Generic Programming Programming with Concepts What is generic
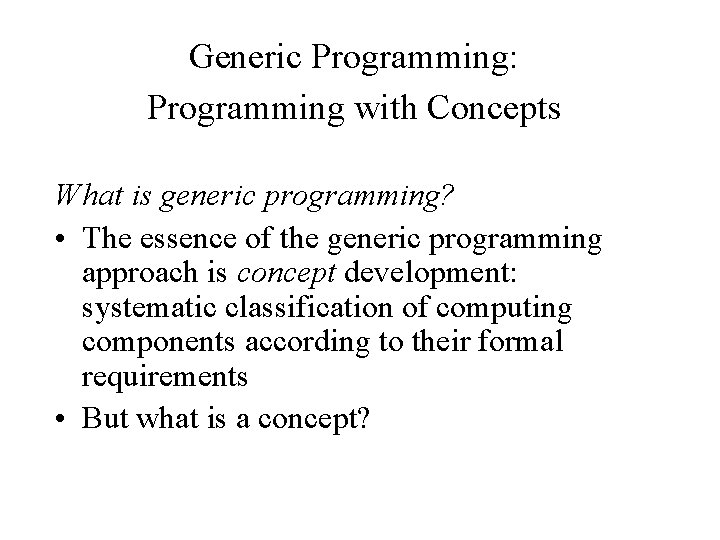
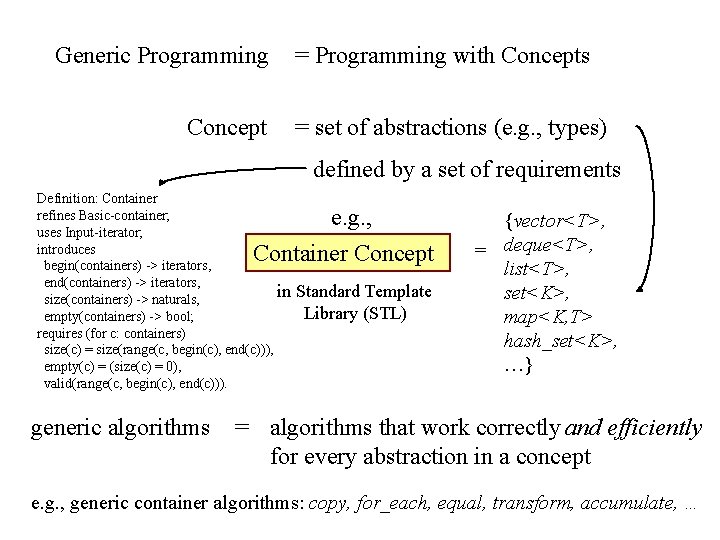
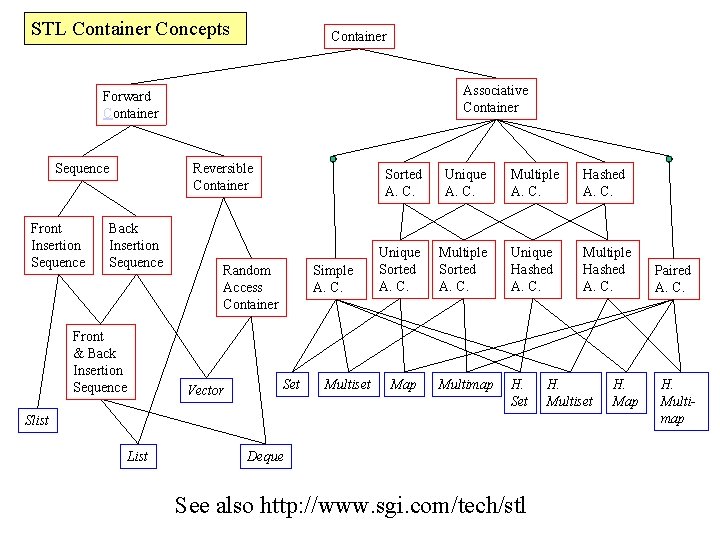
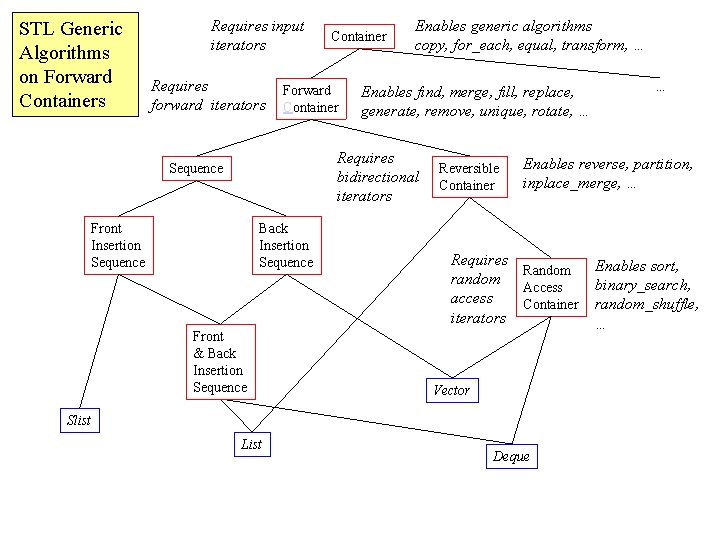
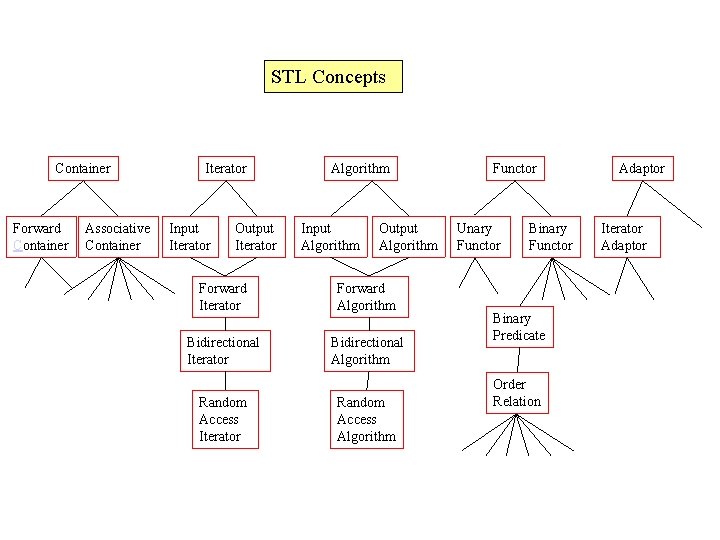
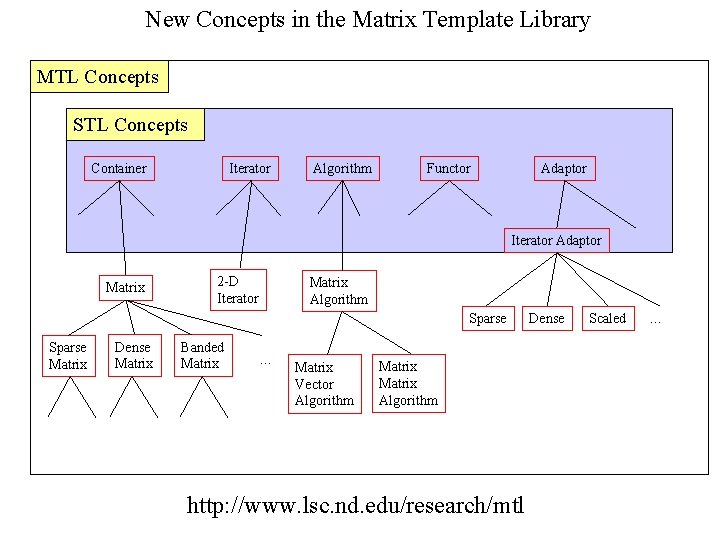
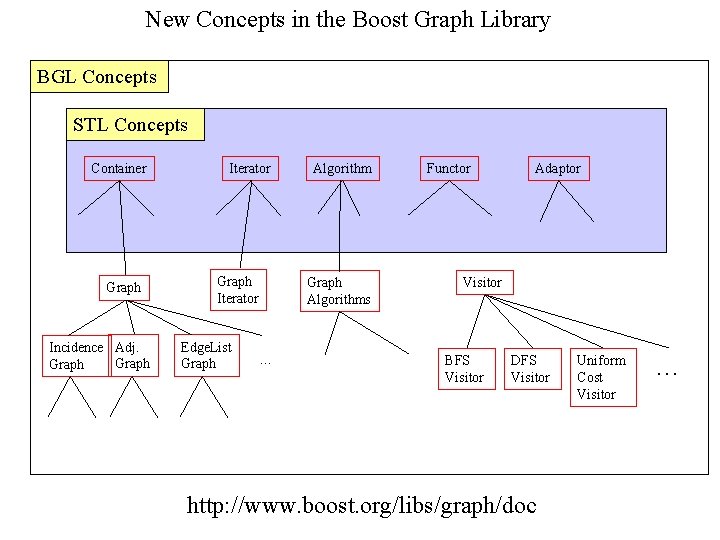
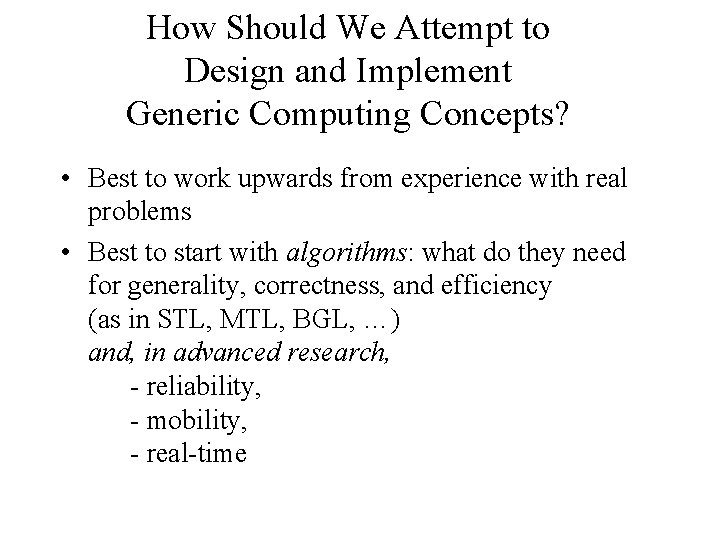
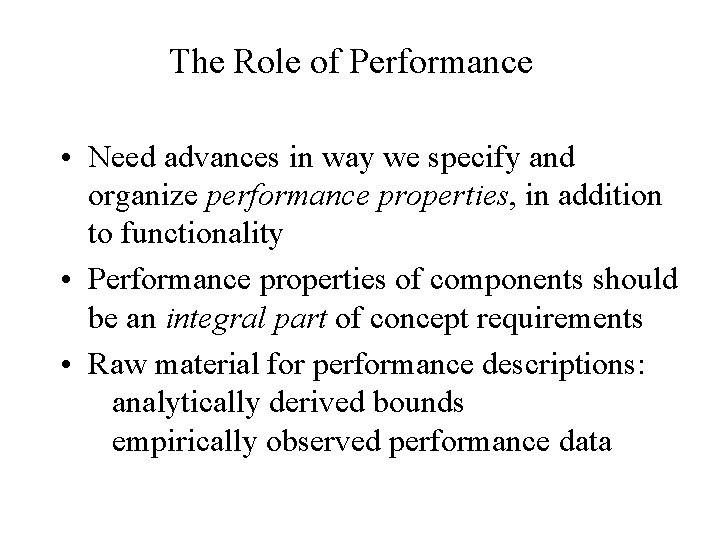
- Slides: 9
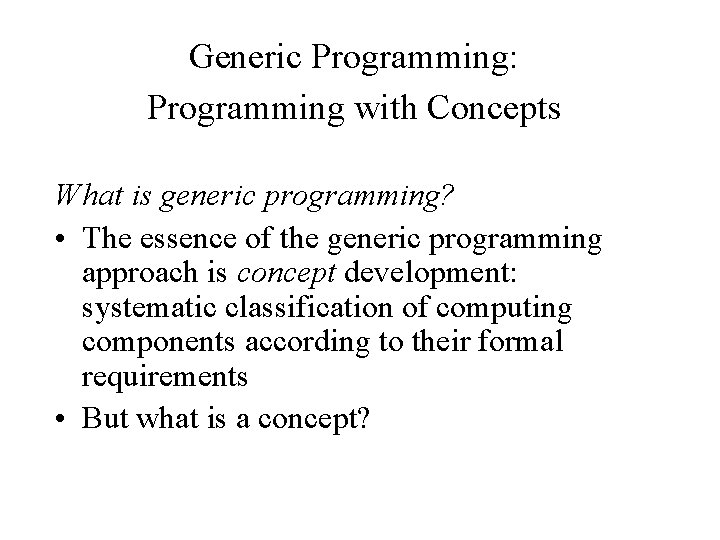
Generic Programming: Programming with Concepts What is generic programming? • The essence of the generic programming approach is concept development: systematic classification of computing components according to their formal requirements • But what is a concept?
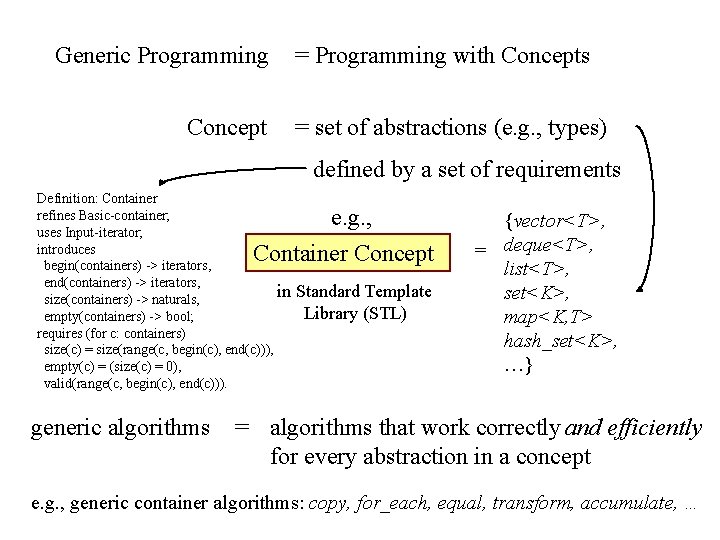
Generic Programming Concept = Programming with Concepts = set of abstractions (e. g. , types) defined by a set of requirements Definition: Container refines Basic-container; uses Input-iterator; introduces begin(containers) -> iterators, end(containers) -> iterators, in size(containers) -> naturals, empty(containers) -> bool; requires (for c: containers) size(c) = size(range(c, begin(c), end(c))), empty(c) = (size(c) = 0), valid(range(c, begin(c), end(c))). e. g. , Container Concept generic algorithms Standard Template Library (STL) {vector<T>, = deque<T>, list<T>, set<K>, map<K, T> hash_set<K>, …} = algorithms that work correctly and efficiently for every abstraction in a concept e. g. , generic container algorithms: copy, for_each, equal, transform, accumulate, …
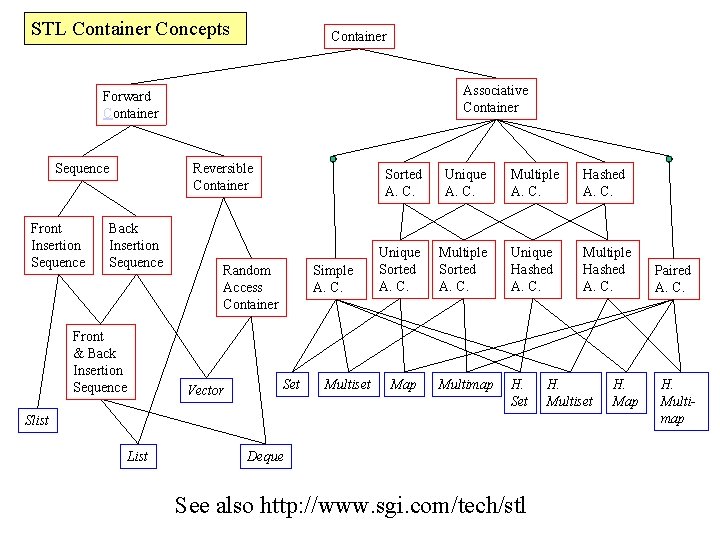
STL Container Concepts Container Associative Container Forward Container Sequence Front Insertion Sequence Reversible Container Back Insertion Sequence Front & Back Insertion Sequence Random Access Container Vector Simple A. C. Set Multiset Sorted A. C. Unique A. C. Multiple A. C. Hashed A. C. Unique Sorted A. C. Multiple Sorted A. C. Unique Hashed A. C. Multiple Hashed A. C. Multimap H. Set Map Slist List Deque See also http: //www. sgi. com/tech/stl H. Multiset H. Map Paired A. C. H. Multimap
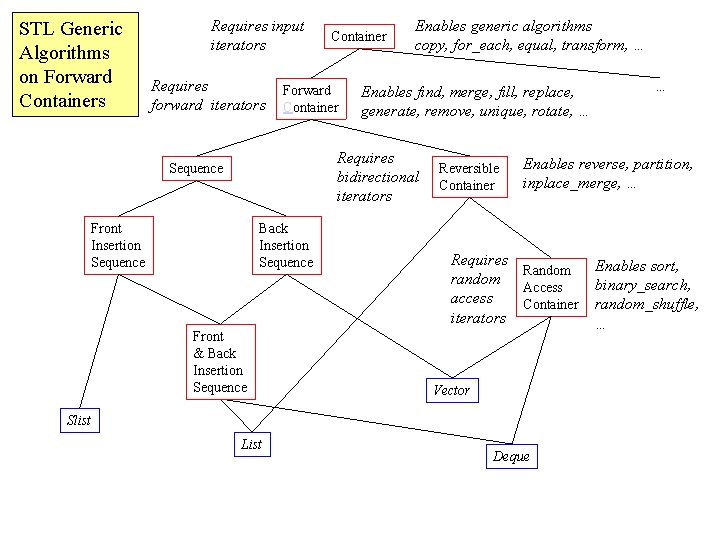
STL Generic Algorithms on Forward Containers Requires input iterators Requires forward iterators Container Forward Container Enables generic algorithms copy, for_each, equal, transform, … Enables find, merge, fill, replace, generate, remove, unique, rotate, … Requires bidirectional iterators Sequence Front Insertion Sequence Back Insertion Sequence Front & Back Insertion Sequence Reversible Container Enables reverse, partition, inplace_merge, … Requires Random random Access access Container iterators Vector Slist List … Deque Enables sort, binary_search, random_shuffle, …
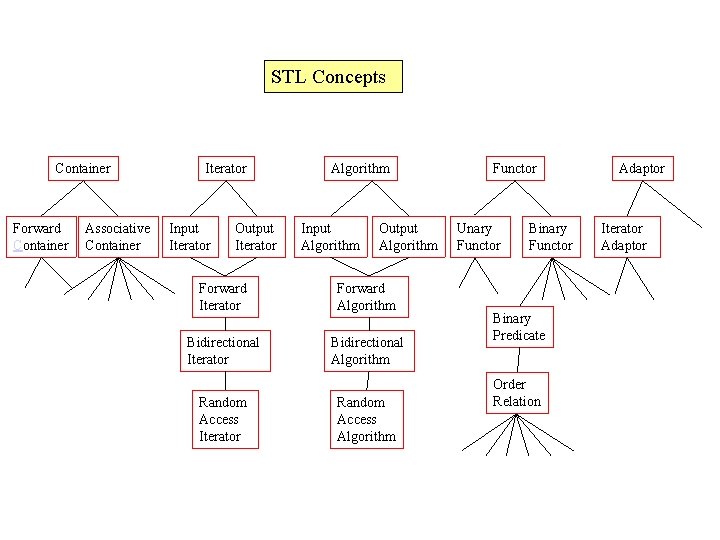
STL Concepts Container Forward Container Associative Container Iterator Input Iterator Output Iterator Algorithm Input Algorithm Output Algorithm Forward Iterator Forward Algorithm Bidirectional Iterator Bidirectional Algorithm Random Access Iterator Random Access Algorithm Functor Unary Functor Binary Predicate Order Relation Adaptor Iterator Adaptor
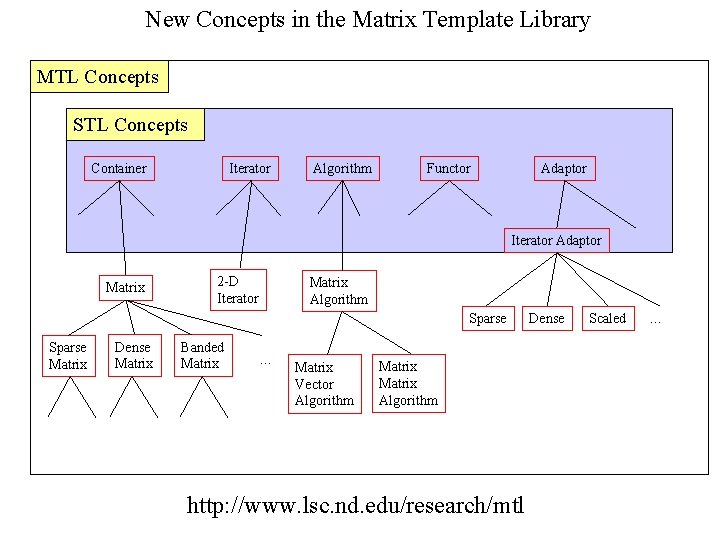
New Concepts in the Matrix Template Library MTL Concepts STL Concepts Container Iterator Algorithm Functor Adaptor Iterator Adaptor Matrix 2 -D Iterator Matrix Algorithm Sparse Matrix Dense Matrix Banded Matrix … Matrix Vector Algorithm Matrix Algorithm http: //www. lsc. nd. edu/research/mtl Dense Scaled …
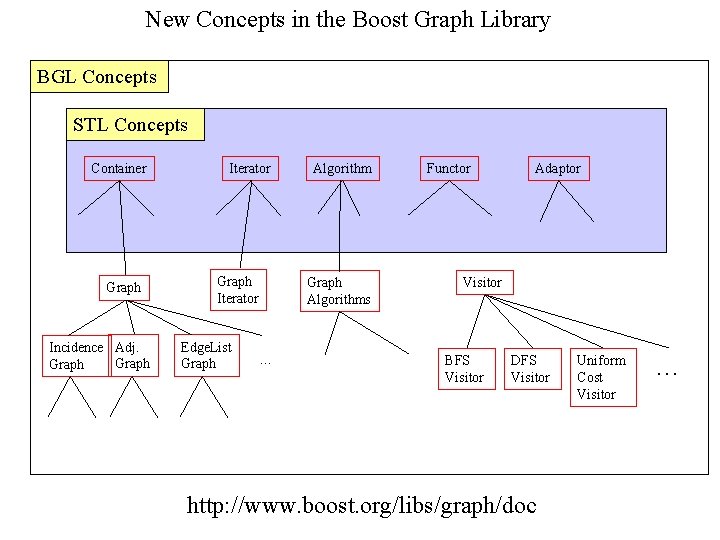
New Concepts in the Boost Graph Library BGL Concepts STL Concepts Container Graph Incidence Adj. Graph Iterator Edge. List Graph Algorithms … Functor Adaptor Visitor BFS Visitor DFS Visitor http: //www. boost. org/libs/graph/doc Uniform Cost Visitor …
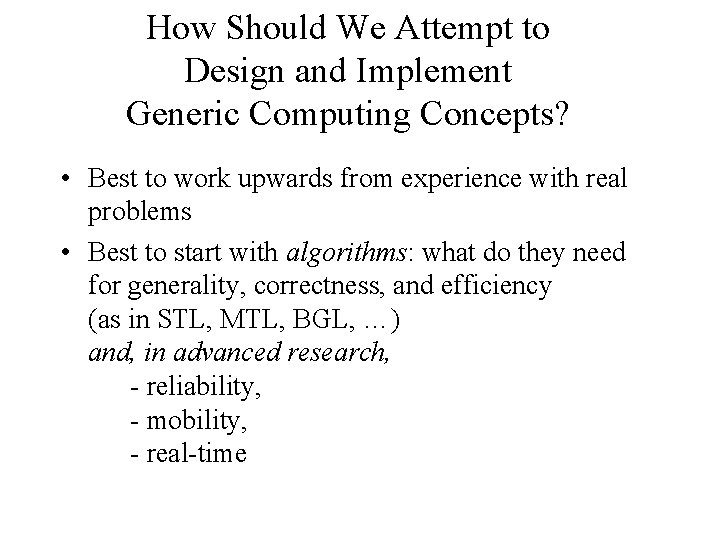
How Should We Attempt to Design and Implement Generic Computing Concepts? • Best to work upwards from experience with real problems • Best to start with algorithms: what do they need for generality, correctness, and efficiency (as in STL, MTL, BGL, …) and, in advanced research, - reliability, - mobility, - real-time
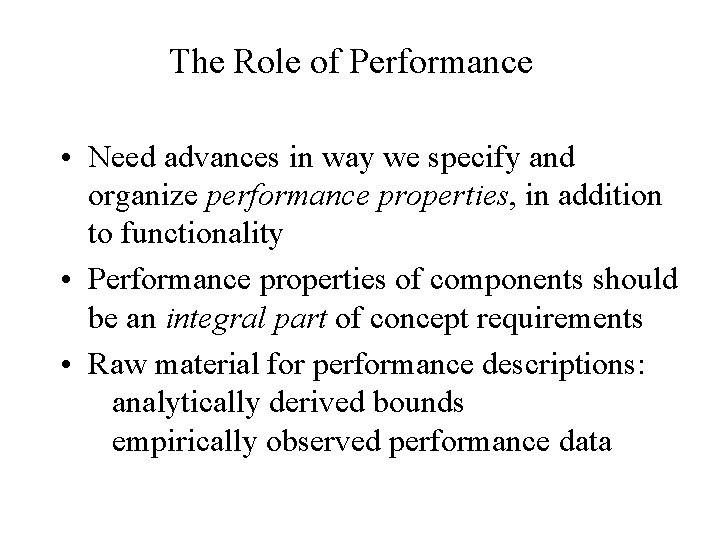
The Role of Performance • Need advances in way we specify and organize performance properties, in addition to functionality • Performance properties of components should be an integral part of concept requirements • Raw material for performance descriptions: analytically derived bounds empirically observed performance data
C generic programming
Generic programming and the stl
Generic subroutine
Generic programming
Reasons for studying concepts of programming languages
Scratch programming concepts
Concepts, techniques, and models of computer programming
Scratch programming concepts
Linear programming definition
Greedy vs dynamic