AVL Trees ITCS 6114 Algorithms and Data Structures
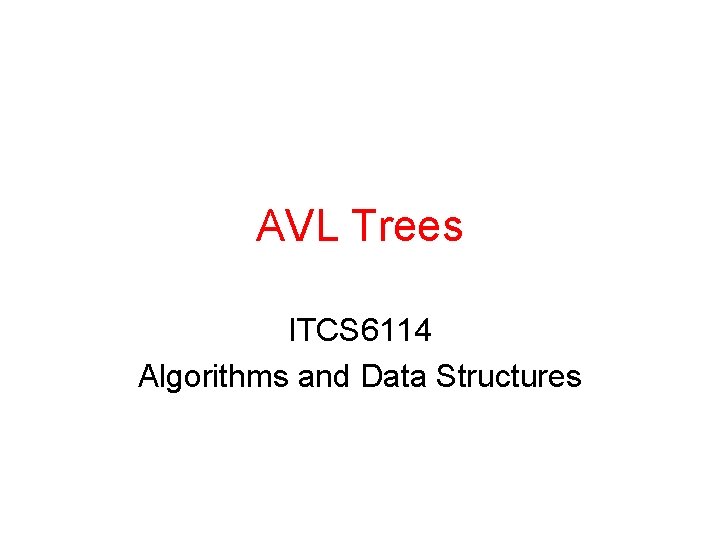
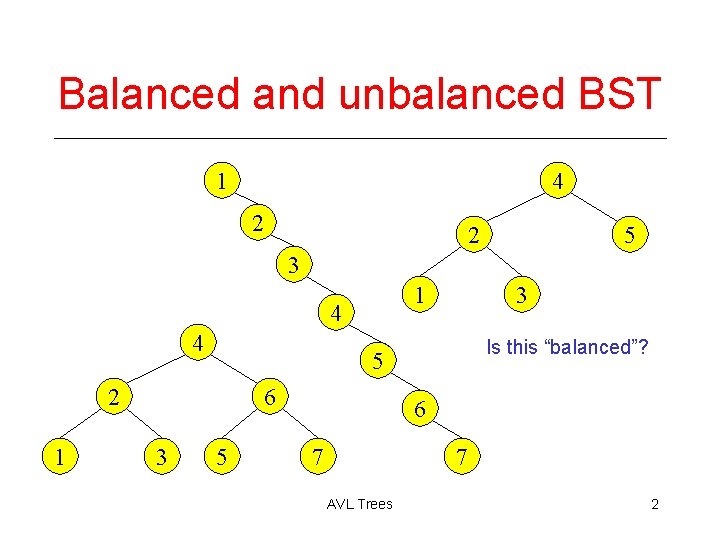
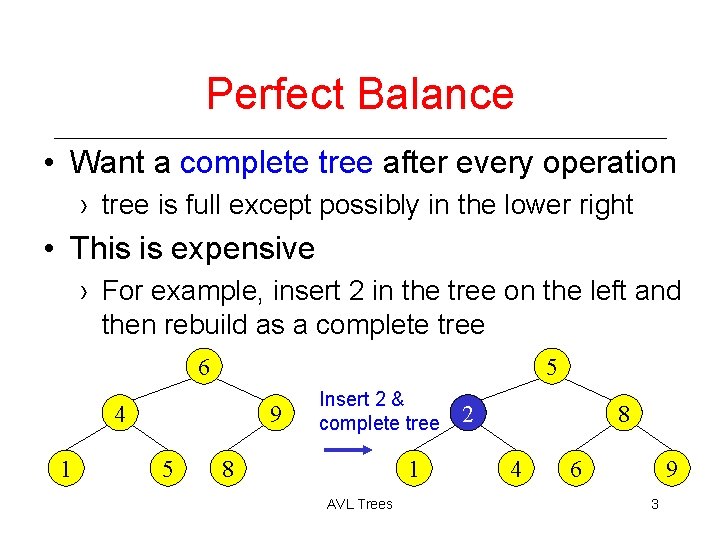
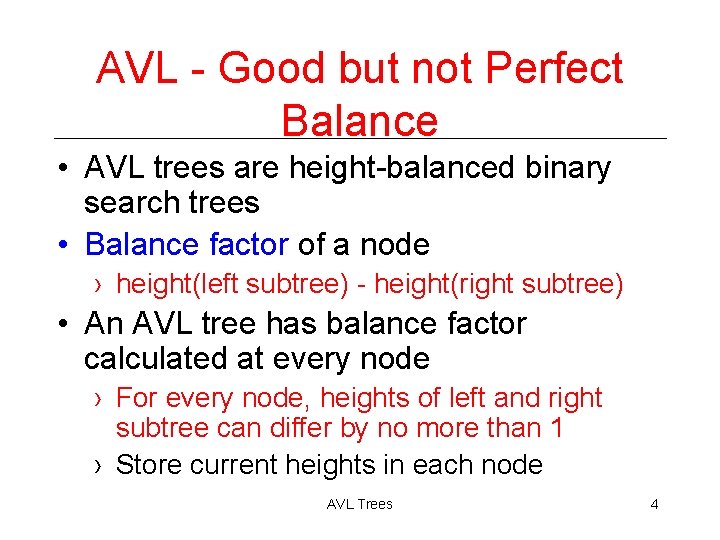
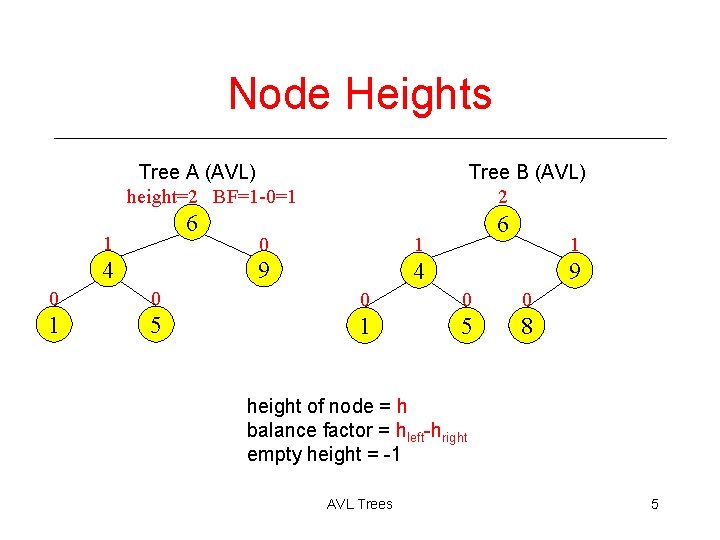
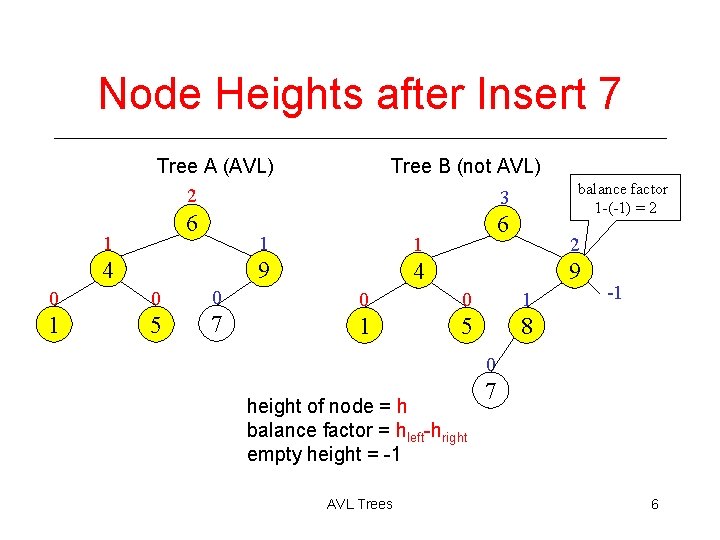
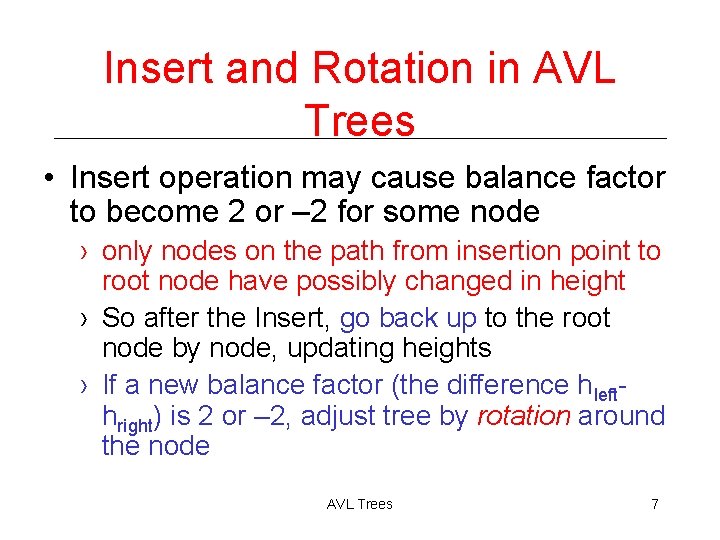
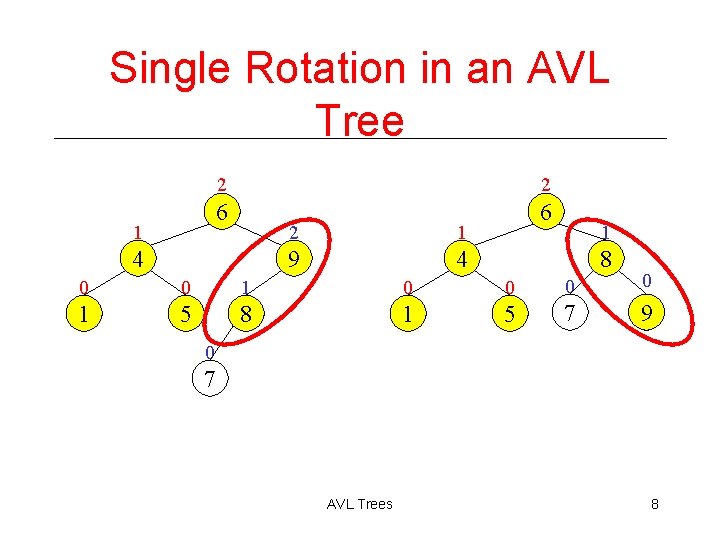
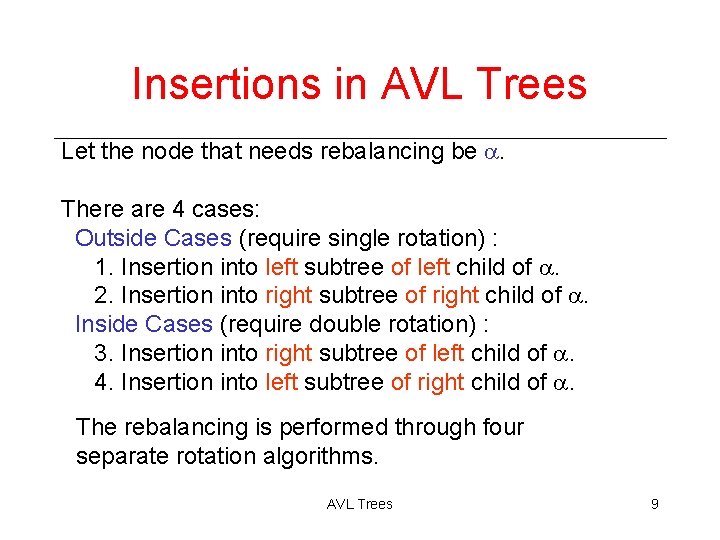
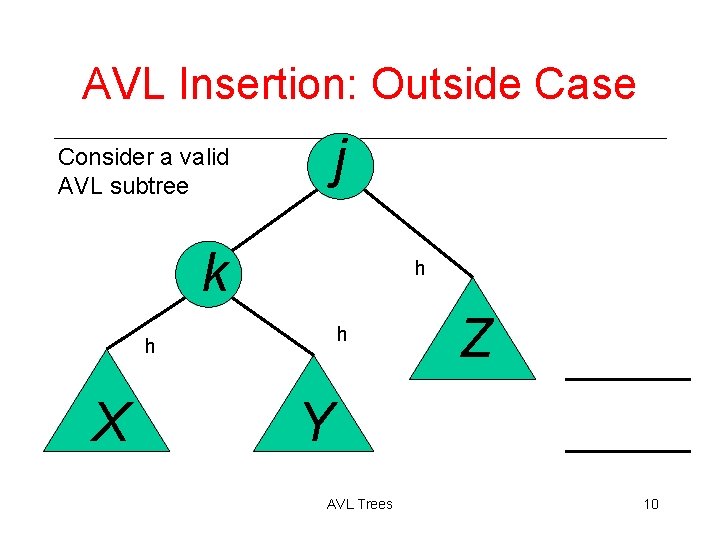
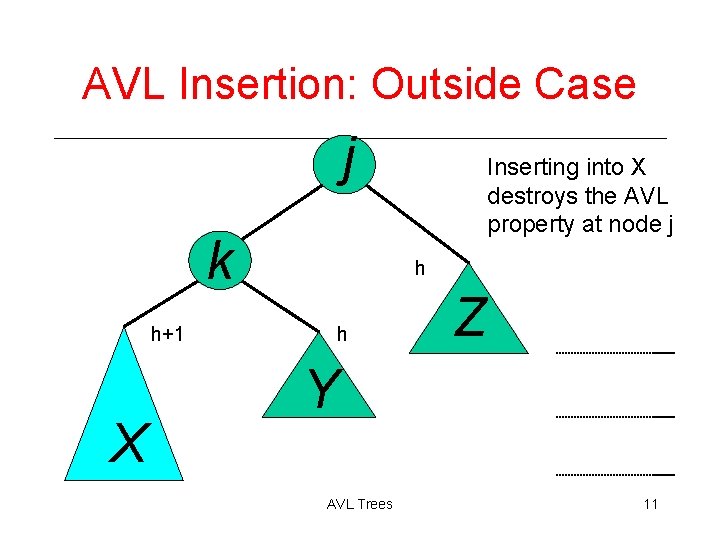
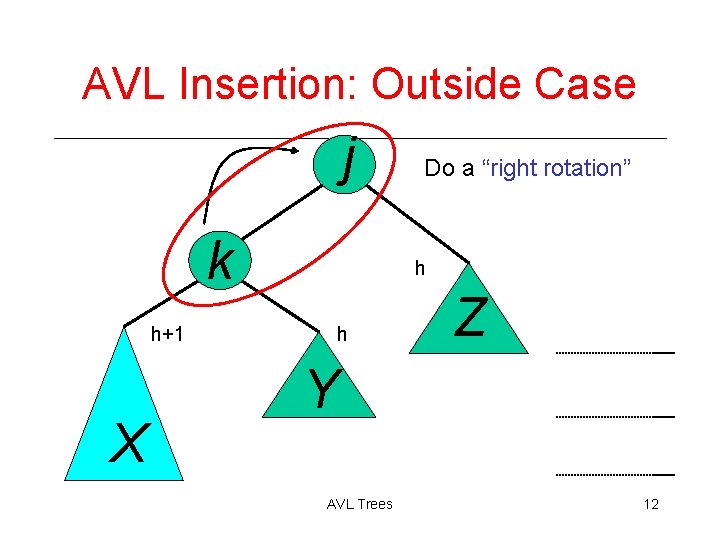
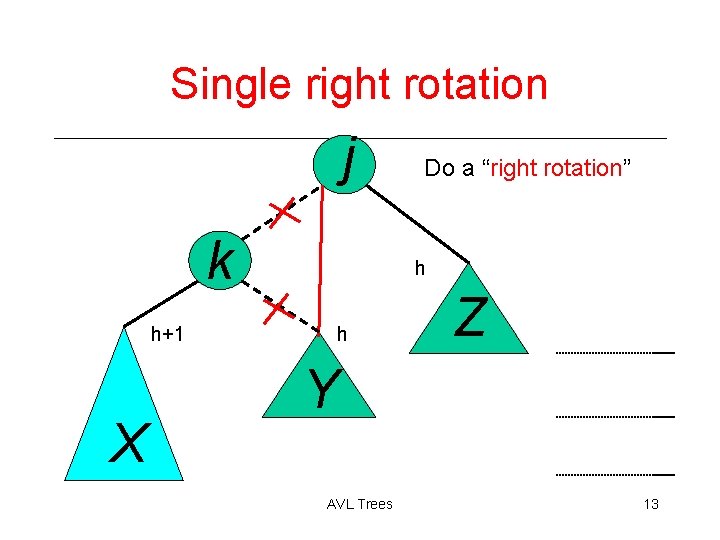
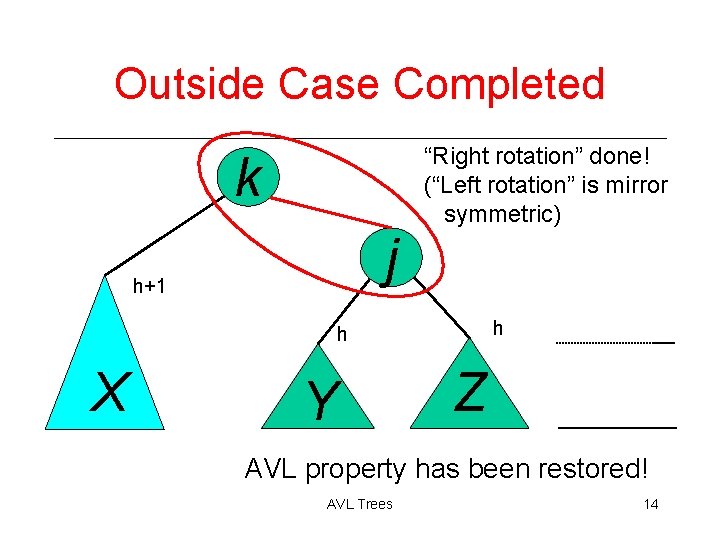
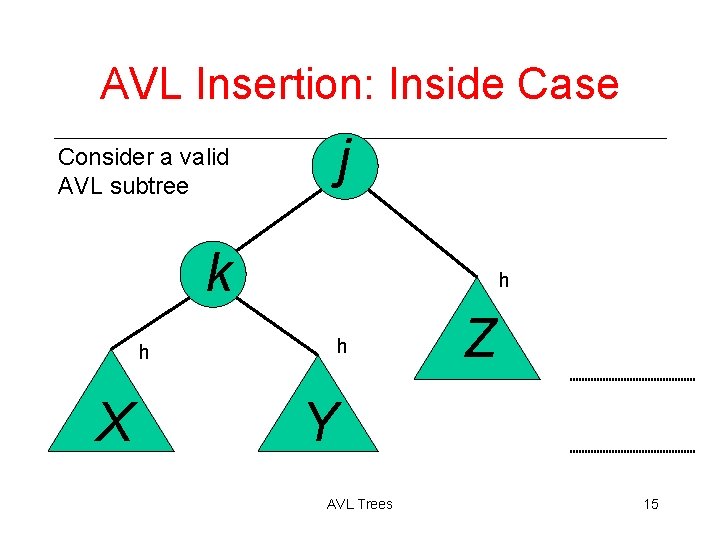
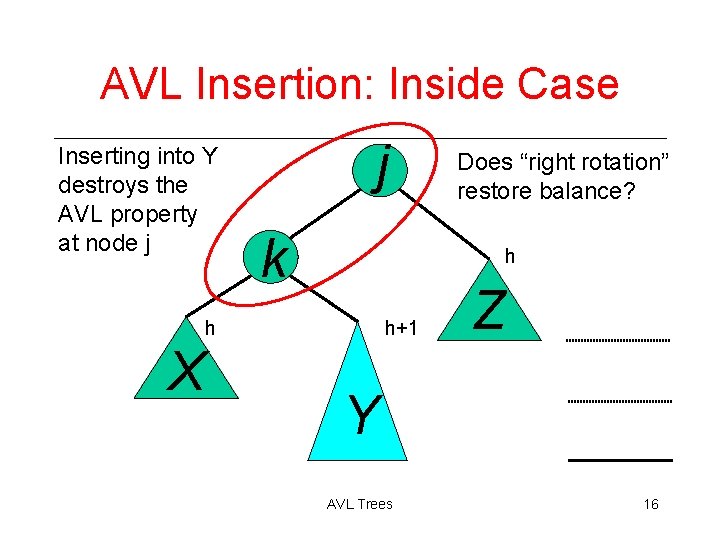
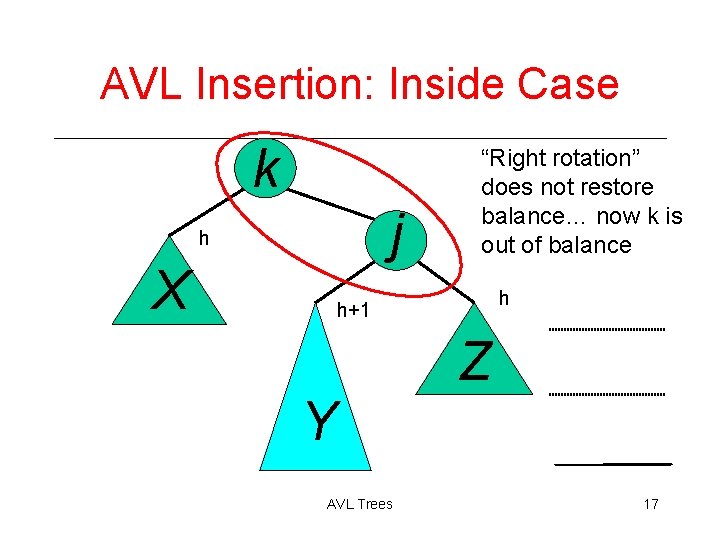
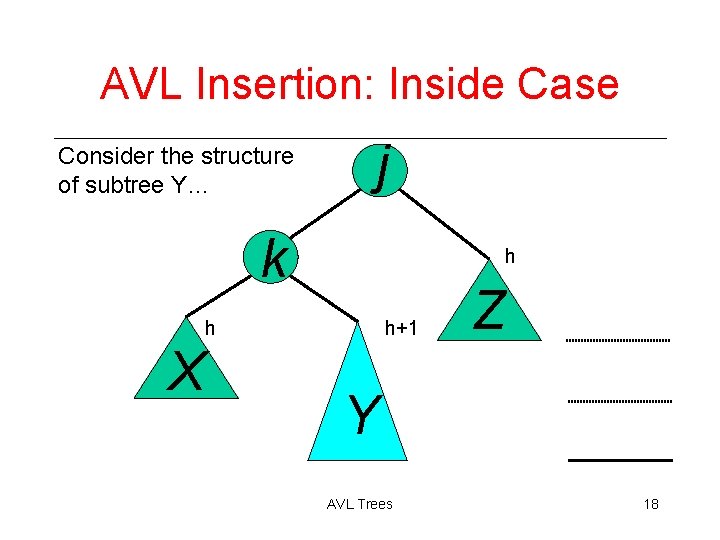
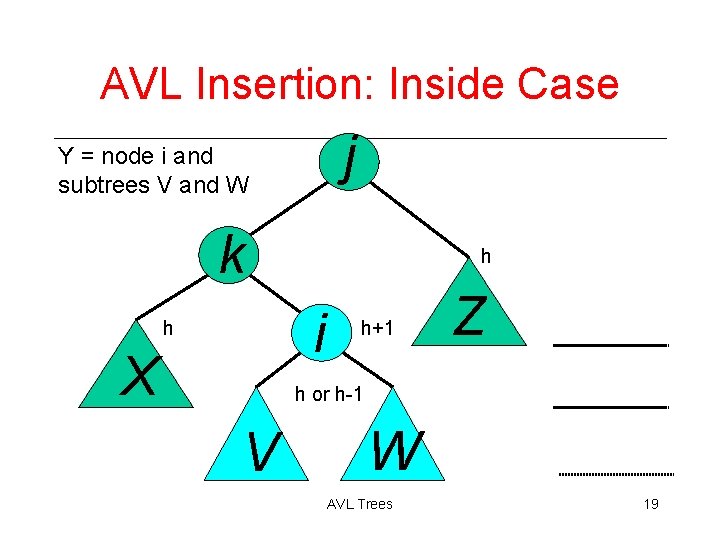
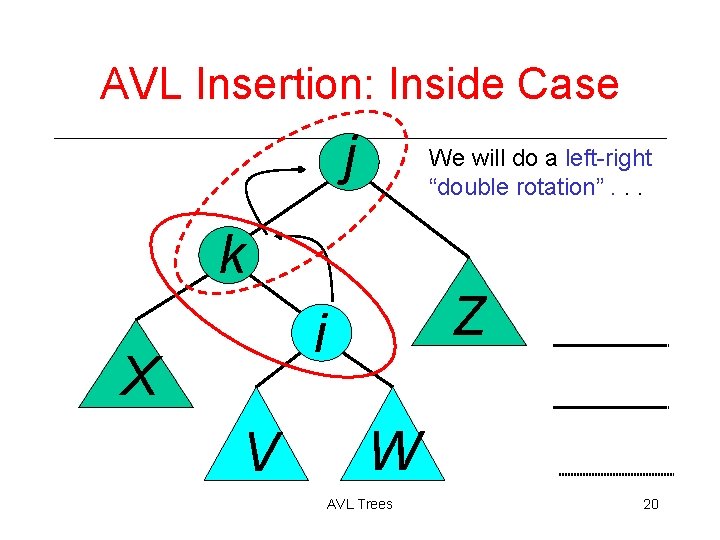
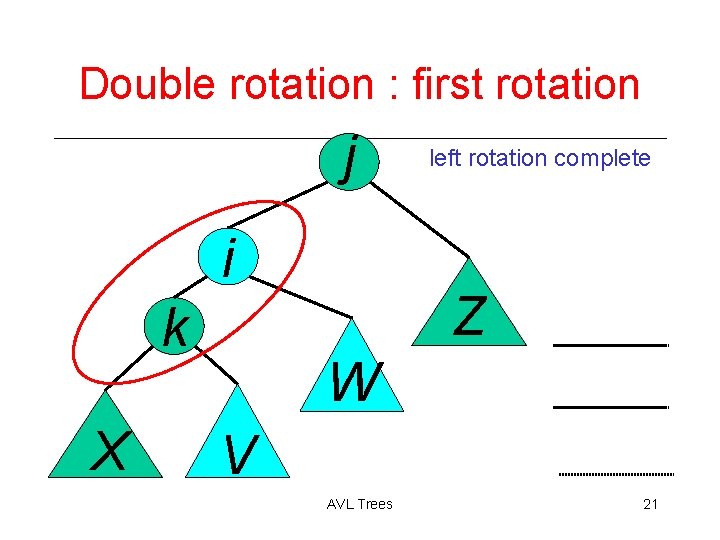
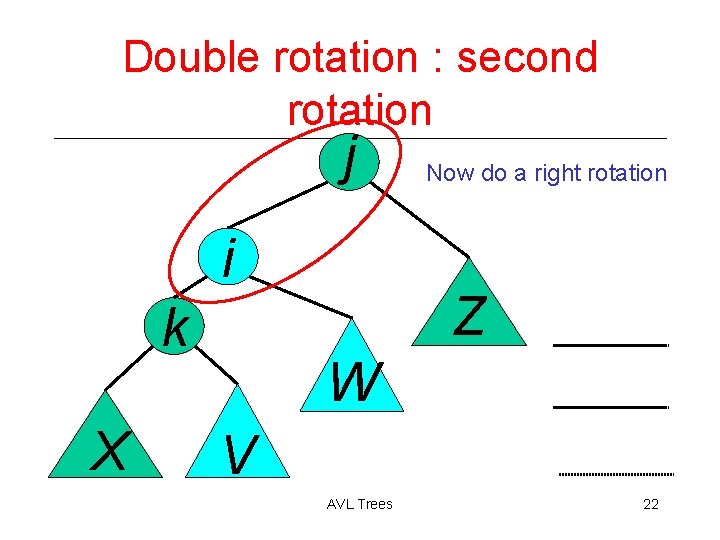
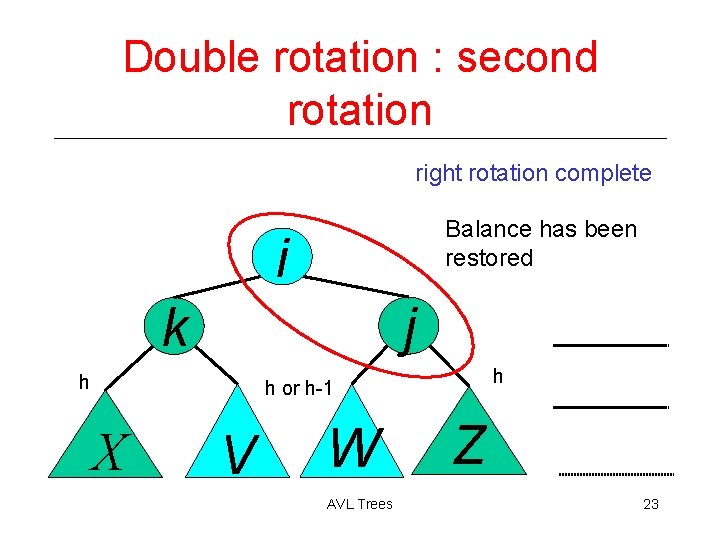
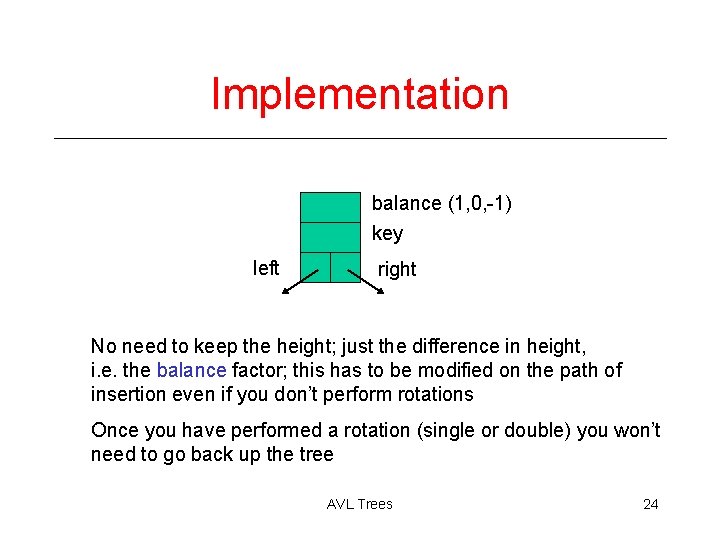
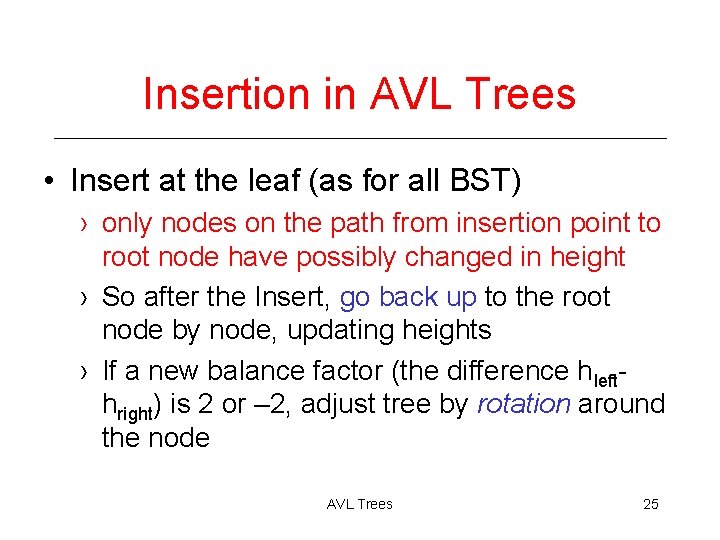
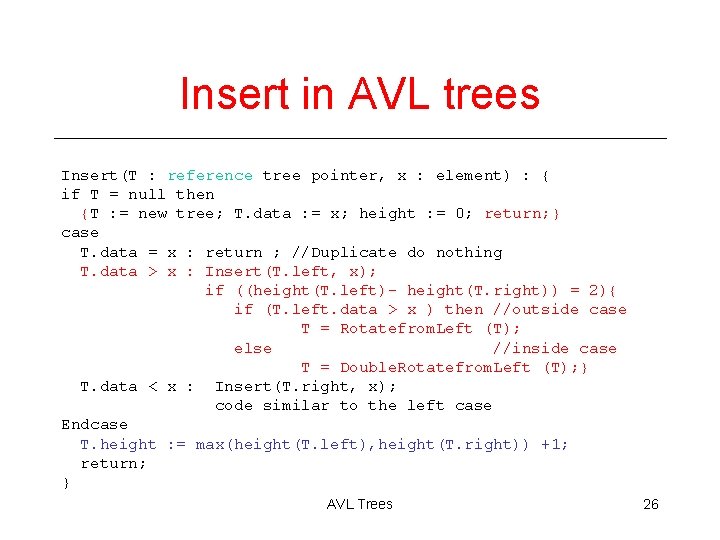
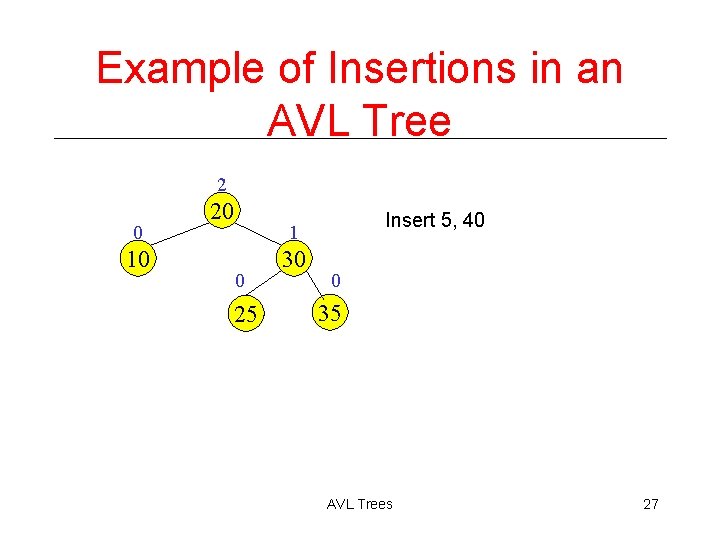
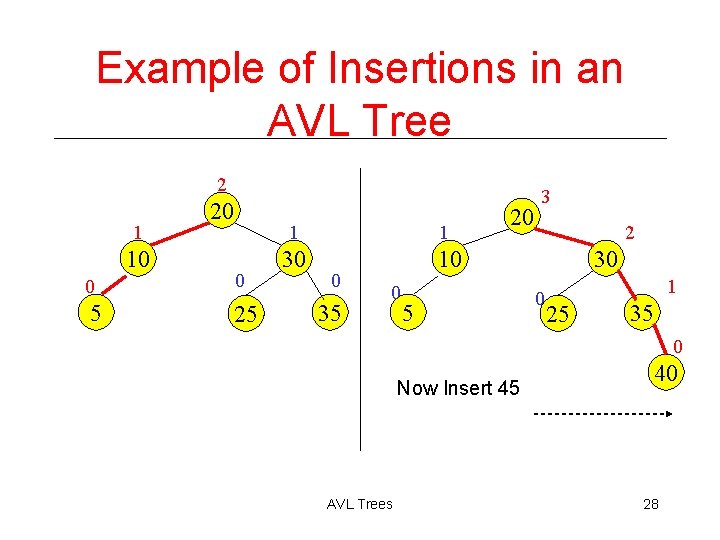
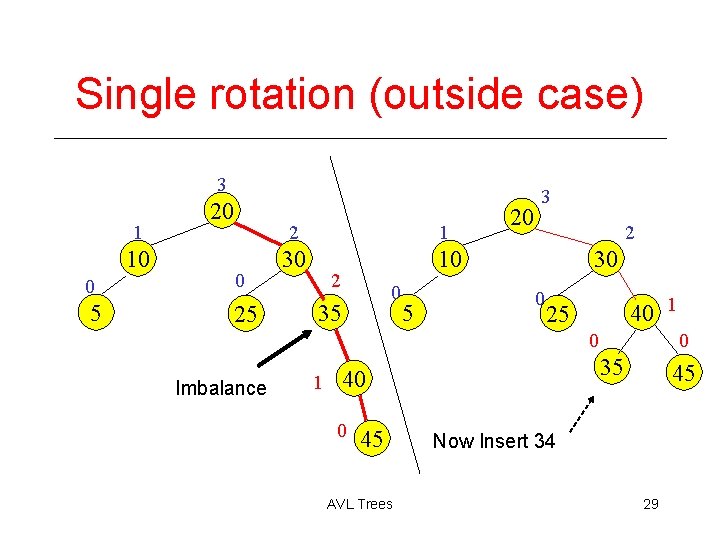
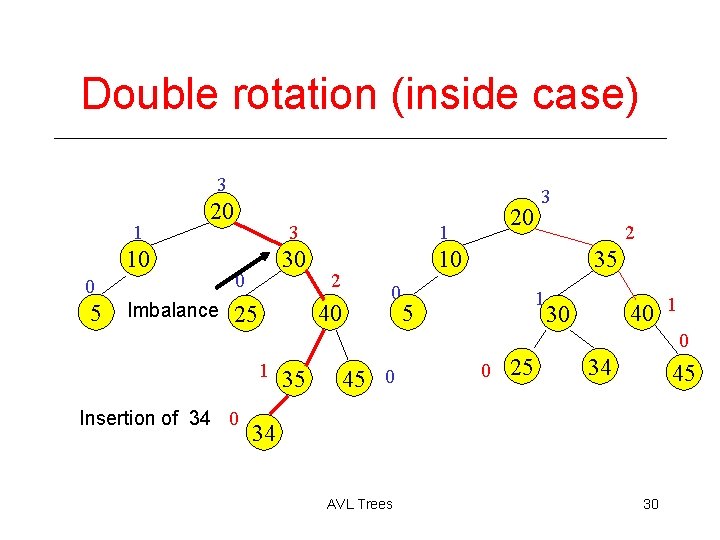
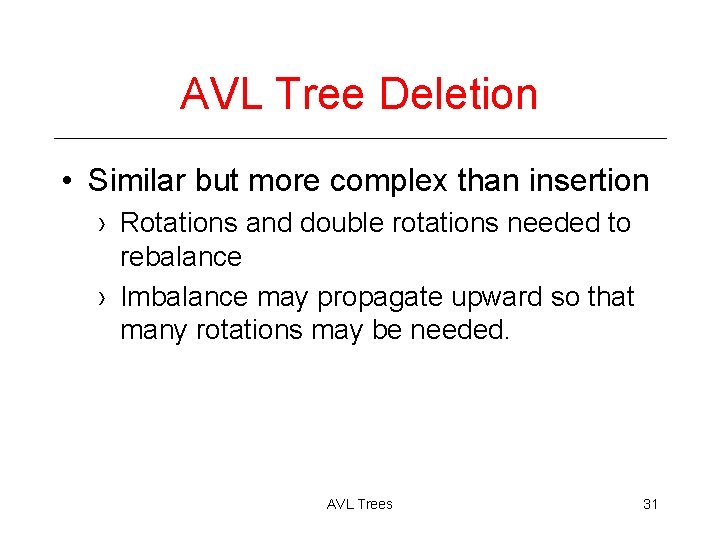
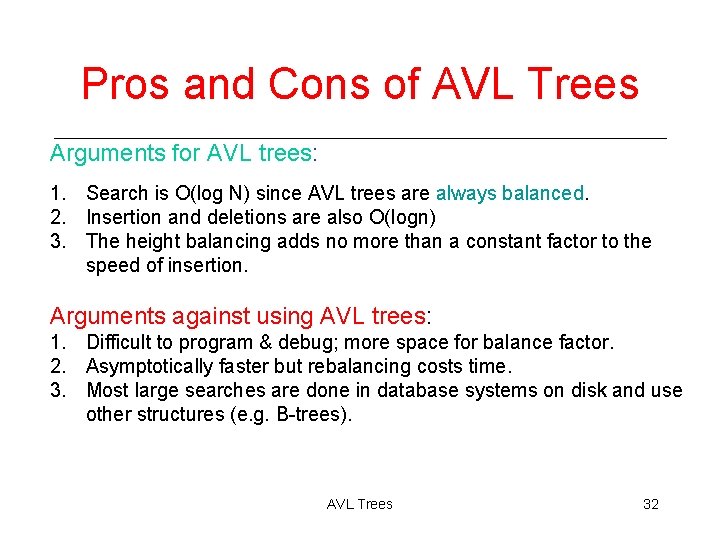
- Slides: 32
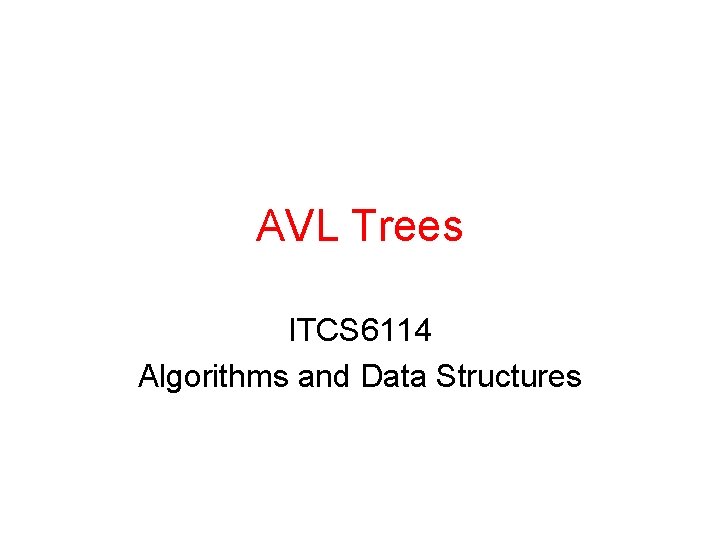
AVL Trees ITCS 6114 Algorithms and Data Structures
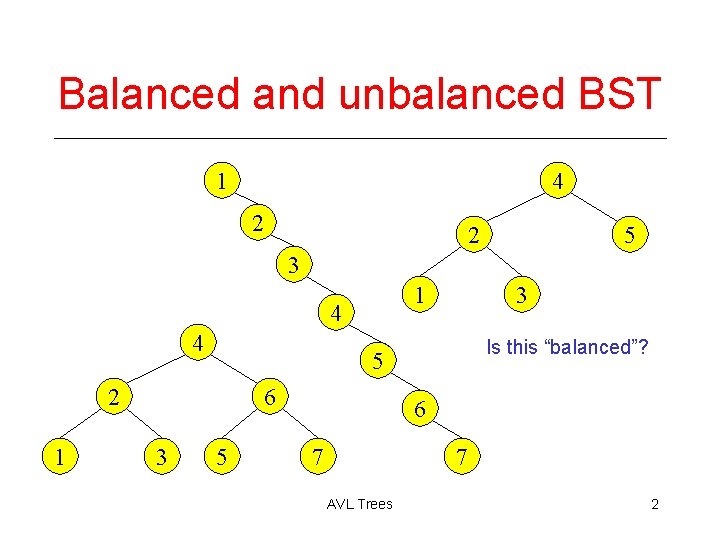
Balanced and unbalanced BST 1 4 2 2 5 3 1 4 4 6 3 Is this “balanced”? 5 2 1 3 5 6 7 7 AVL Trees 2
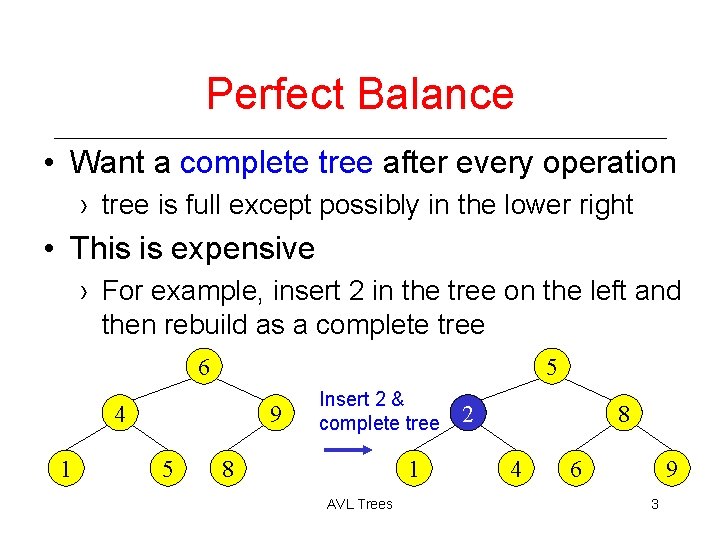
Perfect Balance • Want a complete tree after every operation › tree is full except possibly in the lower right • This is expensive › For example, insert 2 in the tree on the left and then rebuild as a complete tree 6 5 4 1 9 5 Insert 2 & complete tree 8 1 AVL Trees 2 8 4 6 9 3
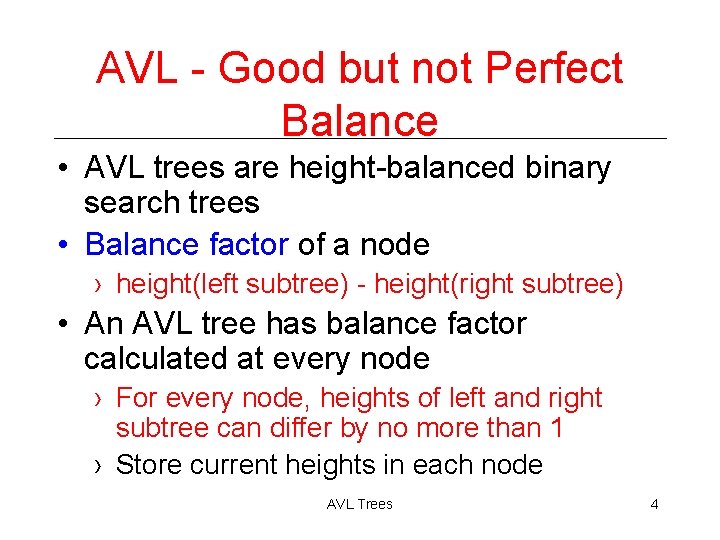
AVL - Good but not Perfect Balance • AVL trees are height-balanced binary search trees • Balance factor of a node › height(left subtree) - height(right subtree) • An AVL tree has balance factor calculated at every node › For every node, heights of left and right subtree can differ by no more than 1 › Store current heights in each node AVL Trees 4
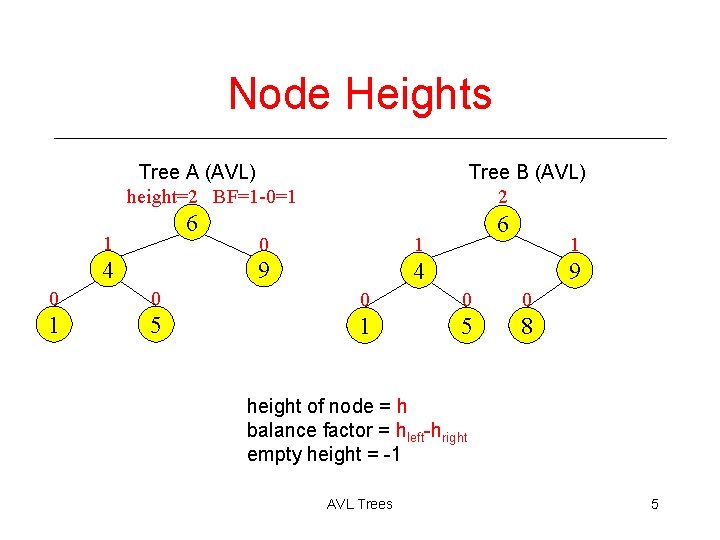
Node Heights Tree A (AVL) height=2 BF=1 -0=1 6 1 4 Tree B (AVL) 2 0 1 9 4 6 1 9 0 0 0 1 5 8 height of node = h balance factor = hleft-hright empty height = -1 AVL Trees 5
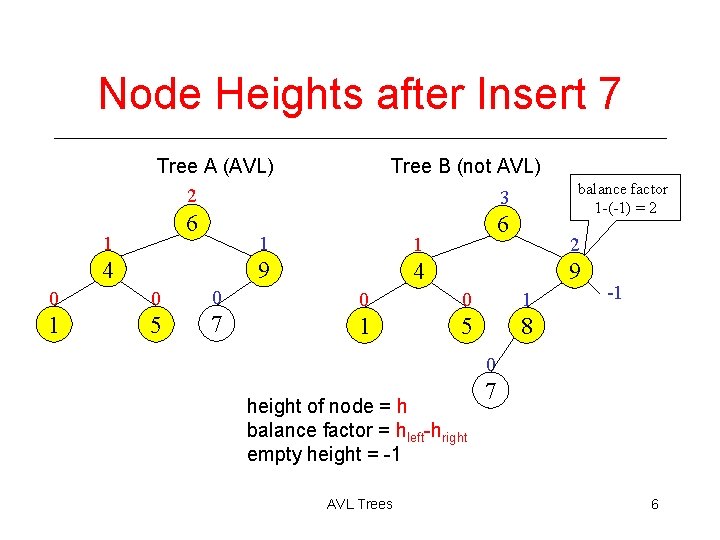
Node Heights after Insert 7 Tree A (AVL) 2 6 1 4 Tree B (not AVL) balance factor 1 -(-1) = 2 3 1 1 9 4 6 2 9 0 0 0 1 1 5 7 1 5 8 -1 0 height of node = h balance factor = hleft-hright empty height = -1 AVL Trees 7 6
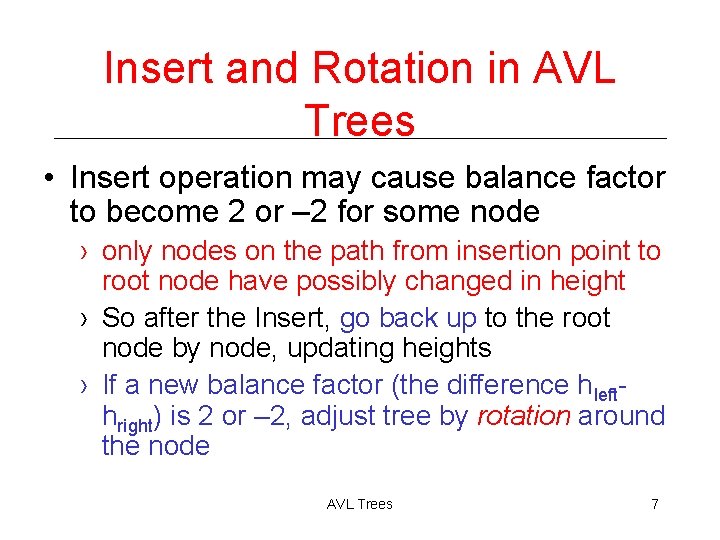
Insert and Rotation in AVL Trees • Insert operation may cause balance factor to become 2 or – 2 for some node › only nodes on the path from insertion point to root node have possibly changed in height › So after the Insert, go back up to the root node by node, updating heights › If a new balance factor (the difference hlefthright) is 2 or – 2, adjust tree by rotation around the node AVL Trees 7
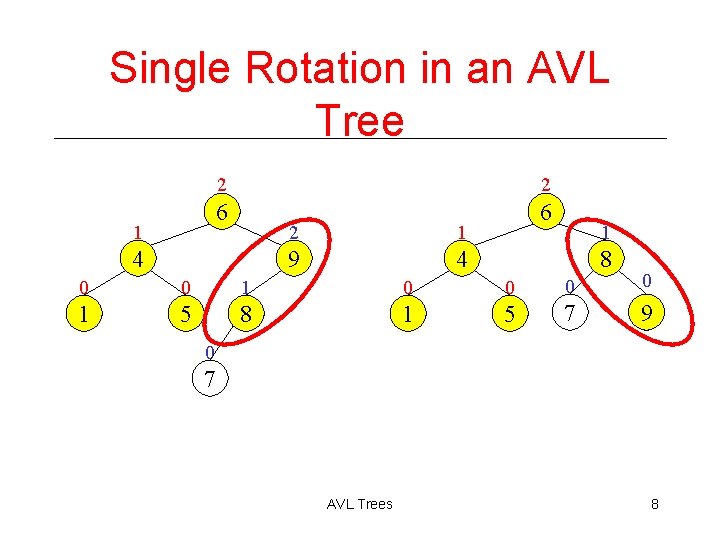
Single Rotation in an AVL Tree 2 6 6 1 2 4 2 1 9 4 1 8 0 0 1 5 8 1 5 7 9 0 7 AVL Trees 8
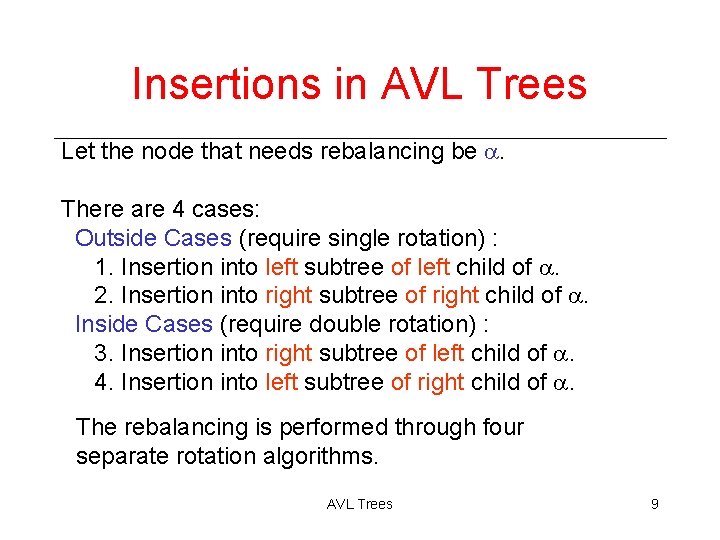
Insertions in AVL Trees Let the node that needs rebalancing be . There are 4 cases: Outside Cases (require single rotation) : 1. Insertion into left subtree of left child of . 2. Insertion into right subtree of right child of . Inside Cases (require double rotation) : 3. Insertion into right subtree of left child of . 4. Insertion into left subtree of right child of . The rebalancing is performed through four separate rotation algorithms. AVL Trees 9
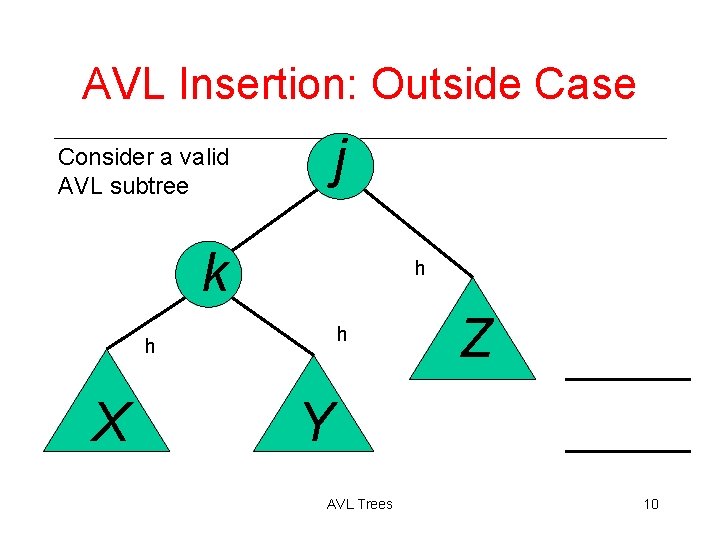
AVL Insertion: Outside Case j Consider a valid AVL subtree k h h h X Z Y AVL Trees 10
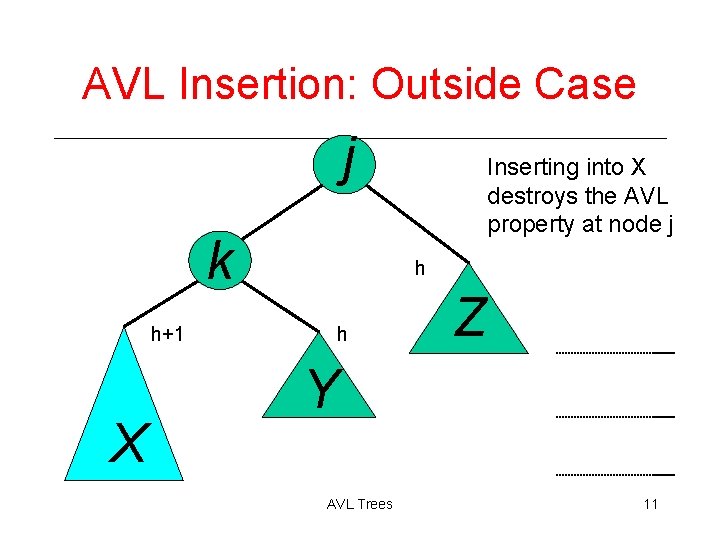
AVL Insertion: Outside Case j k h+1 X Inserting into X destroys the AVL property at node j h h Z Y AVL Trees 11
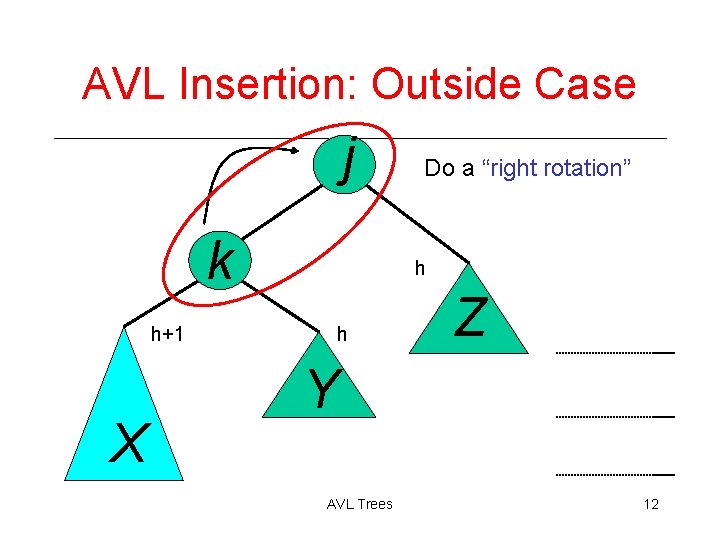
AVL Insertion: Outside Case j k h+1 X Do a “right rotation” h h Z Y AVL Trees 12
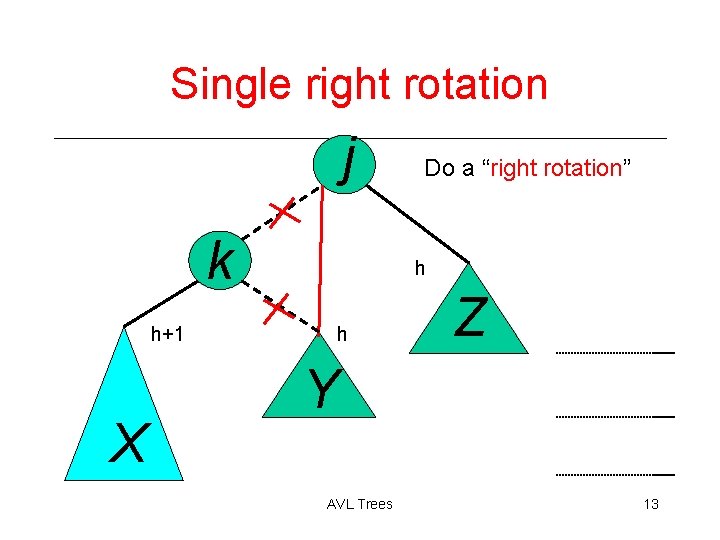
Single right rotation j k h+1 X Do a “right rotation” h h Z Y AVL Trees 13
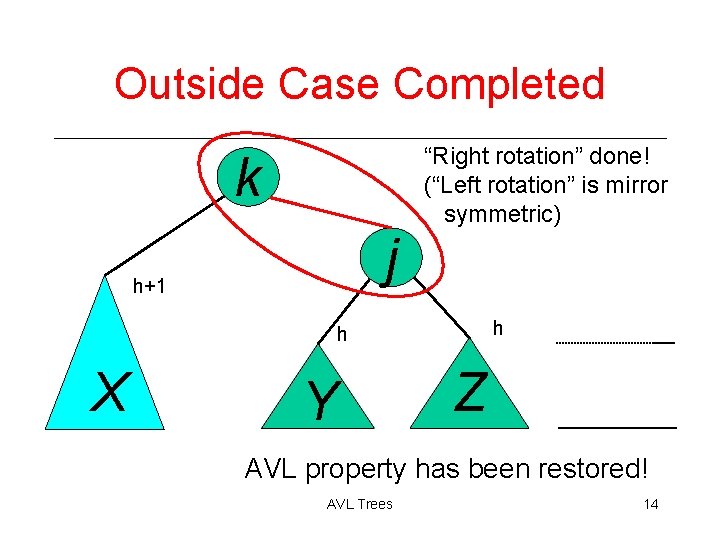
Outside Case Completed “Right rotation” done! (“Left rotation” is mirror symmetric) k j h+1 h h X Y Z AVL property has been restored! AVL Trees 14
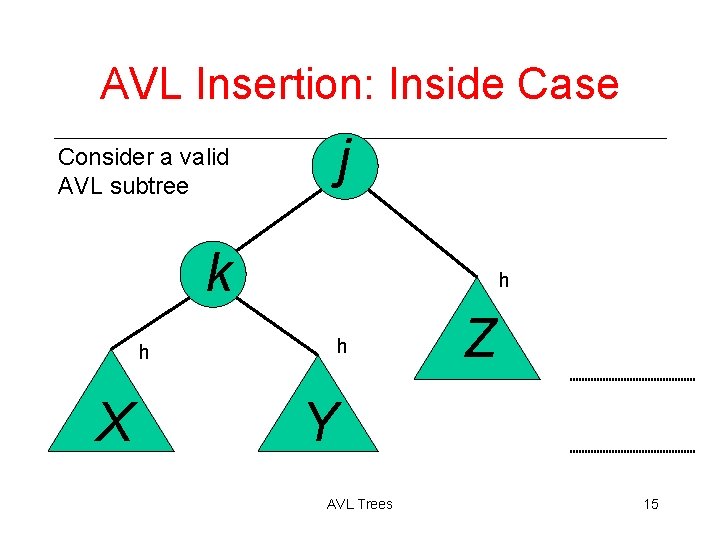
AVL Insertion: Inside Case j Consider a valid AVL subtree k h h h X Z Y AVL Trees 15
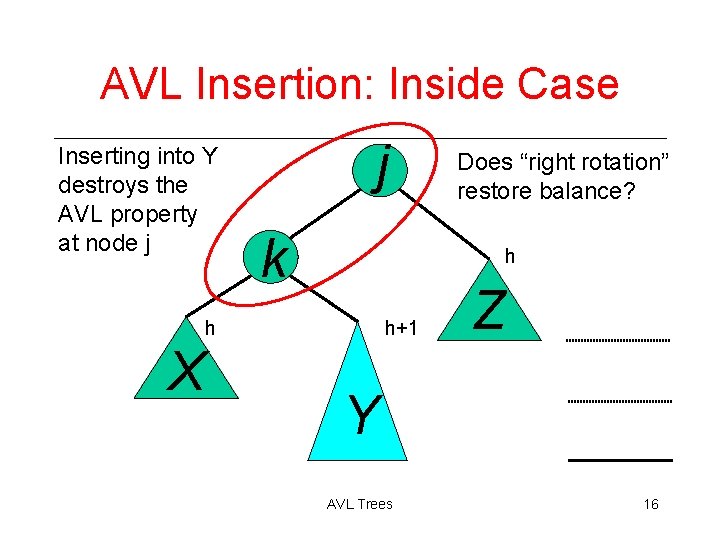
AVL Insertion: Inside Case Inserting into Y destroys the AVL property at node j j k h h X Does “right rotation” restore balance? h+1 Z Y AVL Trees 16
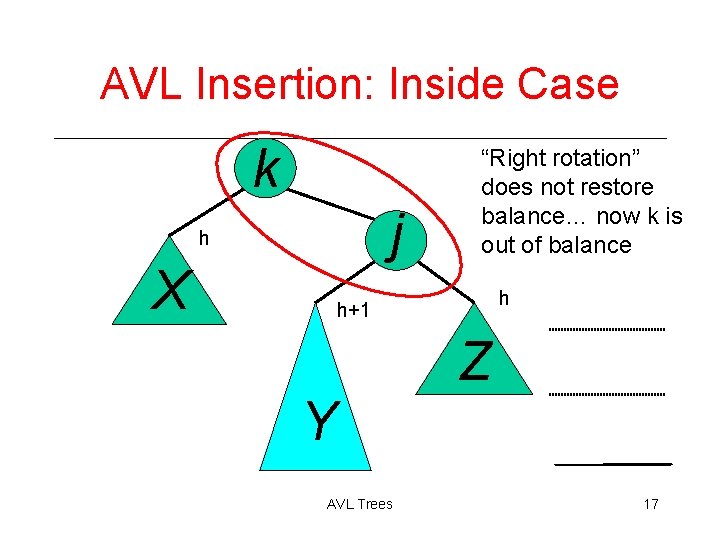
AVL Insertion: Inside Case k j h X “Right rotation” does not restore balance… now k is out of balance h h+1 Y AVL Trees Z 17
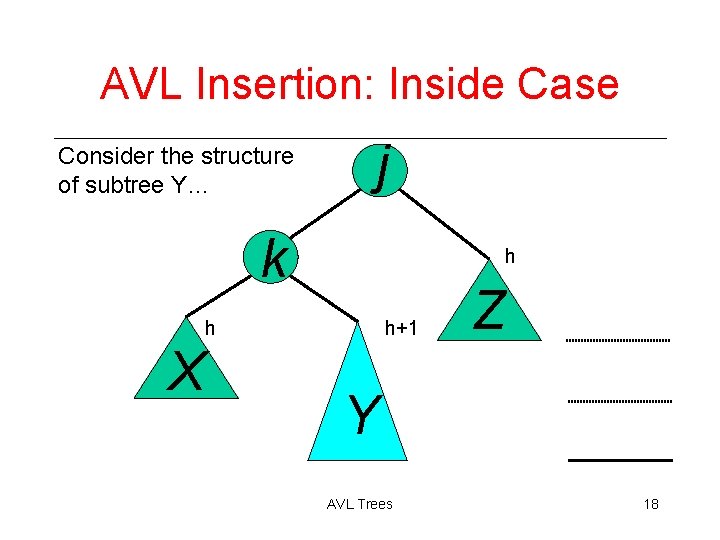
AVL Insertion: Inside Case Consider the structure of subtree Y… j k h h X h+1 Z Y AVL Trees 18
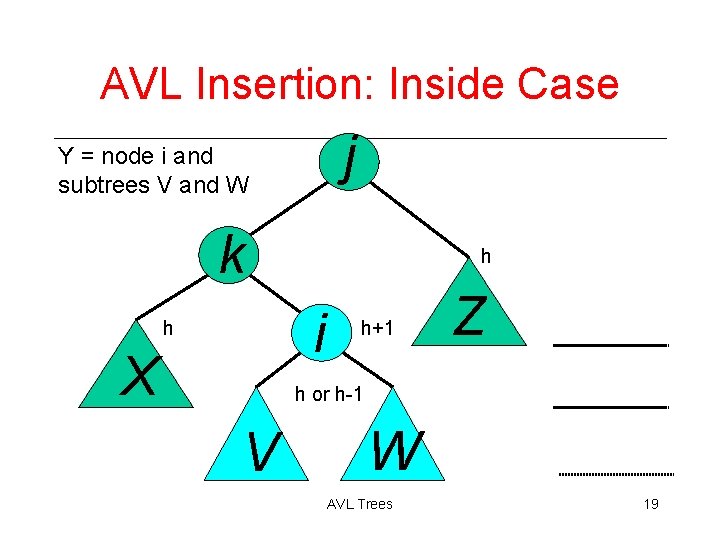
AVL Insertion: Inside Case j Y = node i and subtrees V and W k h i h X h+1 Z h or h-1 V W AVL Trees 19
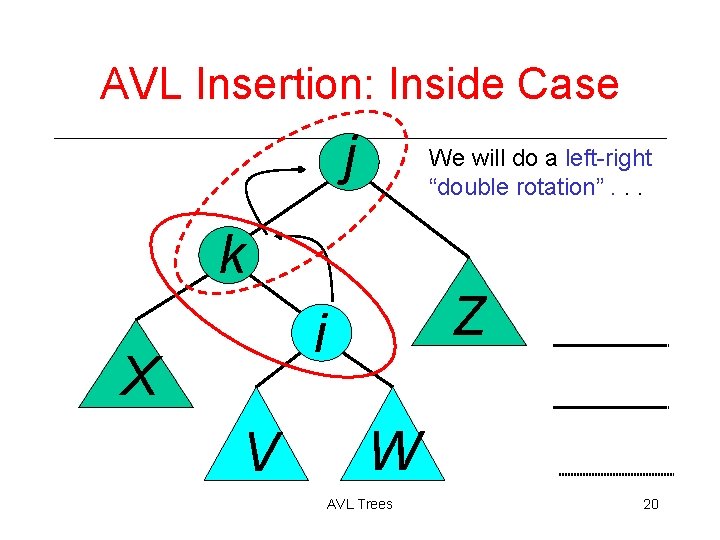
AVL Insertion: Inside Case j We will do a left-right “double rotation”. . . k Z i X V W AVL Trees 20
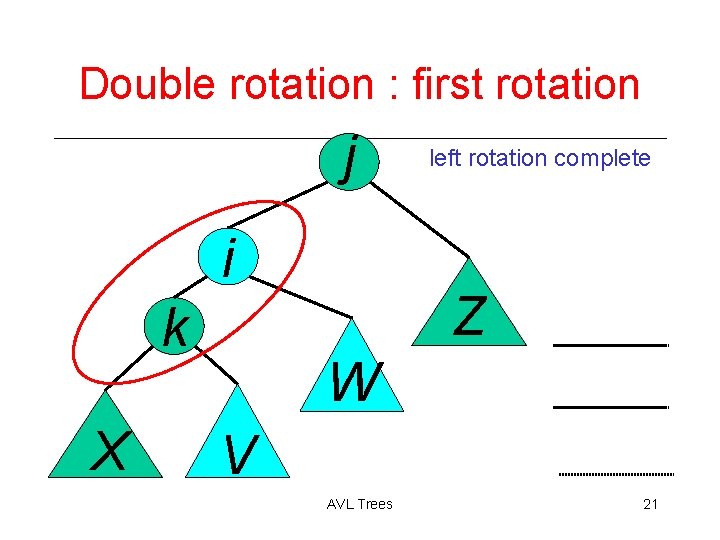
Double rotation : first rotation j i k X left rotation complete Z W V AVL Trees 21
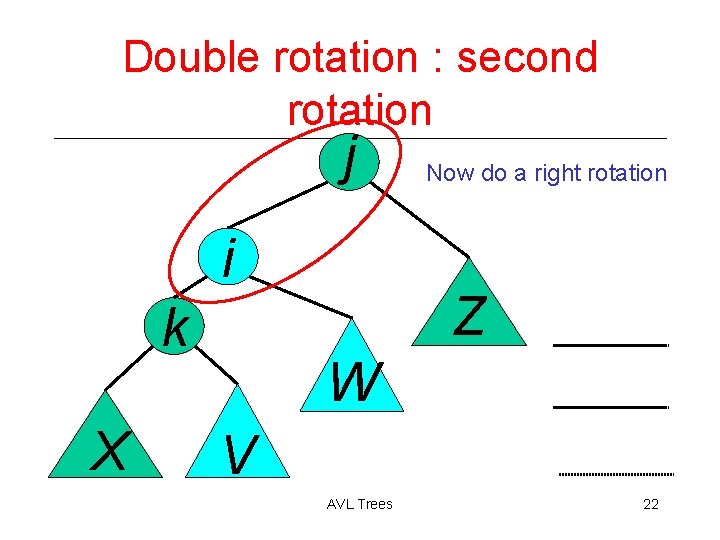
Double rotation : second rotation j i k X Now do a right rotation Z W V AVL Trees 22
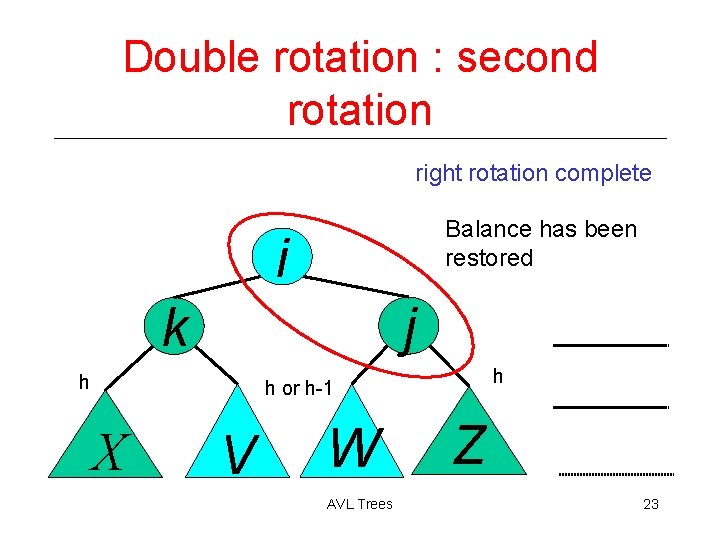
Double rotation : second rotation right rotation complete Balance has been restored i j k h h h or h-1 X V W AVL Trees Z 23
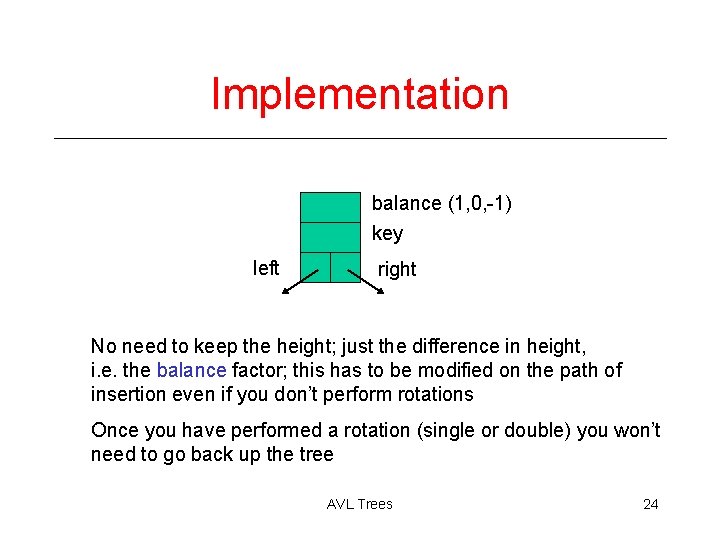
Implementation balance (1, 0, -1) key left right No need to keep the height; just the difference in height, i. e. the balance factor; this has to be modified on the path of insertion even if you don’t perform rotations Once you have performed a rotation (single or double) you won’t need to go back up the tree AVL Trees 24
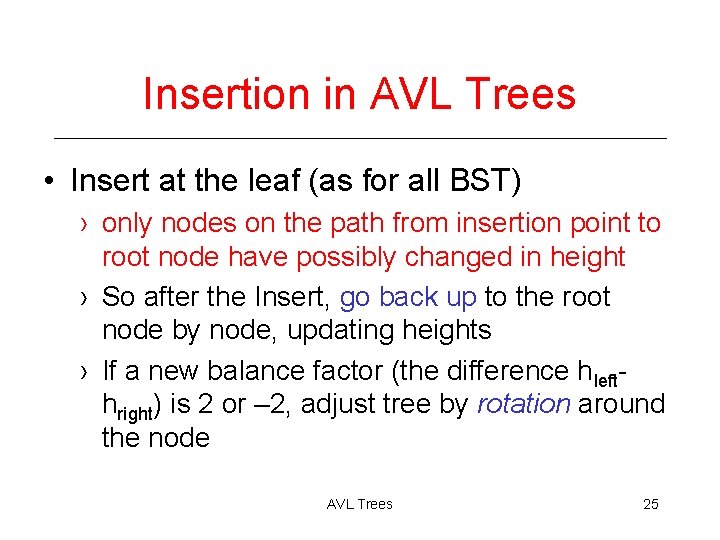
Insertion in AVL Trees • Insert at the leaf (as for all BST) › only nodes on the path from insertion point to root node have possibly changed in height › So after the Insert, go back up to the root node by node, updating heights › If a new balance factor (the difference hlefthright) is 2 or – 2, adjust tree by rotation around the node AVL Trees 25
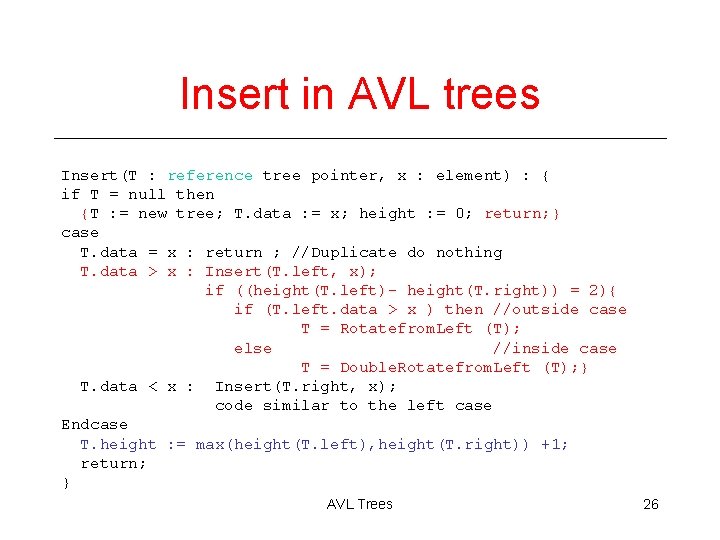
Insert in AVL trees Insert(T : reference tree pointer, x : element) : { if T = null then {T : = new tree; T. data : = x; height : = 0; return; } case T. data = x : return ; //Duplicate do nothing T. data > x : Insert(T. left, x); if ((height(T. left)- height(T. right)) = 2){ if (T. left. data > x ) then //outside case T = Rotatefrom. Left (T); else //inside case T = Double. Rotatefrom. Left (T); } T. data < x : Insert(T. right, x); code similar to the left case Endcase T. height : = max(height(T. left), height(T. right)) +1; return; } AVL Trees 26
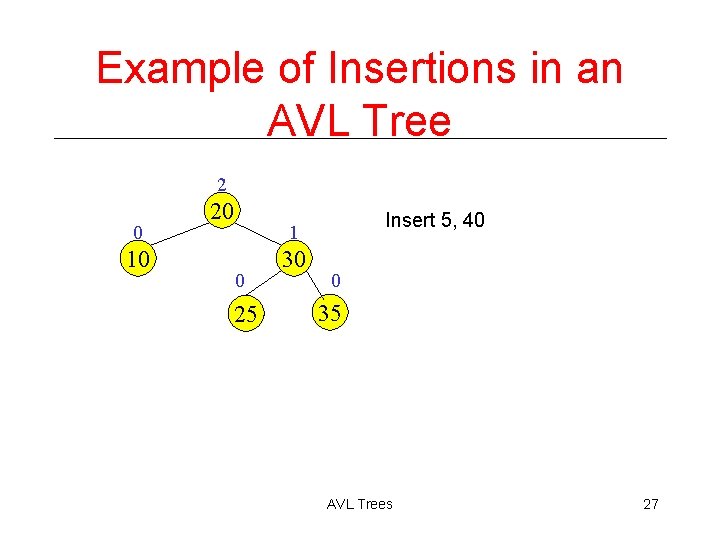
Example of Insertions in an AVL Tree 2 0 10 20 Insert 5, 40 1 0 25 30 0 35 AVL Trees 27
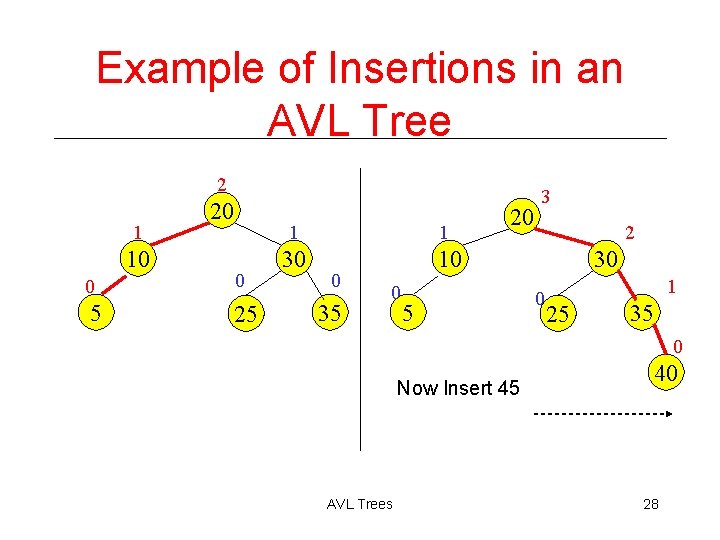
Example of Insertions in an AVL Tree 2 1 10 20 0 0 5 25 1 1 30 10 0 35 0 20 5 3 2 30 0 1 25 35 0 Now Insert 45 AVL Trees 40 28
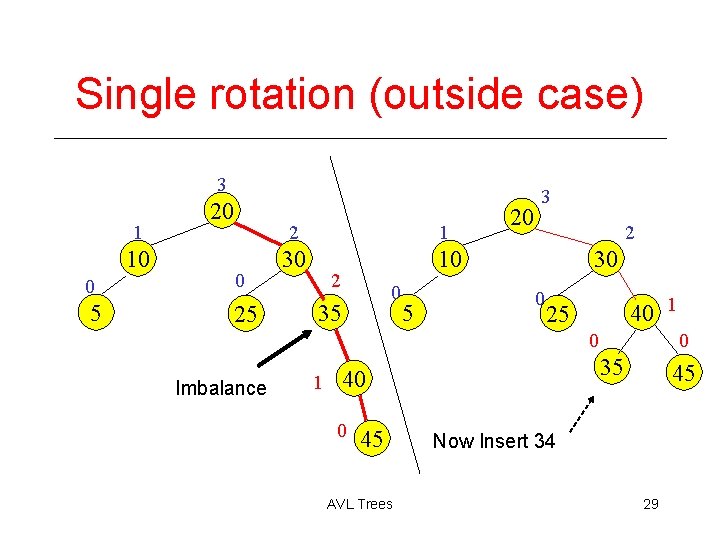
Single rotation (outside case) 3 1 10 20 0 0 5 25 2 1 30 10 2 0 35 5 20 3 2 30 0 40 1 25 0 Imbalance 35 1 40 0 45 AVL Trees 0 45 Now Insert 34 29
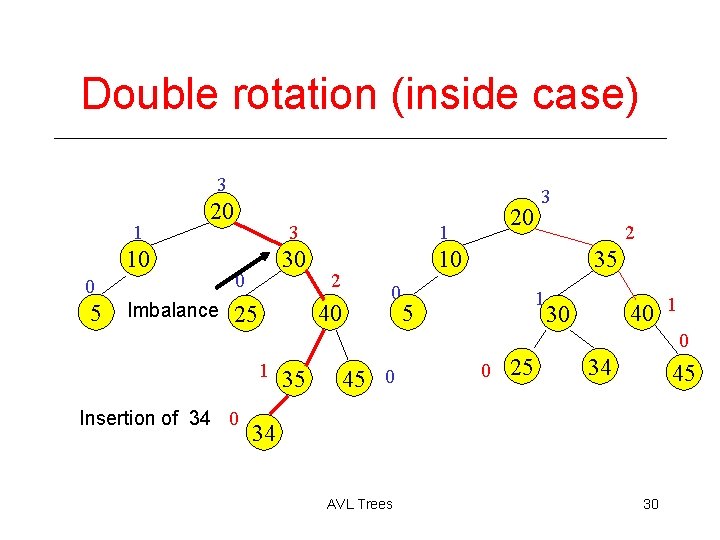
Double rotation (inside case) 3 1 20 10 0 5 Imbalance 0 3 1 30 10 2 40 0 25 20 3 2 35 1 5 40 1 30 0 1 Insertion of 34 0 35 45 0 0 25 34 45 34 AVL Trees 30
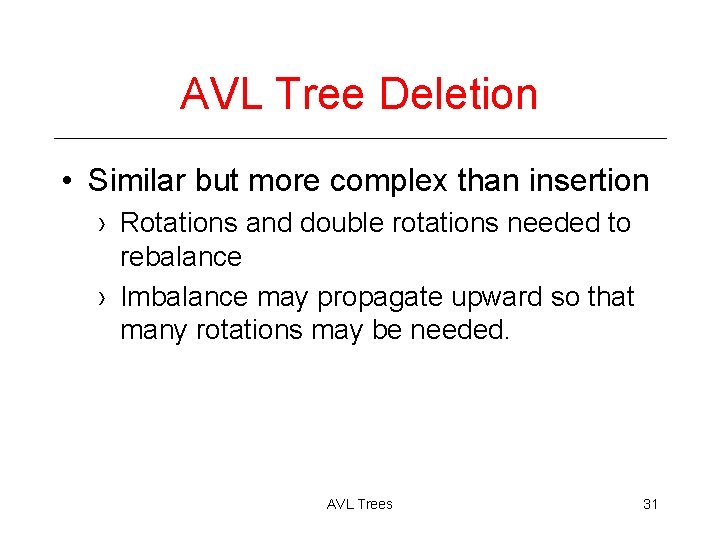
AVL Tree Deletion • Similar but more complex than insertion › Rotations and double rotations needed to rebalance › Imbalance may propagate upward so that many rotations may be needed. AVL Trees 31
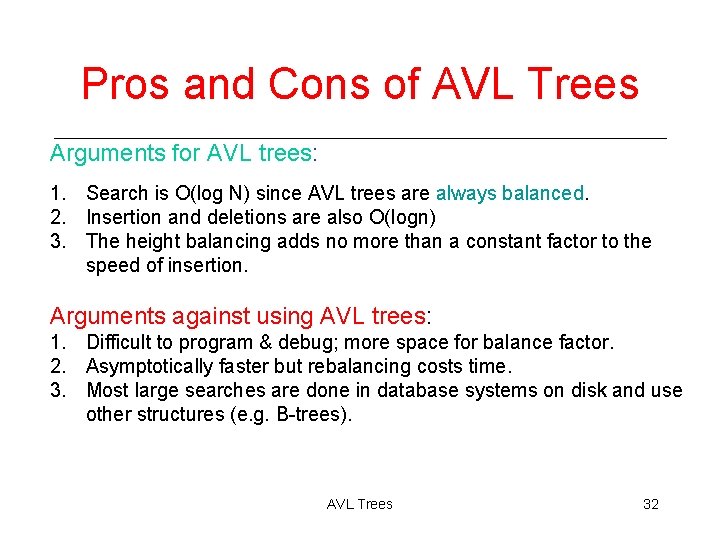
Pros and Cons of AVL Trees Arguments for AVL trees: 1. Search is O(log N) since AVL trees are always balanced. 2. Insertion and deletions are also O(logn) 3. The height balancing adds no more than a constant factor to the speed of insertion. Arguments against using AVL trees: 1. Difficult to program & debug; more space for balance factor. 2. Asymptotically faster but rebalancing costs time. 3. Most large searches are done in database systems on disk and use other structures (e. g. B-trees). AVL Trees 32
Itcs 6114
Itcs 6114
Ajit diwan iit bombay
Amit agarwal princeton
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Data structures and algorithms iit bombay
Data structures and algorithms
Data structures and algorithms
Ian munro waterloo
Information retrieval data structures and algorithms
Data structures and algorithms
Algorithms + data structures = programs
Ecu itcs
Ic primo levi bollate
Primo levi the gray zone
Homologous structures definition
Build an avl tree with the following values
Avl data structure
Stream data model
Threaded tree
Zegour
Simulador avl
Avl tutorial
Avl tree ll rotation
Split avl tree
Avl binary search tree
Razes avl
Arbre avl
Les arbres avl
Arbre avl algorithme
Sifat struktur data tree