CS 1101 Group 1 Discussion 1 Lek Hsiang
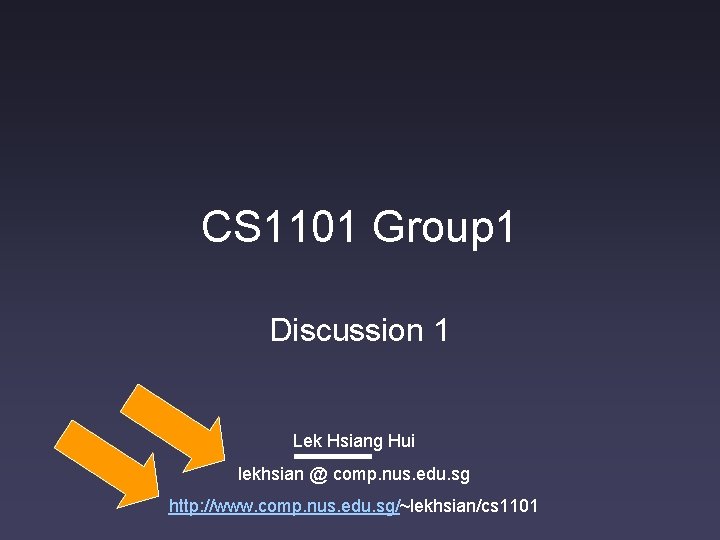
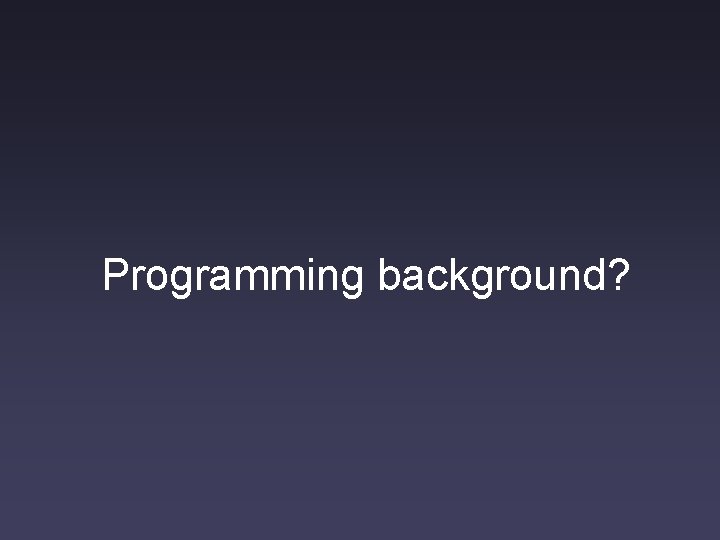
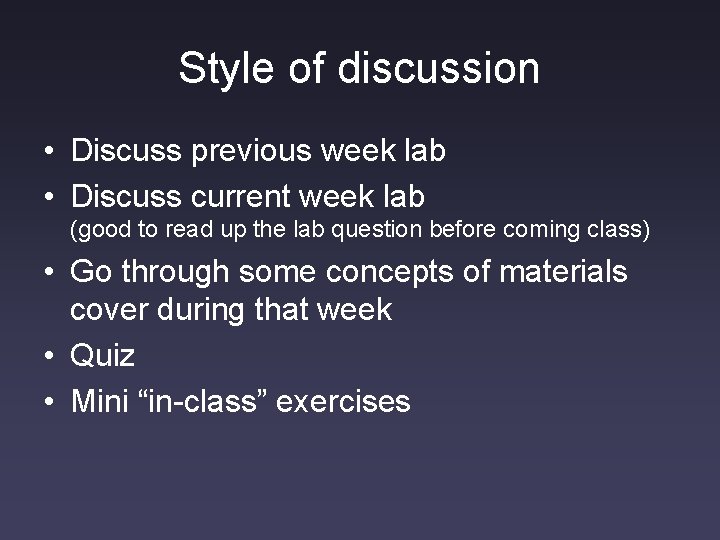
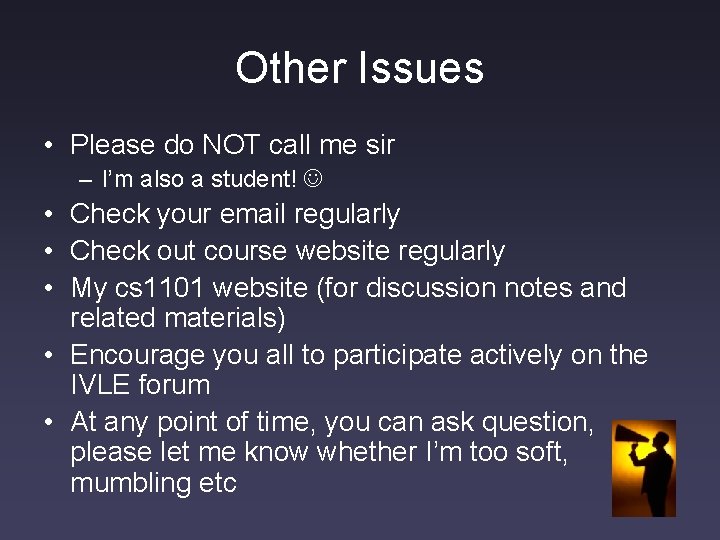
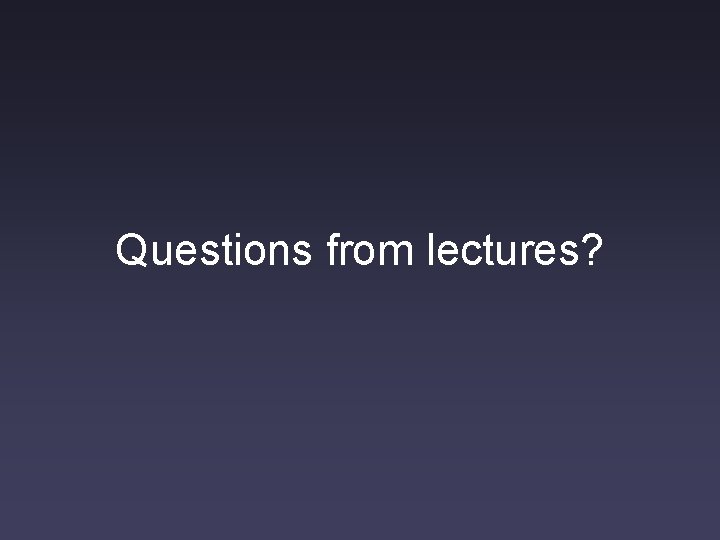
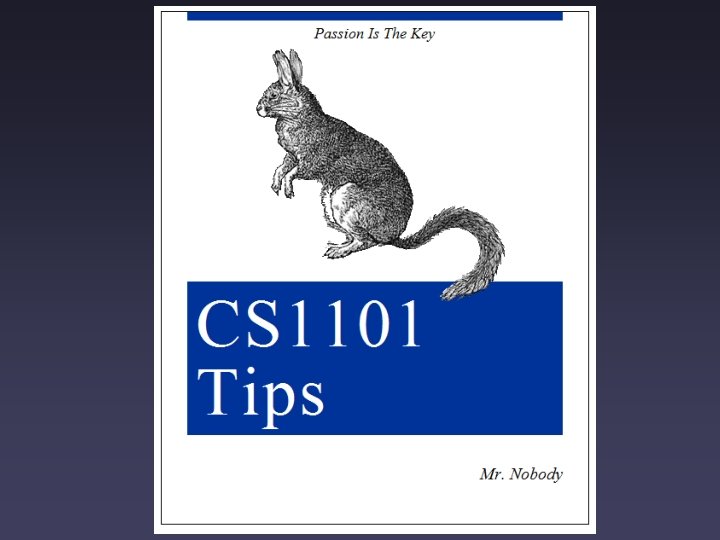
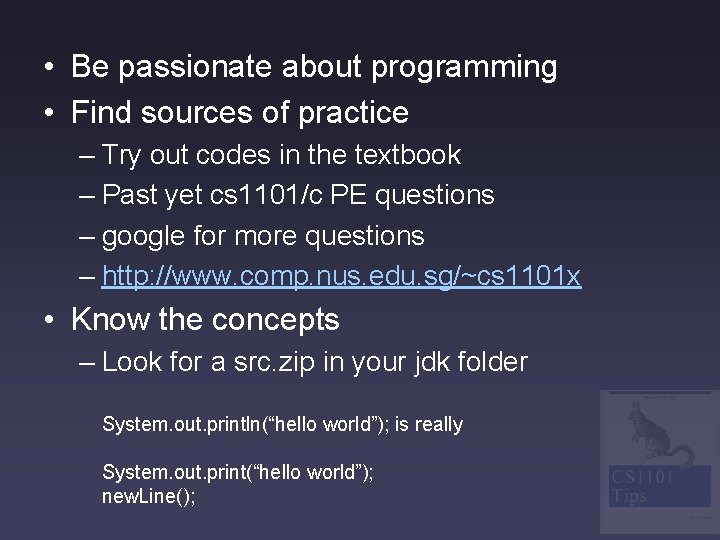
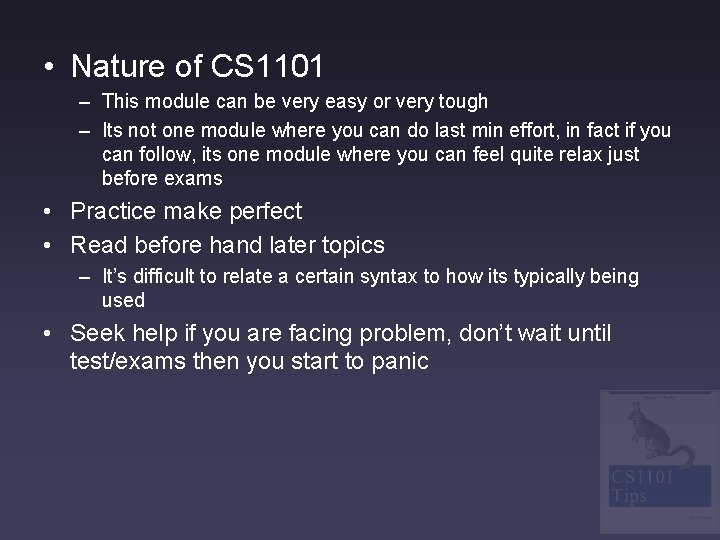
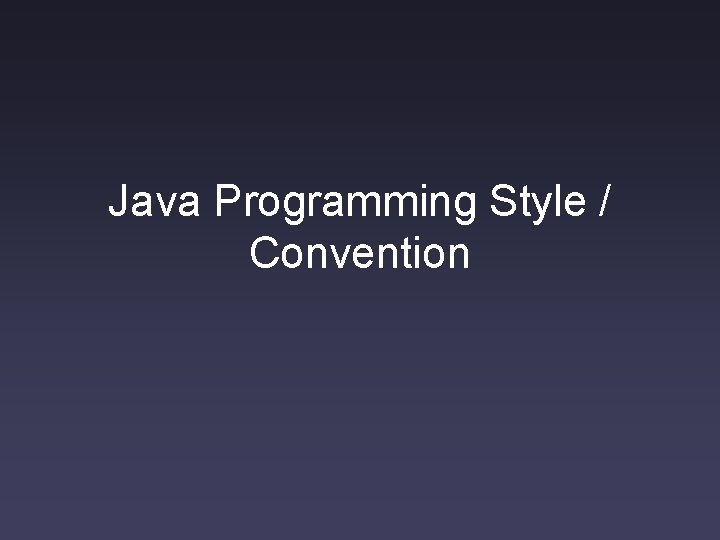
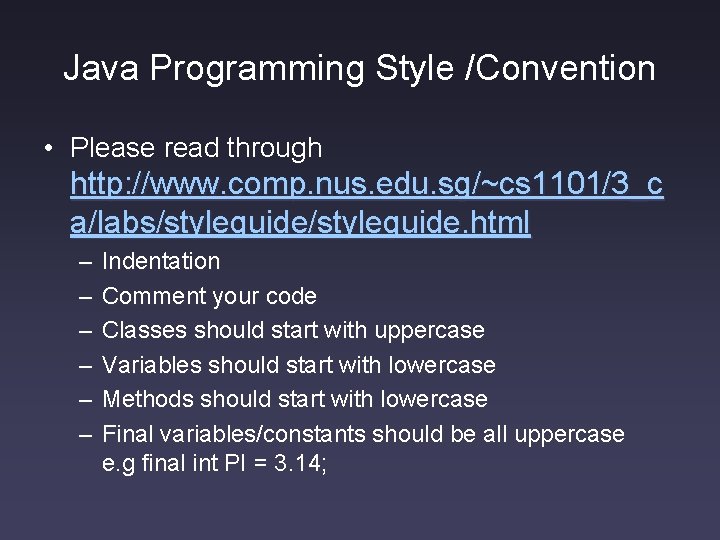
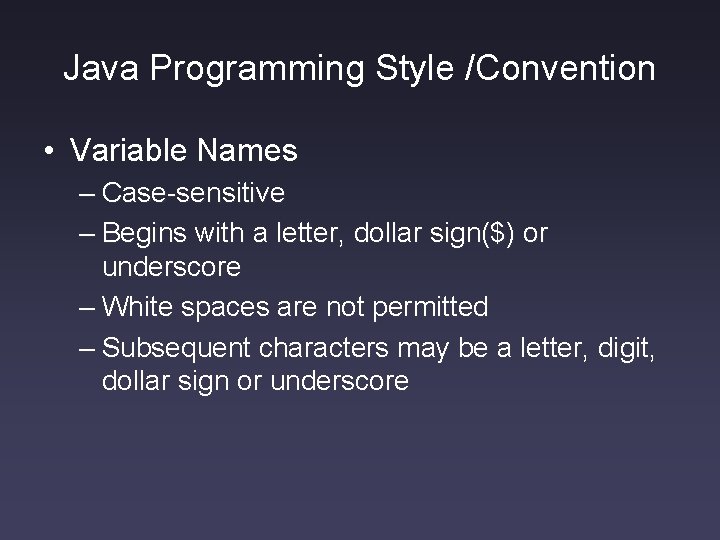
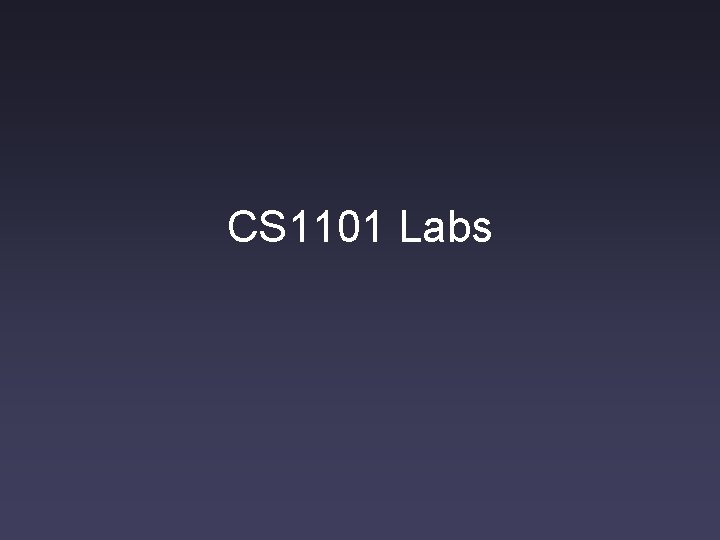
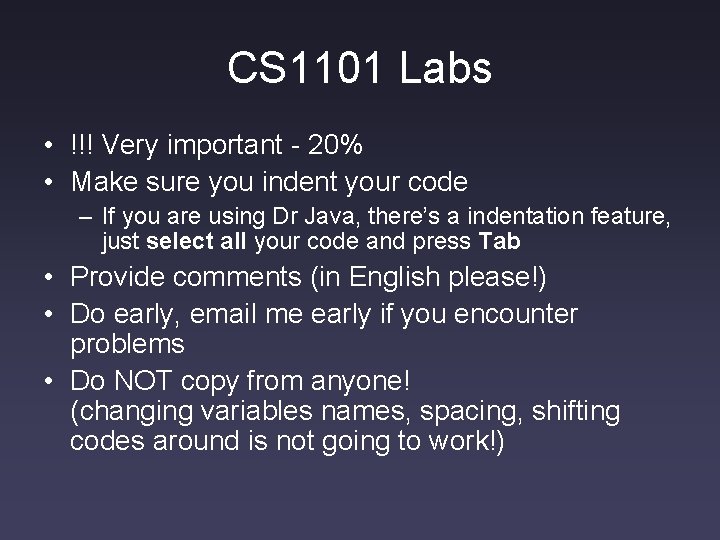
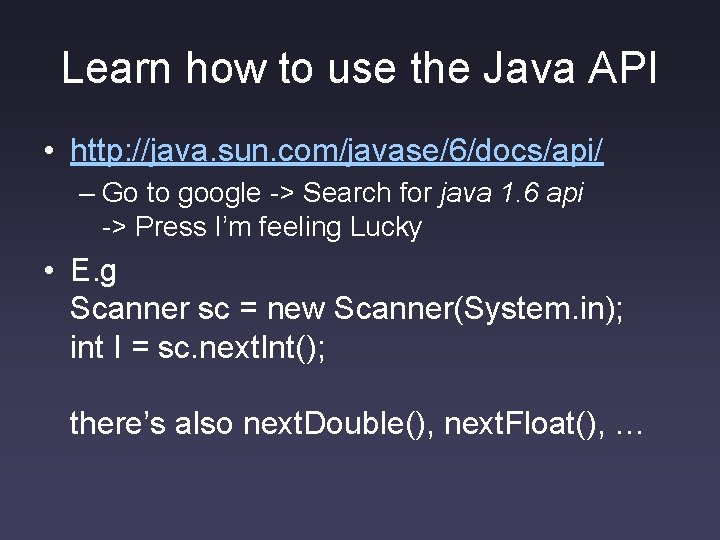
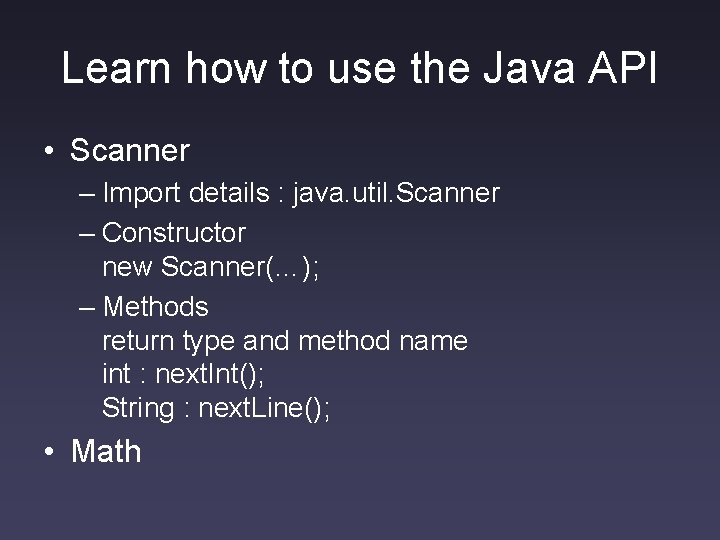
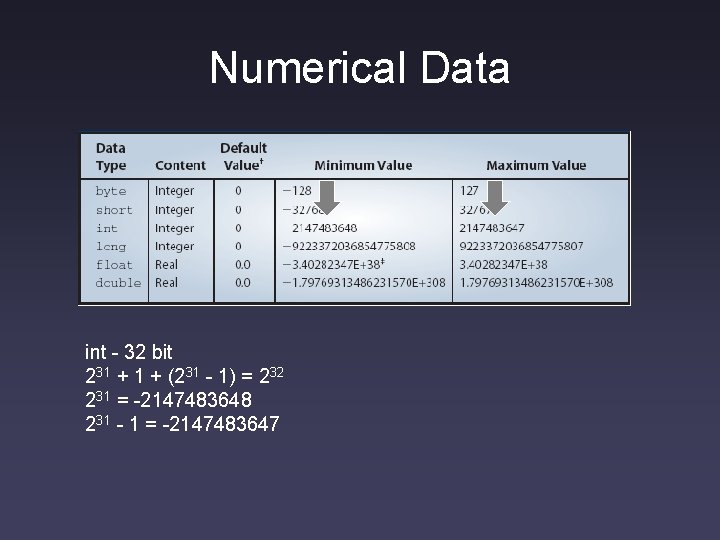
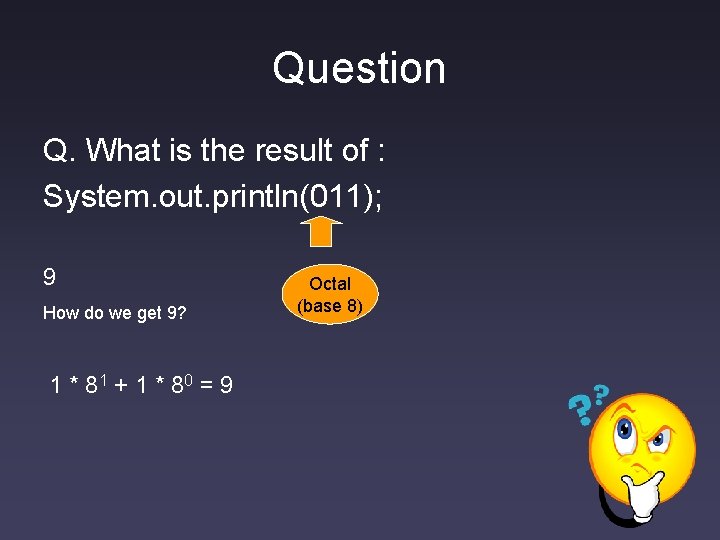
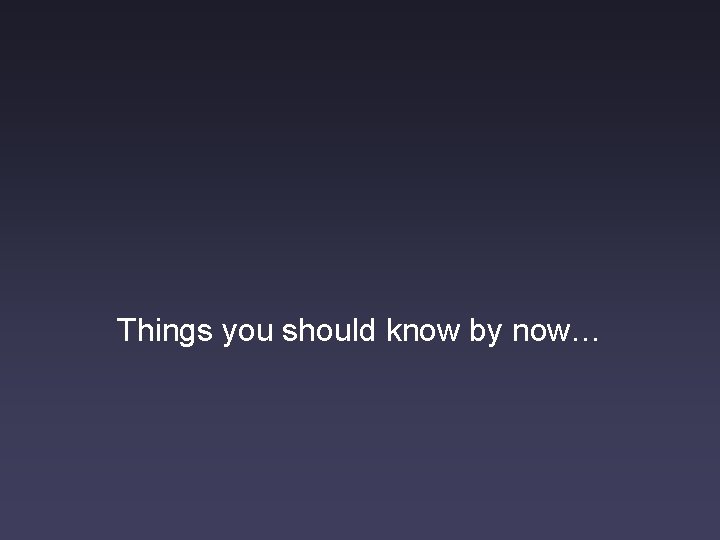
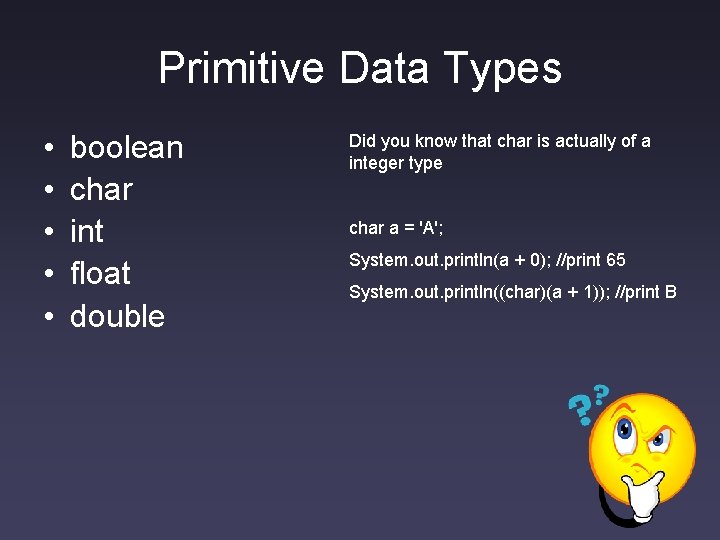
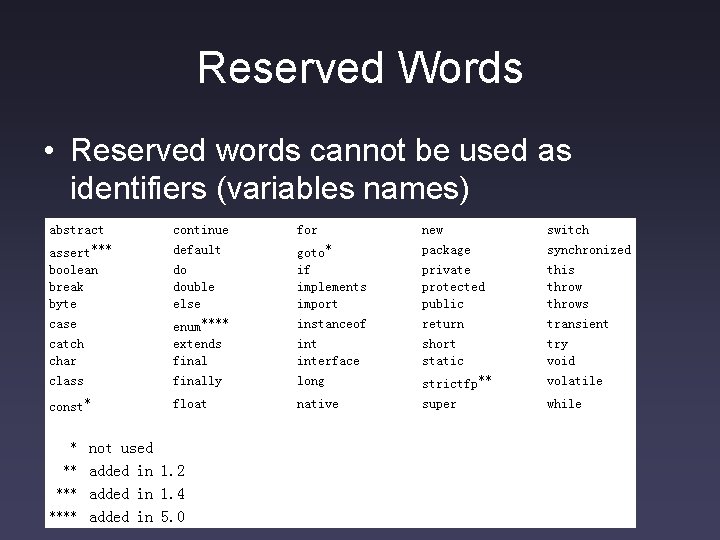
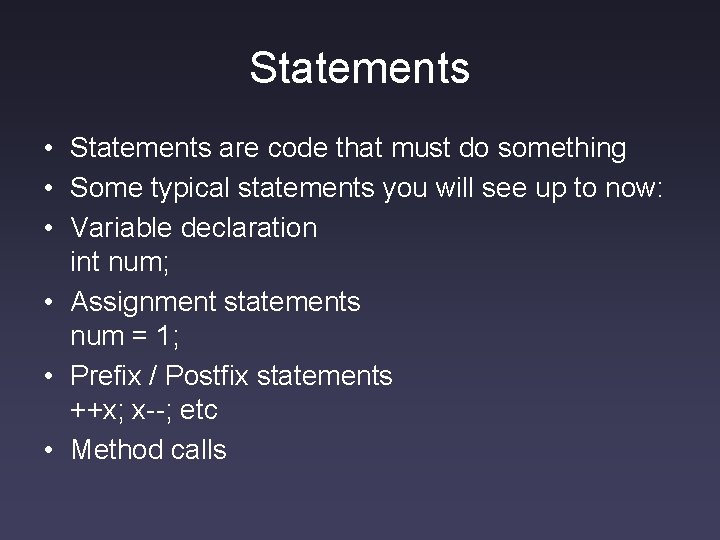
![Statements • Java also allow you to define blocks public static void main(String args[]){ Statements • Java also allow you to define blocks public static void main(String args[]){](https://slidetodoc.com/presentation_image/4adac3a28e0a877142cedb35e1d69cbf/image-22.jpg)
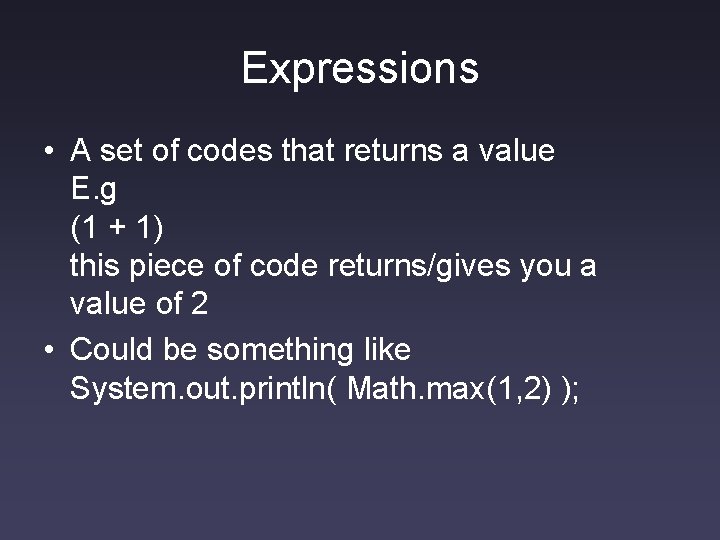
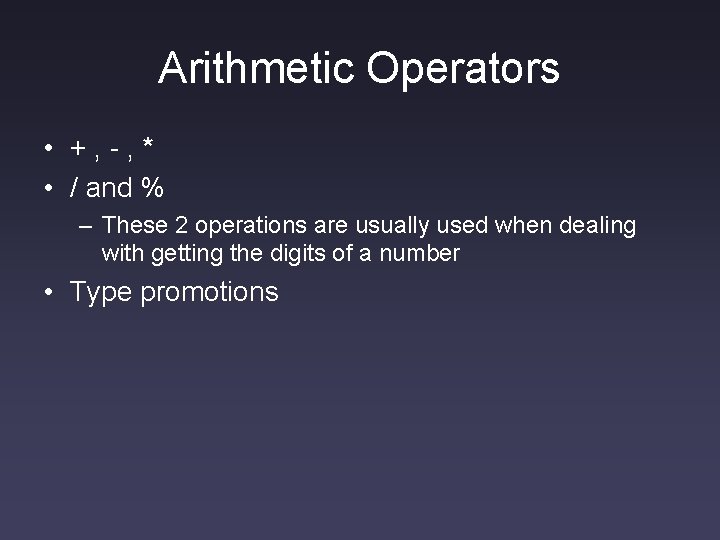
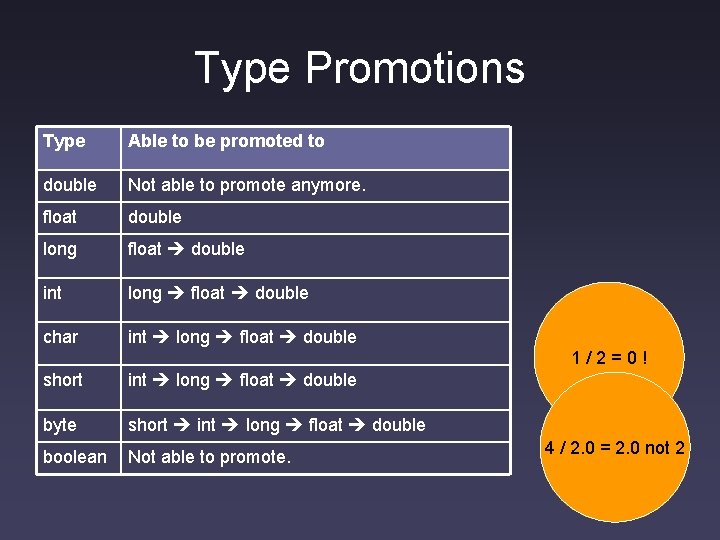
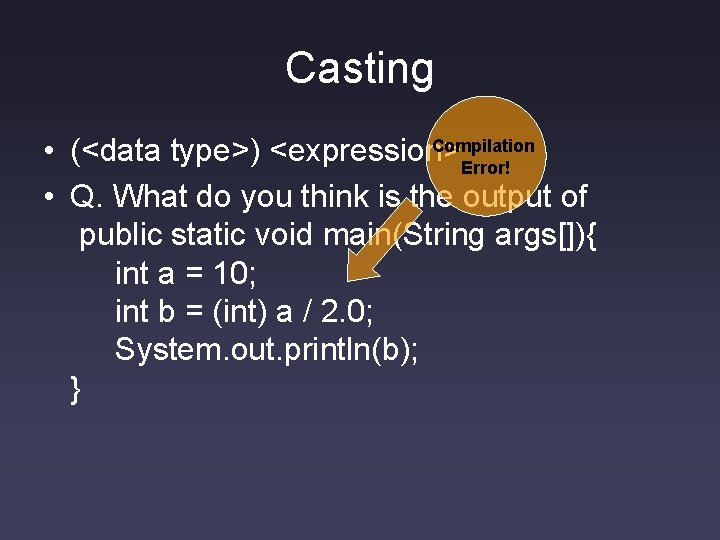
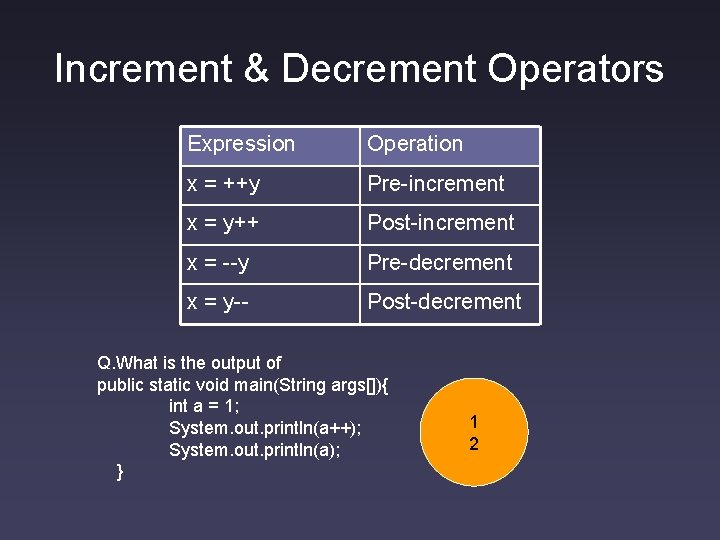
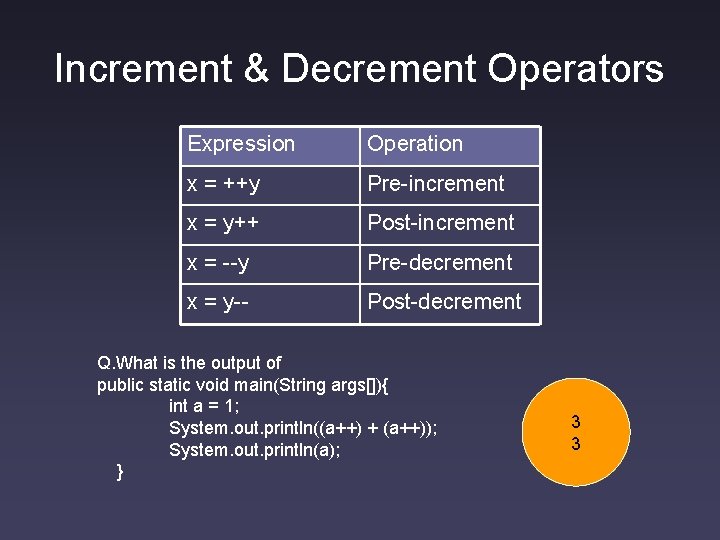
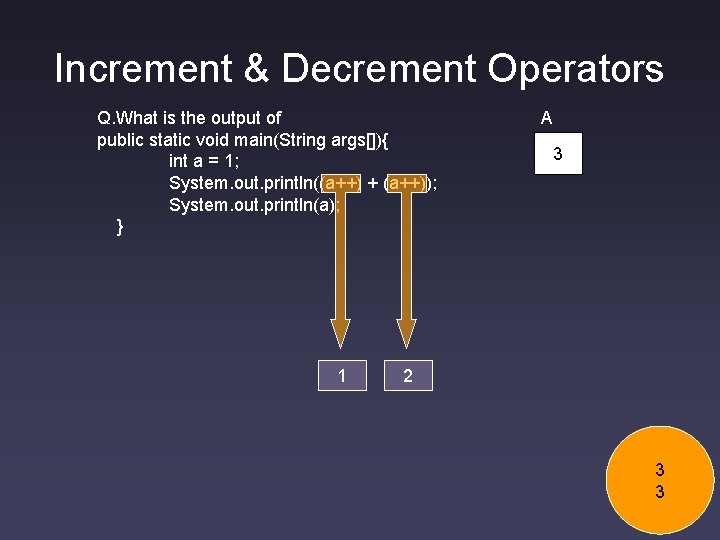
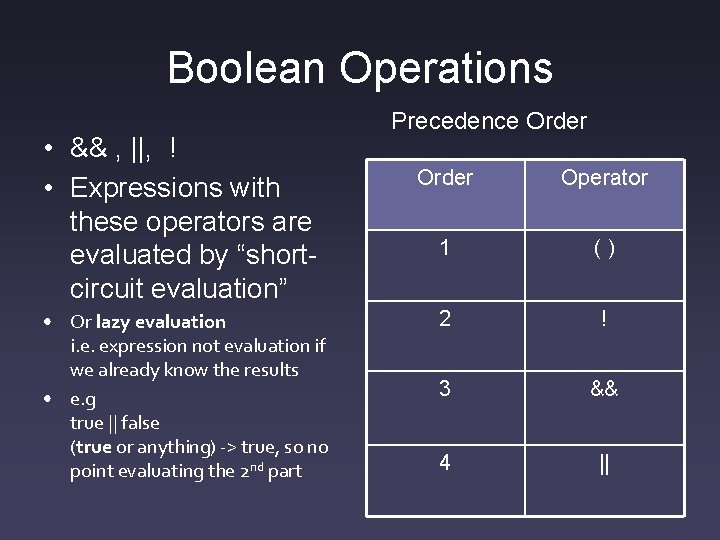
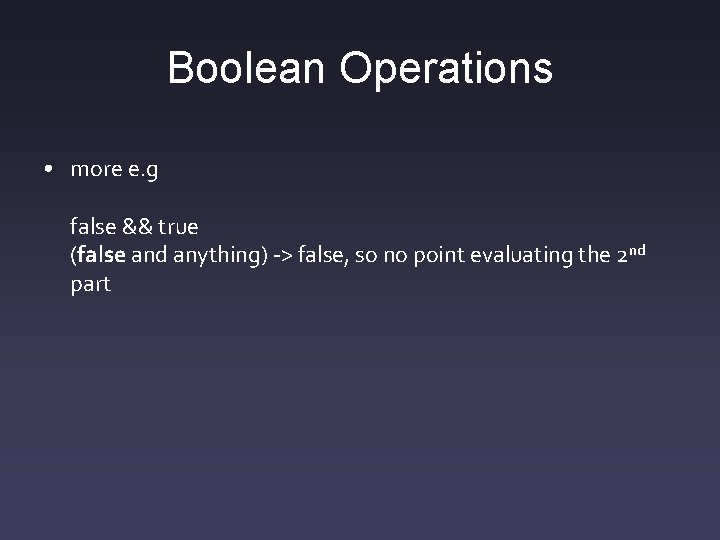
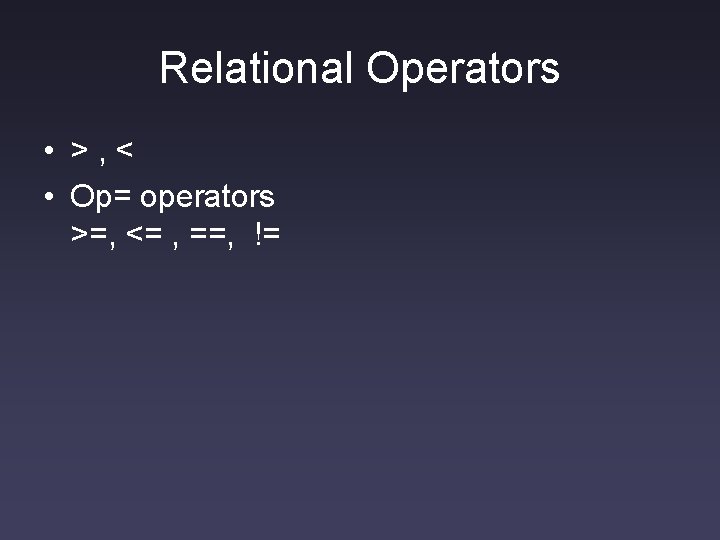
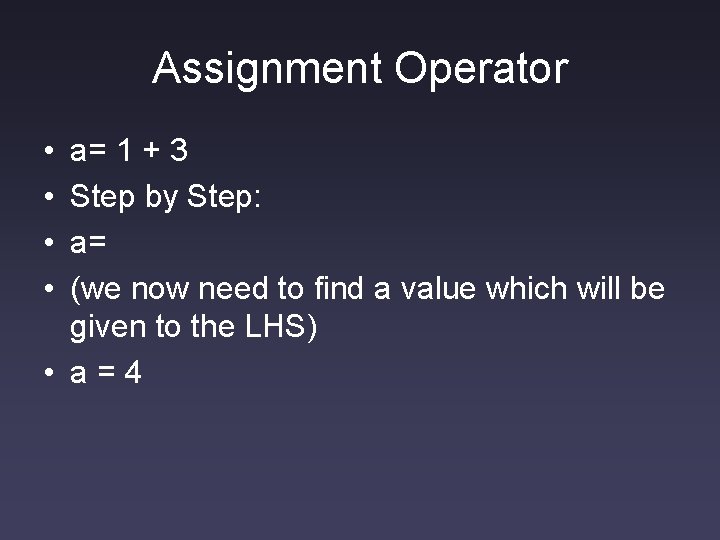
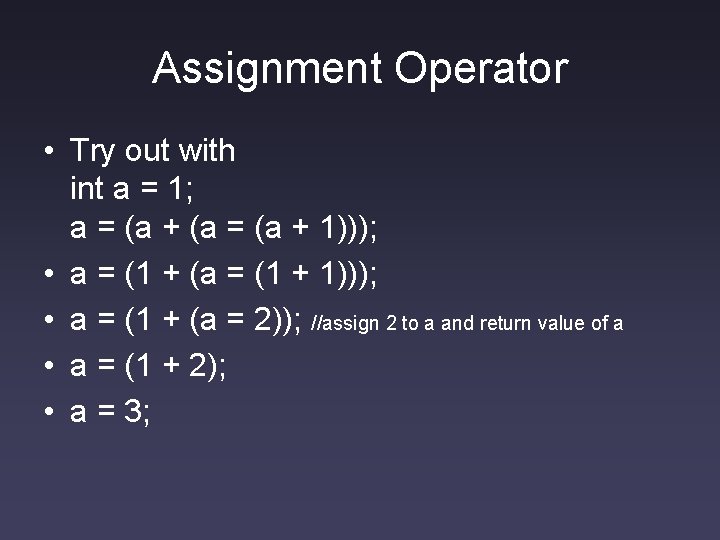
![Precedence and Associativity Operator Type Operator Precedence Level Associativity Unary []. (params) E++ E-- Precedence and Associativity Operator Type Operator Precedence Level Associativity Unary []. (params) E++ E--](https://slidetodoc.com/presentation_image/4adac3a28e0a877142cedb35e1d69cbf/image-35.jpg)
- Slides: 35
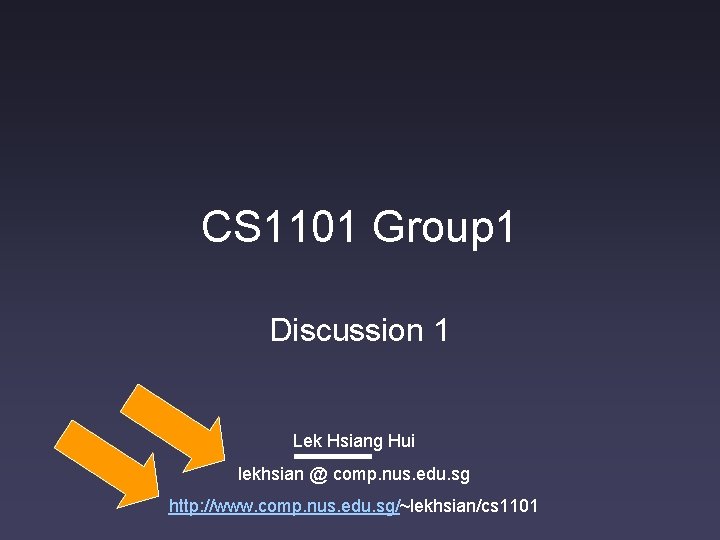
CS 1101 Group 1 Discussion 1 Lek Hsiang Hui lekhsian @ comp. nus. edu. sg http: //www. comp. nus. edu. sg/~lekhsian/cs 1101
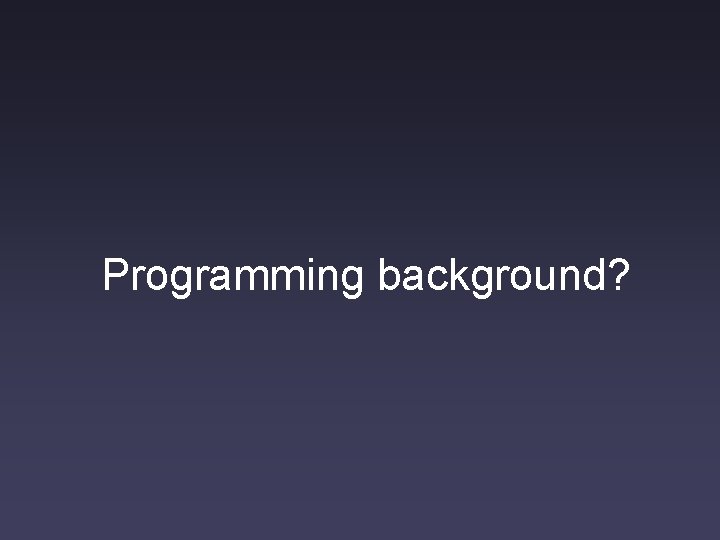
Programming background?
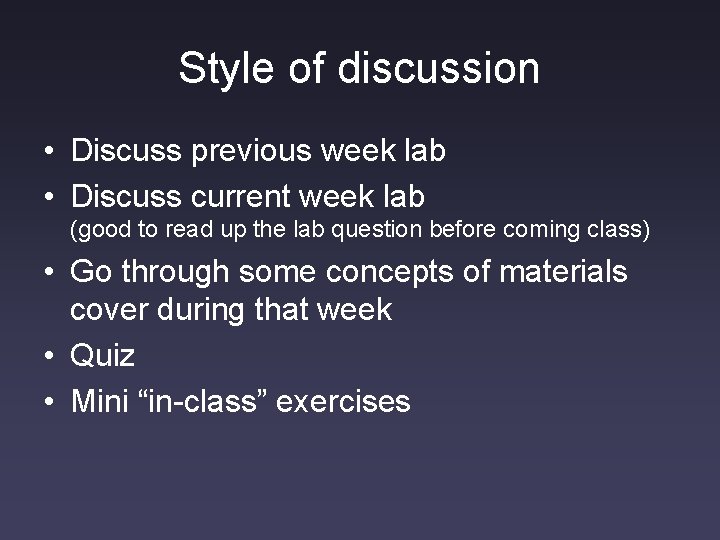
Style of discussion • Discuss previous week lab • Discuss current week lab (good to read up the lab question before coming class) • Go through some concepts of materials cover during that week • Quiz • Mini “in-class” exercises
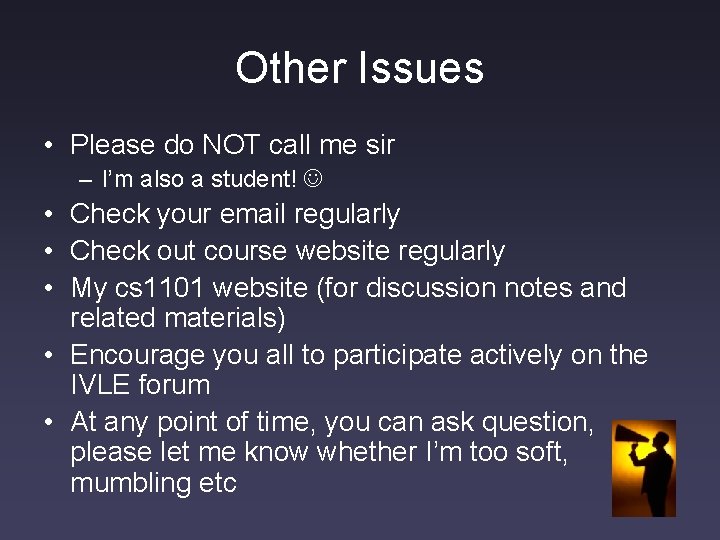
Other Issues • Please do NOT call me sir – I’m also a student! • Check your email regularly • Check out course website regularly • My cs 1101 website (for discussion notes and related materials) • Encourage you all to participate actively on the IVLE forum • At any point of time, you can ask question, please let me know whether I’m too soft, mumbling etc
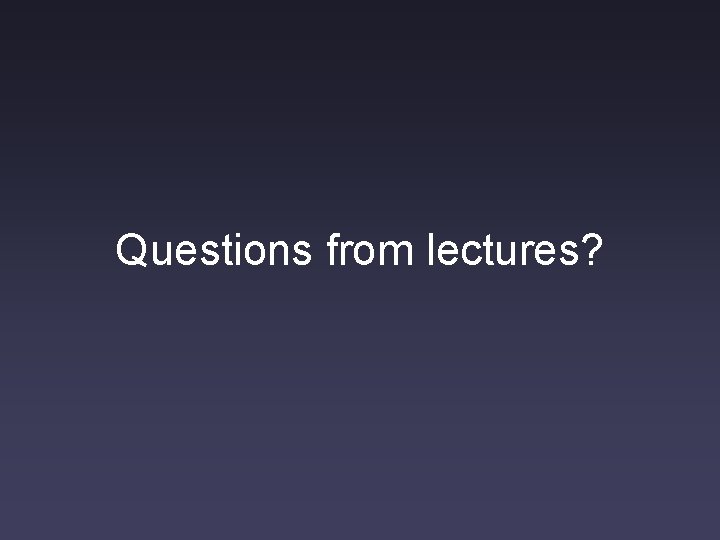
Questions from lectures?
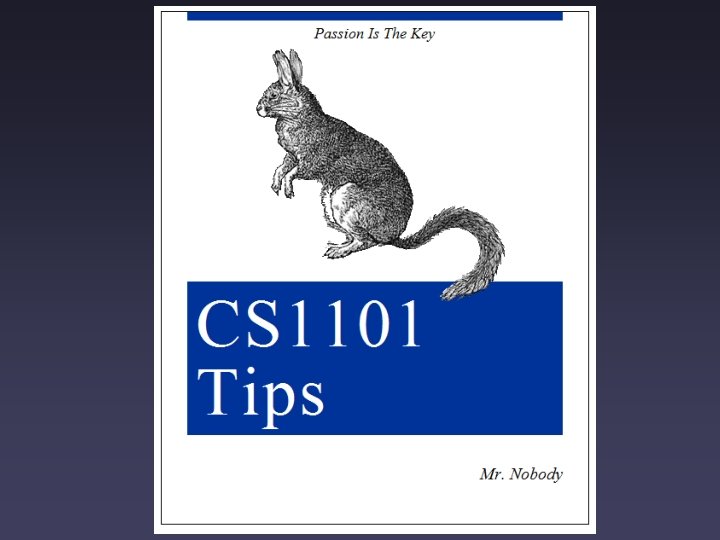
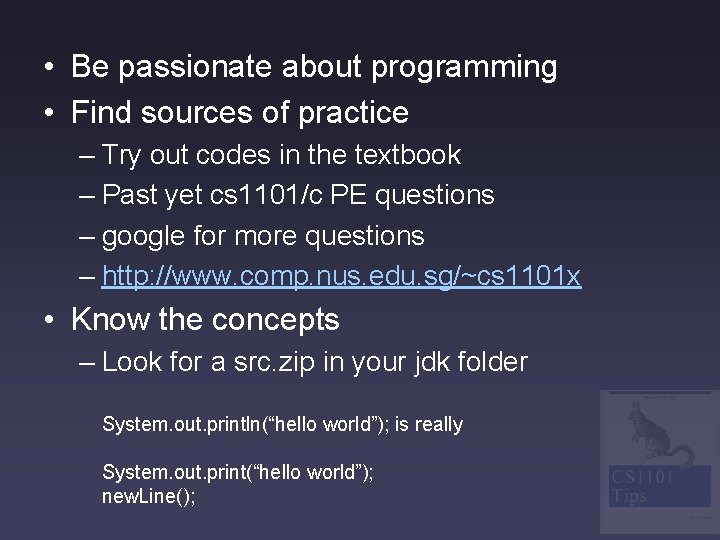
• Be passionate about programming • Find sources of practice – Try out codes in the textbook – Past yet cs 1101/c PE questions – google for more questions – http: //www. comp. nus. edu. sg/~cs 1101 x • Know the concepts – Look for a src. zip in your jdk folder System. out. println(“hello world”); is really System. out. print(“hello world”); new. Line();
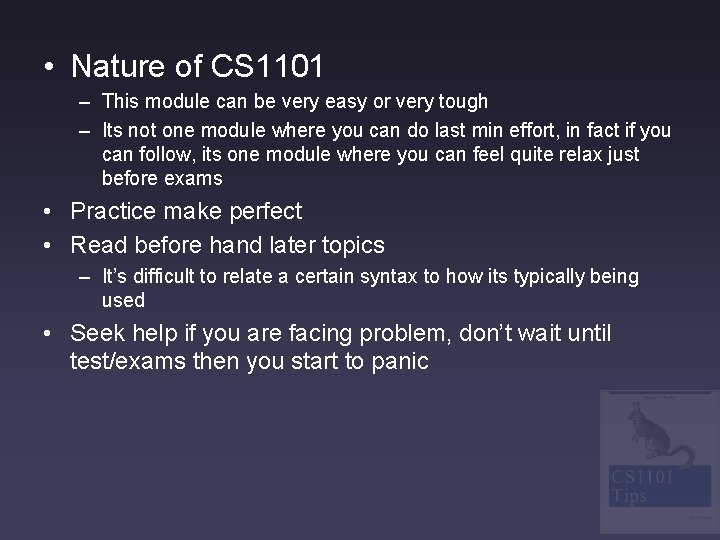
• Nature of CS 1101 – This module can be very easy or very tough – Its not one module where you can do last min effort, in fact if you can follow, its one module where you can feel quite relax just before exams • Practice make perfect • Read before hand later topics – It’s difficult to relate a certain syntax to how its typically being used • Seek help if you are facing problem, don’t wait until test/exams then you start to panic
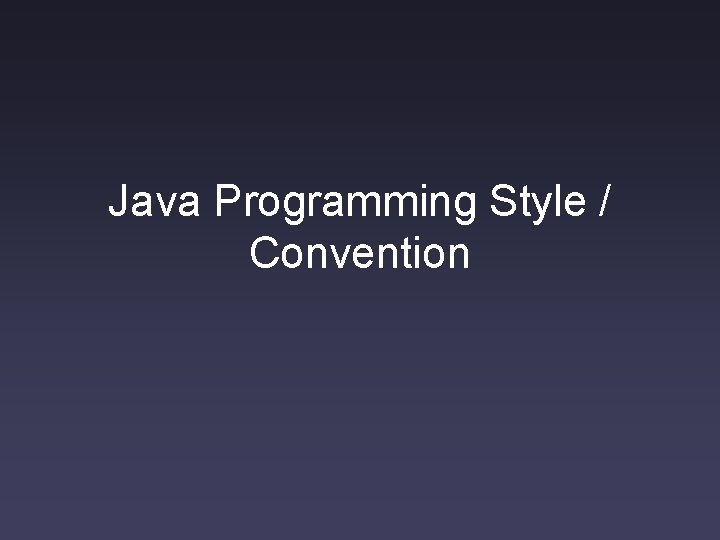
Java Programming Style / Convention
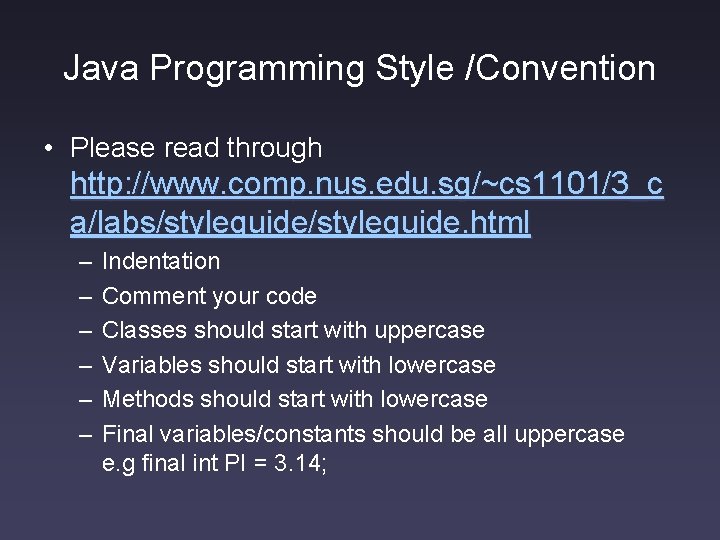
Java Programming Style /Convention • Please read through http: //www. comp. nus. edu. sg/~cs 1101/3_c a/labs/styleguide. html – – – Indentation Comment your code Classes should start with uppercase Variables should start with lowercase Methods should start with lowercase Final variables/constants should be all uppercase e. g final int PI = 3. 14;
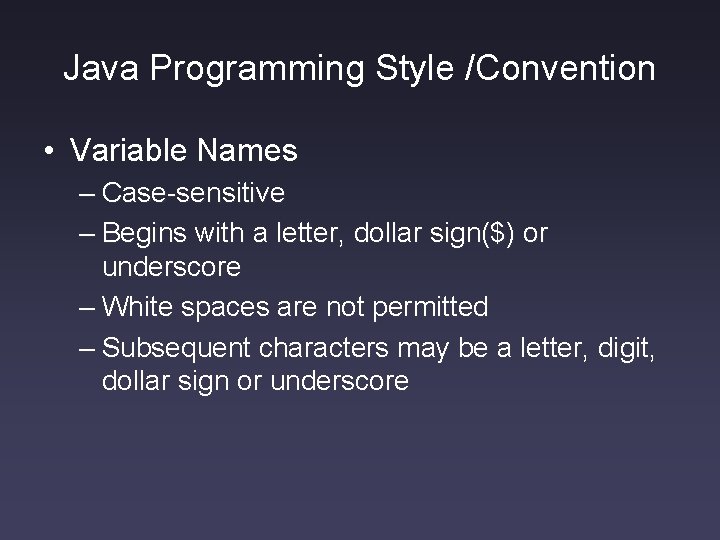
Java Programming Style /Convention • Variable Names – Case-sensitive – Begins with a letter, dollar sign($) or underscore – White spaces are not permitted – Subsequent characters may be a letter, digit, dollar sign or underscore
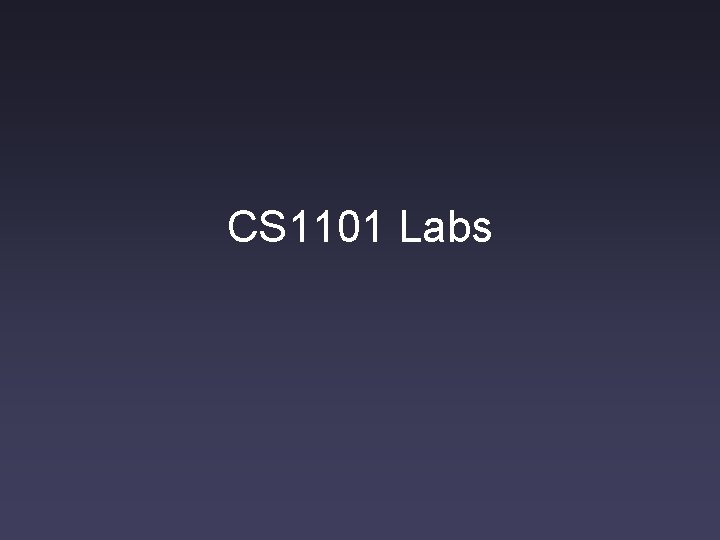
CS 1101 Labs
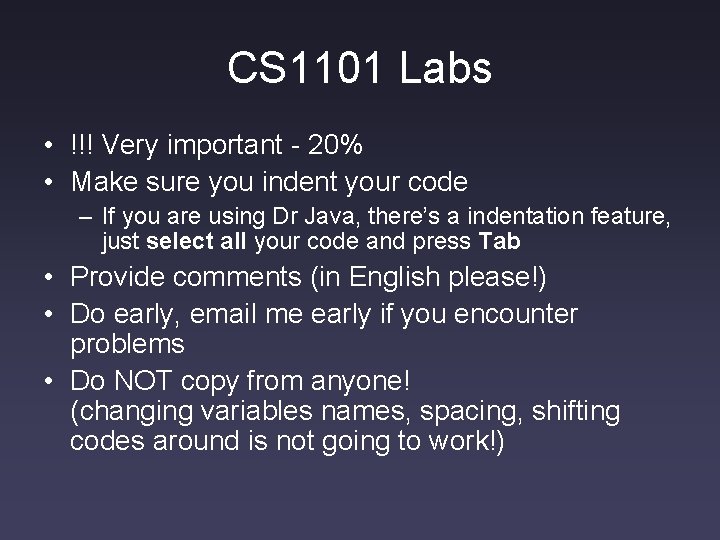
CS 1101 Labs • !!! Very important - 20% • Make sure you indent your code – If you are using Dr Java, there’s a indentation feature, just select all your code and press Tab • Provide comments (in English please!) • Do early, email me early if you encounter problems • Do NOT copy from anyone! (changing variables names, spacing, shifting codes around is not going to work!)
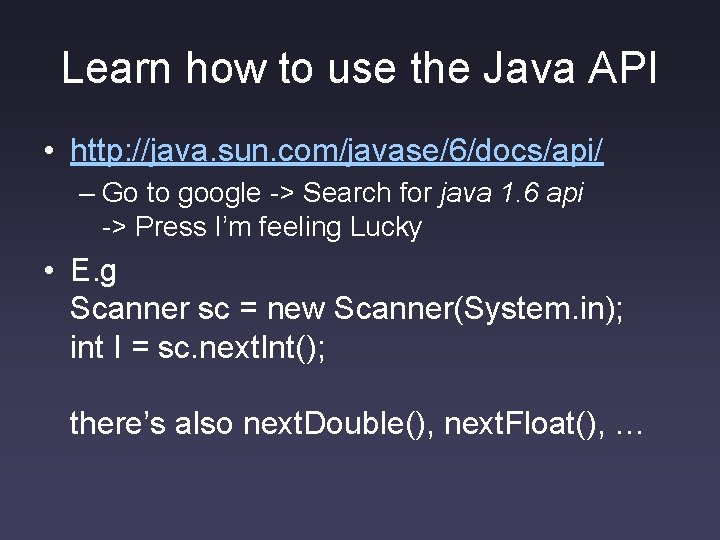
Learn how to use the Java API • http: //java. sun. com/javase/6/docs/api/ – Go to google -> Search for java 1. 6 api -> Press I’m feeling Lucky • E. g Scanner sc = new Scanner(System. in); int I = sc. next. Int(); there’s also next. Double(), next. Float(), …
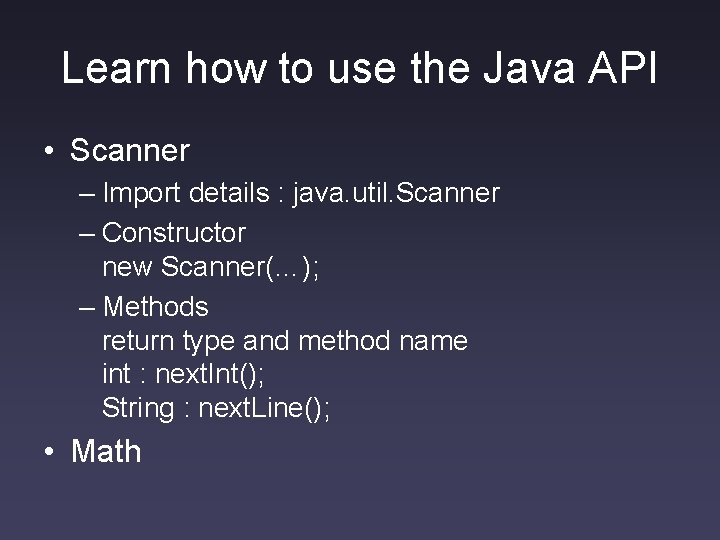
Learn how to use the Java API • Scanner – Import details : java. util. Scanner – Constructor new Scanner(…); – Methods return type and method name int : next. Int(); String : next. Line(); • Math
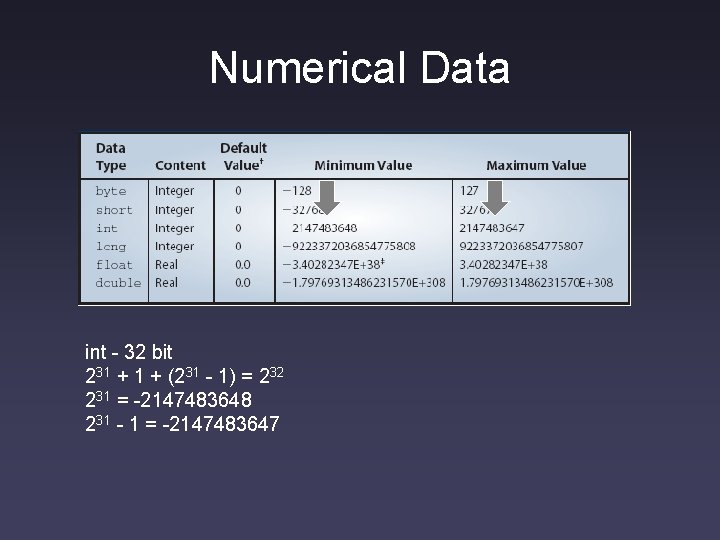
Numerical Data int - 32 bit 231 + (231 - 1) = 232 231 = -2147483648 231 - 1 = -2147483647
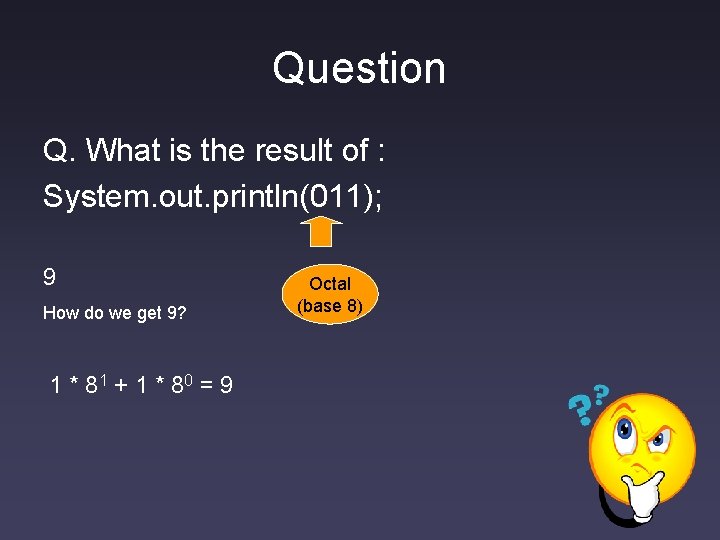
Question Q. What is the result of : System. out. println(011); 9 How do we get 9? 1 * 81 + 1 * 8 0 = 9 Octal (base 8)
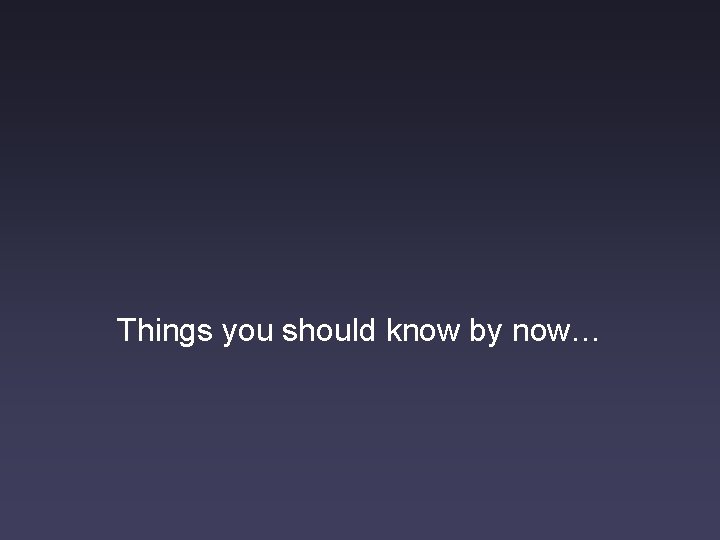
Things you should know by now…
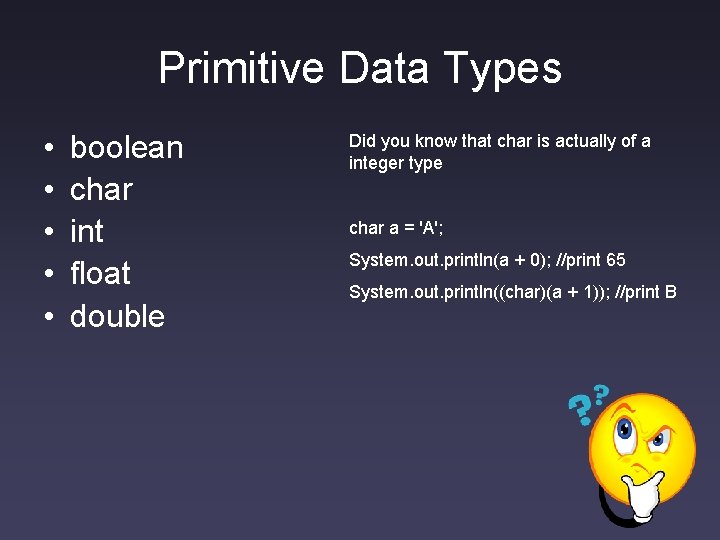
Primitive Data Types • • • boolean char int float double Did you know that char is actually of a integer type char a = 'A'; System. out. println(a + 0); //print 65 System. out. println((char)(a + 1)); //print B
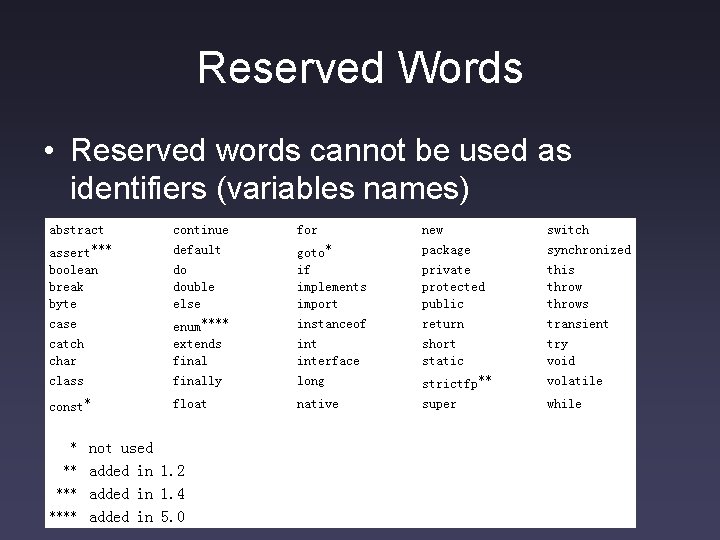
Reserved Words • Reserved words cannot be used as identifiers (variables names)
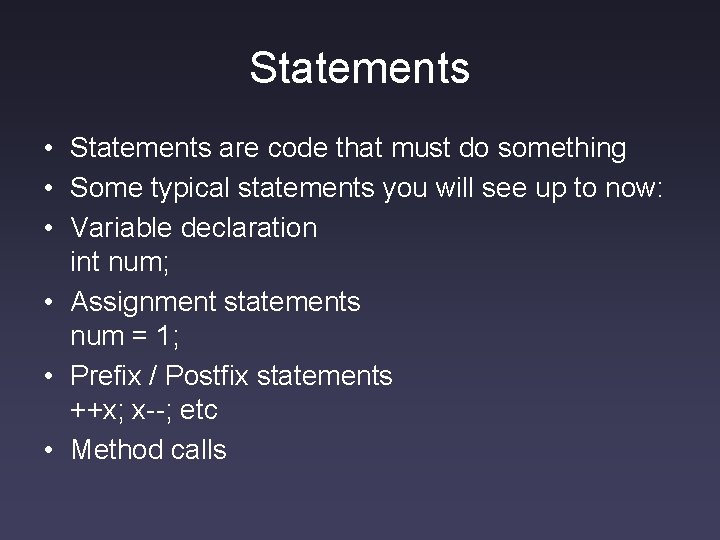
Statements • Statements are code that must do something • Some typical statements you will see up to now: • Variable declaration int num; • Assignment statements num = 1; • Prefix / Postfix statements ++x; x--; etc • Method calls
![Statements Java also allow you to define blocks public static void mainString args Statements • Java also allow you to define blocks public static void main(String args[]){](https://slidetodoc.com/presentation_image/4adac3a28e0a877142cedb35e1d69cbf/image-22.jpg)
Statements • Java also allow you to define blocks public static void main(String args[]){ { int num = 1; System. out. println(num); } int num = 2; System. out. println(num); } prints 1 2
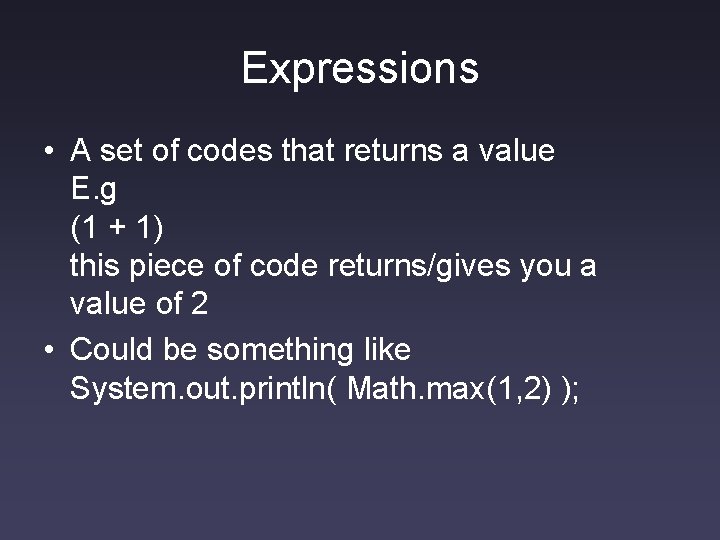
Expressions • A set of codes that returns a value E. g (1 + 1) this piece of code returns/gives you a value of 2 • Could be something like System. out. println( Math. max(1, 2) );
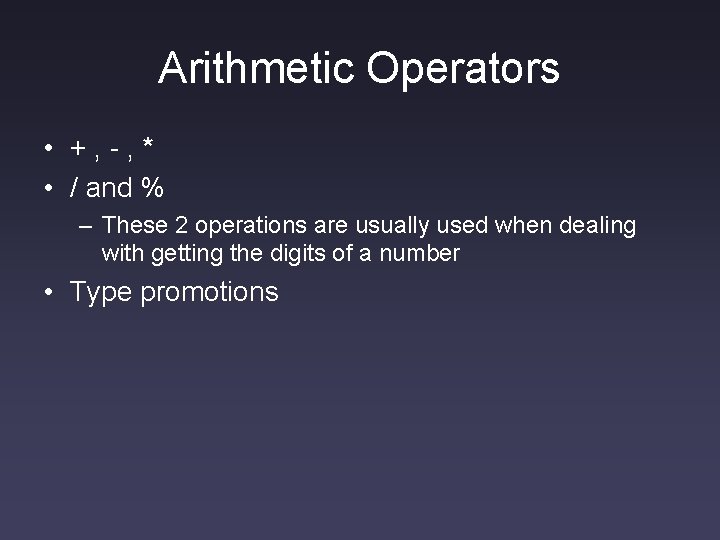
Arithmetic Operators • +, -, * • / and % – These 2 operations are usually used when dealing with getting the digits of a number • Type promotions
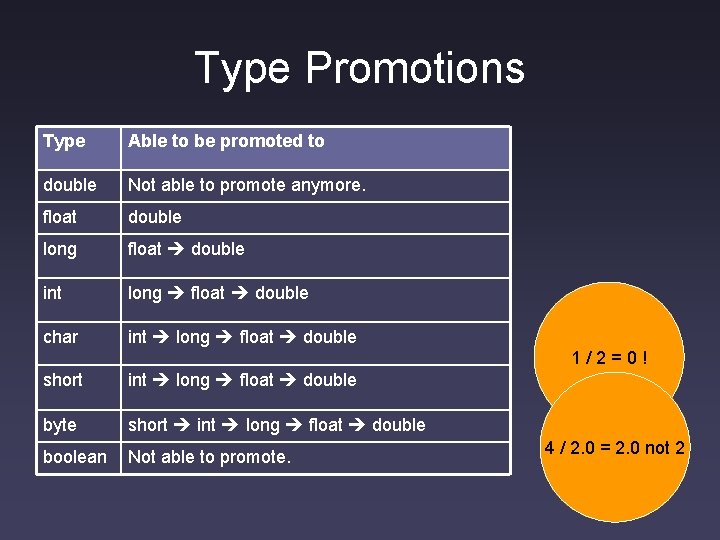
Type Promotions Type Able to be promoted to double Not able to promote anymore. float double long float double int long float double char int long float double 1/2=0! short int long float double byte short int long float double boolean Not able to promote. 4 / 2. 0 = 2. 0 not 2
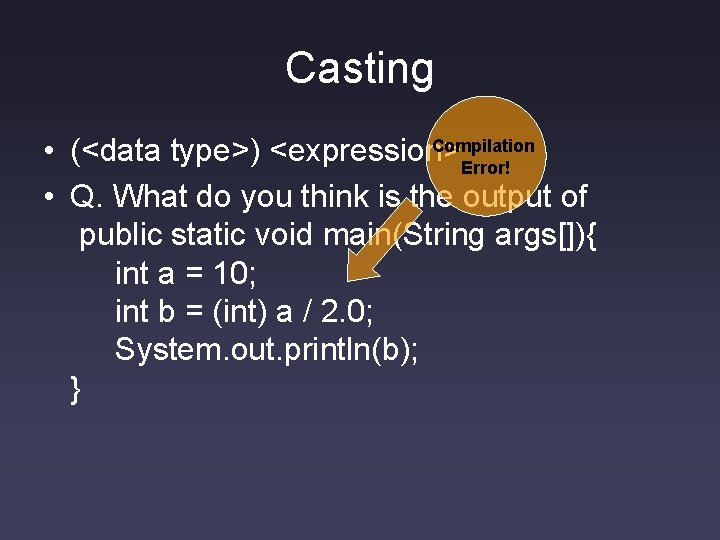
Casting Compilation • (<data type>) <expression> Error! • Q. What do you think is the output of public static void main(String args[]){ int a = 10; int b = (int) a / 2. 0; System. out. println(b); }
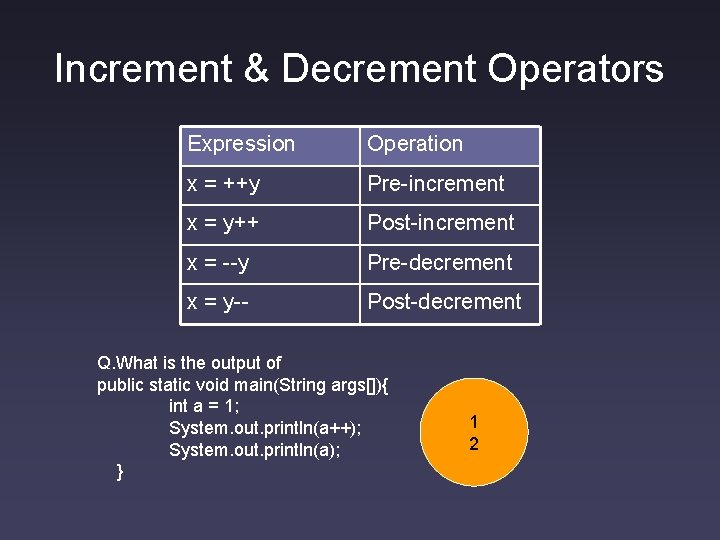
Increment & Decrement Operators Expression Operation x = ++y Pre-increment x = y++ Post-increment x = --y Pre-decrement x = y-- Post-decrement Q. What is the output of public static void main(String args[]){ int a = 1; System. out. println(a++); System. out. println(a); } 1 2
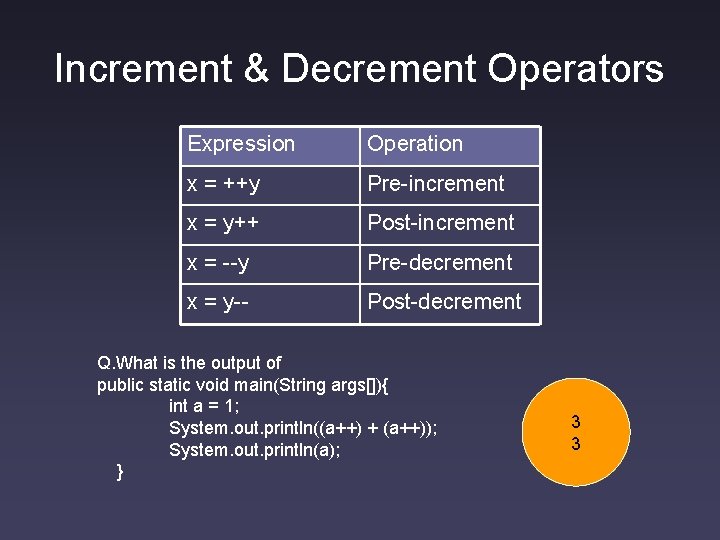
Increment & Decrement Operators Expression Operation x = ++y Pre-increment x = y++ Post-increment x = --y Pre-decrement x = y-- Post-decrement Q. What is the output of public static void main(String args[]){ int a = 1; System. out. println((a++) + (a++)); System. out. println(a); } 3 3
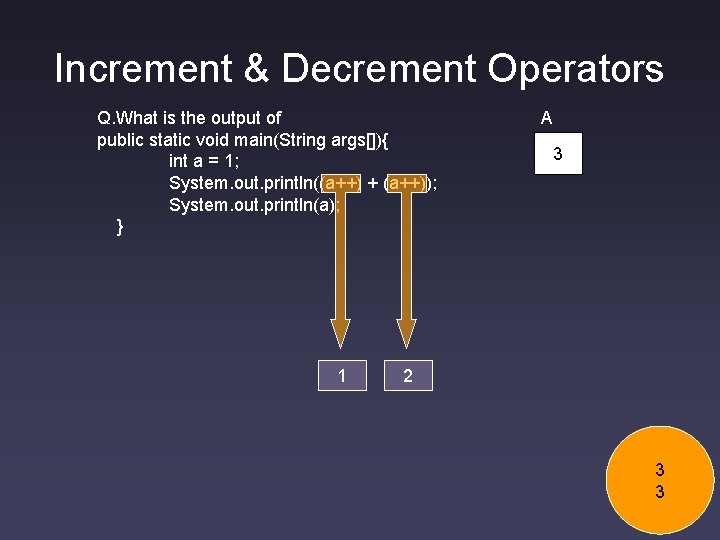
Increment & Decrement Operators Q. What is the output of public static void main(String args[]){ int a = 1; System. out. println((a++) + (a++)); System. out. println(a); } 1 A 2 1 3 2 3 3
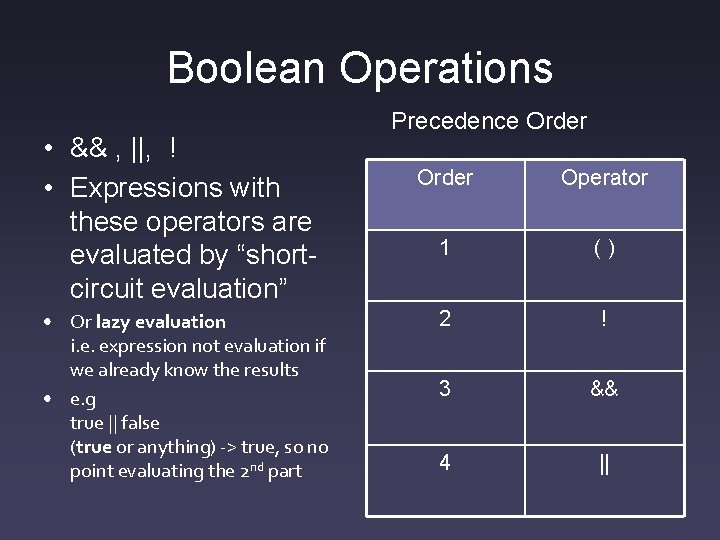
Boolean Operations • && , ||, ! • Expressions with these operators are evaluated by “shortcircuit evaluation” • Or lazy evaluation i. e. expression not evaluation if we already know the results • e. g true || false (true or anything) -> true, so no point evaluating the 2 nd part Precedence Order Operator 1 () 2 ! 3 && 4 ||
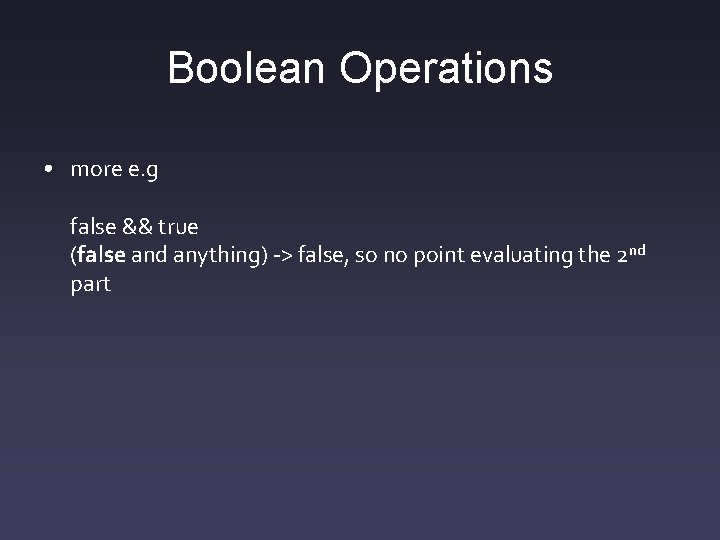
Boolean Operations • more e. g false && true (false and anything) -> false, so no point evaluating the 2 nd part
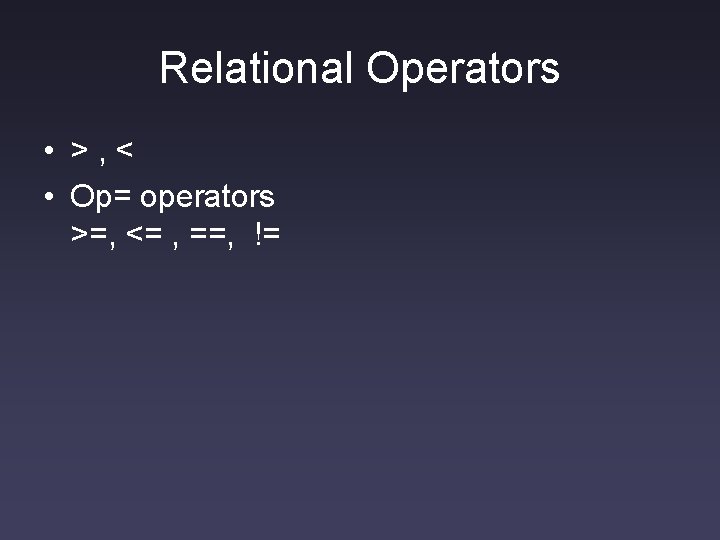
Relational Operators • >, < • Op= operators >=, <= , ==, !=
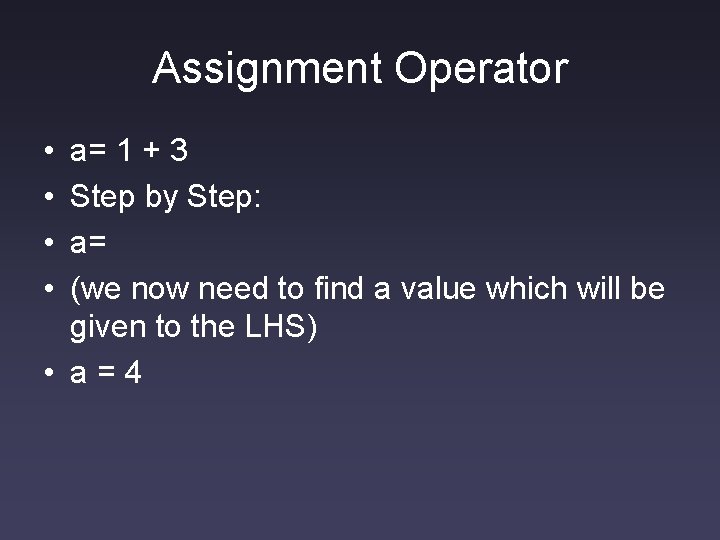
Assignment Operator • • a= 1 + 3 Step by Step: a= (we now need to find a value which will be given to the LHS) • a=4
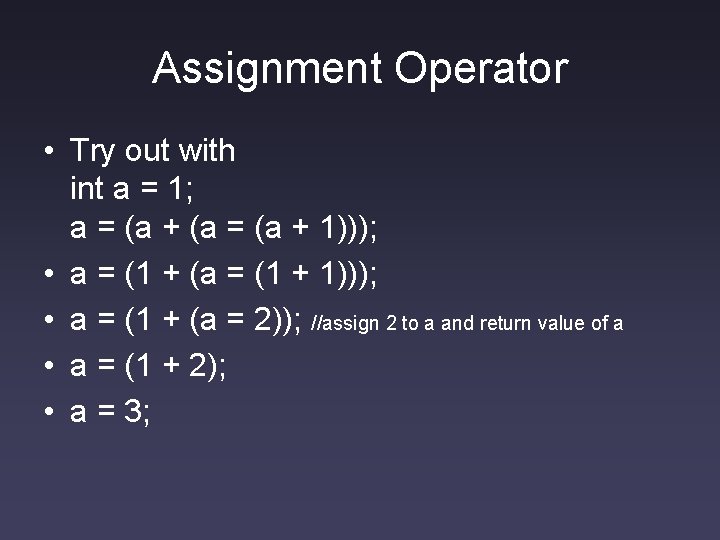
Assignment Operator • Try out with int a = 1; a = (a + (a = (a + 1))); • a = (1 + (a = (1 + 1))); • a = (1 + (a = 2)); //assign 2 to a and return value of a • a = (1 + 2); • a = 3;
![Precedence and Associativity Operator Type Operator Precedence Level Associativity Unary params E E Precedence and Associativity Operator Type Operator Precedence Level Associativity Unary []. (params) E++ E--](https://slidetodoc.com/presentation_image/4adac3a28e0a877142cedb35e1d69cbf/image-35.jpg)
Precedence and Associativity Operator Type Operator Precedence Level Associativity Unary []. (params) E++ E-- 1 Left to right Unary unary operators: -E !E ~E ++E --E 2 Right to left Object creation / Cast new (type)E 3 Right to left Arithmetic */% 4 Left to right Arithmetic +- 5 Left to right Bitwise >> << >>> 6 Left to right Relational < > <= >= 7 Left to right Relational == != 8 Left to right Bitwise & 9 Left to right Bitwise ^ 10 Left to right Bitwise | 11 Left to right Logical && 12 Left to right Logical || 13 Left to right Conditional ? : 14 Right to left Assignment = += -= *= /= %= >>= <<= &= ^= |= 15 Right to left
Lek hsiang hui
Jennifer hsiang
Lu xun new year's sacrifice
Simple distillation introduction
Benefits of polyandry
Skala weissa
Playa thong kut
Asoprisnil lek
Anatol lek
Motivational intervieuwing
Tf kbt
Ovciji loj
Lewosimendan
Lek bloemenservice
Dys-lek-see-uh
Sistemas de apareamiento
Talizer lek
Co to znaczy ikarowy lot
Pravni lek znacenje
Instruments in thailand
Lek trombolityczny
Contergan lek
Parallell lek
Lek med geometriska former
Devolutivni pravni lijek
Litijum lek
Cs 1101 programming fundamentals
Zastępcze elementy geometryczne
Cst 1101
Sls 1101
Bio 1101
Cs 1101 final
Cs 1101 programming fundamentals
Enc 1101 final exam
1010 1101
Dr ben leong