j Query j Query JQuery is a lightweight
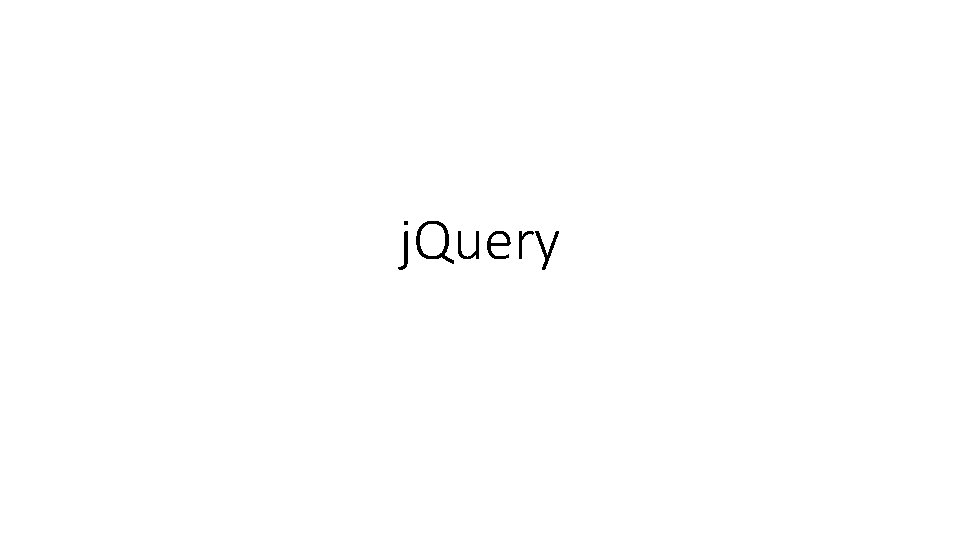
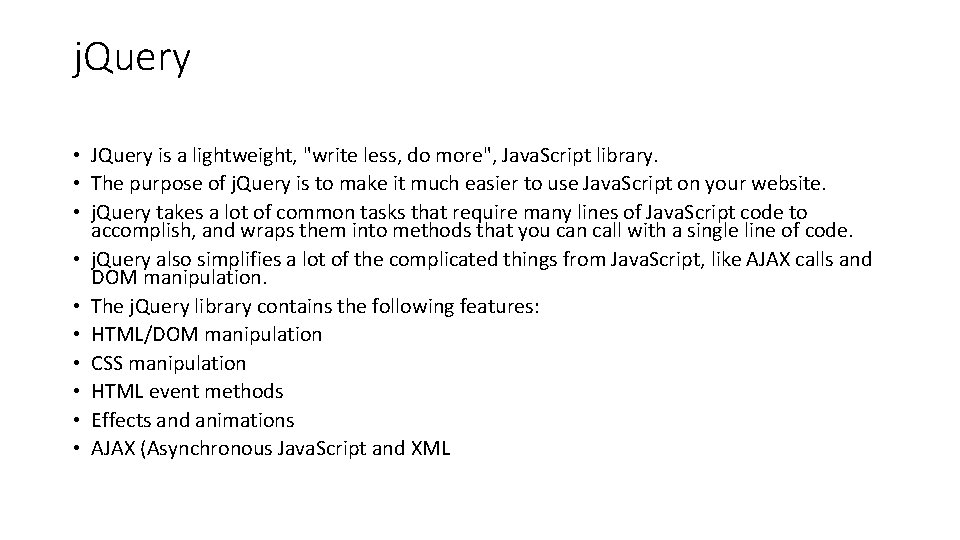
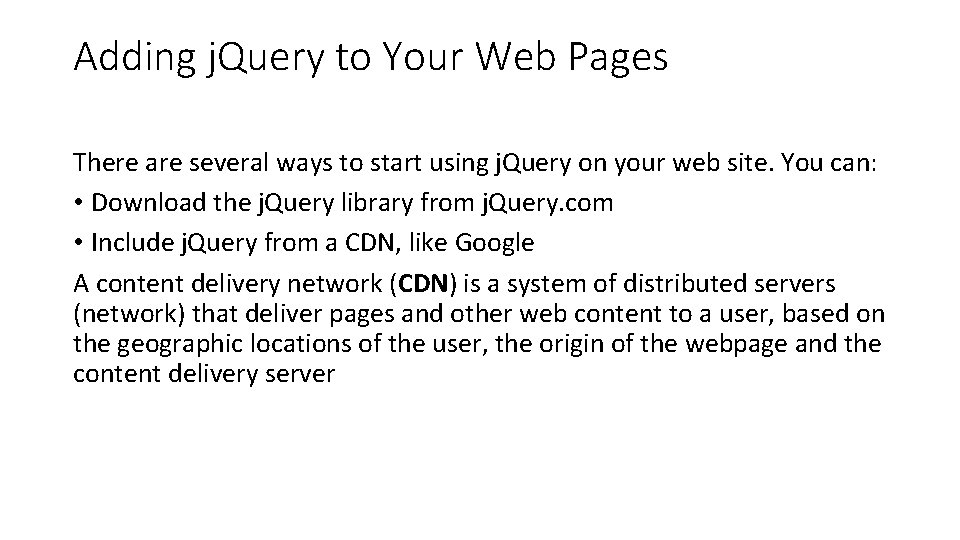
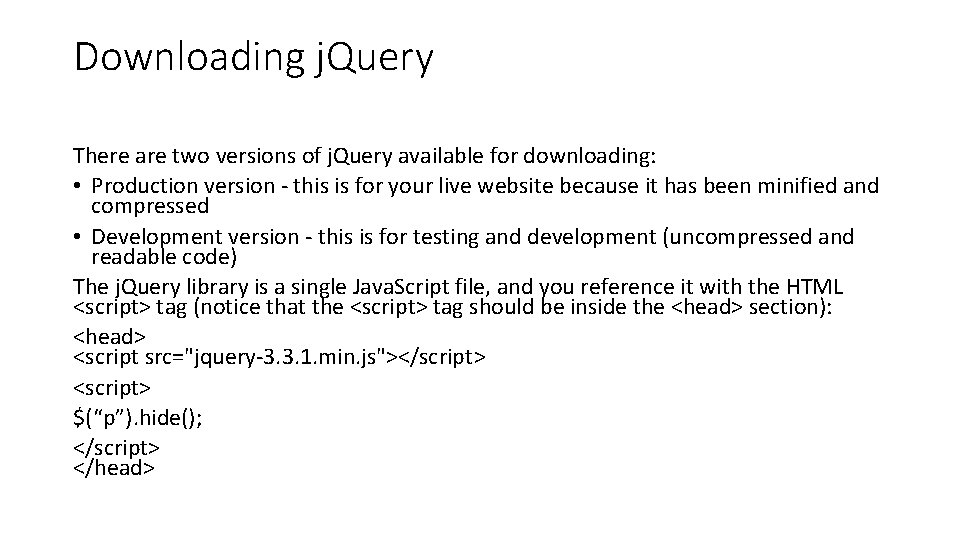
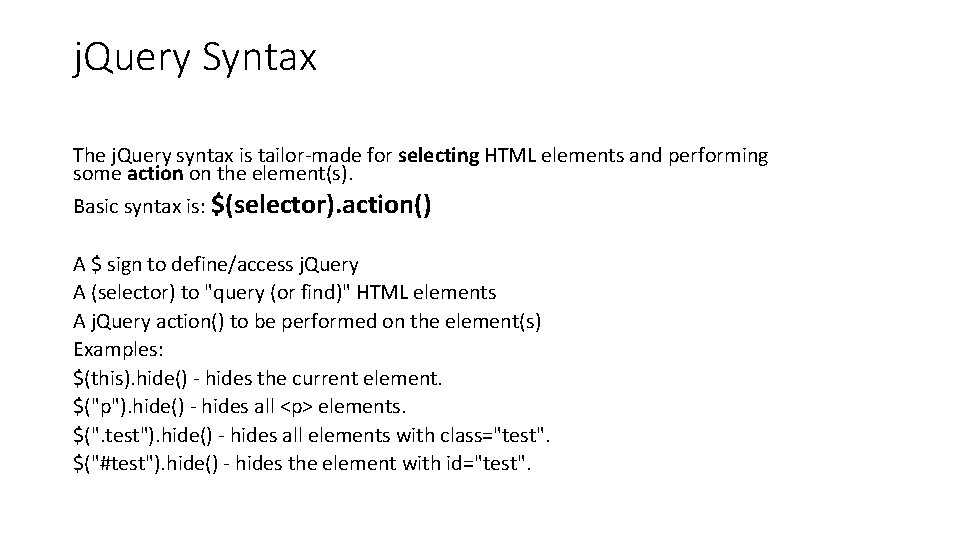
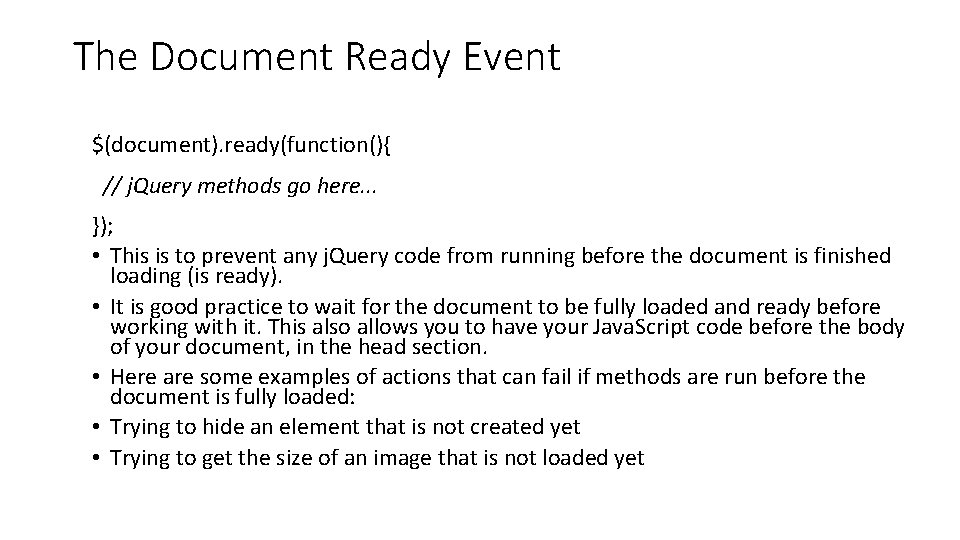
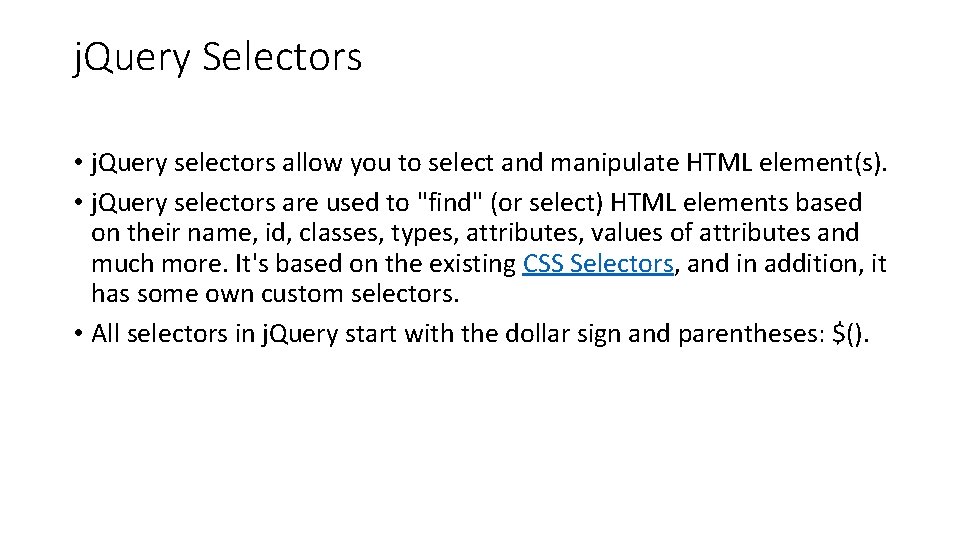
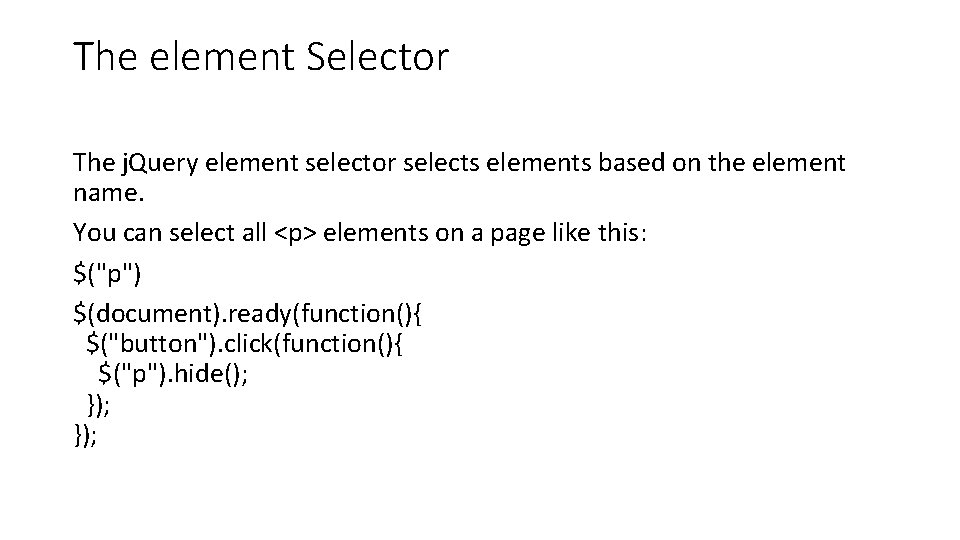
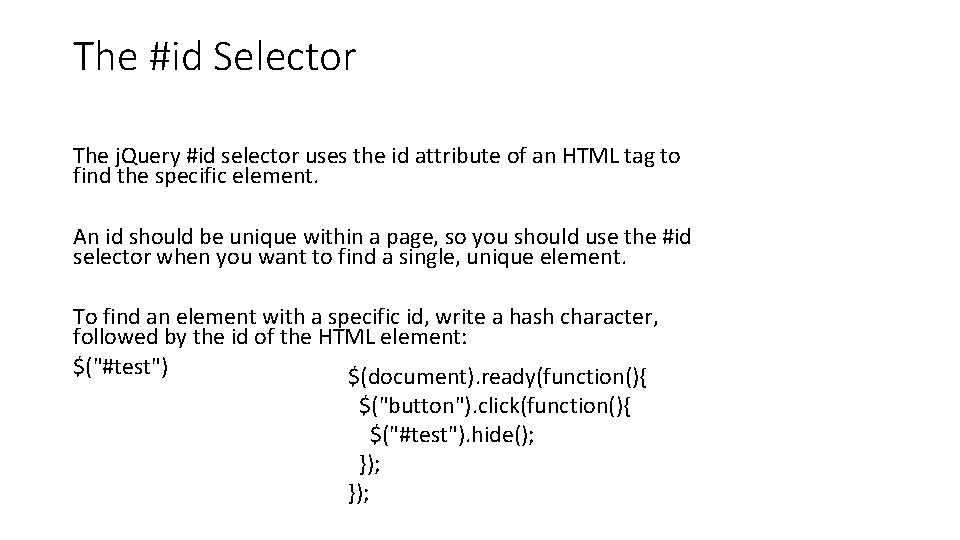
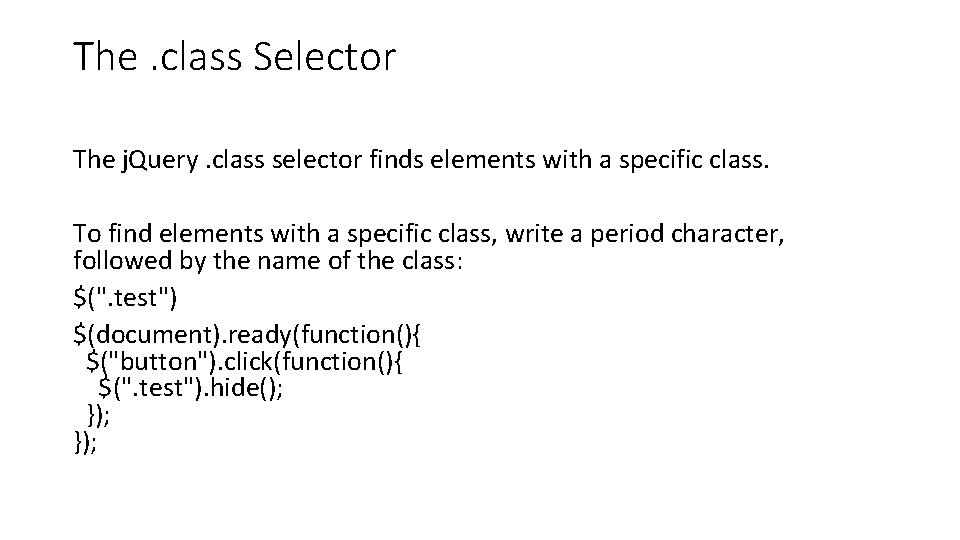
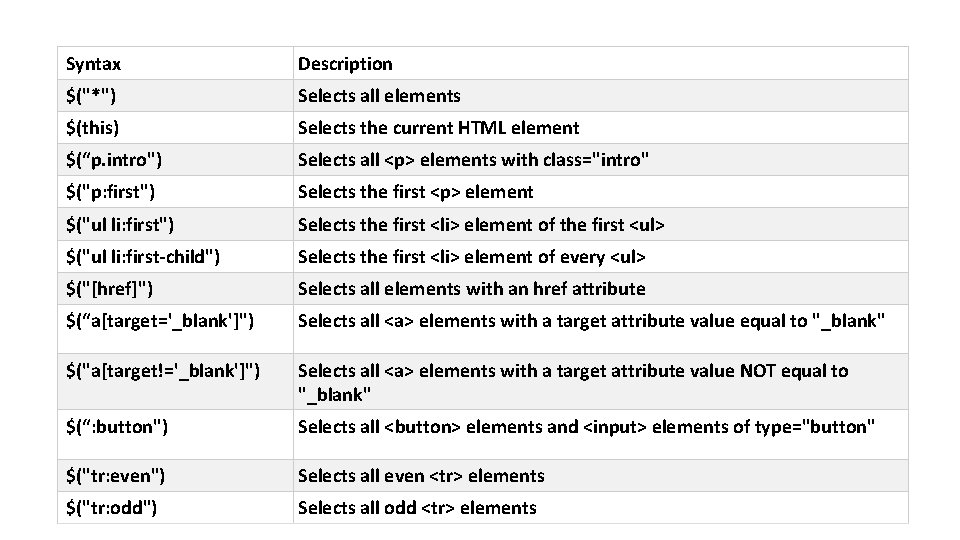
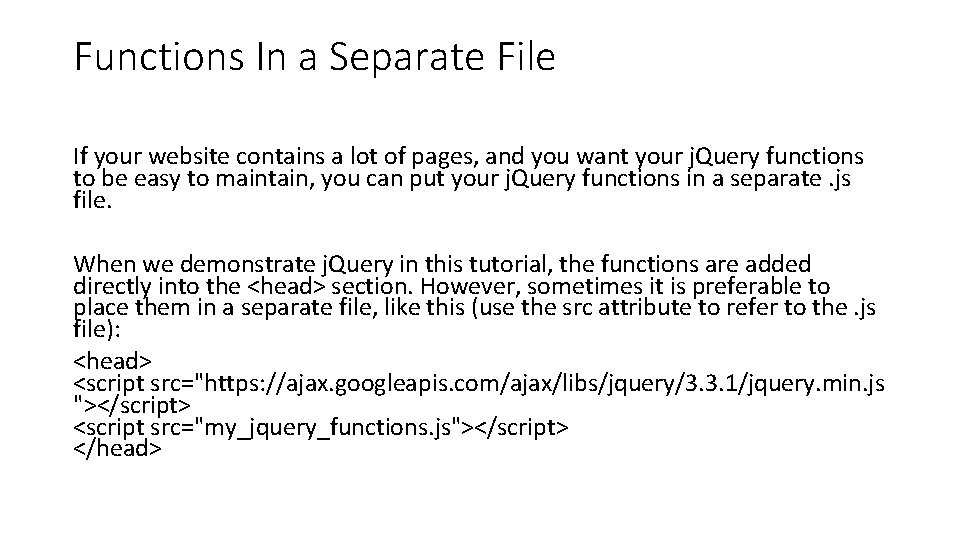
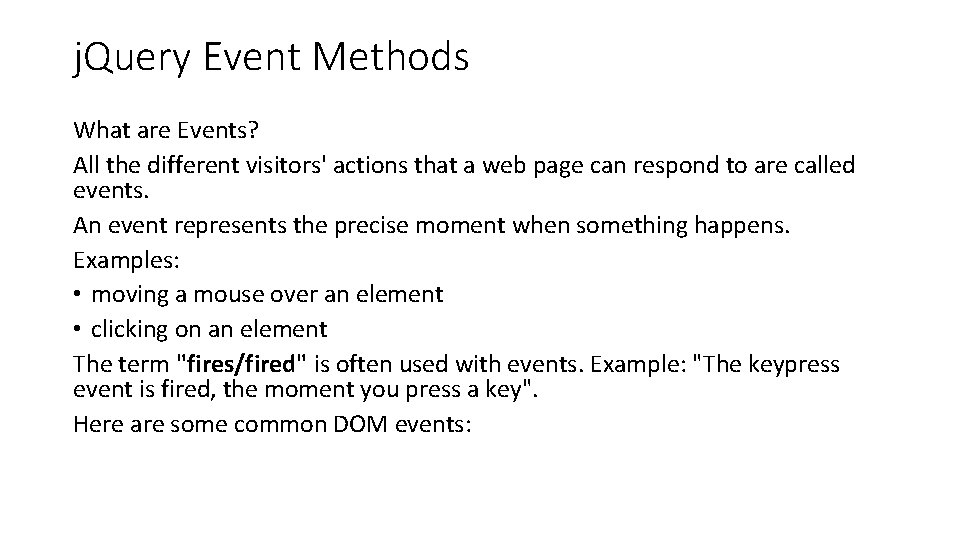
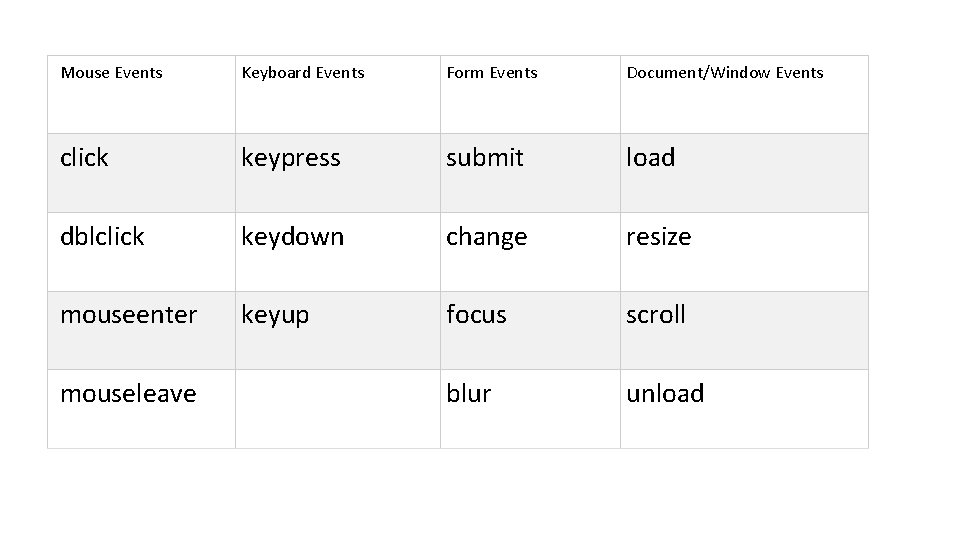
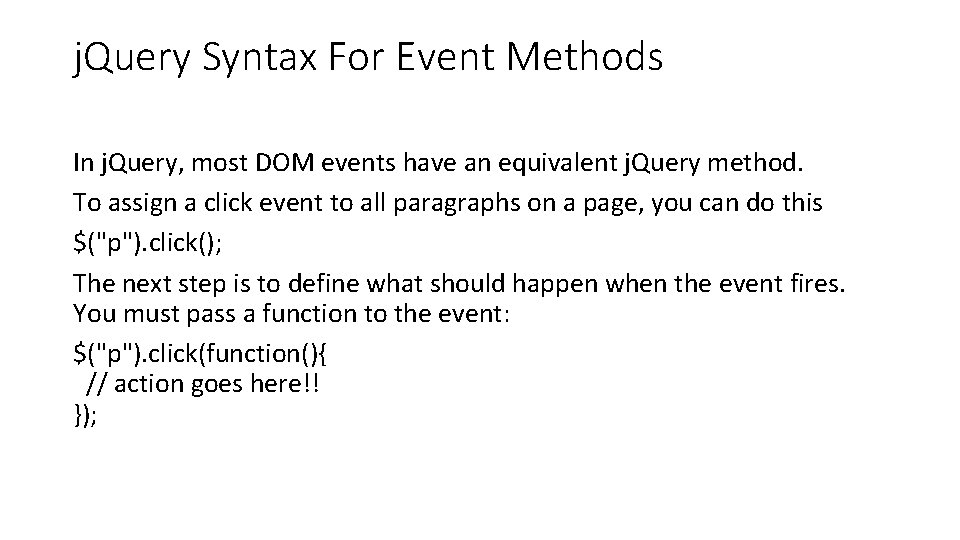
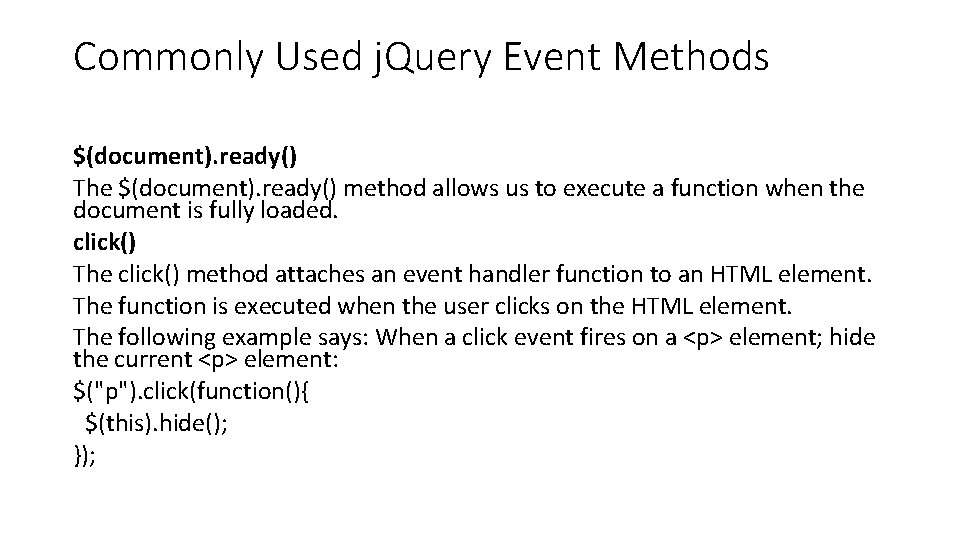
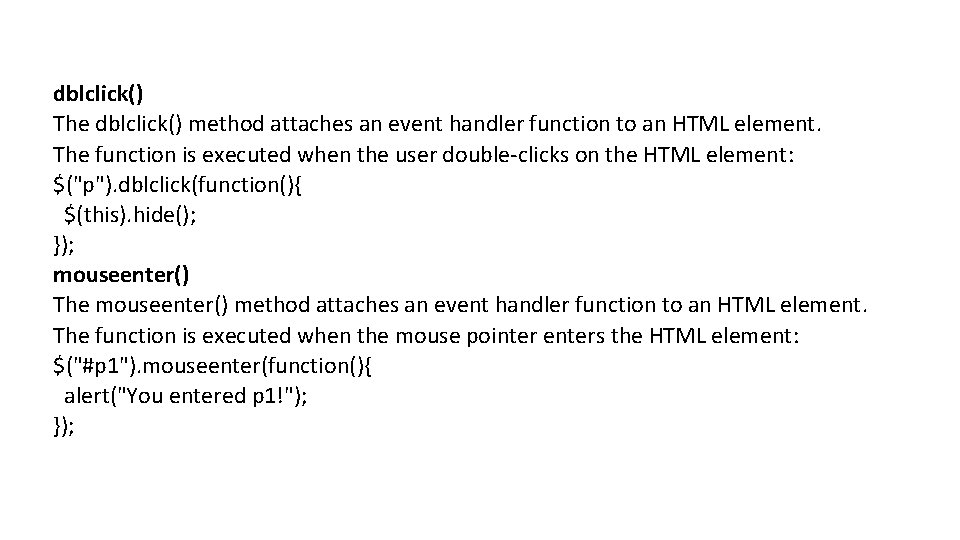
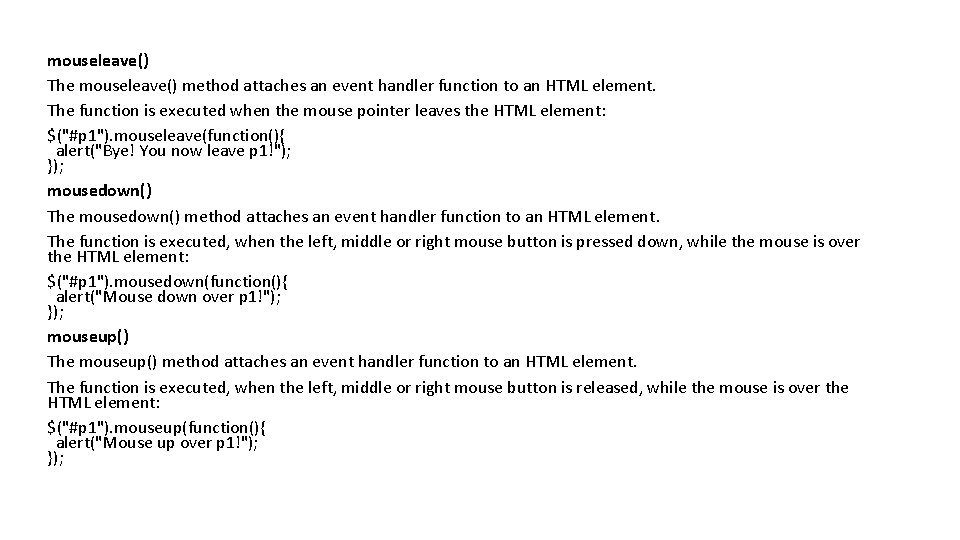
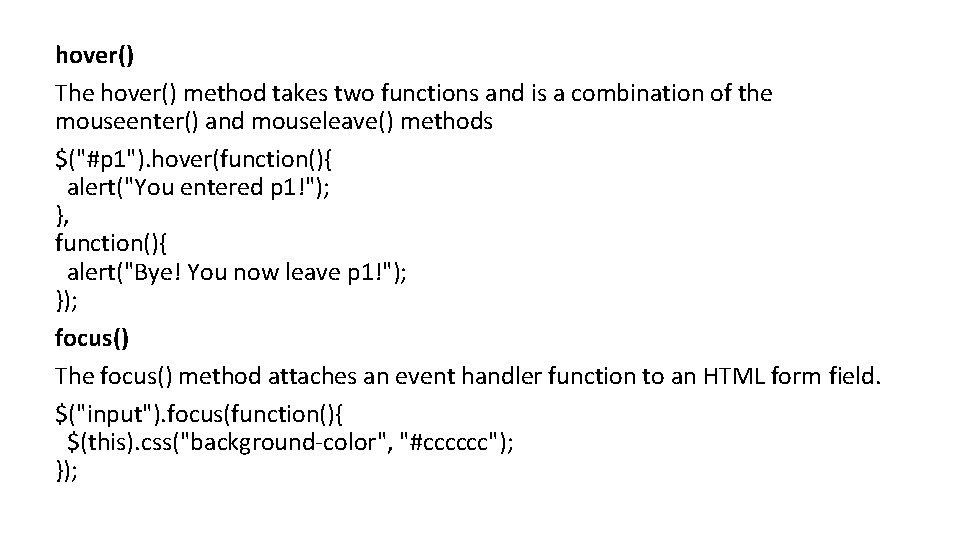
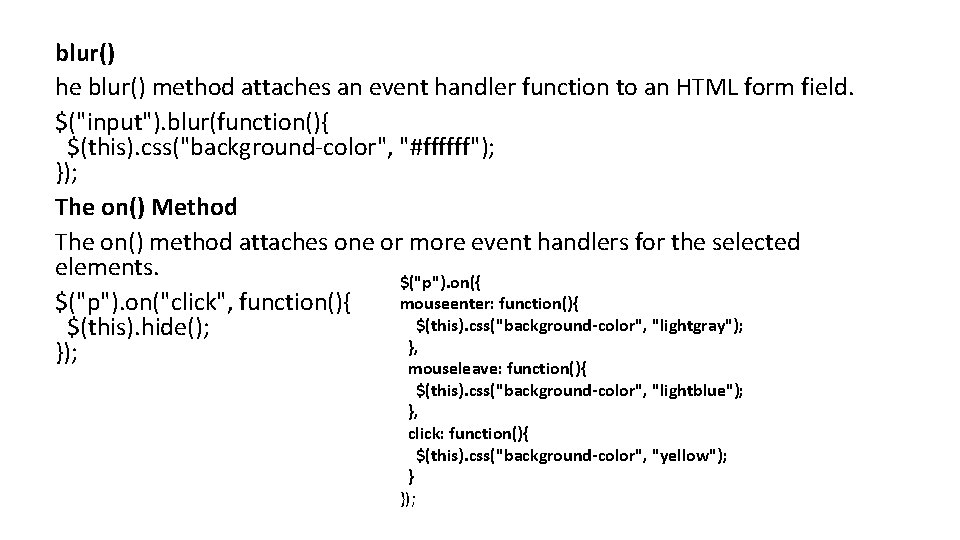
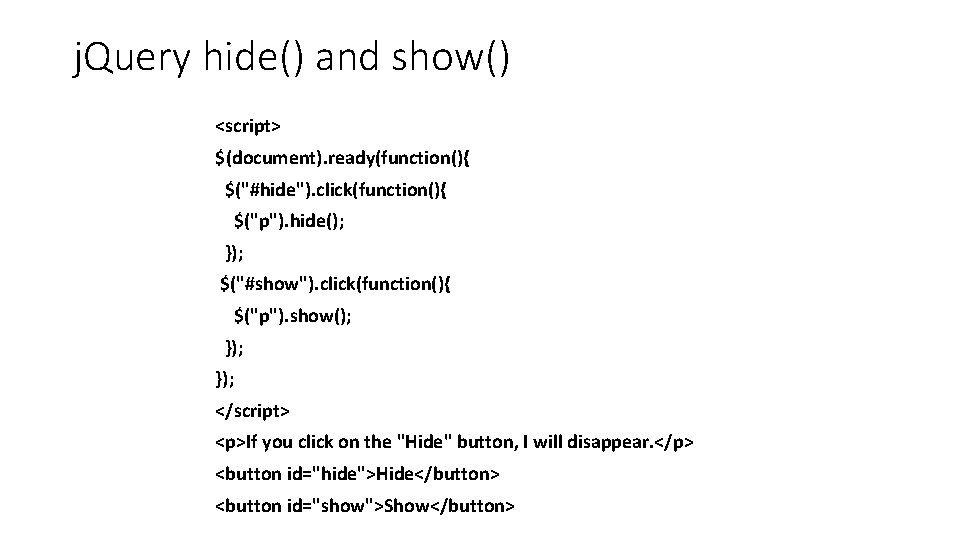
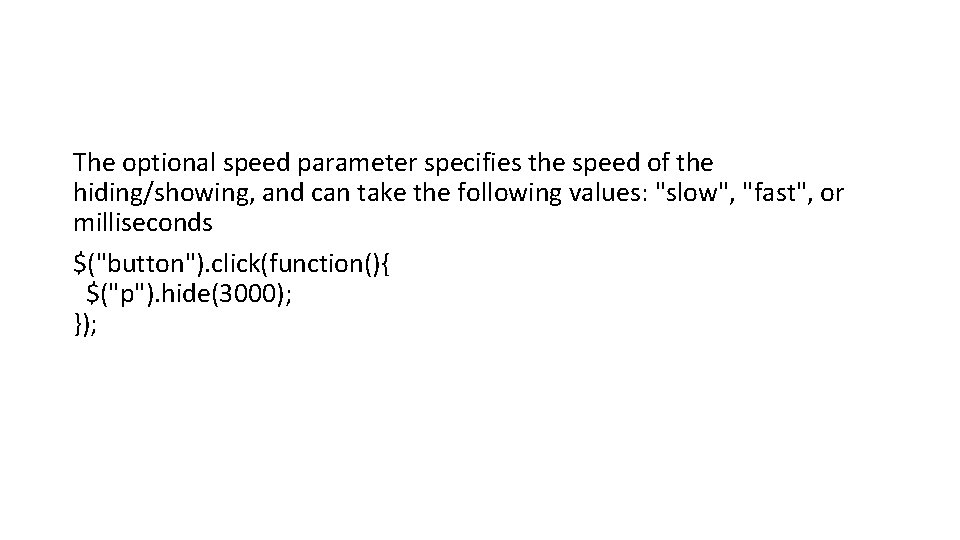
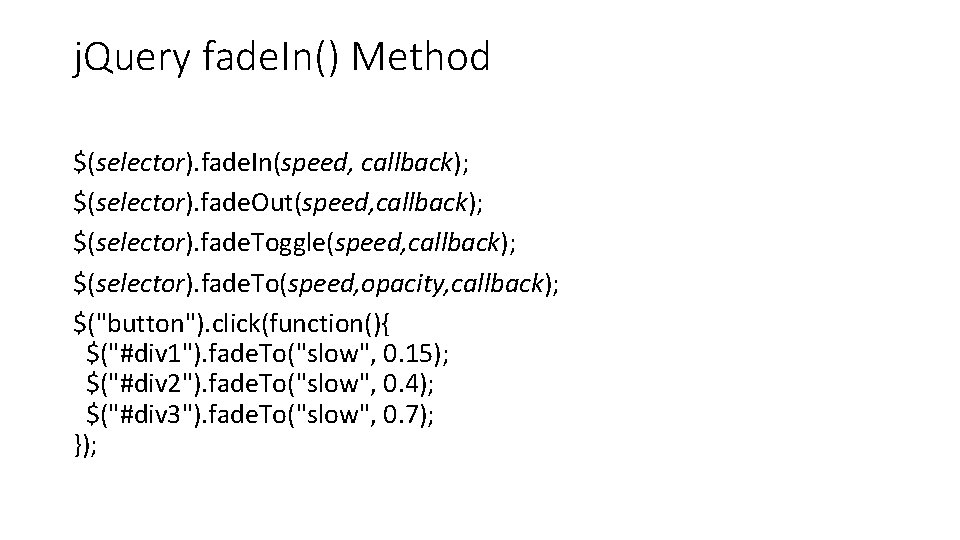
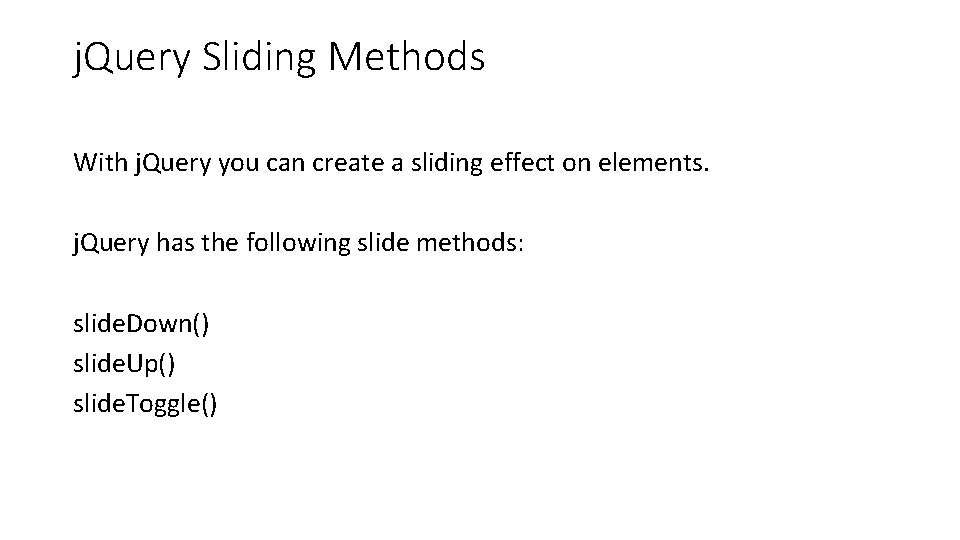
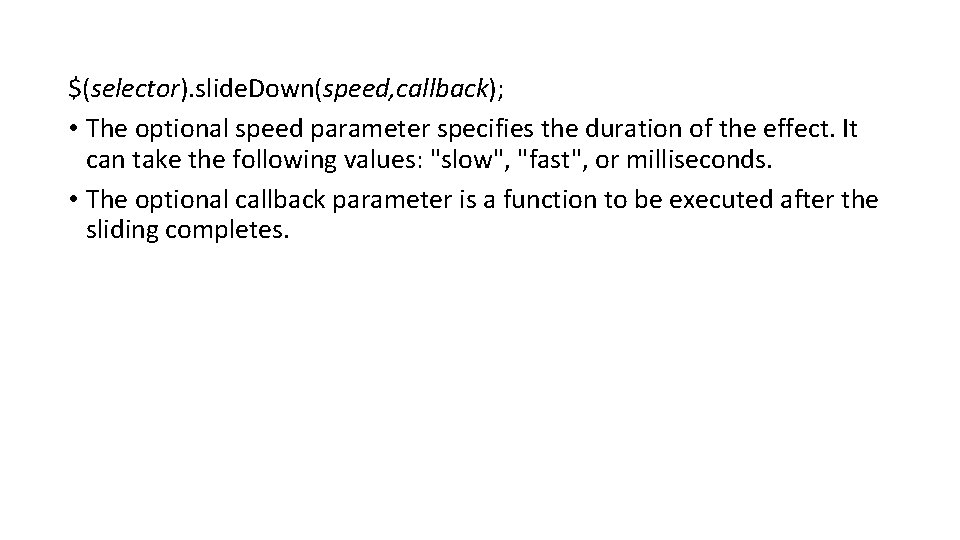
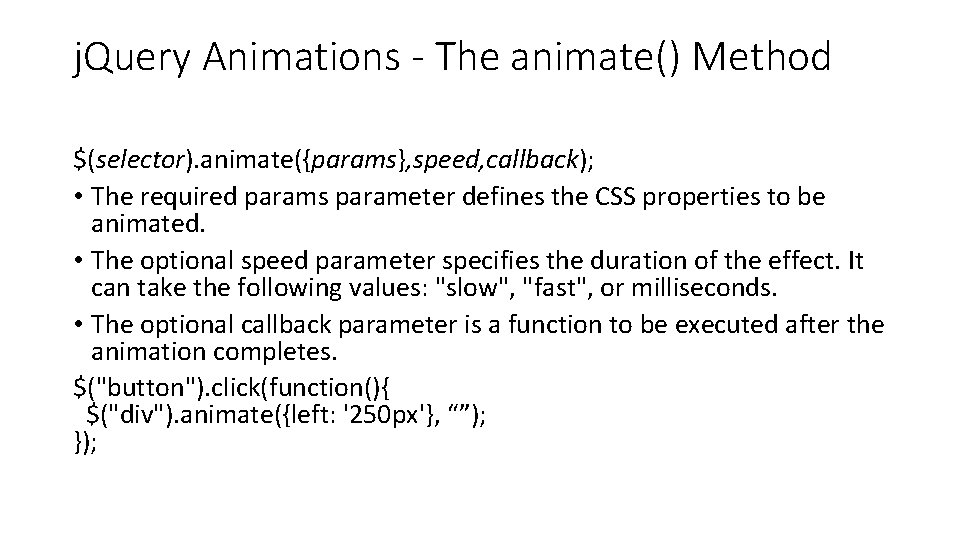
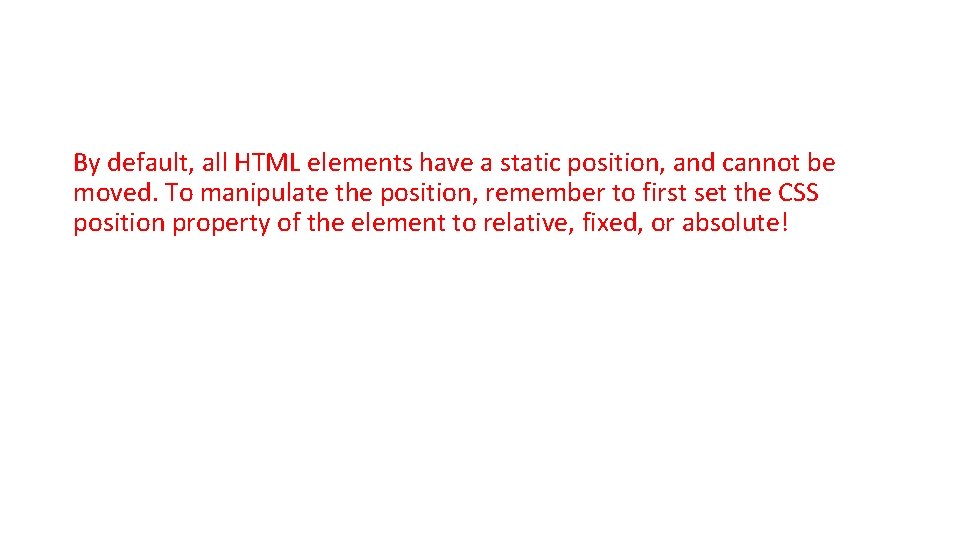
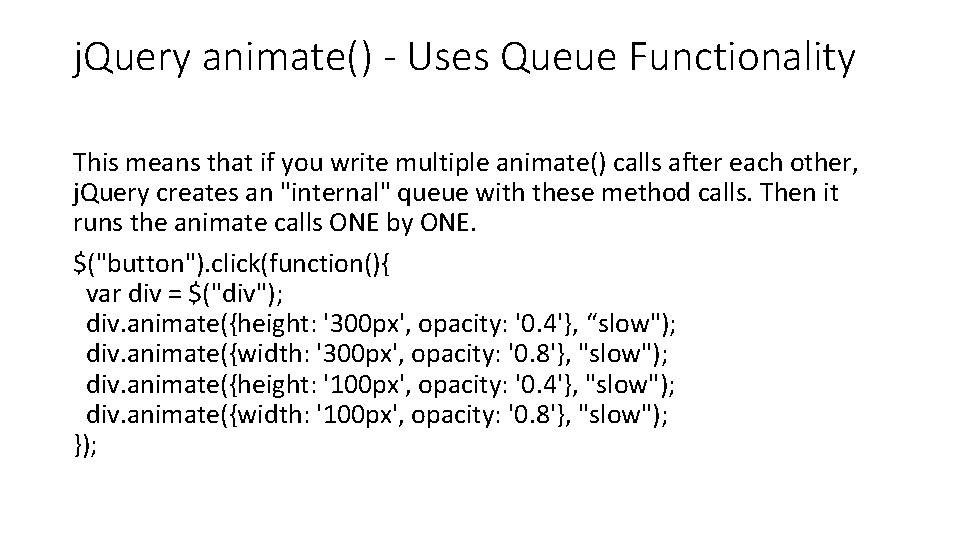
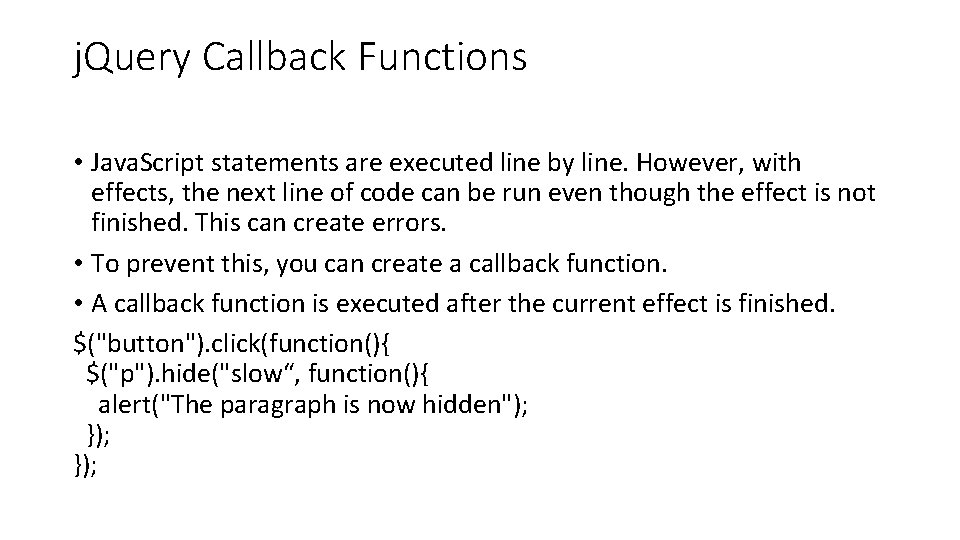
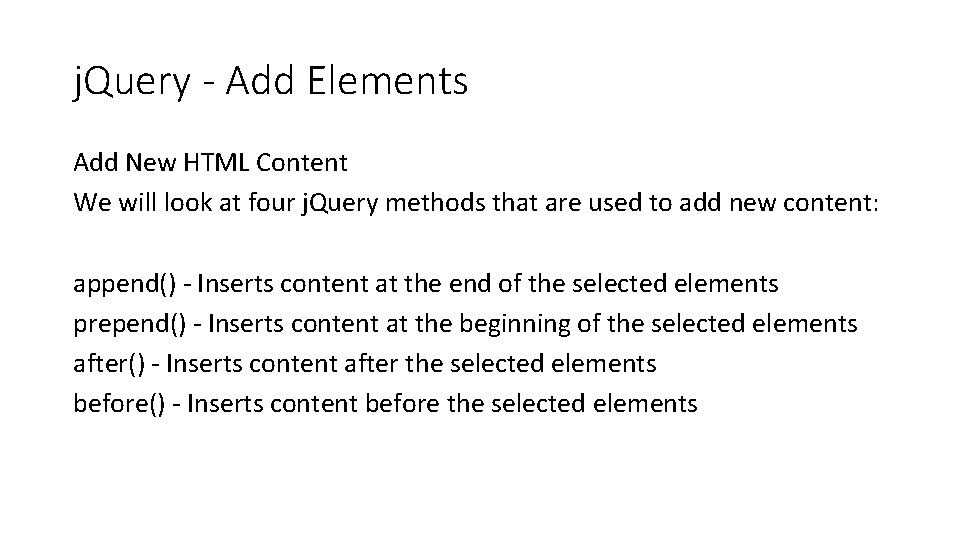
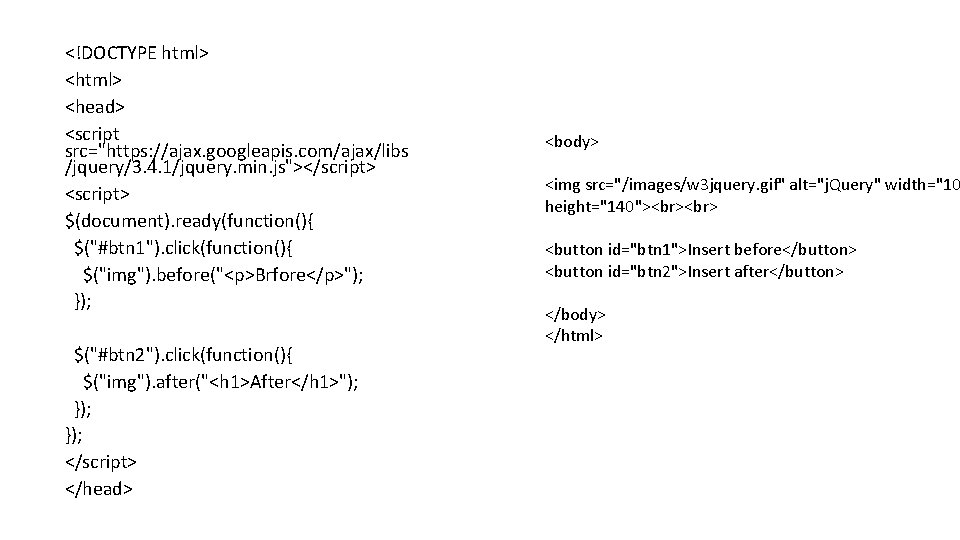
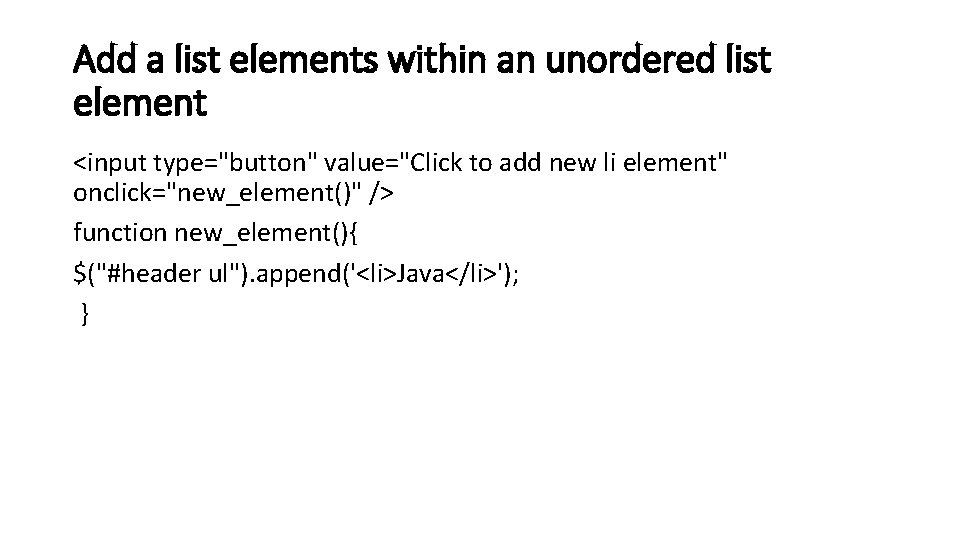
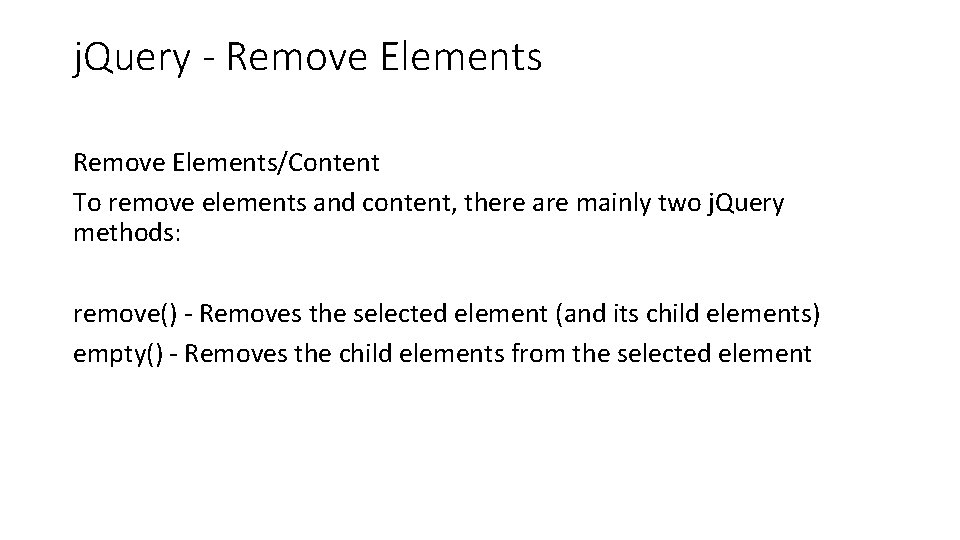
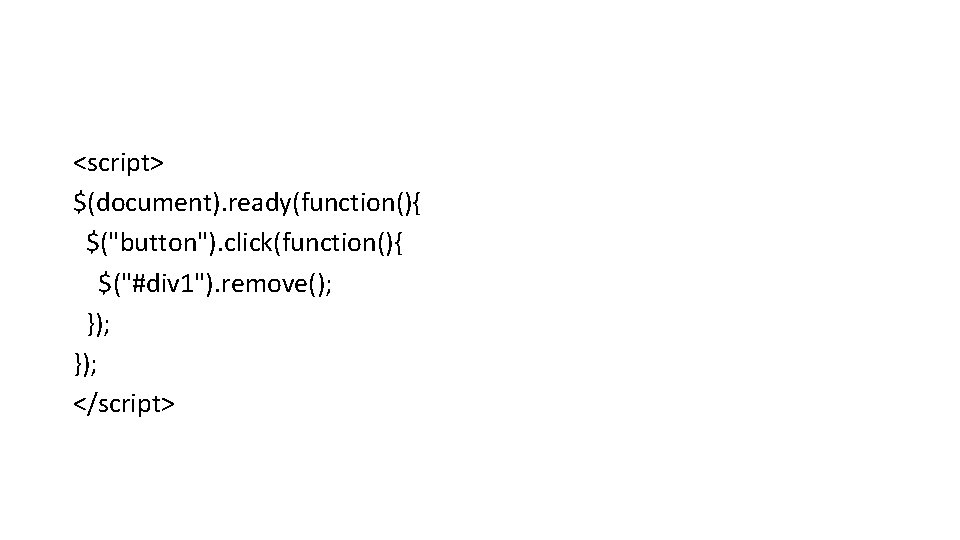
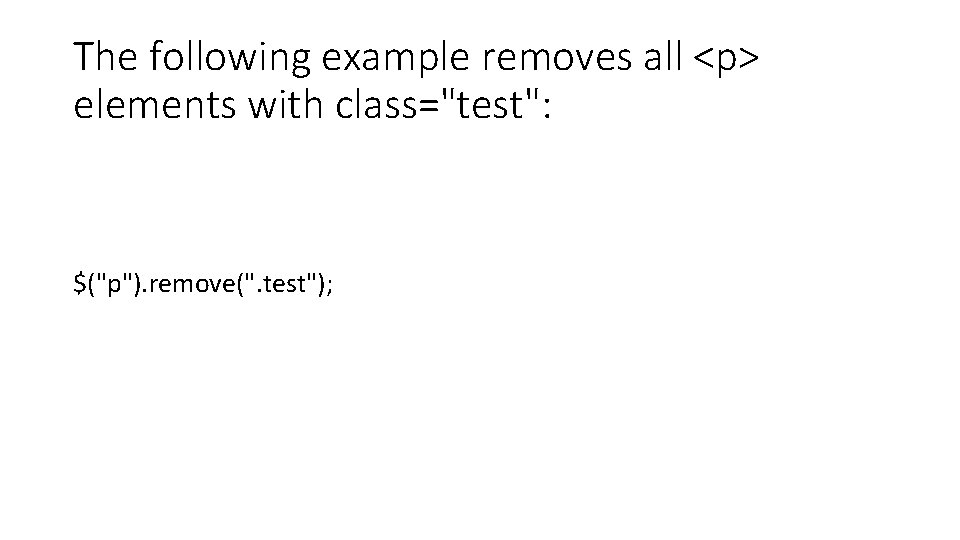
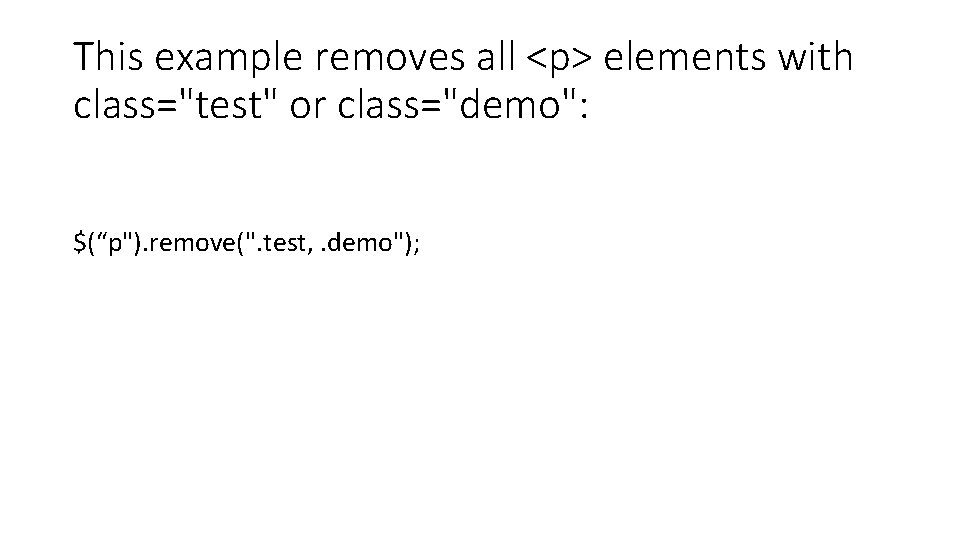
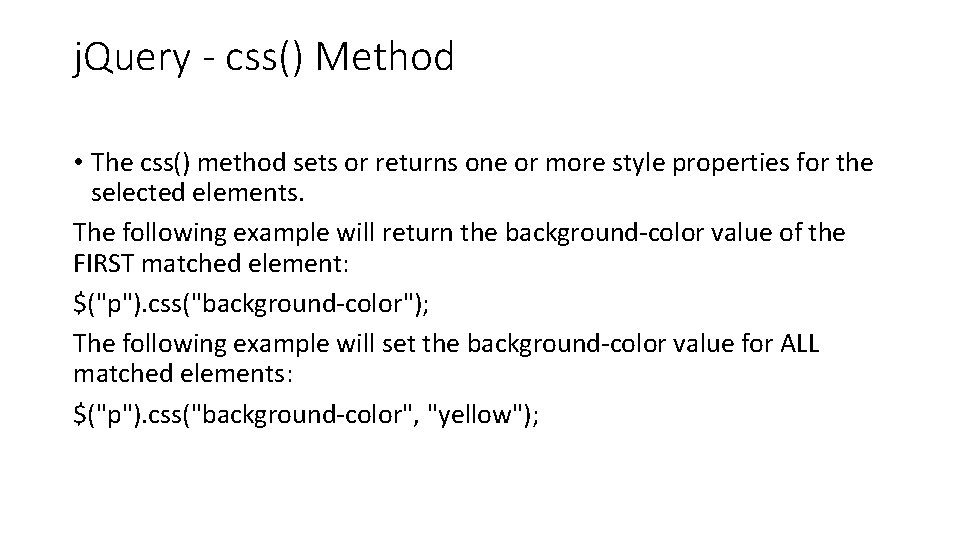
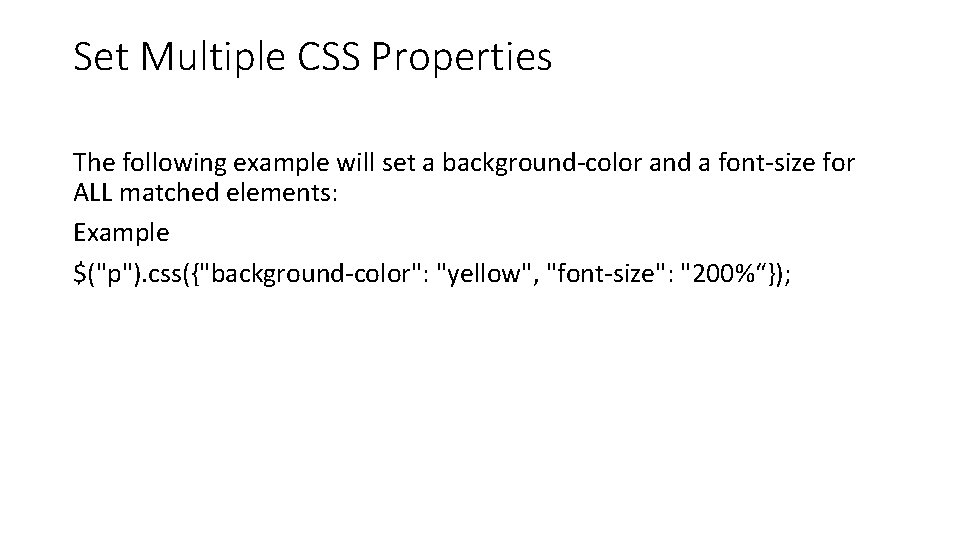
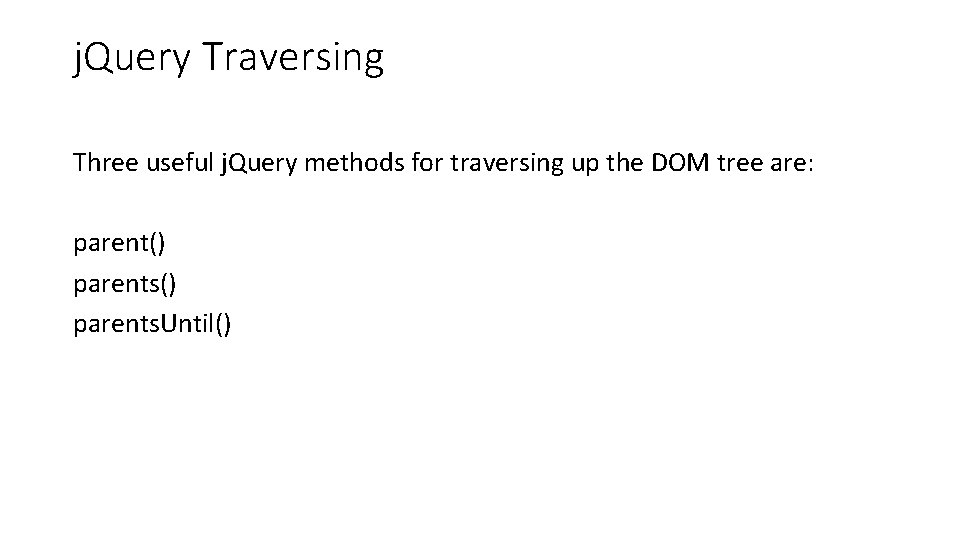
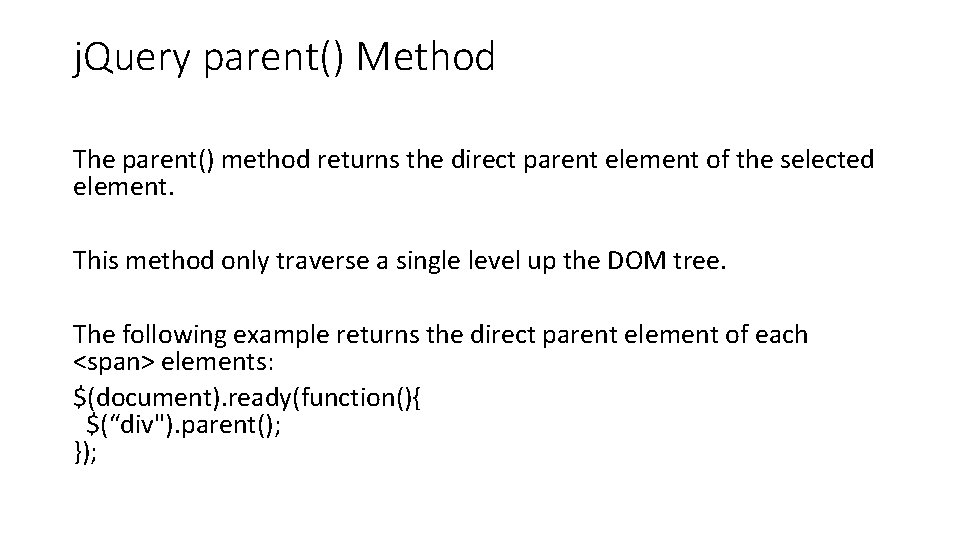
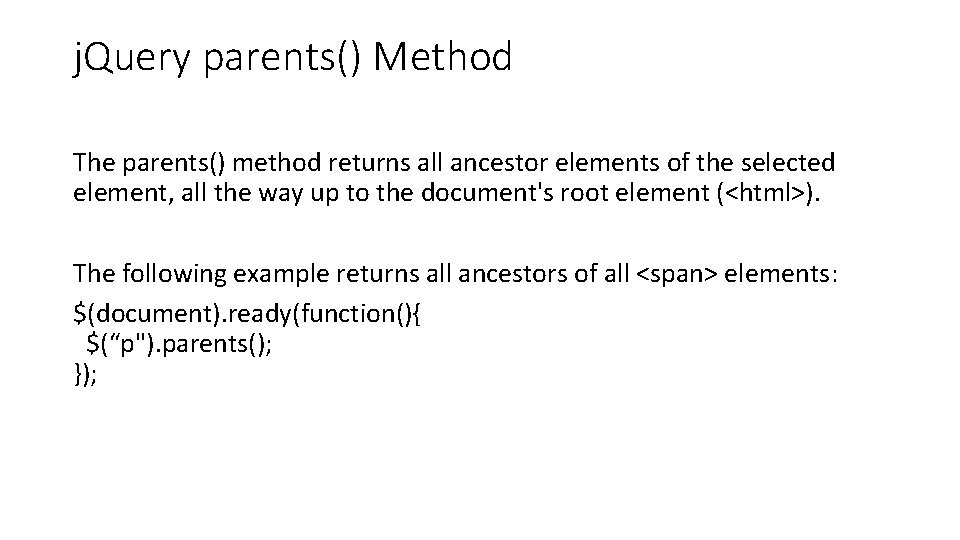
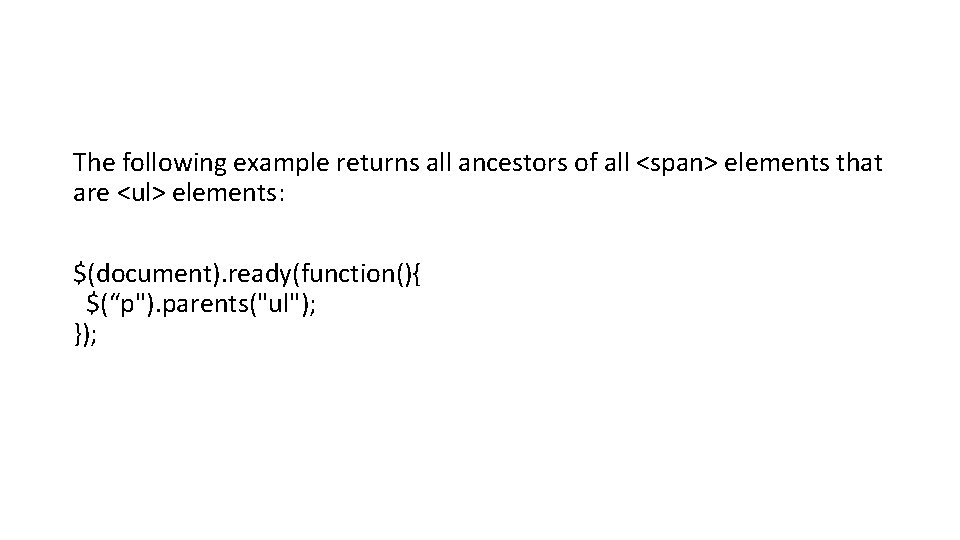
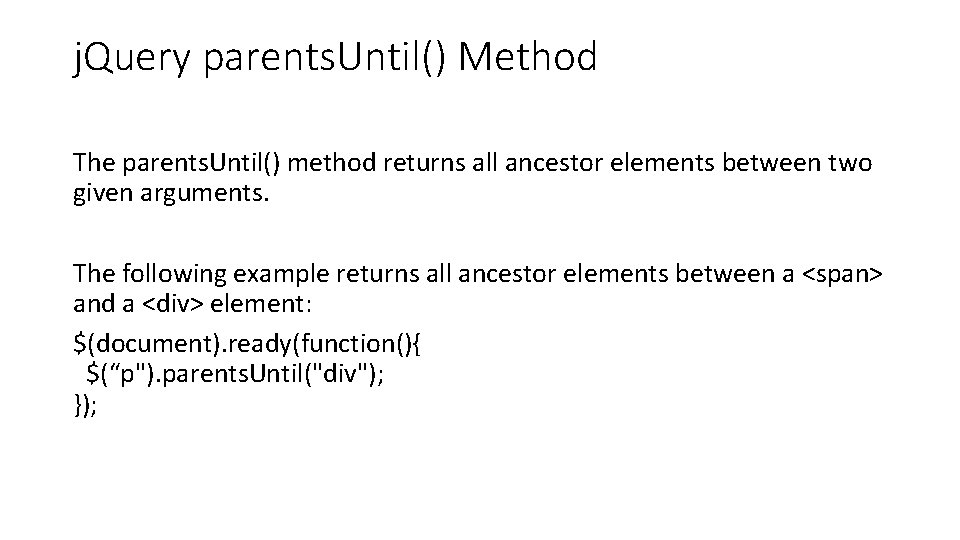
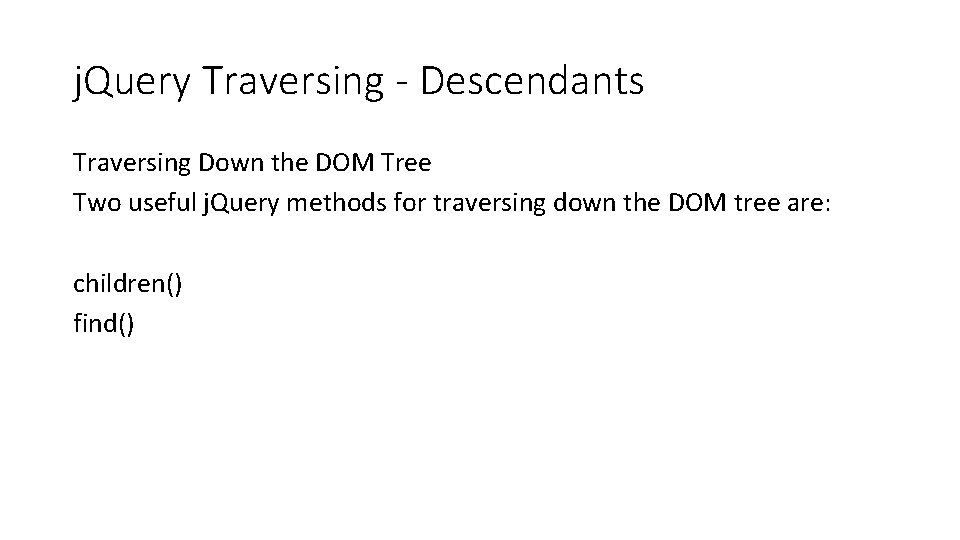
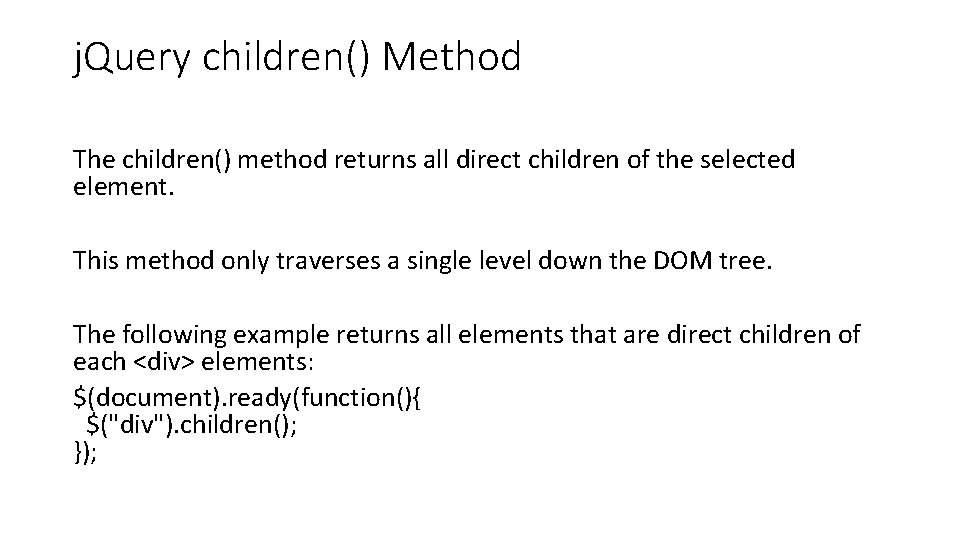
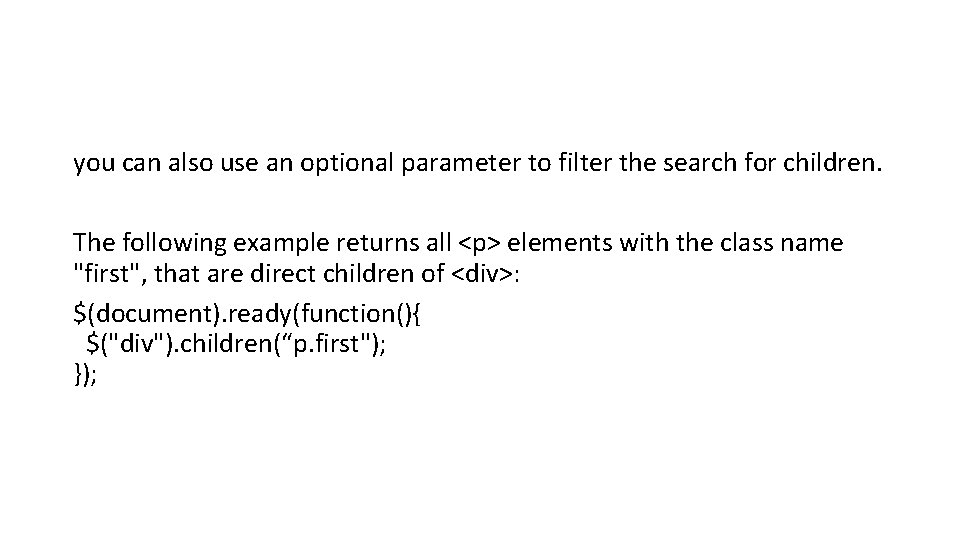
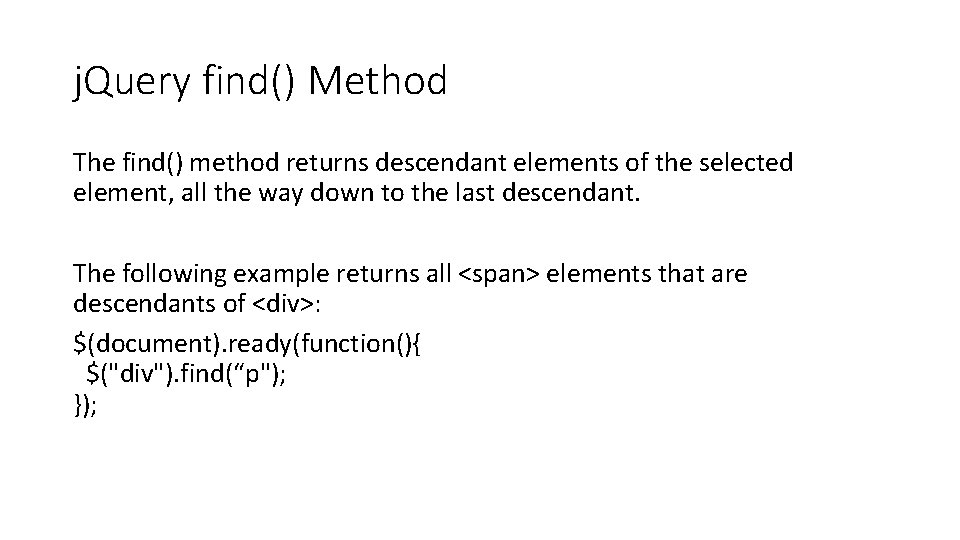
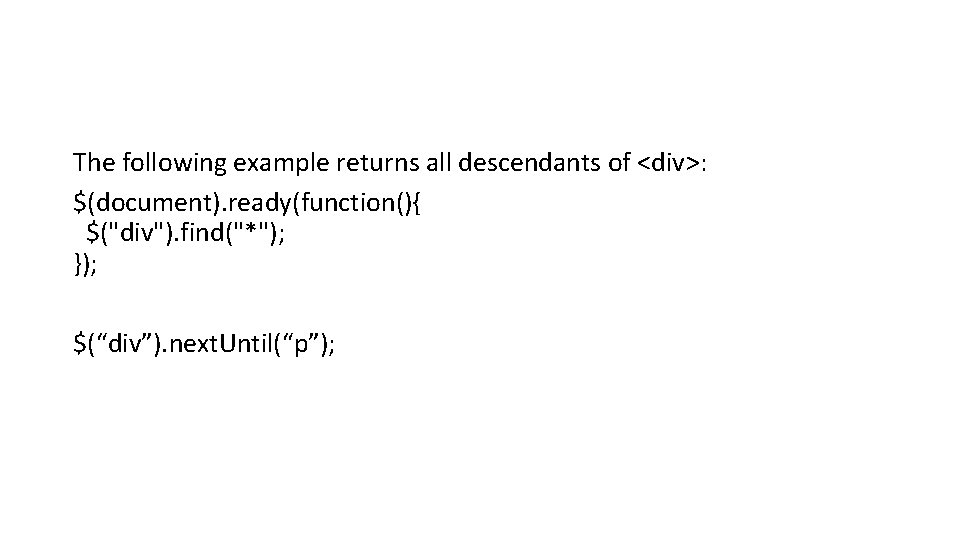
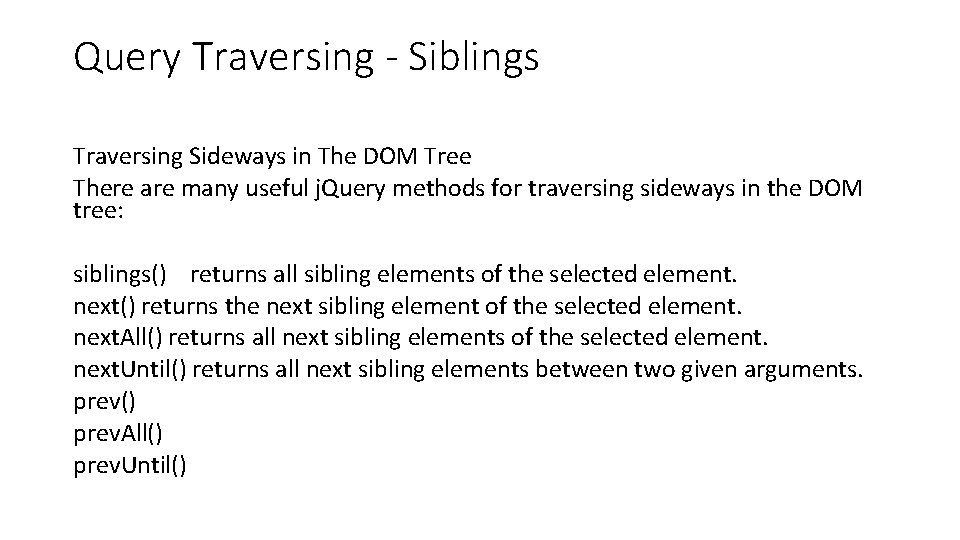
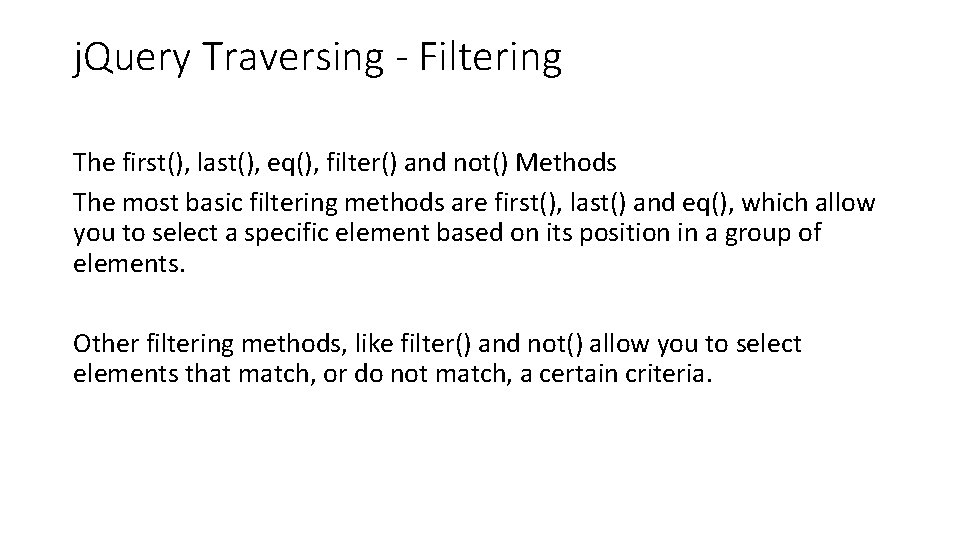
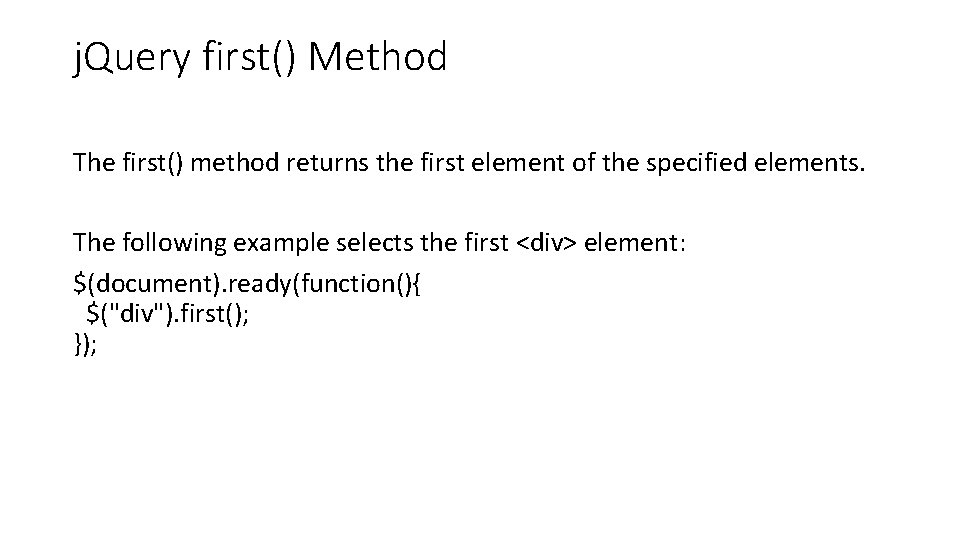
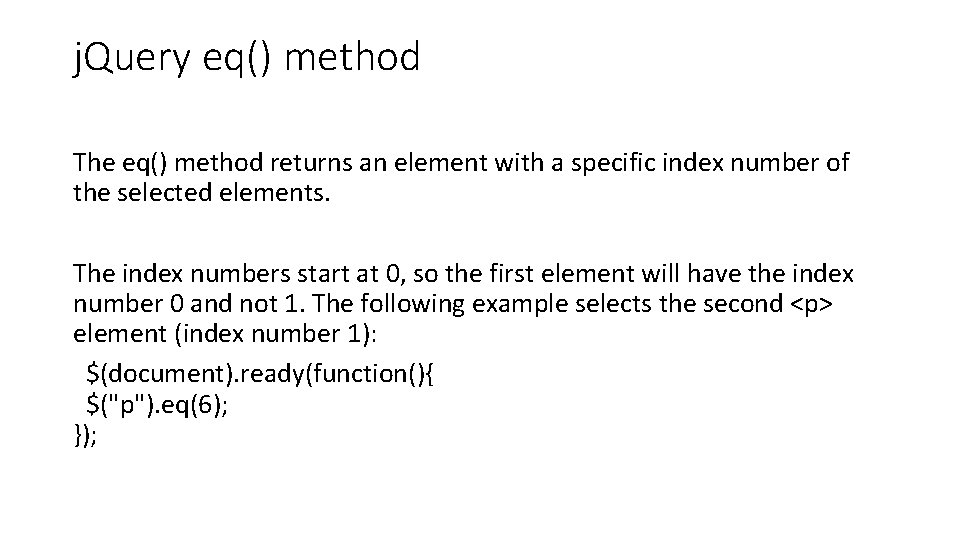
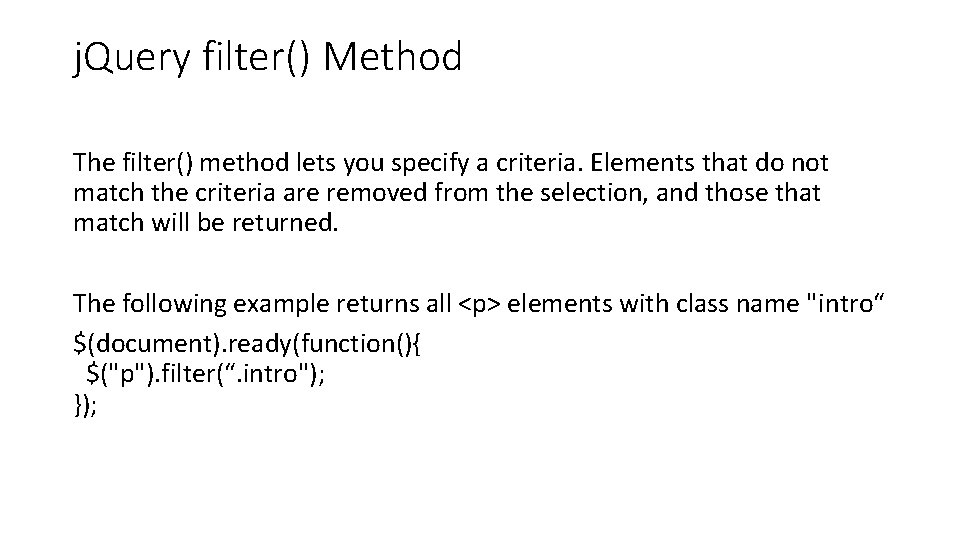
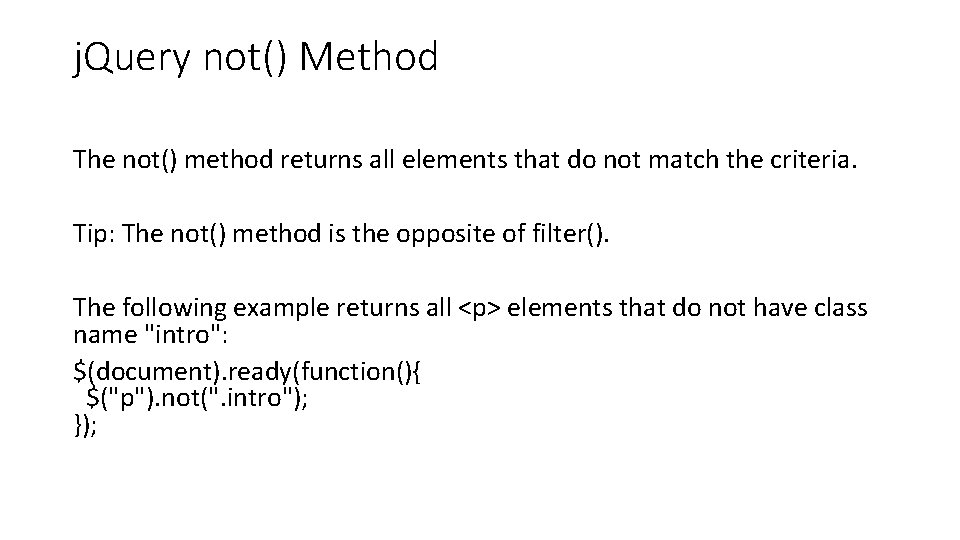
- Slides: 54
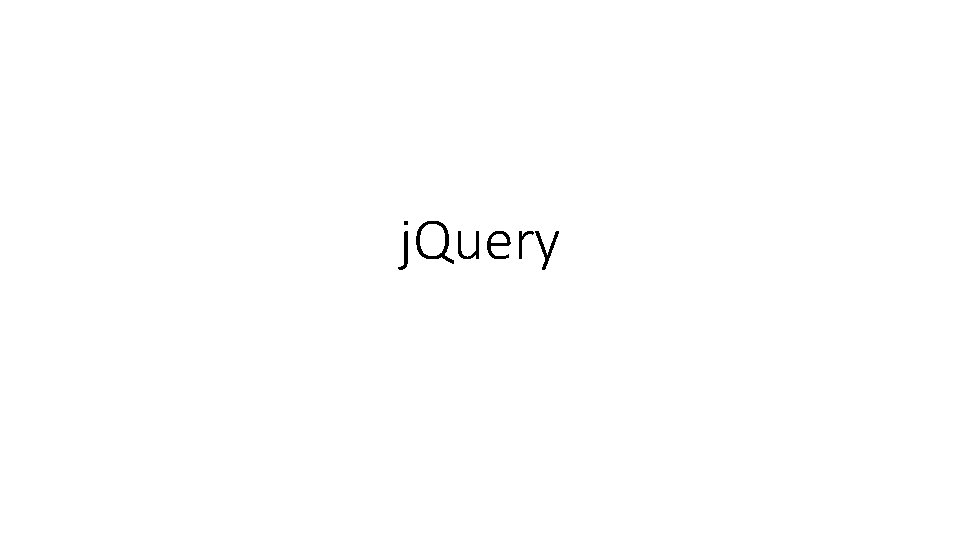
j. Query
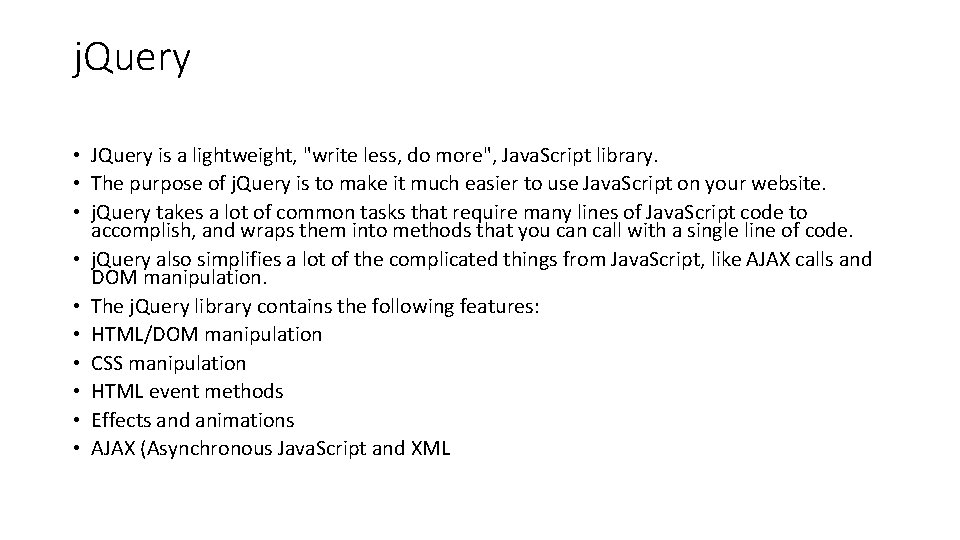
j. Query • JQuery is a lightweight, "write less, do more", Java. Script library. • The purpose of j. Query is to make it much easier to use Java. Script on your website. • j. Query takes a lot of common tasks that require many lines of Java. Script code to accomplish, and wraps them into methods that you can call with a single line of code. • j. Query also simplifies a lot of the complicated things from Java. Script, like AJAX calls and DOM manipulation. • The j. Query library contains the following features: • HTML/DOM manipulation • CSS manipulation • HTML event methods • Effects and animations • AJAX (Asynchronous Java. Script and XML
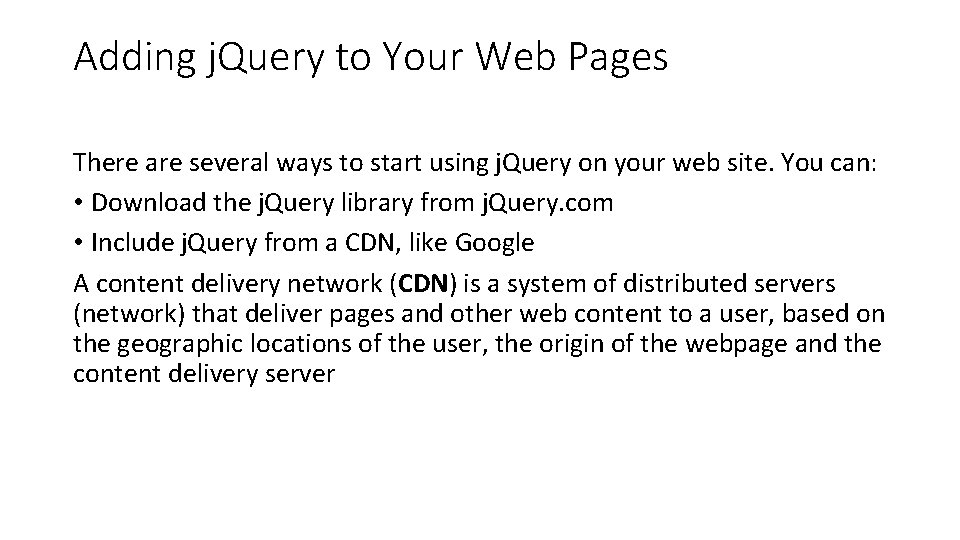
Adding j. Query to Your Web Pages There are several ways to start using j. Query on your web site. You can: • Download the j. Query library from j. Query. com • Include j. Query from a CDN, like Google A content delivery network (CDN) is a system of distributed servers (network) that deliver pages and other web content to a user, based on the geographic locations of the user, the origin of the webpage and the content delivery server
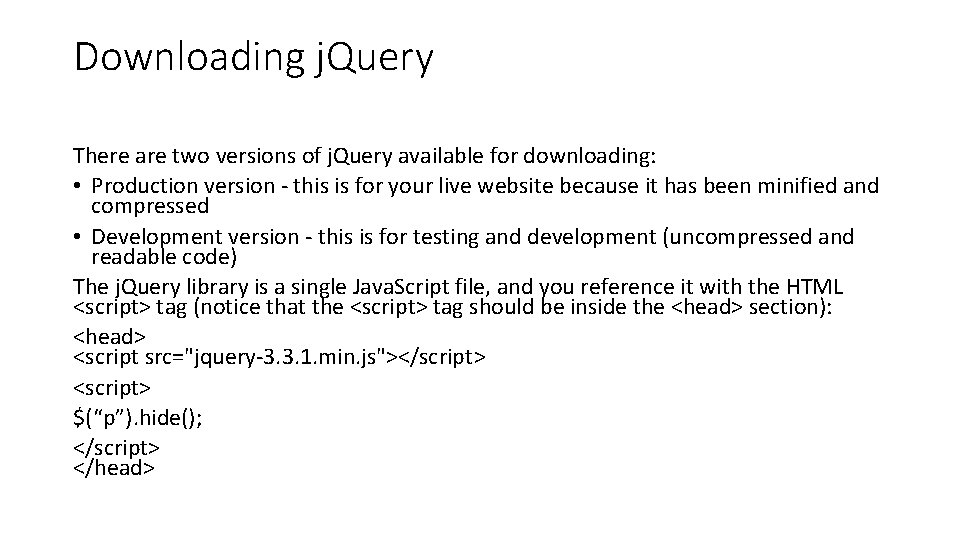
Downloading j. Query There are two versions of j. Query available for downloading: • Production version - this is for your live website because it has been minified and compressed • Development version - this is for testing and development (uncompressed and readable code) The j. Query library is a single Java. Script file, and you reference it with the HTML <script> tag (notice that the <script> tag should be inside the <head> section): <head> <script src="jquery-3. 3. 1. min. js"></script> <script> $(“p”). hide(); </script> </head>
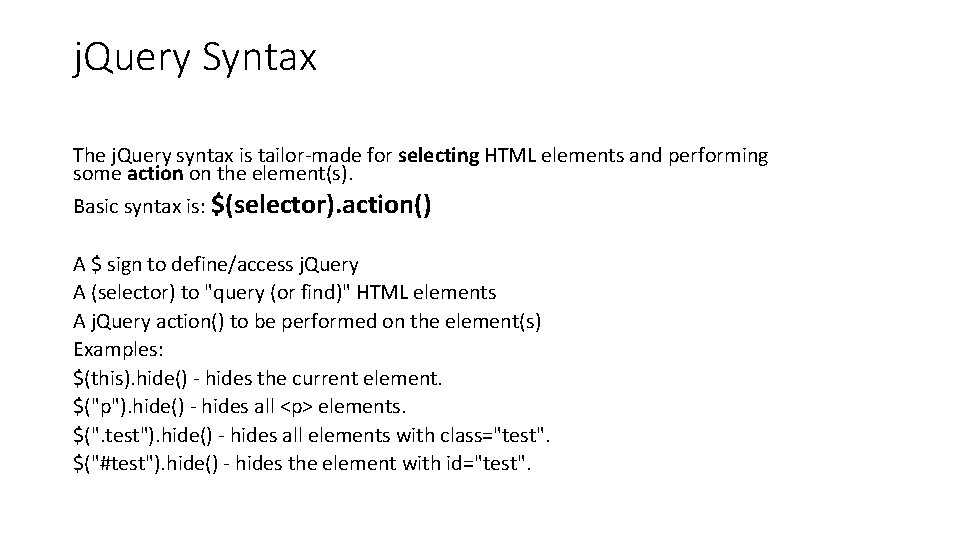
j. Query Syntax The j. Query syntax is tailor-made for selecting HTML elements and performing some action on the element(s). Basic syntax is: $(selector). action() A $ sign to define/access j. Query A (selector) to "query (or find)" HTML elements A j. Query action() to be performed on the element(s) Examples: $(this). hide() - hides the current element. $("p"). hide() - hides all <p> elements. $(". test"). hide() - hides all elements with class="test". $("#test"). hide() - hides the element with id="test".
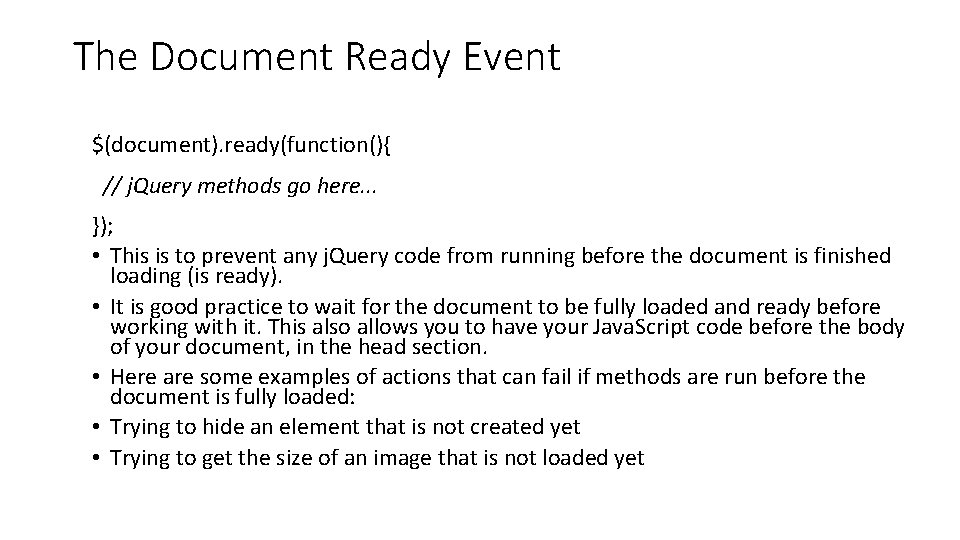
The Document Ready Event $(document). ready(function(){ // j. Query methods go here. . . }); • This is to prevent any j. Query code from running before the document is finished loading (is ready). • It is good practice to wait for the document to be fully loaded and ready before working with it. This also allows you to have your Java. Script code before the body of your document, in the head section. • Here are some examples of actions that can fail if methods are run before the document is fully loaded: • Trying to hide an element that is not created yet • Trying to get the size of an image that is not loaded yet
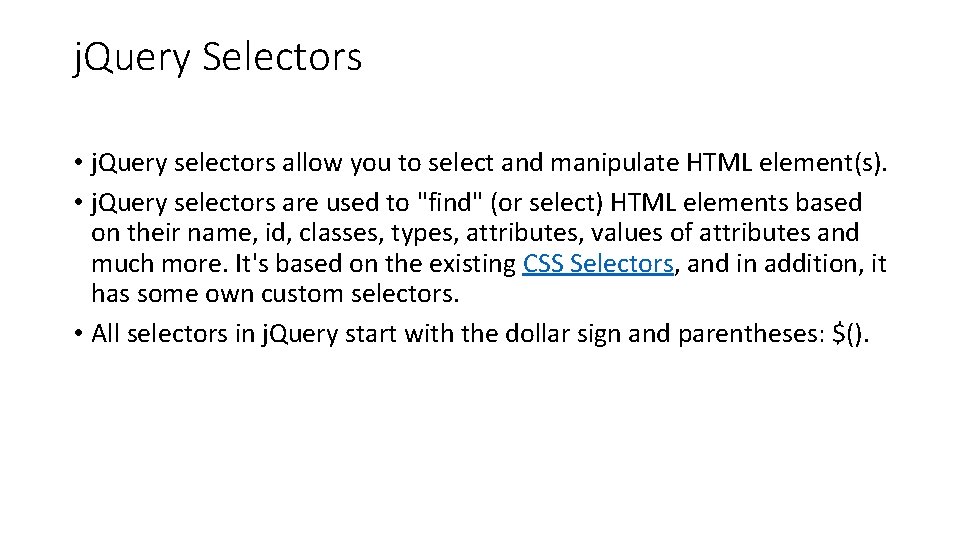
j. Query Selectors • j. Query selectors allow you to select and manipulate HTML element(s). • j. Query selectors are used to "find" (or select) HTML elements based on their name, id, classes, types, attributes, values of attributes and much more. It's based on the existing CSS Selectors, and in addition, it has some own custom selectors. • All selectors in j. Query start with the dollar sign and parentheses: $().
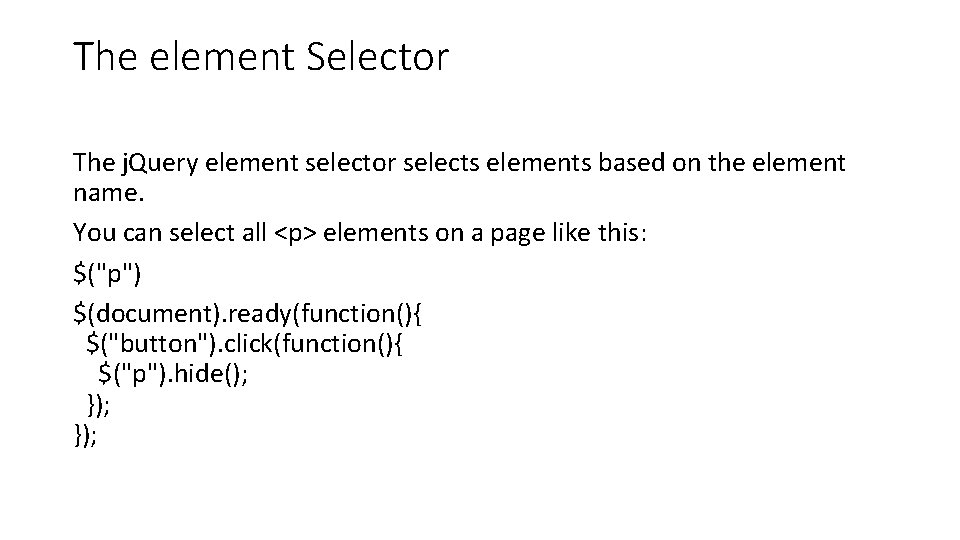
The element Selector The j. Query element selector selects elements based on the element name. You can select all <p> elements on a page like this: $("p") $(document). ready(function(){ $("button"). click(function(){ $("p"). hide(); });
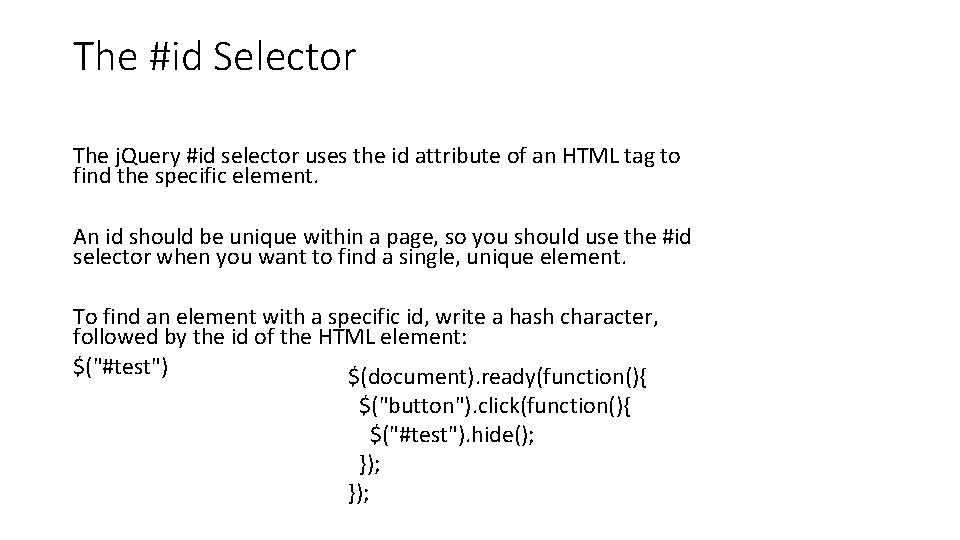
The #id Selector The j. Query #id selector uses the id attribute of an HTML tag to find the specific element. An id should be unique within a page, so you should use the #id selector when you want to find a single, unique element. To find an element with a specific id, write a hash character, followed by the id of the HTML element: $("#test") $(document). ready(function(){ $("button"). click(function(){ $("#test"). hide(); });
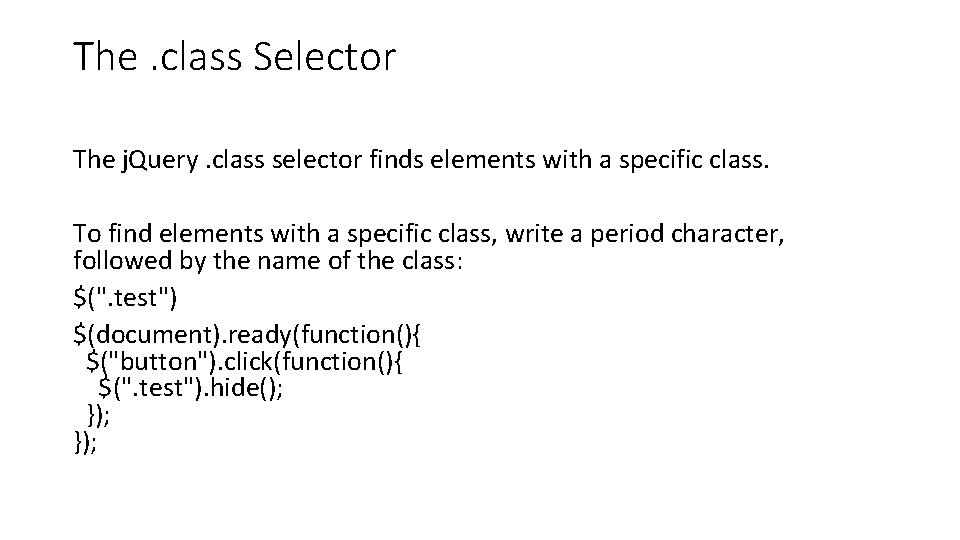
The. class Selector The j. Query. class selector finds elements with a specific class. To find elements with a specific class, write a period character, followed by the name of the class: $(". test") $(document). ready(function(){ $("button"). click(function(){ $(". test"). hide(); });
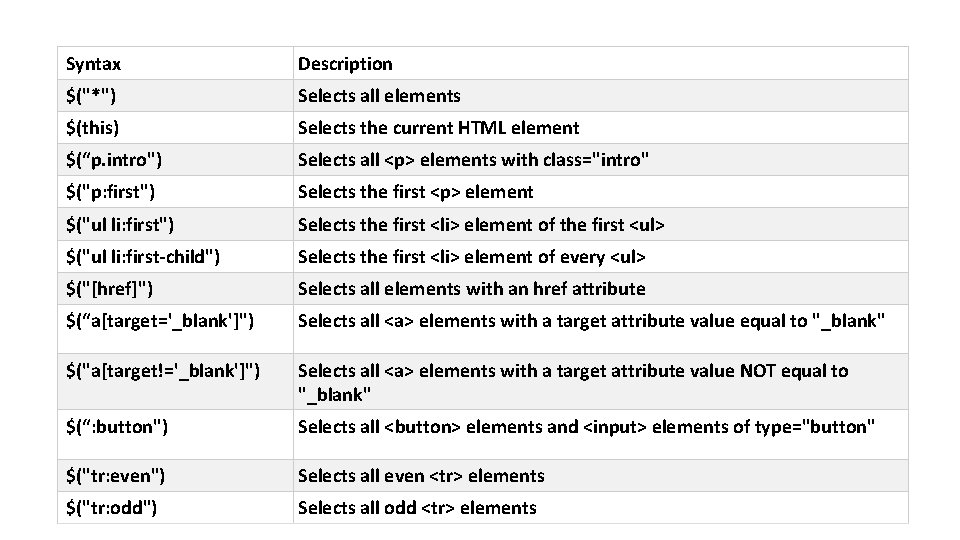
Syntax Description $("*") Selects all elements $(this) Selects the current HTML element $(“p. intro") Selects all <p> elements with class="intro" $("p: first") Selects the first <p> element $("ul li: first") Selects the first <li> element of the first <ul> $("ul li: first-child") Selects the first <li> element of every <ul> $("[href]") Selects all elements with an href attribute $(“a[target='_blank']") Selects all <a> elements with a target attribute value equal to "_blank" $("a[target!='_blank']") Selects all <a> elements with a target attribute value NOT equal to "_blank" $(“: button") Selects all <button> elements and <input> elements of type="button" $("tr: even") Selects all even <tr> elements $("tr: odd") Selects all odd <tr> elements
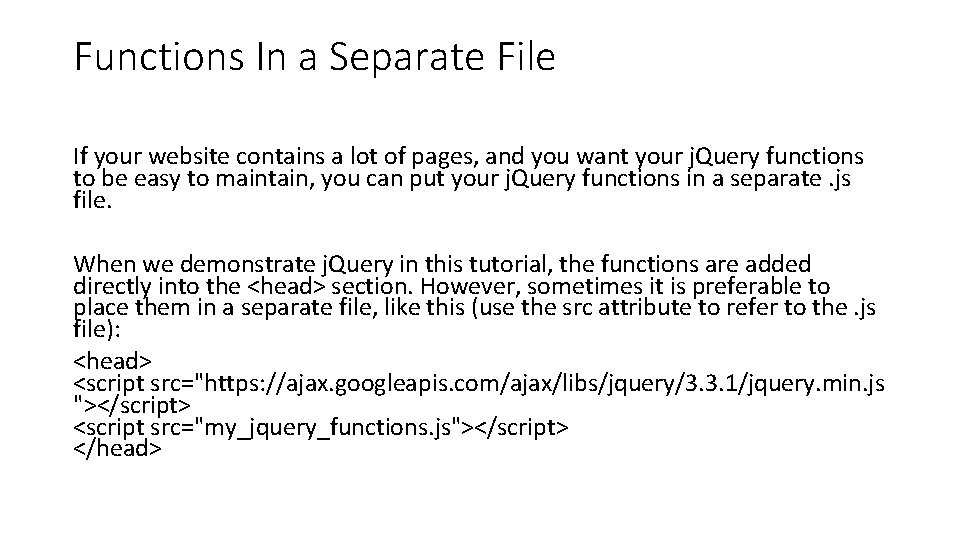
Functions In a Separate File If your website contains a lot of pages, and you want your j. Query functions to be easy to maintain, you can put your j. Query functions in a separate. js file. When we demonstrate j. Query in this tutorial, the functions are added directly into the <head> section. However, sometimes it is preferable to place them in a separate file, like this (use the src attribute to refer to the. js file): <head> <script src="https: //ajax. googleapis. com/ajax/libs/jquery/3. 3. 1/jquery. min. js "></script> <script src="my_jquery_functions. js"></script> </head>
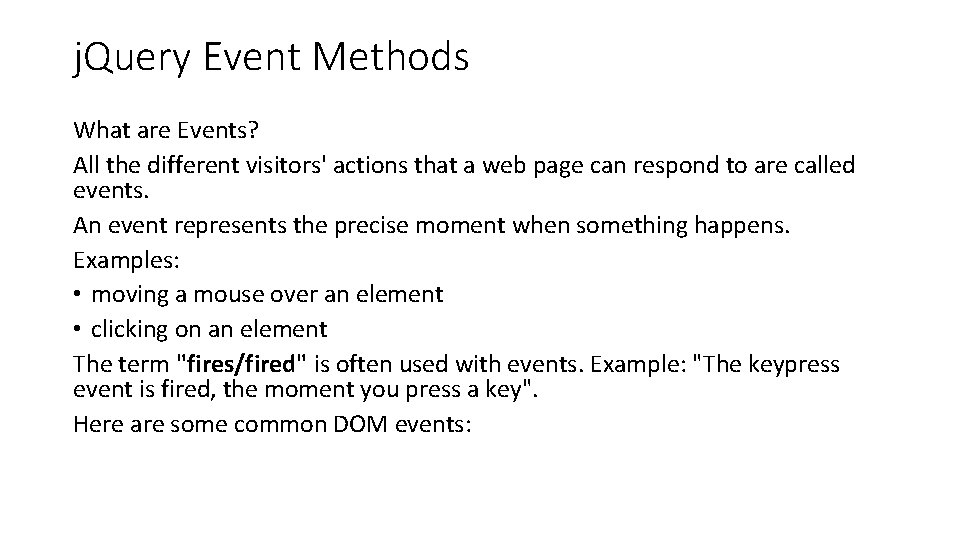
j. Query Event Methods What are Events? All the different visitors' actions that a web page can respond to are called events. An event represents the precise moment when something happens. Examples: • moving a mouse over an element • clicking on an element The term "fires/fired" is often used with events. Example: "The keypress event is fired, the moment you press a key". Here are some common DOM events:
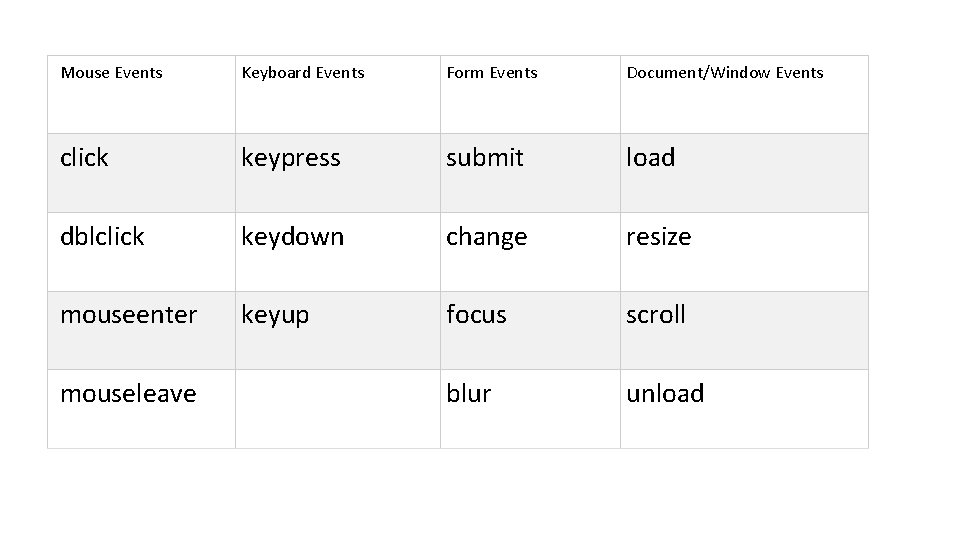
Mouse Events Keyboard Events Form Events Document/Window Events click keypress submit load dblclick keydown change resize mouseenter keyup focus scroll mouseleave blur unload
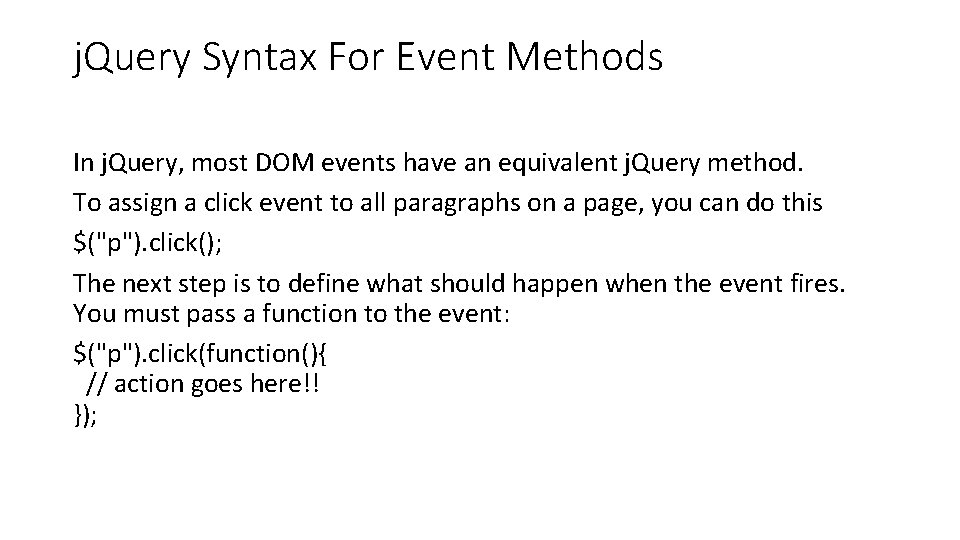
j. Query Syntax For Event Methods In j. Query, most DOM events have an equivalent j. Query method. To assign a click event to all paragraphs on a page, you can do this $("p"). click(); The next step is to define what should happen when the event fires. You must pass a function to the event: $("p"). click(function(){ // action goes here!! });
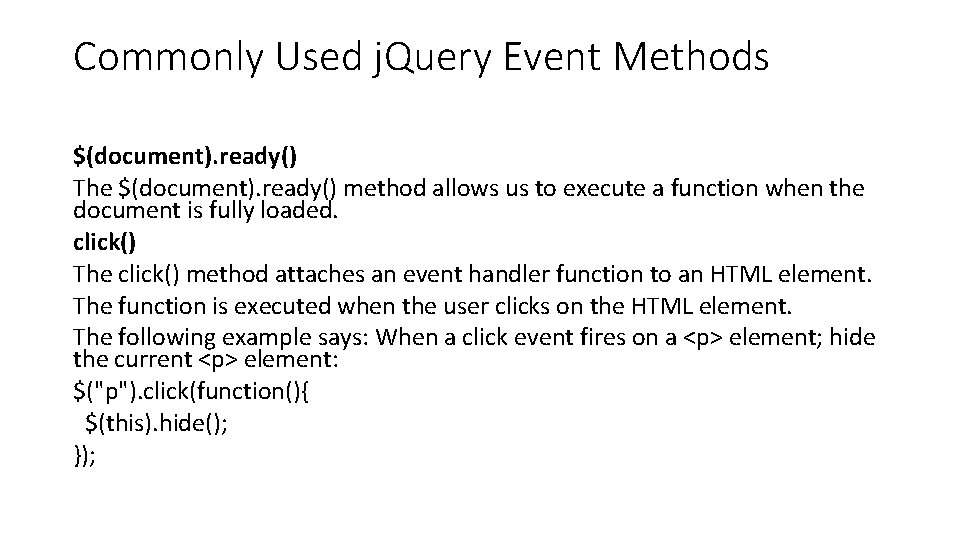
Commonly Used j. Query Event Methods $(document). ready() The $(document). ready() method allows us to execute a function when the document is fully loaded. click() The click() method attaches an event handler function to an HTML element. The function is executed when the user clicks on the HTML element. The following example says: When a click event fires on a <p> element; hide the current <p> element: $("p"). click(function(){ $(this). hide(); });
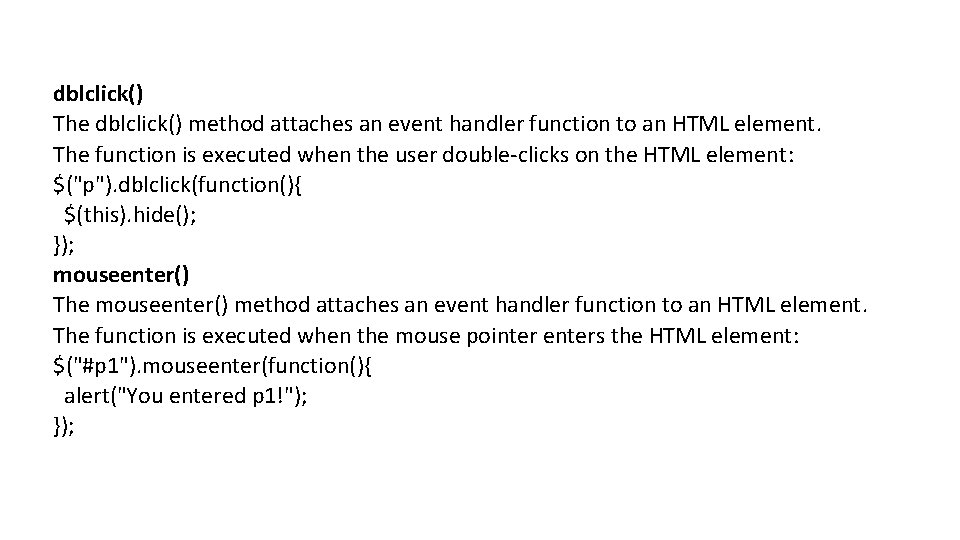
dblclick() The dblclick() method attaches an event handler function to an HTML element. The function is executed when the user double-clicks on the HTML element: $("p"). dblclick(function(){ $(this). hide(); }); mouseenter() The mouseenter() method attaches an event handler function to an HTML element. The function is executed when the mouse pointer enters the HTML element: $("#p 1"). mouseenter(function(){ alert("You entered p 1!"); });
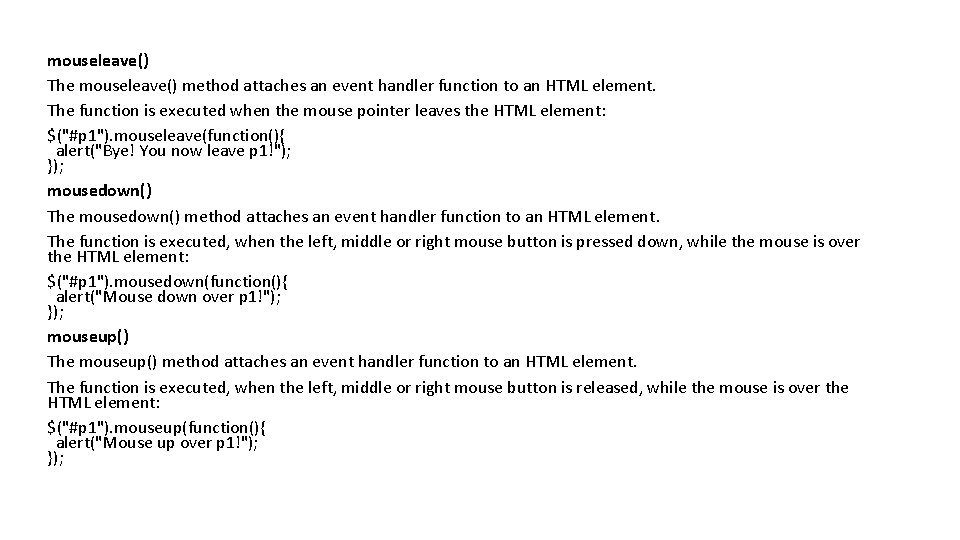
mouseleave() The mouseleave() method attaches an event handler function to an HTML element. The function is executed when the mouse pointer leaves the HTML element: $("#p 1"). mouseleave(function(){ alert("Bye! You now leave p 1!"); }); mousedown() The mousedown() method attaches an event handler function to an HTML element. The function is executed, when the left, middle or right mouse button is pressed down, while the mouse is over the HTML element: $("#p 1"). mousedown(function(){ alert("Mouse down over p 1!"); }); mouseup() The mouseup() method attaches an event handler function to an HTML element. The function is executed, when the left, middle or right mouse button is released, while the mouse is over the HTML element: $("#p 1"). mouseup(function(){ alert("Mouse up over p 1!"); });
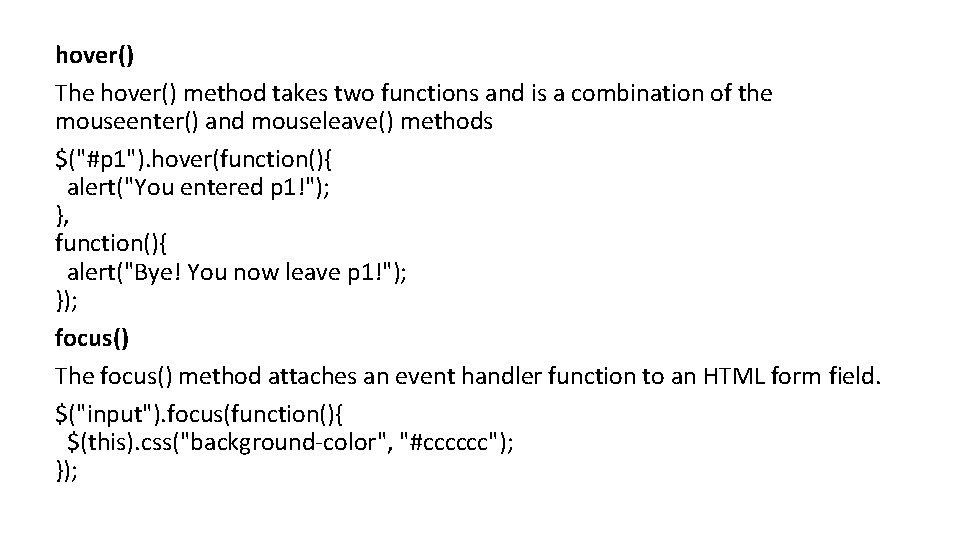
hover() The hover() method takes two functions and is a combination of the mouseenter() and mouseleave() methods $("#p 1"). hover(function(){ alert("You entered p 1!"); }, function(){ alert("Bye! You now leave p 1!"); }); focus() The focus() method attaches an event handler function to an HTML form field. $("input"). focus(function(){ $(this). css("background-color", "#cccccc"); });
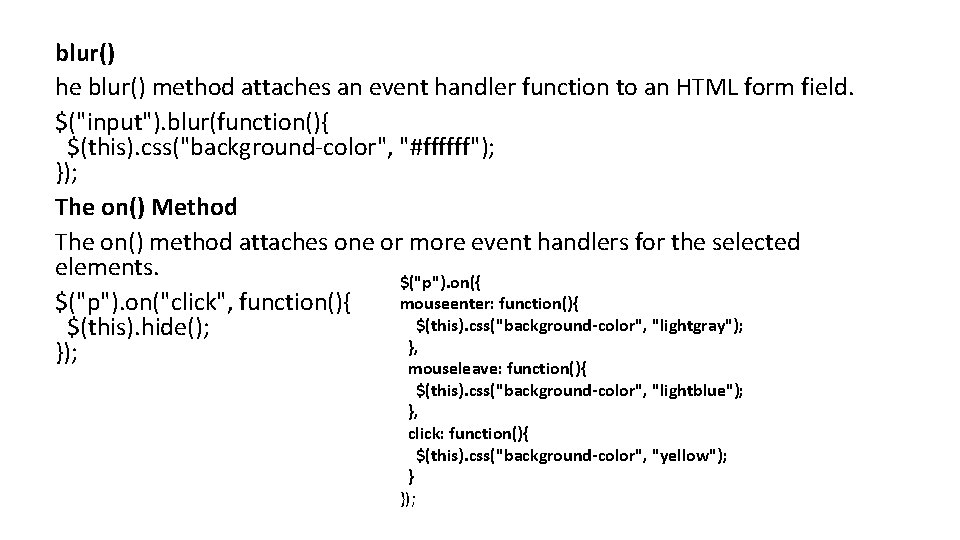
blur() he blur() method attaches an event handler function to an HTML form field. $("input"). blur(function(){ $(this). css("background-color", "#ffffff"); }); The on() Method The on() method attaches one or more event handlers for the selected elements. $("p"). on({ mouseenter: function(){ $("p"). on("click", function(){ $(this). css("background-color", "lightgray"); $(this). hide(); }, }); mouseleave: function(){ $(this). css("background-color", "lightblue"); }, click: function(){ $(this). css("background-color", "yellow"); } });
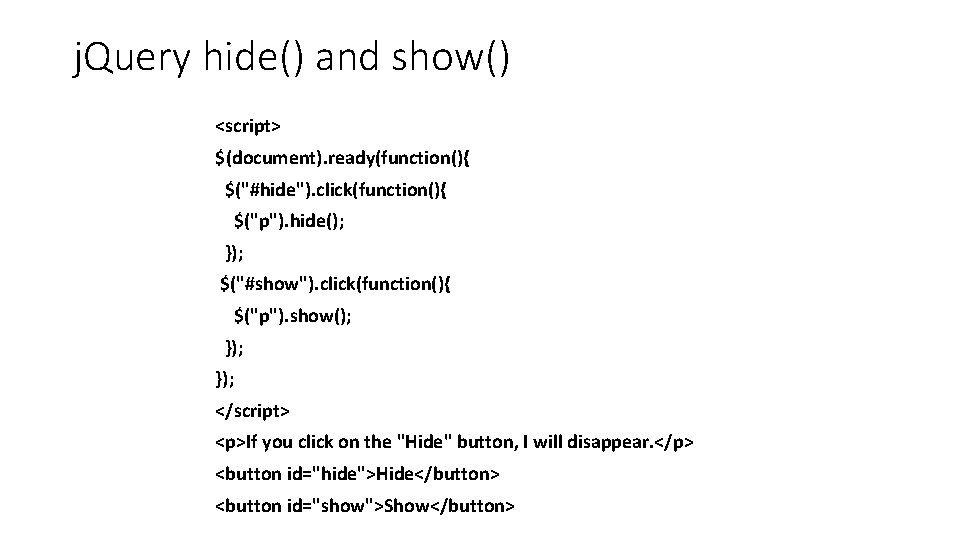
j. Query hide() and show() <script> $(document). ready(function(){ $("#hide"). click(function(){ $("p"). hide(); }); $("#show"). click(function(){ $("p"). show(); }); </script> <p>If you click on the "Hide" button, I will disappear. </p> <button id="hide">Hide</button> <button id="show">Show</button>
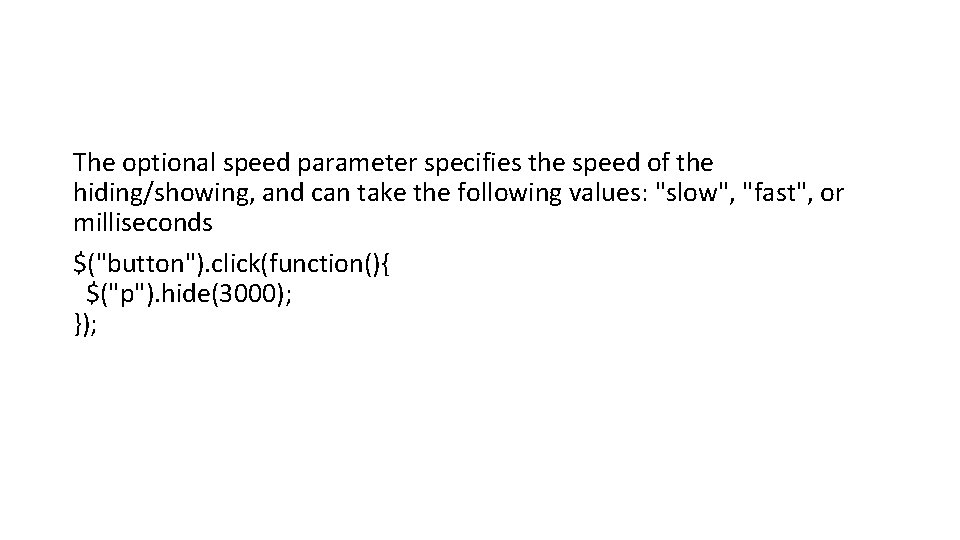
The optional speed parameter specifies the speed of the hiding/showing, and can take the following values: "slow", "fast", or milliseconds $("button"). click(function(){ $("p"). hide(3000); });
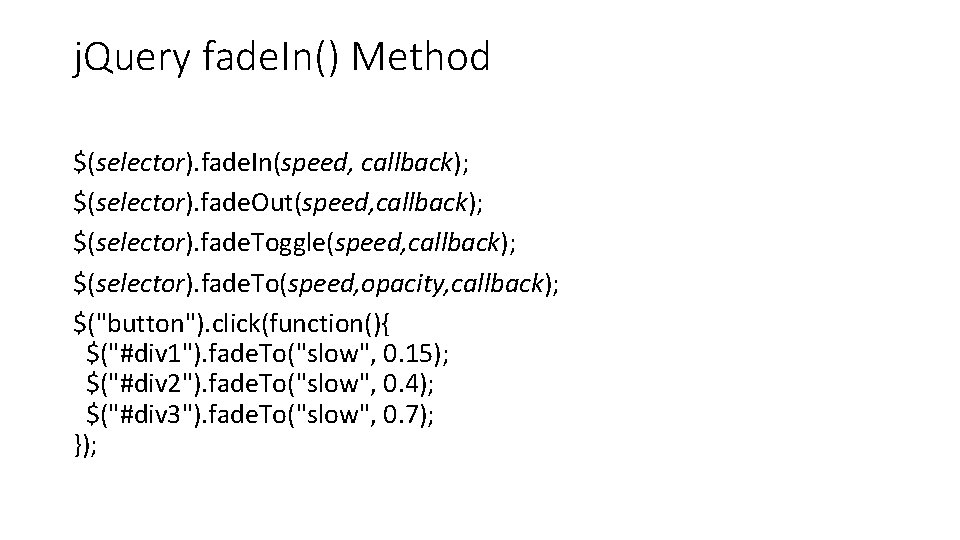
j. Query fade. In() Method $(selector). fade. In(speed, callback); $(selector). fade. Out(speed, callback); $(selector). fade. Toggle(speed, callback); $(selector). fade. To(speed, opacity, callback); $("button"). click(function(){ $("#div 1"). fade. To("slow", 0. 15); $("#div 2"). fade. To("slow", 0. 4); $("#div 3"). fade. To("slow", 0. 7); });
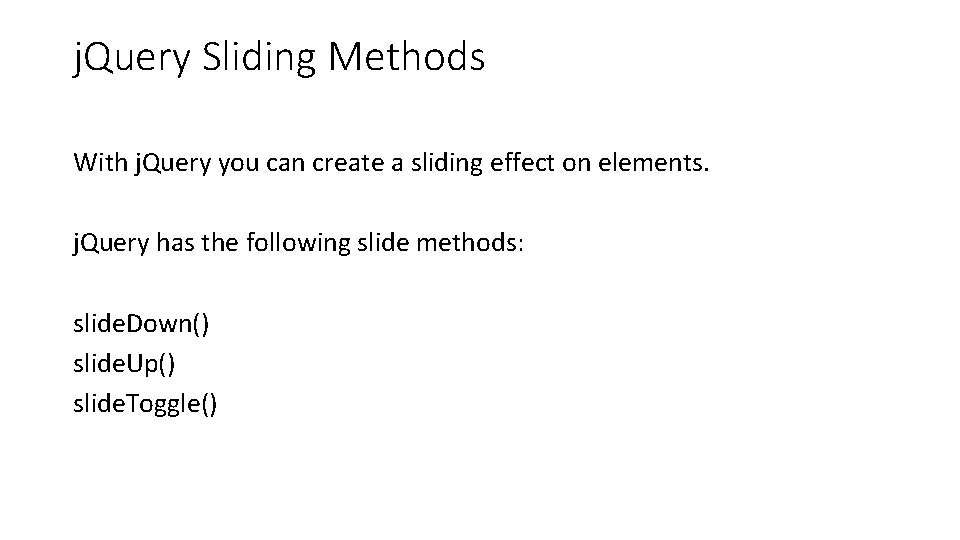
j. Query Sliding Methods With j. Query you can create a sliding effect on elements. j. Query has the following slide methods: slide. Down() slide. Up() slide. Toggle()
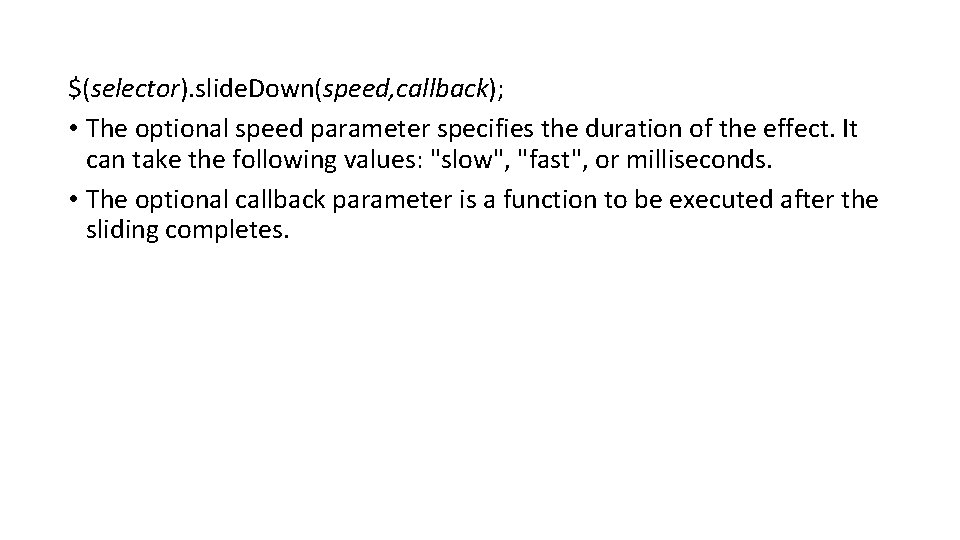
$(selector). slide. Down(speed, callback); • The optional speed parameter specifies the duration of the effect. It can take the following values: "slow", "fast", or milliseconds. • The optional callback parameter is a function to be executed after the sliding completes.
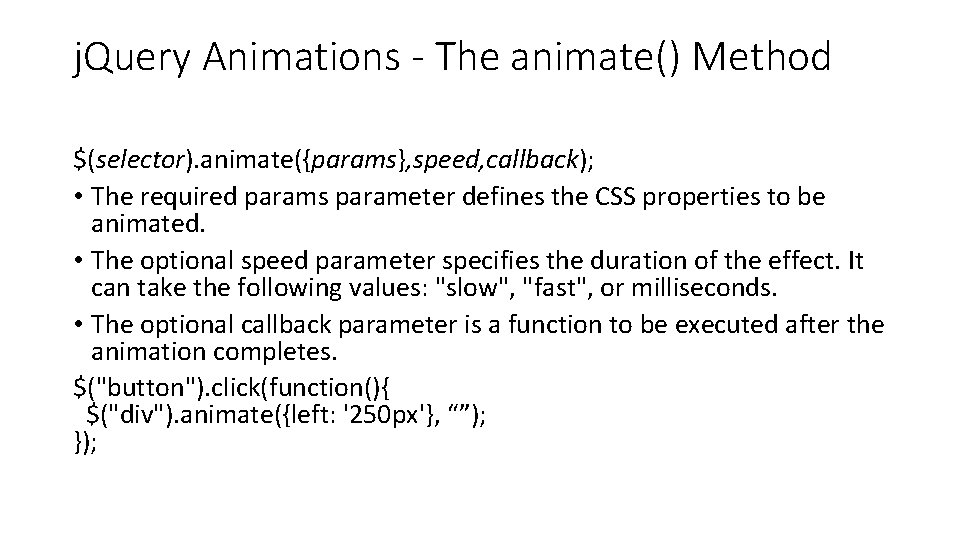
j. Query Animations - The animate() Method $(selector). animate({params}, speed, callback); • The required params parameter defines the CSS properties to be animated. • The optional speed parameter specifies the duration of the effect. It can take the following values: "slow", "fast", or milliseconds. • The optional callback parameter is a function to be executed after the animation completes. $("button"). click(function(){ $("div"). animate({left: '250 px'}, “”); });
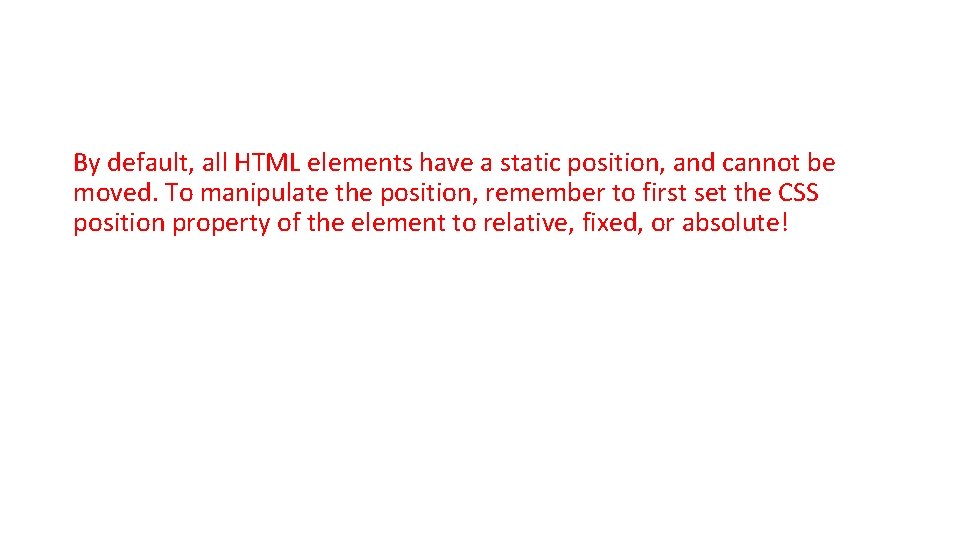
By default, all HTML elements have a static position, and cannot be moved. To manipulate the position, remember to first set the CSS position property of the element to relative, fixed, or absolute!
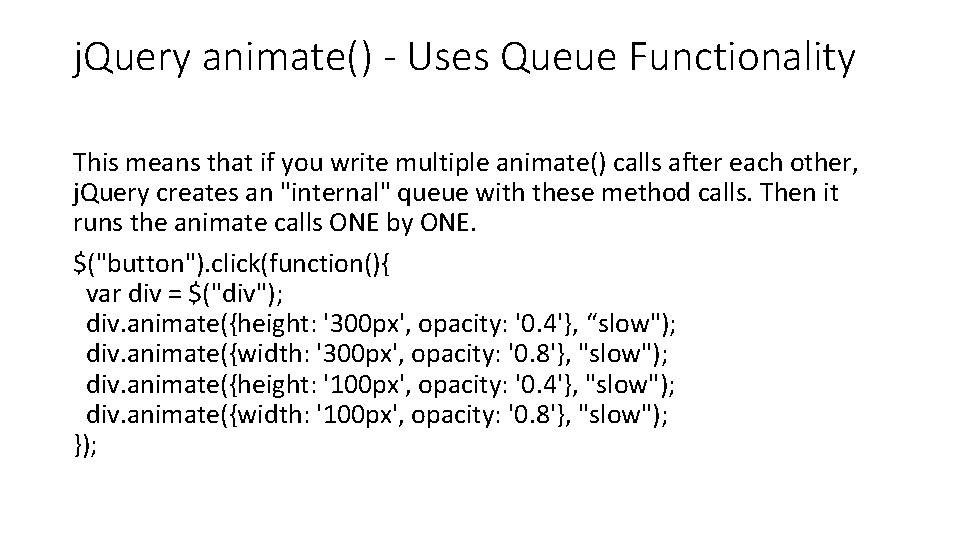
j. Query animate() - Uses Queue Functionality This means that if you write multiple animate() calls after each other, j. Query creates an "internal" queue with these method calls. Then it runs the animate calls ONE by ONE. $("button"). click(function(){ var div = $("div"); div. animate({height: '300 px', opacity: '0. 4'}, “slow"); div. animate({width: '300 px', opacity: '0. 8'}, "slow"); div. animate({height: '100 px', opacity: '0. 4'}, "slow"); div. animate({width: '100 px', opacity: '0. 8'}, "slow"); });
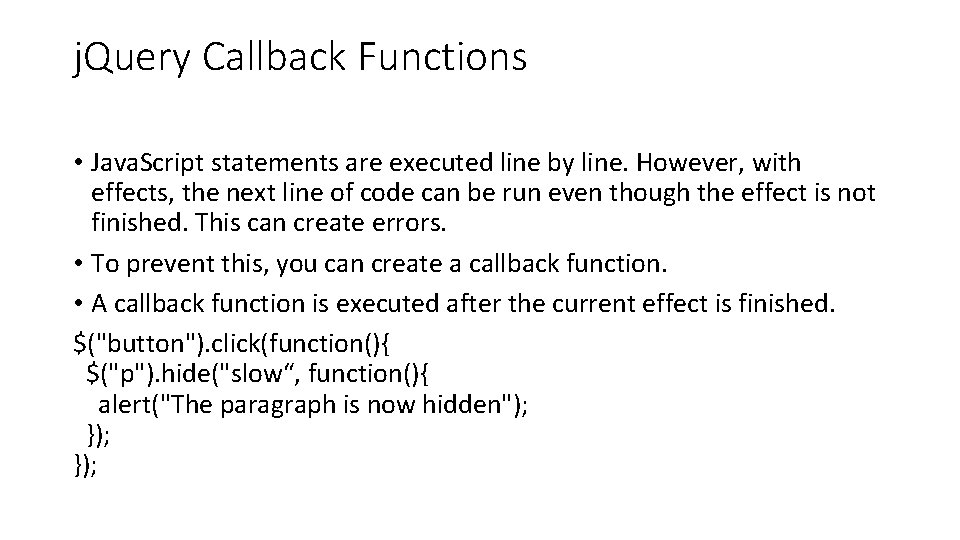
j. Query Callback Functions • Java. Script statements are executed line by line. However, with effects, the next line of code can be run even though the effect is not finished. This can create errors. • To prevent this, you can create a callback function. • A callback function is executed after the current effect is finished. $("button"). click(function(){ $("p"). hide("slow“, function(){ alert("The paragraph is now hidden"); });
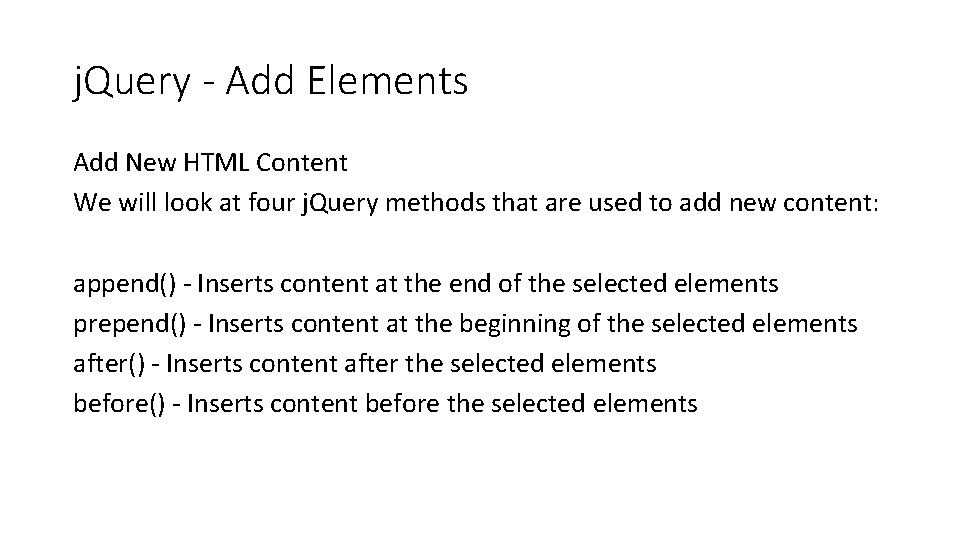
j. Query - Add Elements Add New HTML Content We will look at four j. Query methods that are used to add new content: append() - Inserts content at the end of the selected elements prepend() - Inserts content at the beginning of the selected elements after() - Inserts content after the selected elements before() - Inserts content before the selected elements
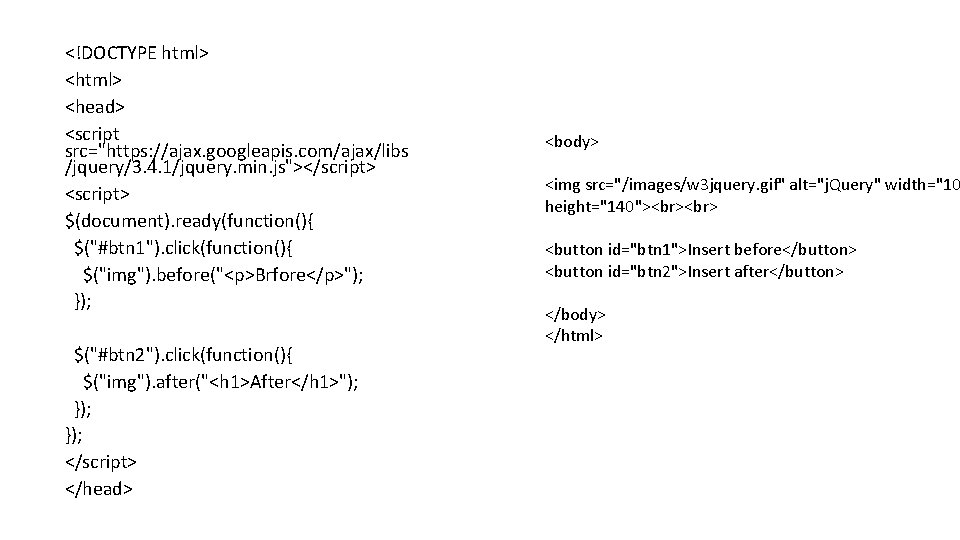
<!DOCTYPE html> <head> <script src="https: //ajax. googleapis. com/ajax/libs /jquery/3. 4. 1/jquery. min. js"></script> <script> $(document). ready(function(){ $("#btn 1"). click(function(){ $("img"). before("<p>Brfore</p>"); }); $("#btn 2"). click(function(){ $("img"). after("<h 1>After</h 1>"); }); </script> </head> <body> <img src="/images/w 3 jquery. gif" alt="j. Query" width="10 height="140"> <button id="btn 1">Insert before</button> <button id="btn 2">Insert after</button> </body> </html>
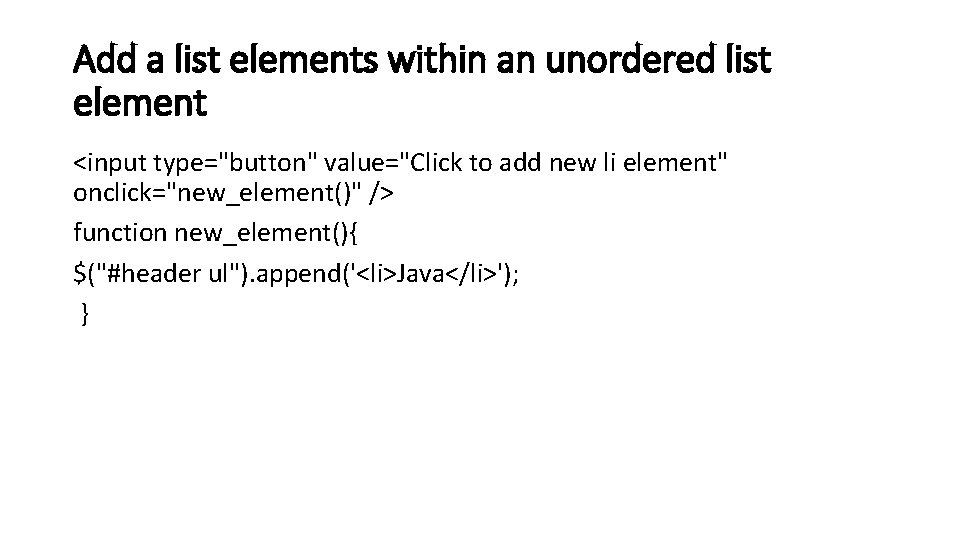
Add a list elements within an unordered list element <input type="button" value="Click to add new li element" onclick="new_element()" /> function new_element(){ $("#header ul"). append('<li>Java</li>'); }
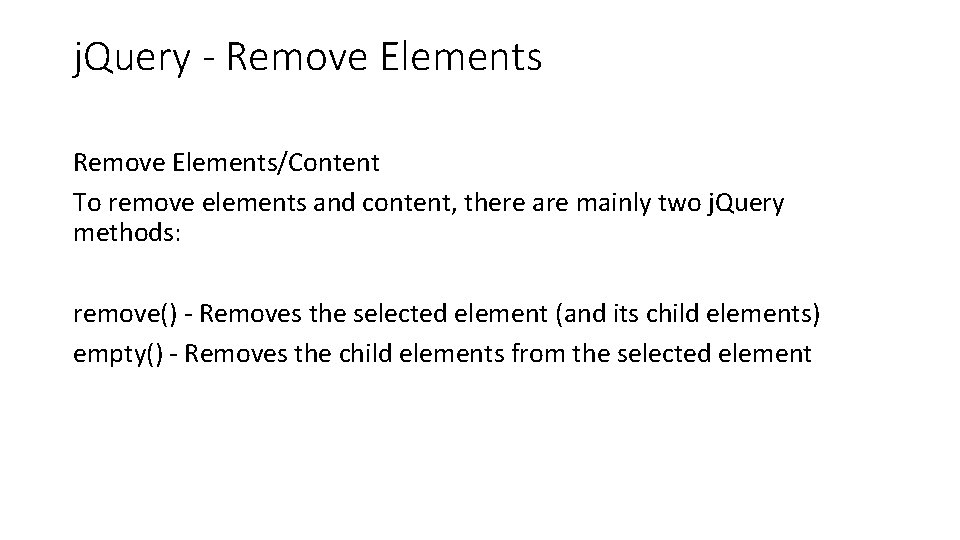
j. Query - Remove Elements/Content To remove elements and content, there are mainly two j. Query methods: remove() - Removes the selected element (and its child elements) empty() - Removes the child elements from the selected element
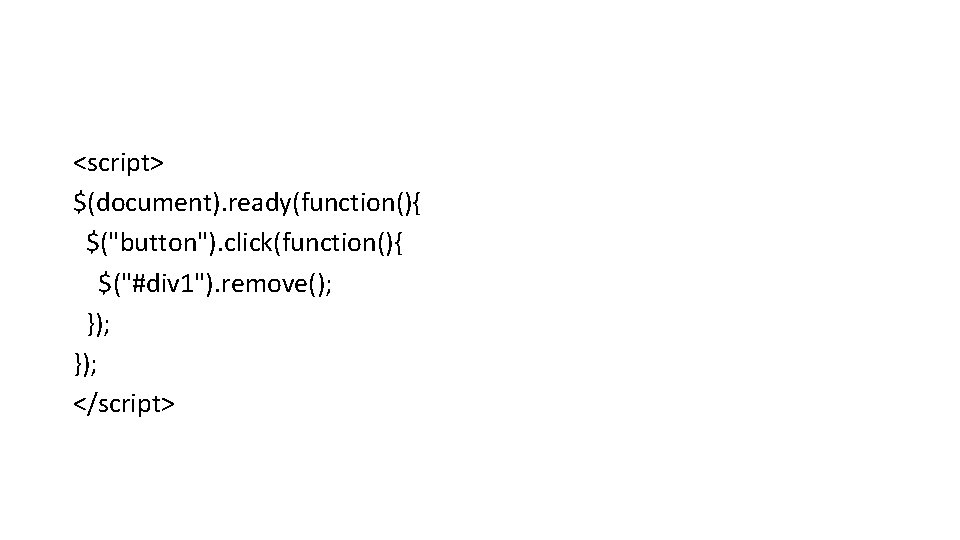
<script> $(document). ready(function(){ $("button"). click(function(){ $("#div 1"). remove(); }); </script>
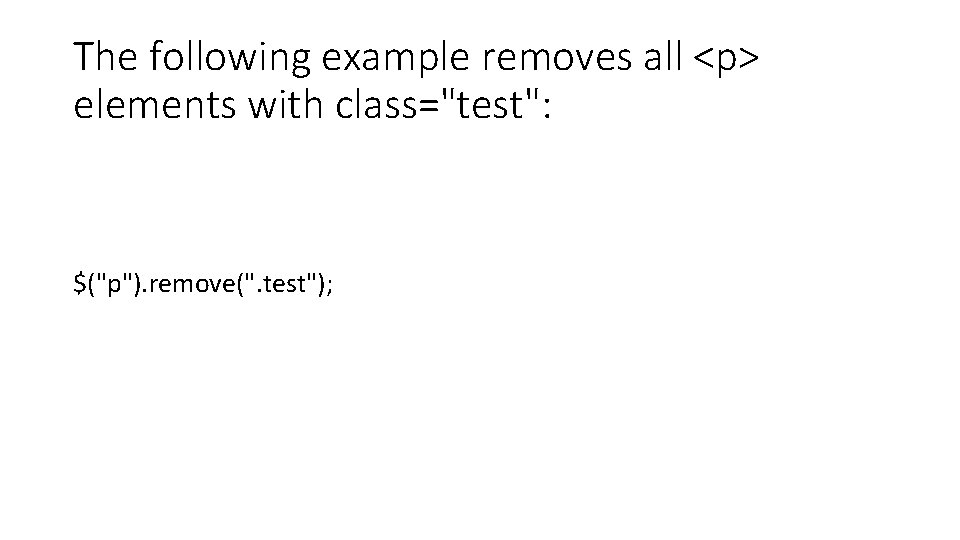
The following example removes all <p> elements with class="test": $("p"). remove(". test");
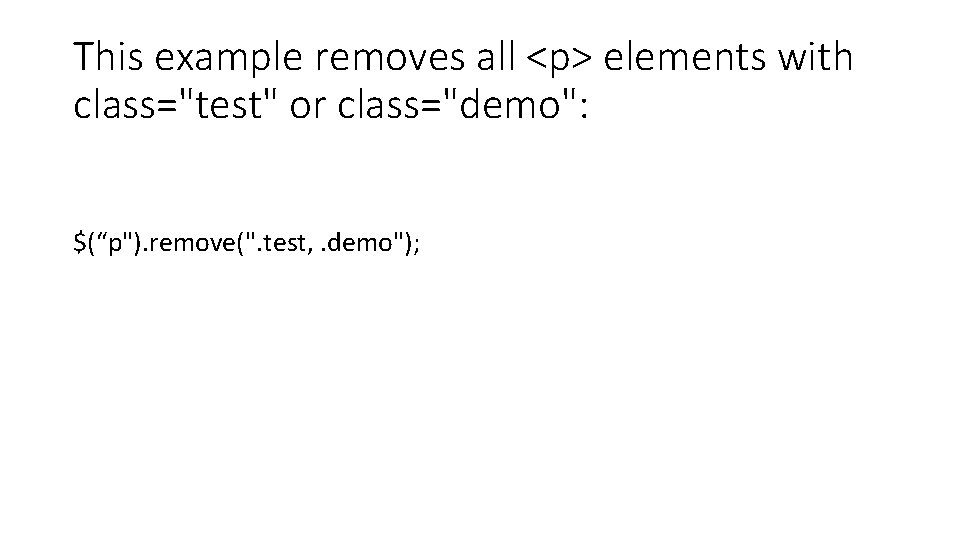
This example removes all <p> elements with class="test" or class="demo": $(“p"). remove(". test, . demo");
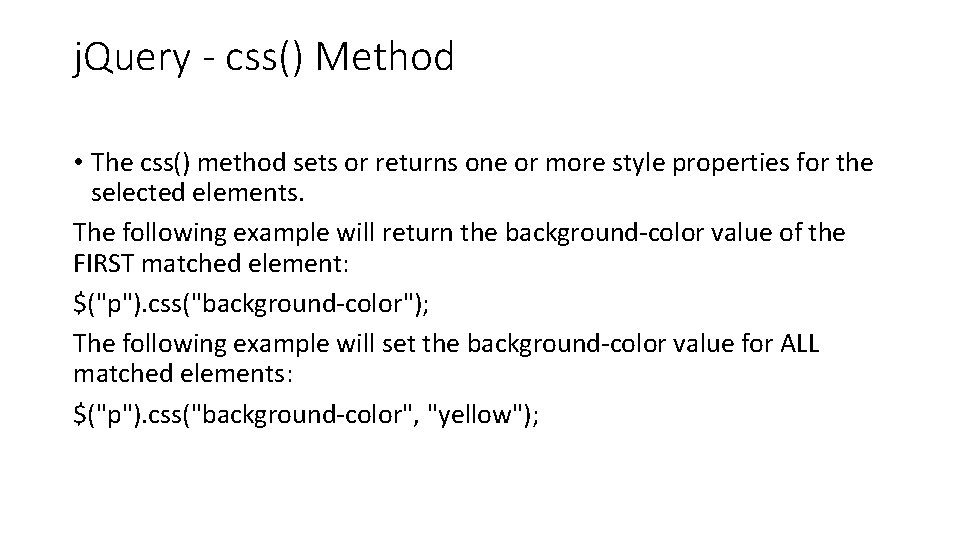
j. Query - css() Method • The css() method sets or returns one or more style properties for the selected elements. The following example will return the background-color value of the FIRST matched element: $("p"). css("background-color"); The following example will set the background-color value for ALL matched elements: $("p"). css("background-color", "yellow");
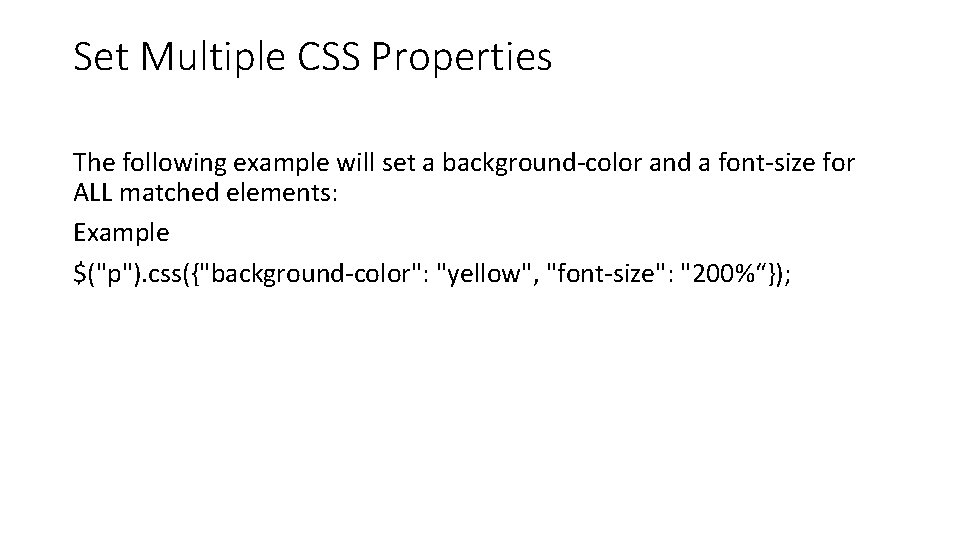
Set Multiple CSS Properties The following example will set a background-color and a font-size for ALL matched elements: Example $("p"). css({"background-color": "yellow", "font-size": "200%“});
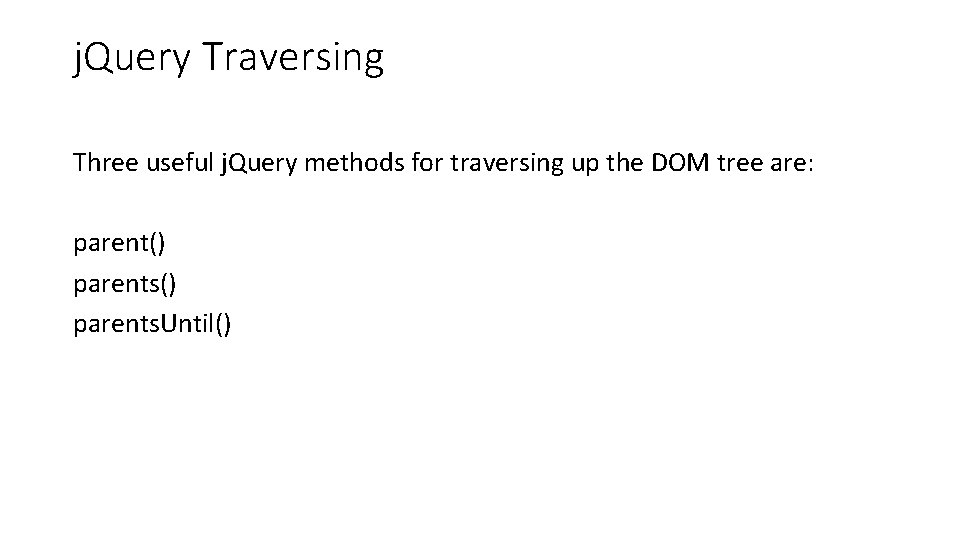
j. Query Traversing Three useful j. Query methods for traversing up the DOM tree are: parent() parents. Until()
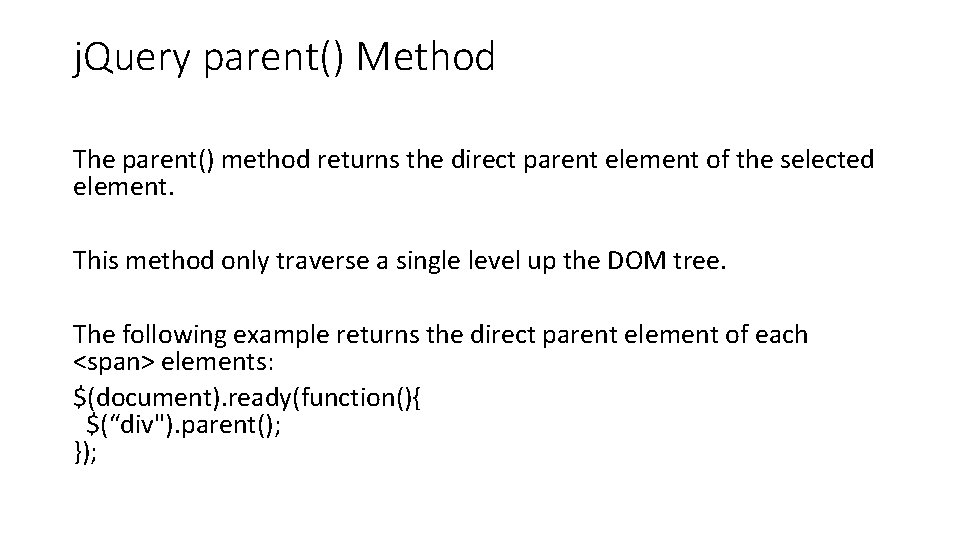
j. Query parent() Method The parent() method returns the direct parent element of the selected element. This method only traverse a single level up the DOM tree. The following example returns the direct parent element of each <span> elements: $(document). ready(function(){ $(“div"). parent(); });
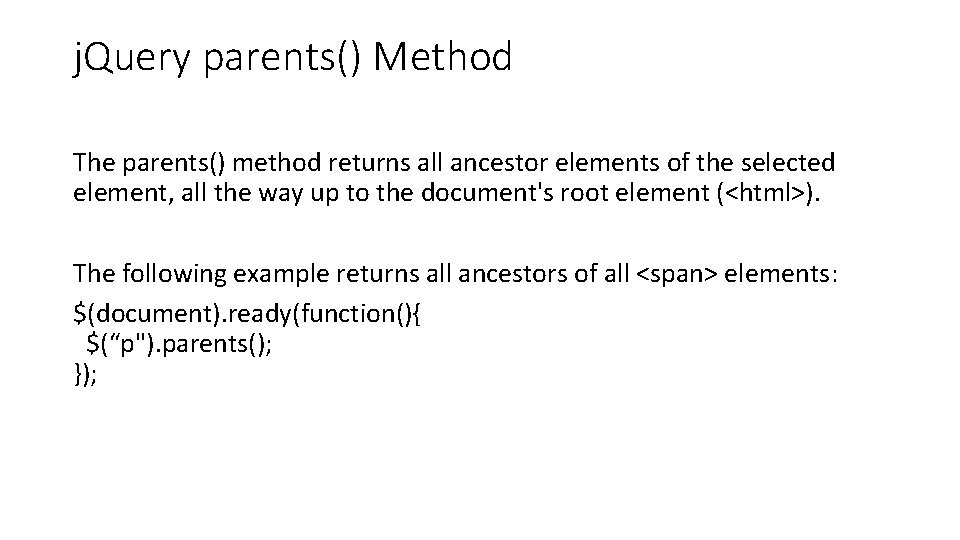
j. Query parents() Method The parents() method returns all ancestor elements of the selected element, all the way up to the document's root element (<html>). The following example returns all ancestors of all <span> elements: $(document). ready(function(){ $(“p"). parents(); });
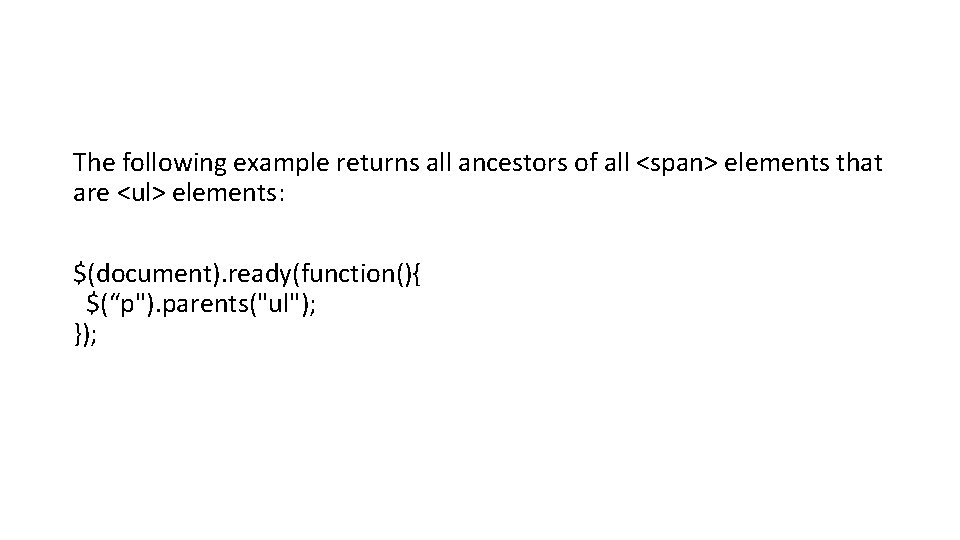
The following example returns all ancestors of all <span> elements that are <ul> elements: $(document). ready(function(){ $(“p"). parents("ul"); });
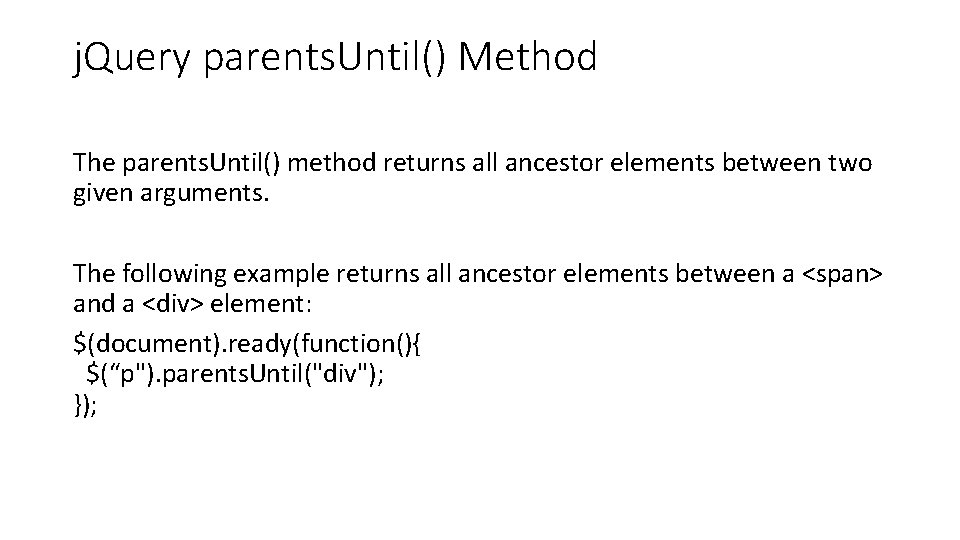
j. Query parents. Until() Method The parents. Until() method returns all ancestor elements between two given arguments. The following example returns all ancestor elements between a <span> and a <div> element: $(document). ready(function(){ $(“p"). parents. Until("div"); });
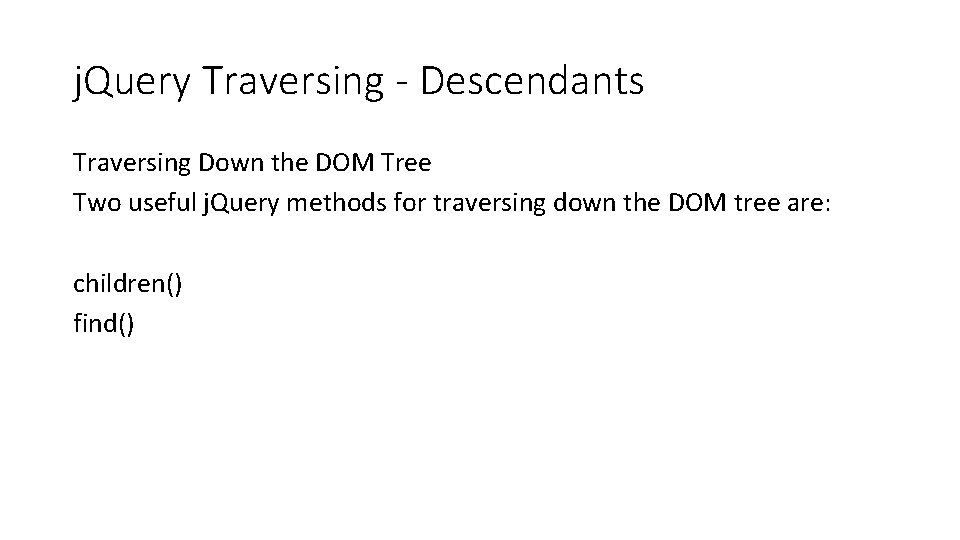
j. Query Traversing - Descendants Traversing Down the DOM Tree Two useful j. Query methods for traversing down the DOM tree are: children() find()
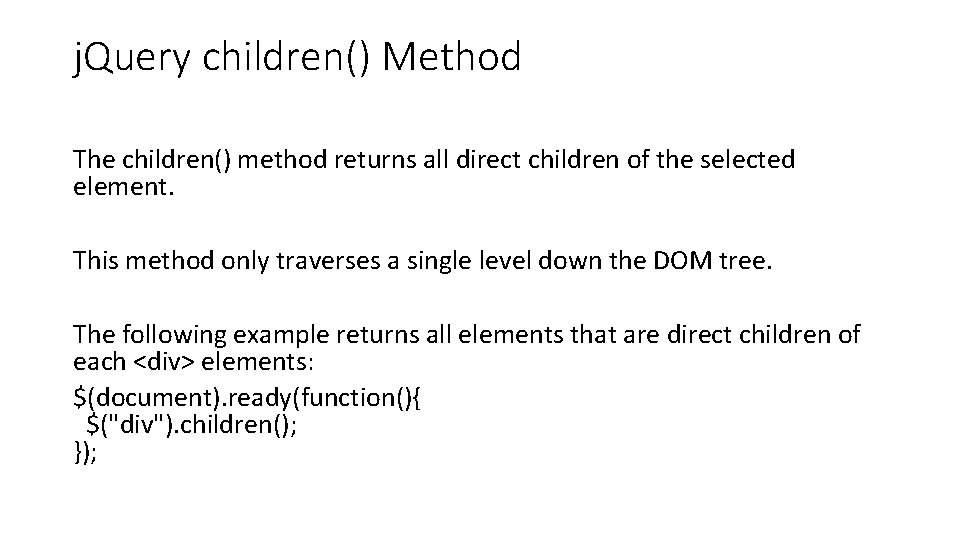
j. Query children() Method The children() method returns all direct children of the selected element. This method only traverses a single level down the DOM tree. The following example returns all elements that are direct children of each <div> elements: $(document). ready(function(){ $("div"). children(); });
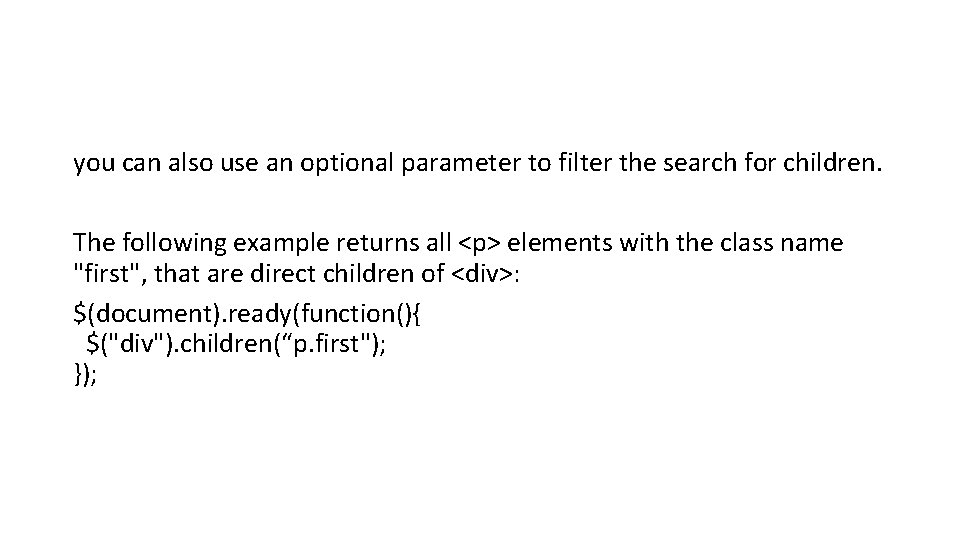
you can also use an optional parameter to filter the search for children. The following example returns all <p> elements with the class name "first", that are direct children of <div>: $(document). ready(function(){ $("div"). children(“p. first"); });
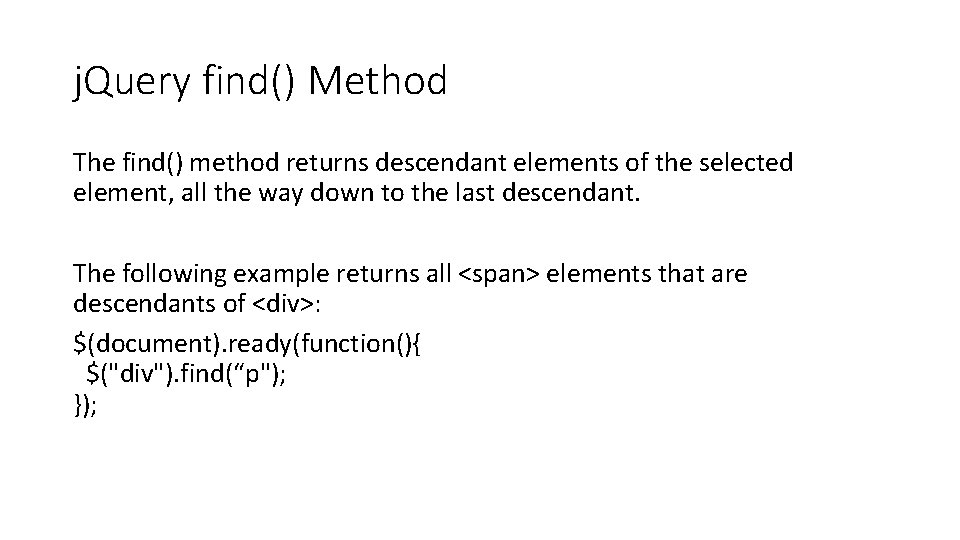
j. Query find() Method The find() method returns descendant elements of the selected element, all the way down to the last descendant. The following example returns all <span> elements that are descendants of <div>: $(document). ready(function(){ $("div"). find(“p"); });
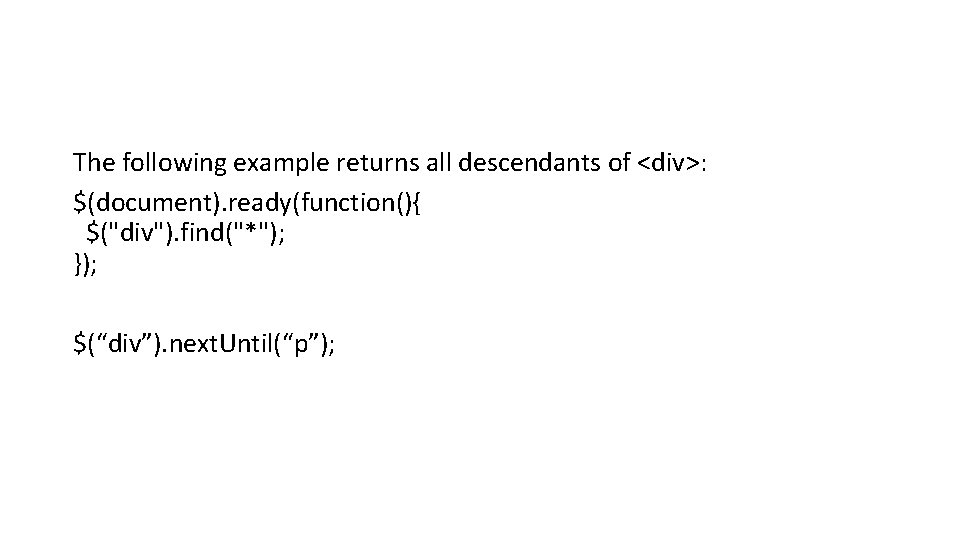
The following example returns all descendants of <div>: $(document). ready(function(){ $("div"). find("*"); }); $(“div”). next. Until(“p”);
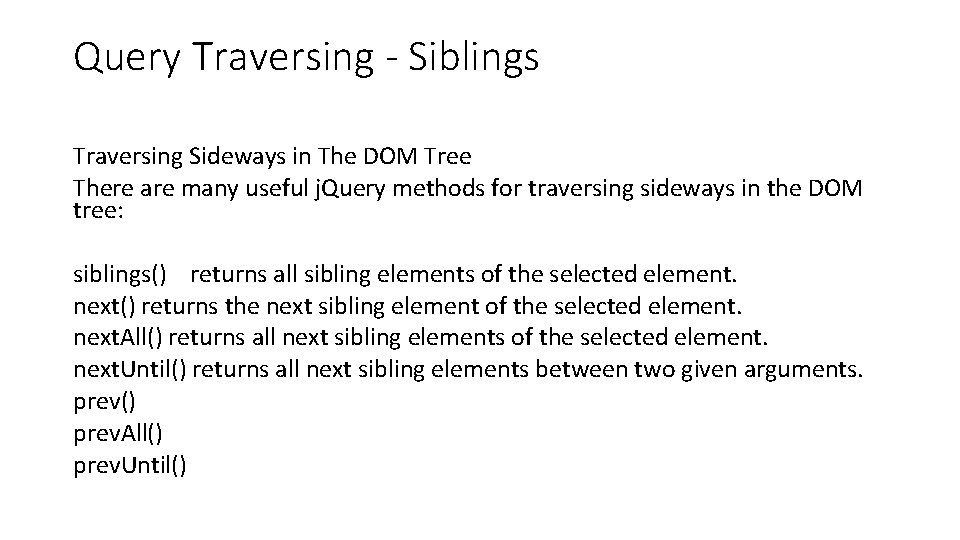
Query Traversing - Siblings Traversing Sideways in The DOM Tree There are many useful j. Query methods for traversing sideways in the DOM tree: siblings() returns all sibling elements of the selected element. next() returns the next sibling element of the selected element. next. All() returns all next sibling elements of the selected element. next. Until() returns all next sibling elements between two given arguments. prev() prev. All() prev. Until()
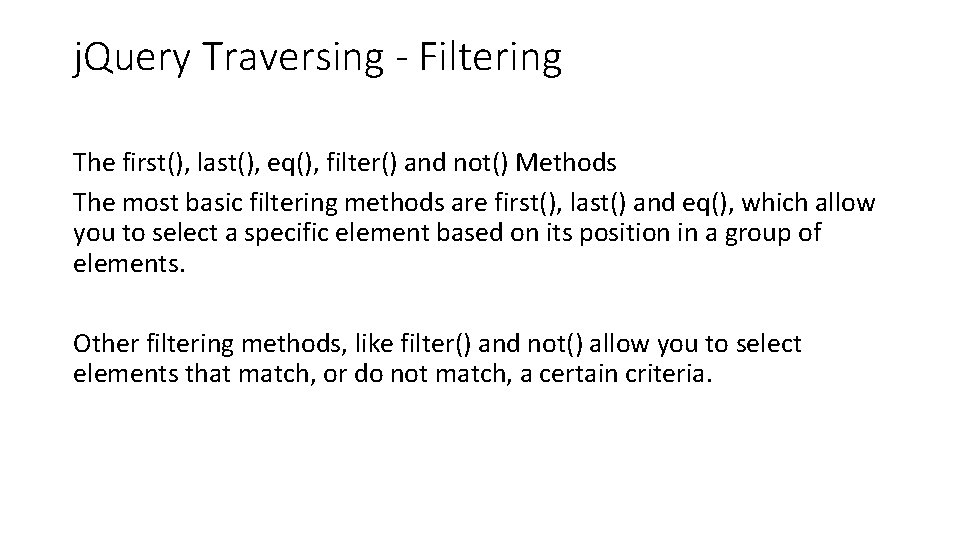
j. Query Traversing - Filtering The first(), last(), eq(), filter() and not() Methods The most basic filtering methods are first(), last() and eq(), which allow you to select a specific element based on its position in a group of elements. Other filtering methods, like filter() and not() allow you to select elements that match, or do not match, a certain criteria.
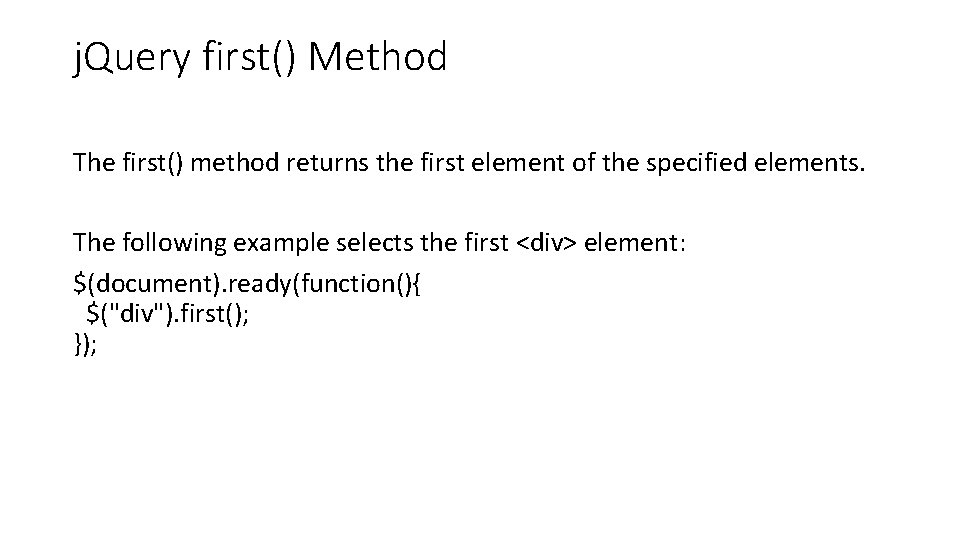
j. Query first() Method The first() method returns the first element of the specified elements. The following example selects the first <div> element: $(document). ready(function(){ $("div"). first(); });
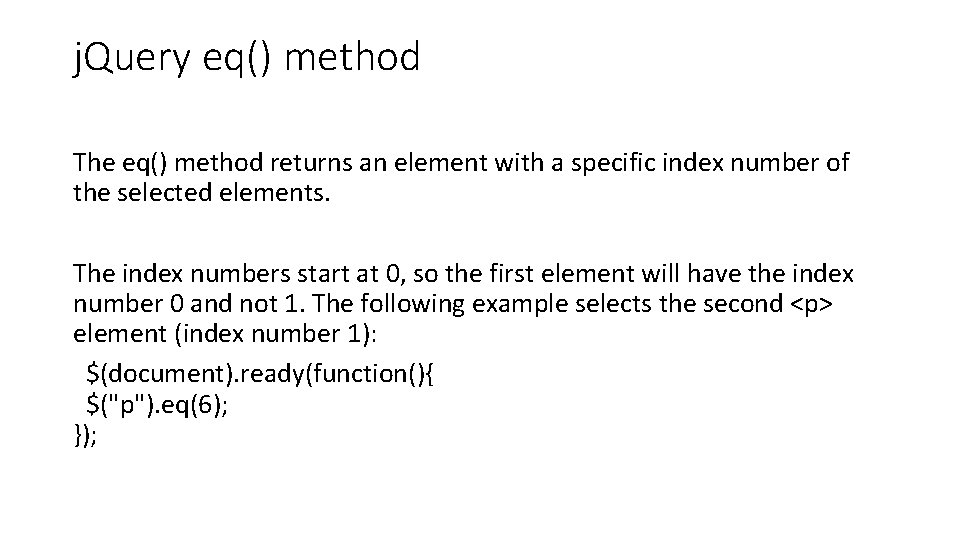
j. Query eq() method The eq() method returns an element with a specific index number of the selected elements. The index numbers start at 0, so the first element will have the index number 0 and not 1. The following example selects the second <p> element (index number 1): $(document). ready(function(){ $("p"). eq(6); });
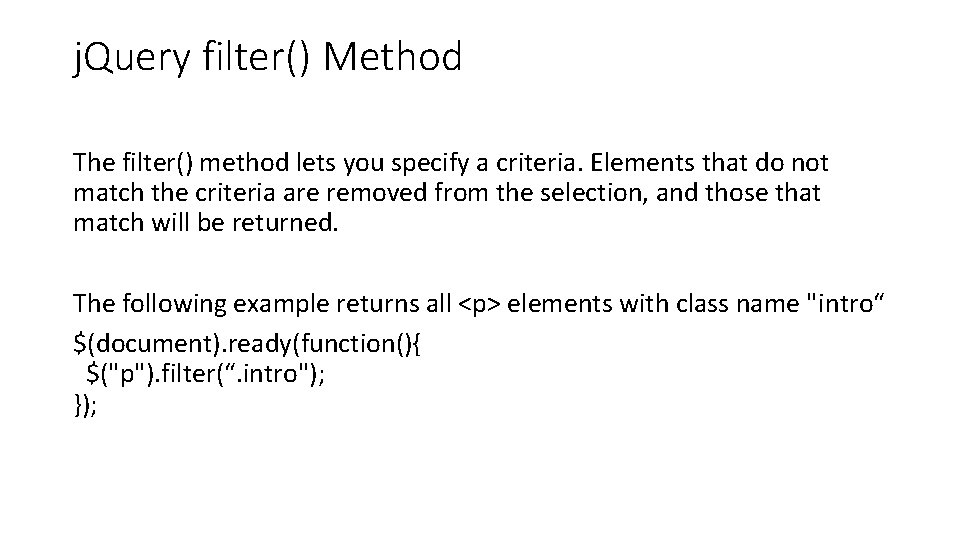
j. Query filter() Method The filter() method lets you specify a criteria. Elements that do not match the criteria are removed from the selection, and those that match will be returned. The following example returns all <p> elements with class name "intro“ $(document). ready(function(){ $("p"). filter(“. intro"); });
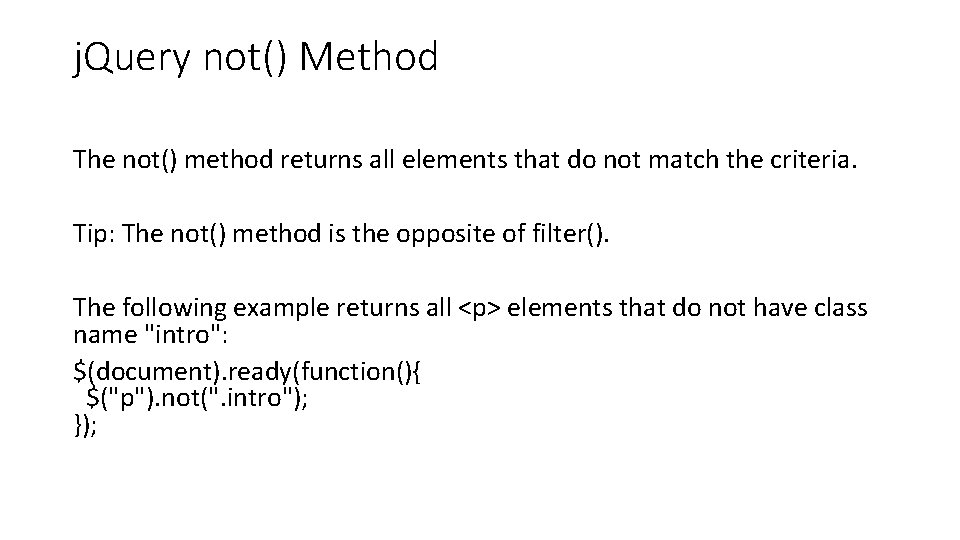
j. Query not() Method The not() method returns all elements that do not match the criteria. Tip: The not() method is the opposite of filter(). The following example returns all <p> elements that do not have class name "intro": $(document). ready(function(){ $("p"). not(". intro"); });