ITCS 6114 Graph Algorithms 1 9142021 DepthFirst Search
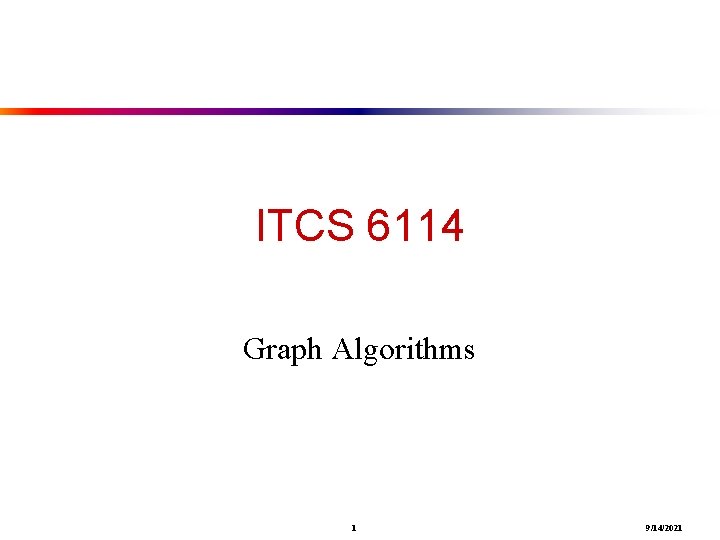
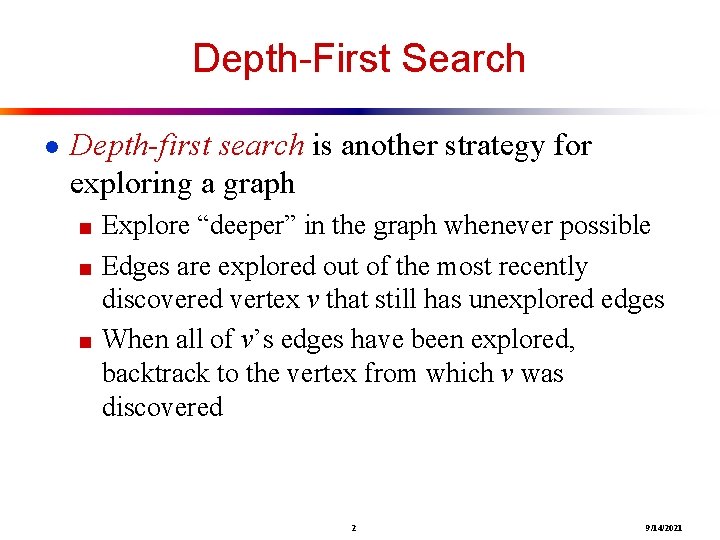
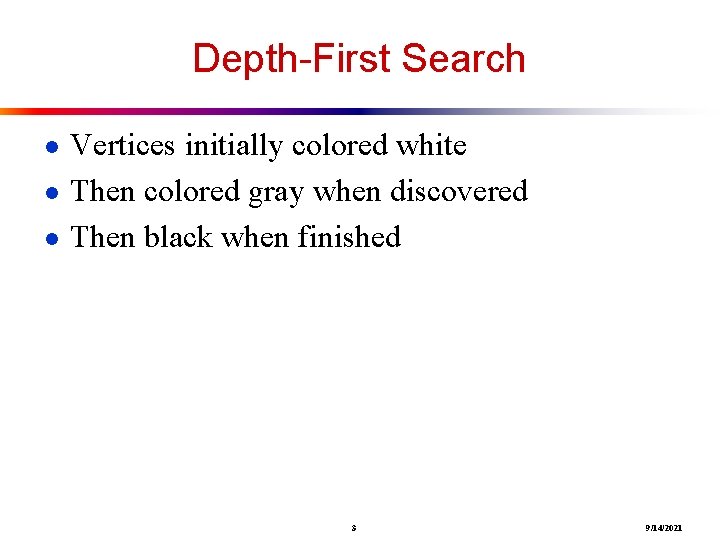
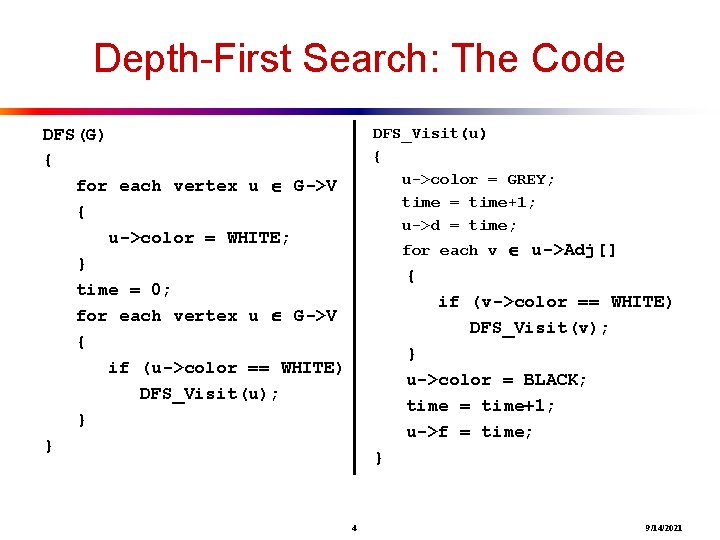
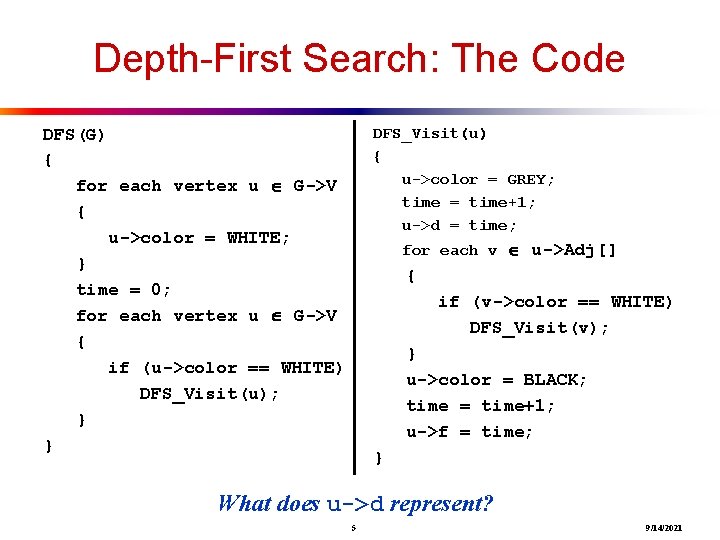
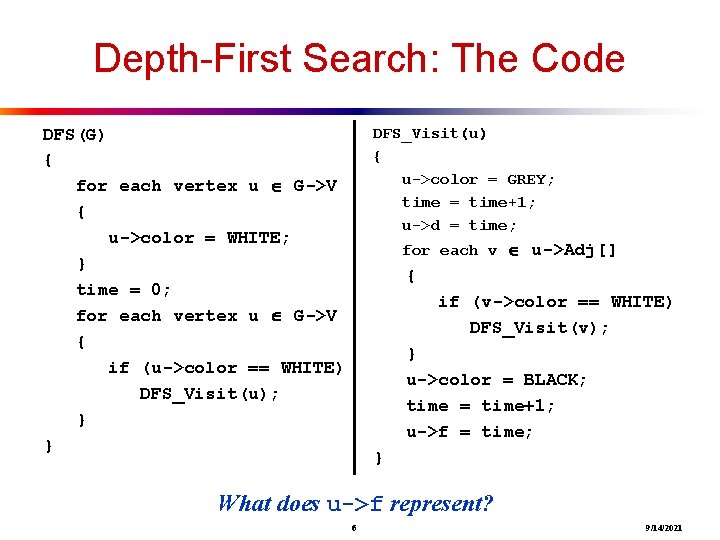
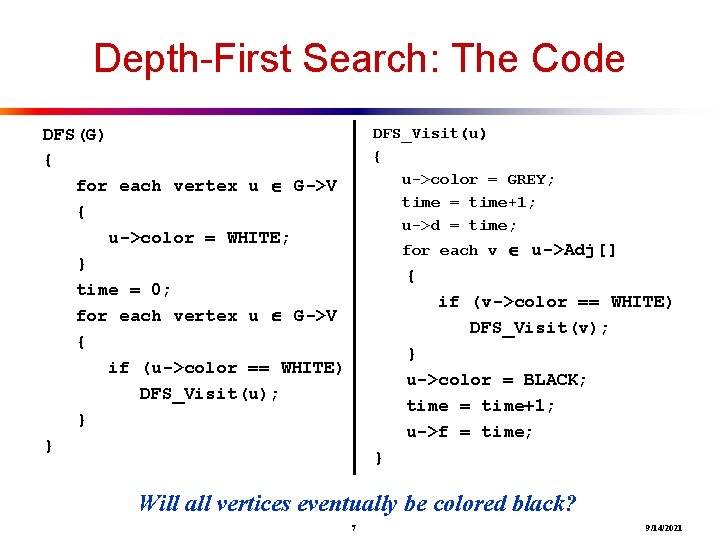
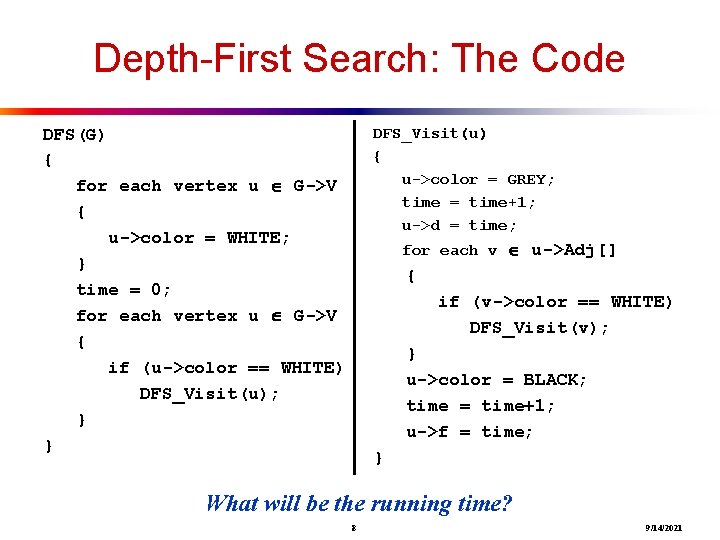
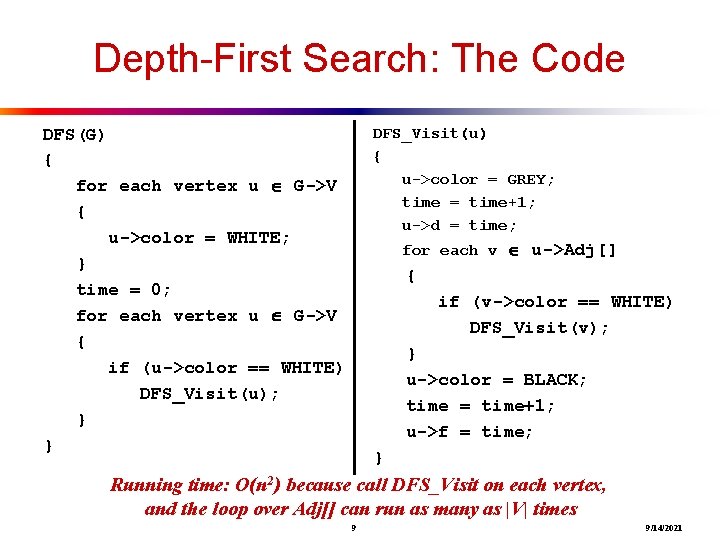
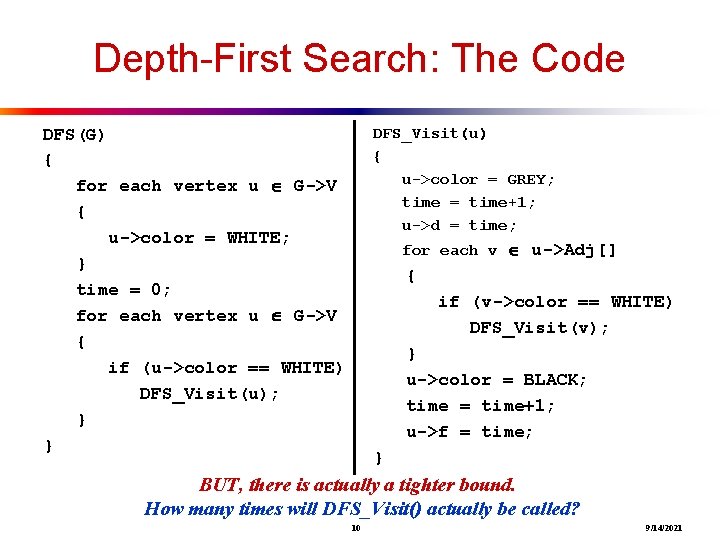
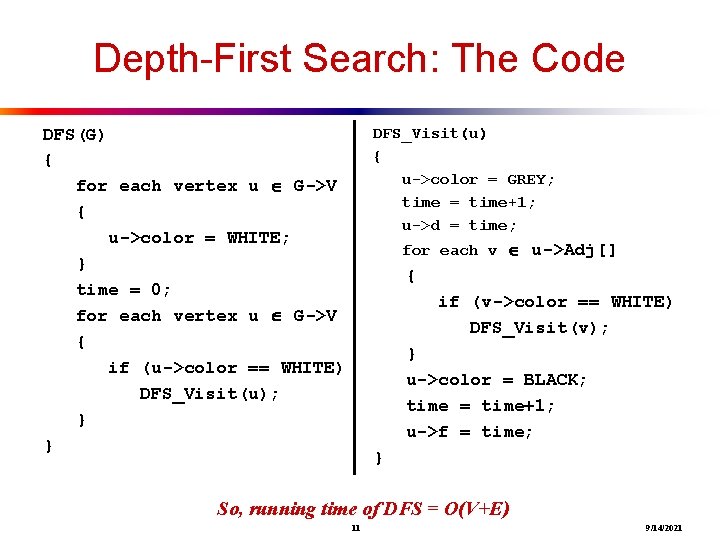
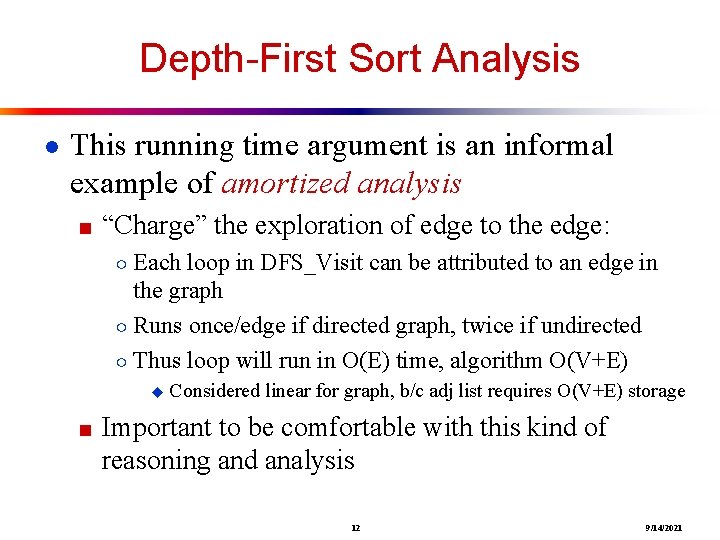
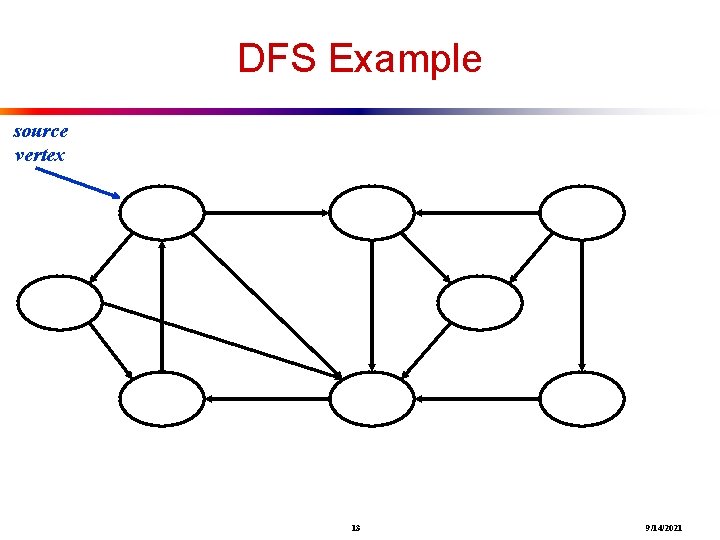
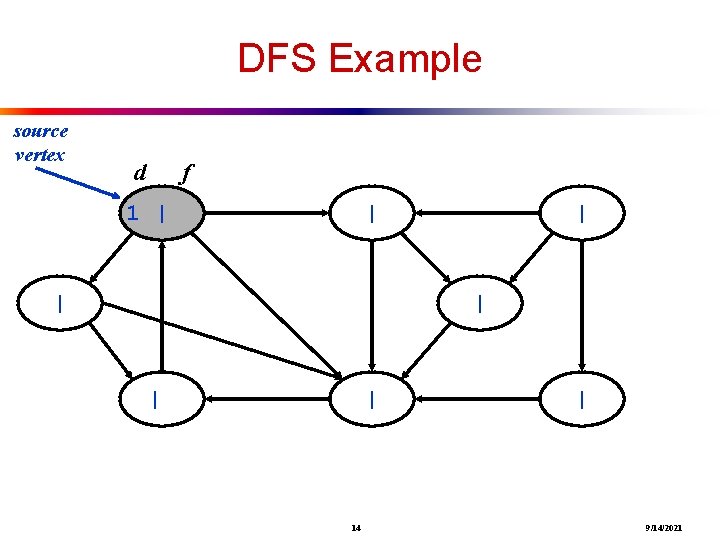
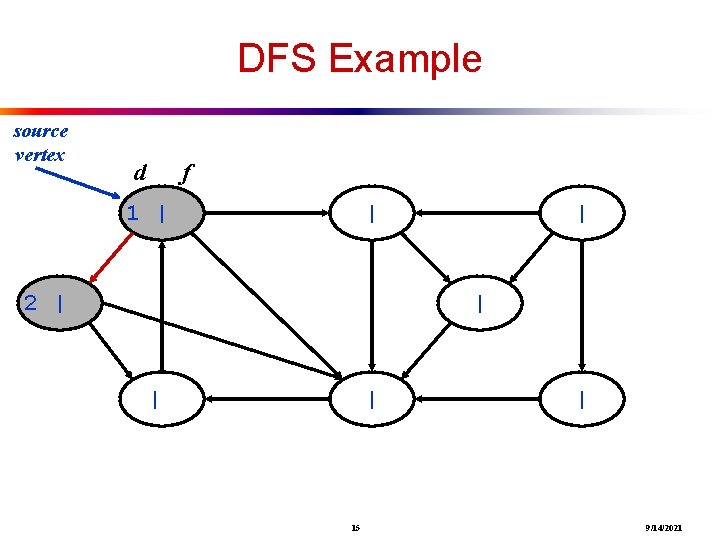
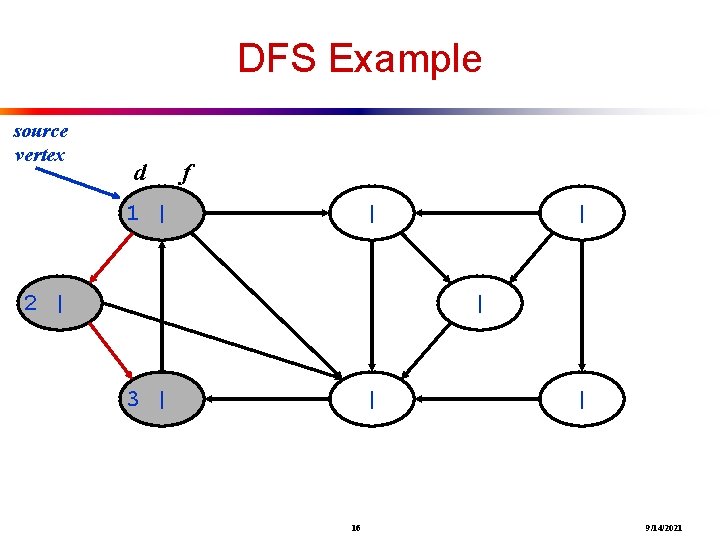
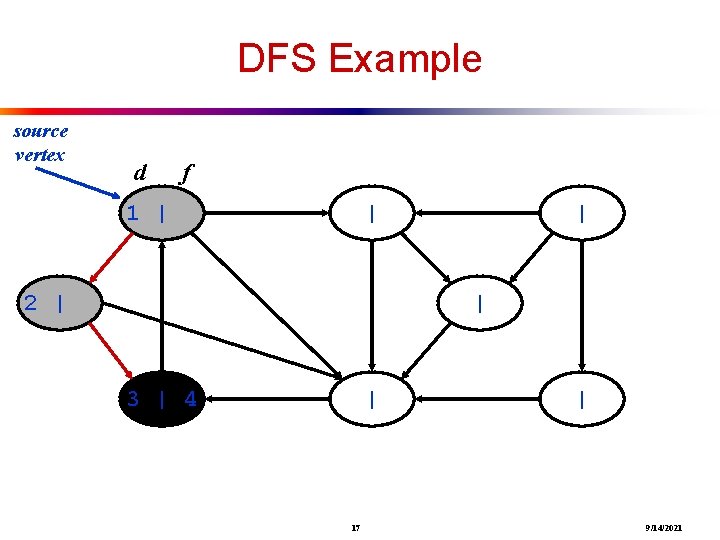
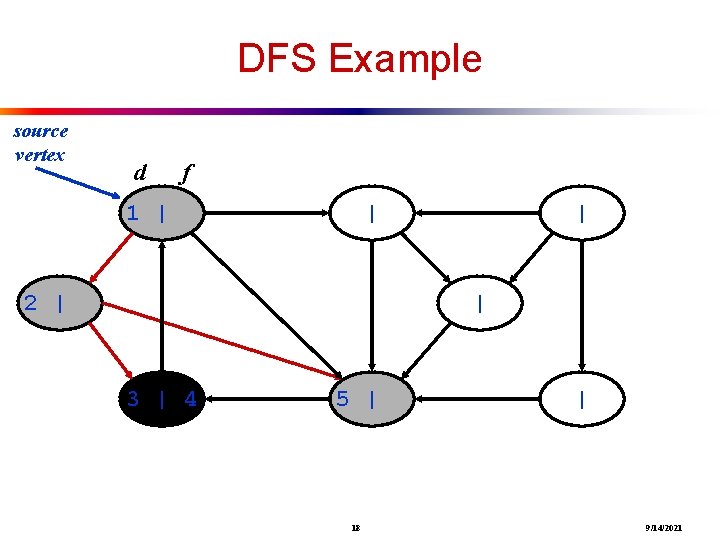
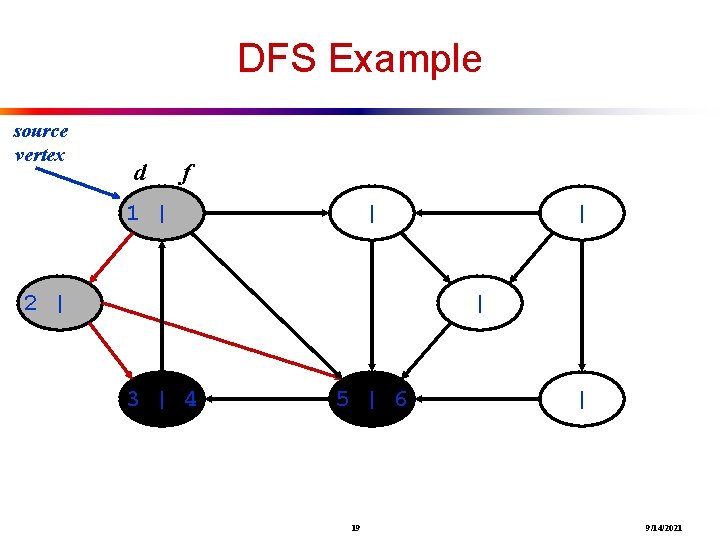
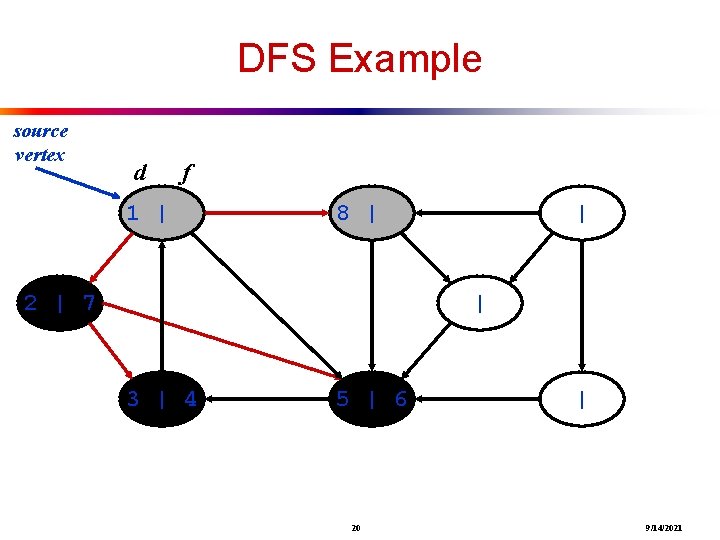
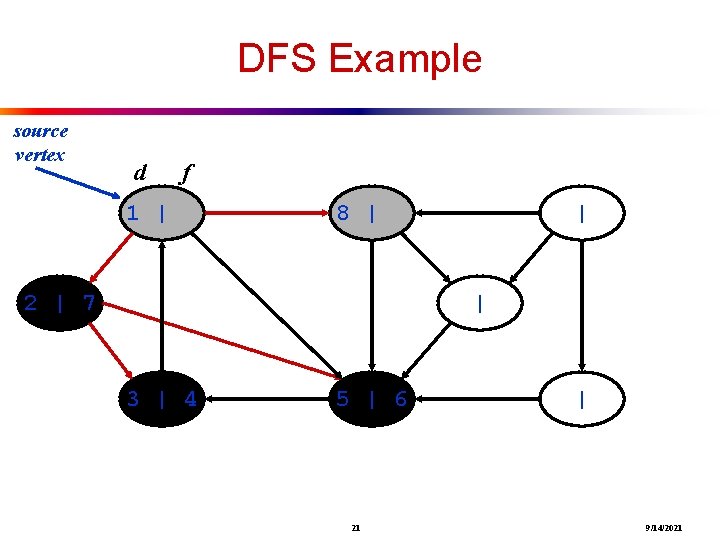
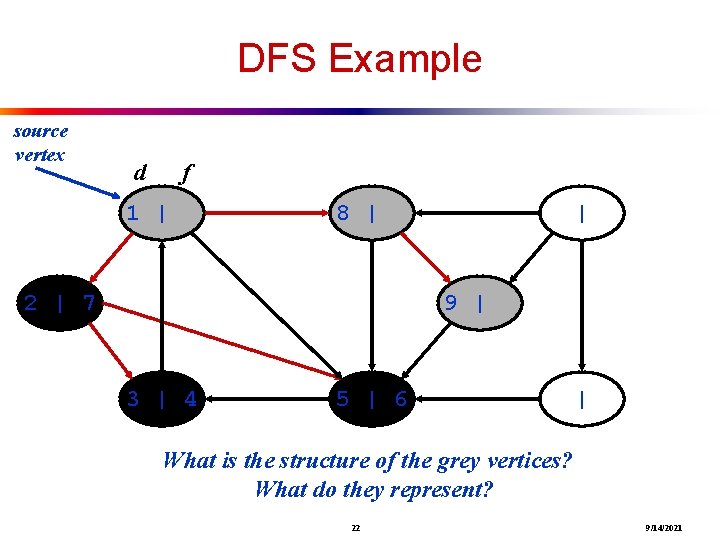
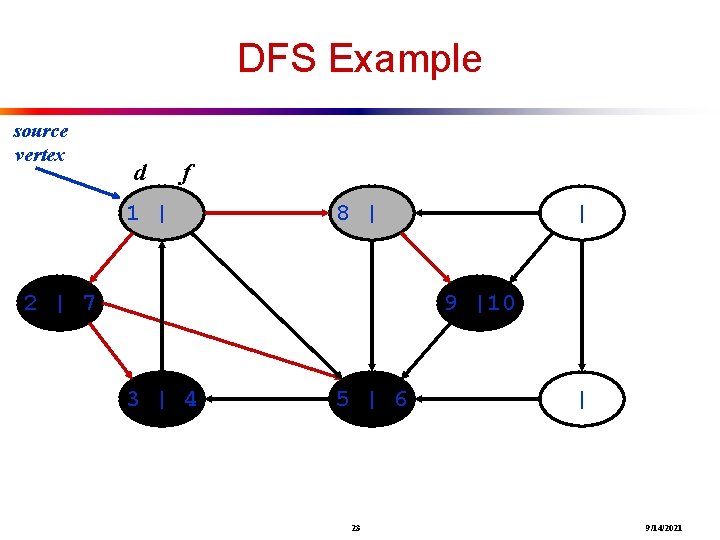
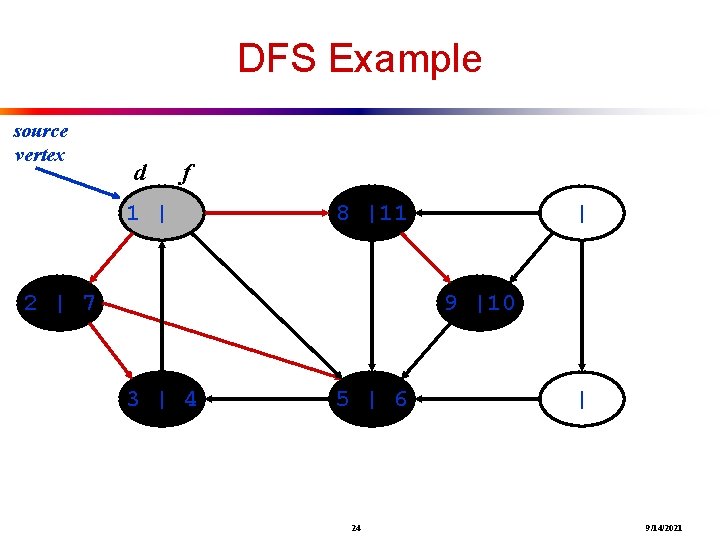
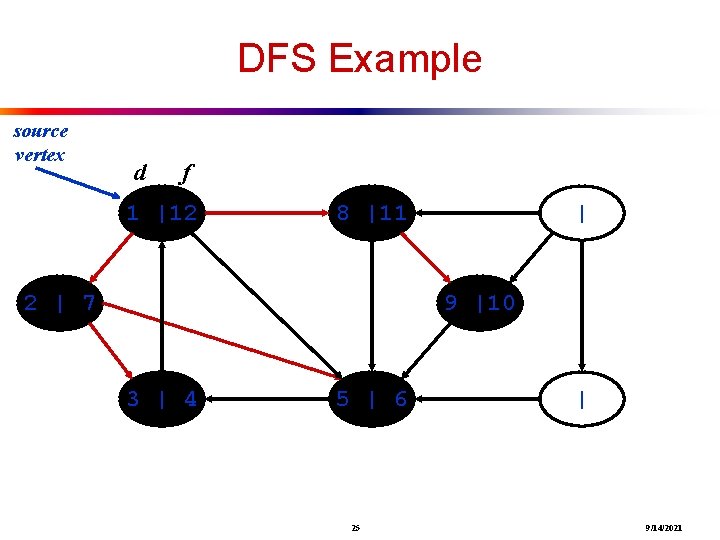
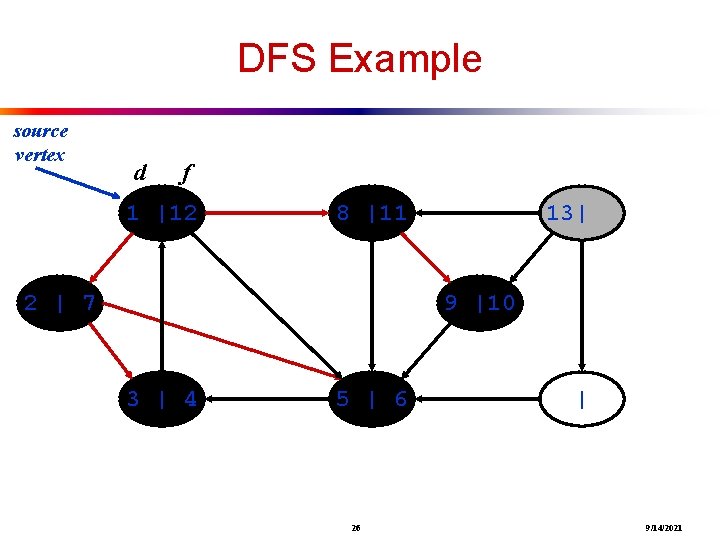
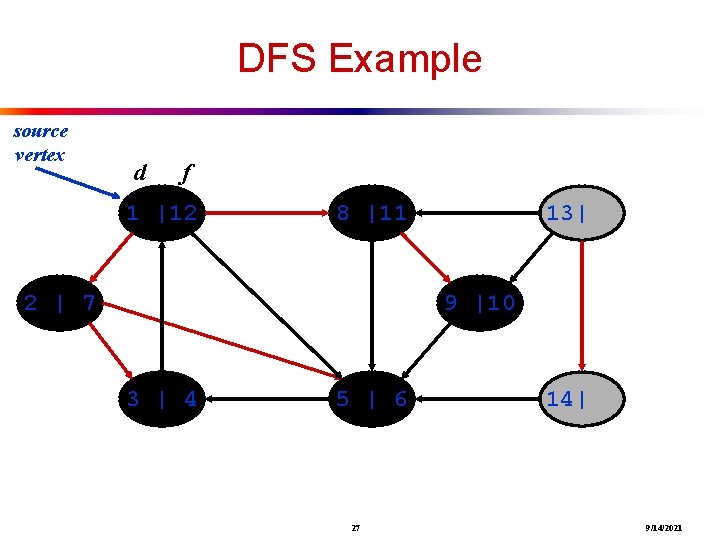
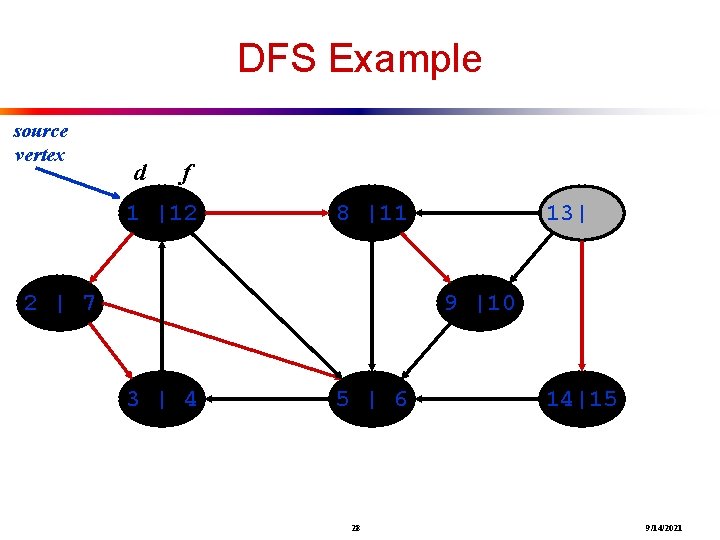
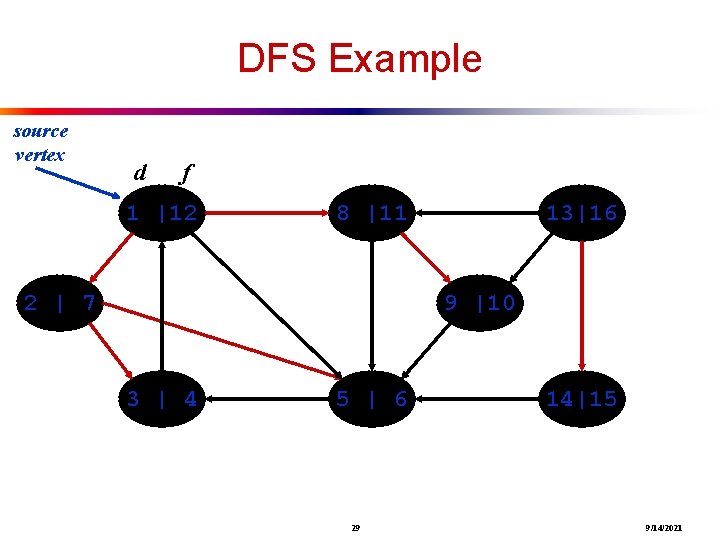
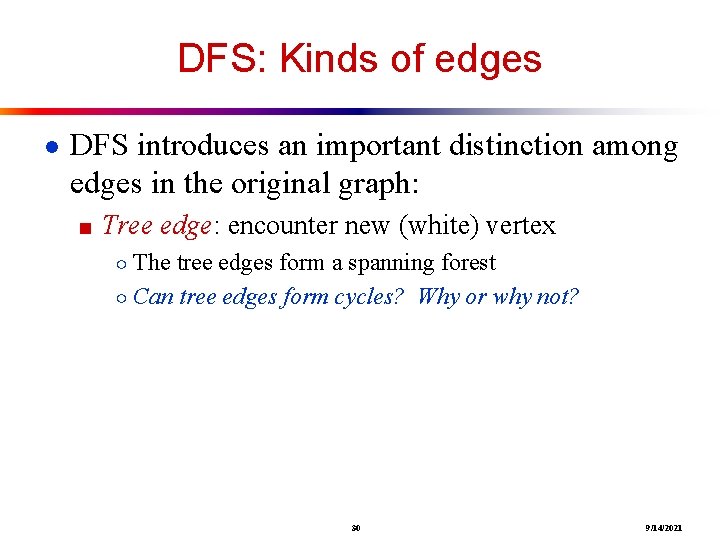
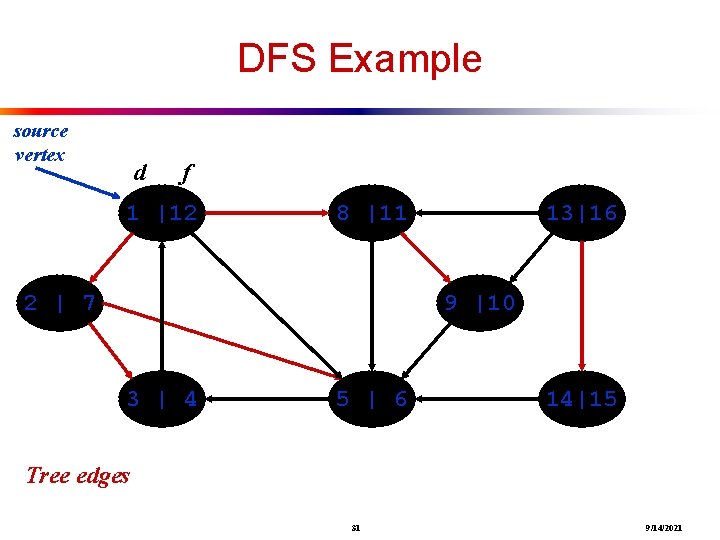
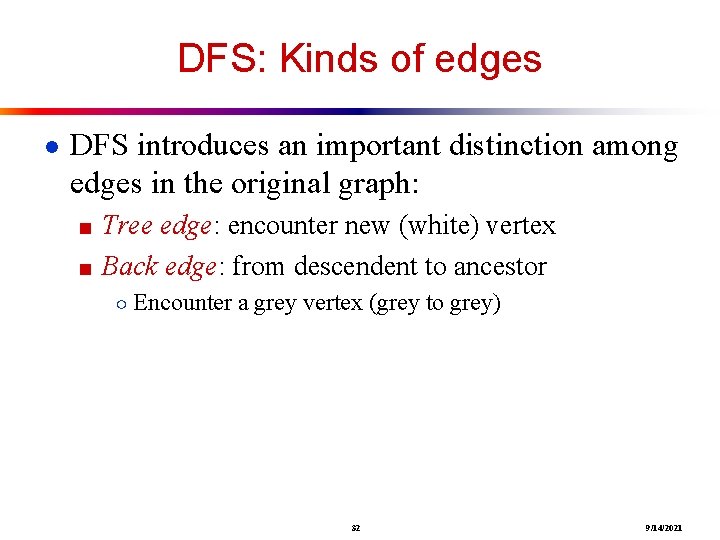
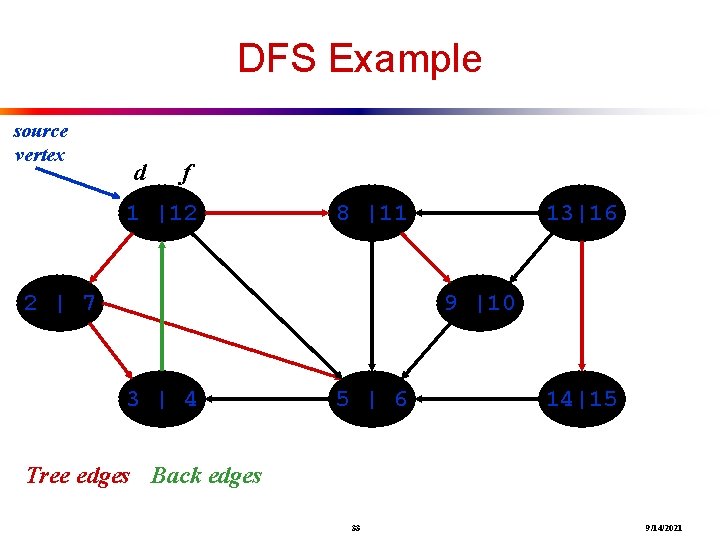
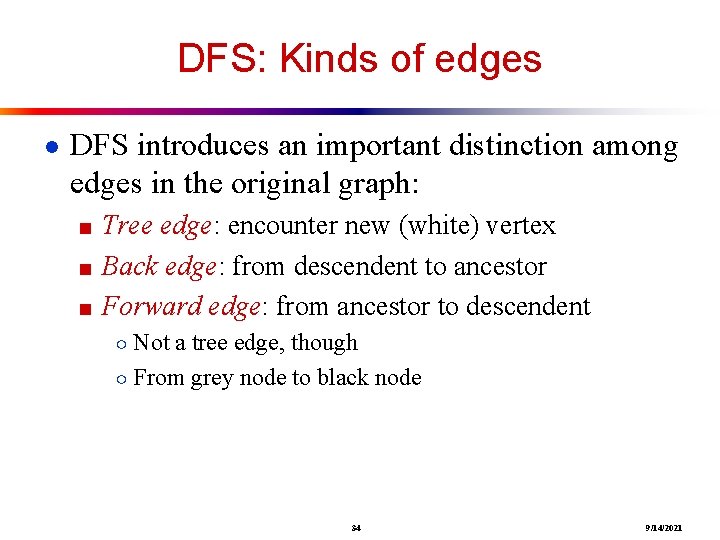
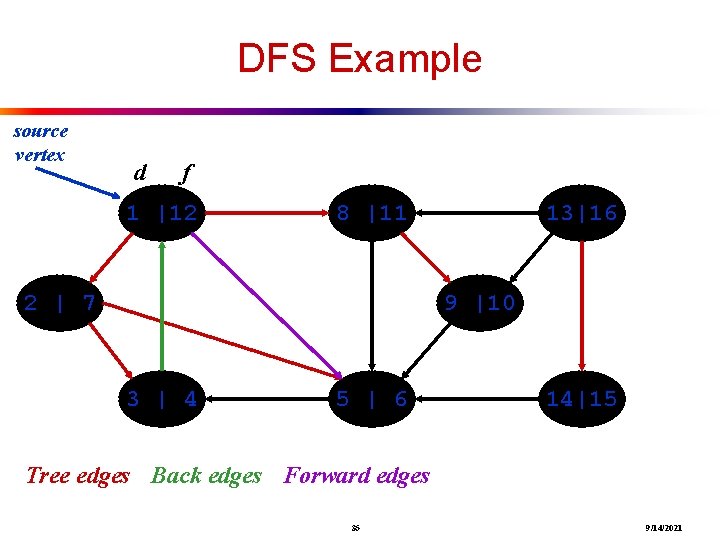
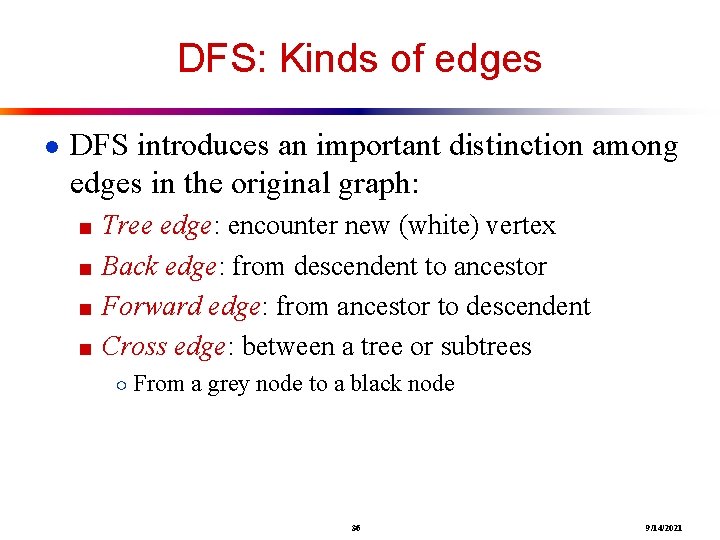
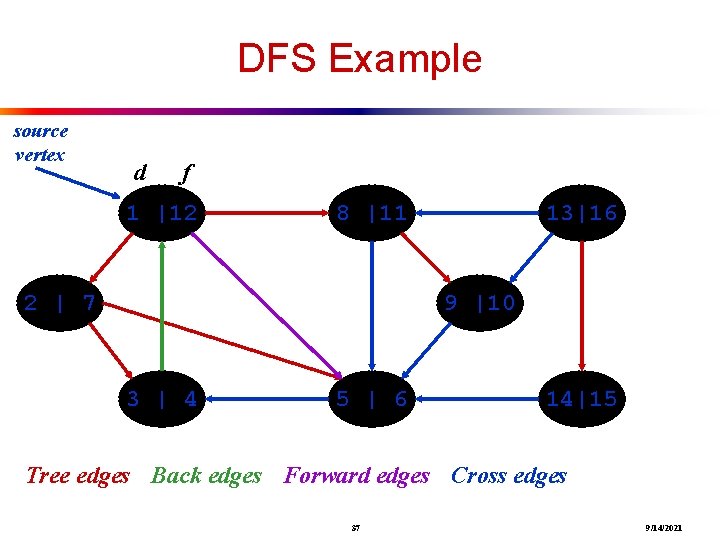
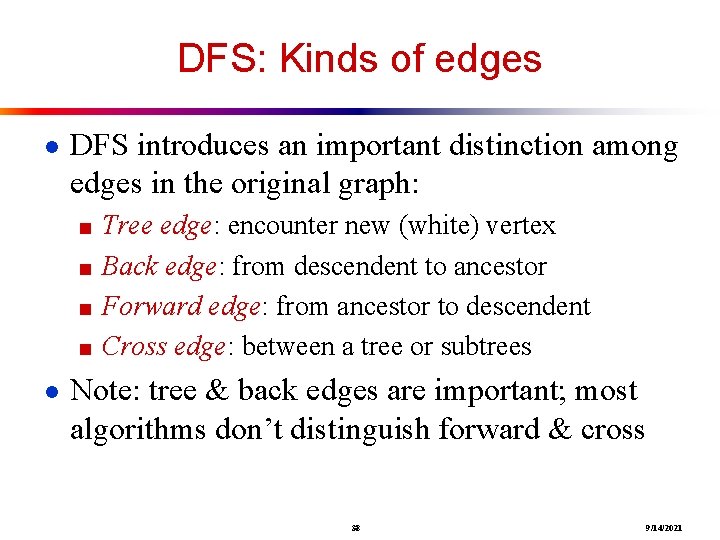
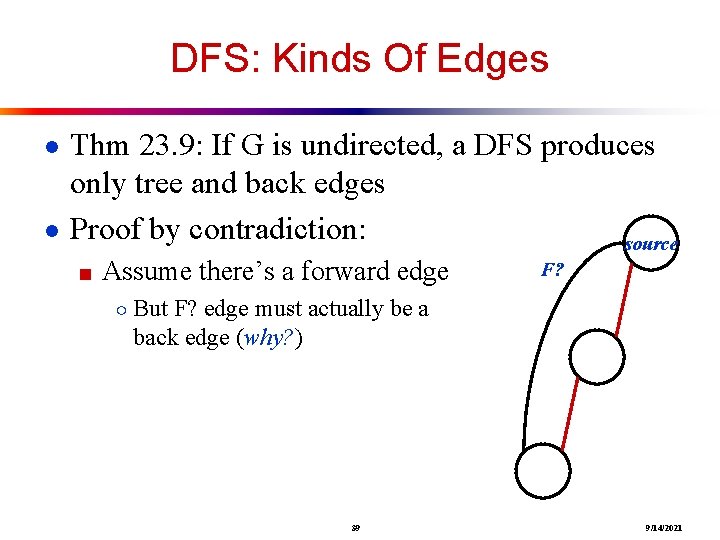
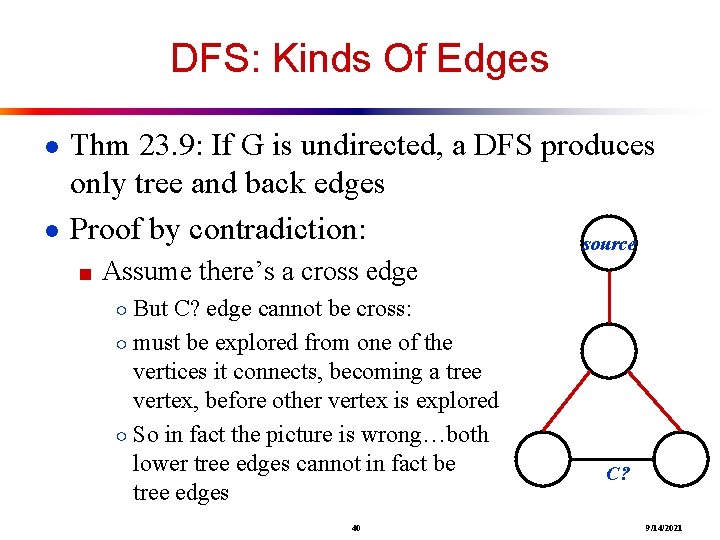
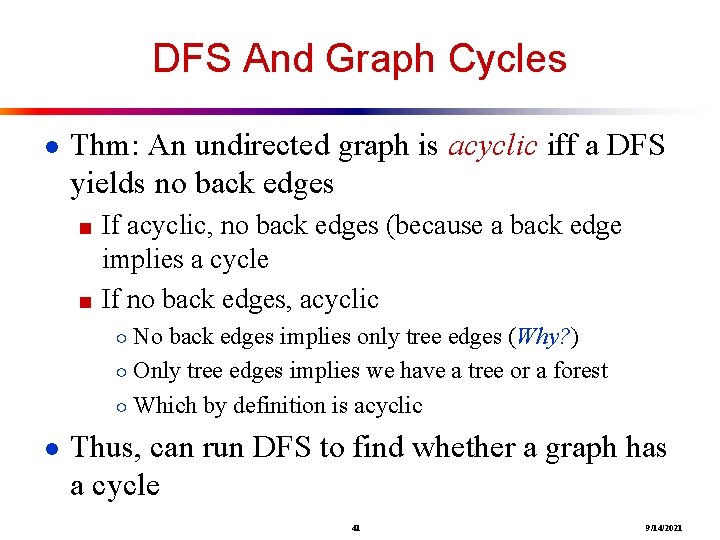
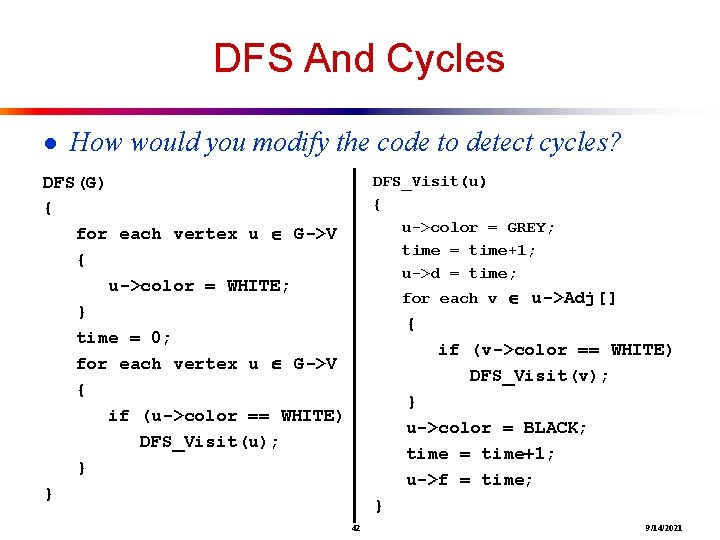
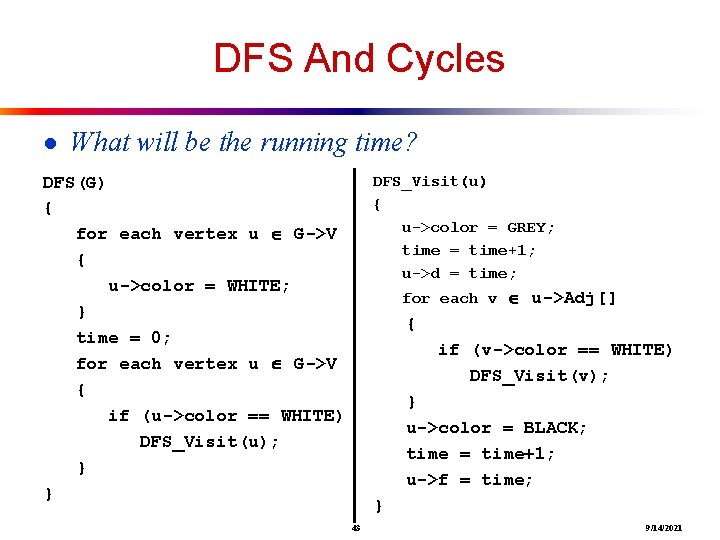
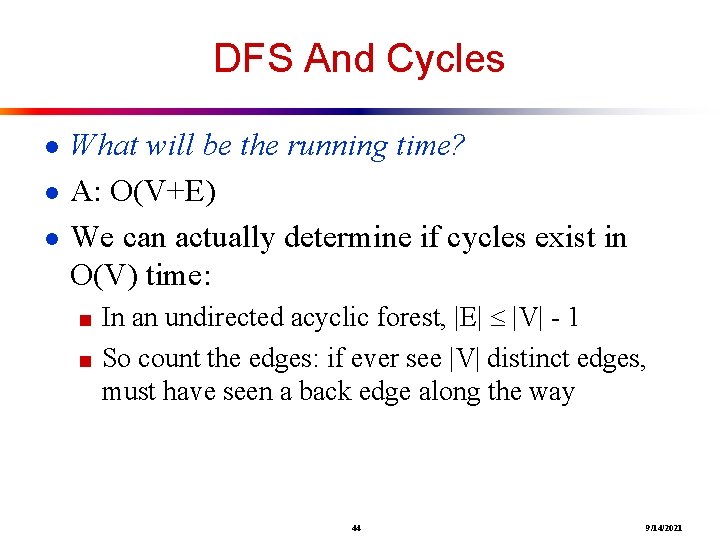
- Slides: 44
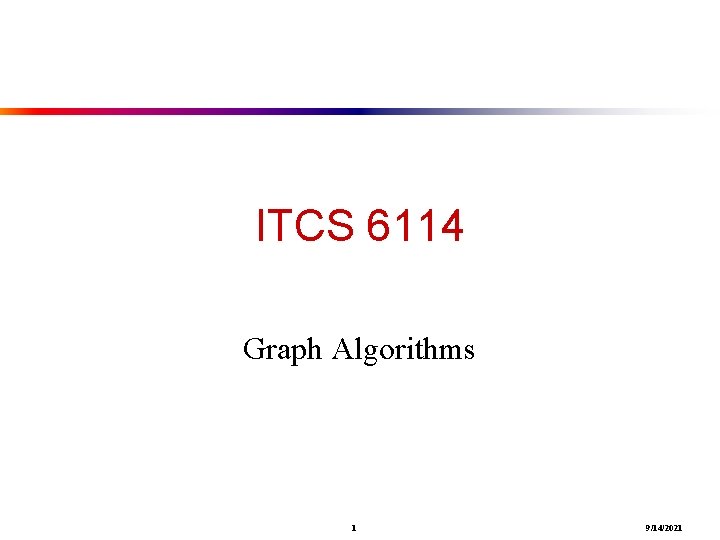
ITCS 6114 Graph Algorithms 1 9/14/2021
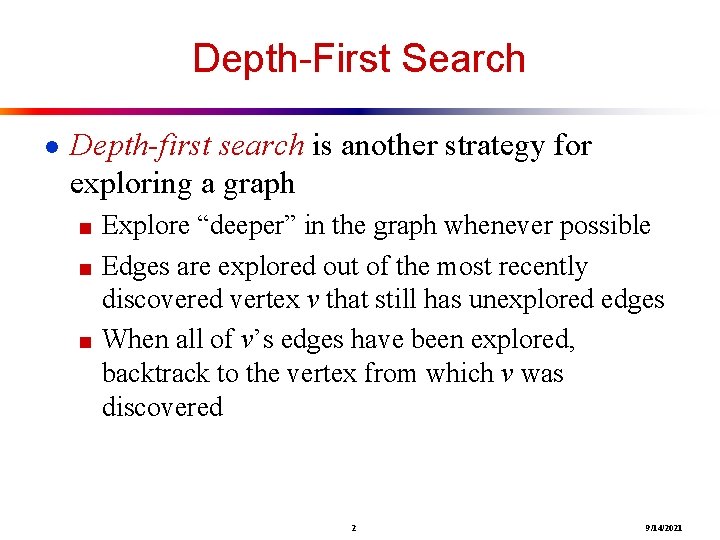
Depth-First Search ● Depth-first search is another strategy for exploring a graph ■ Explore “deeper” in the graph whenever possible ■ Edges are explored out of the most recently discovered vertex v that still has unexplored edges ■ When all of v’s edges have been explored, backtrack to the vertex from which v was discovered 2 9/14/2021
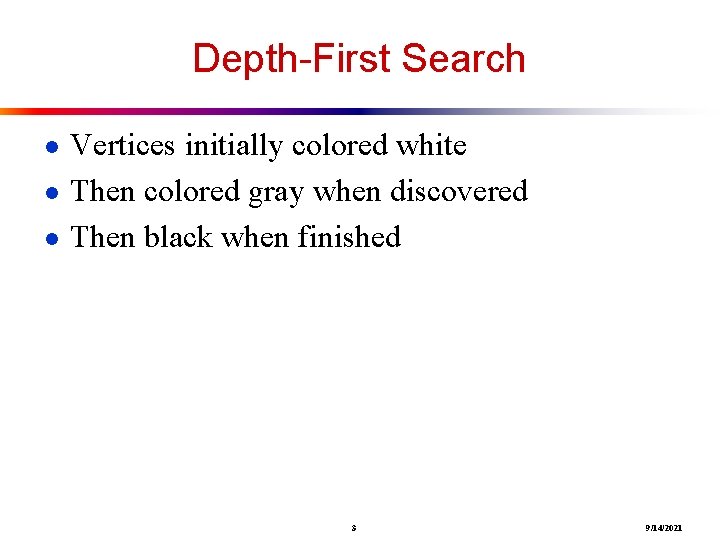
Depth-First Search ● Vertices initially colored white ● Then colored gray when discovered ● Then black when finished 3 9/14/2021
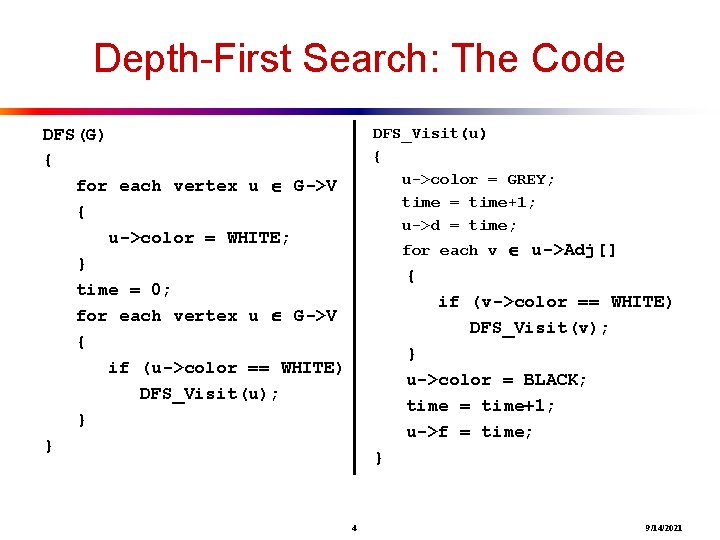
Depth-First Search: The Code DFS_Visit(u) { u->color = GREY; time = time+1; u->d = time; DFS(G) { for each vertex u G->V { u->color = WHITE; } time = 0; for each vertex u G->V { if (u->color == WHITE) DFS_Visit(u); } } for each v u->Adj[] { if (v->color == WHITE) DFS_Visit(v); } u->color = BLACK; time = time+1; u->f = time; } 4 9/14/2021
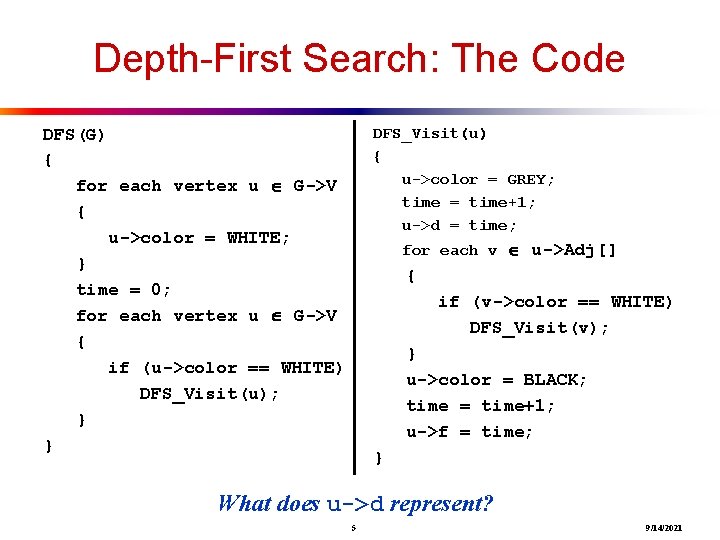
Depth-First Search: The Code DFS_Visit(u) { u->color = GREY; time = time+1; u->d = time; DFS(G) { for each vertex u G->V { u->color = WHITE; } time = 0; for each vertex u G->V { if (u->color == WHITE) DFS_Visit(u); } } for each v u->Adj[] { if (v->color == WHITE) DFS_Visit(v); } u->color = BLACK; time = time+1; u->f = time; } What does u->d represent? 5 9/14/2021
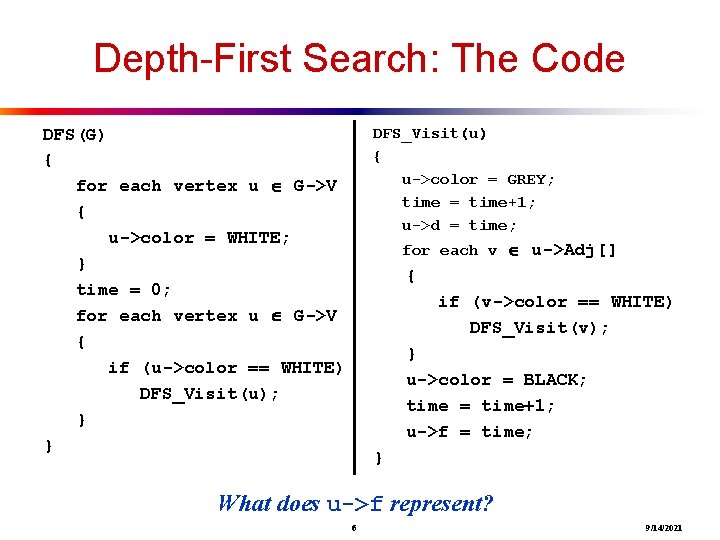
Depth-First Search: The Code DFS_Visit(u) { u->color = GREY; time = time+1; u->d = time; DFS(G) { for each vertex u G->V { u->color = WHITE; } time = 0; for each vertex u G->V { if (u->color == WHITE) DFS_Visit(u); } } for each v u->Adj[] { if (v->color == WHITE) DFS_Visit(v); } u->color = BLACK; time = time+1; u->f = time; } What does u->f represent? 6 9/14/2021
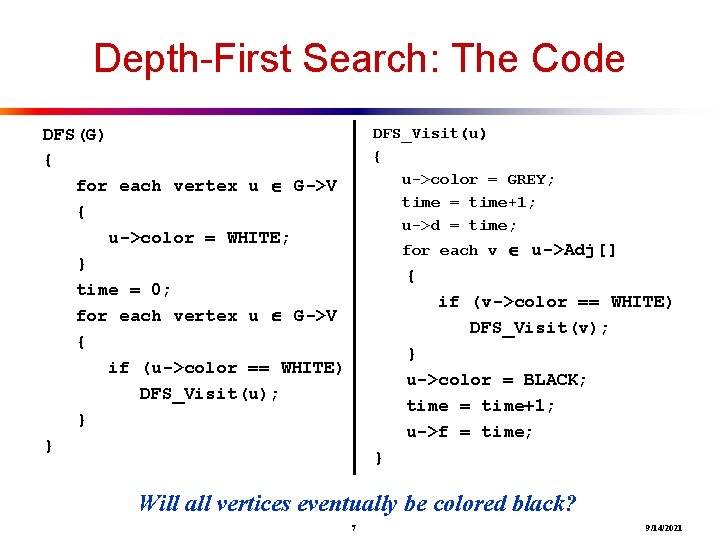
Depth-First Search: The Code DFS_Visit(u) { u->color = GREY; time = time+1; u->d = time; DFS(G) { for each vertex u G->V { u->color = WHITE; } time = 0; for each vertex u G->V { if (u->color == WHITE) DFS_Visit(u); } } for each v u->Adj[] { if (v->color == WHITE) DFS_Visit(v); } u->color = BLACK; time = time+1; u->f = time; } Will all vertices eventually be colored black? 7 9/14/2021
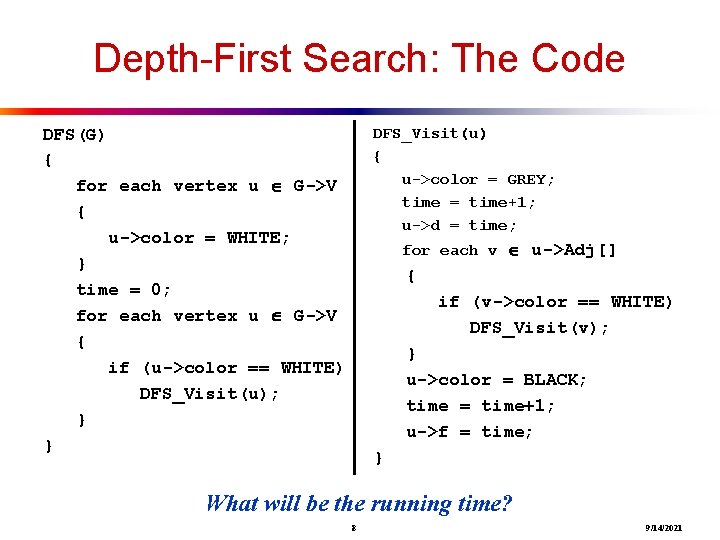
Depth-First Search: The Code DFS_Visit(u) { u->color = GREY; time = time+1; u->d = time; DFS(G) { for each vertex u G->V { u->color = WHITE; } time = 0; for each vertex u G->V { if (u->color == WHITE) DFS_Visit(u); } } for each v u->Adj[] { if (v->color == WHITE) DFS_Visit(v); } u->color = BLACK; time = time+1; u->f = time; } What will be the running time? 8 9/14/2021
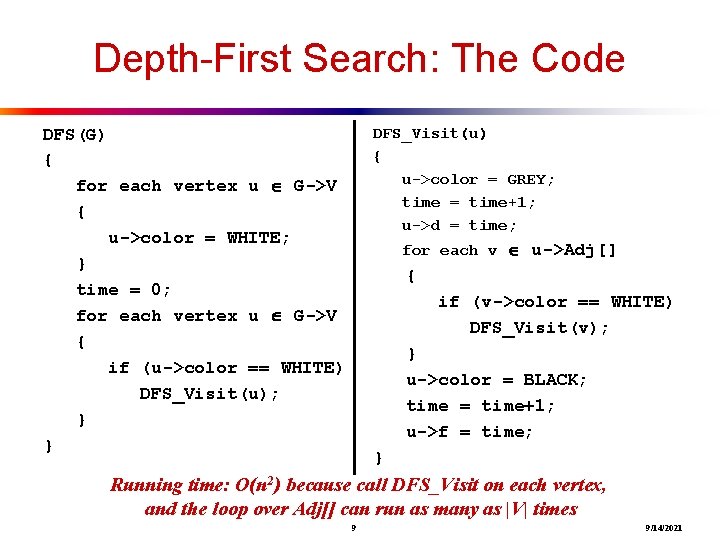
Depth-First Search: The Code DFS_Visit(u) { u->color = GREY; time = time+1; u->d = time; DFS(G) { for each vertex u G->V { u->color = WHITE; } time = 0; for each vertex u G->V { if (u->color == WHITE) DFS_Visit(u); } } for each v u->Adj[] { if (v->color == WHITE) DFS_Visit(v); } u->color = BLACK; time = time+1; u->f = time; } Running time: O(n 2) because call DFS_Visit on each vertex, and the loop over Adj[] can run as many as |V| times 9 9/14/2021
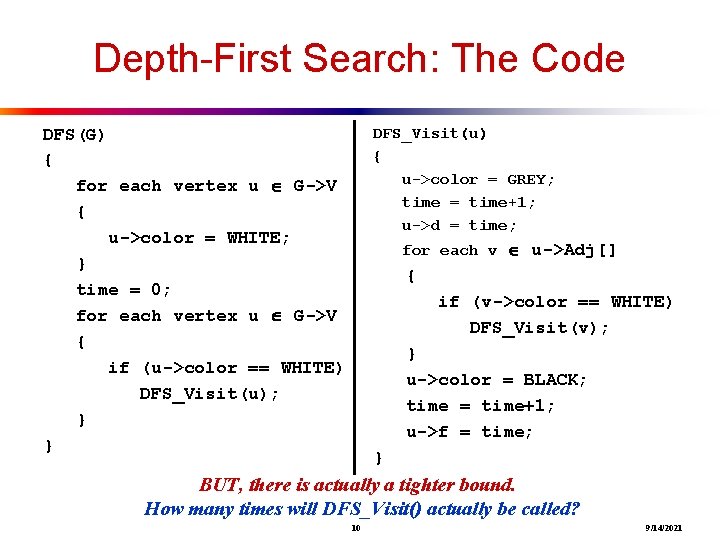
Depth-First Search: The Code DFS_Visit(u) { u->color = GREY; time = time+1; u->d = time; DFS(G) { for each vertex u G->V { u->color = WHITE; } time = 0; for each vertex u G->V { if (u->color == WHITE) DFS_Visit(u); } } for each v u->Adj[] { if (v->color == WHITE) DFS_Visit(v); } u->color = BLACK; time = time+1; u->f = time; } BUT, there is actually a tighter bound. How many times will DFS_Visit() actually be called? 10 9/14/2021
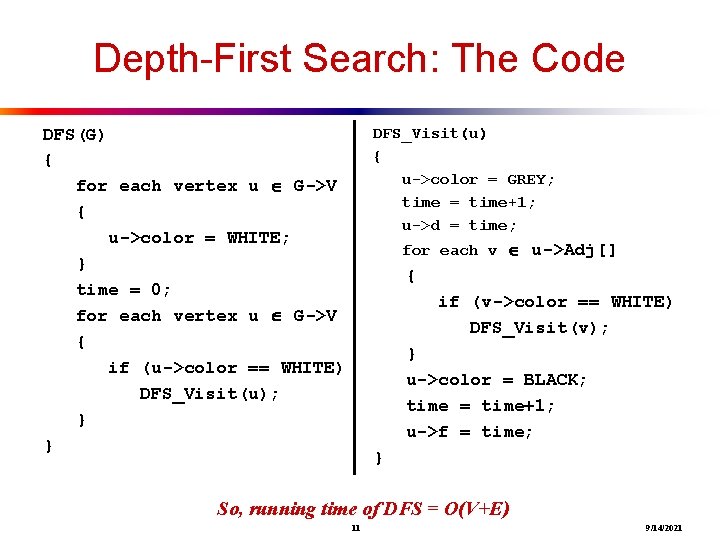
Depth-First Search: The Code DFS_Visit(u) { u->color = GREY; time = time+1; u->d = time; DFS(G) { for each vertex u G->V { u->color = WHITE; } time = 0; for each vertex u G->V { if (u->color == WHITE) DFS_Visit(u); } } for each v u->Adj[] { if (v->color == WHITE) DFS_Visit(v); } u->color = BLACK; time = time+1; u->f = time; } So, running time of DFS = O(V+E) 11 9/14/2021
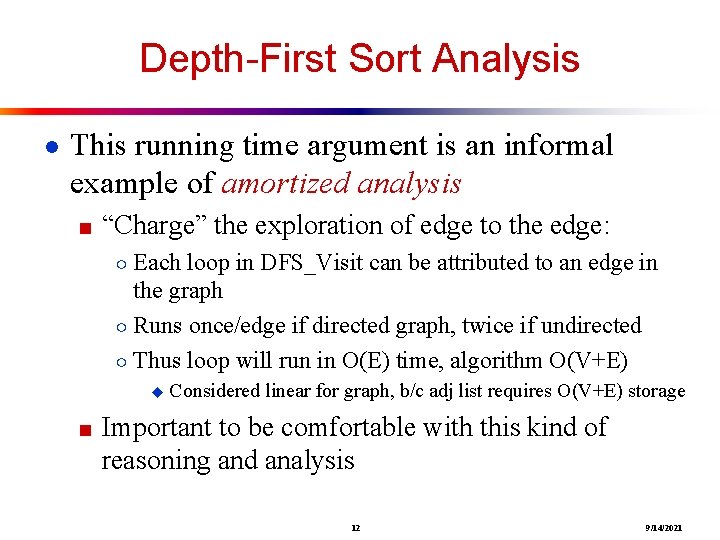
Depth-First Sort Analysis ● This running time argument is an informal example of amortized analysis ■ “Charge” the exploration of edge to the edge: ○ Each loop in DFS_Visit can be attributed to an edge in the graph ○ Runs once/edge if directed graph, twice if undirected ○ Thus loop will run in O(E) time, algorithm O(V+E) u Considered linear for graph, b/c adj list requires O(V+E) storage ■ Important to be comfortable with this kind of reasoning and analysis 12 9/14/2021
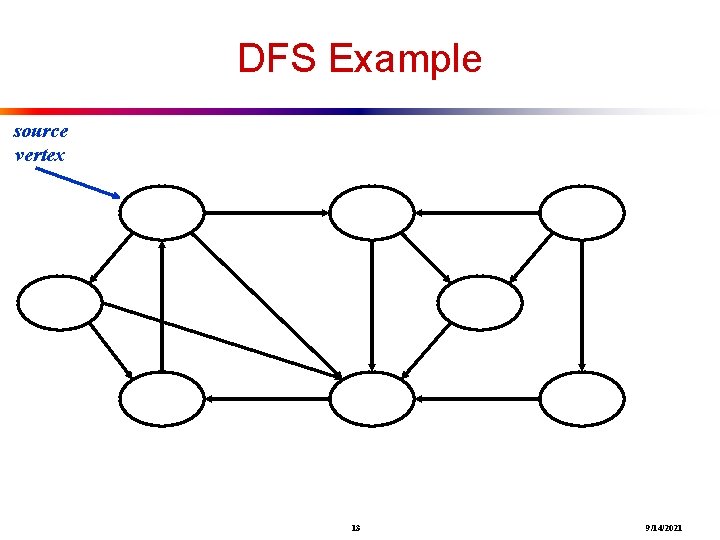
DFS Example source vertex 13 9/14/2021
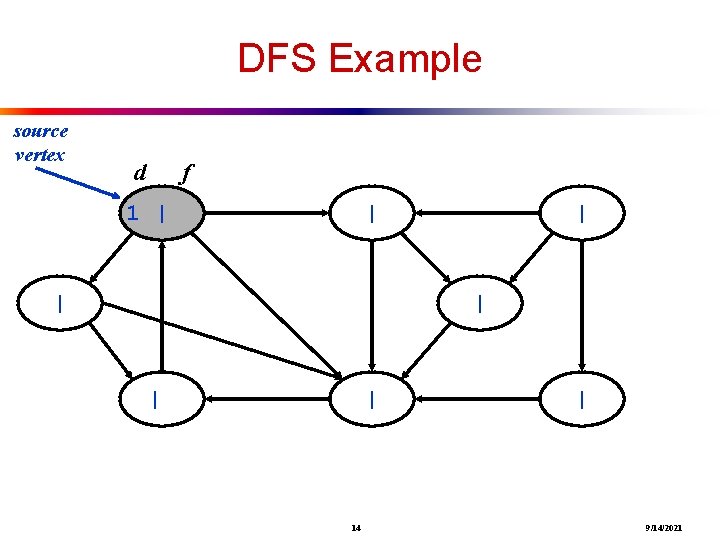
DFS Example source vertex d f 1 | | | | 14 | 9/14/2021
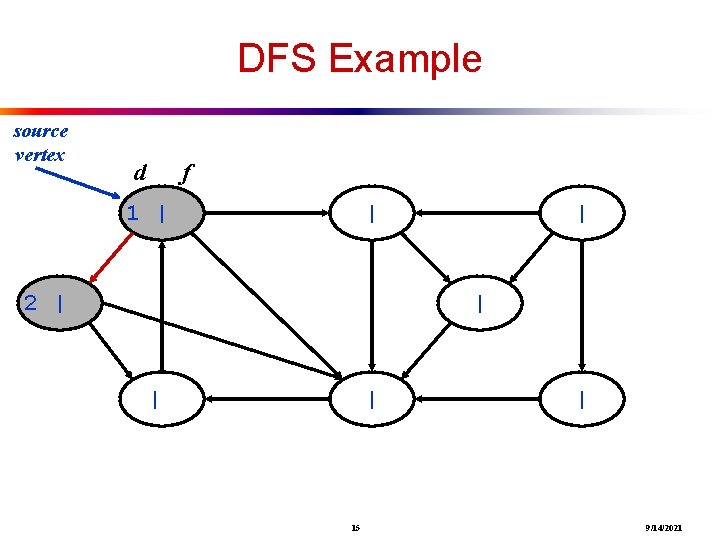
DFS Example source vertex d f 1 | | 2 | | | 15 | 9/14/2021
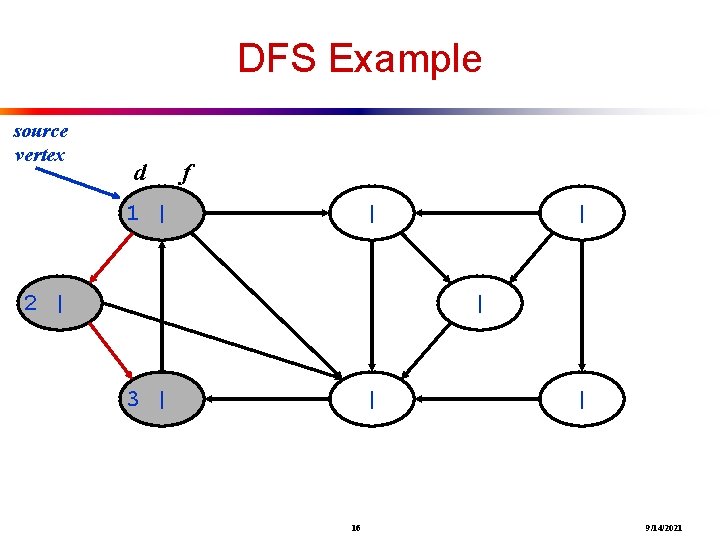
DFS Example source vertex d f 1 | | 2 | | | 3 | | 16 | 9/14/2021
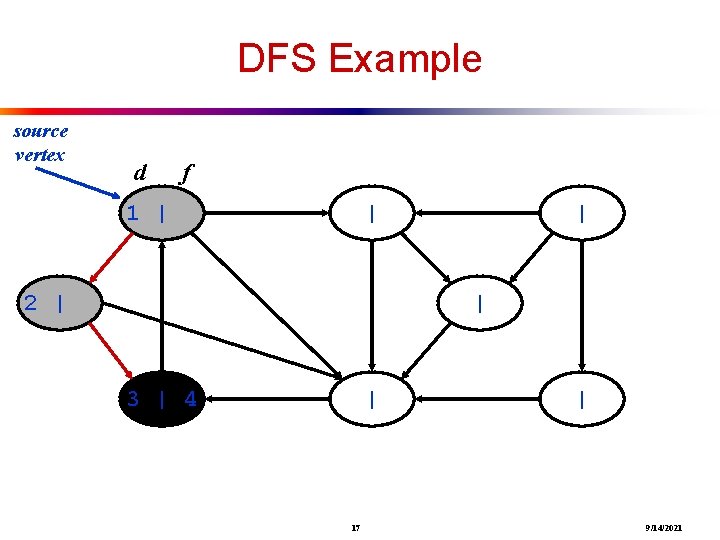
DFS Example source vertex d f 1 | | 2 | | | 3 | 4 | 17 | 9/14/2021
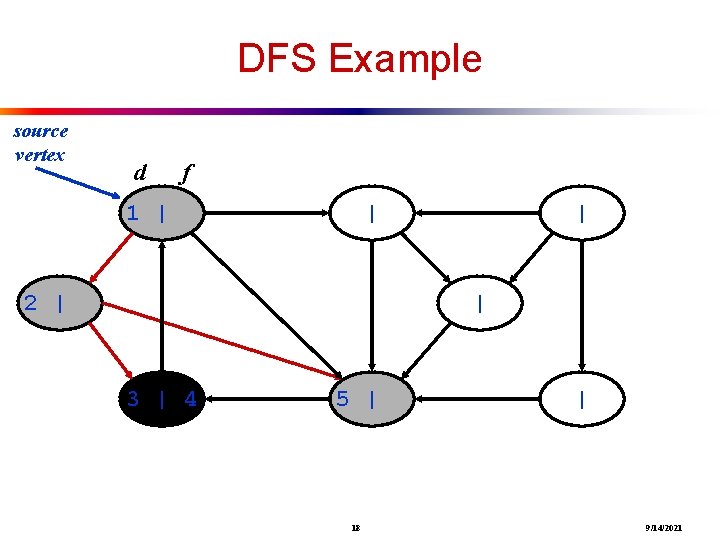
DFS Example source vertex d f 1 | | 2 | | | 3 | 4 5 | 18 | 9/14/2021
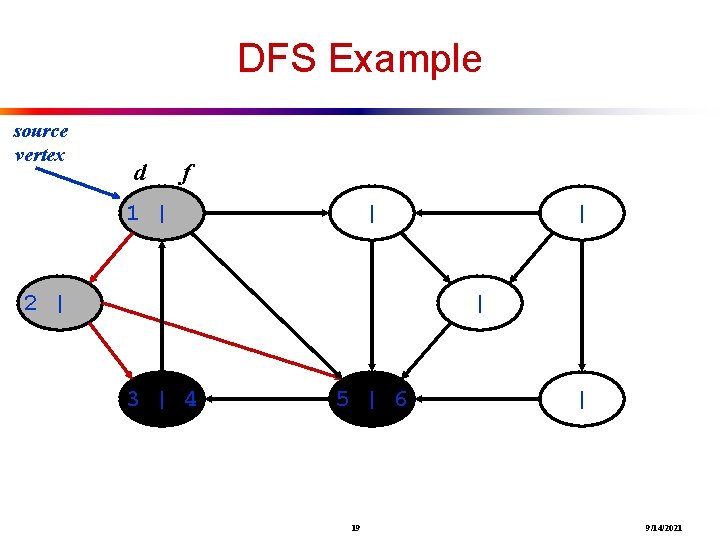
DFS Example source vertex d f 1 | | 2 | | | 3 | 4 5 | 6 19 | 9/14/2021
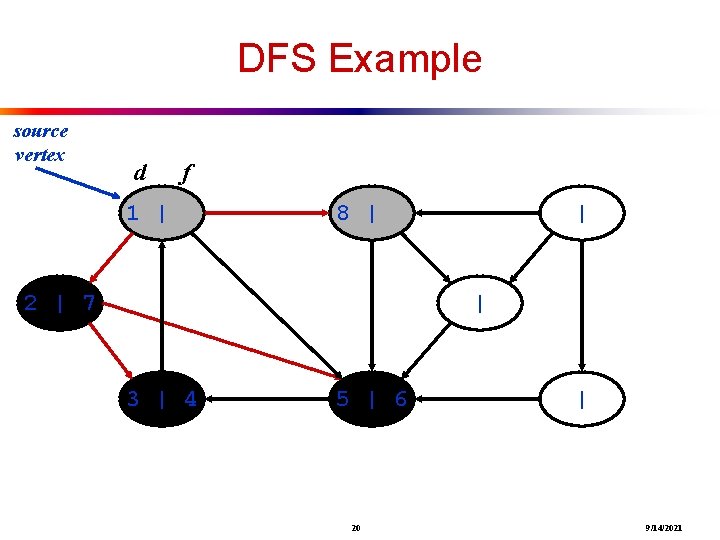
DFS Example source vertex d f 1 | 8 | 2 | 7 | | 3 | 4 5 | 6 20 | 9/14/2021
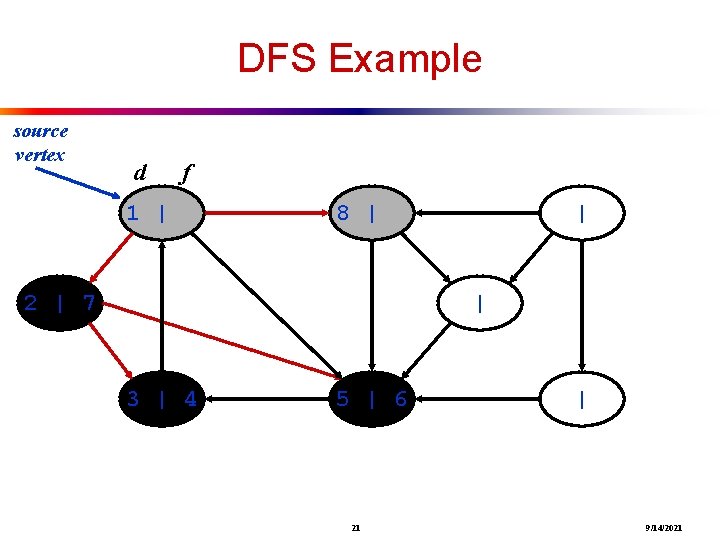
DFS Example source vertex d f 1 | 8 | 2 | 7 | | 3 | 4 5 | 6 21 | 9/14/2021
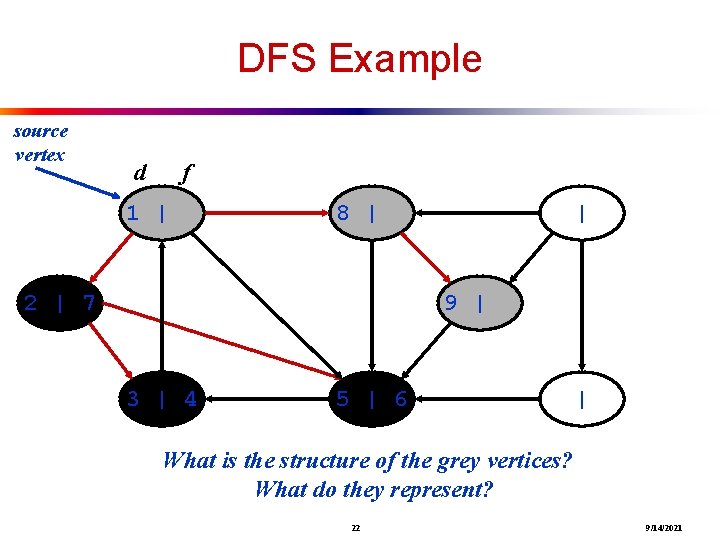
DFS Example source vertex d f 1 | 8 | 2 | 7 | 9 | 3 | 4 5 | 6 | What is the structure of the grey vertices? What do they represent? 22 9/14/2021
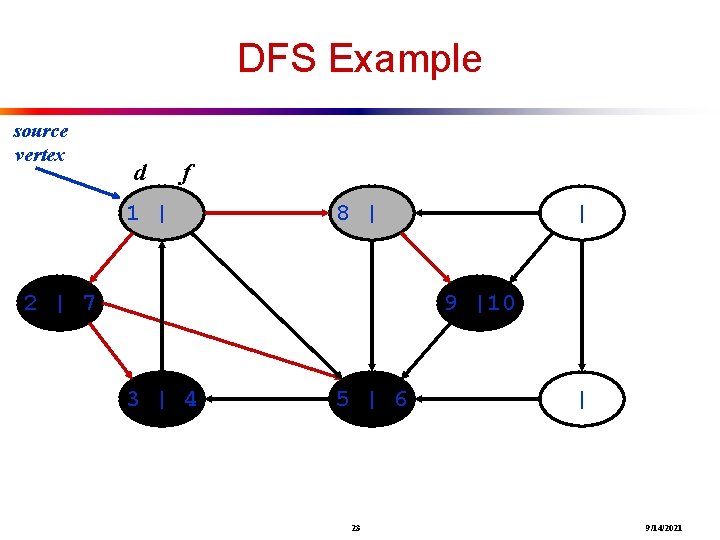
DFS Example source vertex d f 1 | 8 | 2 | 7 | 9 |10 3 | 4 5 | 6 23 | 9/14/2021
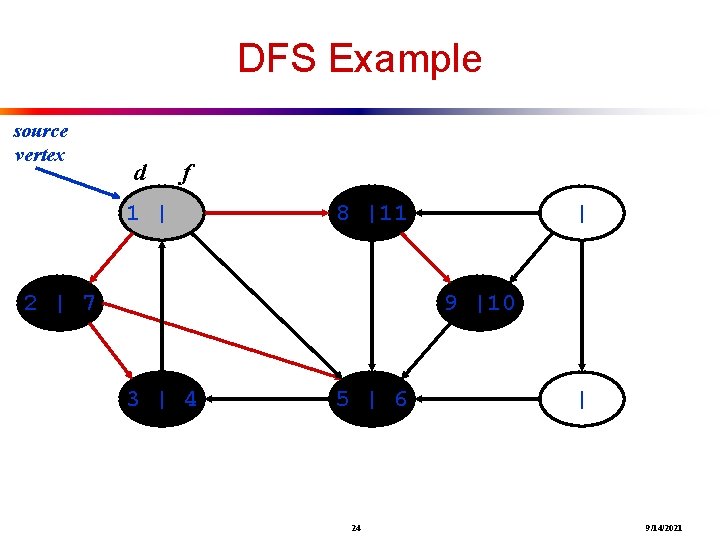
DFS Example source vertex d f 1 | 8 |11 2 | 7 | 9 |10 3 | 4 5 | 6 24 | 9/14/2021
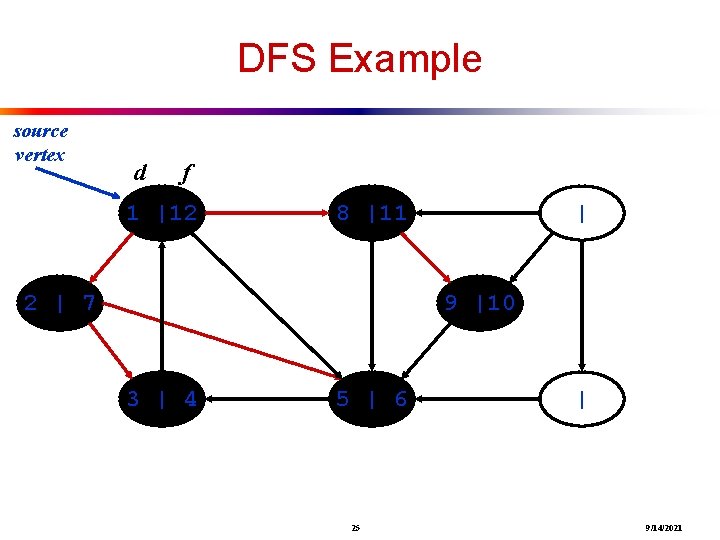
DFS Example source vertex d f 1 |12 8 |11 2 | 7 | 9 |10 3 | 4 5 | 6 25 | 9/14/2021
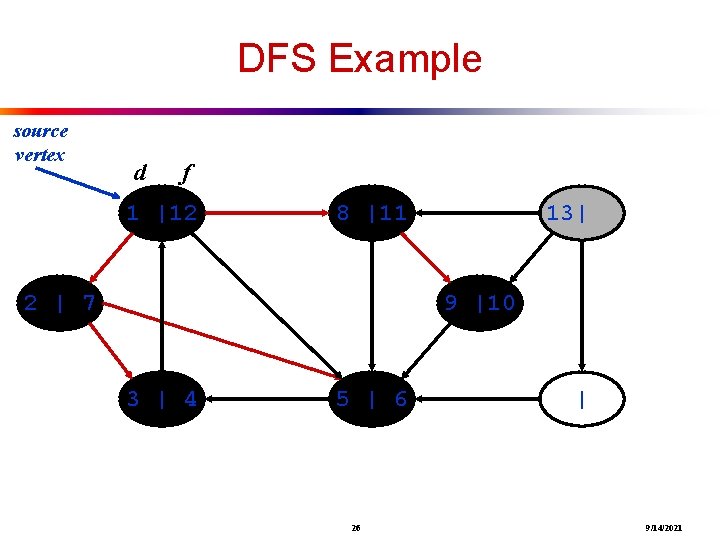
DFS Example source vertex d f 1 |12 8 |11 2 | 7 13| 9 |10 3 | 4 5 | 6 26 | 9/14/2021
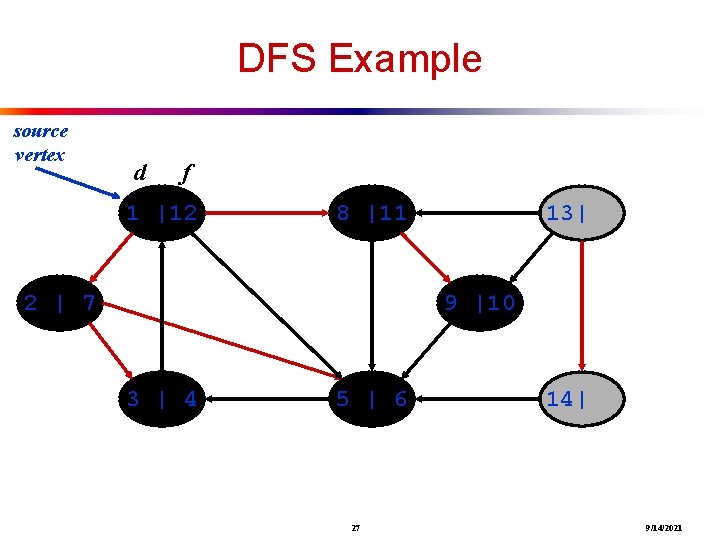
DFS Example source vertex d f 1 |12 8 |11 2 | 7 13| 9 |10 3 | 4 5 | 6 27 14| 9/14/2021
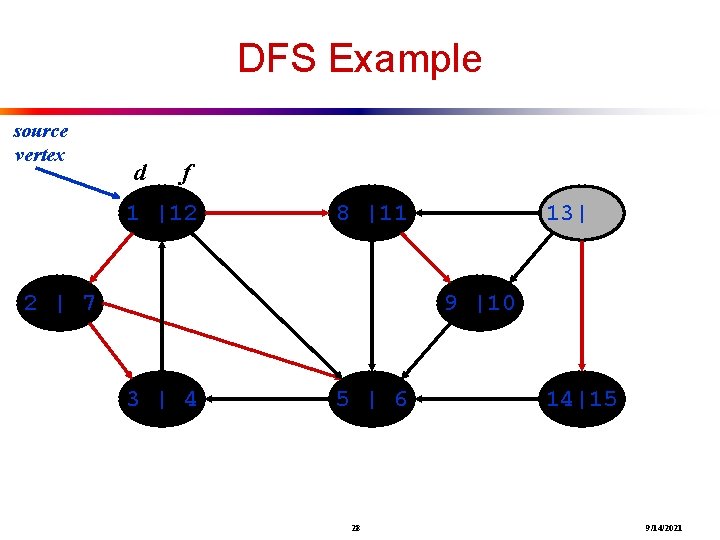
DFS Example source vertex d f 1 |12 8 |11 2 | 7 13| 9 |10 3 | 4 5 | 6 28 14|15 9/14/2021
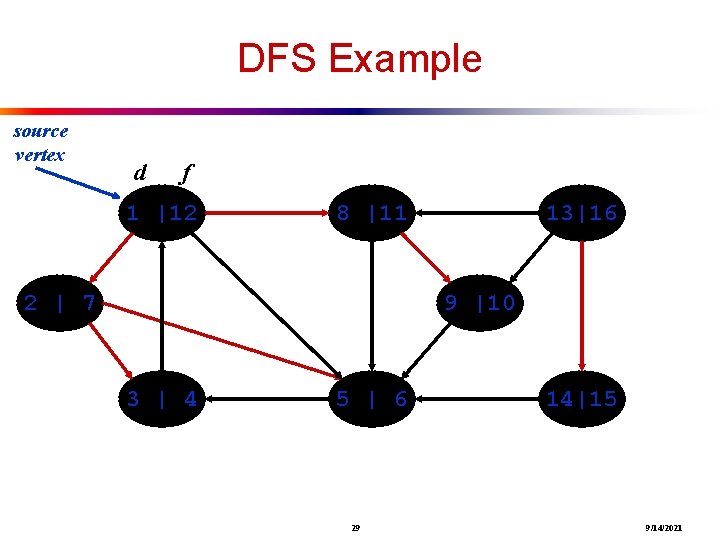
DFS Example source vertex d f 1 |12 8 |11 2 | 7 13|16 9 |10 3 | 4 5 | 6 29 14|15 9/14/2021
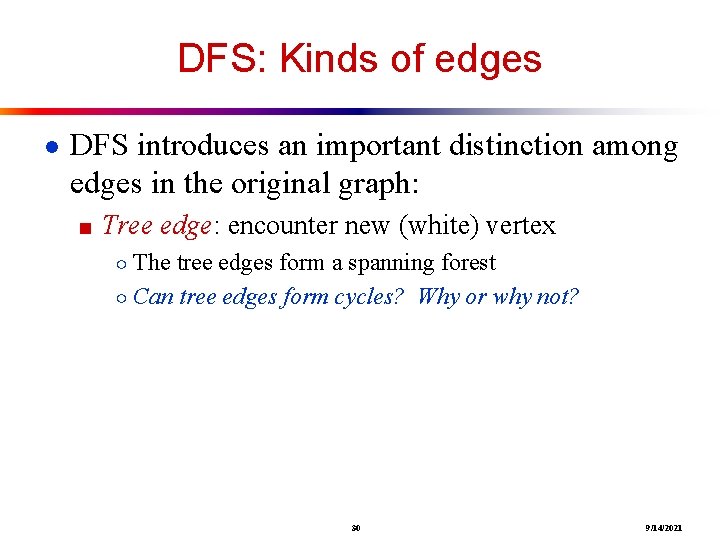
DFS: Kinds of edges ● DFS introduces an important distinction among edges in the original graph: ■ Tree edge: encounter new (white) vertex ○ The tree edges form a spanning forest ○ Can tree edges form cycles? Why or why not? 30 9/14/2021
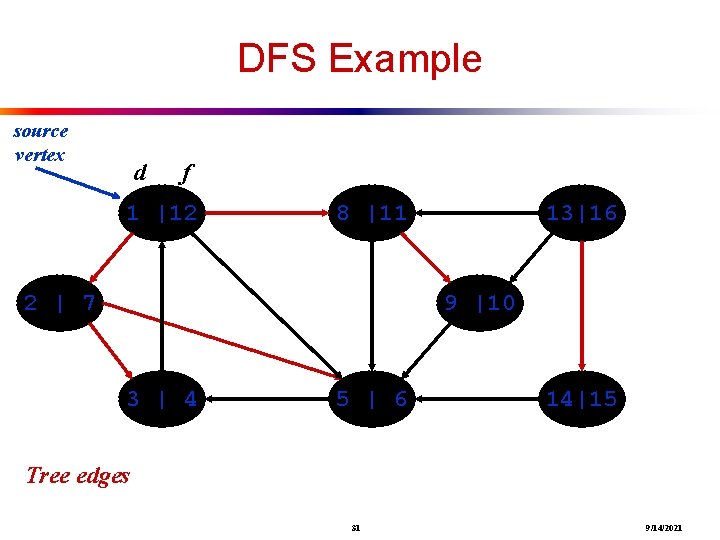
DFS Example source vertex d f 1 |12 8 |11 2 | 7 13|16 9 |10 3 | 4 5 | 6 14|15 Tree edges 31 9/14/2021
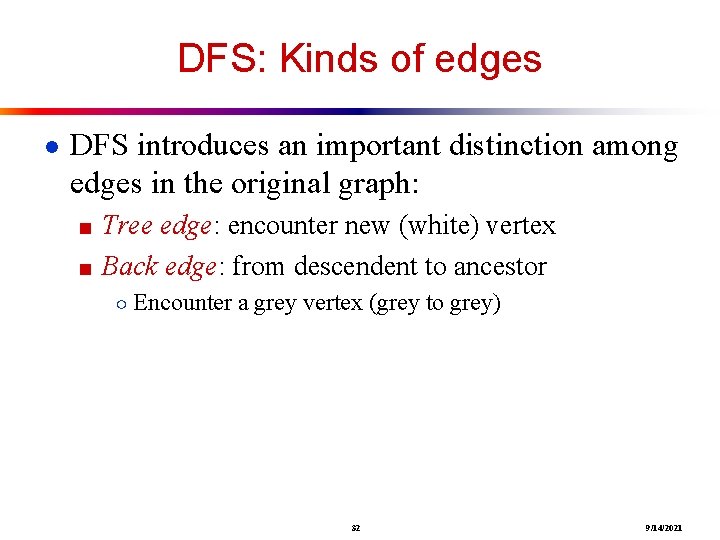
DFS: Kinds of edges ● DFS introduces an important distinction among edges in the original graph: ■ Tree edge: encounter new (white) vertex ■ Back edge: from descendent to ancestor ○ Encounter a grey vertex (grey to grey) 32 9/14/2021
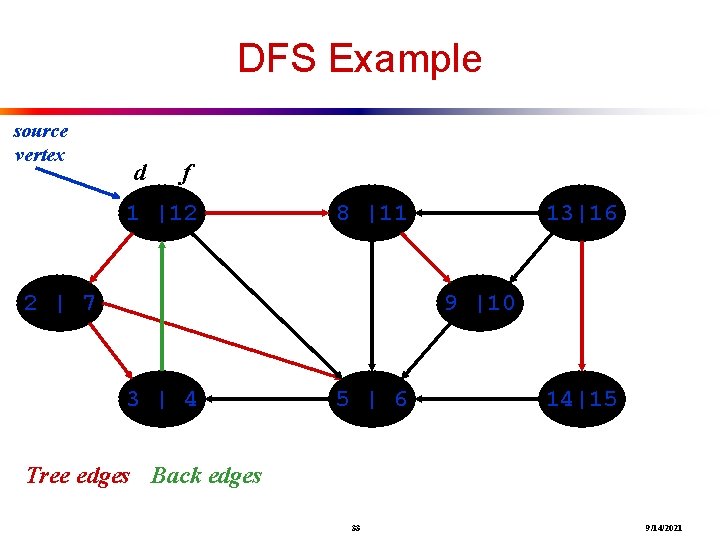
DFS Example source vertex d f 1 |12 8 |11 2 | 7 13|16 9 |10 3 | 4 5 | 6 14|15 Tree edges Back edges 33 9/14/2021
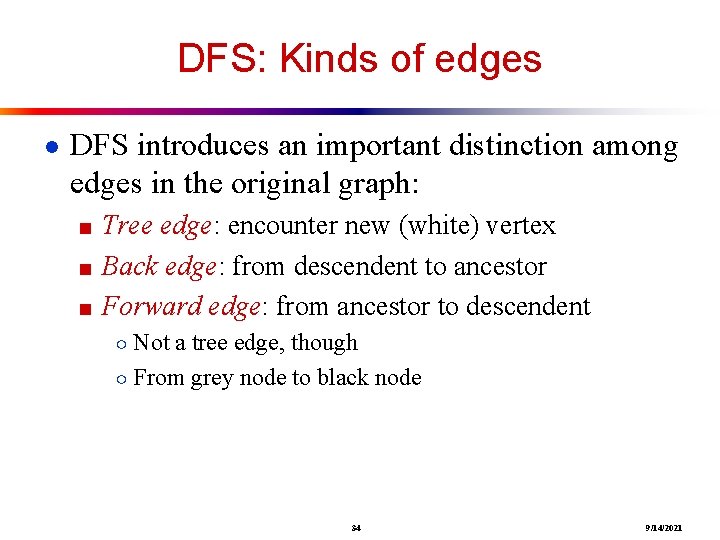
DFS: Kinds of edges ● DFS introduces an important distinction among edges in the original graph: ■ Tree edge: encounter new (white) vertex ■ Back edge: from descendent to ancestor ■ Forward edge: from ancestor to descendent ○ Not a tree edge, though ○ From grey node to black node 34 9/14/2021
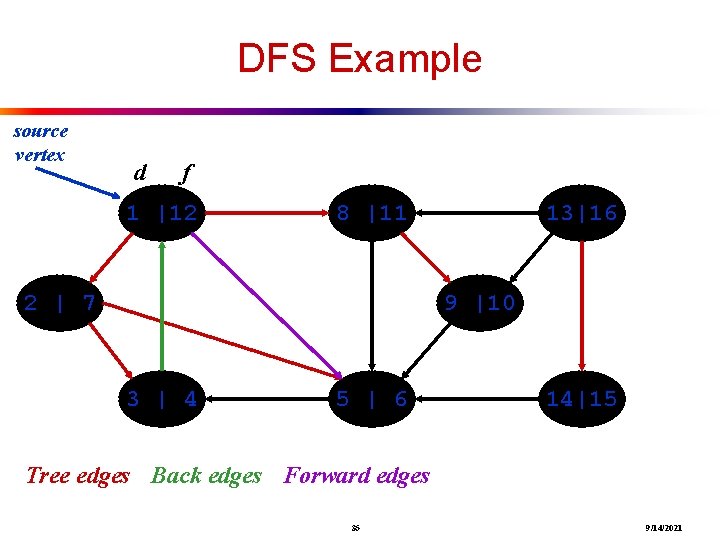
DFS Example source vertex d f 1 |12 8 |11 2 | 7 13|16 9 |10 3 | 4 5 | 6 14|15 Tree edges Back edges Forward edges 35 9/14/2021
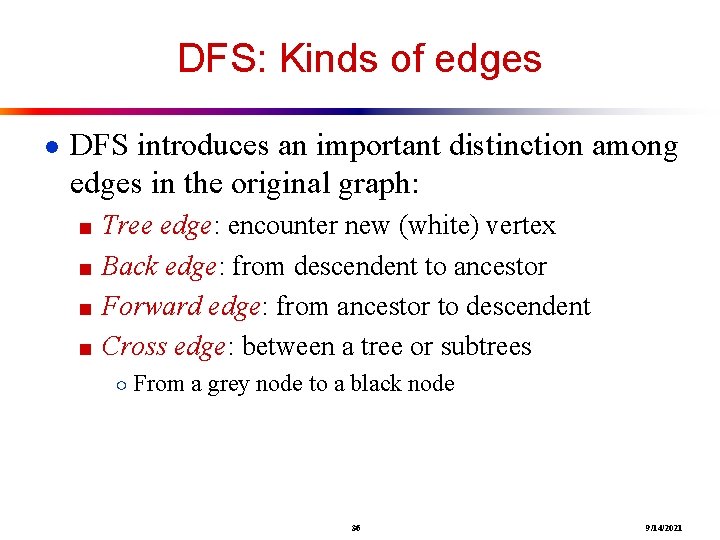
DFS: Kinds of edges ● DFS introduces an important distinction among edges in the original graph: ■ Tree edge: encounter new (white) vertex ■ Back edge: from descendent to ancestor ■ Forward edge: from ancestor to descendent ■ Cross edge: between a tree or subtrees ○ From a grey node to a black node 36 9/14/2021
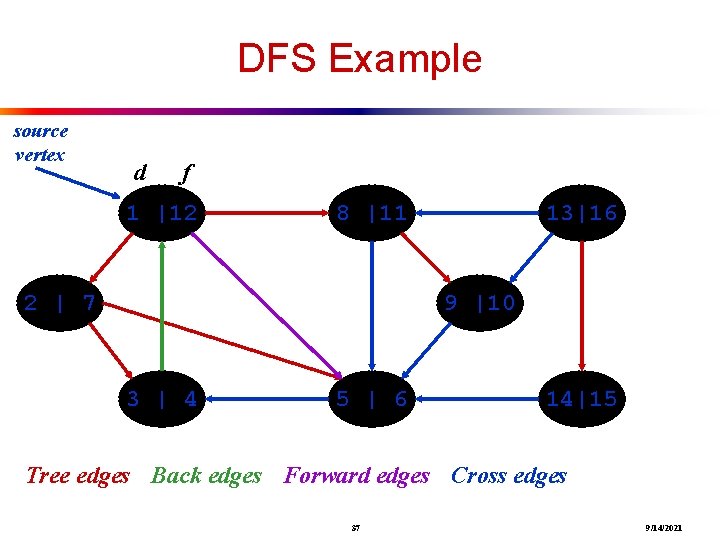
DFS Example source vertex d f 1 |12 8 |11 2 | 7 13|16 9 |10 3 | 4 5 | 6 14|15 Tree edges Back edges Forward edges Cross edges 37 9/14/2021
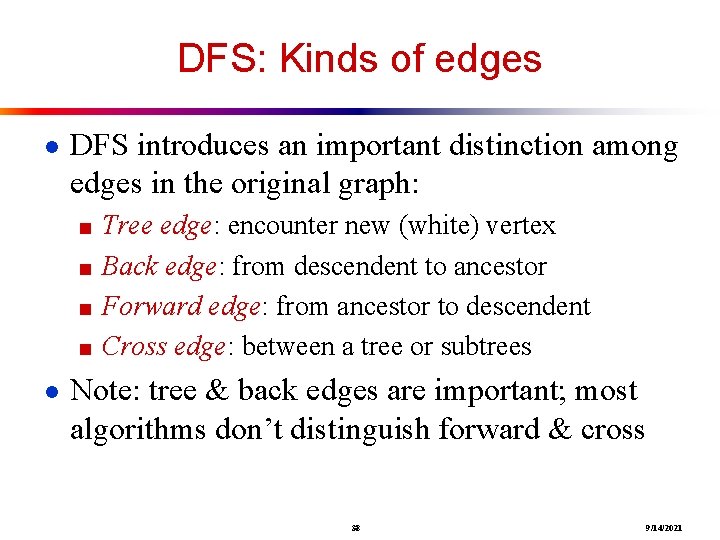
DFS: Kinds of edges ● DFS introduces an important distinction among edges in the original graph: ■ Tree edge: encounter new (white) vertex ■ Back edge: from descendent to ancestor ■ Forward edge: from ancestor to descendent ■ Cross edge: between a tree or subtrees ● Note: tree & back edges are important; most algorithms don’t distinguish forward & cross 38 9/14/2021
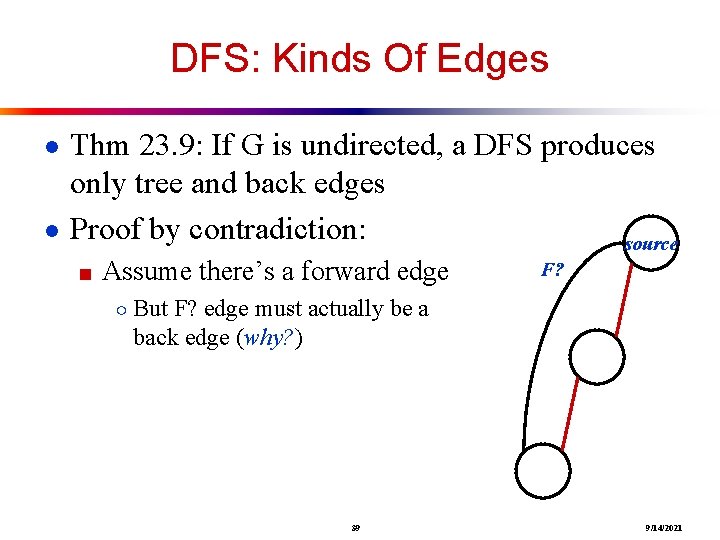
DFS: Kinds Of Edges ● Thm 23. 9: If G is undirected, a DFS produces only tree and back edges ● Proof by contradiction: ■ Assume there’s a forward edge ○ But F? edge must actually be a back edge (why? ) 39 source F? 9/14/2021
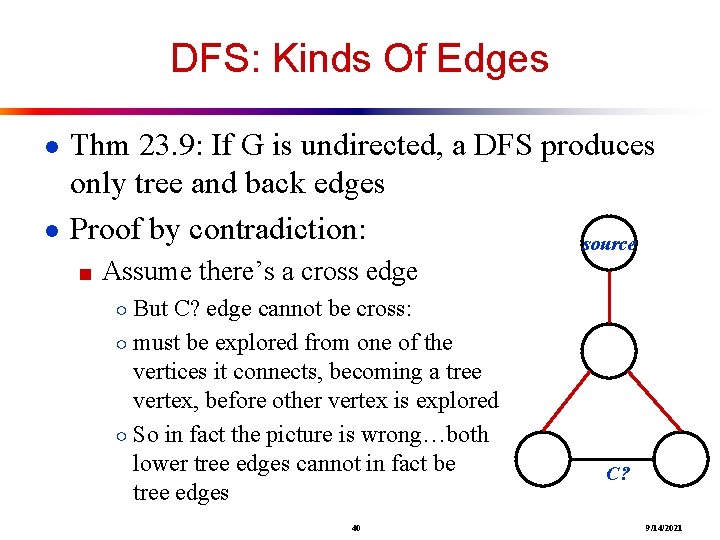
DFS: Kinds Of Edges ● Thm 23. 9: If G is undirected, a DFS produces only tree and back edges ● Proof by contradiction: ■ Assume there’s a cross edge ○ But C? edge cannot be cross: ○ must be explored from one of the vertices it connects, becoming a tree vertex, before other vertex is explored ○ So in fact the picture is wrong…both lower tree edges cannot in fact be tree edges 40 source C? 9/14/2021
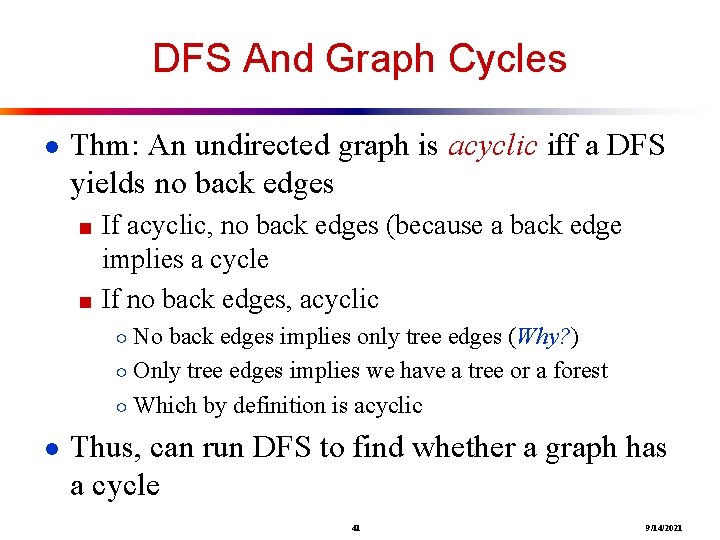
DFS And Graph Cycles ● Thm: An undirected graph is acyclic iff a DFS yields no back edges ■ If acyclic, no back edges (because a back edge implies a cycle ■ If no back edges, acyclic ○ No back edges implies only tree edges (Why? ) ○ Only tree edges implies we have a tree or a forest ○ Which by definition is acyclic ● Thus, can run DFS to find whether a graph has a cycle 41 9/14/2021
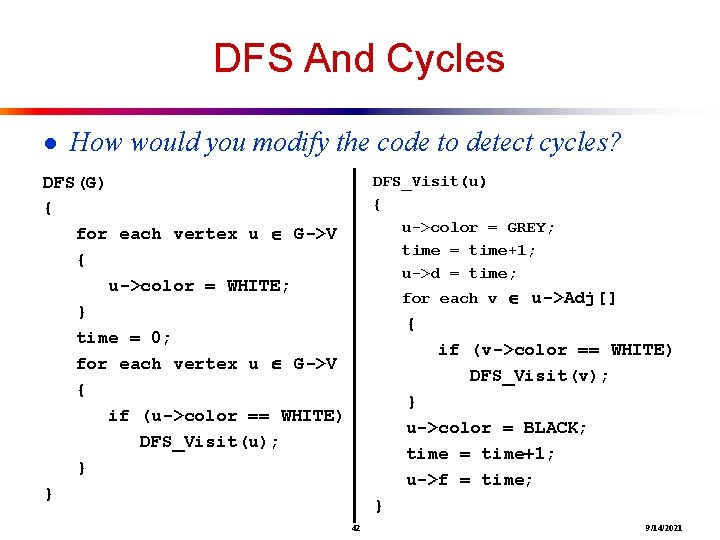
DFS And Cycles ● How would you modify the code to detect cycles? DFS_Visit(u) { u->color = GREY; time = time+1; u->d = time; DFS(G) { for each vertex u G->V { u->color = WHITE; } time = 0; for each vertex u G->V { if (u->color == WHITE) DFS_Visit(u); } } for each v u->Adj[] { if (v->color == WHITE) DFS_Visit(v); } u->color = BLACK; time = time+1; u->f = time; } 42 9/14/2021
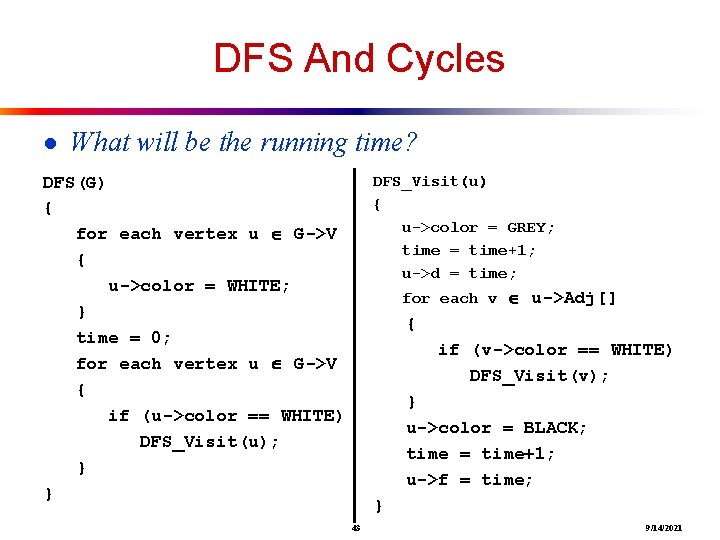
DFS And Cycles ● What will be the running time? DFS_Visit(u) { u->color = GREY; time = time+1; u->d = time; DFS(G) { for each vertex u G->V { u->color = WHITE; } time = 0; for each vertex u G->V { if (u->color == WHITE) DFS_Visit(u); } } for each v u->Adj[] { if (v->color == WHITE) DFS_Visit(v); } u->color = BLACK; time = time+1; u->f = time; } 43 9/14/2021
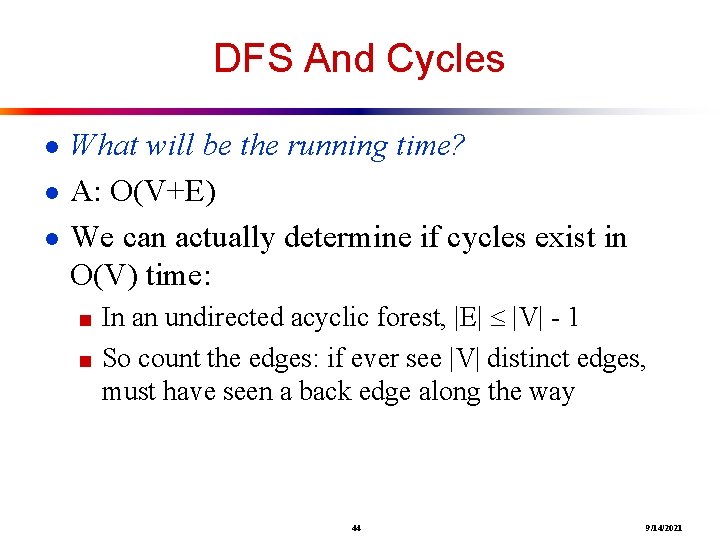
DFS And Cycles ● What will be the running time? ● A: O(V+E) ● We can actually determine if cycles exist in O(V) time: ■ In an undirected acyclic forest, |E| |V| - 1 ■ So count the edges: if ever see |V| distinct edges, must have seen a back edge along the way 44 9/14/2021
Itcs 6114
Itcs 6114
Depthfirst search
Ecu itcs
Liceo scientifico primo levi bollate
Primo levi the grey zone
Difference between informed and uninformed search
Search by image
Binary search in design and analysis of algorithms
Advanced search algorithms
Elementary graph
Parallelizing sequential graph computations
W graph
Undirected graph algorithms
Federated search vs discovery
èinterest
Federated search vs distributed search
What is informed search and uninformed search
Httpstw
Best first search is a type of informed search which uses
Blind search dan heuristic search
Video.search.yahoo.com search video
Videos yahoo search
Benefits of binary search
Linear search vs binary search
Http://tw
Semantic search vs cognitive search
Comparison of uninformed search strategies
1http
Binary search graph
Floor planning concept in vlsi ppt
Search graph
Graph search
Wav2letter
Handshaking theorem
Wait-for graph
Computational thinking algorithms and programming
Algorithms types
Recursive algorithms
1001 design
Safe patient handling algorithms
Recursion in java
Types of randomized algorithms
Process mining algorithms
Evolution of logistics ppt