Chapter 6 Shell Programming Shell Scripts Using the
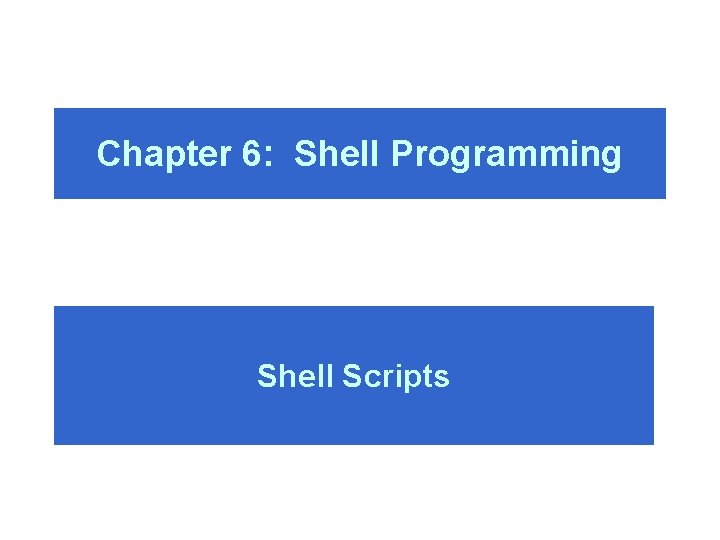
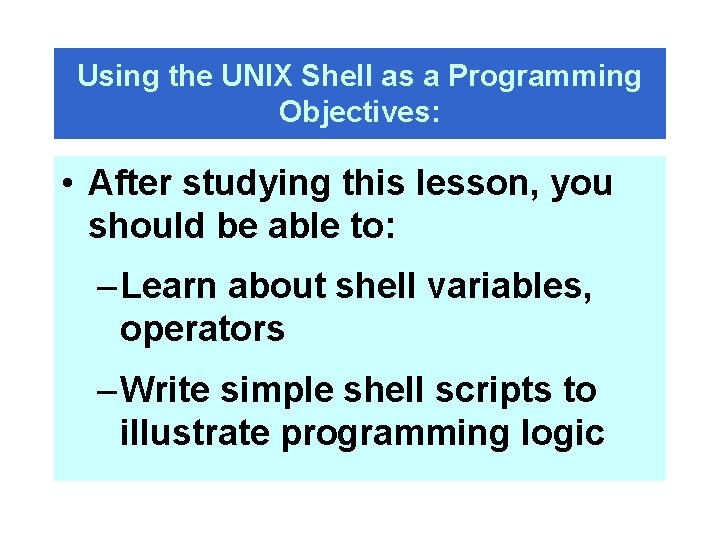
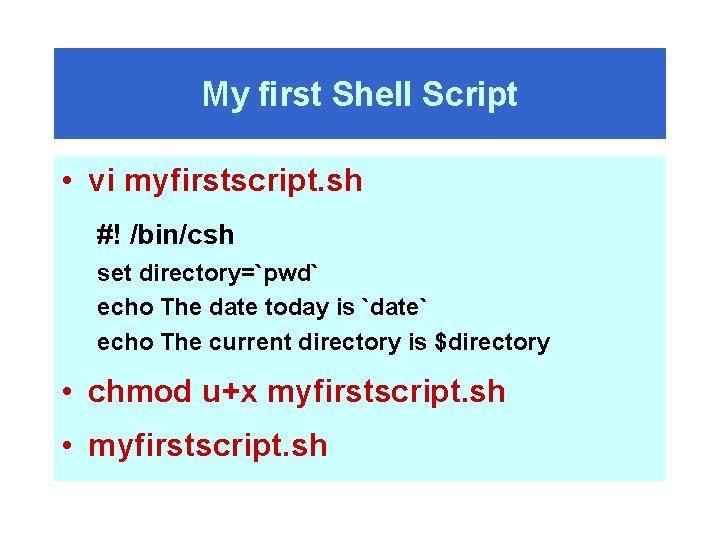
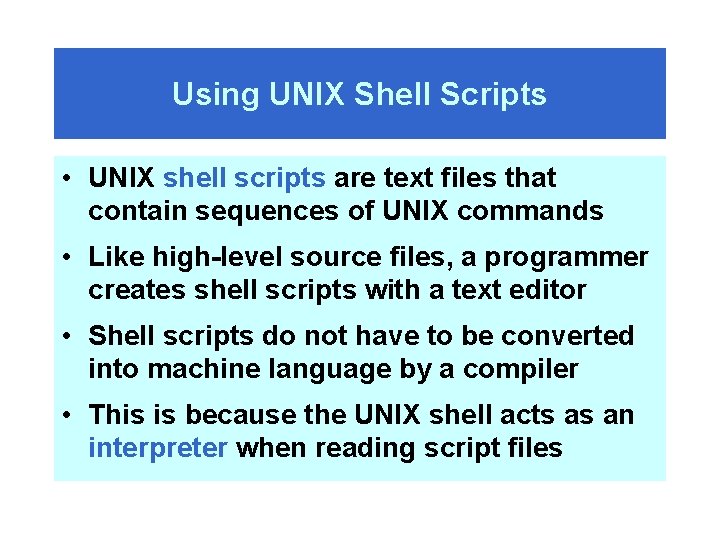
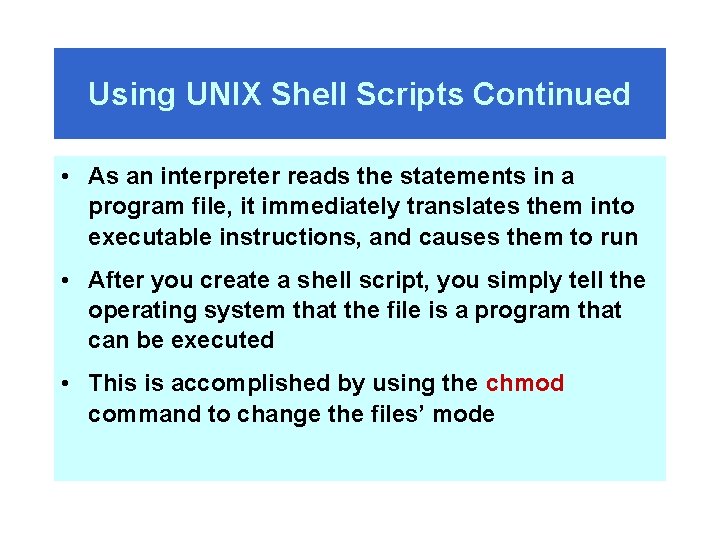
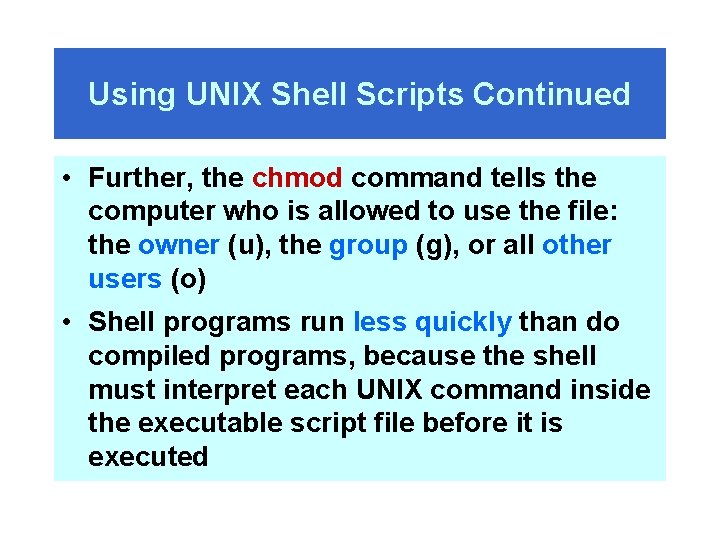
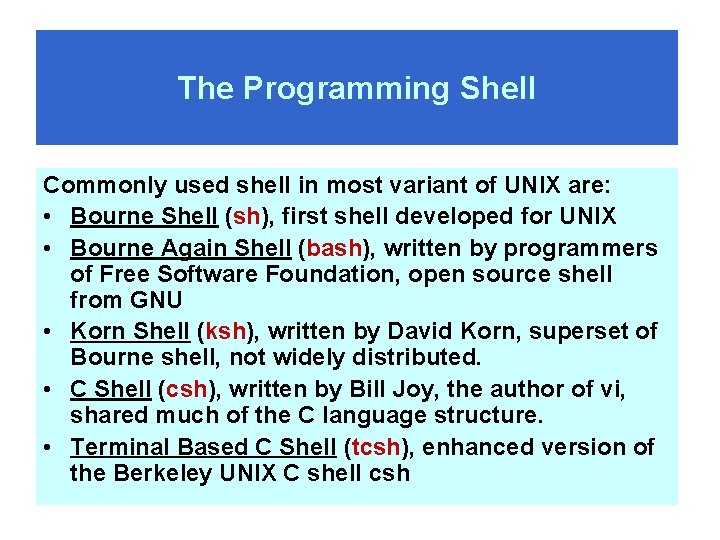
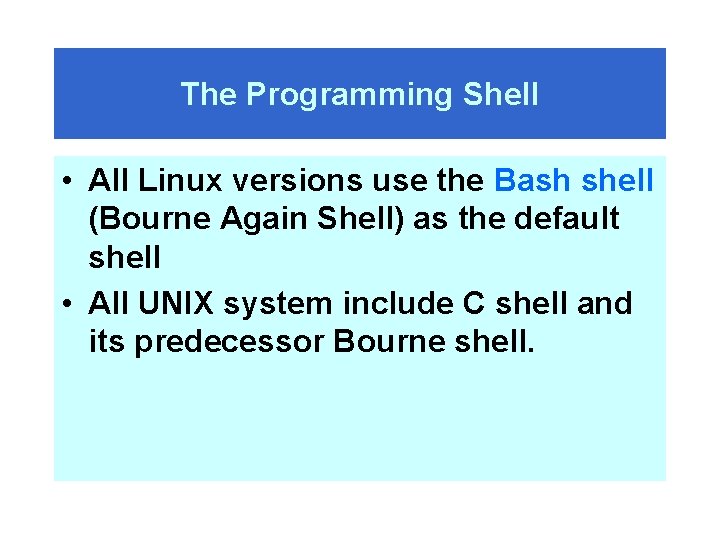
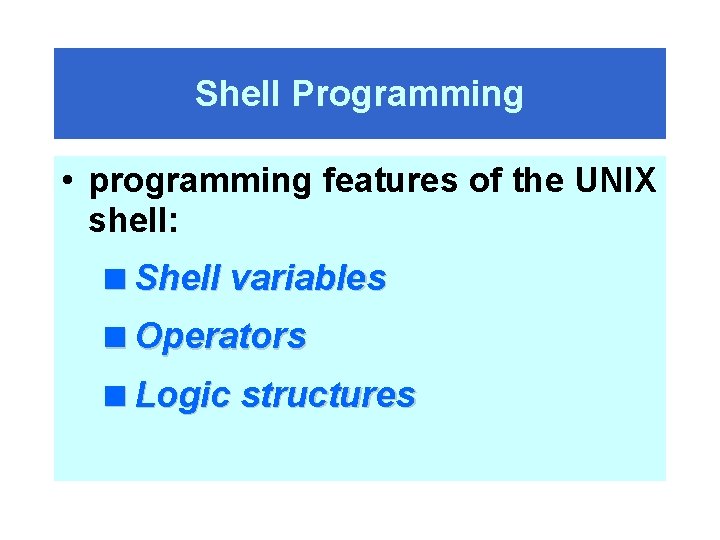
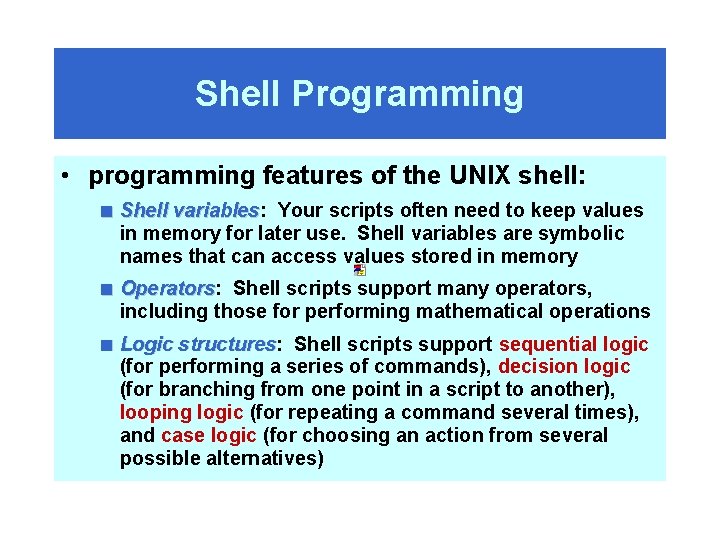
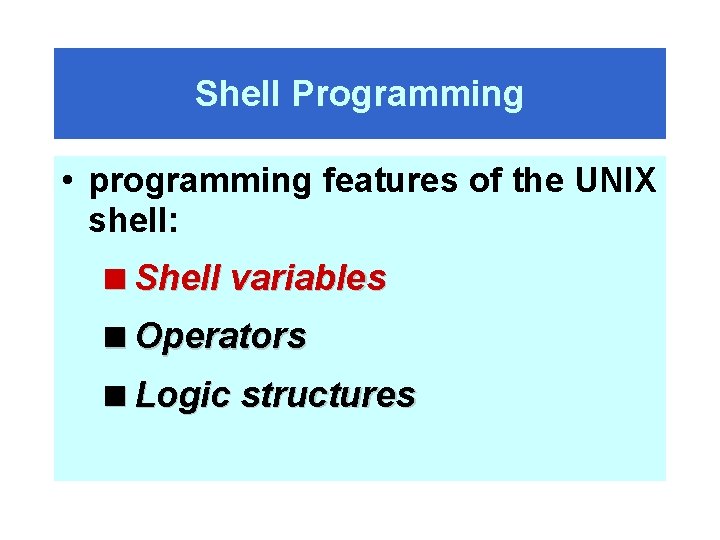
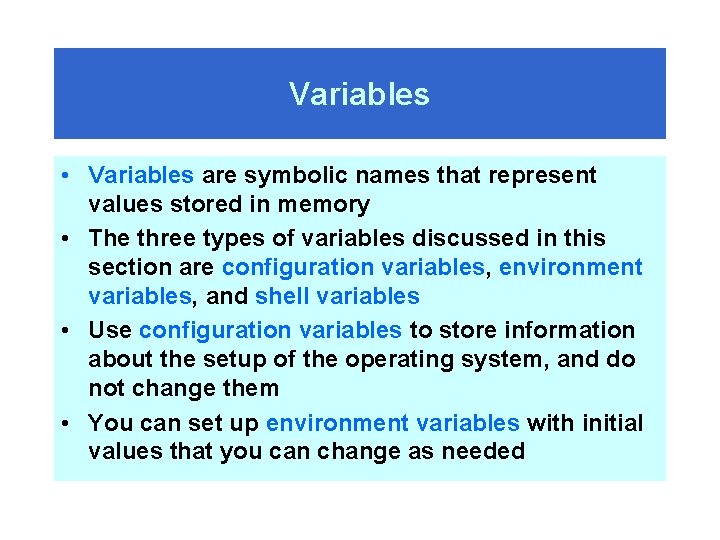
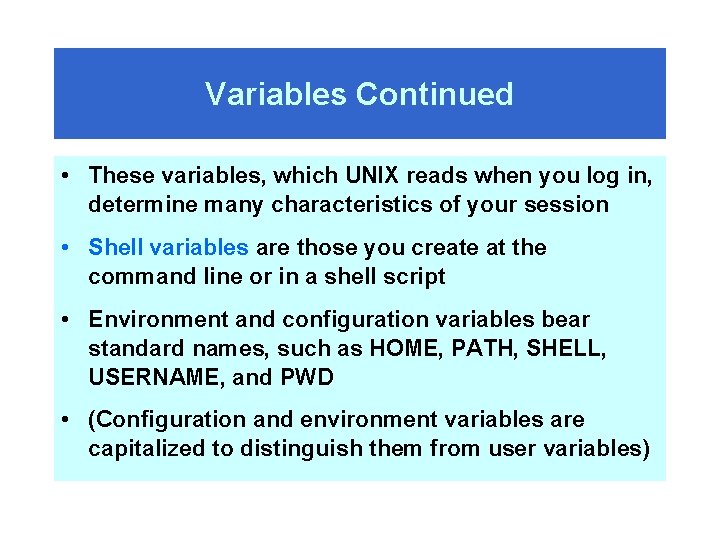
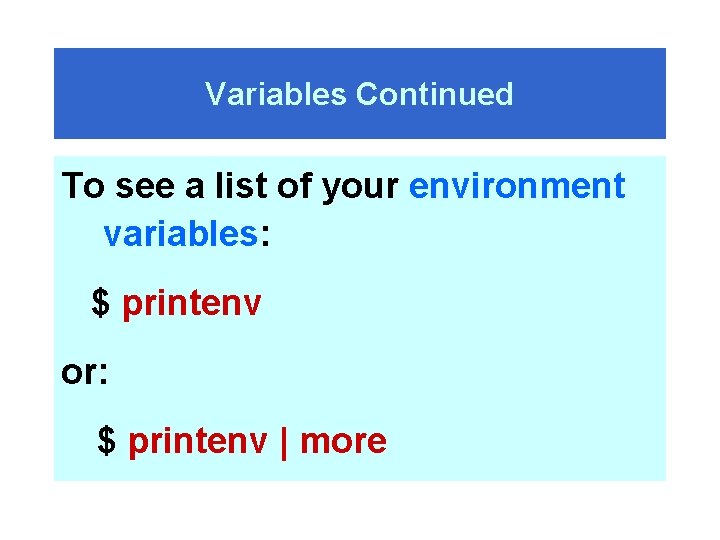
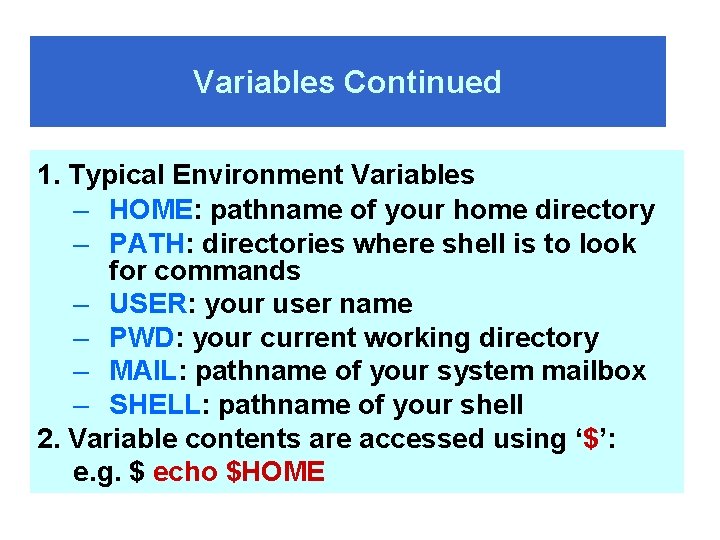
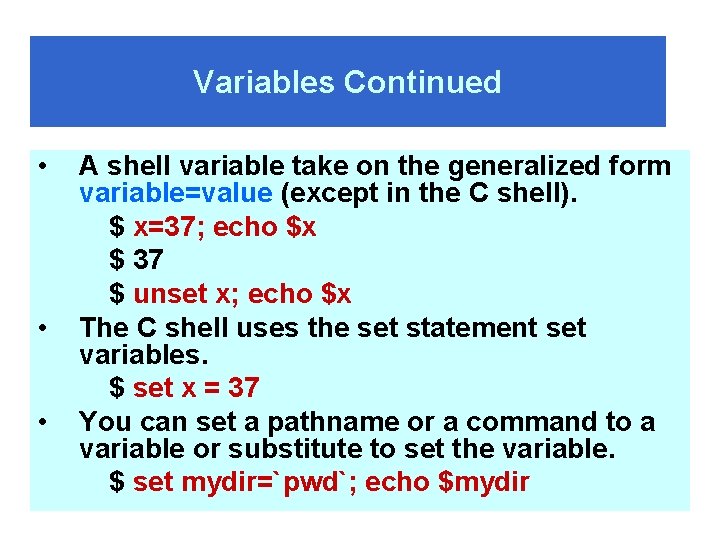
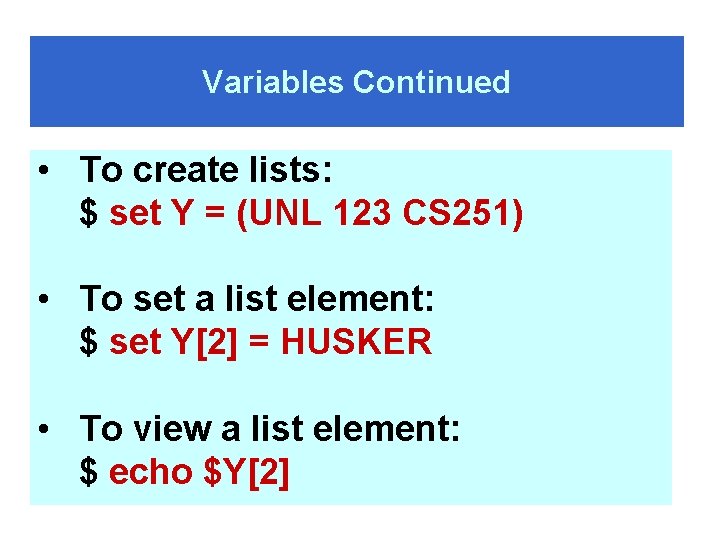
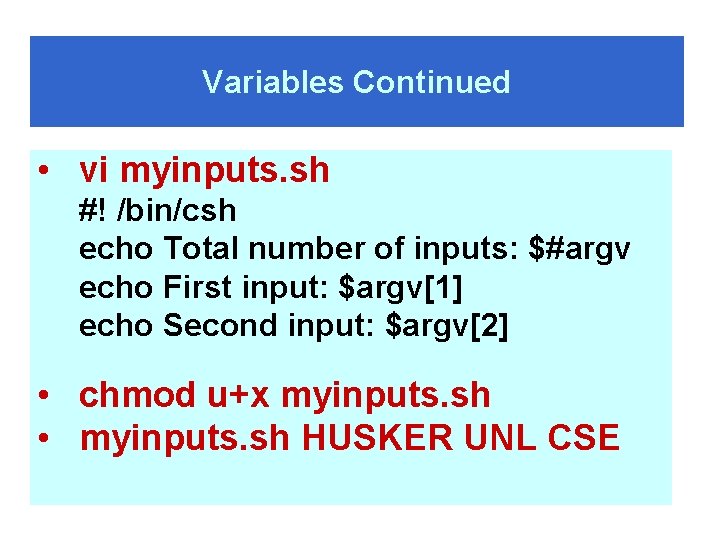
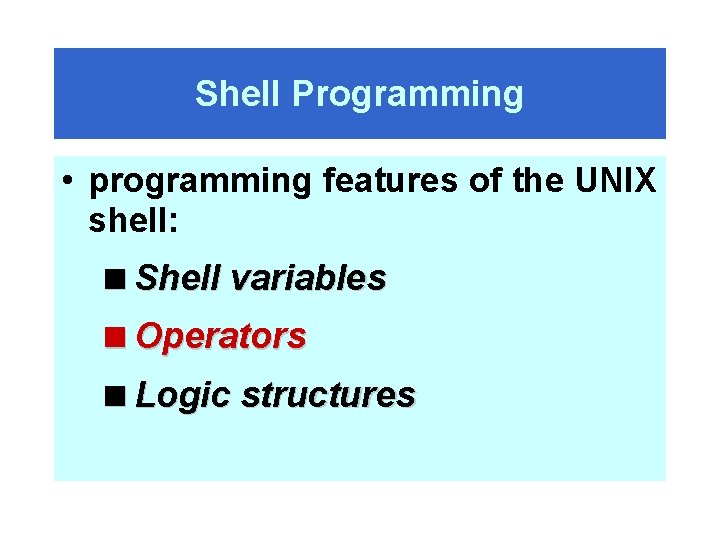
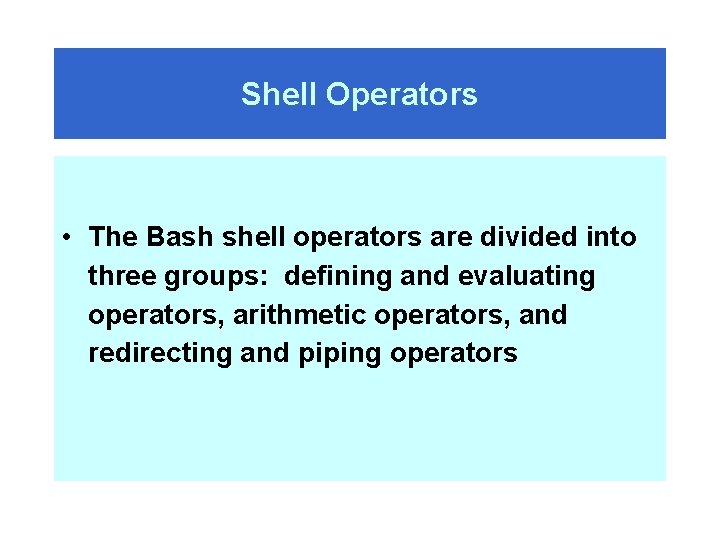
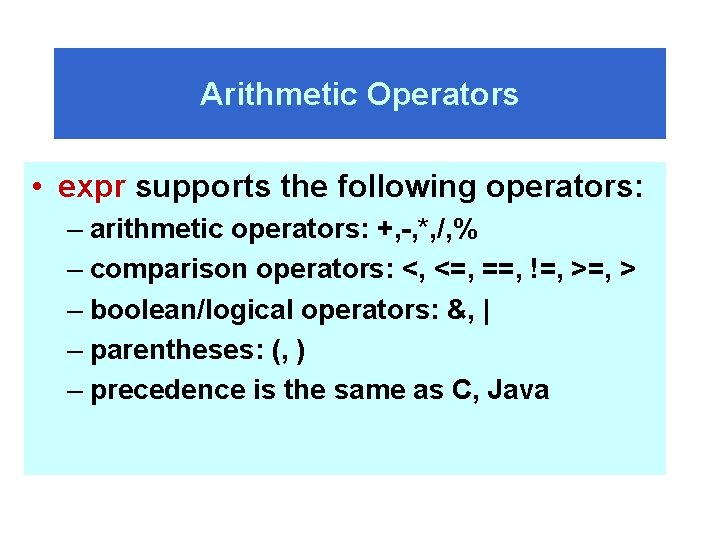
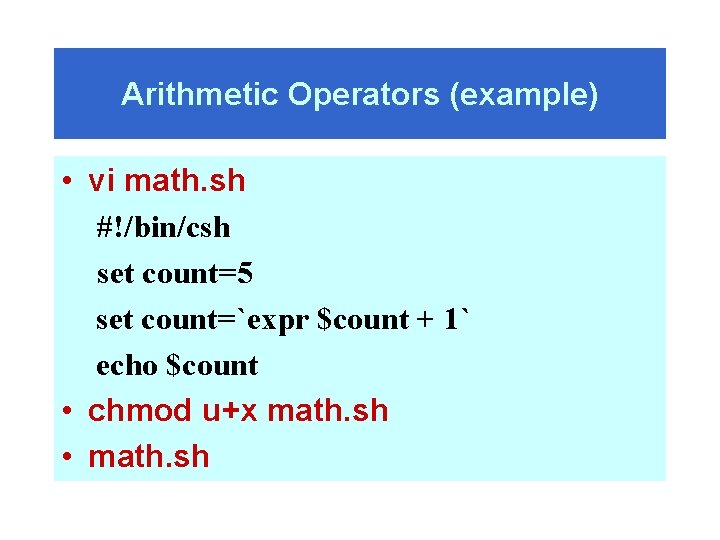
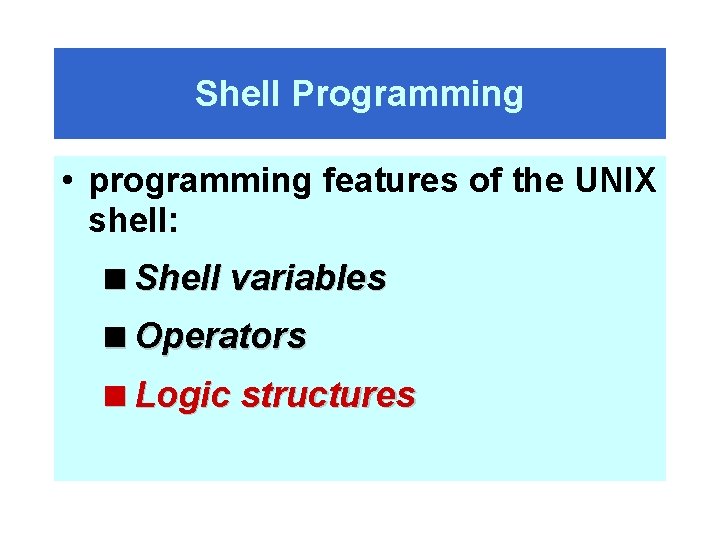
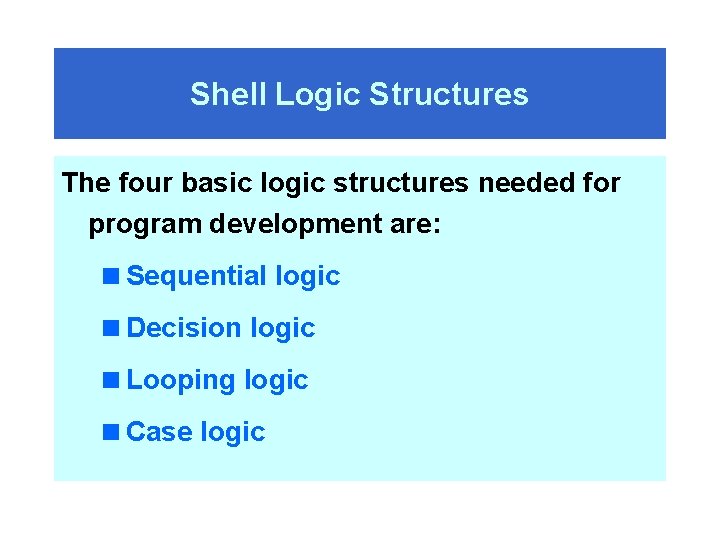
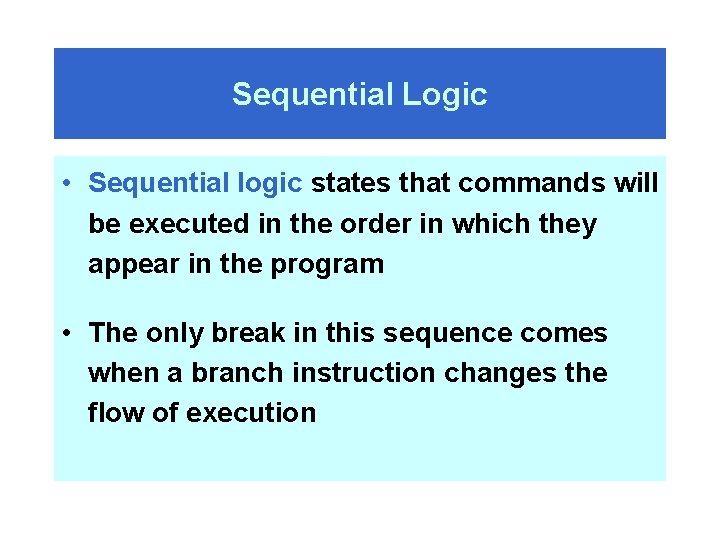
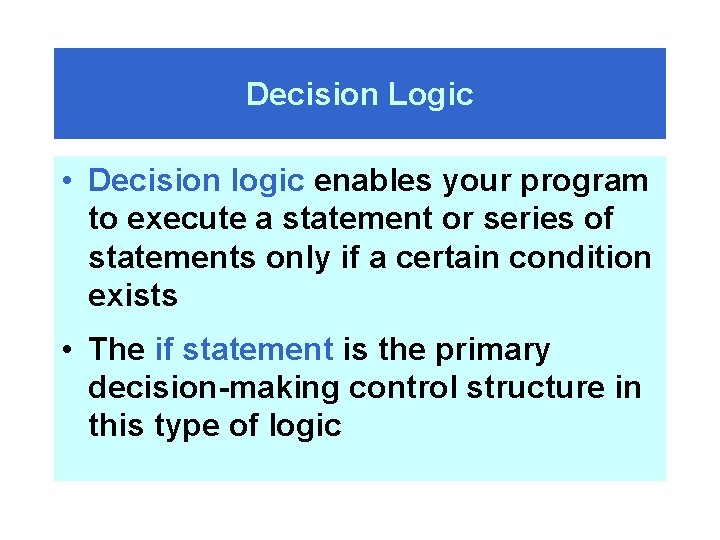
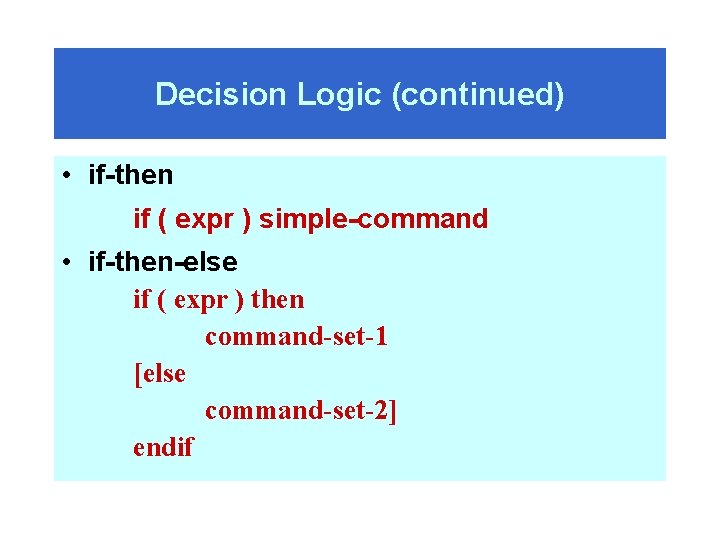
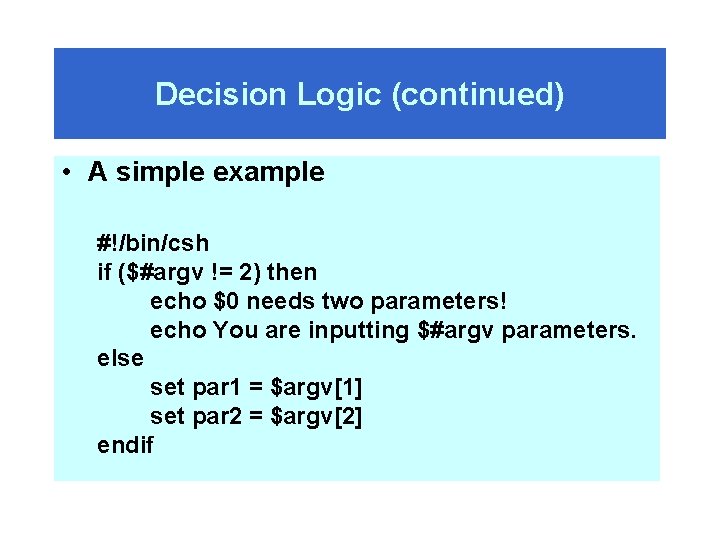
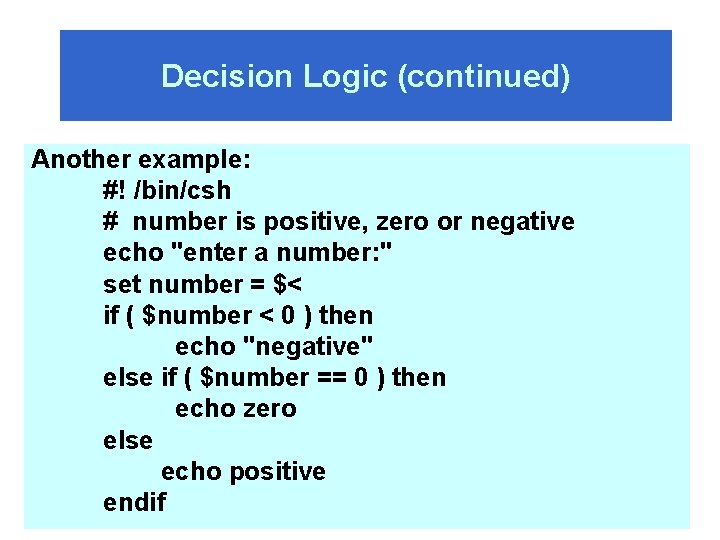
![Decision Logic (continued) Another example: #!/bin/csh if {( grep UNIX $argv[1] > /dev/null )} Decision Logic (continued) Another example: #!/bin/csh if {( grep UNIX $argv[1] > /dev/null )}](https://slidetodoc.com/presentation_image_h/1c51733e96a686e95259978a7a034515/image-30.jpg)
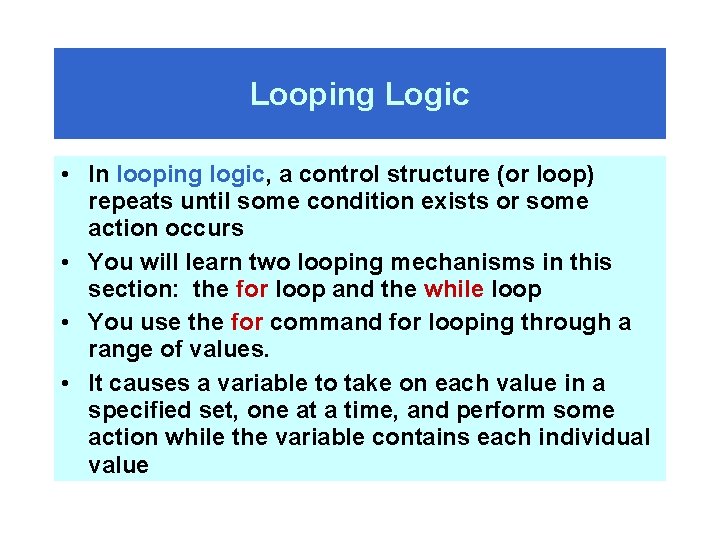
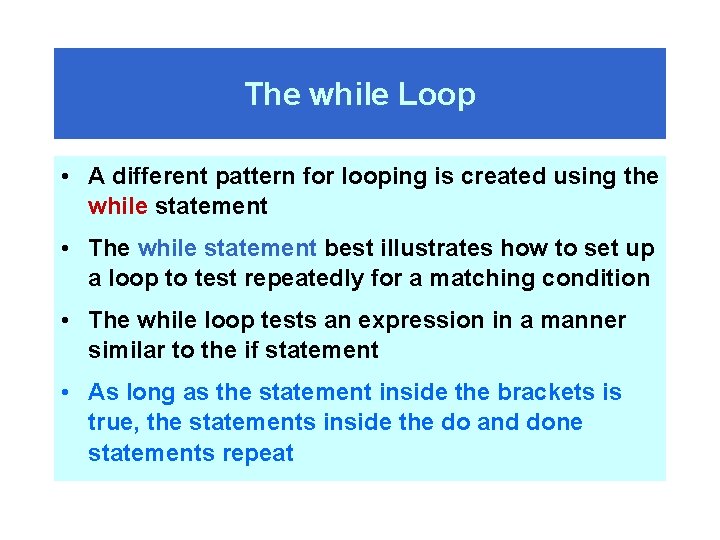
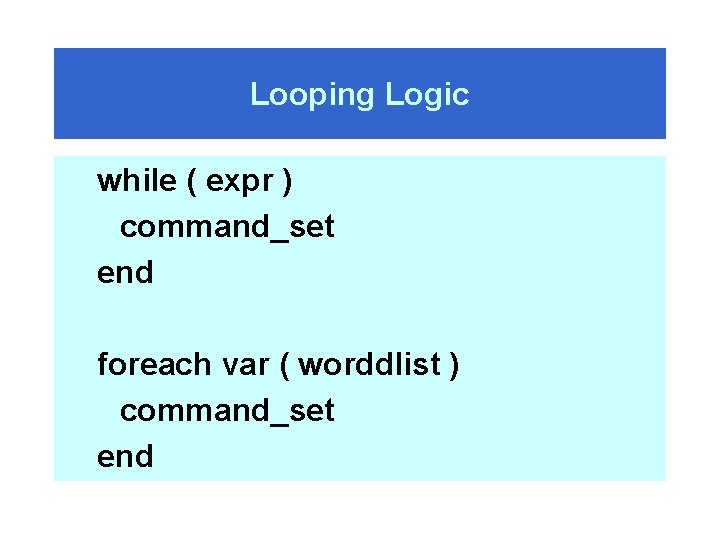
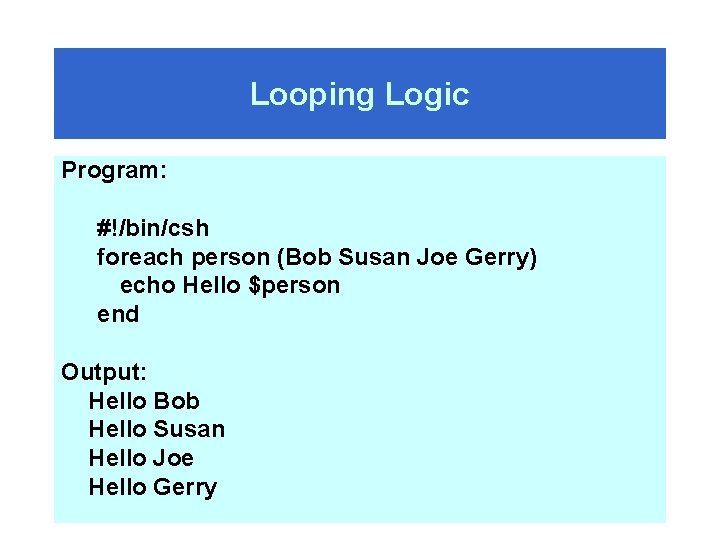
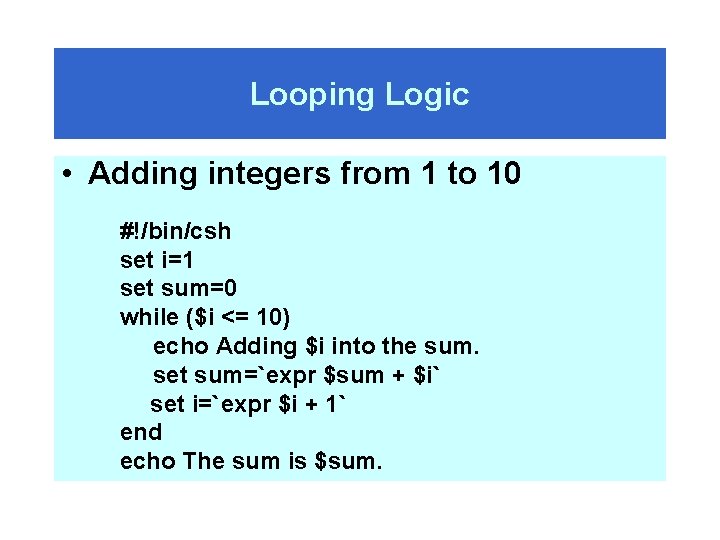
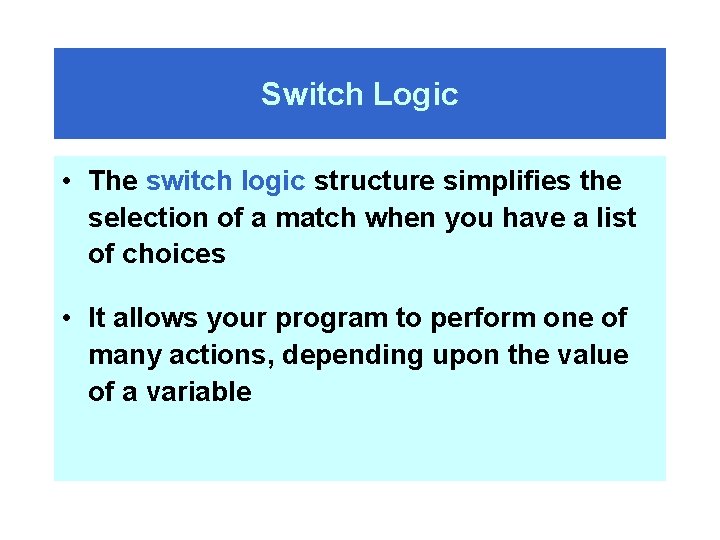
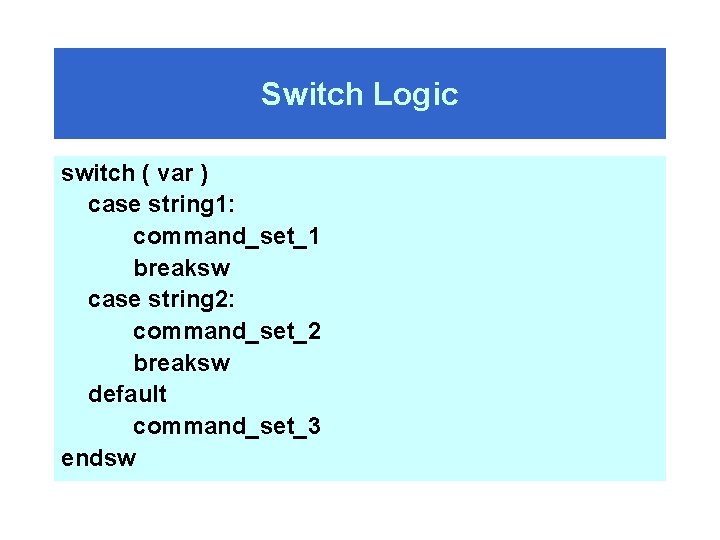
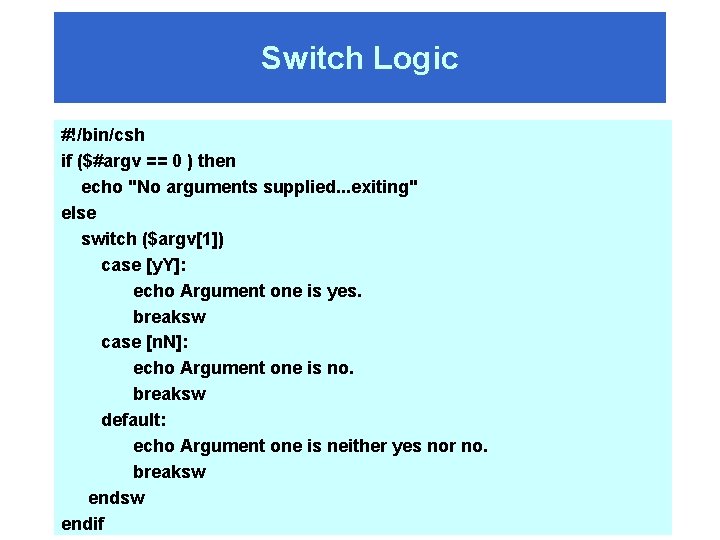
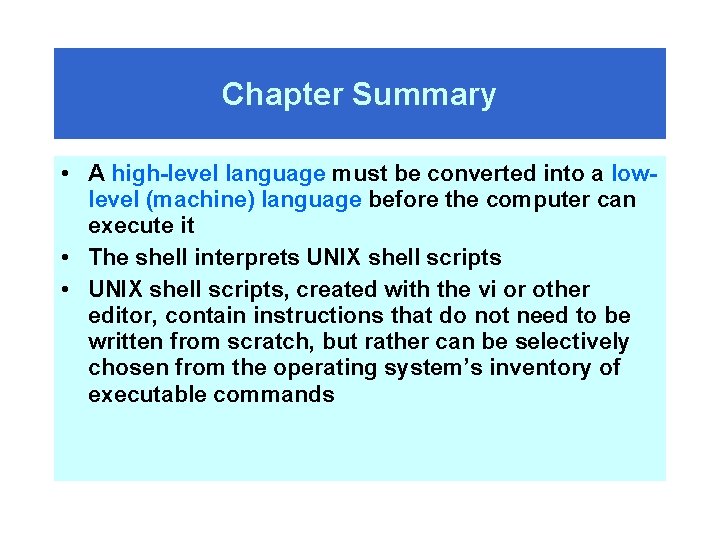
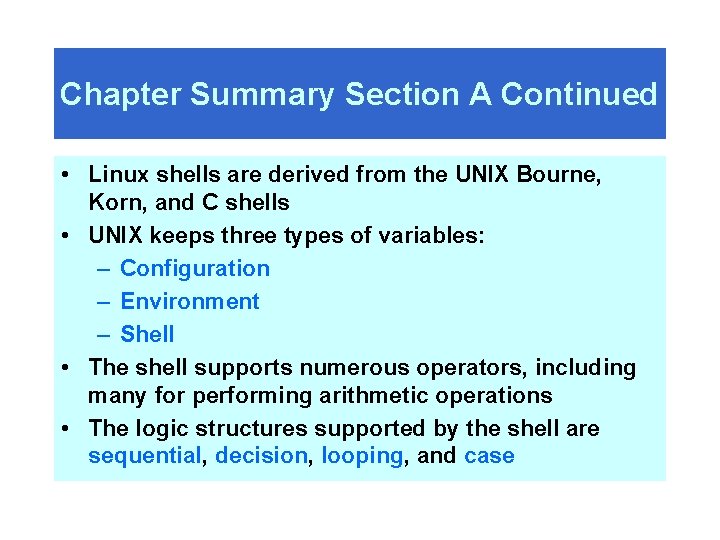
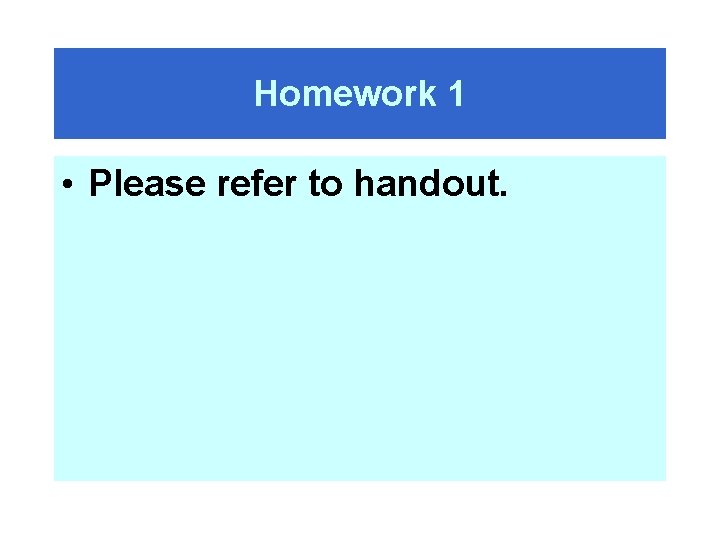
- Slides: 41
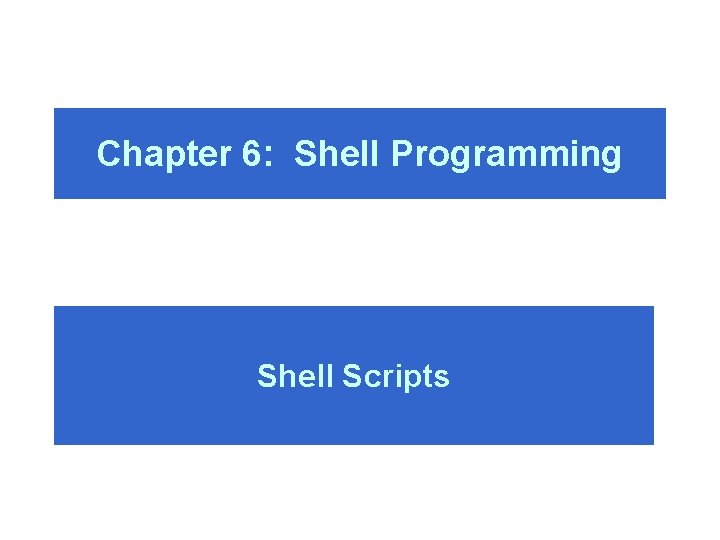
Chapter 6: Shell Programming Shell Scripts
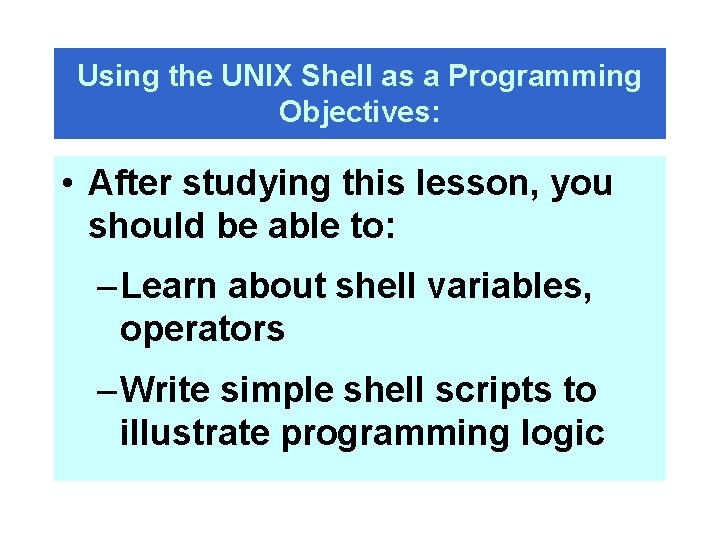
Using the UNIX Shell as a Programming Objectives: • After studying this lesson, you should be able to: – Learn about shell variables, operators – Write simple shell scripts to illustrate programming logic
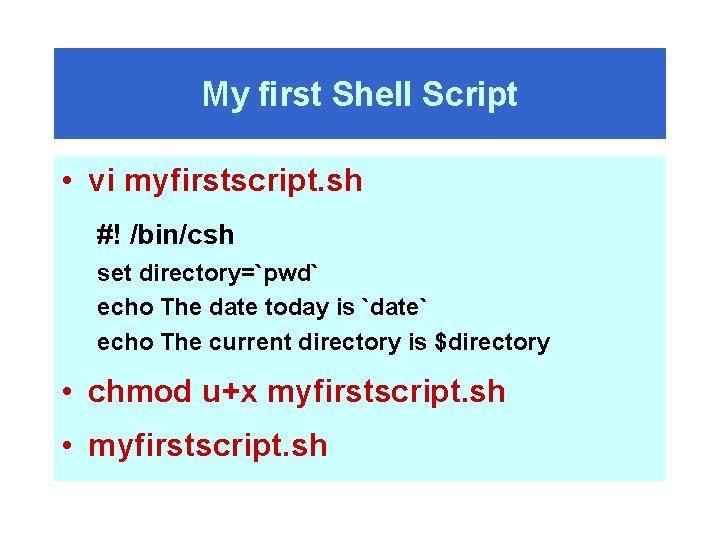
My first Shell Script • vi myfirstscript. sh #! /bin/csh set directory=`pwd` echo The date today is `date` echo The current directory is $directory • chmod u+x myfirstscript. sh • myfirstscript. sh
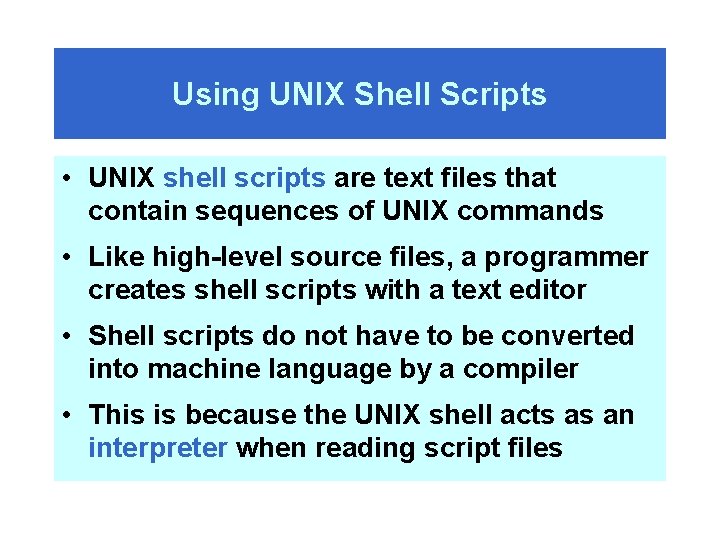
Using UNIX Shell Scripts • UNIX shell scripts are text files that contain sequences of UNIX commands • Like high-level source files, a programmer creates shell scripts with a text editor • Shell scripts do not have to be converted into machine language by a compiler • This is because the UNIX shell acts as an interpreter when reading script files
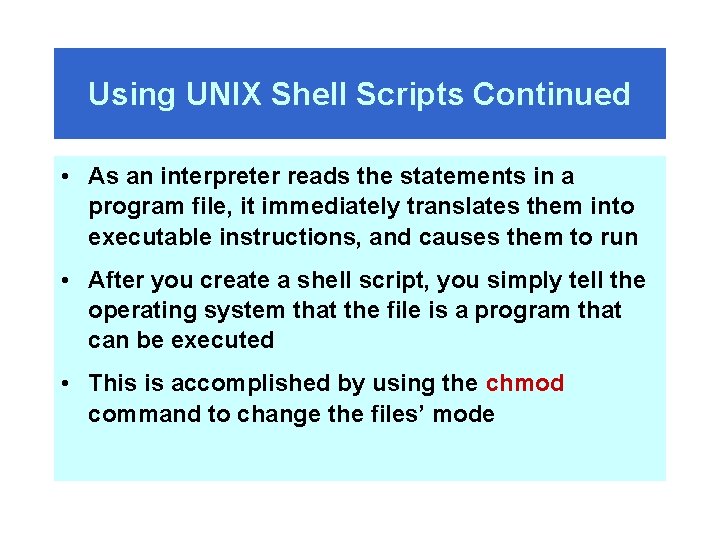
Using UNIX Shell Scripts Continued • As an interpreter reads the statements in a program file, it immediately translates them into executable instructions, and causes them to run • After you create a shell script, you simply tell the operating system that the file is a program that can be executed • This is accomplished by using the chmod command to change the files’ mode
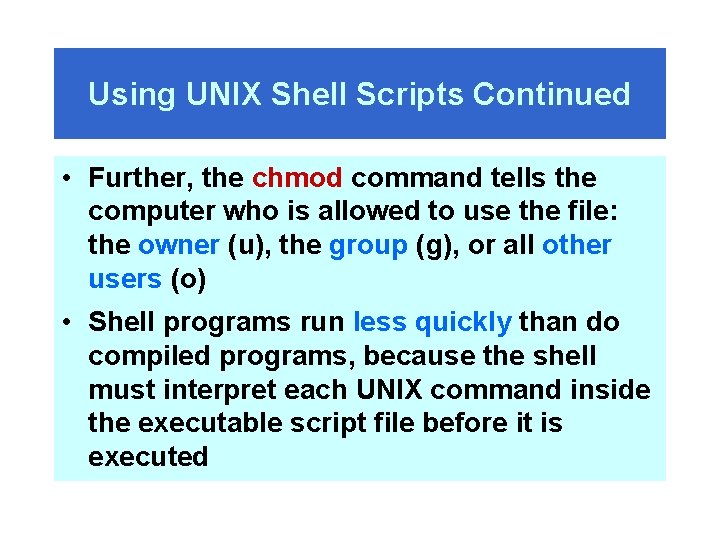
Using UNIX Shell Scripts Continued • Further, the chmod command tells the computer who is allowed to use the file: the owner (u), the group (g), or all other users (o) • Shell programs run less quickly than do compiled programs, because the shell must interpret each UNIX command inside the executable script file before it is executed
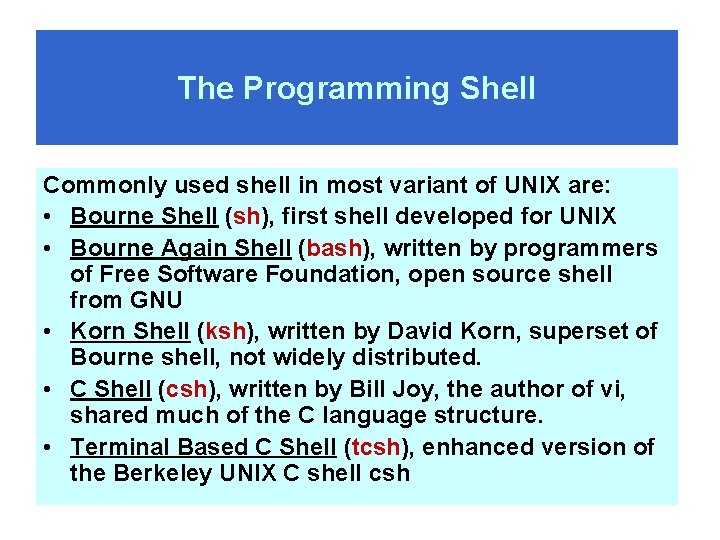
The Programming Shell Commonly used shell in most variant of UNIX are: • Bourne Shell (sh), first shell developed for UNIX • Bourne Again Shell (bash), written by programmers of Free Software Foundation, open source shell from GNU • Korn Shell (ksh), written by David Korn, superset of Bourne shell, not widely distributed. • C Shell (csh), written by Bill Joy, the author of vi, shared much of the C language structure. • Terminal Based C Shell (tcsh), enhanced version of the Berkeley UNIX C shell csh
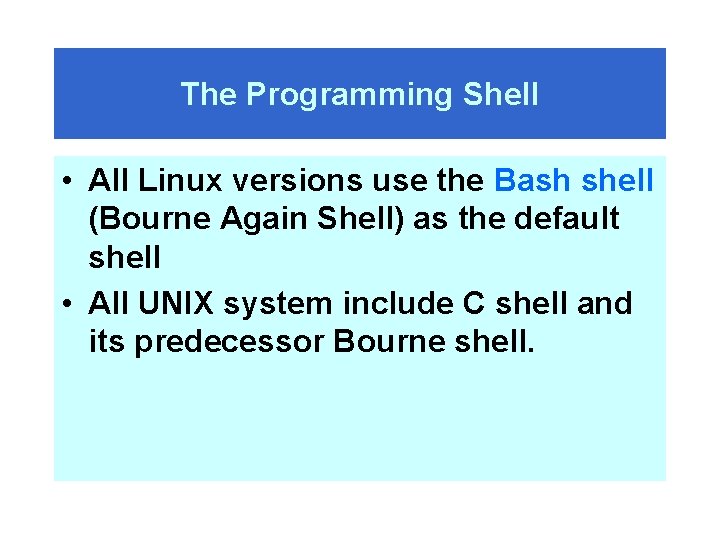
The Programming Shell • All Linux versions use the Bash shell (Bourne Again Shell) as the default shell • All UNIX system include C shell and its predecessor Bourne shell.
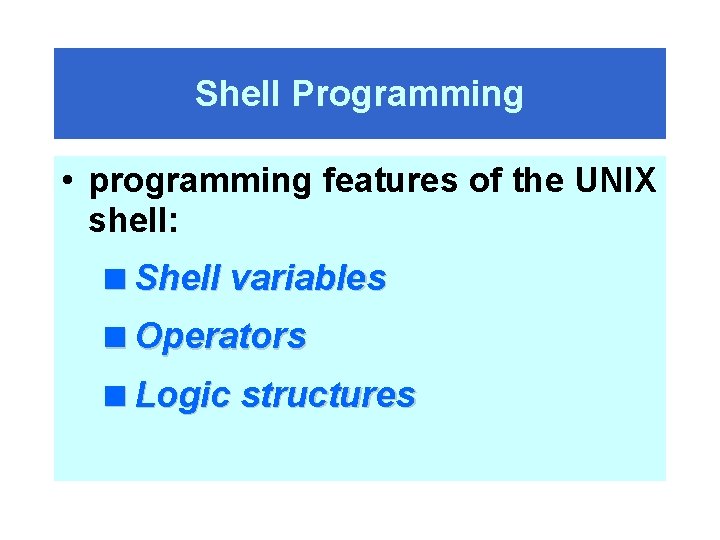
Shell Programming • programming features of the UNIX shell: <Shell variables <Operators <Logic structures
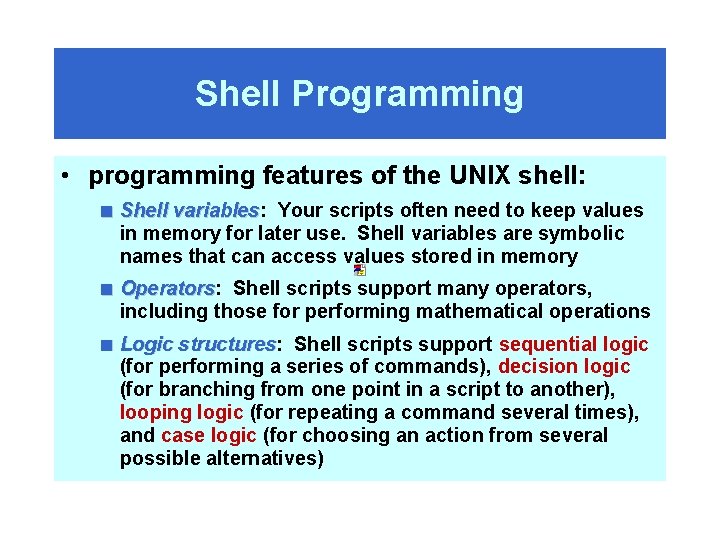
Shell Programming • programming features of the UNIX shell: < Shell variables: variables Your scripts often need to keep values in memory for later use. Shell variables are symbolic names that can access values stored in memory < Operators: Operators Shell scripts support many operators, including those for performing mathematical operations < Logic structures: structures Shell scripts support sequential logic (for performing a series of commands), decision logic (for branching from one point in a script to another), looping logic (for repeating a command several times), and case logic (for choosing an action from several possible alternatives)
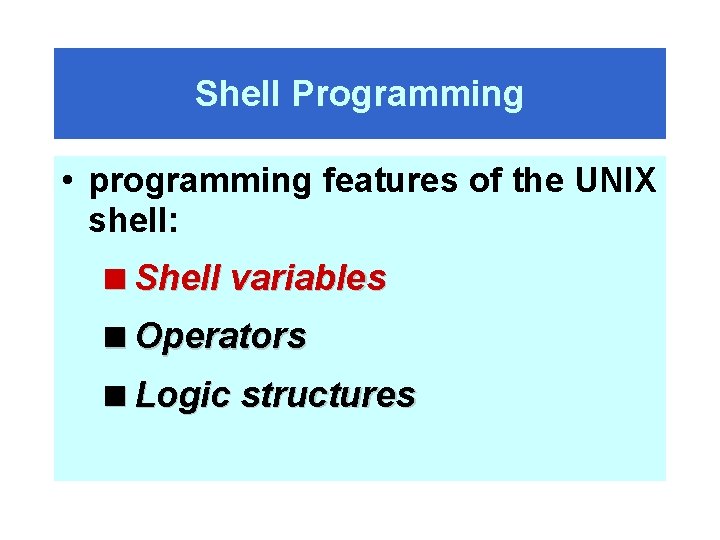
Shell Programming • programming features of the UNIX shell: <Shell variables <Operators <Logic structures
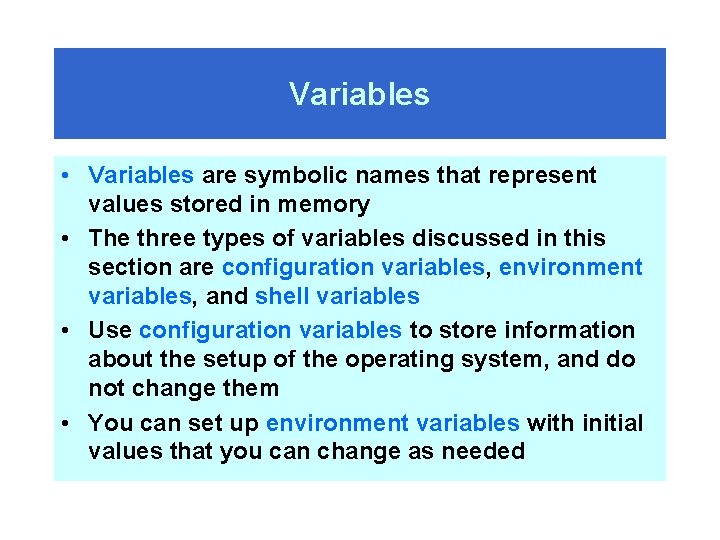
Variables • Variables are symbolic names that represent values stored in memory • The three types of variables discussed in this section are configuration variables, environment variables, and shell variables • Use configuration variables to store information about the setup of the operating system, and do not change them • You can set up environment variables with initial values that you can change as needed
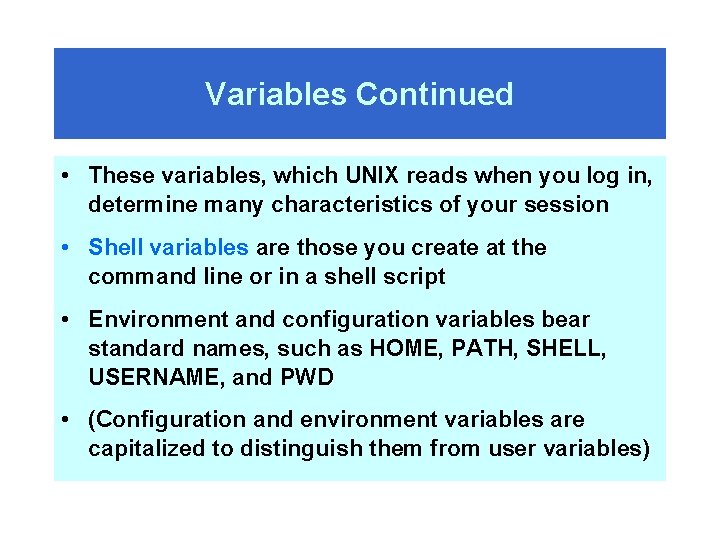
Variables Continued • These variables, which UNIX reads when you log in, determine many characteristics of your session • Shell variables are those you create at the command line or in a shell script • Environment and configuration variables bear standard names, such as HOME, PATH, SHELL, USERNAME, and PWD • (Configuration and environment variables are capitalized to distinguish them from user variables)
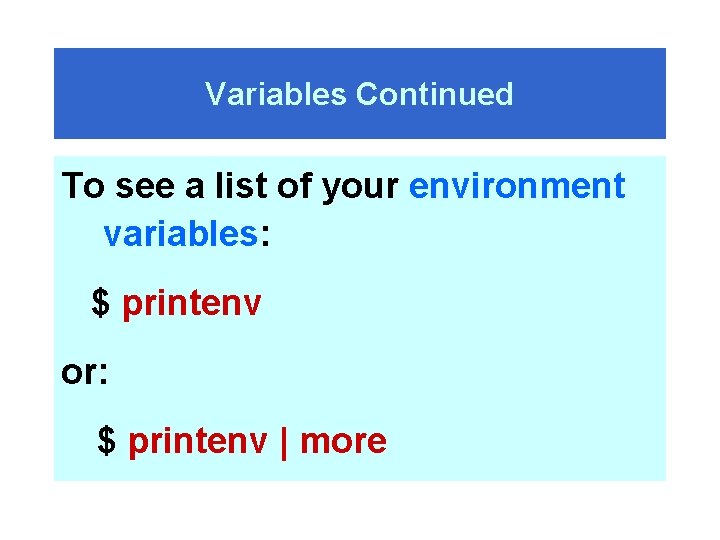
Variables Continued To see a list of your environment variables: $ printenv or: $ printenv | more
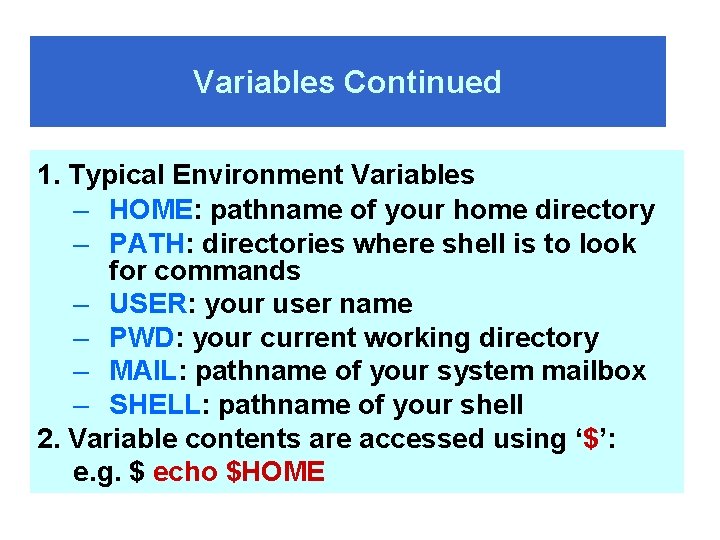
Variables Continued 1. Typical Environment Variables – HOME: pathname of your home directory – PATH: directories where shell is to look for commands – USER: your user name – PWD: your current working directory – MAIL: pathname of your system mailbox – SHELL: pathname of your shell 2. Variable contents are accessed using ‘$’: e. g. $ echo $HOME
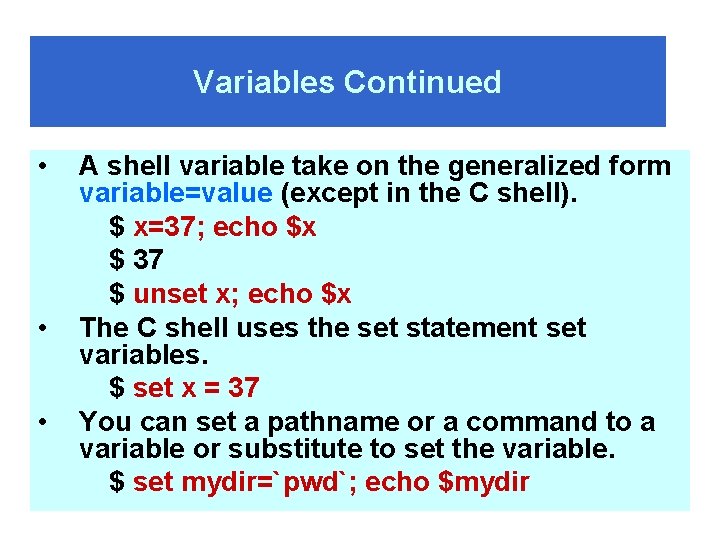
Variables Continued • • • A shell variable take on the generalized form variable=value (except in the C shell). $ x=37; echo $x $ 37 $ unset x; echo $x The C shell uses the set statement set variables. $ set x = 37 You can set a pathname or a command to a variable or substitute to set the variable. $ set mydir=`pwd`; echo $mydir
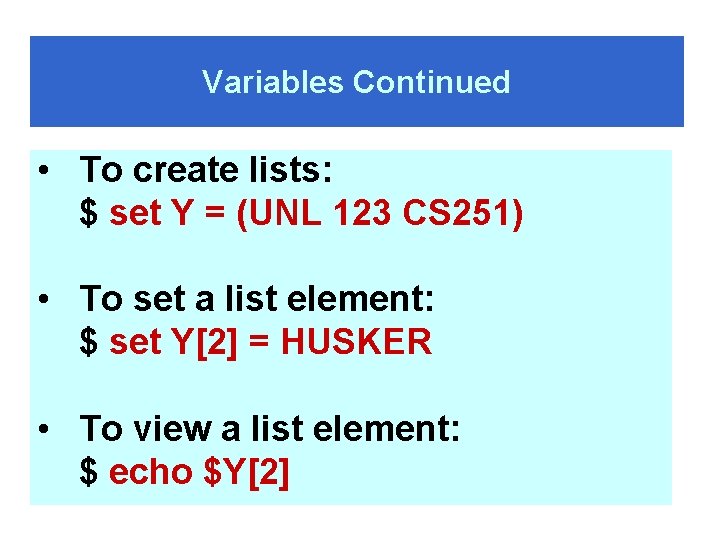
Variables Continued • To create lists: $ set Y = (UNL 123 CS 251) • To set a list element: $ set Y[2] = HUSKER • To view a list element: $ echo $Y[2]
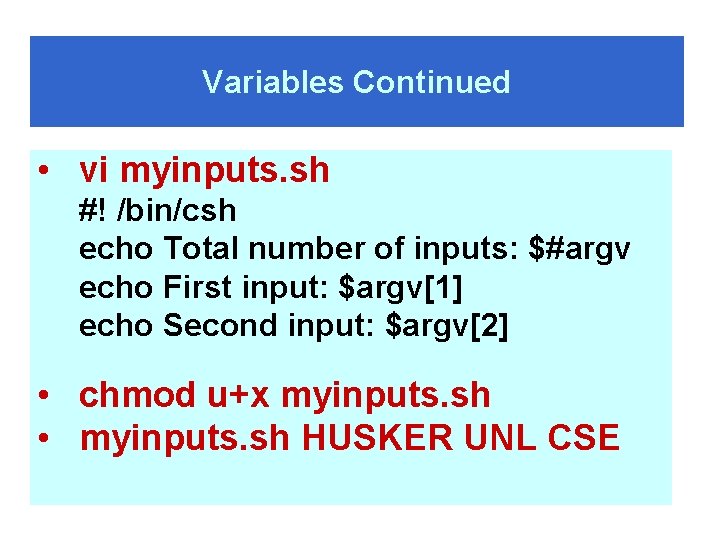
Variables Continued • vi myinputs. sh #! /bin/csh echo Total number of inputs: $#argv echo First input: $argv[1] echo Second input: $argv[2] • chmod u+x myinputs. sh • myinputs. sh HUSKER UNL CSE
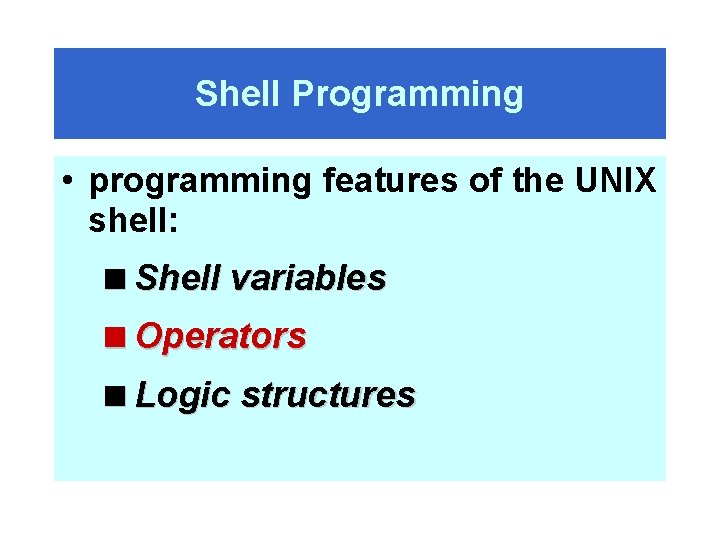
Shell Programming • programming features of the UNIX shell: <Shell variables <Operators <Logic structures
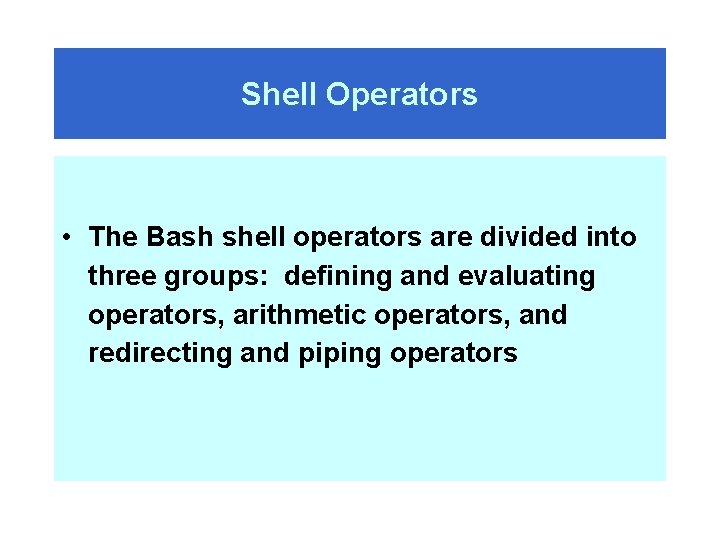
Shell Operators • The Bash shell operators are divided into three groups: defining and evaluating operators, arithmetic operators, and redirecting and piping operators
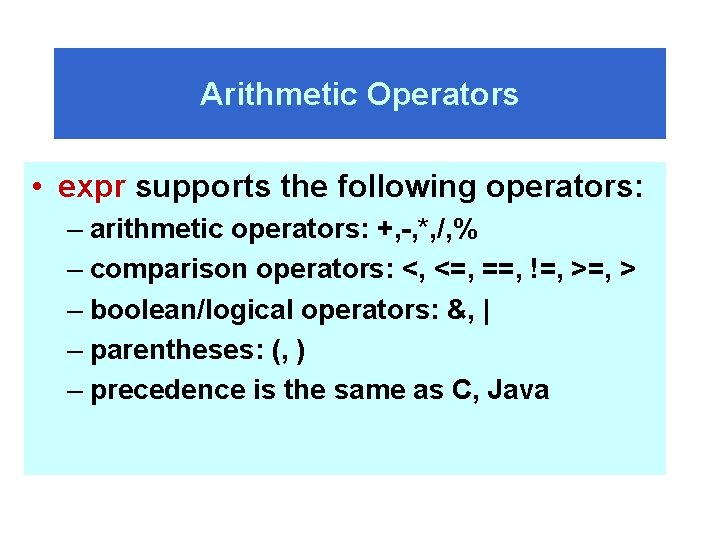
Arithmetic Operators • expr supports the following operators: – arithmetic operators: +, -, *, /, % – comparison operators: <, <=, ==, !=, > – boolean/logical operators: &, | – parentheses: (, ) – precedence is the same as C, Java
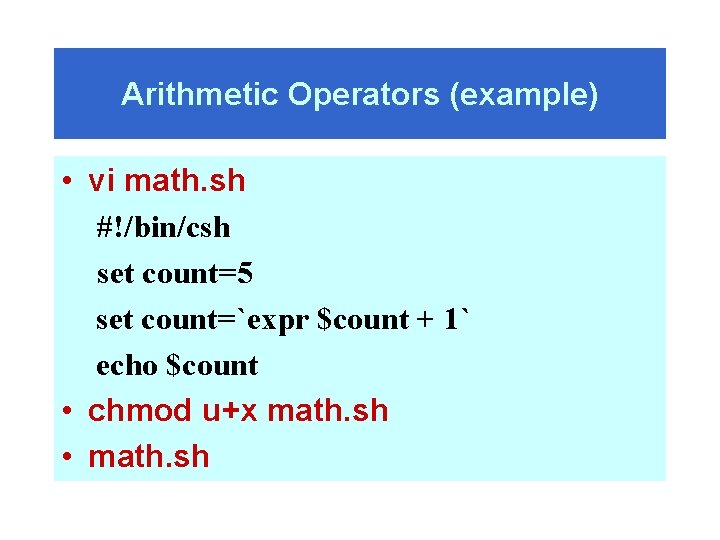
Arithmetic Operators (example) • vi math. sh #!/bin/csh set count=5 set count=`expr $count + 1` echo $count • chmod u+x math. sh • math. sh
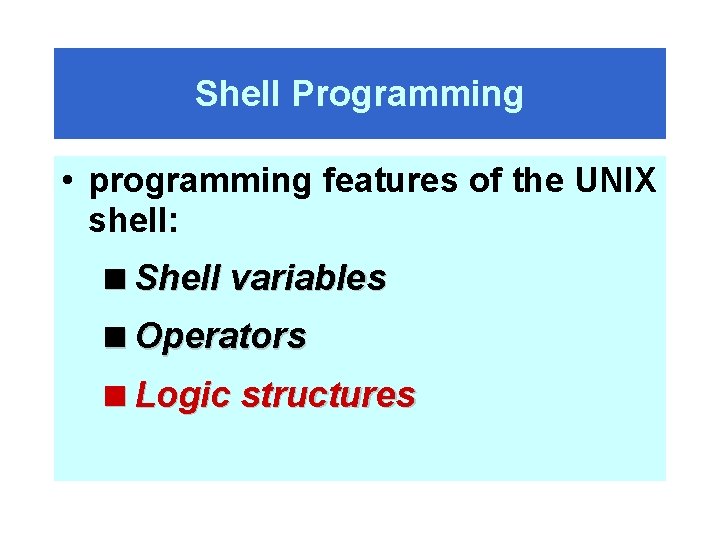
Shell Programming • programming features of the UNIX shell: <Shell variables <Operators <Logic structures
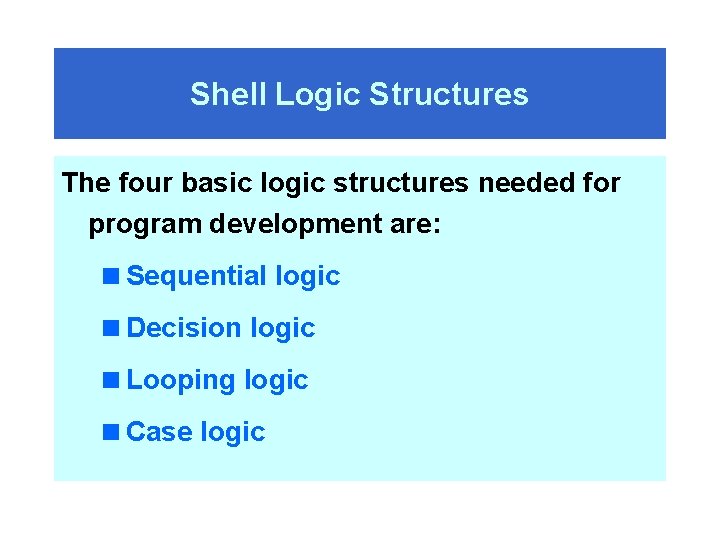
Shell Logic Structures The four basic logic structures needed for program development are: <Sequential logic <Decision logic <Looping logic <Case logic
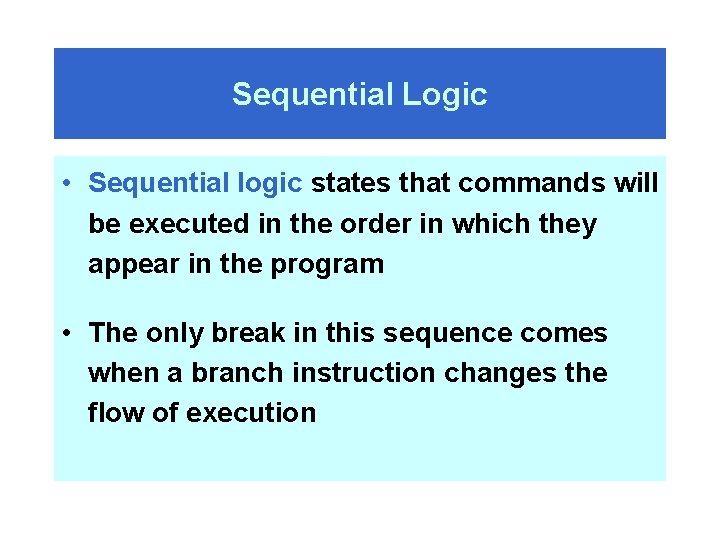
Sequential Logic • Sequential logic states that commands will be executed in the order in which they appear in the program • The only break in this sequence comes when a branch instruction changes the flow of execution
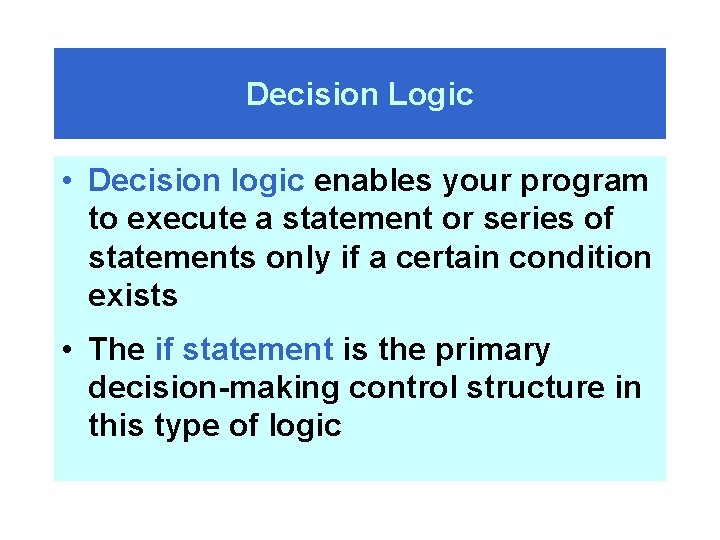
Decision Logic • Decision logic enables your program to execute a statement or series of statements only if a certain condition exists • The if statement is the primary decision-making control structure in this type of logic
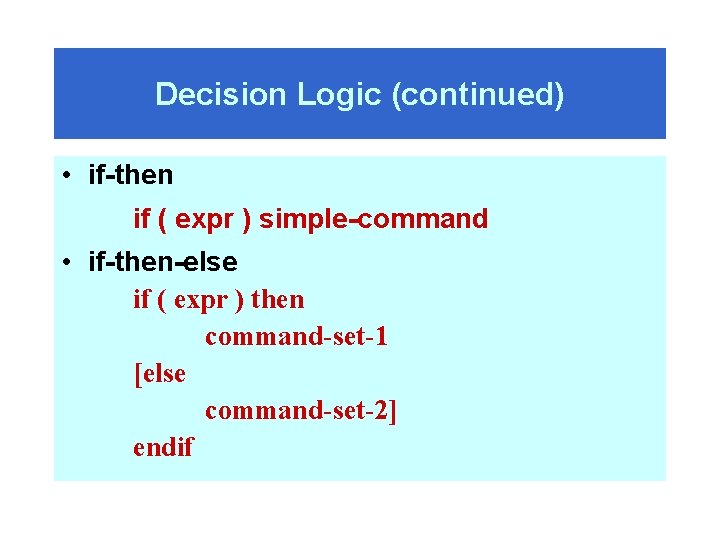
Decision Logic (continued) • if-then if ( expr ) simple-command • if-then-else if ( expr ) then command-set-1 [else command-set-2] endif
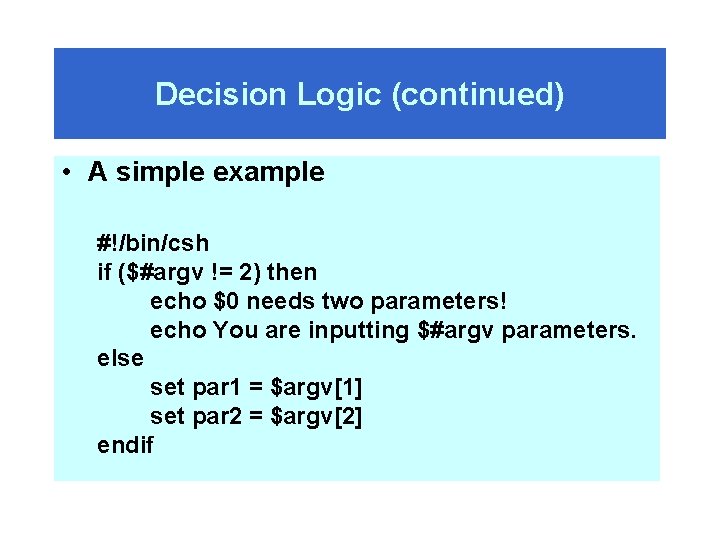
Decision Logic (continued) • A simple example #!/bin/csh if ($#argv != 2) then echo $0 needs two parameters! echo You are inputting $#argv parameters. else set par 1 = $argv[1] set par 2 = $argv[2] endif
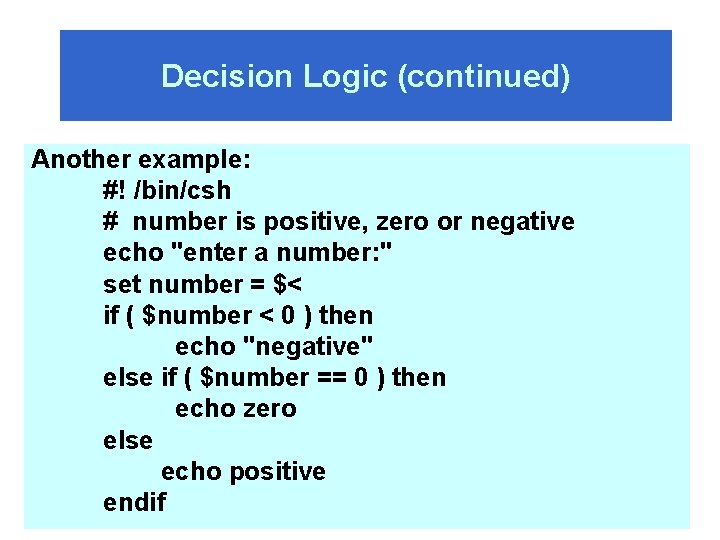
Decision Logic (continued) Another example: #! /bin/csh # number is positive, zero or negative echo "enter a number: " set number = $< if ( $number < 0 ) then echo "negative" else if ( $number == 0 ) then echo zero else echo positive endif
![Decision Logic continued Another example bincsh if grep UNIX argv1 devnull Decision Logic (continued) Another example: #!/bin/csh if {( grep UNIX $argv[1] > /dev/null )}](https://slidetodoc.com/presentation_image_h/1c51733e96a686e95259978a7a034515/image-30.jpg)
Decision Logic (continued) Another example: #!/bin/csh if {( grep UNIX $argv[1] > /dev/null )} then echo UNIX occurs in $argv[1] else echo No! echo UNIX does not occur in $argv[1] endif Redirect intermediate results into >/dev/null, instead of showing on the screen.
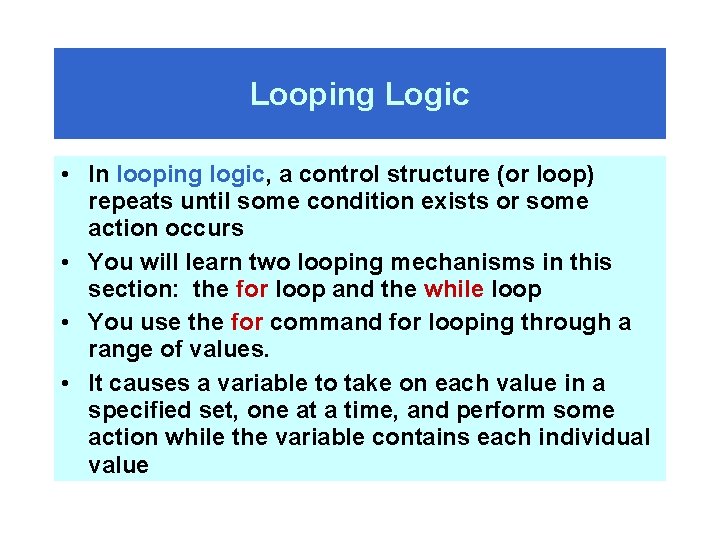
Looping Logic • In looping logic, a control structure (or loop) repeats until some condition exists or some action occurs • You will learn two looping mechanisms in this section: the for loop and the while loop • You use the for command for looping through a range of values. • It causes a variable to take on each value in a specified set, one at a time, and perform some action while the variable contains each individual value
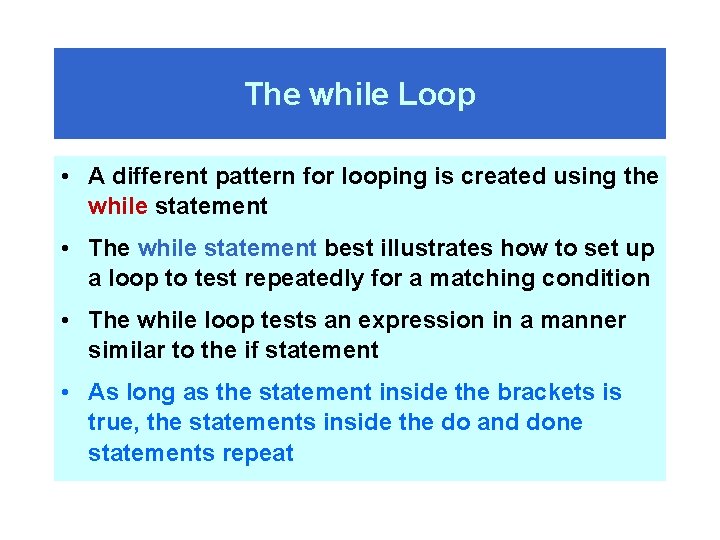
The while Loop • A different pattern for looping is created using the while statement • The while statement best illustrates how to set up a loop to test repeatedly for a matching condition • The while loop tests an expression in a manner similar to the if statement • As long as the statement inside the brackets is true, the statements inside the do and done statements repeat
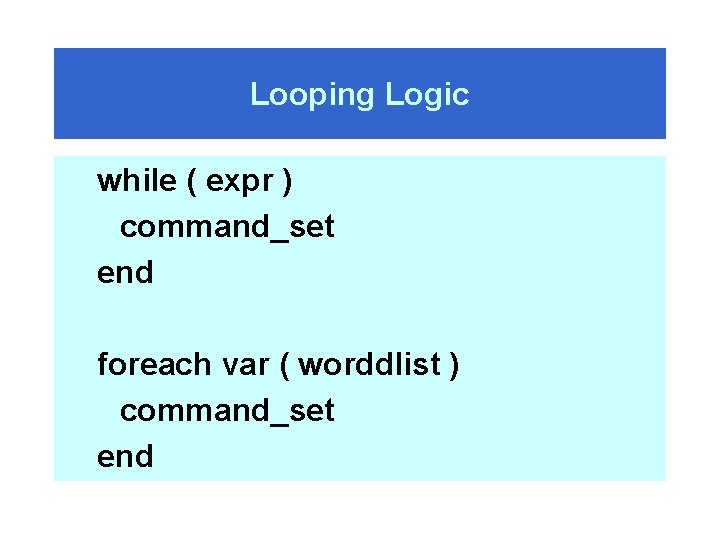
Looping Logic while ( expr ) command_set end foreach var ( worddlist ) command_set end
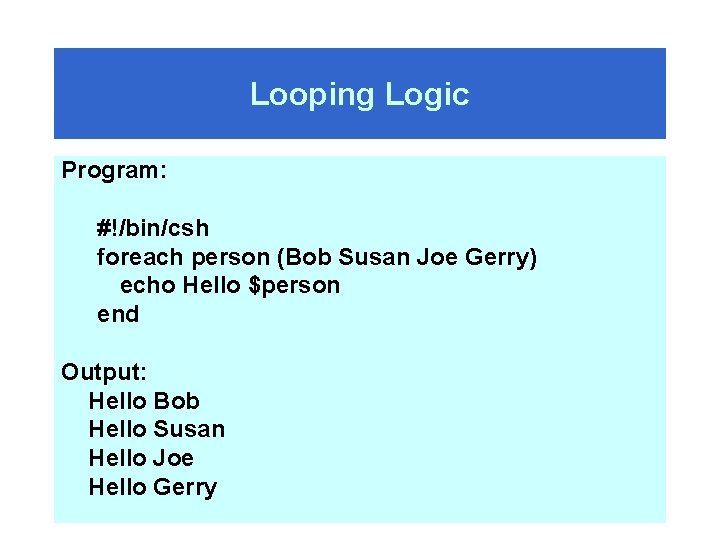
Looping Logic Program: #!/bin/csh foreach person (Bob Susan Joe Gerry) echo Hello $person end Output: Hello Bob Hello Susan Hello Joe Hello Gerry
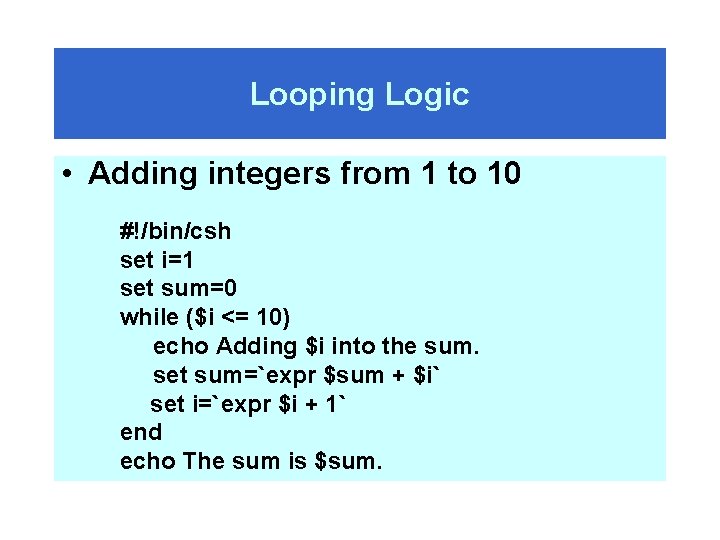
Looping Logic • Adding integers from 1 to 10 #!/bin/csh set i=1 set sum=0 while ($i <= 10) echo Adding $i into the sum. set sum=`expr $sum + $i` set i=`expr $i + 1` end echo The sum is $sum.
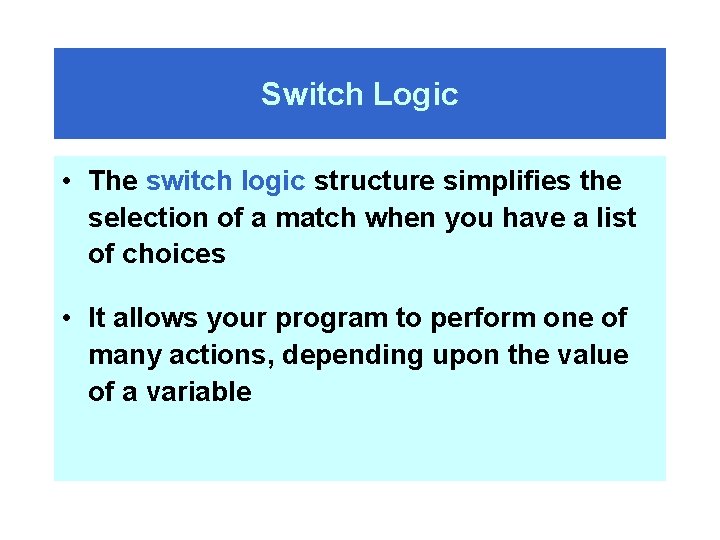
Switch Logic • The switch logic structure simplifies the selection of a match when you have a list of choices • It allows your program to perform one of many actions, depending upon the value of a variable
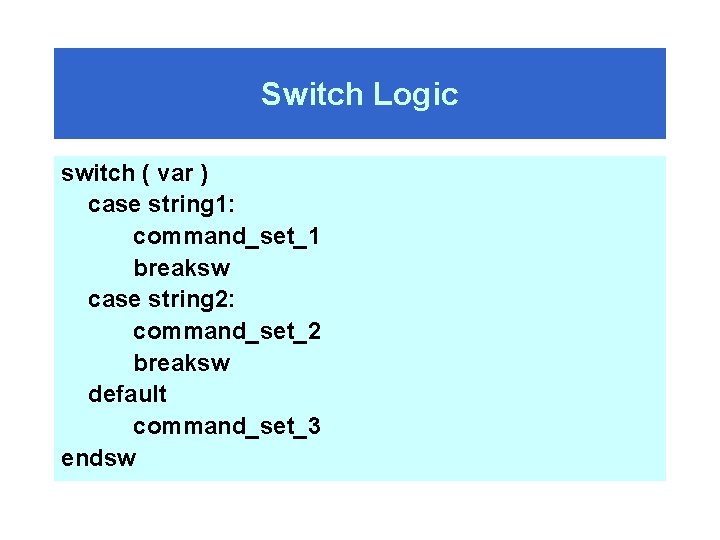
Switch Logic switch ( var ) case string 1: command_set_1 breaksw case string 2: command_set_2 breaksw default command_set_3 endsw
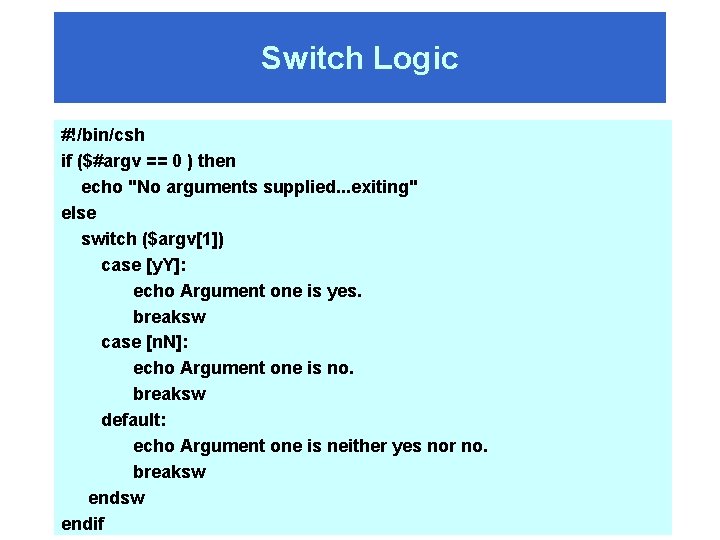
Switch Logic #!/bin/csh if ($#argv == 0 ) then echo "No arguments supplied. . . exiting" else switch ($argv[1]) case [y. Y]: echo Argument one is yes. breaksw case [n. N]: echo Argument one is no. breaksw default: echo Argument one is neither yes nor no. breaksw endif
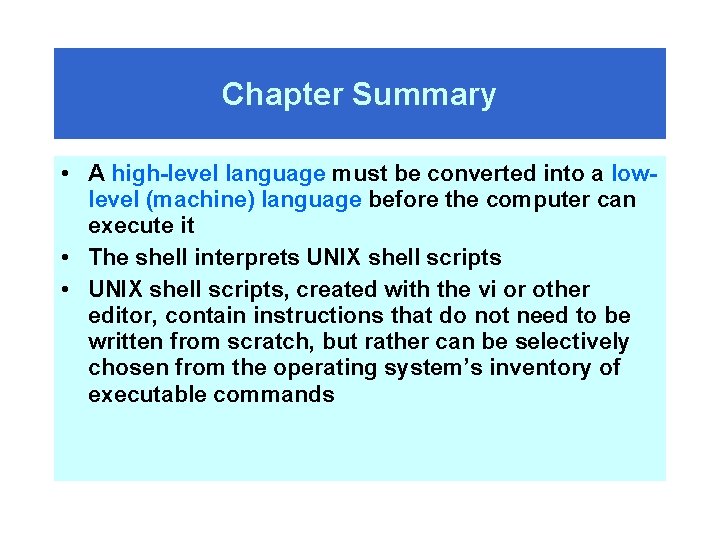
Chapter Summary • A high-level language must be converted into a lowlevel (machine) language before the computer can execute it • The shell interprets UNIX shell scripts • UNIX shell scripts, created with the vi or other editor, contain instructions that do not need to be written from scratch, but rather can be selectively chosen from the operating system’s inventory of executable commands
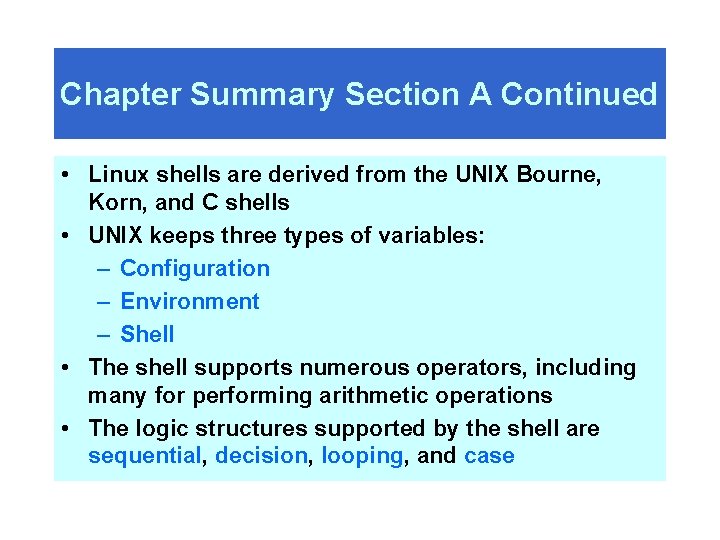
Chapter Summary Section A Continued • Linux shells are derived from the UNIX Bourne, Korn, and C shells • UNIX keeps three types of variables: – Configuration – Environment – Shell • The shell supports numerous operators, including many for performing arithmetic operations • The logic structures supported by the shell are sequential, decision, looping, and case
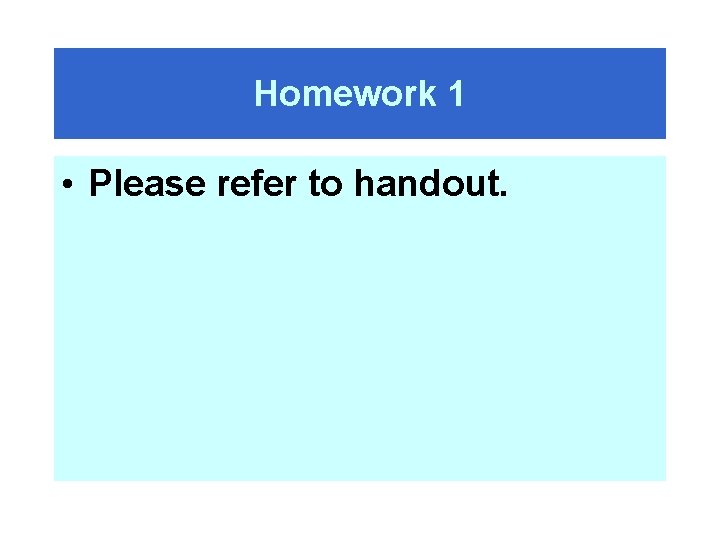
Homework 1 • Please refer to handout.
Shell cleanliness shell soundness shell texture shell shape
Ropey strands of egg white
Fun shell scripts
Are shell scripts compiled or interpreted
Command line arguments in unix
Eagle scout ceremony script
Fungsi dari scripts motion (gerakan) adalah
Spanish skits scripts
Lab 7-1 scripting in the bash shell
Java scrept
Glen marino
Skit script example
Identity scripts examples
Express scripts warehouse
Romeo and juliet script
List three commands the robomind can see.
Emotion coaching scripts
Tabular editor 3
Scripts png
Greasemonkey tutorial
Acting scripts
How to use ola hallengren scripts
Acting scripts
Selenium migration
Serverside scripts
Scripts to rule them all
Moliere scripts
Illness scripts
What shell am i using
Perbedaan linear programming dan integer programming
Greedy vs dynamic
What is system program
Integer programming vs linear programming
Definisi integer
Interrupt programming in 8051 examples
Binomial coefficient using dynamic programming
Solving goal programming problems using simplex method
Apprenticeship learning using linear programming
Hình ảnh bộ gõ cơ thể búng tay
Frameset trong html5
Bổ thể
Tỉ lệ cơ thể trẻ em