CSCI 330 THE UNIX SYSTEM C Shell Programming
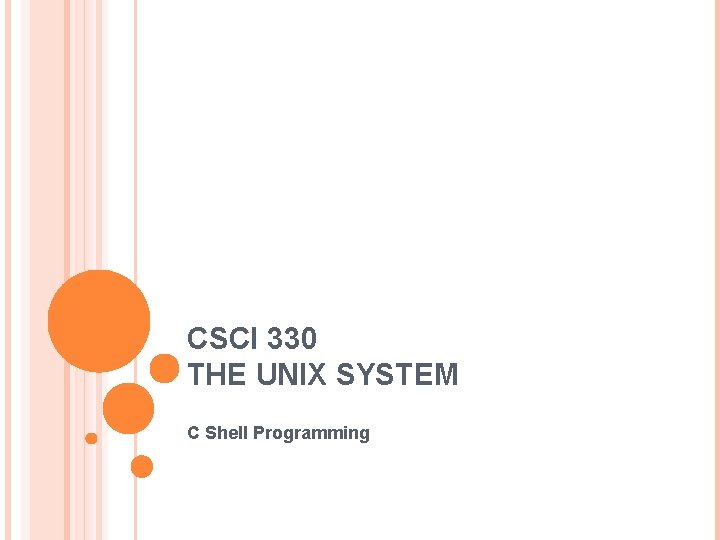
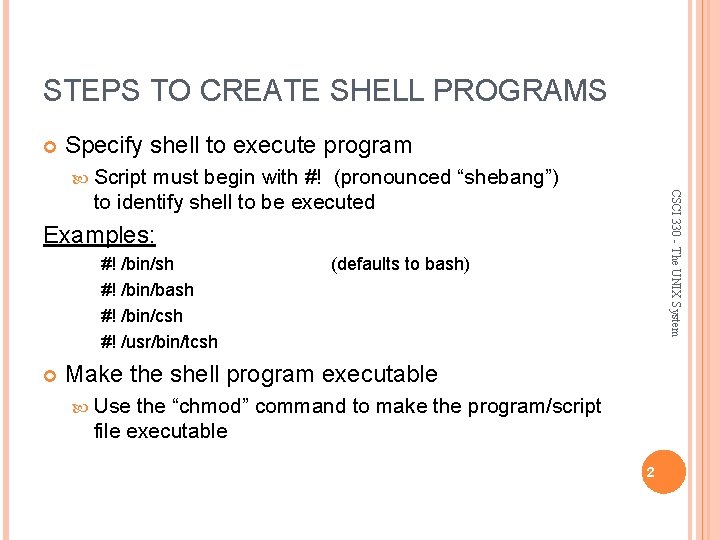
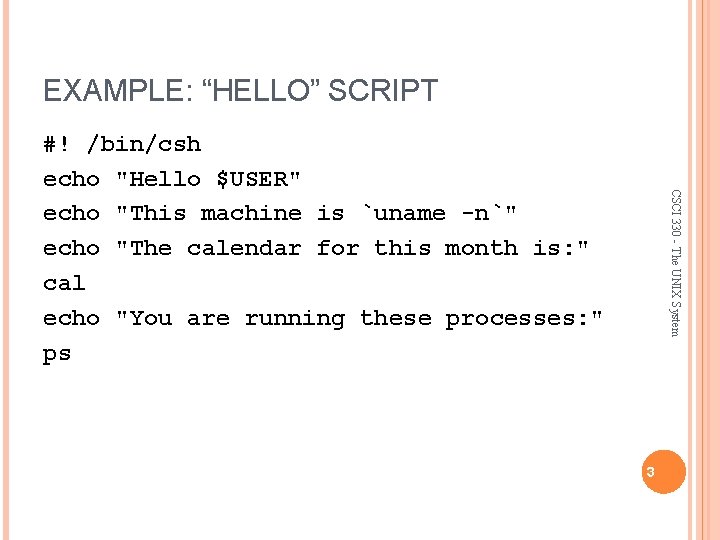
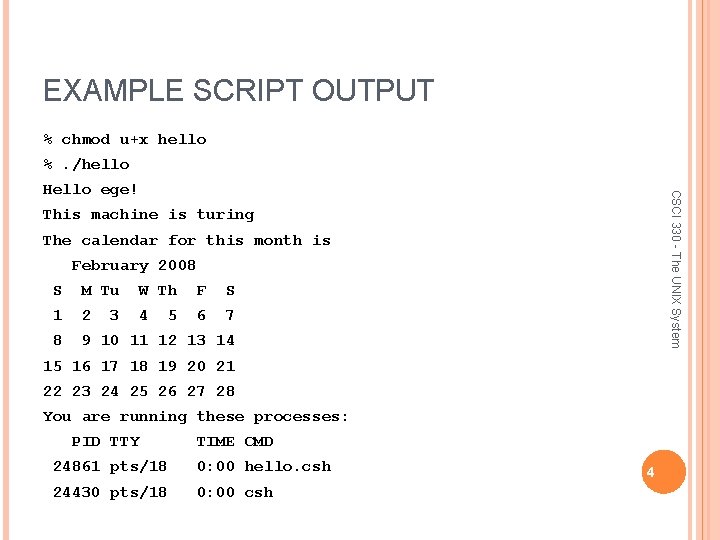
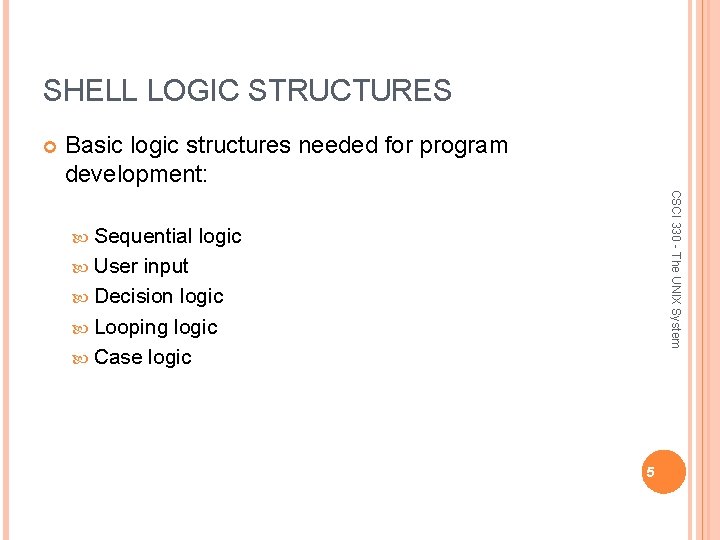
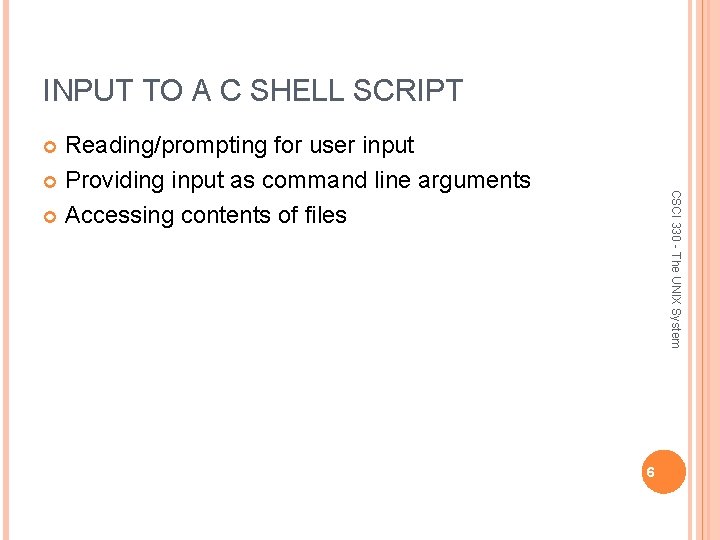
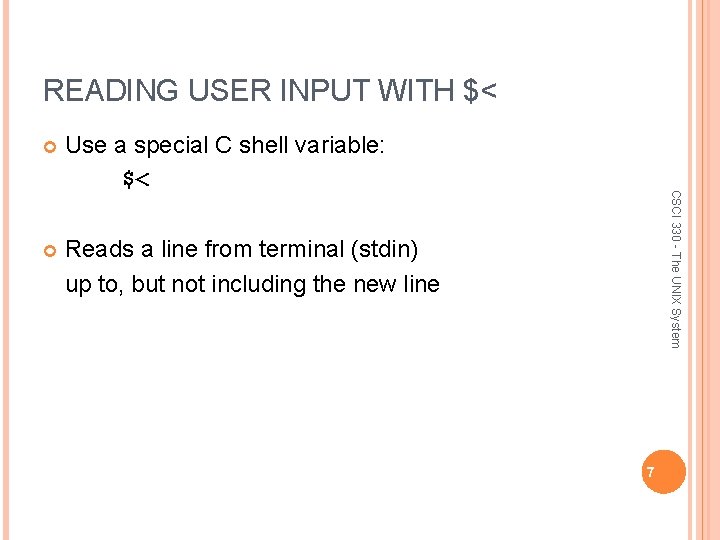
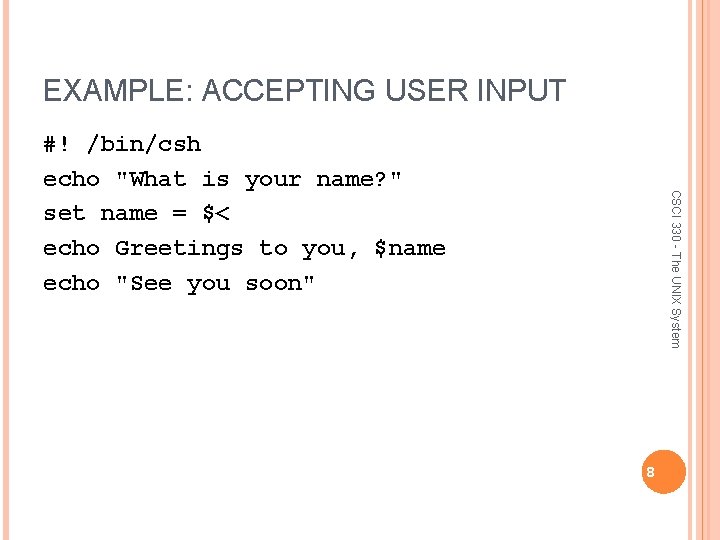
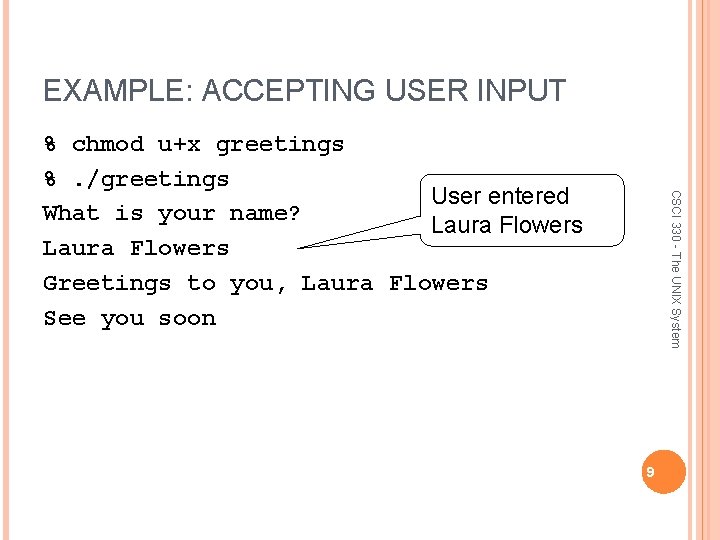
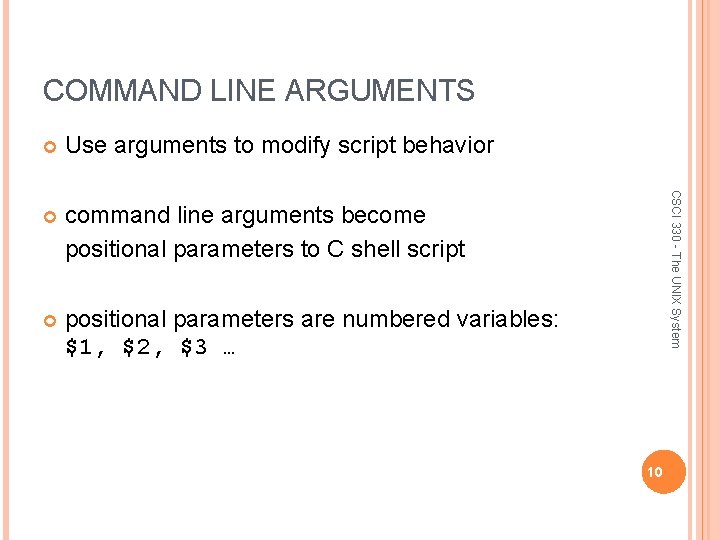
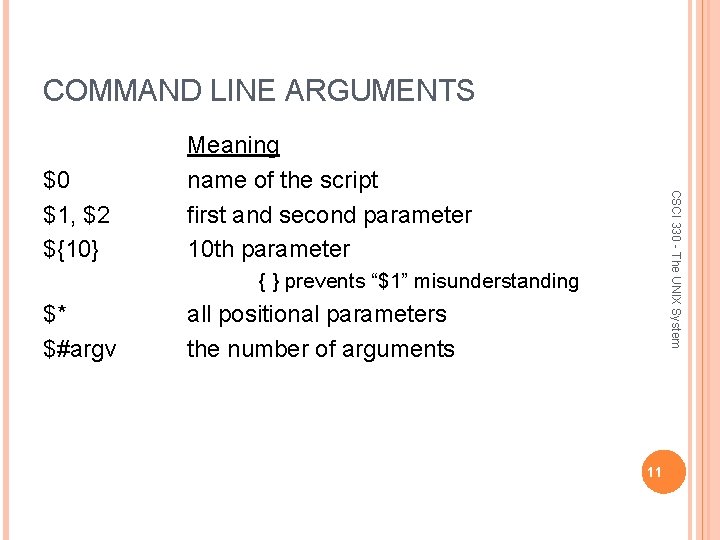
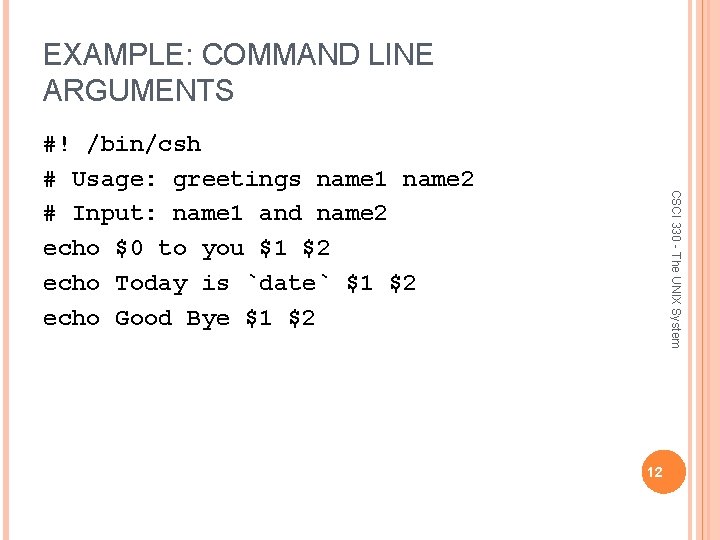
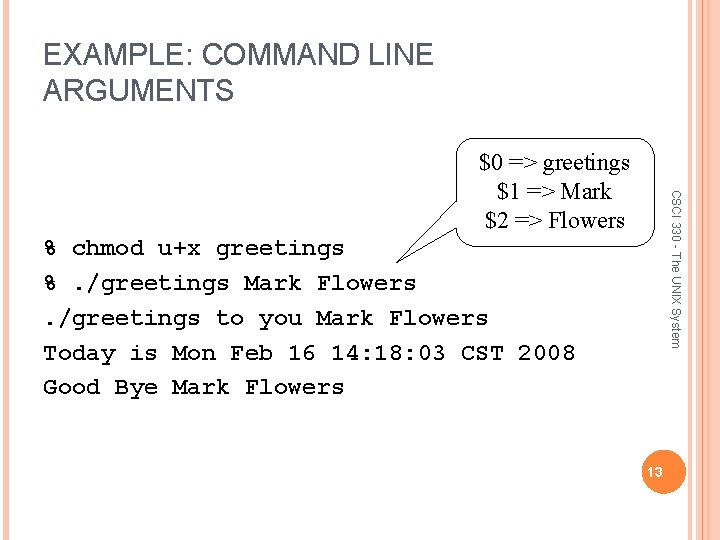
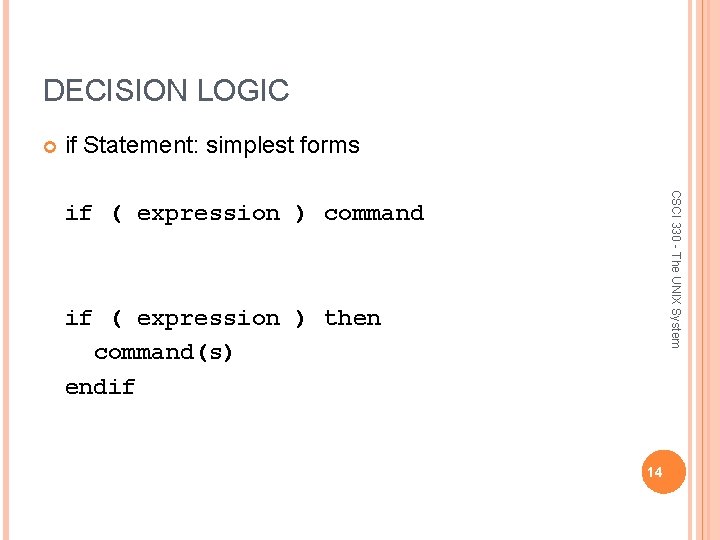
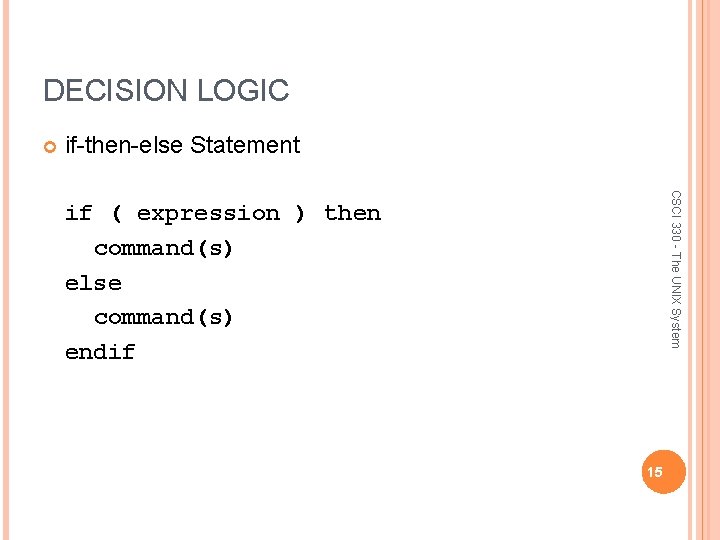
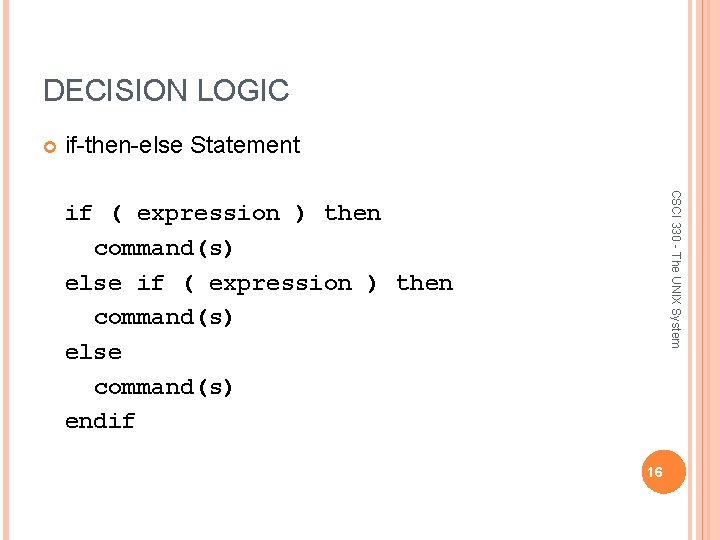
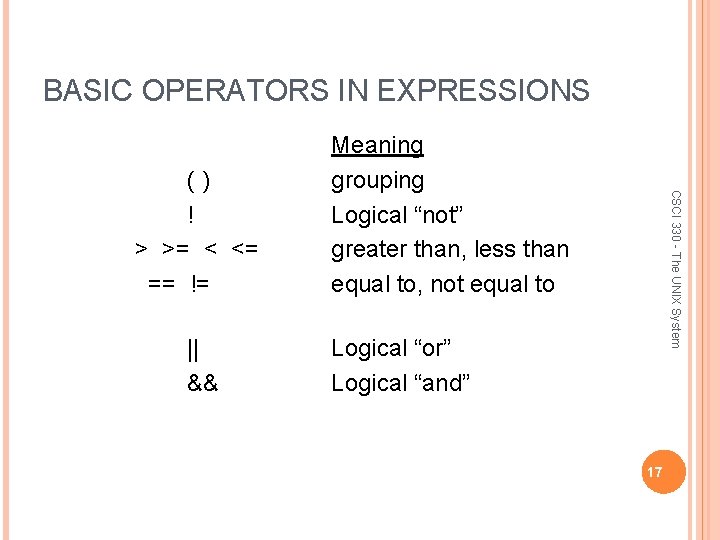
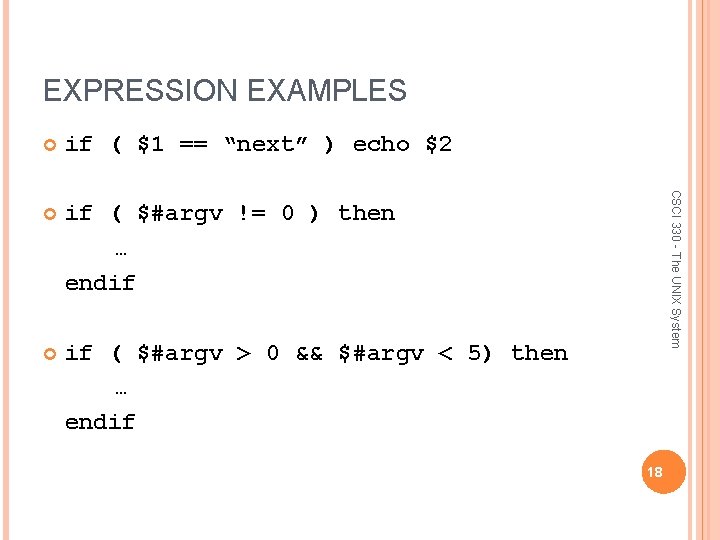
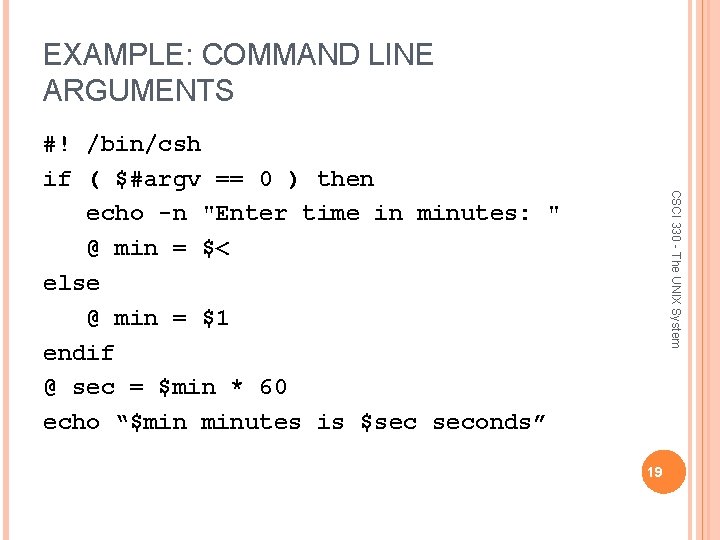
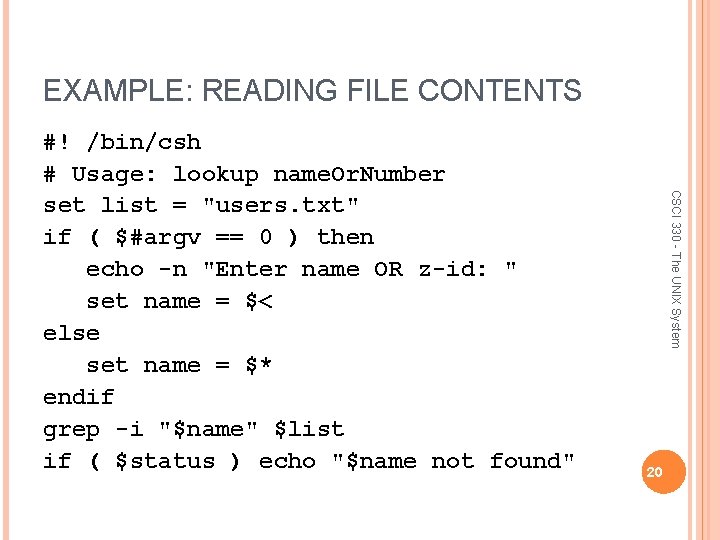
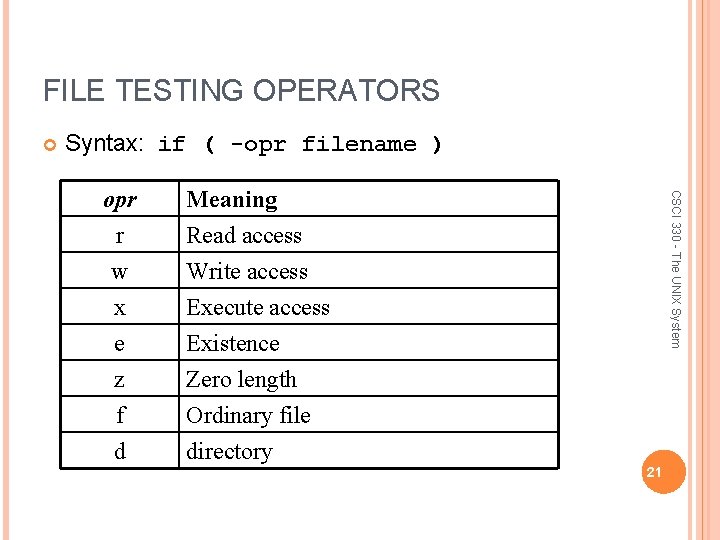
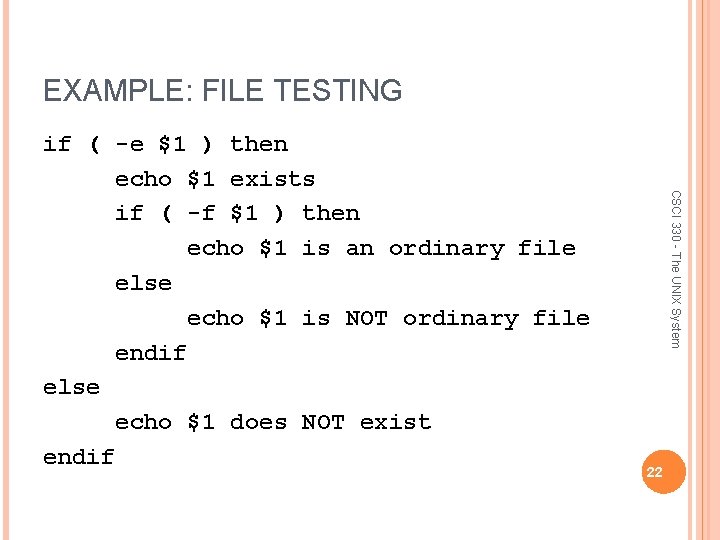
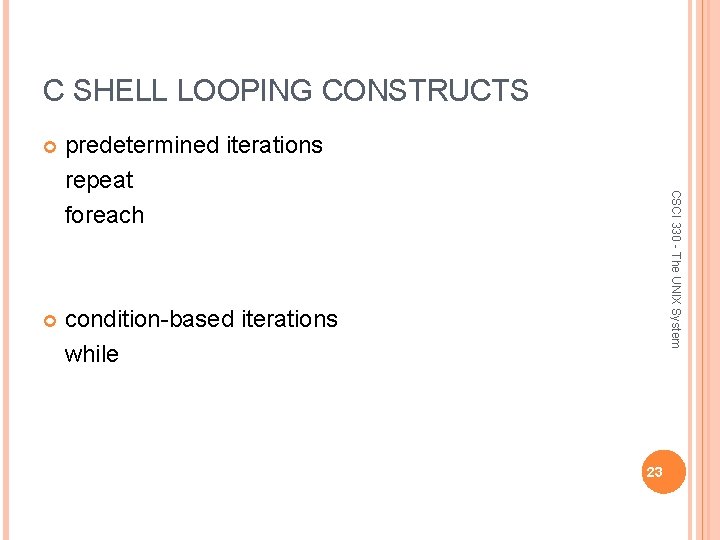
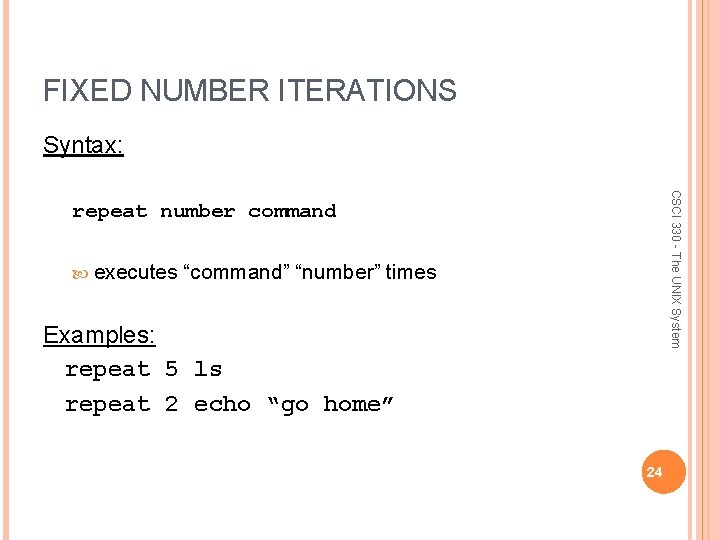
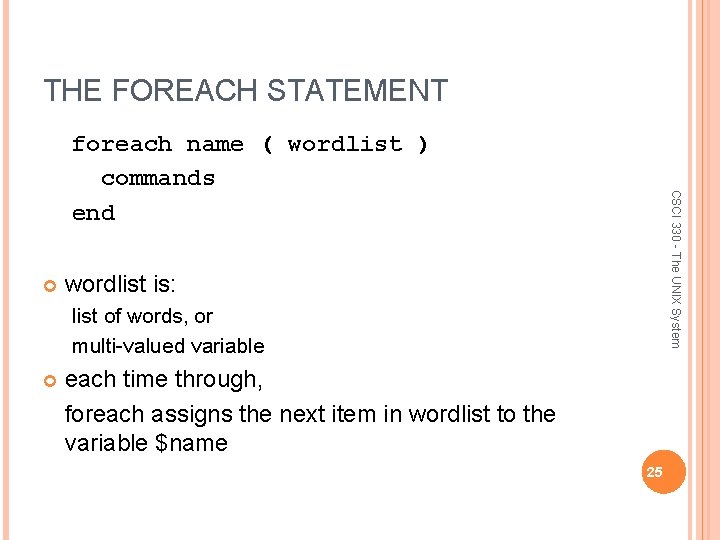
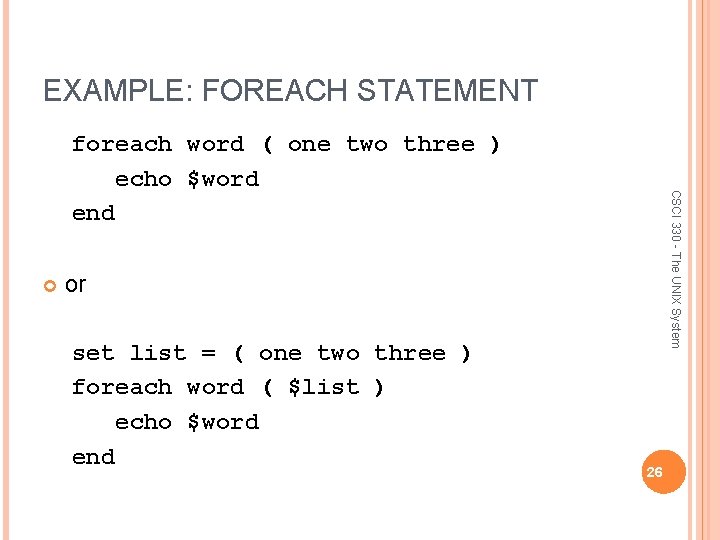
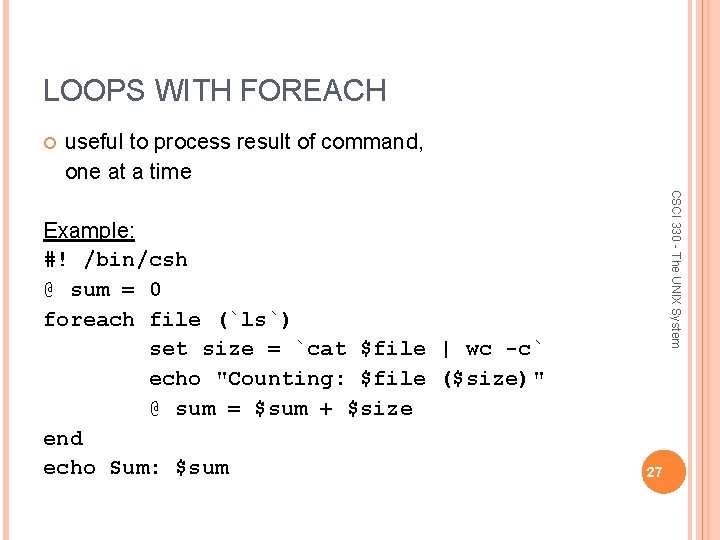
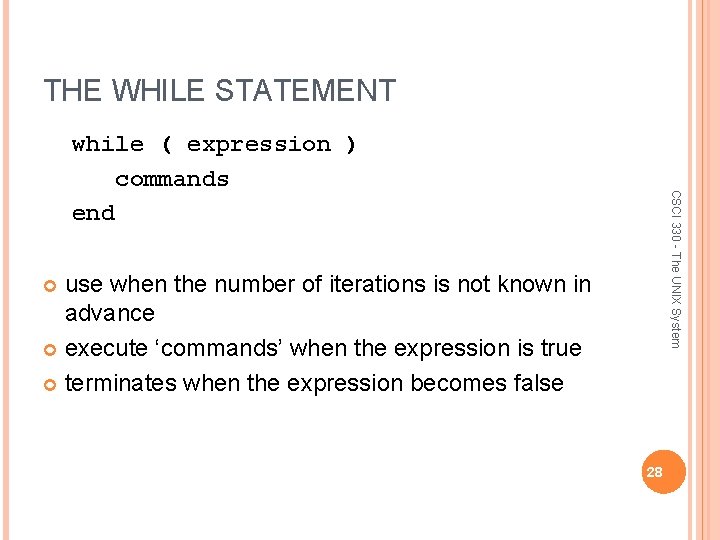
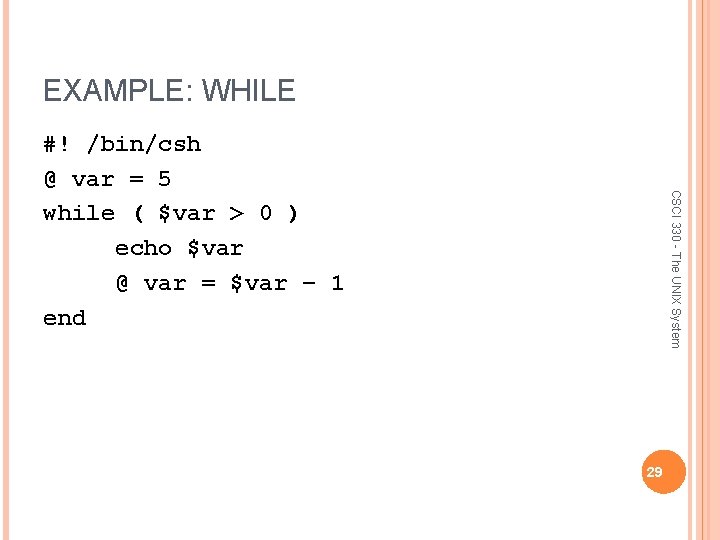
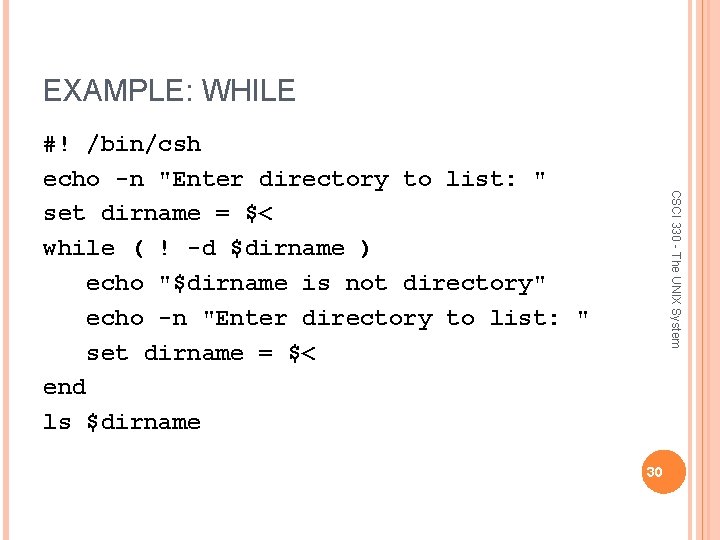
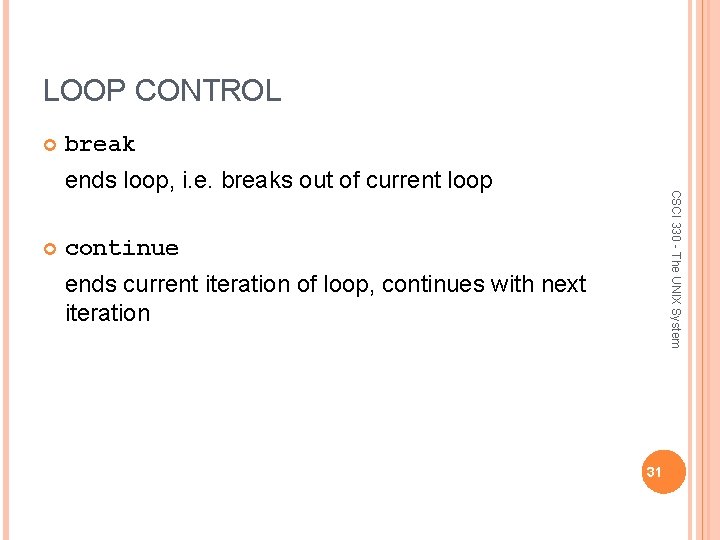
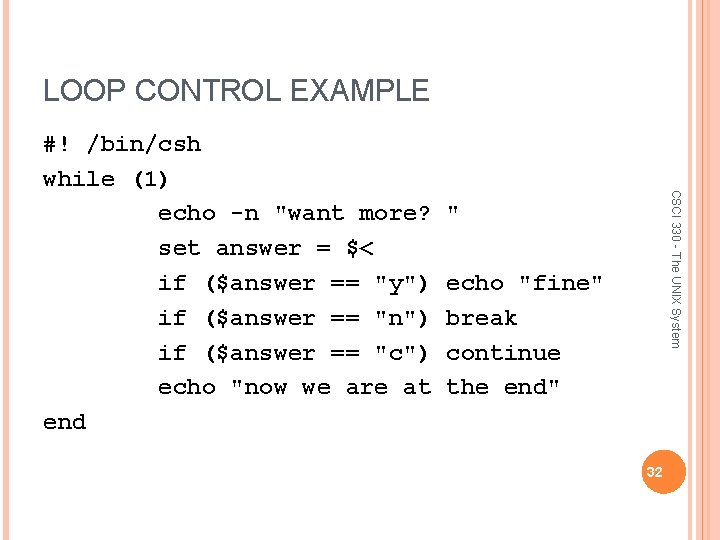
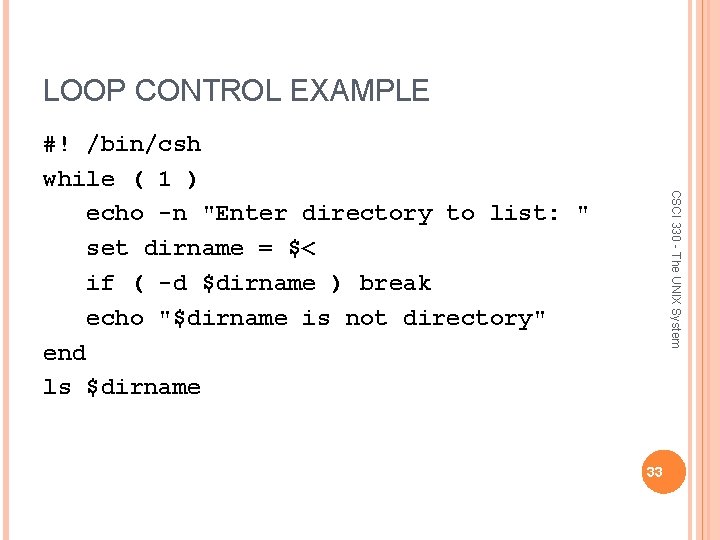
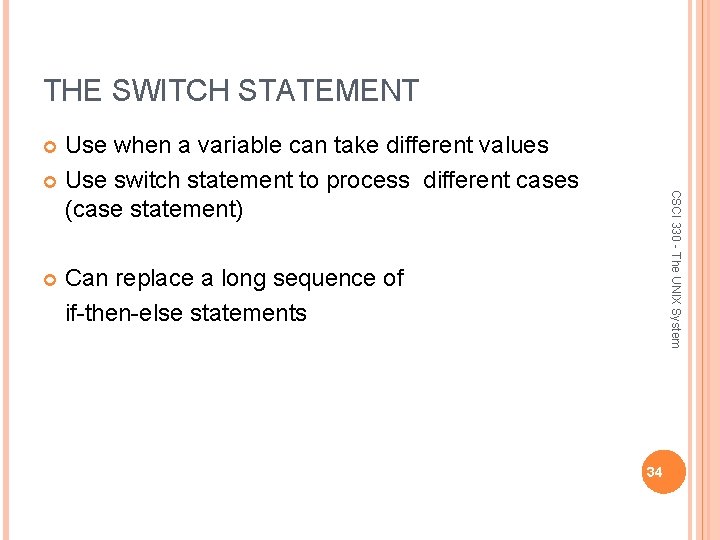
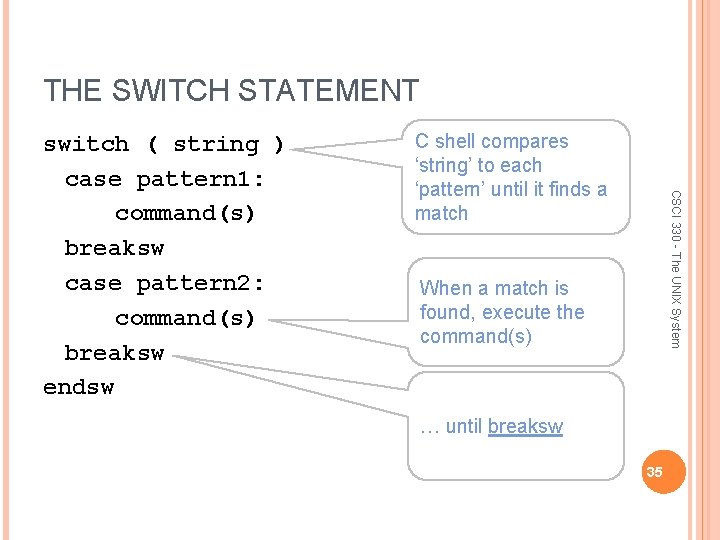
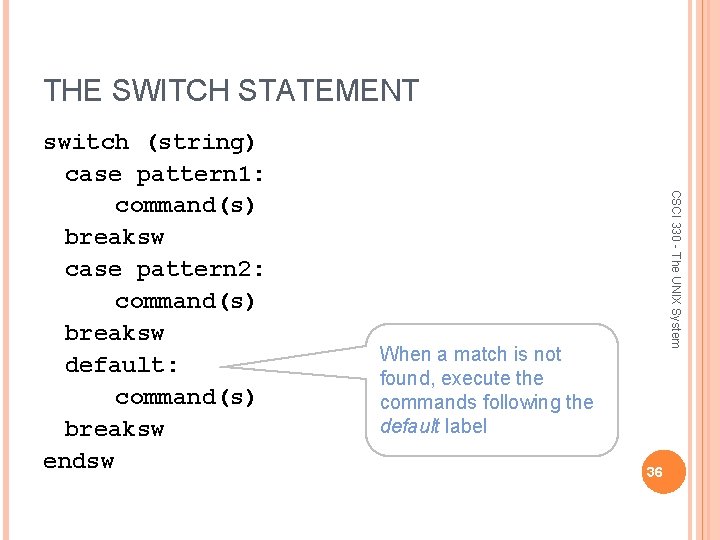
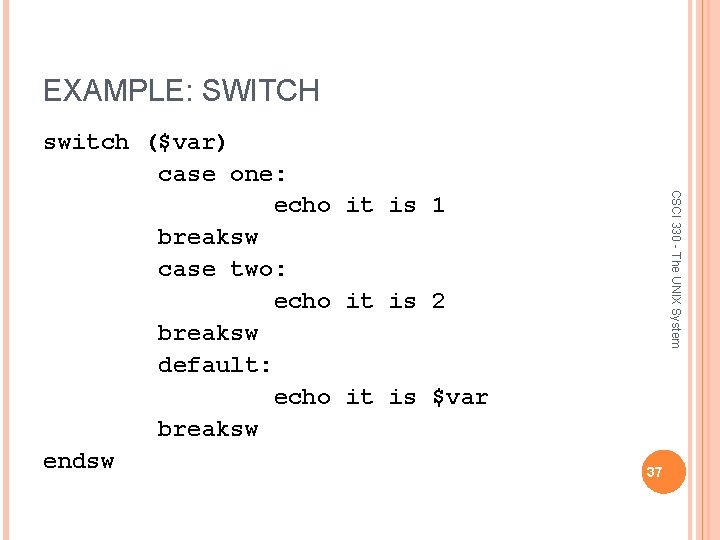
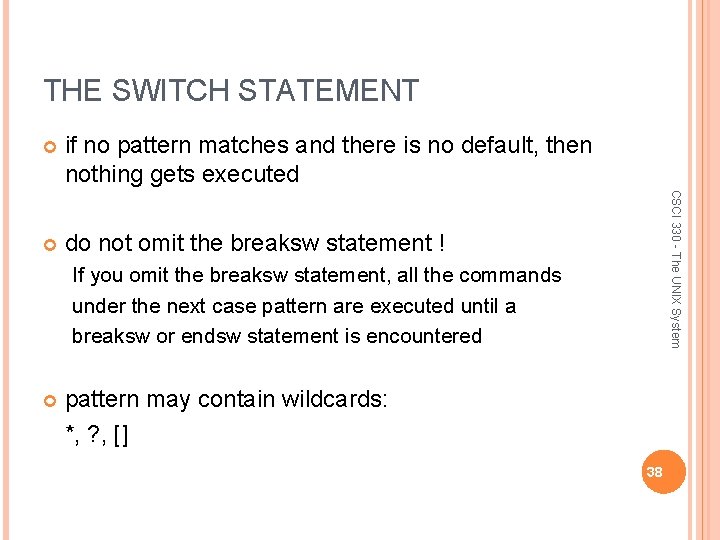
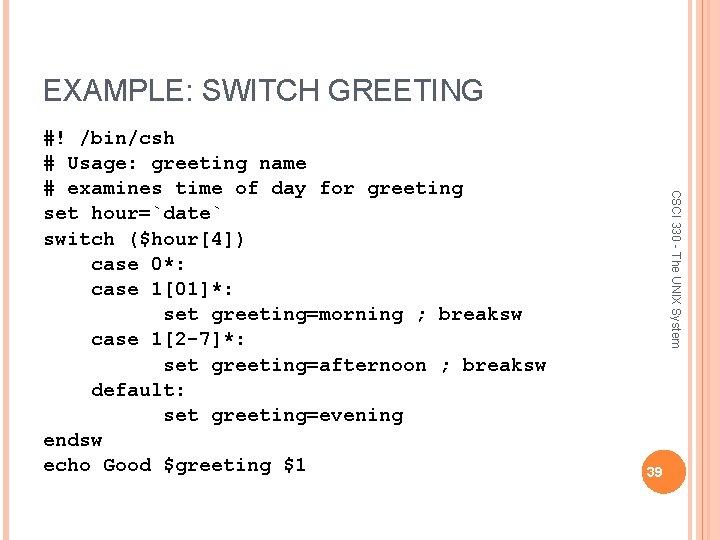
![EXAMPLE C SHELL PROGRAM CSCI 330 - The UNIX System AVAILABLE OPTIONS ********** [1] EXAMPLE C SHELL PROGRAM CSCI 330 - The UNIX System AVAILABLE OPTIONS ********** [1]](https://slidetodoc.com/presentation_image_h/d2c8f951bd1d559f75b5e67861683dda/image-40.jpg)
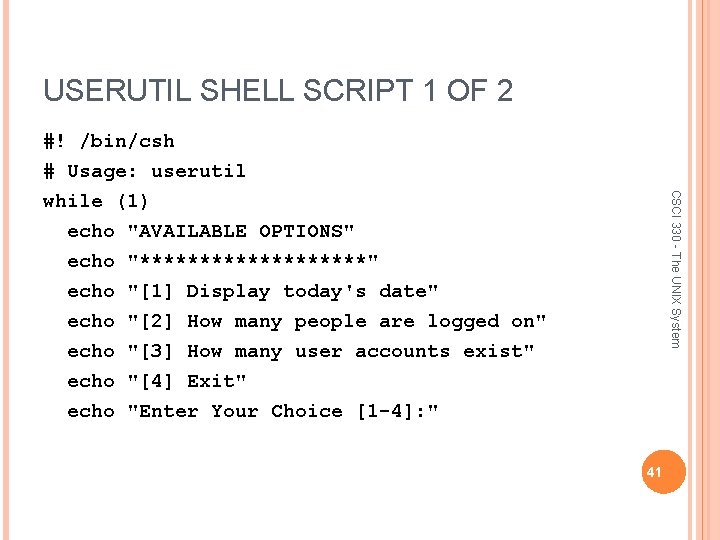
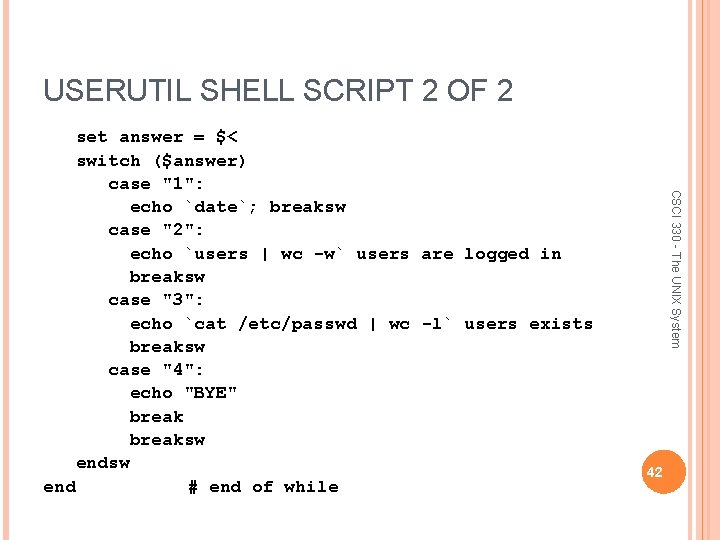
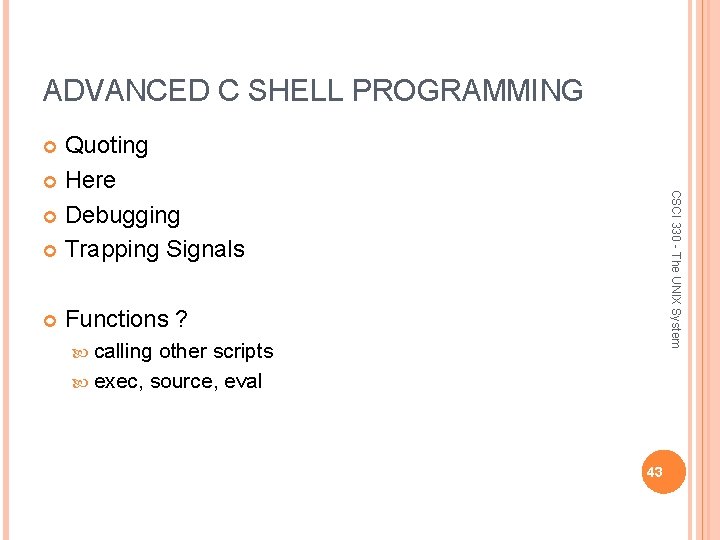
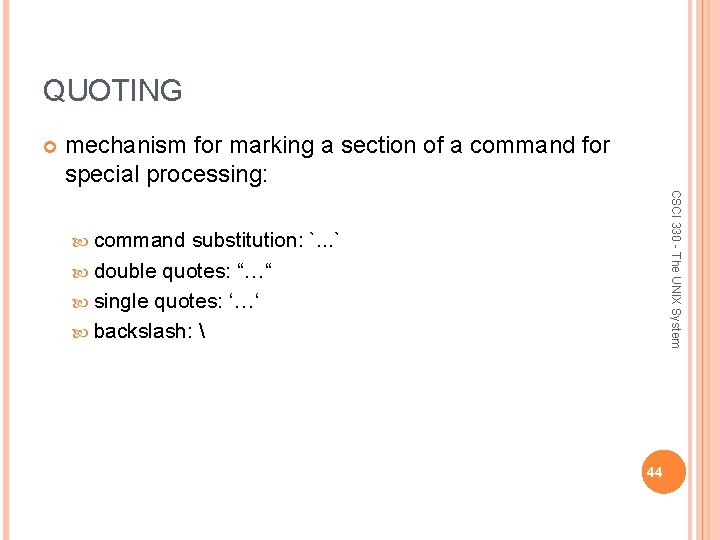
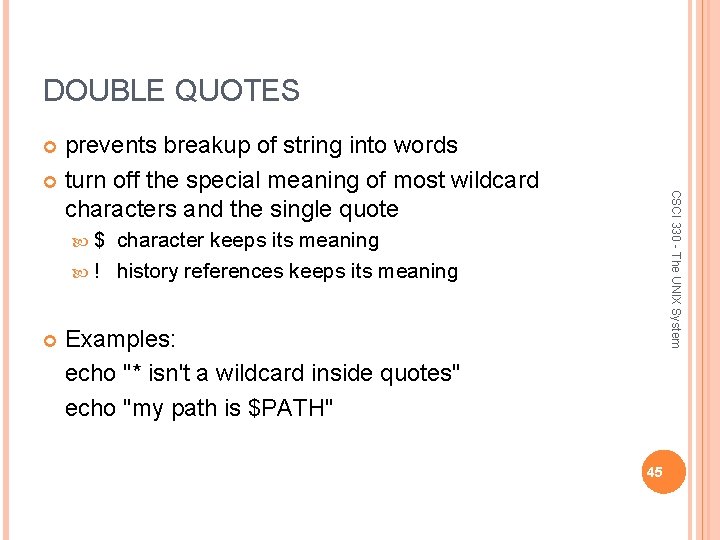
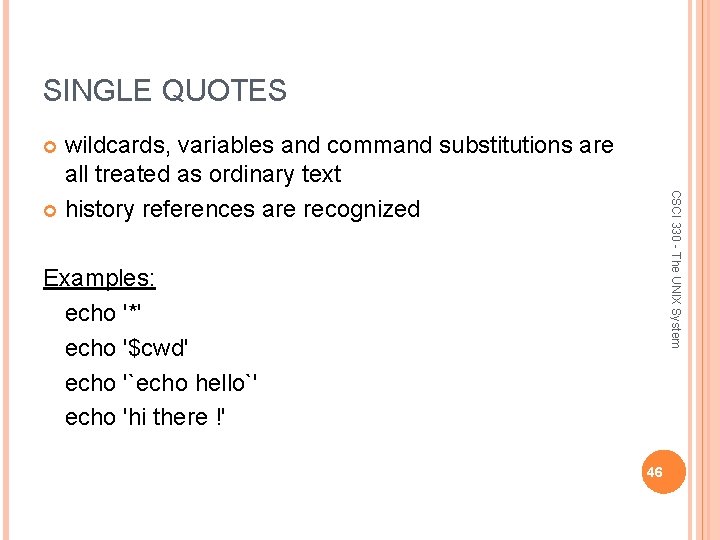
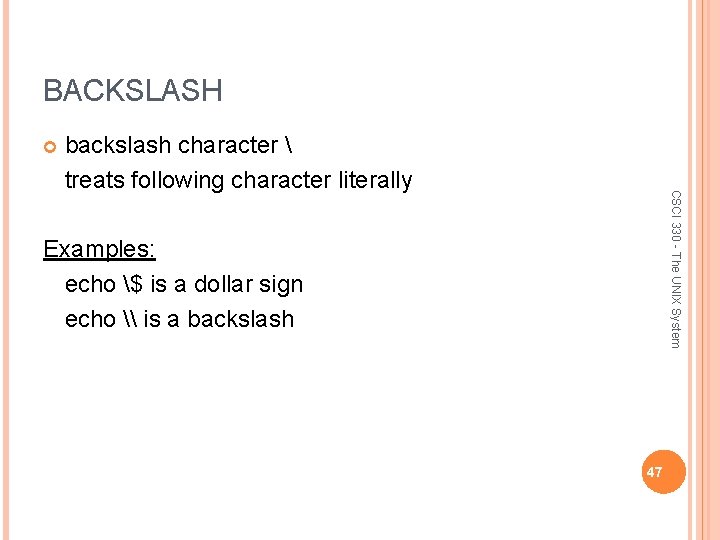
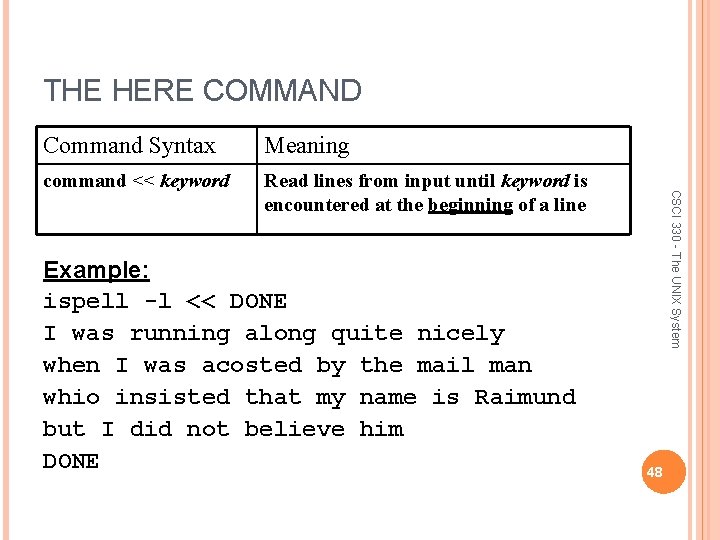
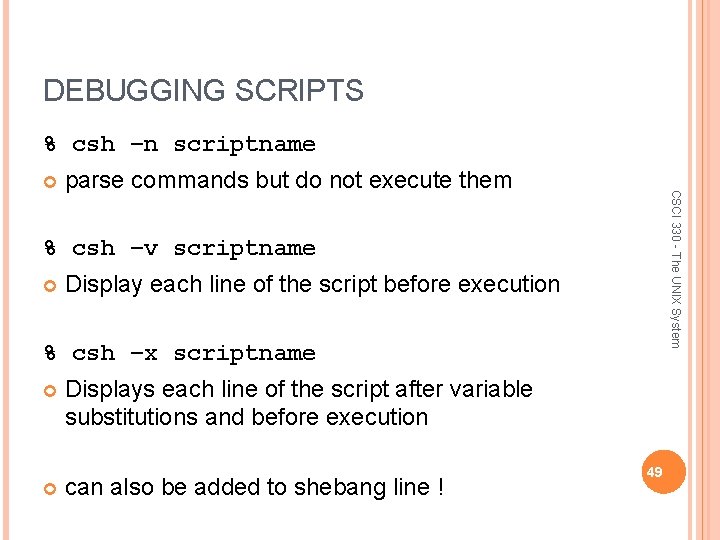
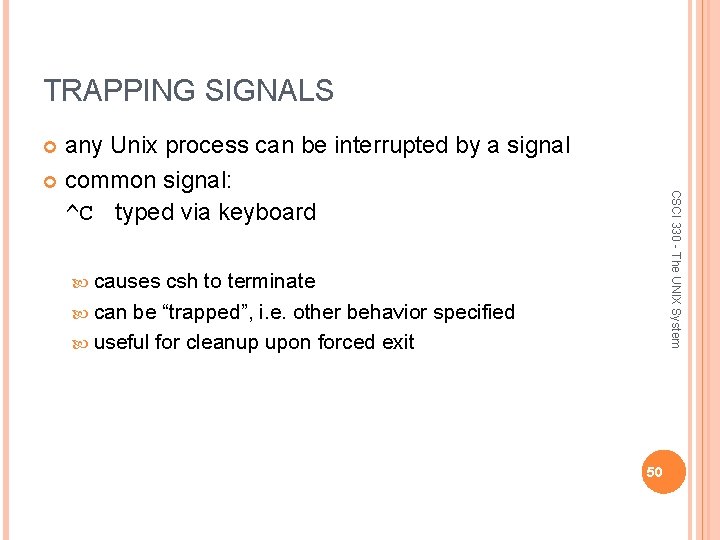
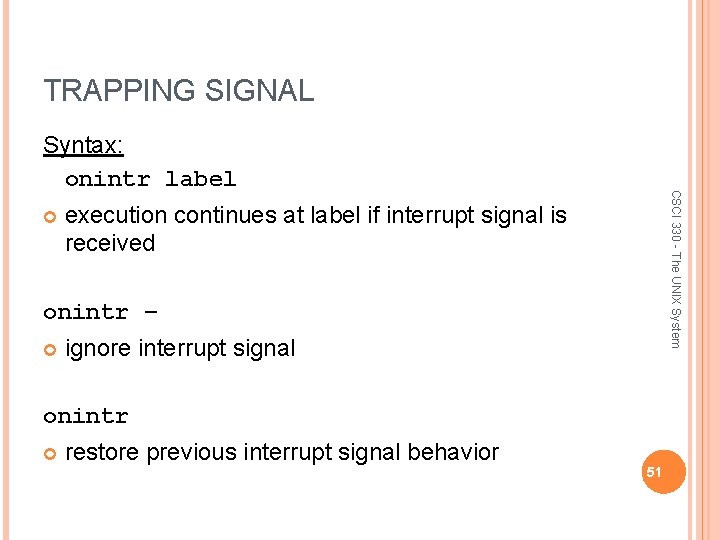
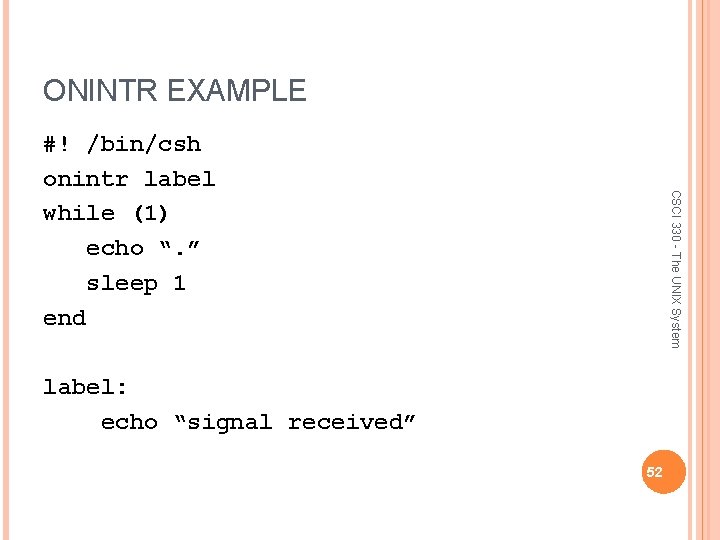
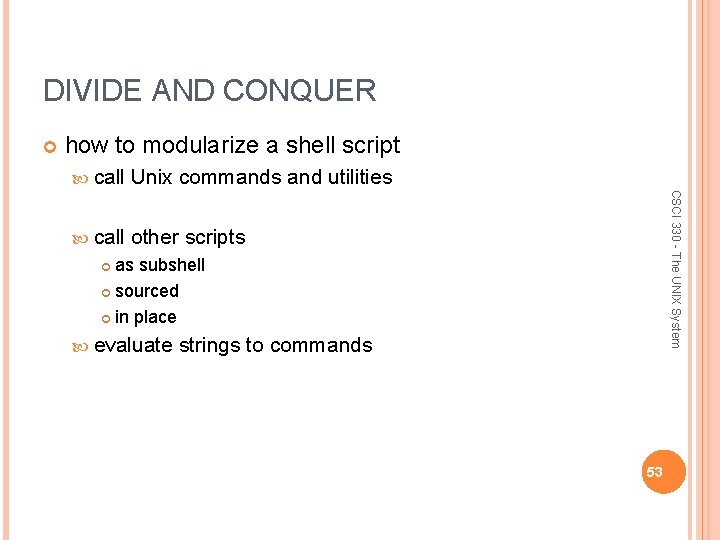
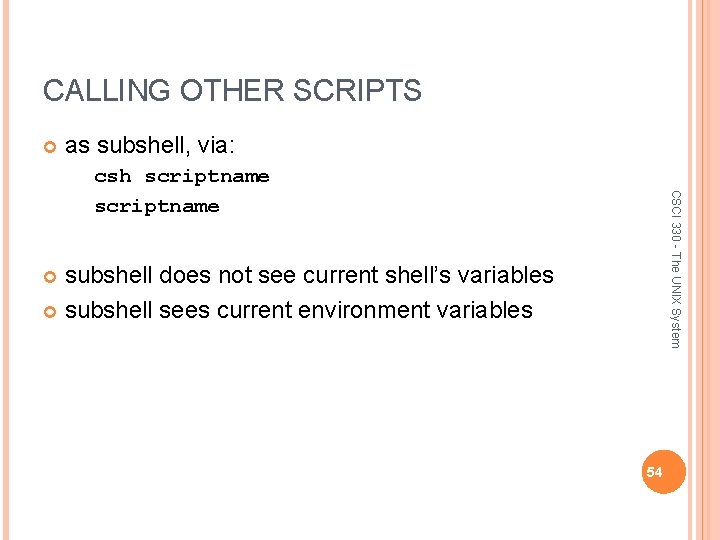
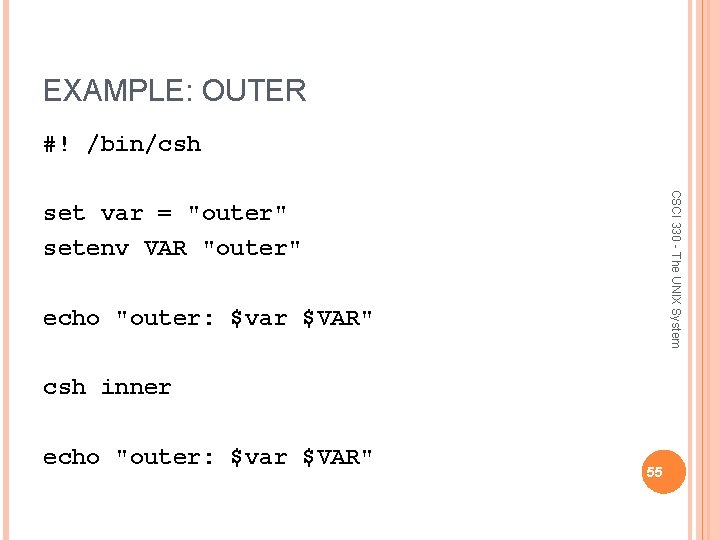
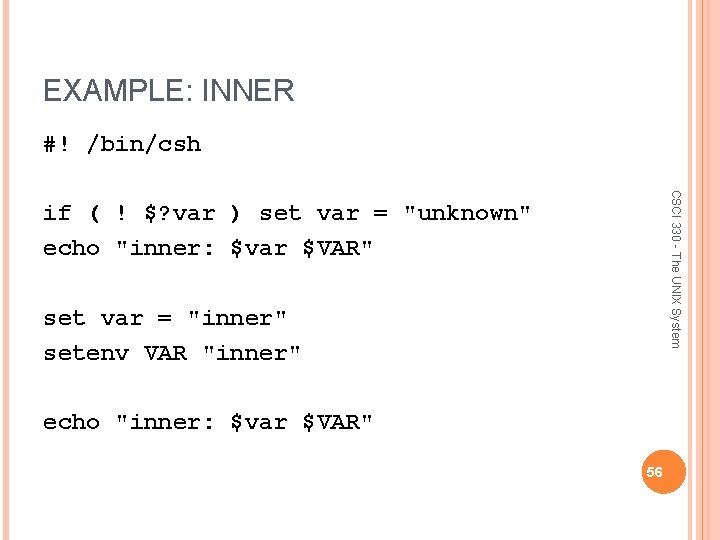
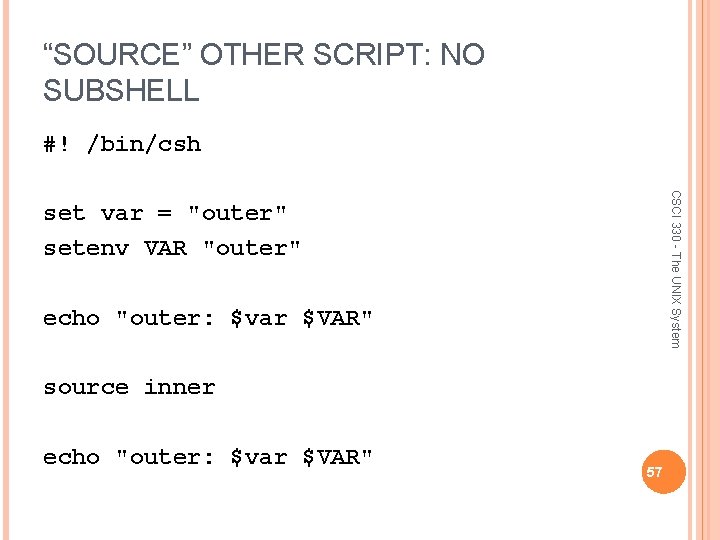
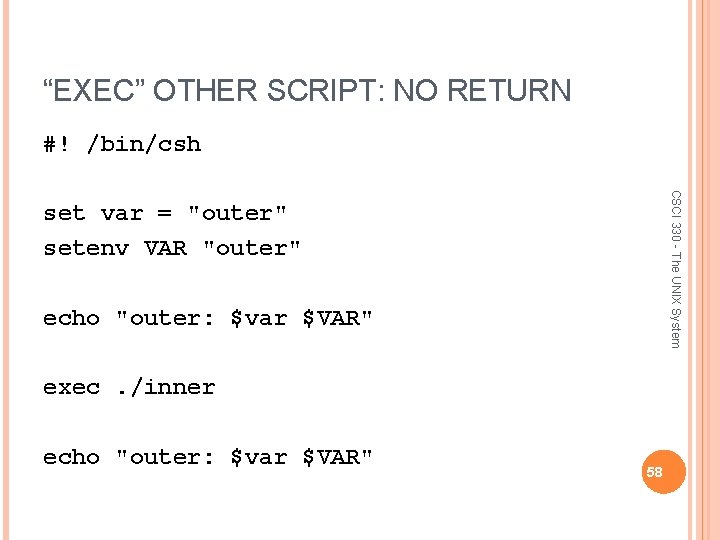
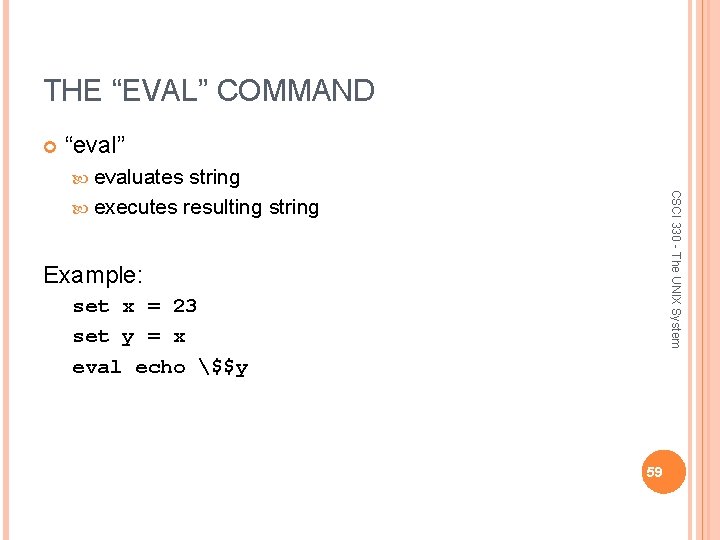
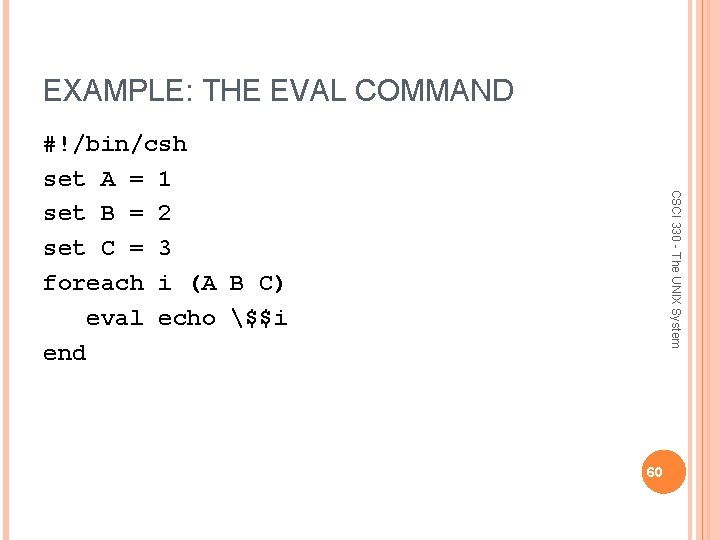
- Slides: 60
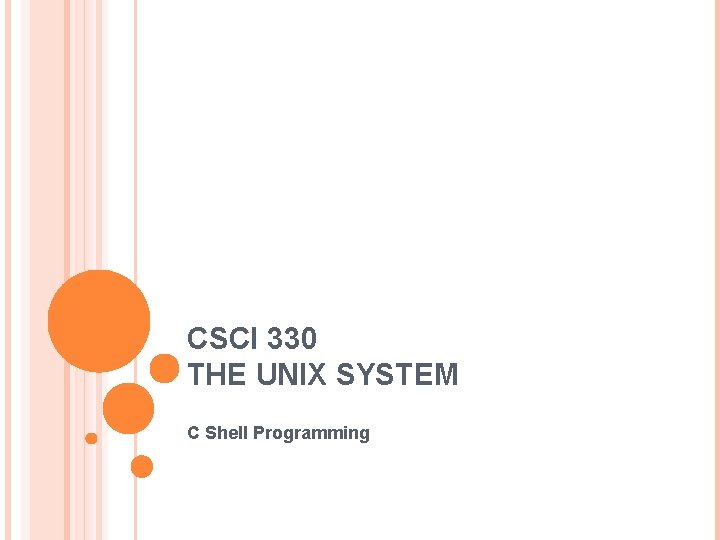
CSCI 330 THE UNIX SYSTEM C Shell Programming
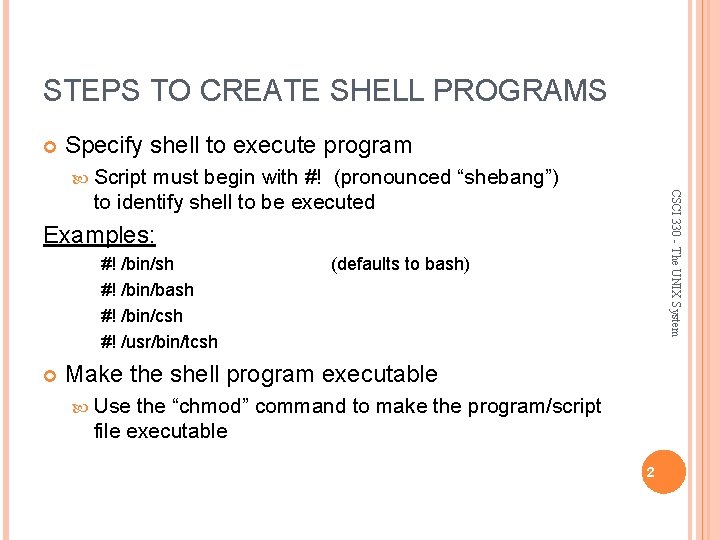
STEPS TO CREATE SHELL PROGRAMS Specify shell to execute program Script CSCI 330 - The UNIX System must begin with #! (pronounced “shebang”) to identify shell to be executed Examples: #! /bin/sh #! /bin/bash #! /bin/csh #! /usr/bin/tcsh (defaults to bash) Make the shell program executable Use the “chmod” command to make the program/script file executable 2
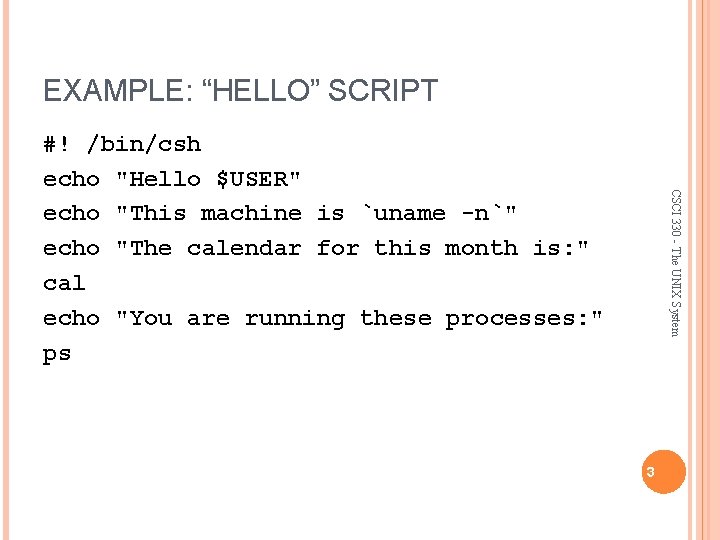
EXAMPLE: “HELLO” SCRIPT CSCI 330 - The UNIX System #! /bin/csh echo "Hello $USER" echo "This machine is `uname -n`" echo "The calendar for this month is: " cal echo "You are running these processes: " ps 3
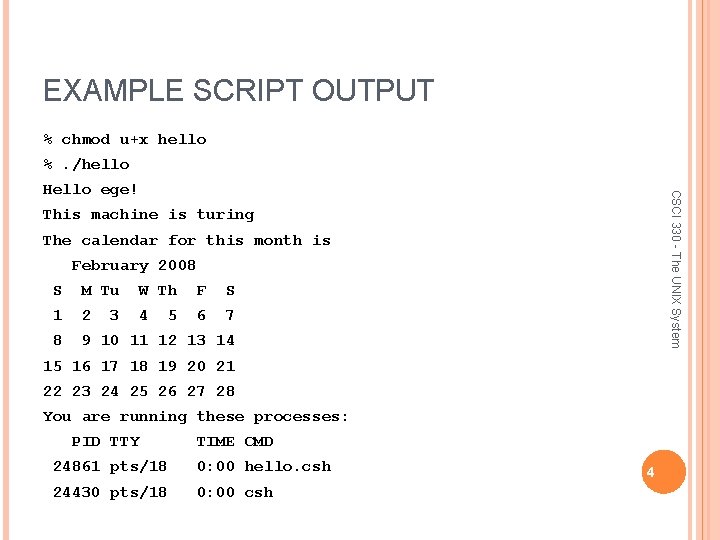
EXAMPLE SCRIPT OUTPUT % chmod u+x hello %. /hello CSCI 330 - The UNIX System Hello ege! This machine is turing The calendar for this month is February 2008 S M Tu W Th F S 1 2 4 6 7 8 9 10 11 12 13 14 3 5 15 16 17 18 19 20 21 22 23 24 25 26 27 28 You are running these processes: PID TTY TIME CMD 24861 pts/18 0: 00 hello. csh 24430 pts/18 0: 00 csh 4
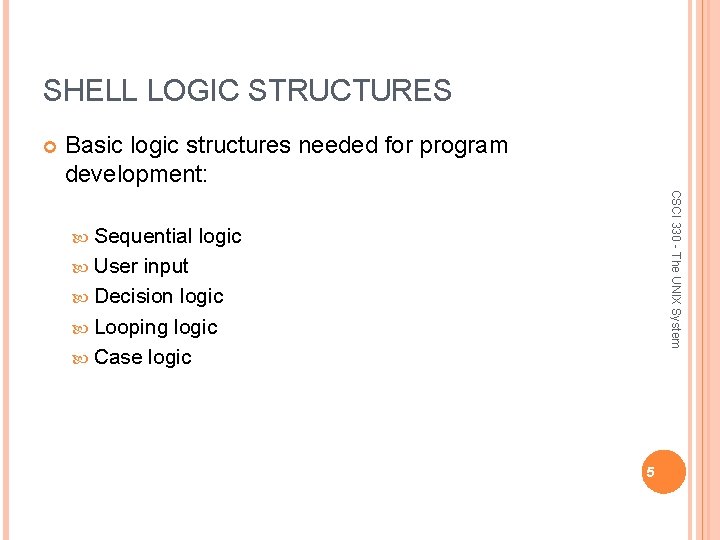
SHELL LOGIC STRUCTURES Basic logic structures needed for program development: CSCI 330 - The UNIX System Sequential logic User input Decision logic Looping logic Case logic 5
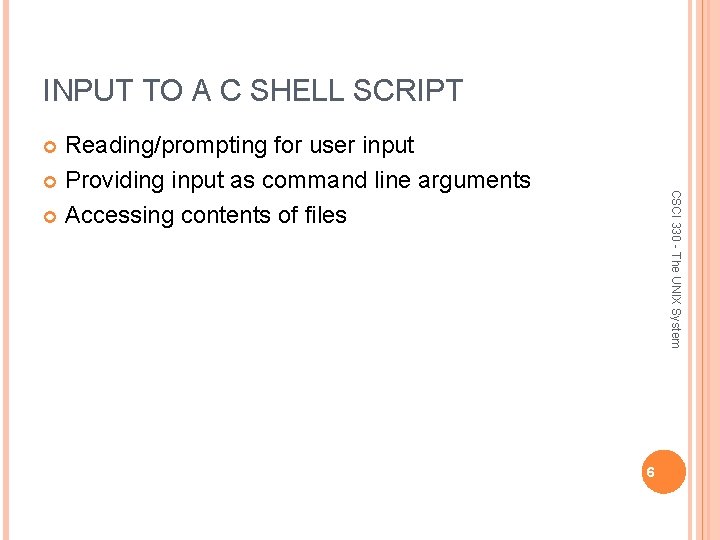
INPUT TO A C SHELL SCRIPT Reading/prompting for user input Providing input as command line arguments Accessing contents of files CSCI 330 - The UNIX System 6
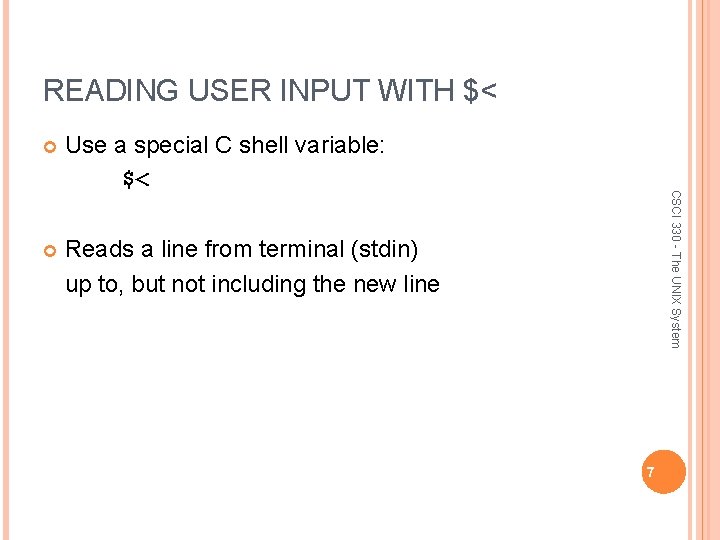
READING USER INPUT WITH $< Use a special C shell variable: $< Reads a line from terminal (stdin) up to, but not including the new line CSCI 330 - The UNIX System 7
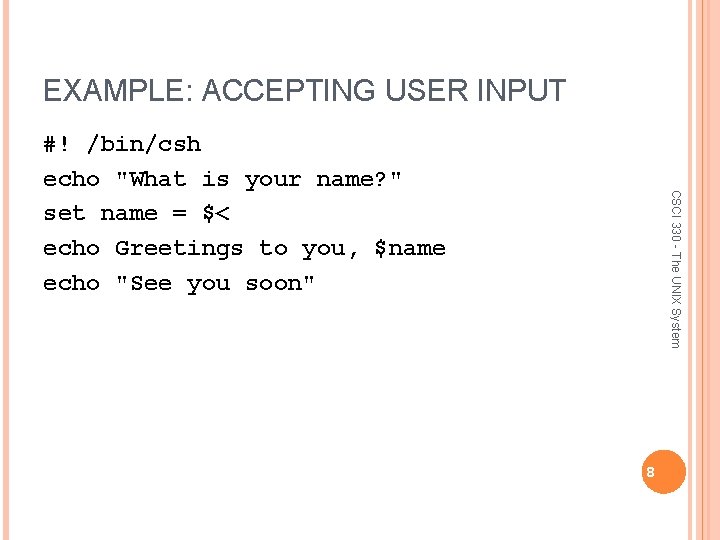
EXAMPLE: ACCEPTING USER INPUT CSCI 330 - The UNIX System #! /bin/csh echo "What is your name? " set name = $< echo Greetings to you, $name echo "See you soon" 8
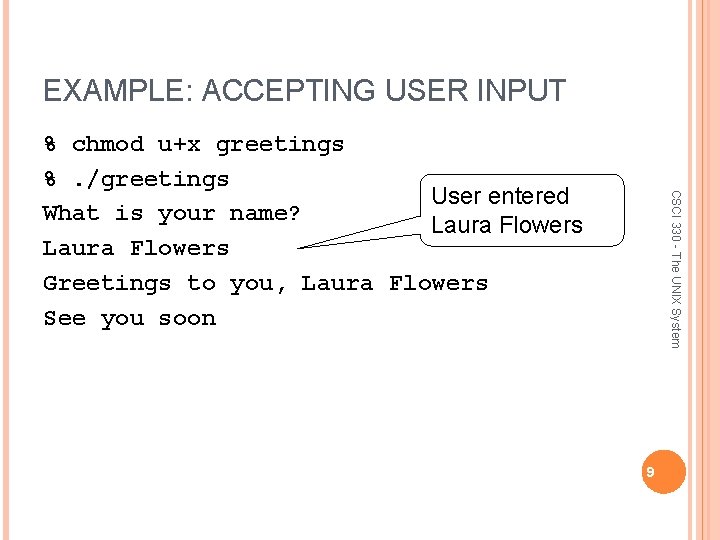
EXAMPLE: ACCEPTING USER INPUT CSCI 330 - The UNIX System % chmod u+x greetings %. /greetings User entered What is your name? Laura Flowers Greetings to you, Laura Flowers See you soon 9
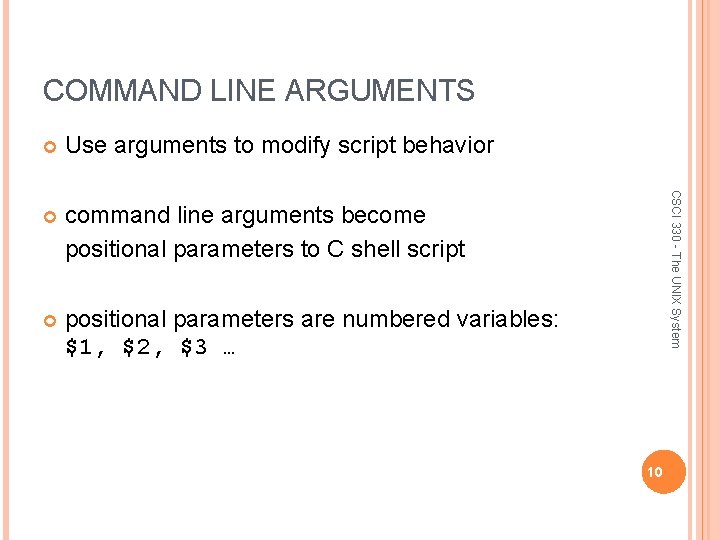
COMMAND LINE ARGUMENTS Use arguments to modify script behavior command line arguments become positional parameters to C shell script positional parameters are numbered variables: $1, $2, $3 … CSCI 330 - The UNIX System 10
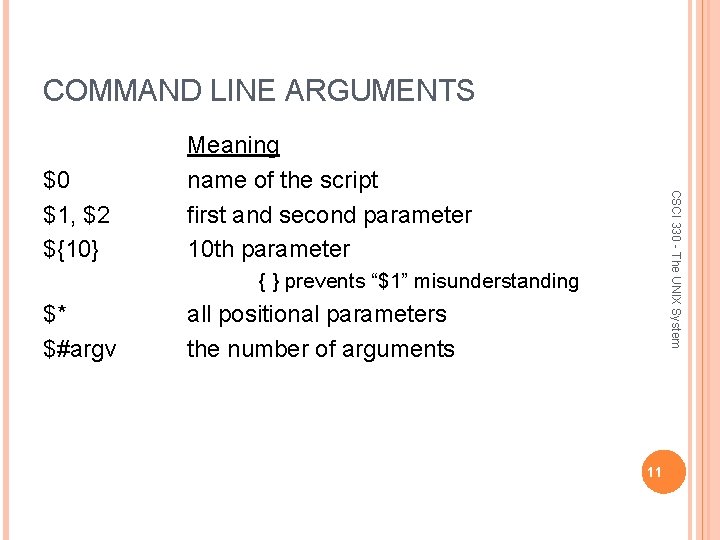
COMMAND LINE ARGUMENTS CSCI 330 - The UNIX System $0 $1, $2 ${10} Meaning name of the script first and second parameter 10 th parameter { } prevents “$1” misunderstanding $* $#argv all positional parameters the number of arguments 11
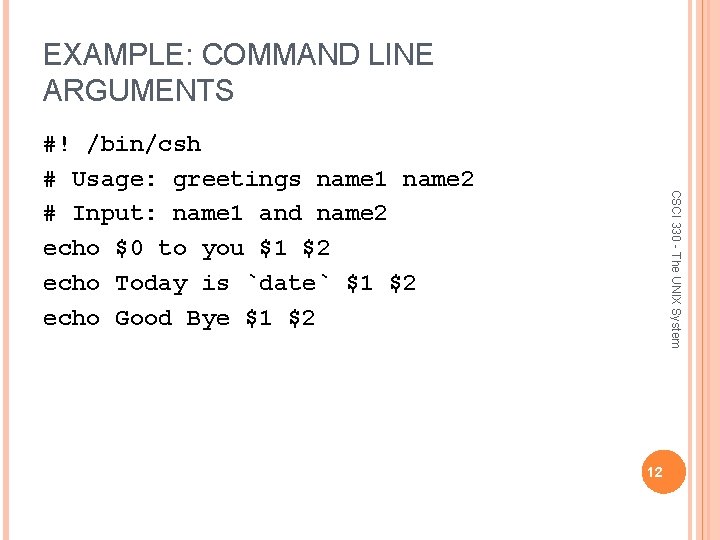
EXAMPLE: COMMAND LINE ARGUMENTS CSCI 330 - The UNIX System #! /bin/csh # Usage: greetings name 1 name 2 # Input: name 1 and name 2 echo $0 to you $1 $2 echo Today is `date` $1 $2 echo Good Bye $1 $2 12
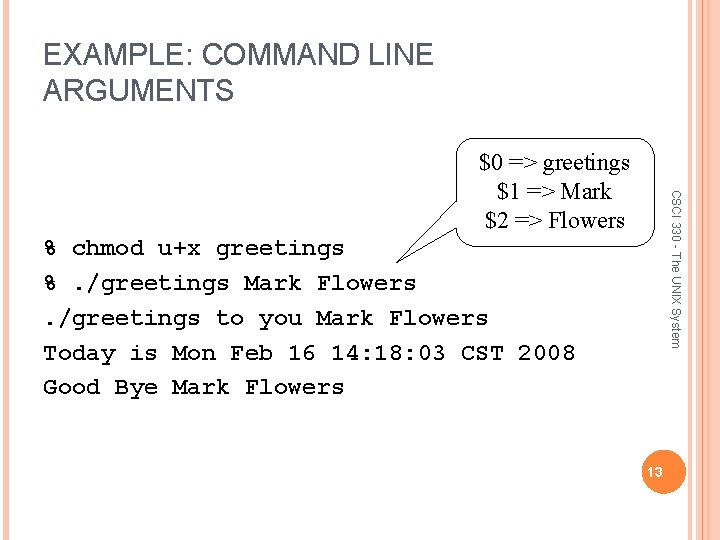
EXAMPLE: COMMAND LINE ARGUMENTS CSCI 330 - The UNIX System $0 => greetings $1 => Mark $2 => Flowers % chmod u+x greetings %. /greetings Mark Flowers. /greetings to you Mark Flowers Today is Mon Feb 16 14: 18: 03 CST 2008 Good Bye Mark Flowers 13
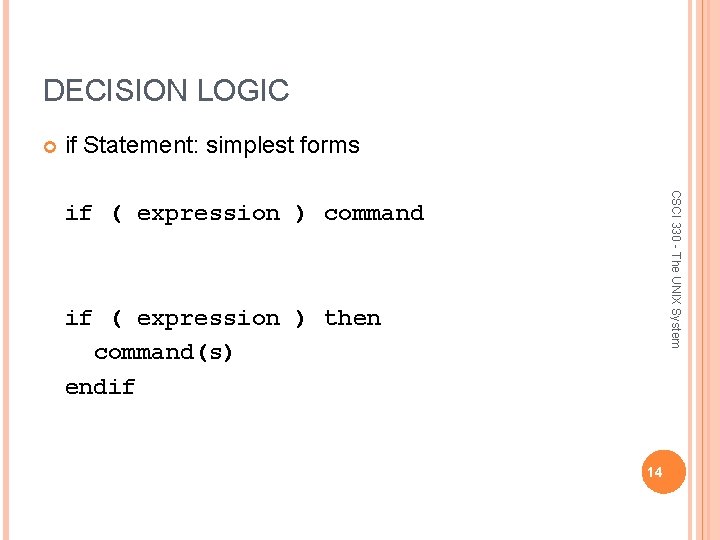
DECISION LOGIC if Statement: simplest forms CSCI 330 - The UNIX System if ( expression ) command if ( expression ) then command(s) endif 14
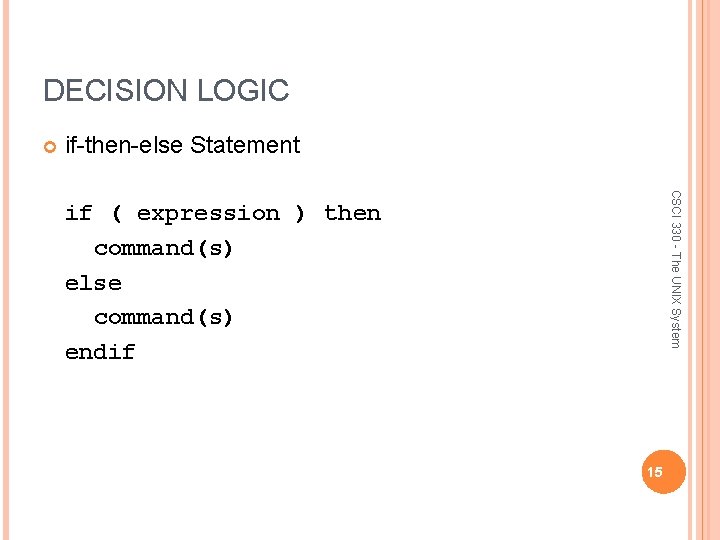
DECISION LOGIC if-then-else Statement CSCI 330 - The UNIX System if ( expression ) then command(s) else command(s) endif 15
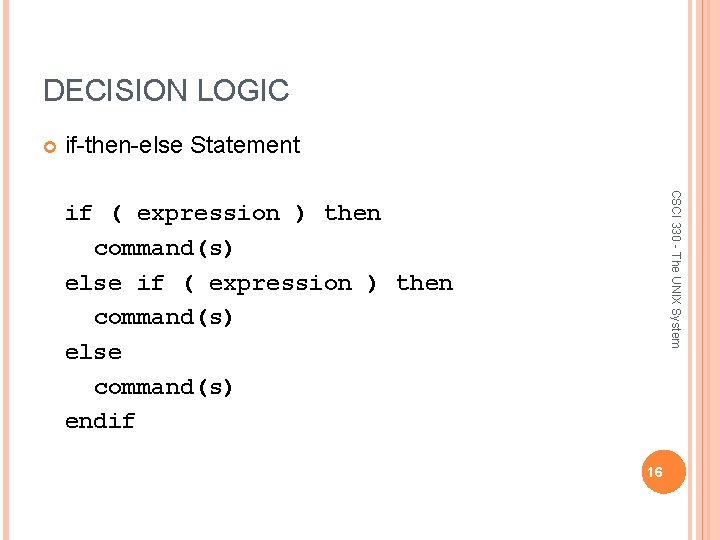
DECISION LOGIC if-then-else Statement CSCI 330 - The UNIX System if ( expression ) then command(s) else command(s) endif 16
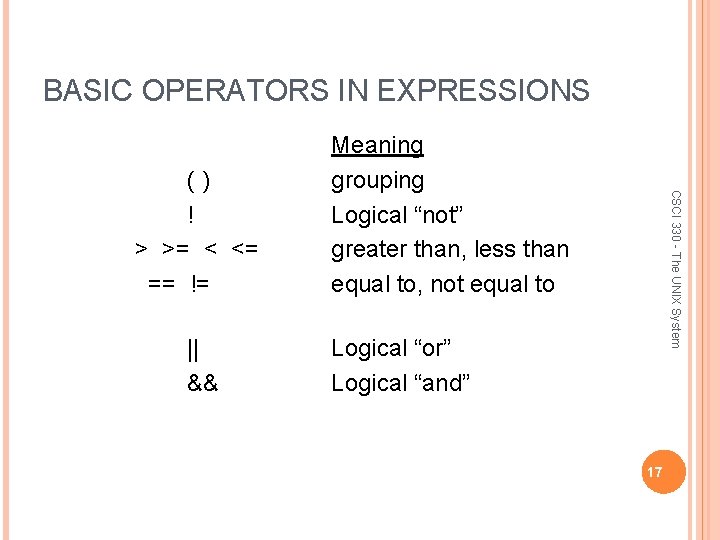
BASIC OPERATORS IN EXPRESSIONS || && CSCI 330 - The UNIX System () ! > >= < <= == != Meaning grouping Logical “not” greater than, less than equal to, not equal to Logical “or” Logical “and” 17
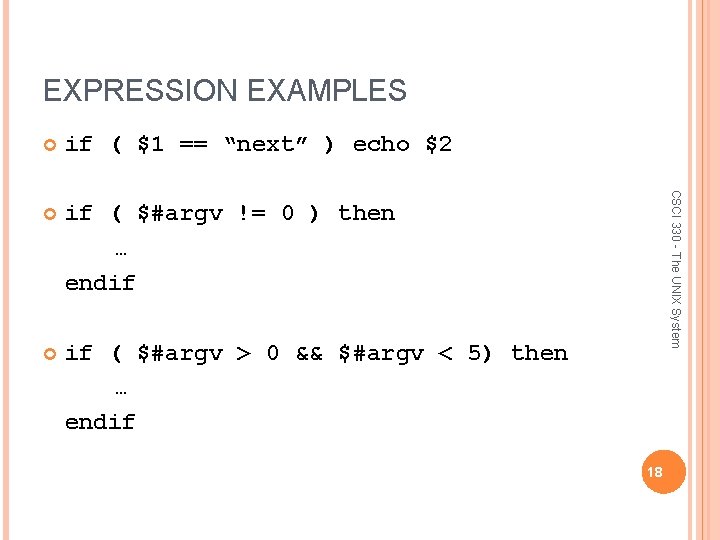
EXPRESSION EXAMPLES if ( $1 == “next” ) echo $2 if ( $#argv != 0 ) then … endif if ( $#argv > 0 && $#argv < 5) then … endif CSCI 330 - The UNIX System 18
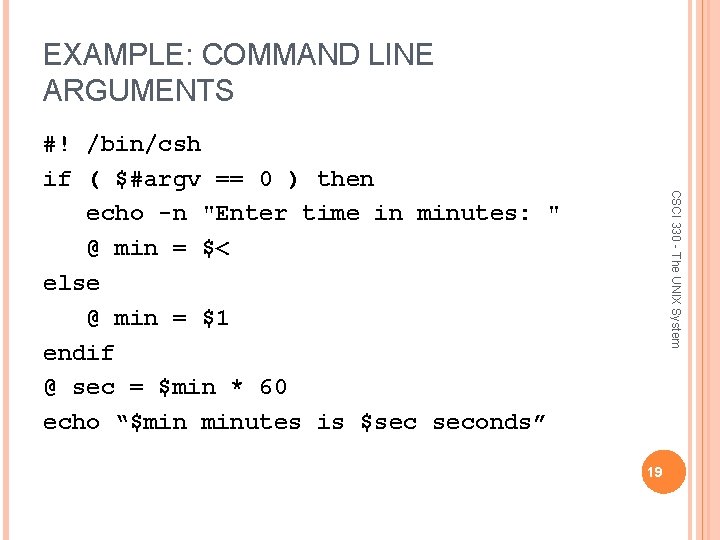
EXAMPLE: COMMAND LINE ARGUMENTS CSCI 330 - The UNIX System #! /bin/csh if ( $#argv == 0 ) then echo -n "Enter time in minutes: " @ min = $< else @ min = $1 endif @ sec = $min * 60 echo “$min minutes is $sec seconds” 19
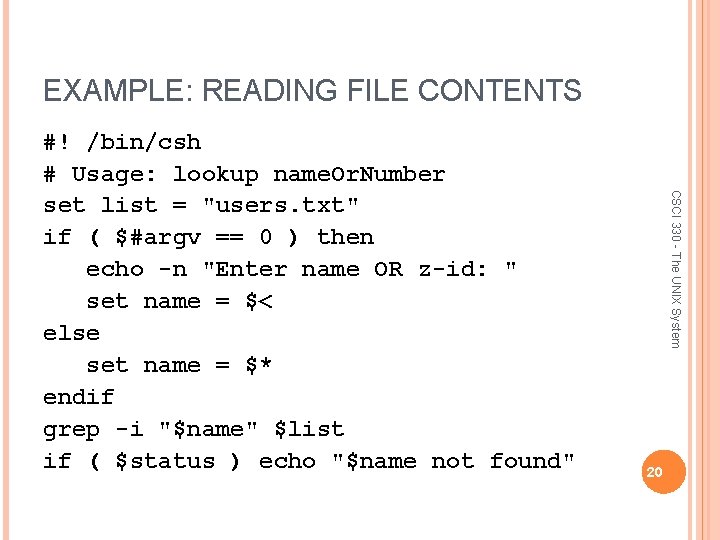
EXAMPLE: READING FILE CONTENTS CSCI 330 - The UNIX System #! /bin/csh # Usage: lookup name. Or. Number set list = "users. txt" if ( $#argv == 0 ) then echo -n "Enter name OR z-id: " set name = $< else set name = $* endif grep -i "$name" $list if ( $status ) echo "$name not found" 20
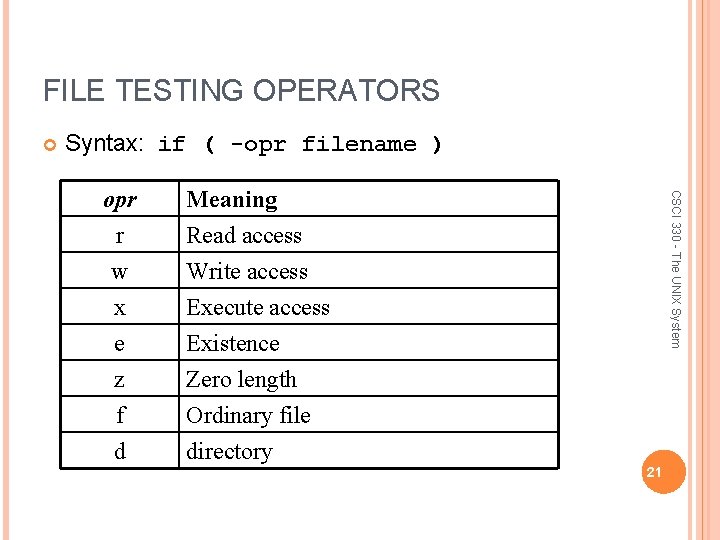
FILE TESTING OPERATORS Syntax: if ( -opr filename ) e z f d Meaning Read access Write access Execute access CSCI 330 - The UNIX System opr r w x Existence Zero length Ordinary file directory 21
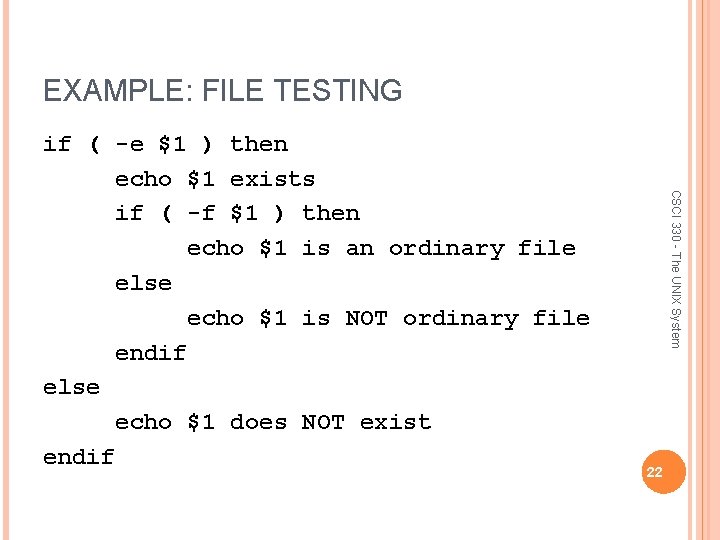
EXAMPLE: FILE TESTING CSCI 330 - The UNIX System if ( -e $1 ) then echo $1 exists if ( -f $1 ) then echo $1 is an ordinary file else echo $1 is NOT ordinary file endif else echo $1 does NOT exist endif 22
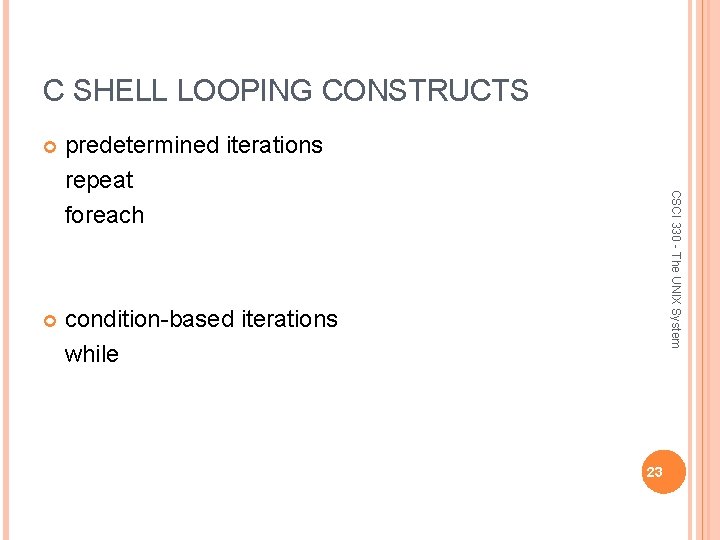
C SHELL LOOPING CONSTRUCTS predetermined iterations repeat foreach condition-based iterations while CSCI 330 - The UNIX System 23
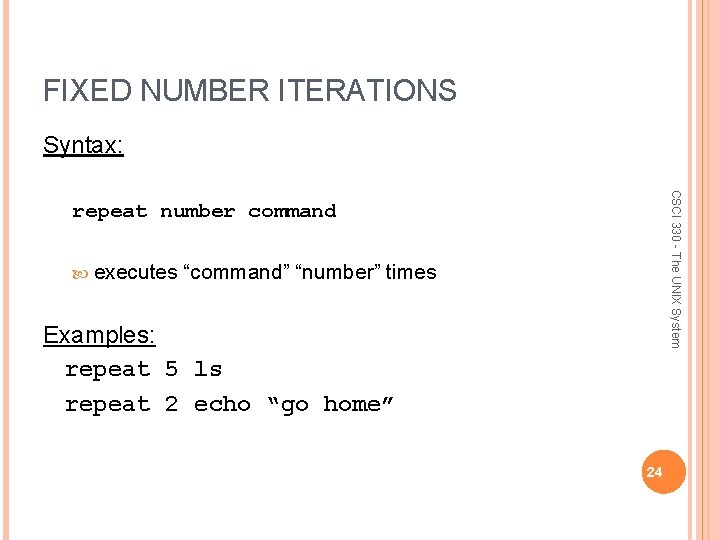
FIXED NUMBER ITERATIONS Syntax: CSCI 330 - The UNIX System repeat number command executes “command” “number” times Examples: repeat 5 ls repeat 2 echo “go home” 24
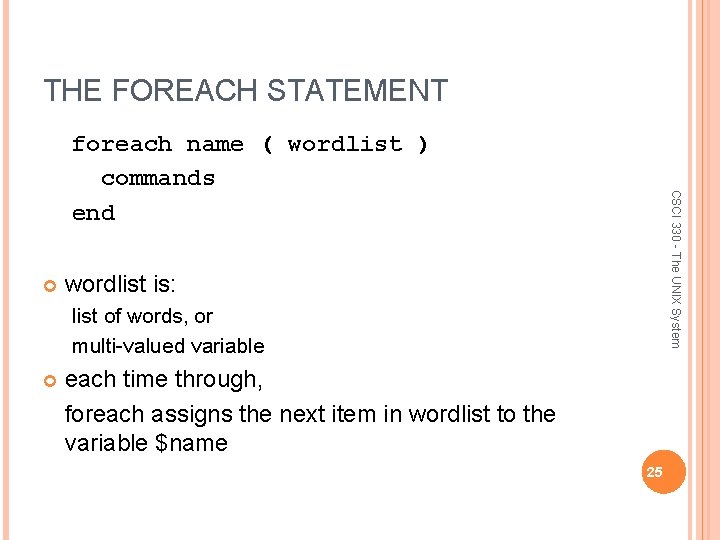
THE FOREACH STATEMENT CSCI 330 - The UNIX System foreach name ( wordlist ) commands end wordlist is: list of words, or multi-valued variable each time through, foreach assigns the next item in wordlist to the variable $name 25
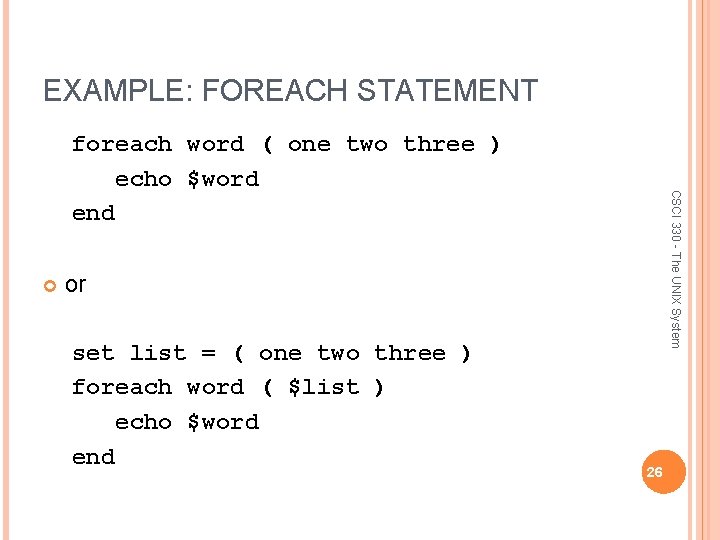
EXAMPLE: FOREACH STATEMENT CSCI 330 - The UNIX System foreach word ( one two three ) echo $word end or set list = ( one two three ) foreach word ( $list ) echo $word end 26
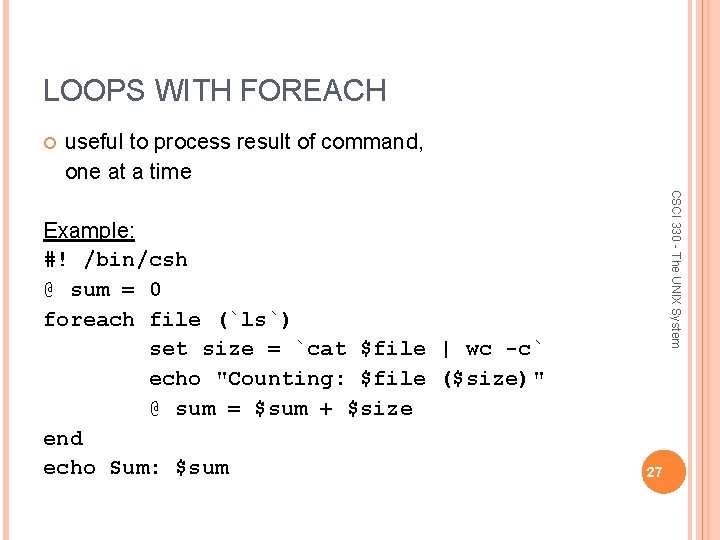
LOOPS WITH FOREACH useful to process result of command, one at a time CSCI 330 - The UNIX System Example: #! /bin/csh @ sum = 0 foreach file (`ls`) set size = `cat $file | wc -c` echo "Counting: $file ($size)" @ sum = $sum + $size end echo Sum: $sum 27
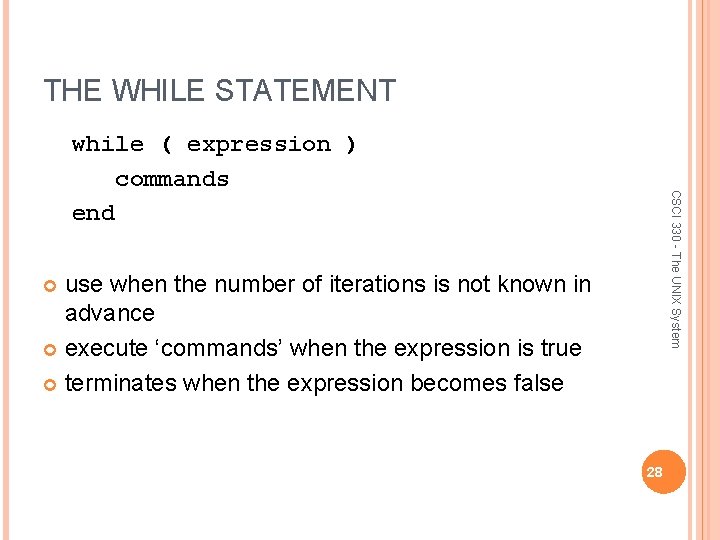
THE WHILE STATEMENT CSCI 330 - The UNIX System while ( expression ) commands end use when the number of iterations is not known in advance execute ‘commands’ when the expression is true terminates when the expression becomes false 28
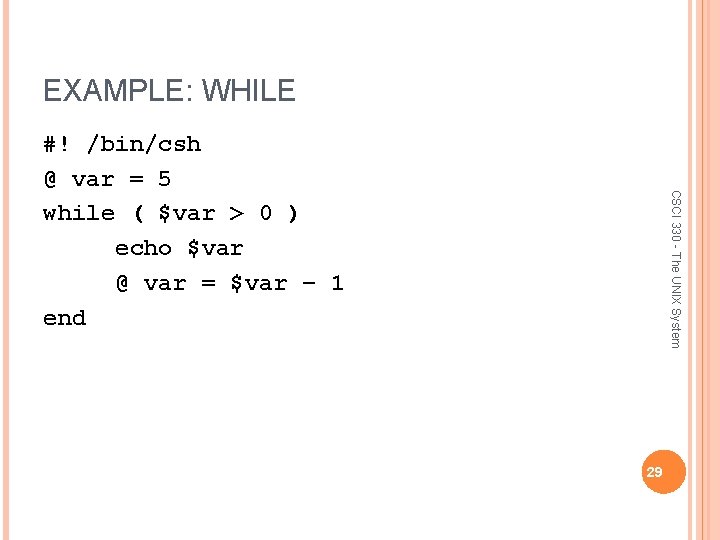
EXAMPLE: WHILE CSCI 330 - The UNIX System #! /bin/csh @ var = 5 while ( $var > 0 ) echo $var @ var = $var – 1 end 29
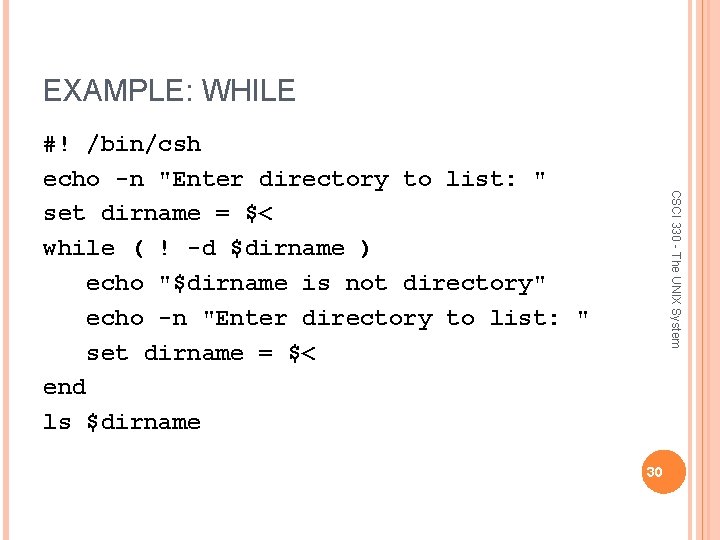
EXAMPLE: WHILE CSCI 330 - The UNIX System #! /bin/csh echo -n "Enter directory to list: " set dirname = $< while ( ! -d $dirname ) echo "$dirname is not directory" echo -n "Enter directory to list: " set dirname = $< end ls $dirname 30
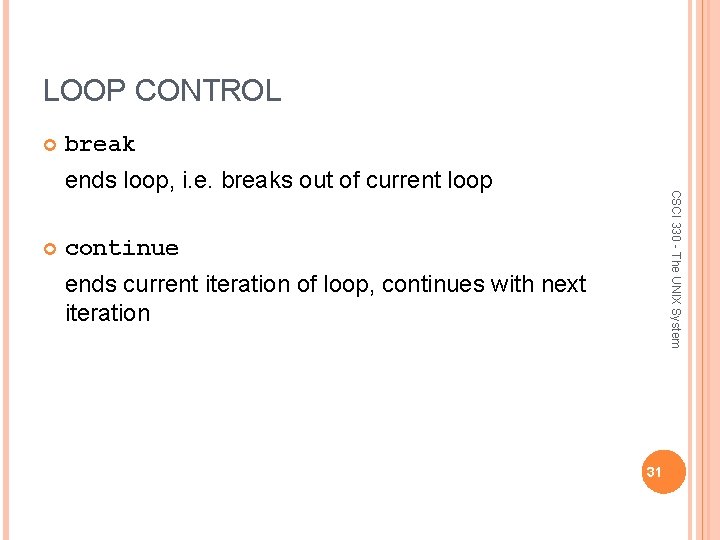
LOOP CONTROL break CSCI 330 - The UNIX System ends loop, i. e. breaks out of current loop continue ends current iteration of loop, continues with next iteration 31
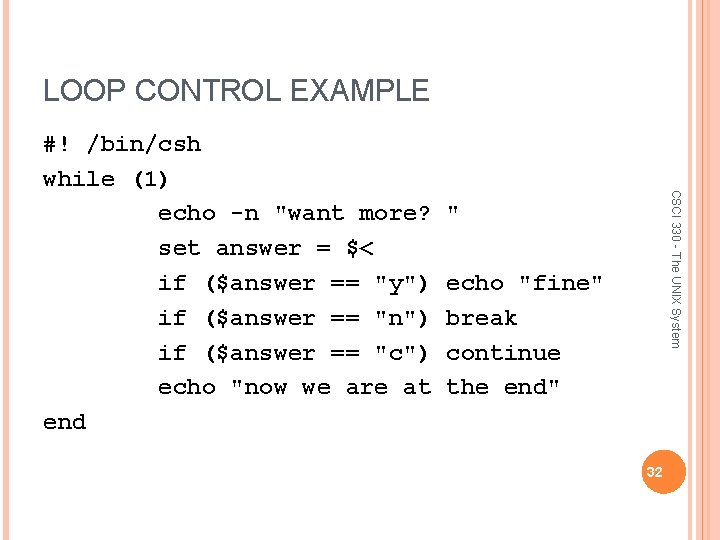
LOOP CONTROL EXAMPLE CSCI 330 - The UNIX System #! /bin/csh while (1) echo -n "want more? set answer = $< if ($answer == "y") if ($answer == "n") if ($answer == "c") echo "now we are at end " echo "fine" break continue the end" 32
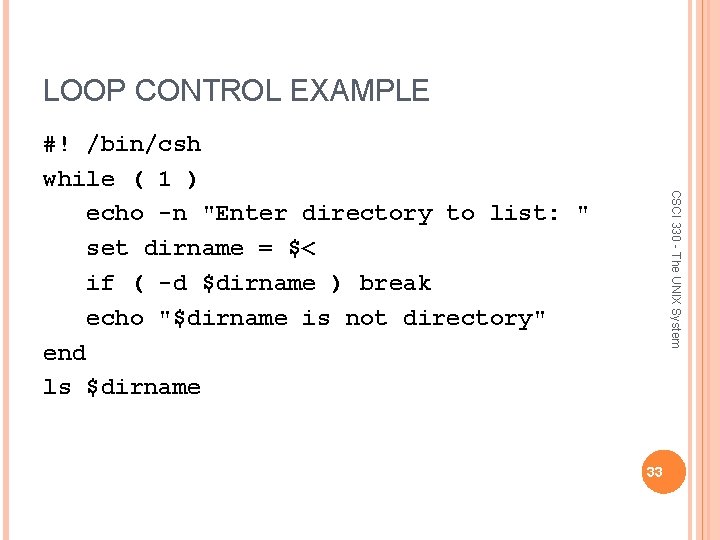
LOOP CONTROL EXAMPLE CSCI 330 - The UNIX System #! /bin/csh while ( 1 ) echo -n "Enter directory to list: " set dirname = $< if ( -d $dirname ) break echo "$dirname is not directory" end ls $dirname 33
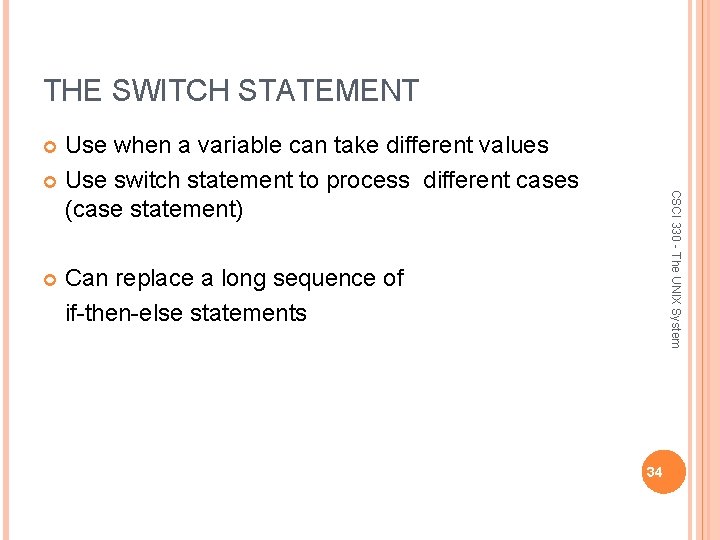
THE SWITCH STATEMENT Use when a variable can take different values Use switch statement to process different cases (case statement) CSCI 330 - The UNIX System Can replace a long sequence of if-then-else statements 34
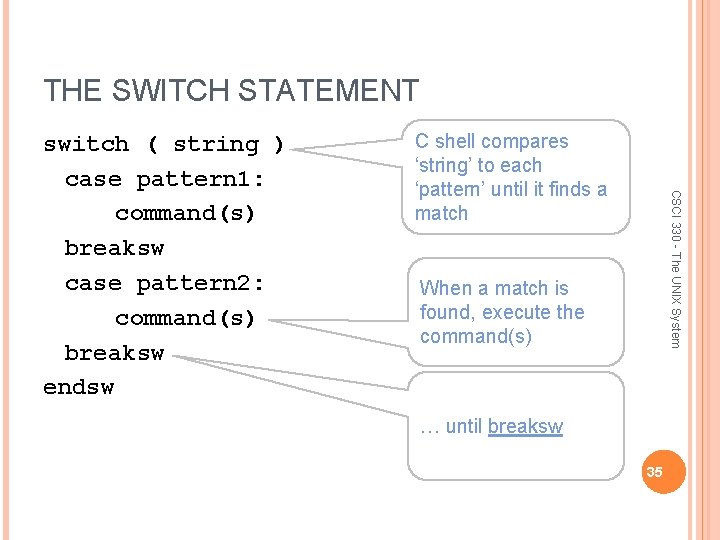
THE SWITCH STATEMENT C shell compares ‘string’ to each ‘pattern’ until it finds a match CSCI 330 - The UNIX System switch ( string ) case pattern 1: command(s) breaksw case pattern 2: command(s) breaksw endsw When a match is found, execute the command(s) … until breaksw 35
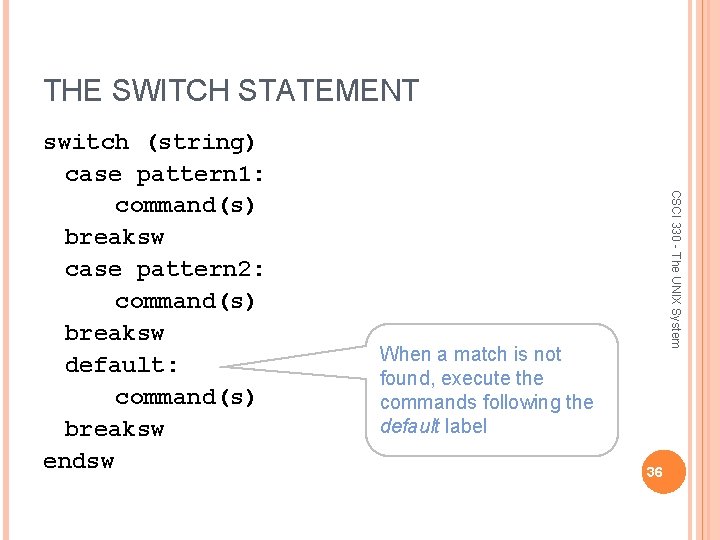
THE SWITCH STATEMENT CSCI 330 - The UNIX System switch (string) case pattern 1: command(s) breaksw case pattern 2: command(s) breaksw default: command(s) breaksw endsw When a match is not found, execute the commands following the default label 36
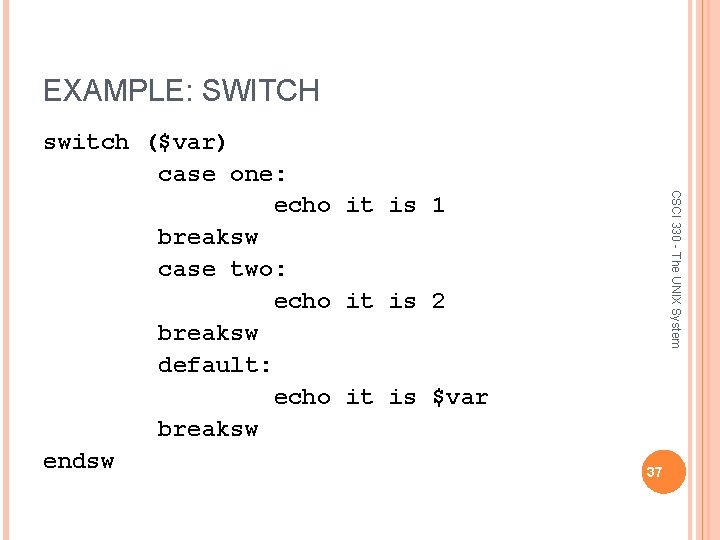
EXAMPLE: SWITCH CSCI 330 - The UNIX System switch ($var) case one: echo it is 1 breaksw case two: echo it is 2 breaksw default: echo it is $var breaksw endsw 37
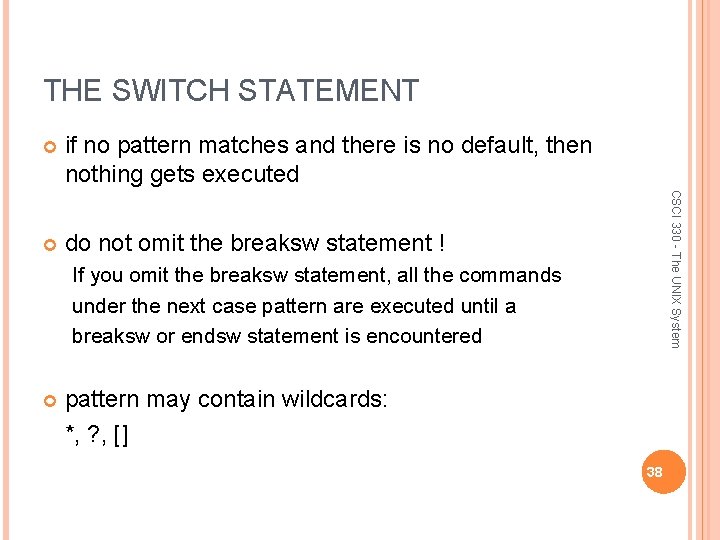
THE SWITCH STATEMENT if no pattern matches and there is no default, then nothing gets executed do not omit the breaksw statement ! CSCI 330 - The UNIX System If you omit the breaksw statement, all the commands under the next case pattern are executed until a breaksw or endsw statement is encountered pattern may contain wildcards: *, ? , [] 38
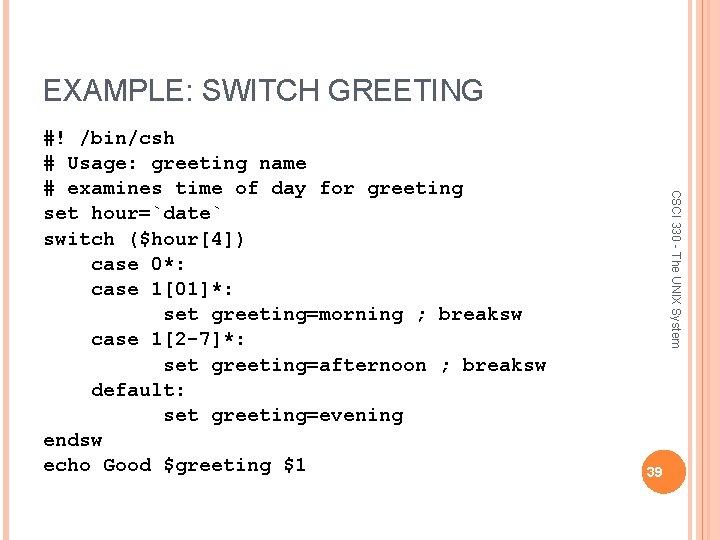
EXAMPLE: SWITCH GREETING CSCI 330 - The UNIX System #! /bin/csh # Usage: greeting name # examines time of day for greeting set hour=`date` switch ($hour[4]) case 0*: case 1[01]*: set greeting=morning ; breaksw case 1[2 -7]*: set greeting=afternoon ; breaksw default: set greeting=evening endsw echo Good $greeting $1 39
![EXAMPLE C SHELL PROGRAM CSCI 330 The UNIX System AVAILABLE OPTIONS 1 EXAMPLE C SHELL PROGRAM CSCI 330 - The UNIX System AVAILABLE OPTIONS ********** [1]](https://slidetodoc.com/presentation_image_h/d2c8f951bd1d559f75b5e67861683dda/image-40.jpg)
EXAMPLE C SHELL PROGRAM CSCI 330 - The UNIX System AVAILABLE OPTIONS ********** [1] Display today's date [2] How many people are logged on [3] How many user accounts exist [4] Exit Enter Your Choice [1 -4]: 40
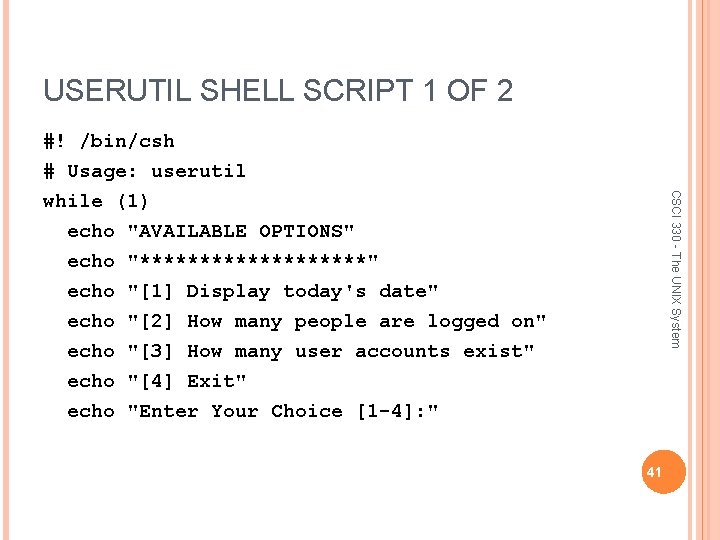
USERUTIL SHELL SCRIPT 1 OF 2 echo echo CSCI 330 - The UNIX System #! /bin/csh # Usage: userutil while (1) echo "AVAILABLE OPTIONS" "**********" "[1] Display today's date" "[2] How many people are logged on" "[3] How many user accounts exist" "[4] Exit" "Enter Your Choice [1 -4]: " 41
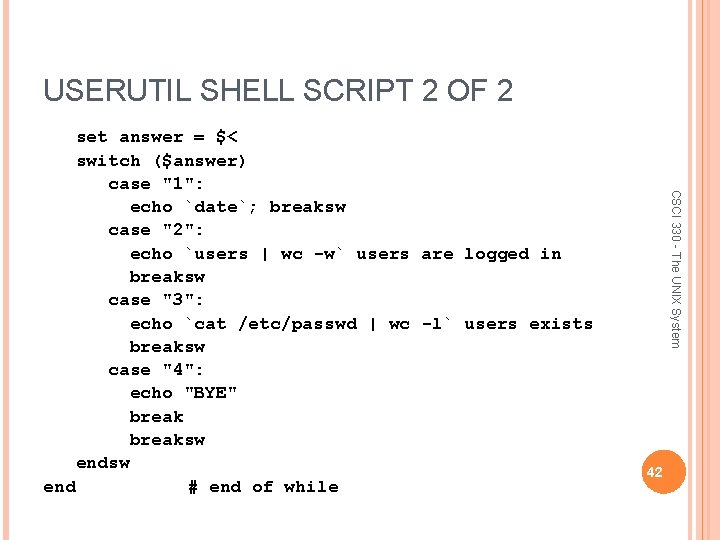
USERUTIL SHELL SCRIPT 2 OF 2 CSCI 330 - The UNIX System set answer = $< switch ($answer) case "1": echo `date`; breaksw case "2": echo `users | wc -w` users are logged in breaksw case "3": echo `cat /etc/passwd | wc -l` users exists breaksw case "4": echo "BYE" breaksw end # end of while 42
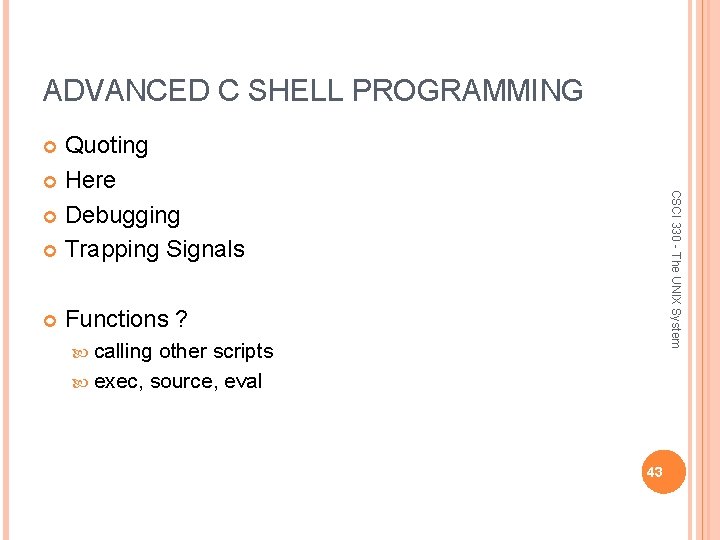
ADVANCED C SHELL PROGRAMMING Quoting Here Debugging Trapping Signals CSCI 330 - The UNIX System Functions ? calling other scripts exec, source, eval 43
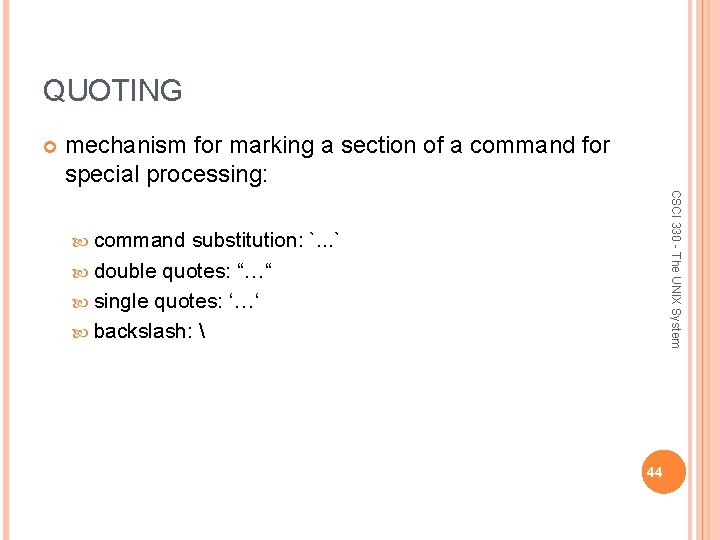
QUOTING mechanism for marking a section of a command for special processing: CSCI 330 - The UNIX System command substitution: `. . . ` double quotes: “…“ single quotes: ‘…‘ backslash: 44
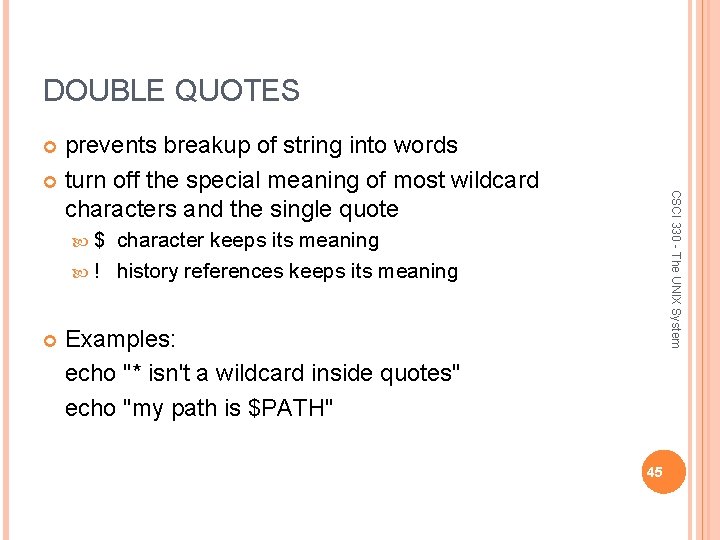
DOUBLE QUOTES prevents breakup of string into words turn off the special meaning of most wildcard characters and the single quote CSCI 330 - The UNIX System $ character keeps its meaning ! history references keeps its meaning Examples: echo "* isn't a wildcard inside quotes" echo "my path is $PATH" 45
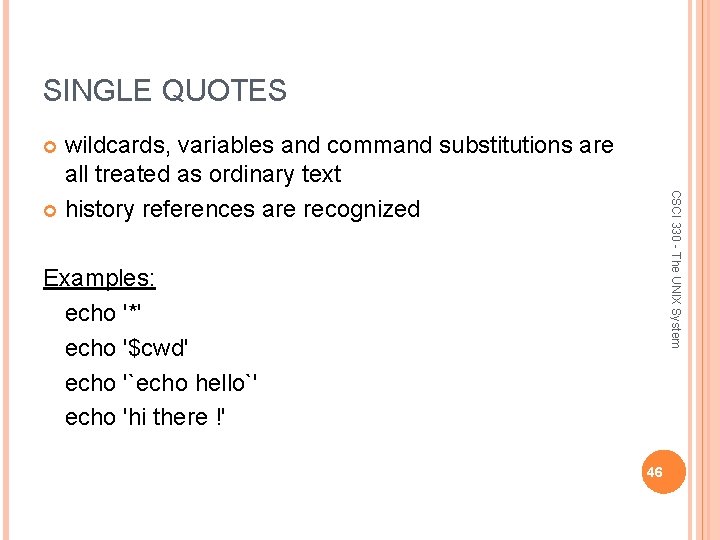
SINGLE QUOTES wildcards, variables and command substitutions are all treated as ordinary text history references are recognized CSCI 330 - The UNIX System Examples: echo '*' echo '$cwd' echo '`echo hello`' echo 'hi there !' 46
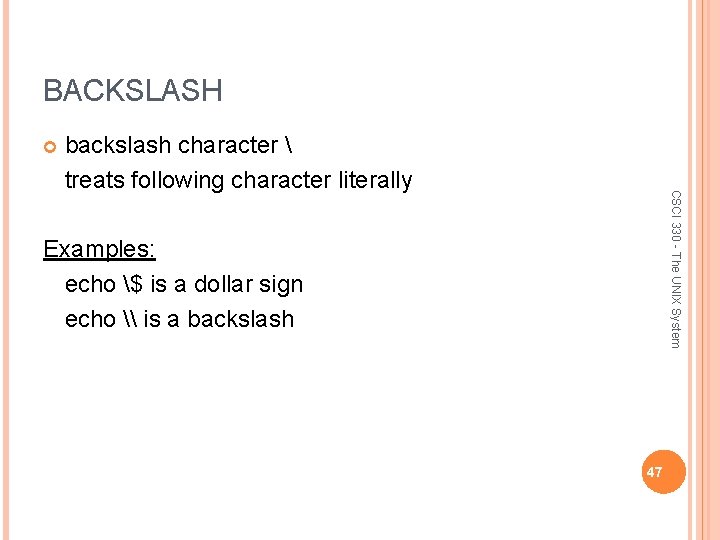
BACKSLASH CSCI 330 - The UNIX System backslash character treats following character literally Examples: echo $ is a dollar sign echo \ is a backslash 47
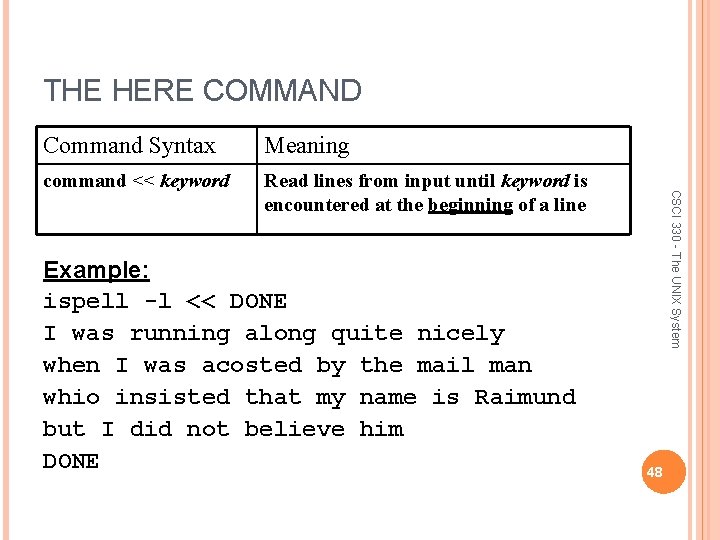
THE HERE COMMAND Meaning command << keyword Read lines from input until keyword is encountered at the beginning of a line Example: ispell -l << DONE I was running along quite nicely when I was acosted by the mail man whio insisted that my name is Raimund but I did not believe him DONE CSCI 330 - The UNIX System Command Syntax 48
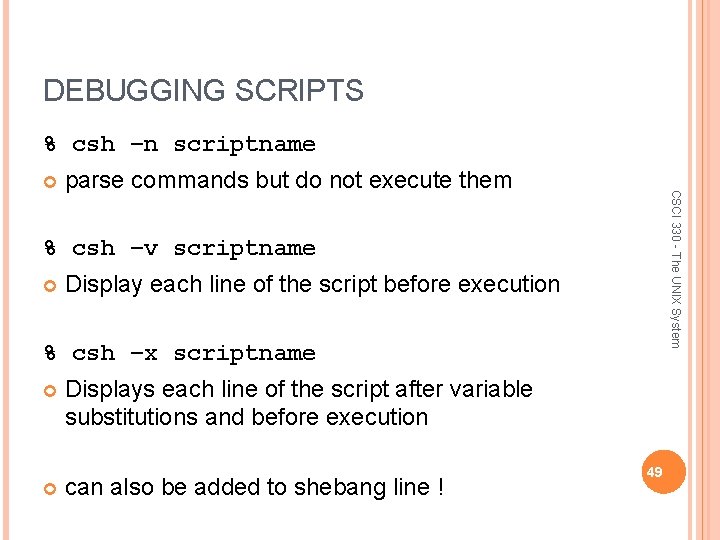
DEBUGGING SCRIPTS % csh –n scriptname parse commands but do not execute them CSCI 330 - The UNIX System % csh –v scriptname Display each line of the script before execution % csh –x scriptname Displays each line of the script after variable substitutions and before execution can also be added to shebang line ! 49
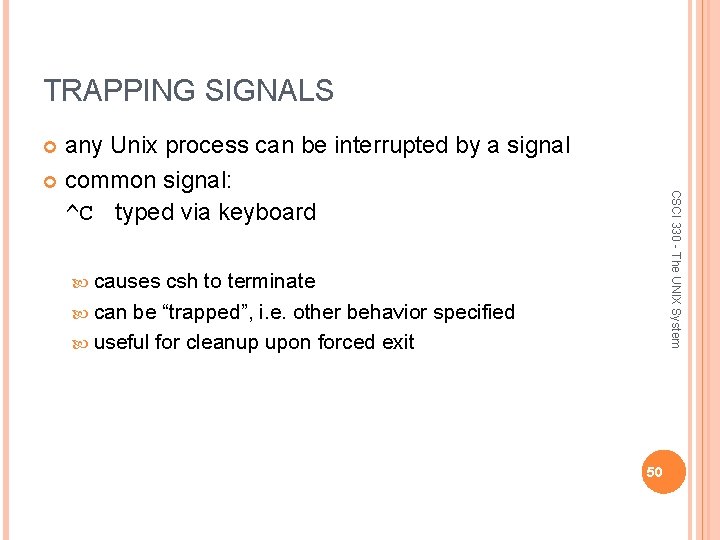
TRAPPING SIGNALS any Unix process can be interrupted by a signal common signal: ^C typed via keyboard CSCI 330 - The UNIX System causes csh to terminate can be “trapped”, i. e. other behavior specified useful for cleanup upon forced exit 50
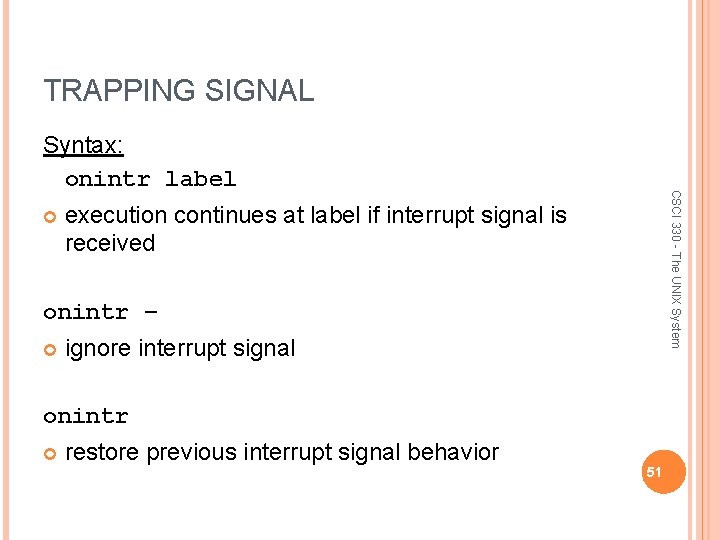
TRAPPING SIGNAL CSCI 330 - The UNIX System Syntax: onintr label execution continues at label if interrupt signal is received onintr – ignore interrupt signal onintr restore previous interrupt signal behavior 51
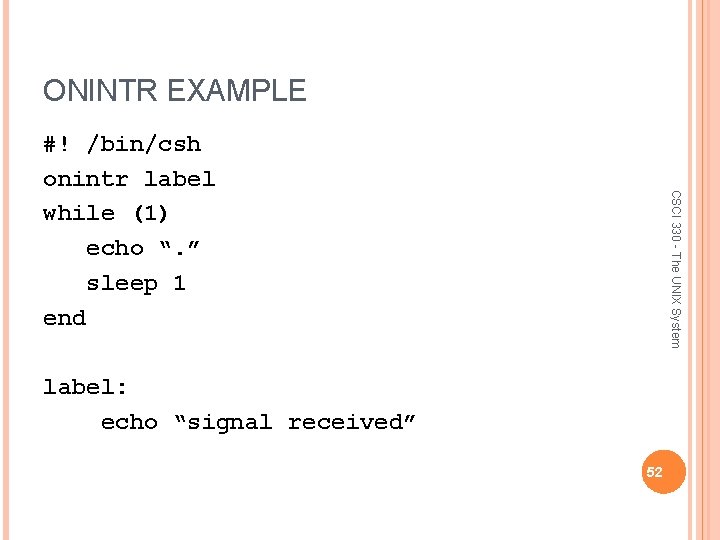
ONINTR EXAMPLE CSCI 330 - The UNIX System #! /bin/csh onintr label while (1) echo “. ” sleep 1 end label: echo “signal received” 52
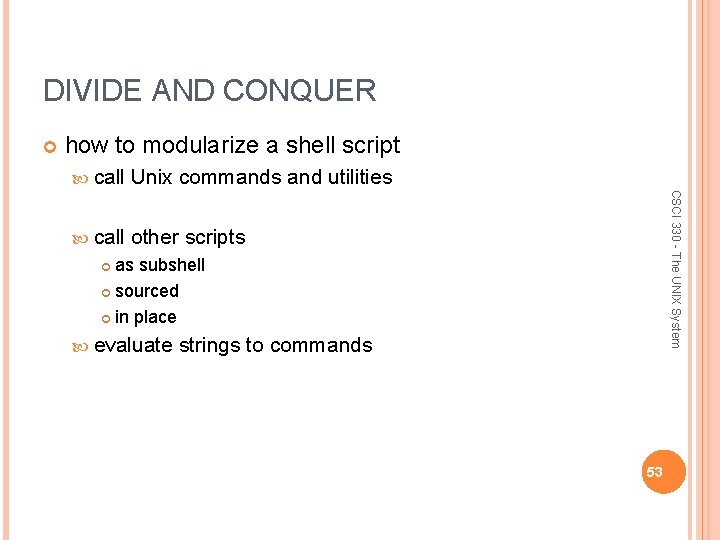
DIVIDE AND CONQUER how to modularize a shell script Unix commands and utilities call other scripts CSCI 330 - The UNIX System call as subshell sourced in place evaluate strings to commands 53
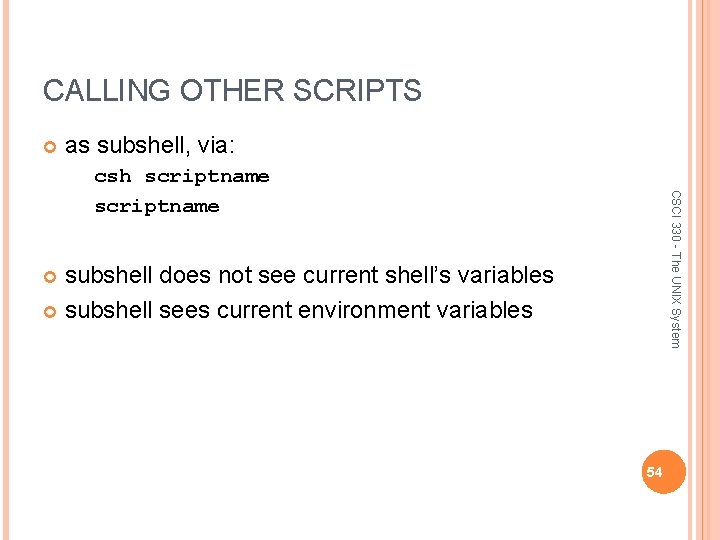
CALLING OTHER SCRIPTS as subshell, via: CSCI 330 - The UNIX System csh scriptname subshell does not see current shell’s variables subshell sees current environment variables 54
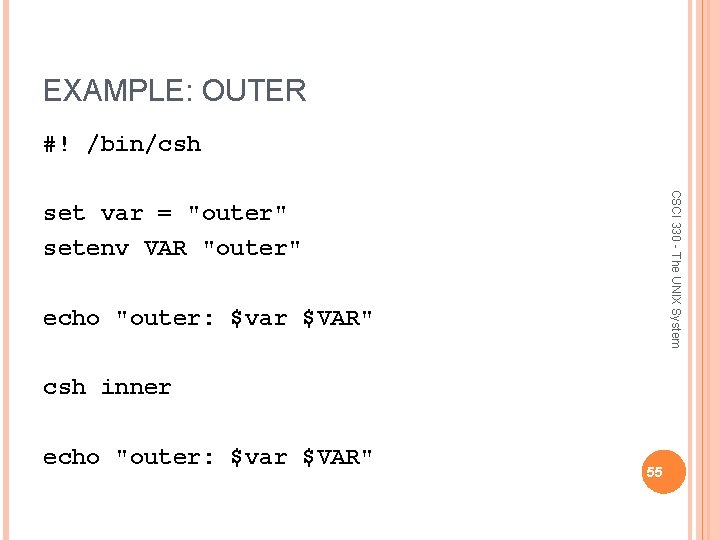
EXAMPLE: OUTER #! /bin/csh CSCI 330 - The UNIX System set var = "outer" setenv VAR "outer" echo "outer: $var $VAR" csh inner echo "outer: $var $VAR" 55
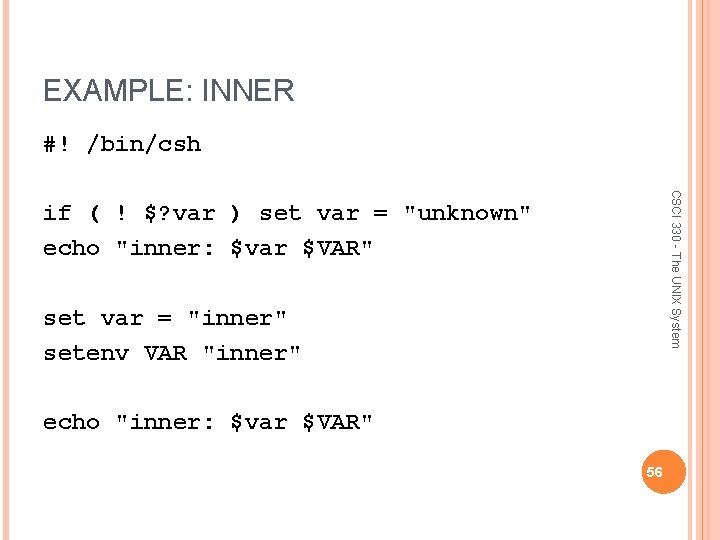
EXAMPLE: INNER #! /bin/csh CSCI 330 - The UNIX System if ( ! $? var ) set var = "unknown" echo "inner: $var $VAR" set var = "inner" setenv VAR "inner" echo "inner: $var $VAR" 56
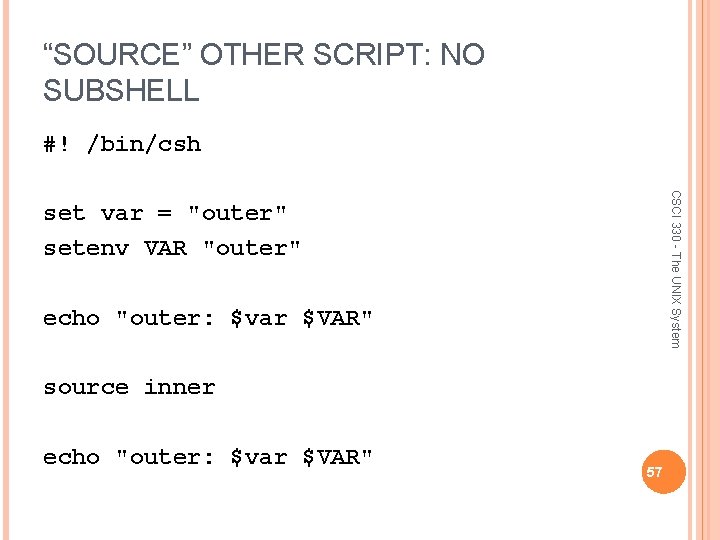
“SOURCE” OTHER SCRIPT: NO SUBSHELL #! /bin/csh CSCI 330 - The UNIX System set var = "outer" setenv VAR "outer" echo "outer: $var $VAR" source inner echo "outer: $var $VAR" 57
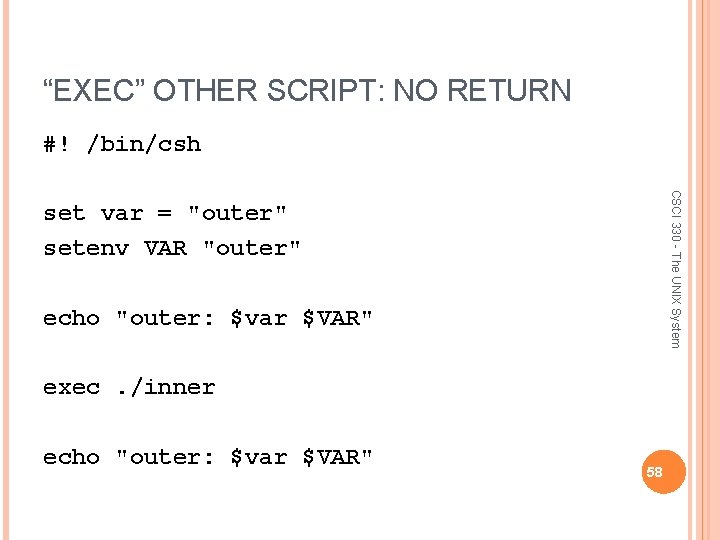
“EXEC” OTHER SCRIPT: NO RETURN #! /bin/csh CSCI 330 - The UNIX System set var = "outer" setenv VAR "outer" echo "outer: $var $VAR" exec. /inner echo "outer: $var $VAR" 58
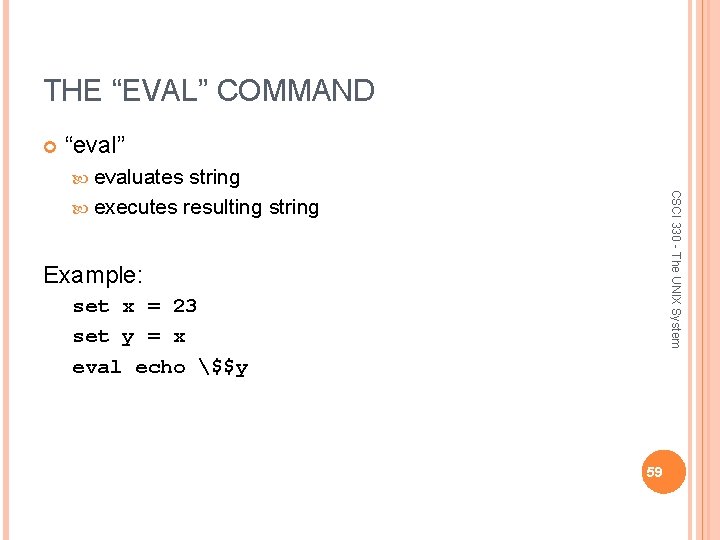
THE “EVAL” COMMAND “eval” evaluates CSCI 330 - The UNIX System string executes resulting string Example: set x = 23 set y = x eval echo $$y 59
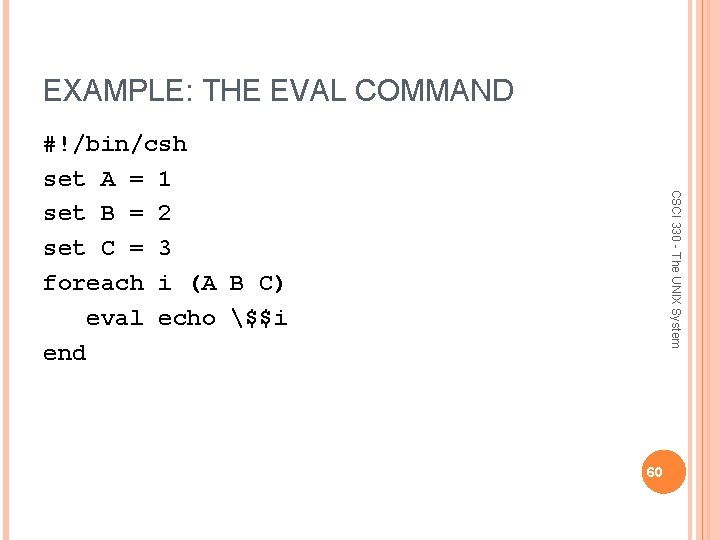
EXAMPLE: THE EVAL COMMAND CSCI 330 - The UNIX System #!/bin/csh set A = 1 set B = 2 set C = 3 foreach i (A B C) eval echo $$i end 60