Cs 288 Intensive Programming in Linux Instructor C
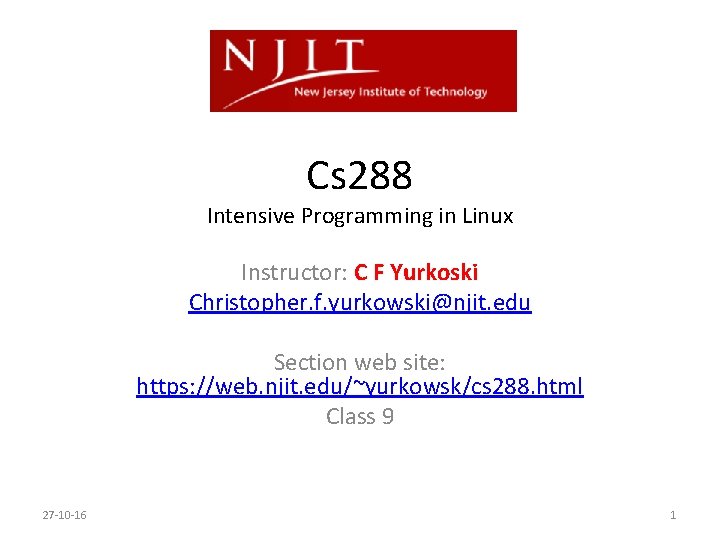
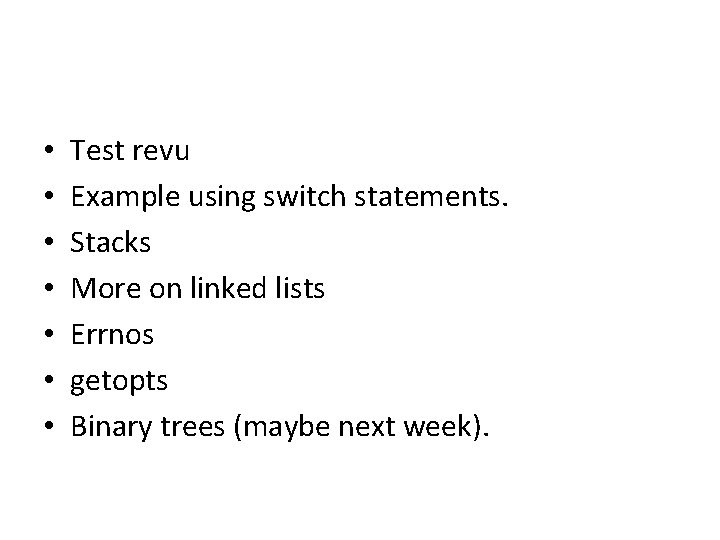
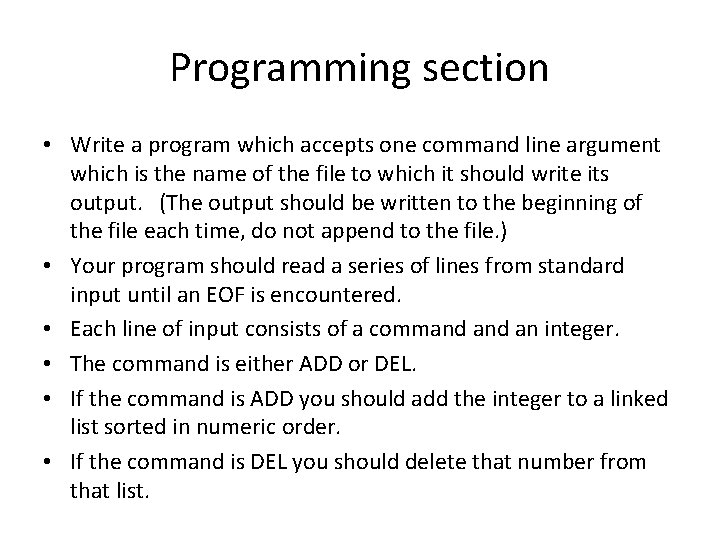
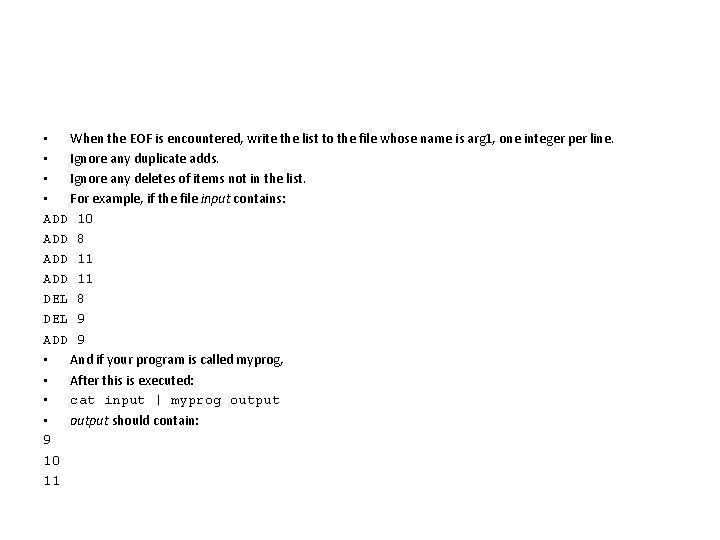
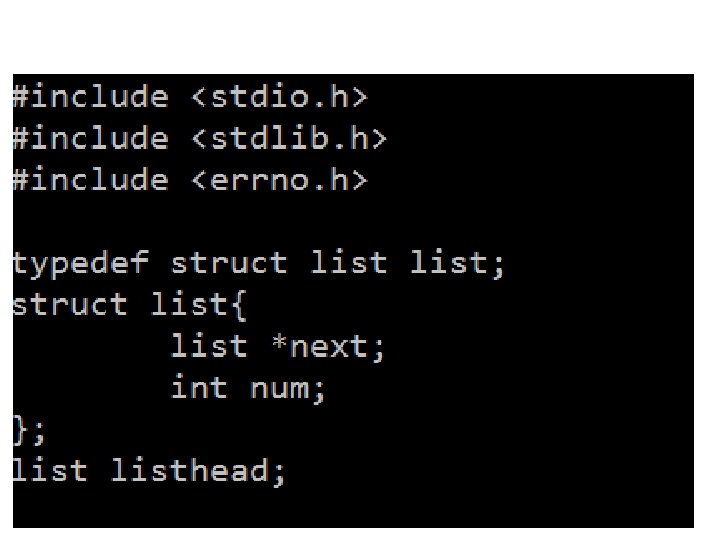
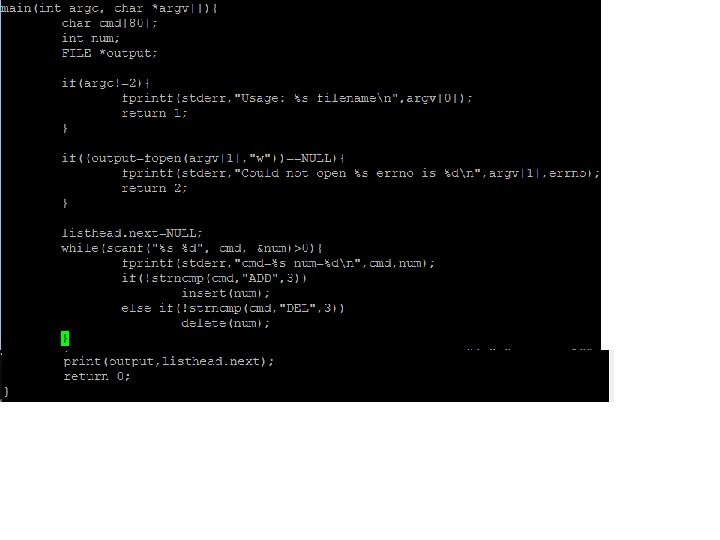
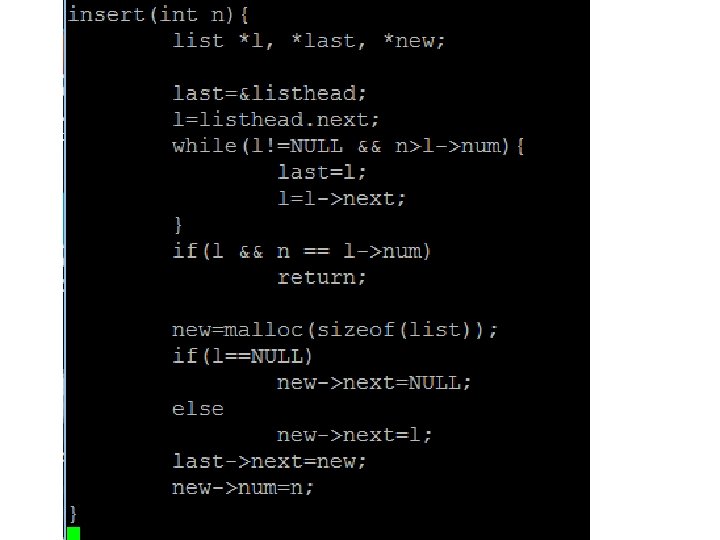
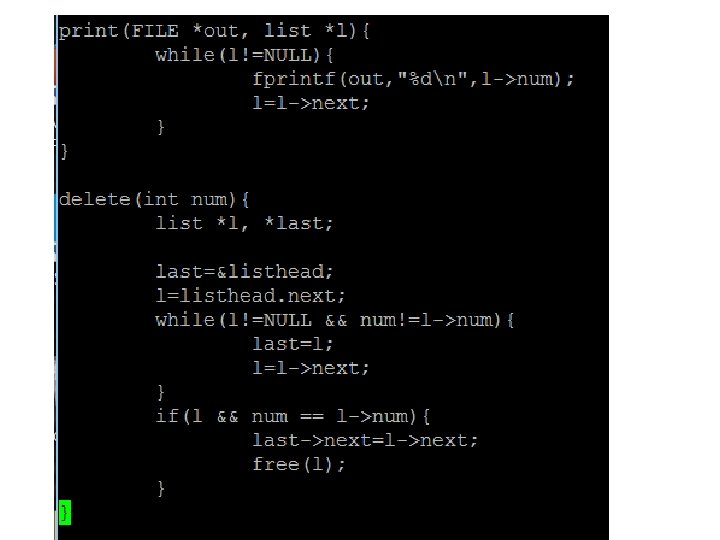
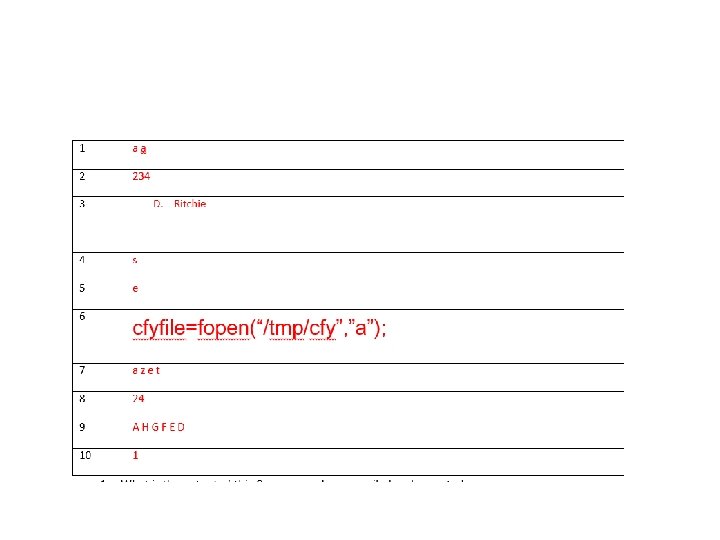
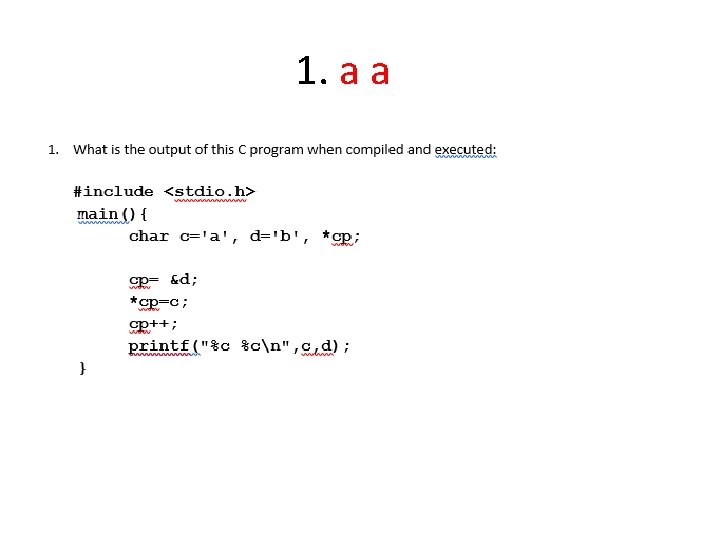
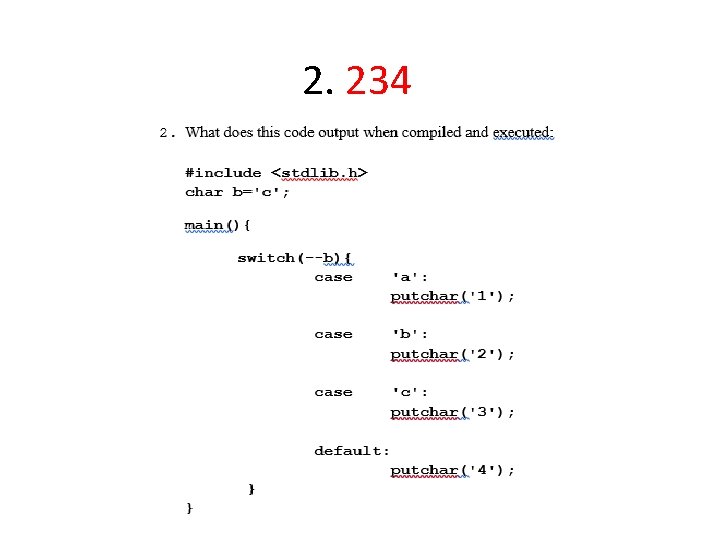
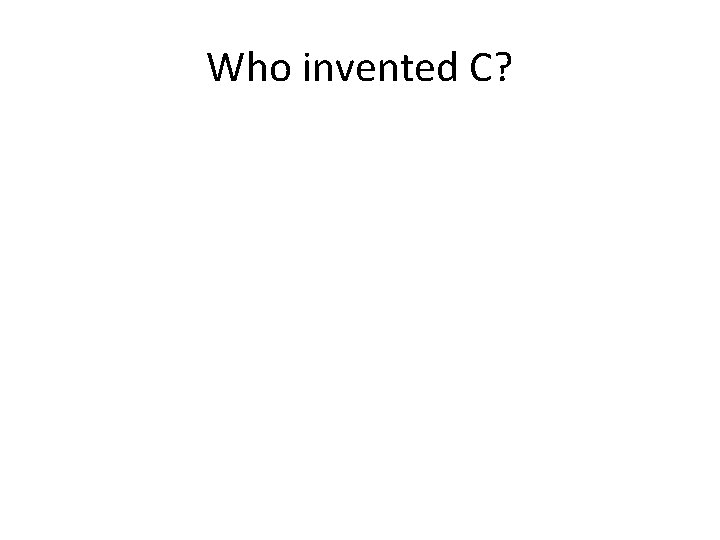
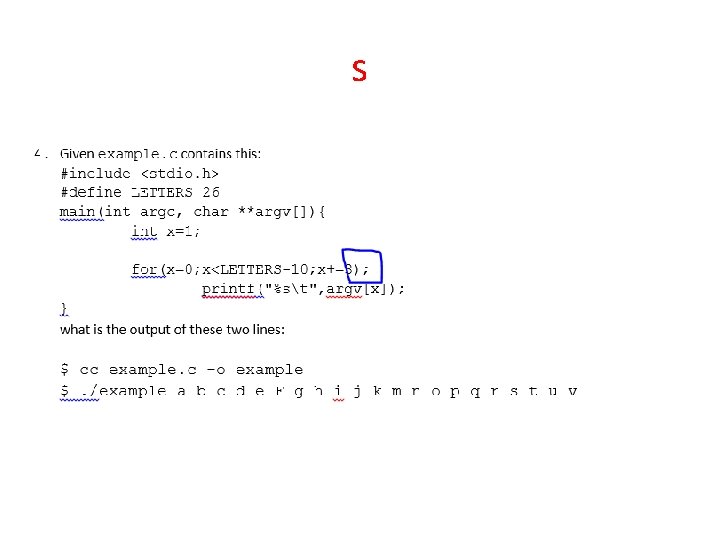
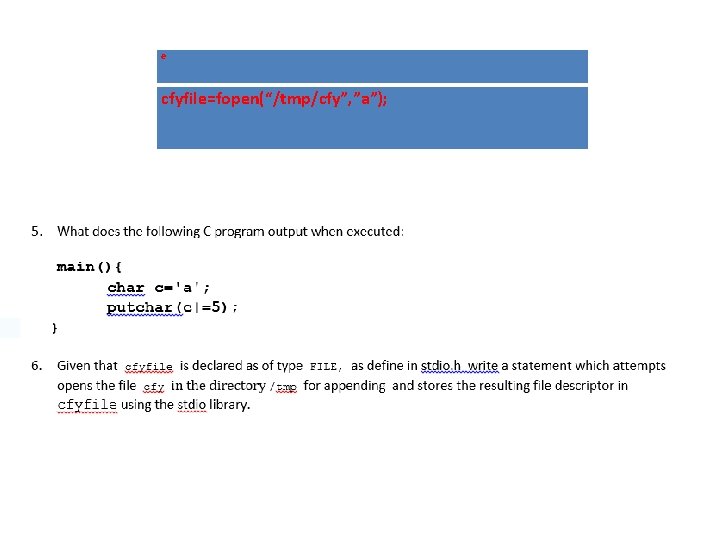
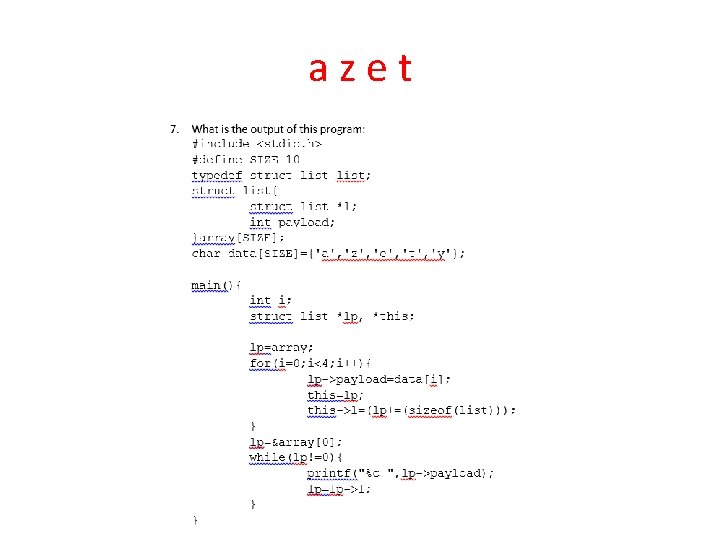
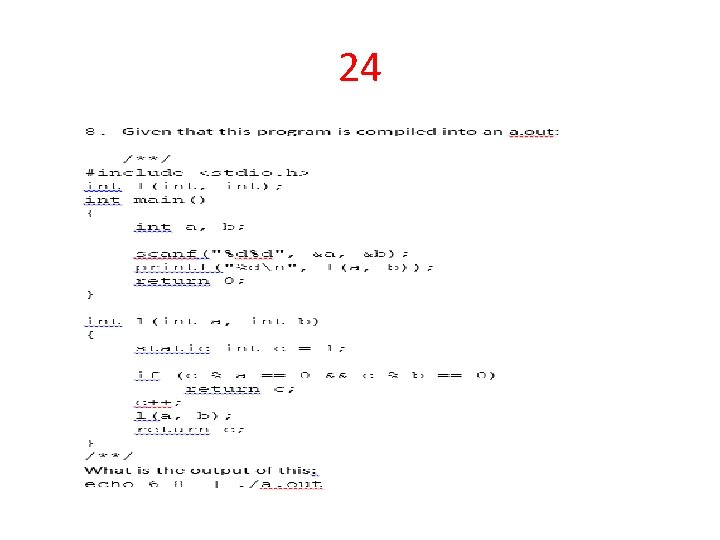
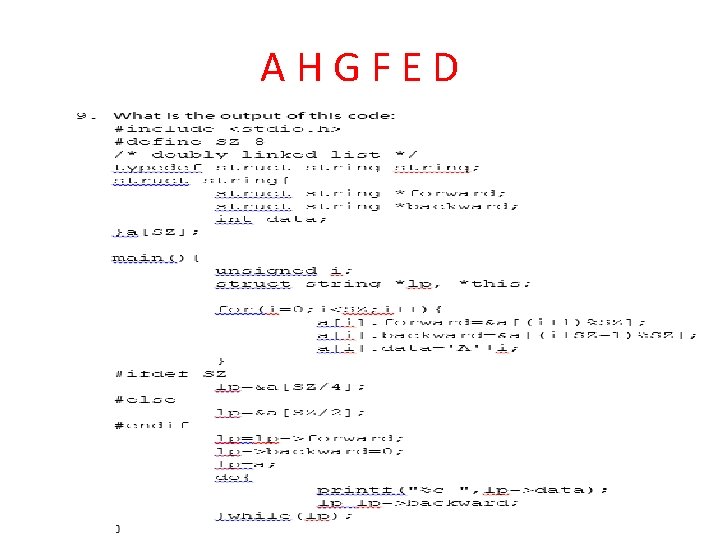
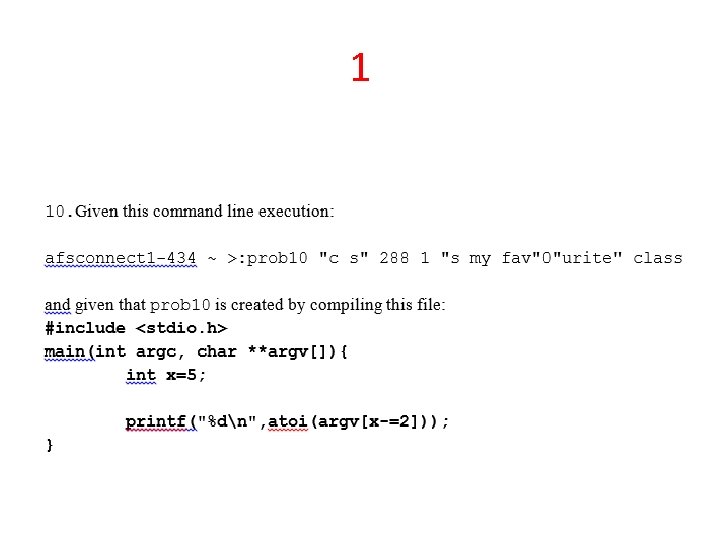
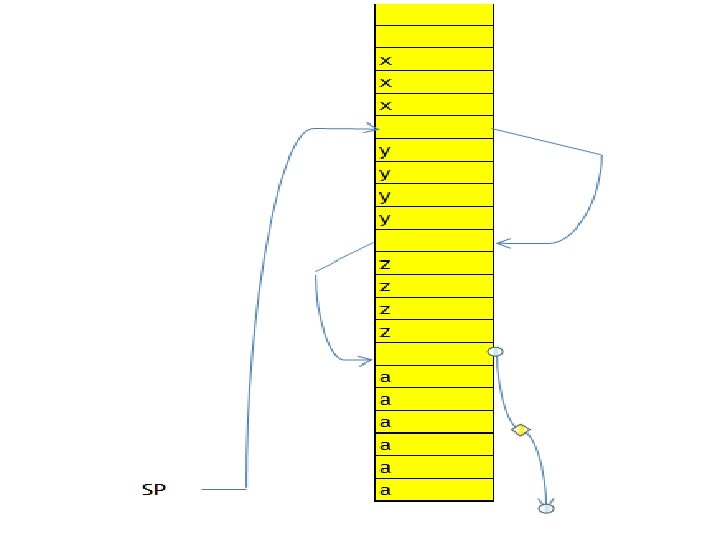
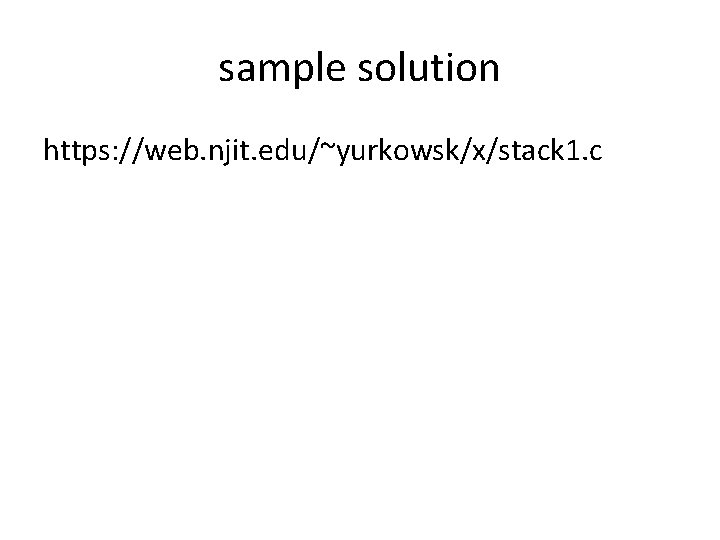
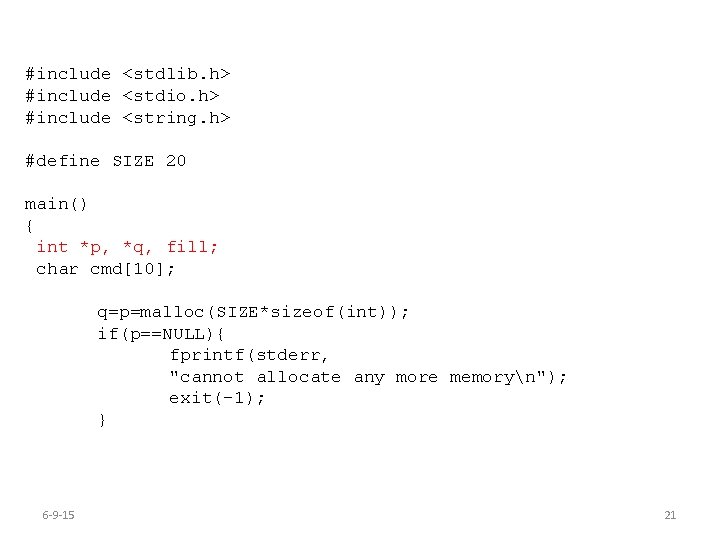
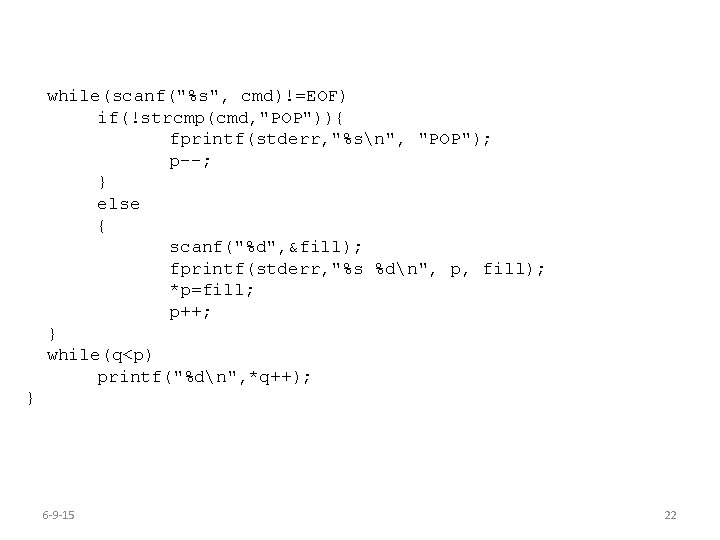
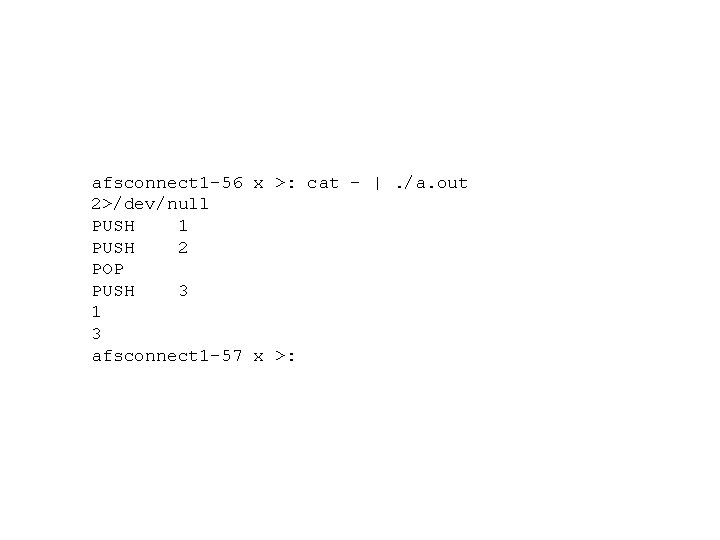
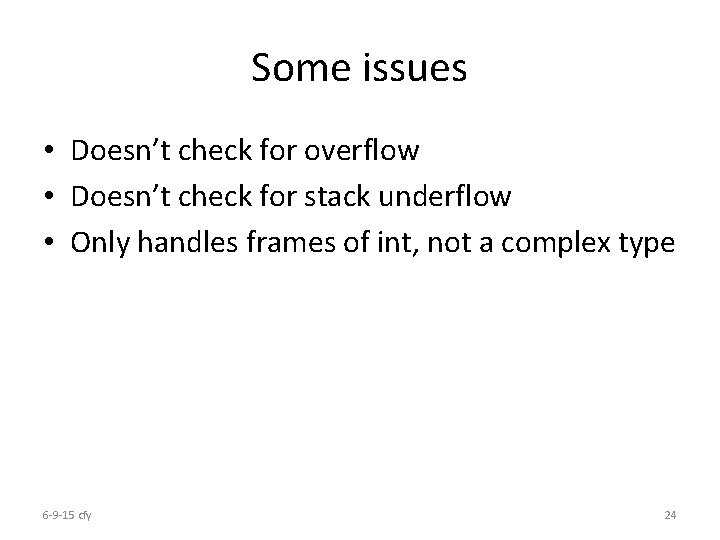
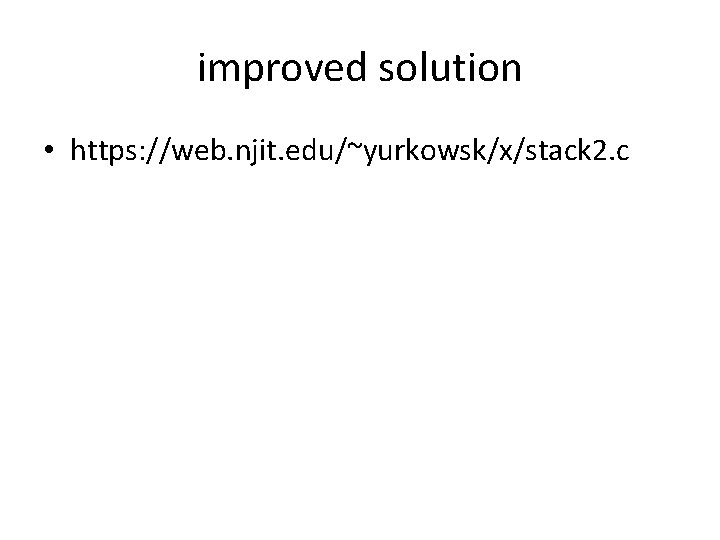
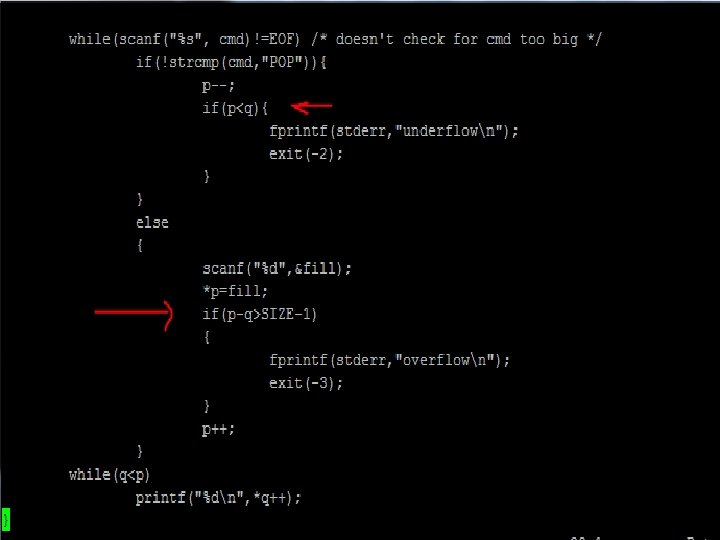
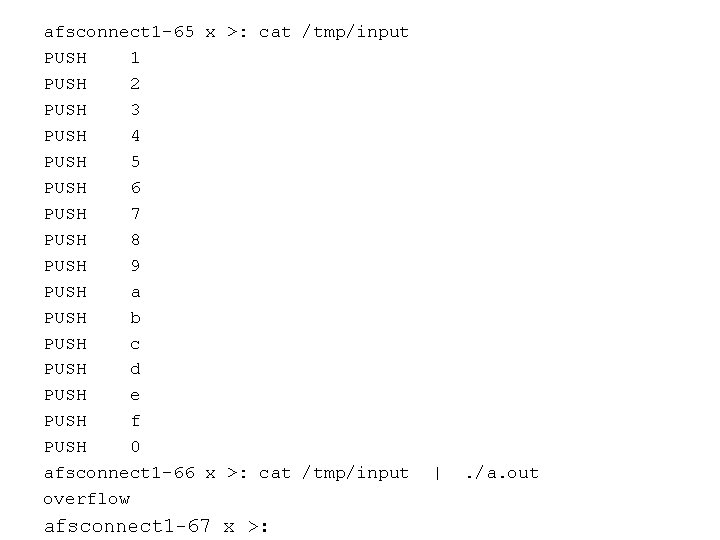
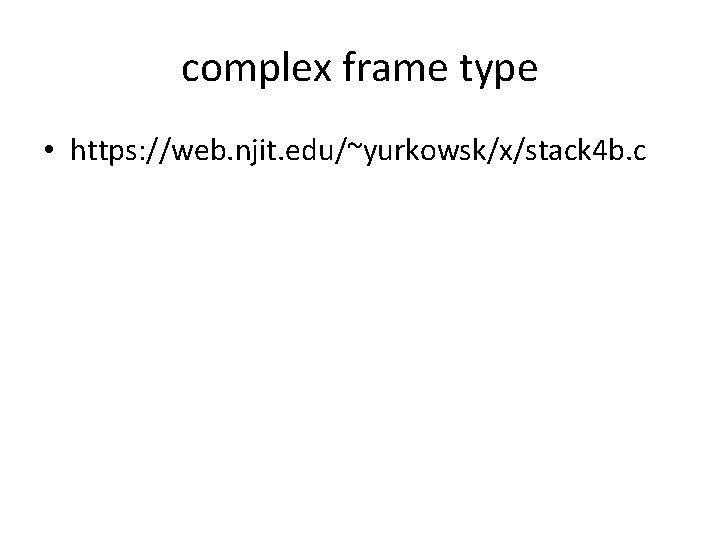
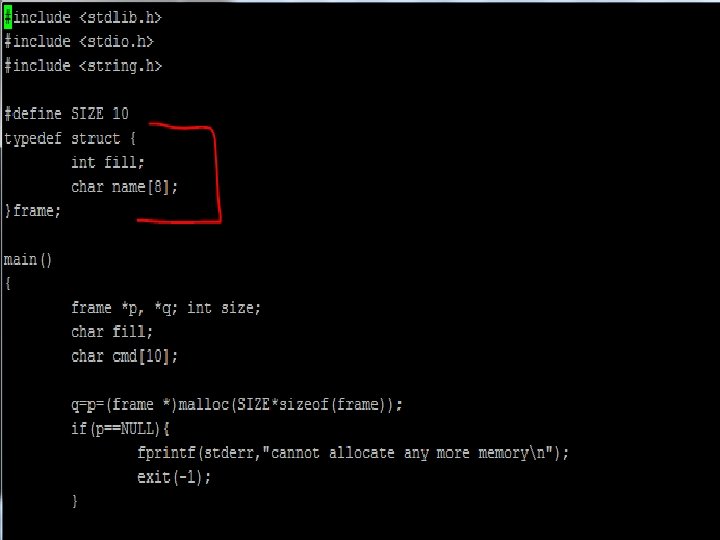
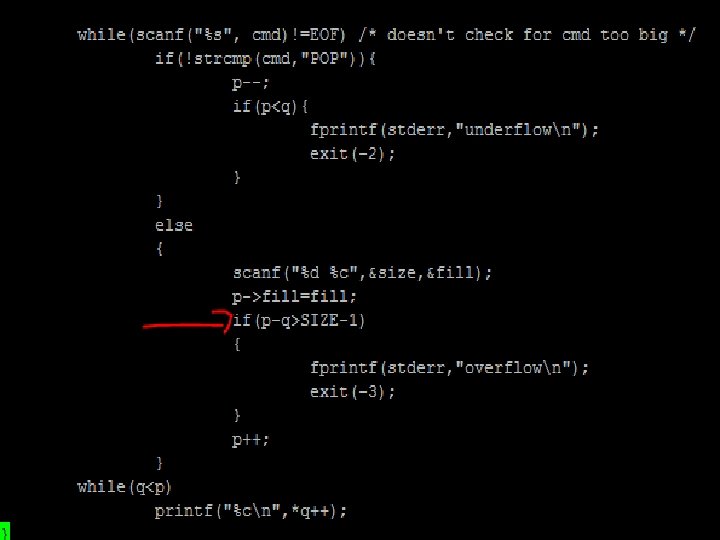
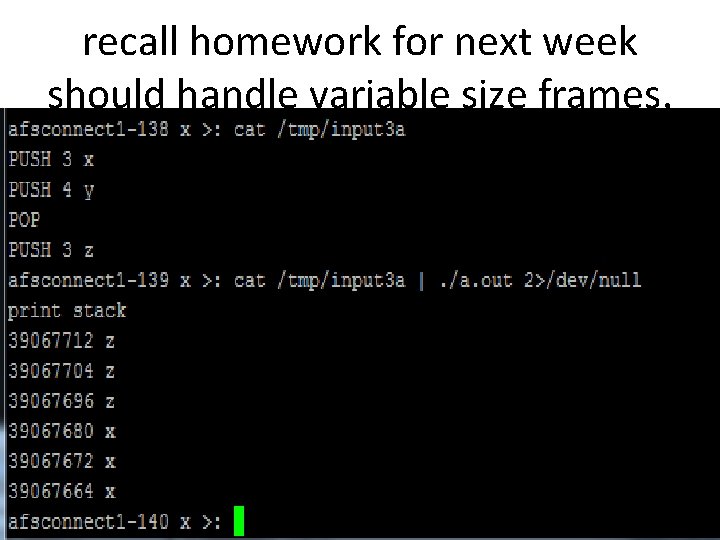
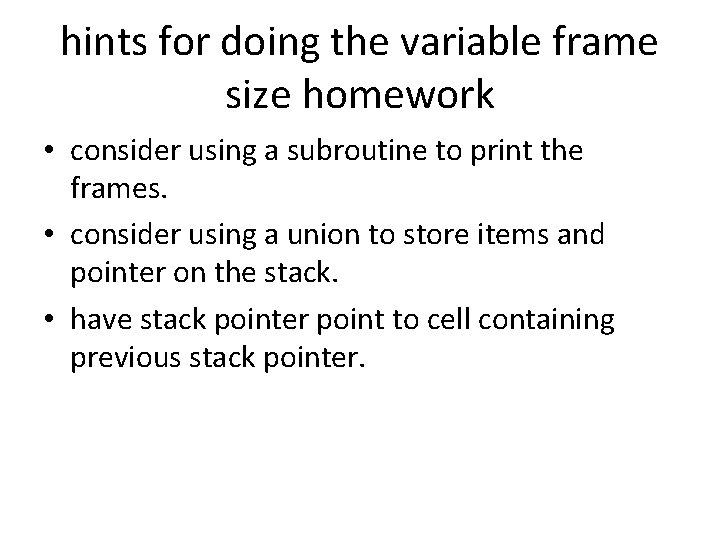
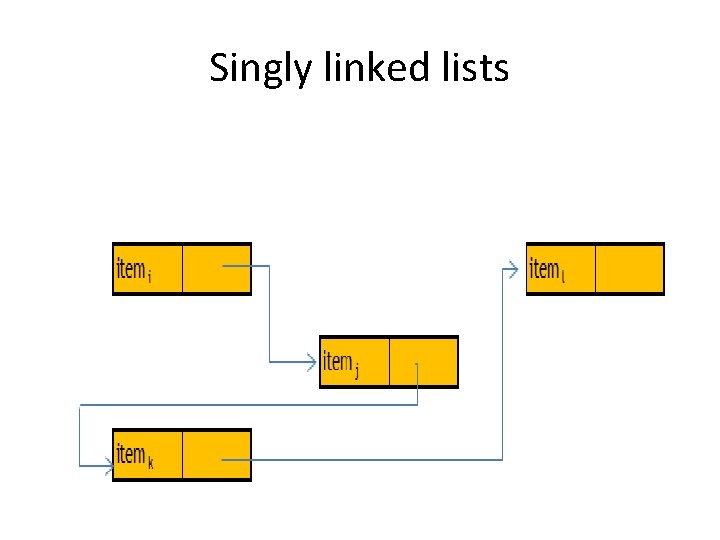
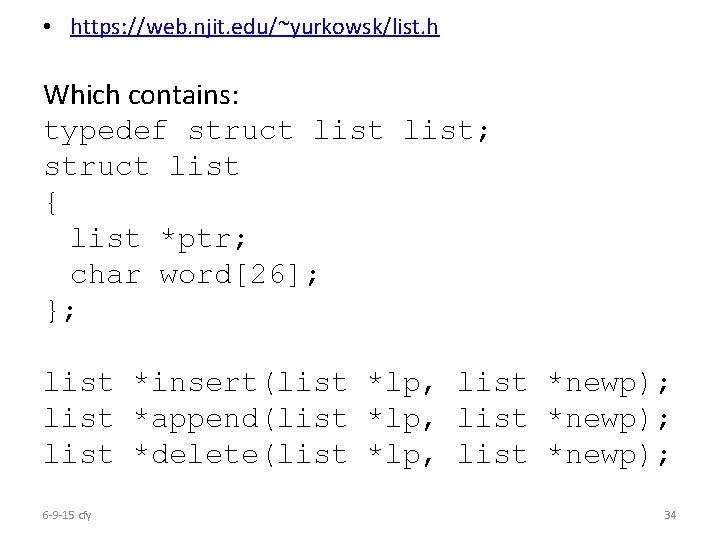
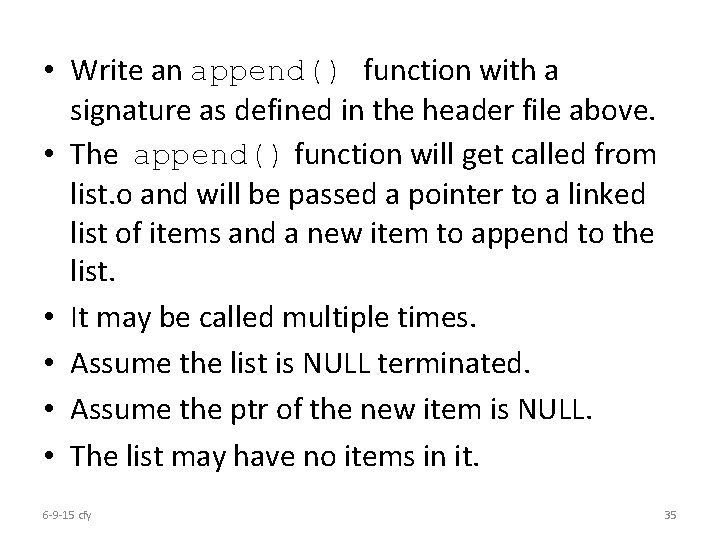
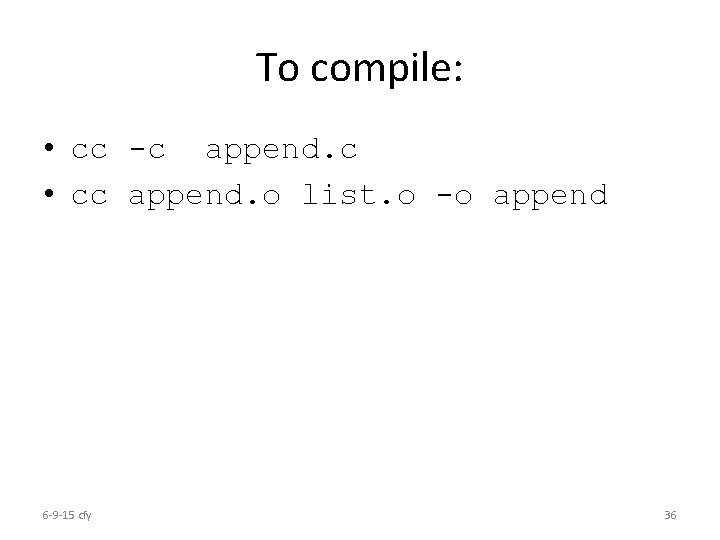
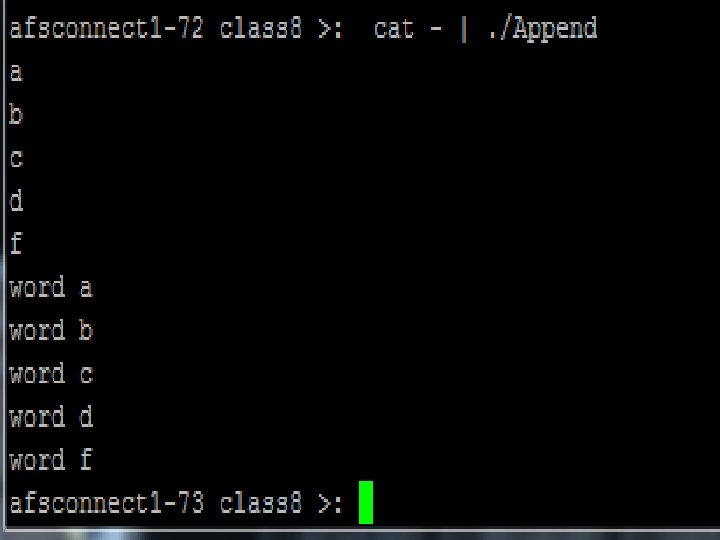
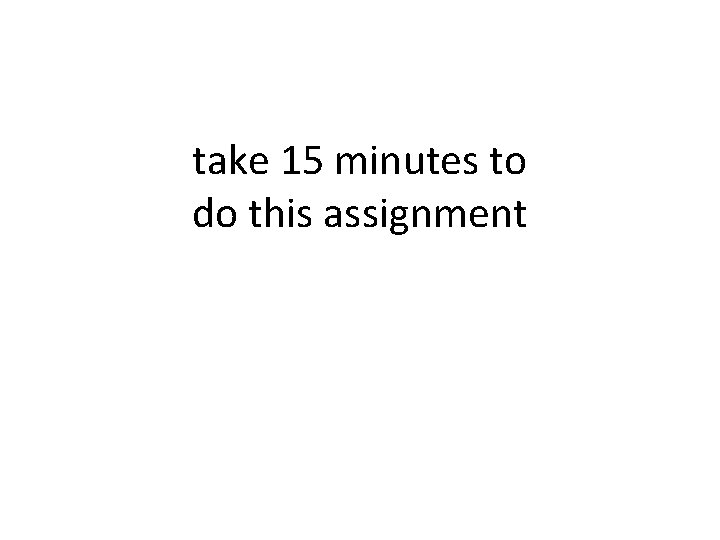
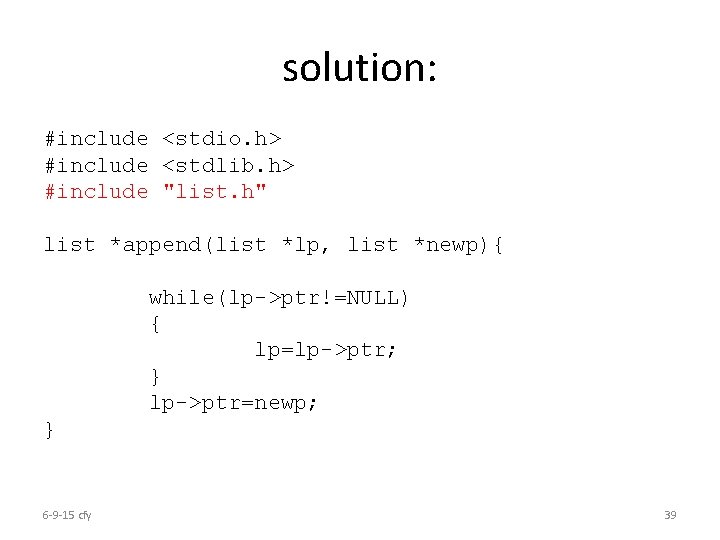
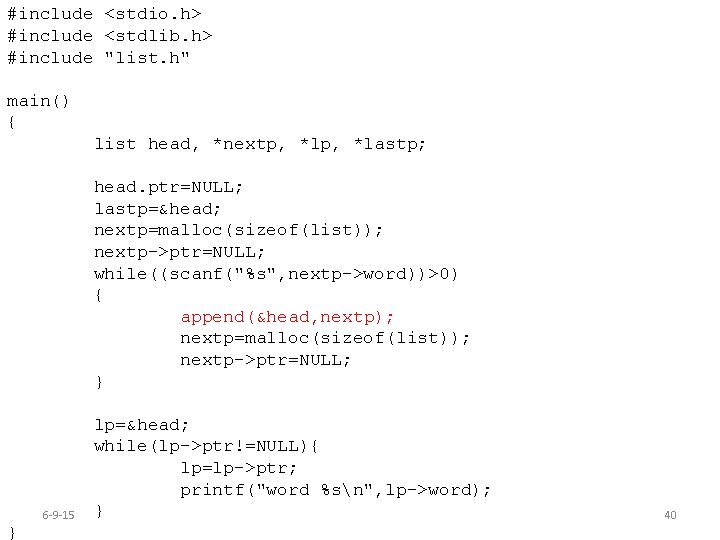
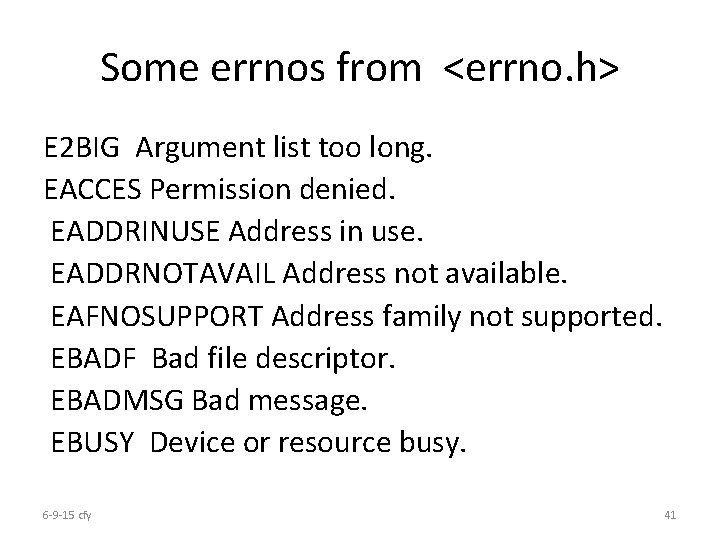
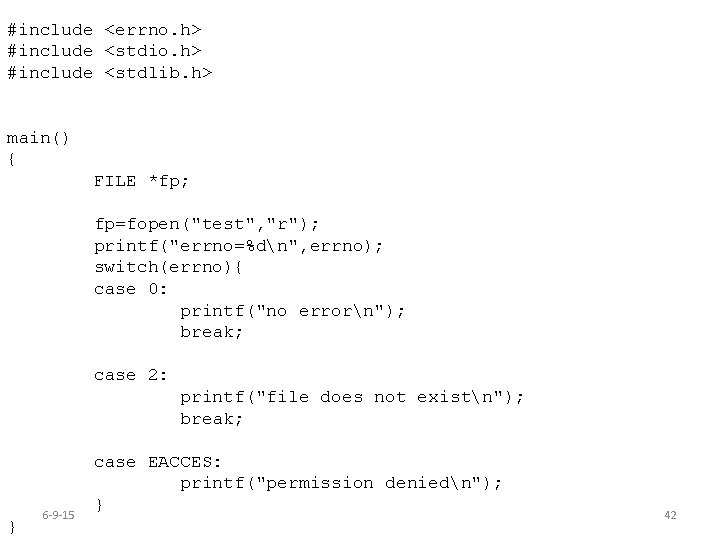
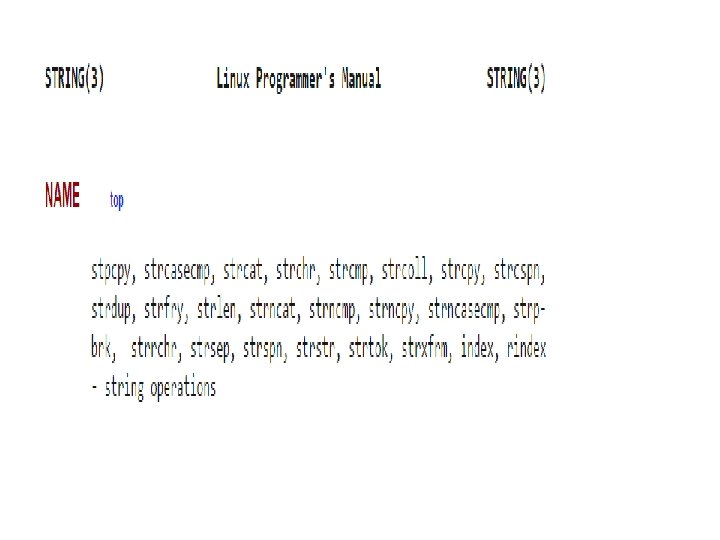
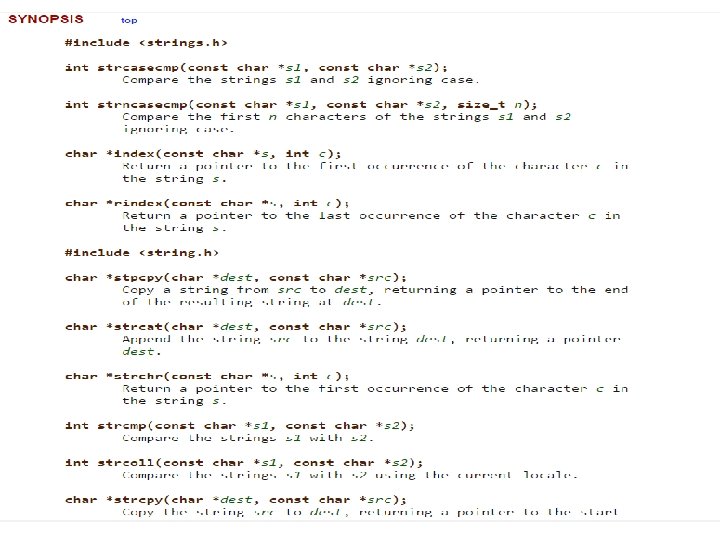
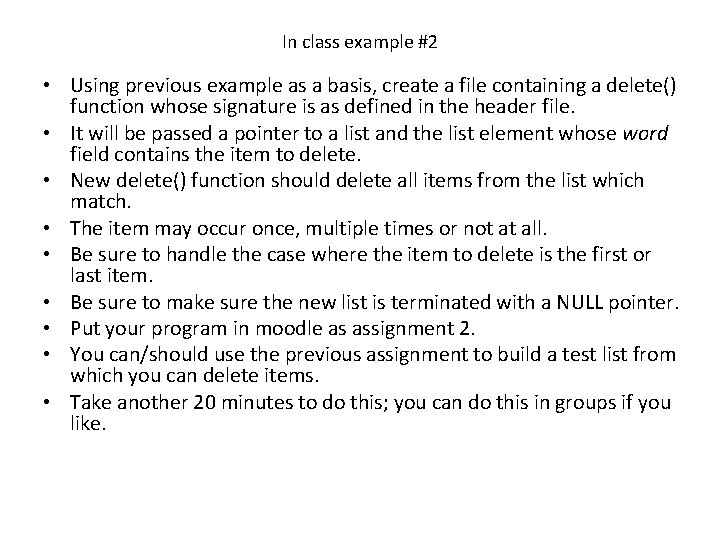
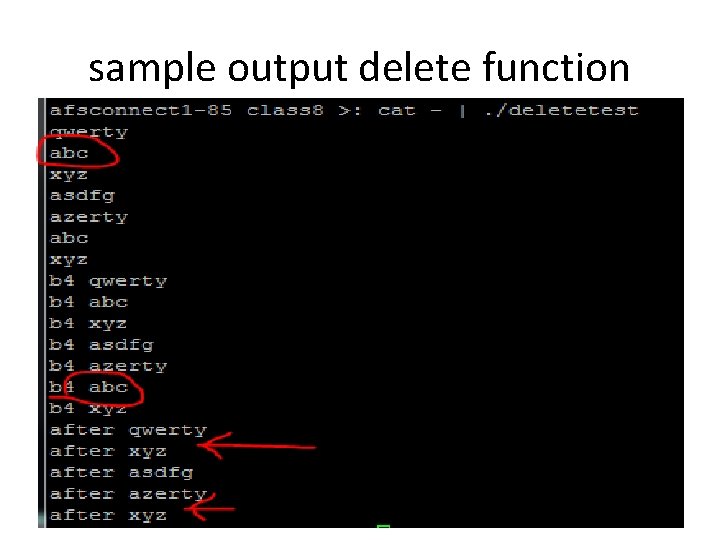
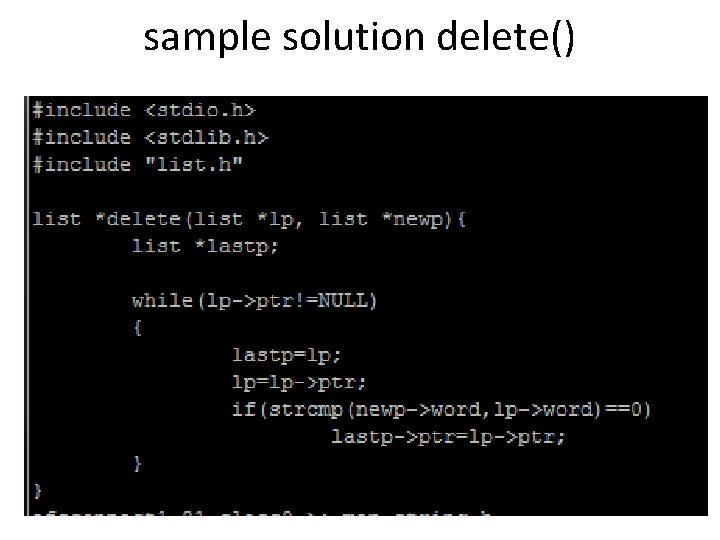
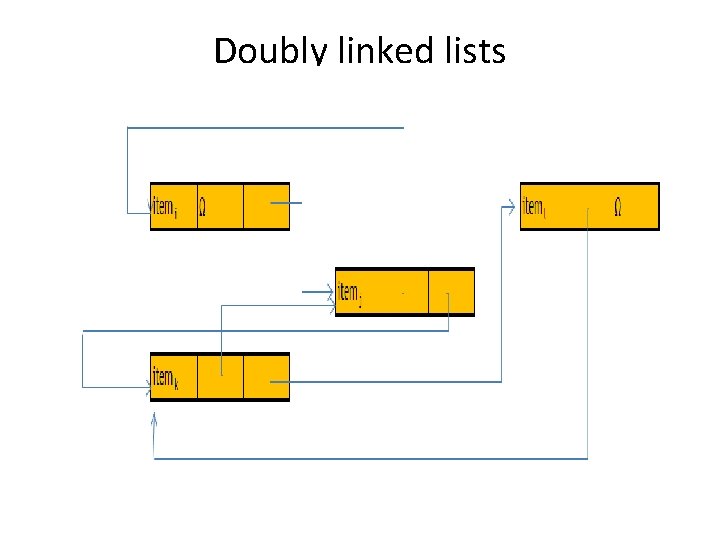
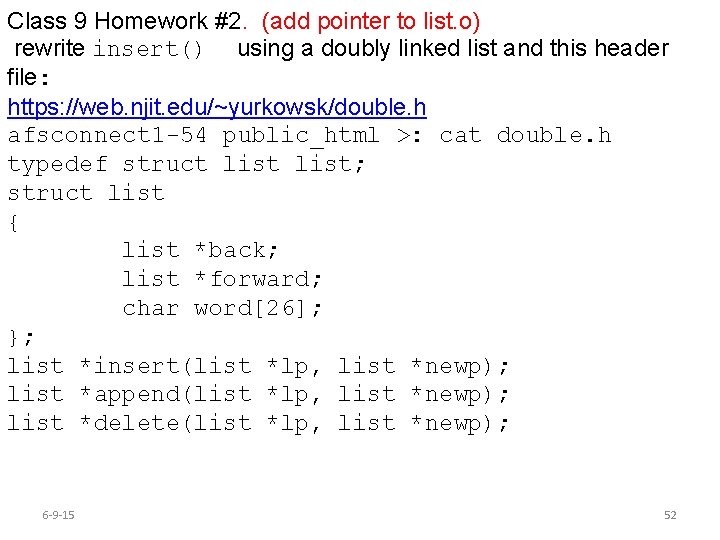
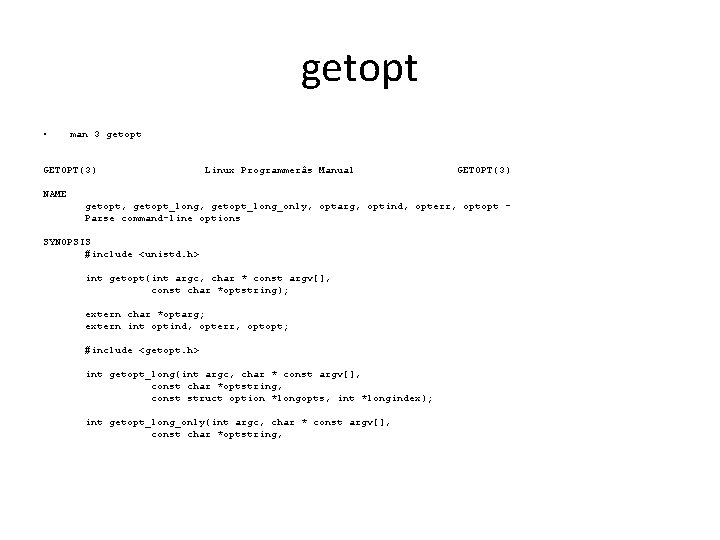
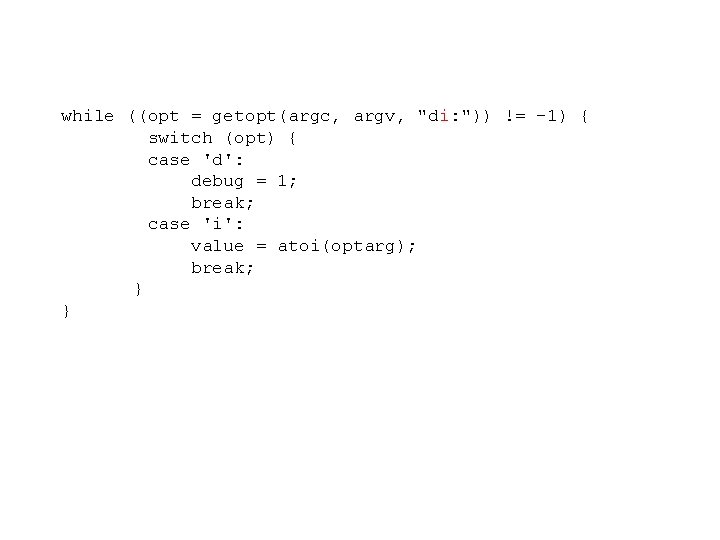
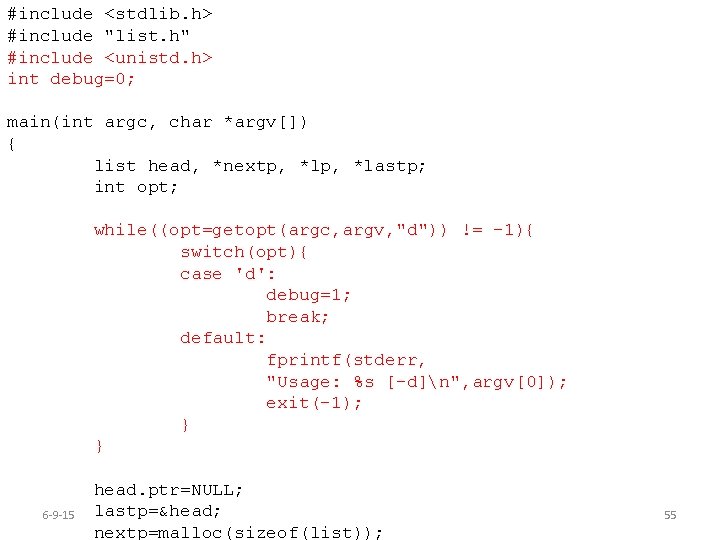
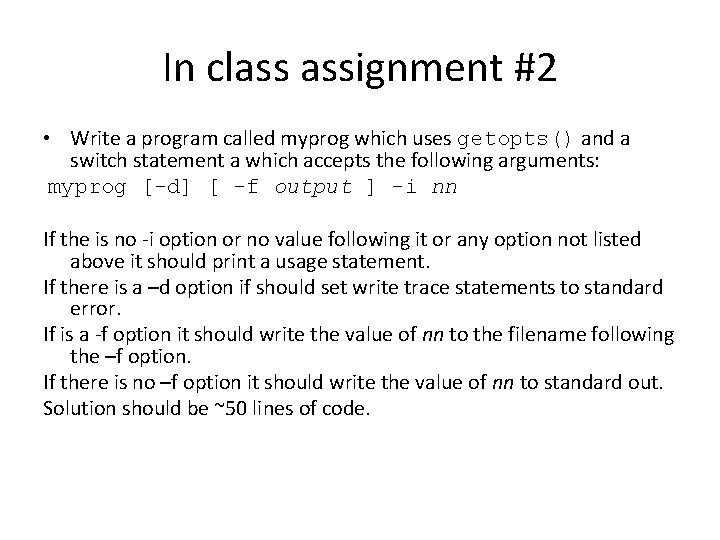
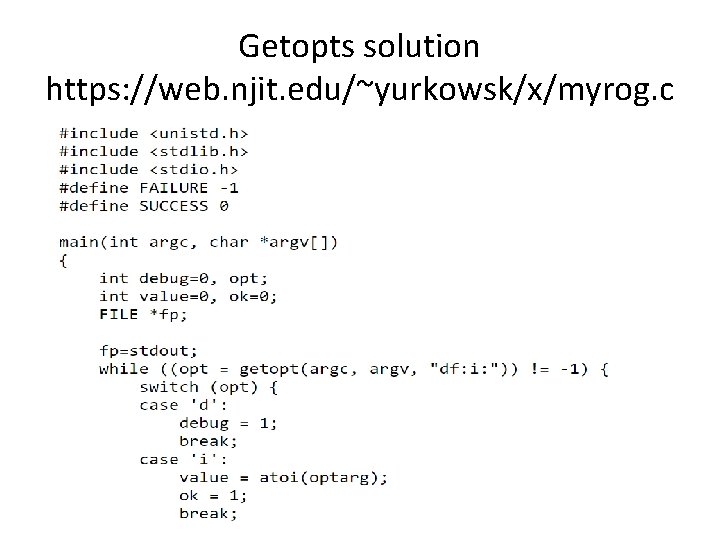
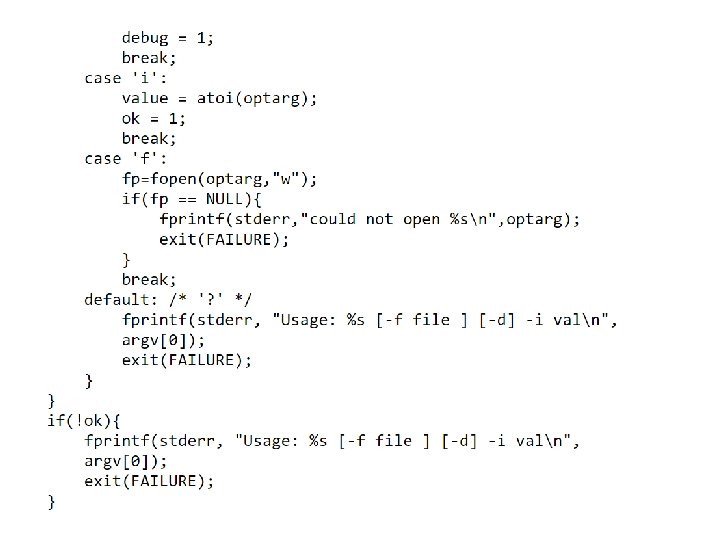
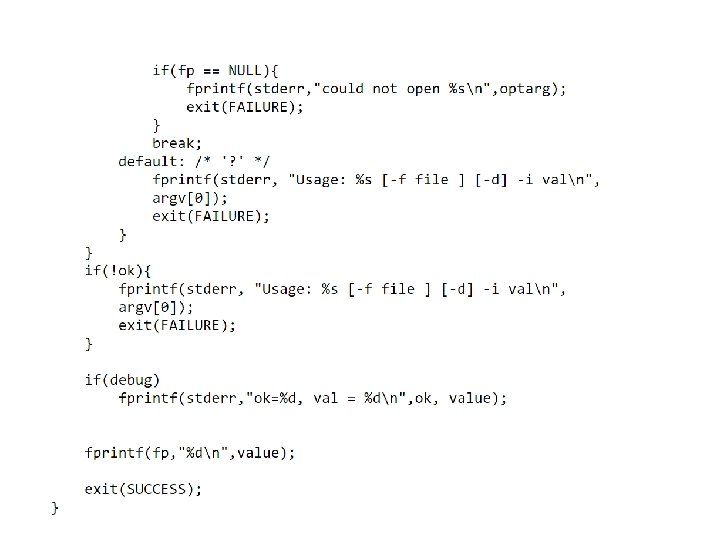
- Slides: 56
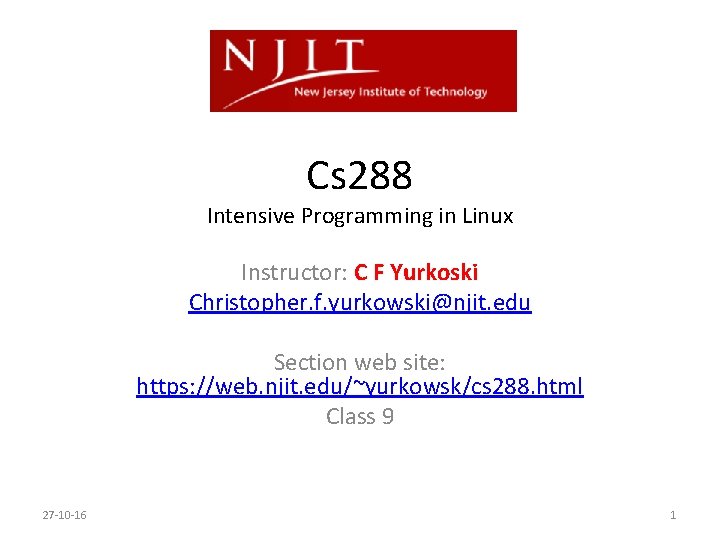
Cs 288 Intensive Programming in Linux Instructor: C F Yurkoski Christopher. f. yurkowski@njit. edu Section web site: https: //web. njit. edu/~yurkowsk/cs 288. html Class 9 27 -10 -16 1
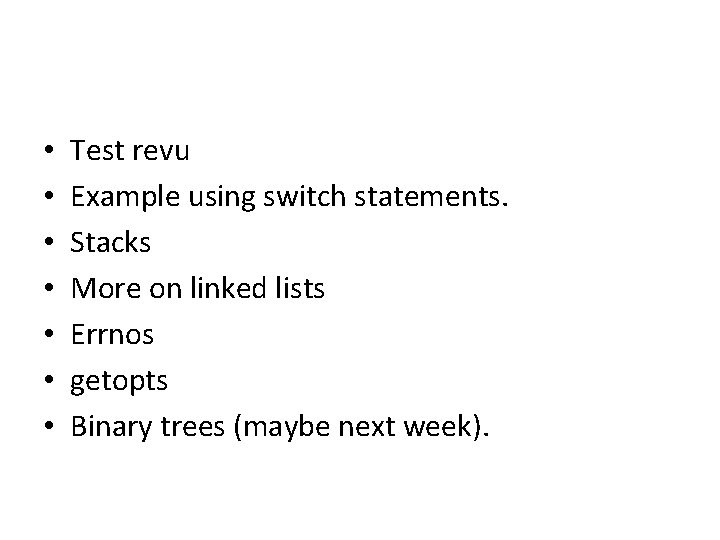
• • Test revu Example using switch statements. Stacks More on linked lists Errnos getopts Binary trees (maybe next week).
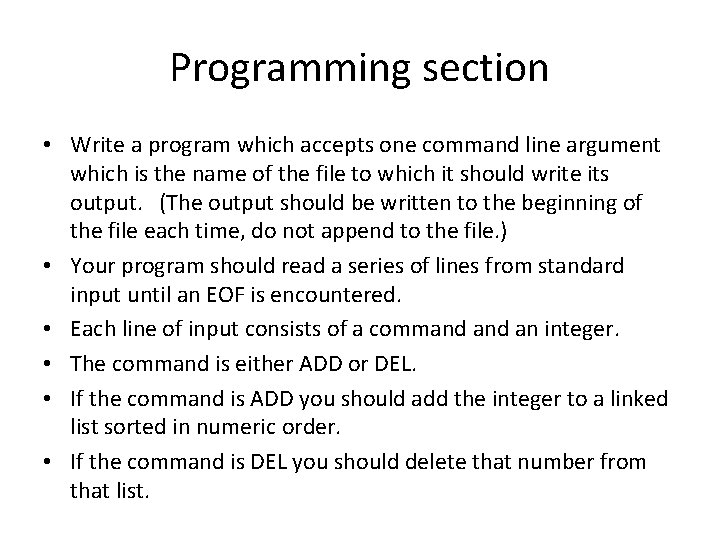
Programming section • Write a program which accepts one command line argument which is the name of the file to which it should write its output. (The output should be written to the beginning of the file each time, do not append to the file. ) • Your program should read a series of lines from standard input until an EOF is encountered. • Each line of input consists of a command an integer. • The command is either ADD or DEL. • If the command is ADD you should add the integer to a linked list sorted in numeric order. • If the command is DEL you should delete that number from that list.
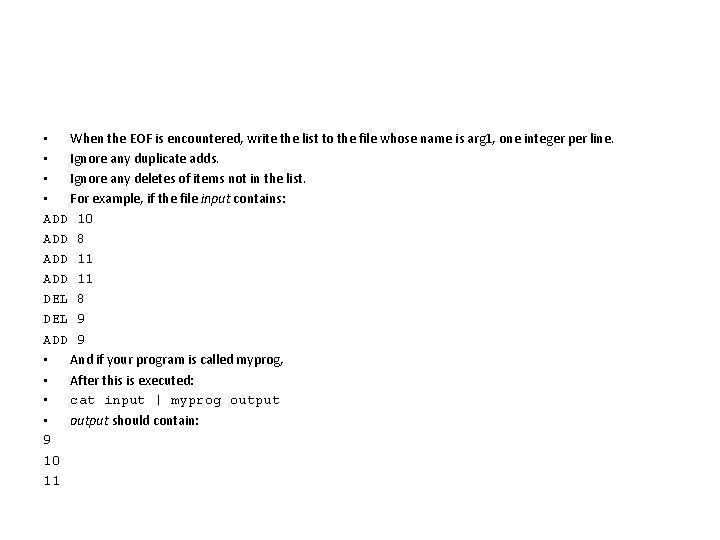
• When the EOF is encountered, write the list to the file whose name is arg 1, one integer per line. • Ignore any duplicate adds. • Ignore any deletes of items not in the list. • For example, if the file input contains: ADD 10 ADD 8 ADD 11 DEL 8 DEL 9 ADD 9 • And if your program is called myprog, • After this is executed: • cat input | myprog output • output should contain: 9 10 11
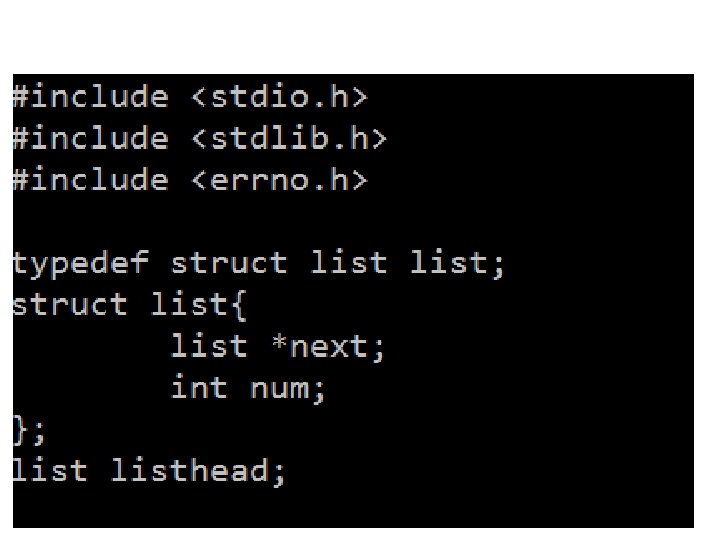
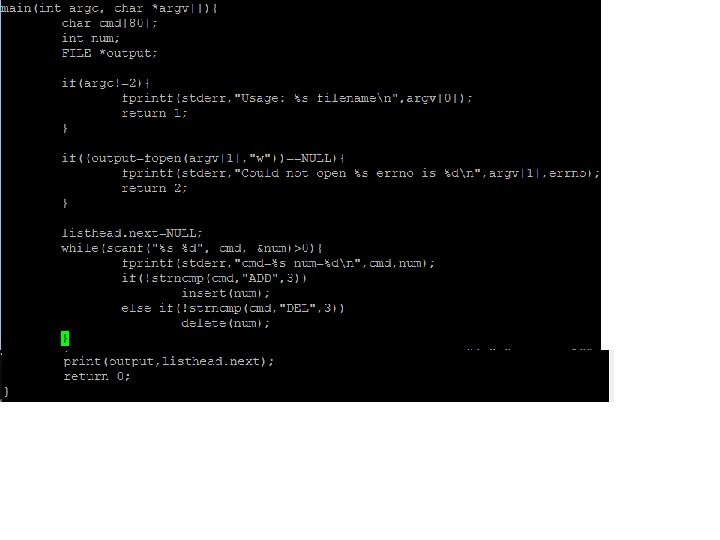
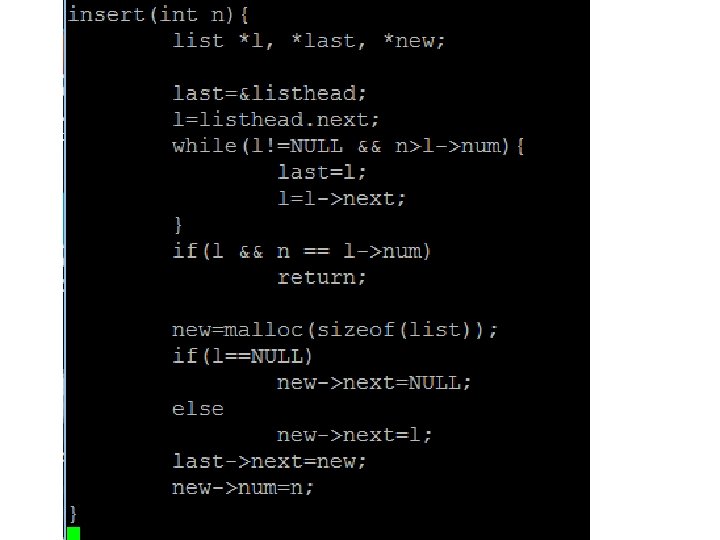
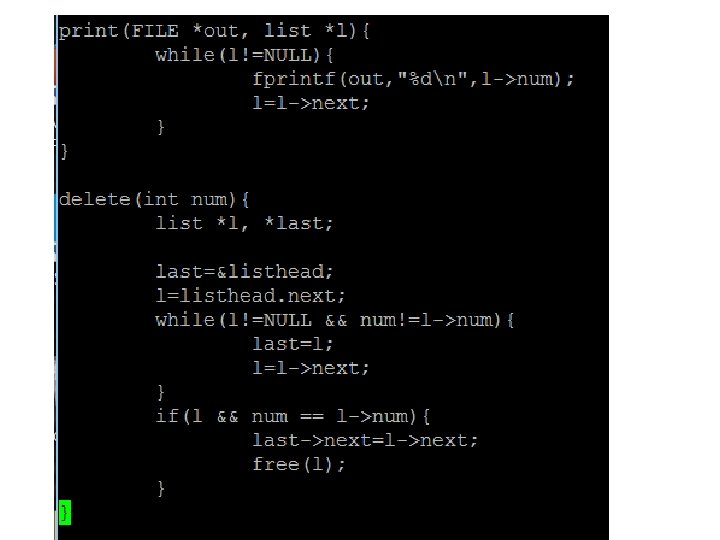
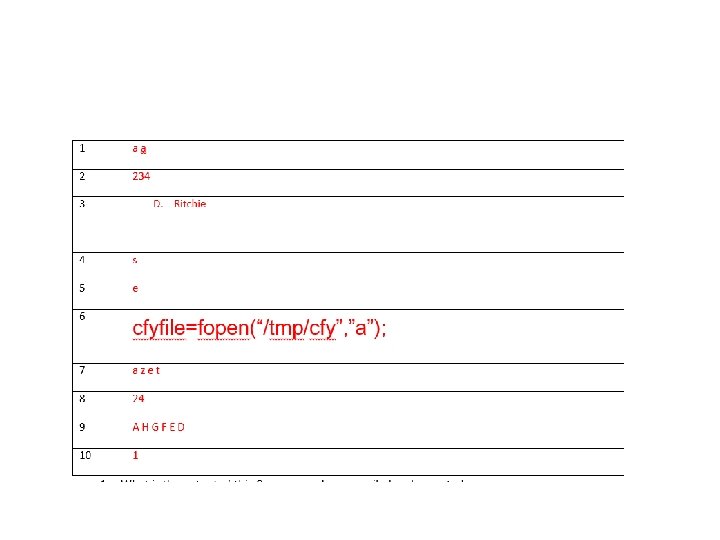
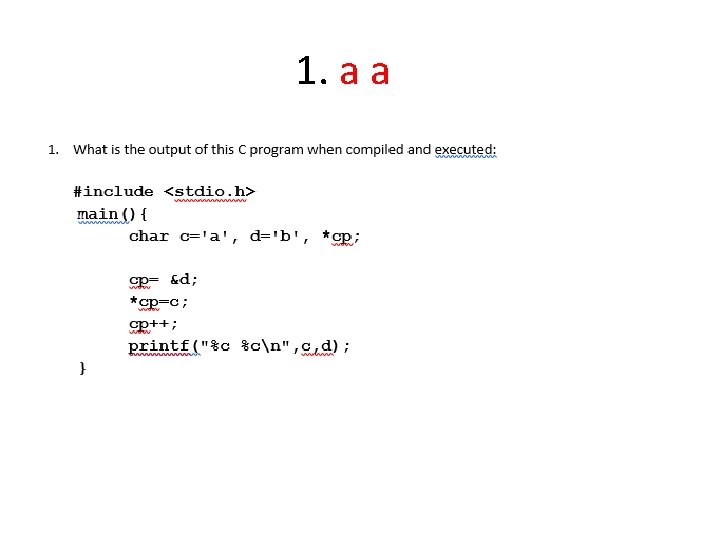
1. a a
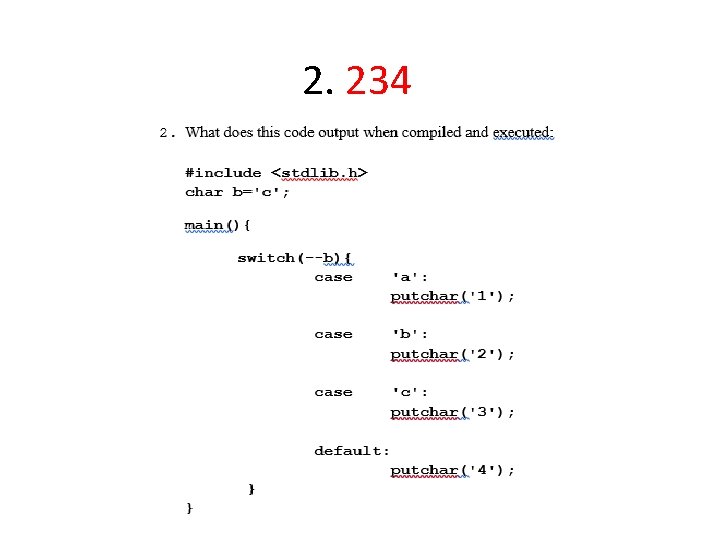
2. 234
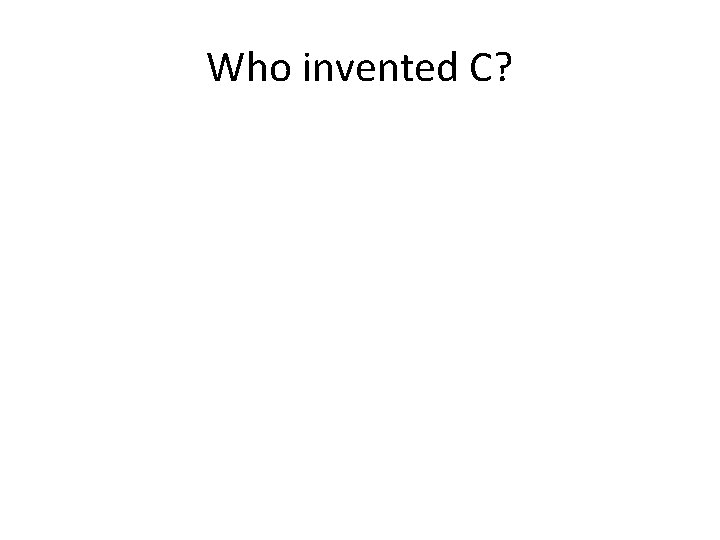
Who invented C?
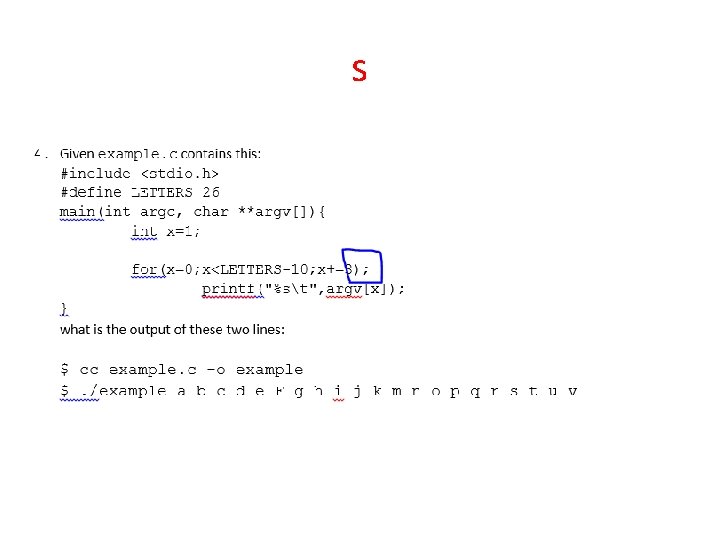
s
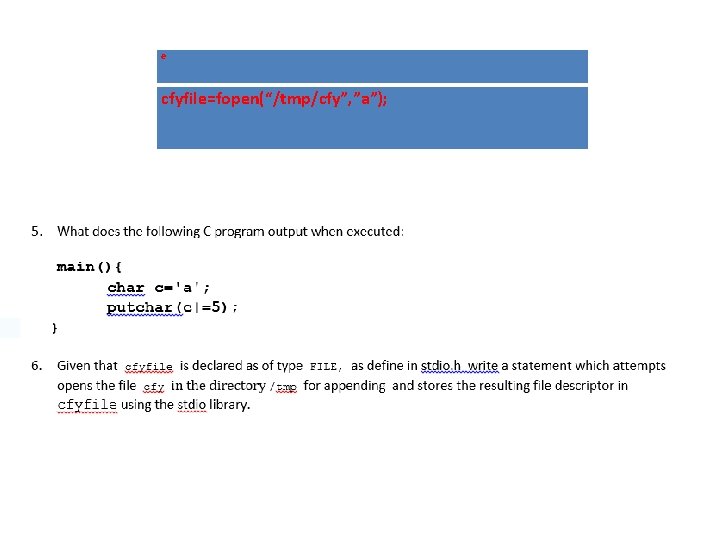
e cfyfile=fopen(“/tmp/cfy”, ”a”);
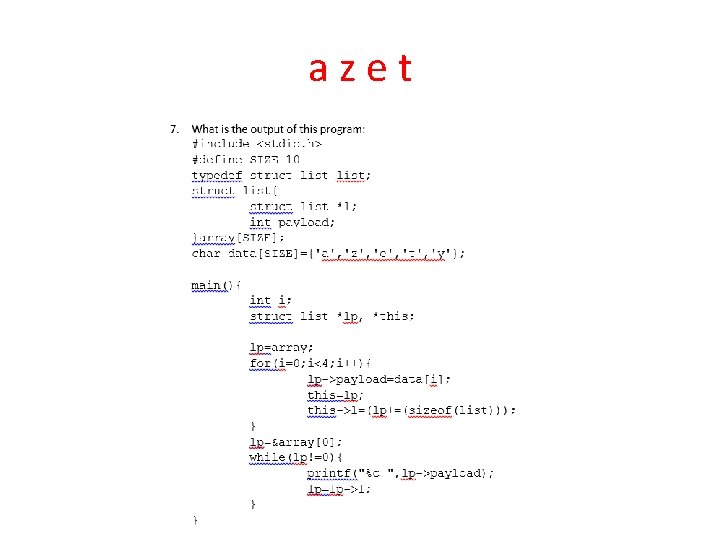
azet
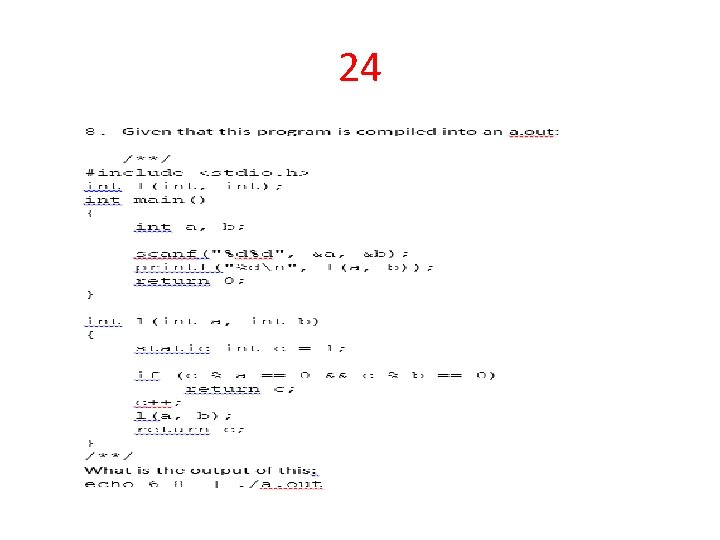
24
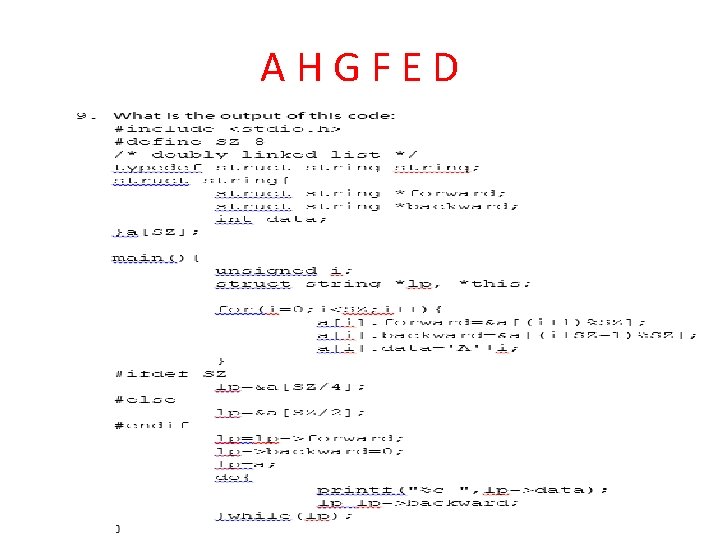
AHGFED
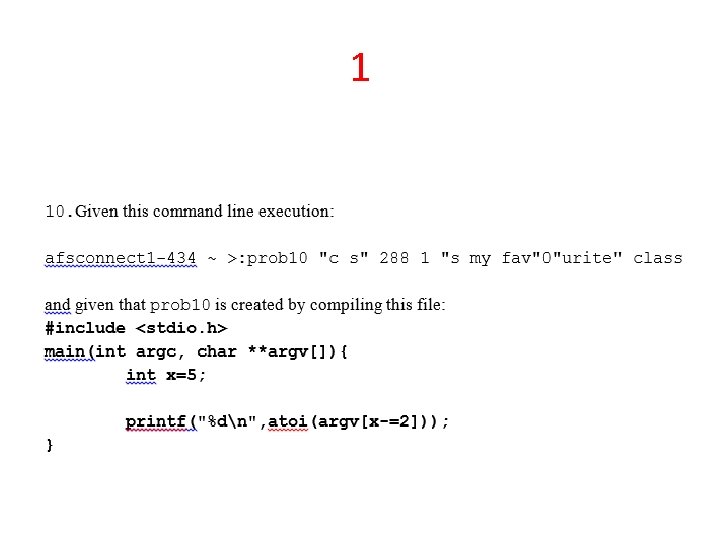
1
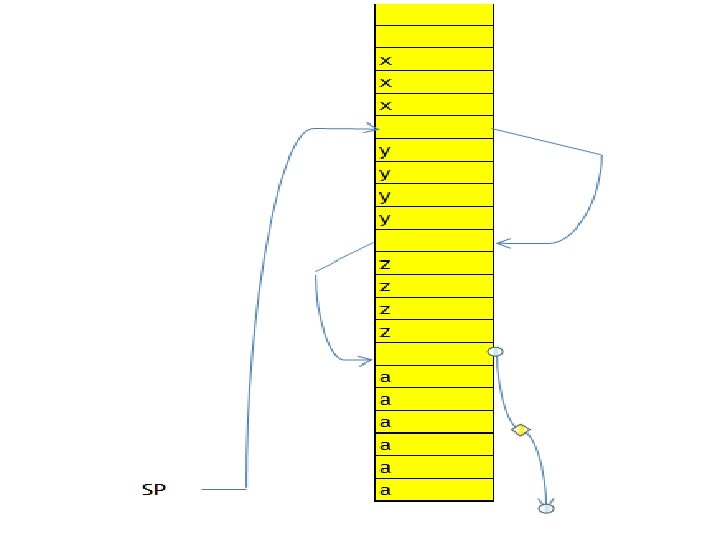
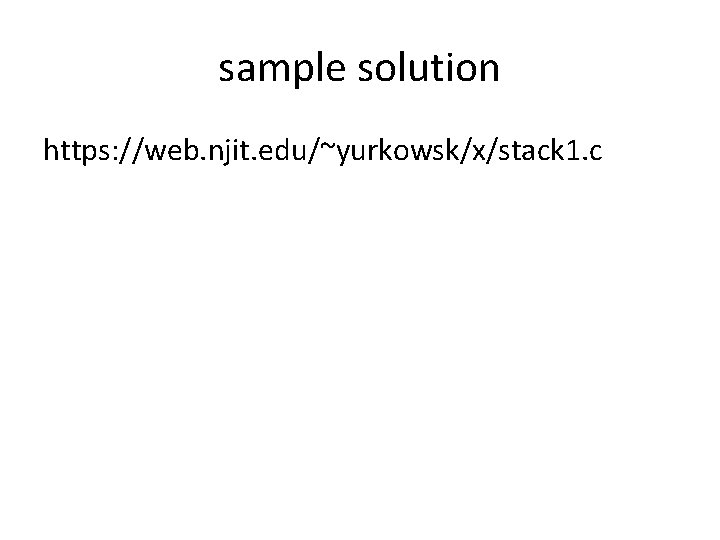
sample solution https: //web. njit. edu/~yurkowsk/x/stack 1. c
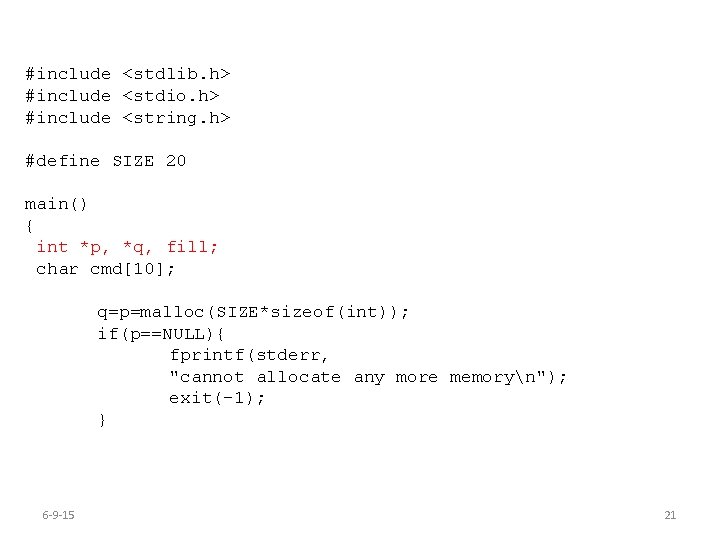
#include <stdlib. h> #include <stdio. h> #include <string. h> #define SIZE 20 main() { int *p, *q, fill; char cmd[10]; q=p=malloc(SIZE*sizeof(int)); if(p==NULL){ fprintf(stderr, "cannot allocate any more memoryn"); exit(-1); } 6 -9 -15 21
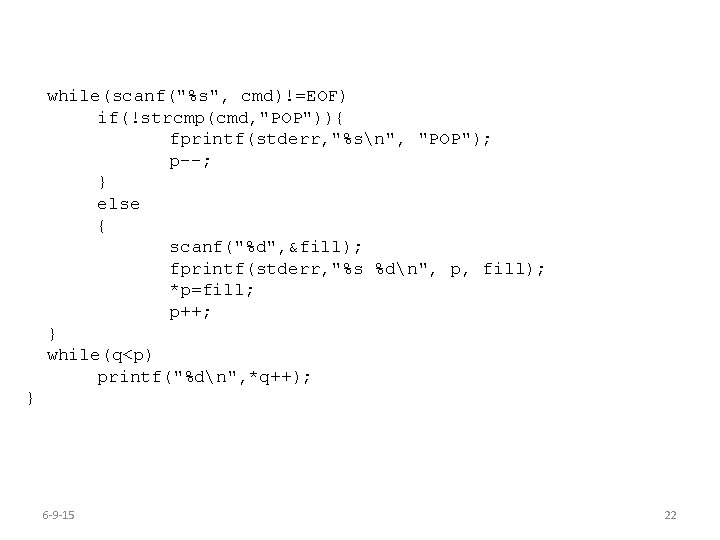
while(scanf("%s", cmd)!=EOF) if(!strcmp(cmd, "POP")){ fprintf(stderr, "%sn", "POP"); p--; } else { scanf("%d", &fill); fprintf(stderr, "%s %dn", p, fill); *p=fill; p++; } while(q<p) printf("%dn", *q++); } 6 -9 -15 22
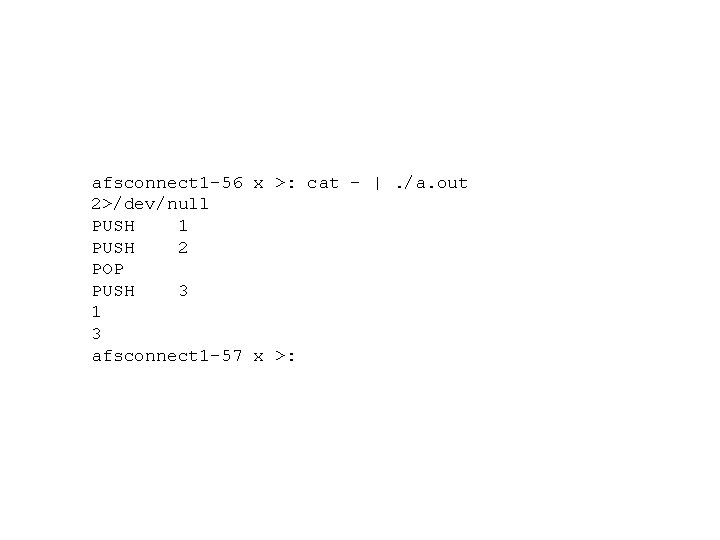
afsconnect 1 -56 x >: cat - |. /a. out 2>/dev/null PUSH 1 PUSH 2 POP PUSH 3 1 3 afsconnect 1 -57 x >:
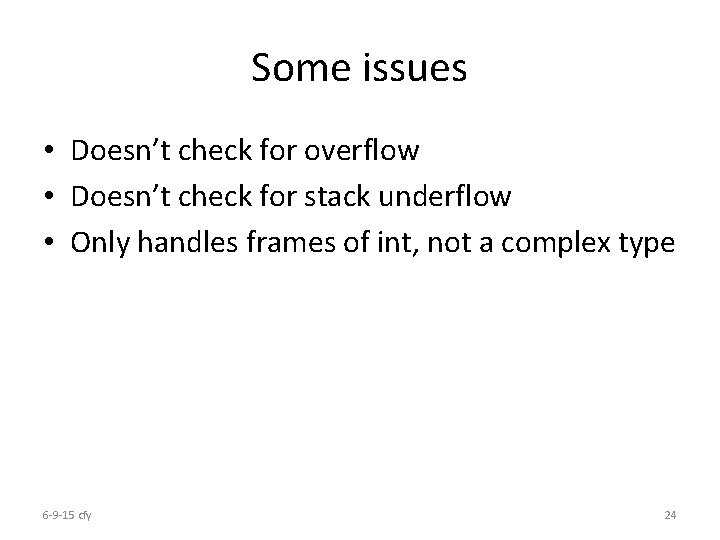
Some issues • Doesn’t check for overflow • Doesn’t check for stack underflow • Only handles frames of int, not a complex type 6 -9 -15 cfy 24
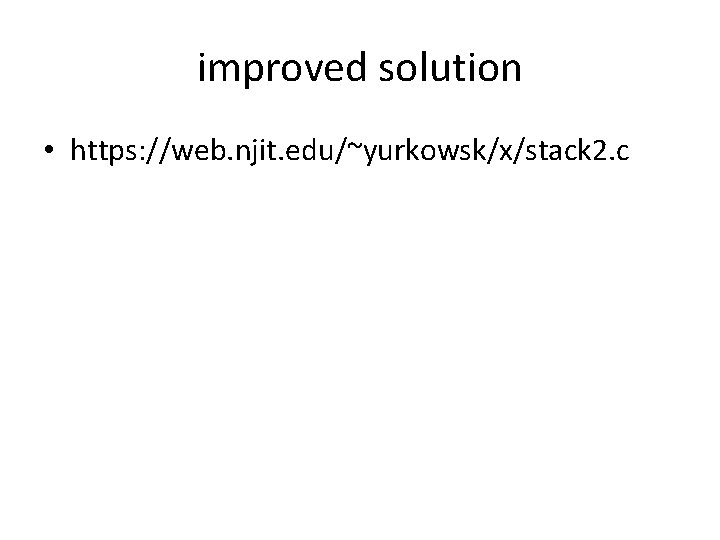
improved solution • https: //web. njit. edu/~yurkowsk/x/stack 2. c
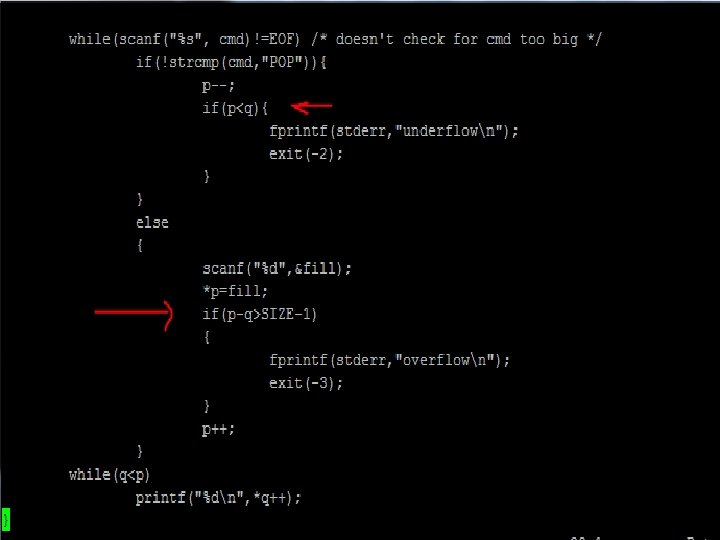
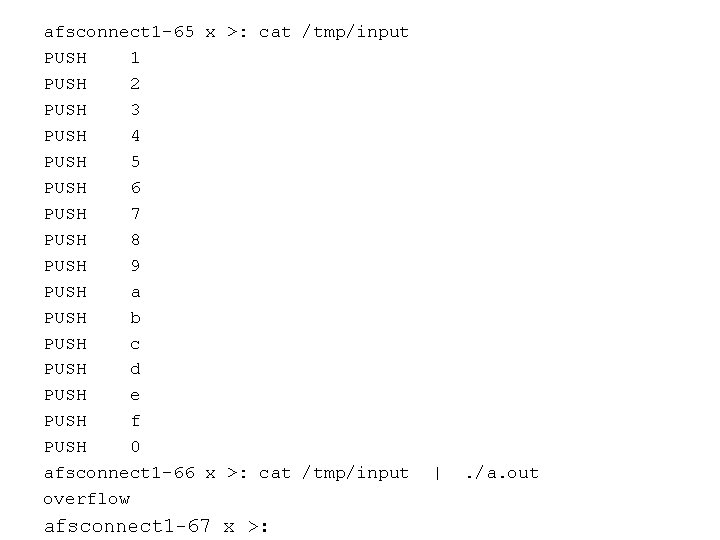
afsconnect 1 -65 x >: cat /tmp/input PUSH 1 PUSH 2 PUSH 3 PUSH 4 PUSH 5 PUSH 6 PUSH 7 PUSH 8 PUSH 9 PUSH a PUSH b PUSH c PUSH d PUSH e PUSH f PUSH 0 afsconnect 1 -66 x >: cat /tmp/input overflow afsconnect 1 -67 x >: | . /a. out
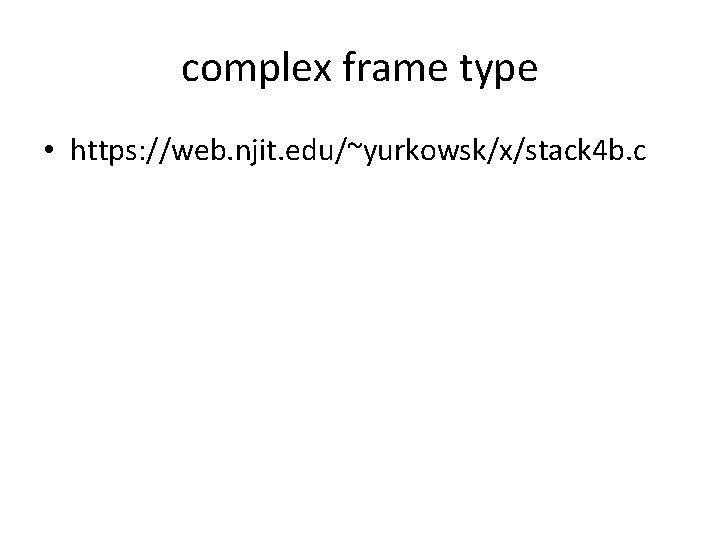
complex frame type • https: //web. njit. edu/~yurkowsk/x/stack 4 b. c
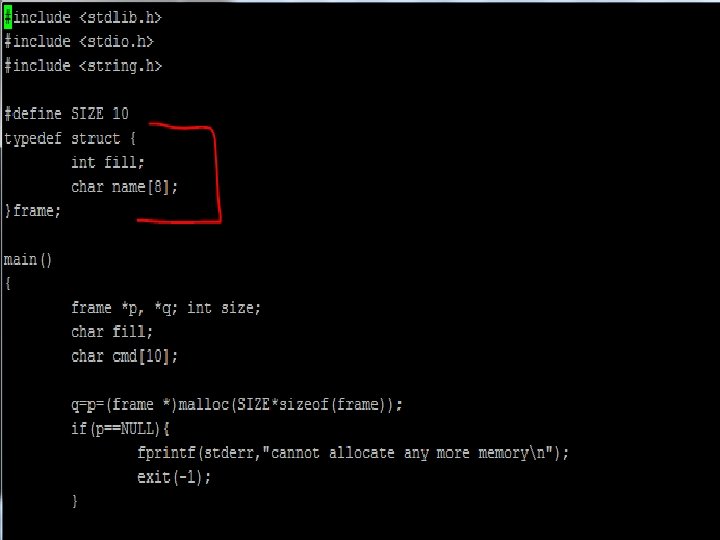
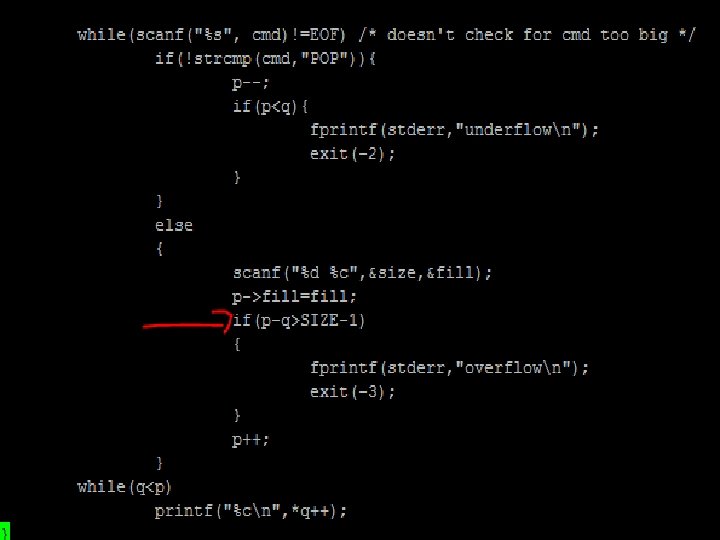
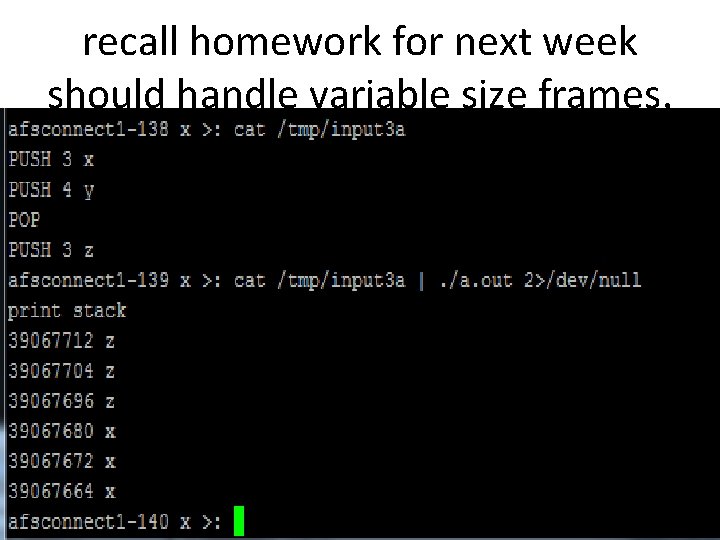
recall homework for next week should handle variable size frames.
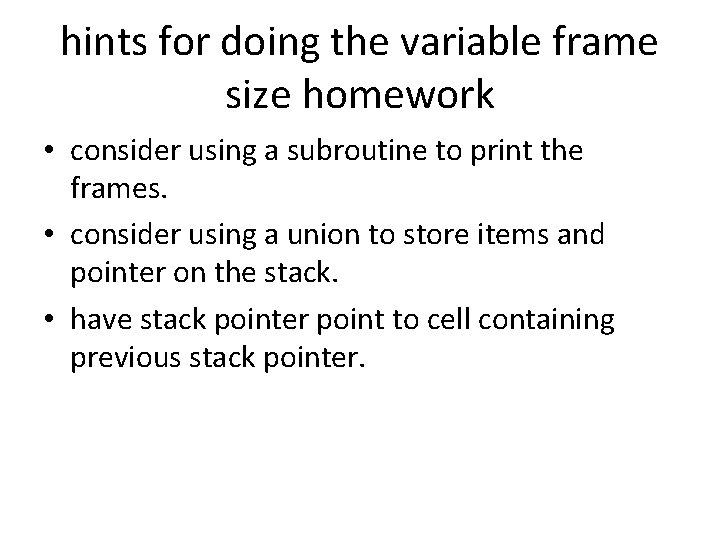
hints for doing the variable frame size homework • consider using a subroutine to print the frames. • consider using a union to store items and pointer on the stack. • have stack pointer point to cell containing previous stack pointer.
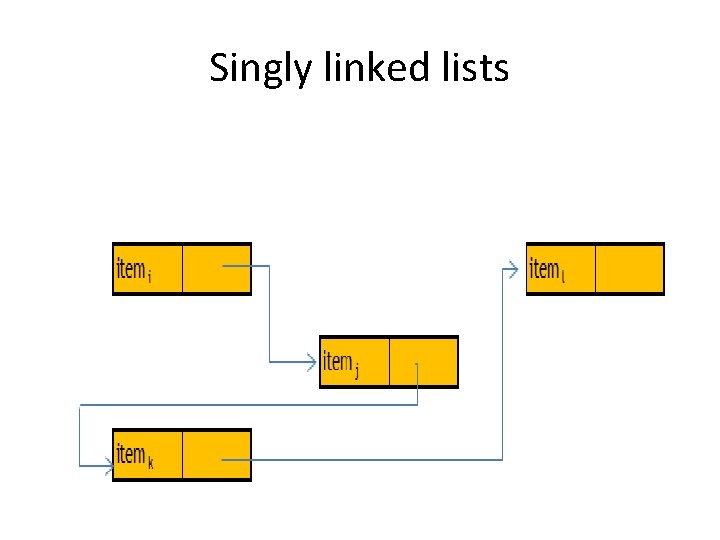
Singly linked lists 6 -9 -15 cfy 33
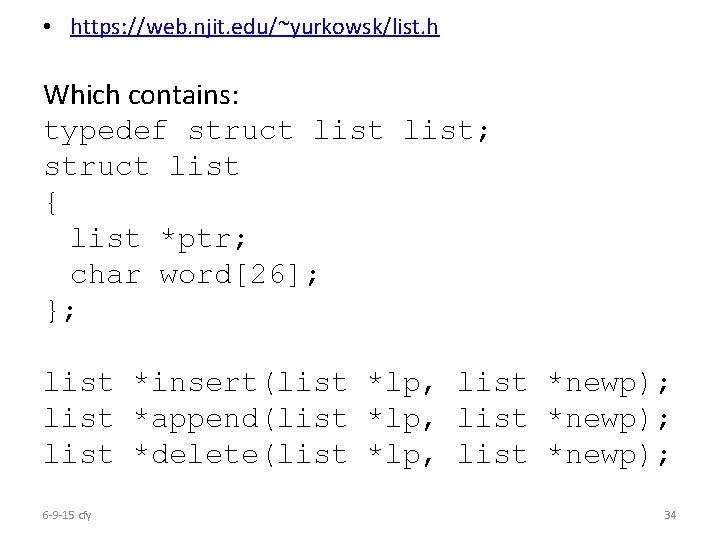
• https: //web. njit. edu/~yurkowsk/list. h Which contains: typedef struct list; struct list { list *ptr; char word[26]; }; list *insert(list *lp, list *newp); list *append(list *lp, list *newp); list *delete(list *lp, list *newp); 6 -9 -15 cfy 34
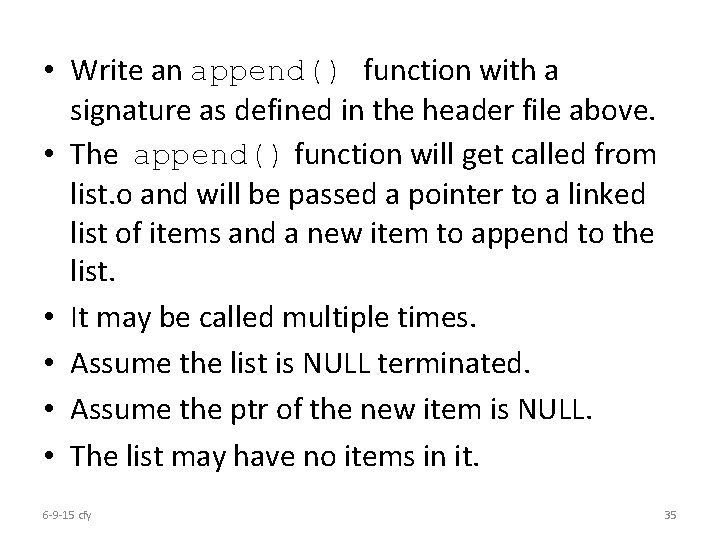
• Write an append() function with a signature as defined in the header file above. • The append() function will get called from list. o and will be passed a pointer to a linked list of items and a new item to append to the list. • It may be called multiple times. • Assume the list is NULL terminated. • Assume the ptr of the new item is NULL. • The list may have no items in it. 6 -9 -15 cfy 35
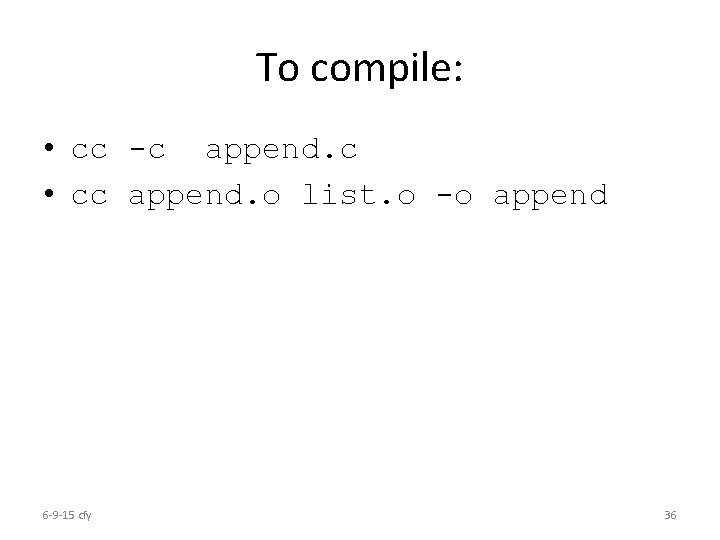
To compile: • cc -c append. c • cc append. o list. o -o append 6 -9 -15 cfy 36
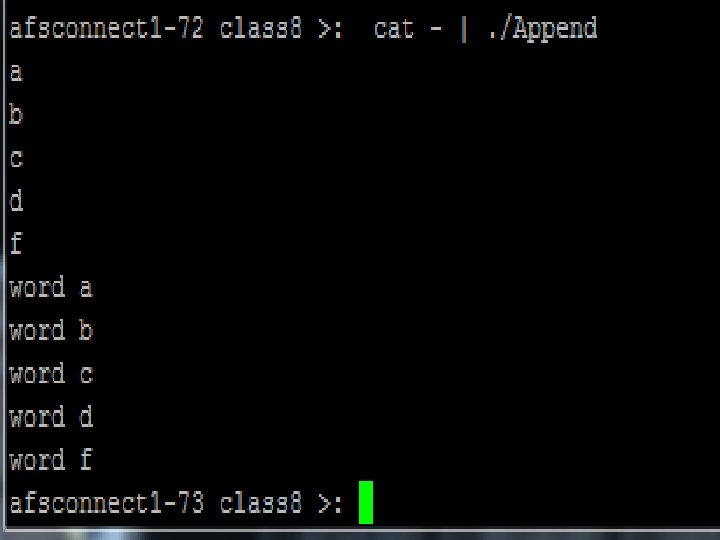
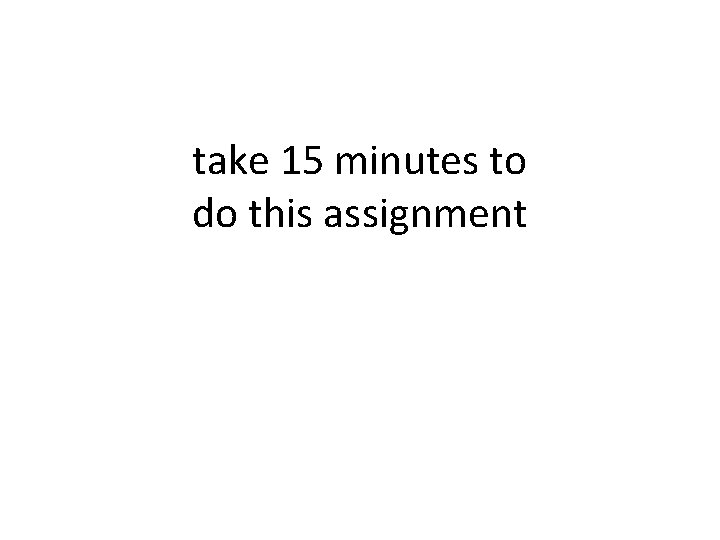
take 15 minutes to do this assignment
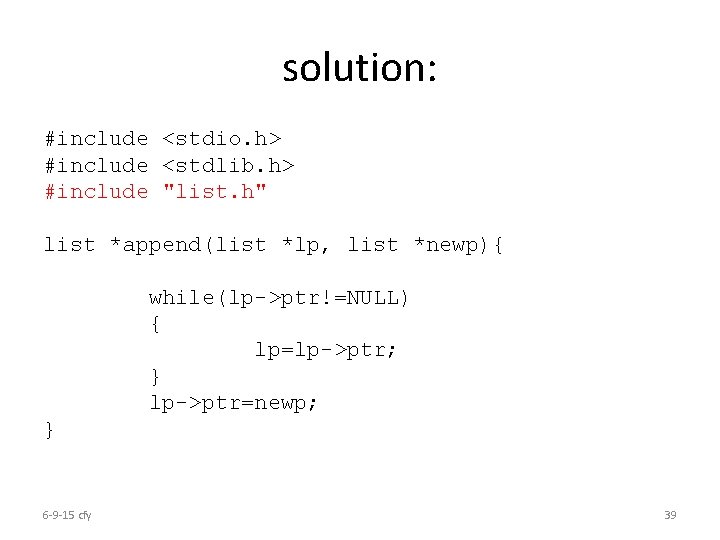
solution: #include <stdio. h> #include <stdlib. h> #include "list. h" list *append(list *lp, list *newp){ while(lp->ptr!=NULL) { lp=lp->ptr; } lp->ptr=newp; } 6 -9 -15 cfy 39
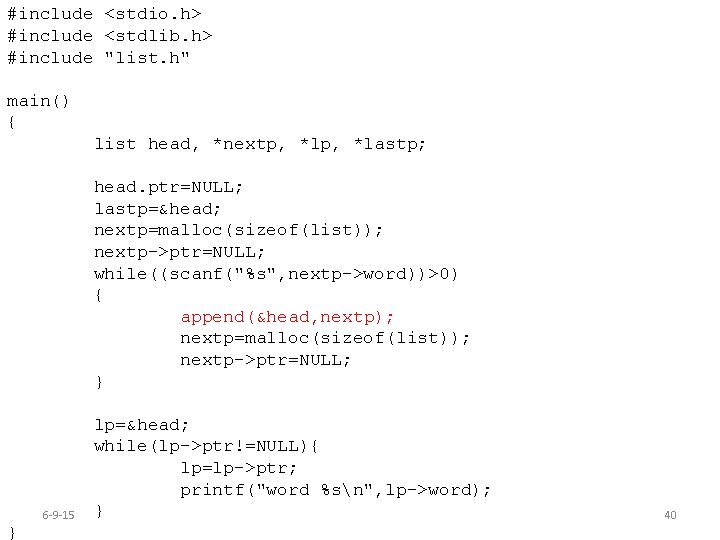
#include <stdio. h> #include <stdlib. h> #include "list. h" main() { list head, *nextp, *lastp; head. ptr=NULL; lastp=&head; nextp=malloc(sizeof(list)); nextp->ptr=NULL; while((scanf("%s", nextp->word))>0) { append(&head, nextp); nextp=malloc(sizeof(list)); nextp->ptr=NULL; } 6 -9 -15 } lp=&head; while(lp->ptr!=NULL){ lp=lp->ptr; printf("word %sn", lp->word); } 40
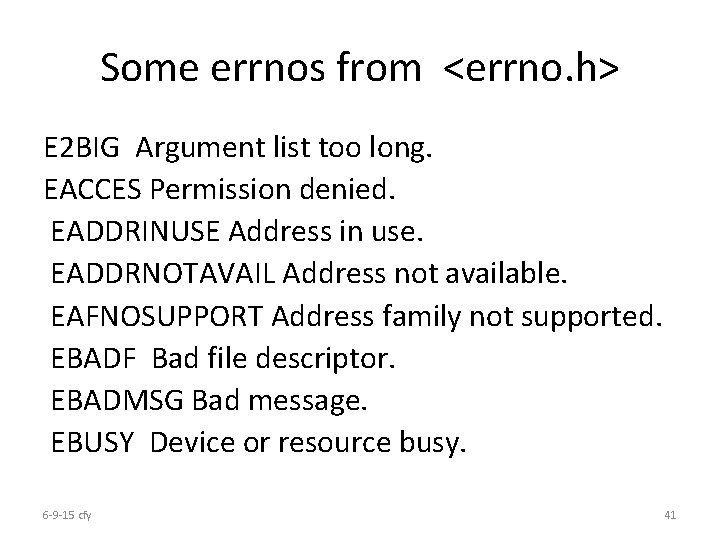
Some errnos from <errno. h> E 2 BIG Argument list too long. EACCES Permission denied. EADDRINUSE Address in use. EADDRNOTAVAIL Address not available. EAFNOSUPPORT Address family not supported. EBADF Bad file descriptor. EBADMSG Bad message. EBUSY Device or resource busy. 6 -9 -15 cfy 41
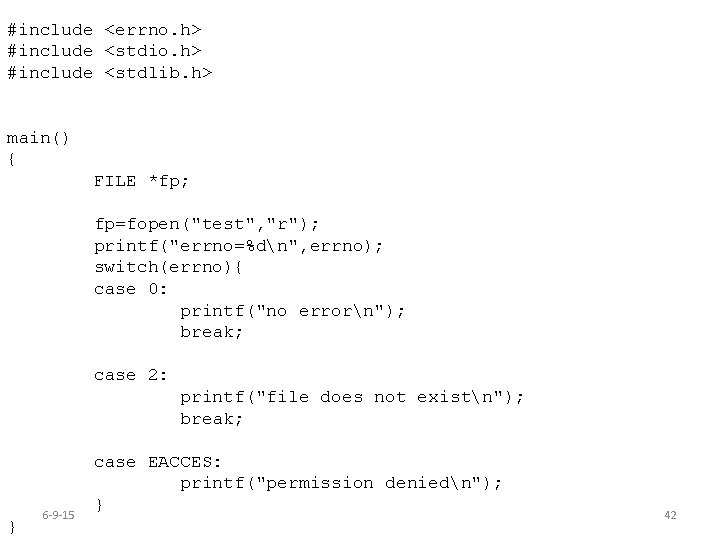
#include <errno. h> #include <stdio. h> #include <stdlib. h> main() { FILE *fp; fp=fopen("test", "r"); printf("errno=%dn", errno); switch(errno){ case 0: printf("no errorn"); break; case 2: printf("file does not existn"); break; } 6 -9 -15 case EACCES: printf("permission deniedn"); } 42
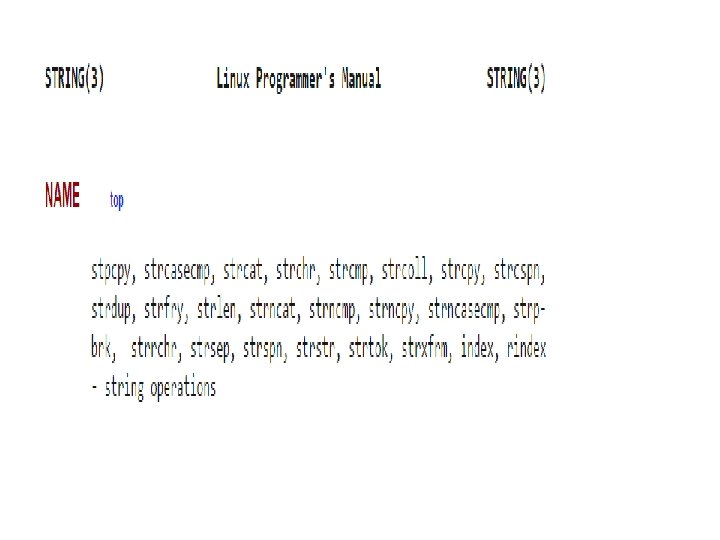
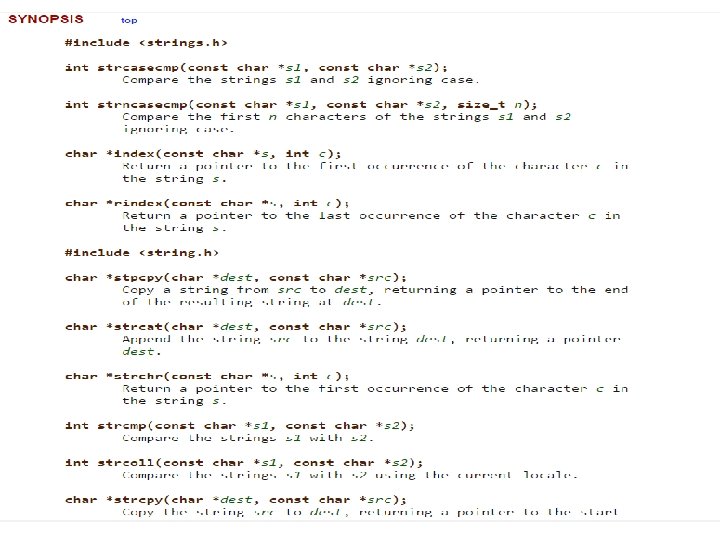
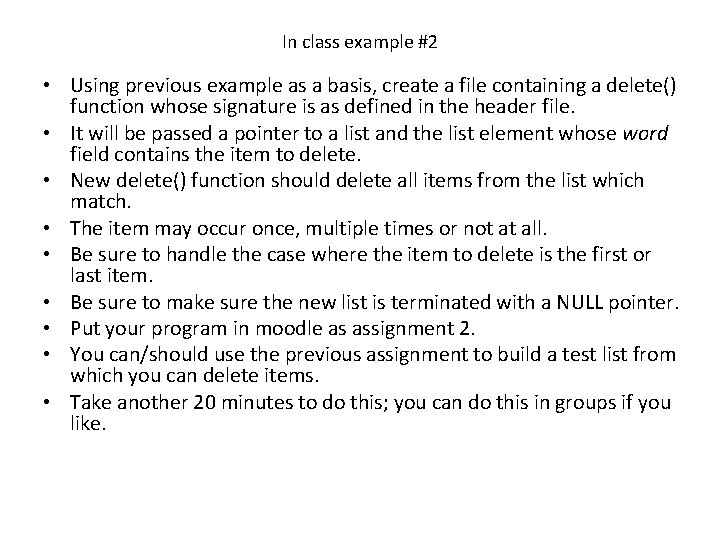
In class example #2 • Using previous example as a basis, create a file containing a delete() function whose signature is as defined in the header file. • It will be passed a pointer to a list and the list element whose word field contains the item to delete. • New delete() function should delete all items from the list which match. • The item may occur once, multiple times or not at all. • Be sure to handle the case where the item to delete is the first or last item. • Be sure to make sure the new list is terminated with a NULL pointer. • Put your program in moodle as assignment 2. • You can/should use the previous assignment to build a test list from which you can delete items. • Take another 20 minutes to do this; you can do this in groups if you like.
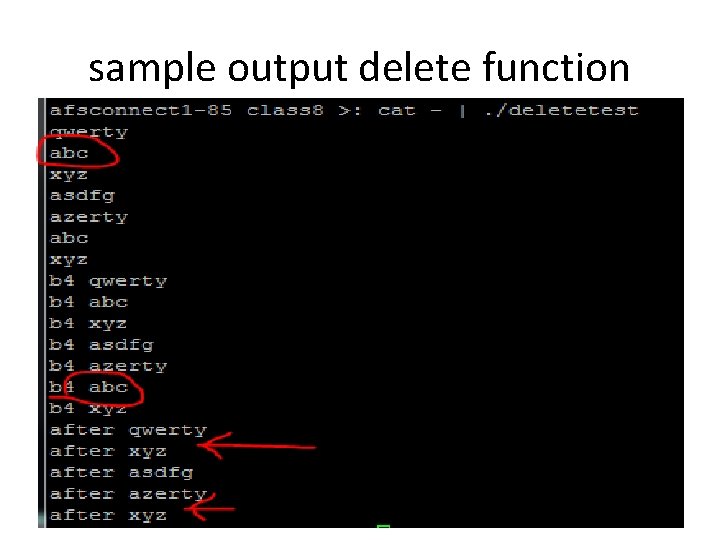
sample output delete function
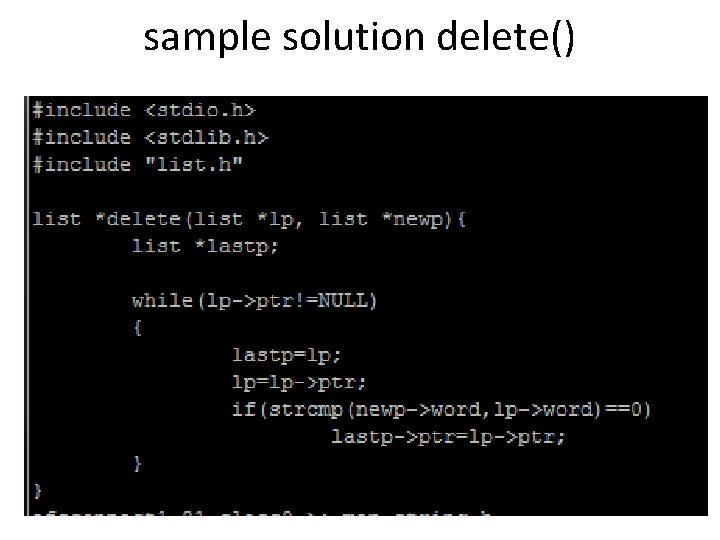
sample solution delete()
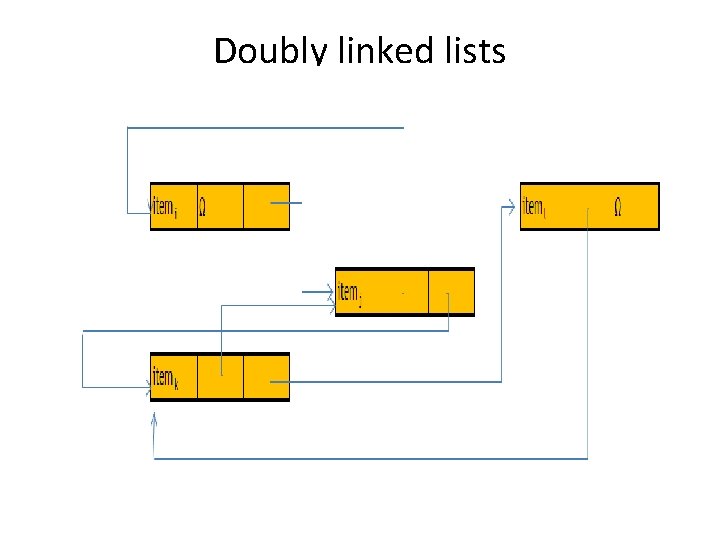
Doubly linked lists 6 -9 -15 cfy 51
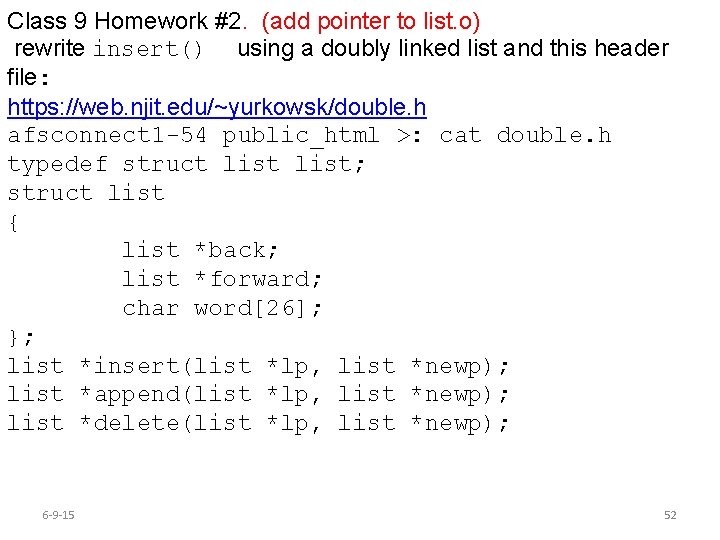
Class 9 Homework #2. (add pointer to list. o) rewrite insert() using a doubly linked list and this header file: https: //web. njit. edu/~yurkowsk/double. h afsconnect 1 -54 public_html >: cat double. h typedef struct list; struct list { list *back; list *forward; char word[26]; }; list *insert(list *lp, list *newp); list *append(list *lp, list *newp); list *delete(list *lp, list *newp); 6 -9 -15 52
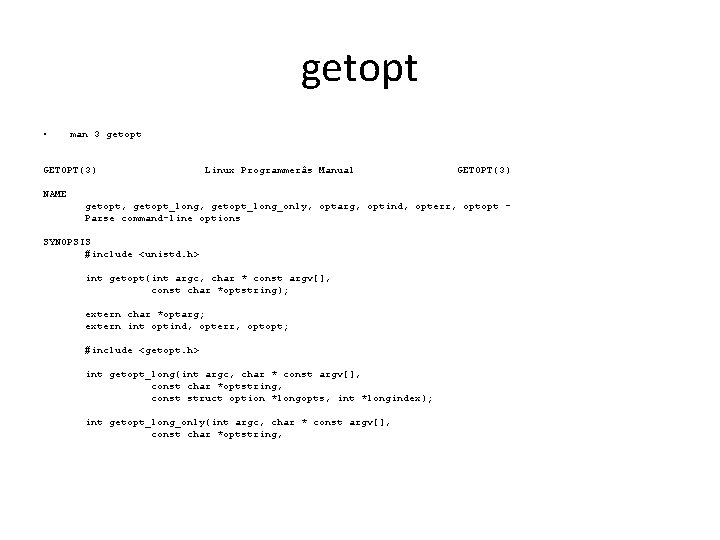
getopt • man 3 getopt GETOPT(3) Linux Programmerâs Manual GETOPT(3) NAME getopt, getopt_long_only, optarg, optind, opterr, optopt Parse command-line options SYNOPSIS #include <unistd. h> int getopt(int argc, char * const argv[], const char *optstring); extern char *optarg; extern int optind, opterr, optopt; #include <getopt. h> int getopt_long(int argc, char * const argv[], const char *optstring, const struct option *longopts, int *longindex); int getopt_long_only(int argc, char * const argv[], const char *optstring,
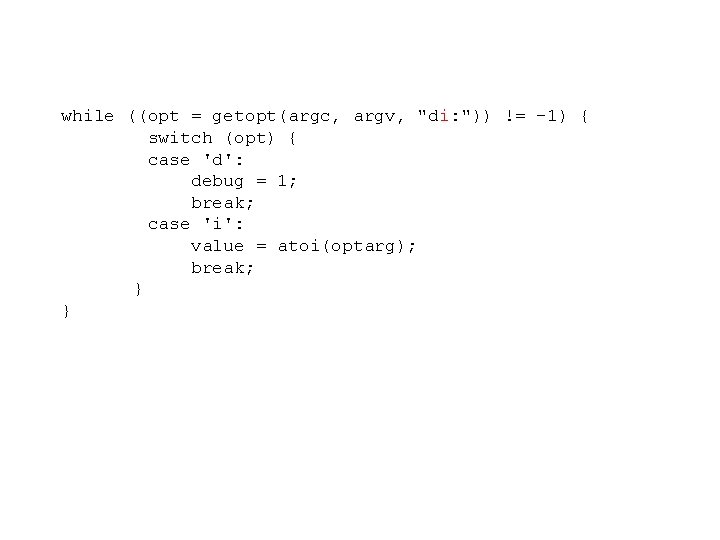
while ((opt = getopt(argc, argv, "di: ")) != -1) { switch (opt) { case 'd': debug = 1; break; case 'i': value = atoi(optarg); break; } }
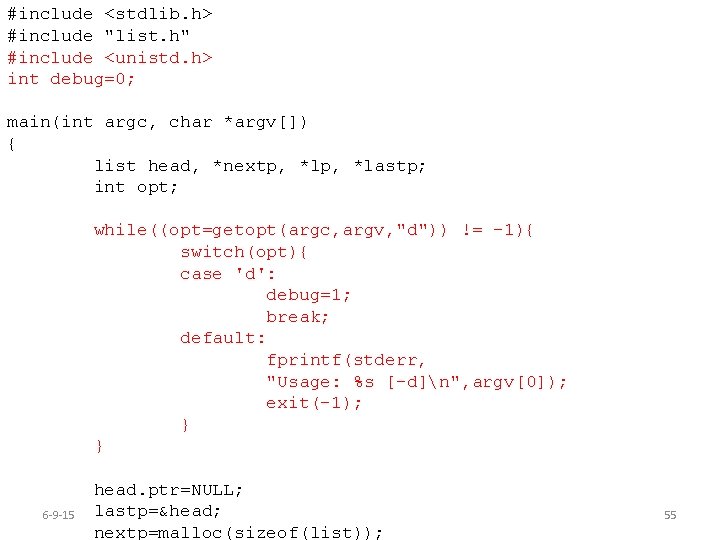
#include <stdlib. h> #include "list. h" #include <unistd. h> int debug=0; main(int argc, char *argv[]) { list head, *nextp, *lastp; int opt; while((opt=getopt(argc, argv, "d")) != -1){ switch(opt){ case 'd': debug=1; break; default: fprintf(stderr, "Usage: %s [-d]n", argv[0]); exit(-1); } } 6 -9 -15 head. ptr=NULL; lastp=&head; nextp=malloc(sizeof(list)); 55
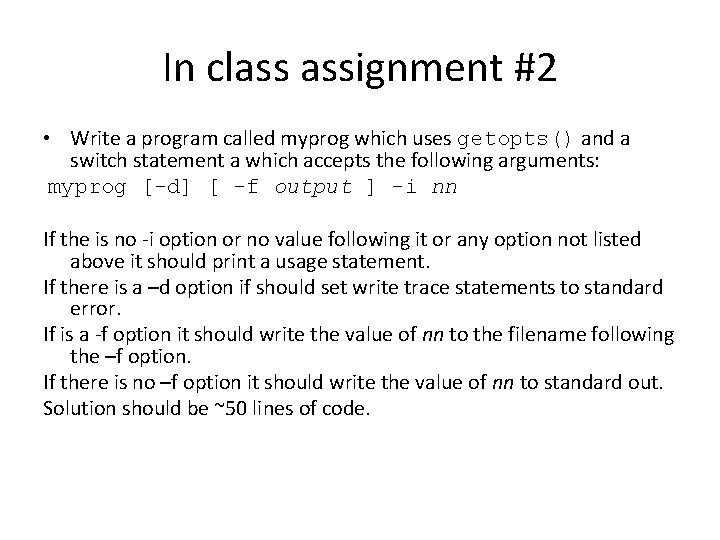
In class assignment #2 • Write a program called myprog which uses getopts() and a switch statement a which accepts the following arguments: myprog [-d] [ -f output ] -i nn If the is no -i option or no value following it or any option not listed above it should print a usage statement. If there is a –d option if should set write trace statements to standard error. If is a -f option it should write the value of nn to the filename following the –f option. If there is no –f option it should write the value of nn to standard out. Solution should be ~50 lines of code.
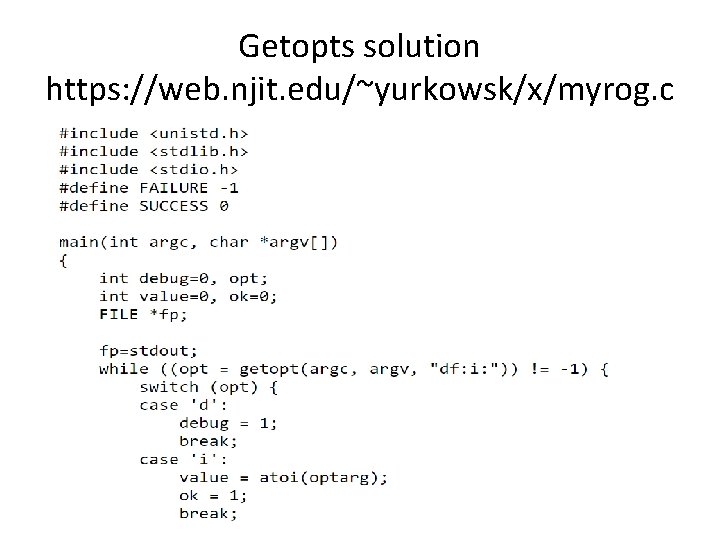
Getopts solution https: //web. njit. edu/~yurkowsk/x/myrog. c
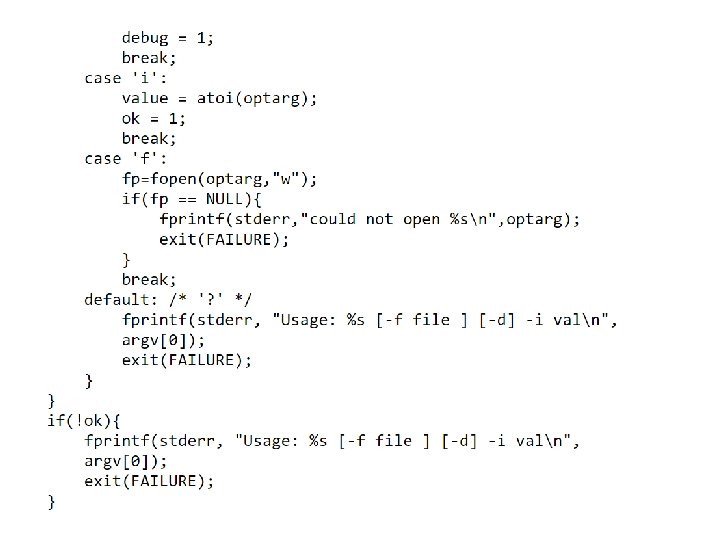
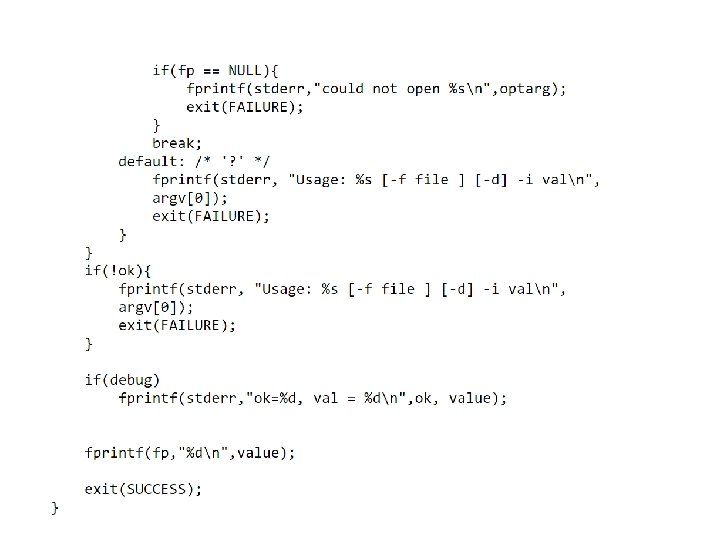
Emily dickinson poem 288
Movint client
Cs 288 njit
Rumah syair
Datacont
Cs 288 njit
Cs288
P 288
Cpre 288
Uclinux
General security
Low level programming language
Linux gui programming
Linux kernel hacking
Definisi integer
Greedy algorithm vs dynamic programming
Perbedaan linear programming dan integer programming
What is system program
Integer programming vs linear programming
Instructor vs teacher
Medical terminology instructor
Nrp instructor toolkit
Tp 12863
Basic instructor course texas
Basic instructor course texas
Instructor office hours
Tcole advanced instructor course
Naismith was an instructor of
Ac61-98 plan of action
Cisco certified trainer
Tipos de participantes y sus características
Basic instructor course #1014
Utp cable
Instructor operating station
Pepperball launcher nomenclature
Jrotc marksmanship instructor course online
Please clean your room
Tcole 1014 basic instructor course
Mptc firearms instructor
Drawing and painting the virtual instructor
Tcole instructor course
Tcole basic instructor course
Cbrf wisconsin registry
Catia instructor
Not only the students but also the instructor
Which line is longer illusion
Njrotc instructor vacancies
Basic instructor course texas
Nfpa 1403 instructor to student ratio
Basic instructor course texas
Delmar cengage learning instructor resources
Nra certified instructor logo
Tcole advanced instructor course
Instructor
Is a a pronoun
Yahya shehabi
Difference between reflexive and intensive