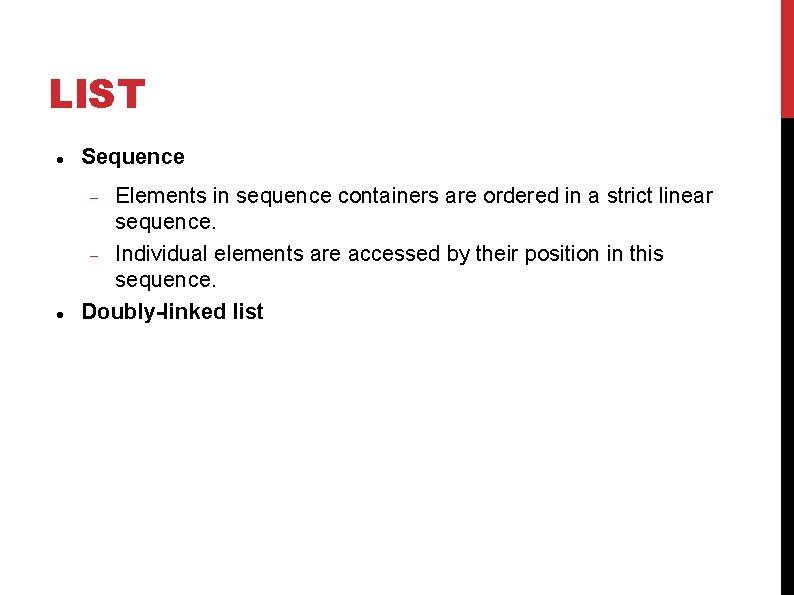
LIST Sequence Elements in sequence containers are ordered in a strict linear sequence. Individual elements are accessed by their position in this sequence. Doubly-linked list
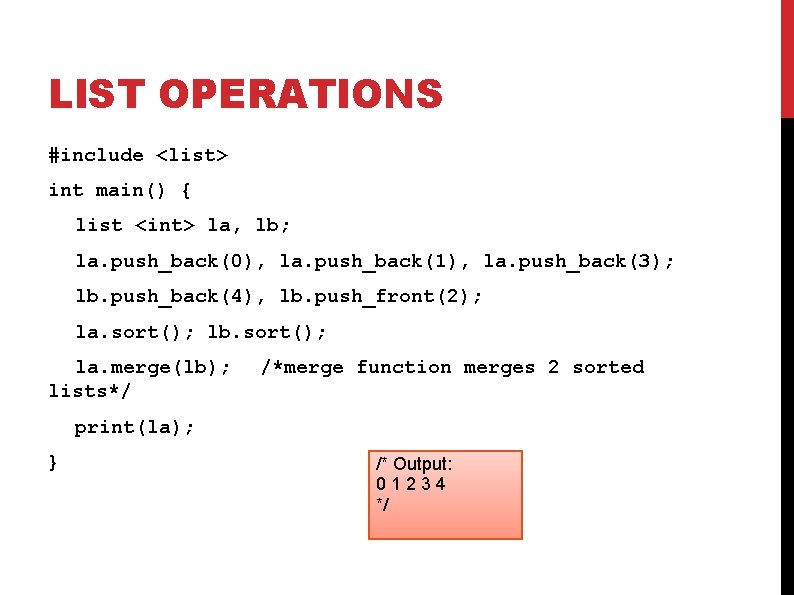
LIST OPERATIONS #include <list> int main() { list <int> la, lb; la. push_back(0), la. push_back(1), la. push_back(3); lb. push_back(4), lb. push_front(2); la. sort(); lb. sort(); la. merge(lb); lists*/ /*merge function merges 2 sorted print(la); } /* Output: 01234 */
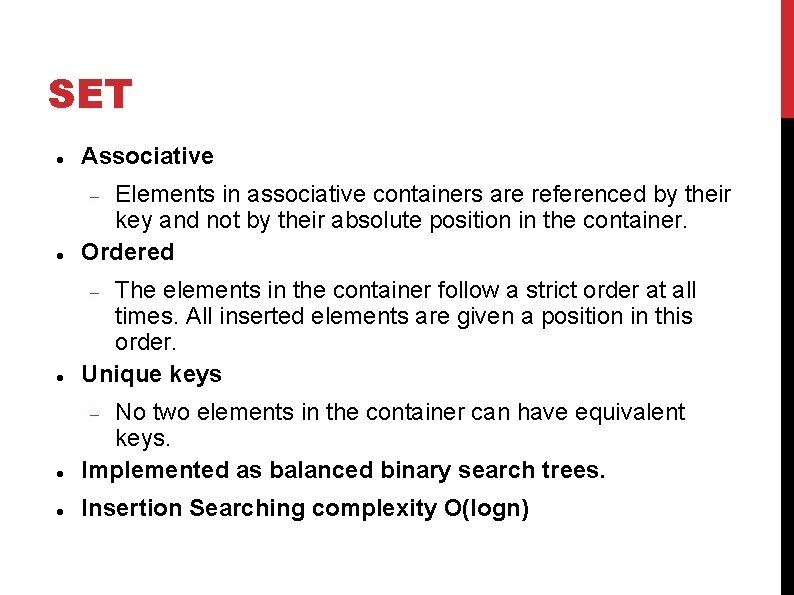
SET Associative Elements in associative containers are referenced by their key and not by their absolute position in the container. Ordered The elements in the container follow a strict order at all times. All inserted elements are given a position in this order. Unique keys No two elements in the container can have equivalent keys. Implemented as balanced binary search trees. Insertion Searching complexity O(logn)
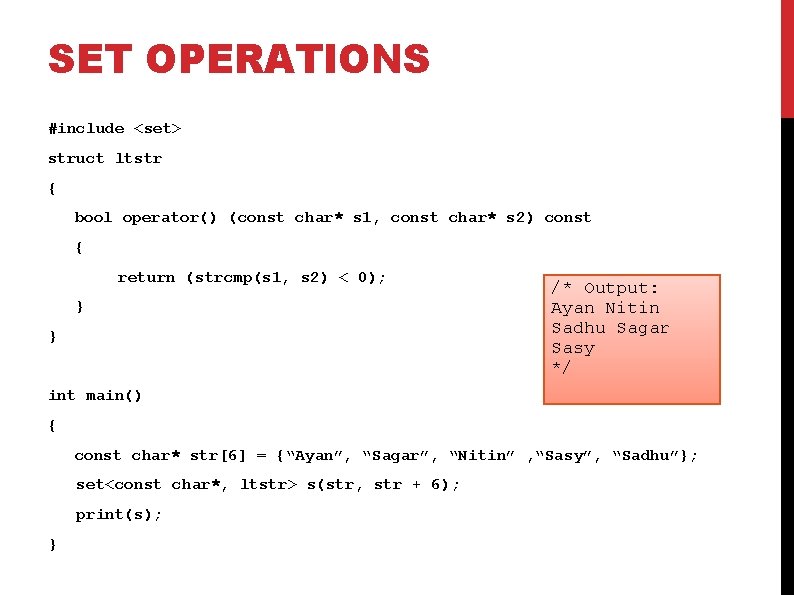
SET OPERATIONS #include <set> struct ltstr { bool operator() (const char* s 1, const char* s 2) const { return (strcmp(s 1, s 2) < 0); } } /* Output: Ayan Nitin Sadhu Sagar Sasy */ int main() { const char* str[6] = {“Ayan”, “Sagar”, “Nitin” , “Sasy”, “Sadhu”}; set<const char*, ltstr> s(str, str + 6); print(s); }
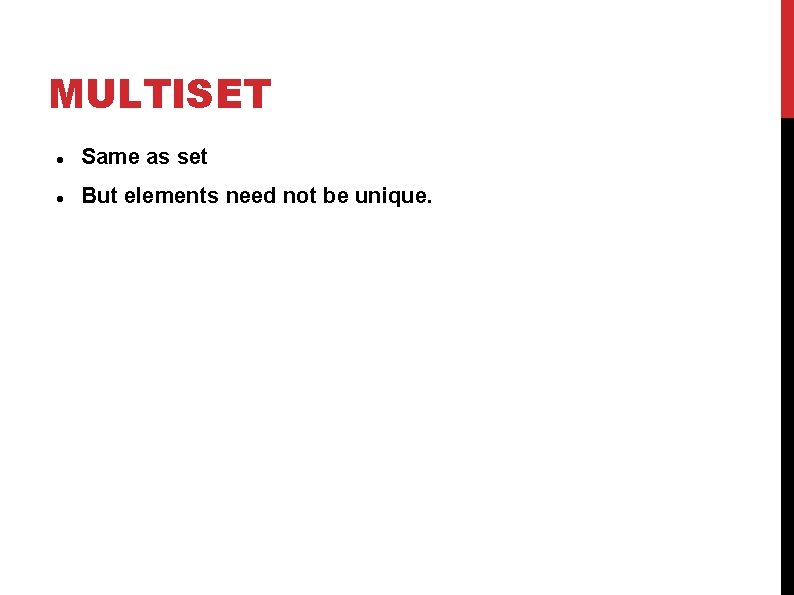
MULTISET Same as set But elements need not be unique.
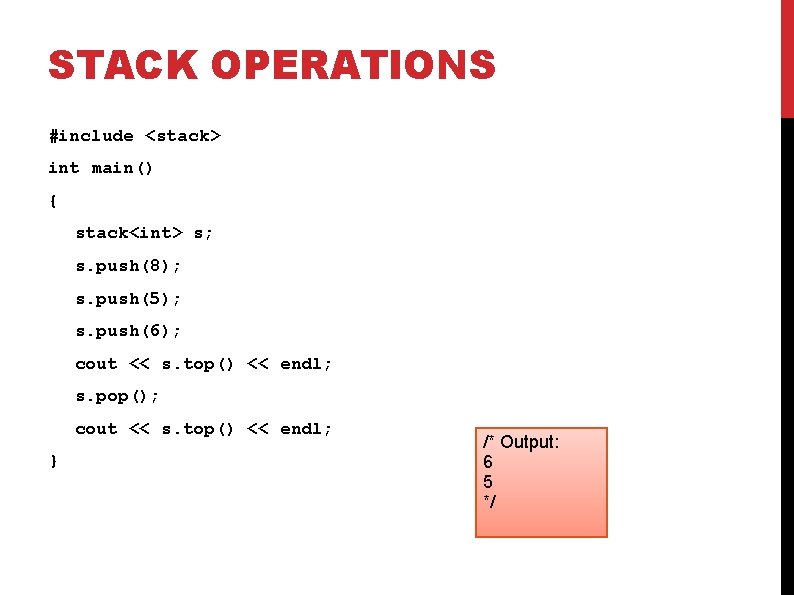
STACK OPERATIONS #include <stack> int main() { stack<int> s; s. push(8); s. push(5); s. push(6); cout << s. top() << endl; s. pop(); cout << s. top() << endl; } /* Output: 6 5 */
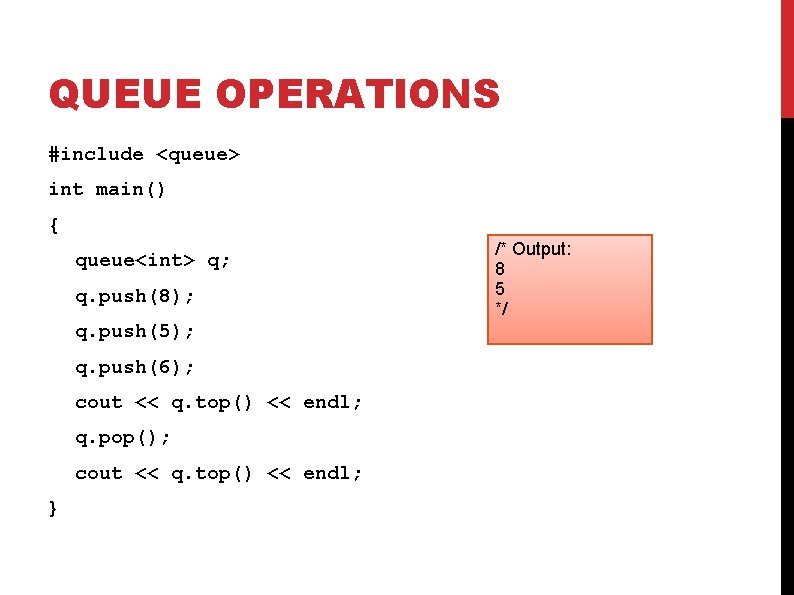
QUEUE OPERATIONS #include <queue> int main() { queue<int> q; q. push(8); q. push(5); q. push(6); cout << q. top() << endl; q. pop(); cout << q. top() << endl; } /* Output: 8 5 */
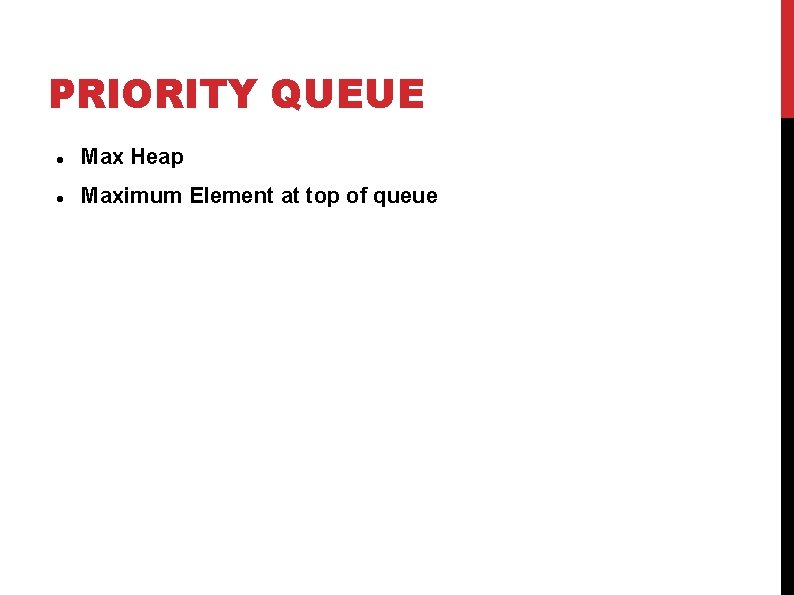
PRIORITY QUEUE Max Heap Maximum Element at top of queue
![PRIORITYQUEUE OPERATIONS include queue int main priorityqueue int pq int ary6 PRIORITY_QUEUE OPERATIONS #include <queue> int main() { priority_queue< int > pq; int ary[6] =](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-15.jpg)
PRIORITY_QUEUE OPERATIONS #include <queue> int main() { priority_queue< int > pq; int ary[6] = {1, 4, 2, 8, 5, 7}; for (int i = 0; i < 6; pq. push(ary[i++])); while (!pq. empty()) { cout << pq. top() << “ “; pq. pop(); } } /* Output: 8 7 5 4 2 1 */
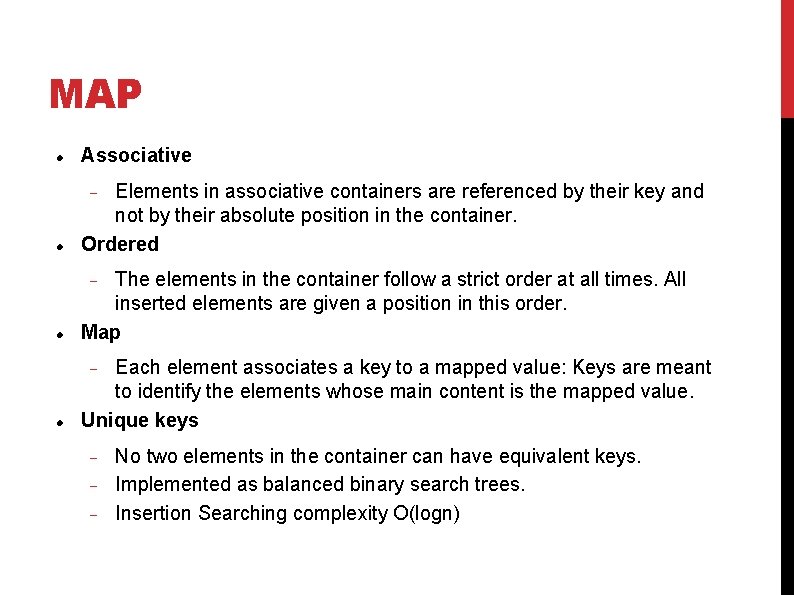
MAP Associative Elements in associative containers are referenced by their key and not by their absolute position in the container. Ordered The elements in the container follow a strict order at all times. All inserted elements are given a position in this order. Map Each element associates a key to a mapped value: Keys are meant to identify the elements whose main content is the mapped value. Unique keys No two elements in the container can have equivalent keys. Implemented as balanced binary search trees. Insertion Searching complexity O(logn)
![MAP OPERATIONS include map int main mapstring int grade gradeMark 95 gradeEdward MAP OPERATIONS #include <map> int main() { map<string, int> grade; grade[“Mark”] = 95; grade[“Edward”]](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-17.jpg)
MAP OPERATIONS #include <map> int main() { map<string, int> grade; grade[“Mark”] = 95; grade[“Edward”] = 87; grade[“Louise”] = 66; /* Output: Allen, 76 Edward, 87 Louise, 66 Mark, 95 76 */ grade[“Allen”] = 76; for(map<string, int> iterator it=grade. begin(); it!=grade. end(); it++) cout<<*it. first<<“ “<<*it. second<<endl; cout << grade[“Allen”] << endl; }
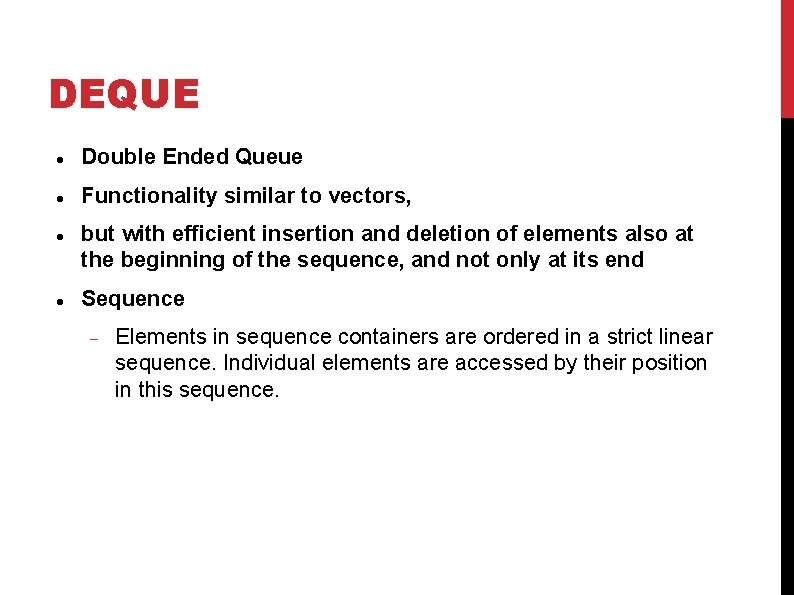
DEQUE Double Ended Queue Functionality similar to vectors, but with efficient insertion and deletion of elements also at the beginning of the sequence, and not only at its end Sequence Elements in sequence containers are ordered in a strict linear sequence. Individual elements are accessed by their position in this sequence.
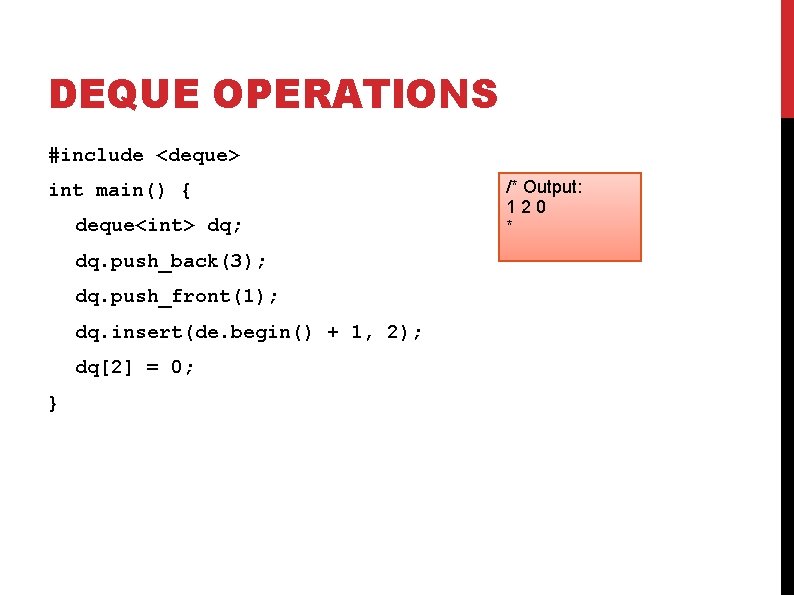
DEQUE OPERATIONS #include <deque> int main() { deque<int> dq; dq. push_back(3); dq. push_front(1); dq. insert(de. begin() + 1, 2); dq[2] = 0; } /* Output: 120 *
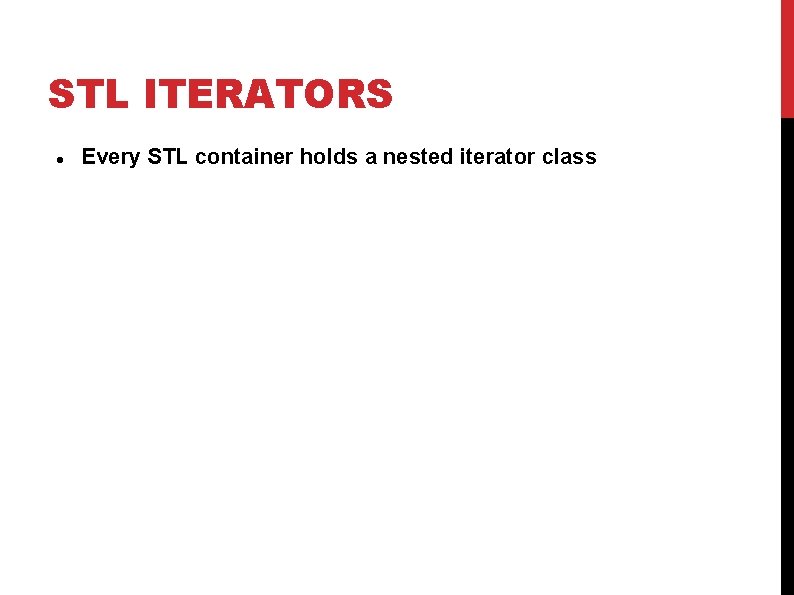
STL ITERATORS Every STL container holds a nested iterator class
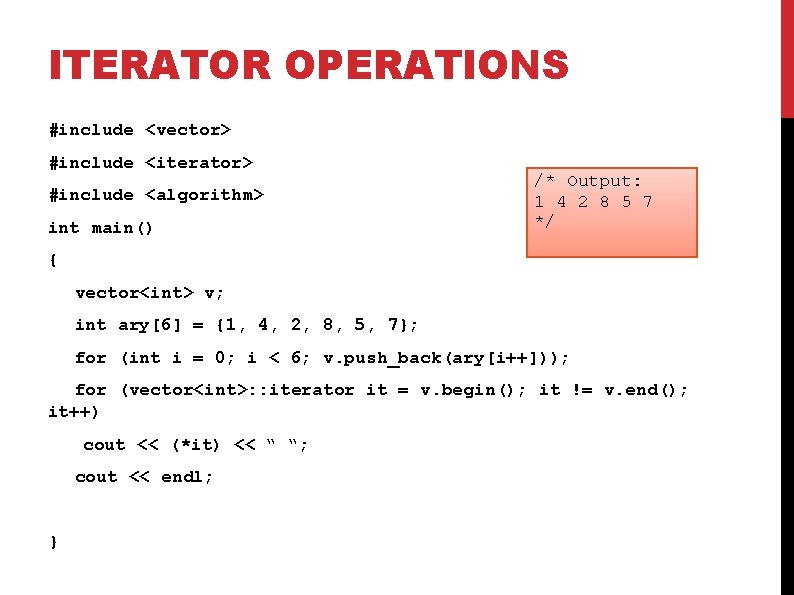
ITERATOR OPERATIONS #include <vector> #include <iterator> #include <algorithm> int main() /* Output: 1 4 2 8 5 7 */ { vector<int> v; int ary[6] = {1, 4, 2, 8, 5, 7}; for (int i = 0; i < 6; v. push_back(ary[i++])); for (vector<int>: : iterator it = v. begin(); it != v. end(); it++) cout << (*it) << “ “; cout << endl; }
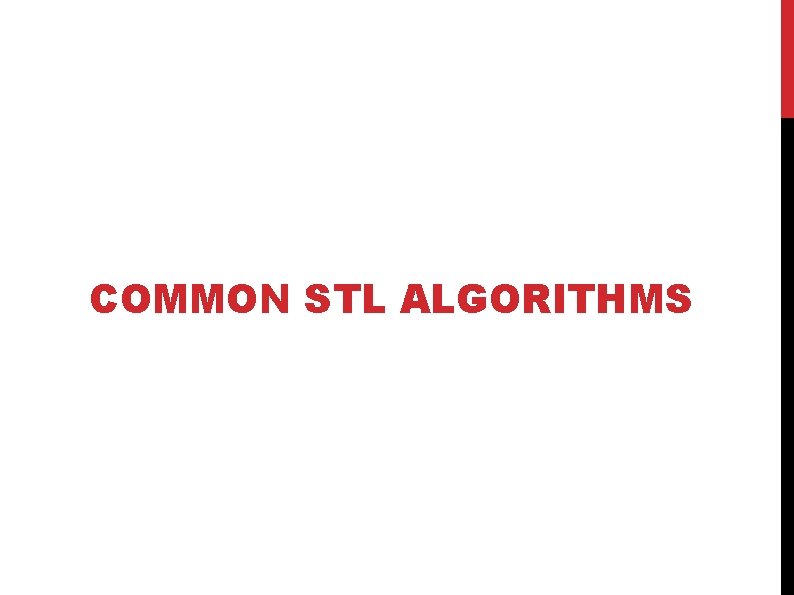
COMMON STL ALGORITHMS
![FIND int main int myints 10 20 30 40 FIND int main () { int myints[] = { 10, 20, 30 , 40](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-23.jpg)
FIND int main () { int myints[] = { 10, 20, 30 , 40 }; int * p; // pointer to array element: p = find (myints, myints+4, 30); ++p; cout << "The element following 30 is " << *p << 'n'; vector<int> myvector (myints, myints+4); vector<int>: : iterator it; // iterator to vector element it = find (myvector. begin(), myvector. end(), 30); ++it; cout << "The element following 30 is " << *it << 'n'; return 0; } The element following 30 is 40
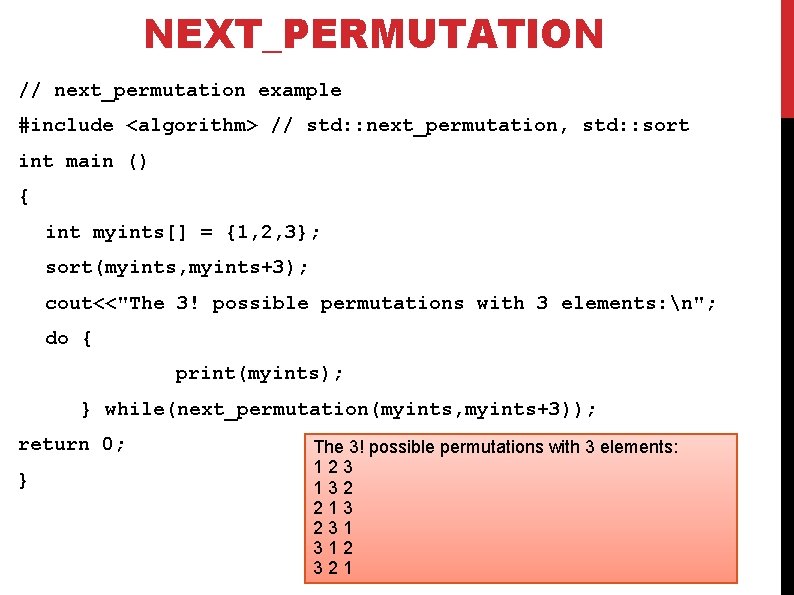
NEXT_PERMUTATION // next_permutation example #include <algorithm> // std: : next_permutation, std: : sort int main () { int myints[] = {1, 2, 3}; sort(myints, myints+3); cout<<"The 3! possible permutations with 3 elements: n"; do { print(myints); } while(next_permutation(myints, myints+3)); return 0; } The 3! possible permutations with 3 elements: 123 132 213 231 312 321
![SORT includealgorithm int main int ar53 2 4 1 5 vectorint v forint SORT() #include<algorithm> int main() { int ar[5]={3, 2, 4, 1, 5}; vector<int> v; for(int](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-25.jpg)
SORT() #include<algorithm> int main() { int ar[5]={3, 2, 4, 1, 5}; vector<int> v; for(int i=0; i<5; i++) v. push_back(ar[i]); sort(ar, ar+5); sort(v. begin(), v. end(), greater<int>); print(ar); print(v); } /* Output 12345 54321 */
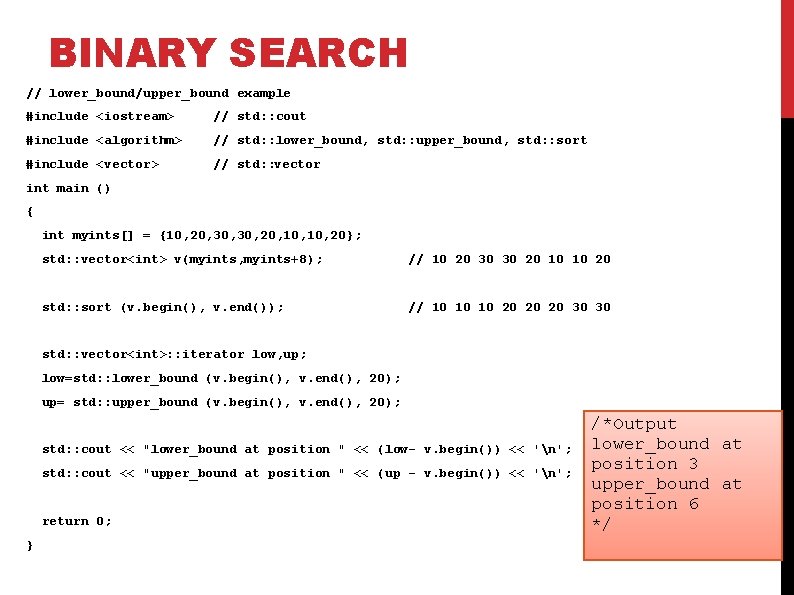
BINARY SEARCH // lower_bound/upper_bound example #include <iostream> // std: : cout #include <algorithm> // std: : lower_bound, std: : upper_bound, std: : sort #include <vector> // std: : vector int main () { int myints[] = {10, 20, 30, 20, 10, 20}; std: : vector<int> v(myints, myints+8); // 10 20 30 30 20 10 10 20 std: : sort (v. begin(), v. end()); // 10 10 10 20 20 20 30 30 std: : vector<int>: : iterator low, up; low=std: : lower_bound (v. begin(), v. end(), 20); up= std: : upper_bound (v. begin(), v. end(), 20); std: : cout << "lower_bound at position " << (low- v. begin()) << 'n'; std: : cout << "upper_bound at position " << (up - v. begin()) << 'n'; return 0; } /*Output lower_bound at position 3 upper_bound at position 6 */
![A SMALL EXAMPLE vectorpairint int v5 forint i0 i5 i vimakepair5 i i A SMALL EXAMPLE vector<pair<int, int> > v(5); for(int i=0; i<5; i++) v[i]=make_pair(5 -i, i);](https://slidetodoc.com/presentation_image/26f4c44e2bf2df69d787a946f7f25299/image-27.jpg)
A SMALL EXAMPLE vector<pair<int, int> > v(5); for(int i=0; i<5; i++) v[i]=make_pair(5 -i, i); sort(v. begin(), v. end()); for(vector<pair<int, int> >: : iterator it=v. begin(); it!=v. end(); it++) cout<<*it. first<<“ “<<*it. second<<endl; for(int i=0; i<5; i++) cout<<v[i]. first<<“ “v[i]. second<<endl; Output 14 23 32 41 50
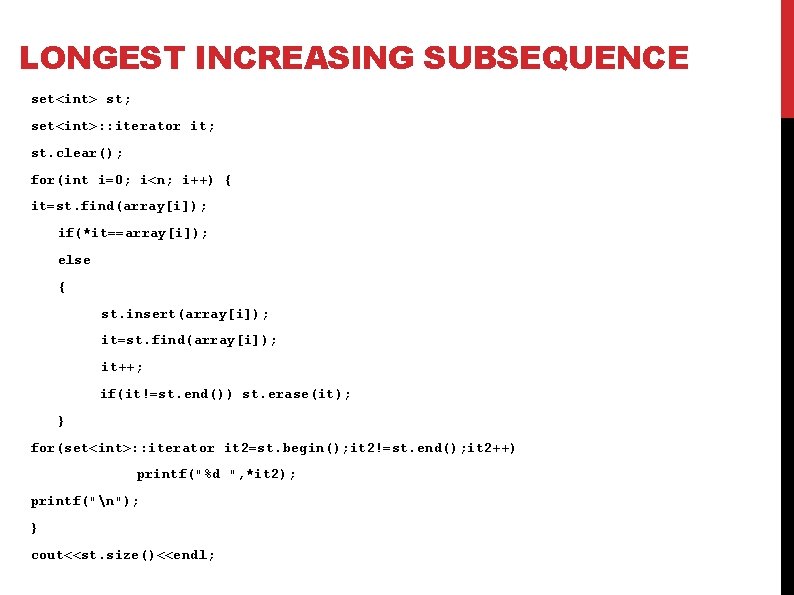
LONGEST INCREASING SUBSEQUENCE set<int> st; set<int>: : iterator it; st. clear(); for(int i=0; i<n; i++) { it=st. find(array[i]); if(*it==array[i]); else { st. insert(array[i]); it=st. find(array[i]); it++; if(it!=st. end()) st. erase(it); } for(set<int>: : iterator it 2=st. begin(); it 2!=st. end(); it 2++) printf("%d ", *it 2); printf("n"); } cout<<st. size()<<endl;
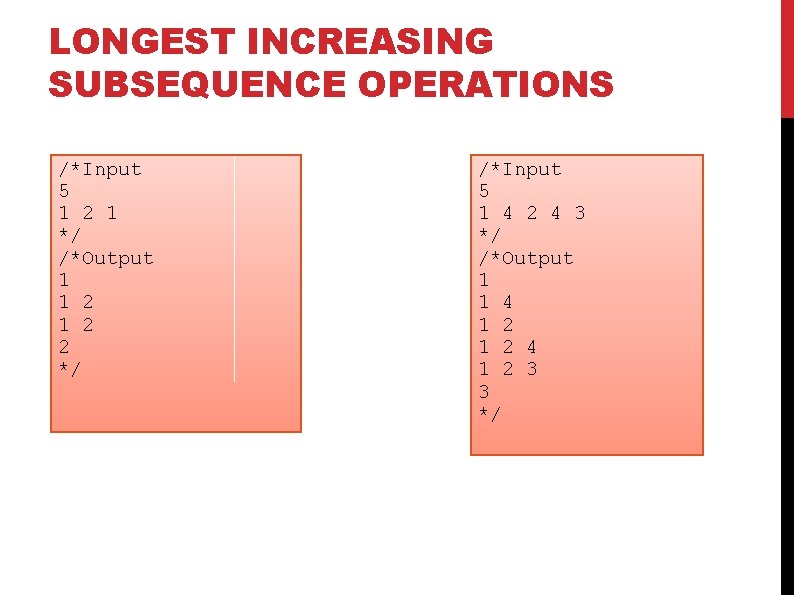
LONGEST INCREASING SUBSEQUENCE OPERATIONS /*Input 5 1 2 1 */ /*Output 1 1 2 2 */ /*Input 5 1 4 2 4 3 */ /*Output 1 1 4 1 2 3 3 */