STL Generic Programming to the extreme TA 5
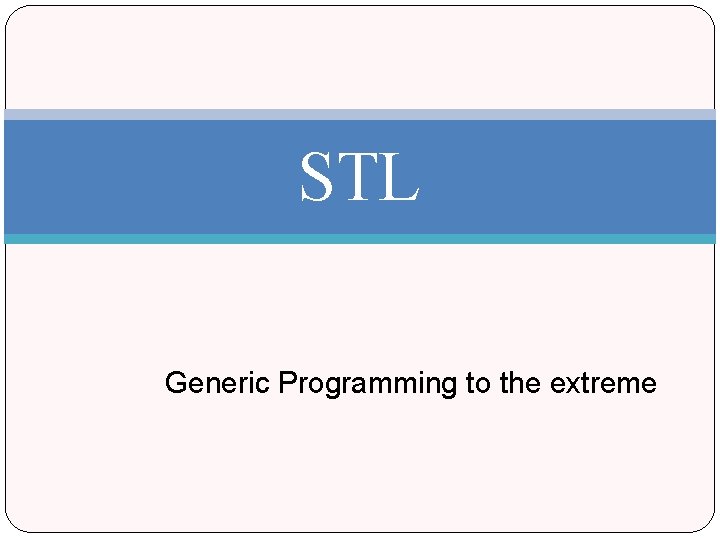
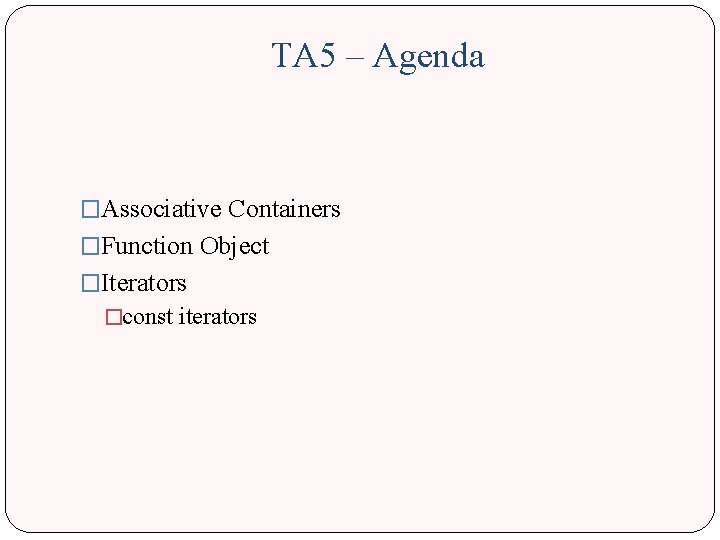
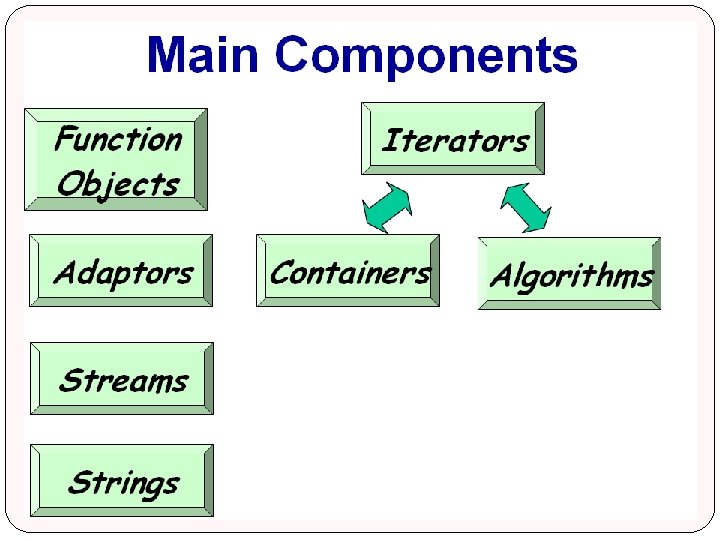
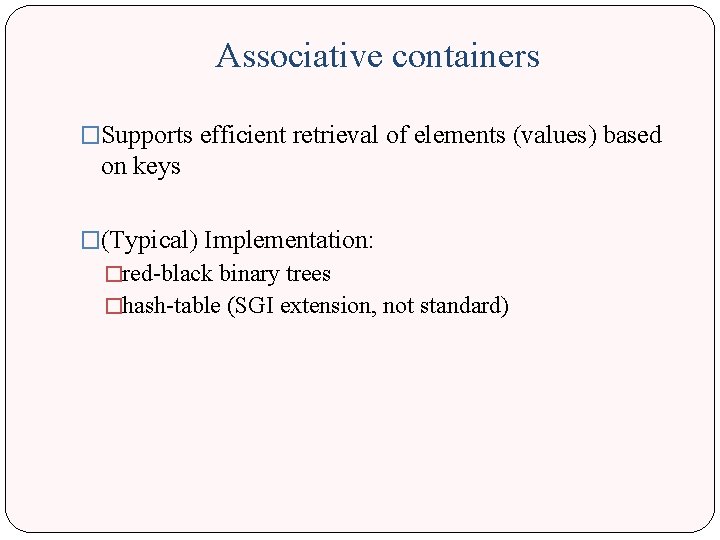
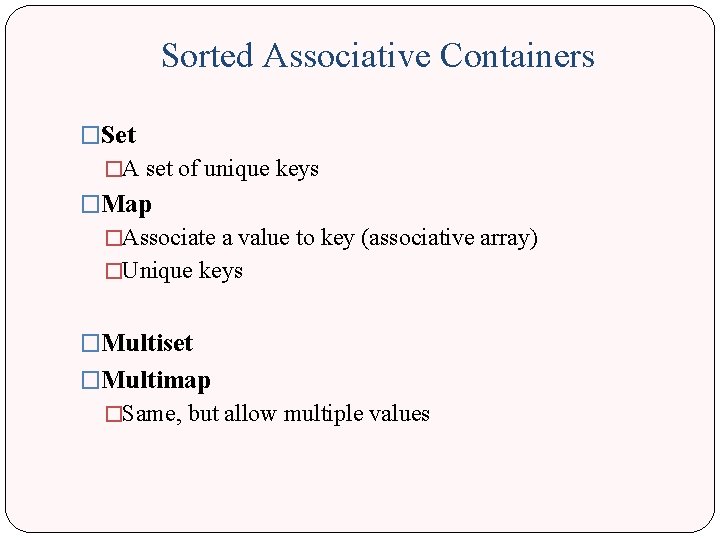
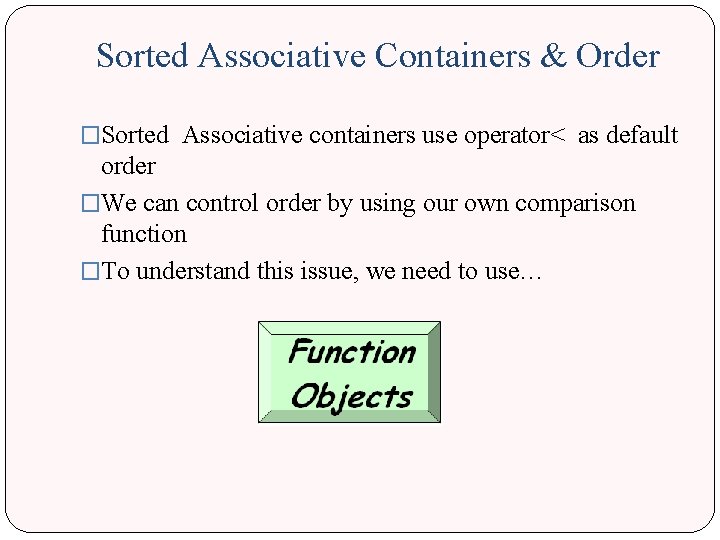
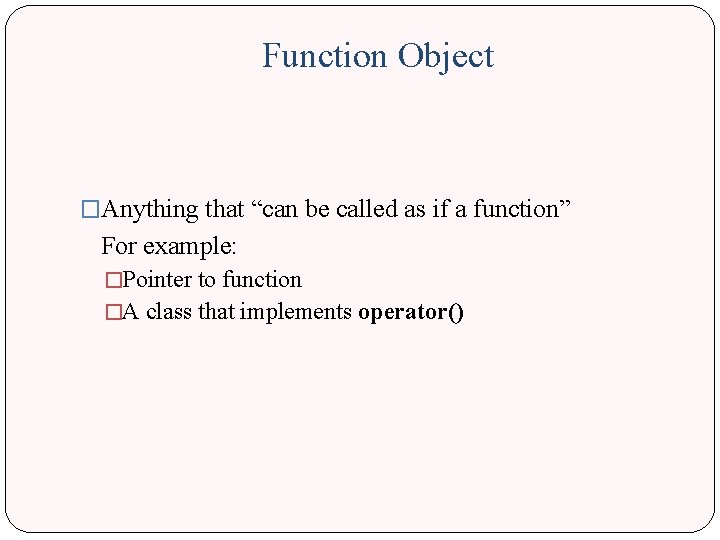
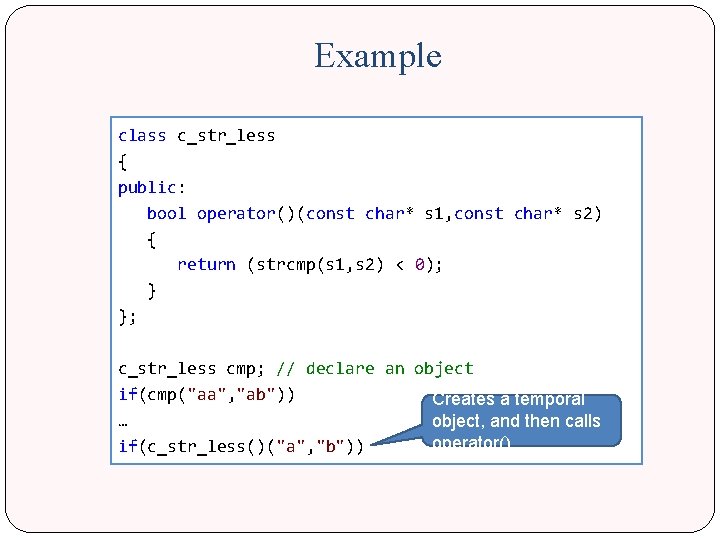
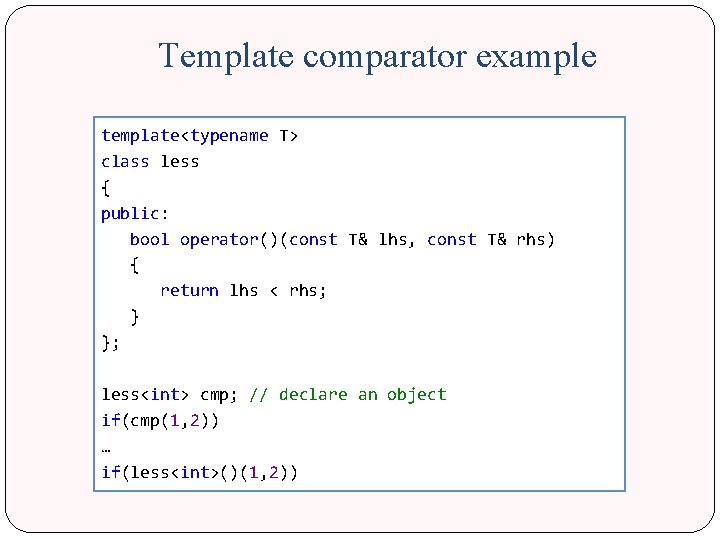
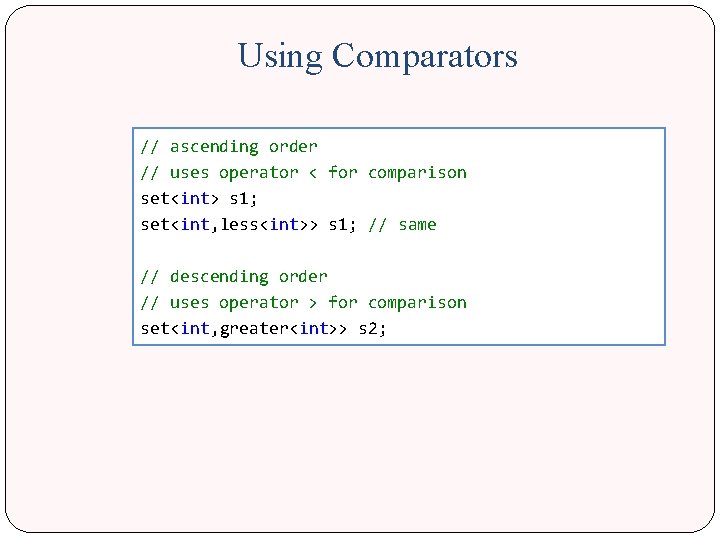
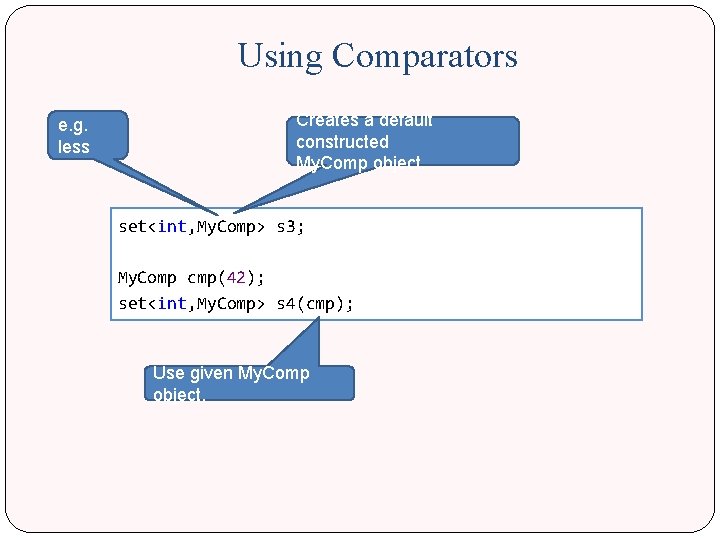
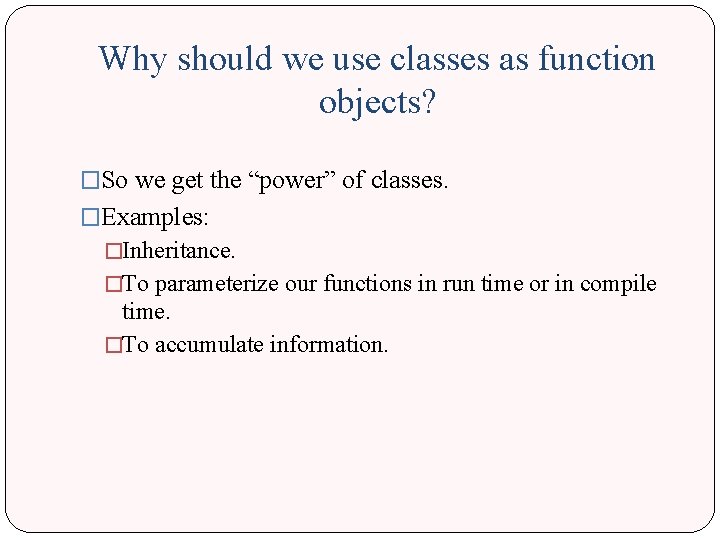
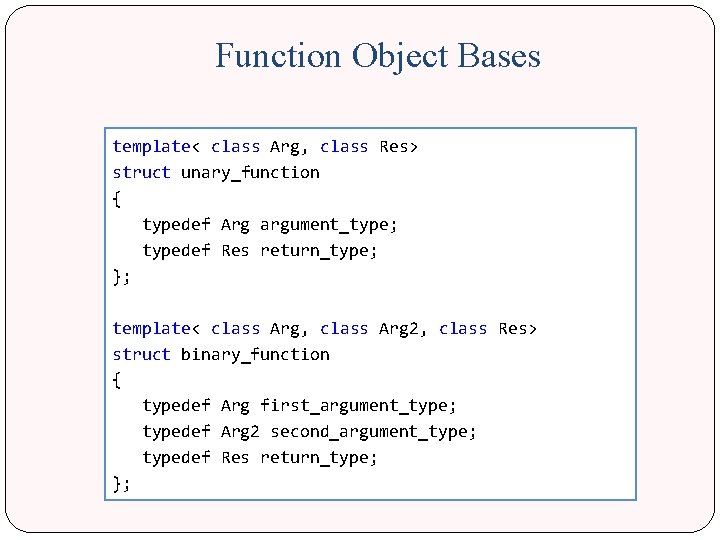
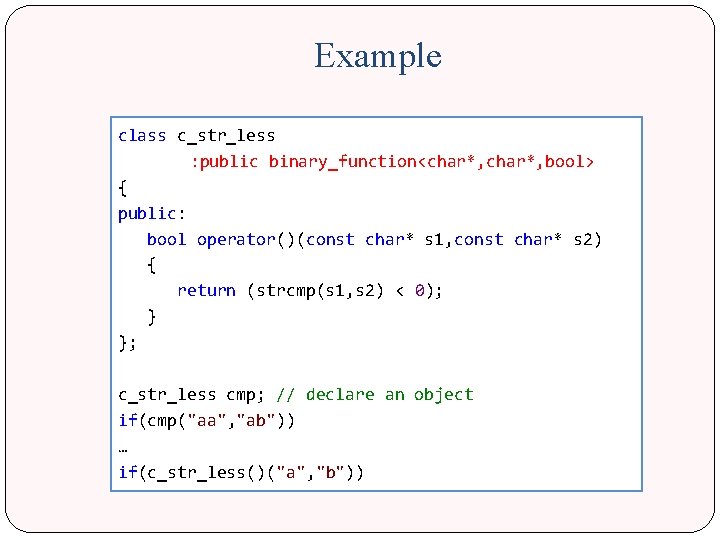
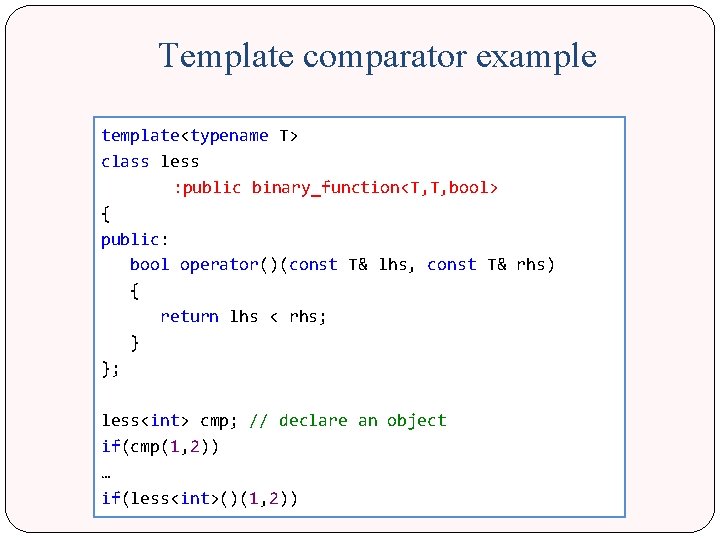
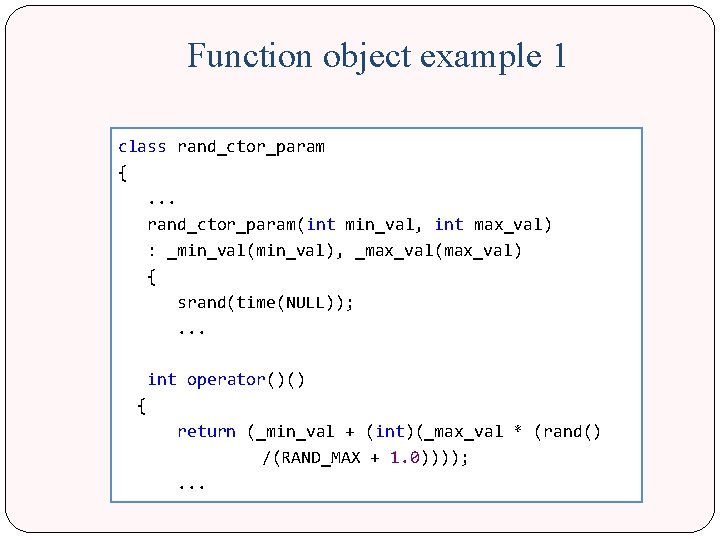
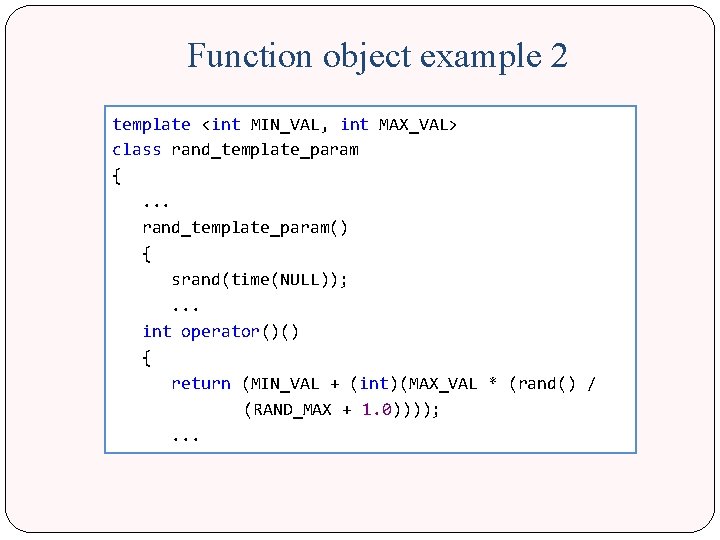
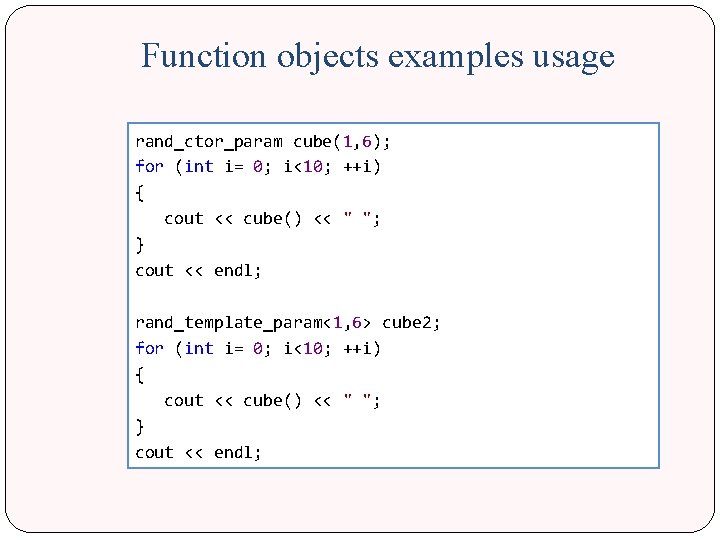
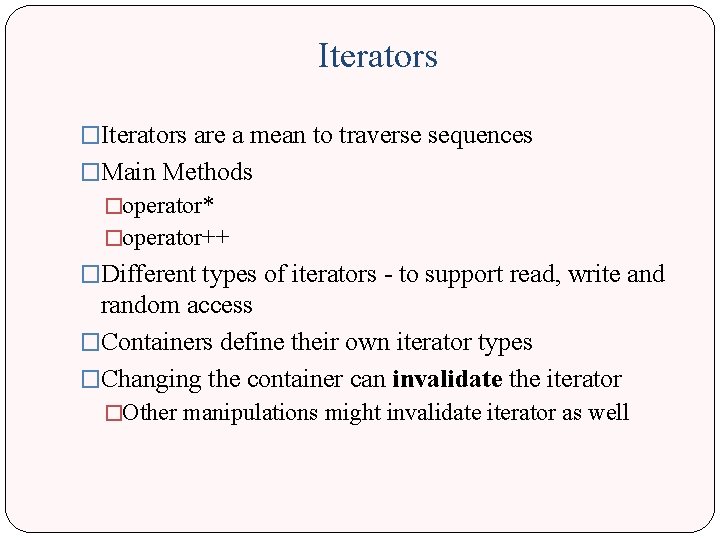
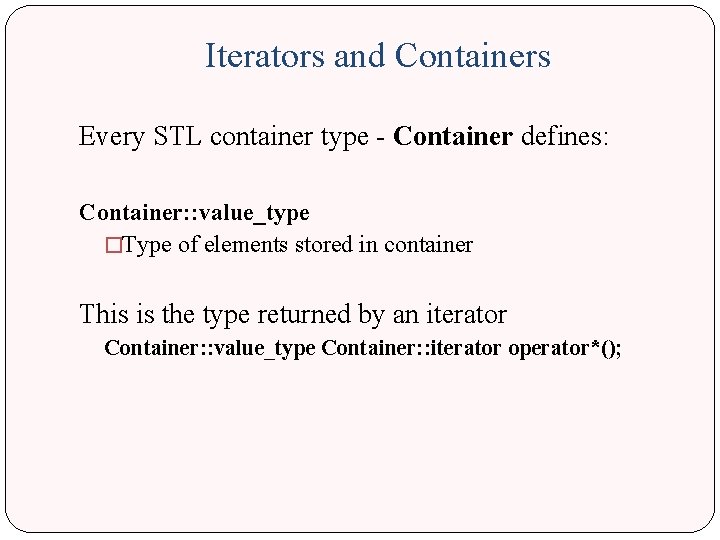
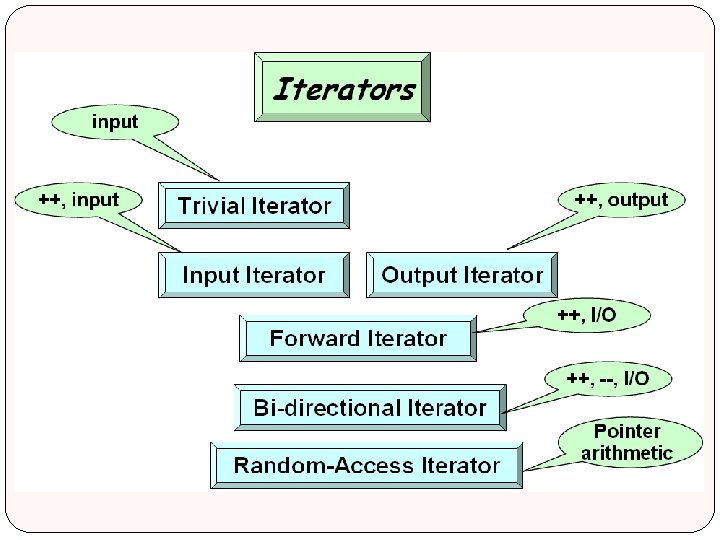
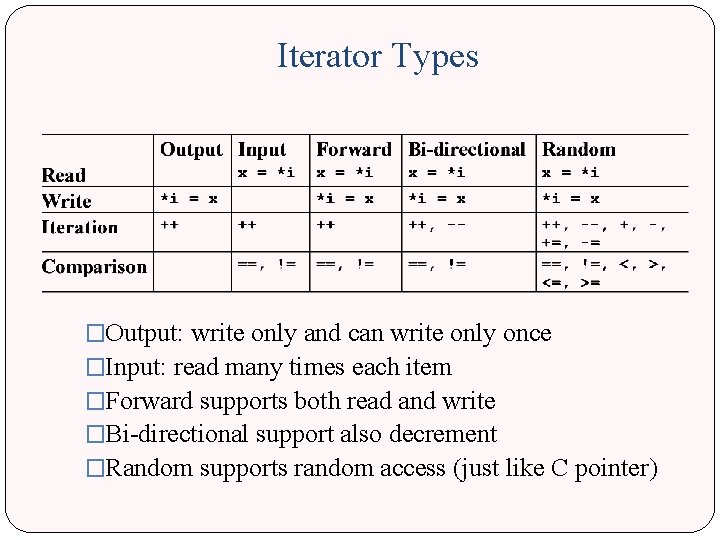
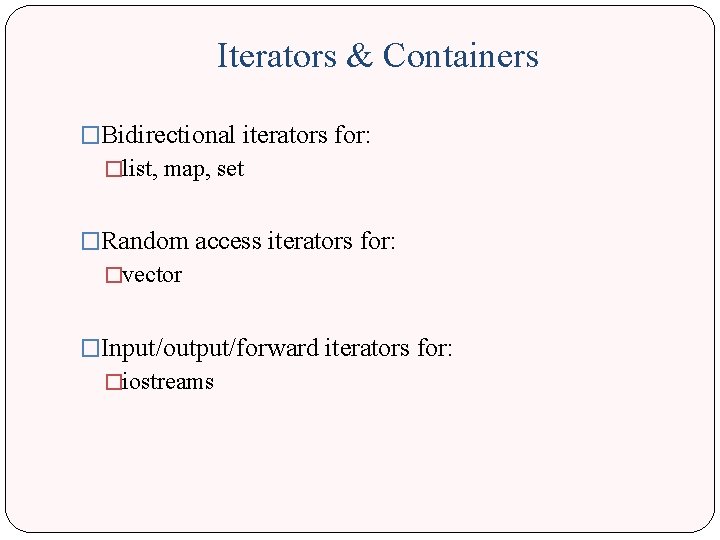
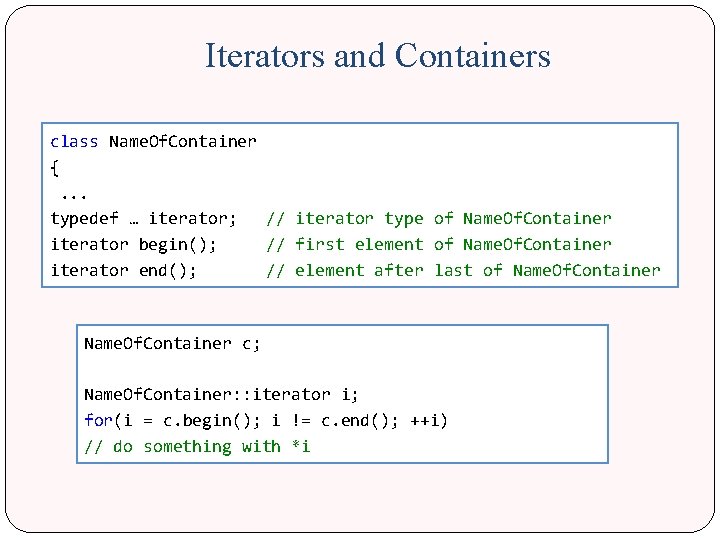
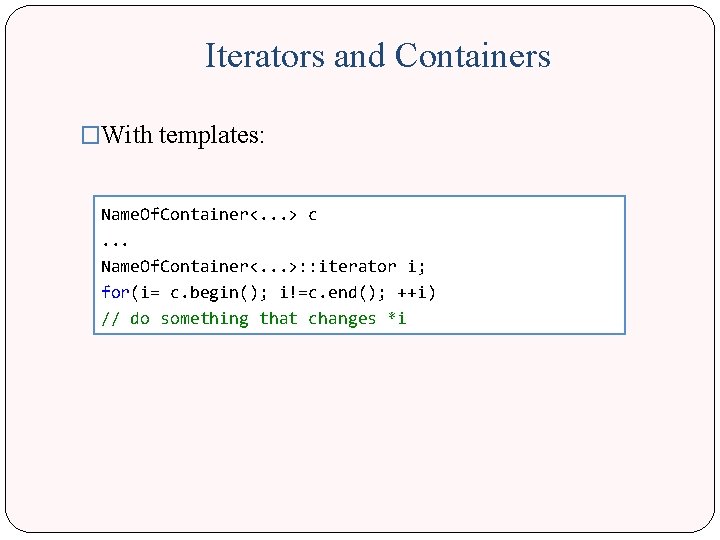
- Slides: 25
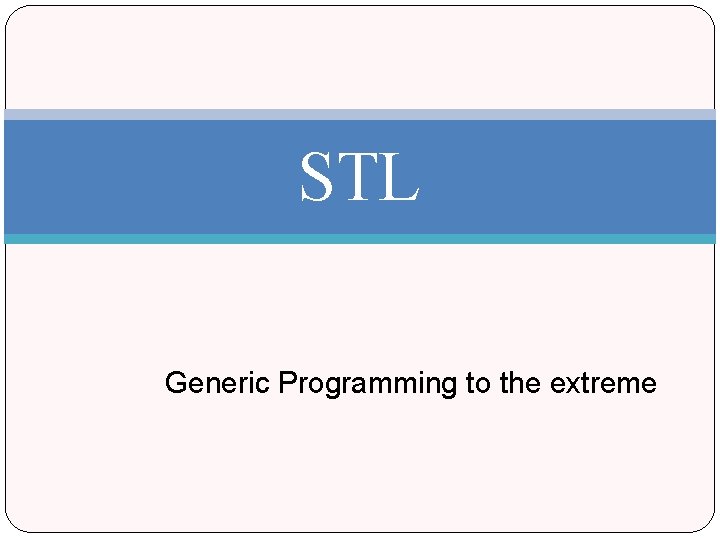
STL Generic Programming to the extreme
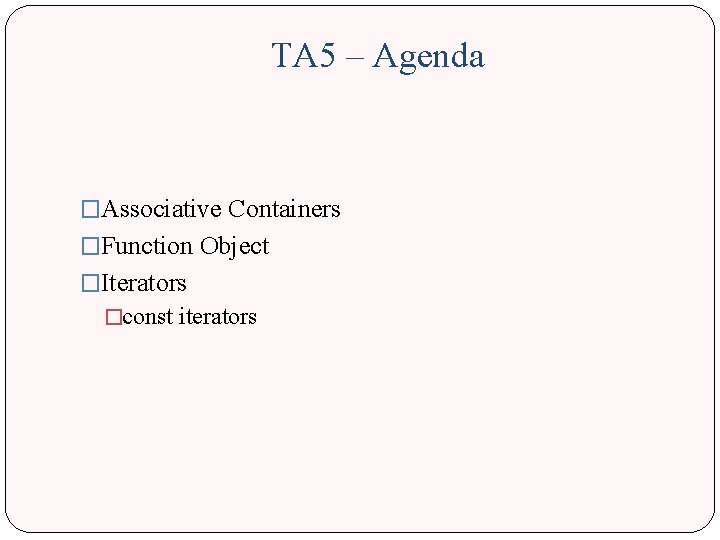
TA 5 – Agenda �Associative Containers �Function Object �Iterators �const iterators
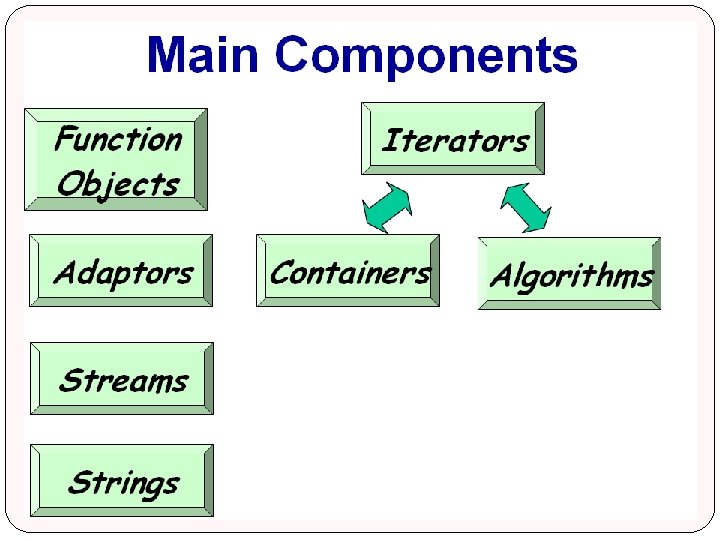
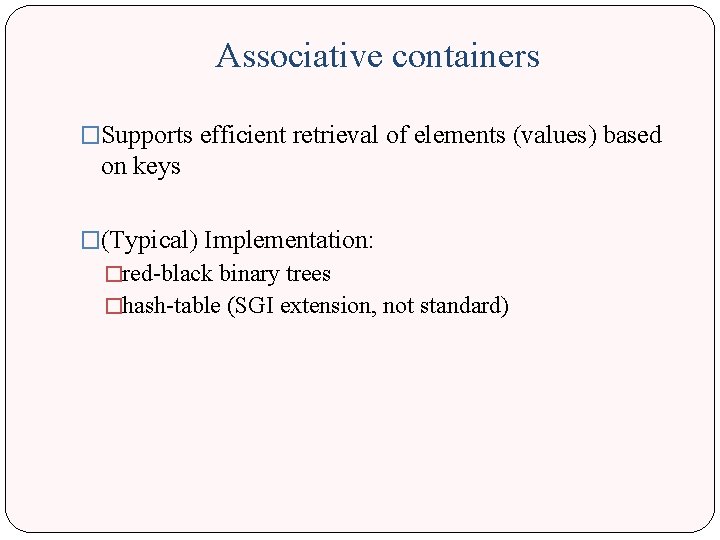
Associative containers �Supports efficient retrieval of elements (values) based on keys �(Typical) Implementation: �red-black binary trees �hash-table (SGI extension, not standard)
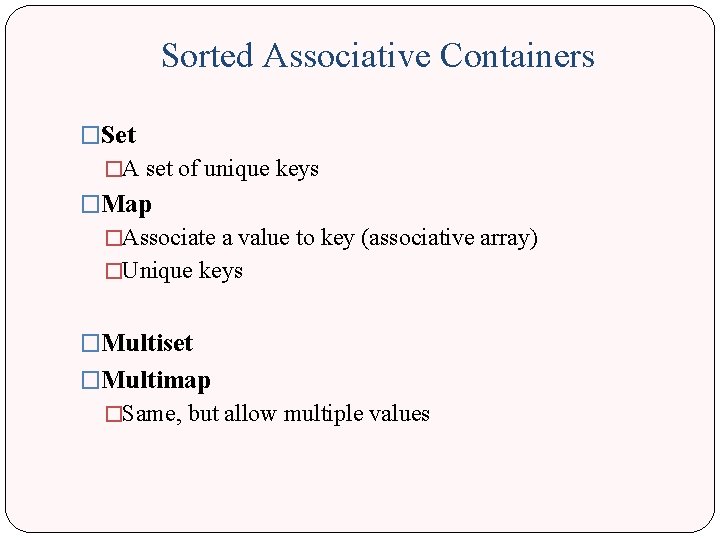
Sorted Associative Containers �Set �A set of unique keys �Map �Associate a value to key (associative array) �Unique keys �Multiset �Multimap �Same, but allow multiple values
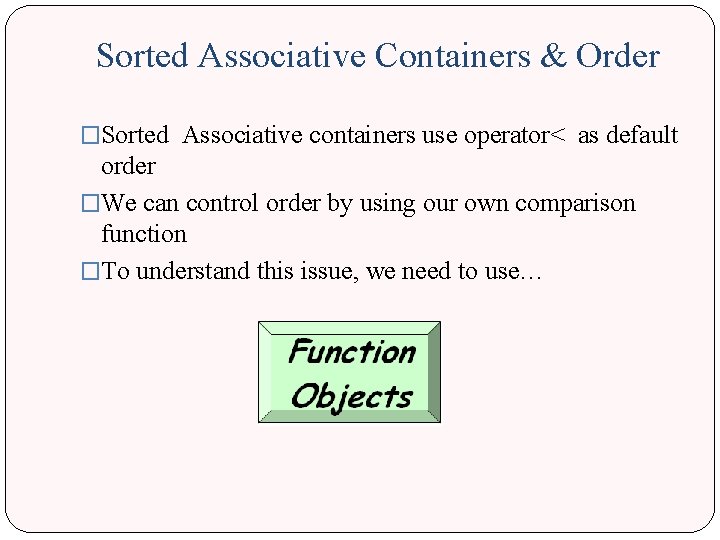
Sorted Associative Containers & Order �Sorted Associative containers use operator< as default order �We can control order by using our own comparison function �To understand this issue, we need to use…
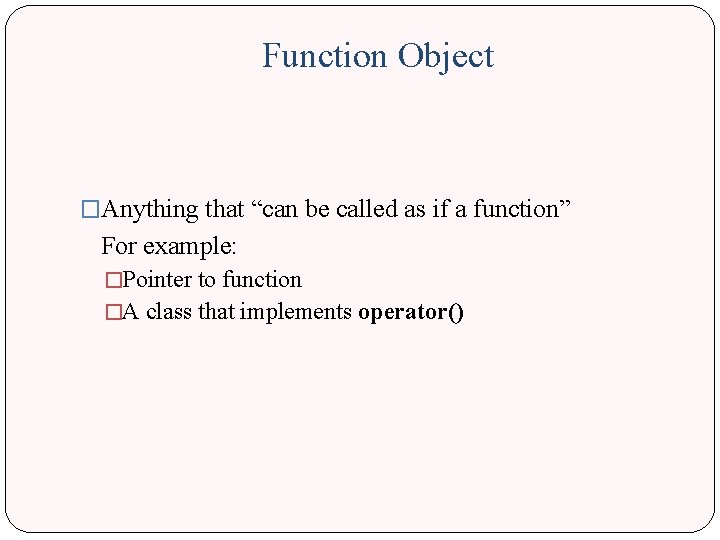
Function Object �Anything that “can be called as if a function” For example: �Pointer to function �A class that implements operator()
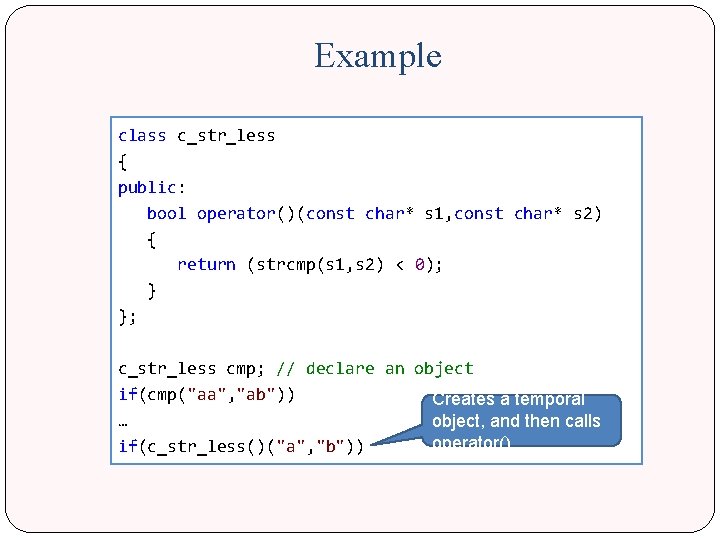
Example class c_str_less { public: bool operator()(const char* s 1, const char* s 2) { return (strcmp(s 1, s 2) < 0); } }; c_str_less cmp; // declare an object if(cmp("aa", "ab")) Creates a temporal … object, and then calls operator() if(c_str_less()("a", "b"))
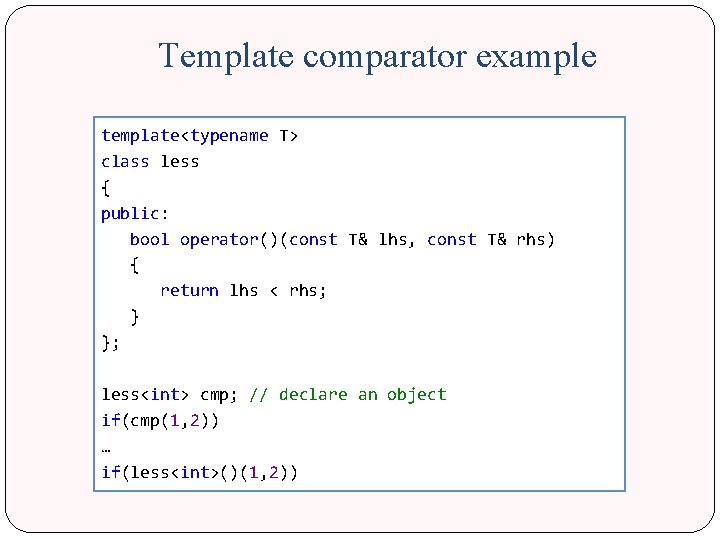
Template comparator example template<typename T> class less { public: bool operator()(const T& lhs, const T& rhs) { return lhs < rhs; } }; less<int> cmp; // declare an object if(cmp(1, 2)) … if(less<int>()(1, 2))
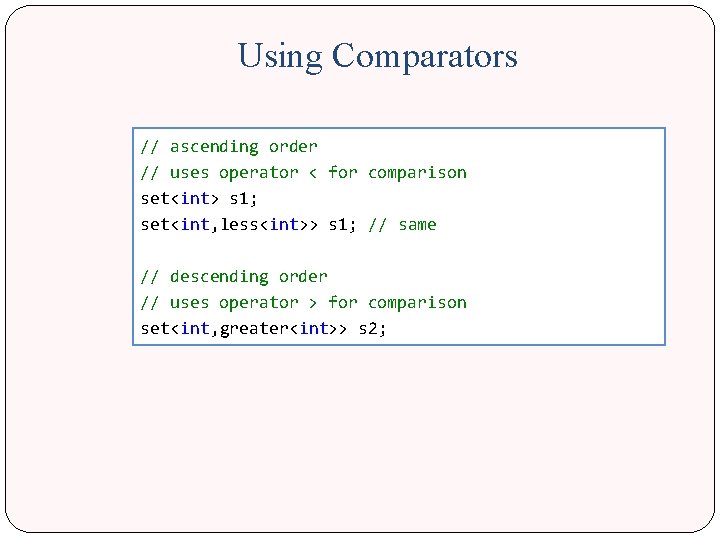
Using Comparators // ascending order // uses operator < for comparison set<int> s 1; set<int, less<int>> s 1; // same // descending order // uses operator > for comparison set<int, greater<int>> s 2;
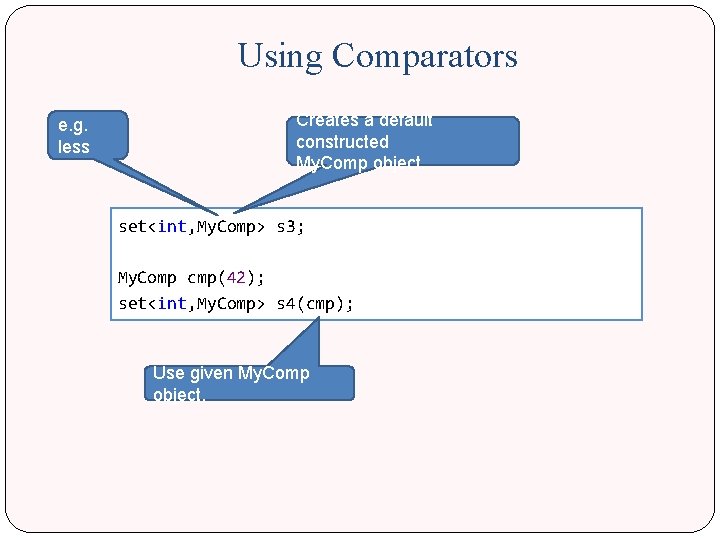
Using Comparators e. g. less Creates a default constructed My. Comp object. set<int, My. Comp> s 3; My. Comp cmp(42); set<int, My. Comp> s 4(cmp); Use given My. Comp object.
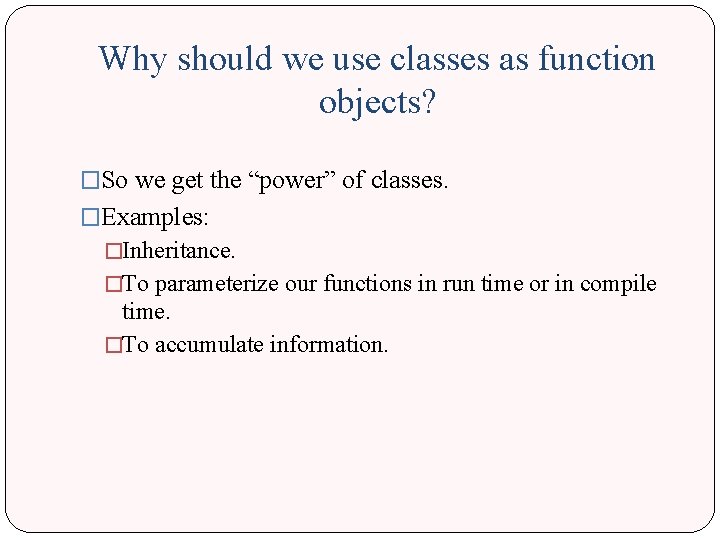
Why should we use classes as function objects? �So we get the “power” of classes. �Examples: �Inheritance. �To parameterize our functions in run time or in compile time. �To accumulate information.
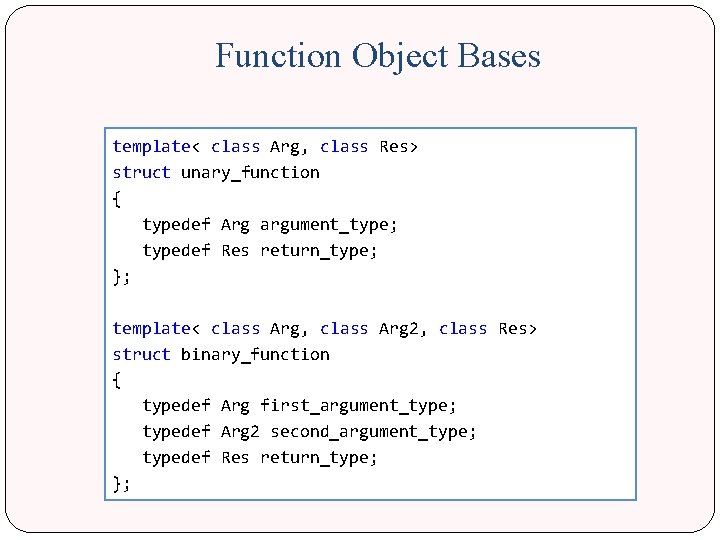
Function Object Bases template< class Arg, class Res> struct unary_function { typedef Arg argument_type; typedef Res return_type; }; template< class Arg, class Arg 2, class Res> struct binary_function { typedef Arg first_argument_type; typedef Arg 2 second_argument_type; typedef Res return_type; };
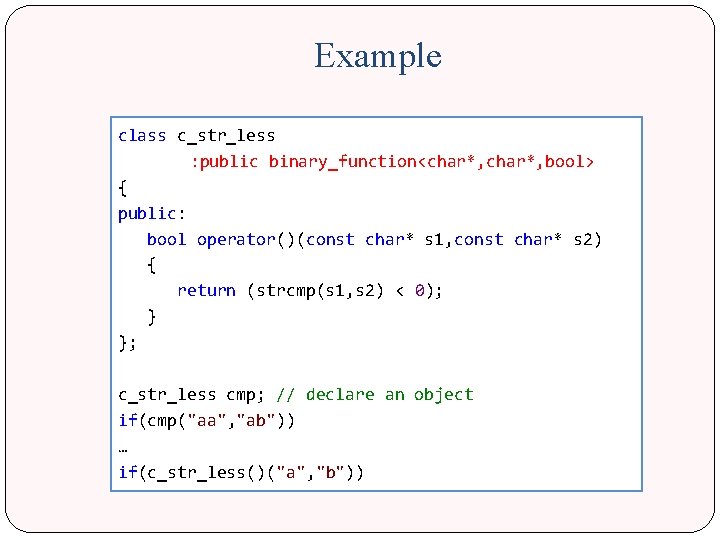
Example class c_str_less : public binary_function<char*, bool> { public: bool operator()(const char* s 1, const char* s 2) { return (strcmp(s 1, s 2) < 0); } }; c_str_less cmp; // declare an object if(cmp("aa", "ab")) … if(c_str_less()("a", "b"))
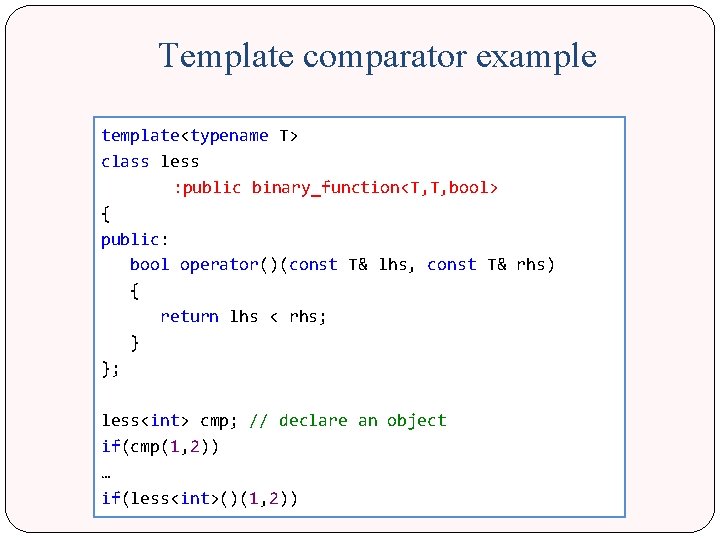
Template comparator example template<typename T> class less : public binary_function<T, T, bool> { public: bool operator()(const T& lhs, const T& rhs) { return lhs < rhs; } }; less<int> cmp; // declare an object if(cmp(1, 2)) … if(less<int>()(1, 2))
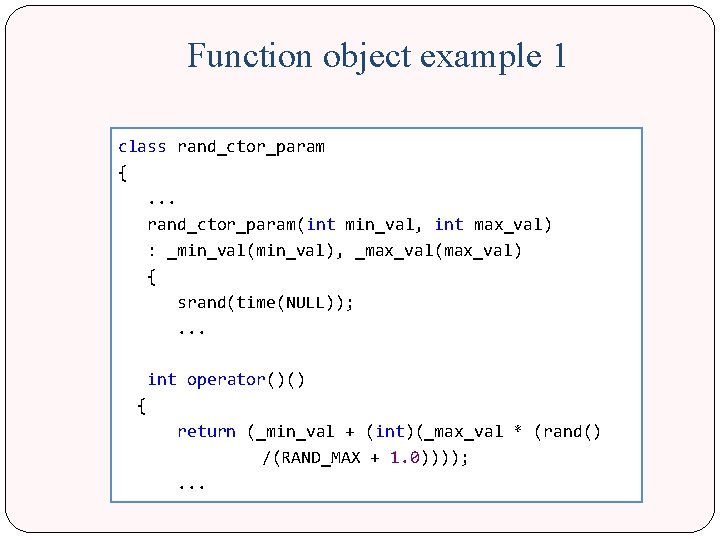
Function object example 1 class rand_ctor_param { . . . rand_ctor_param(int min_val, int max_val) : _min_val(min_val), _max_val(max_val) { srand(time(NULL)); . . . int operator()() { return (_min_val + (int)(_max_val * (rand() /(RAND_MAX + 1. 0)))); . . .
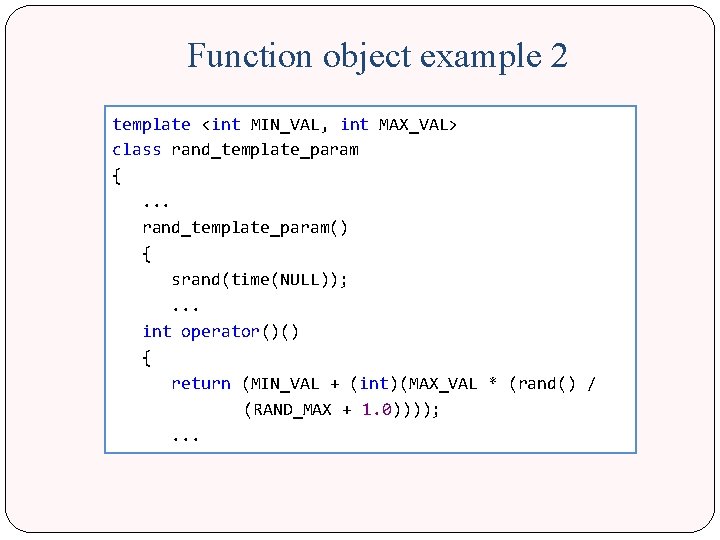
Function object example 2 template <int MIN_VAL, int MAX_VAL> class rand_template_param { . . . rand_template_param() { srand(time(NULL)); . . . int operator()() { return (MIN_VAL + (int)(MAX_VAL * (rand() / (RAND_MAX + 1. 0)))); . . .
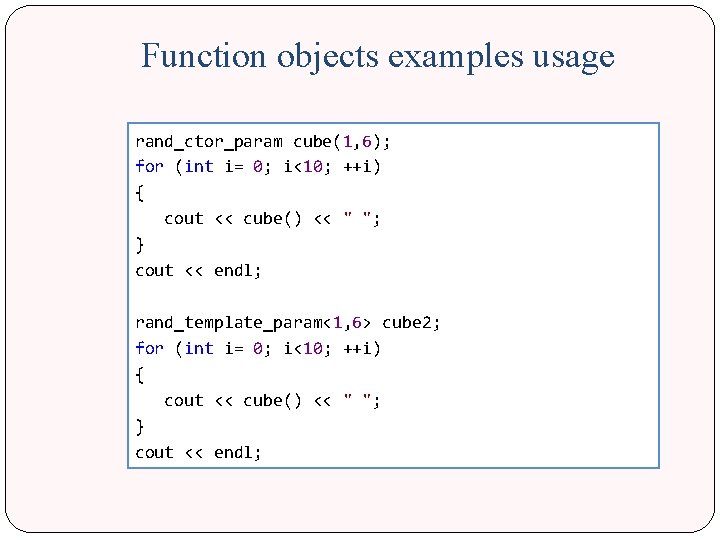
Function objects examples usage rand_ctor_param cube(1, 6); for (int i= 0; i<10; ++i) { cout << cube() << " "; } cout << endl; rand_template_param<1, 6> cube 2; for (int i= 0; i<10; ++i) { cout << cube() << " "; } cout << endl;
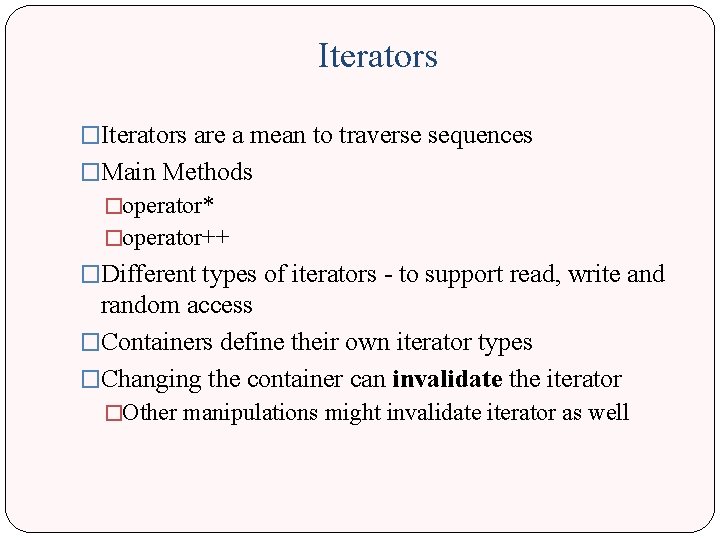
Iterators �Iterators are a mean to traverse sequences �Main Methods �operator* �operator++ �Different types of iterators - to support read, write and random access �Containers define their own iterator types �Changing the container can invalidate the iterator �Other manipulations might invalidate iterator as well
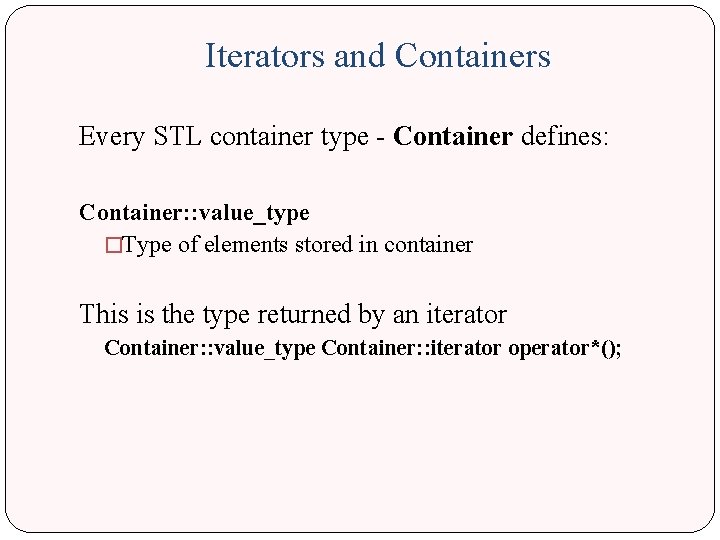
Iterators and Containers Every STL container type - Container defines: Container: : value_type �Type of elements stored in container This is the type returned by an iterator Container: : value_type Container: : iterator operator*();
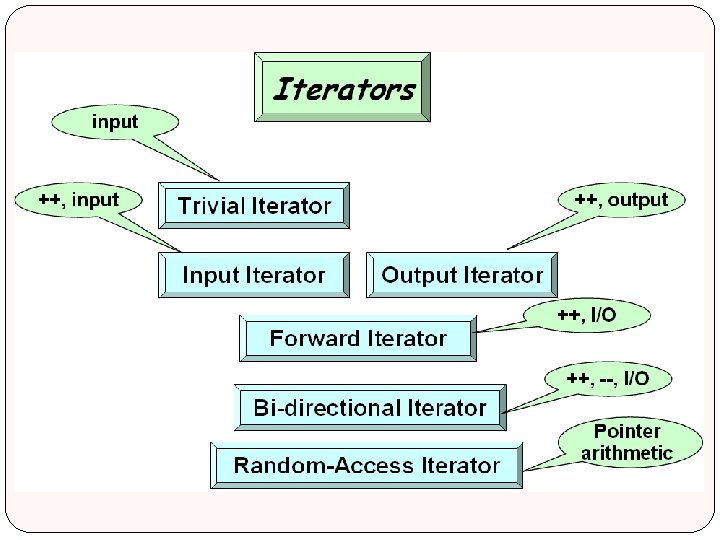
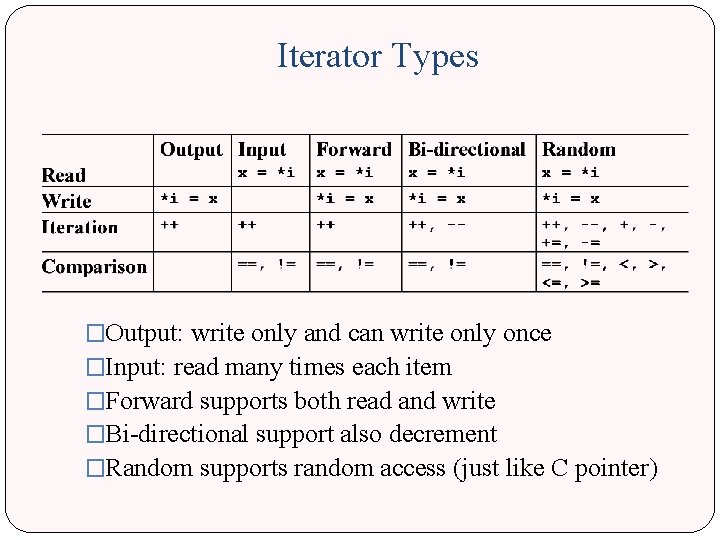
Iterator Types �Output: write only and can write only once �Input: read many times each item �Forward supports both read and write �Bi-directional support also decrement �Random supports random access (just like C pointer)
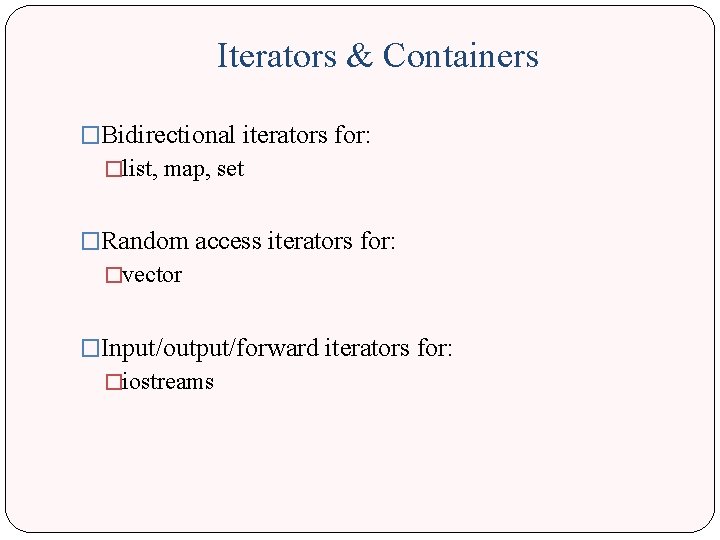
Iterators & Containers �Bidirectional iterators for: �list, map, set �Random access iterators for: �vector �Input/output/forward iterators for: �iostreams
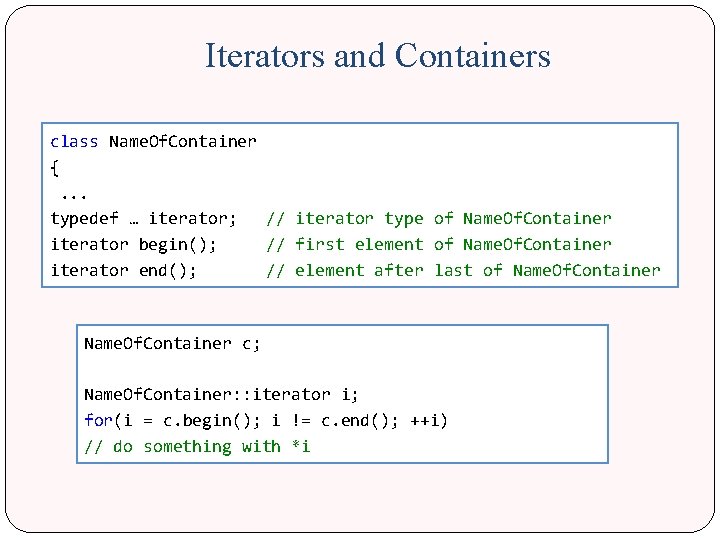
Iterators and Containers class Name. Of. Container { . . . typedef … iterator; // iterator type of Name. Of. Container iterator begin(); // first element of Name. Of. Container iterator end(); // element after last of Name. Of. Container c; Name. Of. Container: : iterator i; for(i = c. begin(); i != c. end(); ++i) // do something with *i
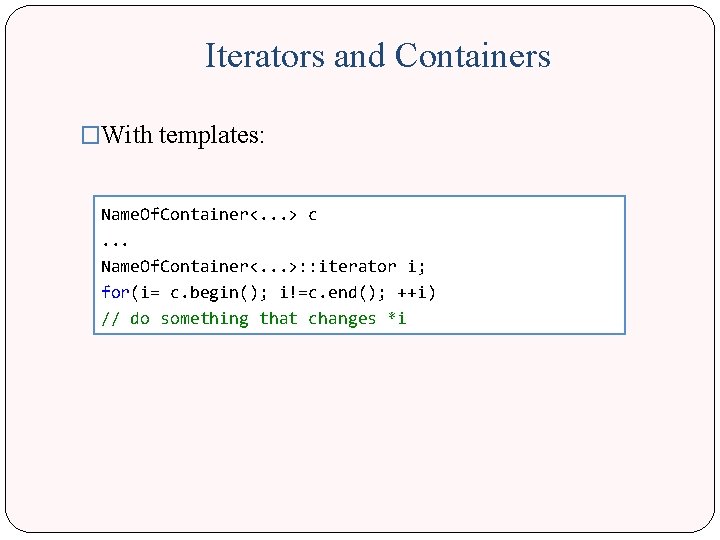
Iterators and Containers �With templates: Name. Of. Container<. . . > c . . . Name. Of. Container<. . . >: : iterator i; for(i= c. begin(); i!=c. end(); ++i) // do something that changes *i
Generic programming and the stl
Extreme wide angle camera shot
Diane pozefsky
Extreme programming
Extreme programming agile
User requirements are expressed as in extreme programming
Extreme programming workflow
Extreme programming advantages and disadvantages
Alistair dilbert
Programming
Extreme point theorem
History of extreme programming
Ventajas y desventajas de la metodologia xp
Refactoring extreme programming
Extreme programming life cycle
Daniel baranowski
Extreme programming in software engineering
Extreme programming
Templates in c++
Generic subroutine
Generic programming
Terminale stl spcl systèmes et procédés
Stl biblioteka
Stl biblioteka
Stl link st leonards
Stl stands for