CMSC 202 Java Primer 1 July 24 2007
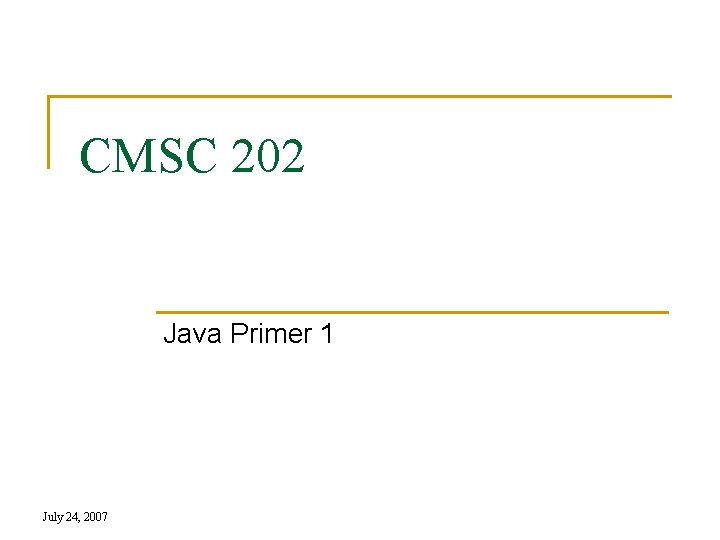
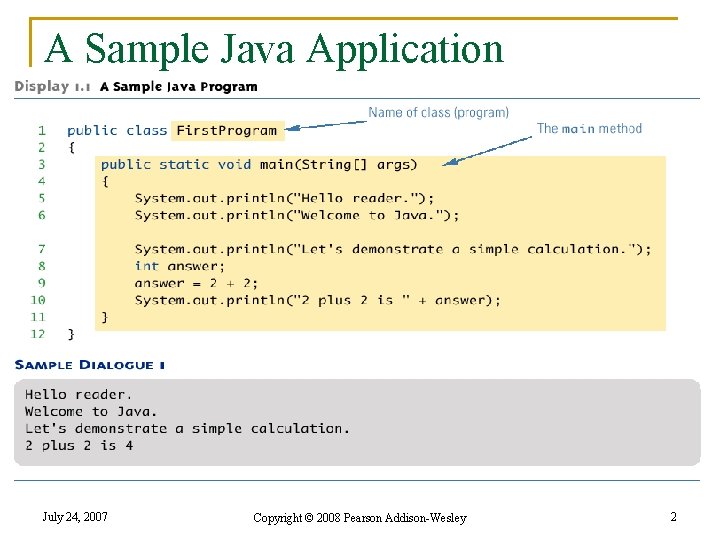
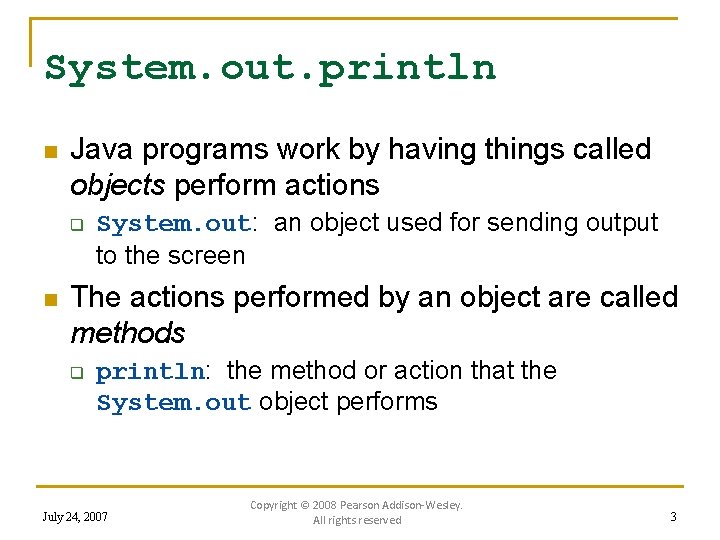
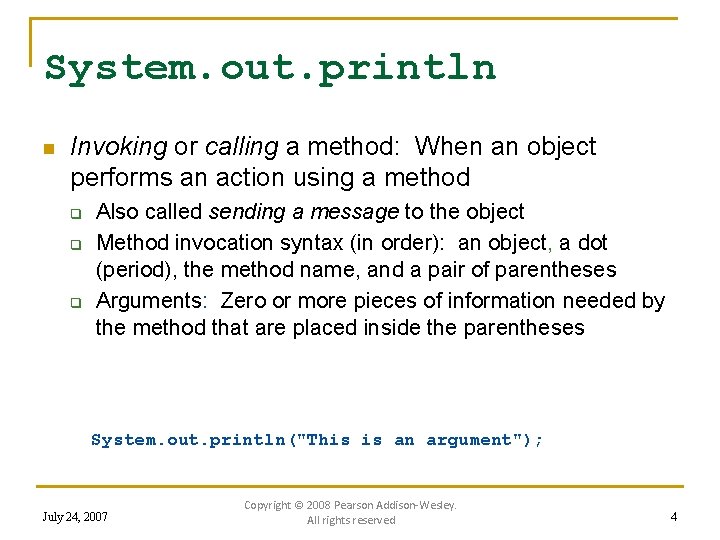
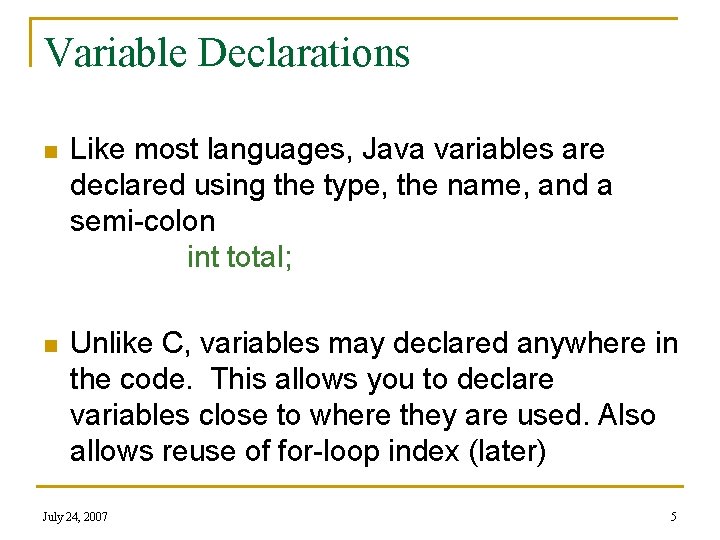
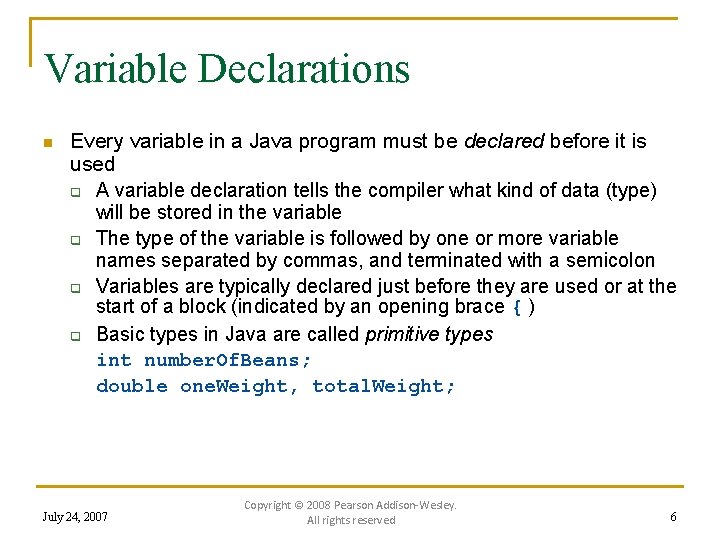
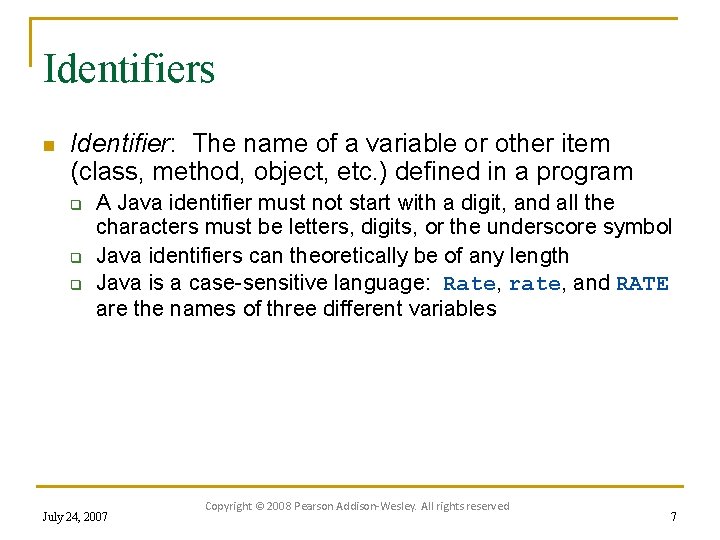
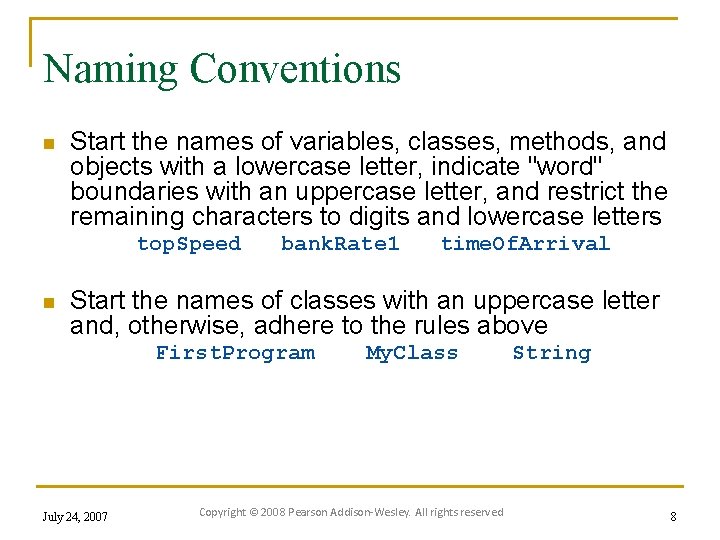
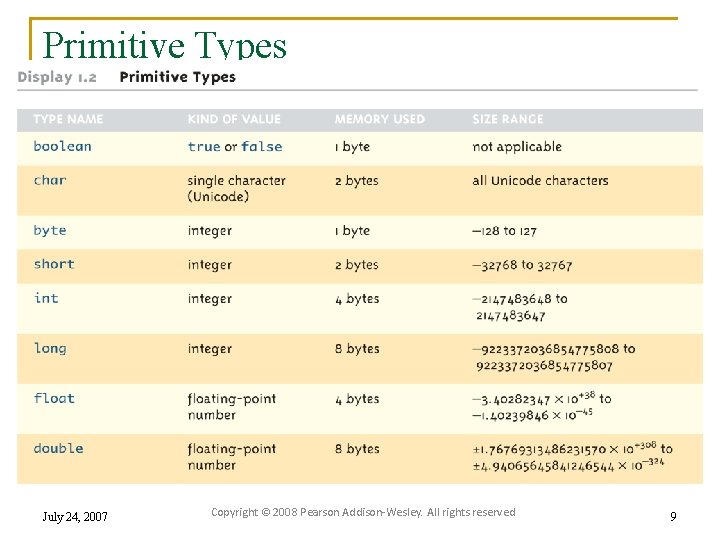
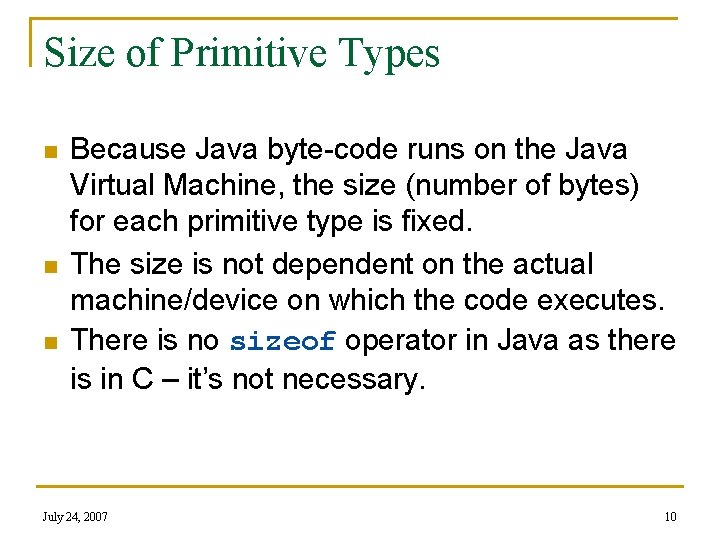
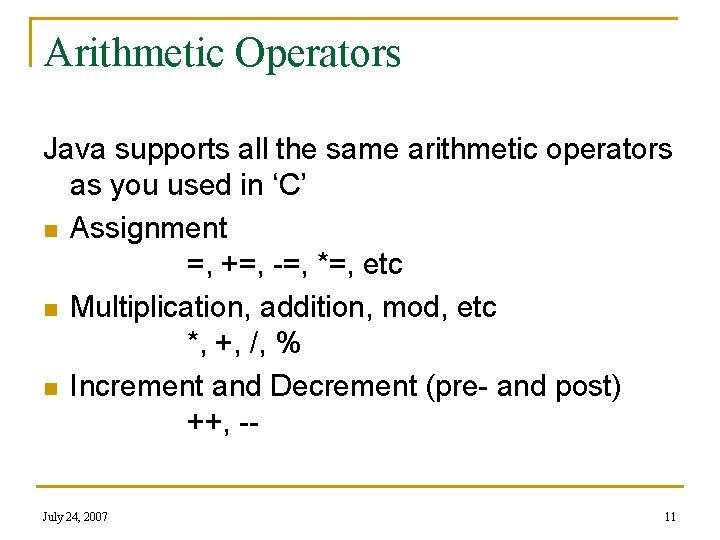
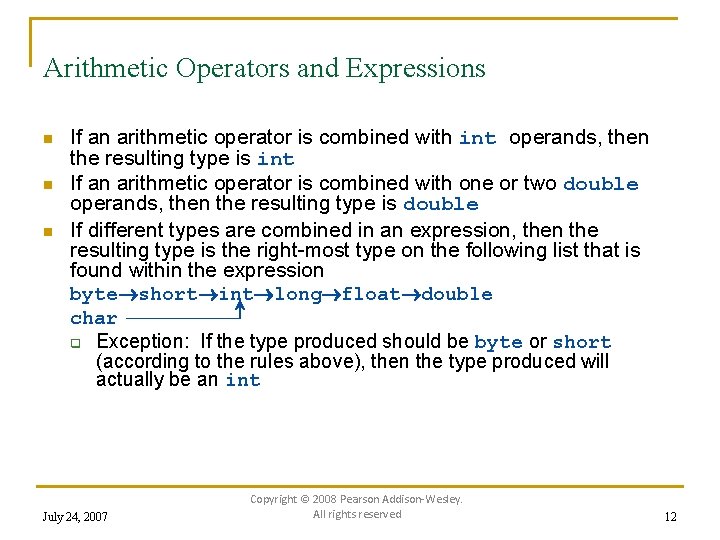
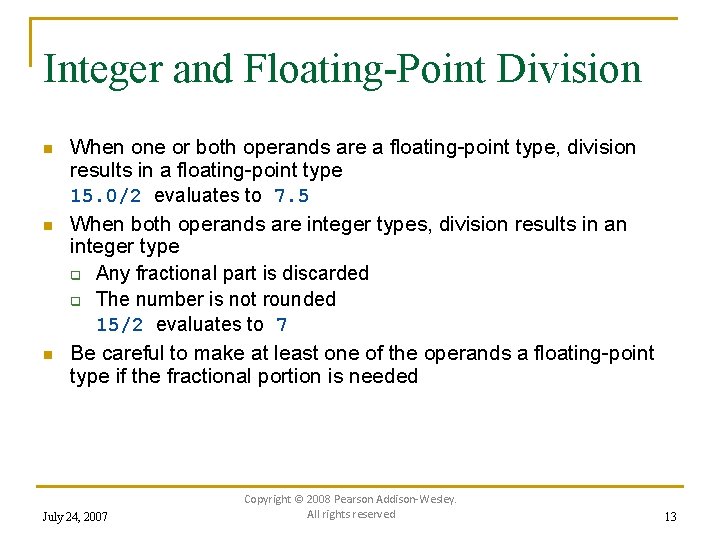
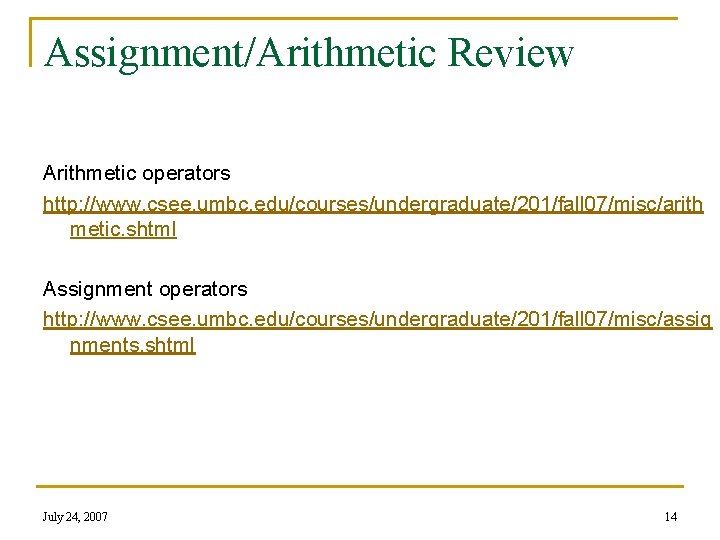
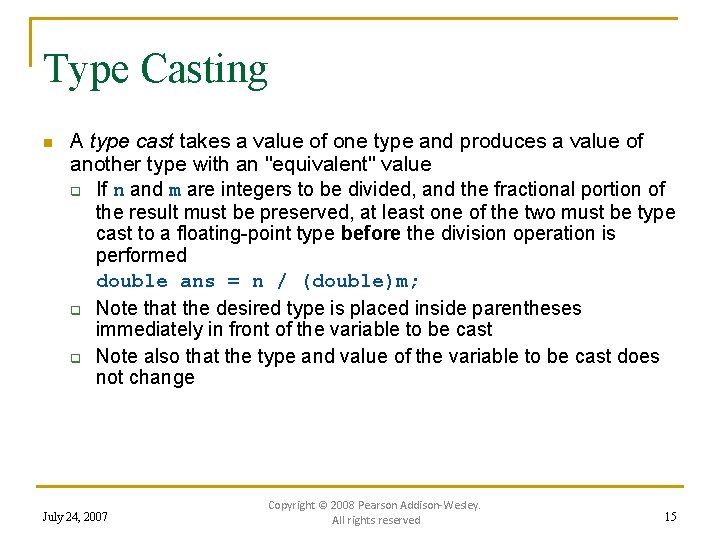
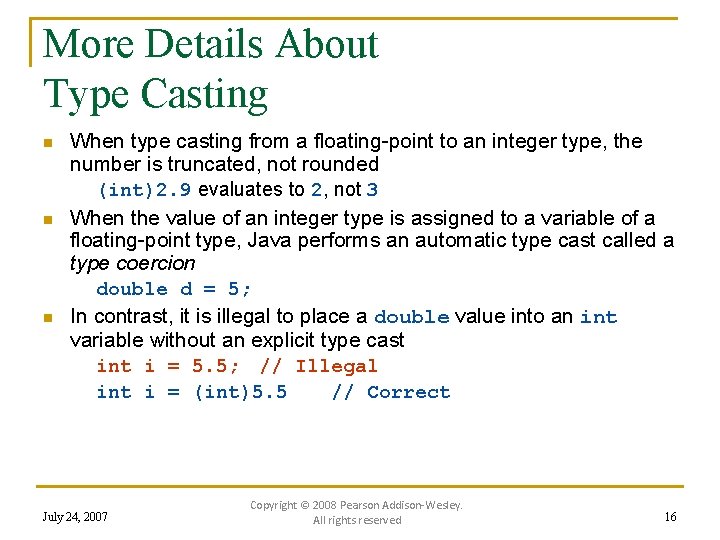
- Slides: 16
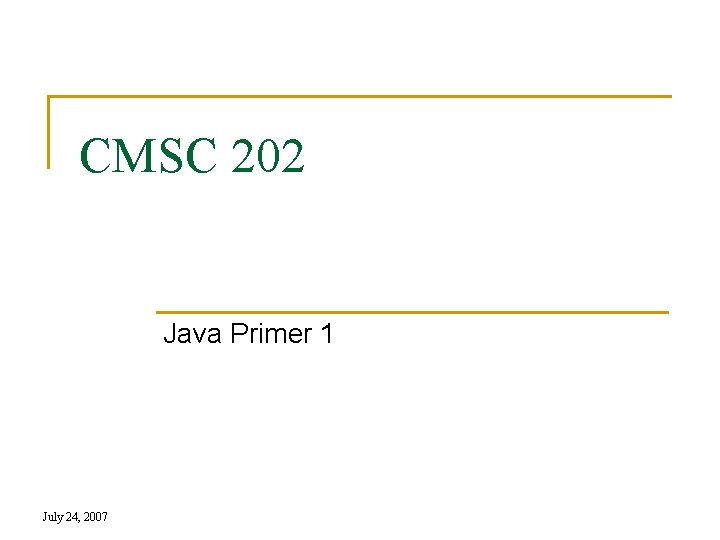
CMSC 202 Java Primer 1 July 24, 2007
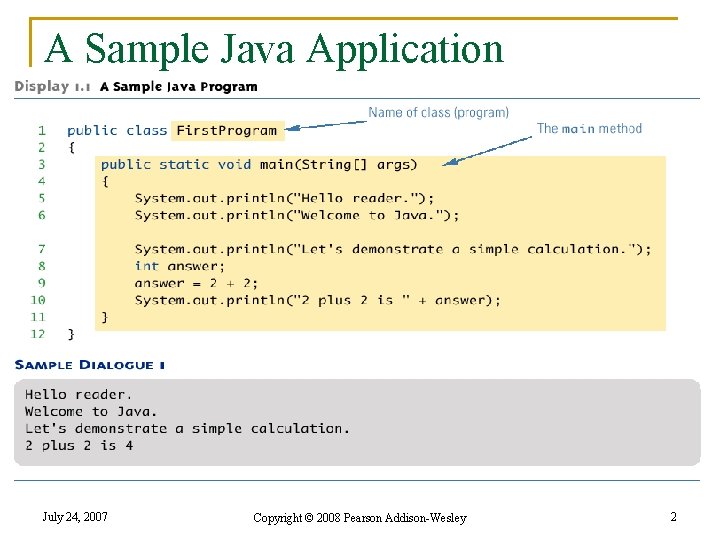
A Sample Java Application July 24, 2007 Copyright © 2008 Pearson Addison-Wesley 2
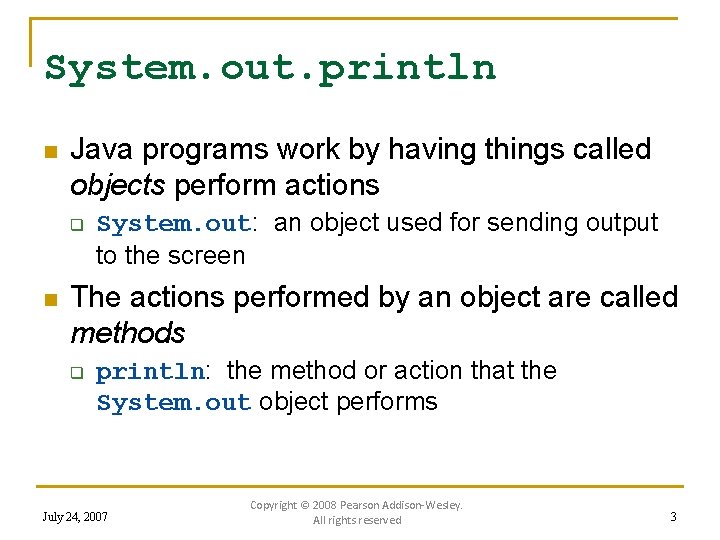
System. out. println n Java programs work by having things called objects perform actions q n System. out: an object used for sending output to the screen The actions performed by an object are called methods q println: the method or action that the System. out object performs July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 3
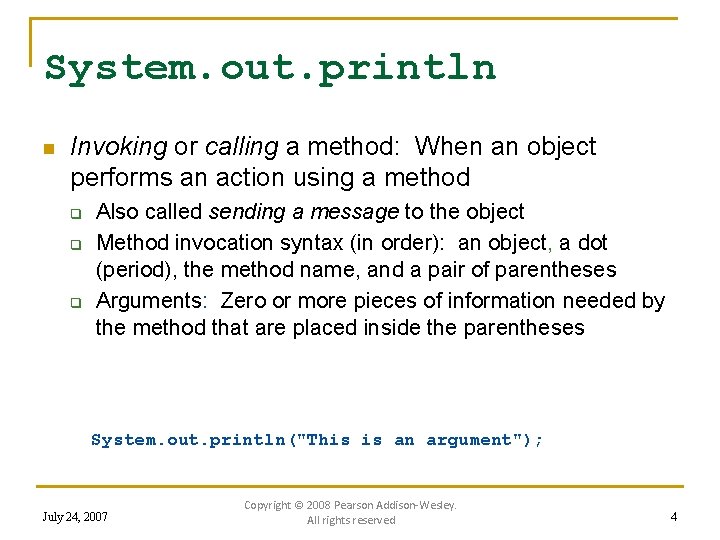
System. out. println n Invoking or calling a method: When an object performs an action using a method q q q Also called sending a message to the object Method invocation syntax (in order): an object, a dot (period), the method name, and a pair of parentheses Arguments: Zero or more pieces of information needed by the method that are placed inside the parentheses System. out. println("This is an argument"); July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 4
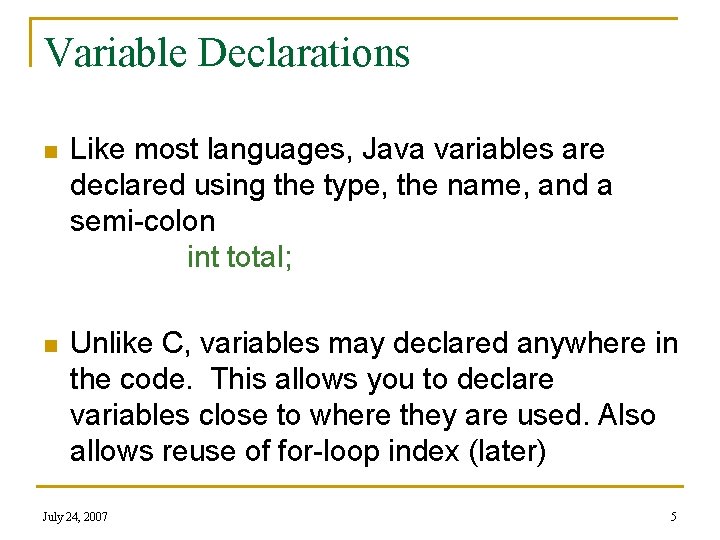
Variable Declarations n Like most languages, Java variables are declared using the type, the name, and a semi-colon int total; n Unlike C, variables may declared anywhere in the code. This allows you to declare variables close to where they are used. Also allows reuse of for-loop index (later) July 24, 2007 5
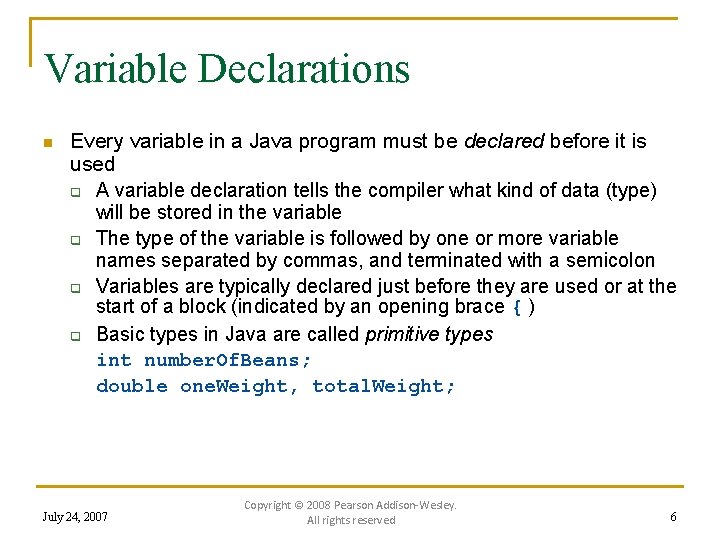
Variable Declarations n Every variable in a Java program must be declared before it is used q A variable declaration tells the compiler what kind of data (type) will be stored in the variable q The type of the variable is followed by one or more variable names separated by commas, and terminated with a semicolon q Variables are typically declared just before they are used or at the start of a block (indicated by an opening brace { ) q Basic types in Java are called primitive types int number. Of. Beans; double one. Weight, total. Weight; July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 6
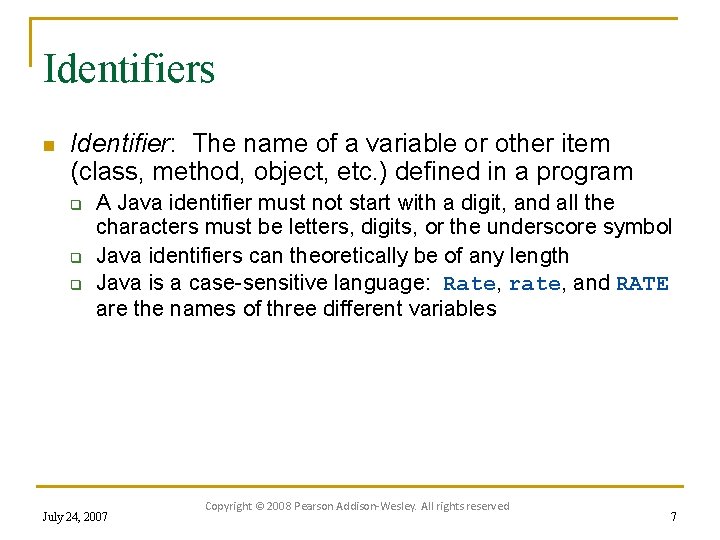
Identifiers n Identifier: The name of a variable or other item (class, method, object, etc. ) defined in a program q q q A Java identifier must not start with a digit, and all the characters must be letters, digits, or the underscore symbol Java identifiers can theoretically be of any length Java is a case-sensitive language: Rate, rate, and RATE are the names of three different variables July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 7
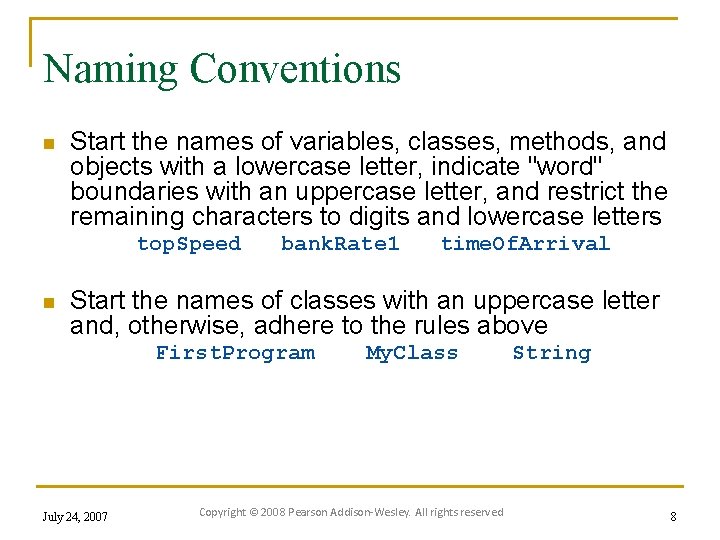
Naming Conventions n Start the names of variables, classes, methods, and objects with a lowercase letter, indicate "word" boundaries with an uppercase letter, and restrict the remaining characters to digits and lowercase letters top. Speed n bank. Rate 1 time. Of. Arrival Start the names of classes with an uppercase letter and, otherwise, adhere to the rules above First. Program July 24, 2007 My. Class Copyright © 2008 Pearson Addison-Wesley. All rights reserved String 8
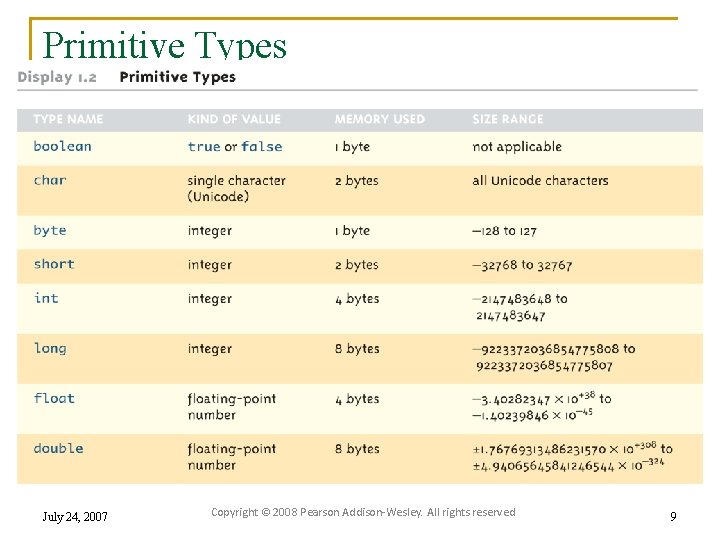
Primitive Types July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 9
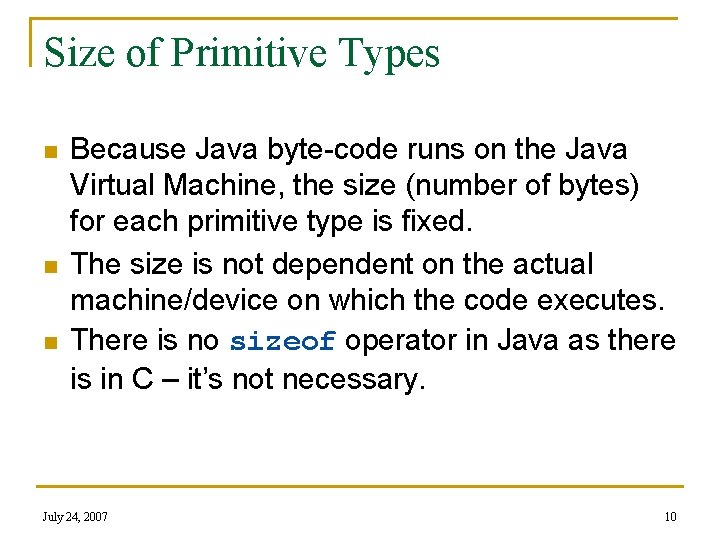
Size of Primitive Types n n n Because Java byte-code runs on the Java Virtual Machine, the size (number of bytes) for each primitive type is fixed. The size is not dependent on the actual machine/device on which the code executes. There is no sizeof operator in Java as there is in C – it’s not necessary. July 24, 2007 10
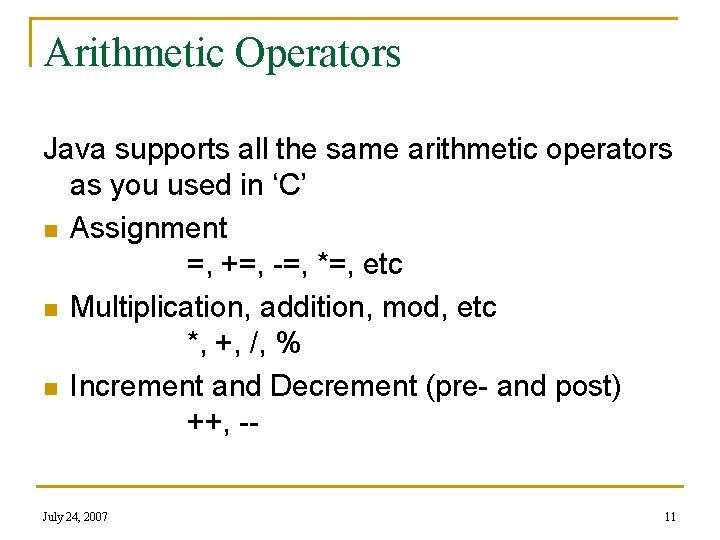
Arithmetic Operators Java supports all the same arithmetic operators as you used in ‘C’ n Assignment =, +=, -=, *=, etc n Multiplication, addition, mod, etc *, +, /, % n Increment and Decrement (pre- and post) ++, -July 24, 2007 11
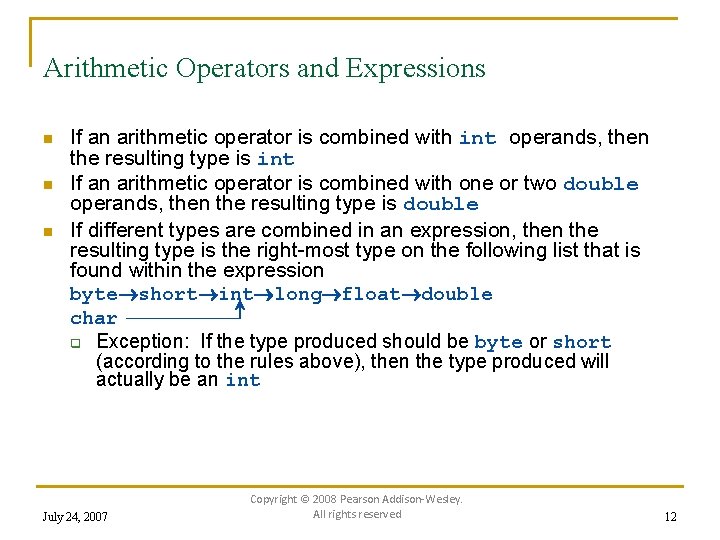
Arithmetic Operators and Expressions n n n If an arithmetic operator is combined with int operands, then the resulting type is int If an arithmetic operator is combined with one or two double operands, then the resulting type is double If different types are combined in an expression, then the resulting type is the right-most type on the following list that is found within the expression byte short int long float double char q Exception: If the type produced should be byte or short (according to the rules above), then the type produced will actually be an int July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 12
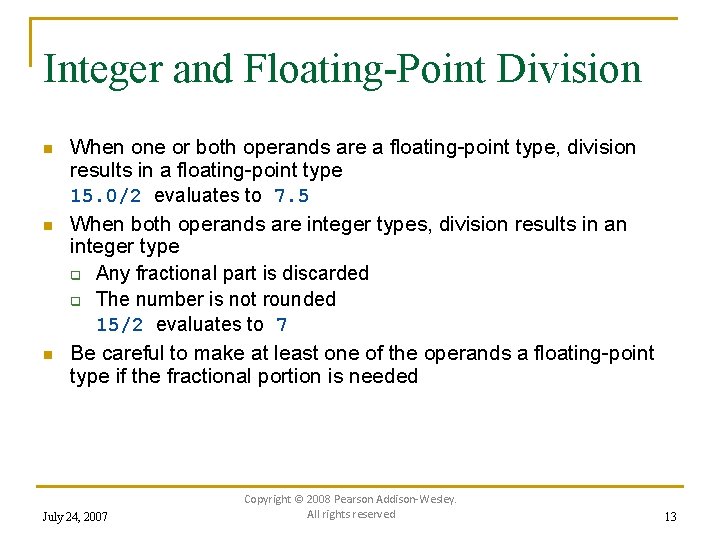
Integer and Floating-Point Division n When one or both operands are a floating-point type, division results in a floating-point type 15. 0/2 evaluates to 7. 5 When both operands are integer types, division results in an integer type q Any fractional part is discarded q The number is not rounded 15/2 evaluates to 7 Be careful to make at least one of the operands a floating-point type if the fractional portion is needed July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 13
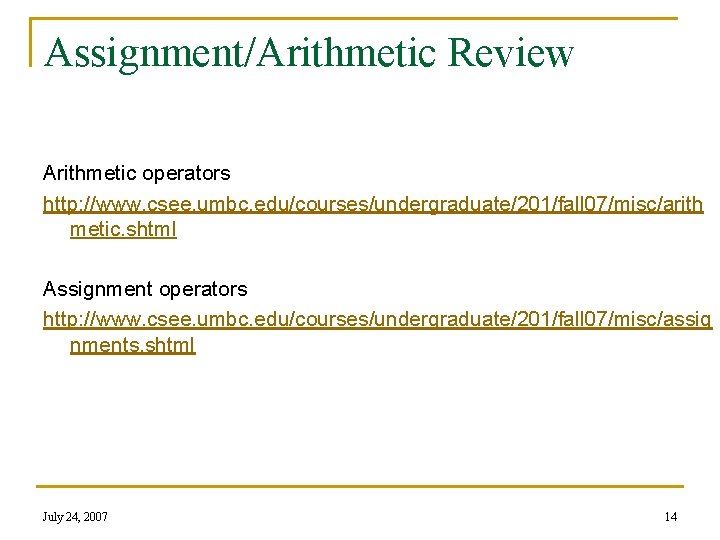
Assignment/Arithmetic Review Arithmetic operators http: //www. csee. umbc. edu/courses/undergraduate/201/fall 07/misc/arith metic. shtml Assignment operators http: //www. csee. umbc. edu/courses/undergraduate/201/fall 07/misc/assig nments. shtml July 24, 2007 14
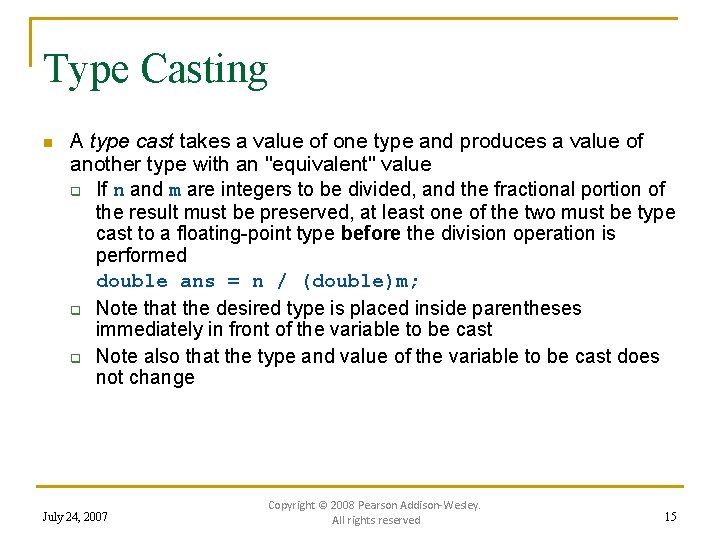
Type Casting n A type cast takes a value of one type and produces a value of another type with an "equivalent" value q If n and m are integers to be divided, and the fractional portion of the result must be preserved, at least one of the two must be type cast to a floating-point type before the division operation is performed double ans = n / (double)m; q Note that the desired type is placed inside parentheses immediately in front of the variable to be cast q Note also that the type and value of the variable to be cast does not change July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 15
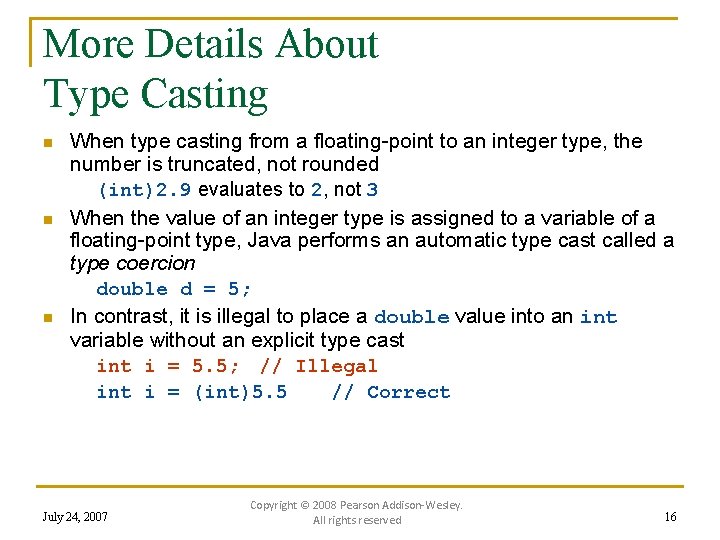
More Details About Type Casting n n n When type casting from a floating-point to an integer type, the number is truncated, not rounded (int)2. 9 evaluates to 2, not 3 When the value of an integer type is assigned to a variable of a floating-point type, Java performs an automatic type cast called a type coercion double d = 5; In contrast, it is illegal to place a double value into an int variable without an explicit type cast int i = 5. 5; // Illegal int i = (int)5. 5 // Correct July 24, 2007 Copyright © 2008 Pearson Addison-Wesley. All rights reserved 16
Cmsc 202 umbc
Umbc cmsc 341
The harp by harris burdick
Just desert harris burdick story
July 1-4 1863
Tender definition
Astronomy picture of the day 11 july 2001
2001 july 15
2003 july 17
July 30 2009 nasa
Sources nso frenchhowell neill mit technology...
May 1775
Sylvia plath poppies in july
Miss cuba receives an invitation
Poppies in july
July 10 1856
Ctdssmap payment schedule july 2021