CMSC 202 Java Primer July 24 2007 A
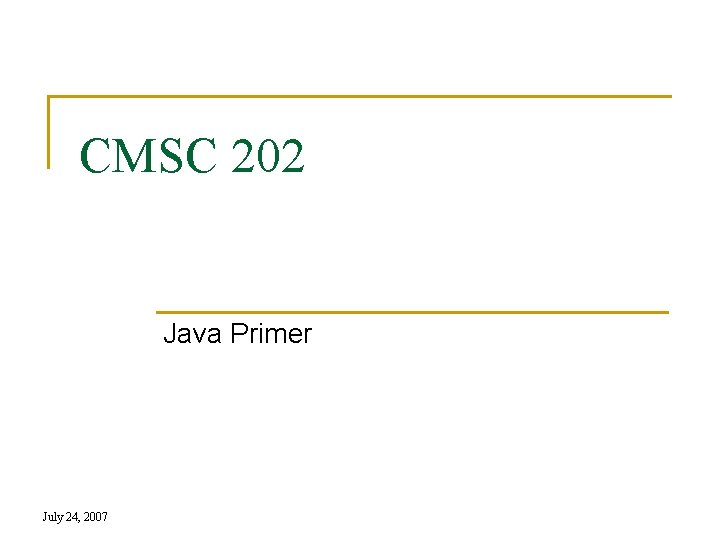
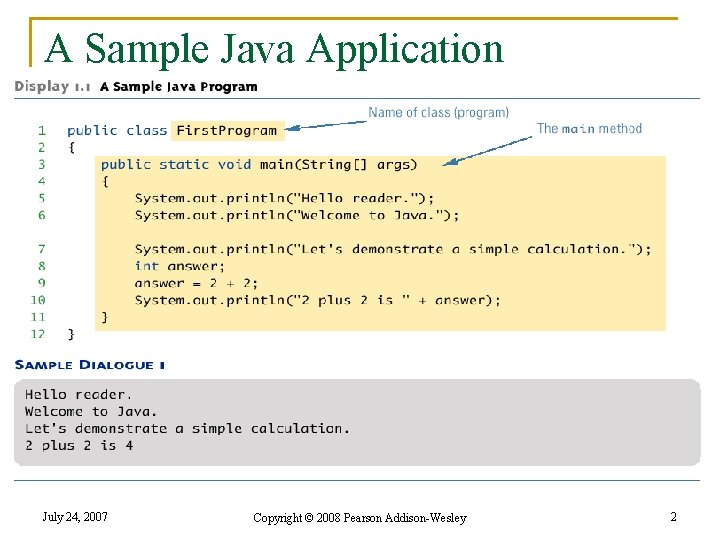
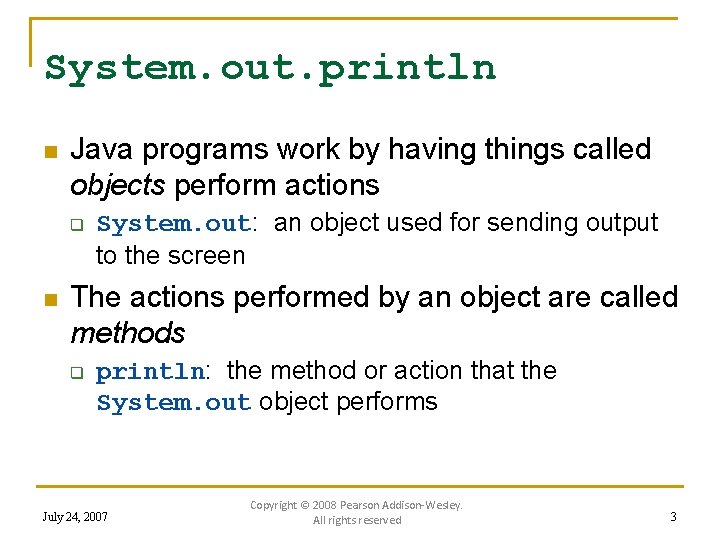
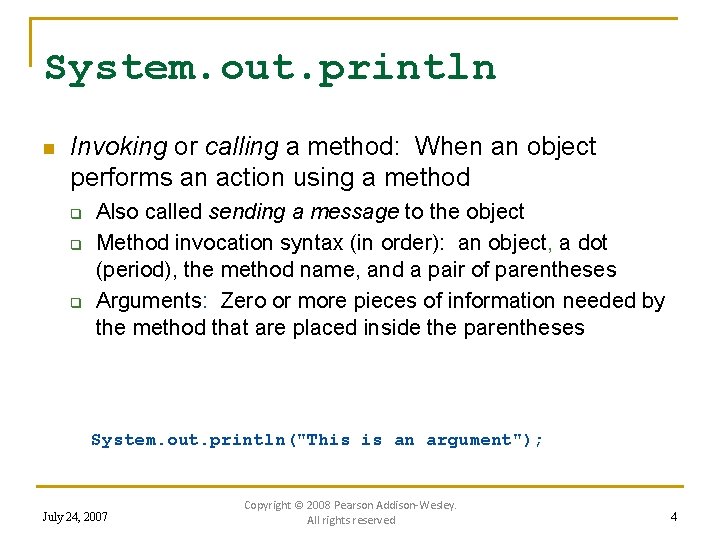
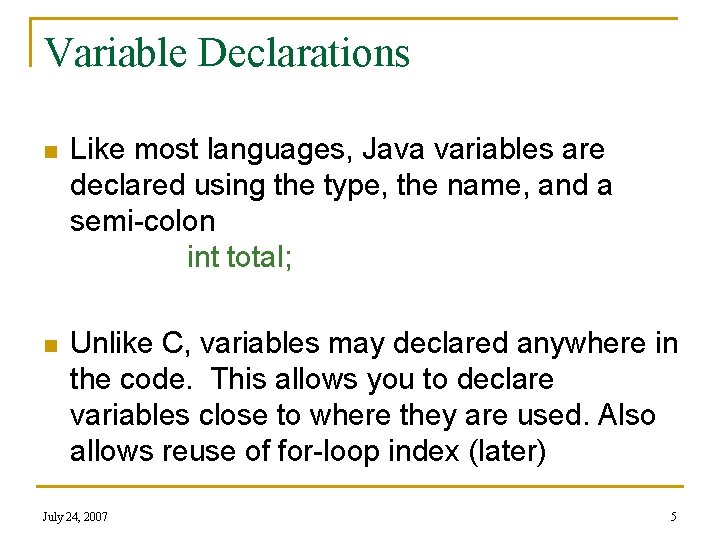
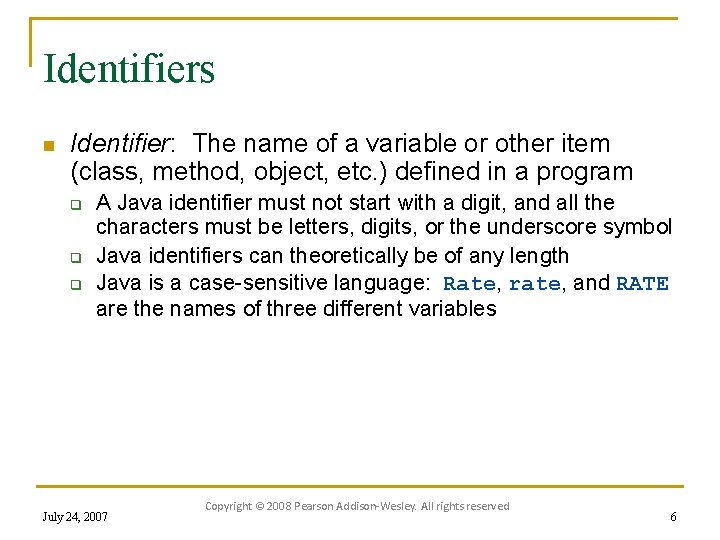
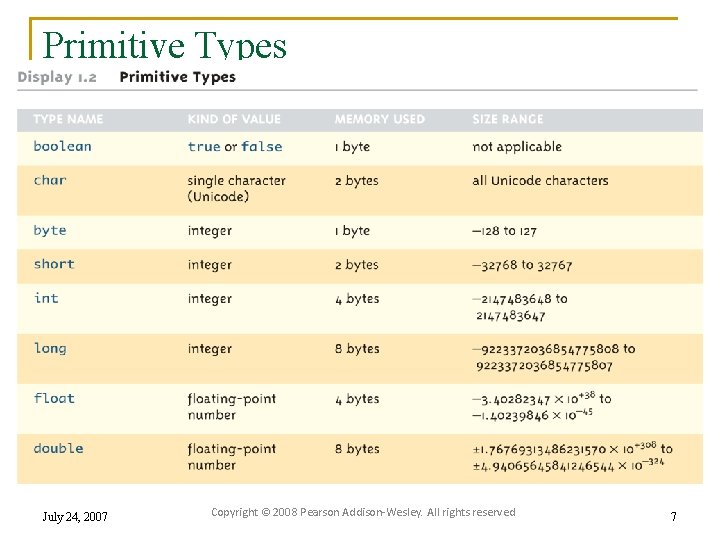
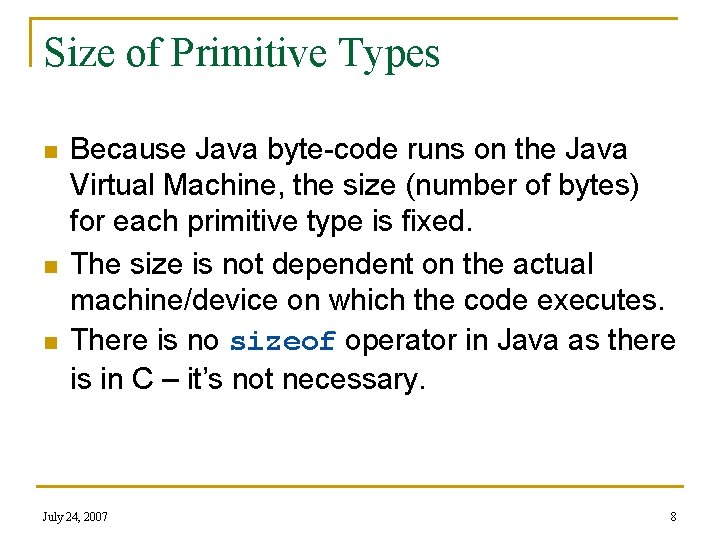
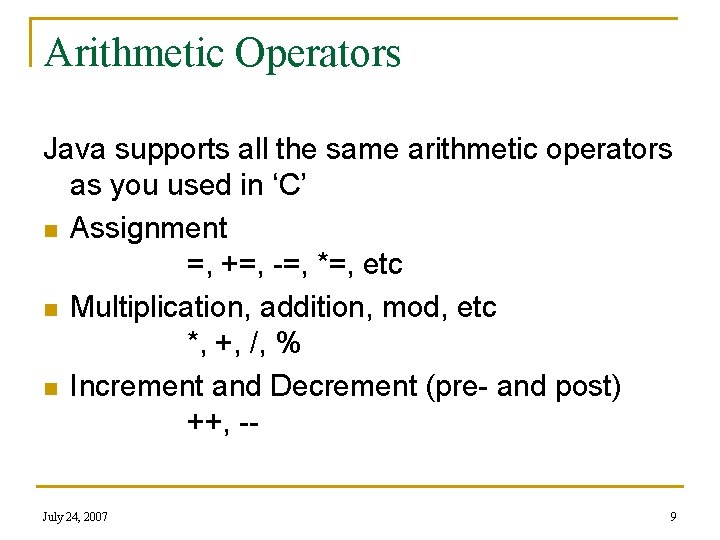
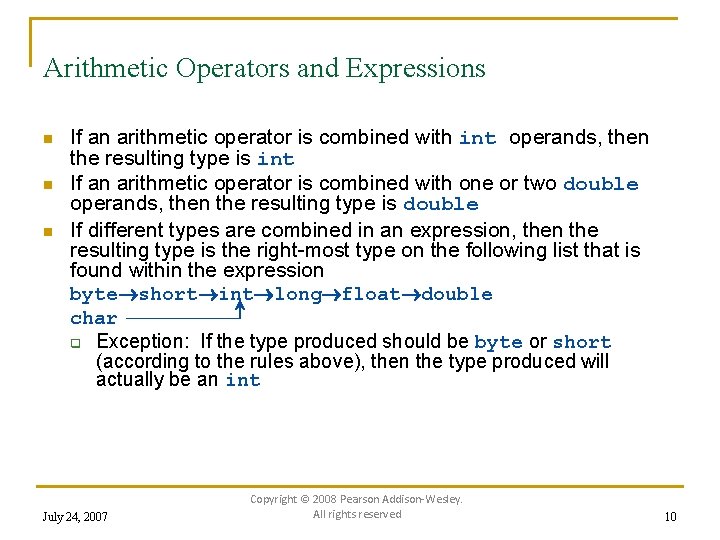
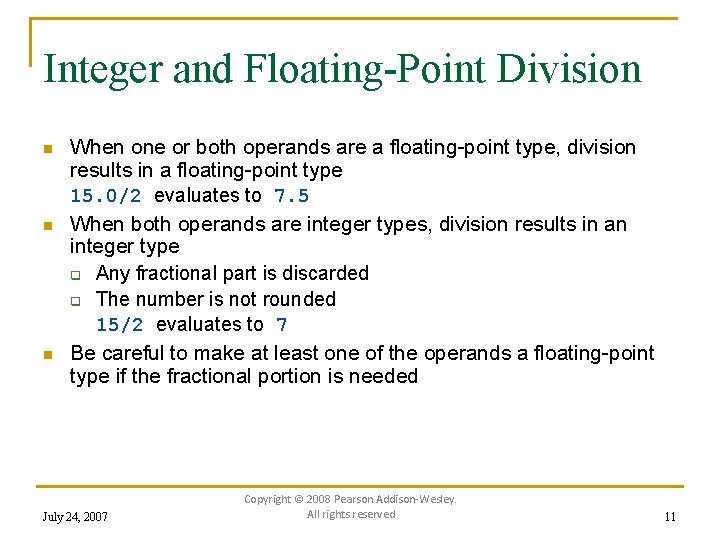
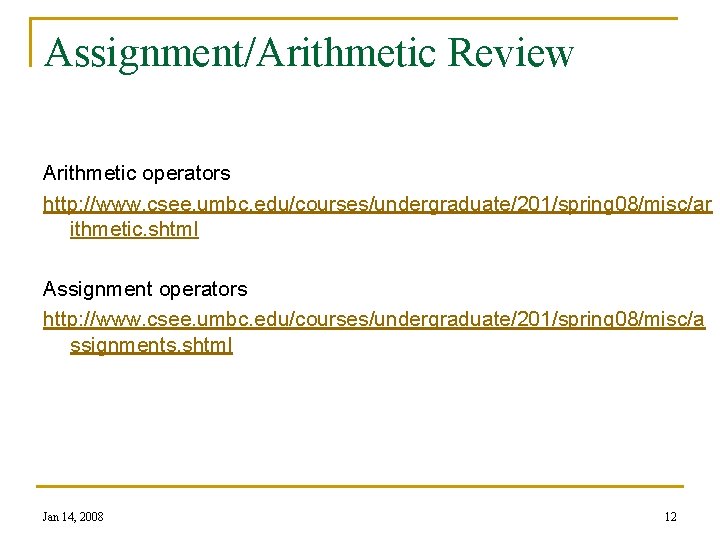
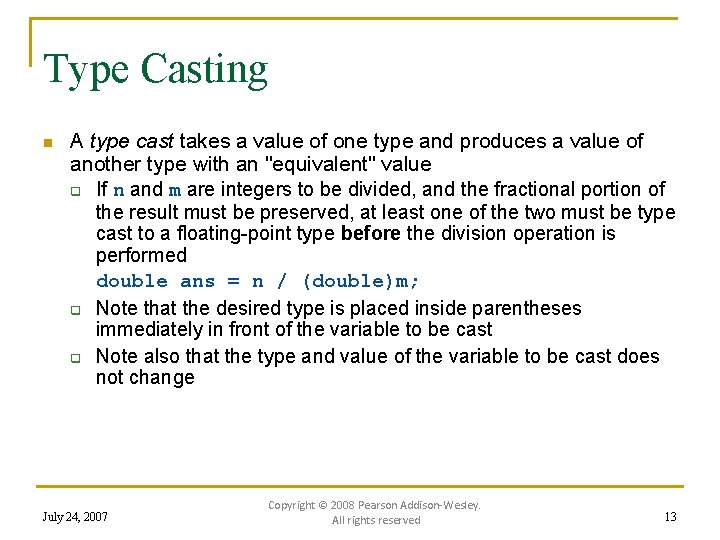
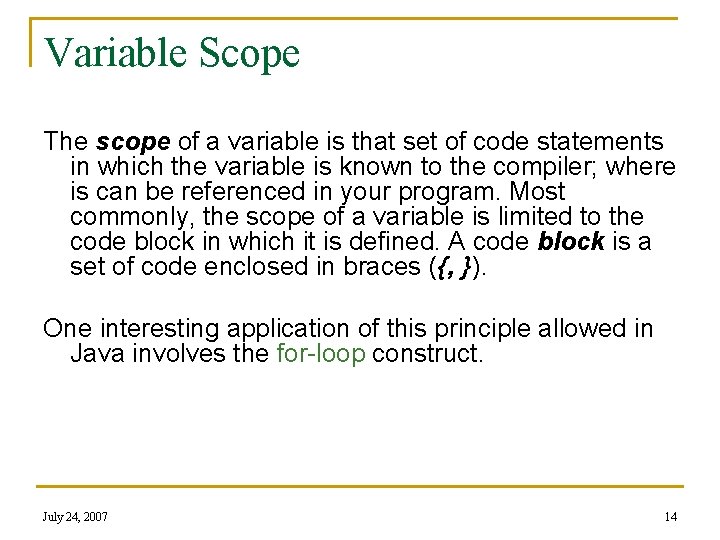
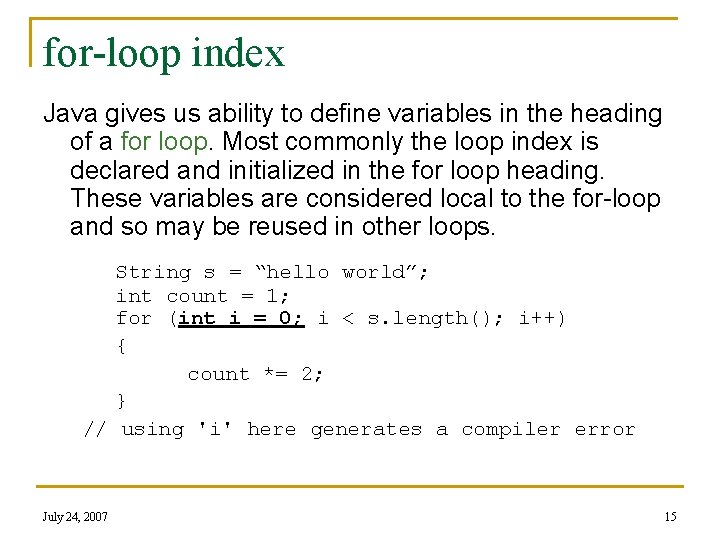
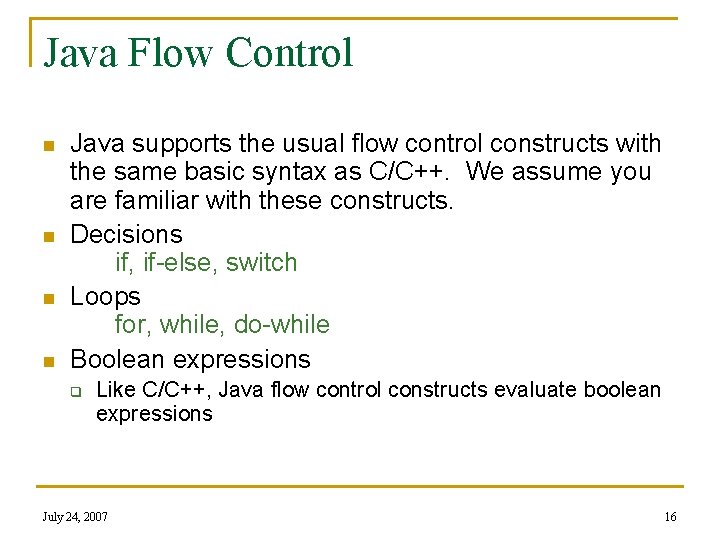
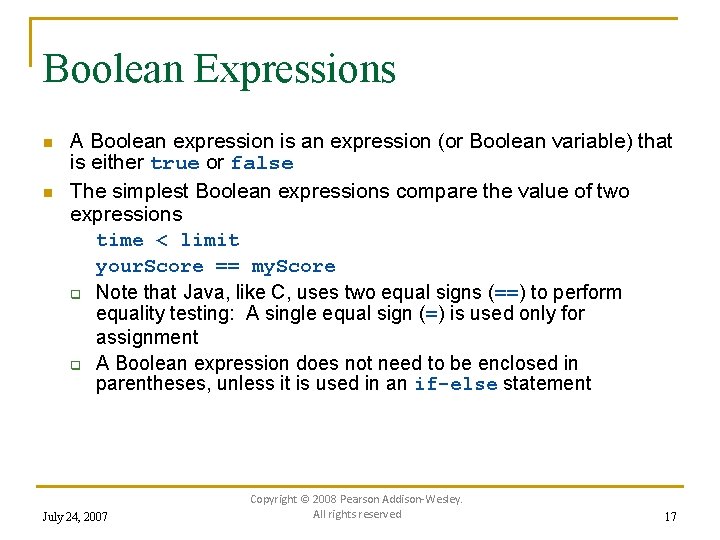
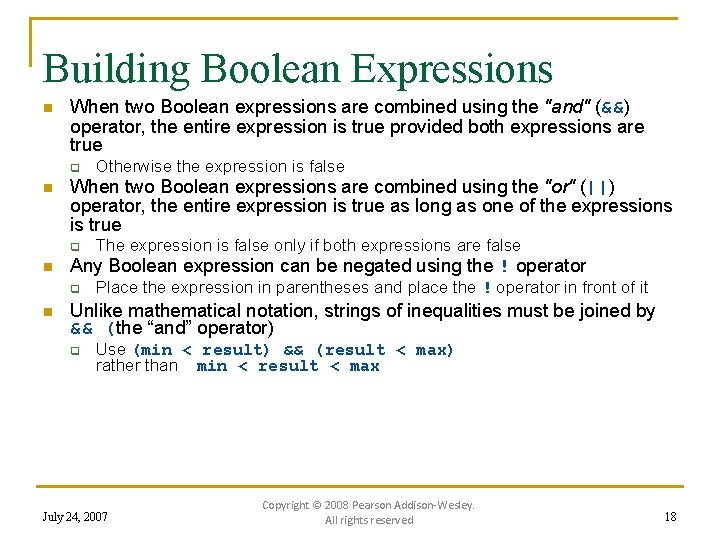
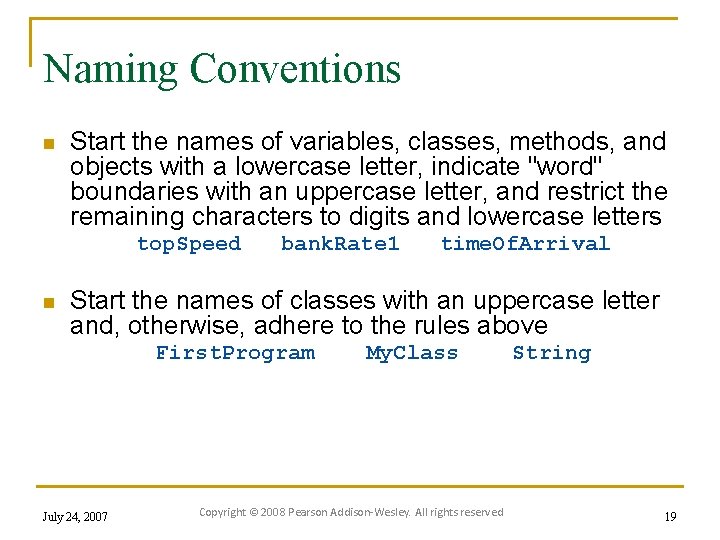
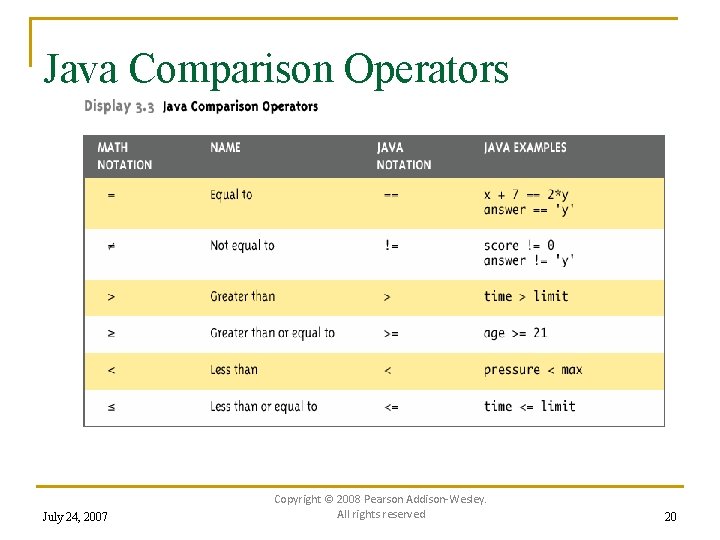
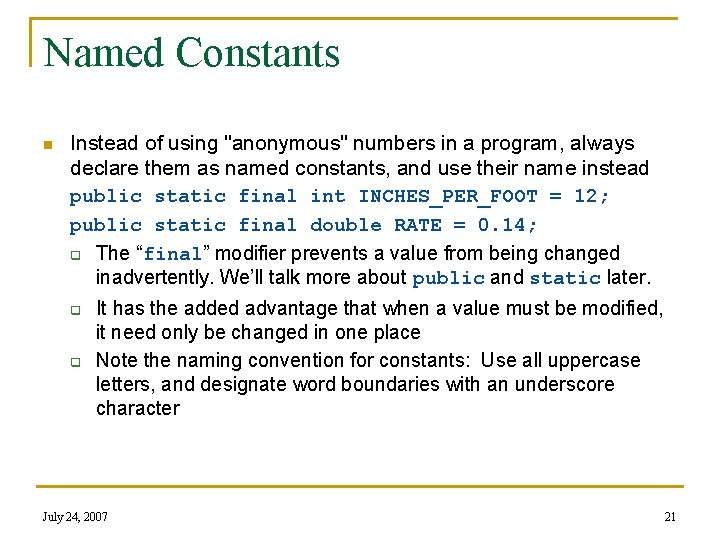
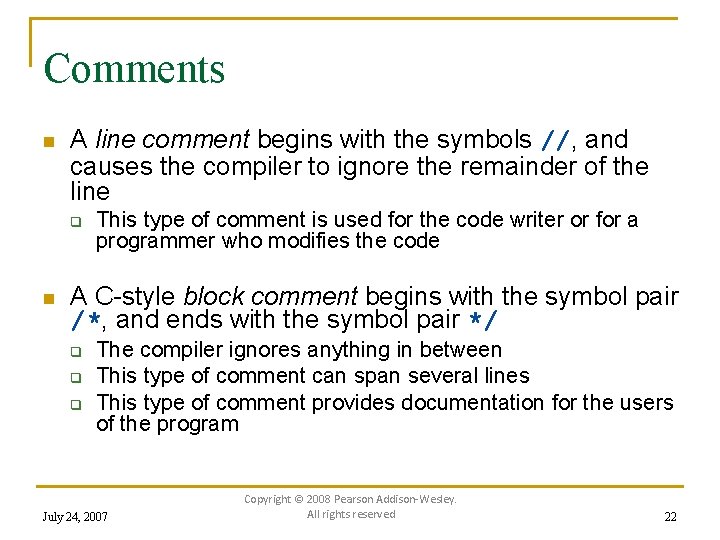
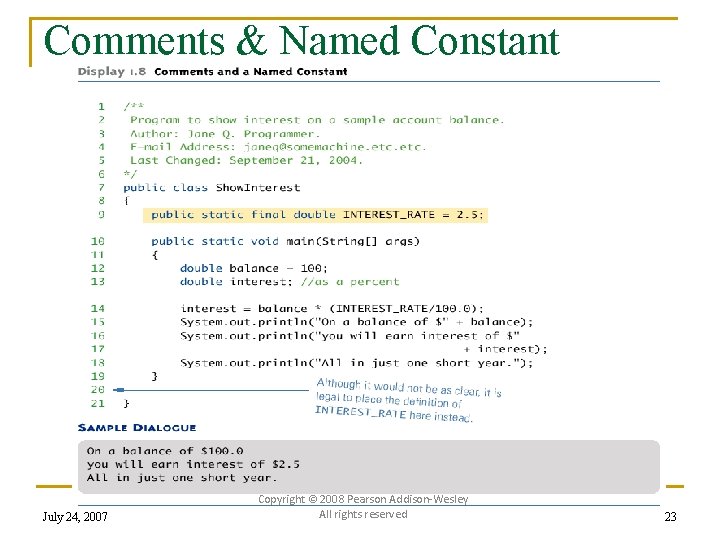
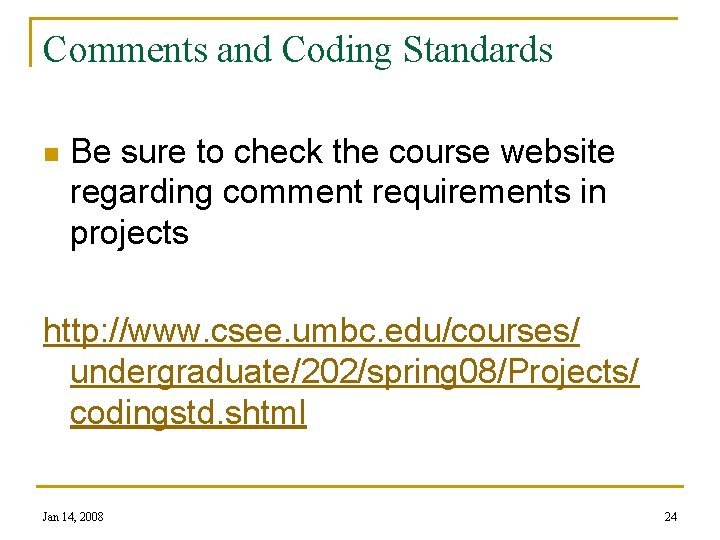
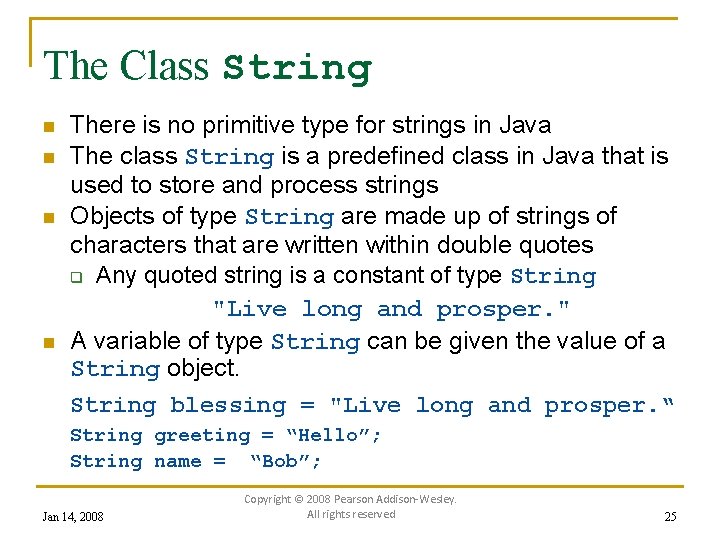
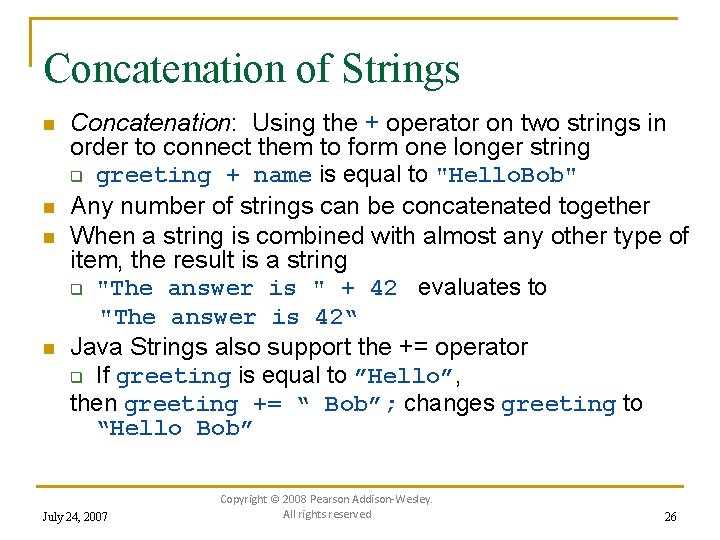
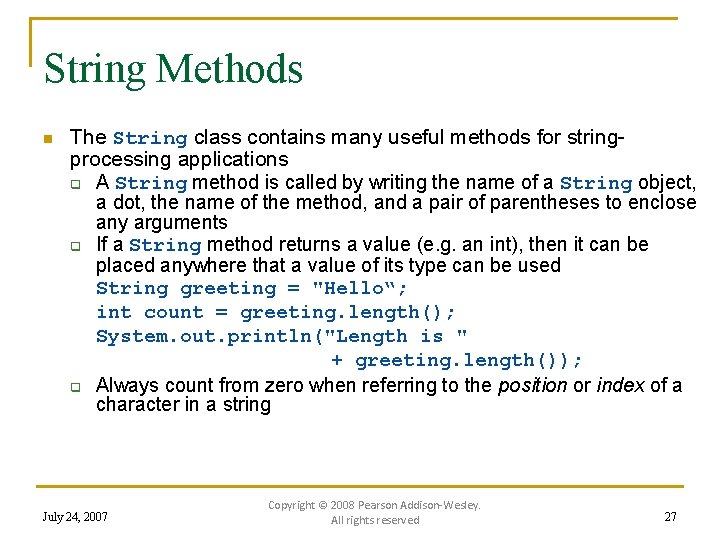
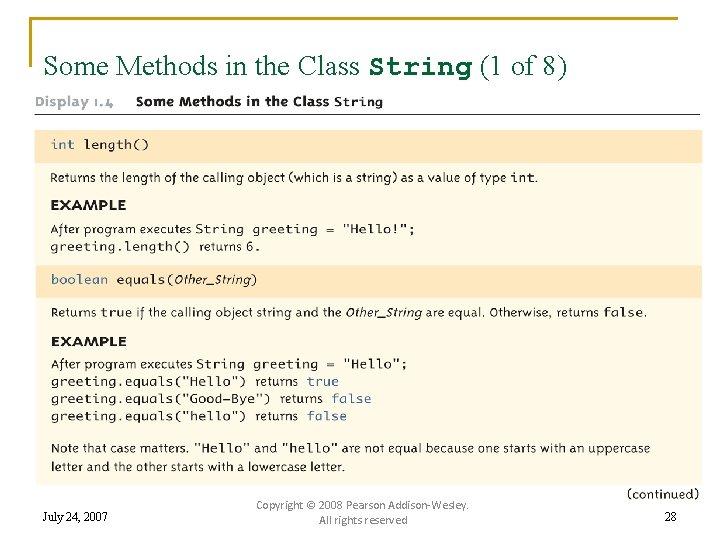
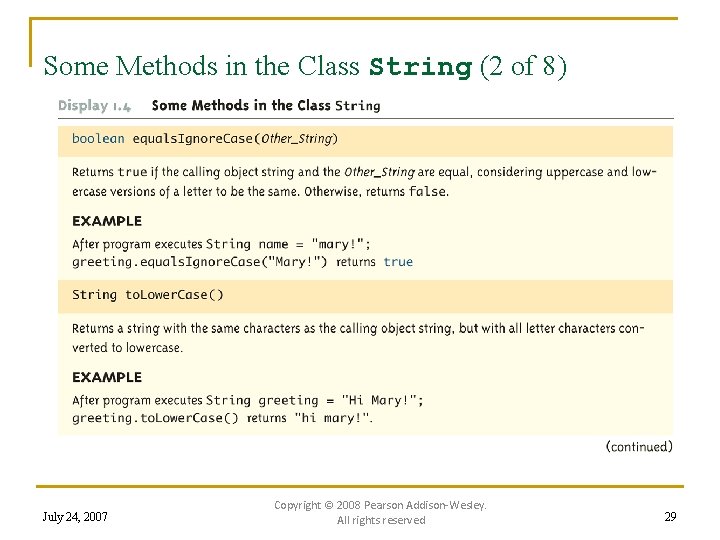
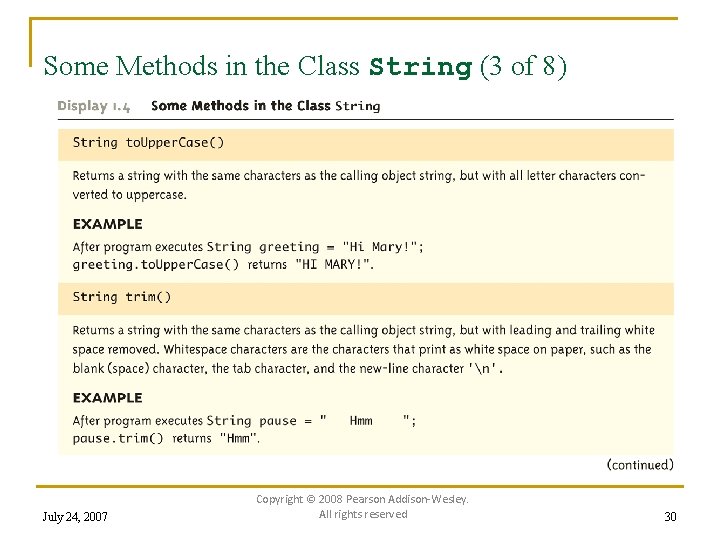
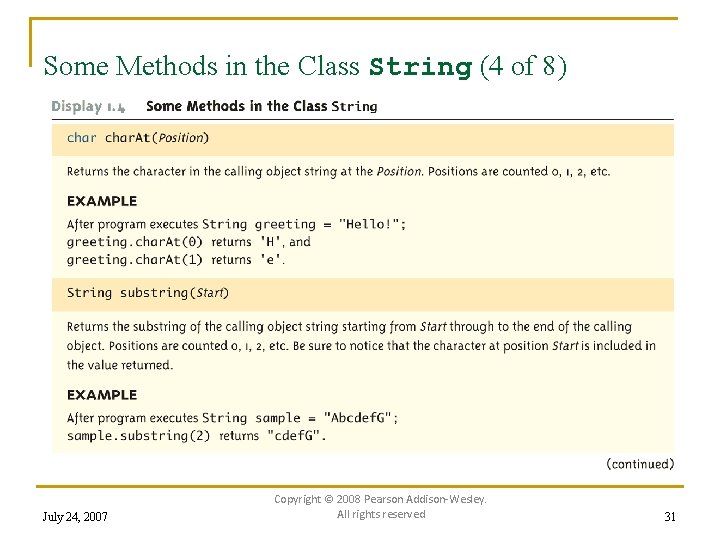
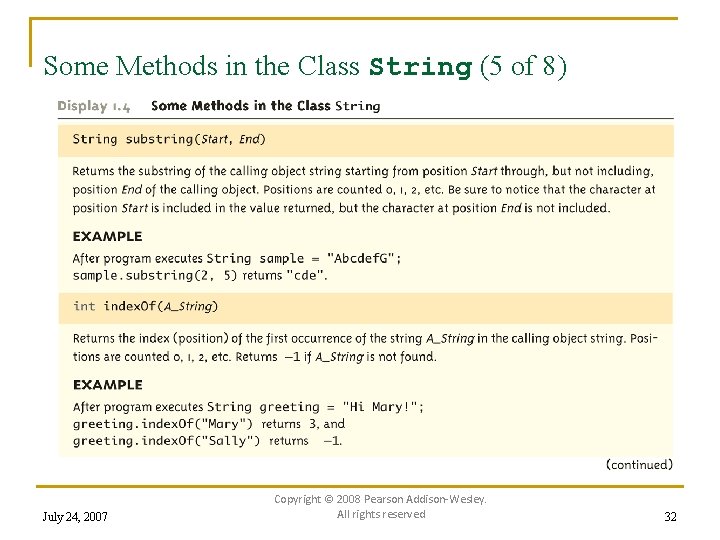
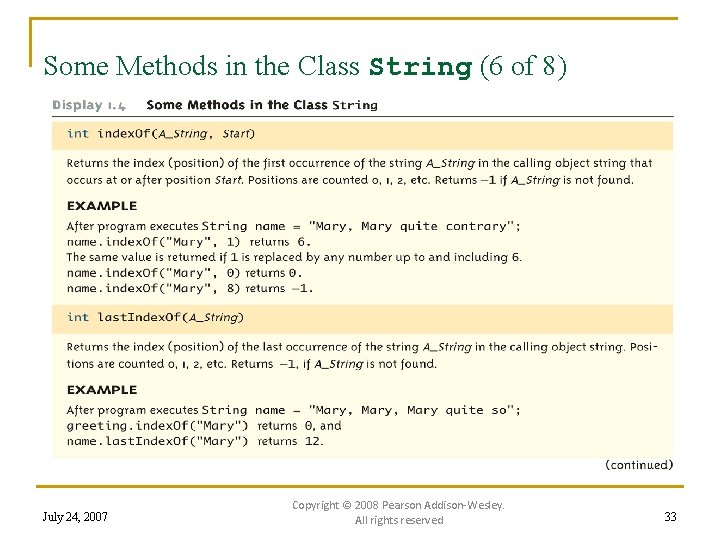
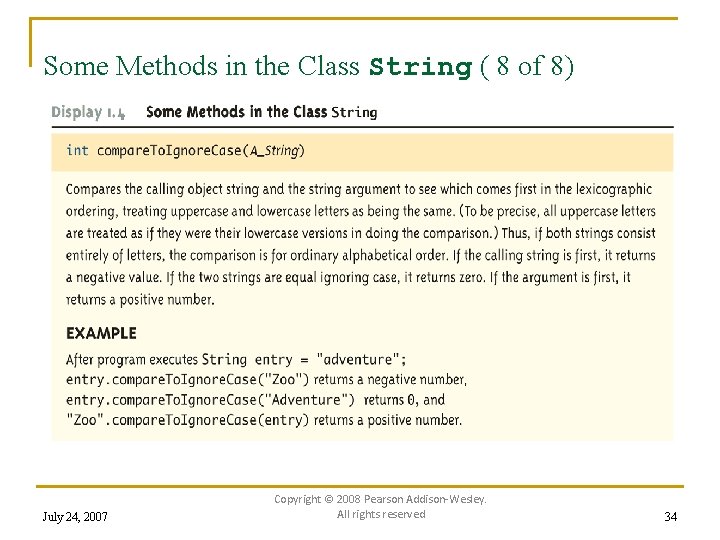
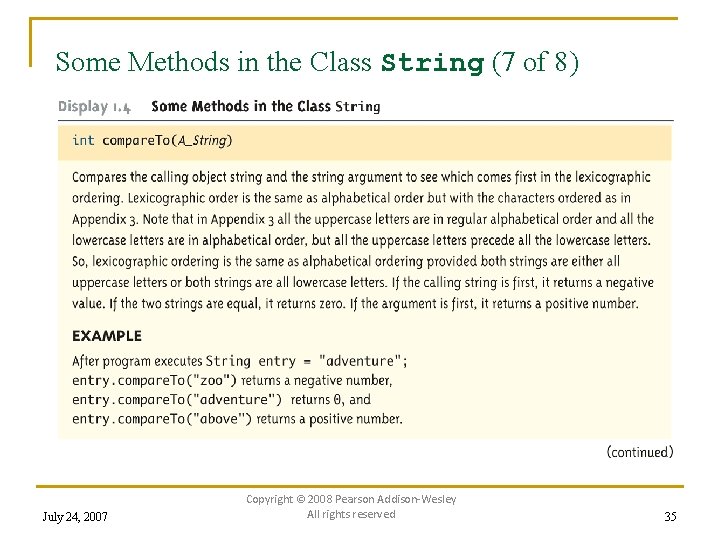
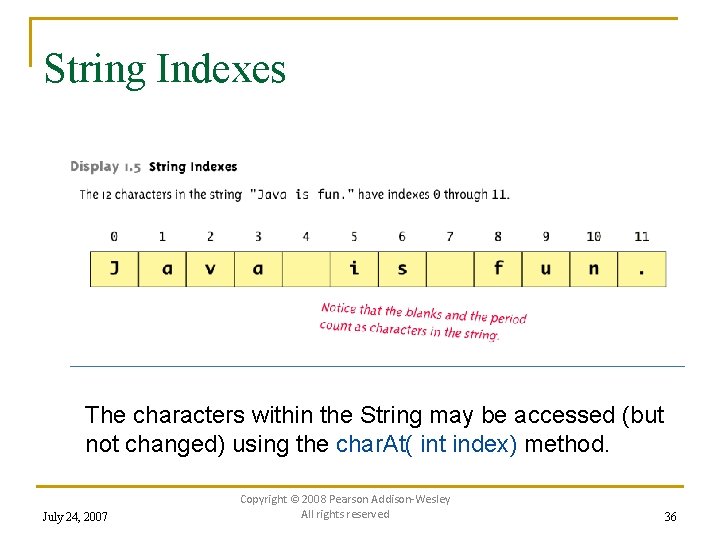
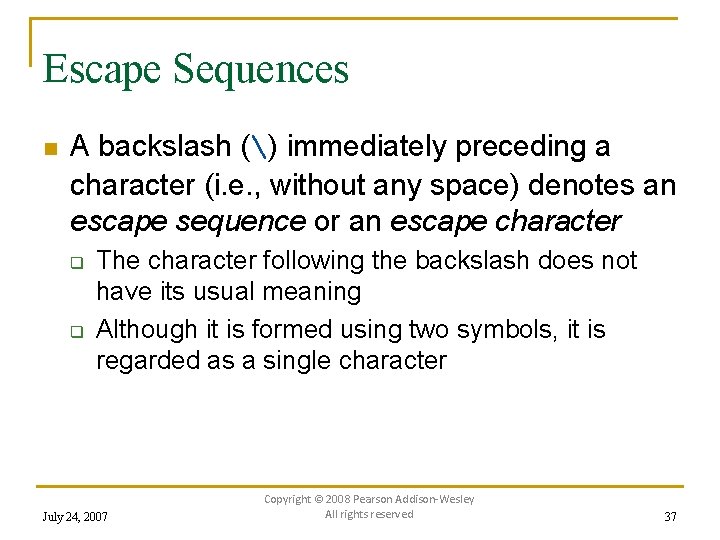
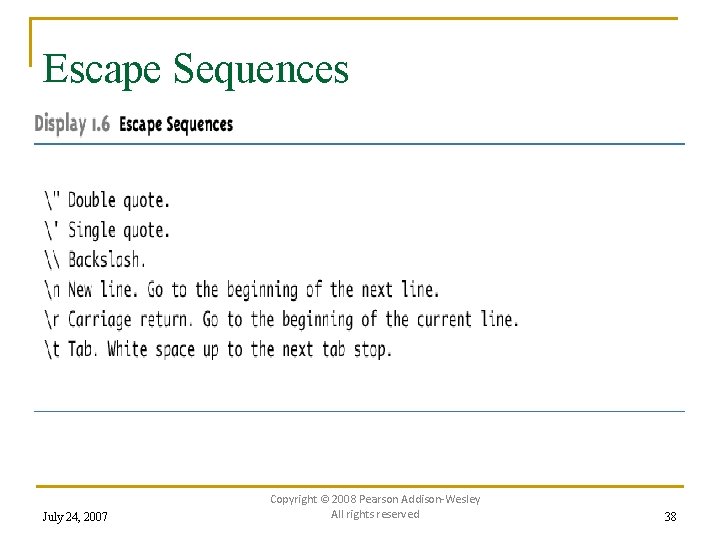
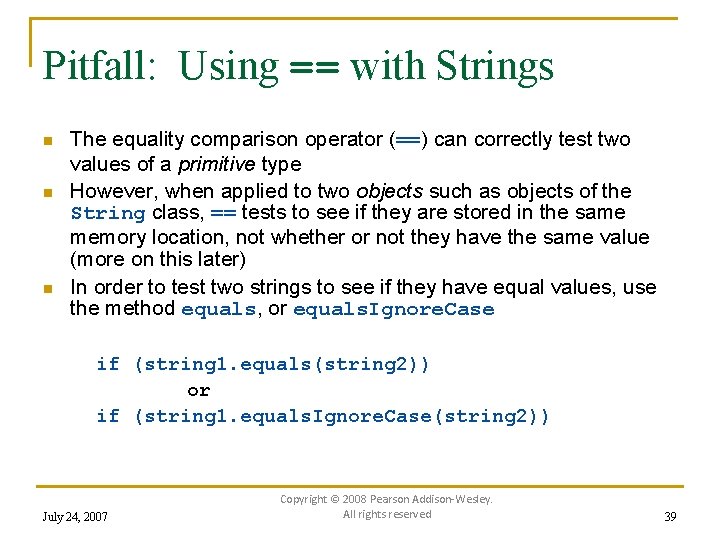
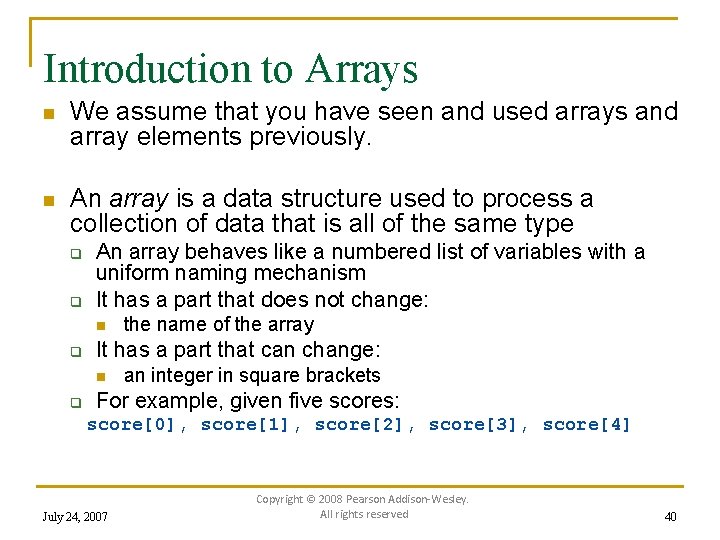
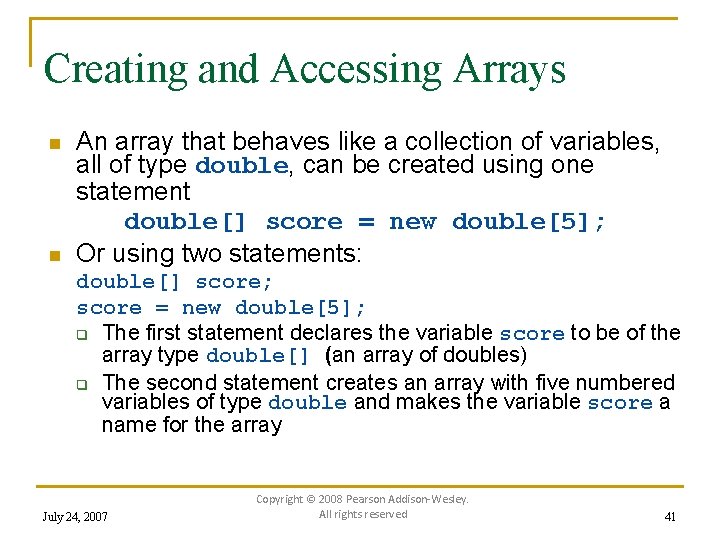
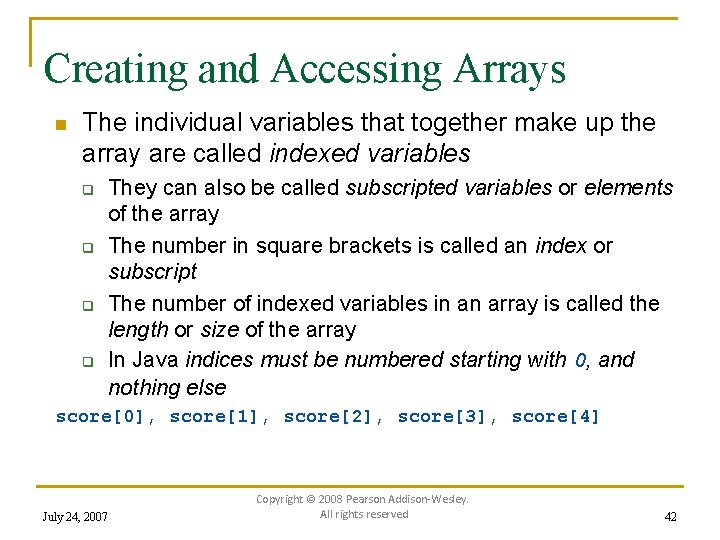
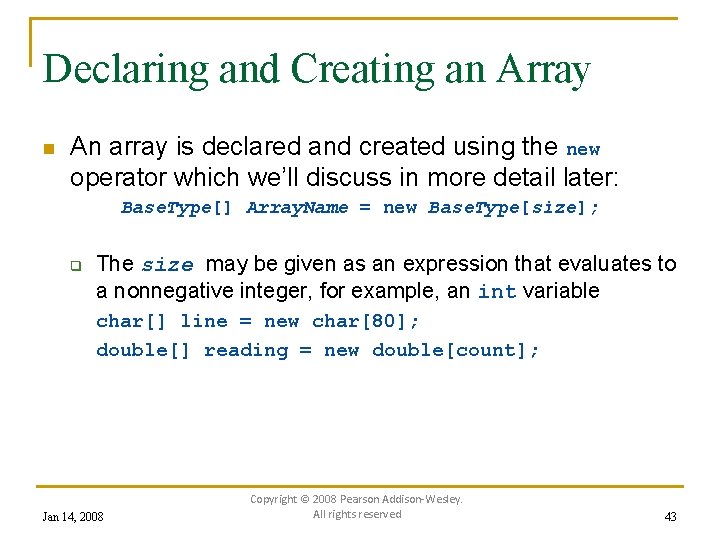
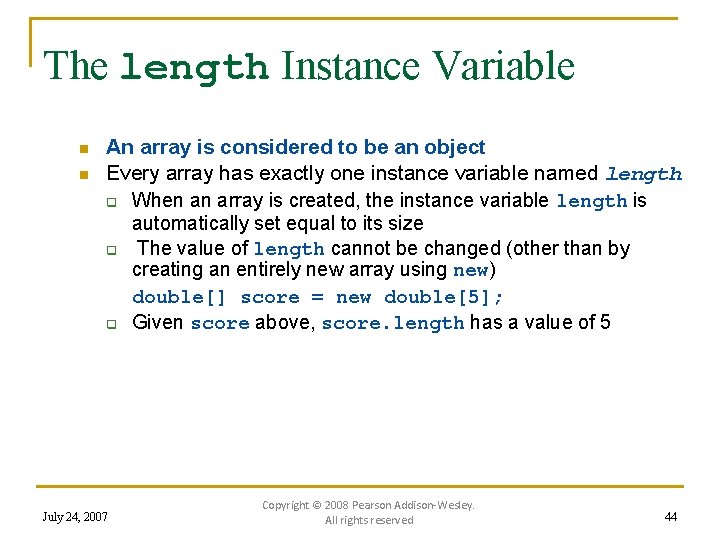
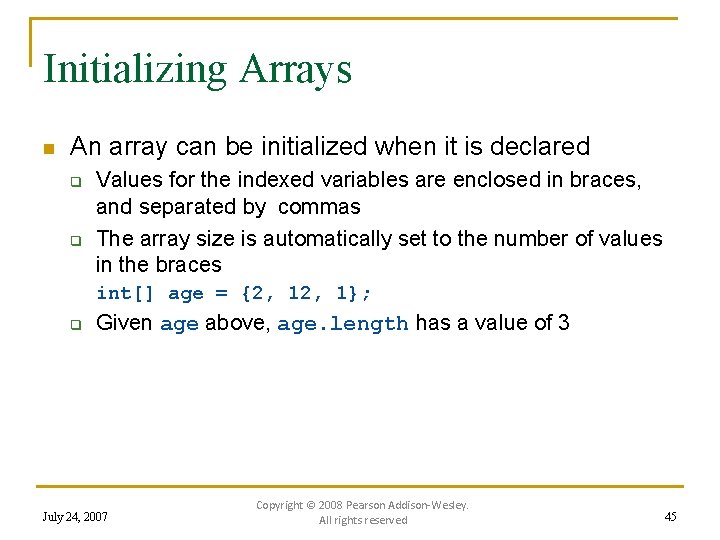
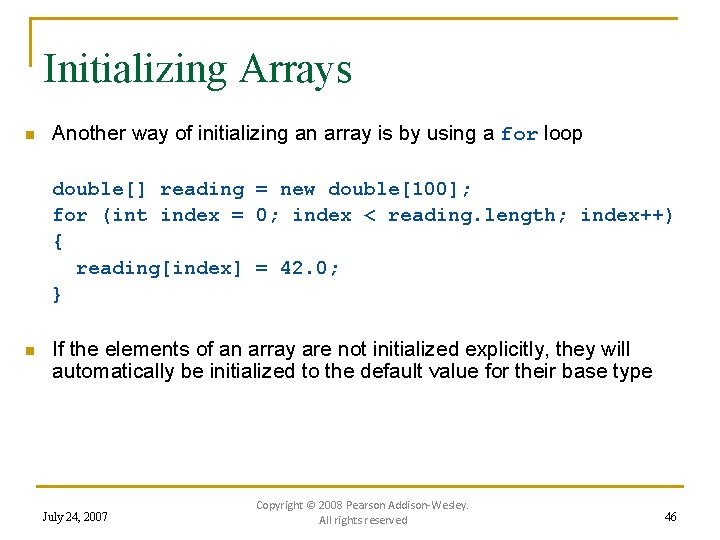
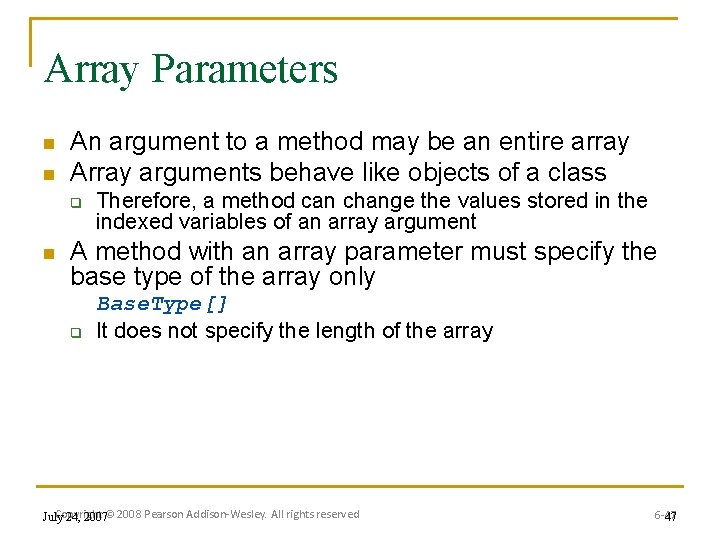
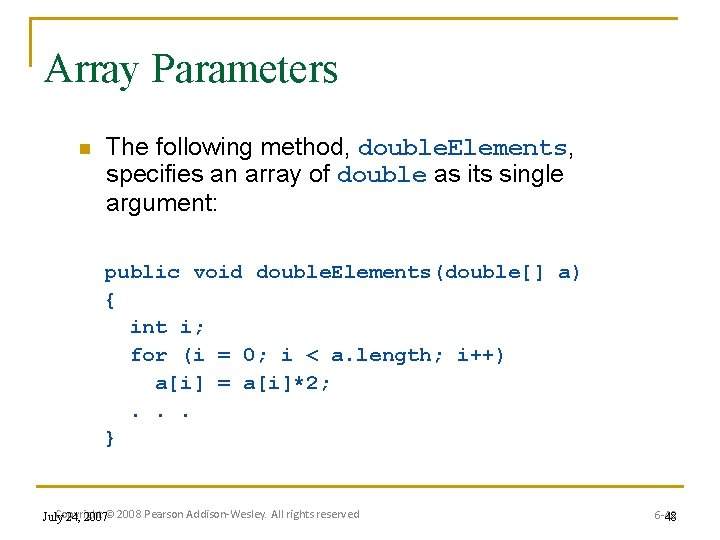
![Array Parameters n n Arrays of double may be defined as follows: double[] a Array Parameters n n Arrays of double may be defined as follows: double[] a](https://slidetodoc.com/presentation_image_h2/bba903afdd485962c17876a8e1e65ad9/image-49.jpg)
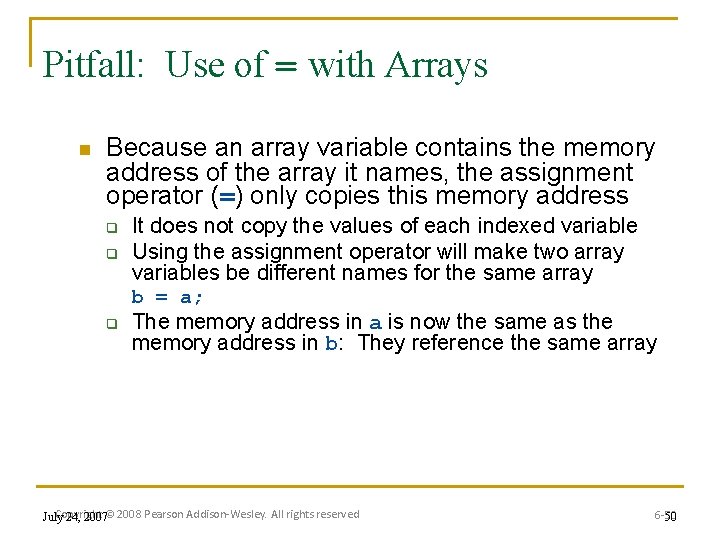
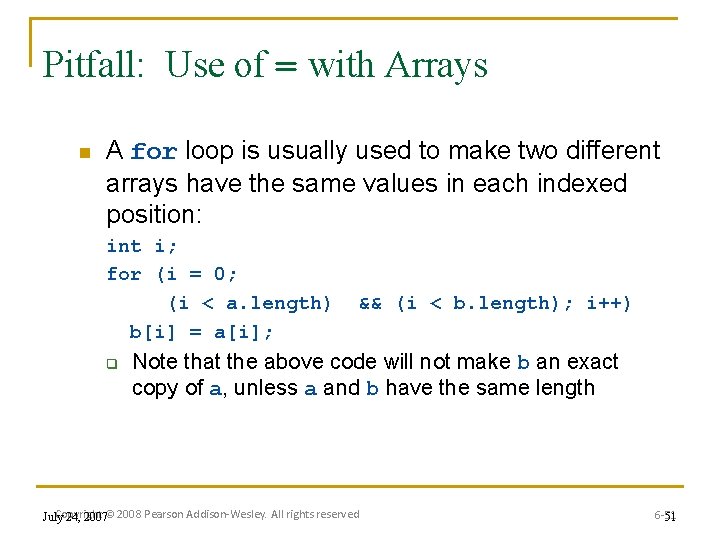
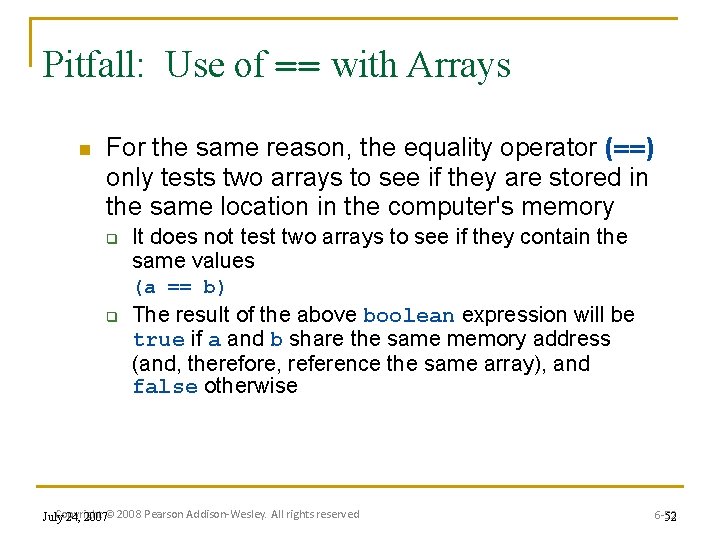
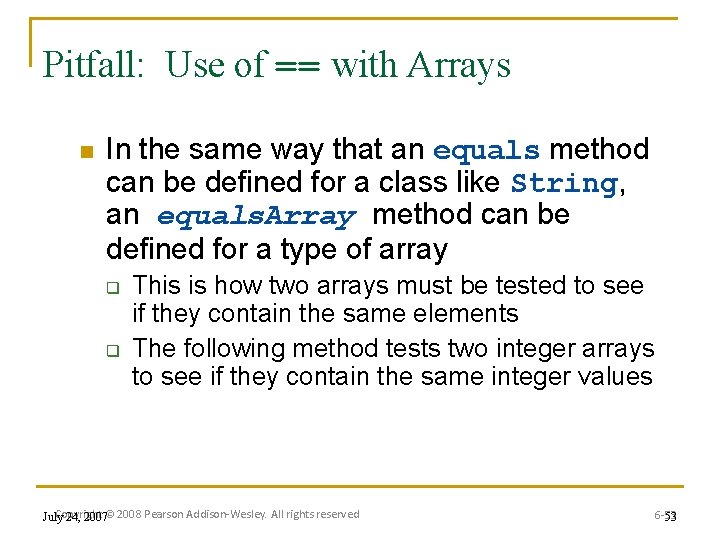
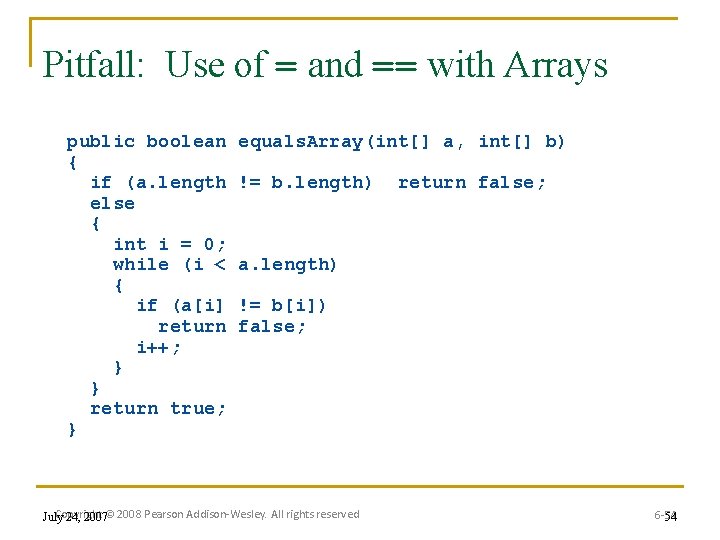
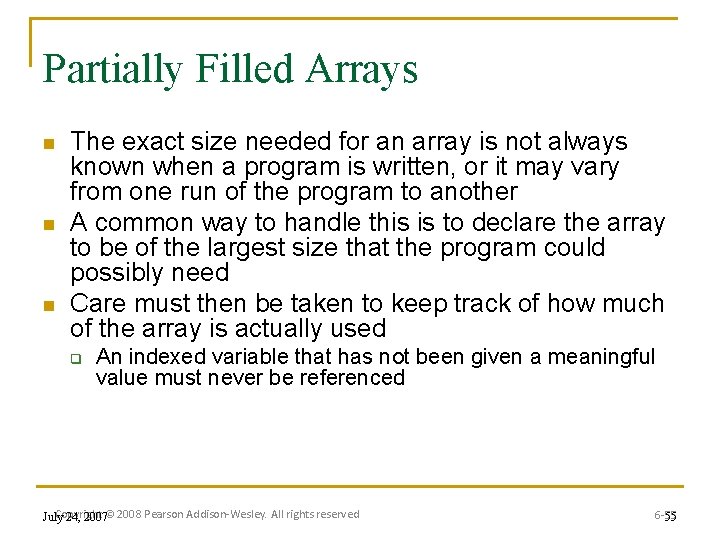
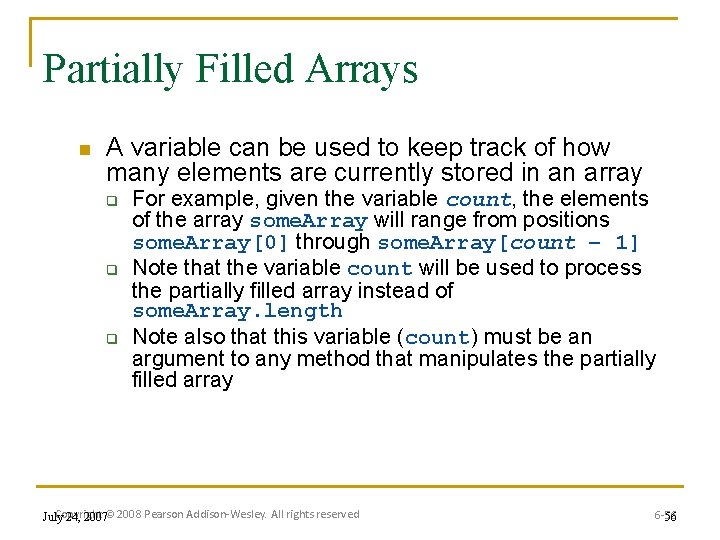
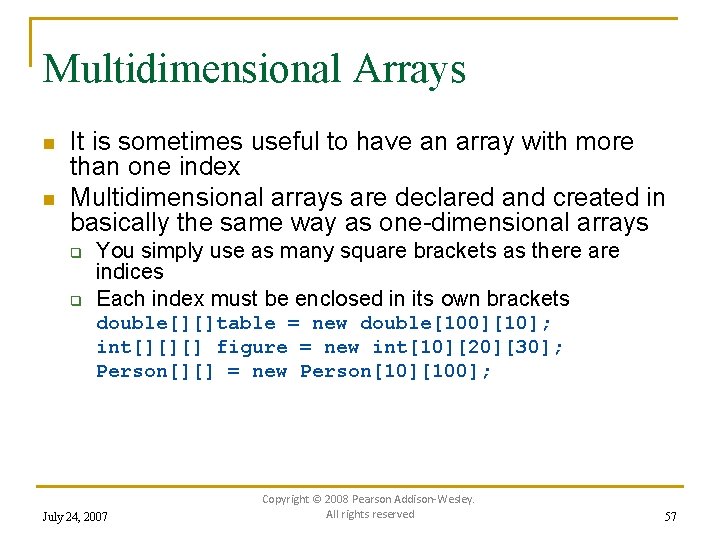
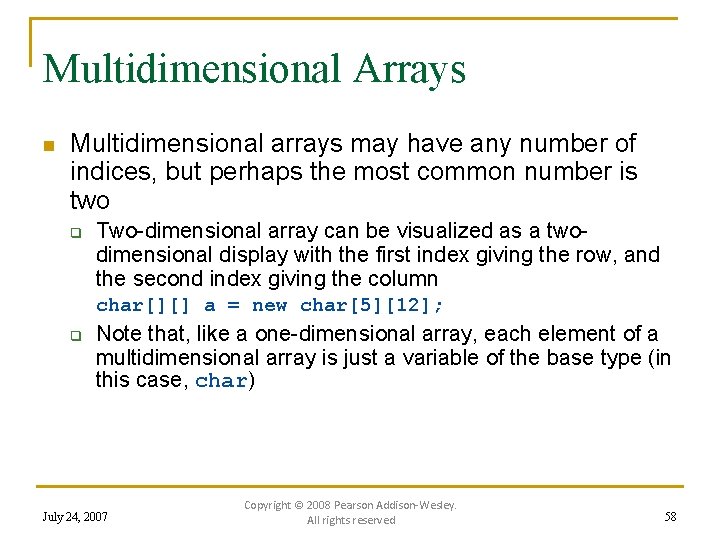
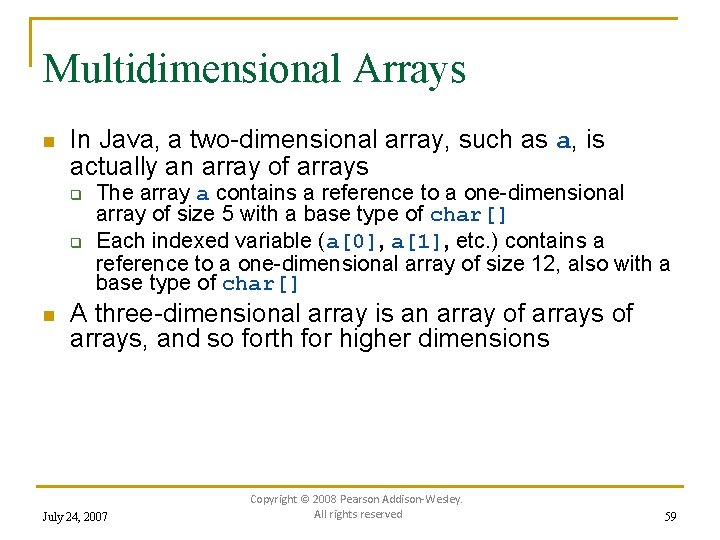
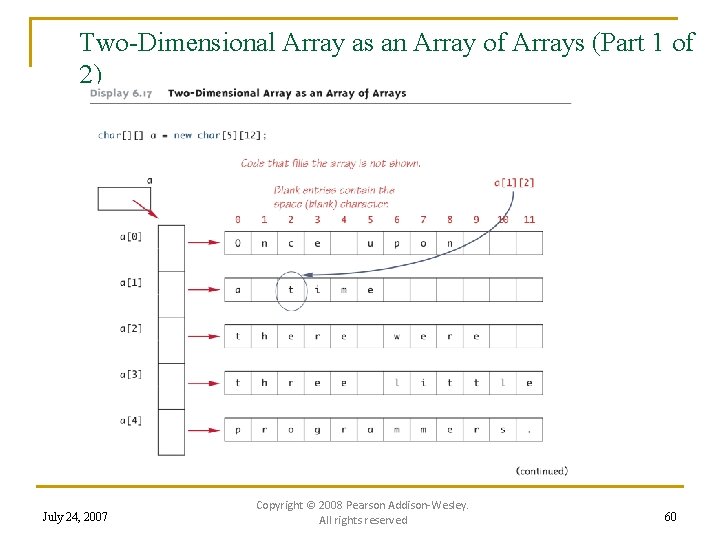
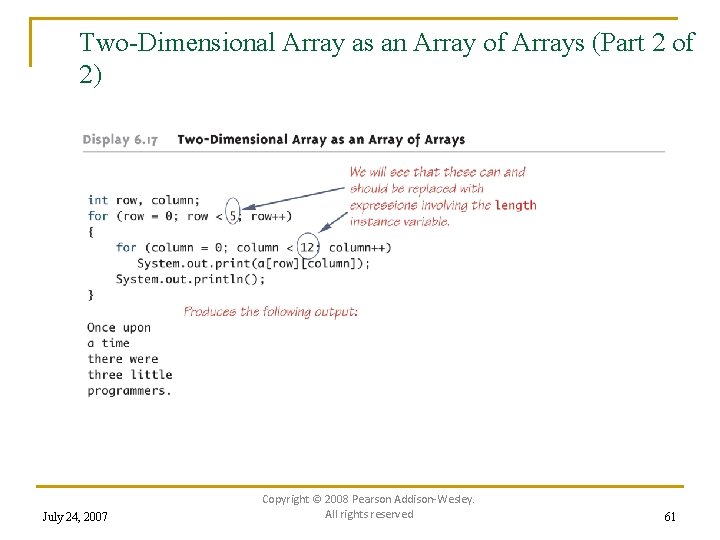
![Using the length Instance Variable char[][] page = new char[30][100]; n The instance variable Using the length Instance Variable char[][] page = new char[30][100]; n The instance variable](https://slidetodoc.com/presentation_image_h2/bba903afdd485962c17876a8e1e65ad9/image-62.jpg)
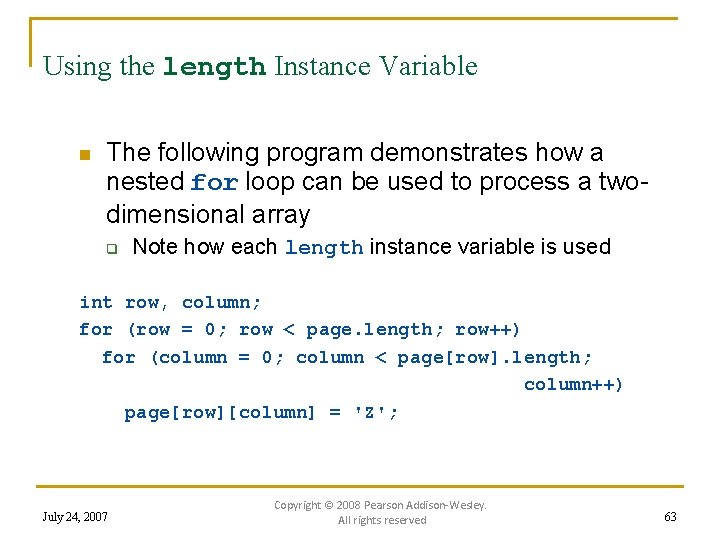
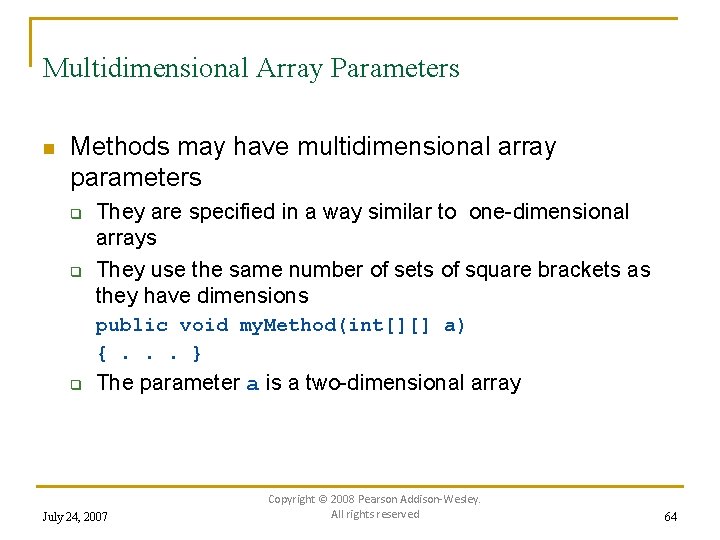
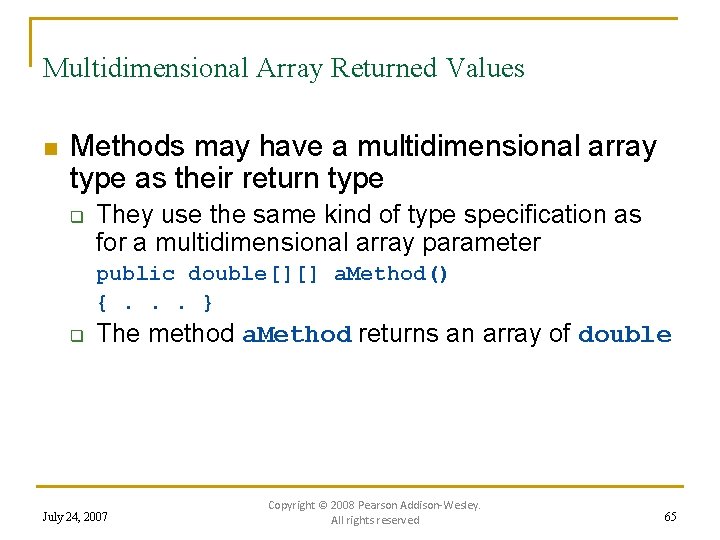
- Slides: 65
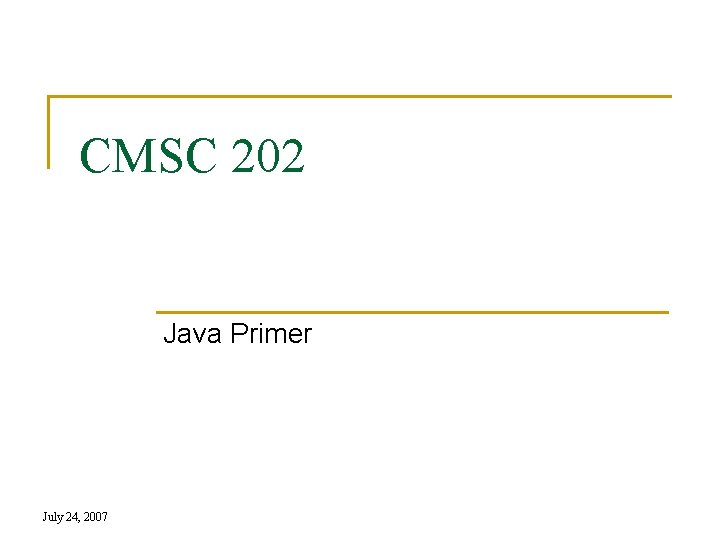
CMSC 202 Java Primer July 24, 2007
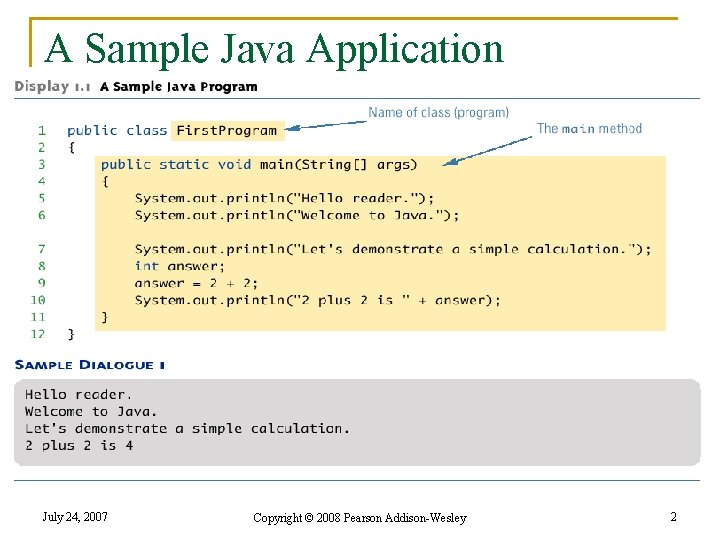
A Sample Java Application July 24, 2007 Copyright © 2008 Pearson Addison-Wesley 2
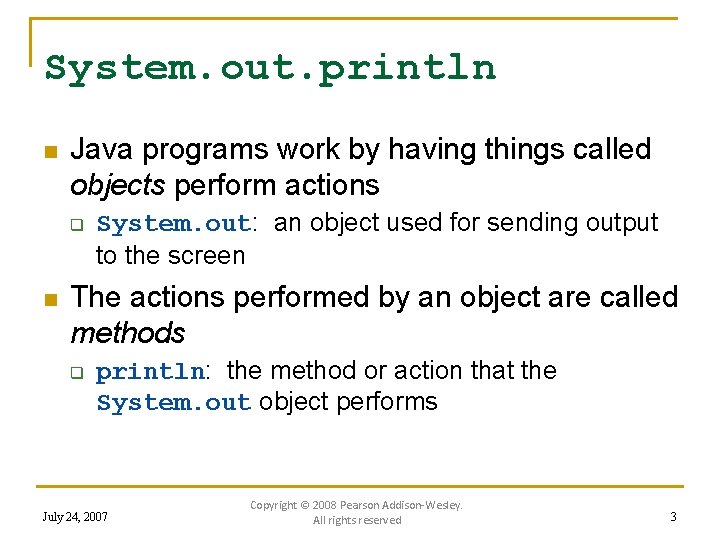
System. out. println n Java programs work by having things called objects perform actions q n System. out: an object used for sending output to the screen The actions performed by an object are called methods q println: the method or action that the System. out object performs July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 3
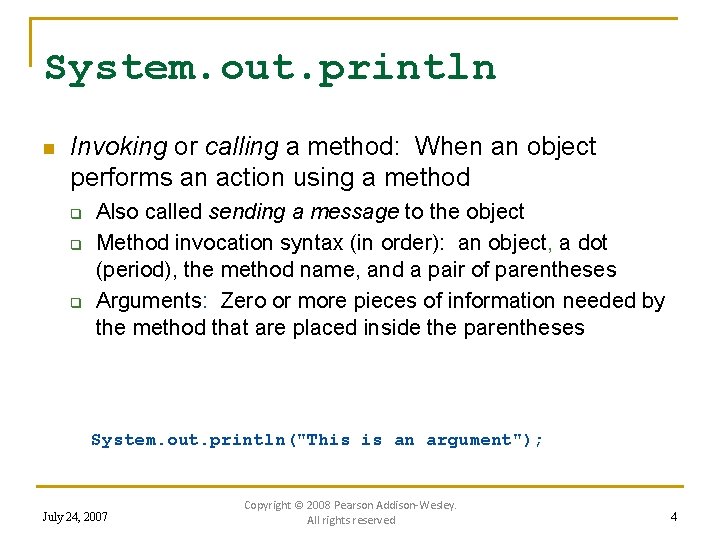
System. out. println n Invoking or calling a method: When an object performs an action using a method q q q Also called sending a message to the object Method invocation syntax (in order): an object, a dot (period), the method name, and a pair of parentheses Arguments: Zero or more pieces of information needed by the method that are placed inside the parentheses System. out. println("This is an argument"); July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 4
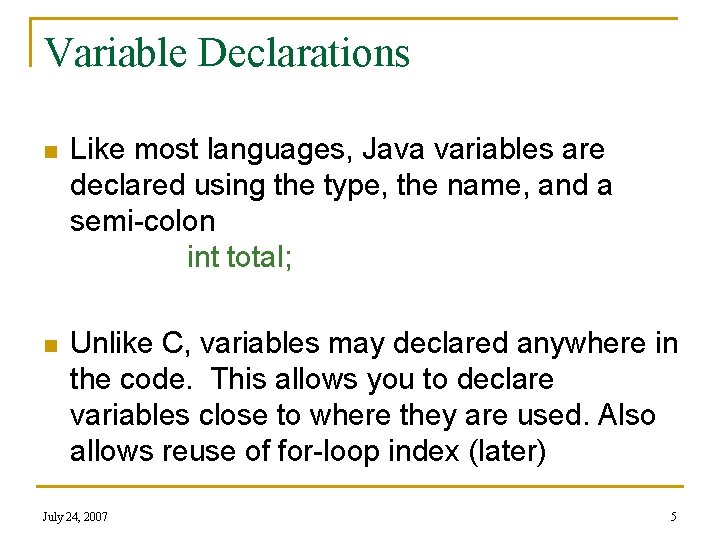
Variable Declarations n Like most languages, Java variables are declared using the type, the name, and a semi-colon int total; n Unlike C, variables may declared anywhere in the code. This allows you to declare variables close to where they are used. Also allows reuse of for-loop index (later) July 24, 2007 5
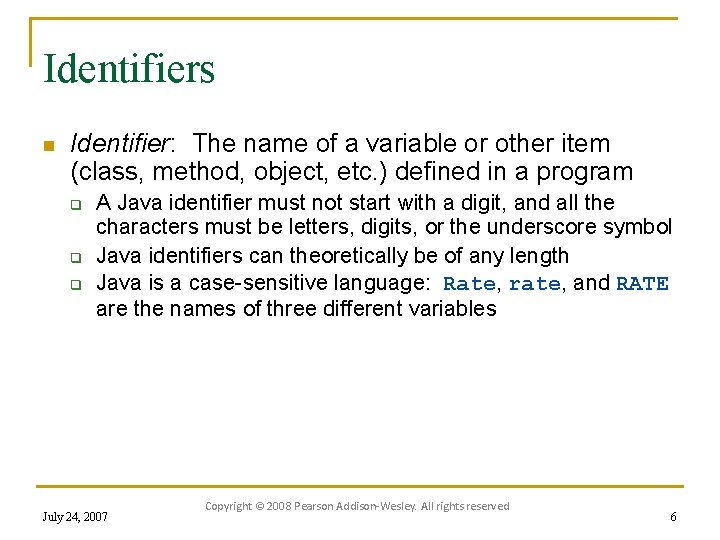
Identifiers n Identifier: The name of a variable or other item (class, method, object, etc. ) defined in a program q q q A Java identifier must not start with a digit, and all the characters must be letters, digits, or the underscore symbol Java identifiers can theoretically be of any length Java is a case-sensitive language: Rate, rate, and RATE are the names of three different variables July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 6
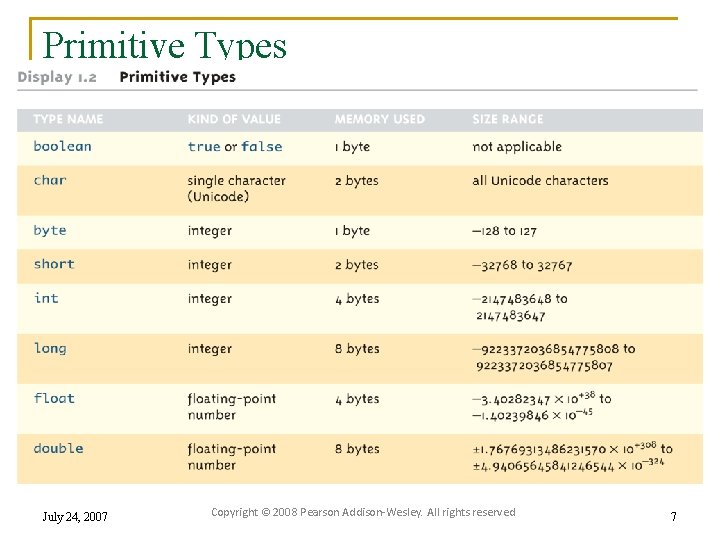
Primitive Types July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 7
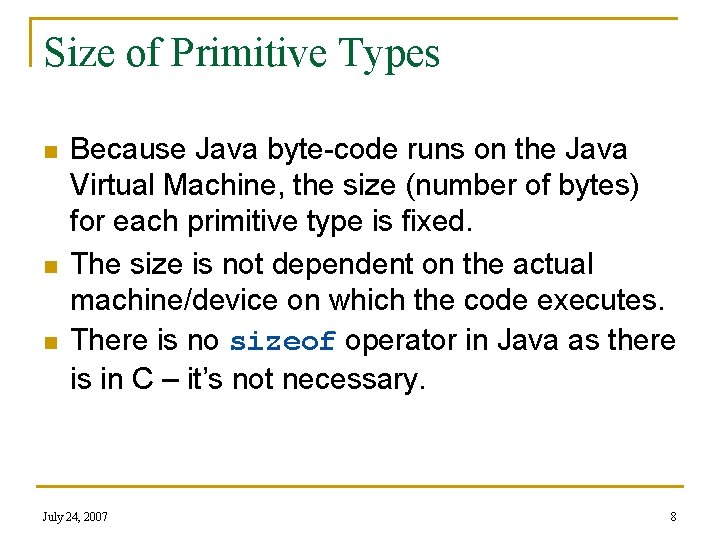
Size of Primitive Types n n n Because Java byte-code runs on the Java Virtual Machine, the size (number of bytes) for each primitive type is fixed. The size is not dependent on the actual machine/device on which the code executes. There is no sizeof operator in Java as there is in C – it’s not necessary. July 24, 2007 8
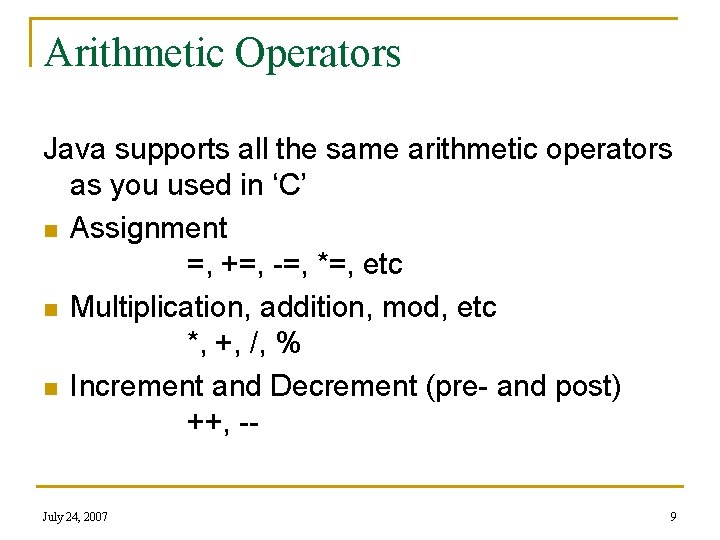
Arithmetic Operators Java supports all the same arithmetic operators as you used in ‘C’ n Assignment =, +=, -=, *=, etc n Multiplication, addition, mod, etc *, +, /, % n Increment and Decrement (pre- and post) ++, -July 24, 2007 9
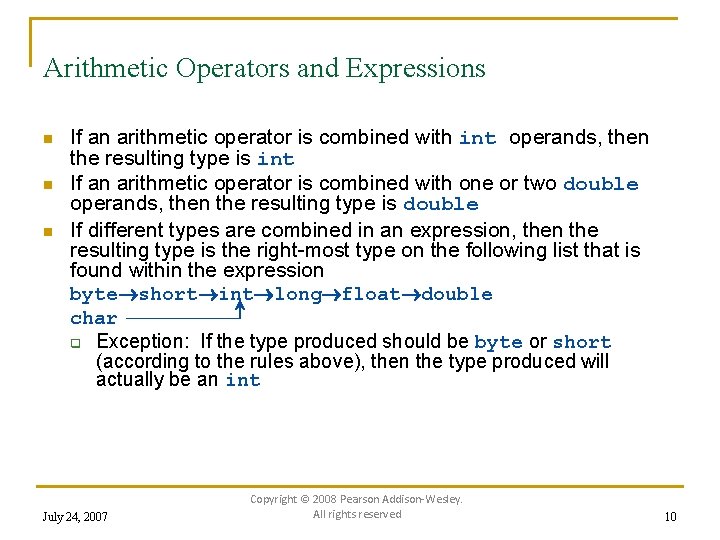
Arithmetic Operators and Expressions n n n If an arithmetic operator is combined with int operands, then the resulting type is int If an arithmetic operator is combined with one or two double operands, then the resulting type is double If different types are combined in an expression, then the resulting type is the right-most type on the following list that is found within the expression byte short int long float double char q Exception: If the type produced should be byte or short (according to the rules above), then the type produced will actually be an int July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 10
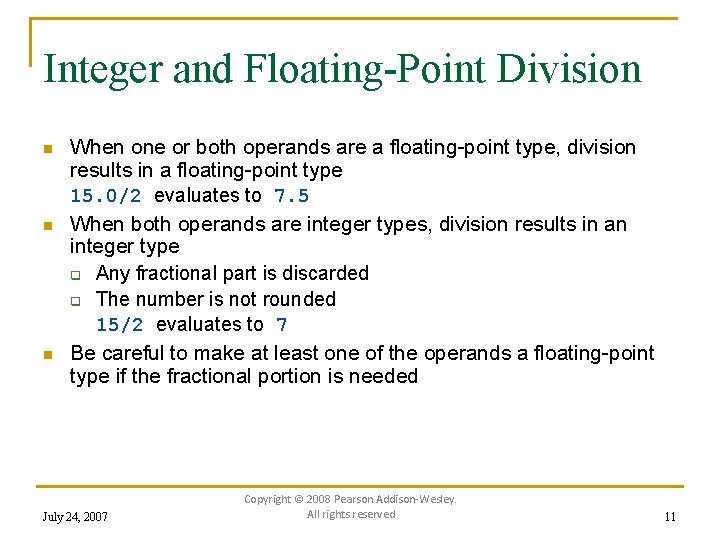
Integer and Floating-Point Division n When one or both operands are a floating-point type, division results in a floating-point type 15. 0/2 evaluates to 7. 5 When both operands are integer types, division results in an integer type q Any fractional part is discarded q The number is not rounded 15/2 evaluates to 7 Be careful to make at least one of the operands a floating-point type if the fractional portion is needed July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 11
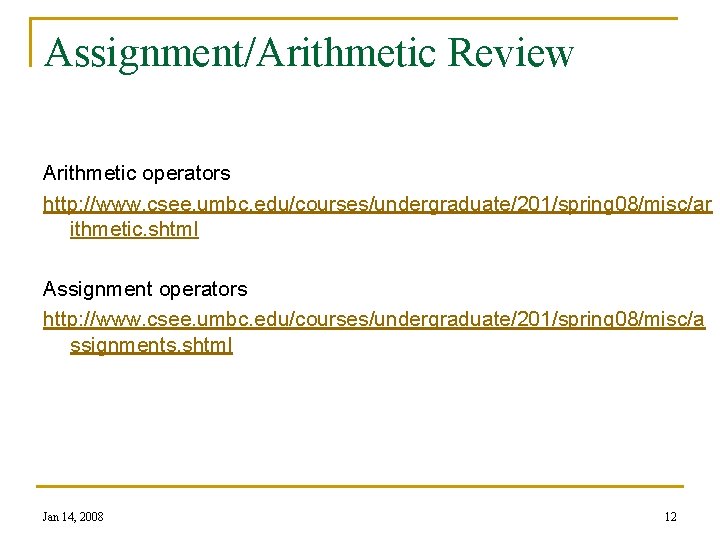
Assignment/Arithmetic Review Arithmetic operators http: //www. csee. umbc. edu/courses/undergraduate/201/spring 08/misc/ar ithmetic. shtml Assignment operators http: //www. csee. umbc. edu/courses/undergraduate/201/spring 08/misc/a ssignments. shtml Jan 14, 2008 12
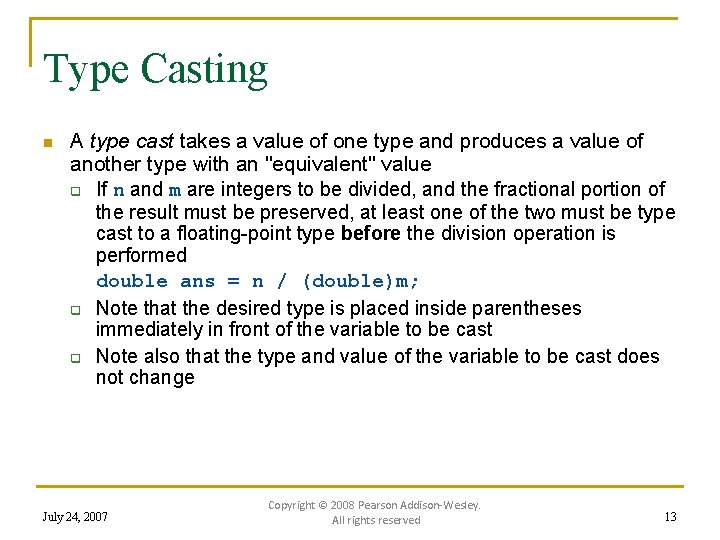
Type Casting n A type cast takes a value of one type and produces a value of another type with an "equivalent" value q If n and m are integers to be divided, and the fractional portion of the result must be preserved, at least one of the two must be type cast to a floating-point type before the division operation is performed double ans = n / (double)m; q Note that the desired type is placed inside parentheses immediately in front of the variable to be cast q Note also that the type and value of the variable to be cast does not change July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 13
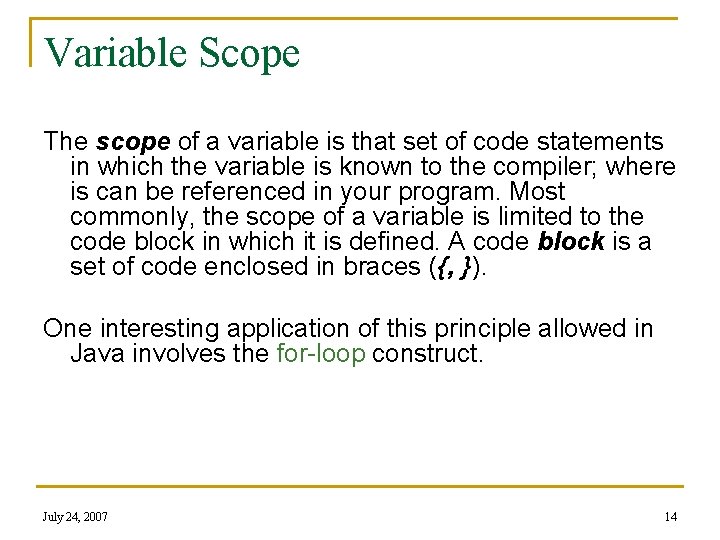
Variable Scope The scope of a variable is that set of code statements in which the variable is known to the compiler; where is can be referenced in your program. Most commonly, the scope of a variable is limited to the code block in which it is defined. A code block is a set of code enclosed in braces ({, }). One interesting application of this principle allowed in Java involves the for-loop construct. July 24, 2007 14
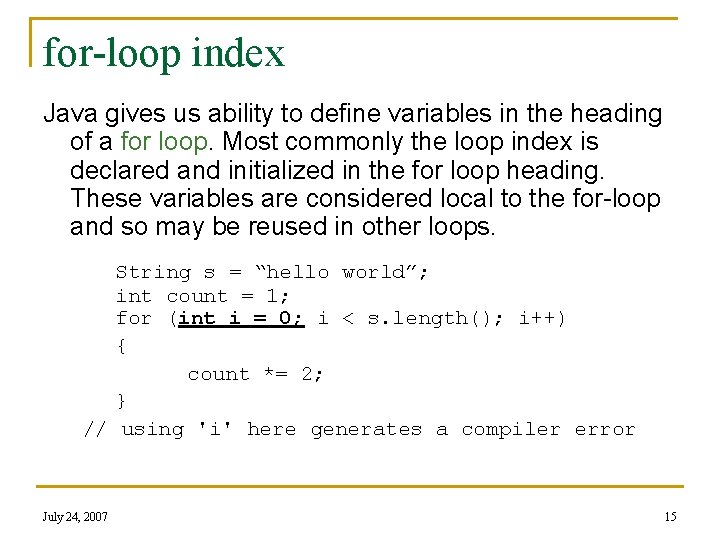
for-loop index Java gives us ability to define variables in the heading of a for loop. Most commonly the loop index is declared and initialized in the for loop heading. These variables are considered local to the for-loop and so may be reused in other loops. String s = “hello world”; int count = 1; for (int i = 0; i < s. length(); i++) { count *= 2; } // using 'i' here generates a compiler error July 24, 2007 15
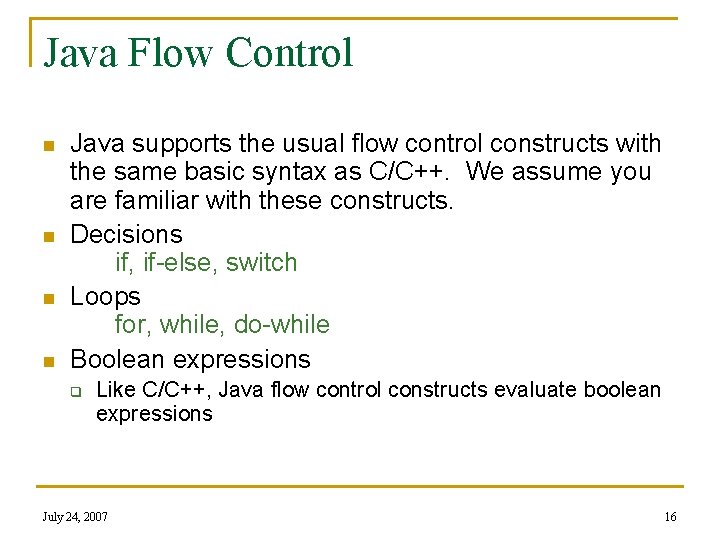
Java Flow Control n n Java supports the usual flow control constructs with the same basic syntax as C/C++. We assume you are familiar with these constructs. Decisions if, if-else, switch Loops for, while, do-while Boolean expressions q Like C/C++, Java flow control constructs evaluate boolean expressions July 24, 2007 16
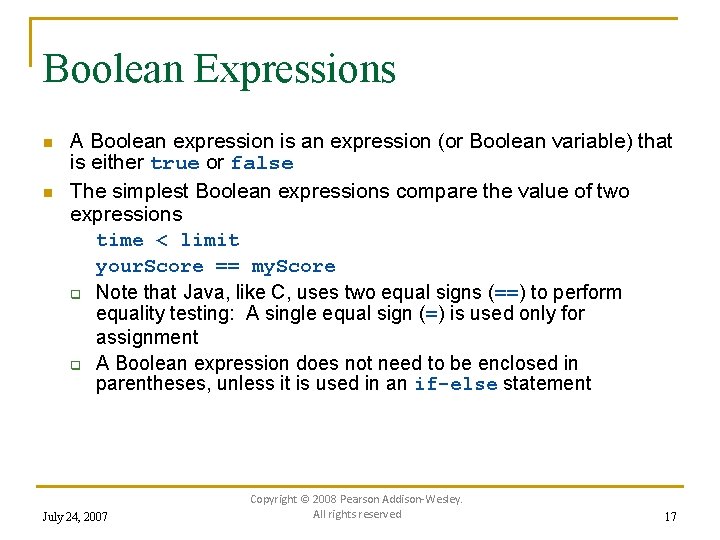
Boolean Expressions n n A Boolean expression is an expression (or Boolean variable) that is either true or false The simplest Boolean expressions compare the value of two expressions time < limit your. Score == my. Score q Note that Java, like C, uses two equal signs (==) to perform equality testing: A single equal sign (=) is used only for assignment q A Boolean expression does not need to be enclosed in parentheses, unless it is used in an if-else statement July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 17
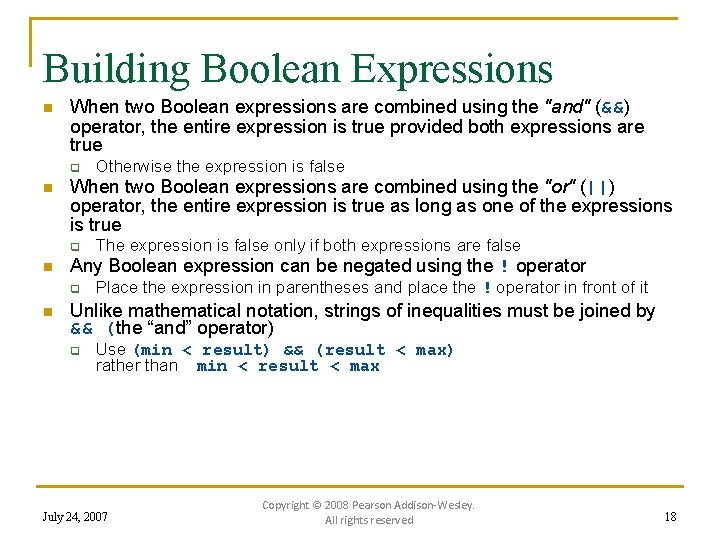
Building Boolean Expressions n When two Boolean expressions are combined using the "and" (&&) operator, the entire expression is true provided both expressions are true q n When two Boolean expressions are combined using the "or" (||) operator, the entire expression is true as long as one of the expressions is true q n The expression is false only if both expressions are false Any Boolean expression can be negated using the ! operator q n Otherwise the expression is false Place the expression in parentheses and place the ! operator in front of it Unlike mathematical notation, strings of inequalities must be joined by && (the “and” operator) q Use (min < result) && (result < max) rather than min < result < max July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 18
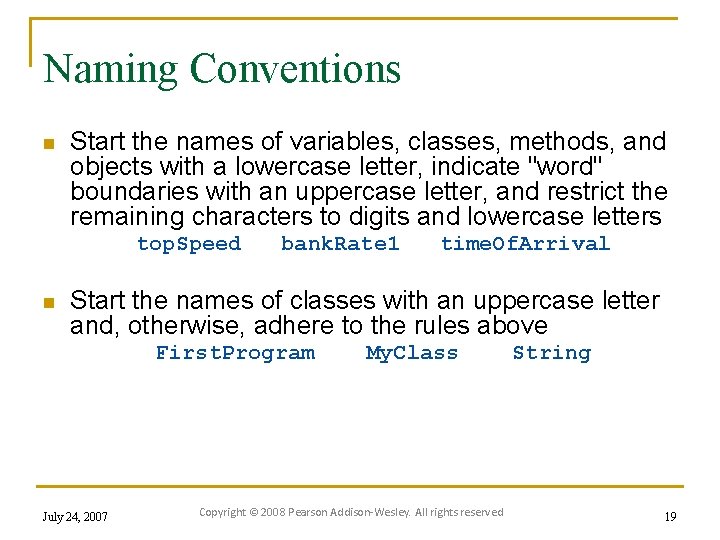
Naming Conventions n Start the names of variables, classes, methods, and objects with a lowercase letter, indicate "word" boundaries with an uppercase letter, and restrict the remaining characters to digits and lowercase letters top. Speed n bank. Rate 1 time. Of. Arrival Start the names of classes with an uppercase letter and, otherwise, adhere to the rules above First. Program July 24, 2007 My. Class Copyright © 2008 Pearson Addison-Wesley. All rights reserved String 19
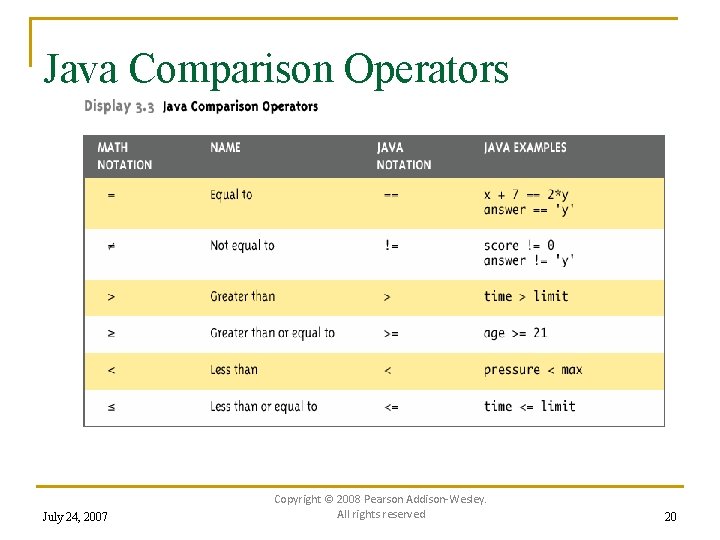
Java Comparison Operators July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 20
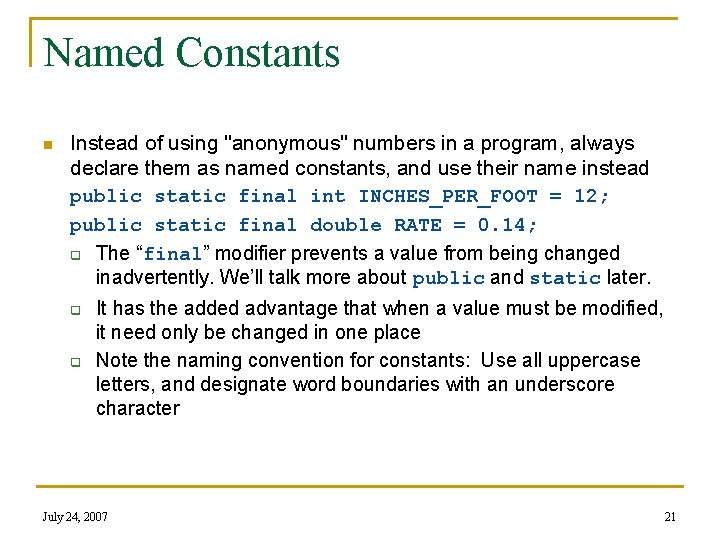
Named Constants n Instead of using "anonymous" numbers in a program, always declare them as named constants, and use their name instead public static final int INCHES_PER_FOOT = 12; public static final double RATE = 0. 14; q The “final” modifier prevents a value from being changed inadvertently. We’ll talk more about public and static later. q It has the added advantage that when a value must be modified, it need only be changed in one place q Note the naming convention for constants: Use all uppercase letters, and designate word boundaries with an underscore character July 24, 2007 21
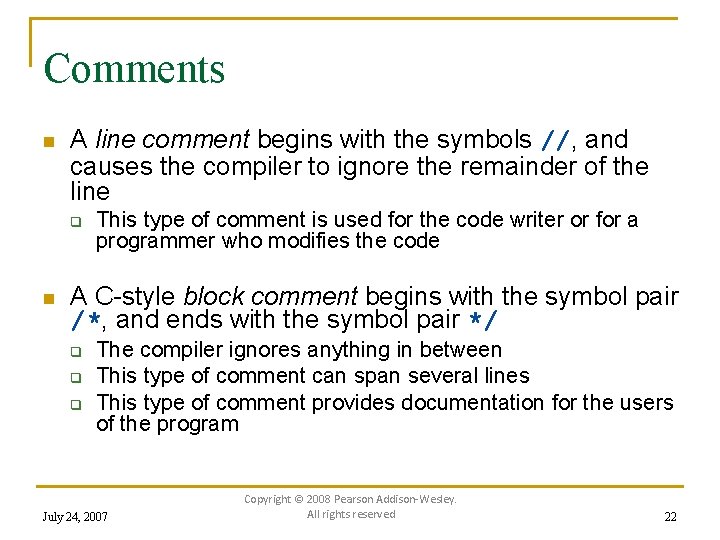
Comments n A line comment begins with the symbols //, and causes the compiler to ignore the remainder of the line q n This type of comment is used for the code writer or for a programmer who modifies the code A C-style block comment begins with the symbol pair /*, and ends with the symbol pair */ q q q The compiler ignores anything in between This type of comment can span several lines This type of comment provides documentation for the users of the program July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 22
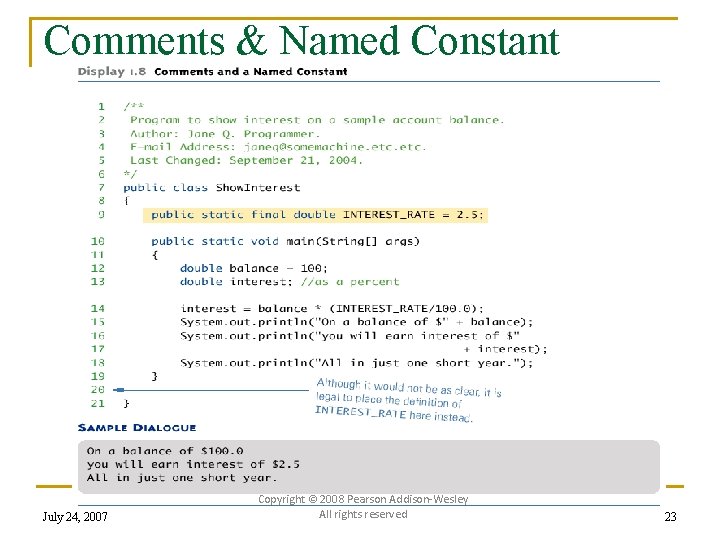
Comments & Named Constant July 24, 2007 Copyright © 2008 Pearson Addison-Wesley All rights reserved 23
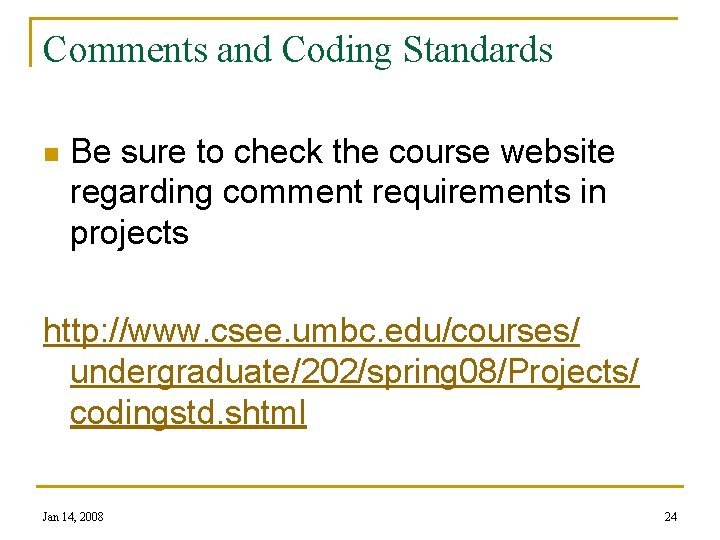
Comments and Coding Standards n Be sure to check the course website regarding comment requirements in projects http: //www. csee. umbc. edu/courses/ undergraduate/202/spring 08/Projects/ codingstd. shtml Jan 14, 2008 24
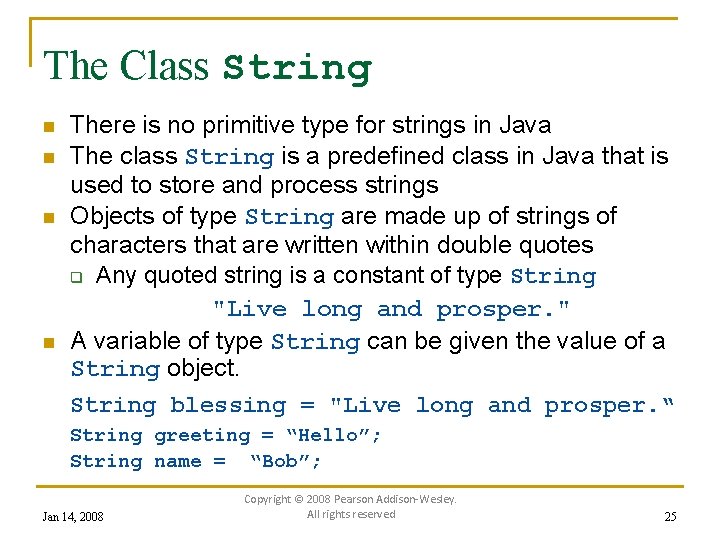
The Class String n n There is no primitive type for strings in Java The class String is a predefined class in Java that is used to store and process strings Objects of type String are made up of strings of characters that are written within double quotes q Any quoted string is a constant of type String "Live long and prosper. " A variable of type String can be given the value of a String object. String blessing = "Live long and prosper. “ String greeting = “Hello”; String name = “Bob”; Jan 14, 2008 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 25
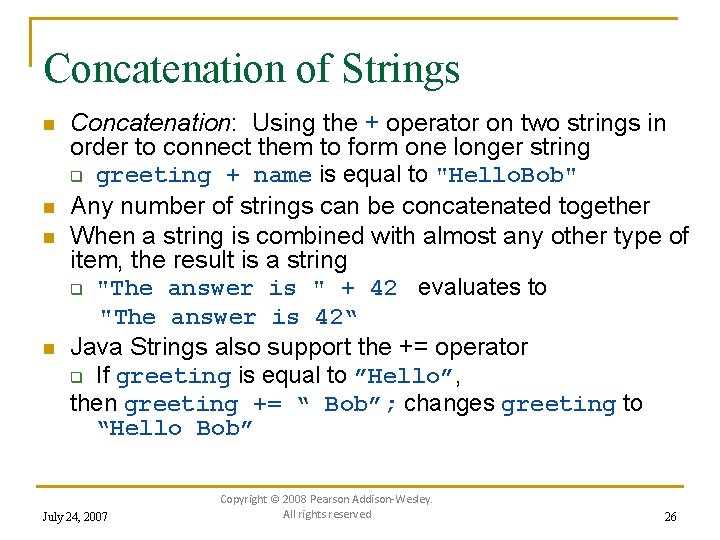
Concatenation of Strings n n Concatenation: Using the + operator on two strings in order to connect them to form one longer string q greeting + name is equal to "Hello. Bob" Any number of strings can be concatenated together When a string is combined with almost any other type of item, the result is a string q "The answer is " + 42 evaluates to "The answer is 42“ Java Strings also support the += operator q If greeting is equal to ”Hello”, then greeting += “ Bob”; changes greeting to “Hello Bob” July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 26
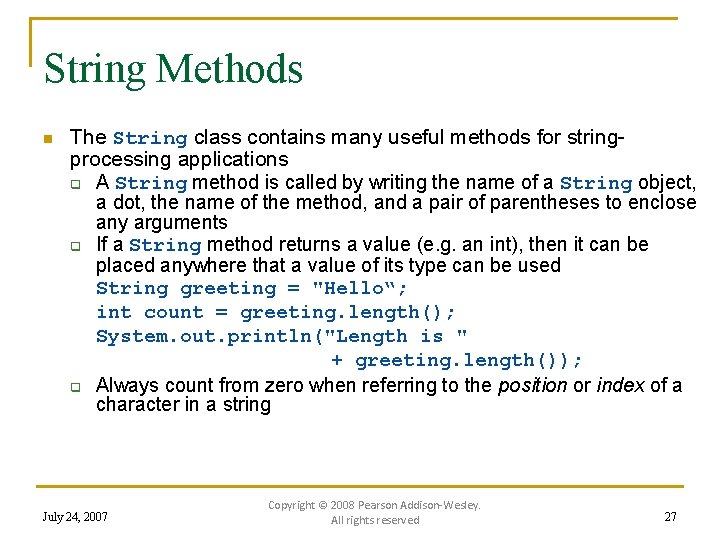
String Methods n The String class contains many useful methods for stringprocessing applications q A String method is called by writing the name of a String object, a dot, the name of the method, and a pair of parentheses to enclose any arguments q If a String method returns a value (e. g. an int), then it can be placed anywhere that a value of its type can be used String greeting = "Hello“; int count = greeting. length(); System. out. println("Length is " + greeting. length()); q Always count from zero when referring to the position or index of a character in a string July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 27
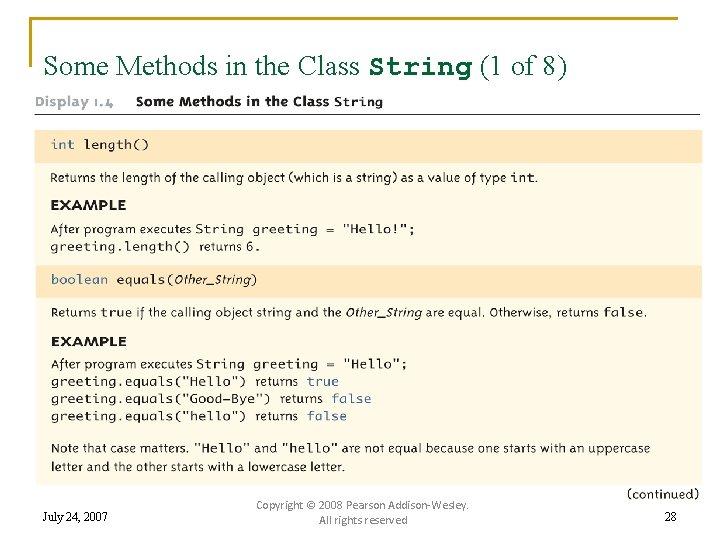
Some Methods in the Class String (1 of 8) July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 28
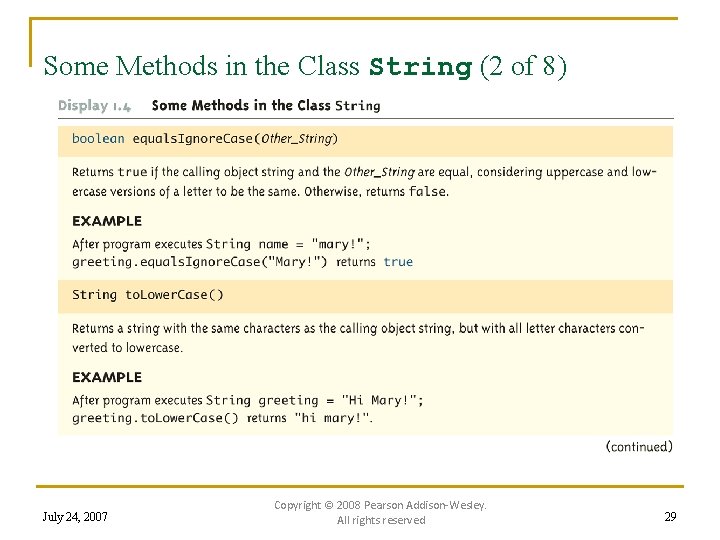
Some Methods in the Class String (2 of 8) July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 29
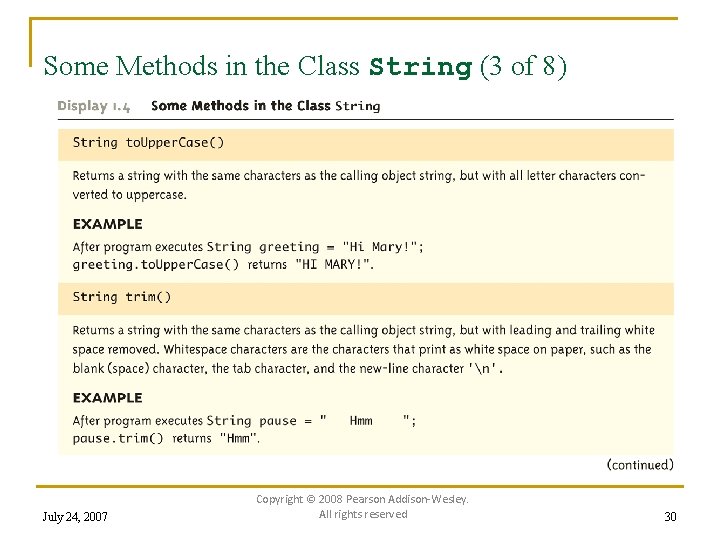
Some Methods in the Class String (3 of 8) July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 30
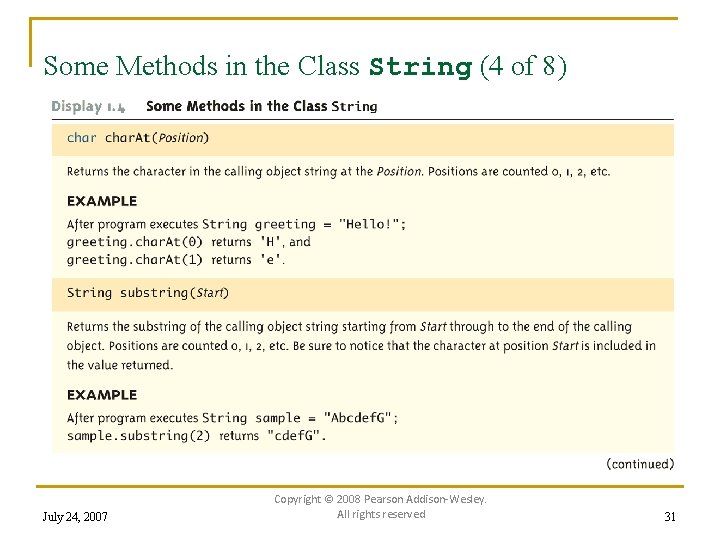
Some Methods in the Class String (4 of 8) July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 31
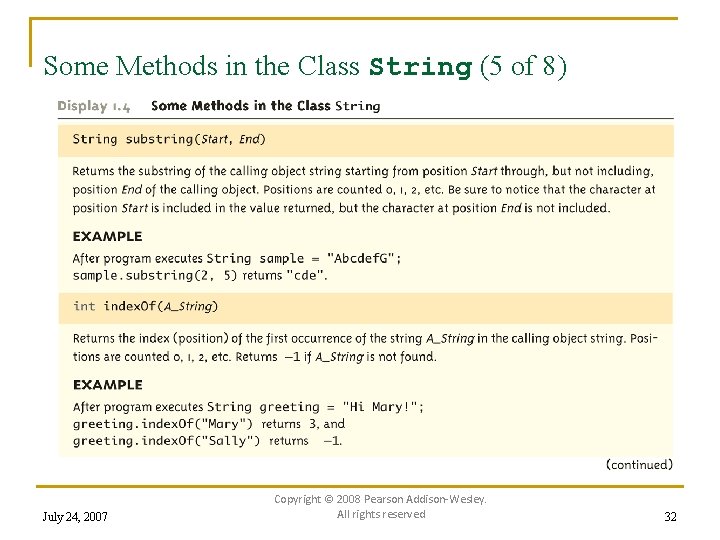
Some Methods in the Class String (5 of 8) July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 32
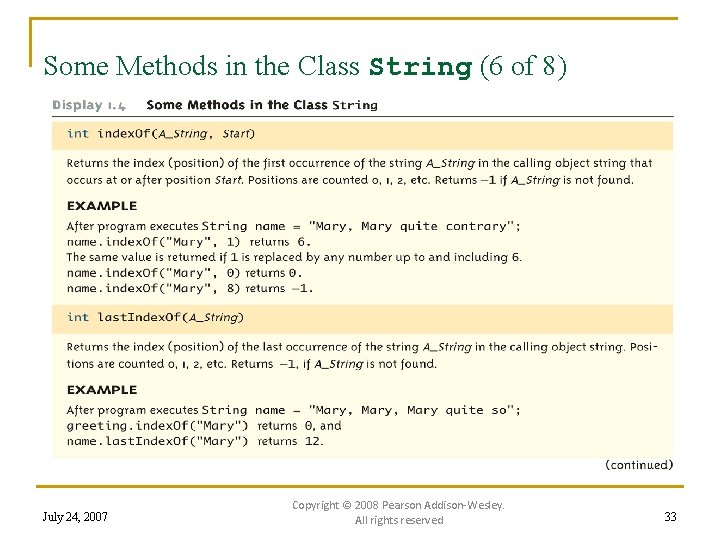
Some Methods in the Class String (6 of 8) July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 33
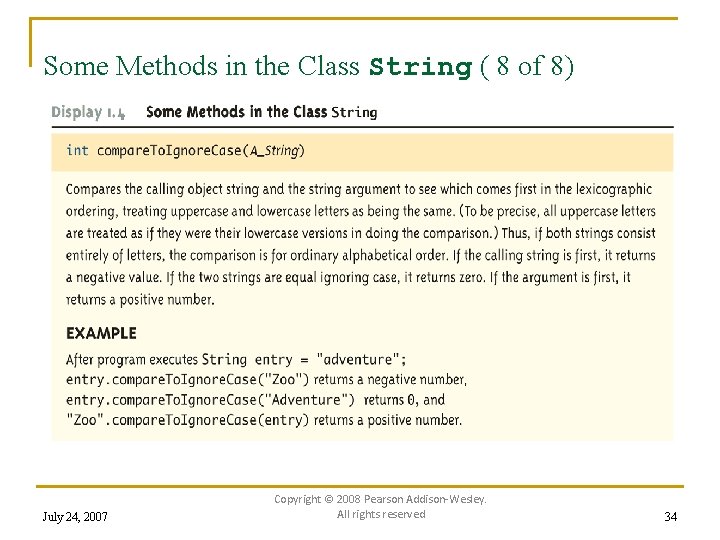
Some Methods in the Class String ( 8 of 8) July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 34
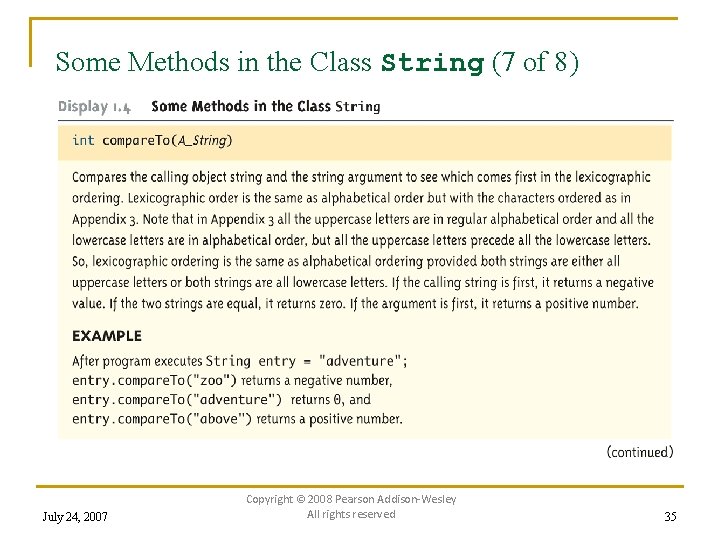
Some Methods in the Class String (7 of 8) July 24, 2007 Copyright © 2008 Pearson Addison-Wesley All rights reserved 35
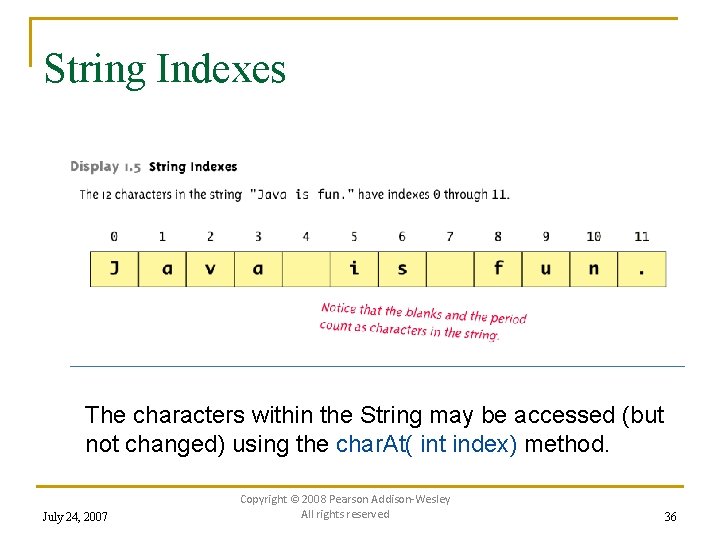
String Indexes The characters within the String may be accessed (but not changed) using the char. At( int index) method. July 24, 2007 Copyright © 2008 Pearson Addison-Wesley All rights reserved 36
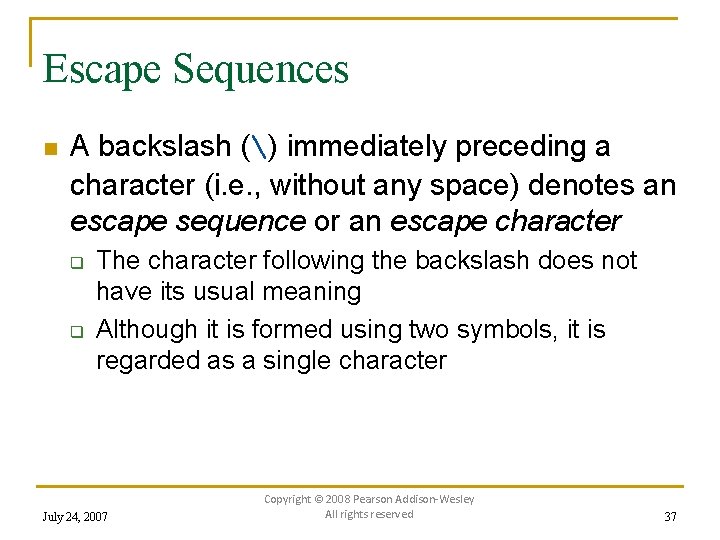
Escape Sequences n A backslash () immediately preceding a character (i. e. , without any space) denotes an escape sequence or an escape character q q The character following the backslash does not have its usual meaning Although it is formed using two symbols, it is regarded as a single character July 24, 2007 Copyright © 2008 Pearson Addison-Wesley All rights reserved 37
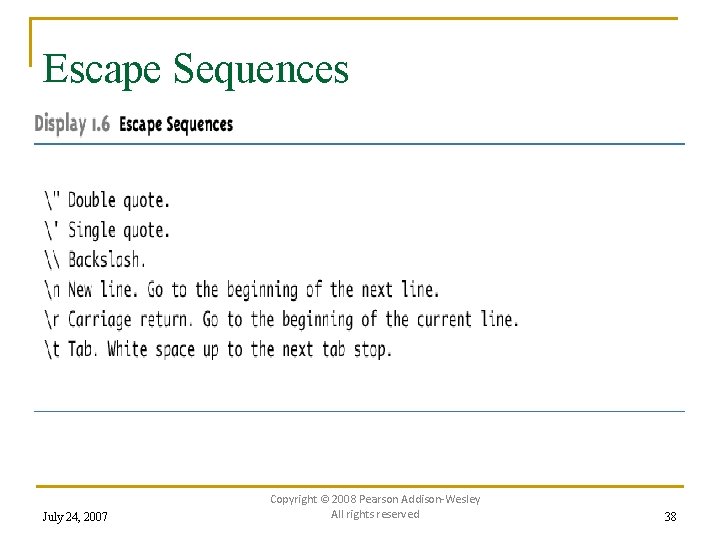
Escape Sequences July 24, 2007 Copyright © 2008 Pearson Addison-Wesley All rights reserved 38
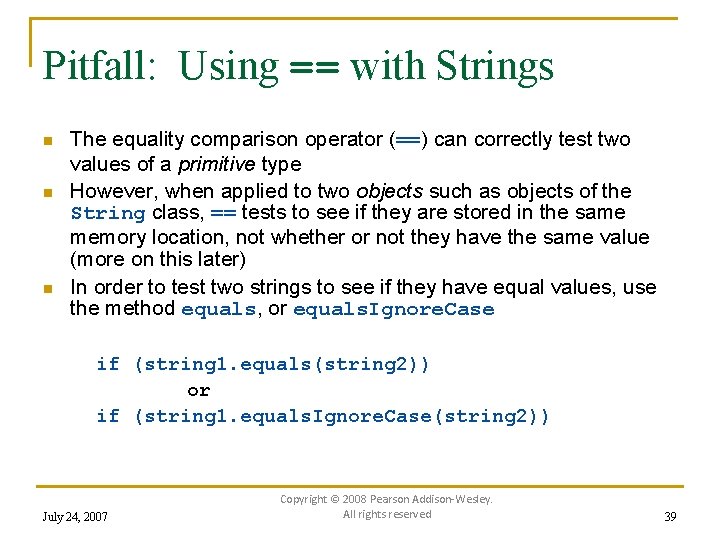
Pitfall: Using == with Strings n n n The equality comparison operator (==) can correctly test two values of a primitive type However, when applied to two objects such as objects of the String class, == tests to see if they are stored in the same memory location, not whether or not they have the same value (more on this later) In order to test two strings to see if they have equal values, use the method equals, or equals. Ignore. Case if (string 1. equals(string 2)) or if (string 1. equals. Ignore. Case(string 2)) July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 39
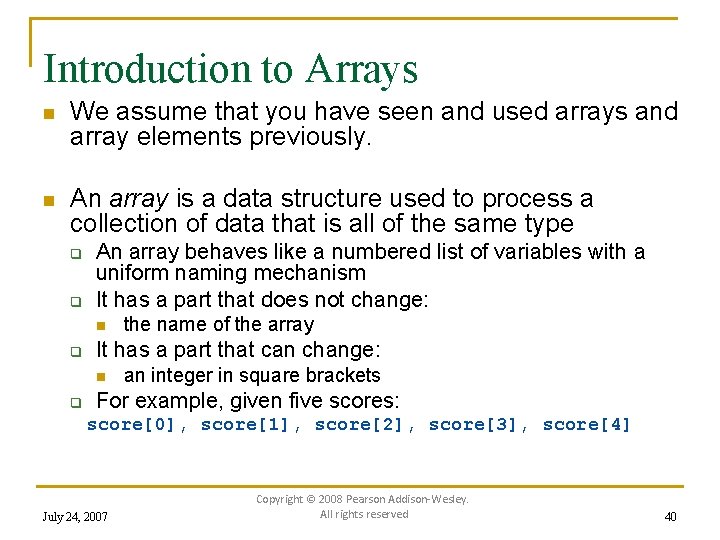
Introduction to Arrays n We assume that you have seen and used arrays and array elements previously. n An array is a data structure used to process a collection of data that is all of the same type q q An array behaves like a numbered list of variables with a uniform naming mechanism It has a part that does not change: n q It has a part that can change: n q the name of the array an integer in square brackets For example, given five scores: score[0], score[1], score[2], score[3], score[4] July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 40
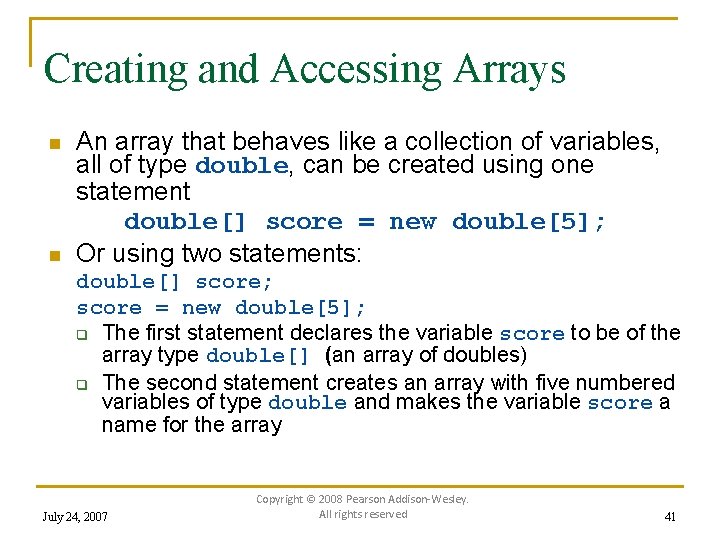
Creating and Accessing Arrays n n An array that behaves like a collection of variables, all of type double, can be created using one statement double[] score = new double[5]; Or using two statements: double[] score; score = new double[5]; q The first statement declares the variable score to be of the array type double[] (an array of doubles) q The second statement creates an array with five numbered variables of type double and makes the variable score a name for the array July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 41
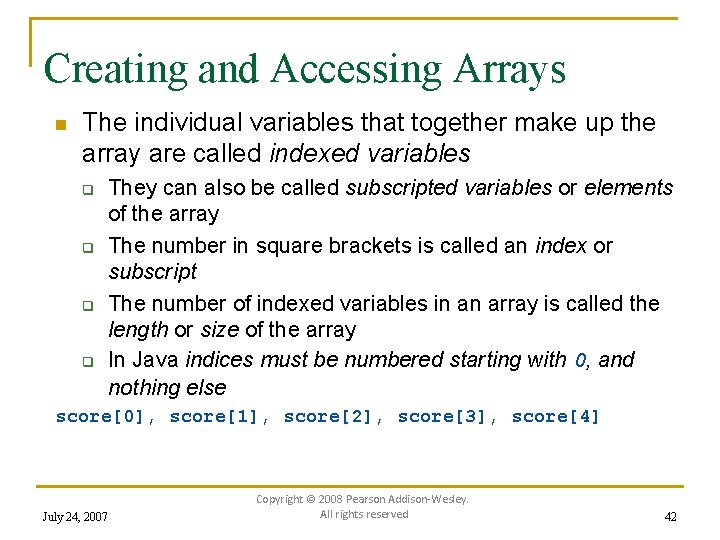
Creating and Accessing Arrays n The individual variables that together make up the array are called indexed variables q q They can also be called subscripted variables or elements of the array The number in square brackets is called an index or subscript The number of indexed variables in an array is called the length or size of the array In Java indices must be numbered starting with 0, and nothing else score[0], score[1], score[2], score[3], score[4] July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 42
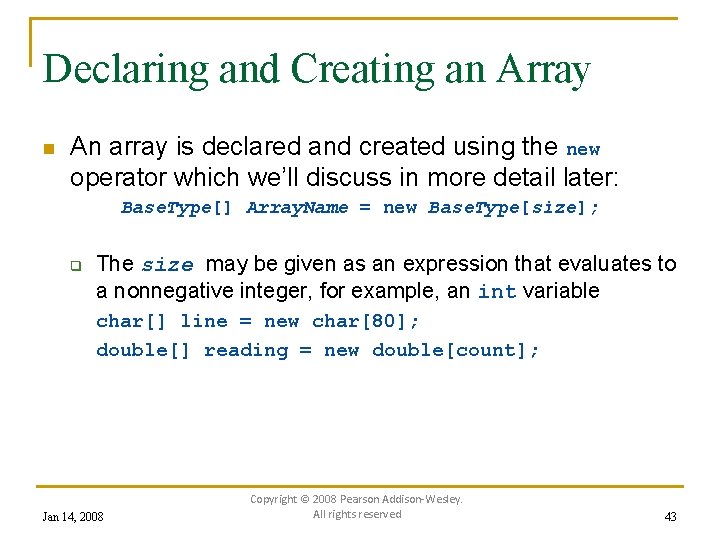
Declaring and Creating an Array n An array is declared and created using the new operator which we’ll discuss in more detail later: Base. Type[] Array. Name = new Base. Type[size]; q The size may be given as an expression that evaluates to a nonnegative integer, for example, an int variable char[] line = new char[80]; double[] reading = new double[count]; Jan 14, 2008 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 43
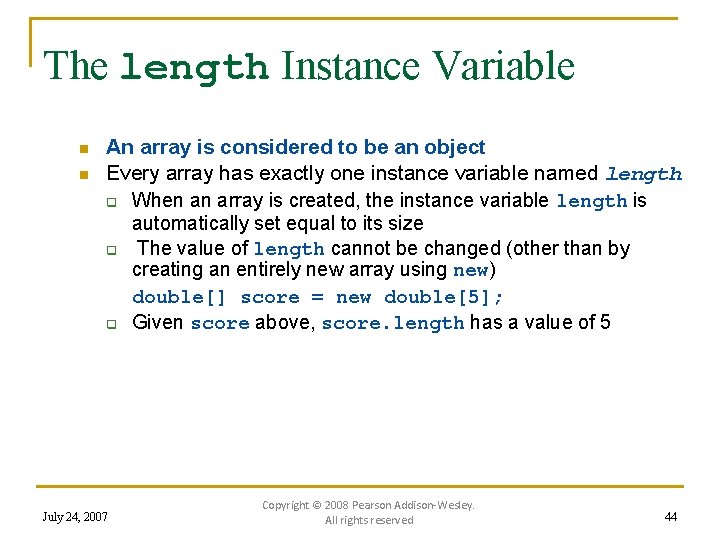
The length Instance Variable n n An array is considered to be an object Every array has exactly one instance variable named length q When an array is created, the instance variable length is automatically set equal to its size q The value of length cannot be changed (other than by creating an entirely new array using new) double[] score = new double[5]; q Given score above, score. length has a value of 5 July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 44
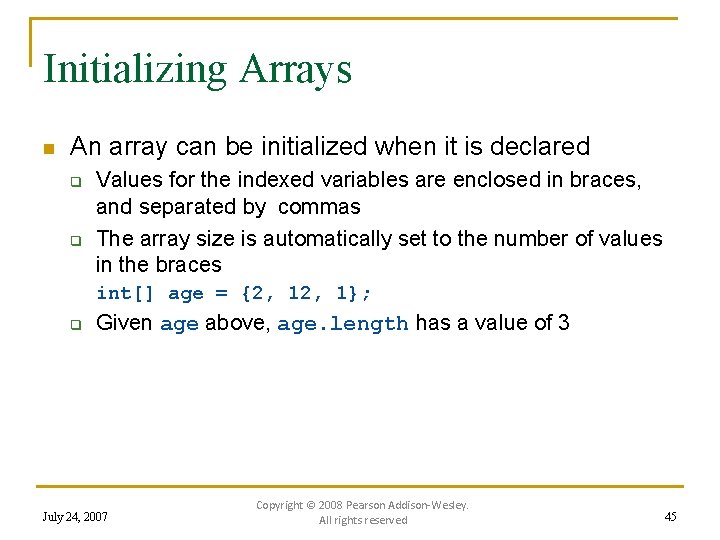
Initializing Arrays n An array can be initialized when it is declared q q Values for the indexed variables are enclosed in braces, and separated by commas The array size is automatically set to the number of values in the braces int[] age = {2, 1}; q Given age above, age. length has a value of 3 July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 45
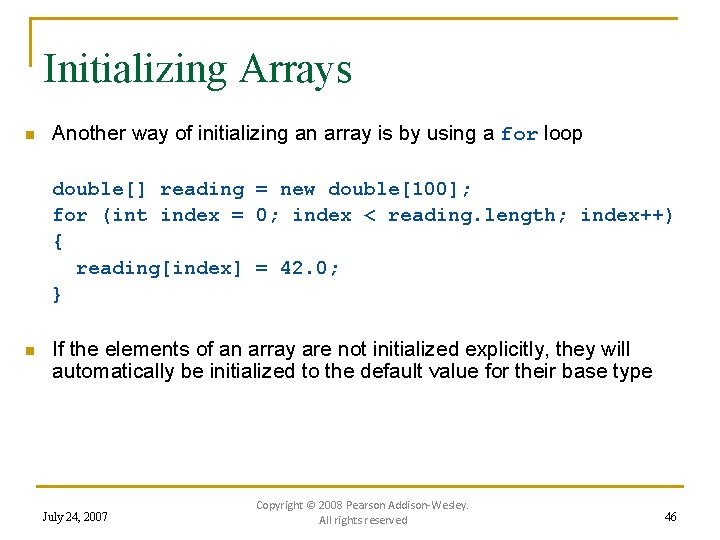
Initializing Arrays n Another way of initializing an array is by using a for loop double[] reading = new double[100]; for (int index = 0; index < reading. length; index++) { reading[index] = 42. 0; } n If the elements of an array are not initialized explicitly, they will automatically be initialized to the default value for their base type July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 46
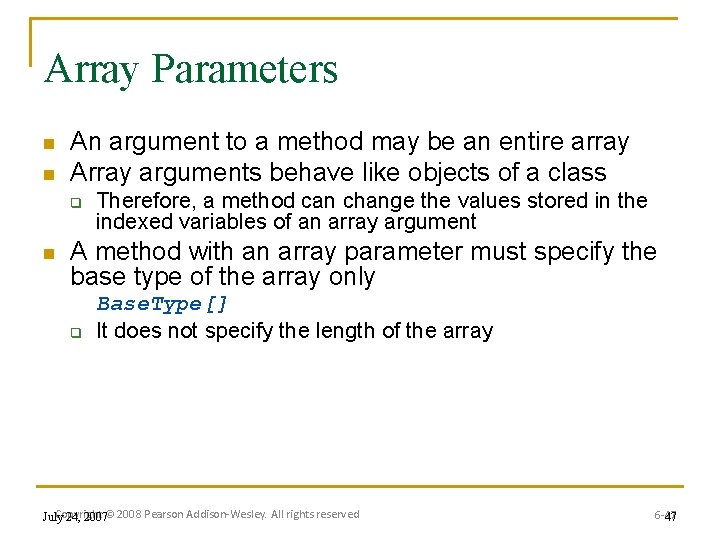
Array Parameters n n An argument to a method may be an entire array Array arguments behave like objects of a class q n Therefore, a method can change the values stored in the indexed variables of an array argument A method with an array parameter must specify the base type of the array only q Base. Type[] It does not specify the length of the array Copyright July 24, 2007© 2008 Pearson Addison-Wesley. All rights reserved 6 -47 47
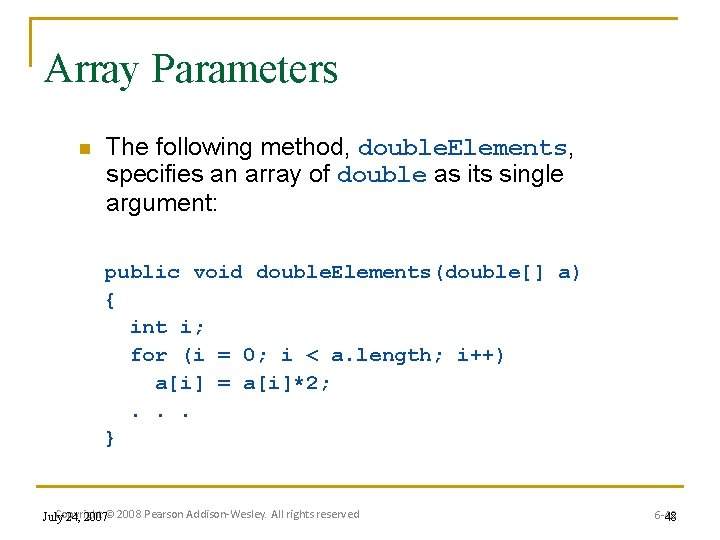
Array Parameters n The following method, double. Elements, specifies an array of double as its single argument: public void double. Elements(double[] a) { int i; for (i = 0; i < a. length; i++) a[i] = a[i]*2; . . . } Copyright July 24, 2007© 2008 Pearson Addison-Wesley. All rights reserved 6 -48 48
![Array Parameters n n Arrays of double may be defined as follows double a Array Parameters n n Arrays of double may be defined as follows: double[] a](https://slidetodoc.com/presentation_image_h2/bba903afdd485962c17876a8e1e65ad9/image-49.jpg)
Array Parameters n n Arrays of double may be defined as follows: double[] a = new double[10]; double[] b = new double[30]; Given the arrays above, the method double. Elements invoked as follows: double. Elements(a); double. Elements(b); q Note that no square brackets are used when an entire array is given as an argument q Note also that a method that specifies an array for a parameter can take an array of any length as an argument Copyright July 24, 2007© 2008 Pearson Addison-Wesley. All rights reserved 6 -49 49
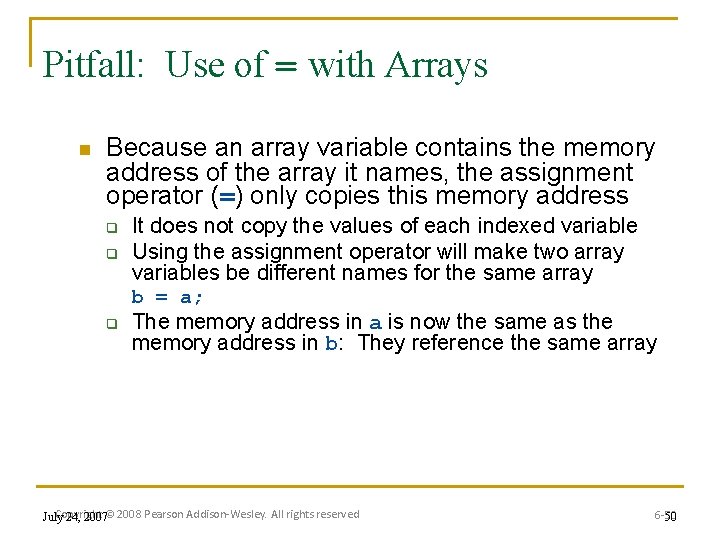
Pitfall: Use of = with Arrays n Because an array variable contains the memory address of the array it names, the assignment operator (=) only copies this memory address q q It does not copy the values of each indexed variable Using the assignment operator will make two array variables be different names for the same array b = a; q The memory address in a is now the same as the memory address in b: They reference the same array Copyright July 24, 2007© 2008 Pearson Addison-Wesley. All rights reserved 6 -50 50
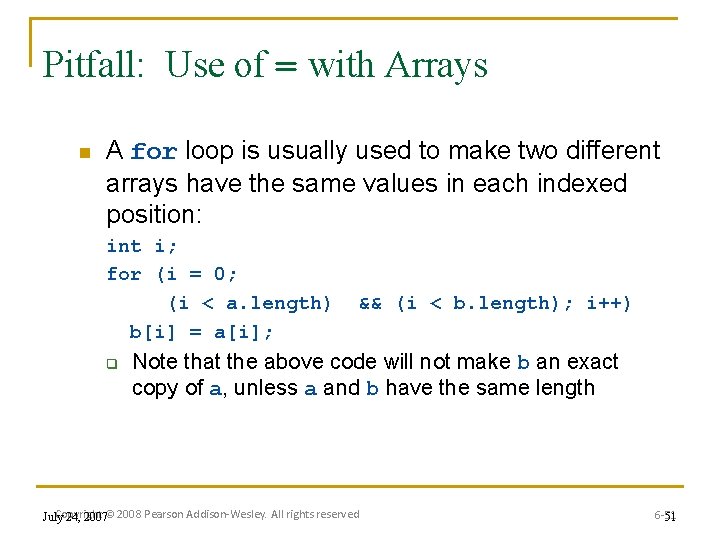
Pitfall: Use of = with Arrays n A for loop is usually used to make two different arrays have the same values in each indexed position: int i; for (i = 0; (i < a. length) b[i] = a[i]; q && (i < b. length); i++) Note that the above code will not make b an exact copy of a, unless a and b have the same length Copyright July 24, 2007© 2008 Pearson Addison-Wesley. All rights reserved 6 -51 51
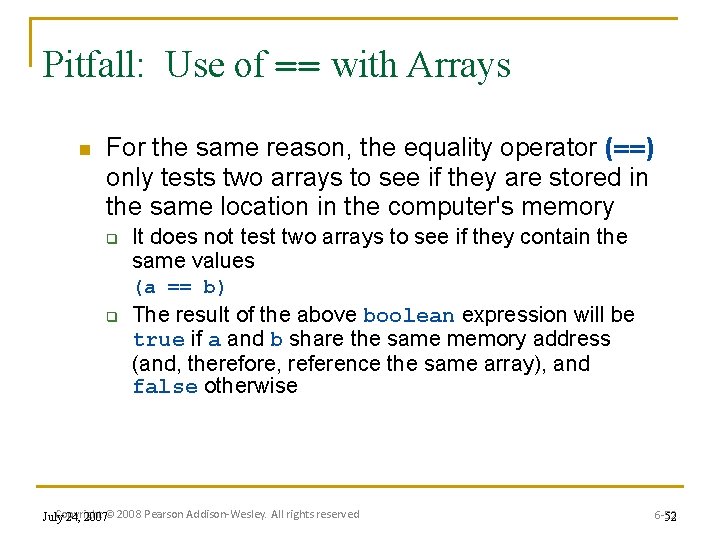
Pitfall: Use of == with Arrays n For the same reason, the equality operator (==) only tests two arrays to see if they are stored in the same location in the computer's memory q It does not test two arrays to see if they contain the same values (a == b) q The result of the above boolean expression will be true if a and b share the same memory address (and, therefore, reference the same array), and false otherwise Copyright July 24, 2007© 2008 Pearson Addison-Wesley. All rights reserved 6 -52 52
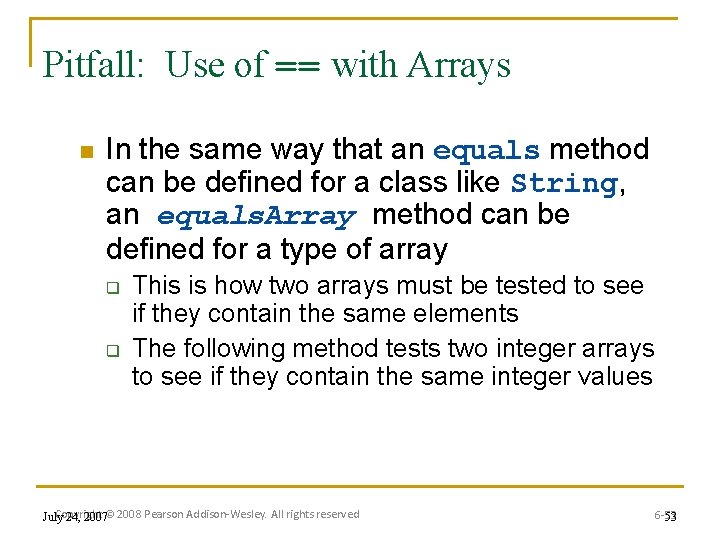
Pitfall: Use of == with Arrays n In the same way that an equals method can be defined for a class like String, an equals. Array method can be defined for a type of array q q This is how two arrays must be tested to see if they contain the same elements The following method tests two integer arrays to see if they contain the same integer values Copyright July 24, 2007© 2008 Pearson Addison-Wesley. All rights reserved 6 -53 53
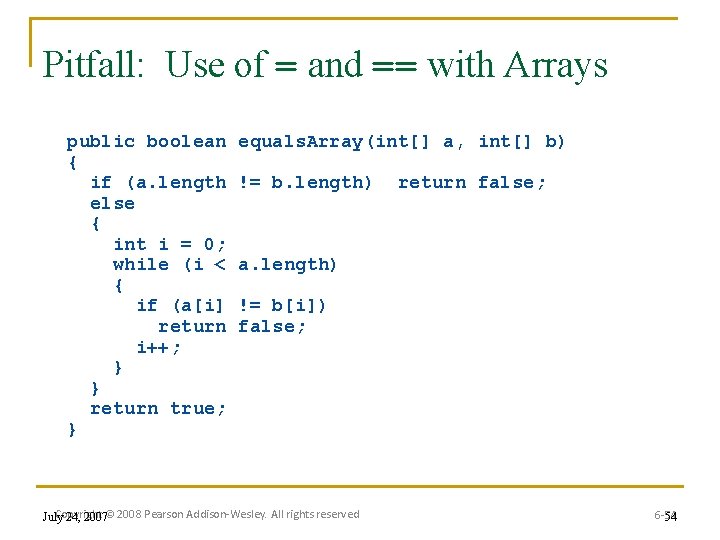
Pitfall: Use of = and == with Arrays public boolean { if (a. length else { int i = 0; while (i < { if (a[i] return i++; } } return true; } equals. Array(int[] a, int[] b) != b. length) return false; a. length) != b[i]) false; Copyright July 24, 2007© 2008 Pearson Addison-Wesley. All rights reserved 6 -54 54
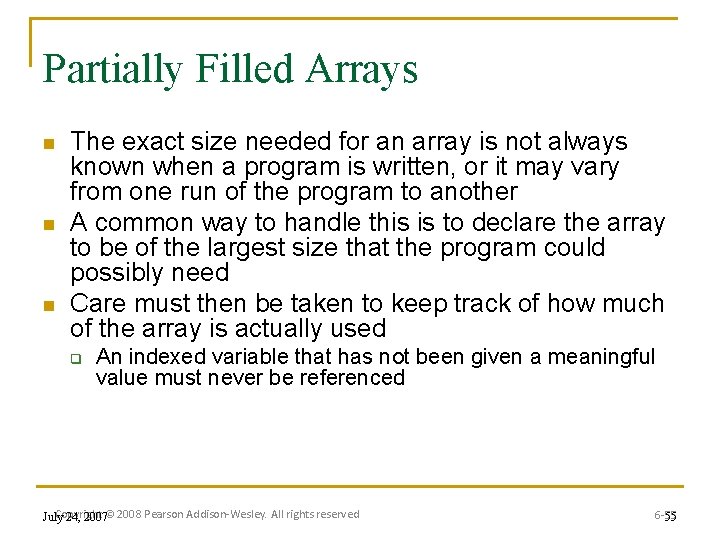
Partially Filled Arrays n n n The exact size needed for an array is not always known when a program is written, or it may vary from one run of the program to another A common way to handle this is to declare the array to be of the largest size that the program could possibly need Care must then be taken to keep track of how much of the array is actually used q An indexed variable that has not been given a meaningful value must never be referenced Copyright July 24, 2007© 2008 Pearson Addison-Wesley. All rights reserved 6 -55 55
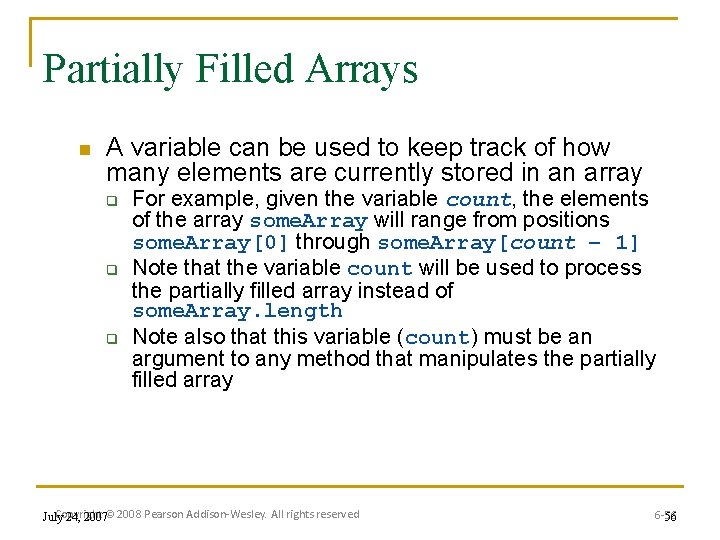
Partially Filled Arrays n A variable can be used to keep track of how many elements are currently stored in an array q q q For example, given the variable count, the elements of the array some. Array will range from positions some. Array[0] through some. Array[count – 1] Note that the variable count will be used to process the partially filled array instead of some. Array. length Note also that this variable (count) must be an argument to any method that manipulates the partially filled array Copyright July 24, 2007© 2008 Pearson Addison-Wesley. All rights reserved 6 -56 56
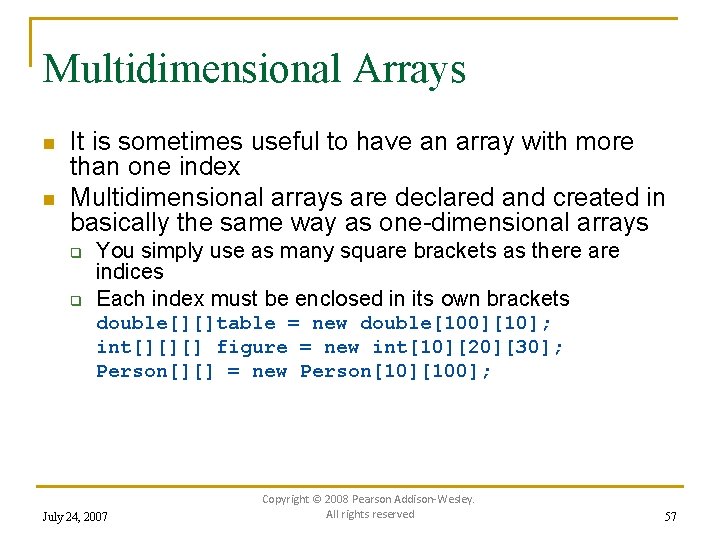
Multidimensional Arrays n n It is sometimes useful to have an array with more than one index Multidimensional arrays are declared and created in basically the same way as one-dimensional arrays q q You simply use as many square brackets as there are indices Each index must be enclosed in its own brackets double[][]table = new double[100][10]; int[][][] figure = new int[10][20][30]; Person[][] = new Person[10][100]; July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 57
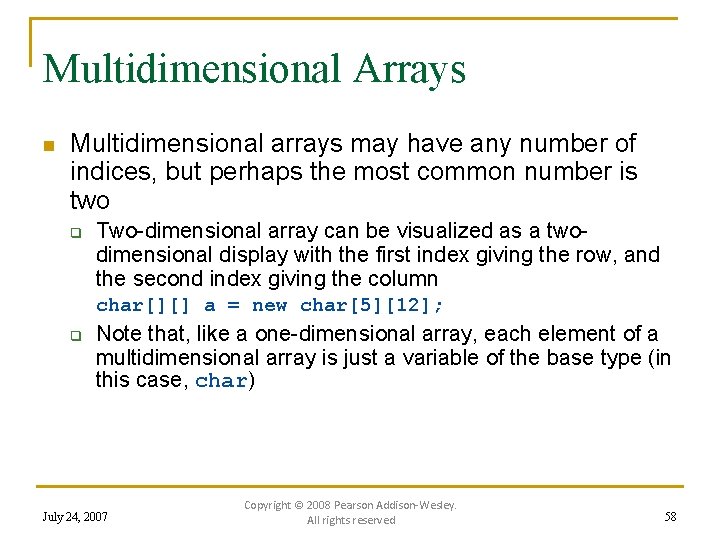
Multidimensional Arrays n Multidimensional arrays may have any number of indices, but perhaps the most common number is two q Two-dimensional array can be visualized as a twodimensional display with the first index giving the row, and the second index giving the column char[][] a = new char[5][12]; q Note that, like a one-dimensional array, each element of a multidimensional array is just a variable of the base type (in this case, char) July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 58
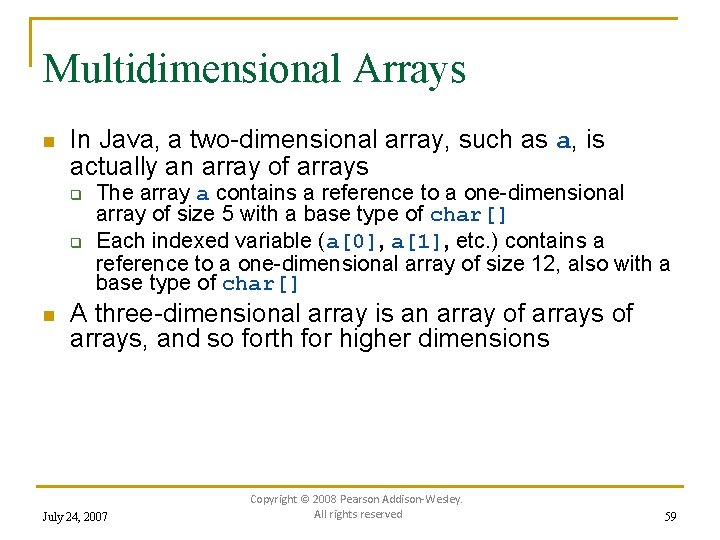
Multidimensional Arrays n In Java, a two-dimensional array, such as a, is actually an array of arrays q q n The array a contains a reference to a one-dimensional array of size 5 with a base type of char[] Each indexed variable (a[0], a[1], etc. ) contains a reference to a one-dimensional array of size 12, also with a base type of char[] A three-dimensional array is an array of arrays, and so forth for higher dimensions July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 59
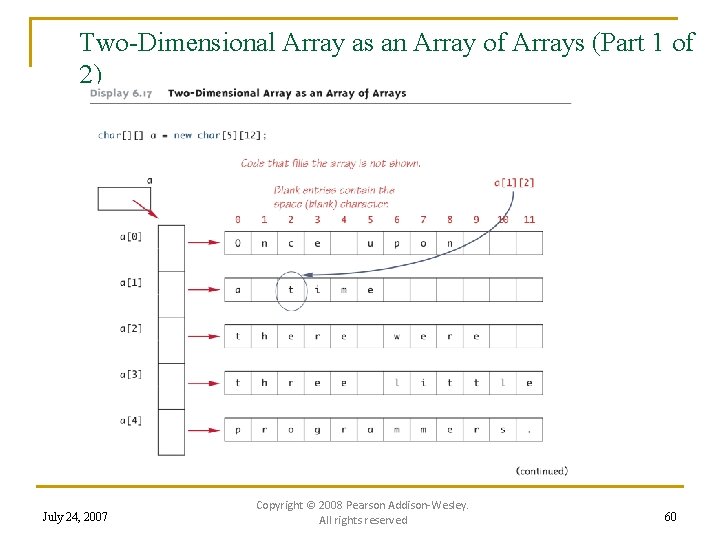
Two-Dimensional Array as an Array of Arrays (Part 1 of 2) July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 60
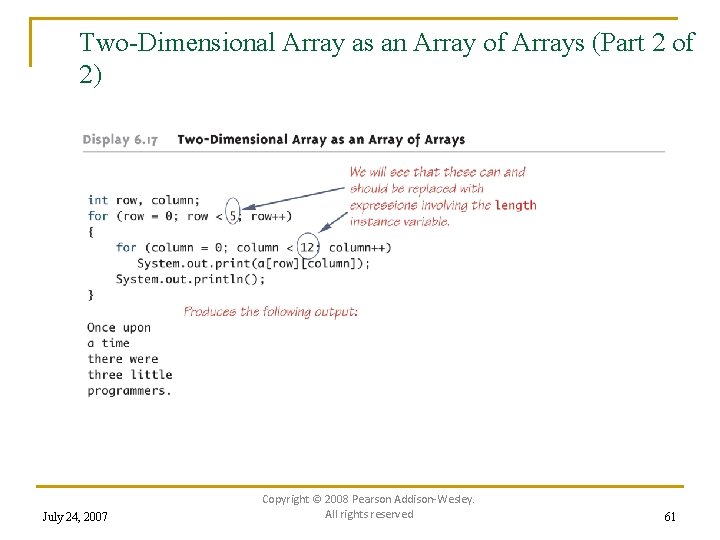
Two-Dimensional Array as an Array of Arrays (Part 2 of 2) July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 61
![Using the length Instance Variable char page new char30100 n The instance variable Using the length Instance Variable char[][] page = new char[30][100]; n The instance variable](https://slidetodoc.com/presentation_image_h2/bba903afdd485962c17876a8e1e65ad9/image-62.jpg)
Using the length Instance Variable char[][] page = new char[30][100]; n The instance variable length does not give the total number of indexed variables in a two-dimensional array q Because a two-dimensional array is actually an array of arrays, the instance variable length gives the number of first indices (or "rows") in the array n page. length is equal to 30 q For the same reason, the number of second indices (or "columns") for a given "row" is given by referencing length for that "row" variable n page[0]. length is equal to 100 July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 62
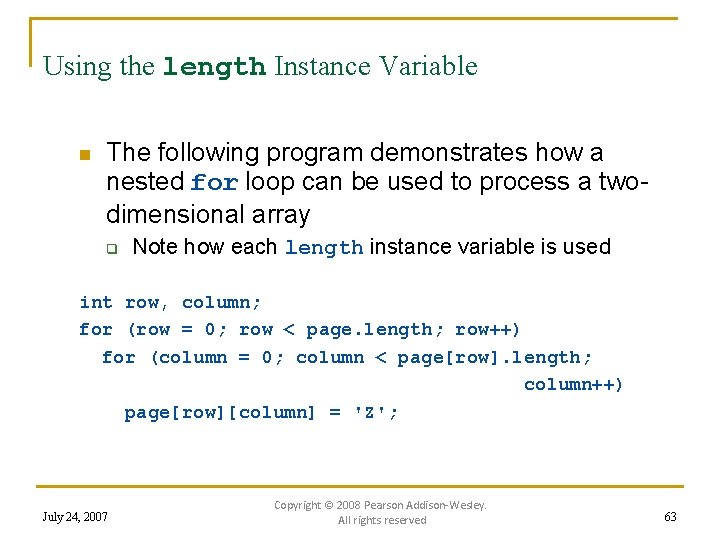
Using the length Instance Variable n The following program demonstrates how a nested for loop can be used to process a twodimensional array q Note how each length instance variable is used int row, column; for (row = 0; row < page. length; row++) for (column = 0; column < page[row]. length; column++) page[row][column] = 'Z'; July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 63
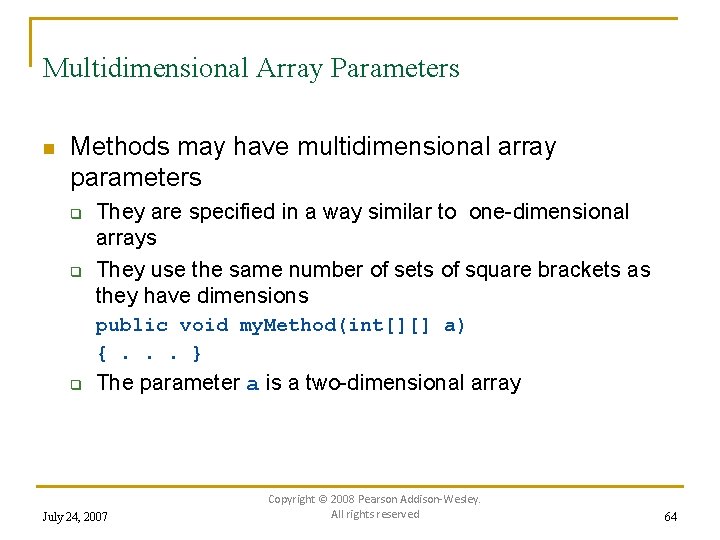
Multidimensional Array Parameters n Methods may have multidimensional array parameters q q They are specified in a way similar to one-dimensional arrays They use the same number of sets of square brackets as they have dimensions public void my. Method(int[][] a) {. . . } q The parameter a is a two-dimensional array July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 64
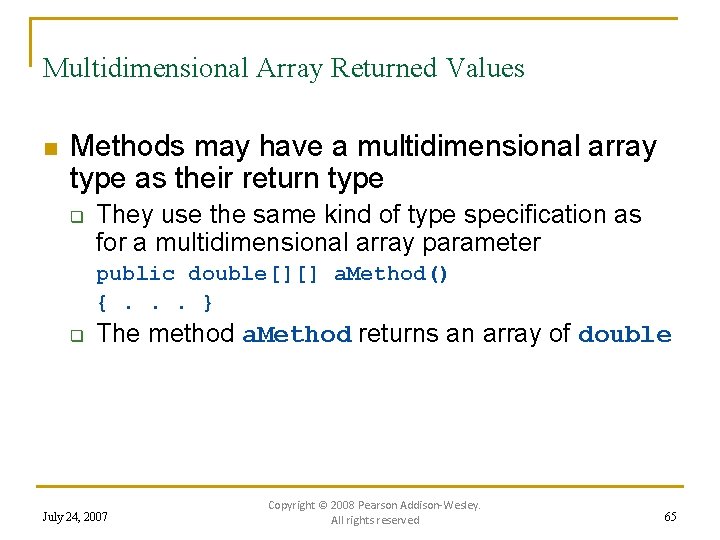
Multidimensional Array Returned Values n Methods may have a multidimensional array type as their return type q They use the same kind of type specification as for a multidimensional array parameter public double[][] a. Method() {. . . } q The method a. Method returns an array of double July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 65
Cmsc 202 umbc
Umbc cmsc 341
Captain tory harris burdick
Captain tory picture
July 1-4 1863
Tender mean
Antwrp
2001 july 15
2003 july 17
July 30 2009 nasa
Sources nso july frenchhowell neill technology...
What is the significance of july 4 1776 brainpop
Poppies in july aoifes notes
The cuban melodrama
Imagery in poppies in july
Gdje se rodio nikola tesla
Ctdssmap payment schedule july 2021
Sergei korolev
6th july 1988
Monday 13th july
On july 18 2001 a train carrying hazardous chemicals
July 4 sermon
June too soon july stand by
July 2 1937 amelia earhart
June 22 to july 22
July 12 1776
Sensory language definition
Catawba indian nation bingo
June july august
July 14 1789
Malaga in july
Leaf yeast
July 26 1953
July 16 1776
Bp 202 form
Oblique cutting example
Cs202 iitk
Mt202 cov swift message format
Linia kolejowa 202
Mt 202
202 accepted
Sfu fraser library
Cve 202
Coe 202
Pos expression can be implemented using
Coe 202
Xxxxxxxs
Cvsp 202 aub
Ashrae standard 202
Helikasa
Cse 202
Cse 202
Cpcs 202
Coe 202
Coe202
Coe202
Jaquet ft3000
Cs 202
Atlas copco sb 202 hydraulic breaker
Hcf and lcm worksheet for class 6
Mt manager inventia download
Five variable k map
Bio 202
Ie 202
Tuseno saat
Cpcs 202