CHAPTER 7 JQUERY WHAT IS JQUERY j Query
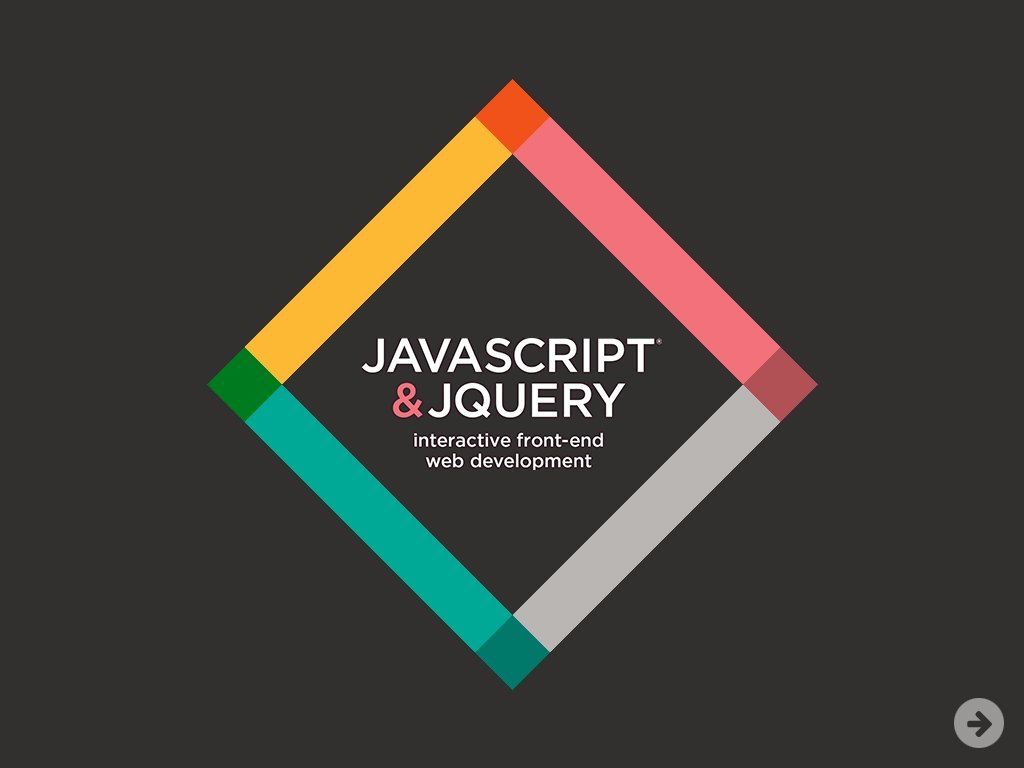
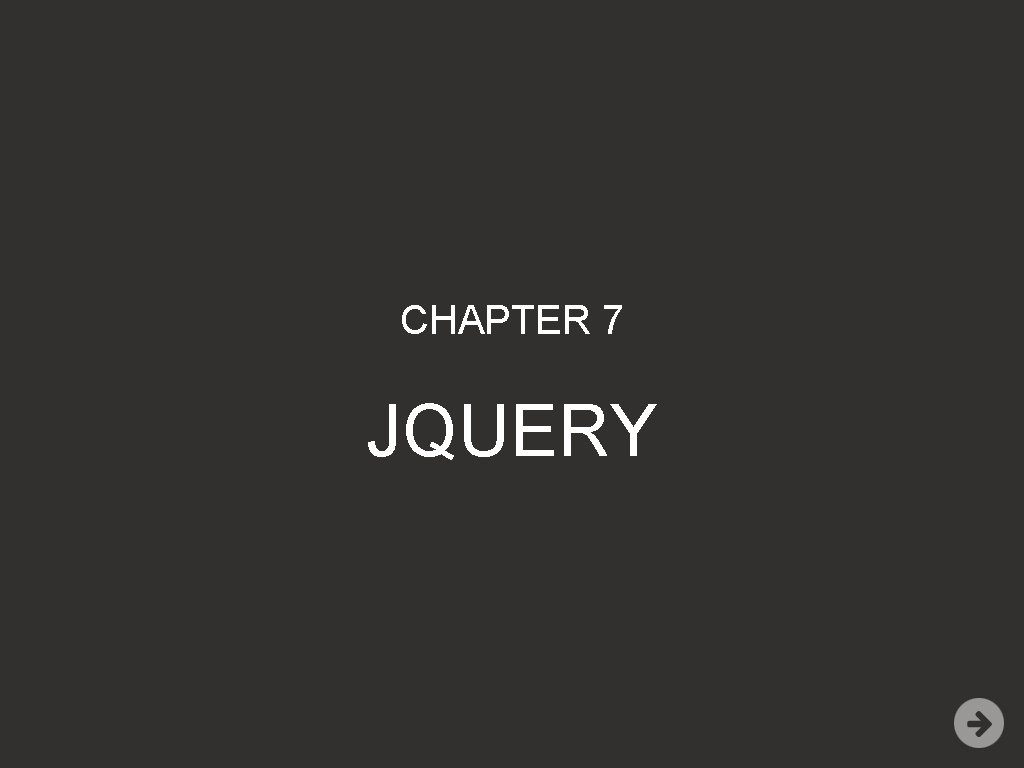
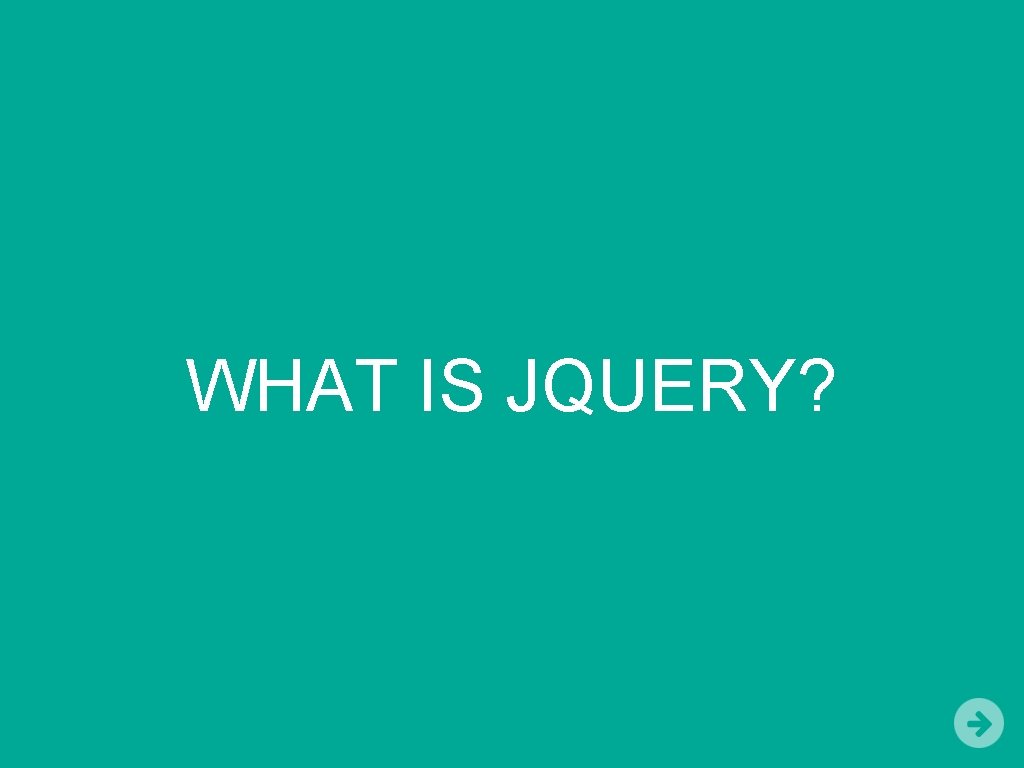
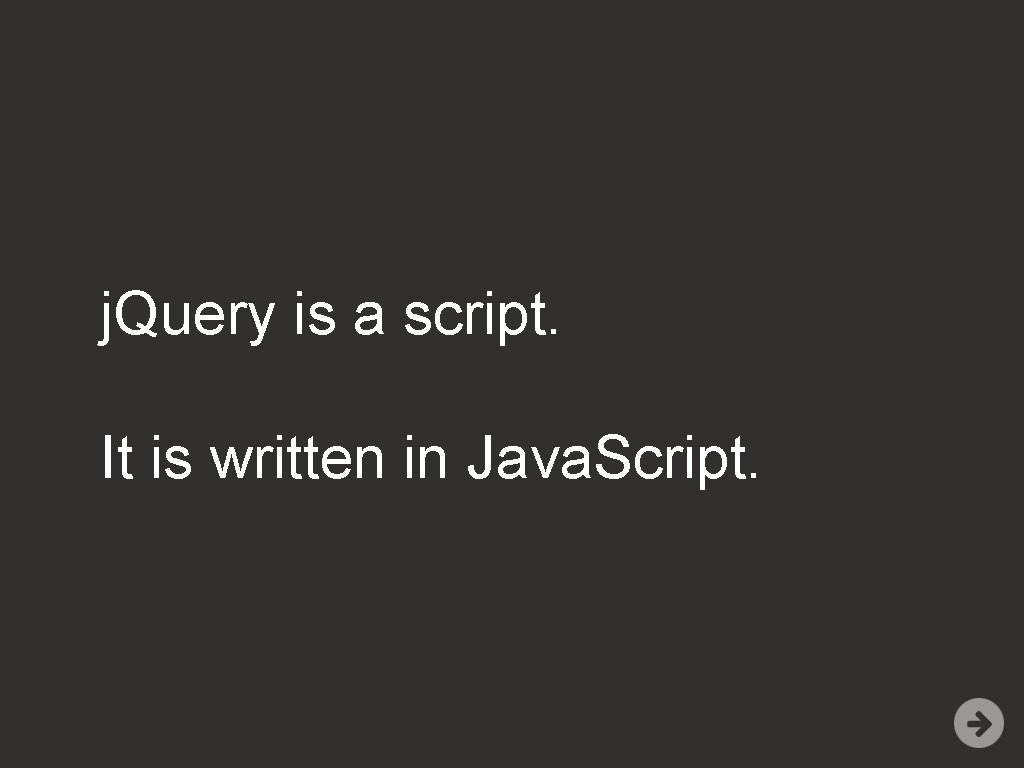
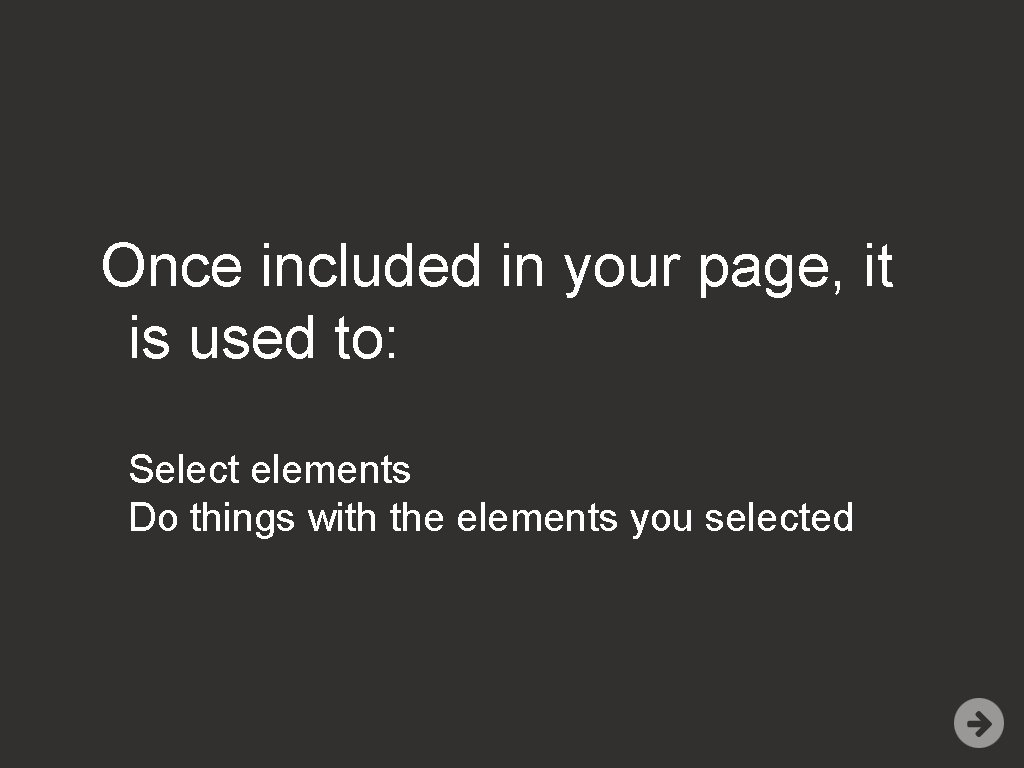
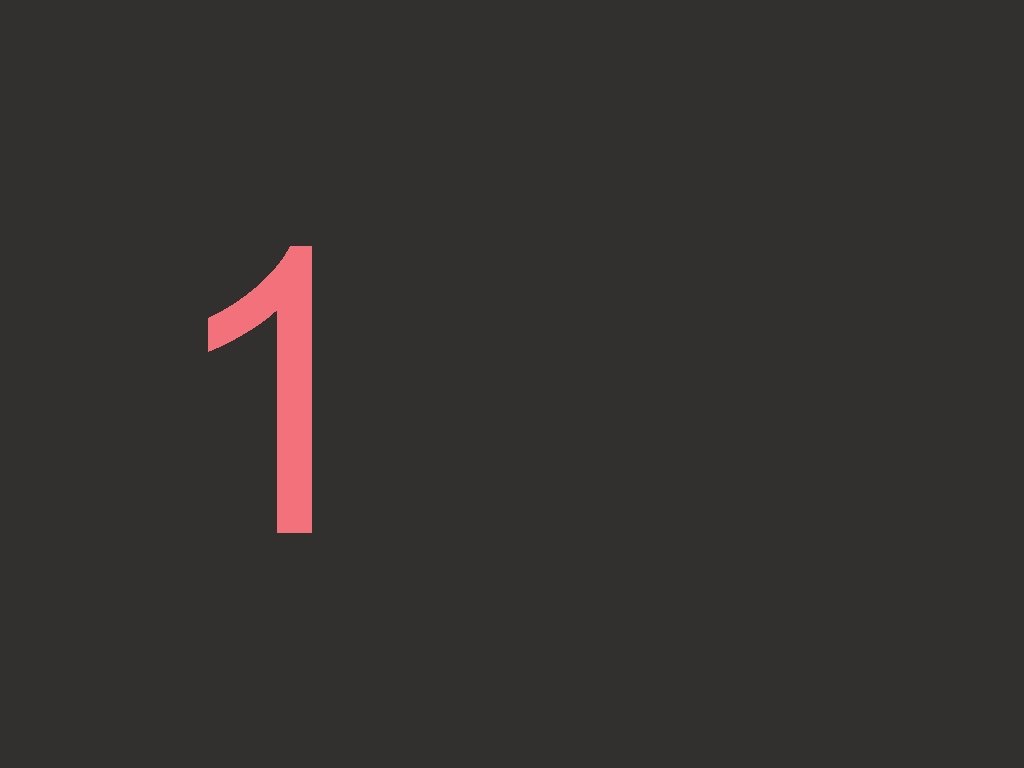
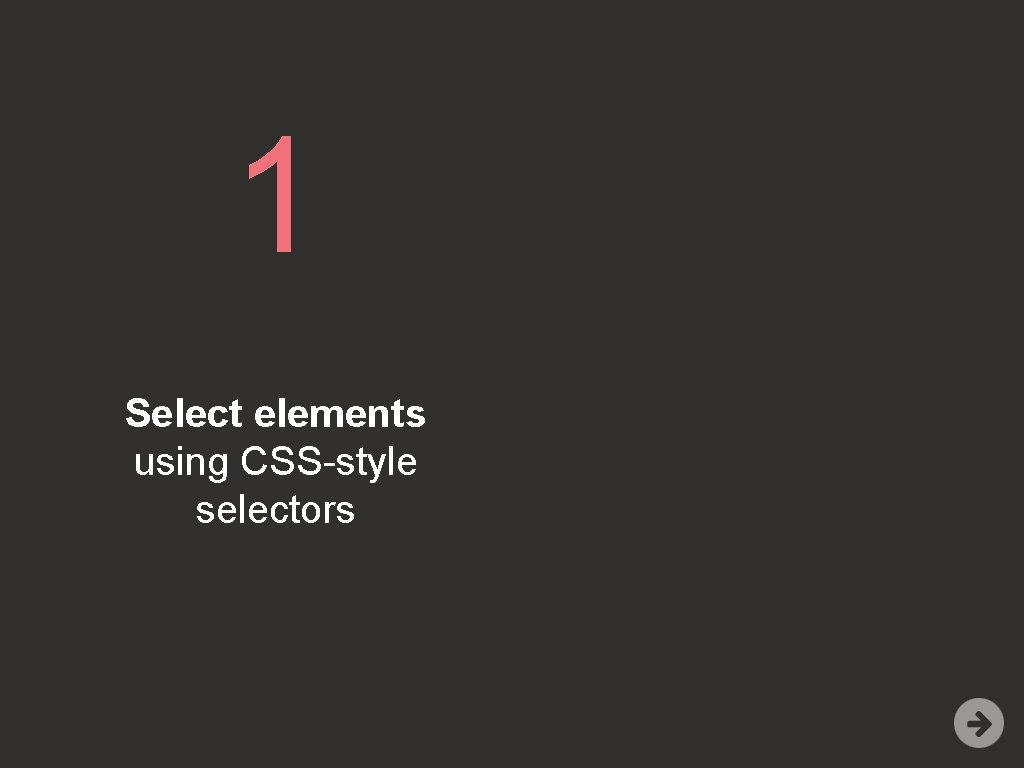
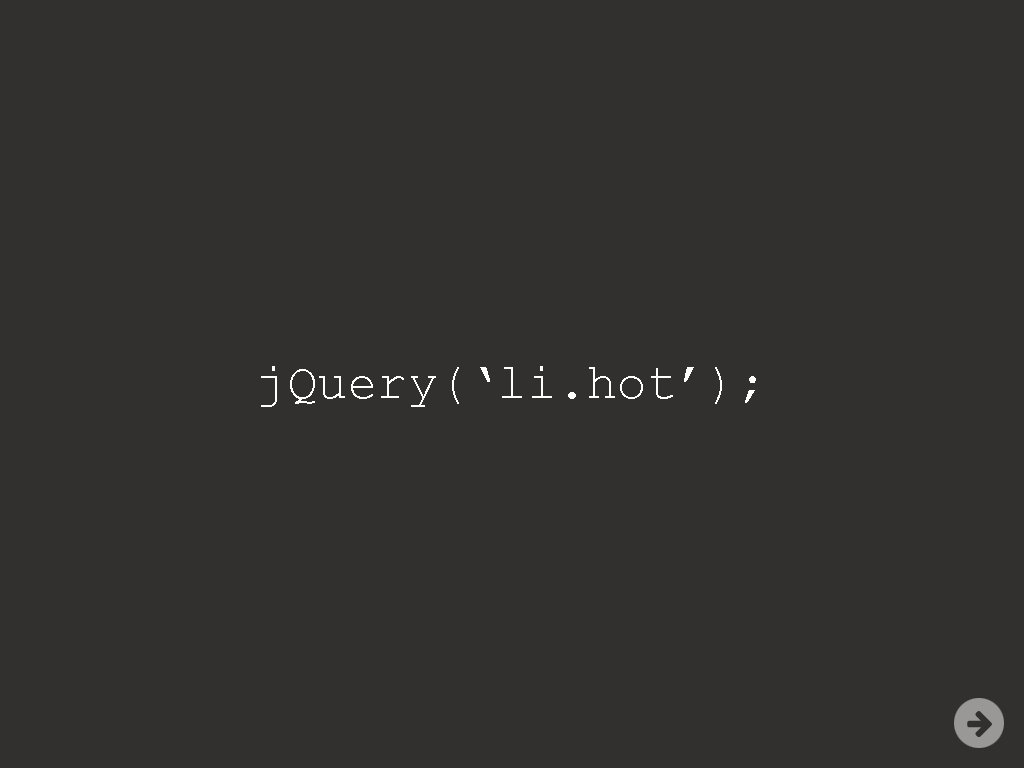
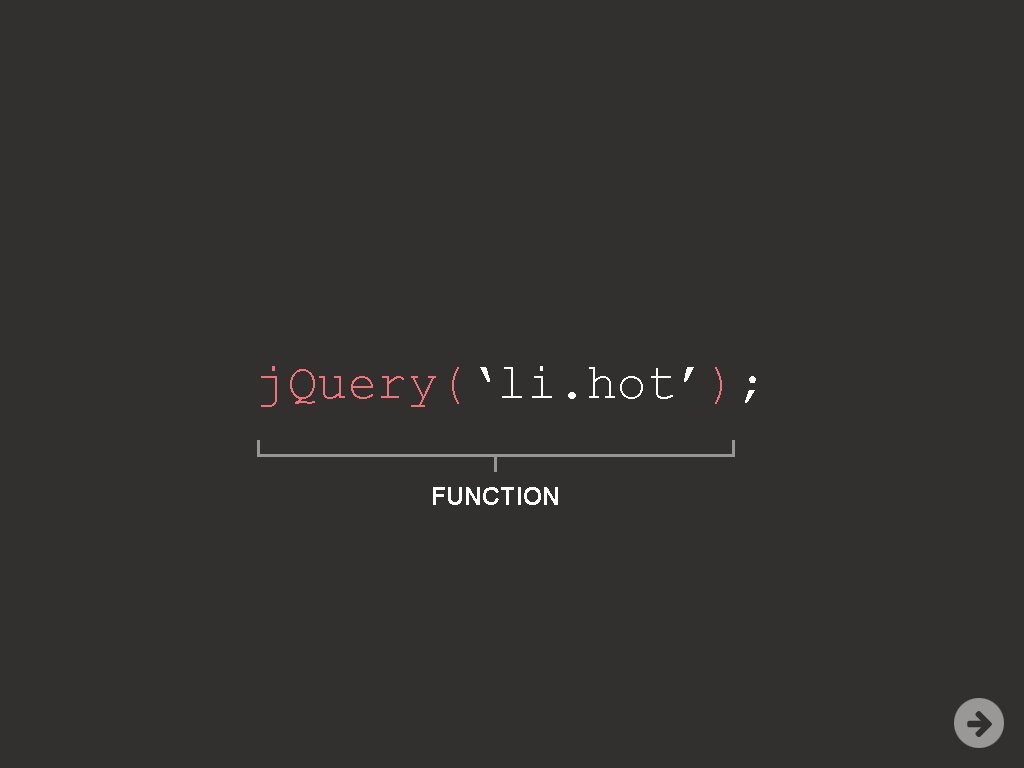
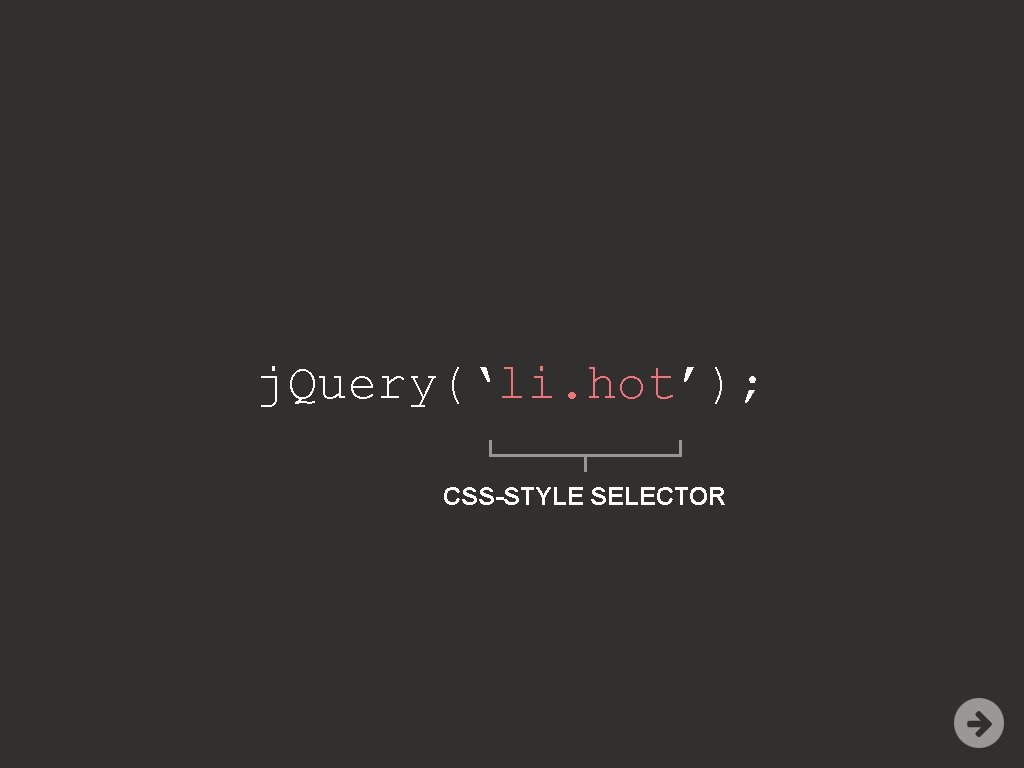
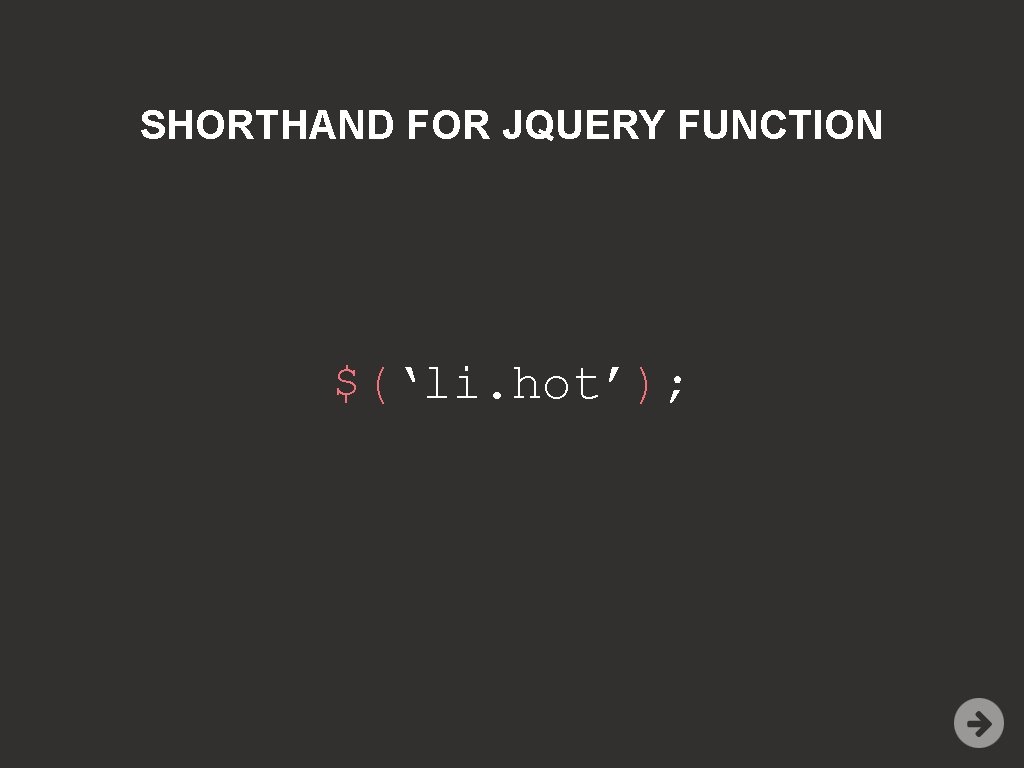
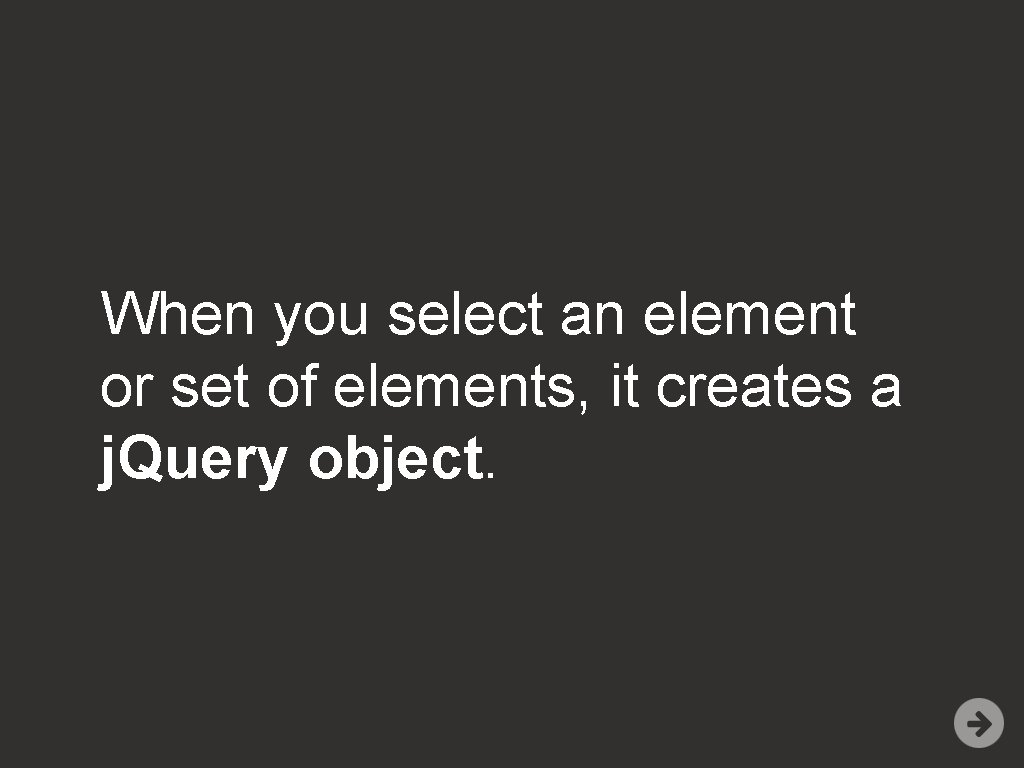
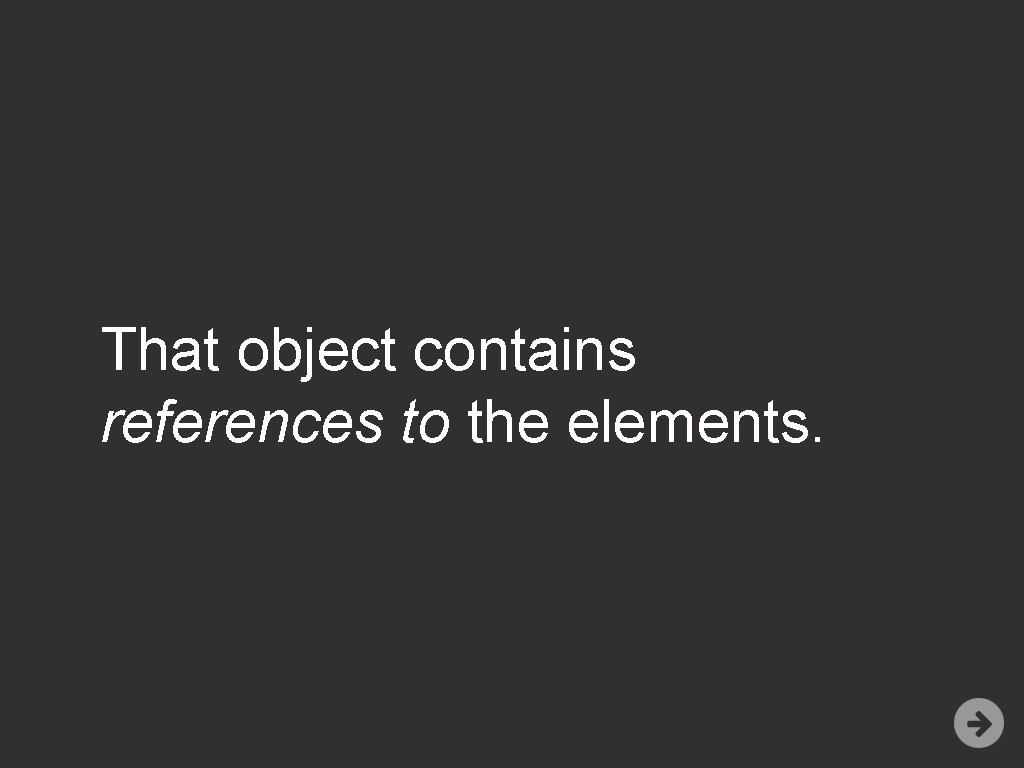
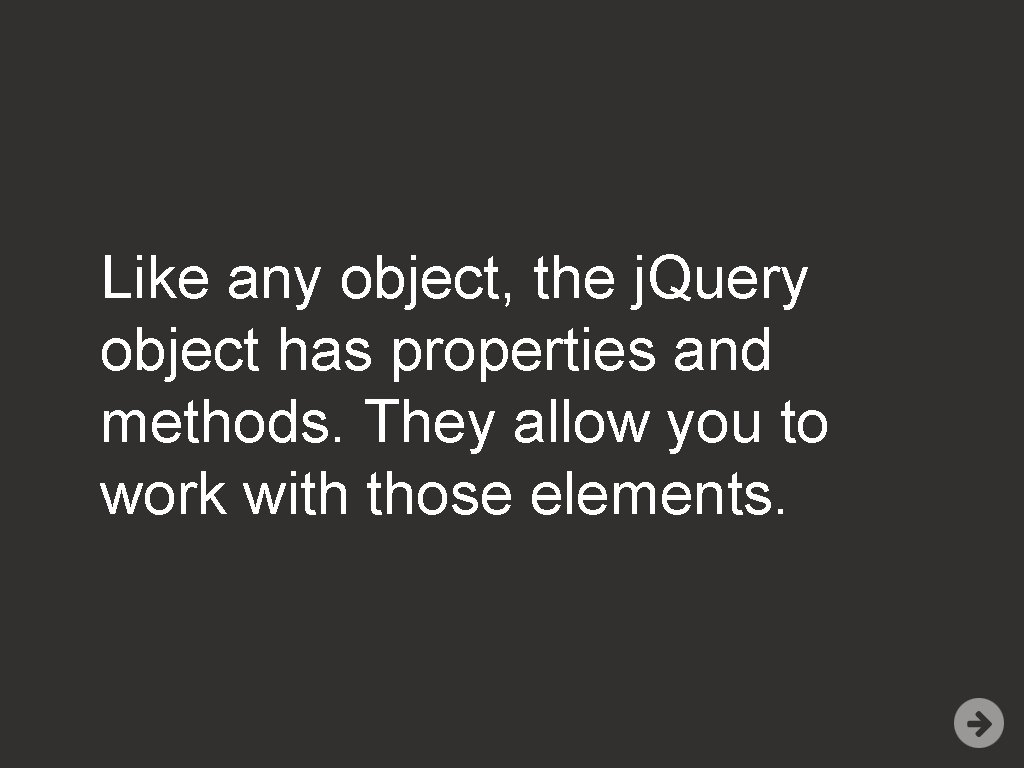
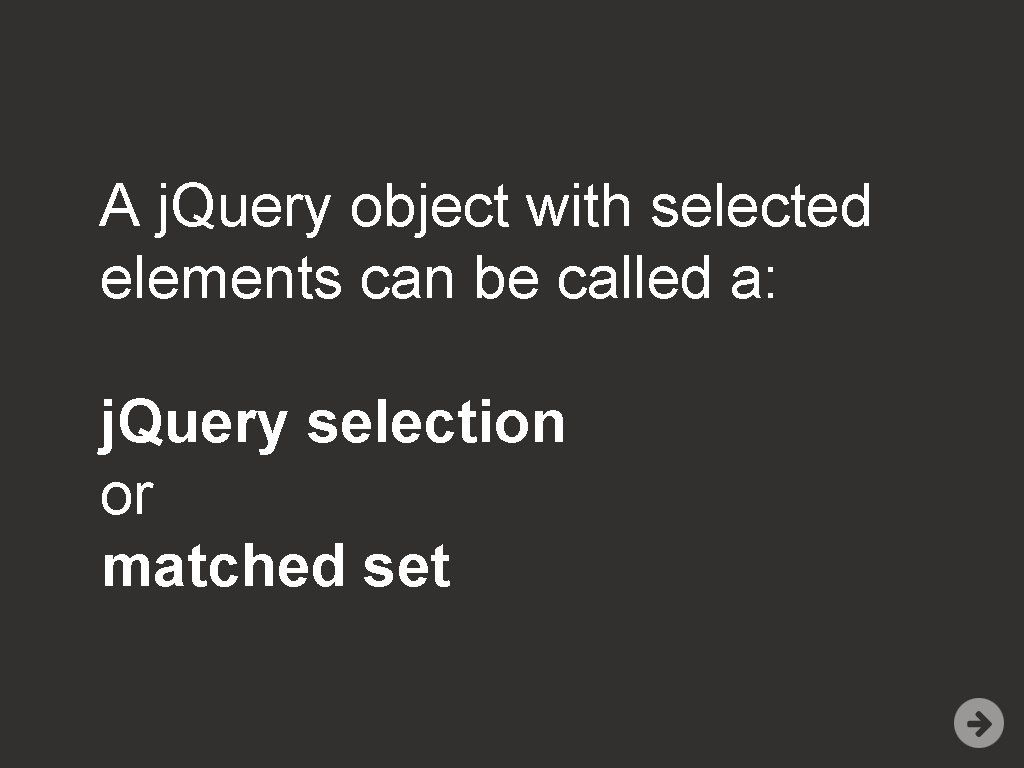
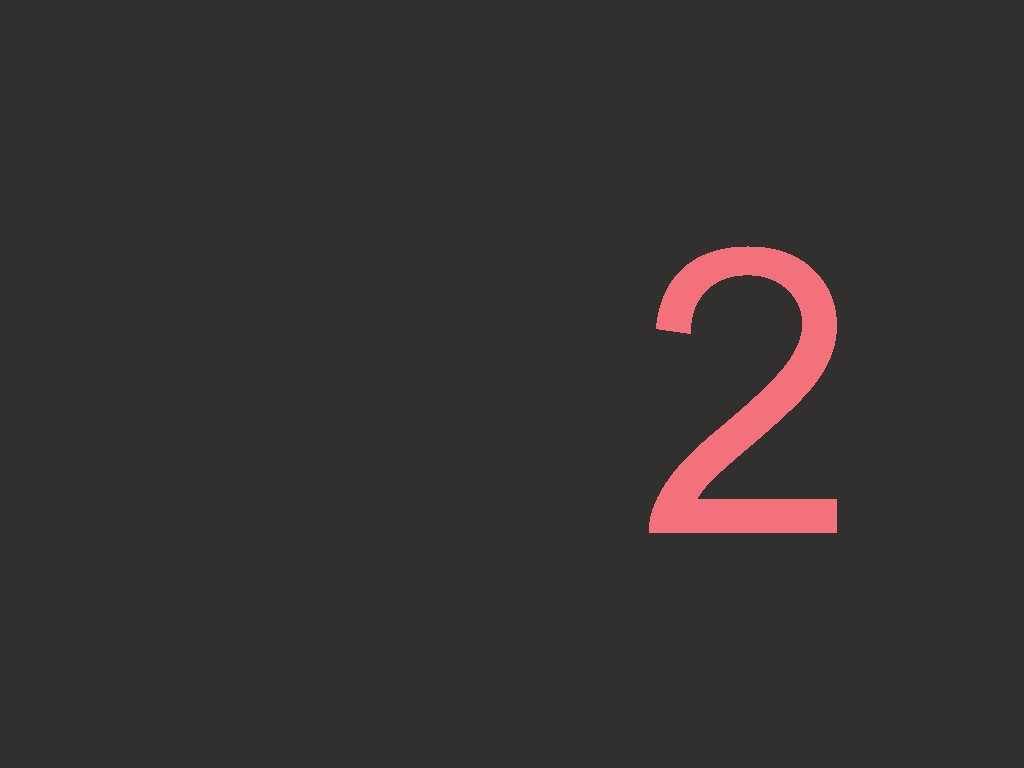
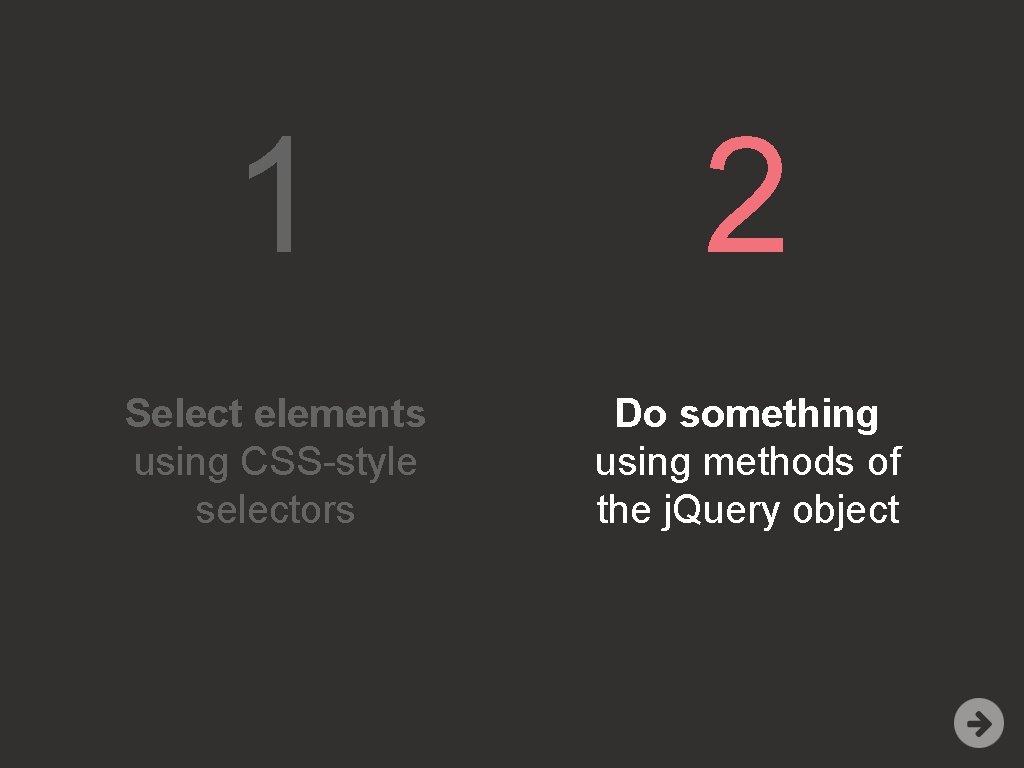
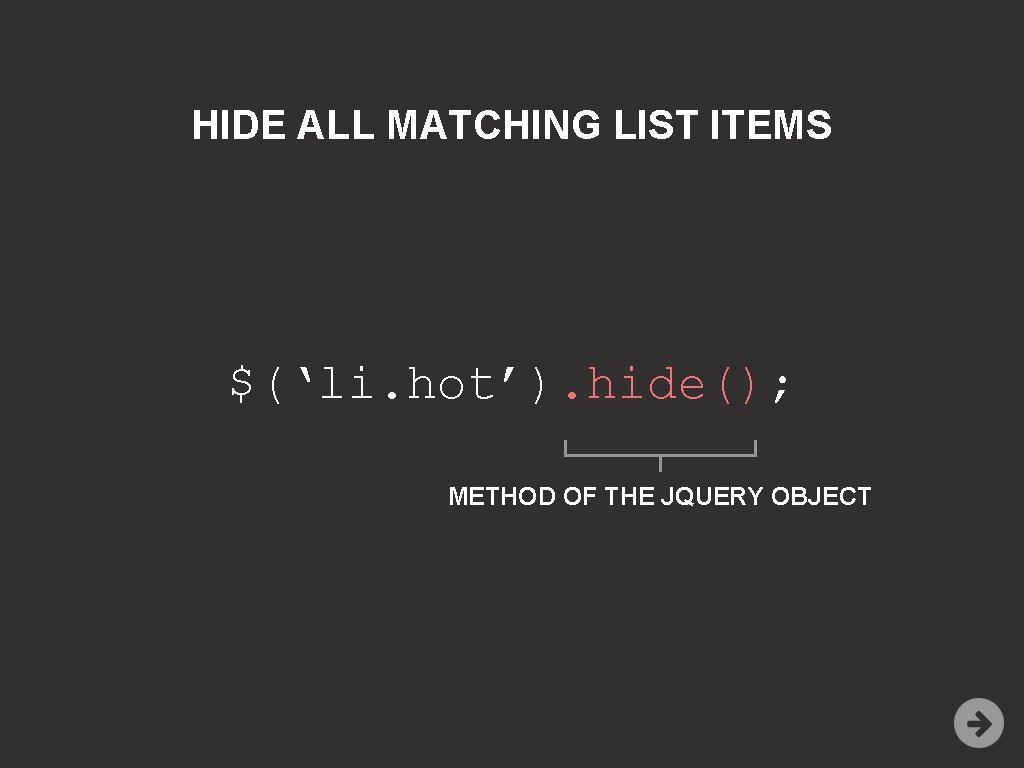
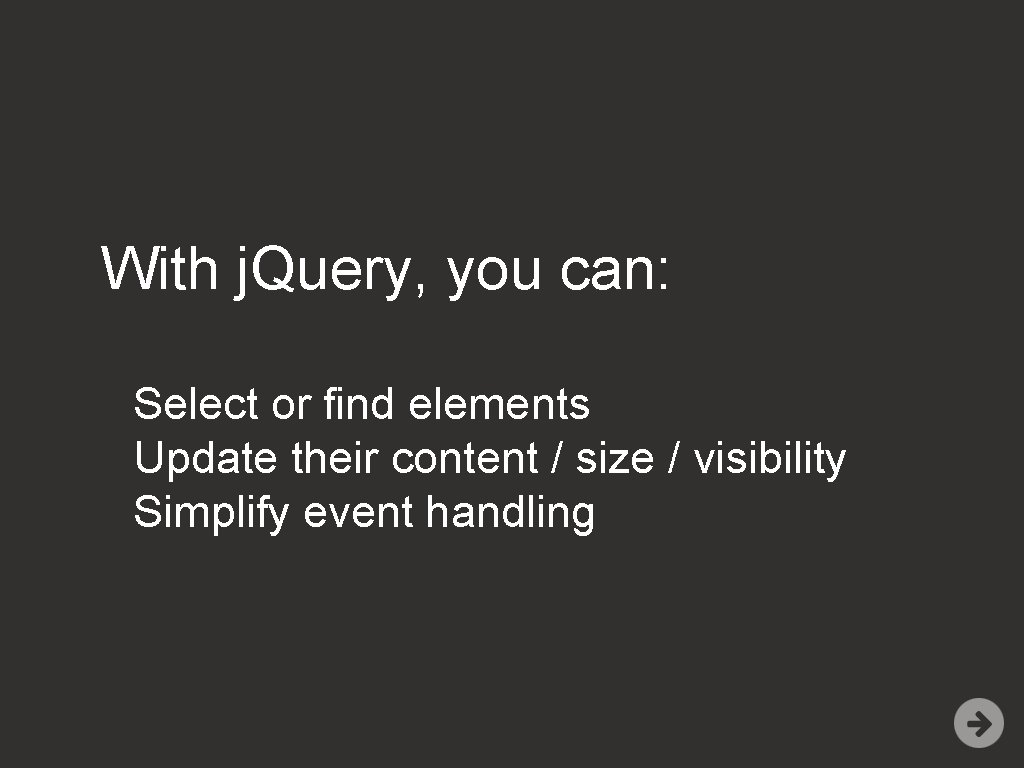
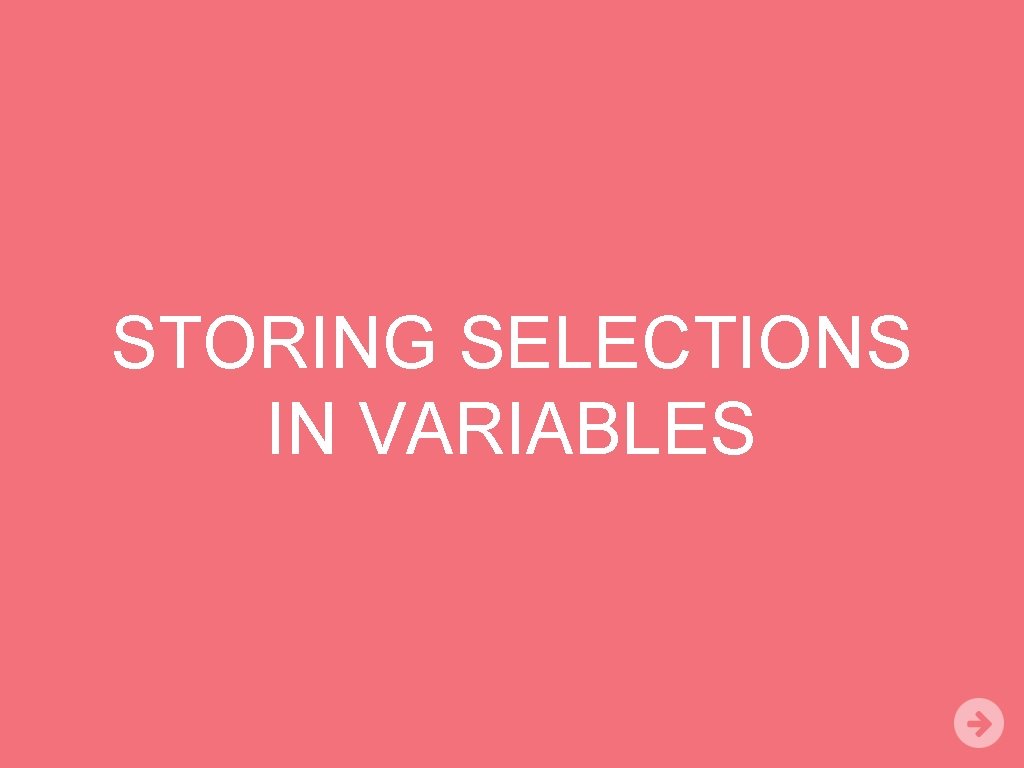
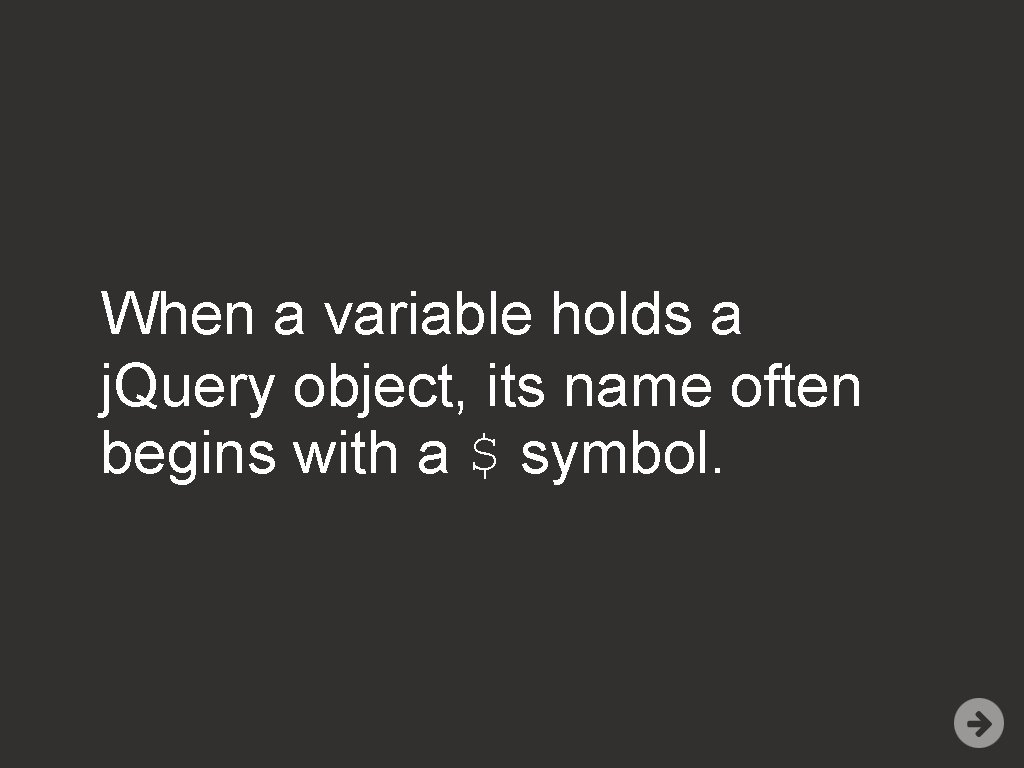
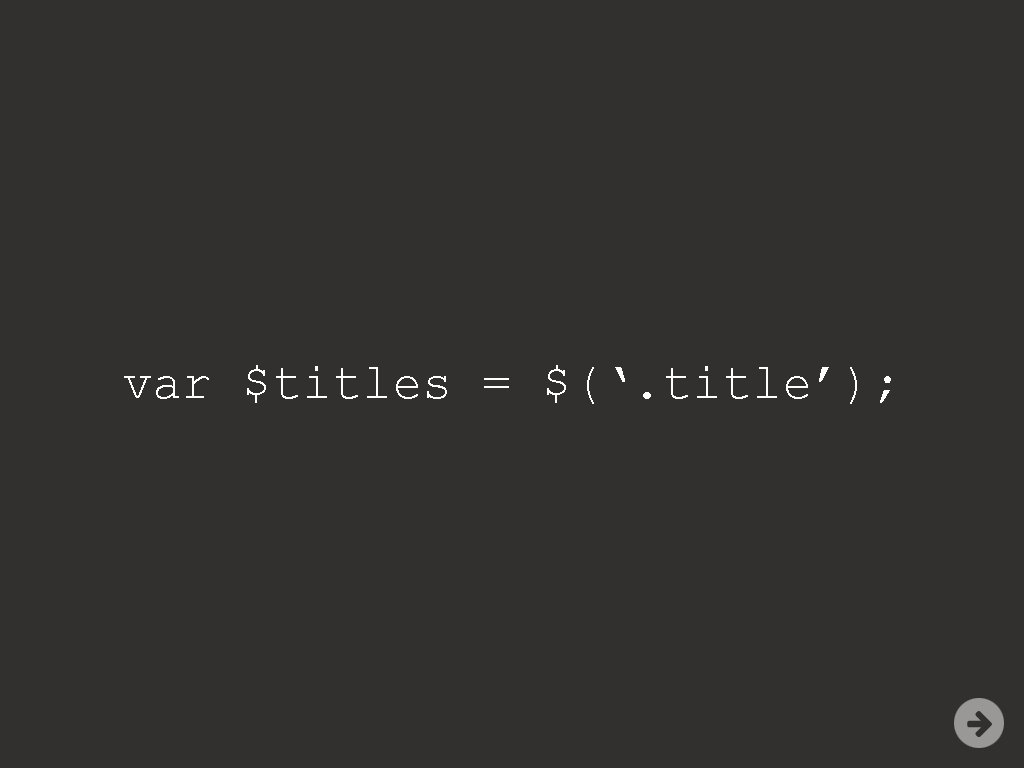
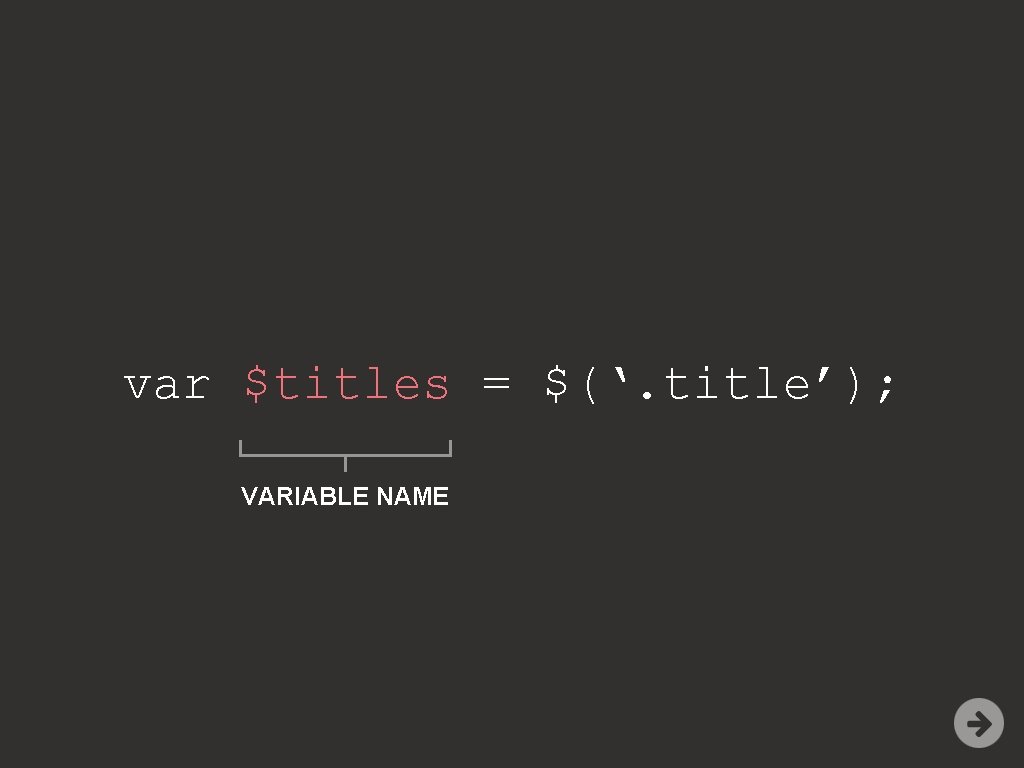
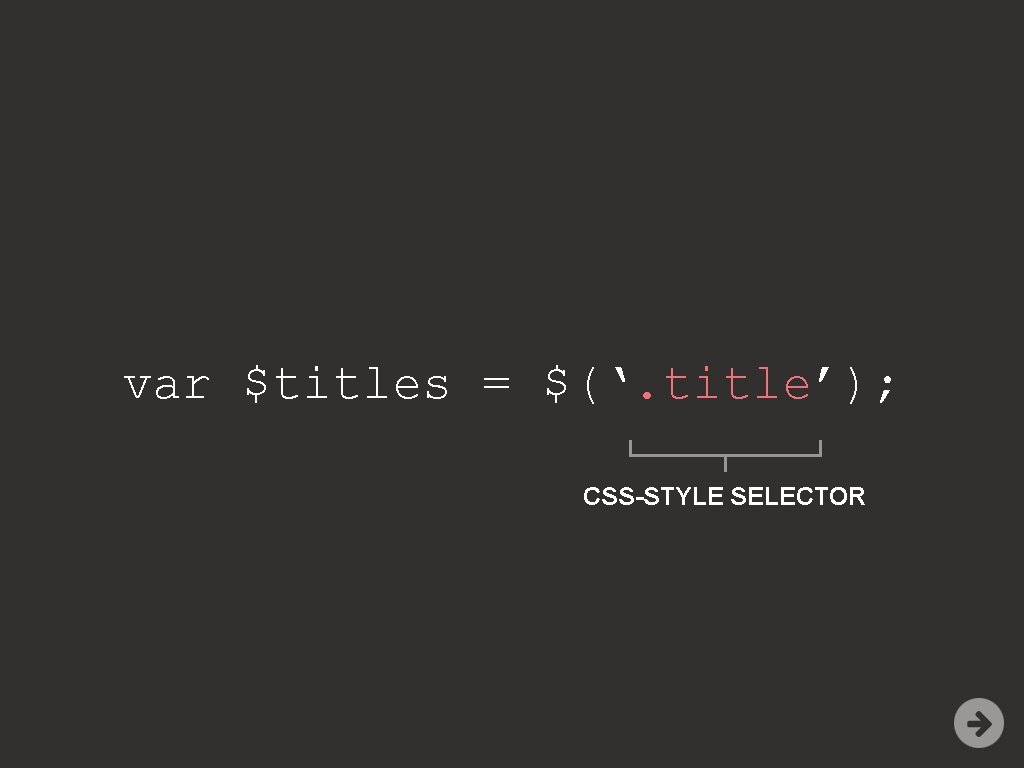
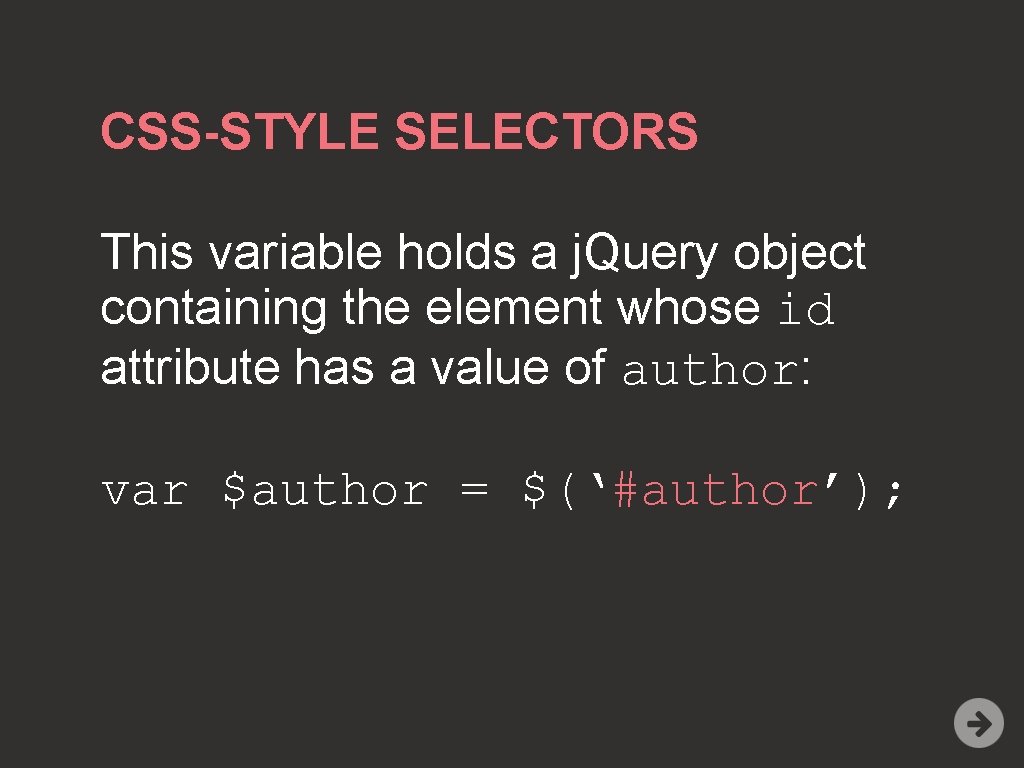
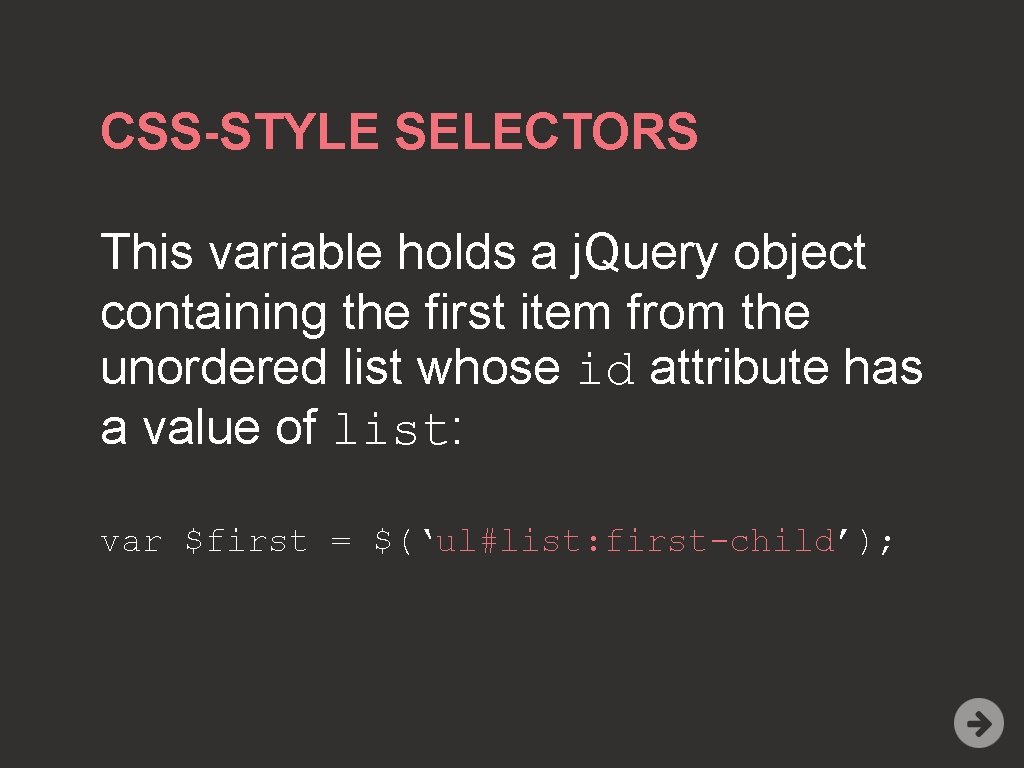
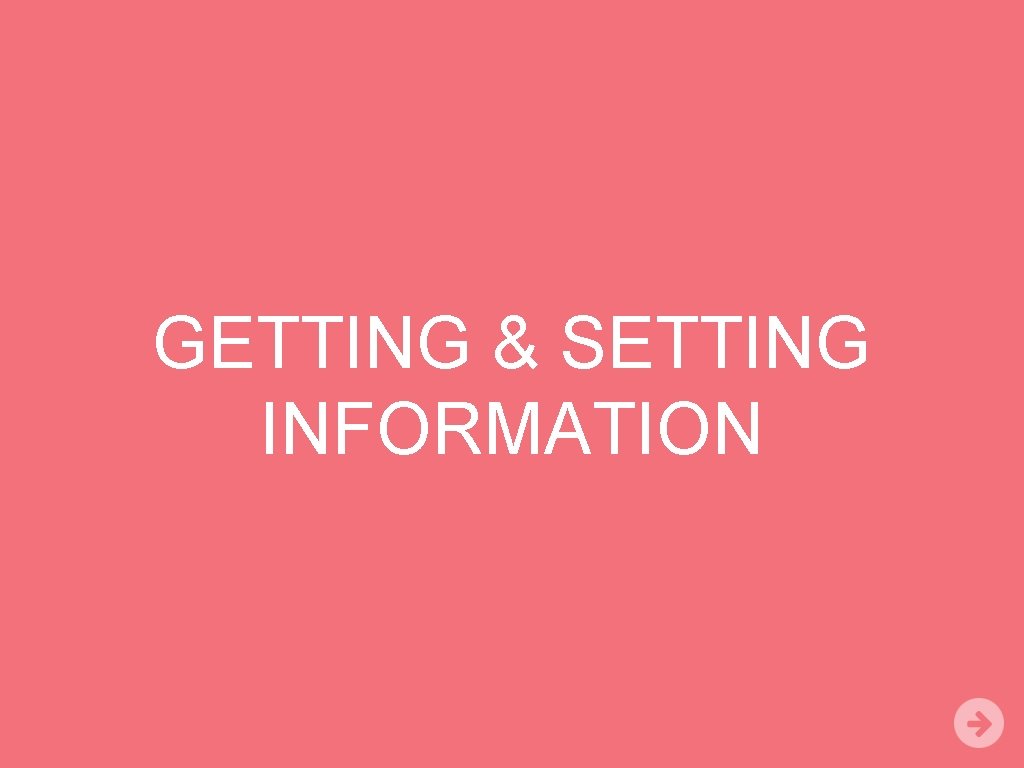
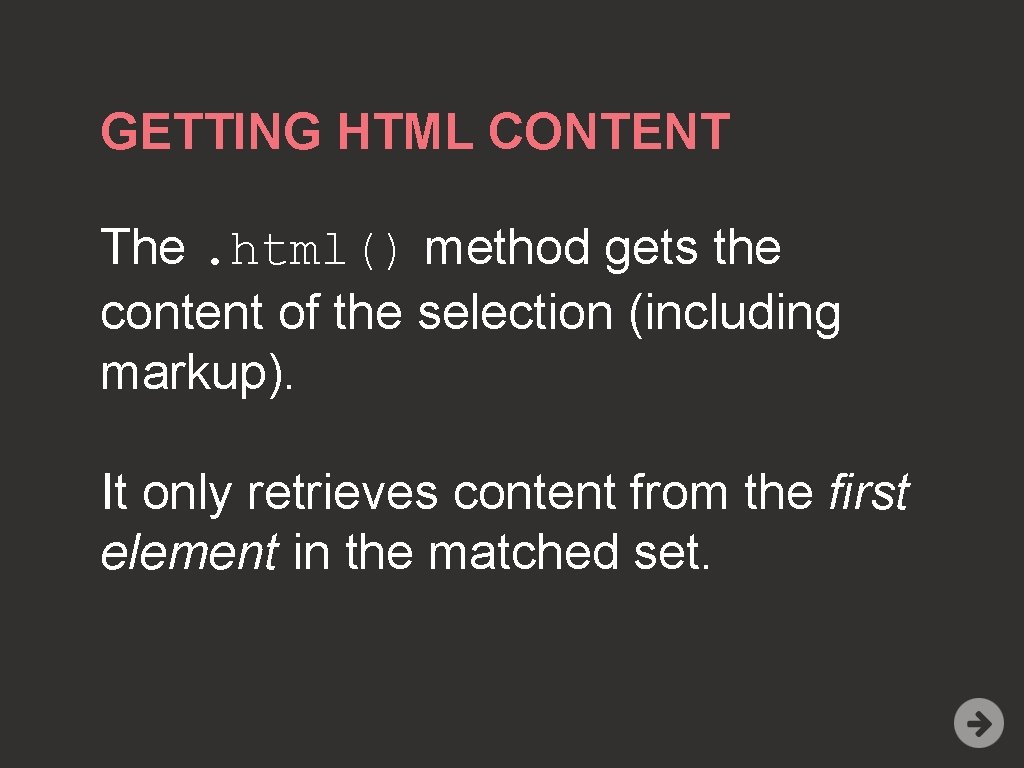
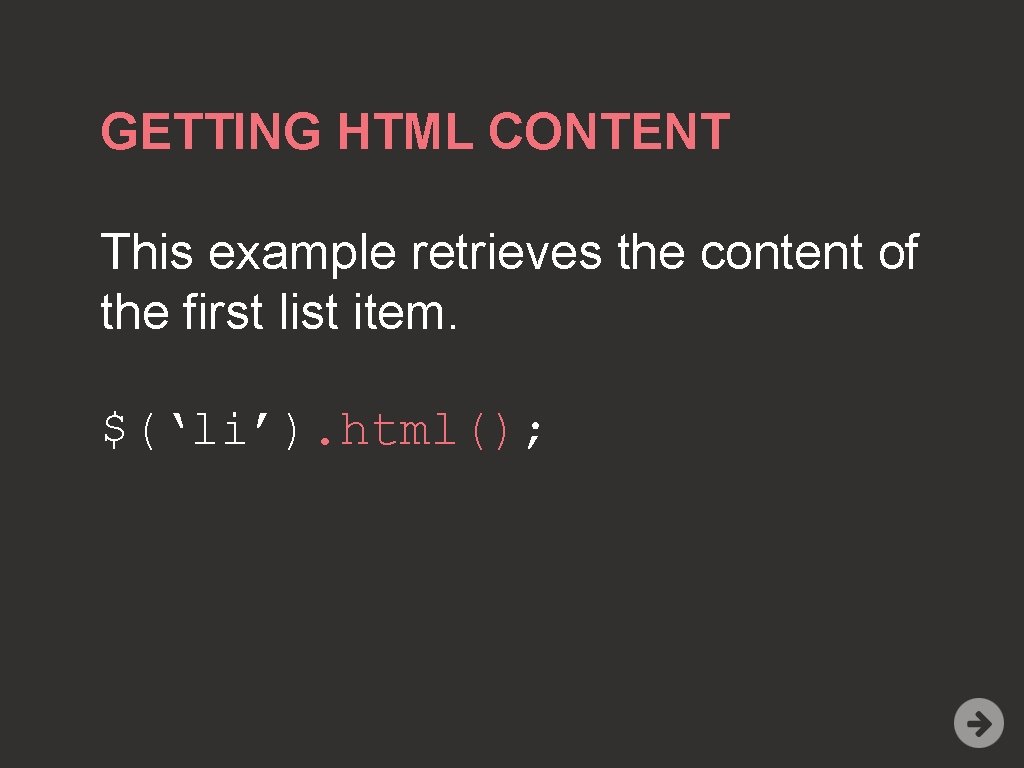
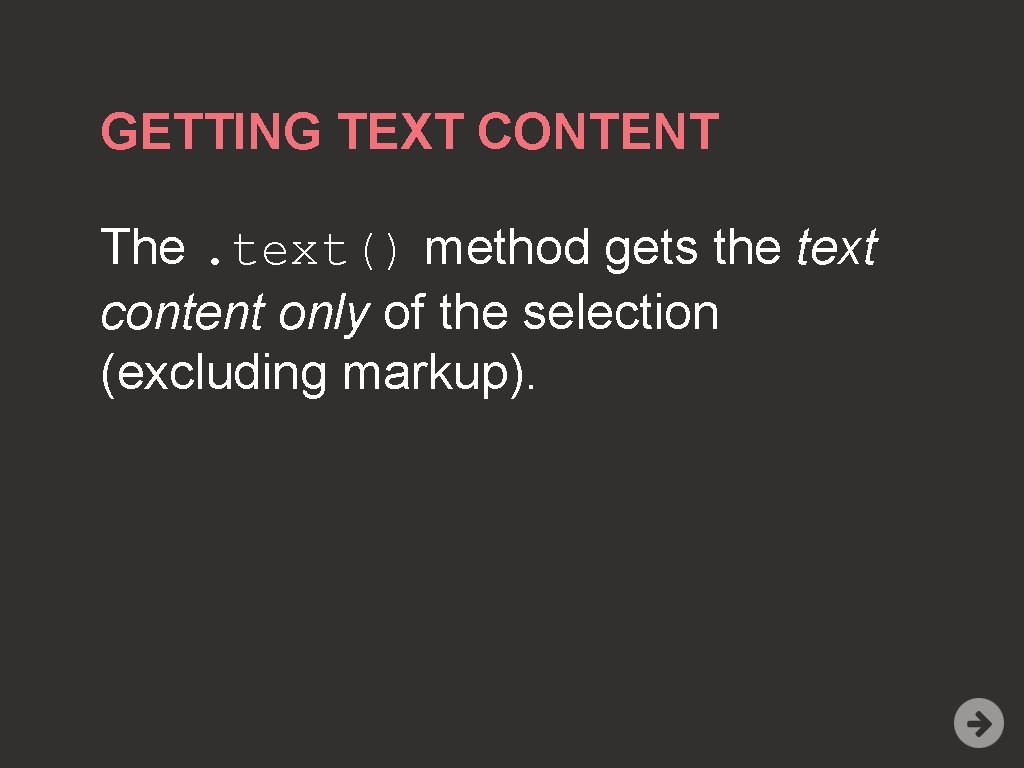
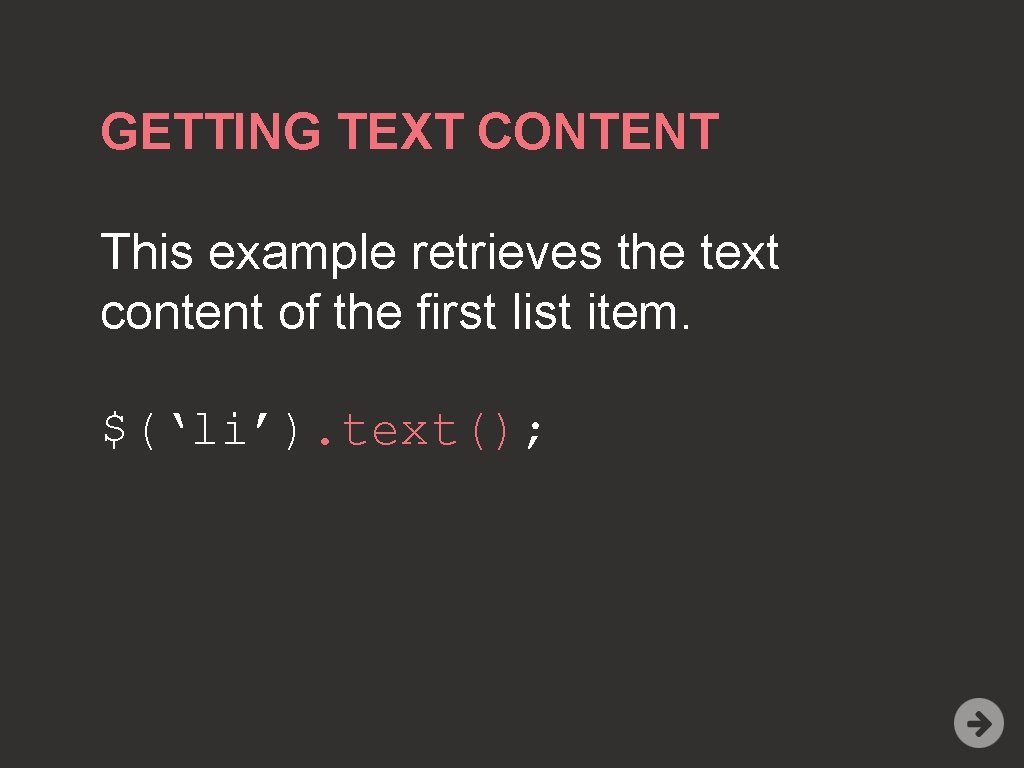
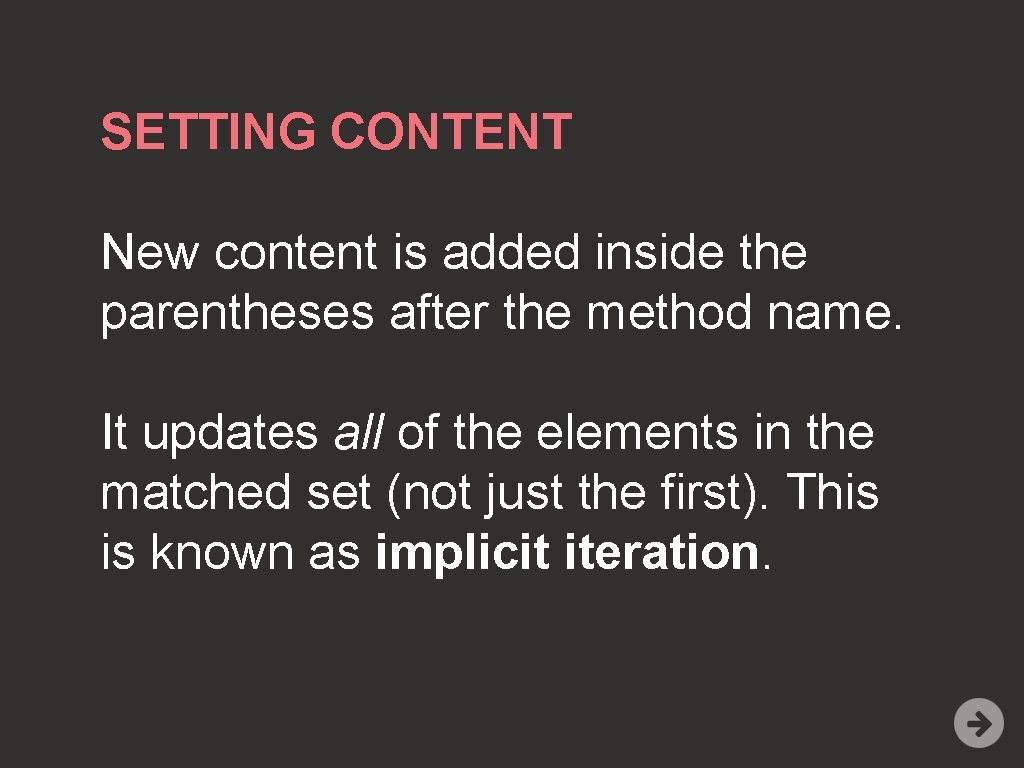
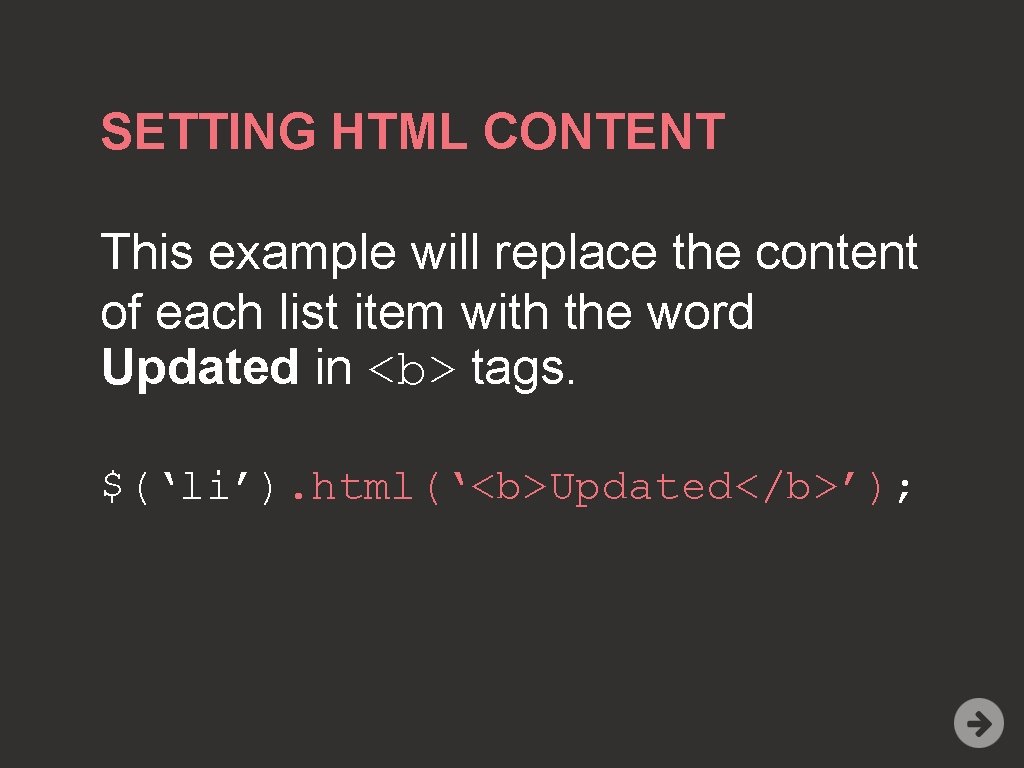
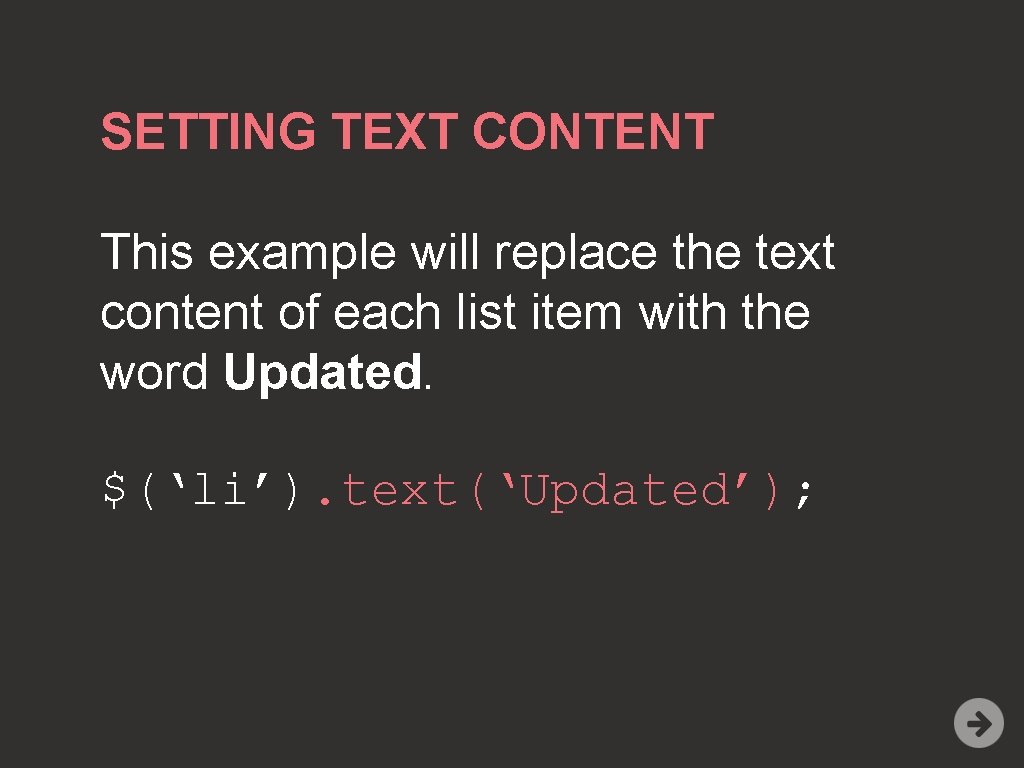
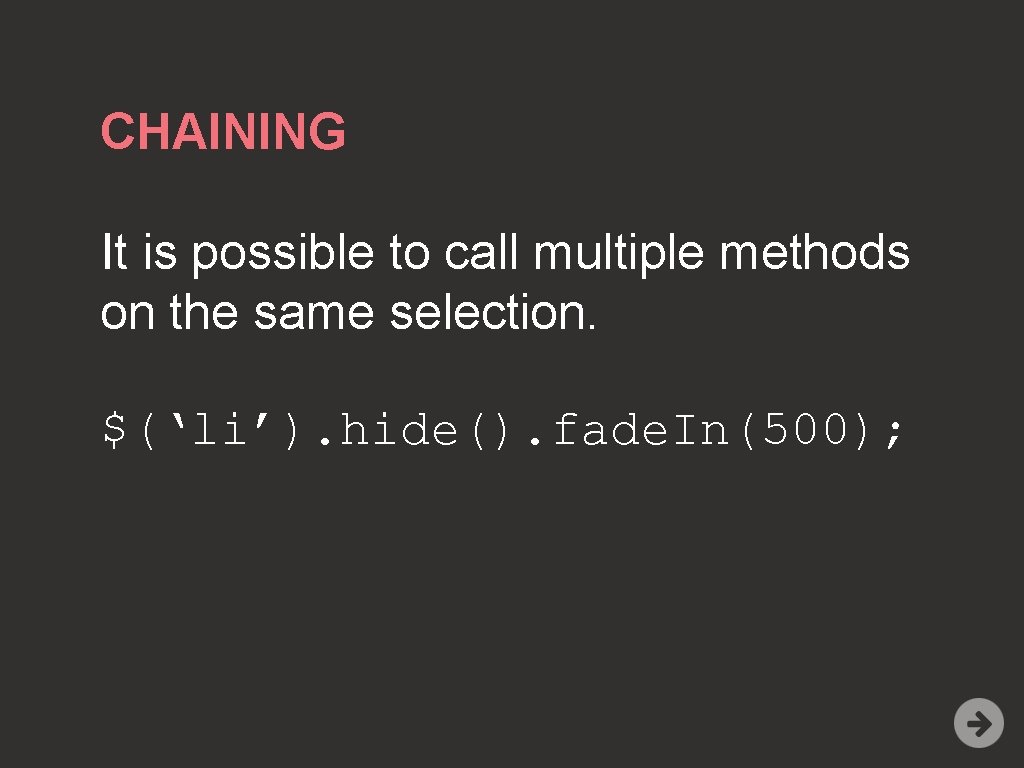
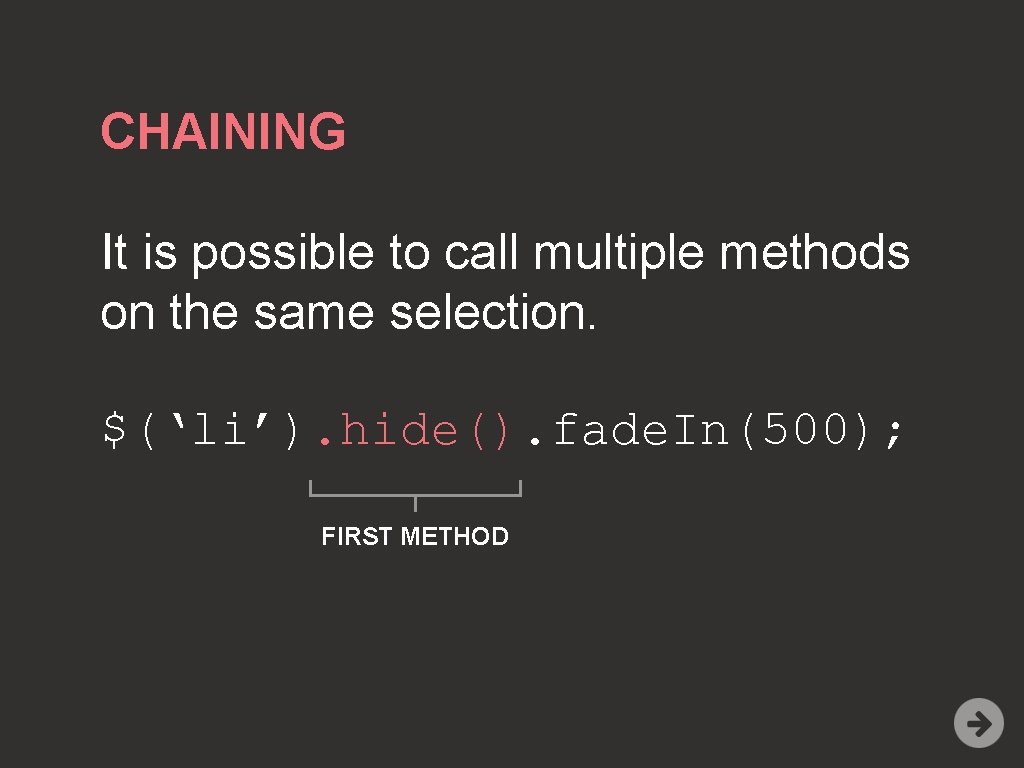
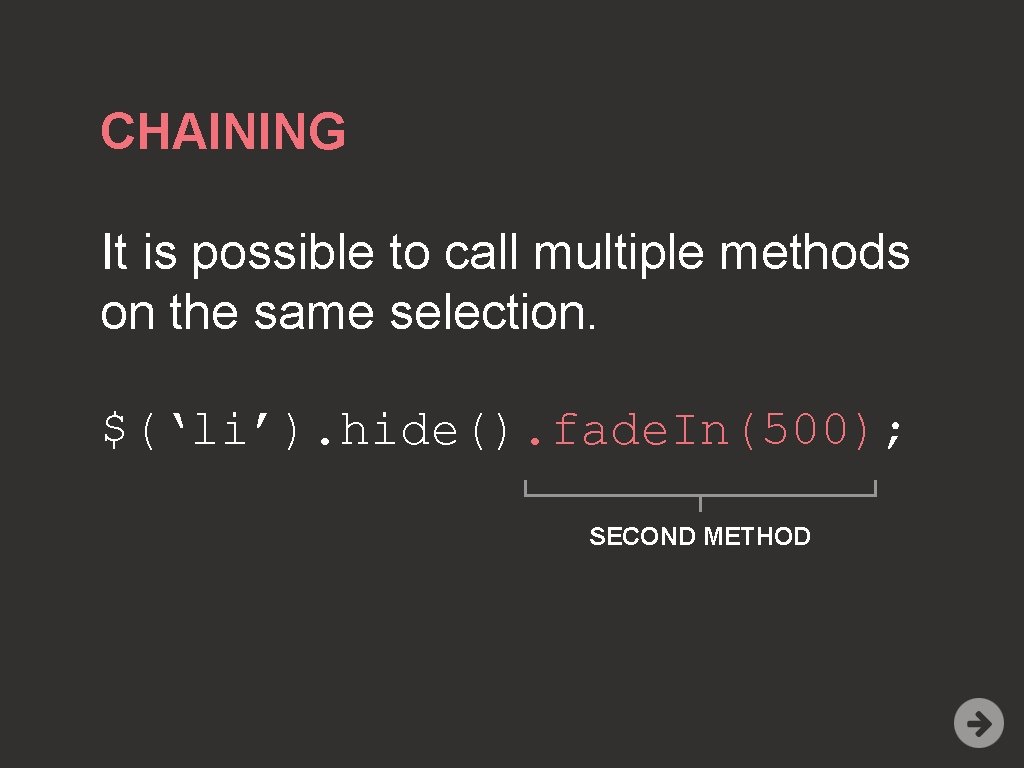
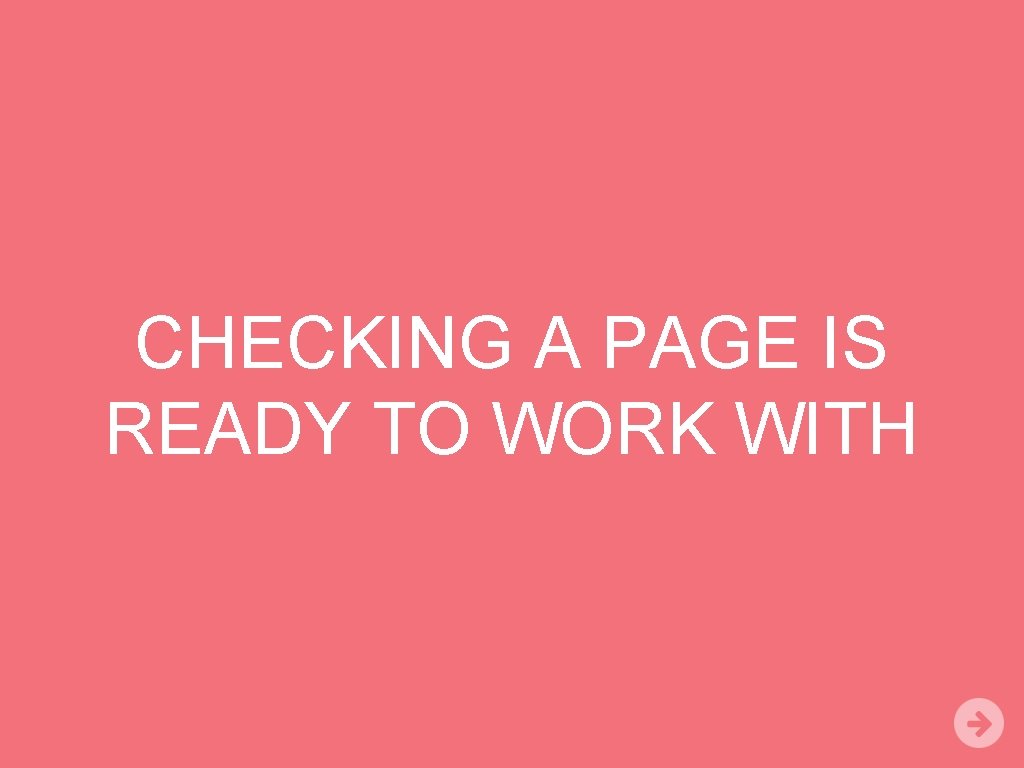
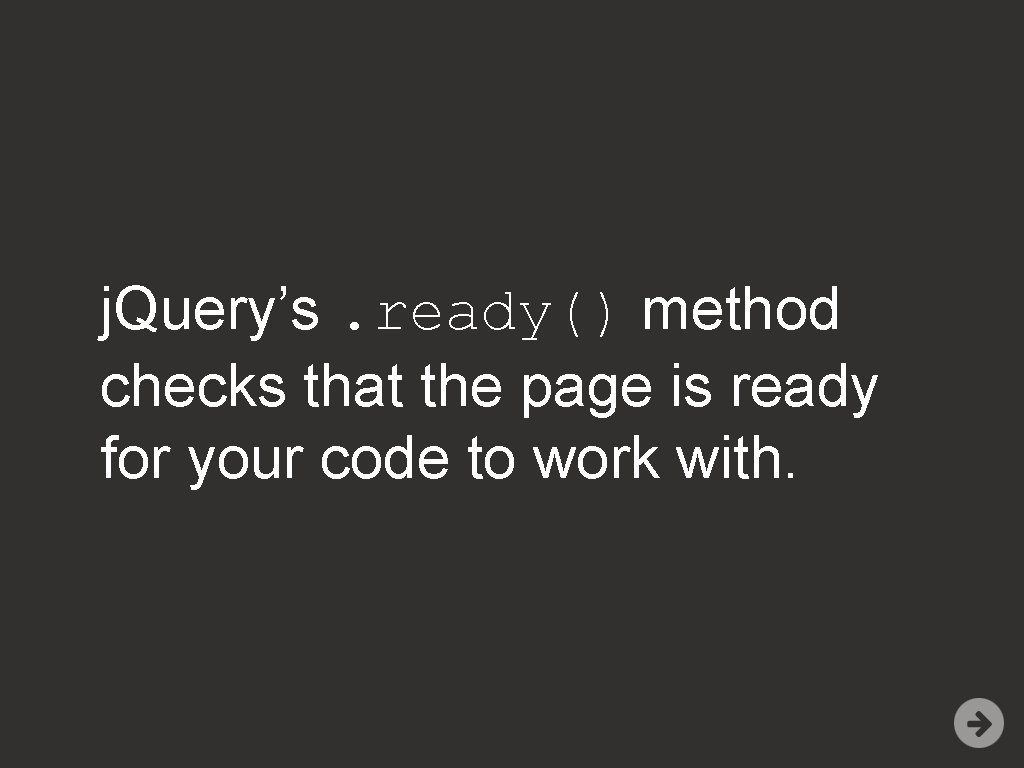
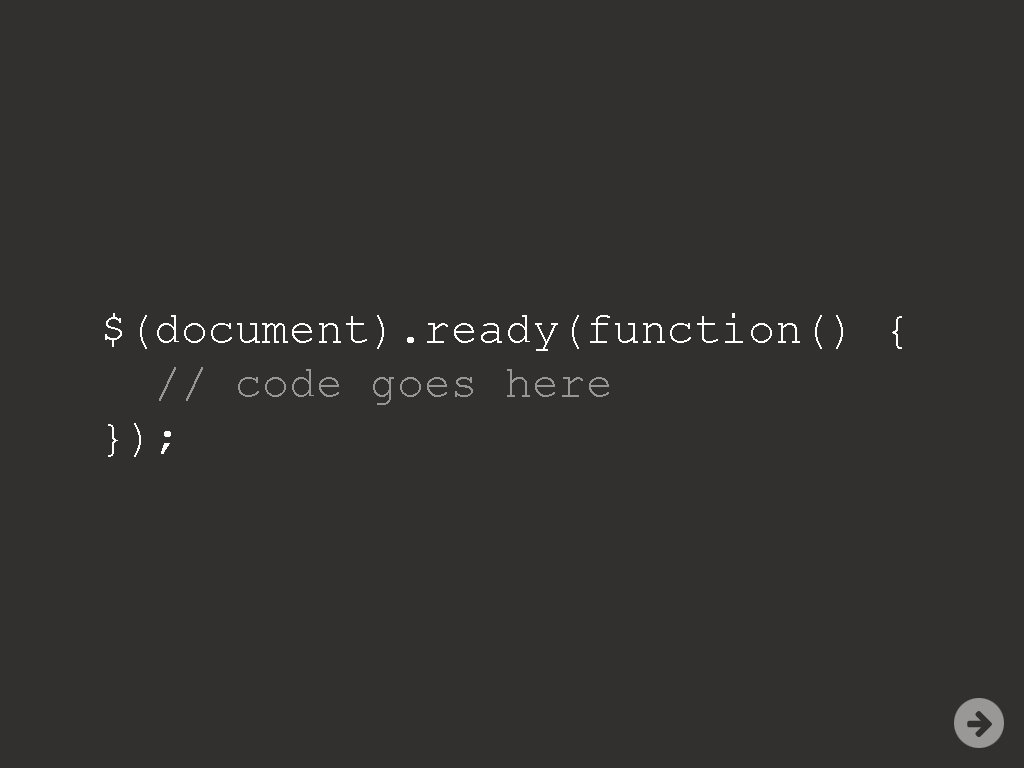
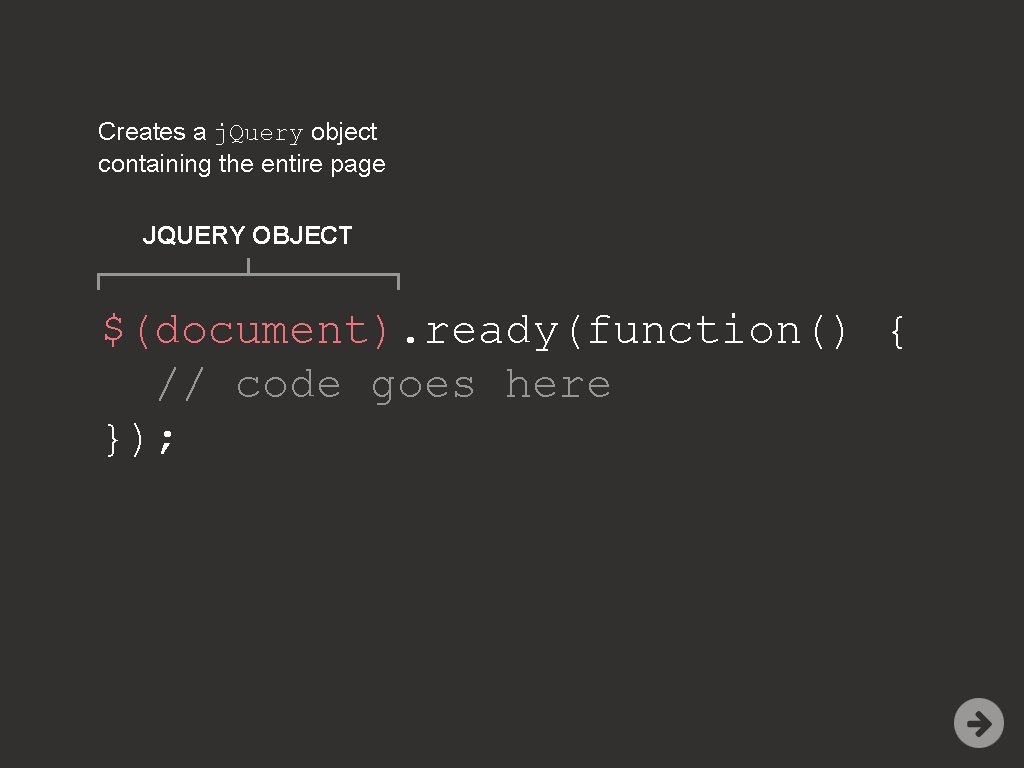
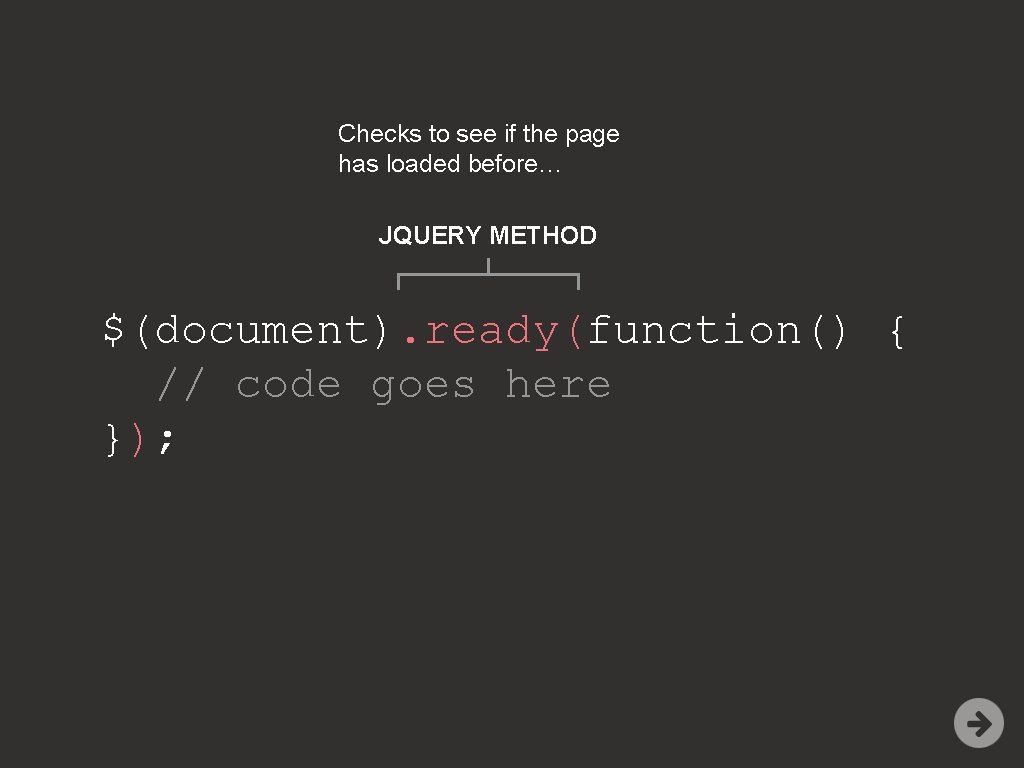
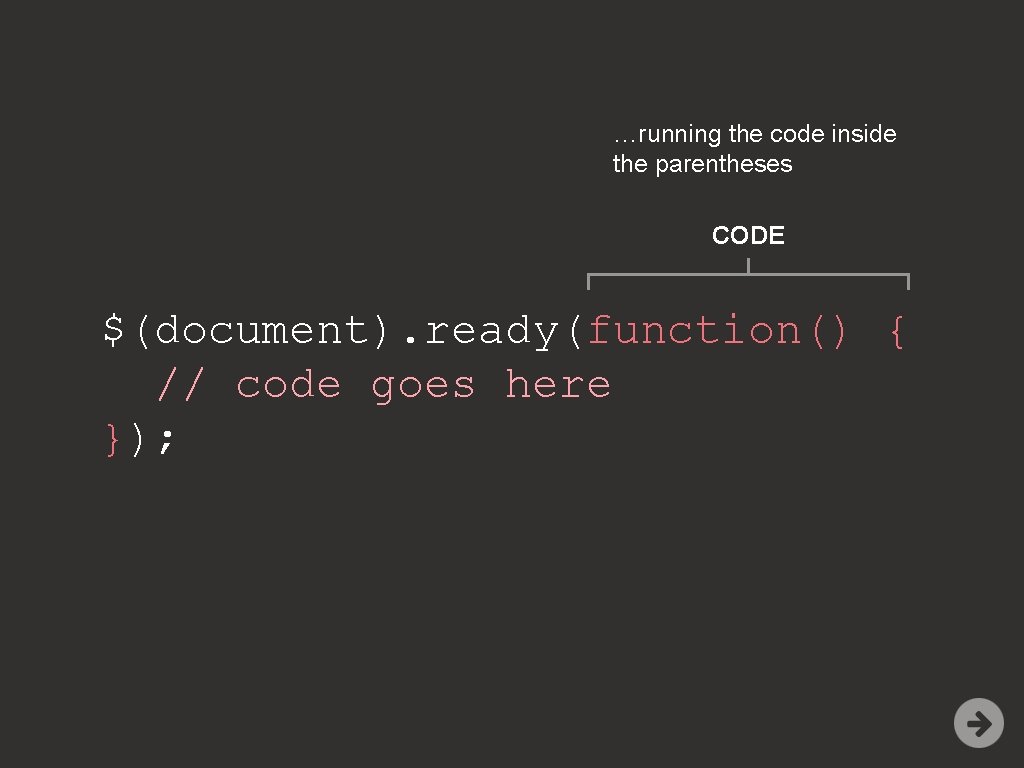
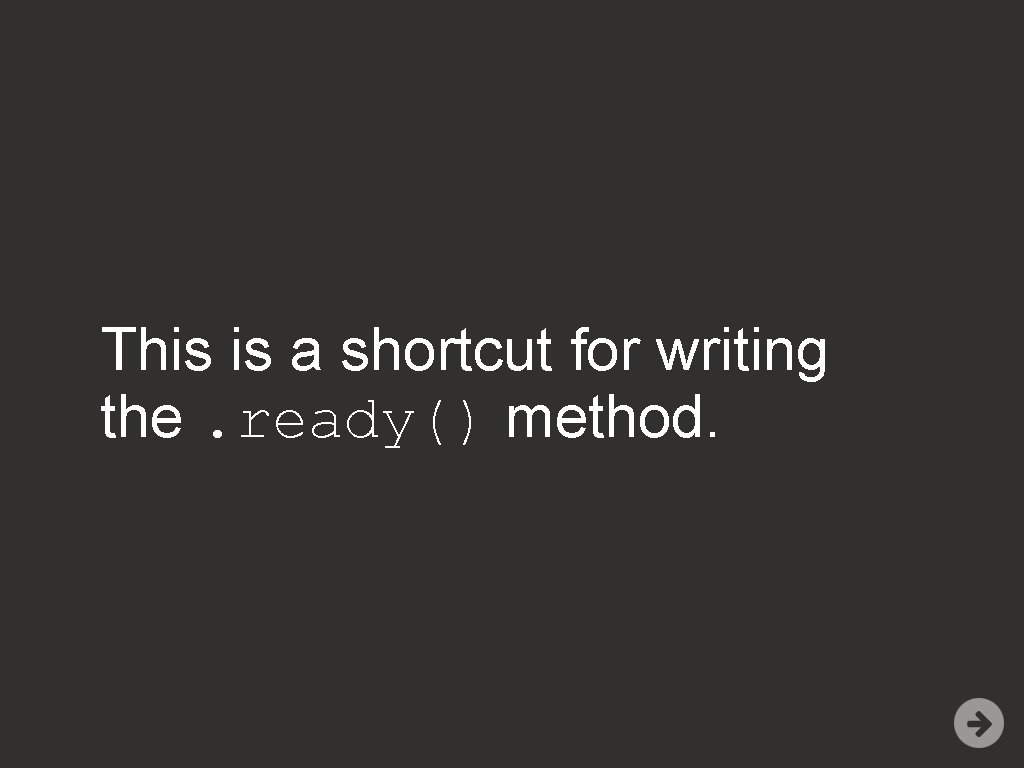
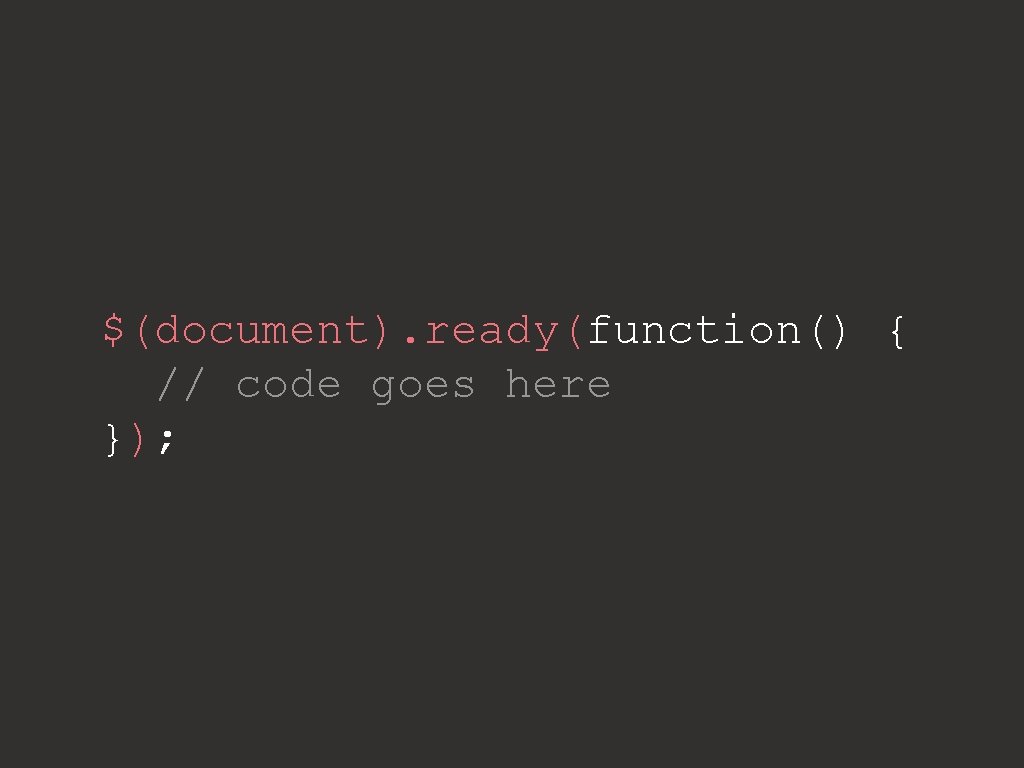
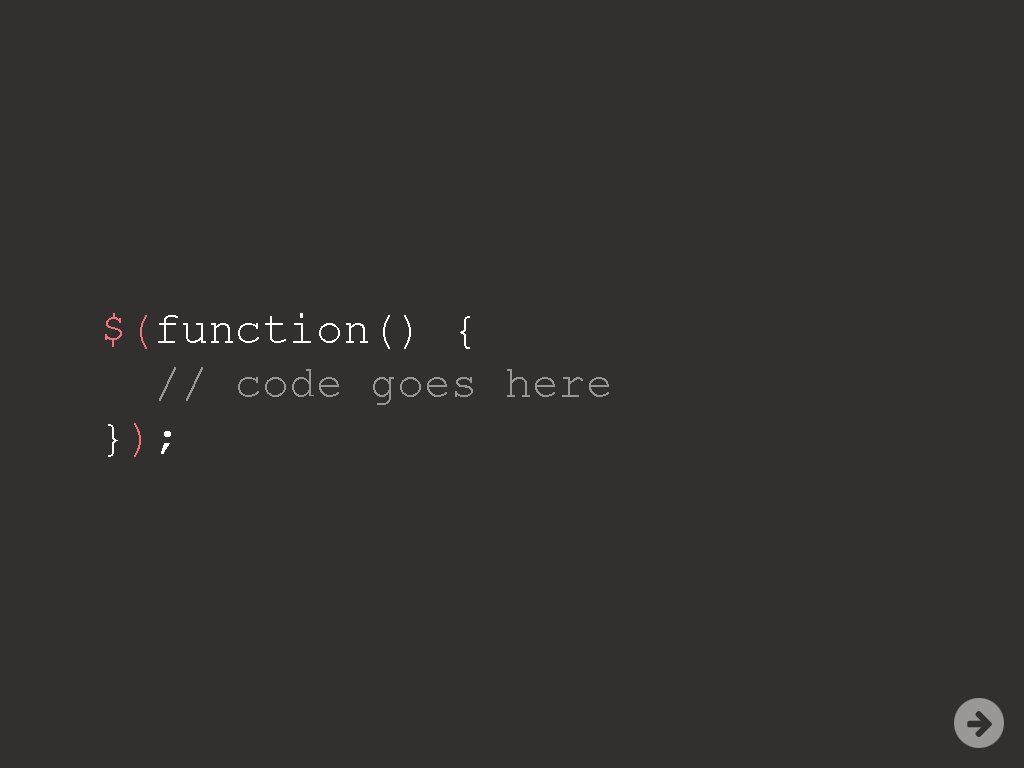
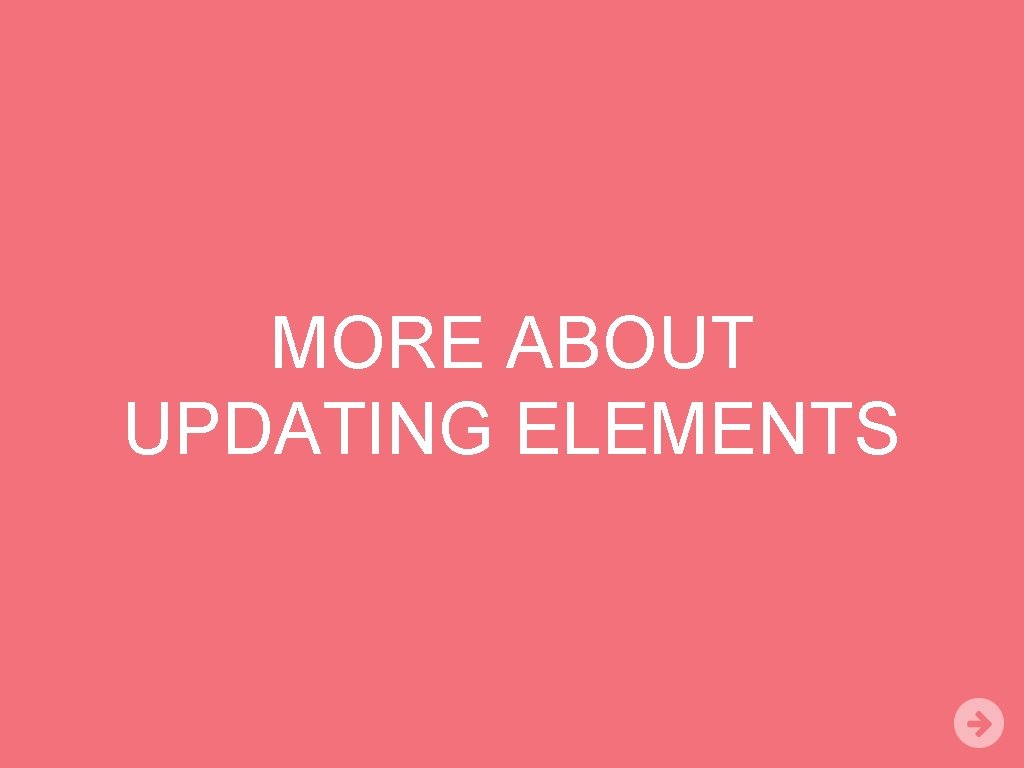
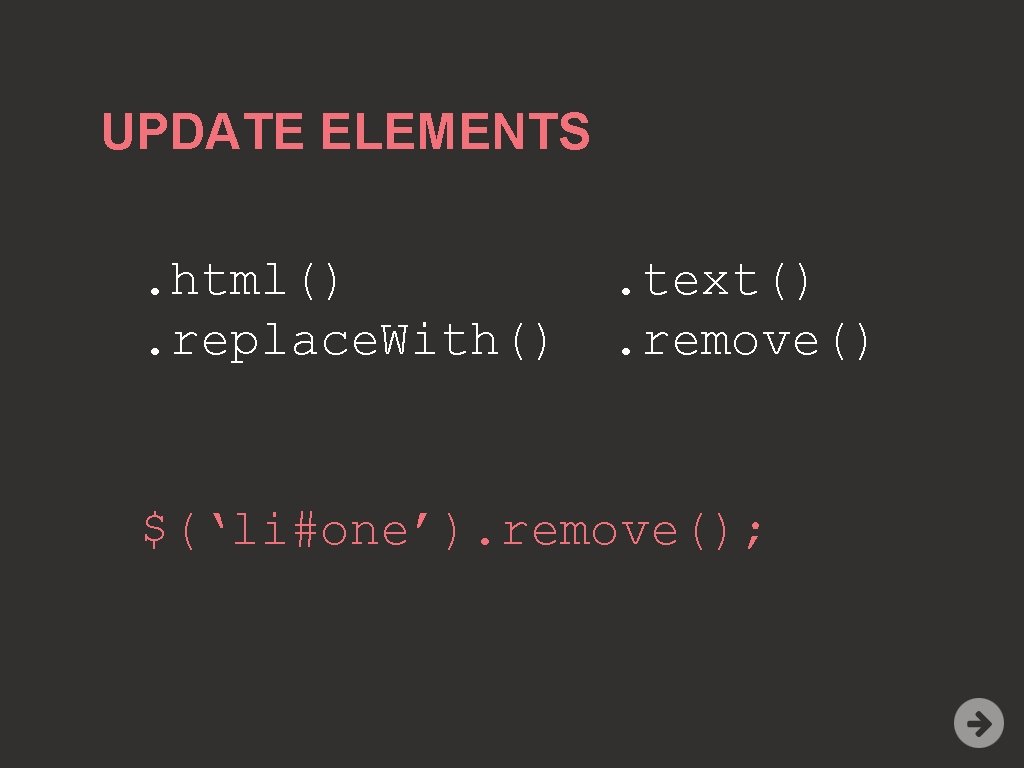
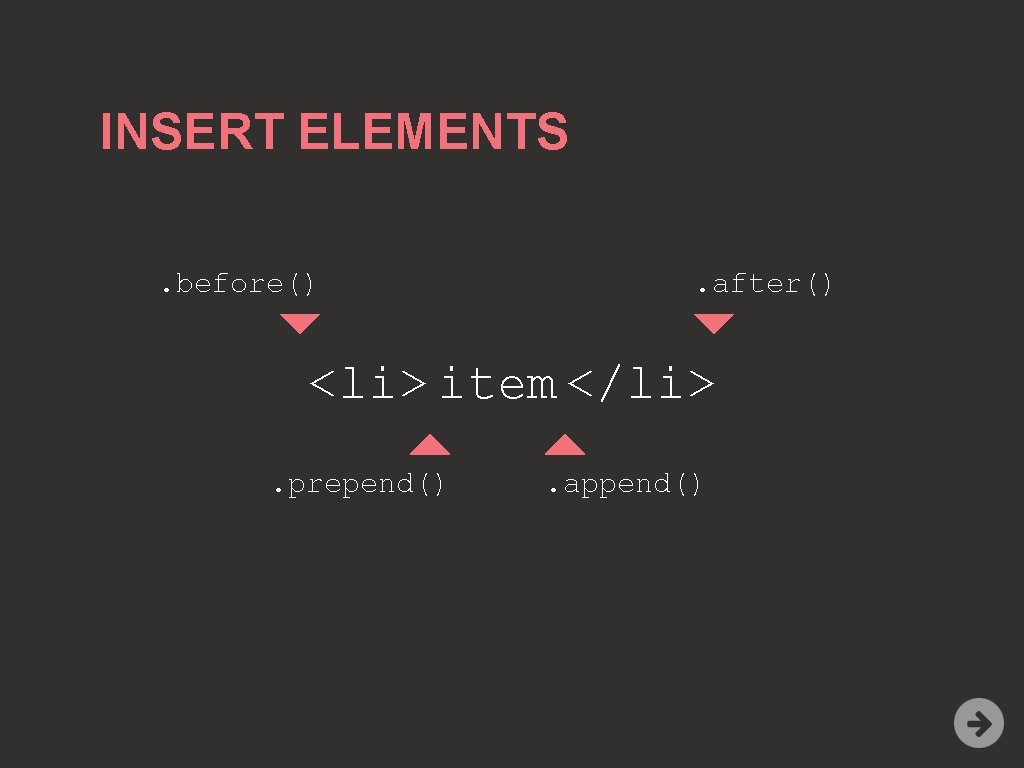
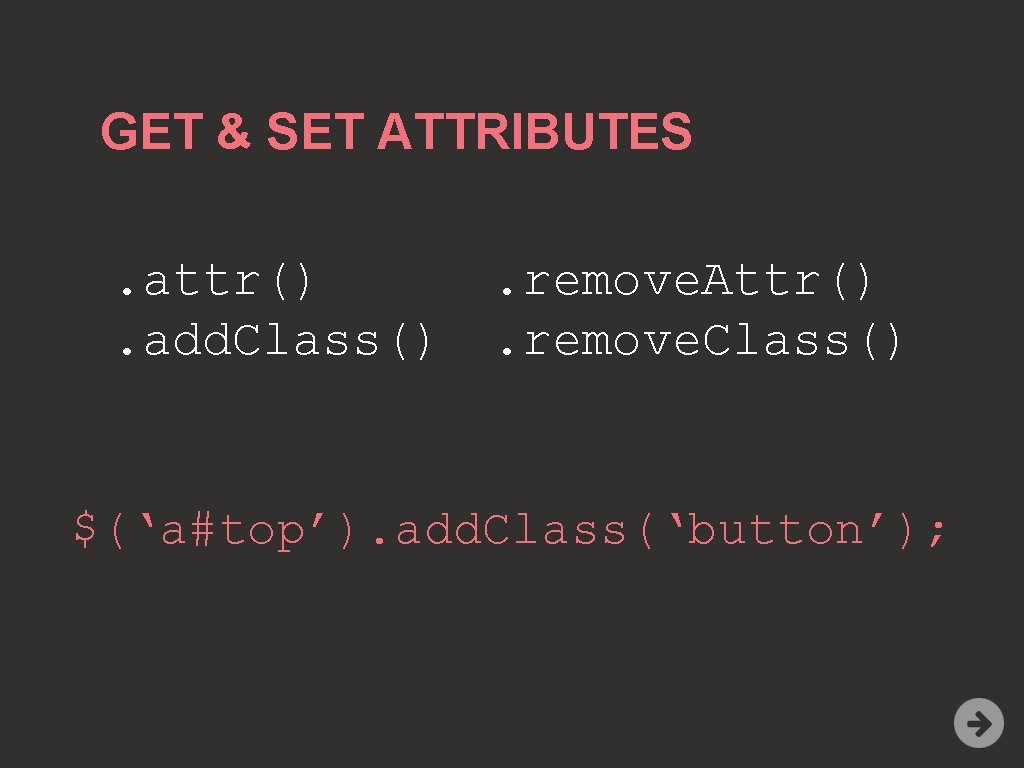
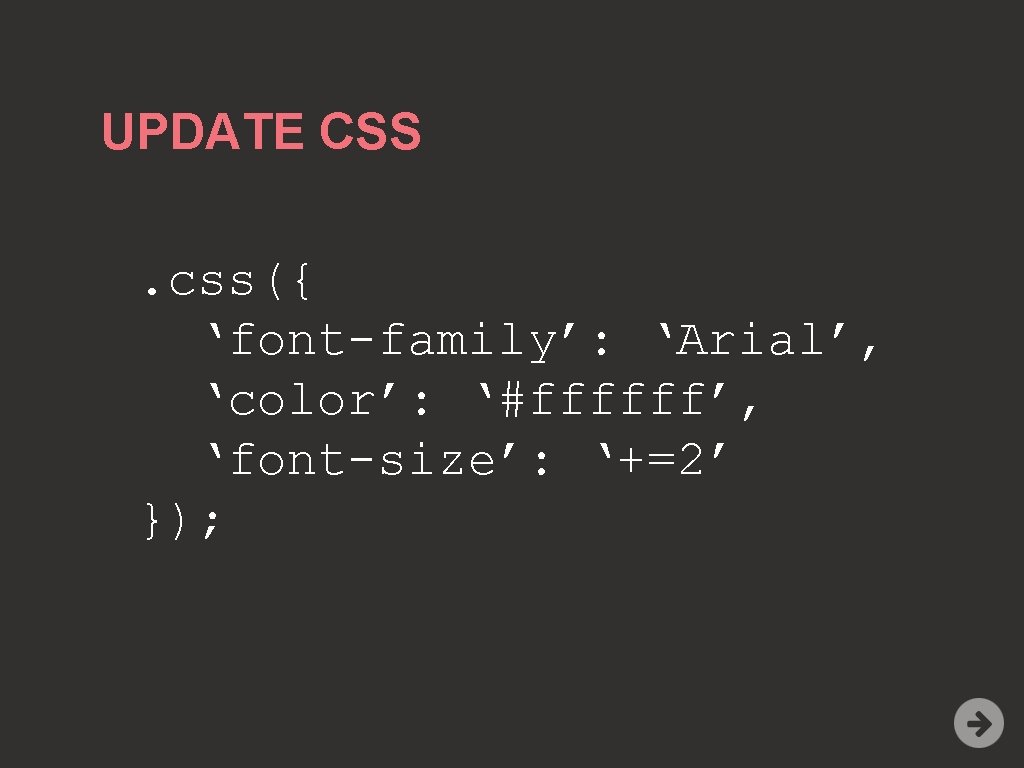
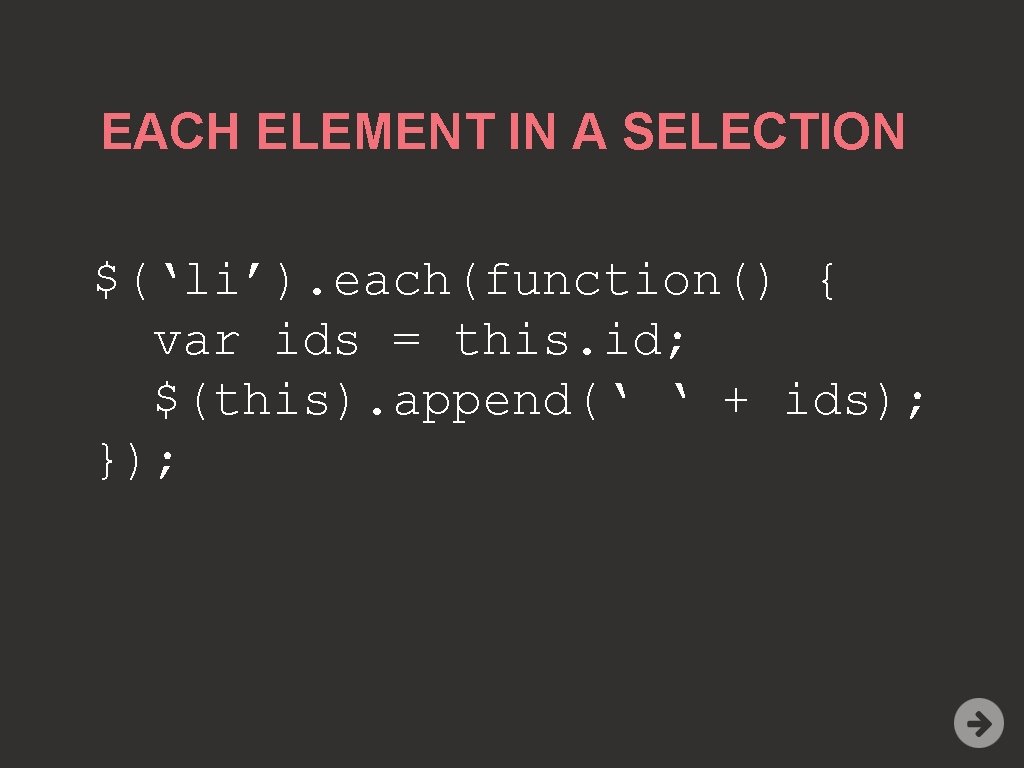
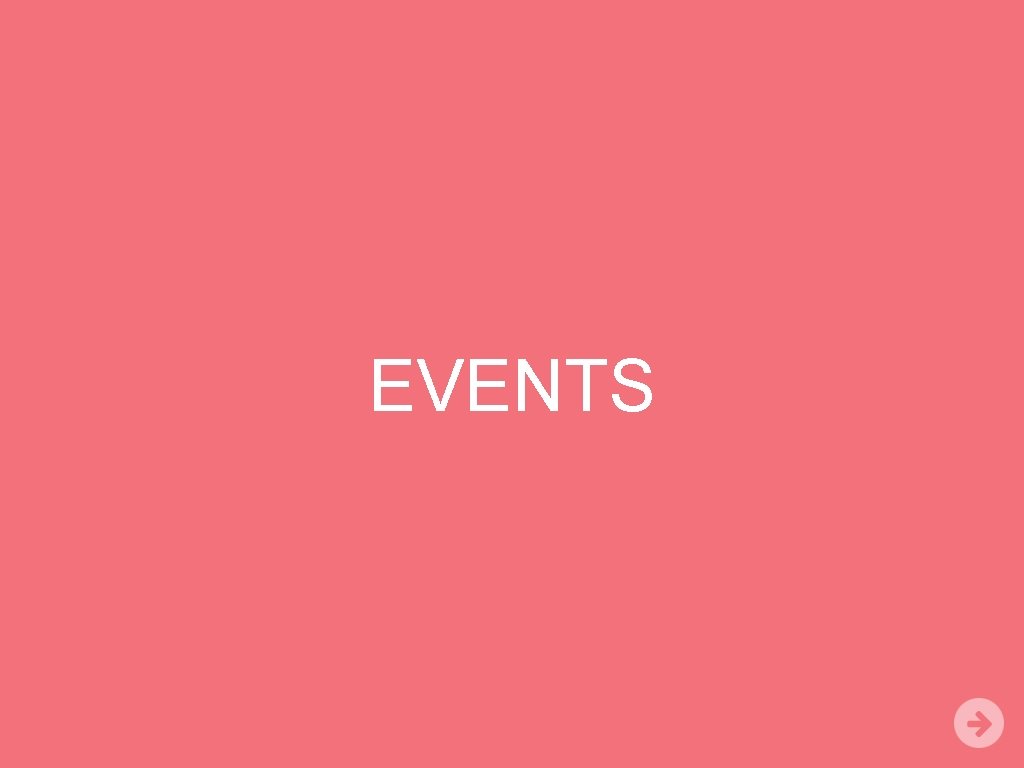
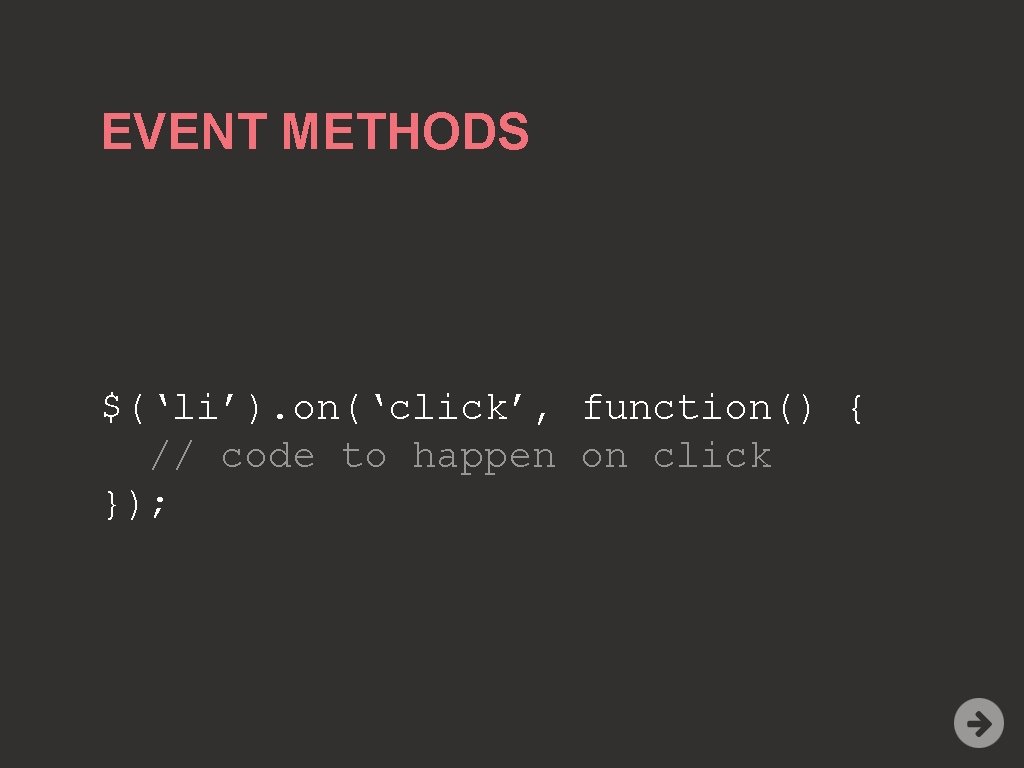
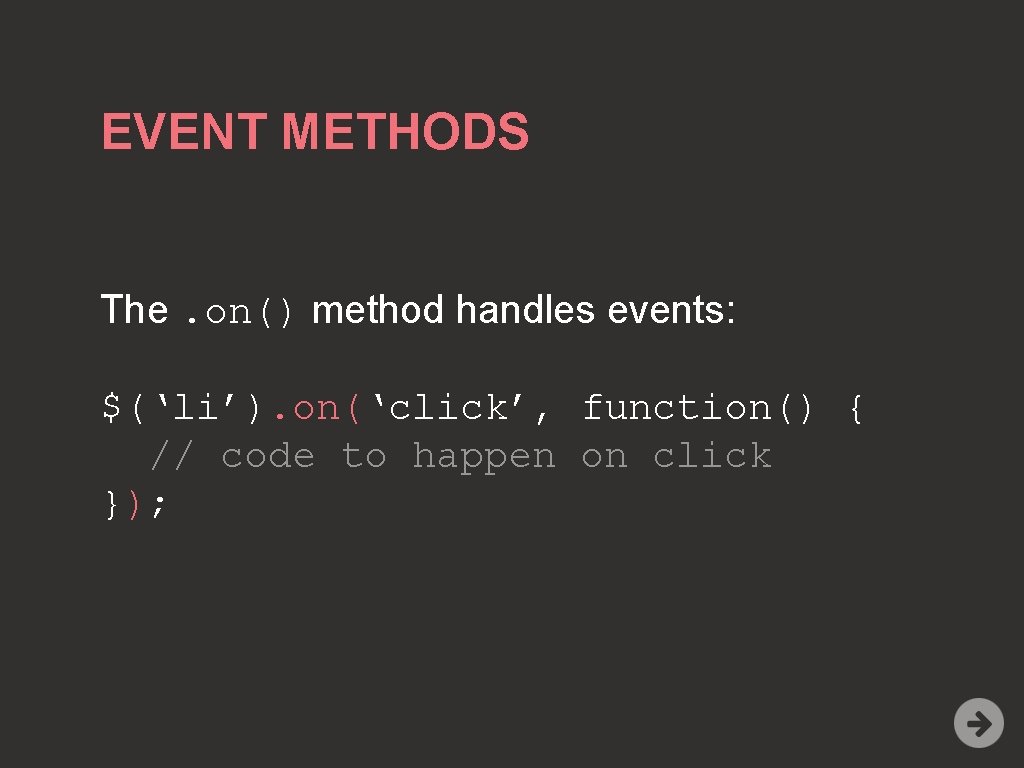
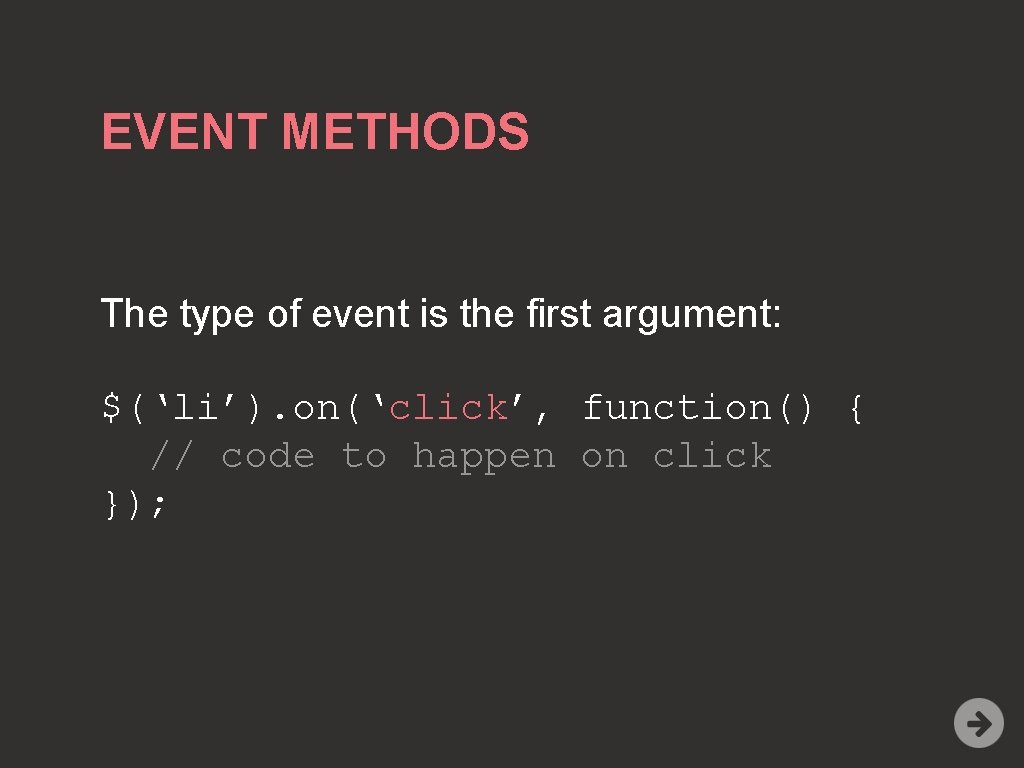
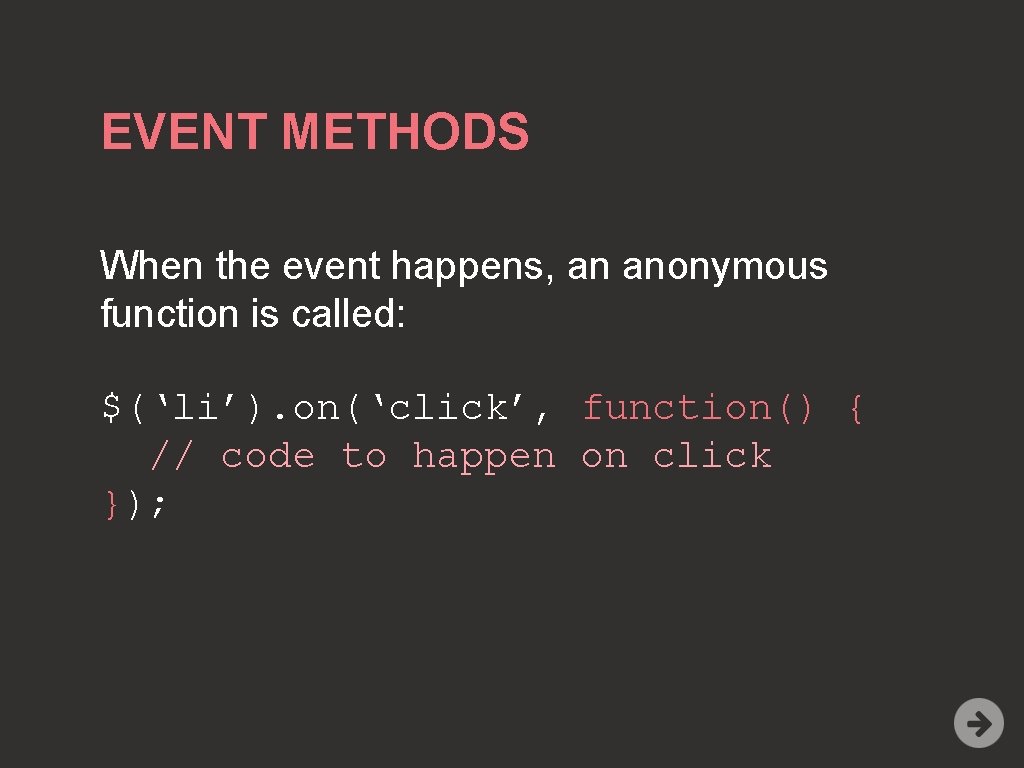
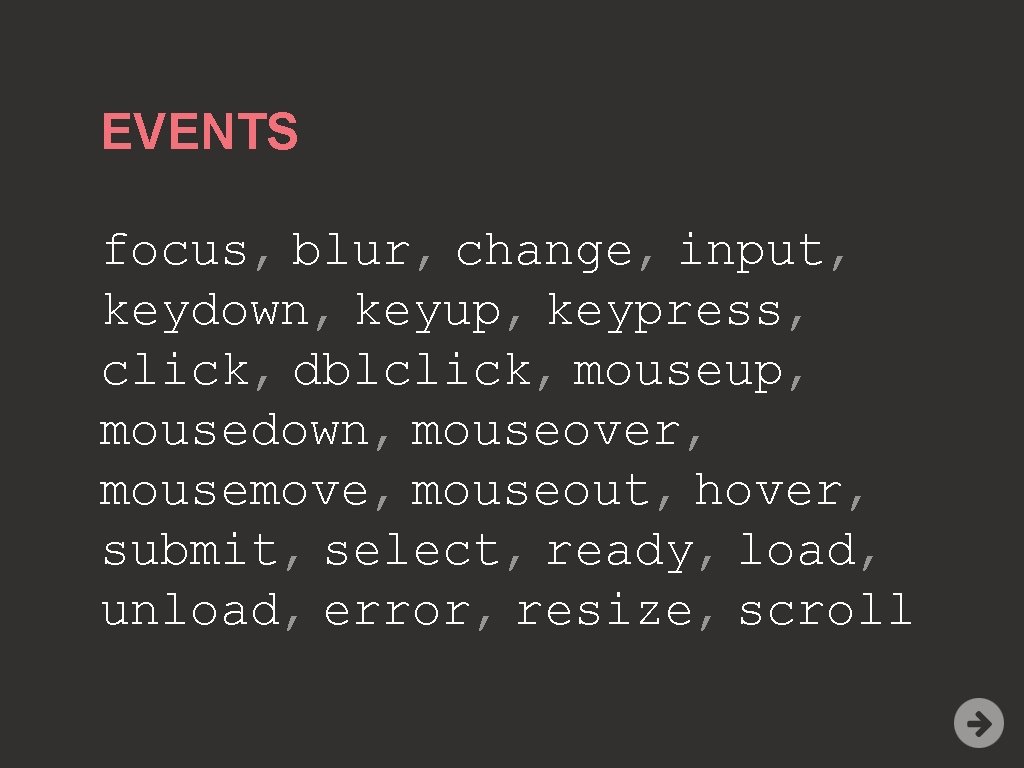
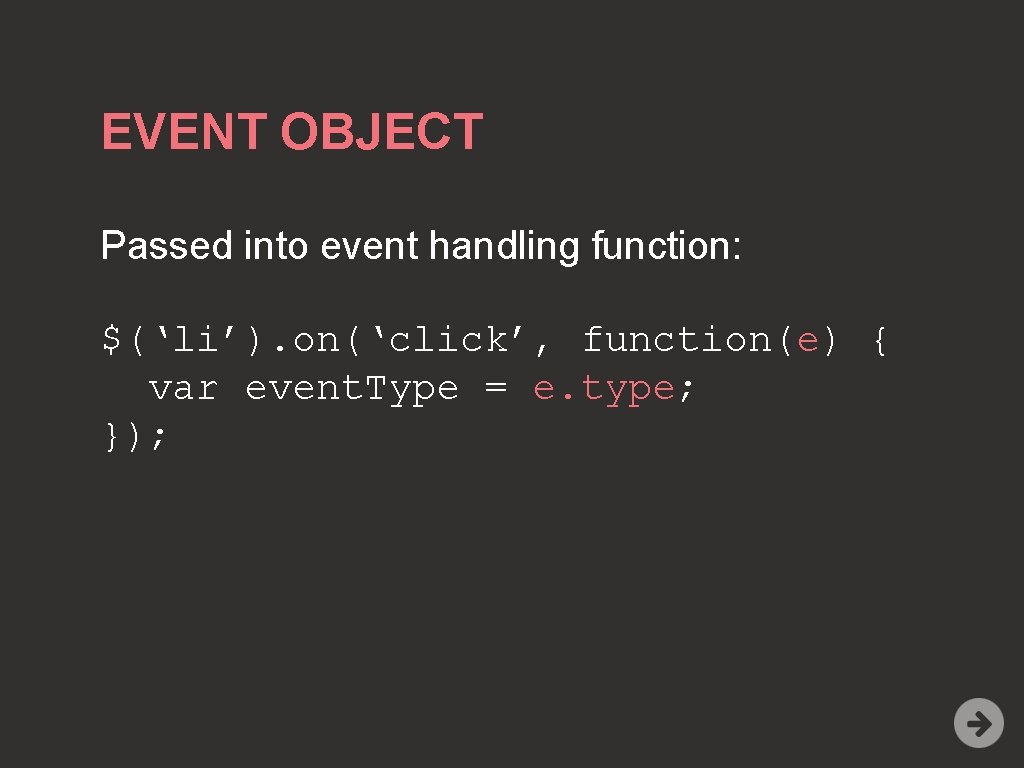
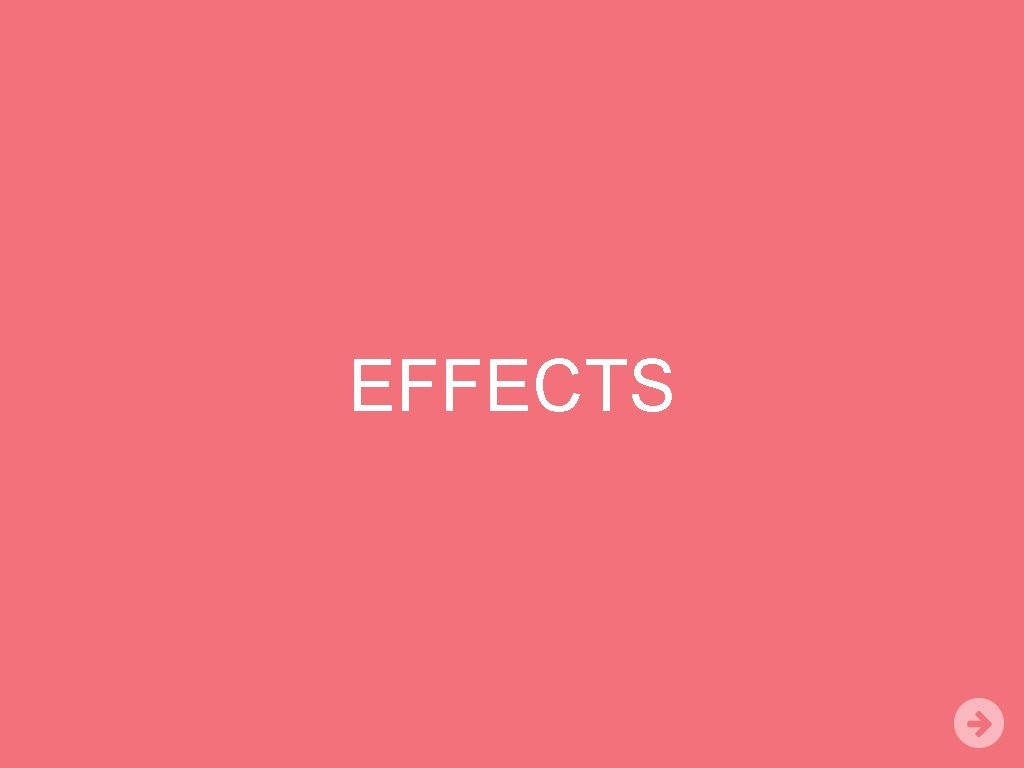
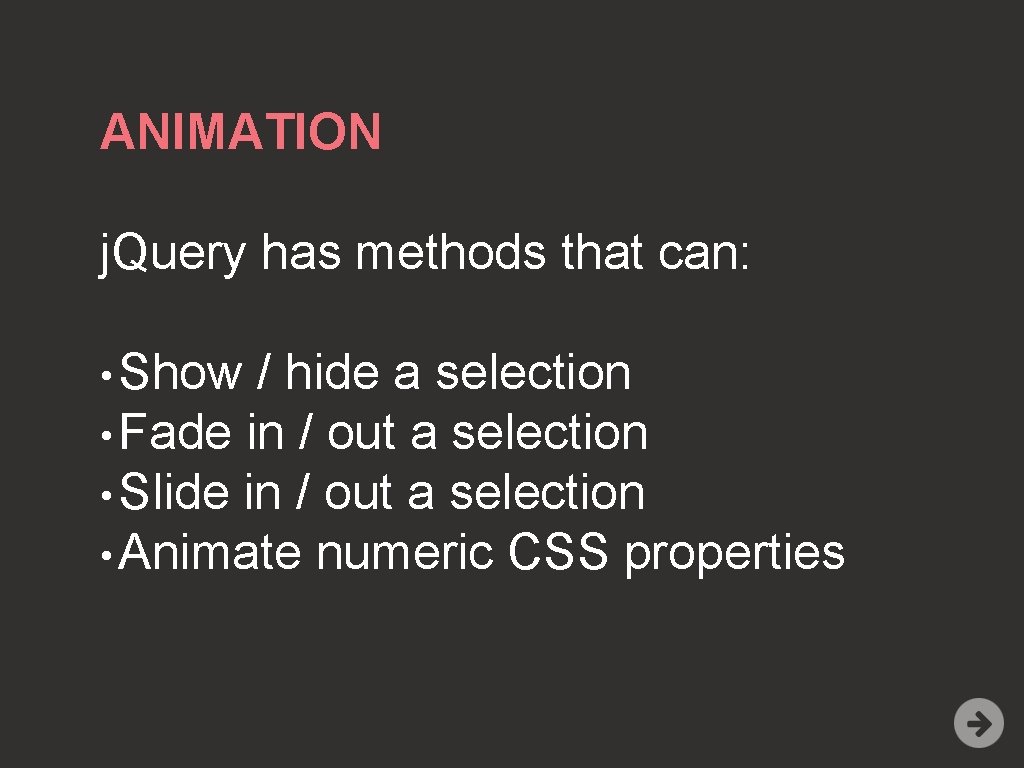
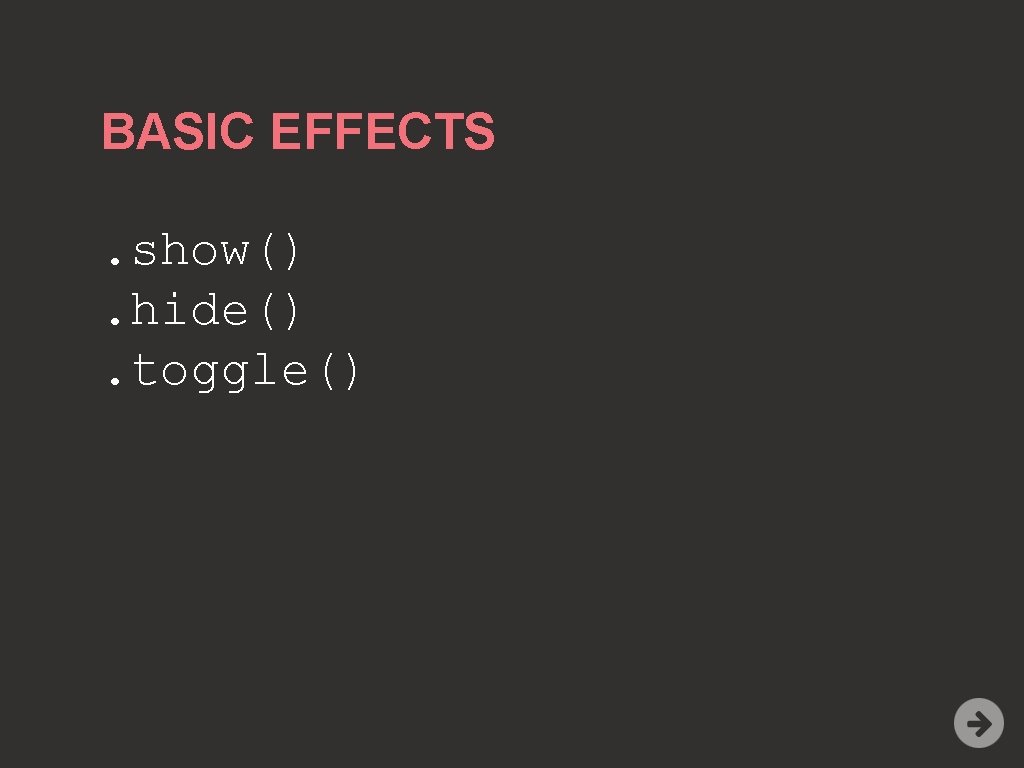
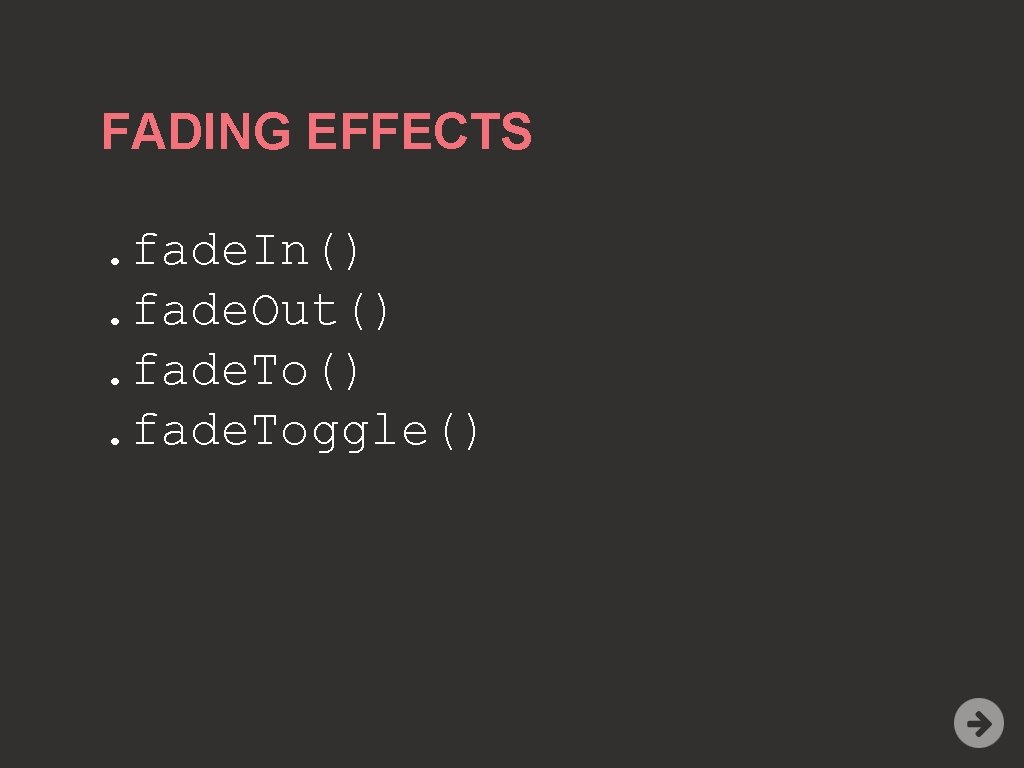
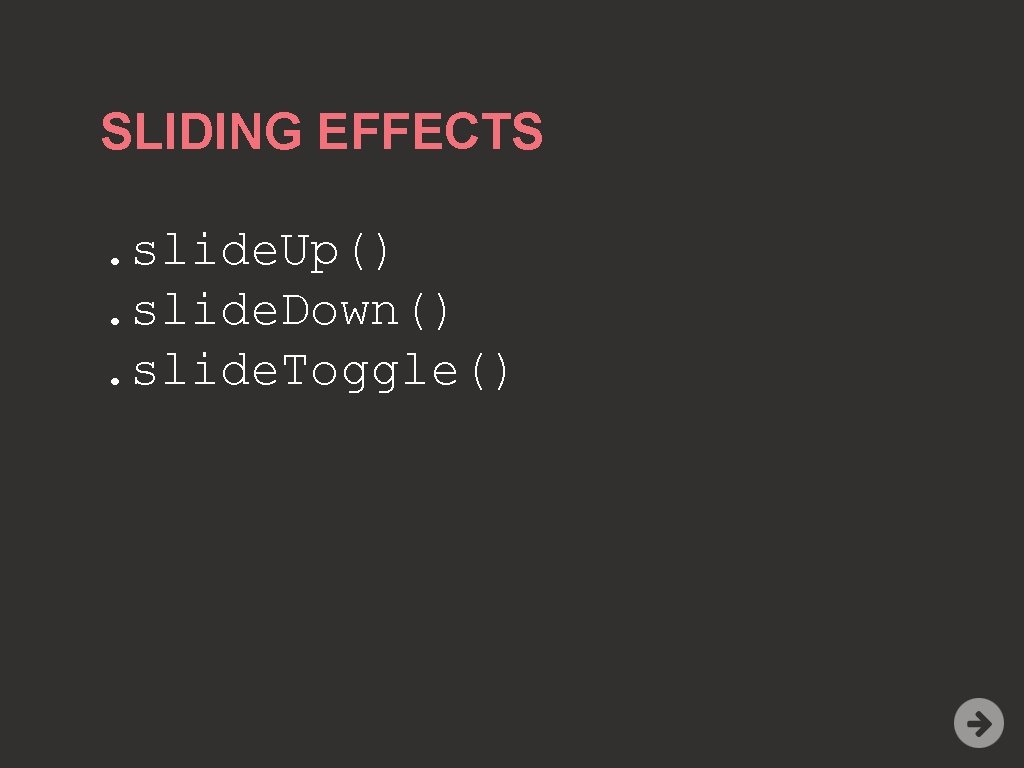
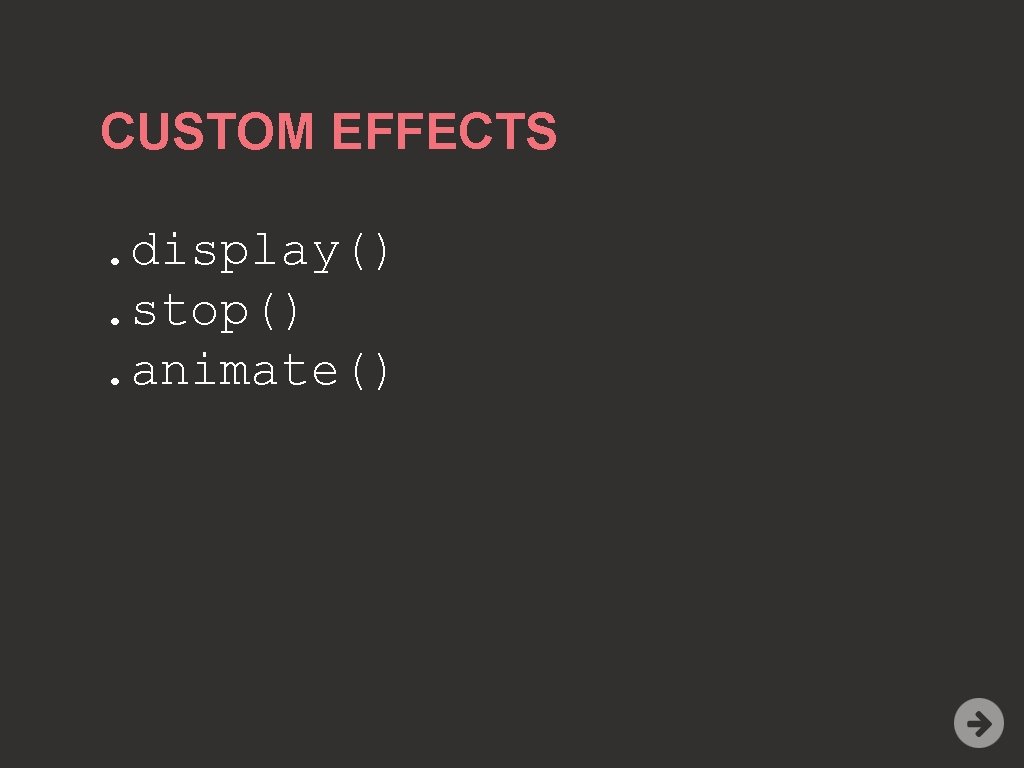
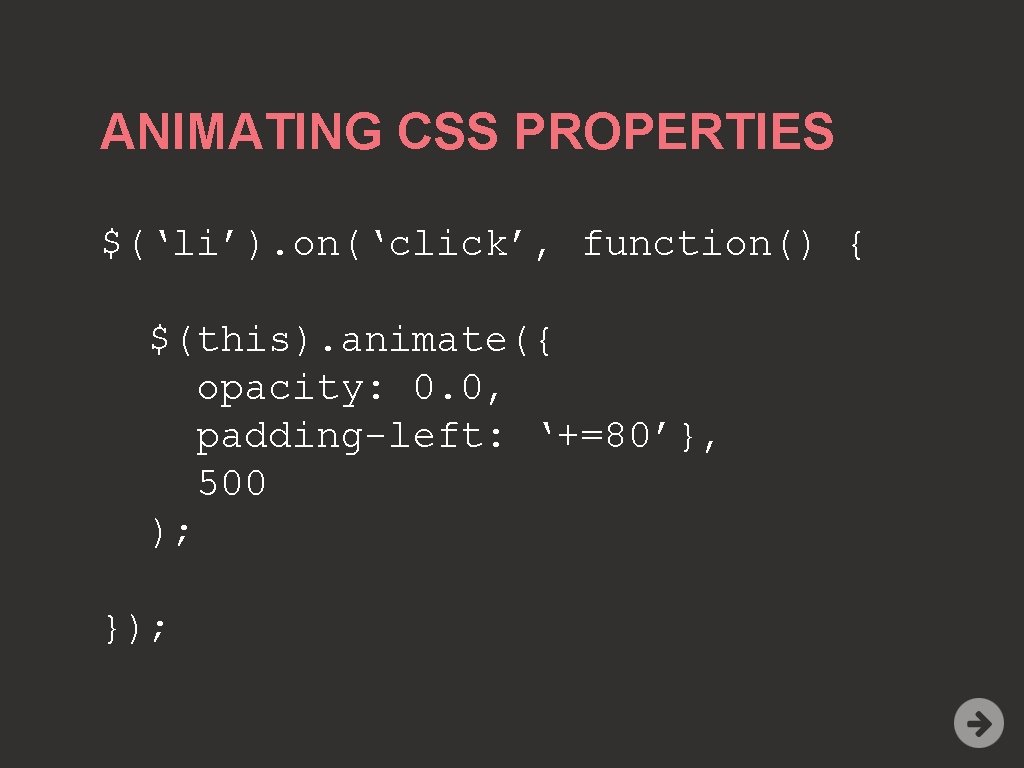
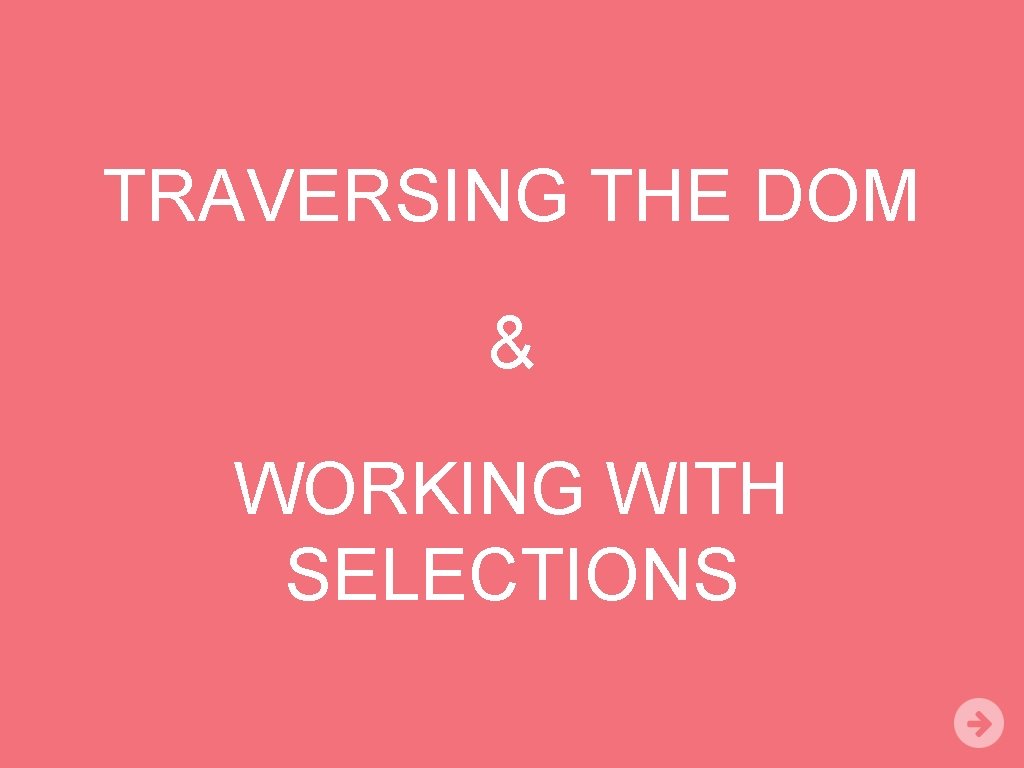
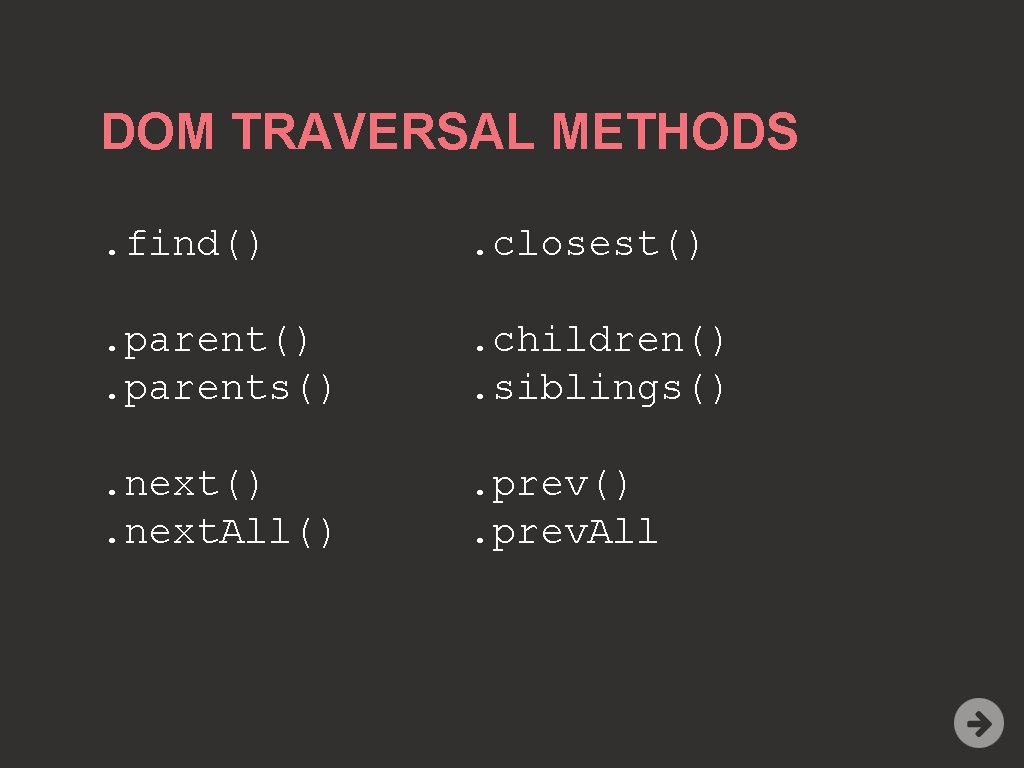
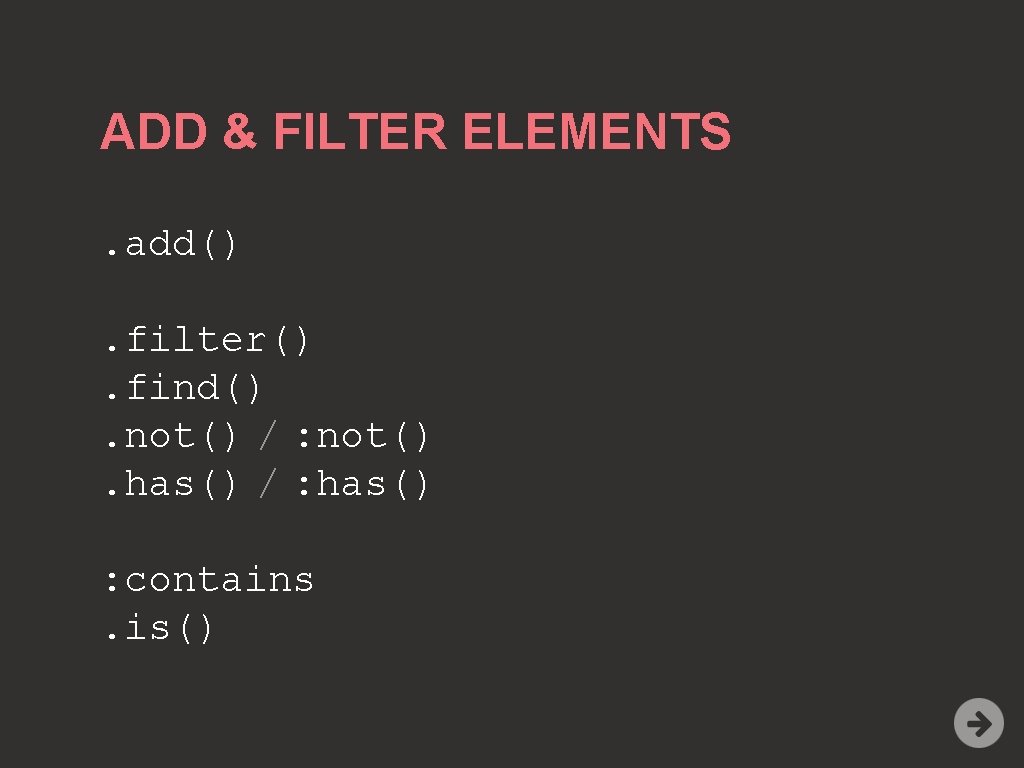
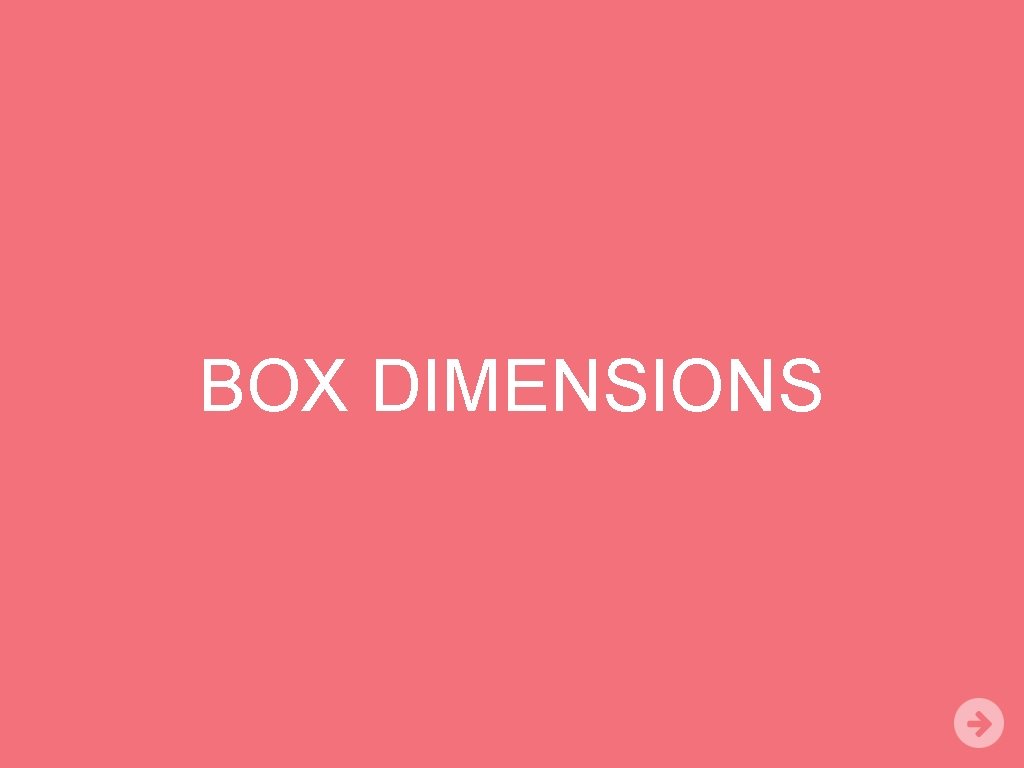
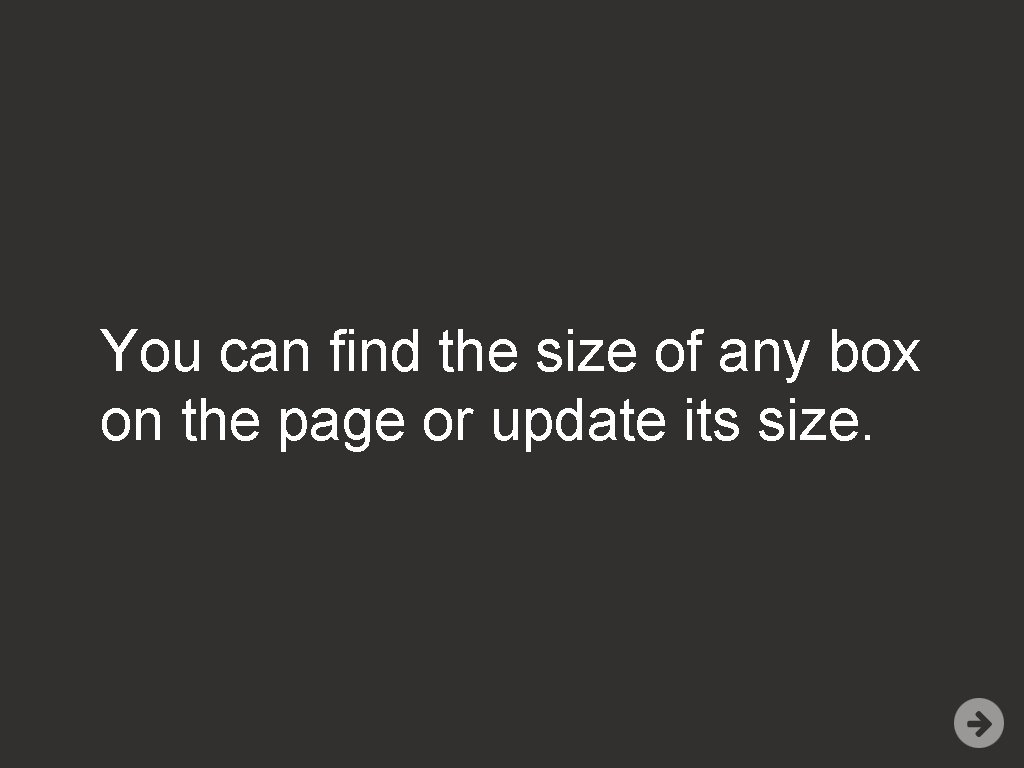
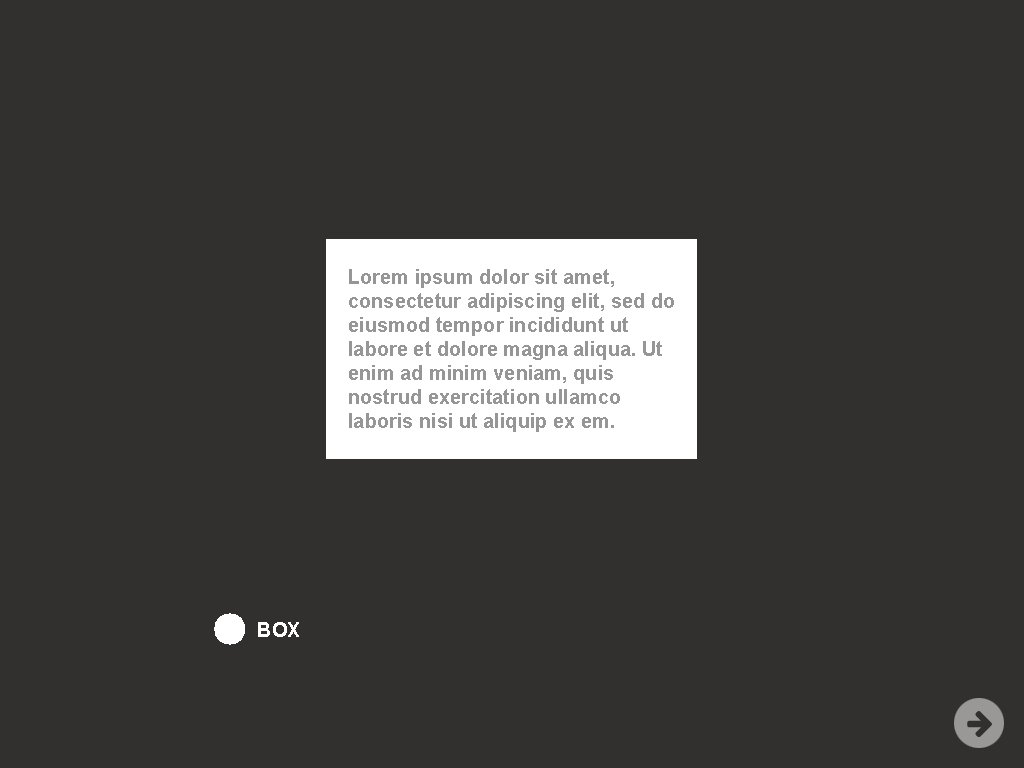
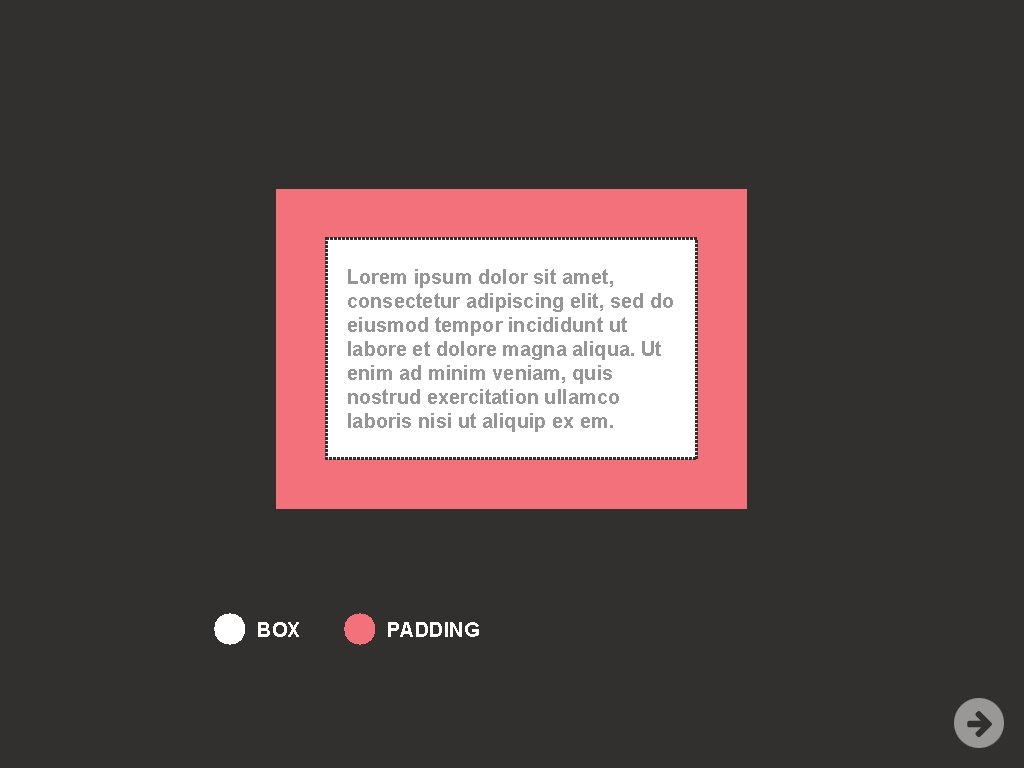
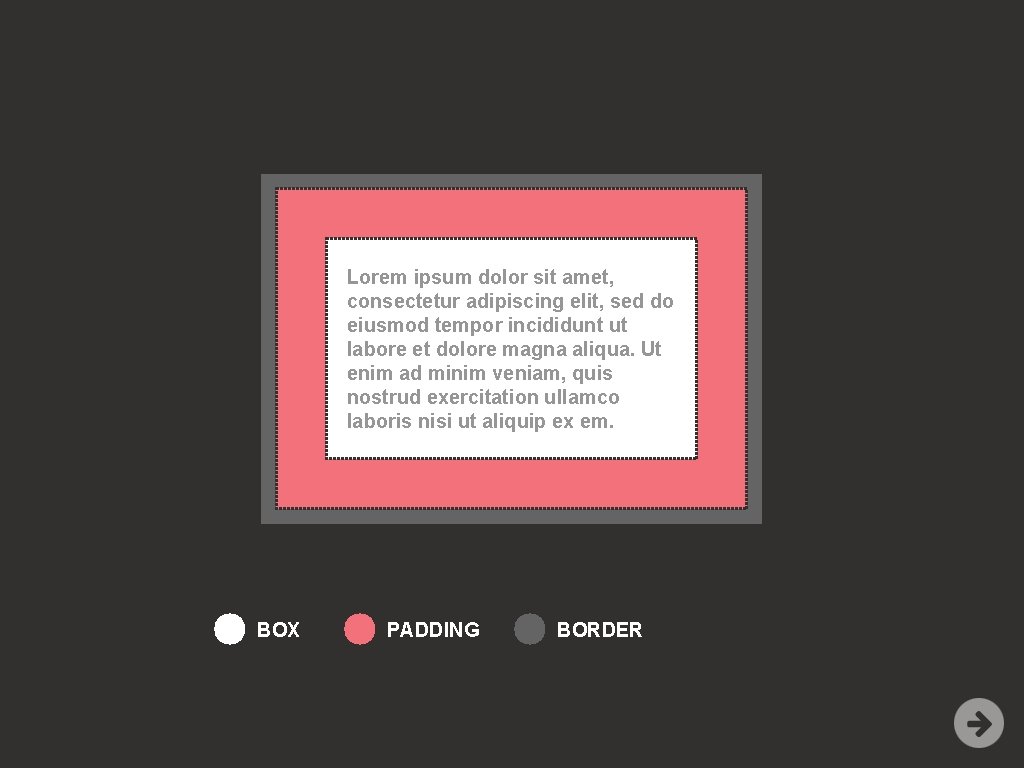
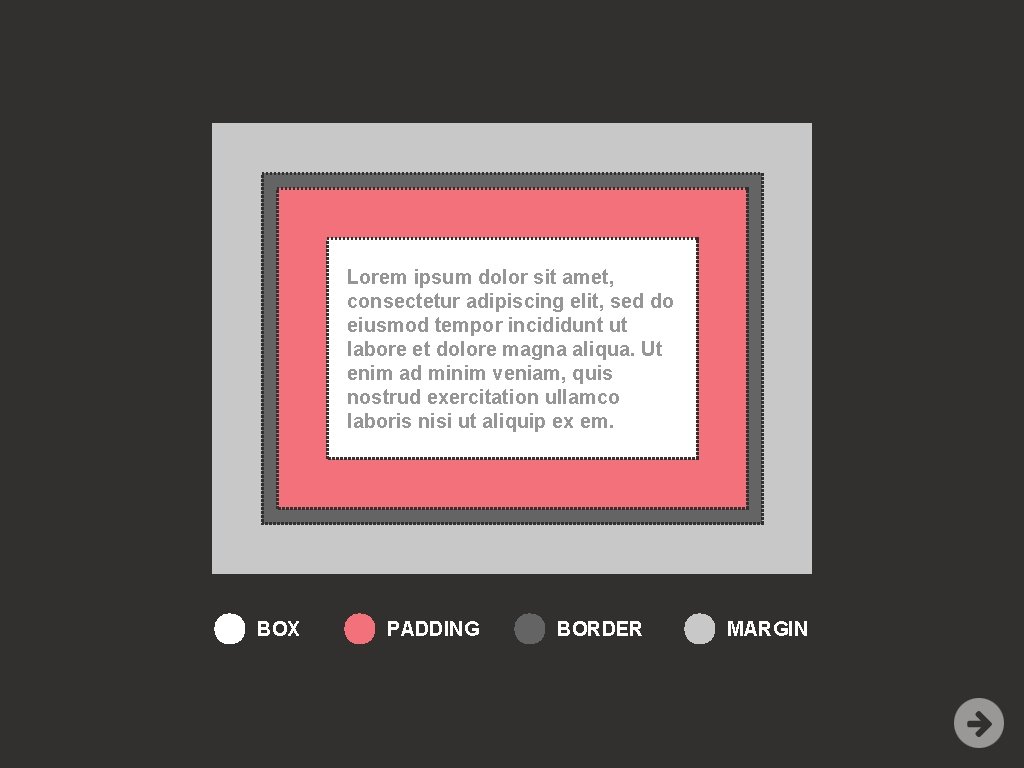
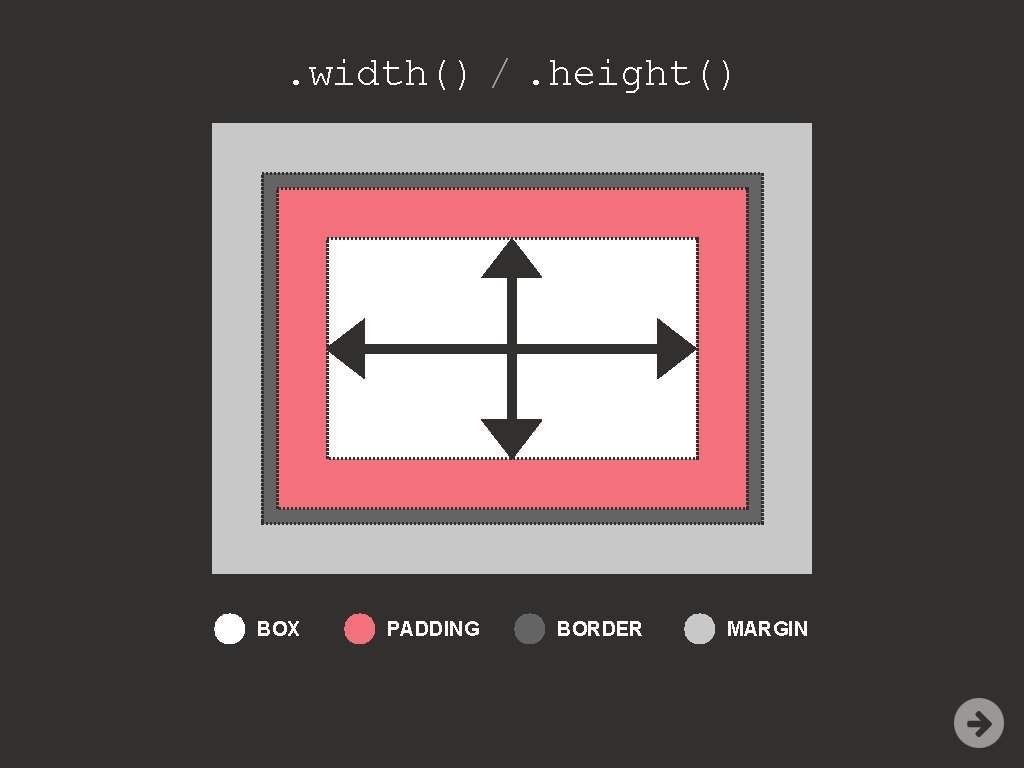
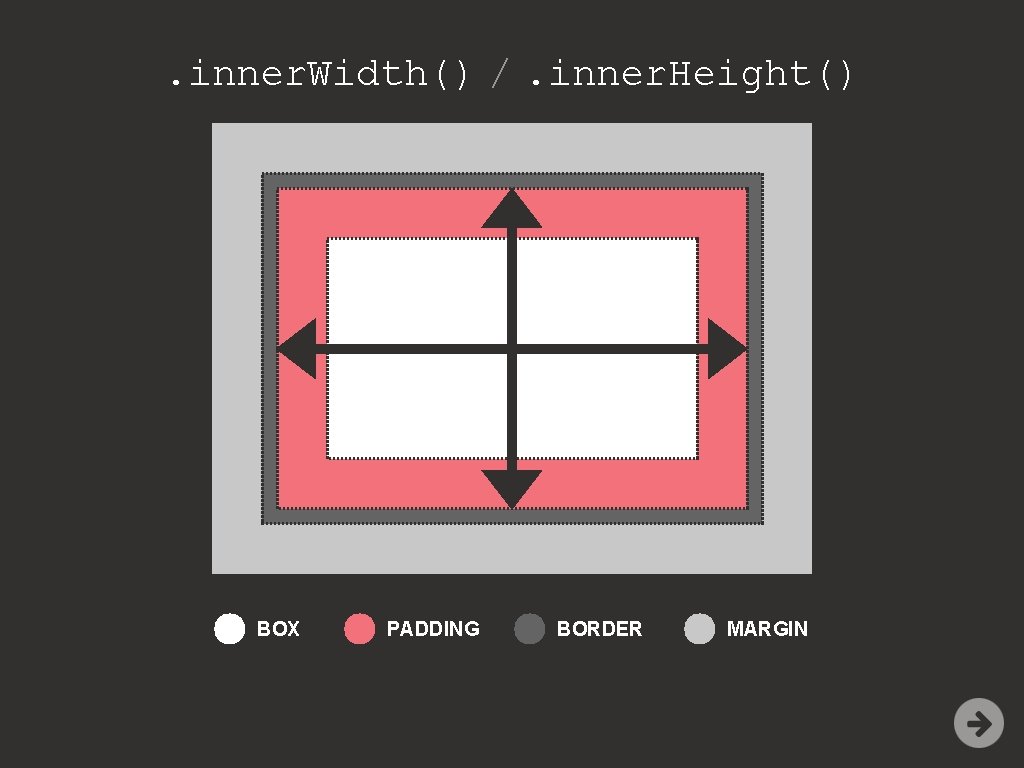
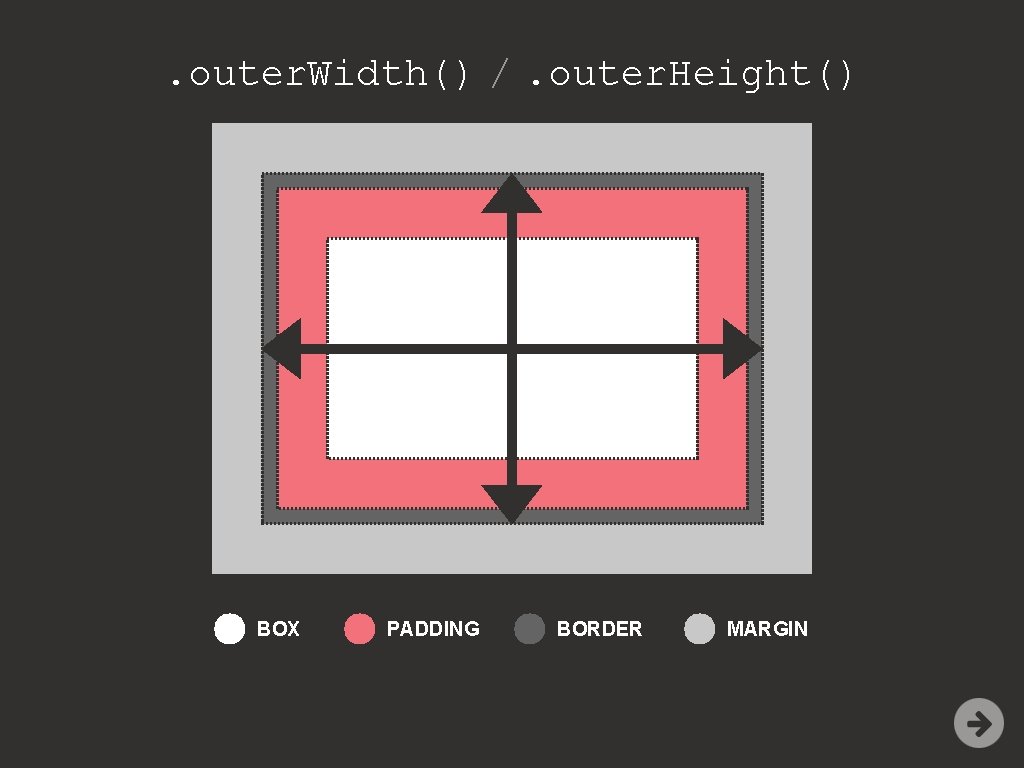
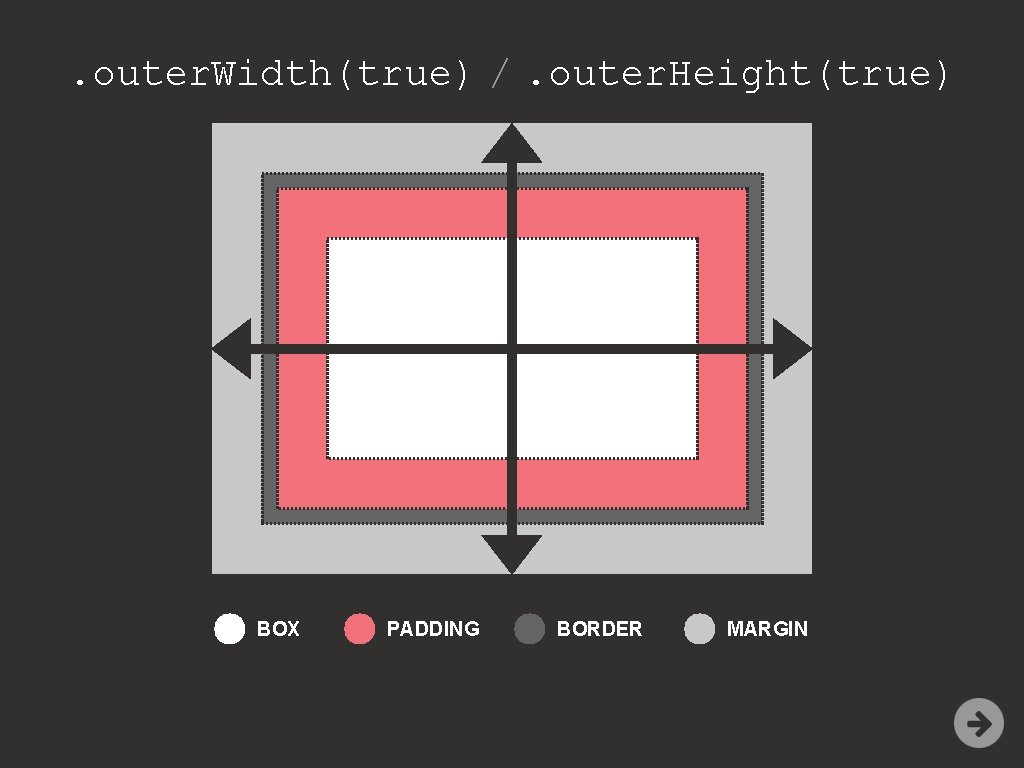
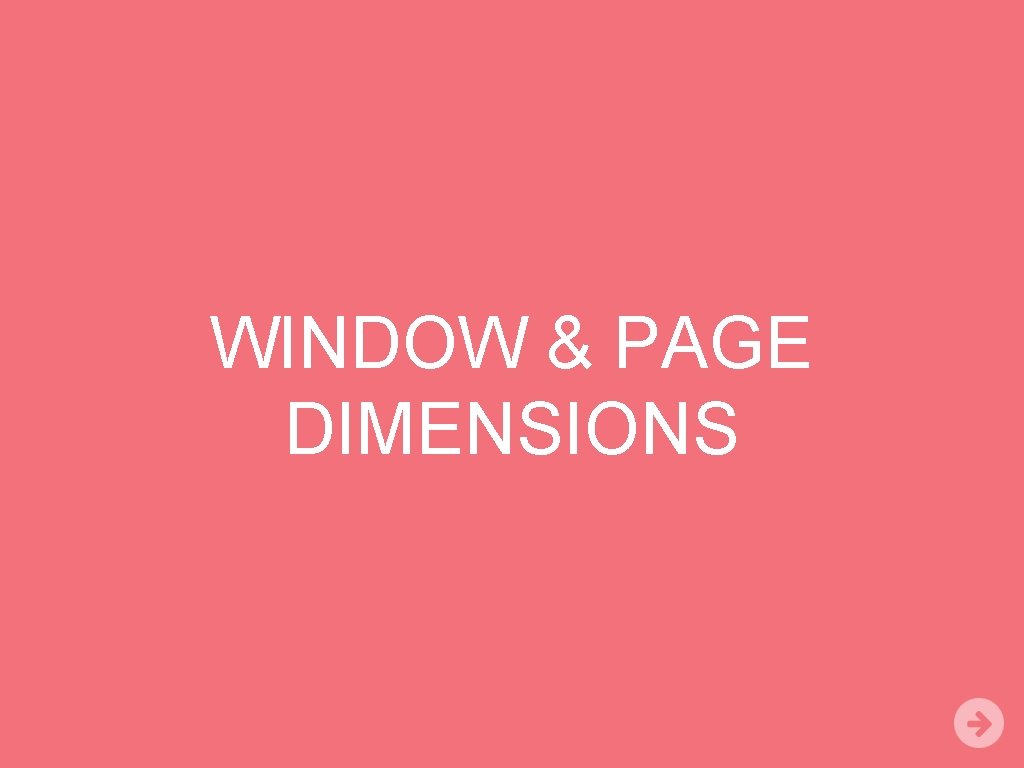
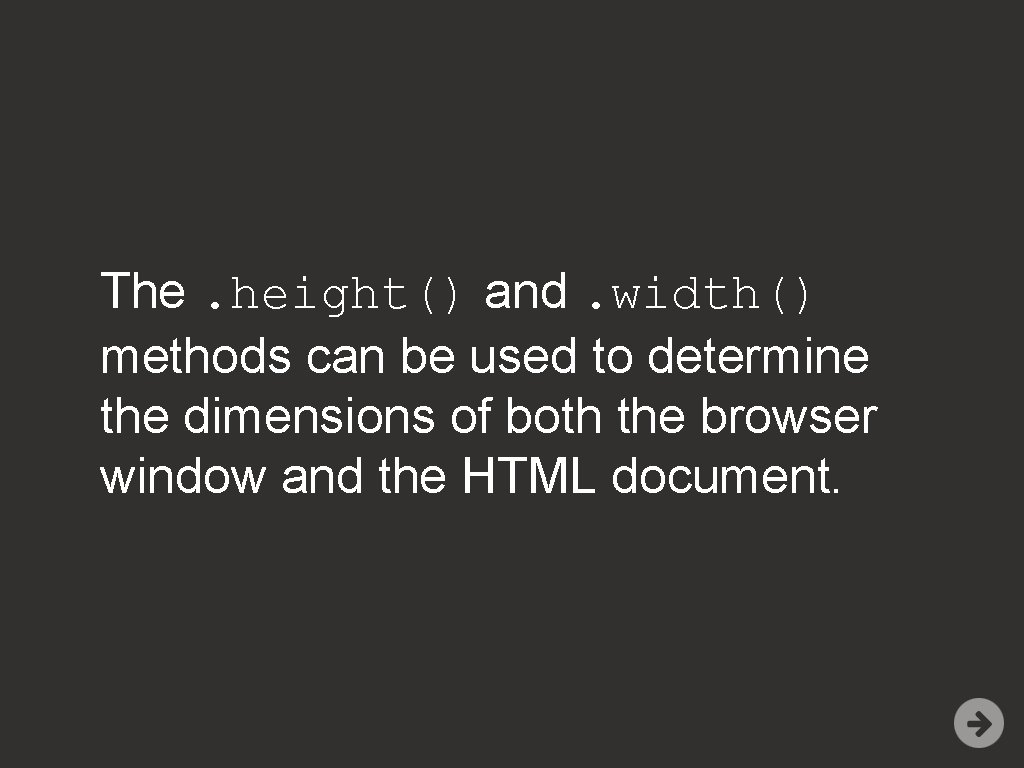
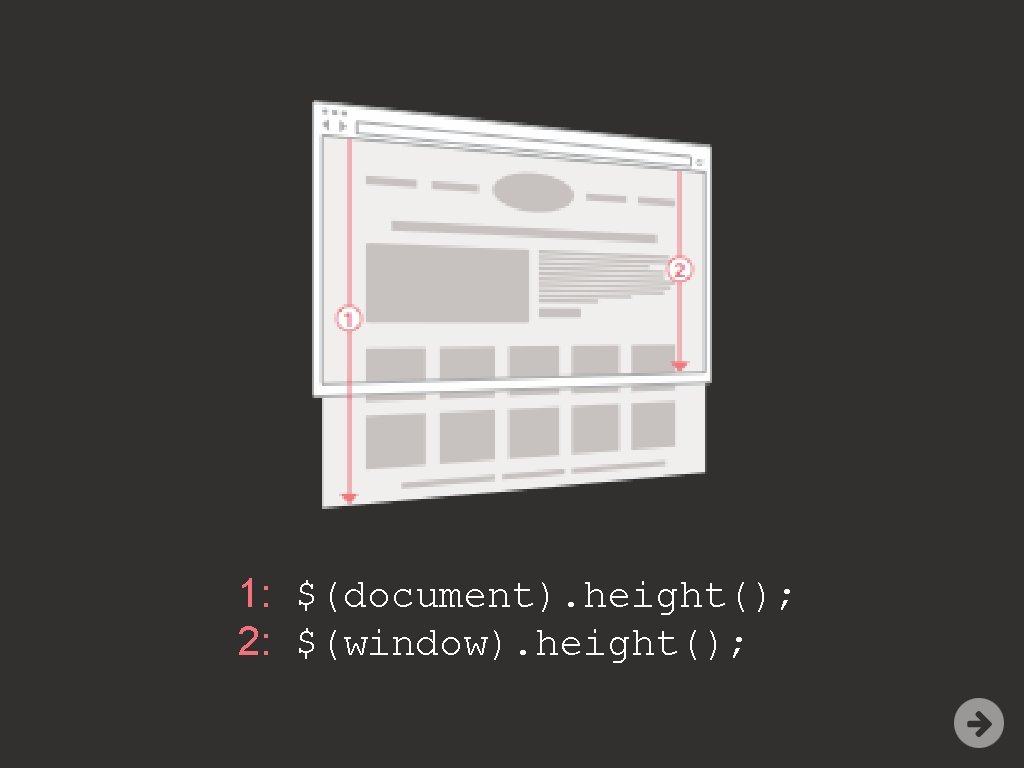
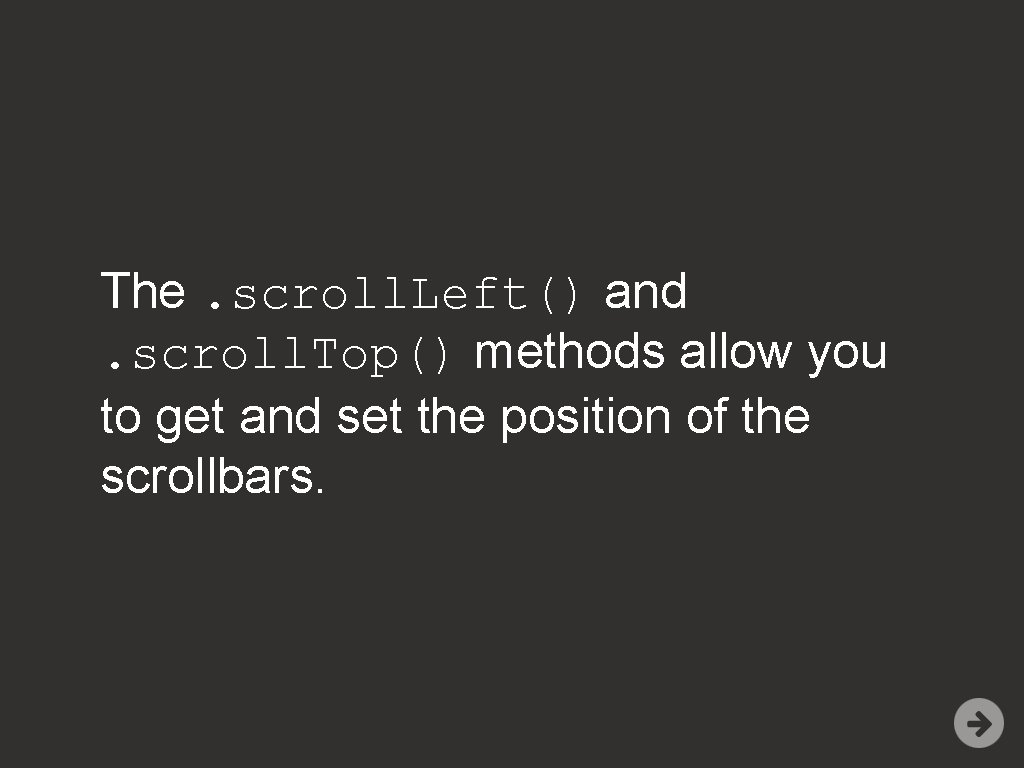
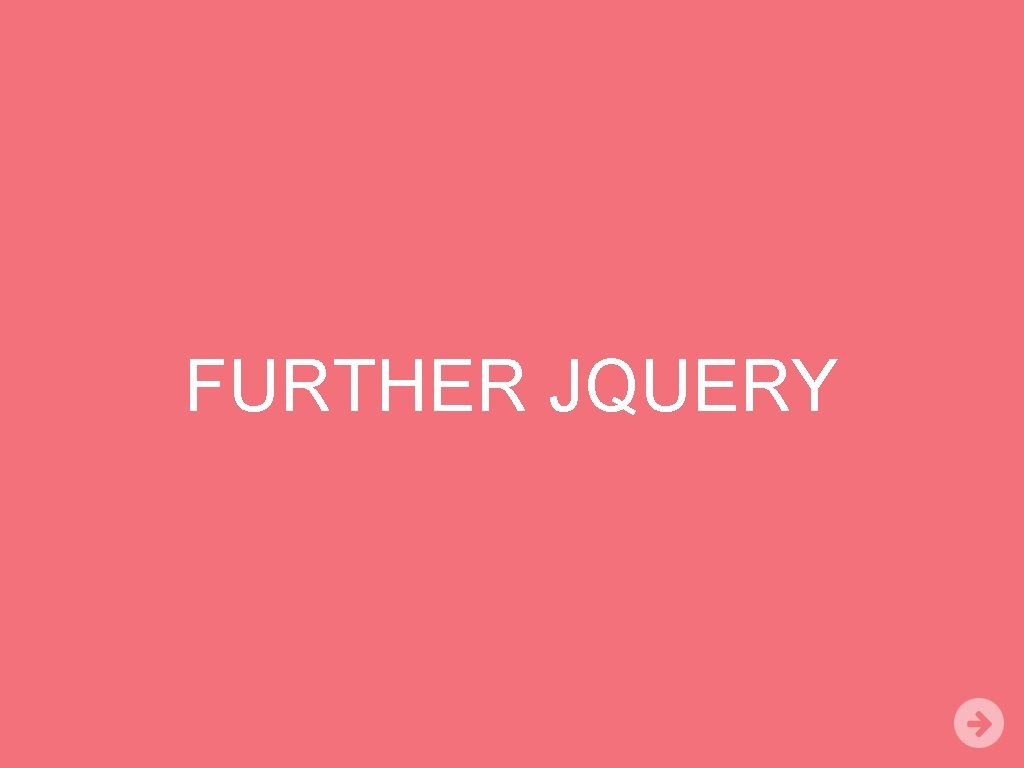
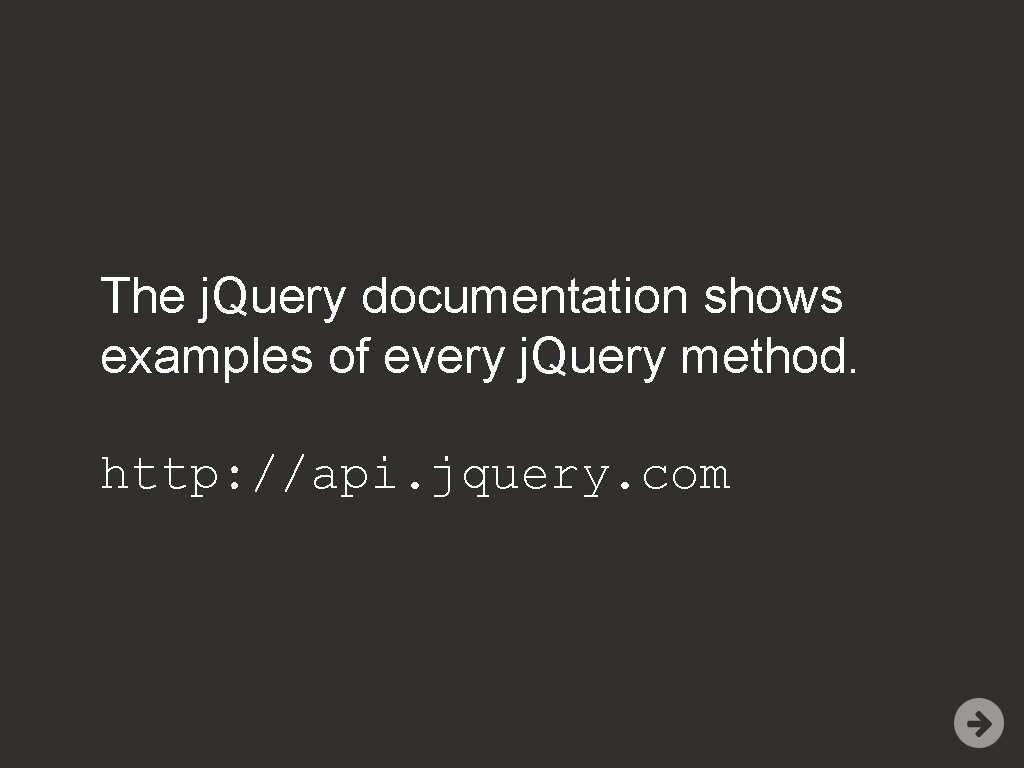
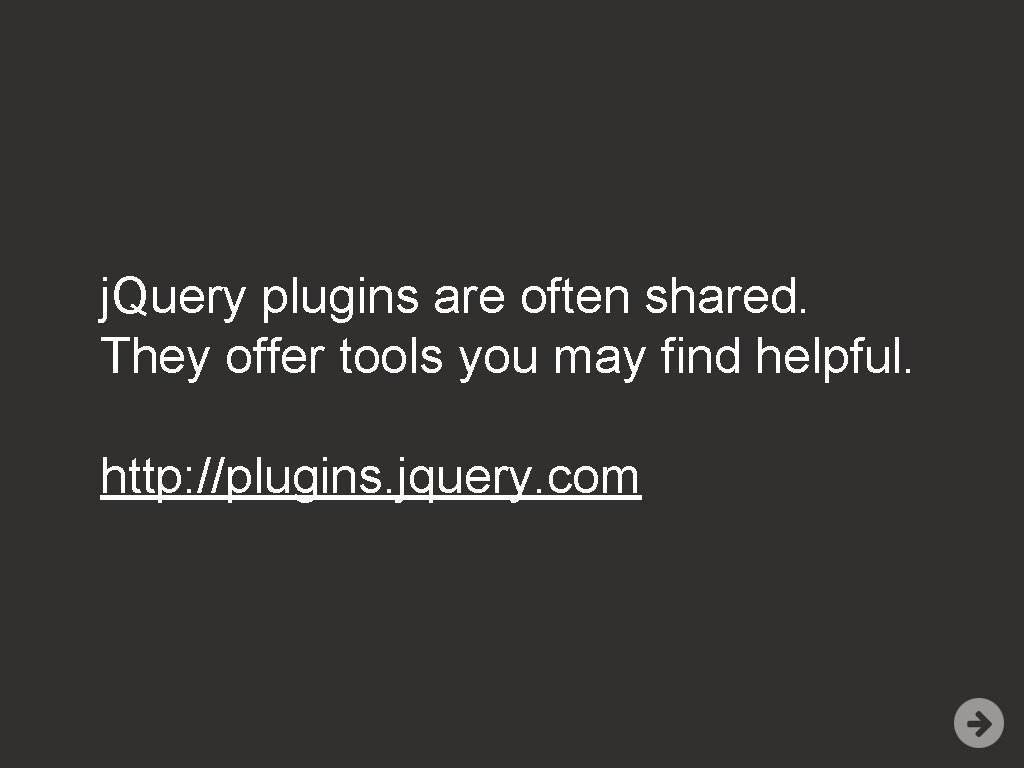
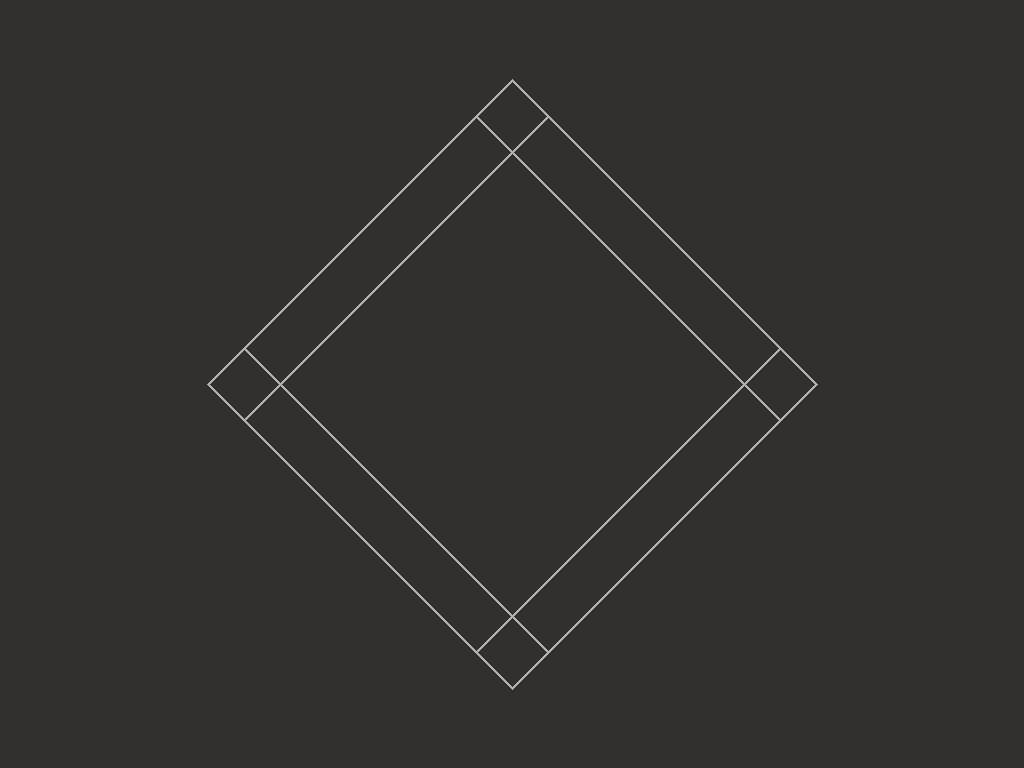
- Slides: 87
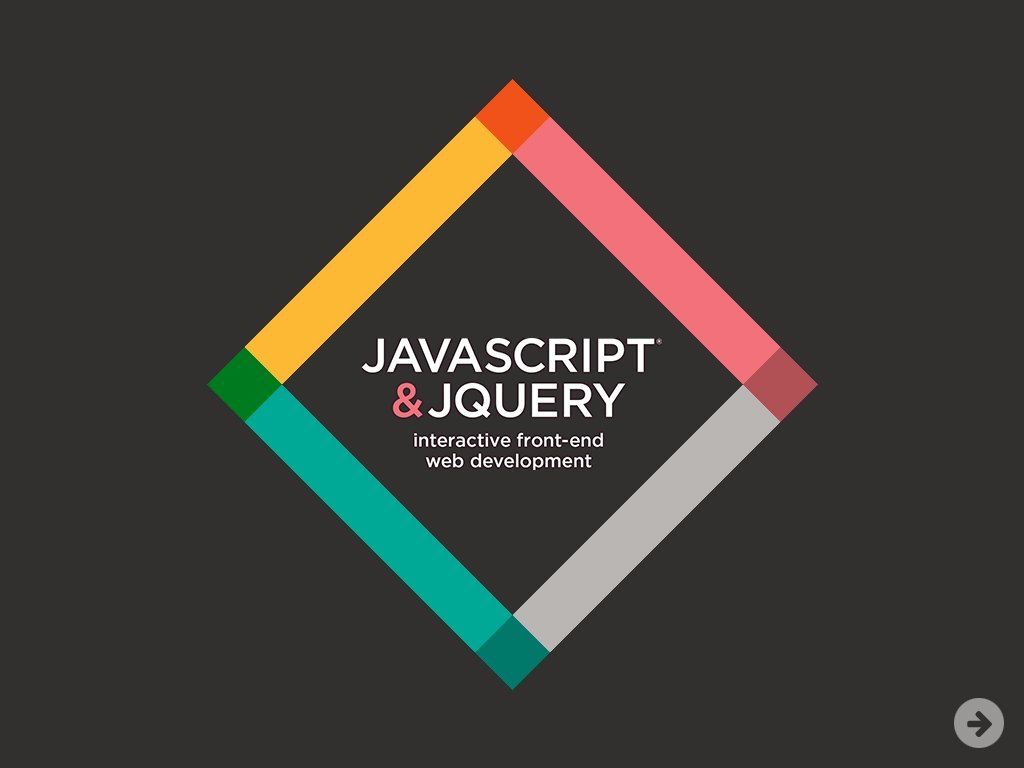
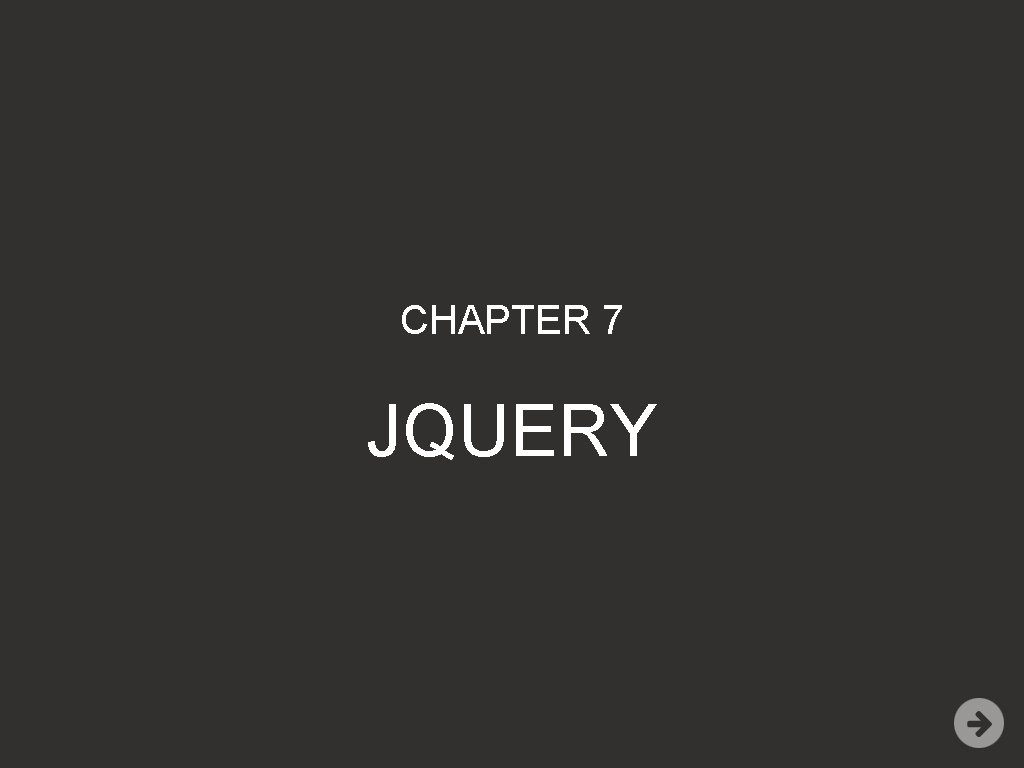
CHAPTER 7 JQUERY
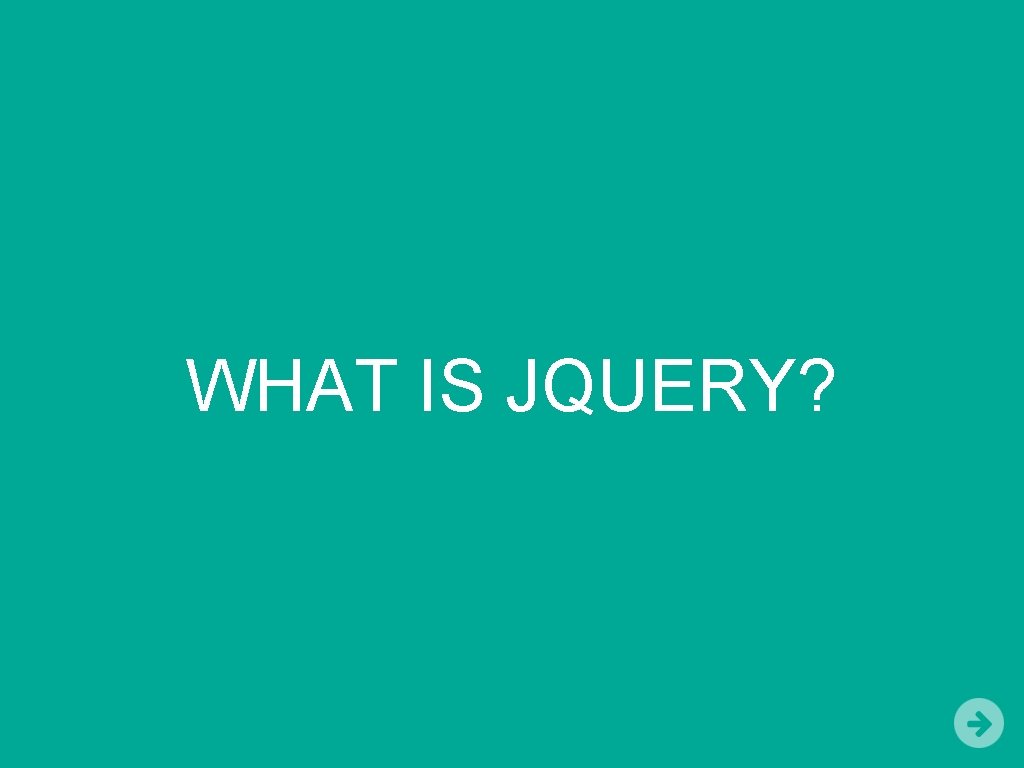
WHAT IS JQUERY?
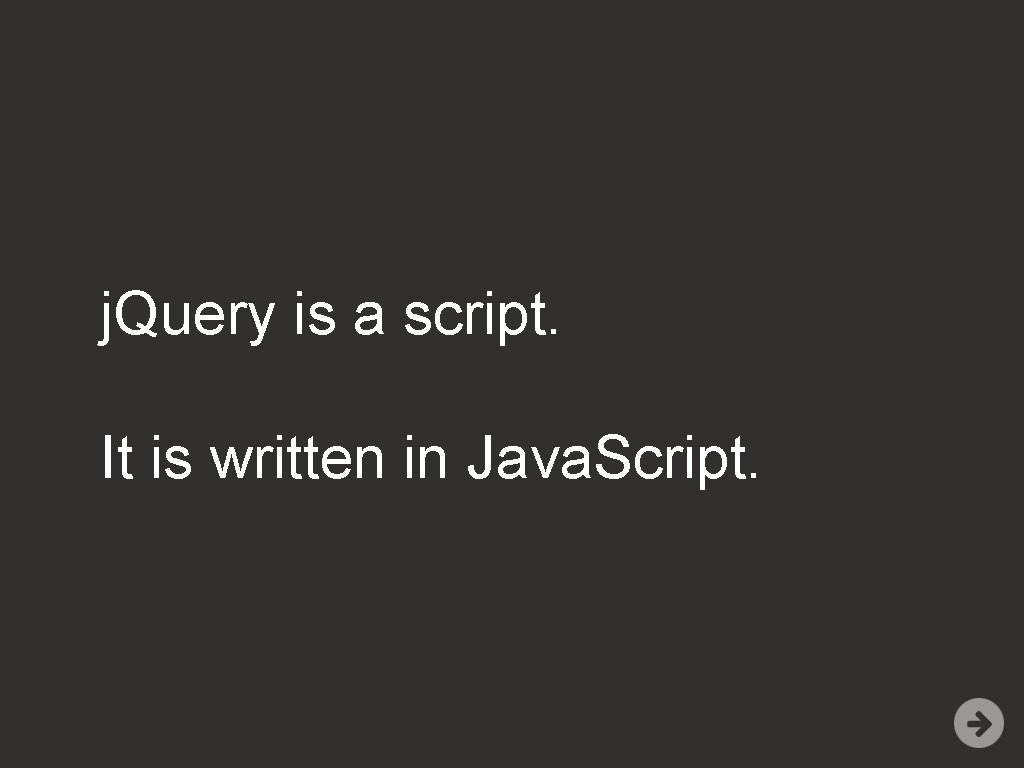
j. Query is a script. It is written in Java. Script.
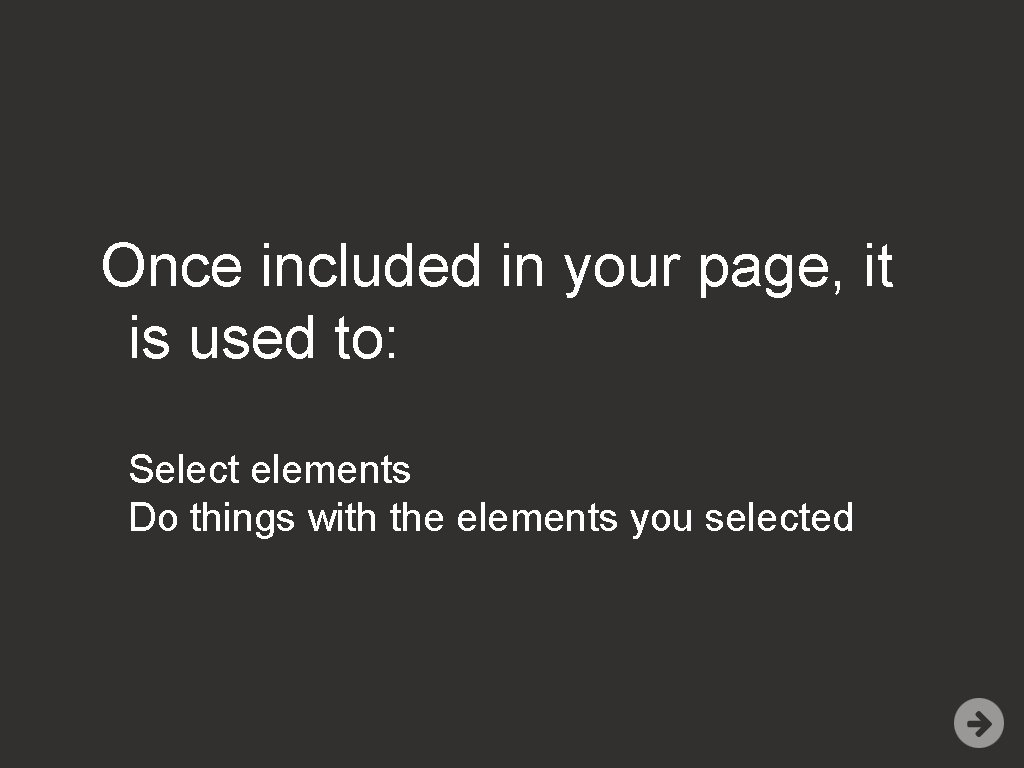
Once included in your page, it is used to: Select elements Do things with the elements you selected
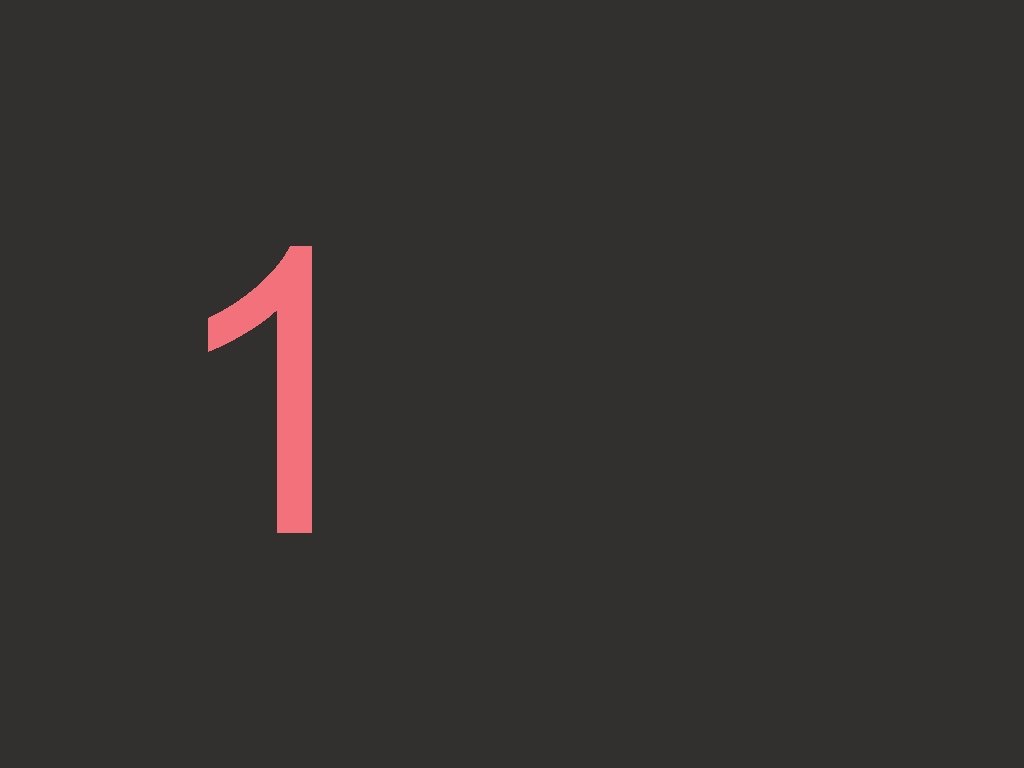
1
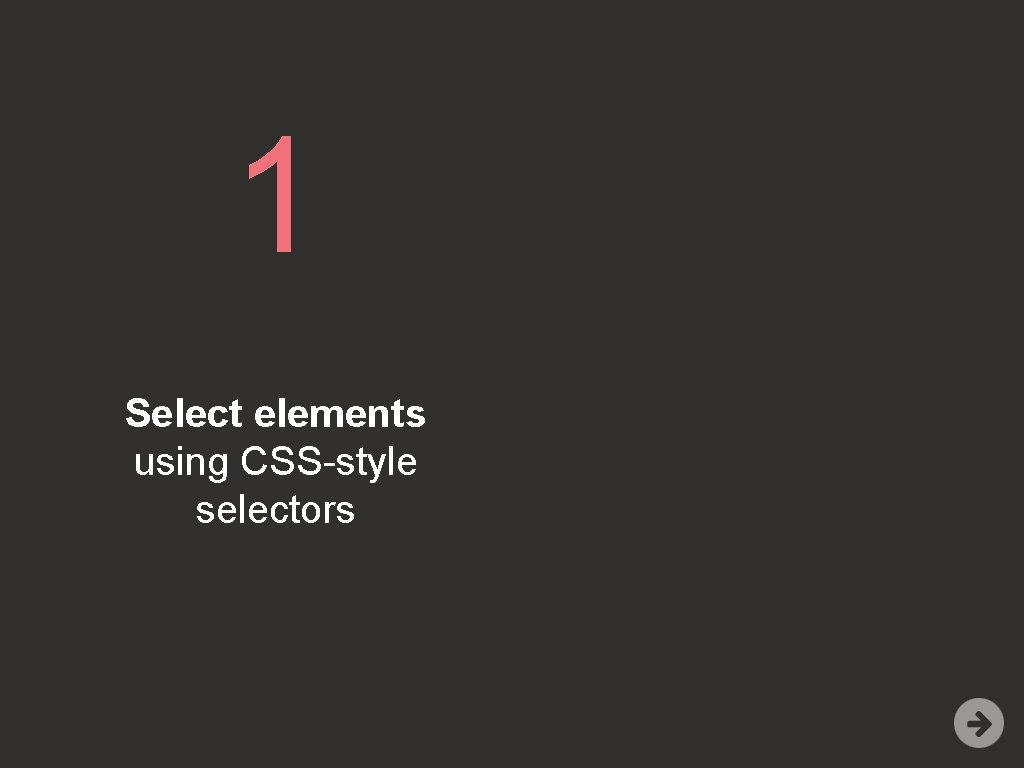
1 Select elements using CSS-style selectors
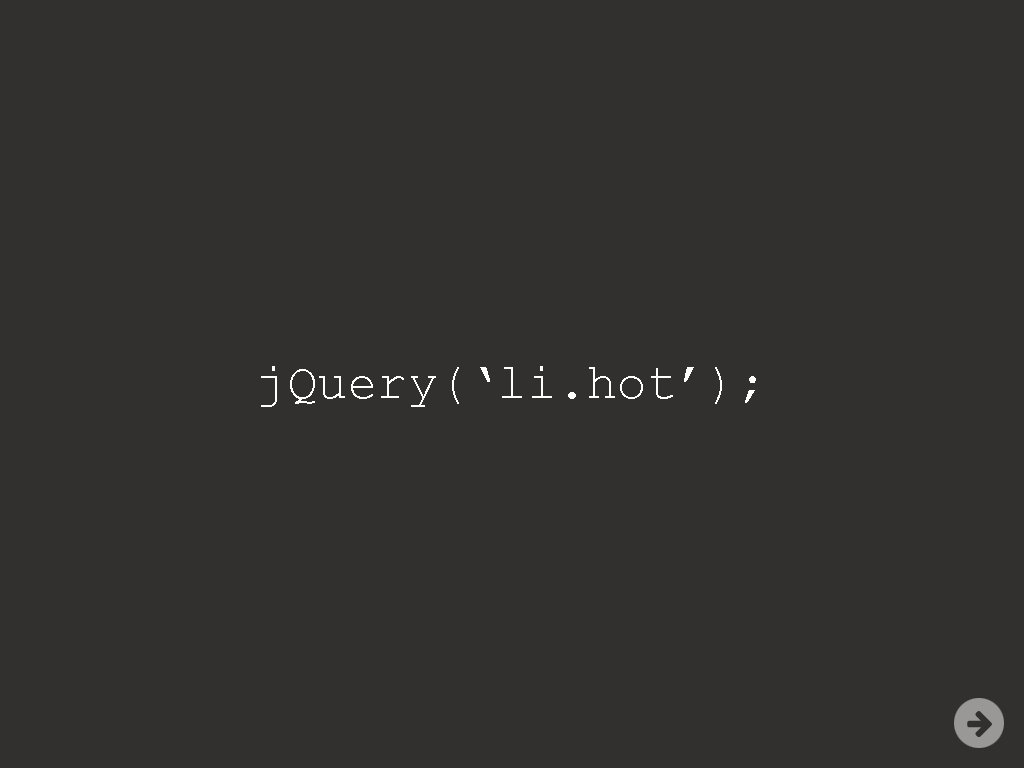
j. Query(‘li. hot’);
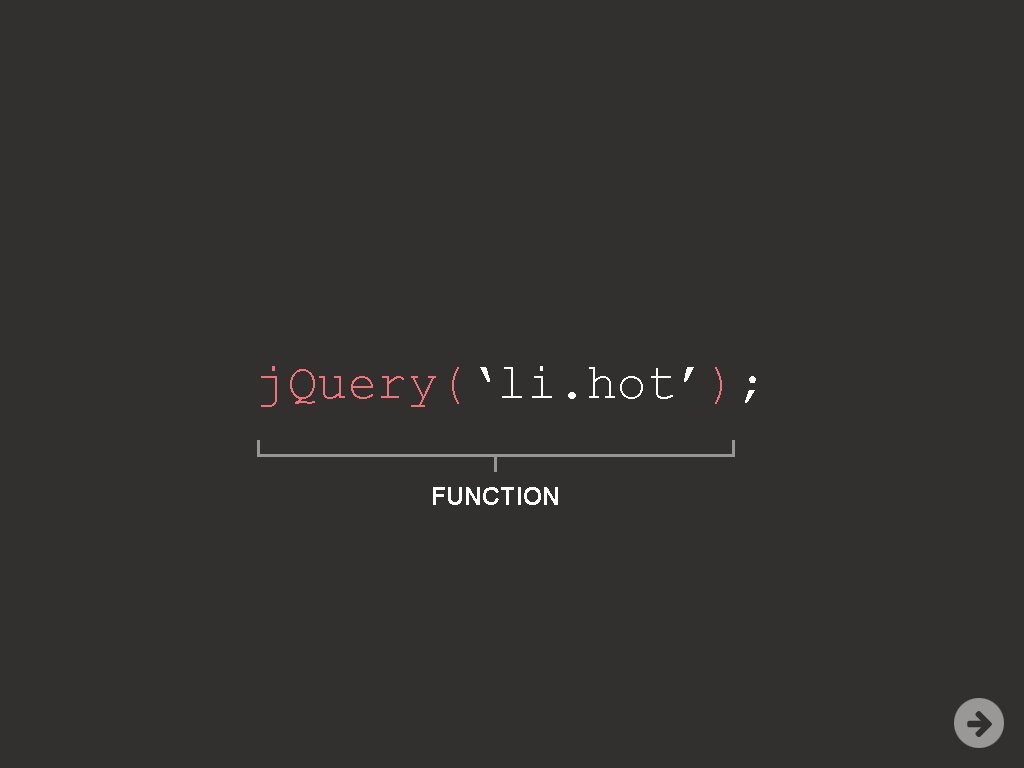
j. Query(‘li. hot’); FUNCTION
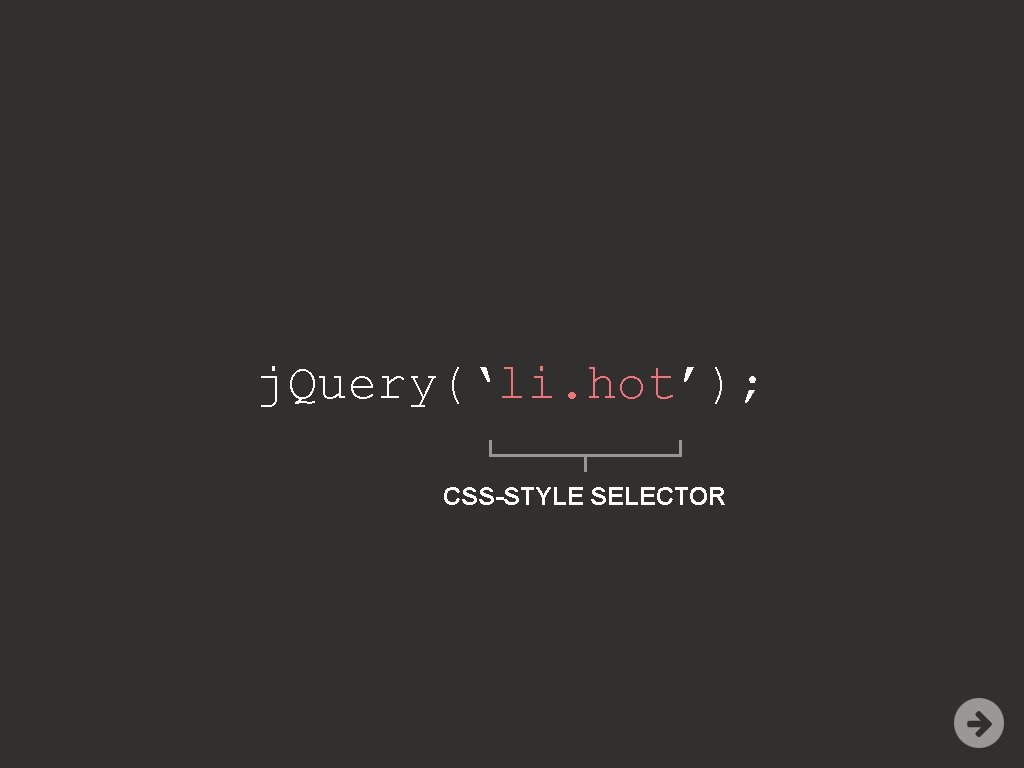
j. Query(‘li. hot’); CSS-STYLE SELECTOR
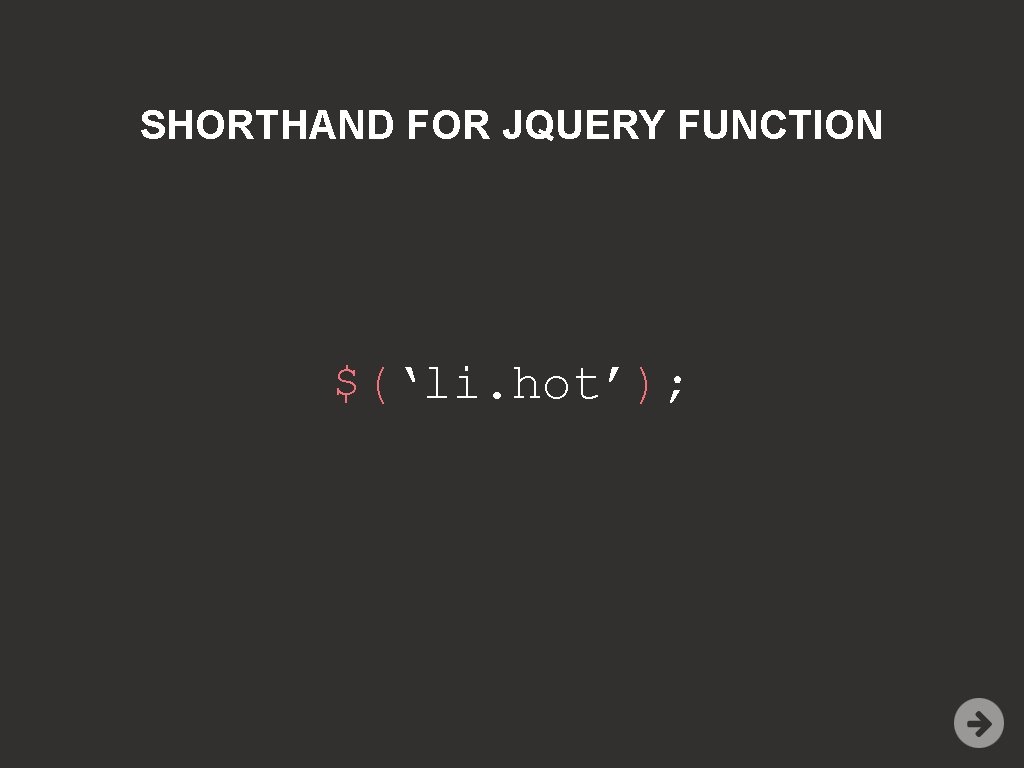
SHORTHAND FOR JQUERY FUNCTION $(‘li. hot’);
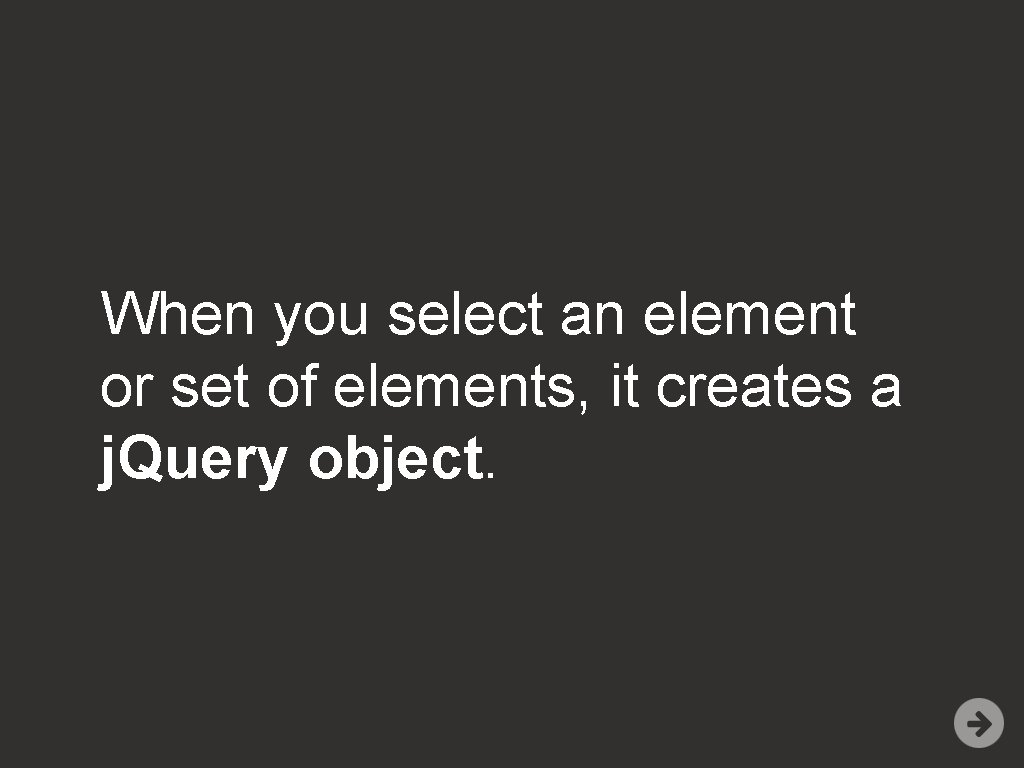
When you select an element or set of elements, it creates a j. Query object.
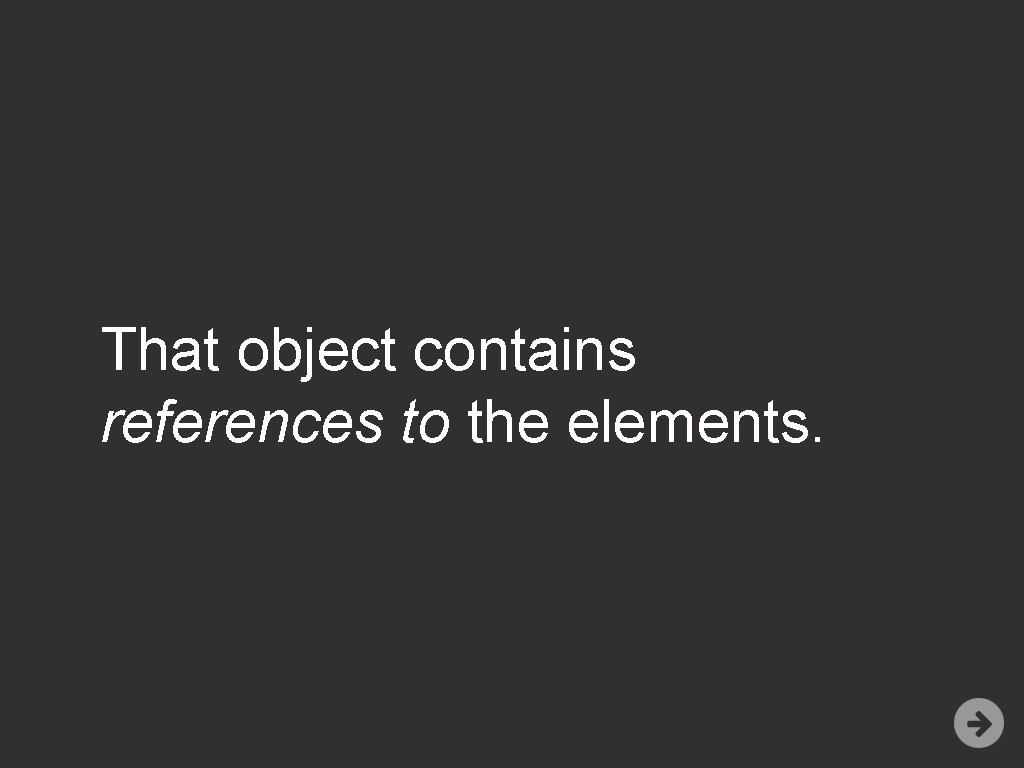
That object contains references to the elements.
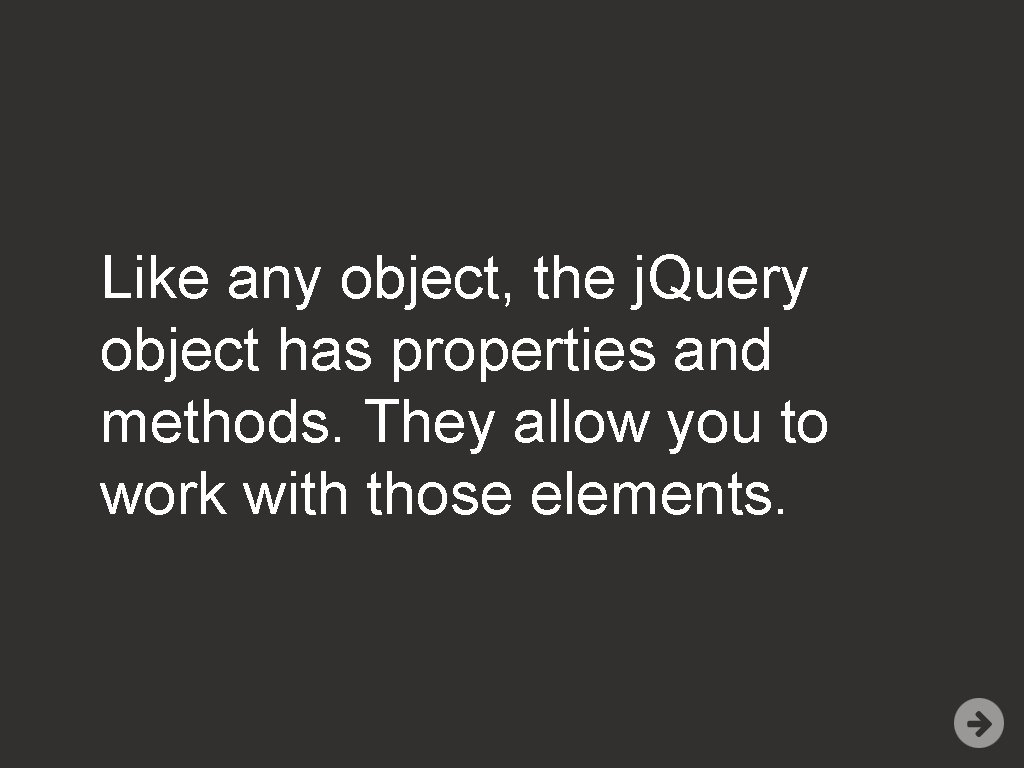
Like any object, the j. Query object has properties and methods. They allow you to work with those elements.
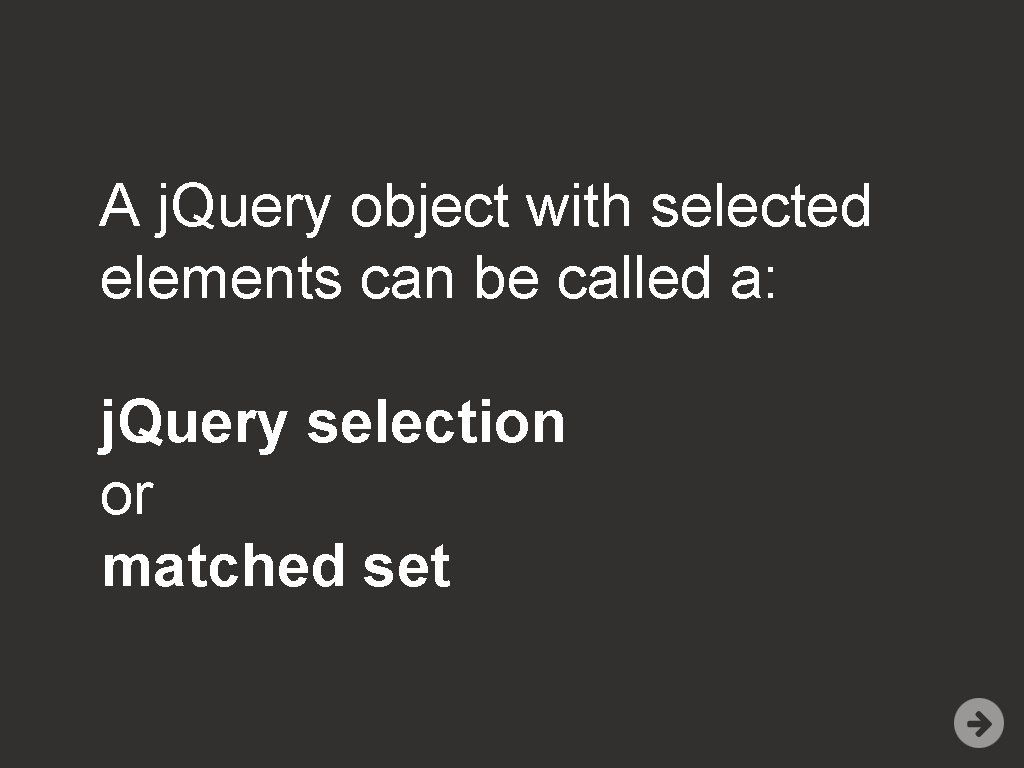
A j. Query object with selected elements can be called a: j. Query selection or matched set
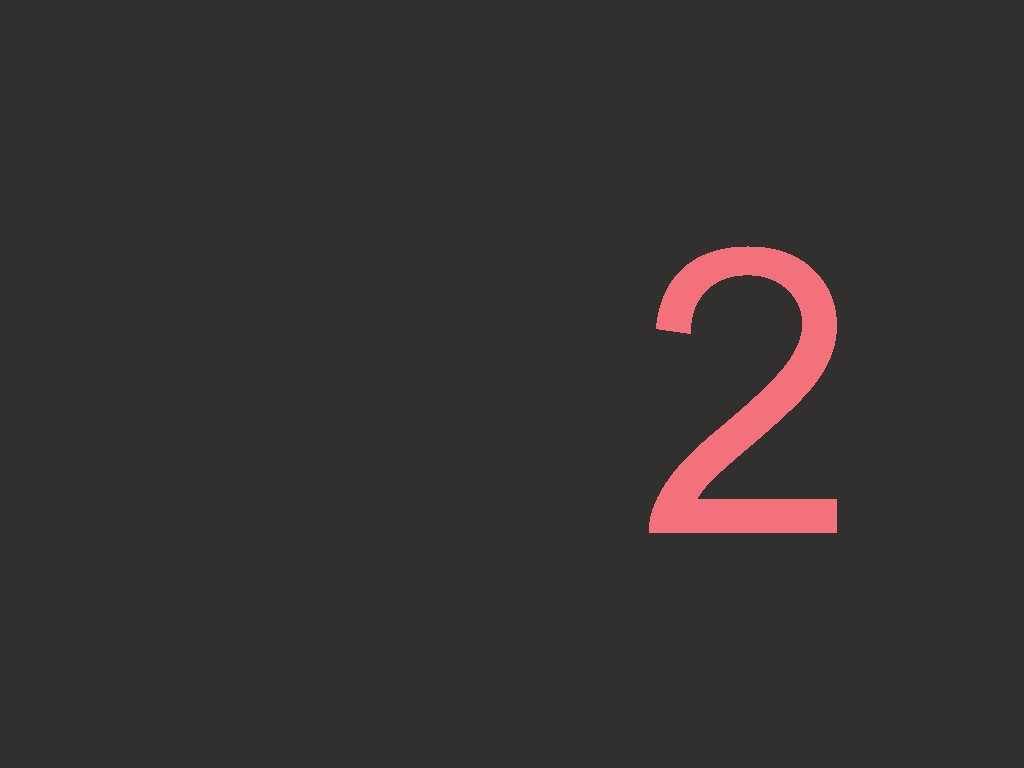
2
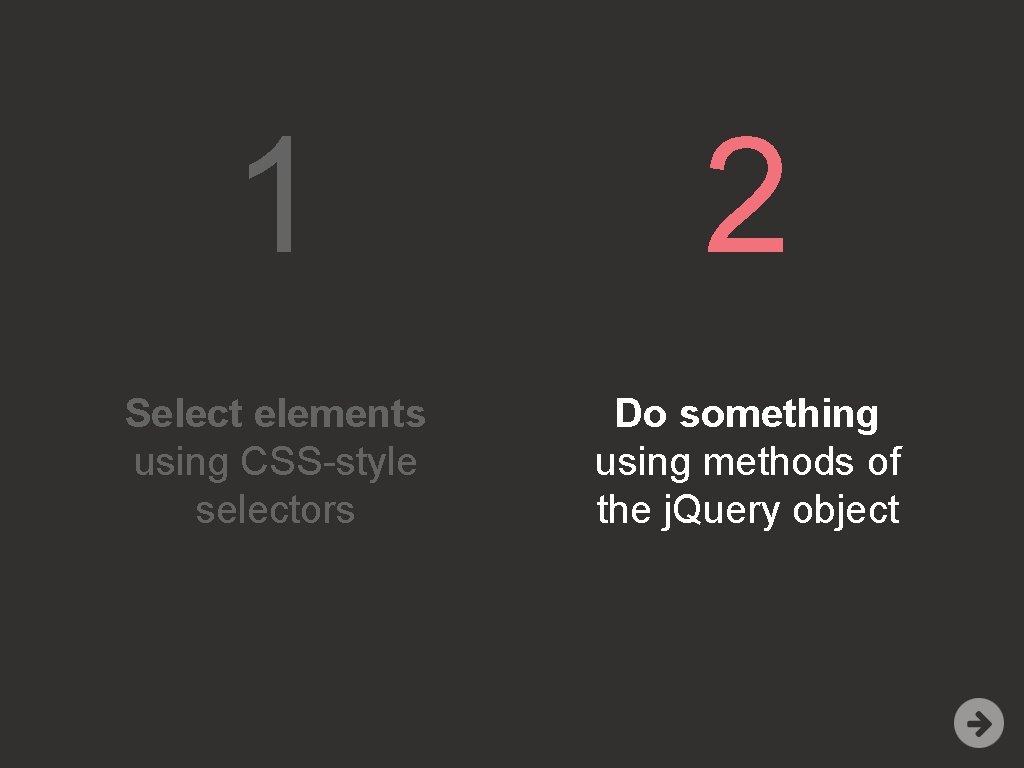
1 2 Select elements using CSS-style selectors Do something using methods of the j. Query object
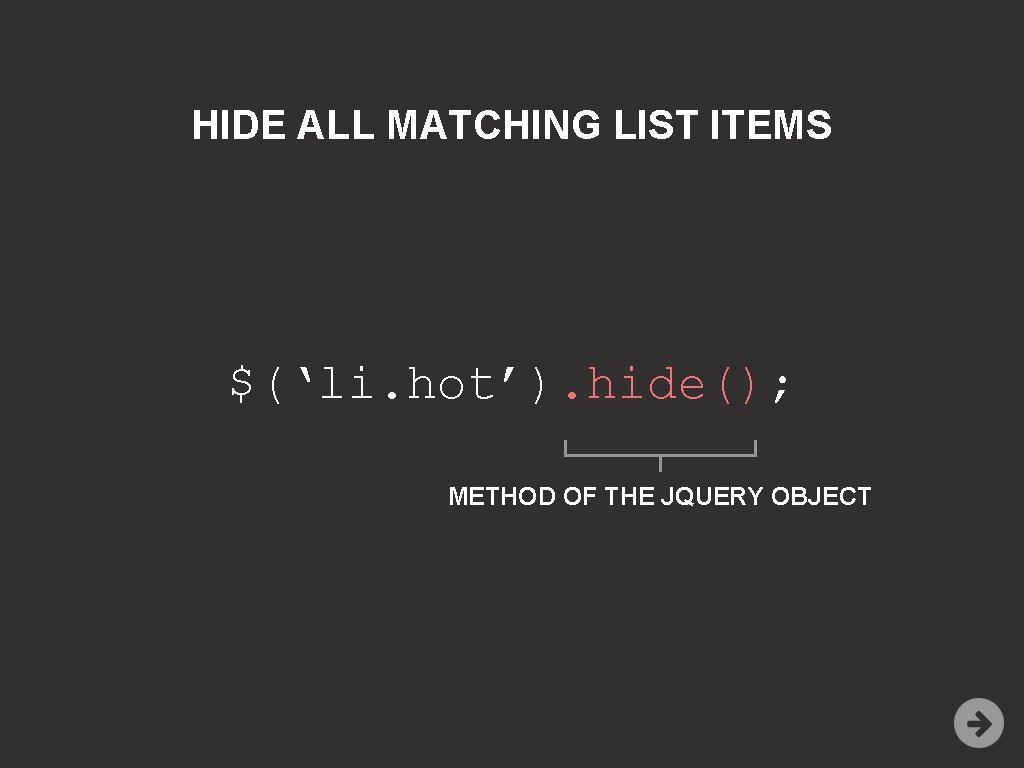
HIDE ALL MATCHING LIST ITEMS $(‘li. hot’). hide(); METHOD OF THE JQUERY OBJECT
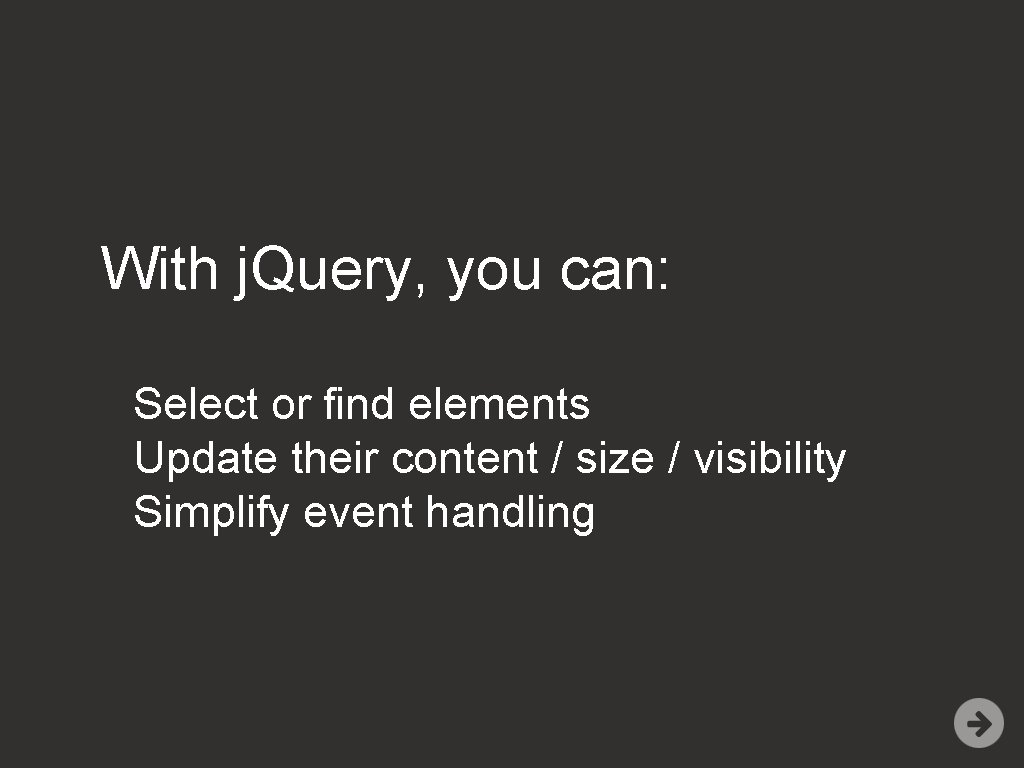
With j. Query, you can: Select or find elements Update their content / size / visibility Simplify event handling
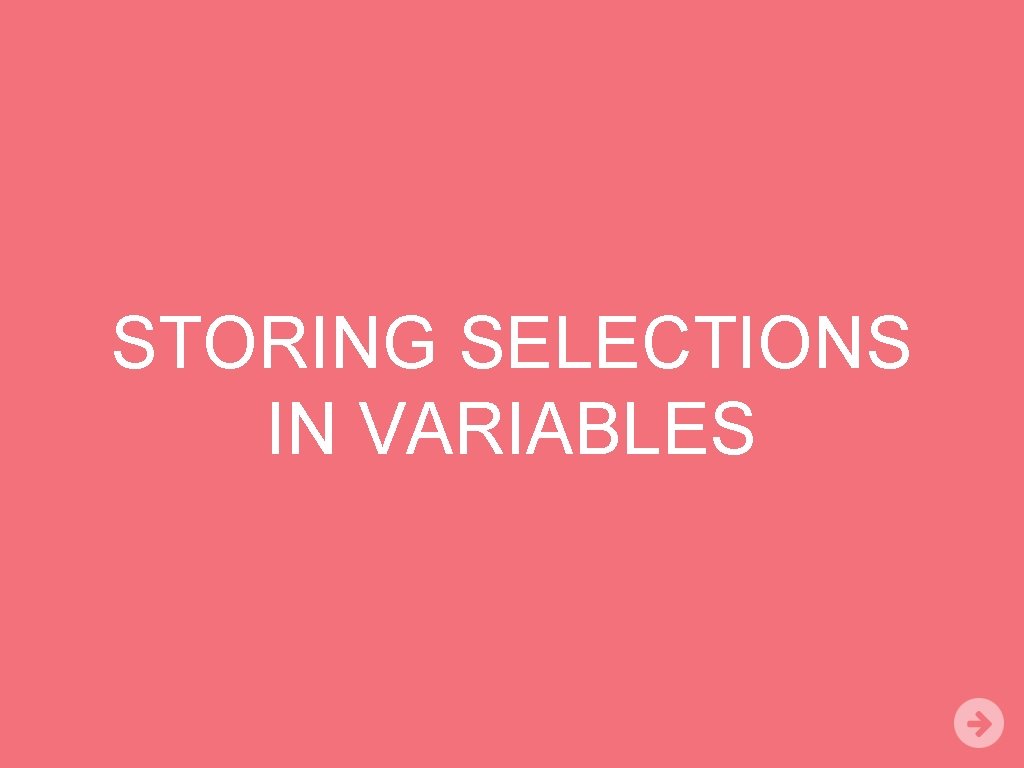
STORING SELECTIONS IN VARIABLES
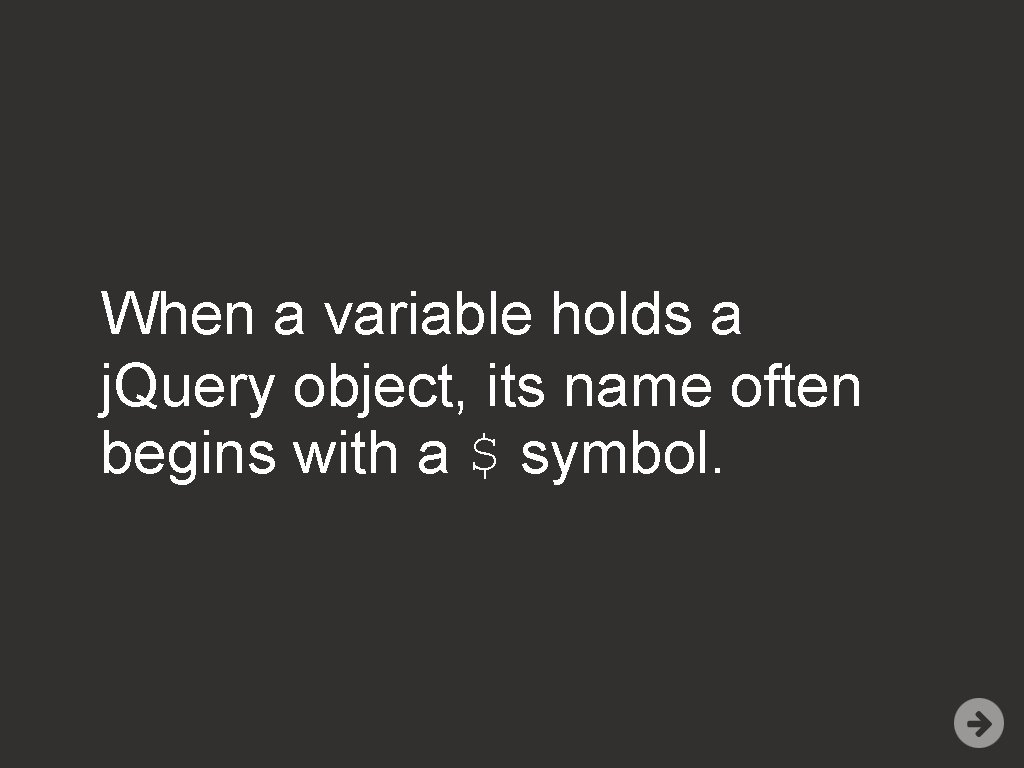
When a variable holds a j. Query object, its name often begins with a $ symbol.
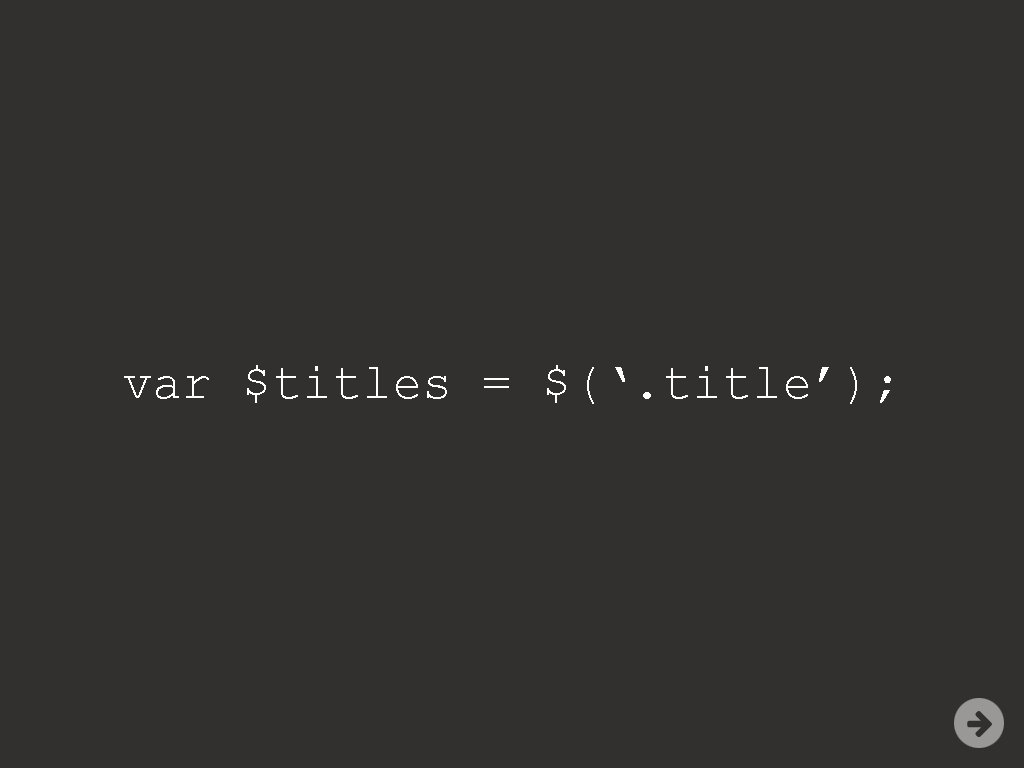
var $titles = $(‘. title’);
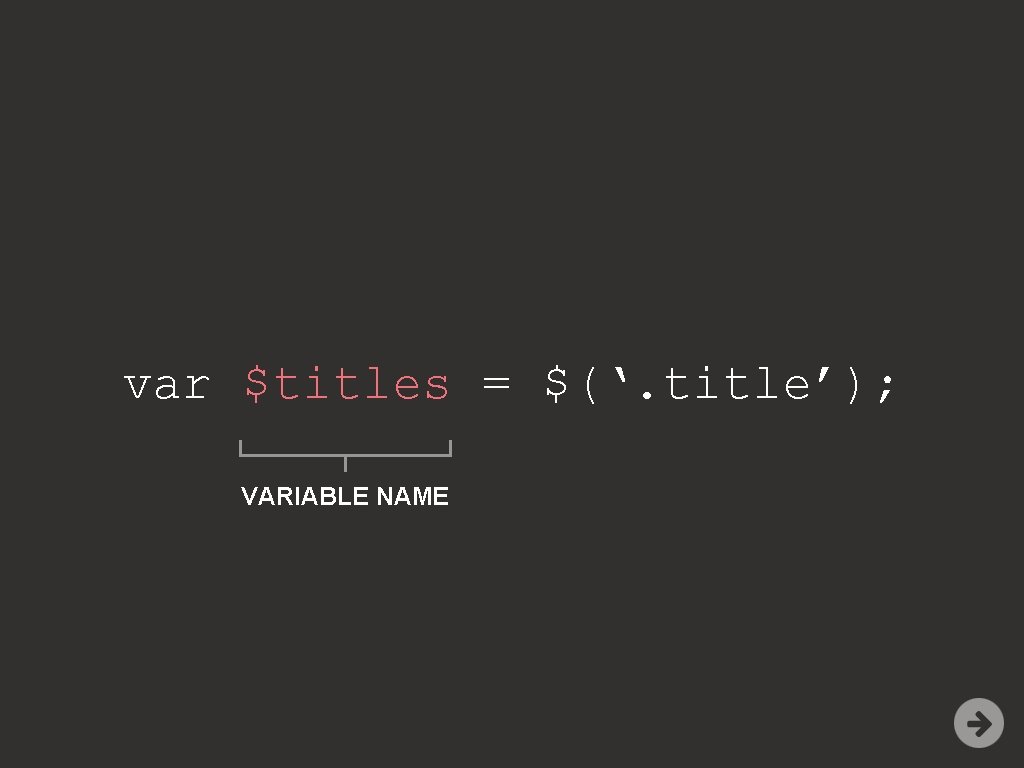
var $titles = $(‘. title’); VARIABLE NAME
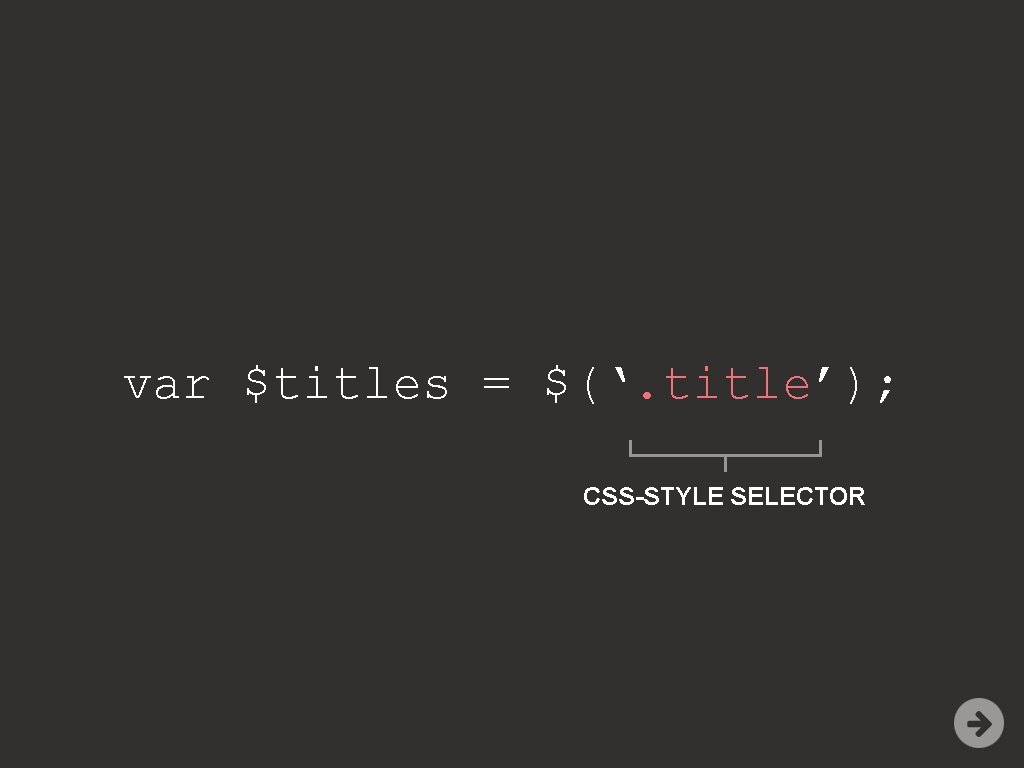
var $titles = $(‘. title’); CSS-STYLE SELECTOR
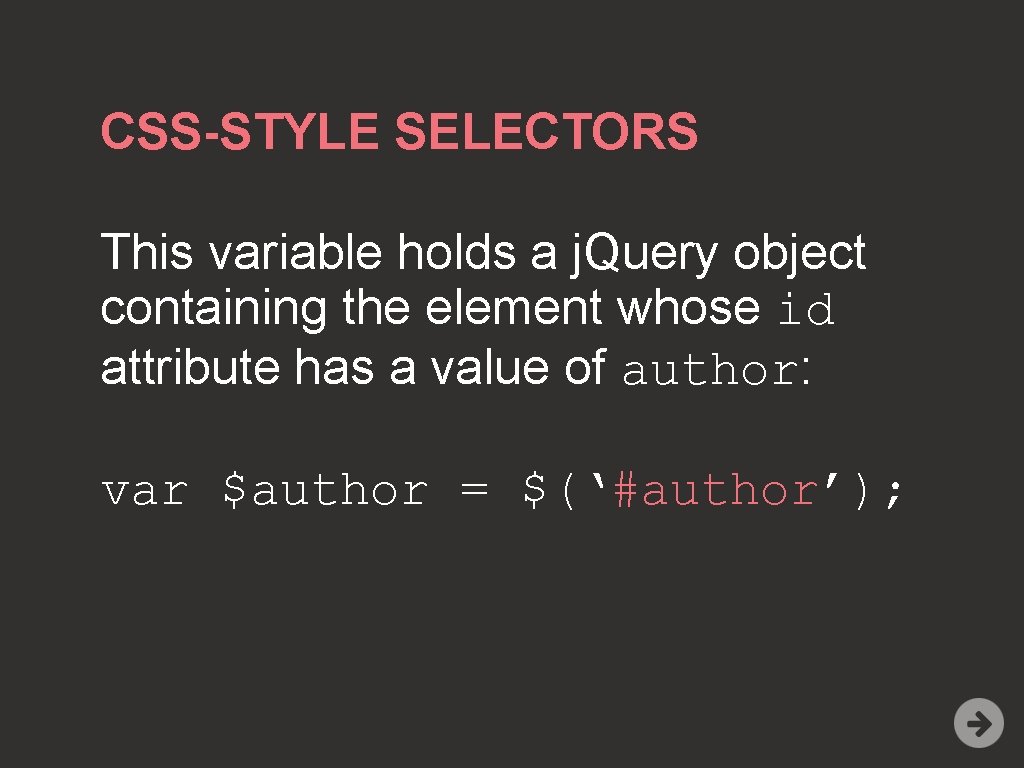
CSS-STYLE SELECTORS This variable holds a j. Query object containing the element whose id attribute has a value of author: var $author = $(‘#author’);
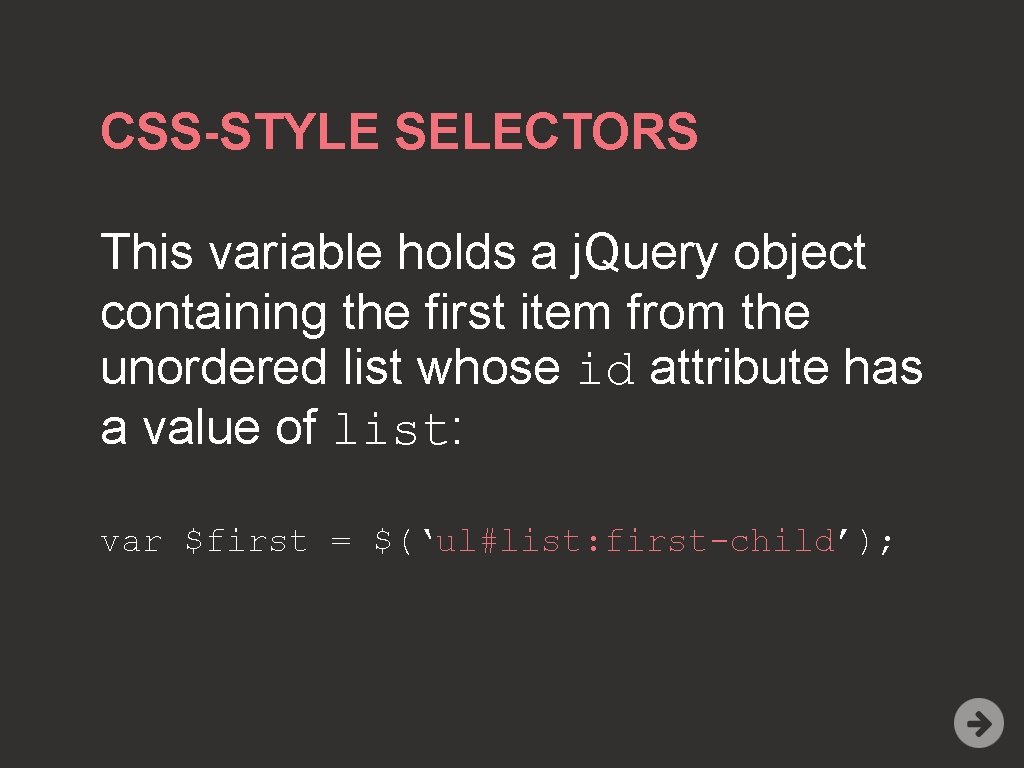
CSS-STYLE SELECTORS This variable holds a j. Query object containing the first item from the unordered list whose id attribute has a value of list: var $first = $(‘ul#list: first-child’);
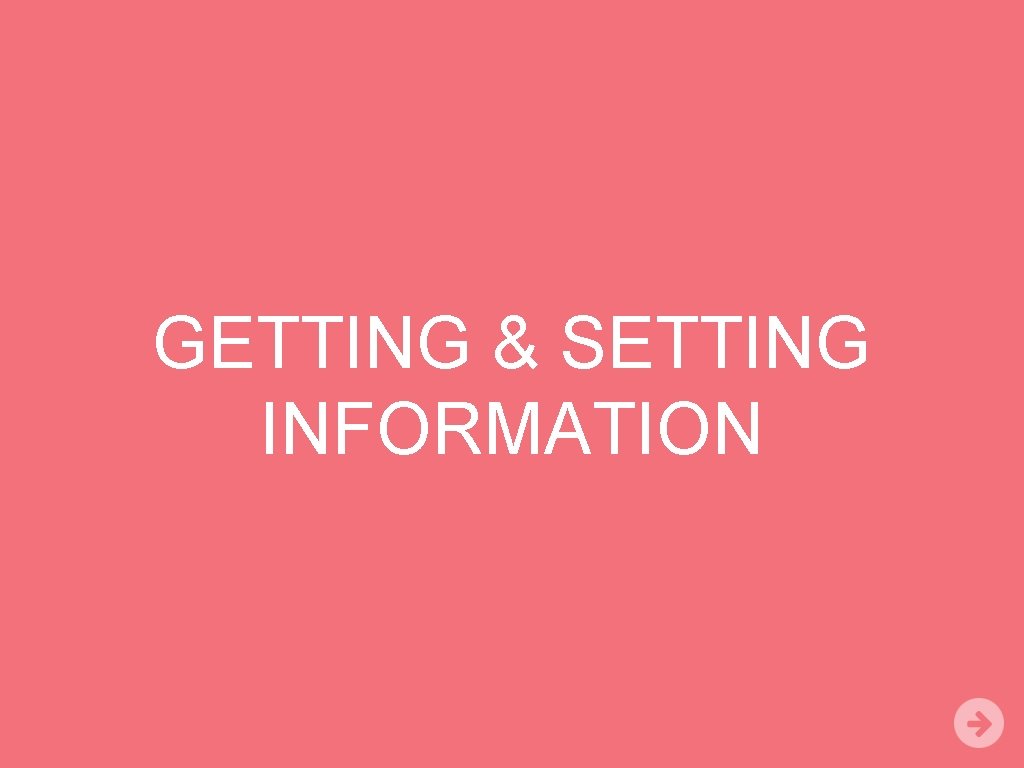
GETTING & SETTING INFORMATION
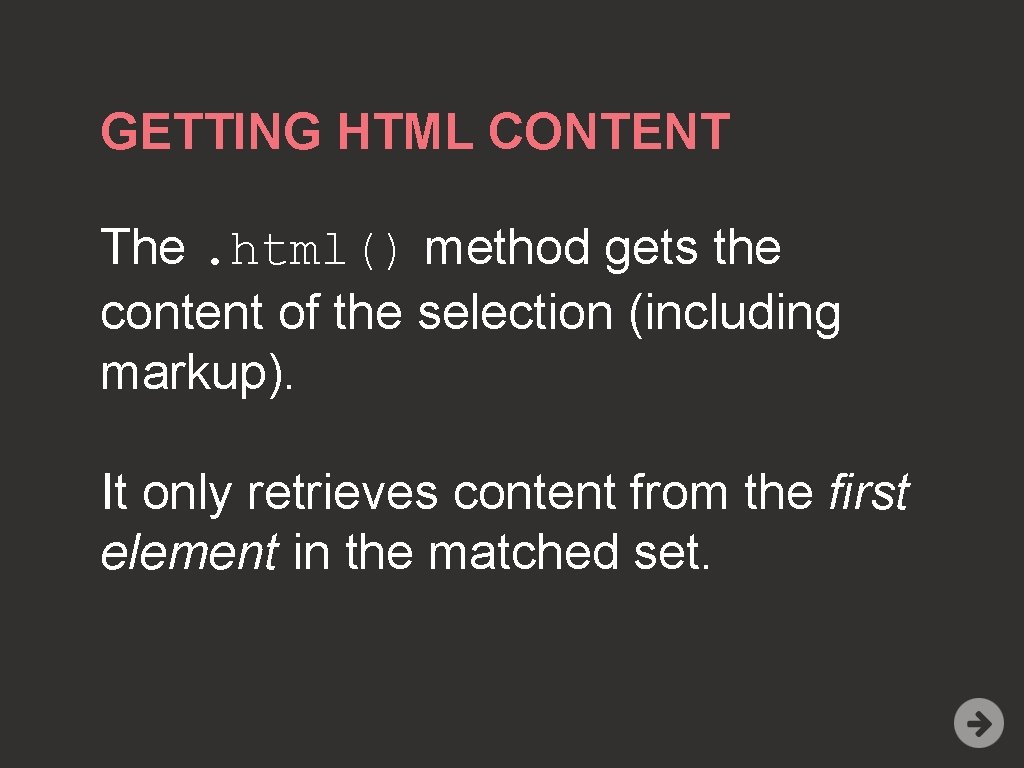
GETTING HTML CONTENT The. html() method gets the content of the selection (including markup). It only retrieves content from the first element in the matched set.
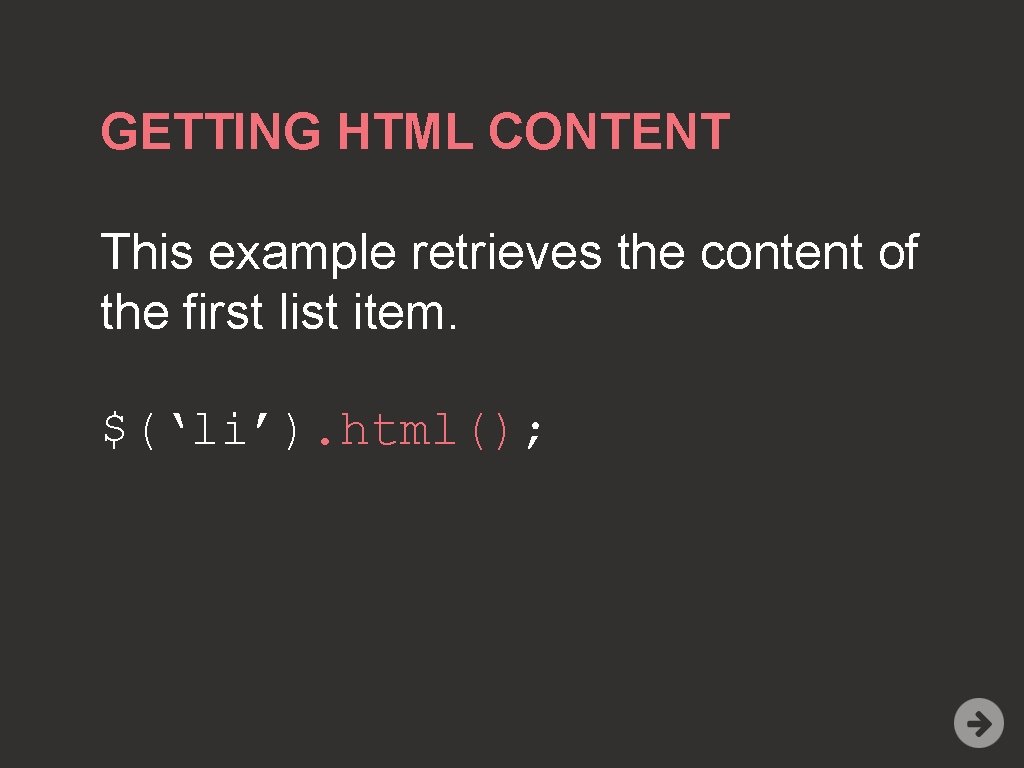
GETTING HTML CONTENT This example retrieves the content of the first list item. $(‘li’). html();
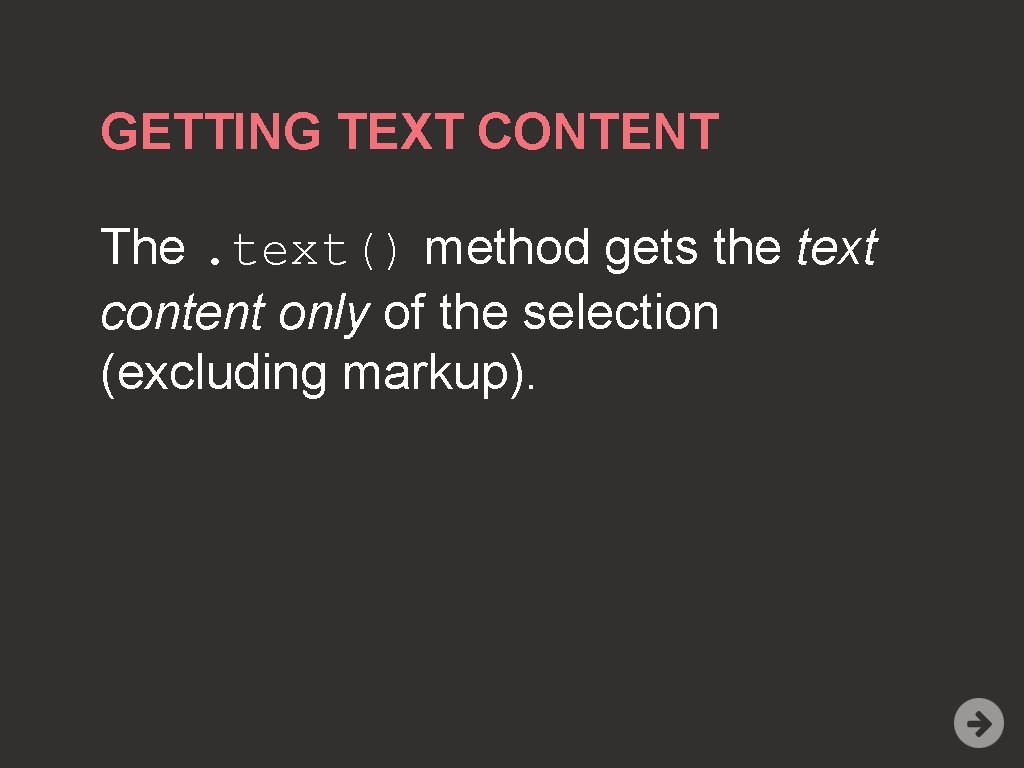
GETTING TEXT CONTENT The. text() method gets the text content only of the selection (excluding markup).
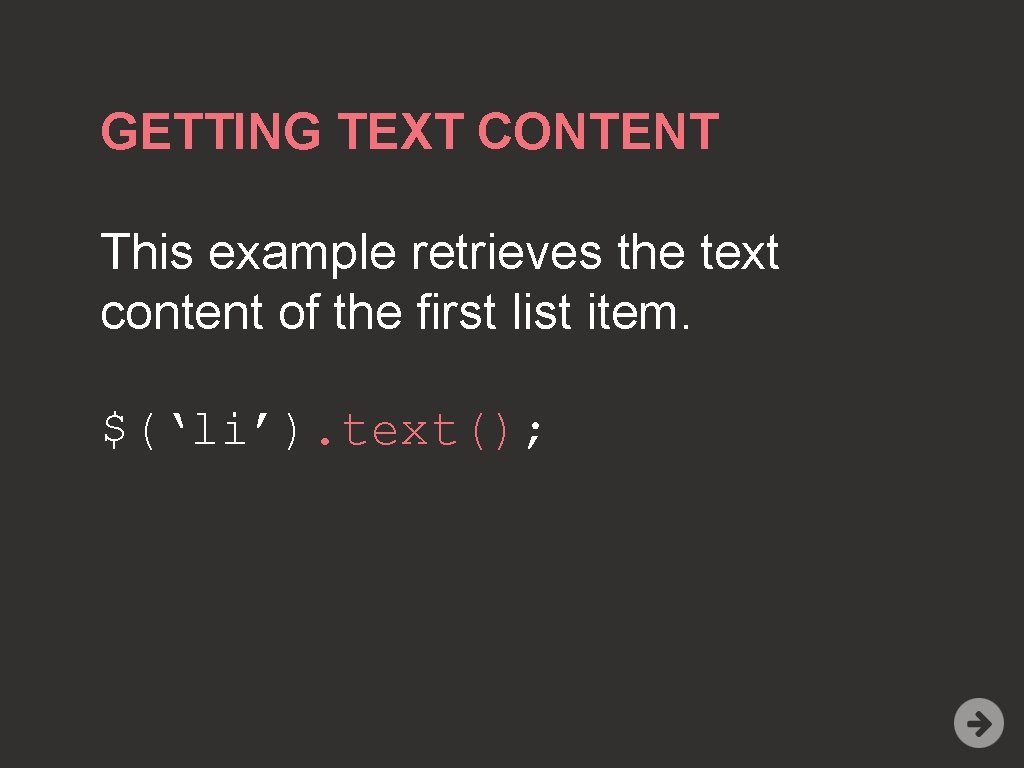
GETTING TEXT CONTENT This example retrieves the text content of the first list item. $(‘li’). text();
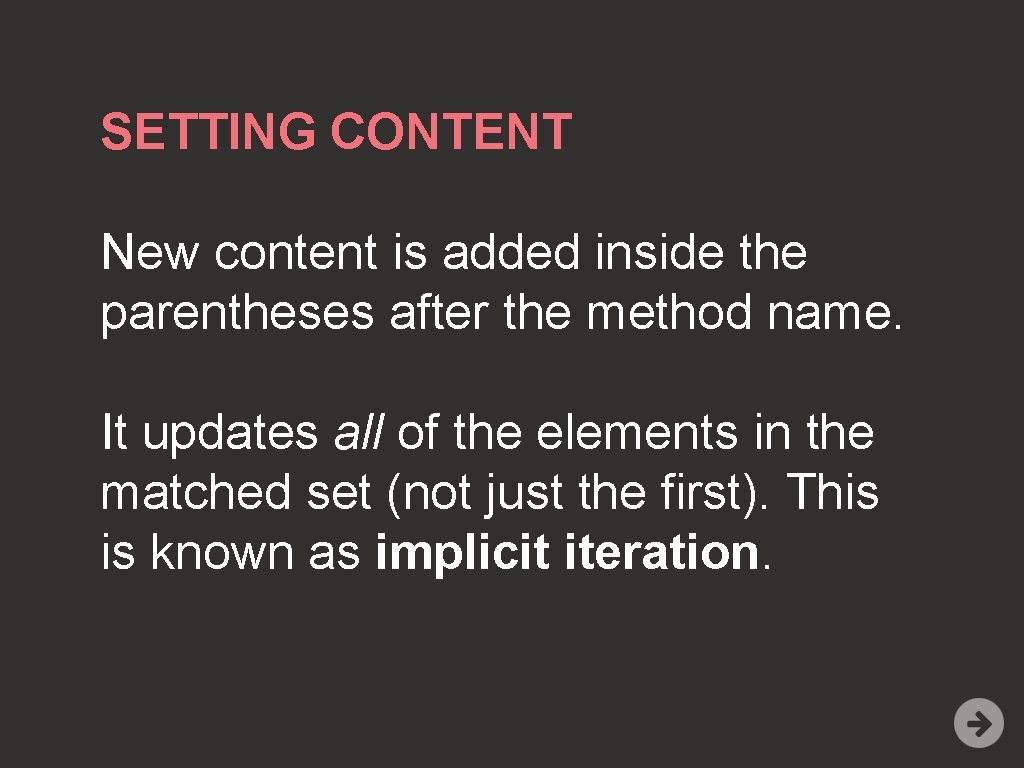
SETTING CONTENT New content is added inside the parentheses after the method name. It updates all of the elements in the matched set (not just the first). This is known as implicit iteration.
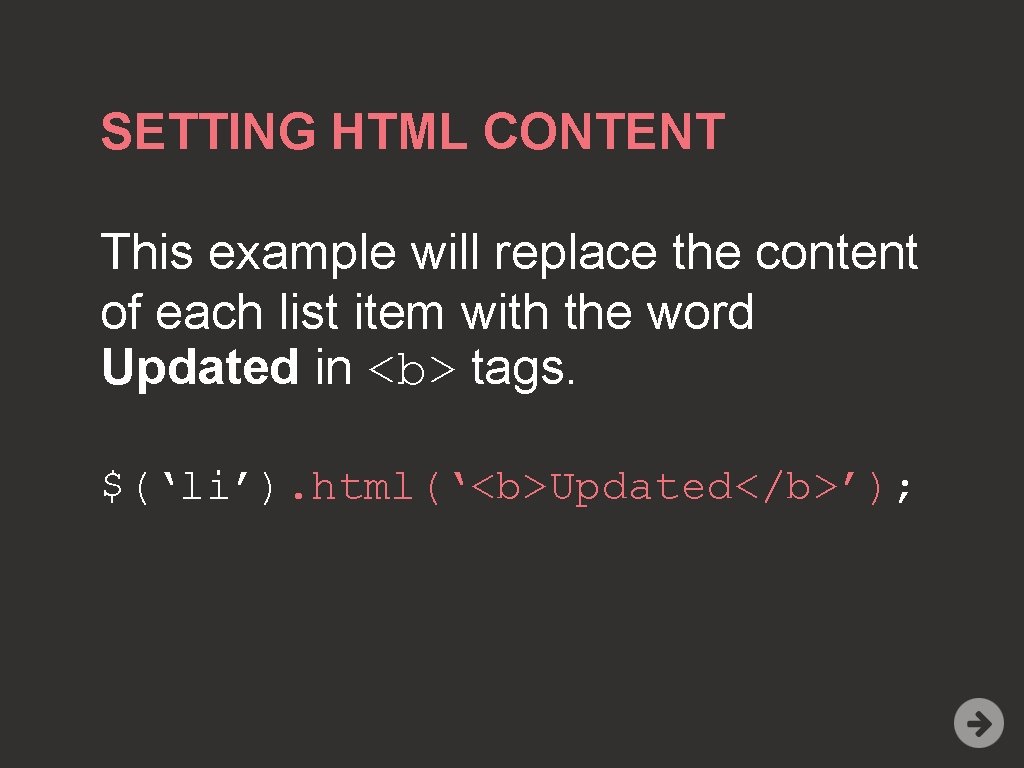
SETTING HTML CONTENT This example will replace the content of each list item with the word Updated in <b> tags. $(‘li’). html(‘<b>Updated</b>’);
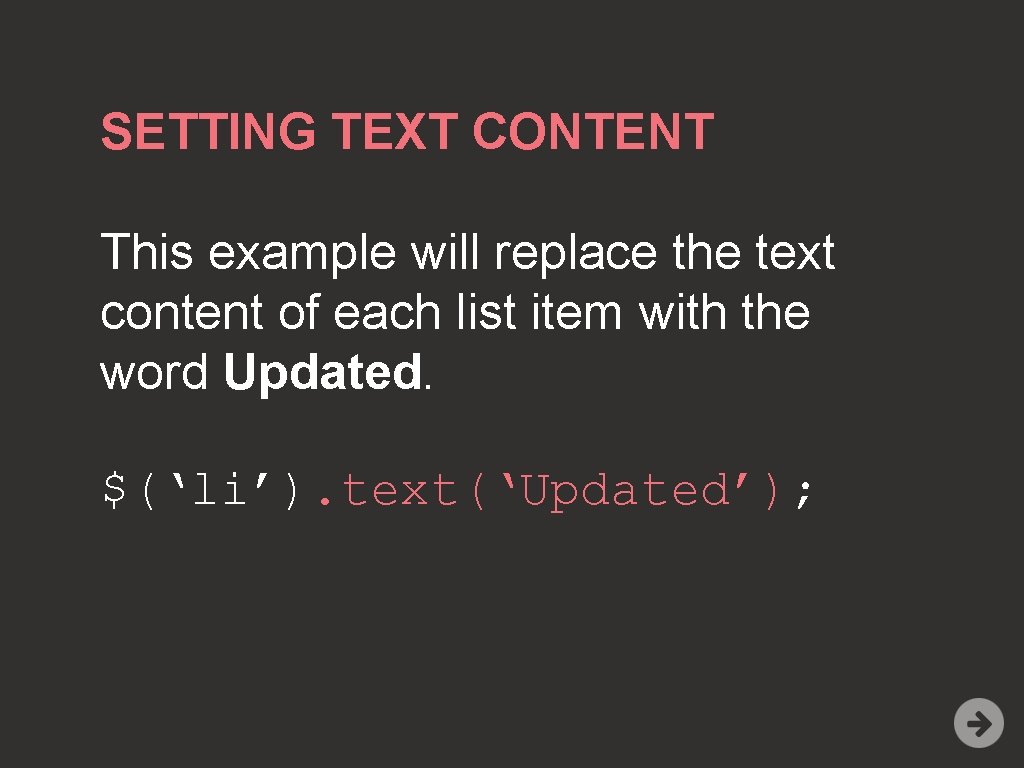
SETTING TEXT CONTENT This example will replace the text content of each list item with the word Updated. $(‘li’). text(‘Updated’);
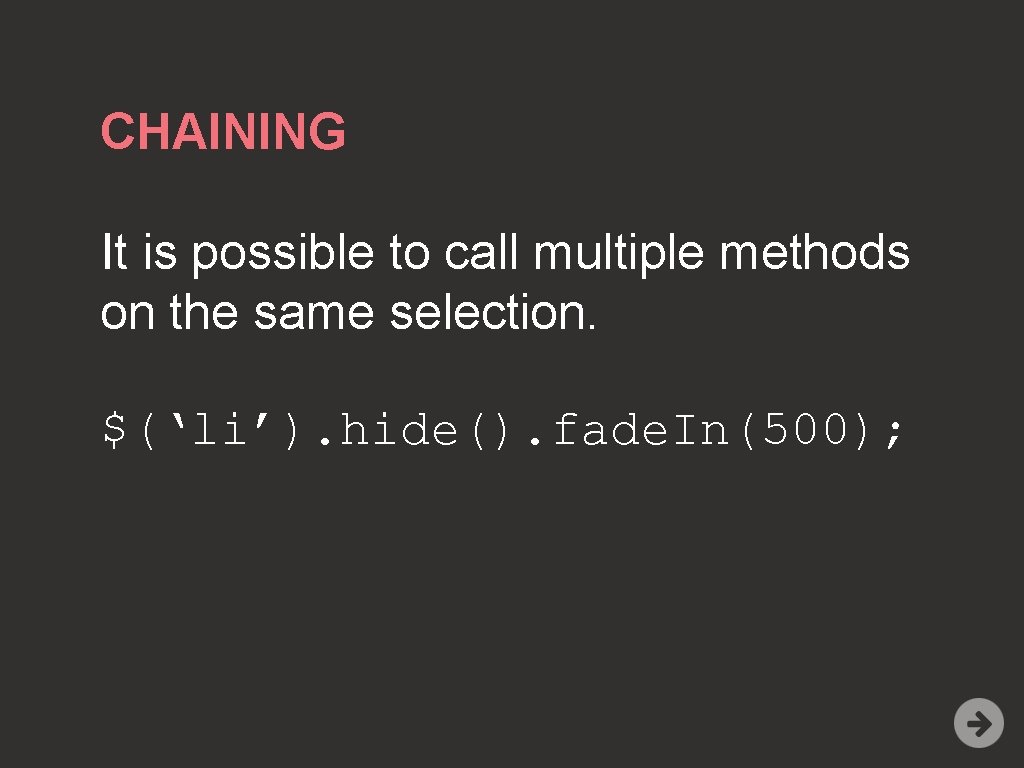
CHAINING It is possible to call multiple methods on the same selection. $(‘li’). hide(). fade. In(500);
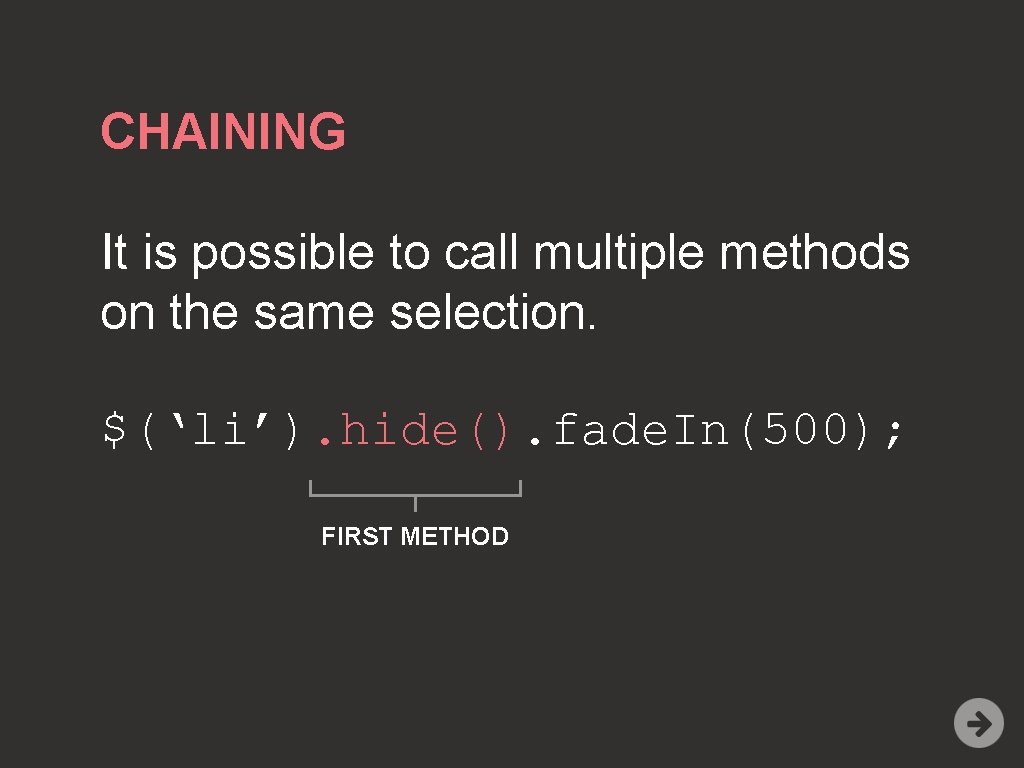
CHAINING It is possible to call multiple methods on the same selection. $(‘li’). hide(). fade. In(500); FIRST METHOD
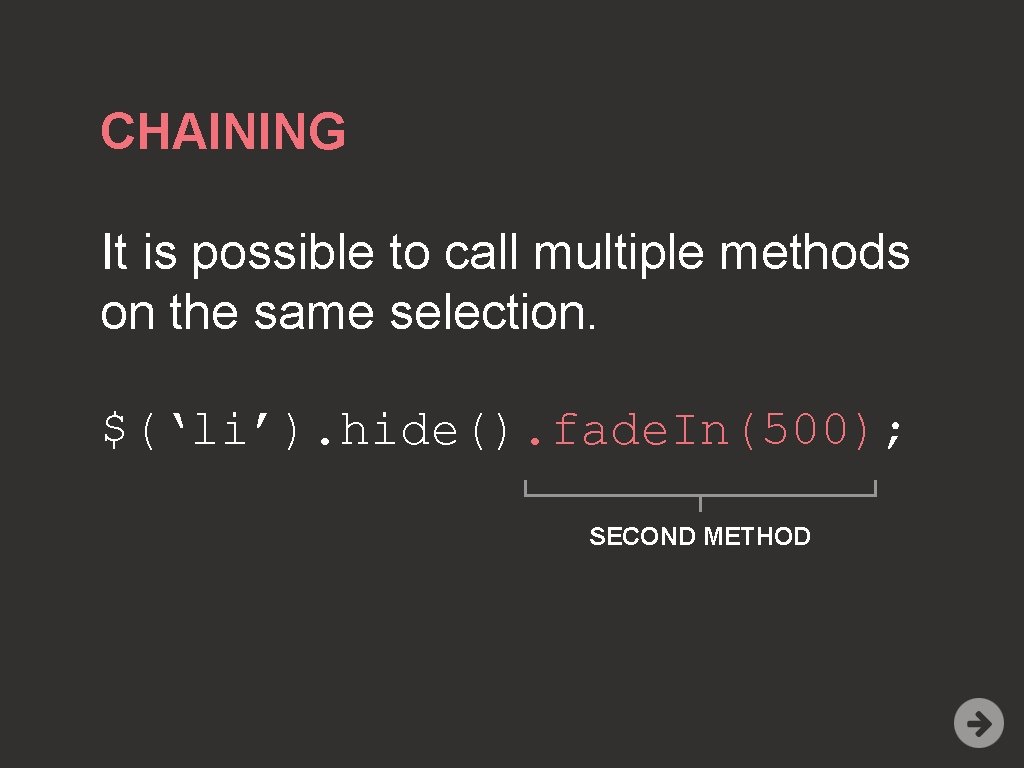
CHAINING It is possible to call multiple methods on the same selection. $(‘li’). hide(). fade. In(500); SECOND METHOD
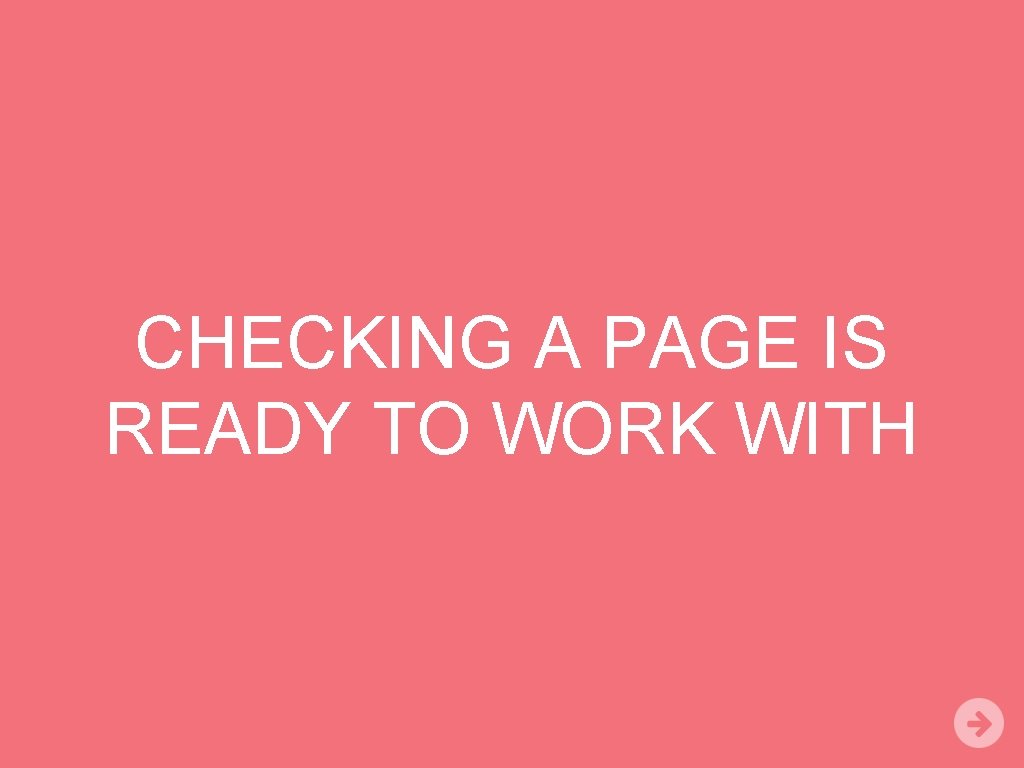
CHECKING A PAGE IS READY TO WORK WITH
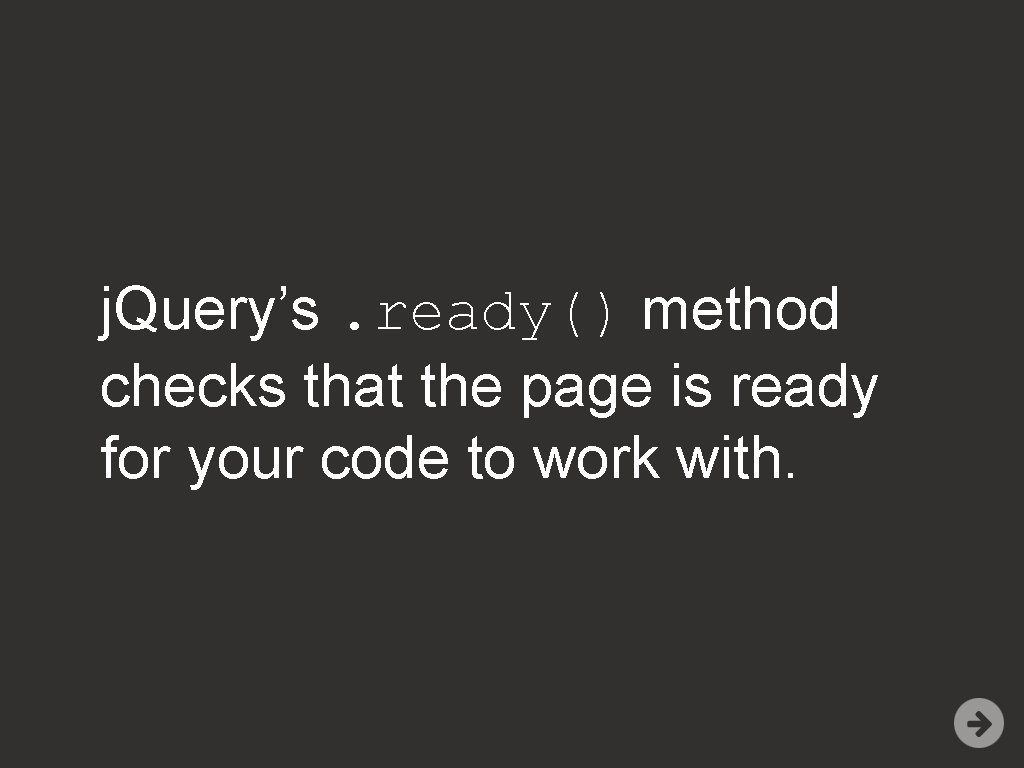
j. Query’s. ready() method checks that the page is ready for your code to work with.
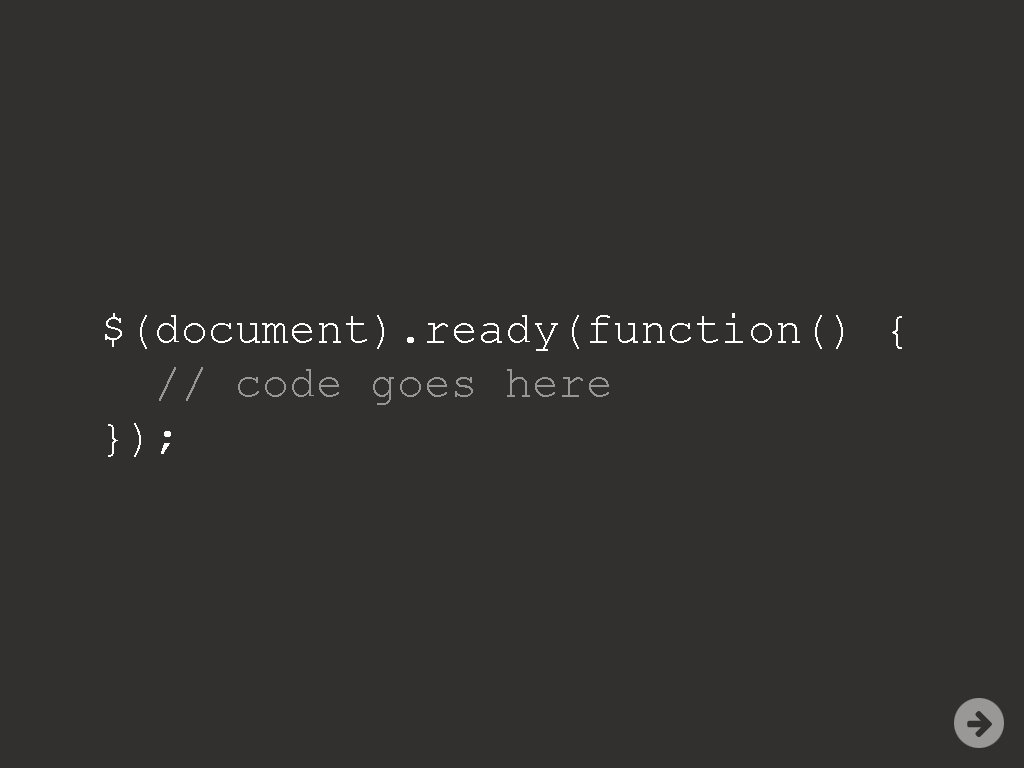
$(document). ready(function() { // code goes here });
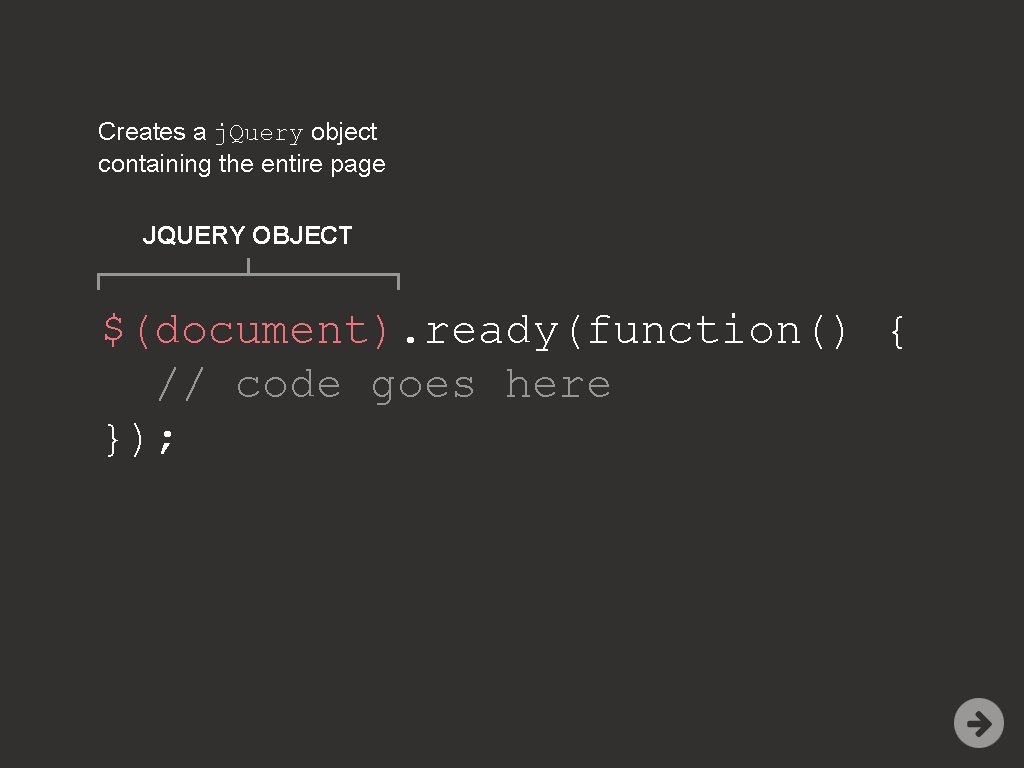
Creates a j. Query object containing the entire page JQUERY OBJECT $(document). ready(function() { // code goes here });
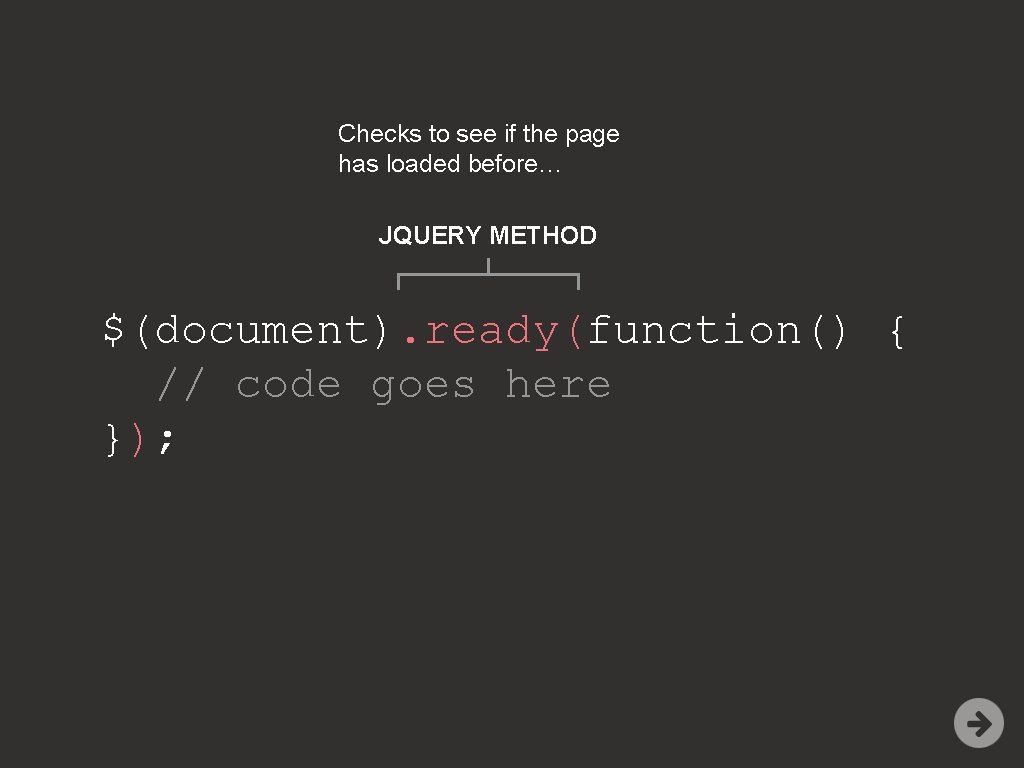
Checks to see if the page has loaded before… JQUERY METHOD $(document). ready(function() { // code goes here });
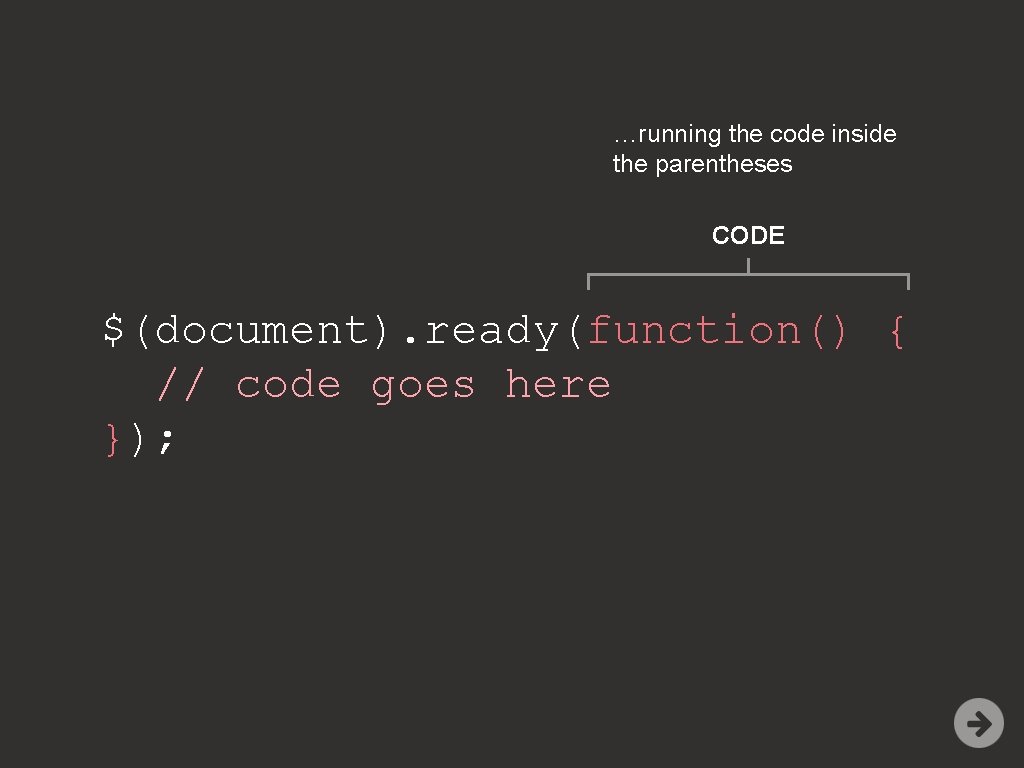
…running the code inside the parentheses CODE $(document). ready(function() { // code goes here });
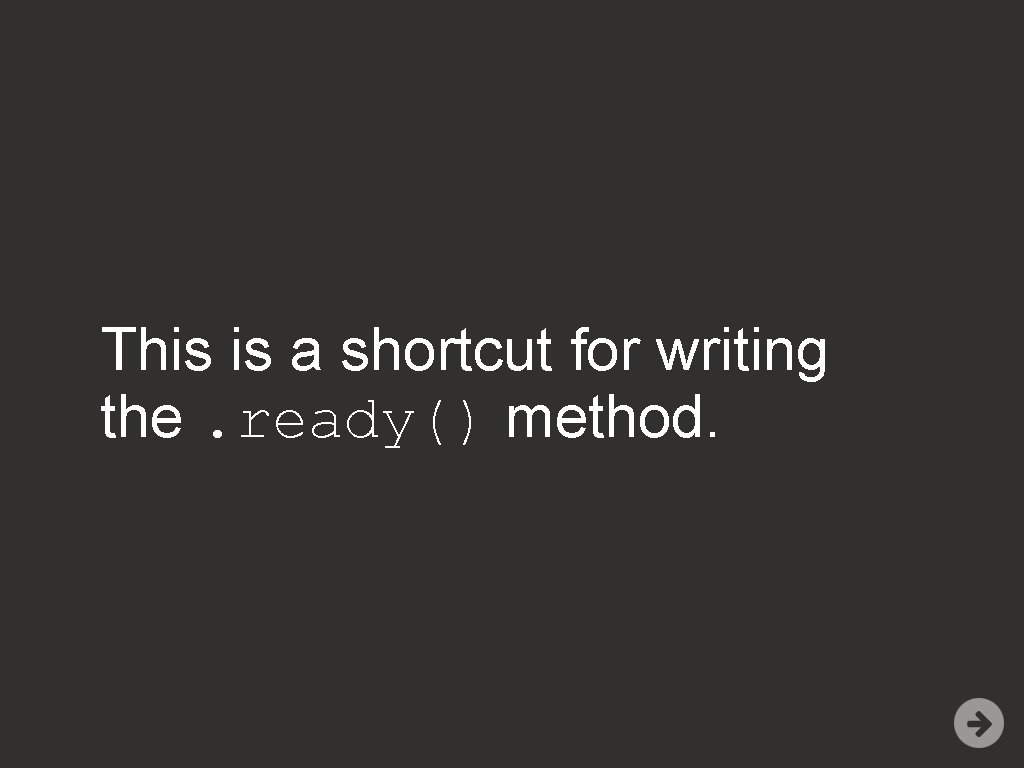
This is a shortcut for writing the. ready() method.
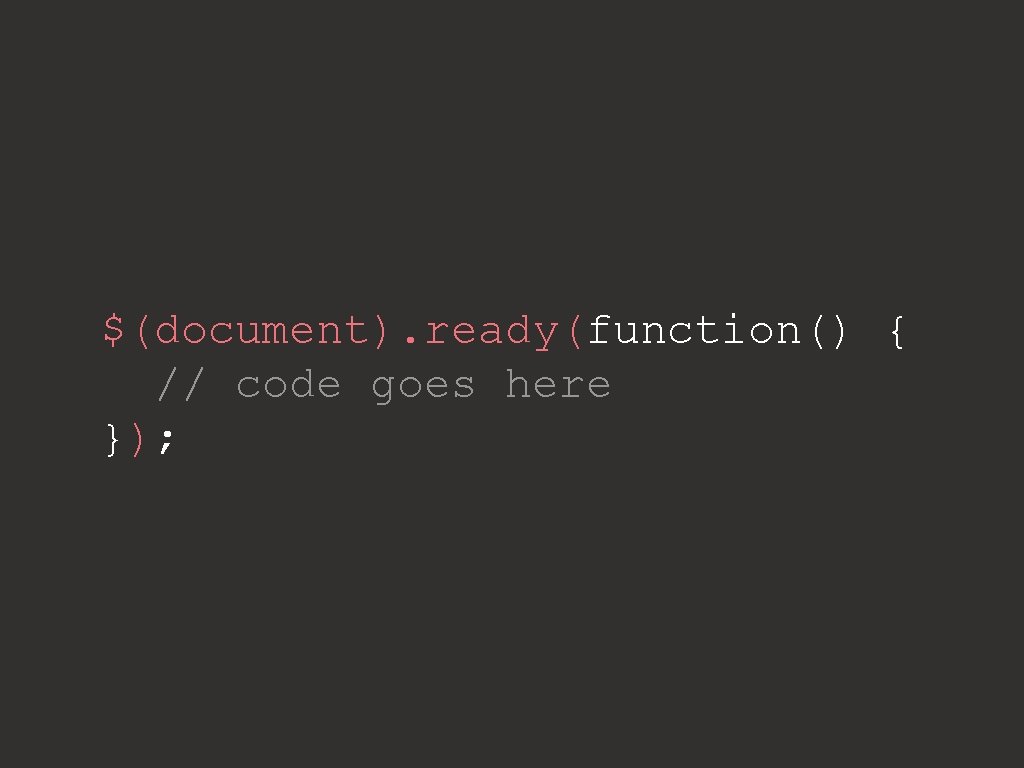
$(document). ready(function() { // code goes here });
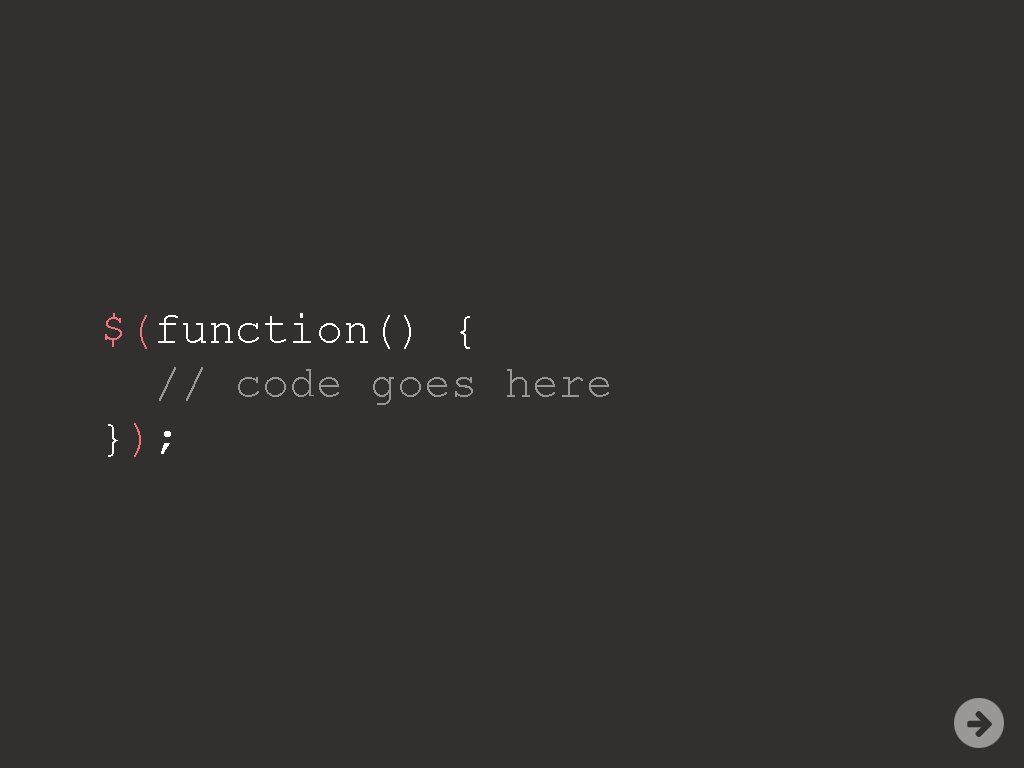
$(function() { // code goes here });
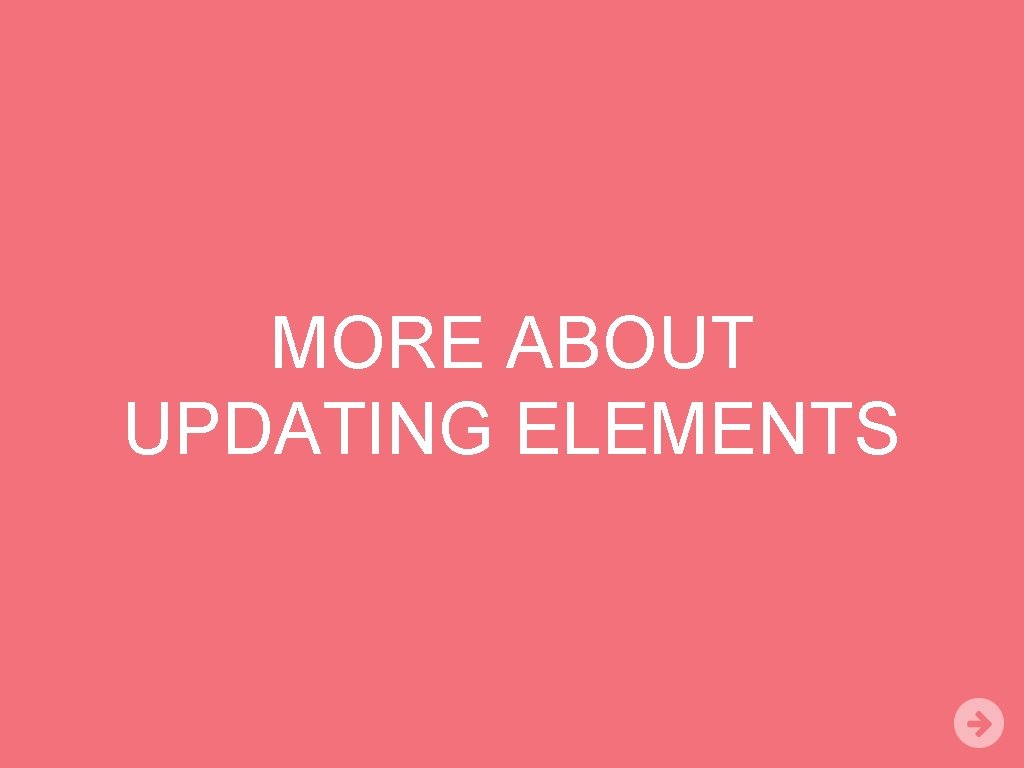
MORE ABOUT UPDATING ELEMENTS
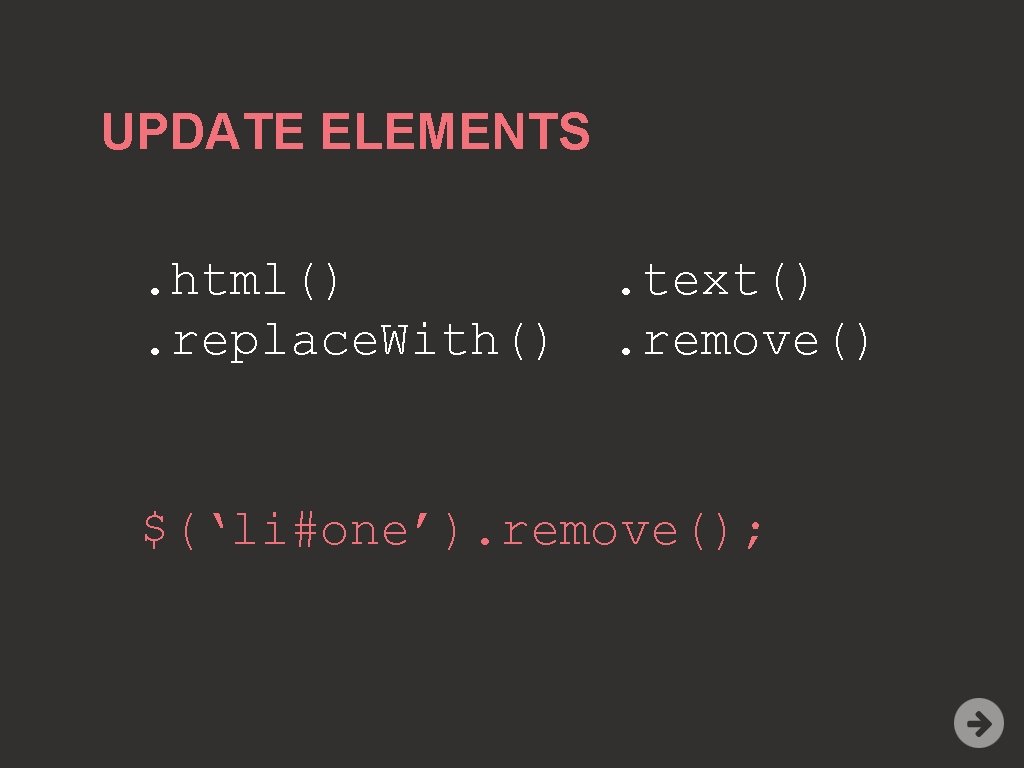
UPDATE ELEMENTS. html(). replace. With() . text(). remove() $(‘li#one’). remove();
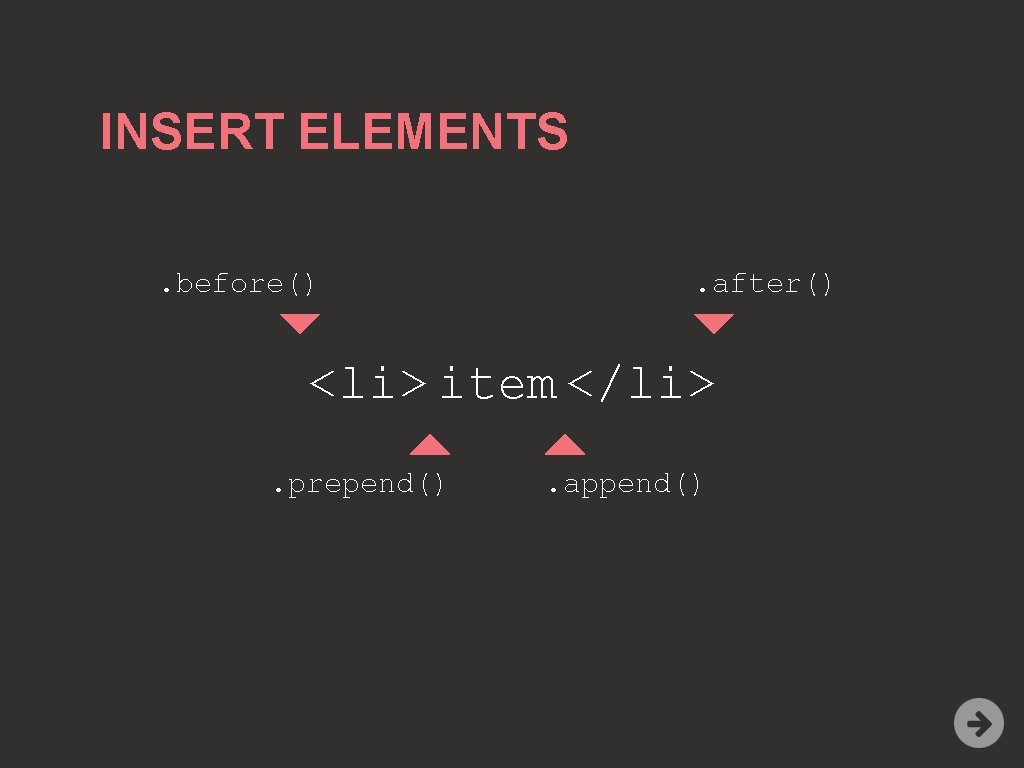
INSERT ELEMENTS. before() . after() <li> item </li>. prepend() . append()
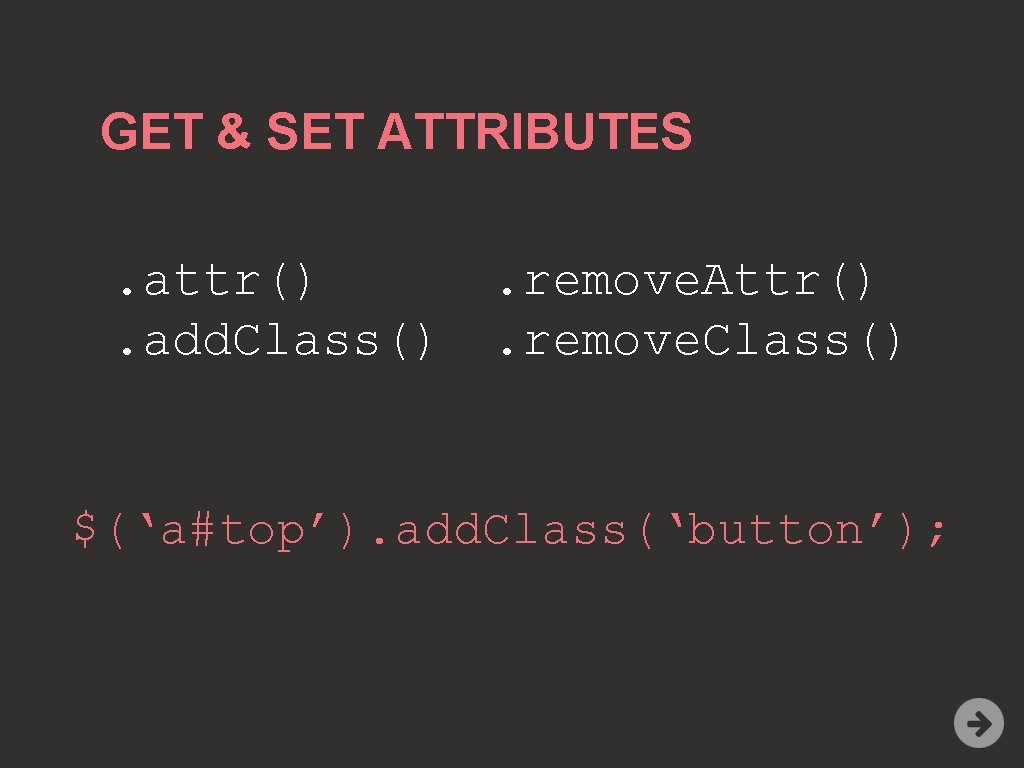
GET & SET ATTRIBUTES. attr(). add. Class() . remove. Attr(). remove. Class() $(‘a#top’). add. Class(‘button’);
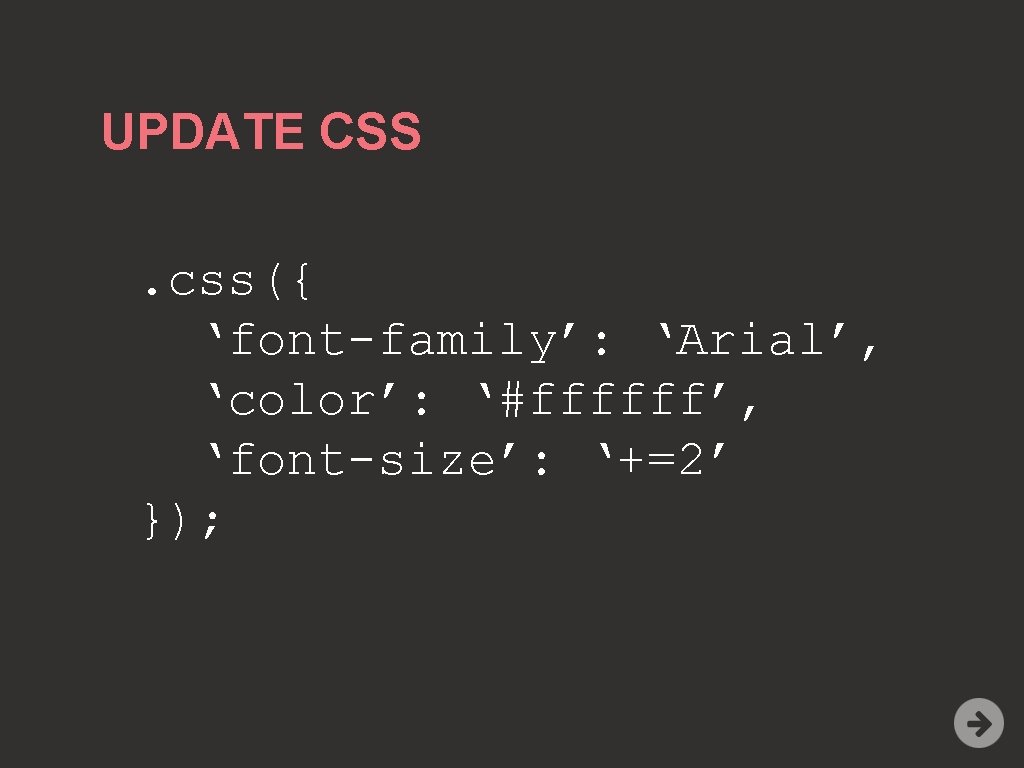
UPDATE CSS. css({ ‘font-family’: ‘Arial’, ‘color’: ‘#ffffff’, ‘font-size’: ‘+=2’ });
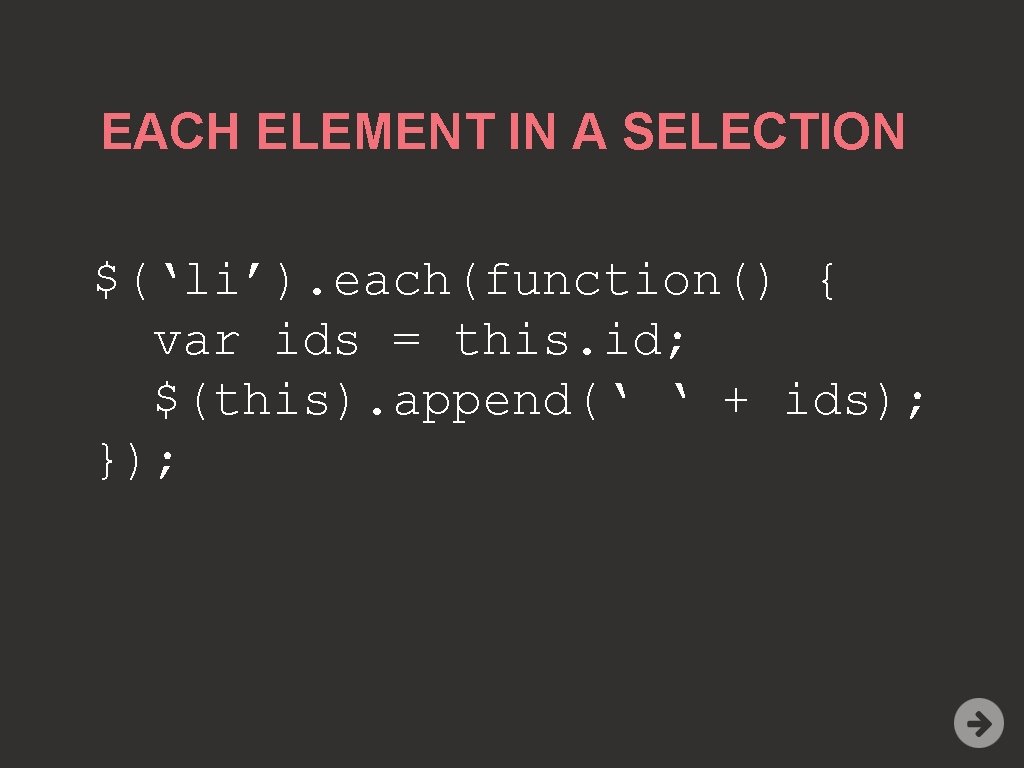
EACH ELEMENT IN A SELECTION $(‘li’). each(function() { var ids = this. id; $(this). append(‘ ‘ + ids); });
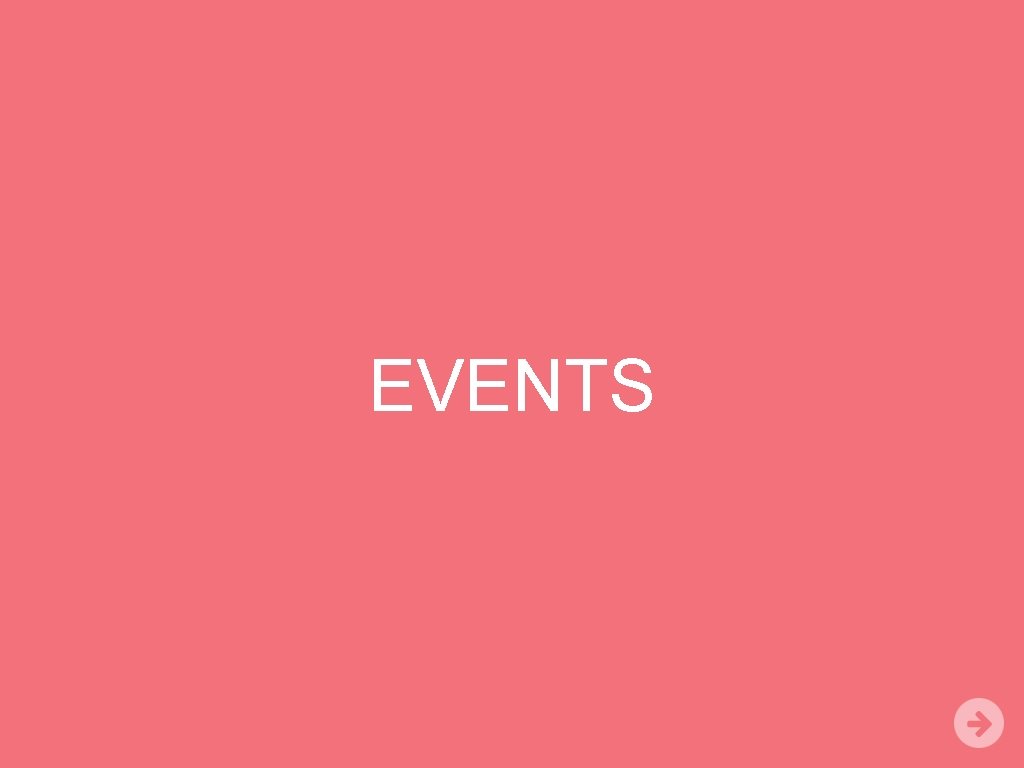
EVENTS
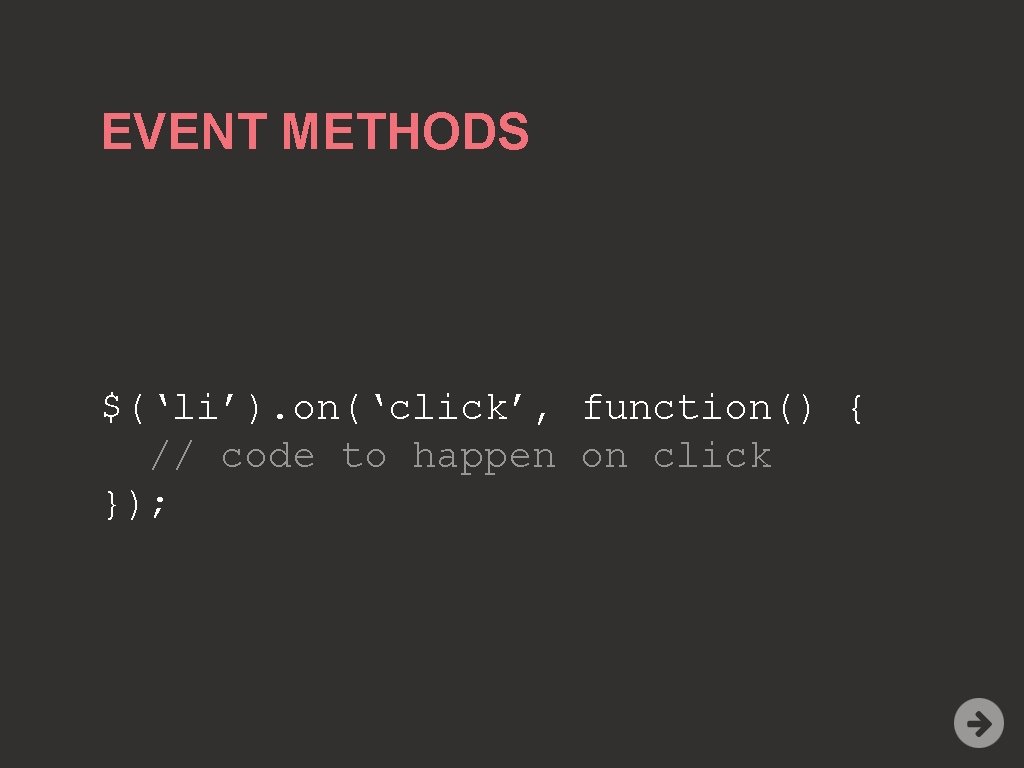
EVENT METHODS $(‘li’). on(‘click’, function() { // code to happen on click });
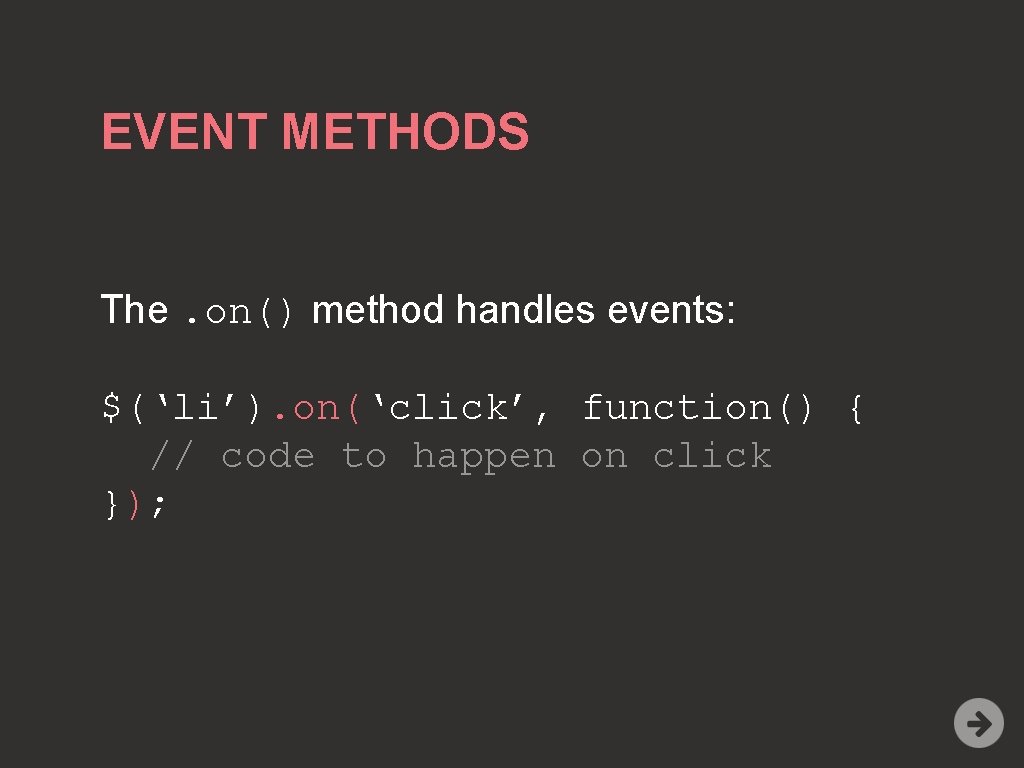
EVENT METHODS The. on() method handles events: $(‘li’). on(‘click’, function() { // code to happen on click });
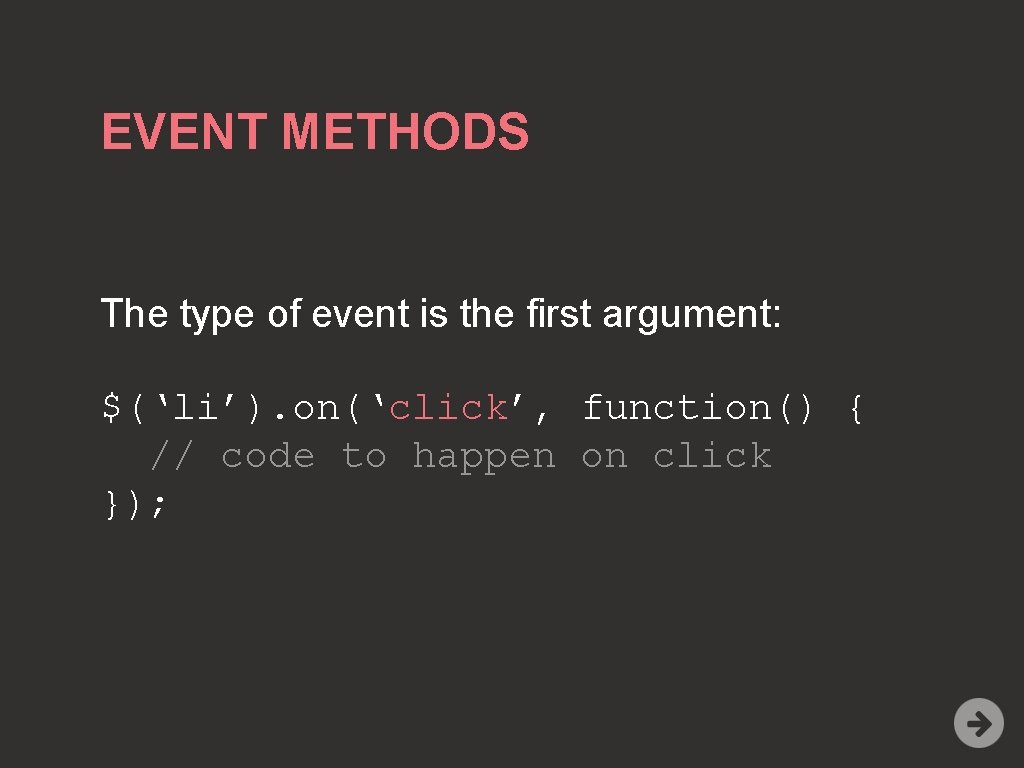
EVENT METHODS The type of event is the first argument: $(‘li’). on(‘click’, function() { // code to happen on click });
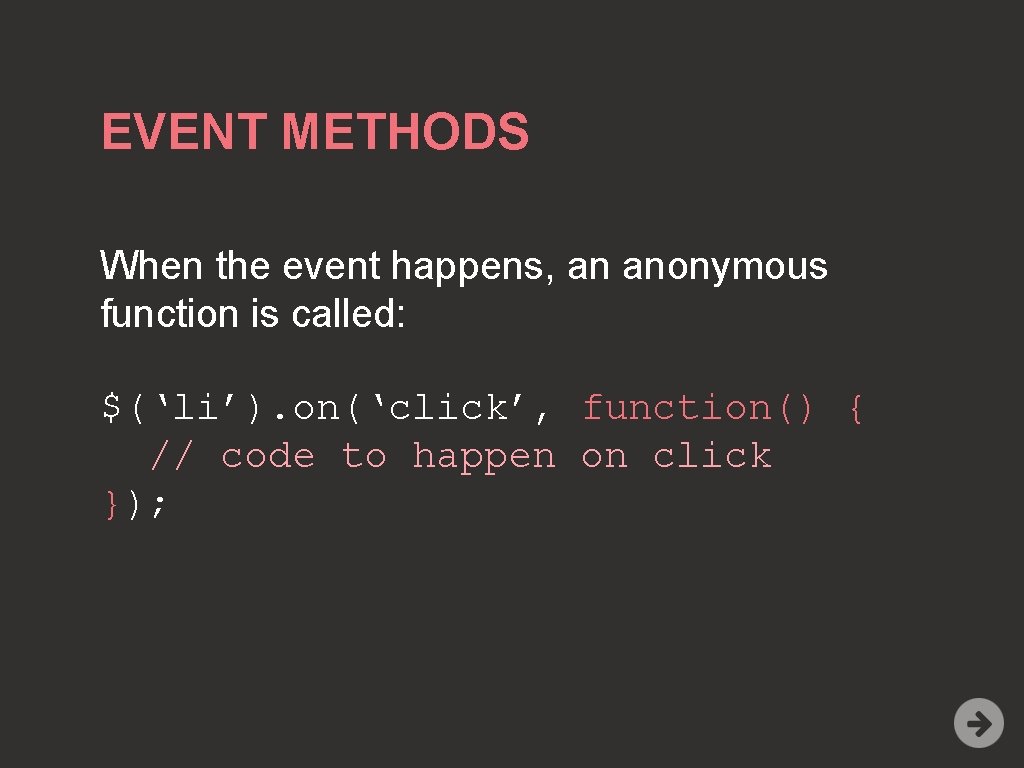
EVENT METHODS When the event happens, an anonymous function is called: $(‘li’). on(‘click’, function() { // code to happen on click });
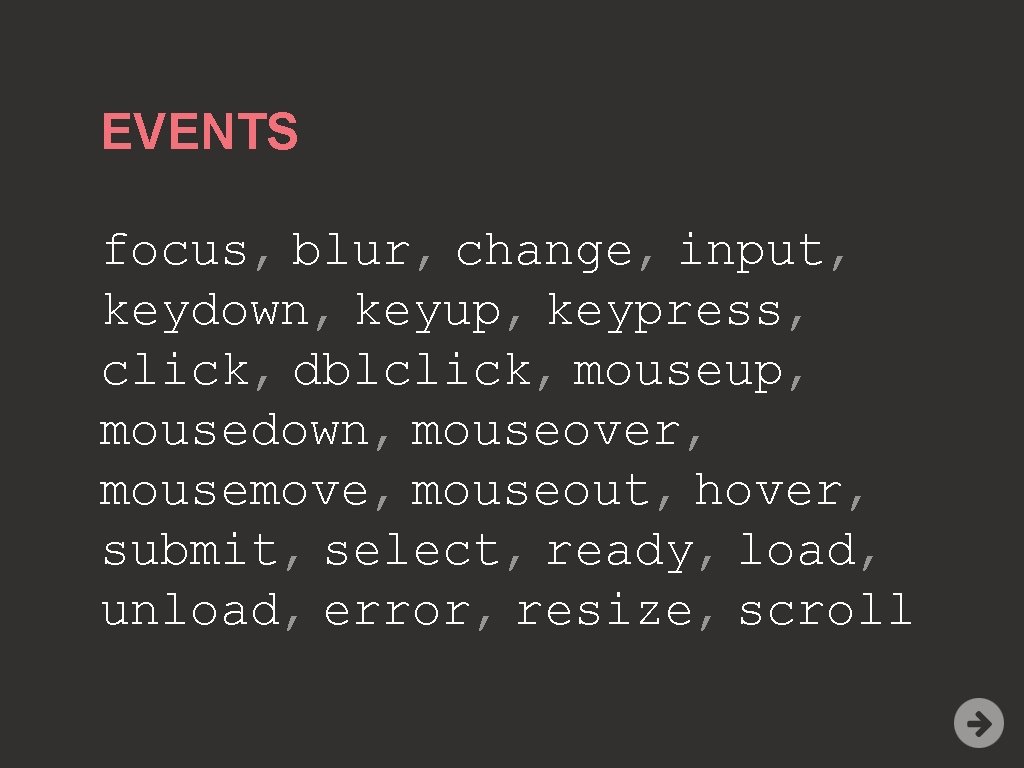
EVENTS focus, blur, change, input, keydown, keyup, keypress, click, dblclick, mouseup, mousedown, mouseover, mousemove, mouseout, hover, submit, select, ready, load, unload, error, resize, scroll
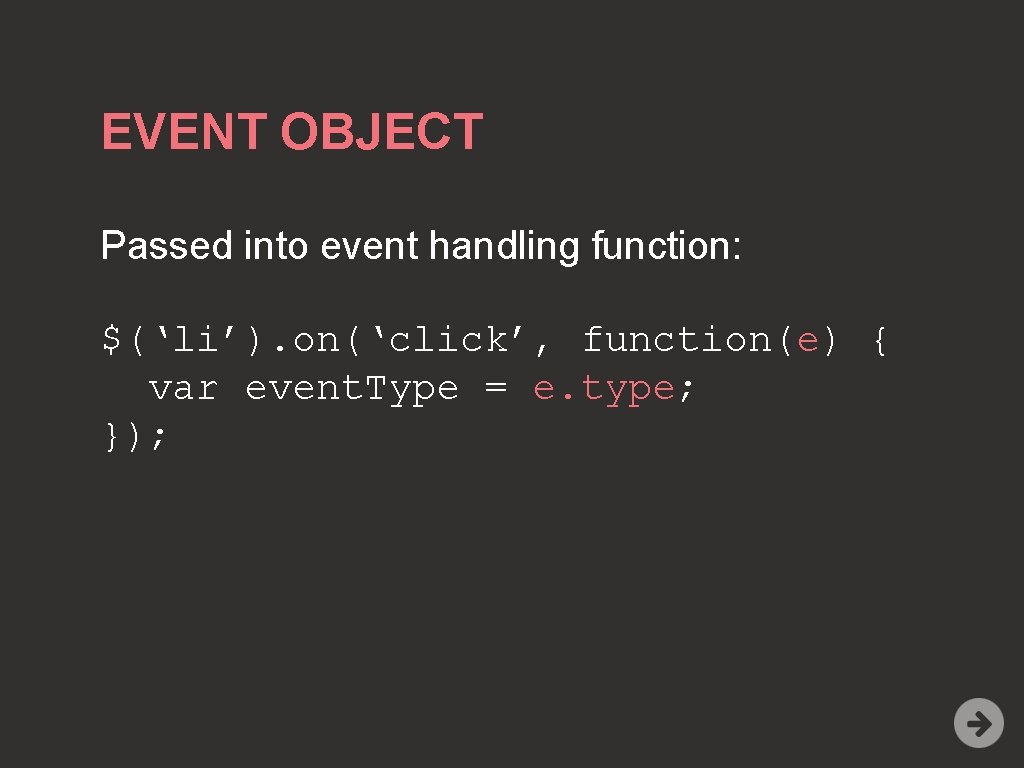
EVENT OBJECT Passed into event handling function: $(‘li’). on(‘click’, function(e) { var event. Type = e. type; });
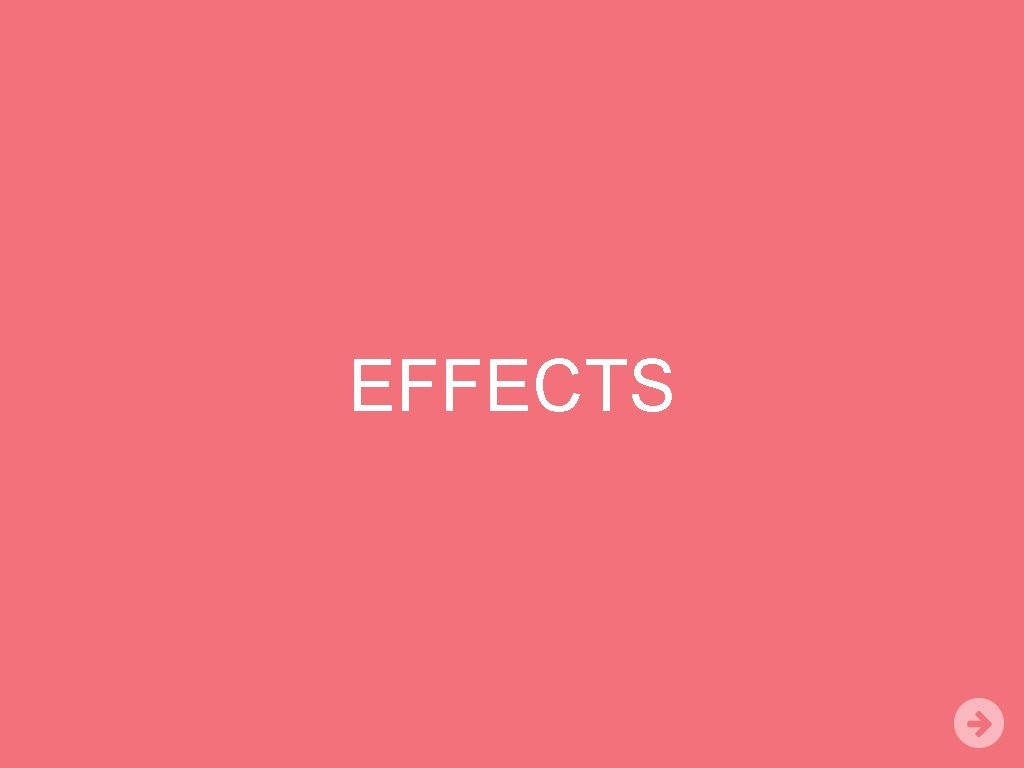
EFFECTS
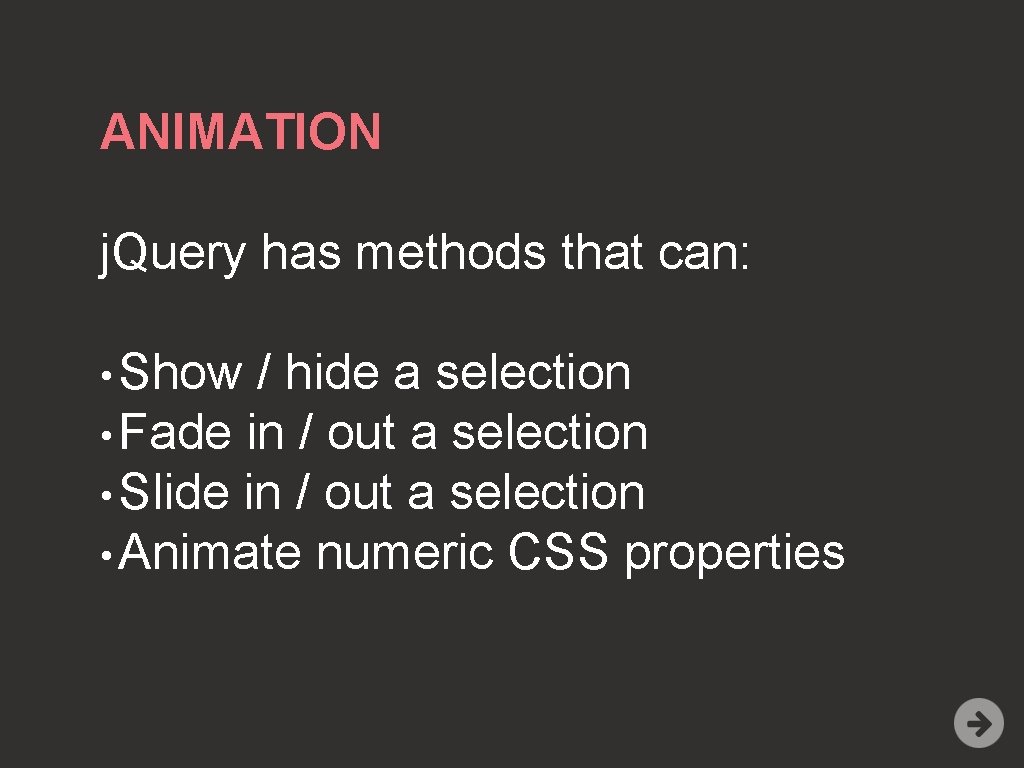
ANIMATION j. Query has methods that can: • Show / hide a selection • Fade in / out a selection • Slide in / out a selection • Animate numeric CSS properties
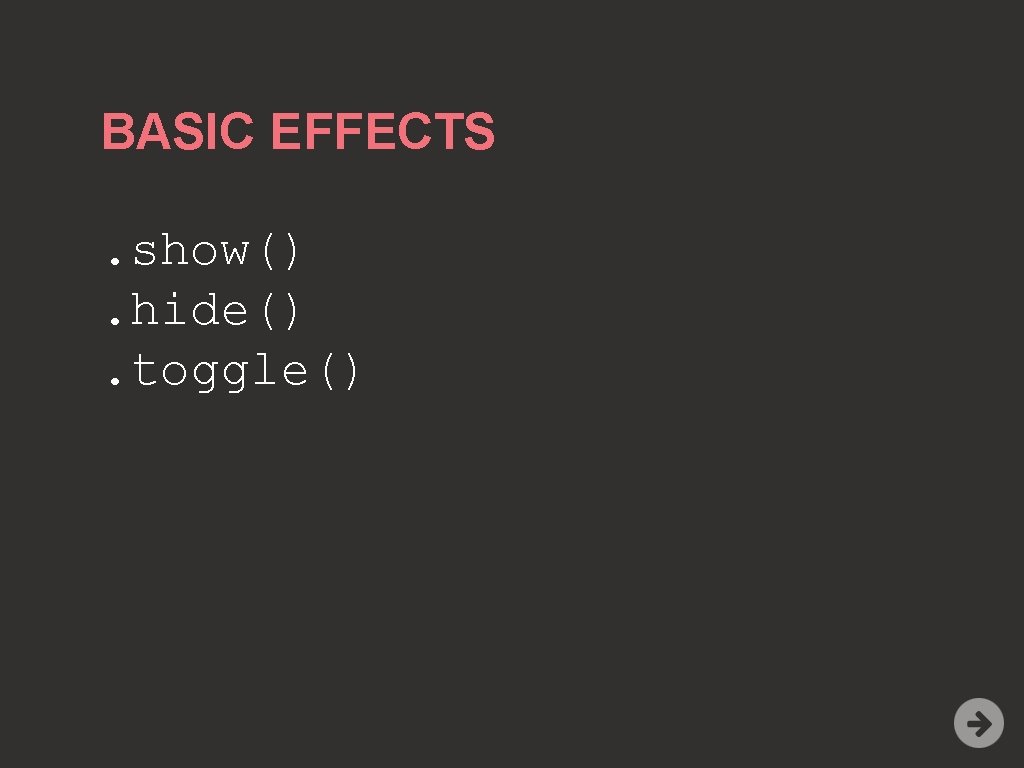
BASIC EFFECTS. show(). hide(). toggle()
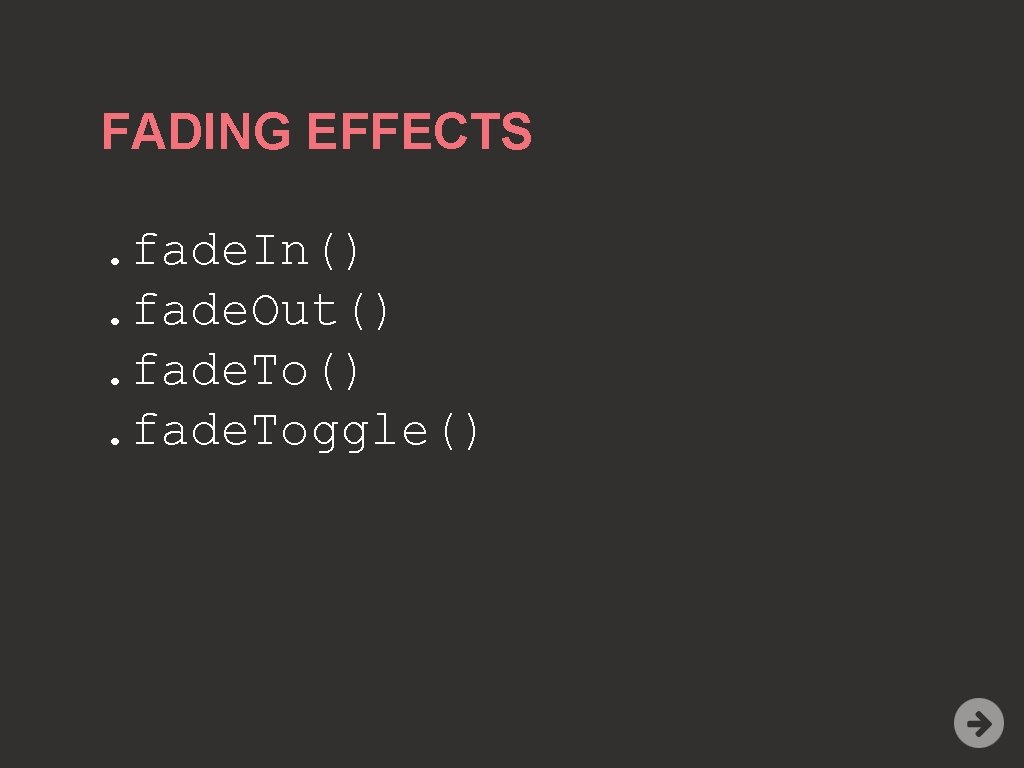
FADING EFFECTS. fade. In(). fade. Out(). fade. Toggle()
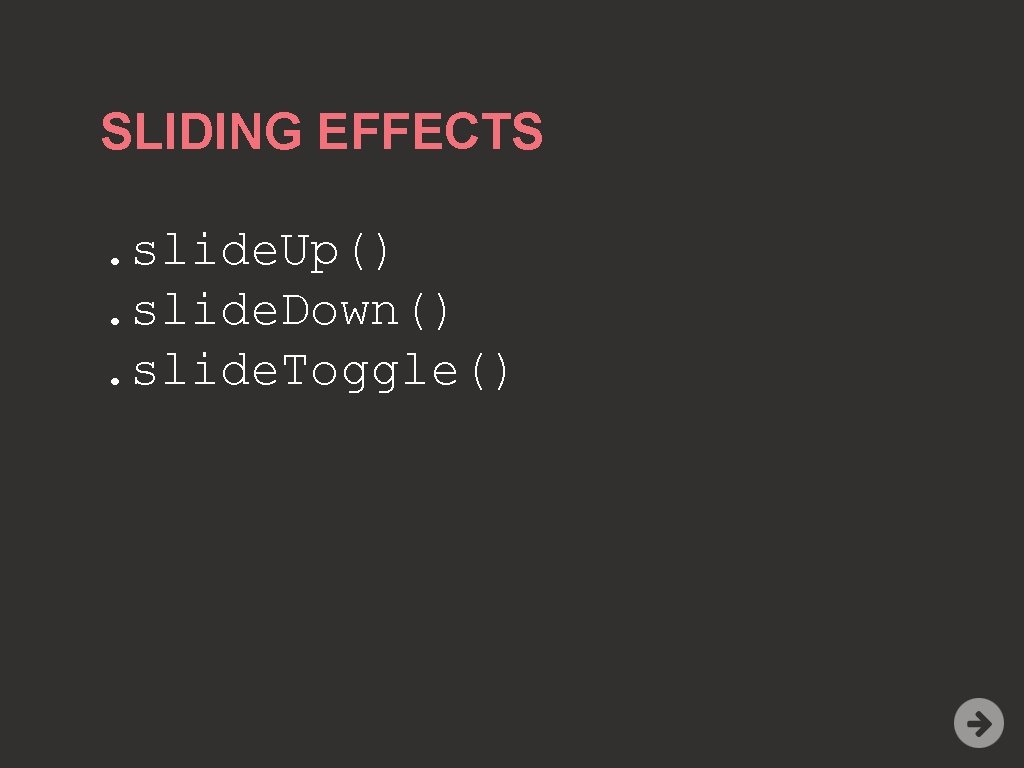
SLIDING EFFECTS. slide. Up(). slide. Down(). slide. Toggle()
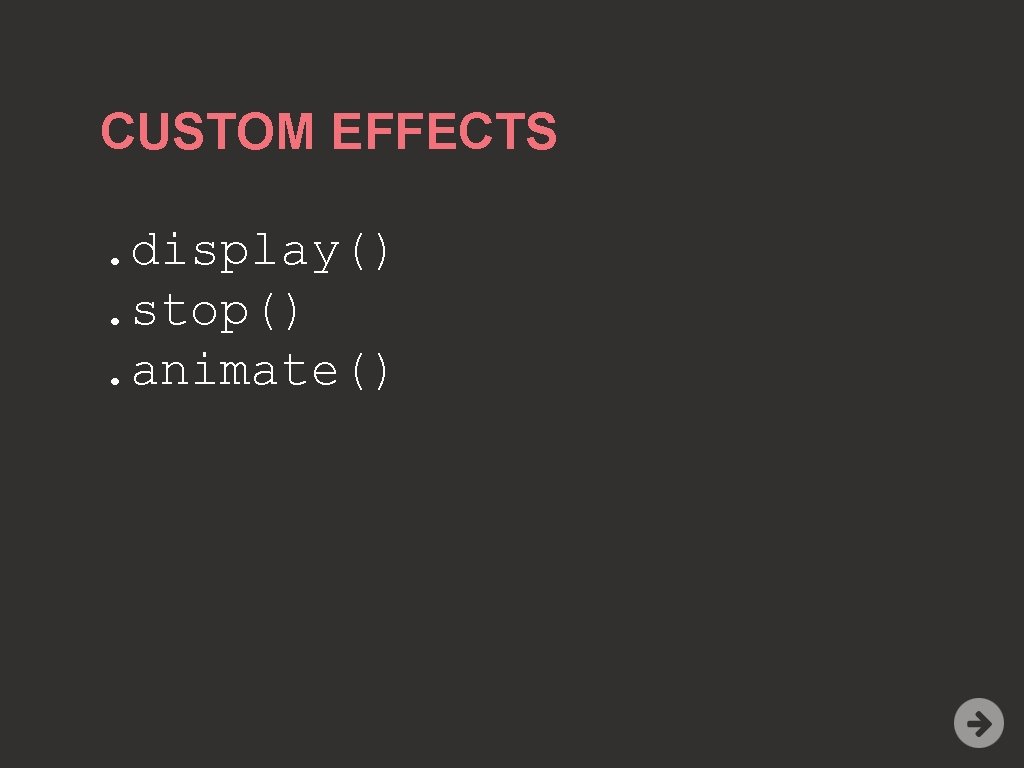
CUSTOM EFFECTS. display(). stop(). animate()
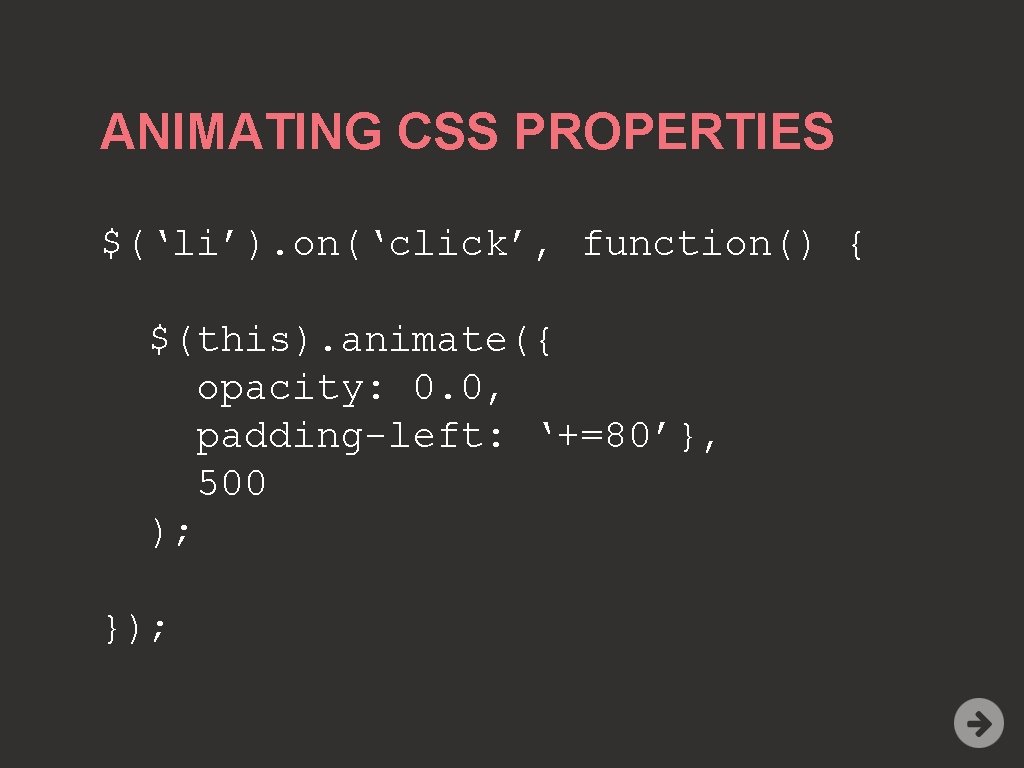
ANIMATING CSS PROPERTIES $(‘li’). on(‘click’, function() { $(this). animate({ opacity: 0. 0, padding-left: ‘+=80’}, 500 ); });
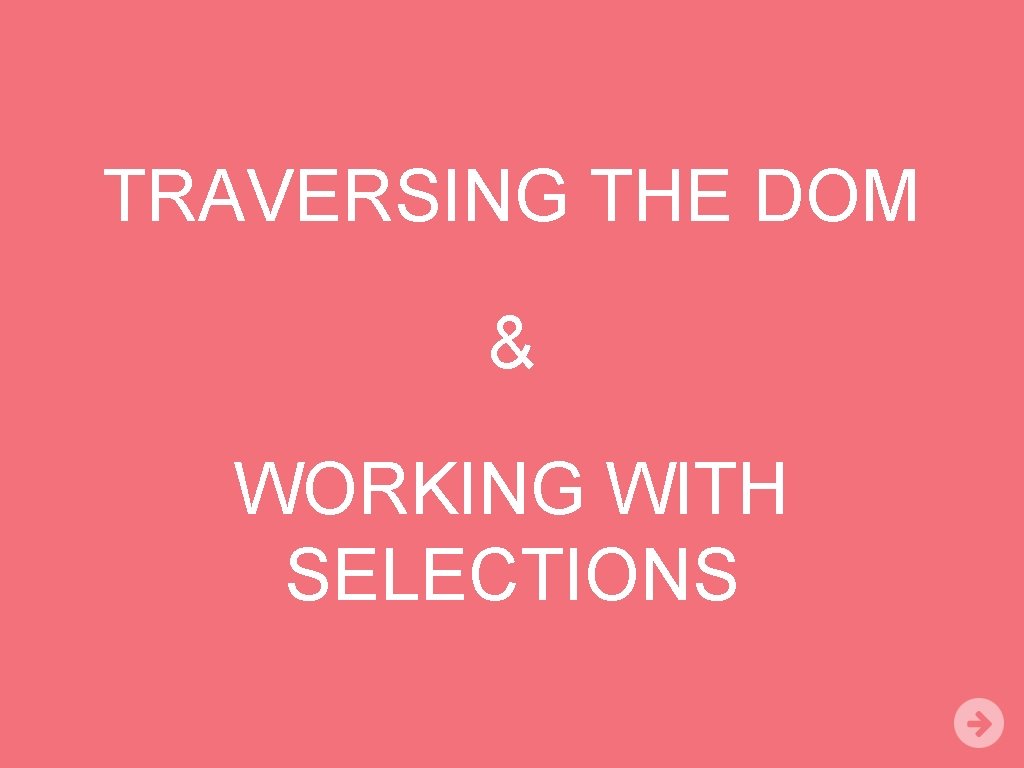
TRAVERSING THE DOM & WORKING WITH SELECTIONS
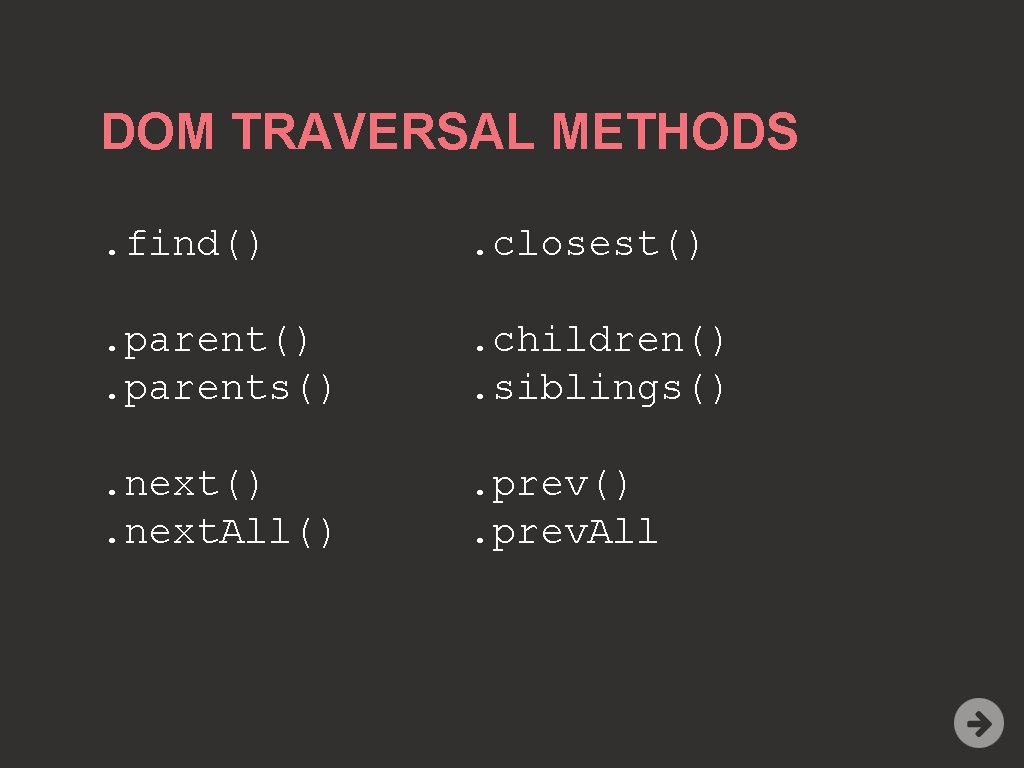
DOM TRAVERSAL METHODS. find() . closest() . parent(). parents() . children(). siblings() . next(). next. All() . prev(). prev. All
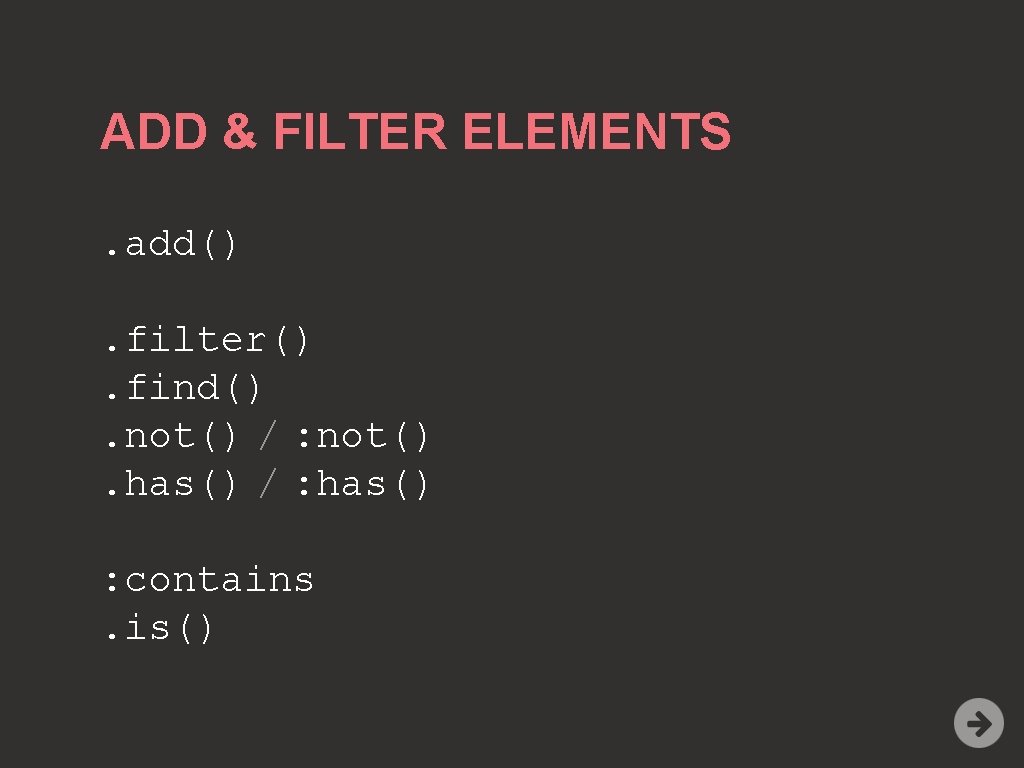
ADD & FILTER ELEMENTS. add(). filter(). find(). not() / : not(). has() / : has() : contains. is()
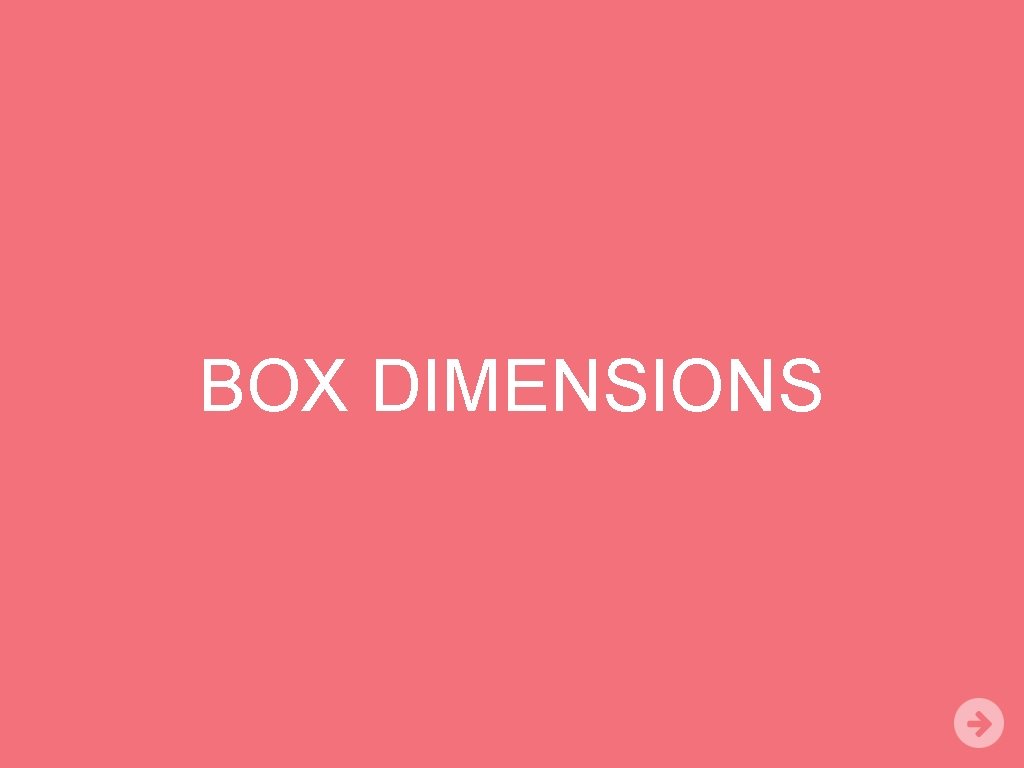
BOX DIMENSIONS
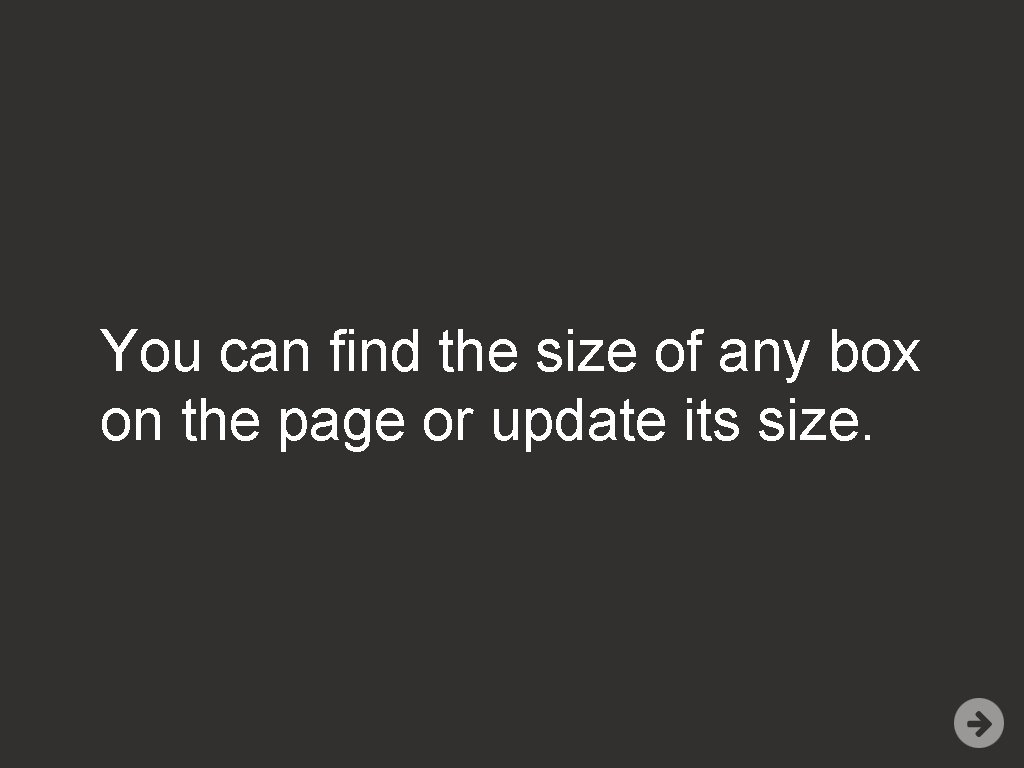
You can find the size of any box on the page or update its size.
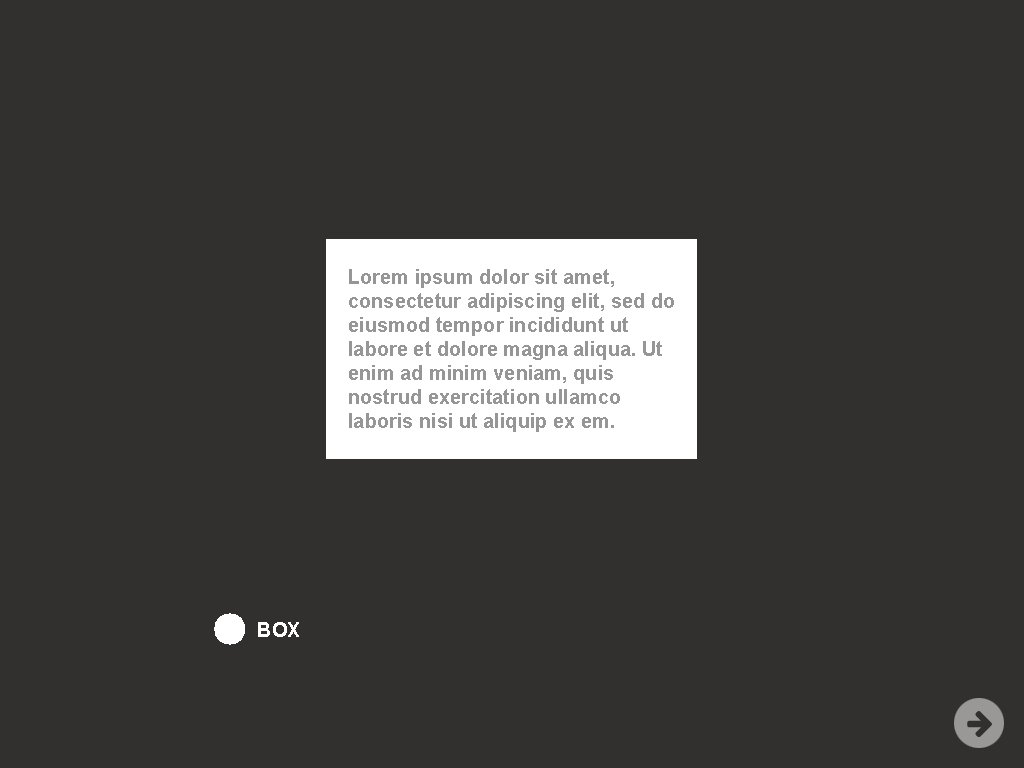
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex em. BOX
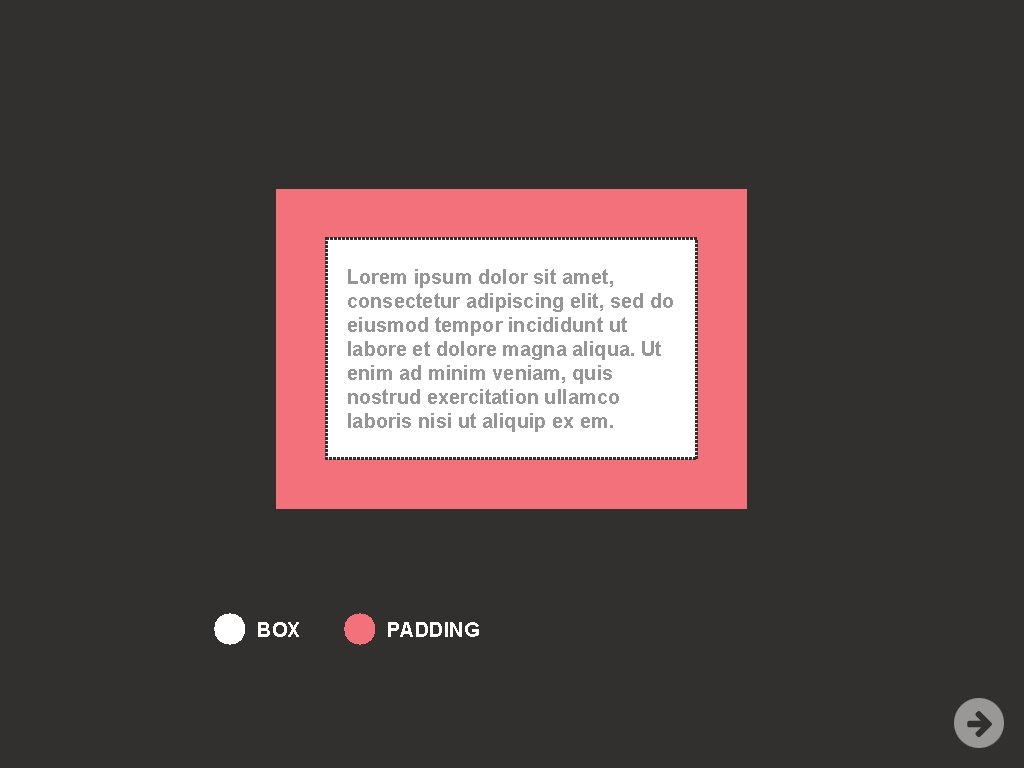
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex em. BOX PADDING
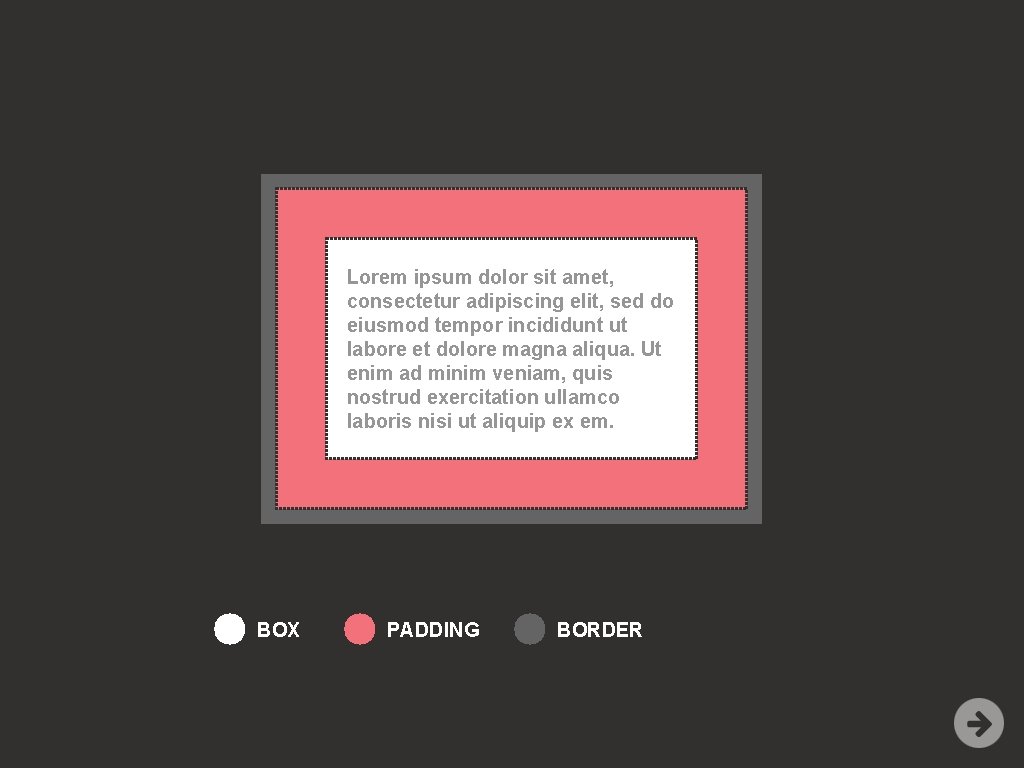
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex em. BOX PADDING BORDER
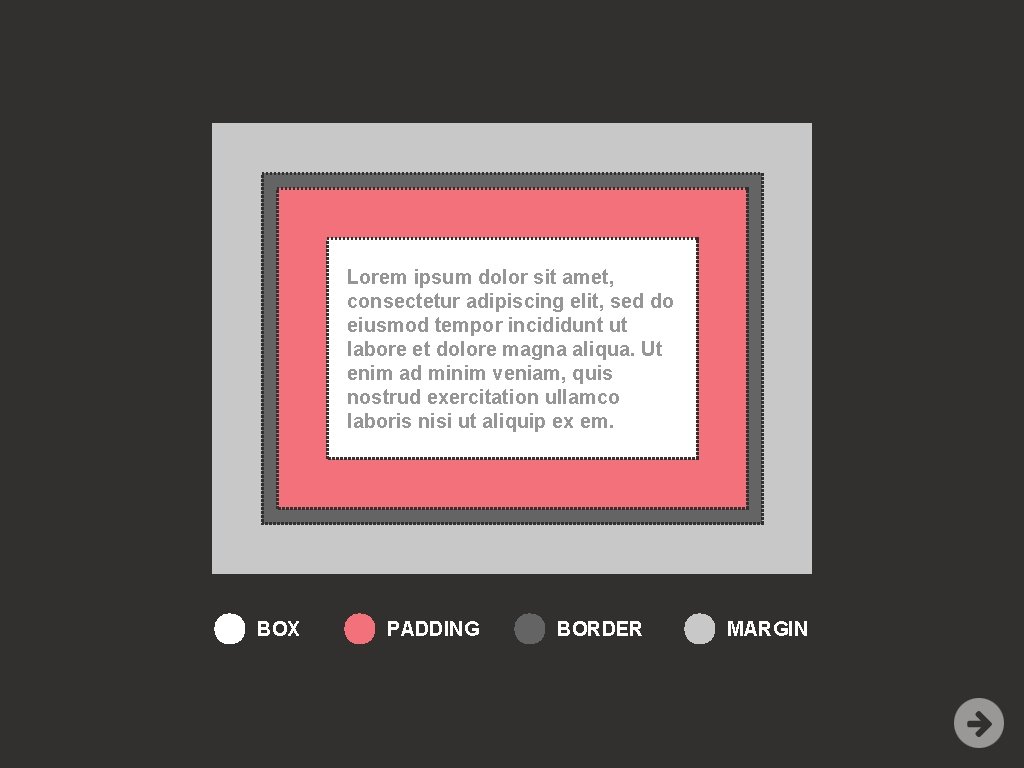
Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex em. BOX PADDING BORDER MARGIN
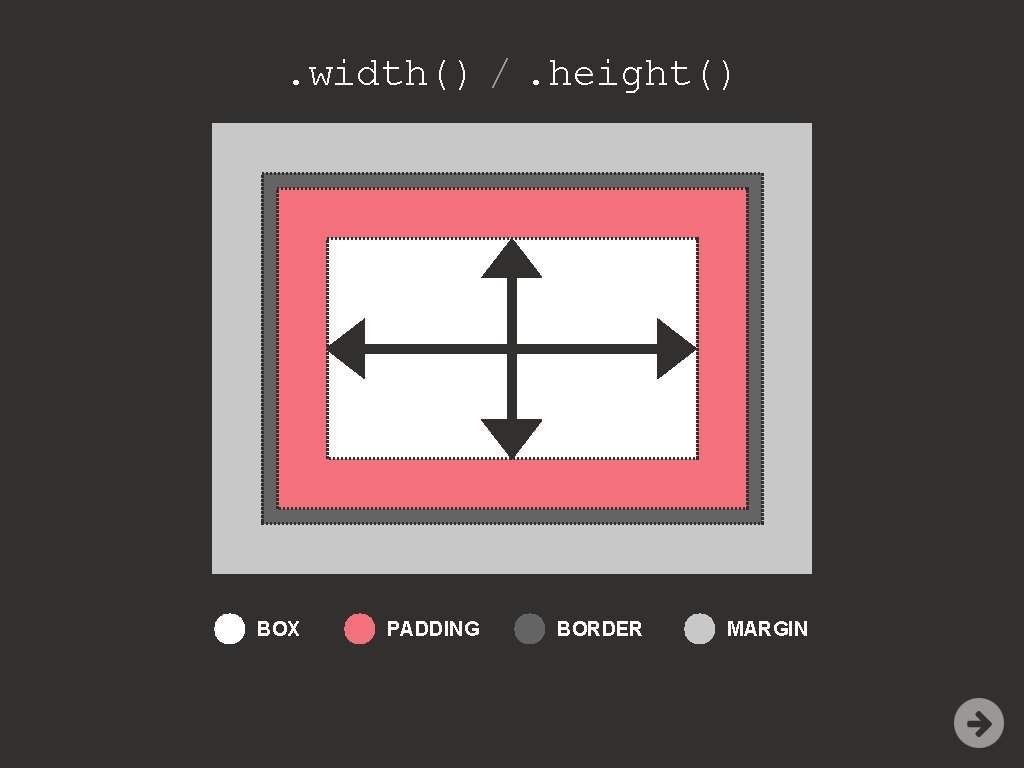
. width() /. height() BOX PADDING BORDER MARGIN
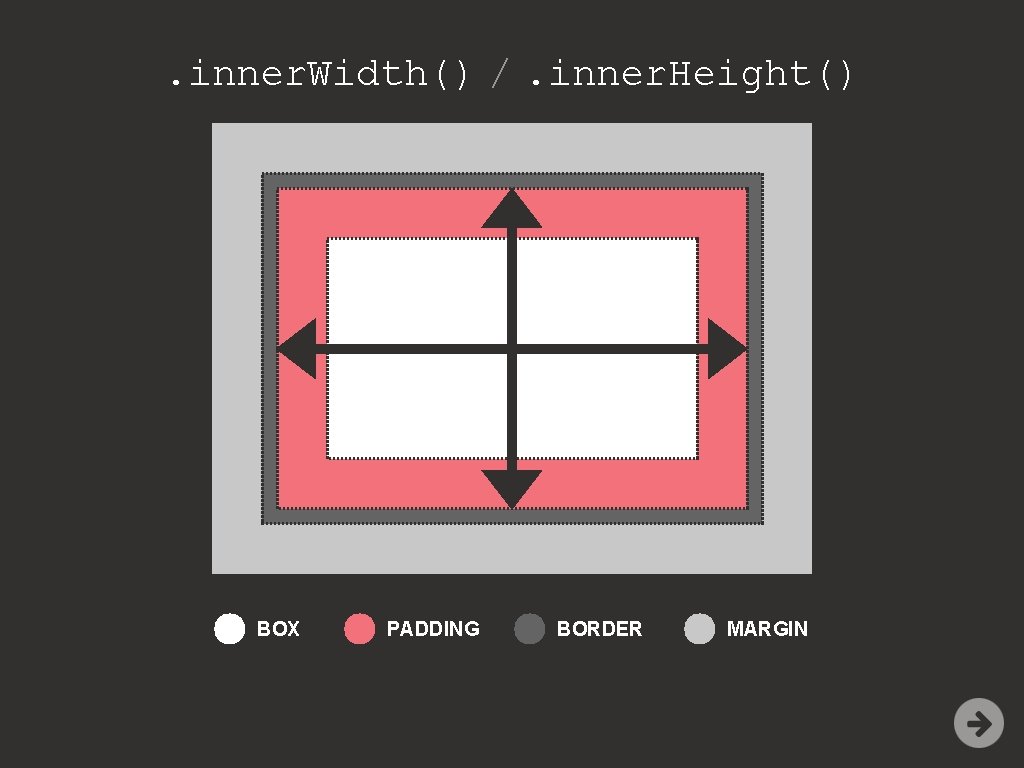
. inner. Width() /. inner. Height() BOX PADDING BORDER MARGIN
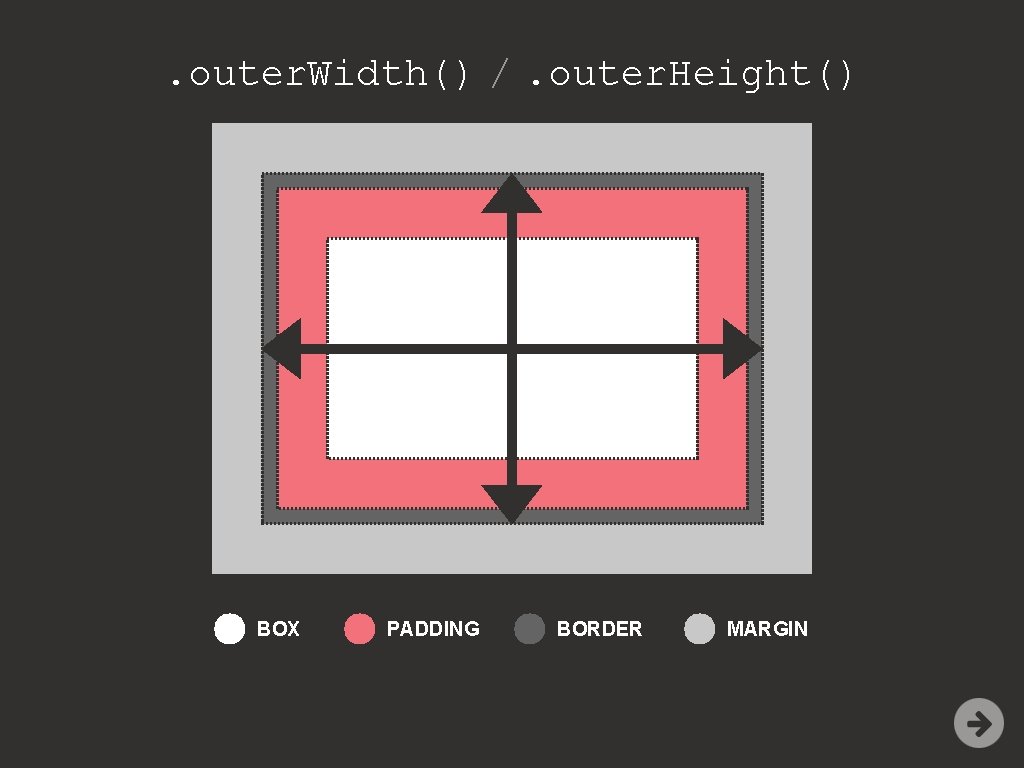
. outer. Width() /. outer. Height() BOX PADDING BORDER MARGIN
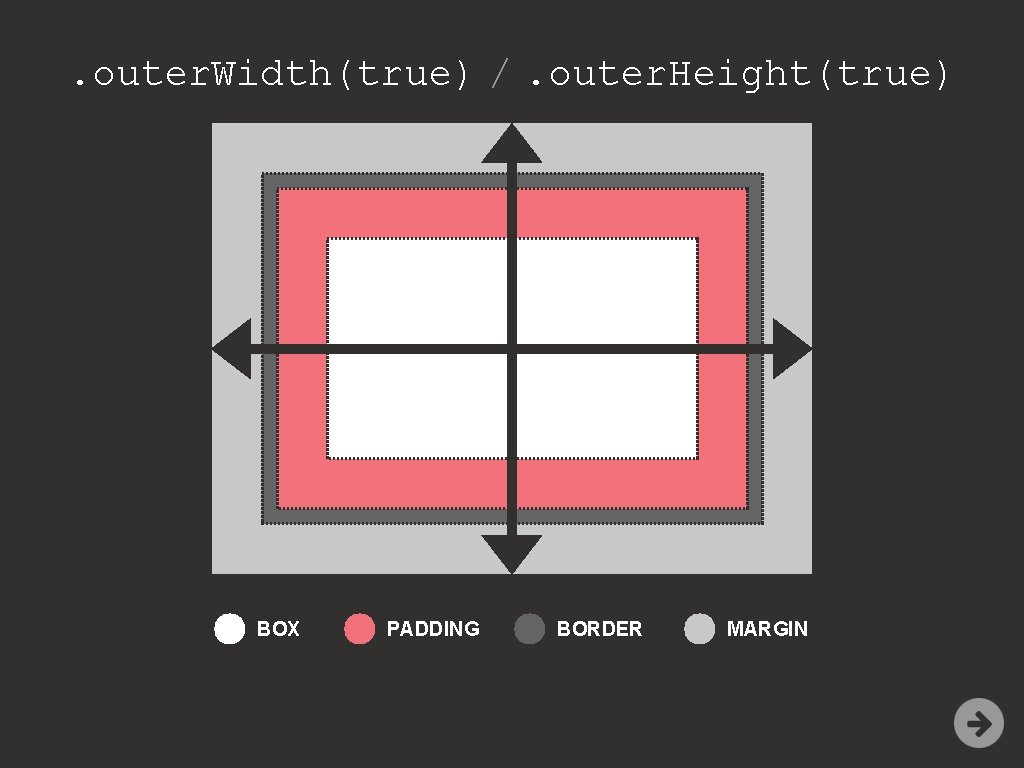
. outer. Width(true) /. outer. Height(true) BOX PADDING BORDER MARGIN
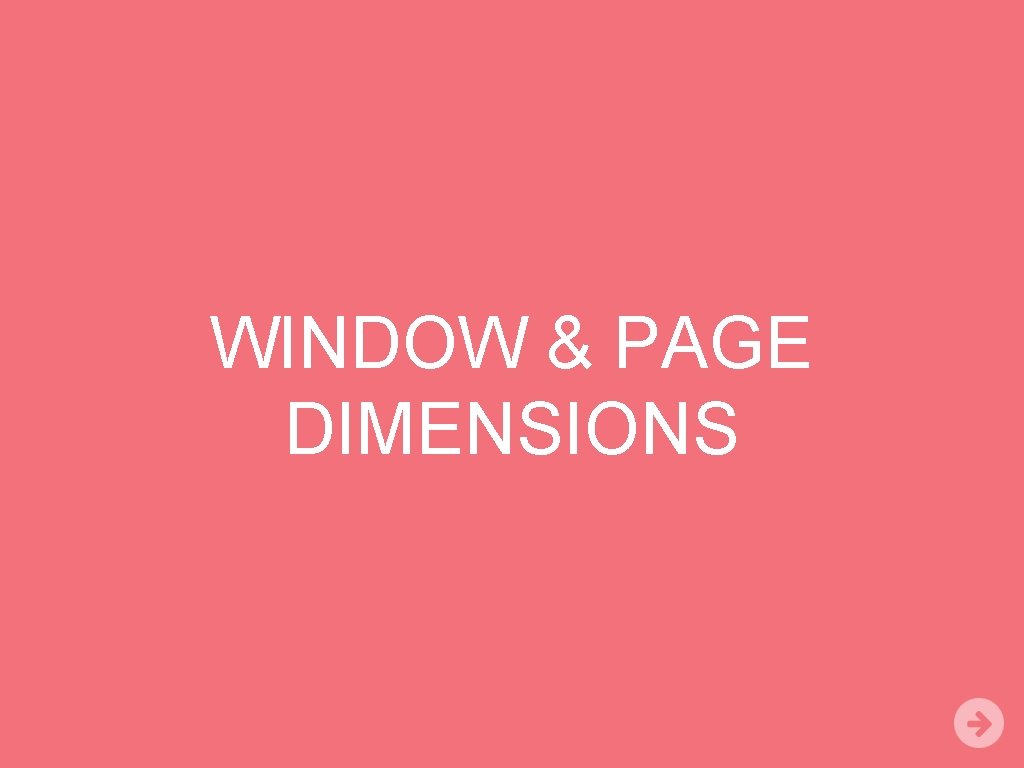
WINDOW & PAGE DIMENSIONS
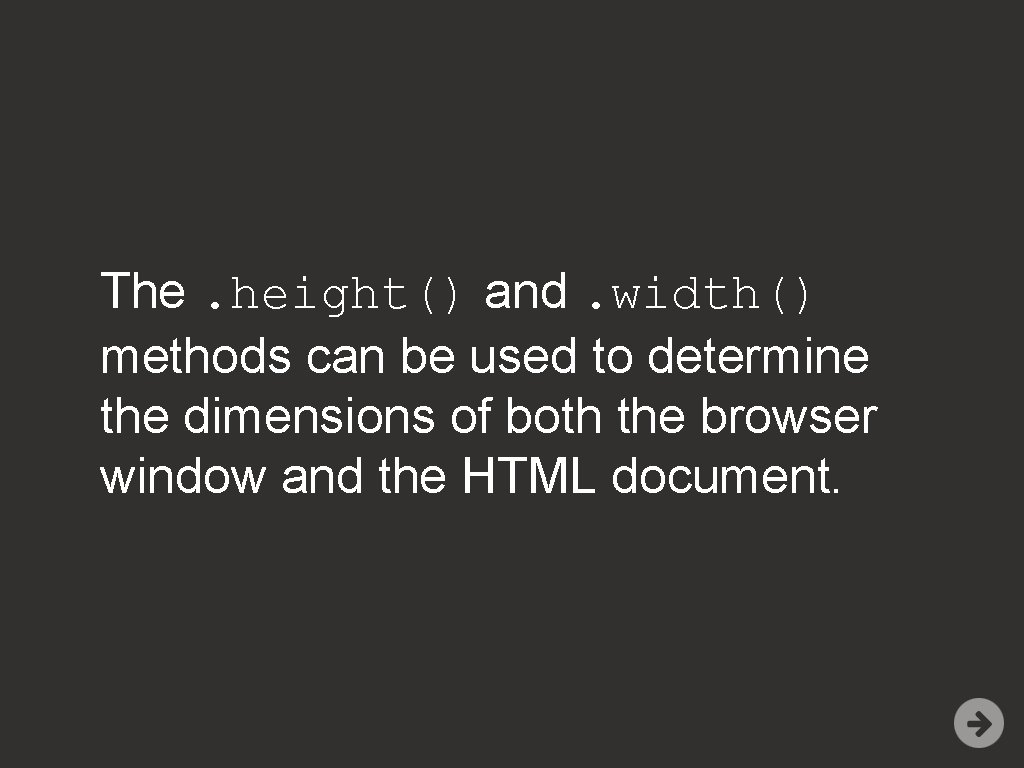
The. height() and. width() methods can be used to determine the dimensions of both the browser window and the HTML document.
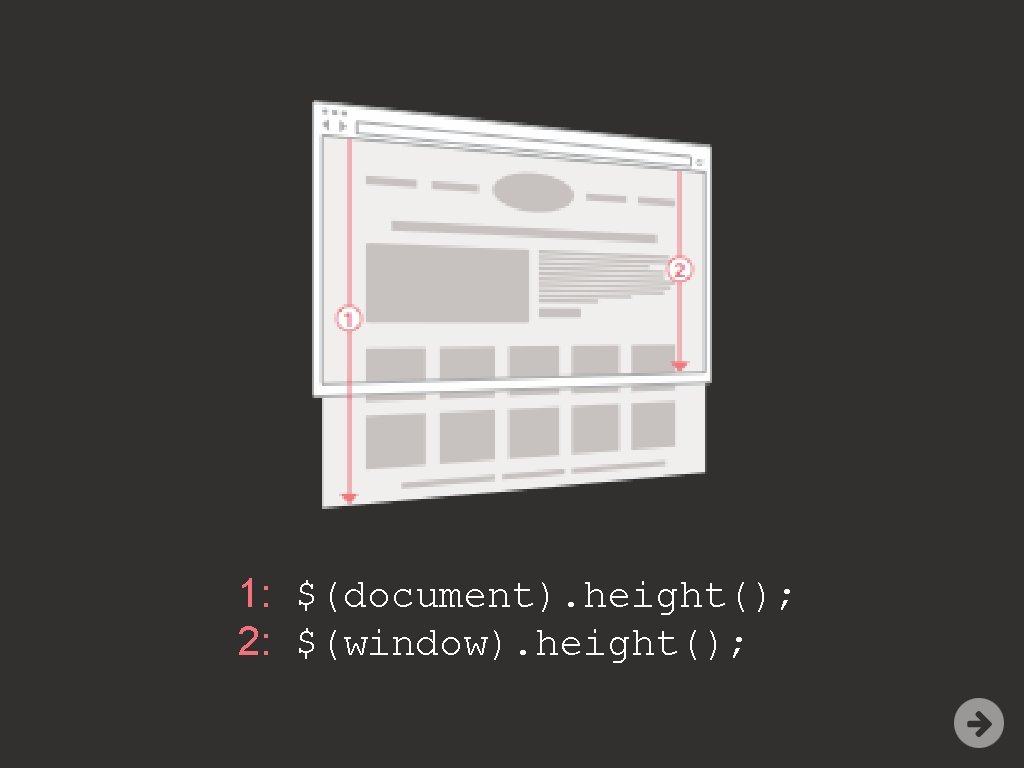
1: $(document). height(); 2: $(window). height();
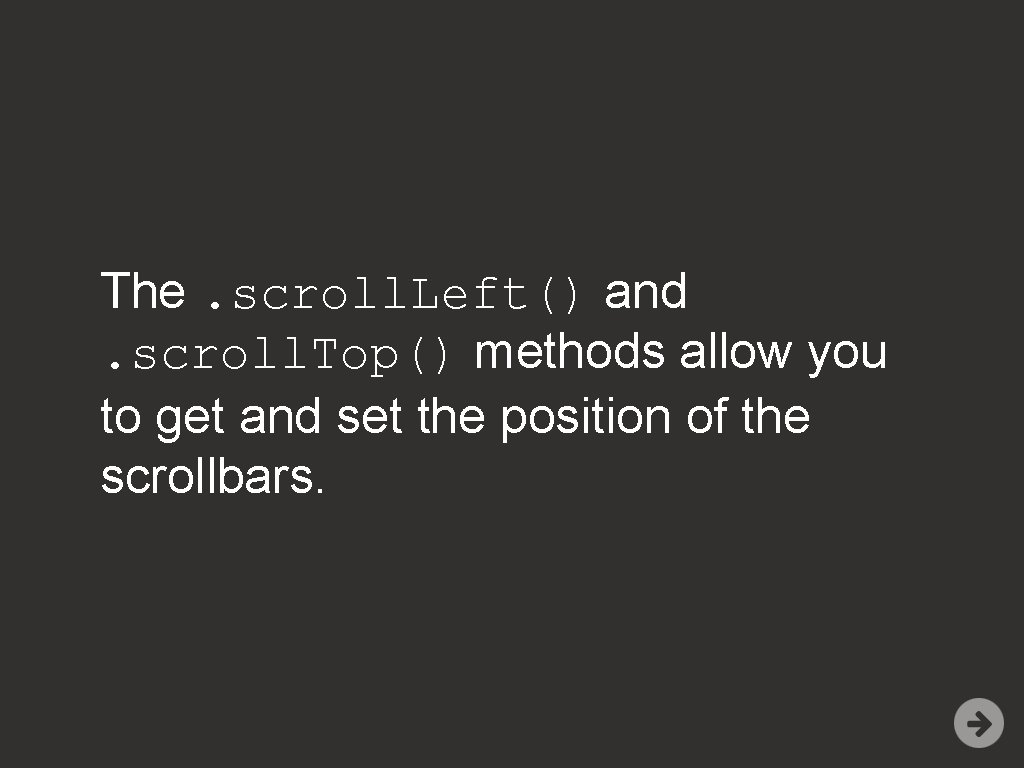
The. scroll. Left() and. scroll. Top() methods allow you to get and set the position of the scrollbars.
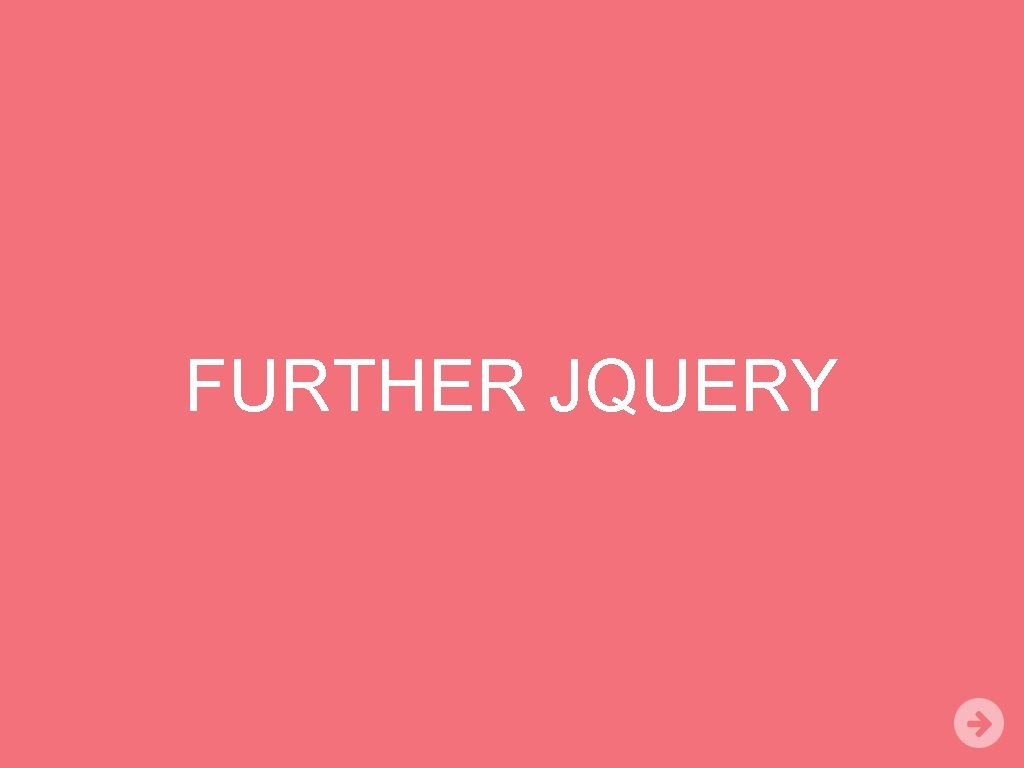
FURTHER JQUERY
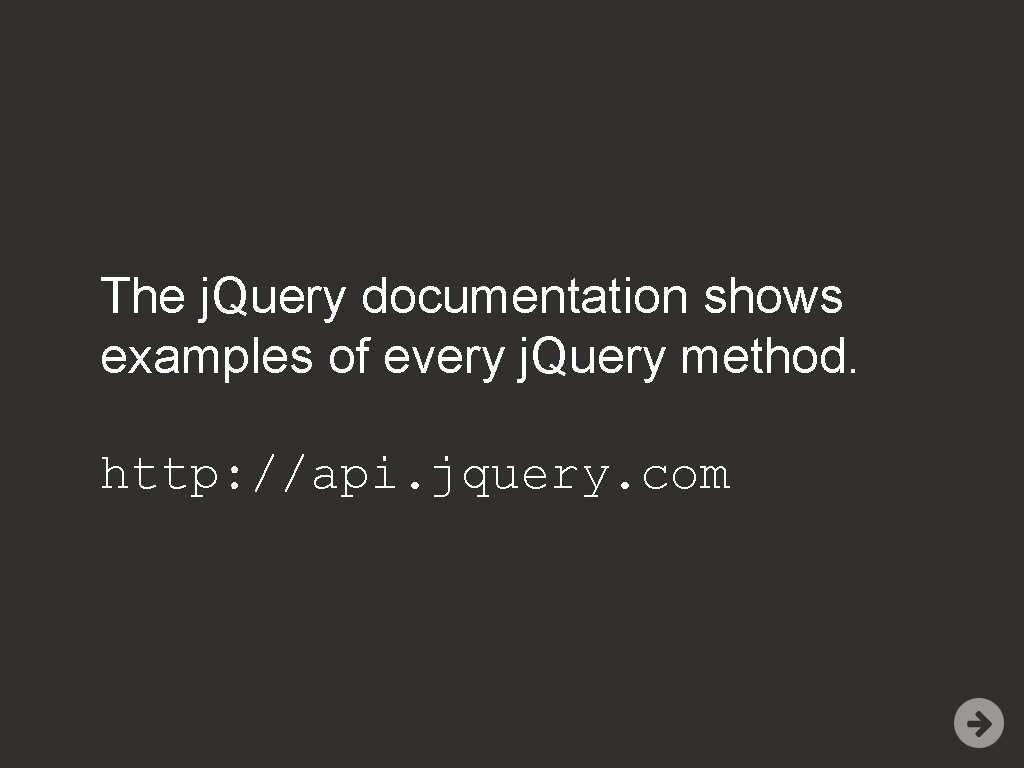
The j. Query documentation shows examples of every j. Query method. http: //api. jquery. com
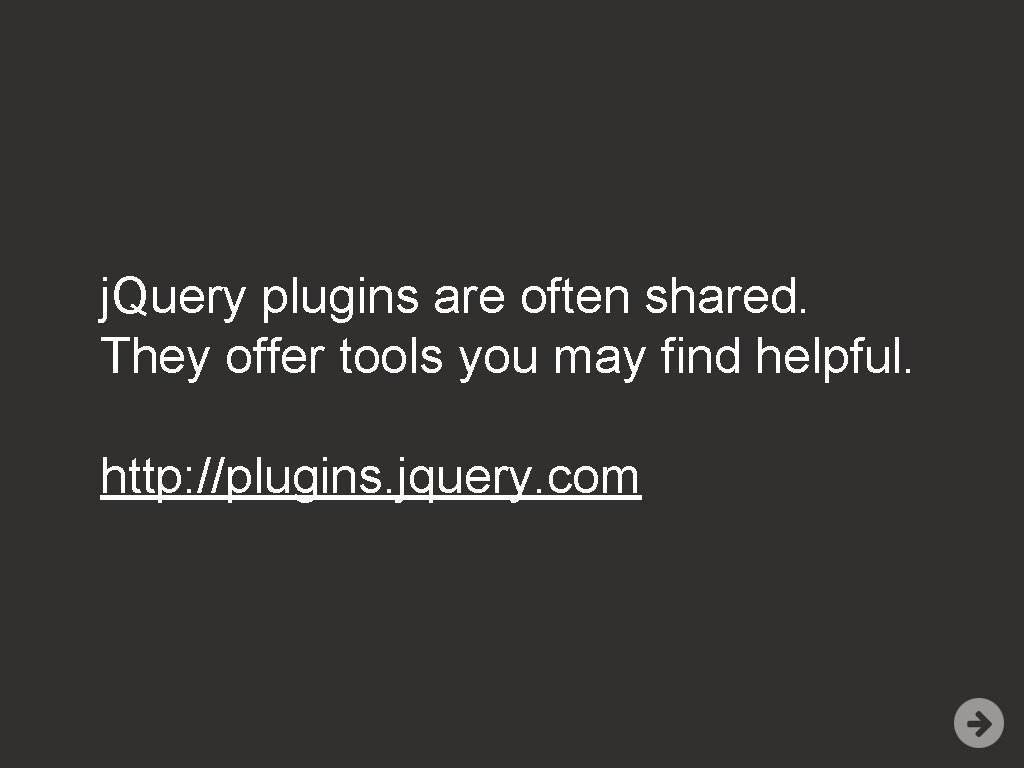
j. Query plugins are often shared. They offer tools you may find helpful. http: //plugins. jquery. com
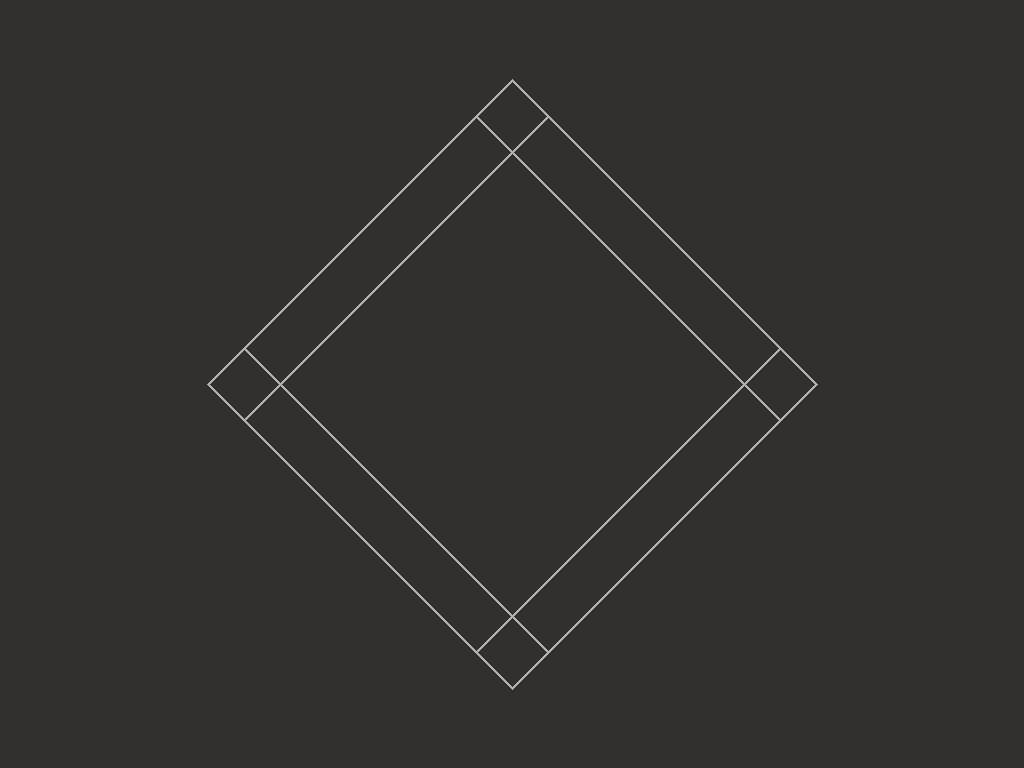
Iterative query vs recursive query
Query tree and query graph
Query tree and query graph
J
Ajax sqlite
W 3 schools
Prepend jquery
Jquery functionality
Infolight
Greensock parallax
Jquery mobile
Ajax async
Jquery optimization selectors
My structured query language
Oql query examples
Suspicious dns query
Starnet query model
Aggregate operation in relational algebra
Sfw query
Rrc wellbore query
Eurostat query builder
Pengertian query
Query adalah
Query optimizer sql server
Course selection ntust
Ripe database query service
Query tools in data mining
Relational query languages in dbms
Texas railroad commission completions query
Rrc wellbore query
Google data visualization api
Steps in query processing
Query decomposition in dbms
Sql
Rakshatpa neft form
Moloch query examples
Query operations in information retrieval
Truyvn
Jmp query builder
Query optimization steps
Wild card queries in information retrieval
Simple text query
Supplier query form unilever
Query management process
Enum query ims
Recursive and iterative query
Recursive and iterative query
Azure cosmos db query cheat sheet
Steps in query processing
Measures of query cost in dbms
Research problems in data warehousing
Convert natural language to sql query
Recursive and iterative query
Introduction to structured query language (sql)
Formal relational query languages
Unary and binary tree in a query
Application layer
Objectives of query processing
Building an elastic query engine on disaggregated storage
Steps of query processing
Analyzing plan diagrams of database query optimizers
Introduction to structured query language (sql)
Montana water rights query
Steps in query processing
Northwind database query exercises
Difference between import and direct query power bi
Cyberquery help
Well log query
An attacker injects the following sql query blah
Sedref query
Similar image search
Clinical validation query example
Optimasi query
Range query elasticsearch
Mimer sql
Cfscript loop
Language integrated query developer
Query engine
Automated analysis in hci
Sqlstress
Query evaluation engine
Brian query 1
Inside the sql server query optimizer
Common query language
Whoisactive sql query
Layers of query processing
Dasl query
Total query trong access