Compiling vs Interpreting We write source code that
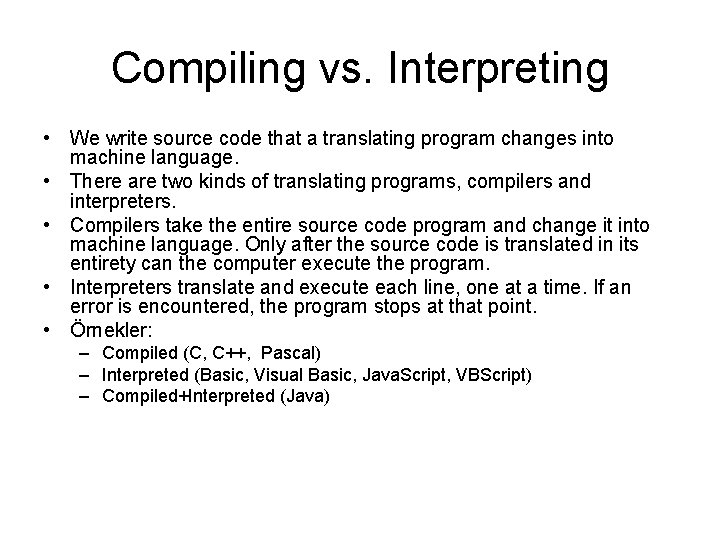
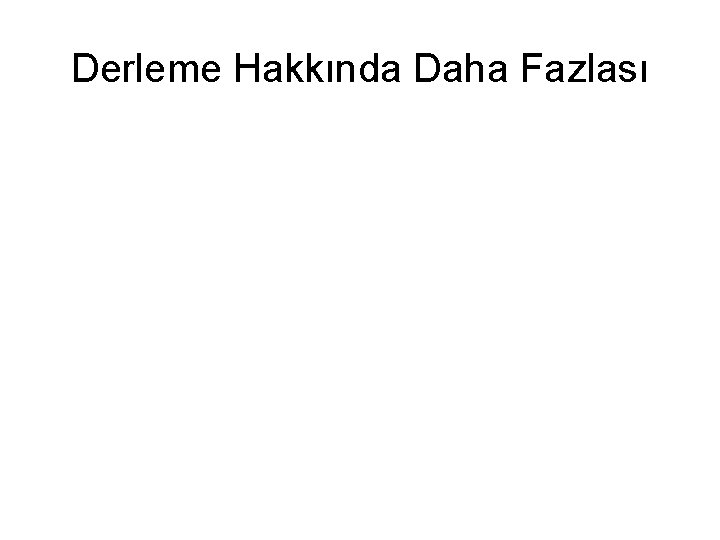
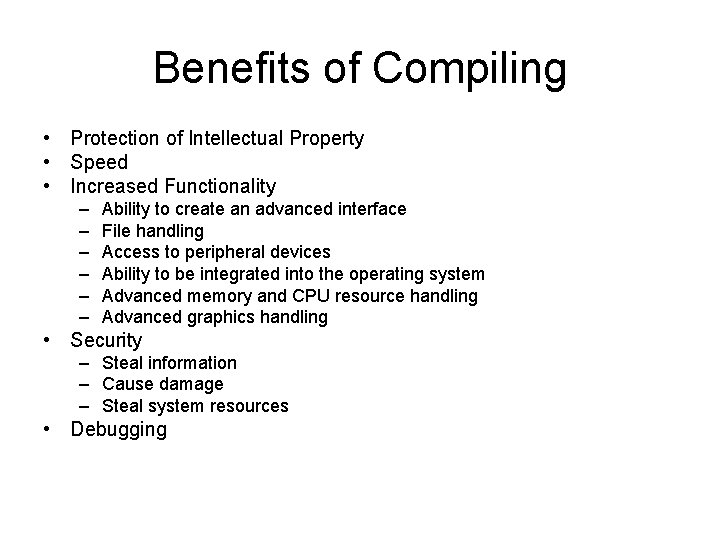
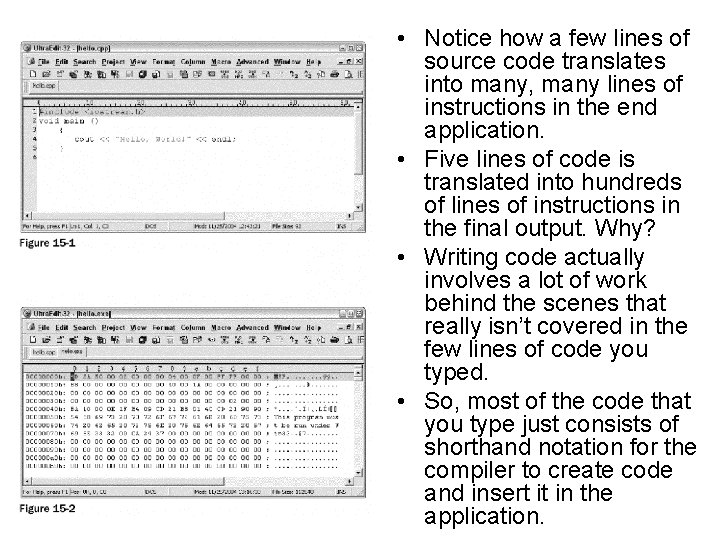
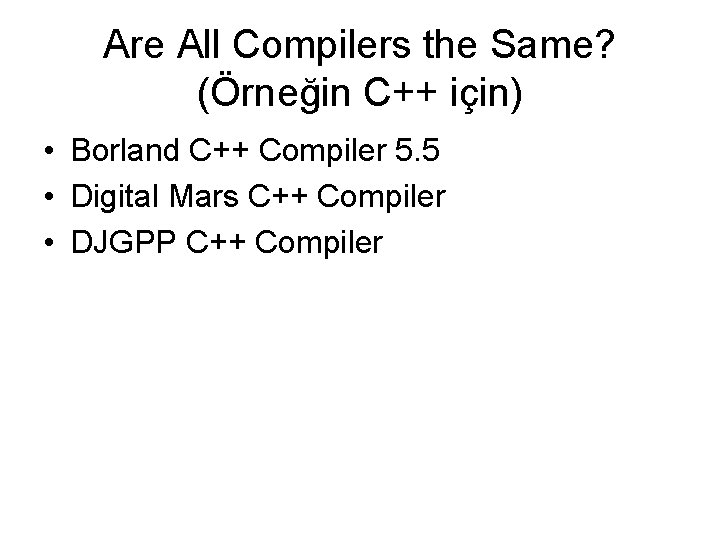
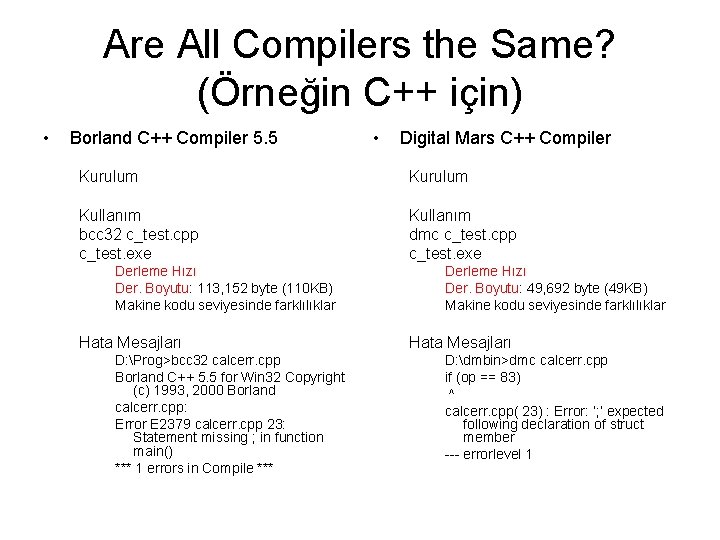
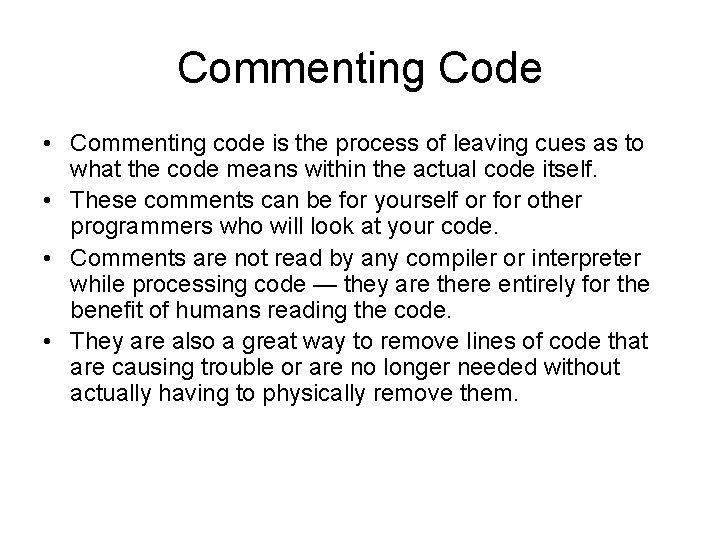
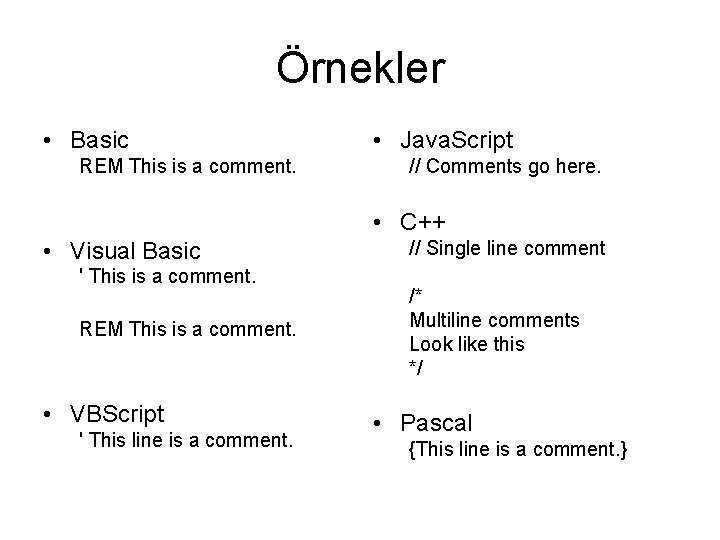
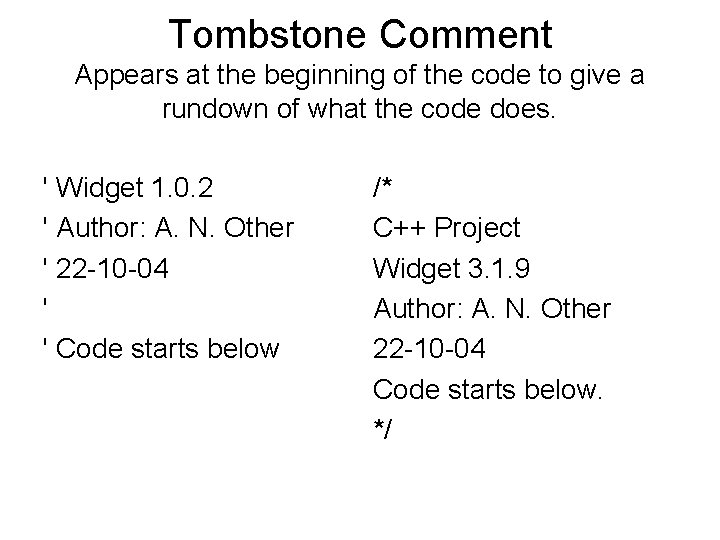
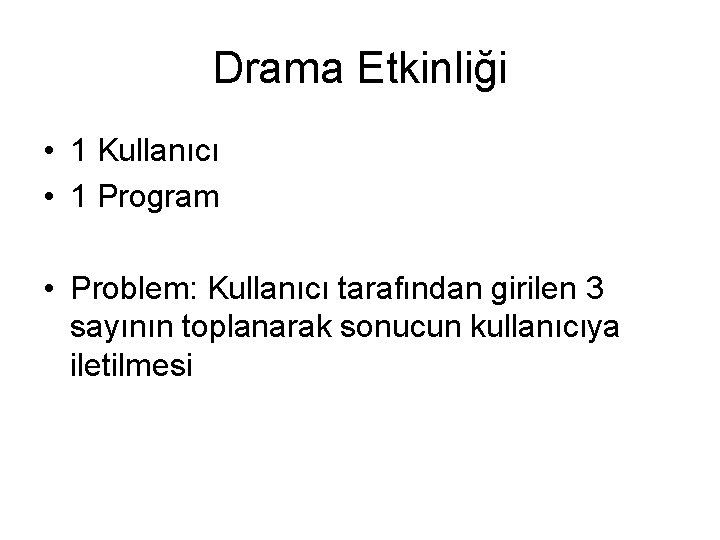
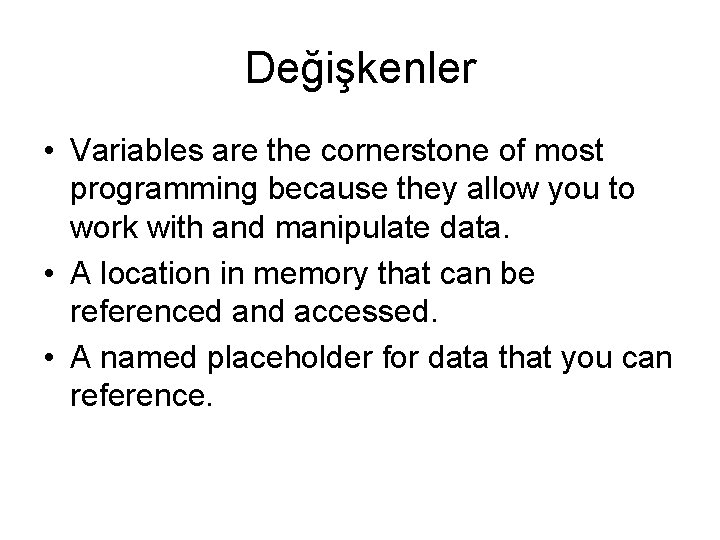
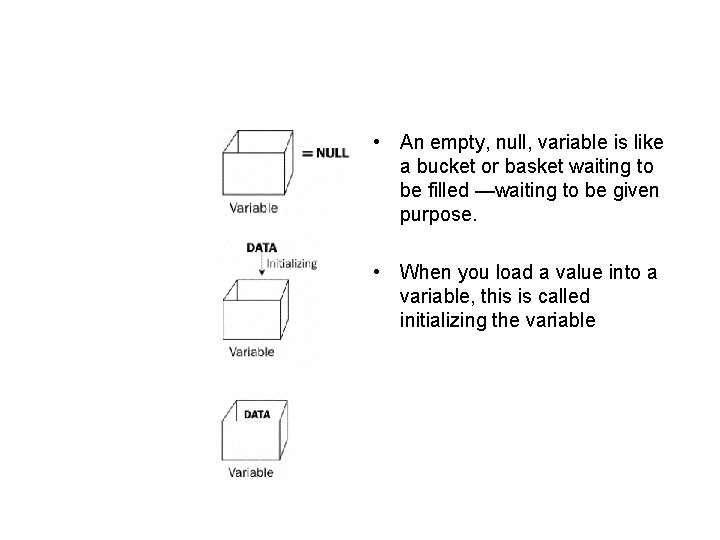
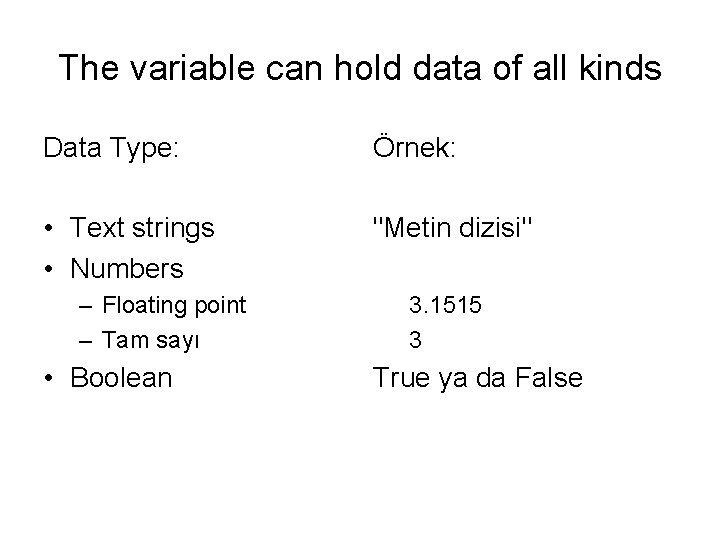
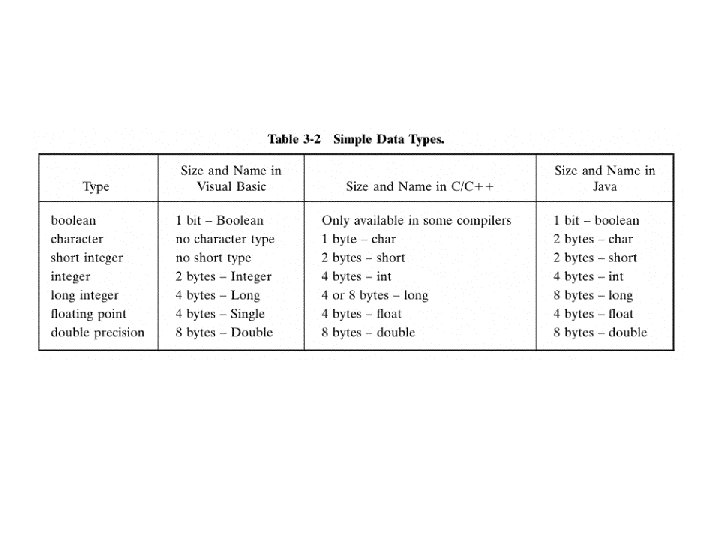
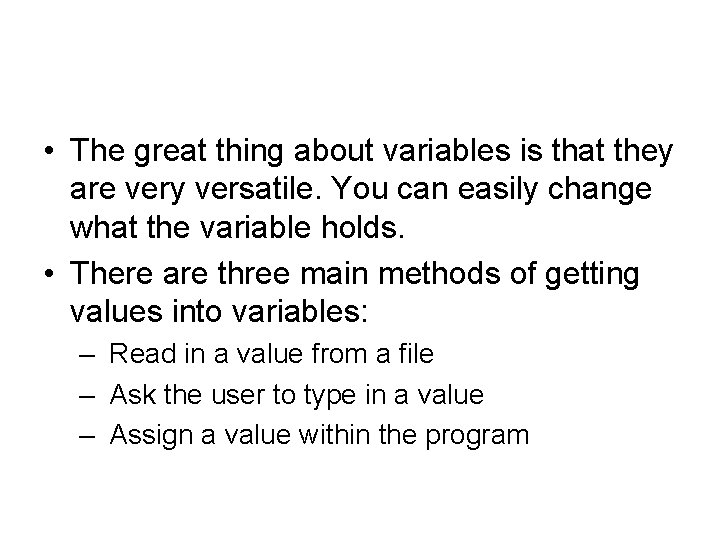
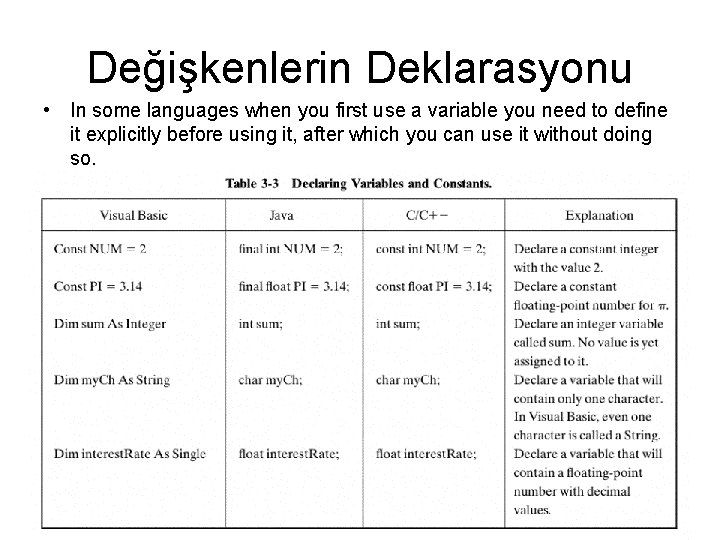
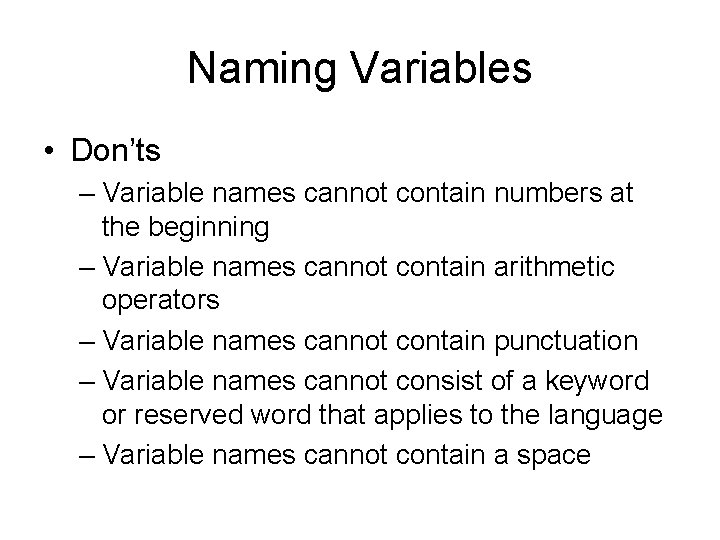
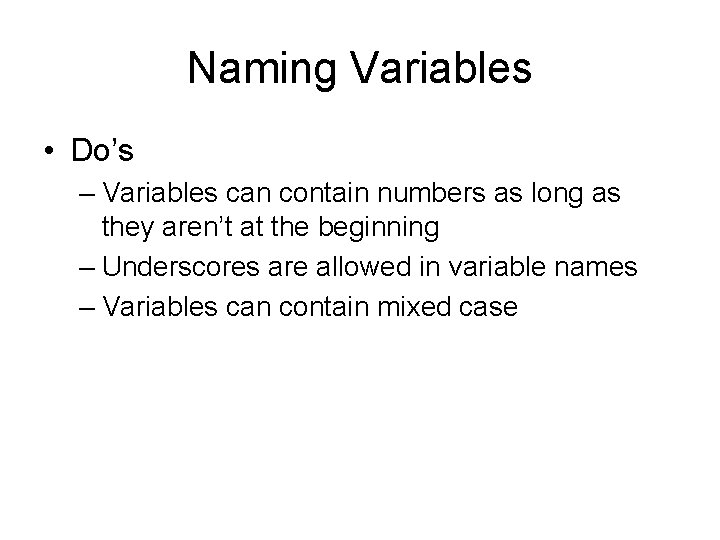
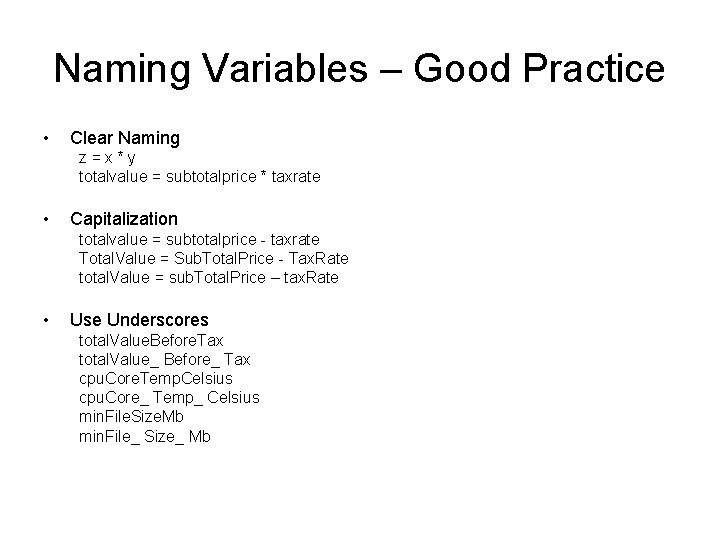
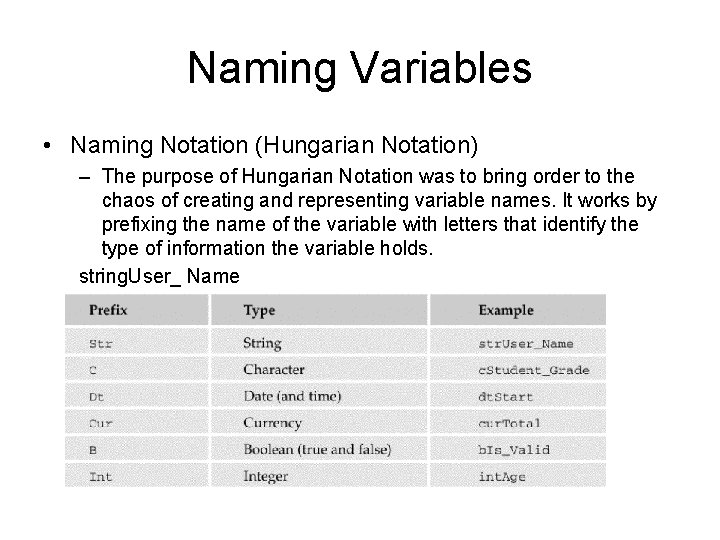
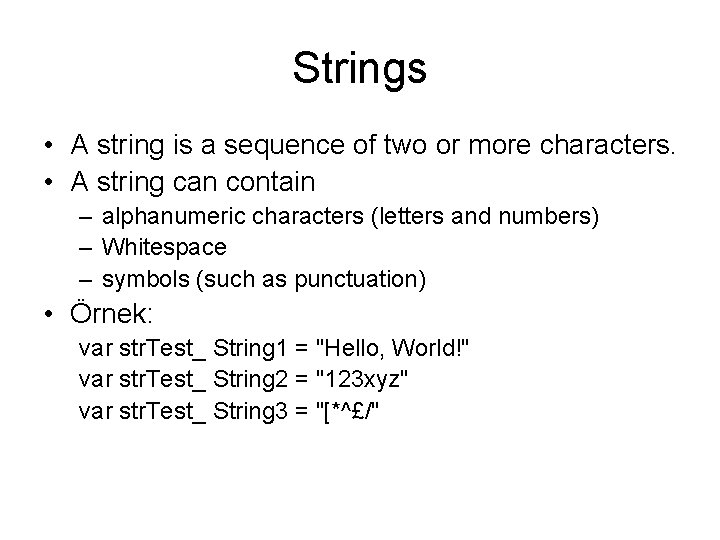
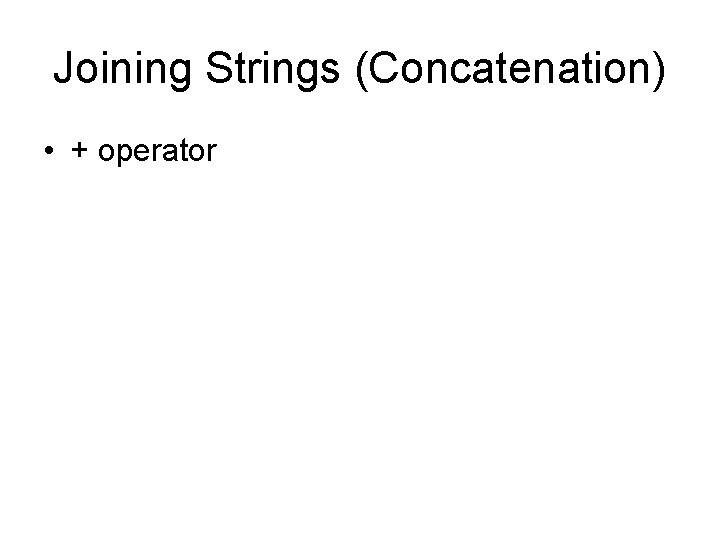
- Slides: 22
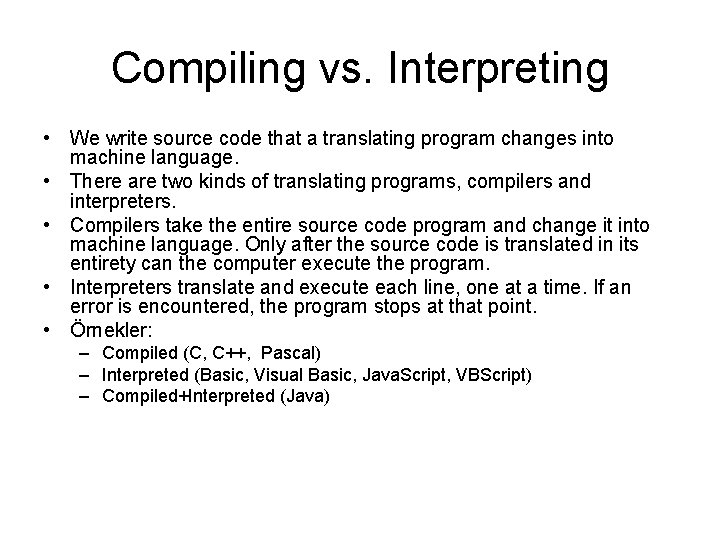
Compiling vs. Interpreting • We write source code that a translating program changes into machine language. • There are two kinds of translating programs, compilers and interpreters. • Compilers take the entire source code program and change it into machine language. Only after the source code is translated in its entirety can the computer execute the program. • Interpreters translate and execute each line, one at a time. If an error is encountered, the program stops at that point. • Örnekler: – Compiled (C, C++, Pascal) – Interpreted (Basic, Visual Basic, Java. Script, VBScript) – Compiled+Interpreted (Java)
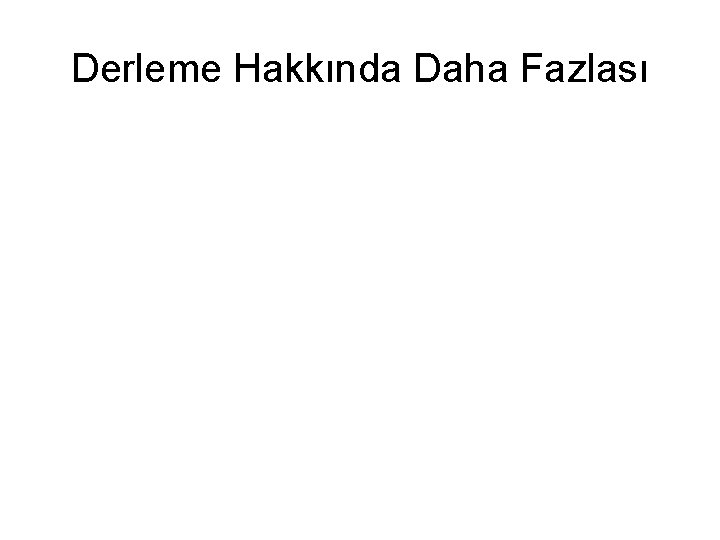
Derleme Hakkında Daha Fazlası
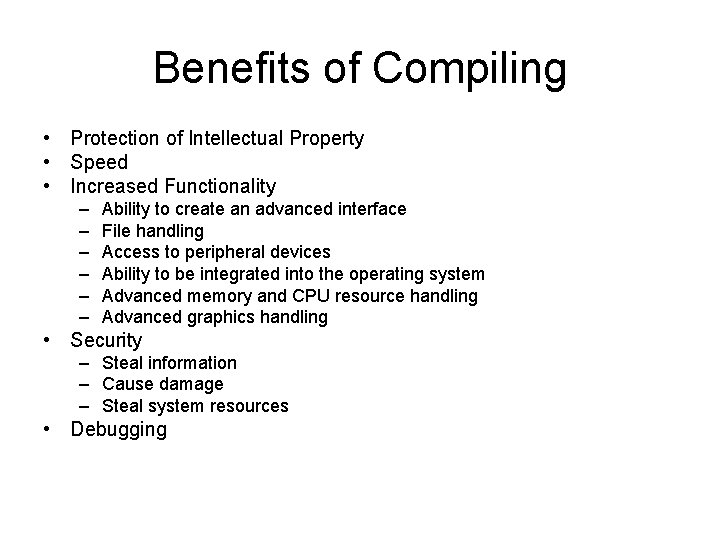
Benefits of Compiling • Protection of Intellectual Property • Speed • Increased Functionality – – – Ability to create an advanced interface File handling Access to peripheral devices Ability to be integrated into the operating system Advanced memory and CPU resource handling Advanced graphics handling • Security – Steal information – Cause damage – Steal system resources • Debugging
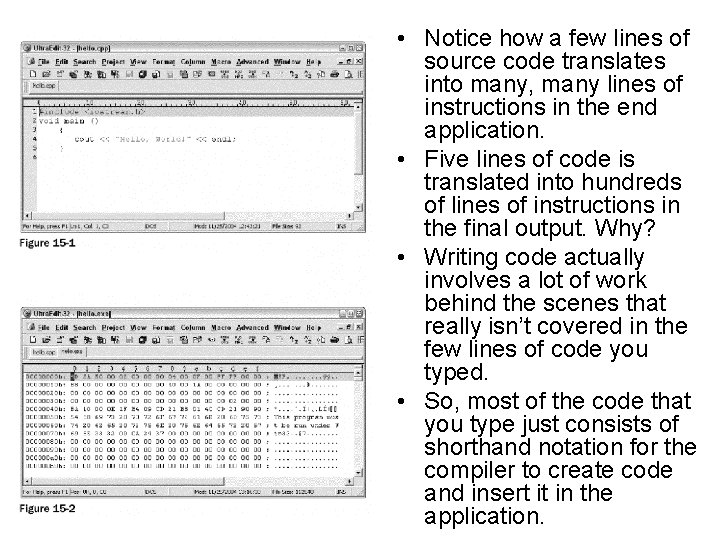
• Notice how a few lines of source code translates into many, many lines of instructions in the end application. • Five lines of code is translated into hundreds of lines of instructions in the final output. Why? • Writing code actually involves a lot of work behind the scenes that really isn’t covered in the few lines of code you typed. • So, most of the code that you type just consists of shorthand notation for the compiler to create code and insert it in the application.
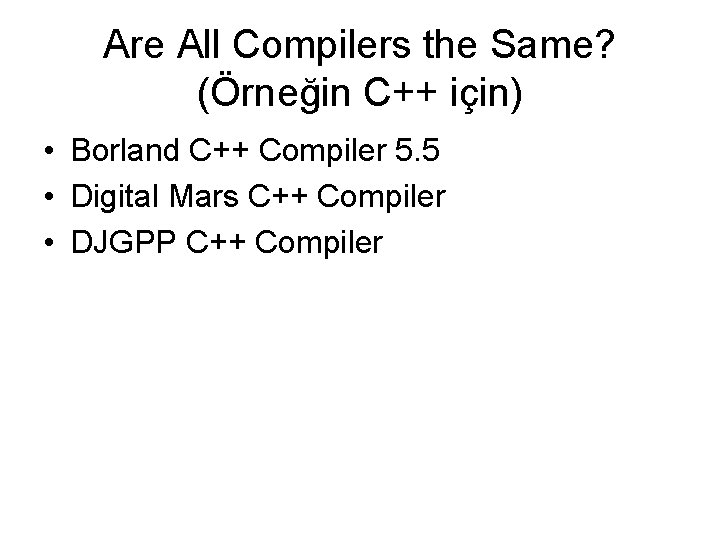
Are All Compilers the Same? (Örneğin C++ için) • Borland C++ Compiler 5. 5 • Digital Mars C++ Compiler • DJGPP C++ Compiler
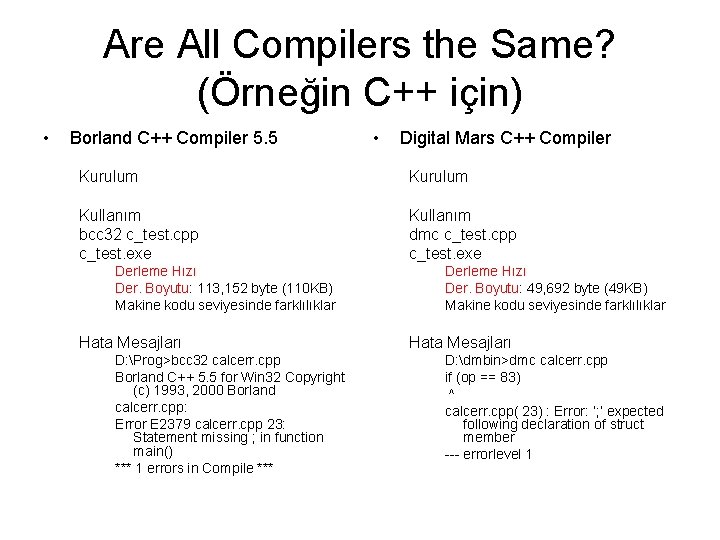
Are All Compilers the Same? (Örneğin C++ için) • Borland C++ Compiler 5. 5 • Digital Mars C++ Compiler Kurulum Kullanım bcc 32 c_test. cpp c_test. exe Kullanım dmc c_test. cpp c_test. exe Derleme Hızı Der. Boyutu: 113, 152 byte (110 KB) Makine kodu seviyesinde farklılıklar Hata Mesajları D: Prog>bcc 32 calcerr. cpp Borland C++ 5. 5 for Win 32 Copyright (c) 1993, 2000 Borland calcerr. cpp: Error E 2379 calcerr. cpp 23: Statement missing ; in function main() *** 1 errors in Compile *** Derleme Hızı Der. Boyutu: 49, 692 byte (49 KB) Makine kodu seviyesinde farklılıklar Hata Mesajları D: dmbin>dmc calcerr. cpp if (op == 83) ^ calcerr. cpp( 23) : Error: ‘; ’ expected following declaration of struct member --- errorlevel 1
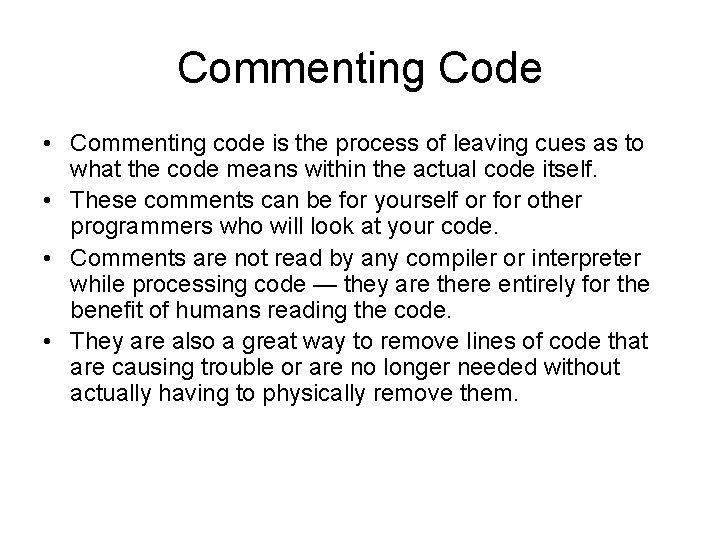
Commenting Code • Commenting code is the process of leaving cues as to what the code means within the actual code itself. • These comments can be for yourself or for other programmers who will look at your code. • Comments are not read by any compiler or interpreter while processing code — they are there entirely for the benefit of humans reading the code. • They are also a great way to remove lines of code that are causing trouble or are no longer needed without actually having to physically remove them.
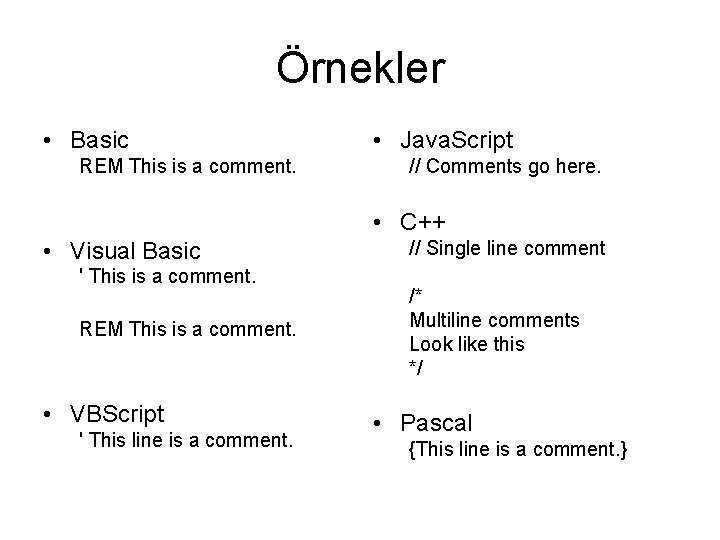
Örnekler • Basic REM This is a comment. • Java. Script // Comments go here. • C++ • Visual Basic ' This is a comment. REM This is a comment. • VBScript ' This line is a comment. // Single line comment /* Multiline comments Look like this */ • Pascal {This line is a comment. }
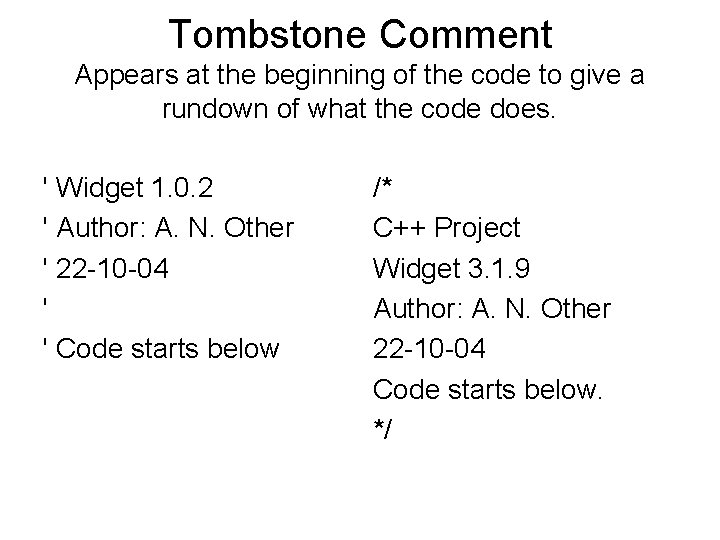
Tombstone Comment Appears at the beginning of the code to give a rundown of what the code does. ' Widget 1. 0. 2 ' Author: A. N. Other ' 22 -10 -04 ' ' Code starts below /* C++ Project Widget 3. 1. 9 Author: A. N. Other 22 -10 -04 Code starts below. */
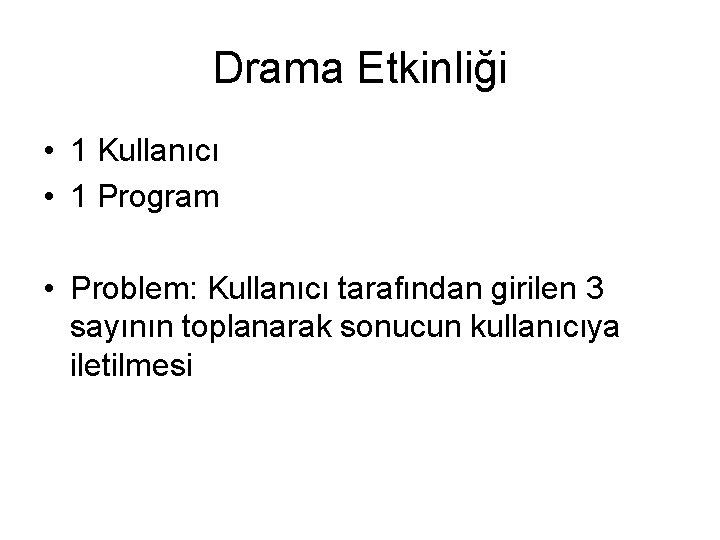
Drama Etkinliği • 1 Kullanıcı • 1 Program • Problem: Kullanıcı tarafından girilen 3 sayının toplanarak sonucun kullanıcıya iletilmesi
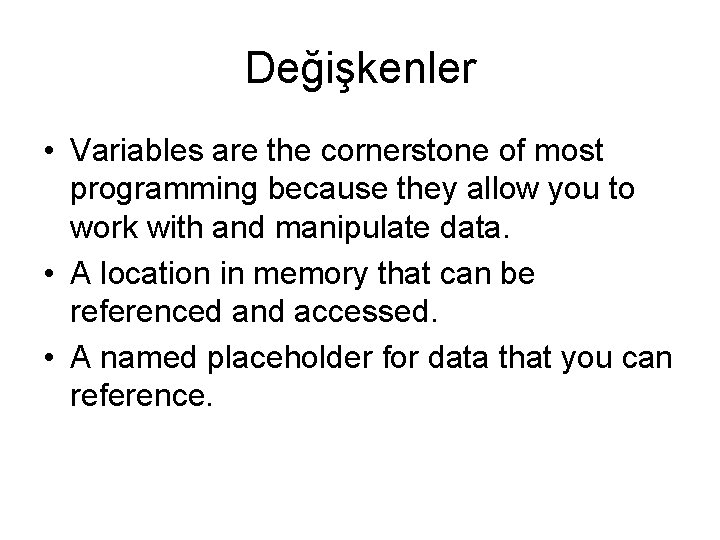
Değişkenler • Variables are the cornerstone of most programming because they allow you to work with and manipulate data. • A location in memory that can be referenced and accessed. • A named placeholder for data that you can reference.
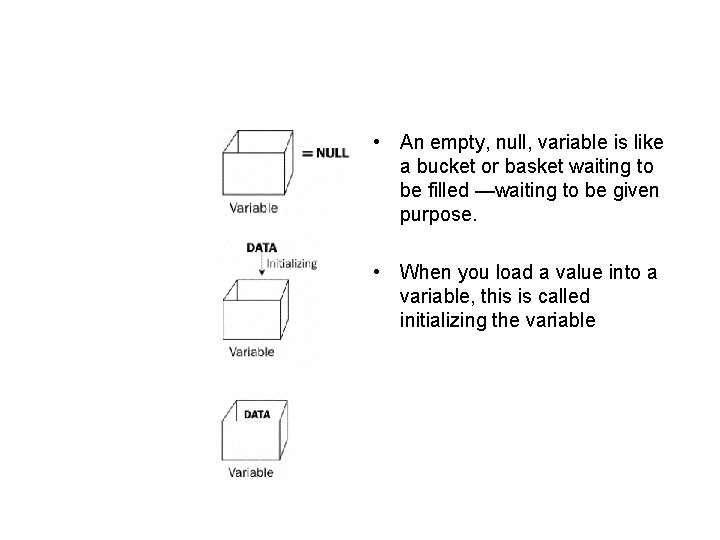
• An empty, null, variable is like a bucket or basket waiting to be filled —waiting to be given purpose. • When you load a value into a variable, this is called initializing the variable
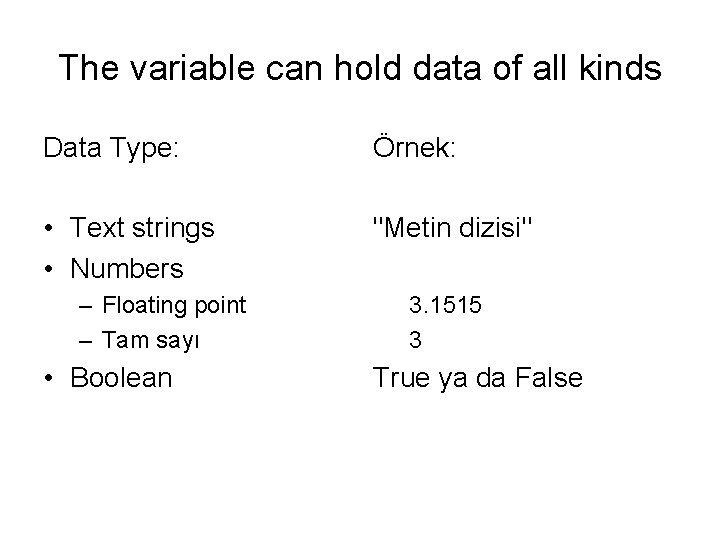
The variable can hold data of all kinds Data Type: Örnek: • Text strings • Numbers "Metin dizisi" – Floating point – Tam sayı • Boolean 3. 1515 3 True ya da False
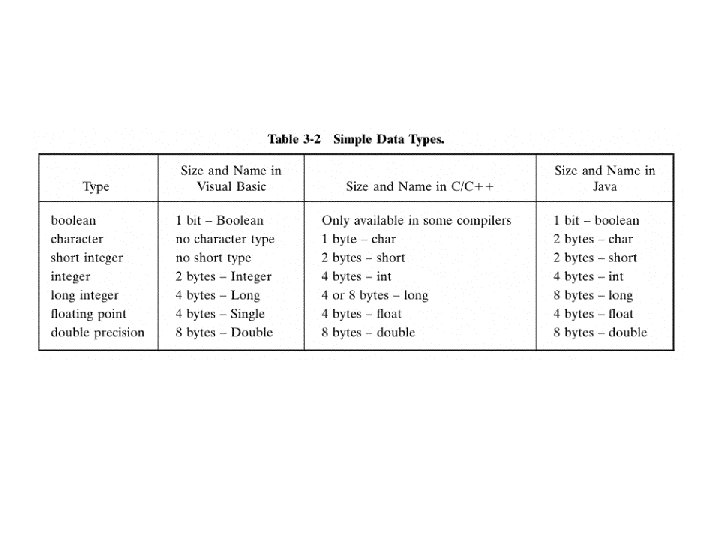
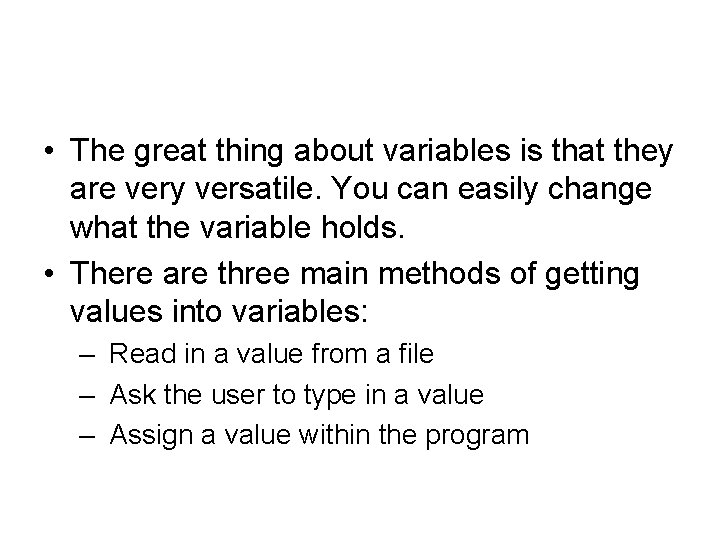
• The great thing about variables is that they are very versatile. You can easily change what the variable holds. • There are three main methods of getting values into variables: – Read in a value from a file – Ask the user to type in a value – Assign a value within the program
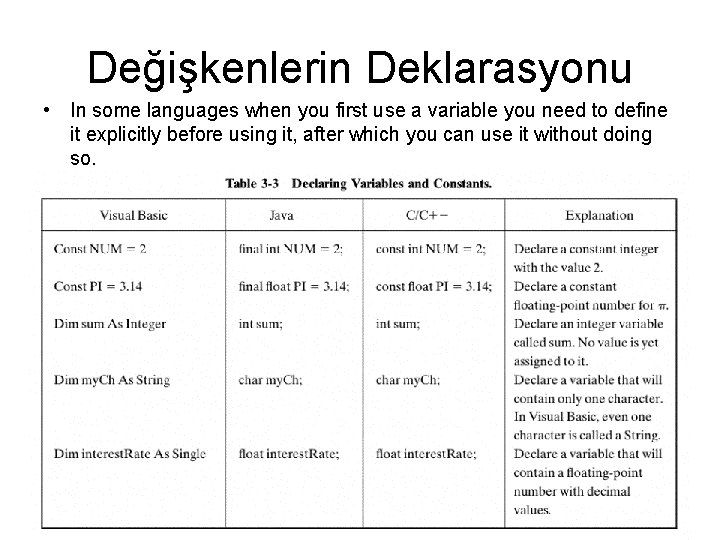
Değişkenlerin Deklarasyonu • In some languages when you first use a variable you need to define it explicitly before using it, after which you can use it without doing so.
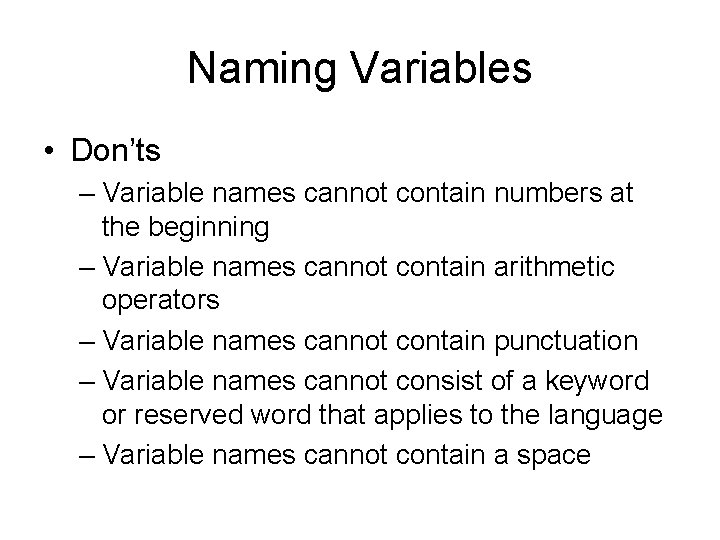
Naming Variables • Don’ts – Variable names cannot contain numbers at the beginning – Variable names cannot contain arithmetic operators – Variable names cannot contain punctuation – Variable names cannot consist of a keyword or reserved word that applies to the language – Variable names cannot contain a space
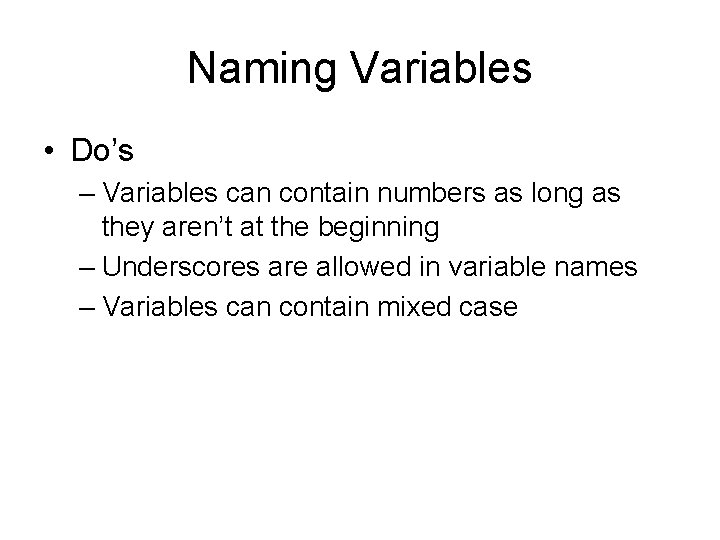
Naming Variables • Do’s – Variables can contain numbers as long as they aren’t at the beginning – Underscores are allowed in variable names – Variables can contain mixed case
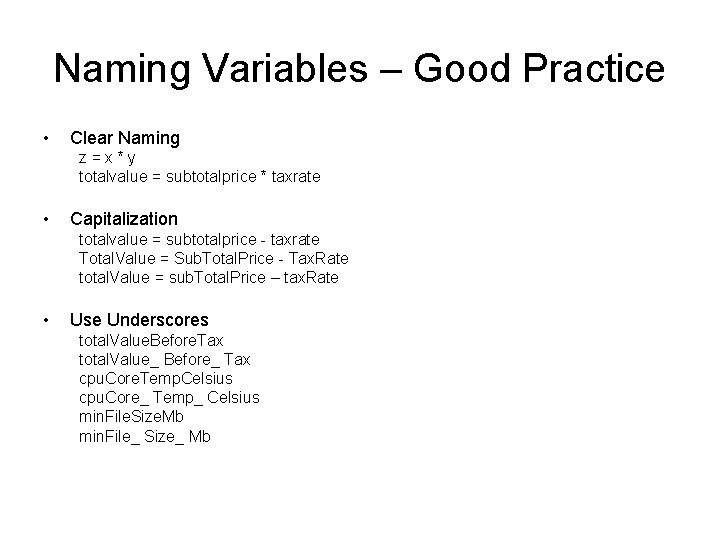
Naming Variables – Good Practice • Clear Naming z=x*y totalvalue = subtotalprice * taxrate • Capitalization totalvalue = subtotalprice - taxrate Total. Value = Sub. Total. Price - Tax. Rate total. Value = sub. Total. Price – tax. Rate • Use Underscores total. Value. Before. Tax total. Value_ Before_ Tax cpu. Core. Temp. Celsius cpu. Core_ Temp_ Celsius min. File. Size. Mb min. File_ Size_ Mb
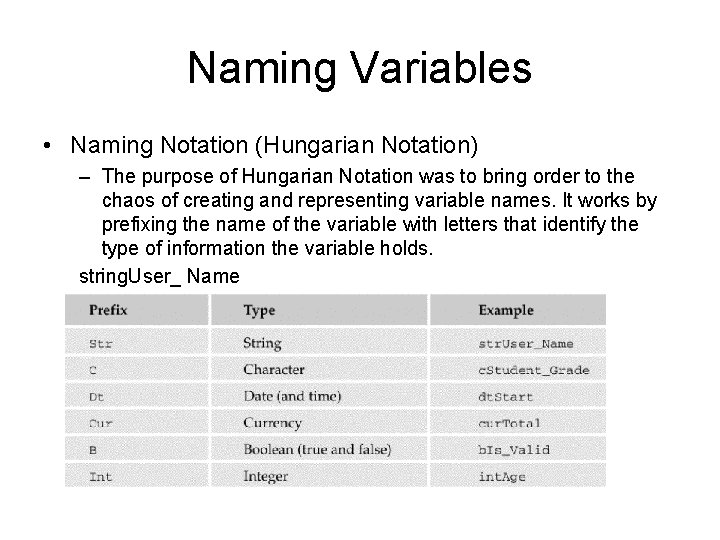
Naming Variables • Naming Notation (Hungarian Notation) – The purpose of Hungarian Notation was to bring order to the chaos of creating and representing variable names. It works by prefixing the name of the variable with letters that identify the type of information the variable holds. string. User_ Name
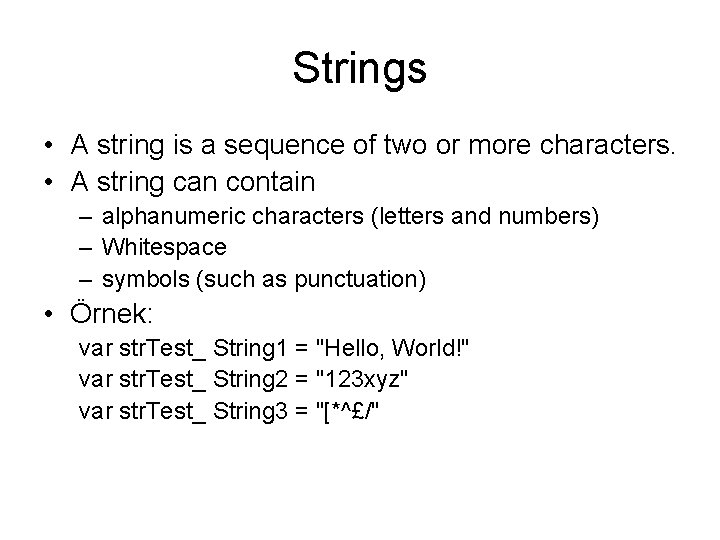
Strings • A string is a sequence of two or more characters. • A string can contain – alphanumeric characters (letters and numbers) – Whitespace – symbols (such as punctuation) • Örnek: var str. Test_ String 1 = "Hello, World!" var str. Test_ String 2 = "123 xyz" var str. Test_ String 3 = "[*^£/"
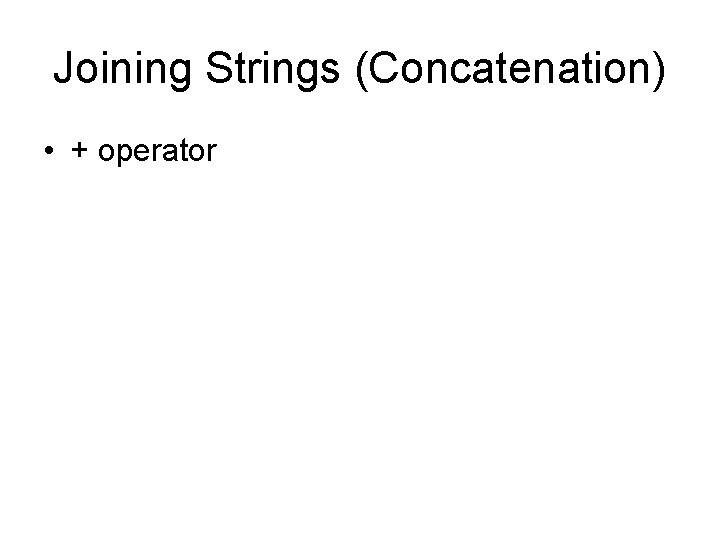
Joining Strings (Concatenation) • + operator
C++ hungarian notation
Fase fase proses kompilasi
Process of compiling data in research
Compiling information
An integral part planning and compiling a calendar is
Thoughts compiling
Compiling creates a
Phân độ lown
Block av độ 2
Thơ thất ngôn tứ tuyệt đường luật
Thơ thất ngôn tứ tuyệt đường luật
Walmart thất bại ở nhật
Tìm độ lớn thật của tam giác abc
Con hãy đưa tay khi thấy người vấp ngã
Tôn thất thuyết là ai
Gây tê cơ vuông thắt lưng
Sau thất bại ở hồ điển triệt
Difference between source code and machine code
Code commit code build code deploy
Tamagotchi 6502
Source code disclosure
Source code structure
Fortify analysis