Advanced Java Programming CS 537 Data Structures and
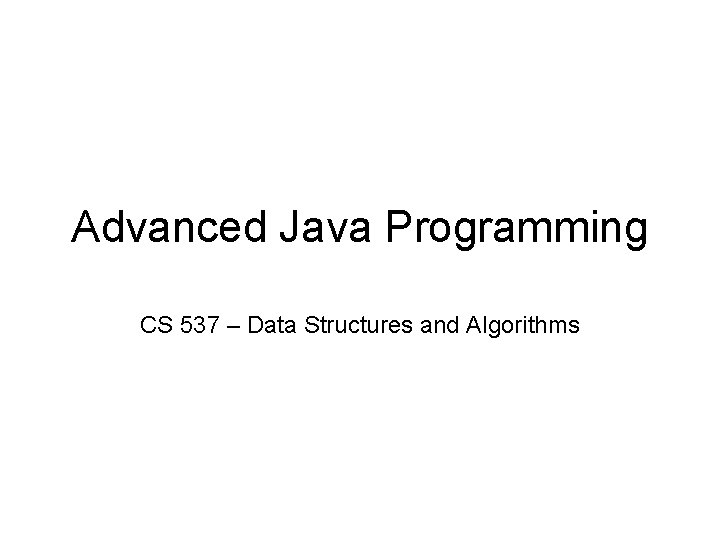
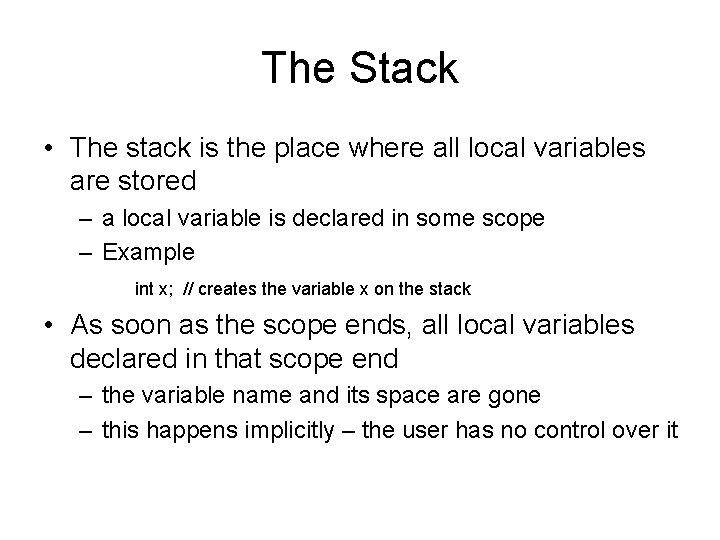
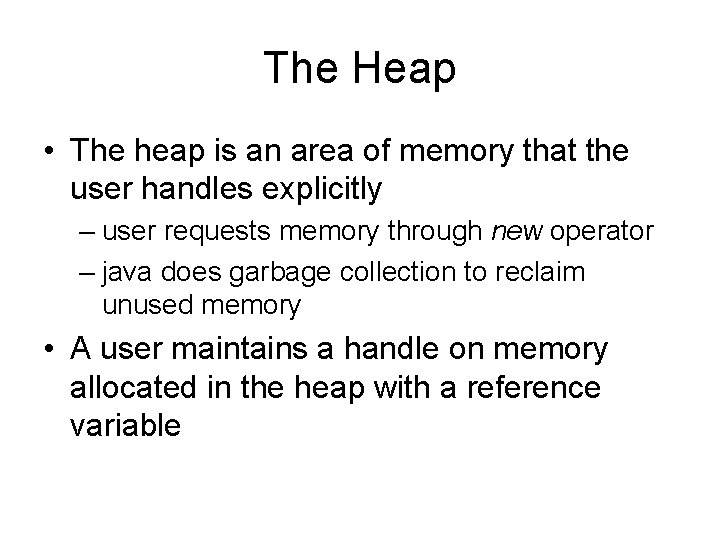
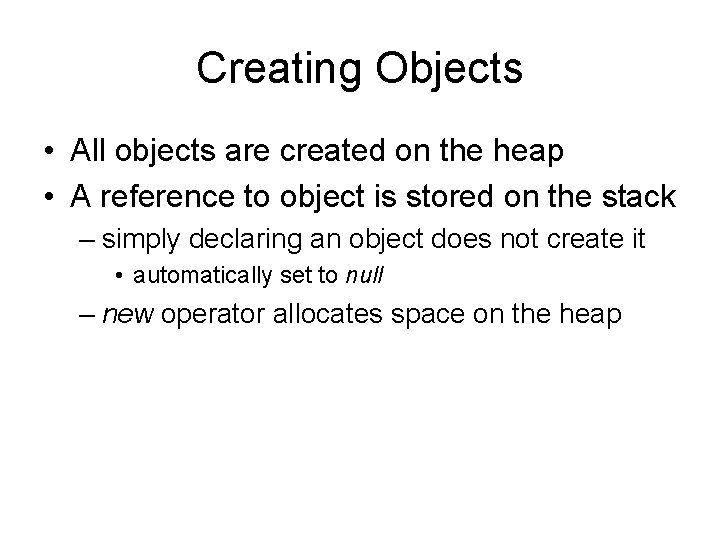
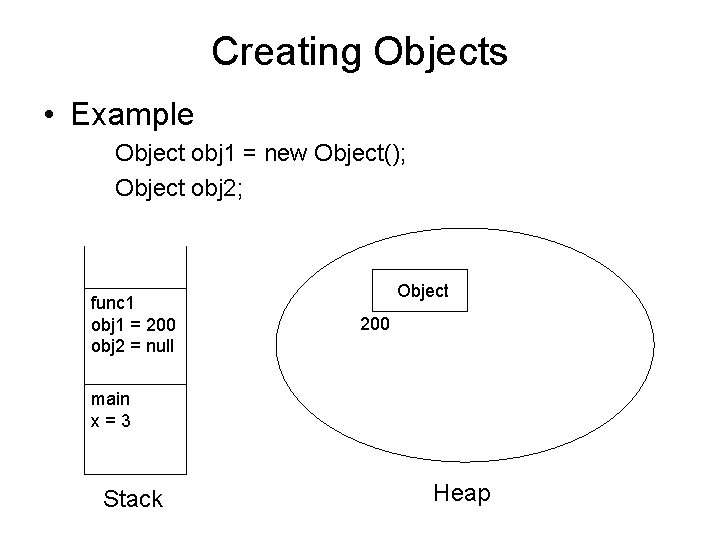
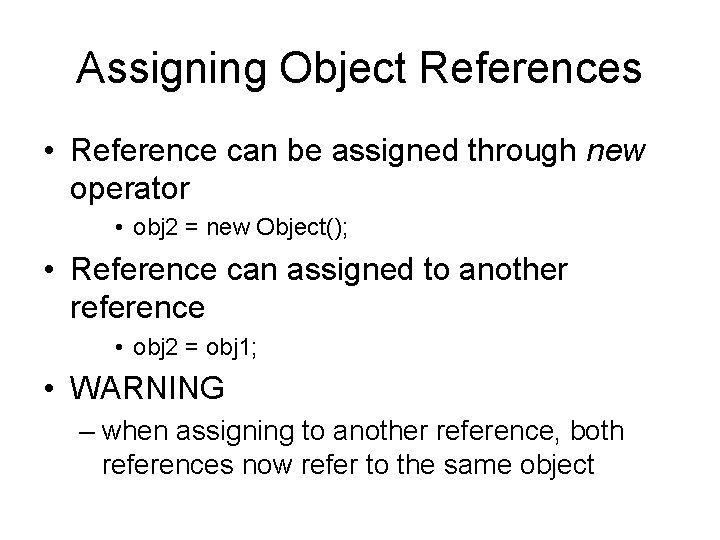
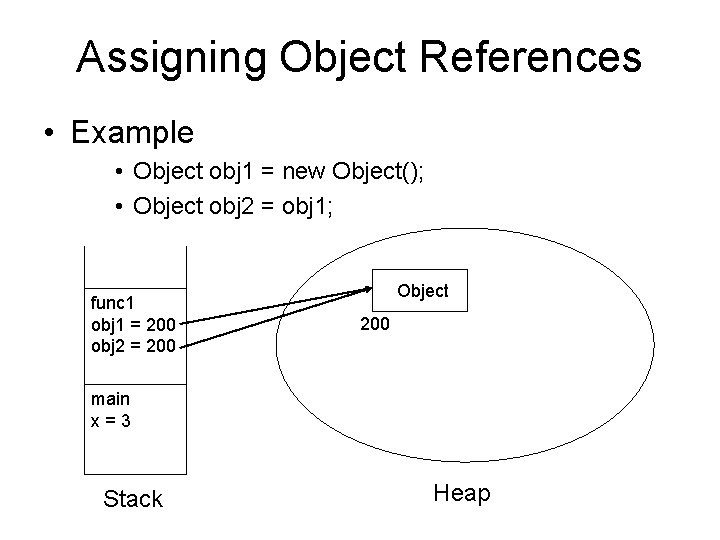
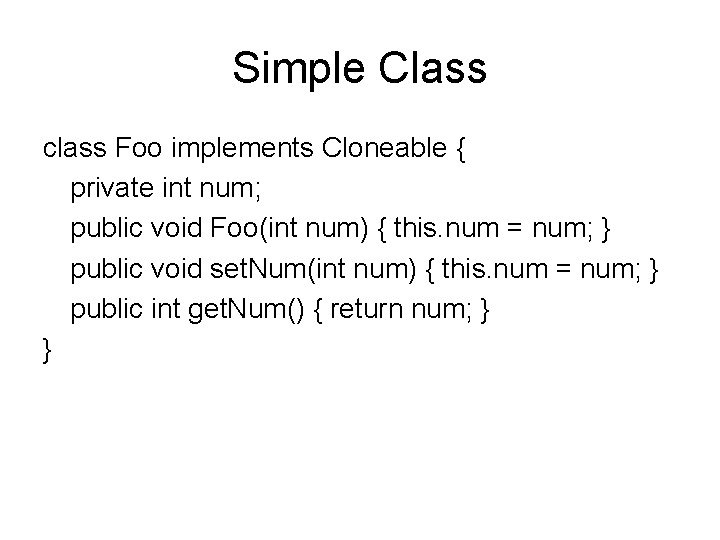
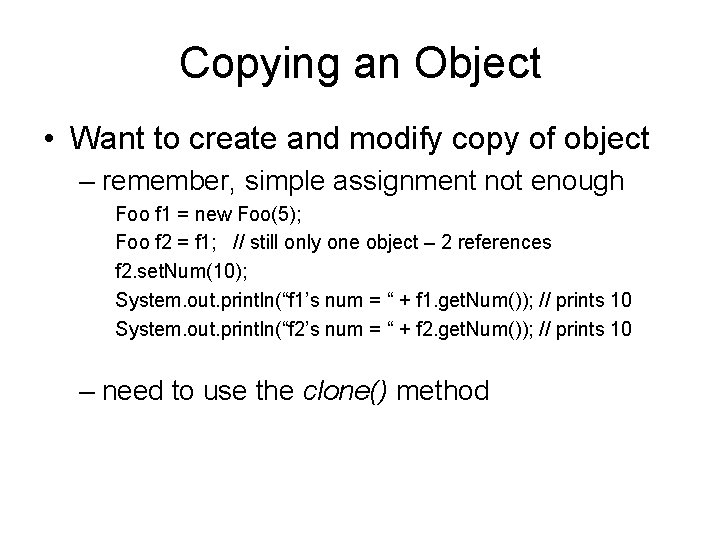
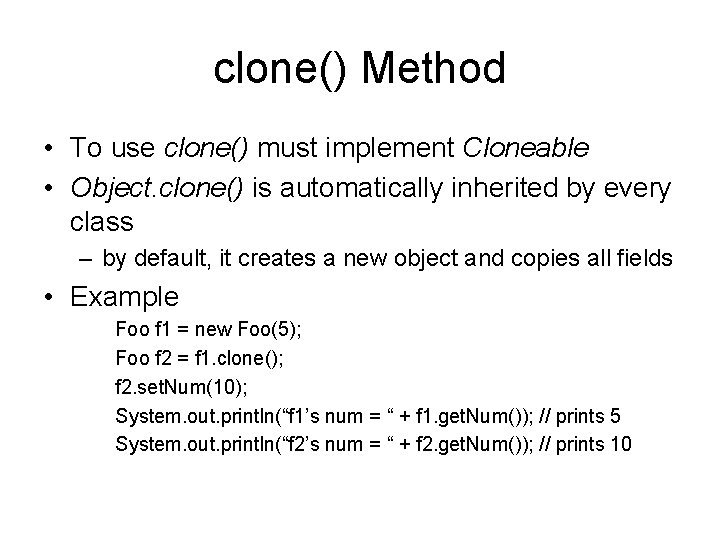
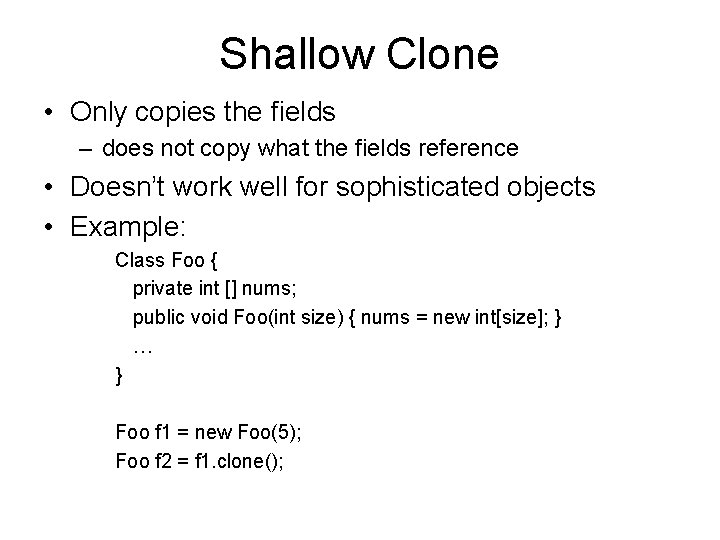
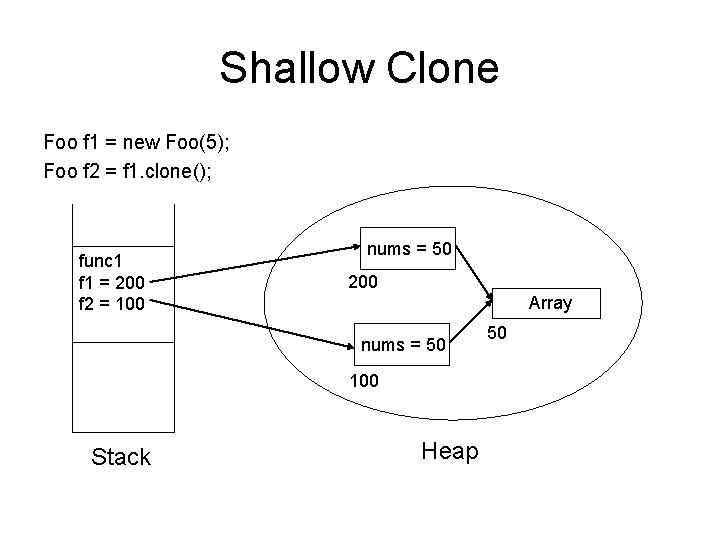
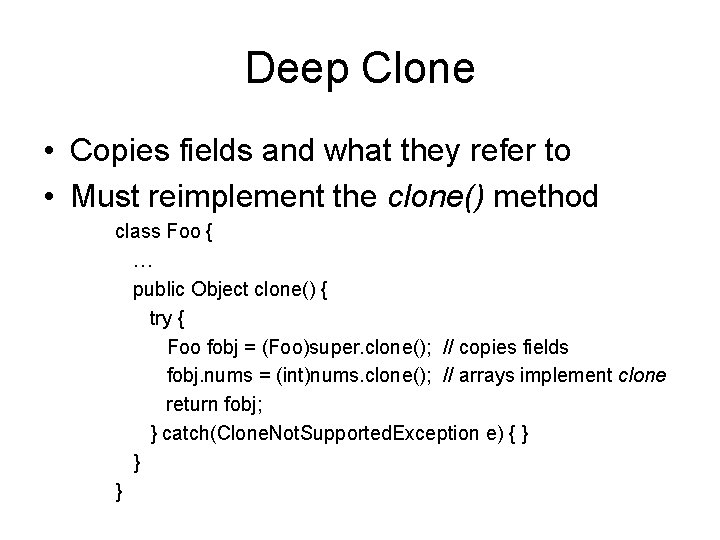
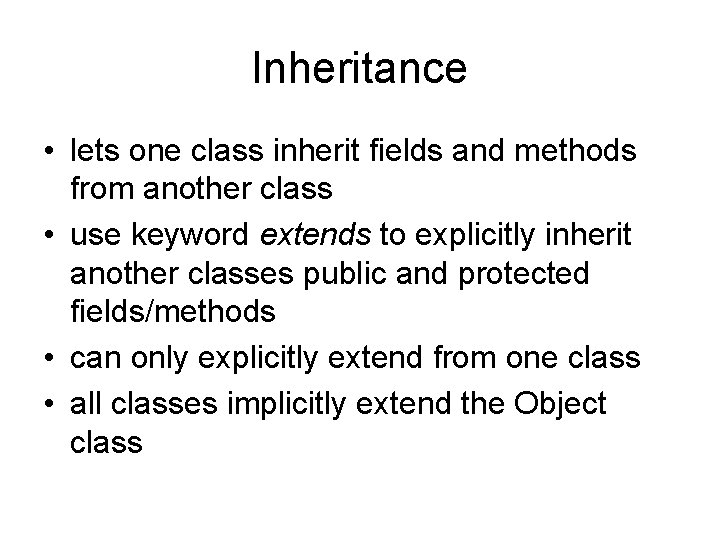
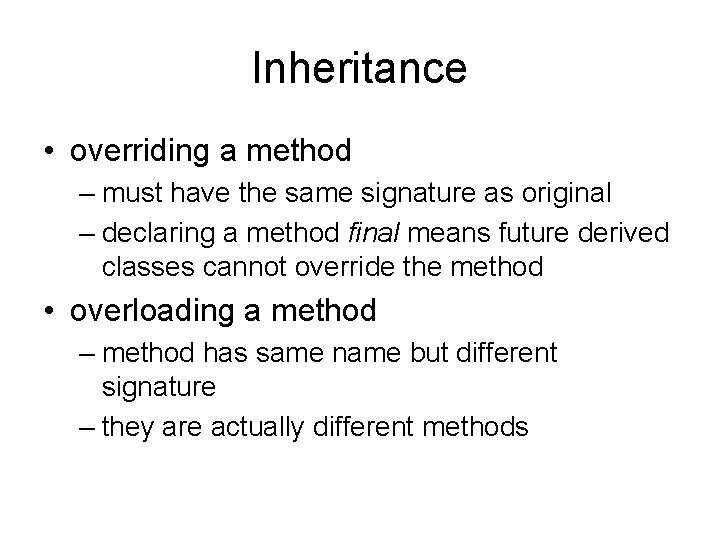
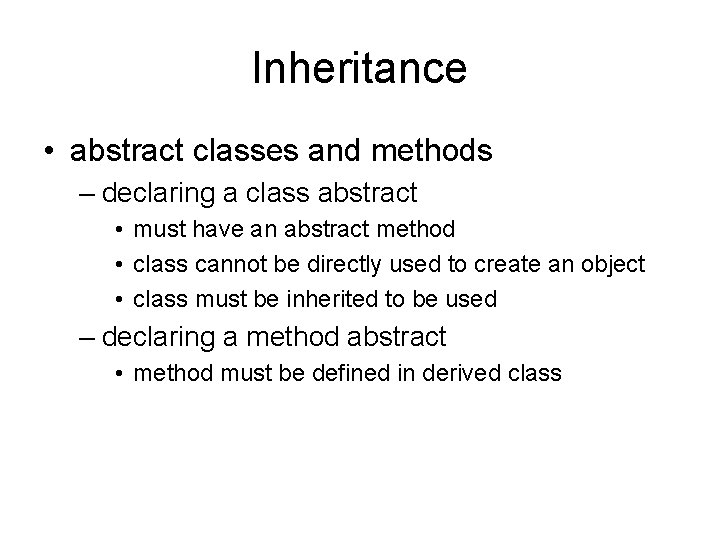
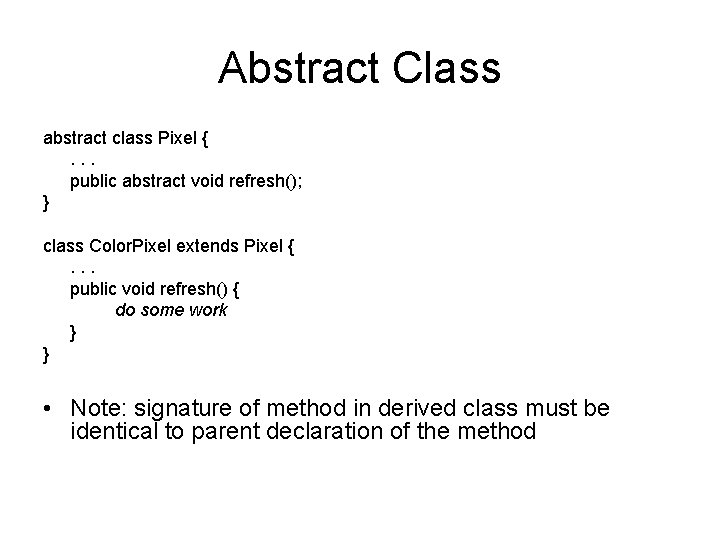
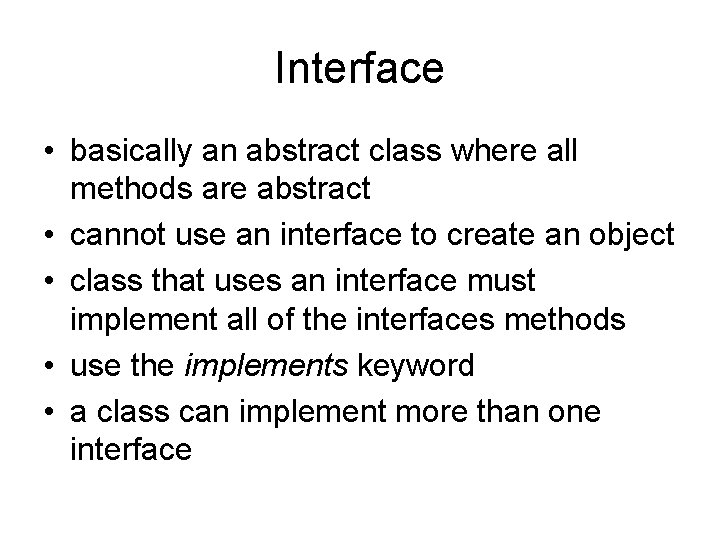
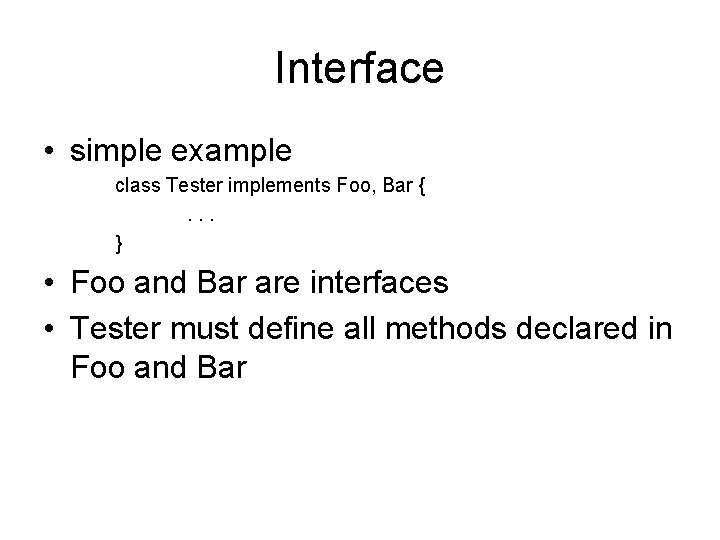
![Array Review • • • Consecutive blocks of memory Creation: int [] grades = Array Review • • • Consecutive blocks of memory Creation: int [] grades =](https://slidetodoc.com/presentation_image/3b17d656250058ef7f6de5a3bfd78f5a/image-20.jpg)
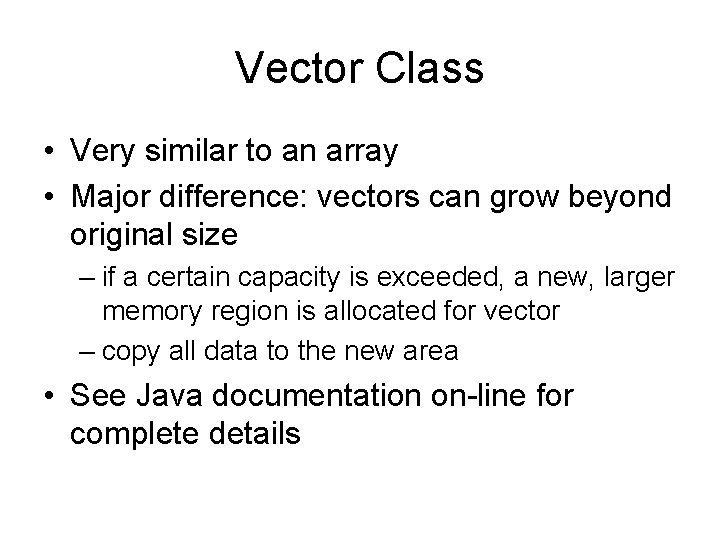
- Slides: 21
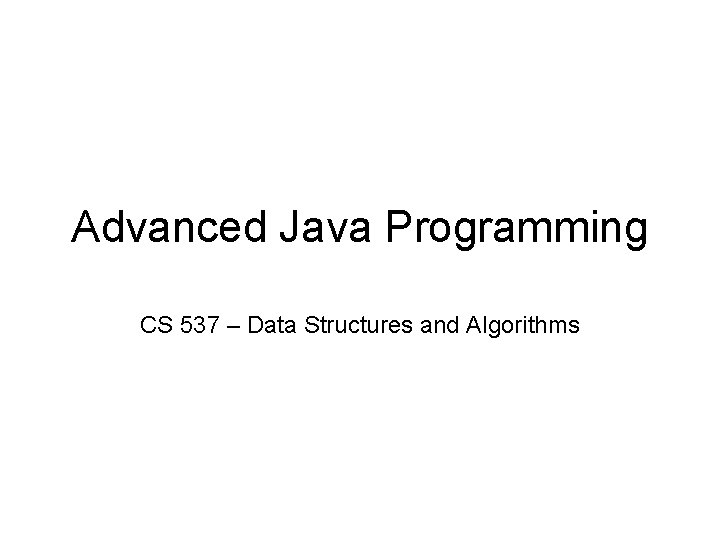
Advanced Java Programming CS 537 – Data Structures and Algorithms
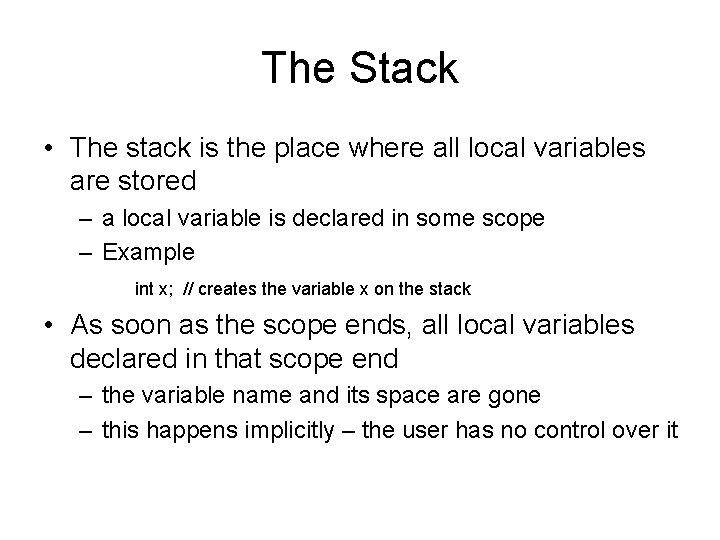
The Stack • The stack is the place where all local variables are stored – a local variable is declared in some scope – Example int x; // creates the variable x on the stack • As soon as the scope ends, all local variables declared in that scope end – the variable name and its space are gone – this happens implicitly – the user has no control over it
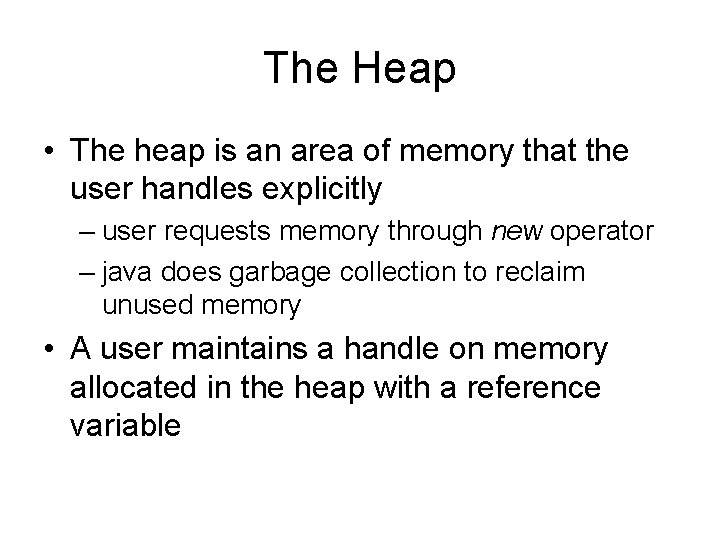
The Heap • The heap is an area of memory that the user handles explicitly – user requests memory through new operator – java does garbage collection to reclaim unused memory • A user maintains a handle on memory allocated in the heap with a reference variable
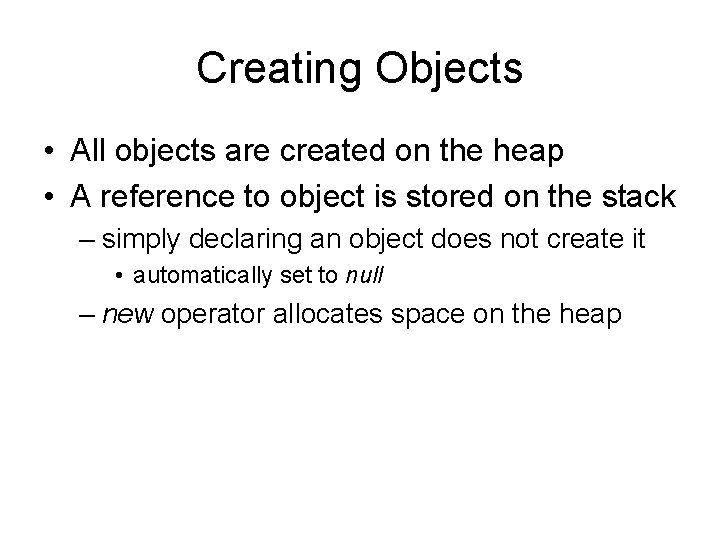
Creating Objects • All objects are created on the heap • A reference to object is stored on the stack – simply declaring an object does not create it • automatically set to null – new operator allocates space on the heap
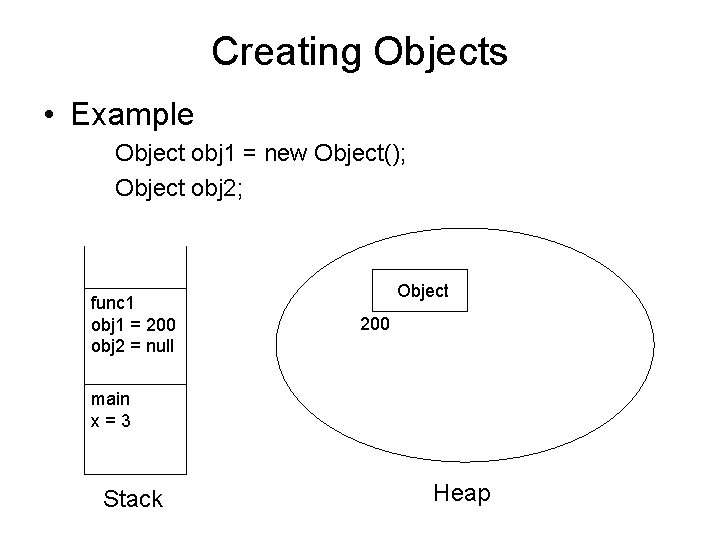
Creating Objects • Example Object obj 1 = new Object(); Object obj 2; func 1 obj 1 = 200 obj 2 = null Object 200 main x=3 Stack Heap
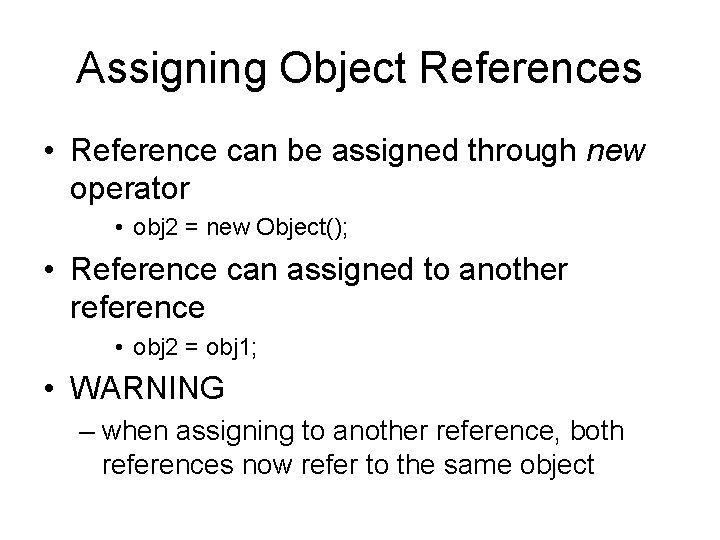
Assigning Object References • Reference can be assigned through new operator • obj 2 = new Object(); • Reference can assigned to another reference • obj 2 = obj 1; • WARNING – when assigning to another reference, both references now refer to the same object
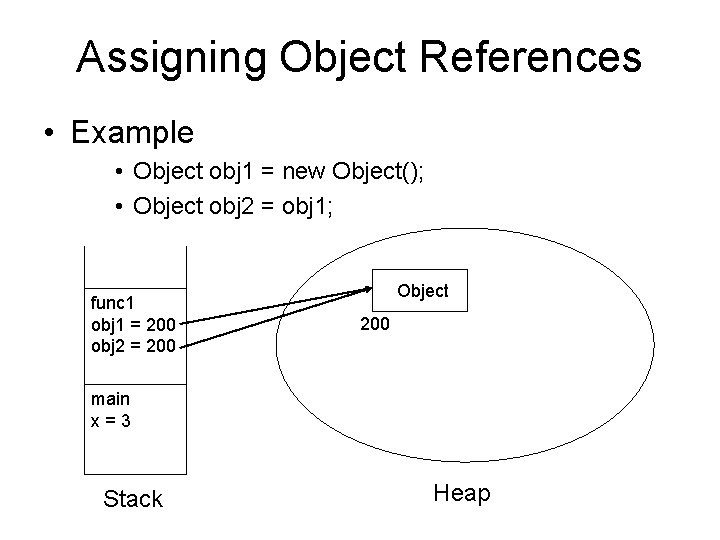
Assigning Object References • Example • Object obj 1 = new Object(); • Object obj 2 = obj 1; func 1 obj 1 = 200 obj 2 = 200 Object 200 main x=3 Stack Heap
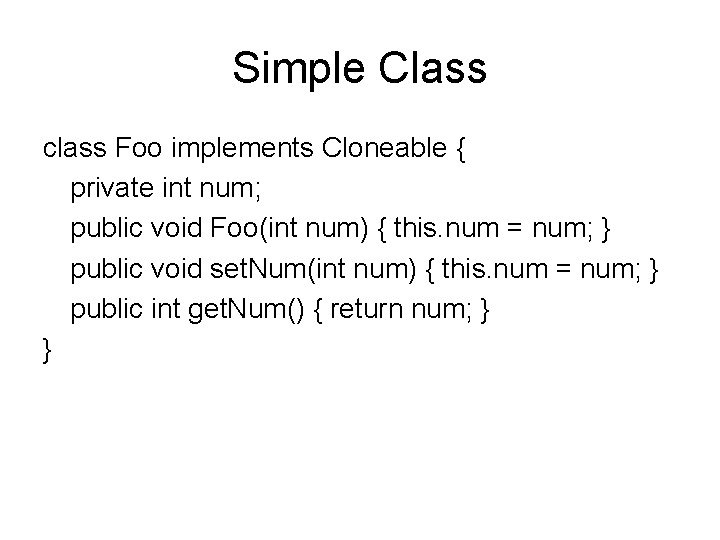
Simple Class class Foo implements Cloneable { private int num; public void Foo(int num) { this. num = num; } public void set. Num(int num) { this. num = num; } public int get. Num() { return num; } }
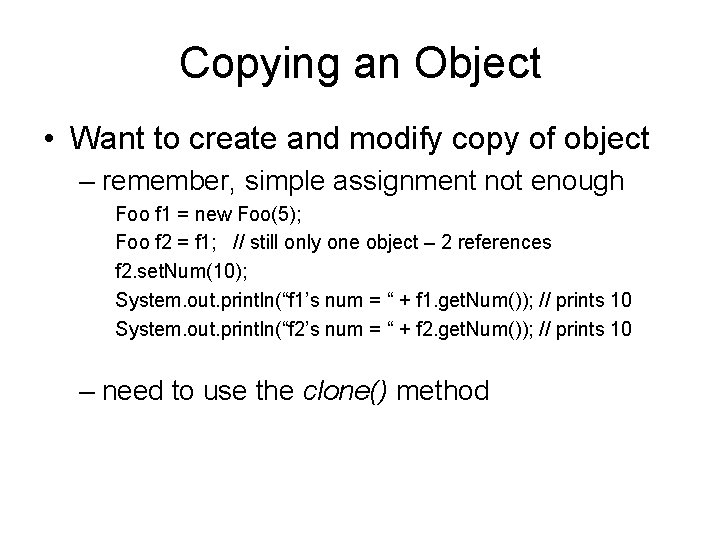
Copying an Object • Want to create and modify copy of object – remember, simple assignment not enough Foo f 1 = new Foo(5); Foo f 2 = f 1; // still only one object – 2 references f 2. set. Num(10); System. out. println(“f 1’s num = “ + f 1. get. Num()); // prints 10 System. out. println(“f 2’s num = “ + f 2. get. Num()); // prints 10 – need to use the clone() method
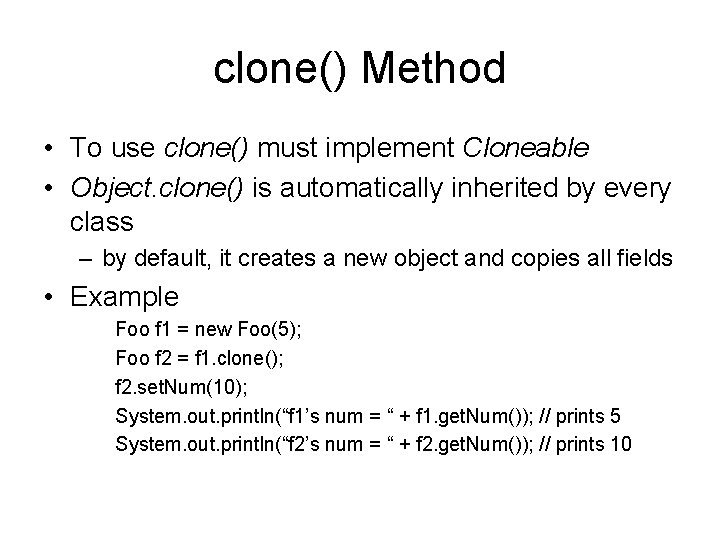
clone() Method • To use clone() must implement Cloneable • Object. clone() is automatically inherited by every class – by default, it creates a new object and copies all fields • Example Foo f 1 = new Foo(5); Foo f 2 = f 1. clone(); f 2. set. Num(10); System. out. println(“f 1’s num = “ + f 1. get. Num()); // prints 5 System. out. println(“f 2’s num = “ + f 2. get. Num()); // prints 10
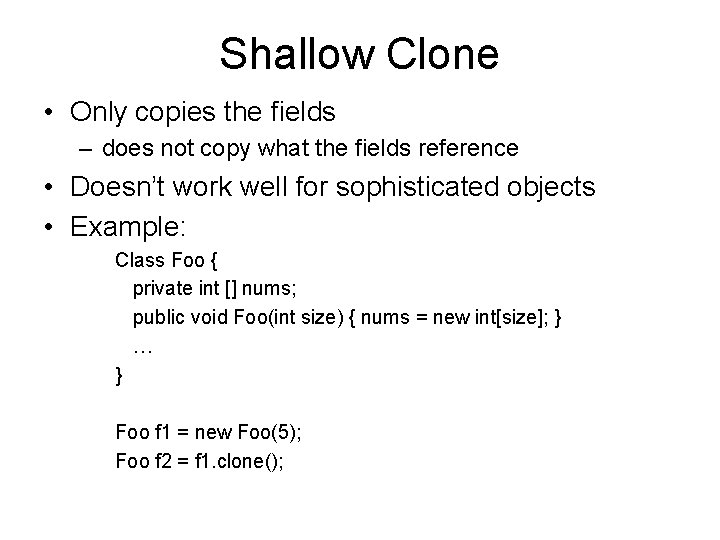
Shallow Clone • Only copies the fields – does not copy what the fields reference • Doesn’t work well for sophisticated objects • Example: Class Foo { private int [] nums; public void Foo(int size) { nums = new int[size]; } … } Foo f 1 = new Foo(5); Foo f 2 = f 1. clone();
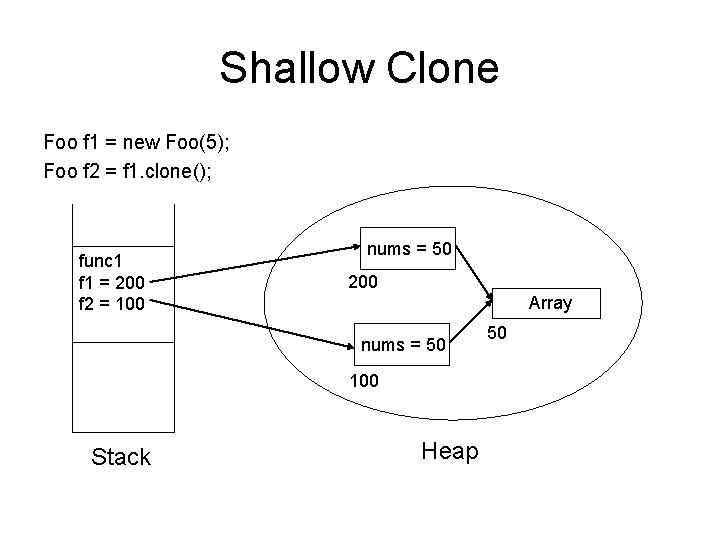
Shallow Clone Foo f 1 = new Foo(5); Foo f 2 = f 1. clone(); func 1 f 1 = 200 f 2 = 100 nums = 50 200 Array nums = 50 100 Stack Heap 50
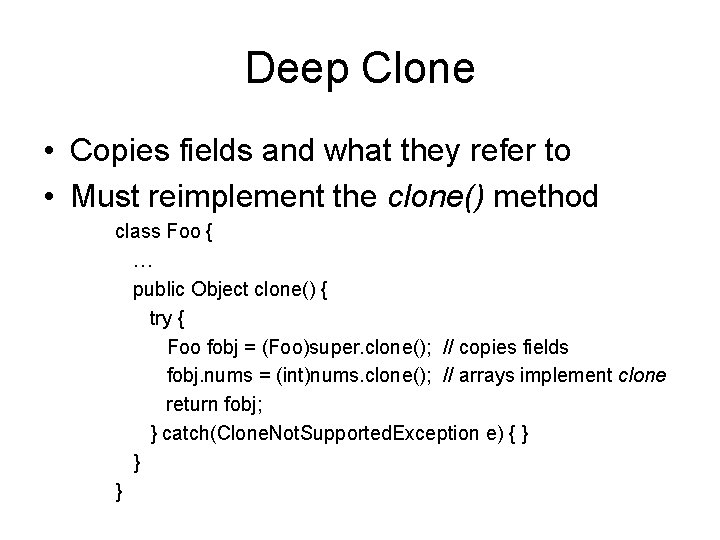
Deep Clone • Copies fields and what they refer to • Must reimplement the clone() method class Foo { … public Object clone() { try { Foo fobj = (Foo)super. clone(); // copies fields fobj. nums = (int)nums. clone(); // arrays implement clone return fobj; } catch(Clone. Not. Supported. Exception e) { } } }
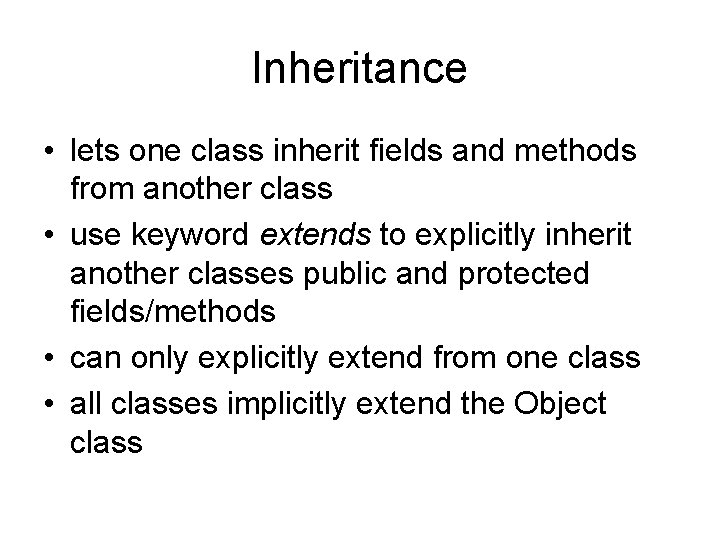
Inheritance • lets one class inherit fields and methods from another class • use keyword extends to explicitly inherit another classes public and protected fields/methods • can only explicitly extend from one class • all classes implicitly extend the Object class
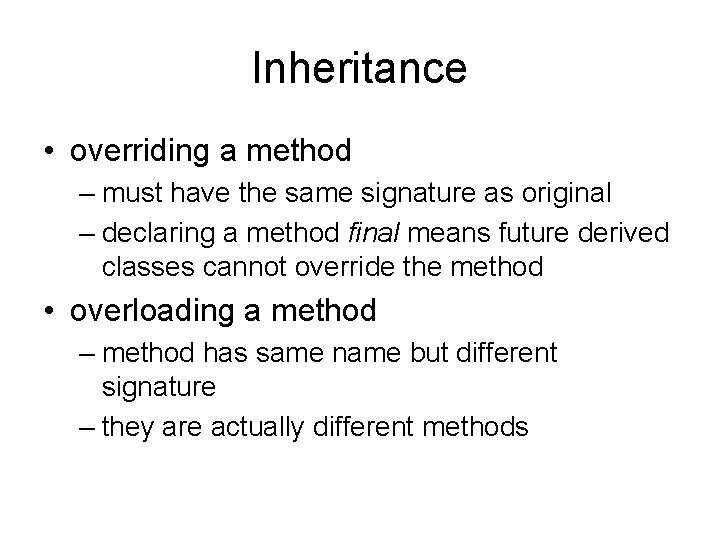
Inheritance • overriding a method – must have the same signature as original – declaring a method final means future derived classes cannot override the method • overloading a method – method has same name but different signature – they are actually different methods
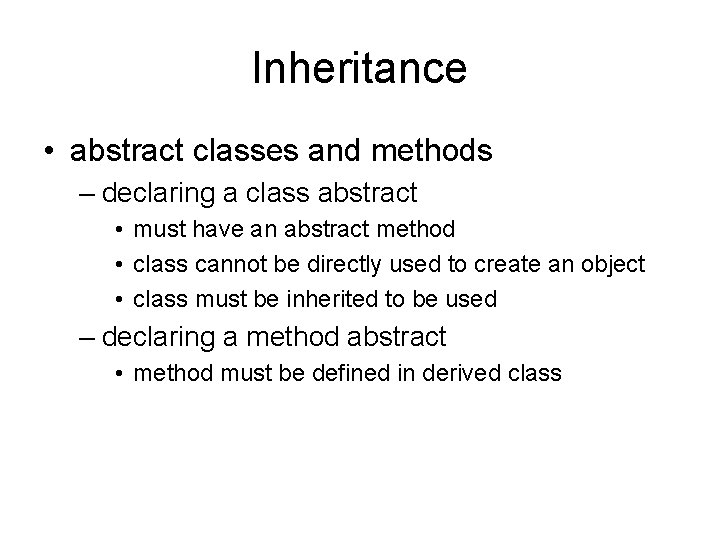
Inheritance • abstract classes and methods – declaring a class abstract • must have an abstract method • class cannot be directly used to create an object • class must be inherited to be used – declaring a method abstract • method must be defined in derived class
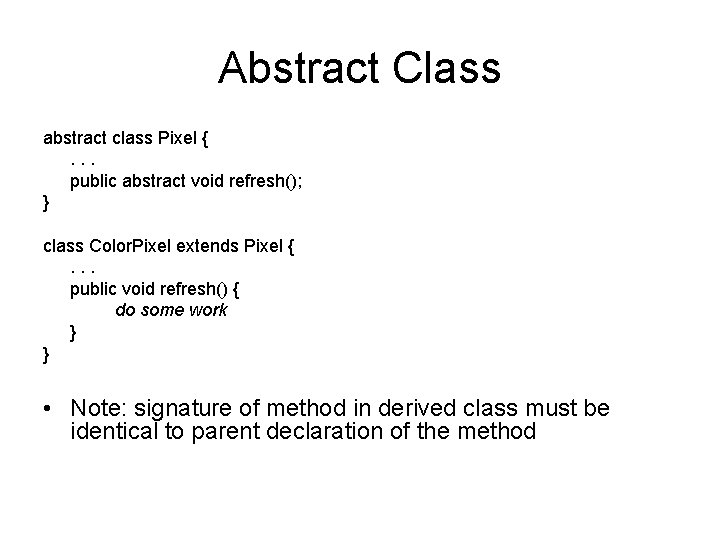
Abstract Class abstract class Pixel {. . . public abstract void refresh(); } class Color. Pixel extends Pixel {. . . public void refresh() { do some work } } • Note: signature of method in derived class must be identical to parent declaration of the method
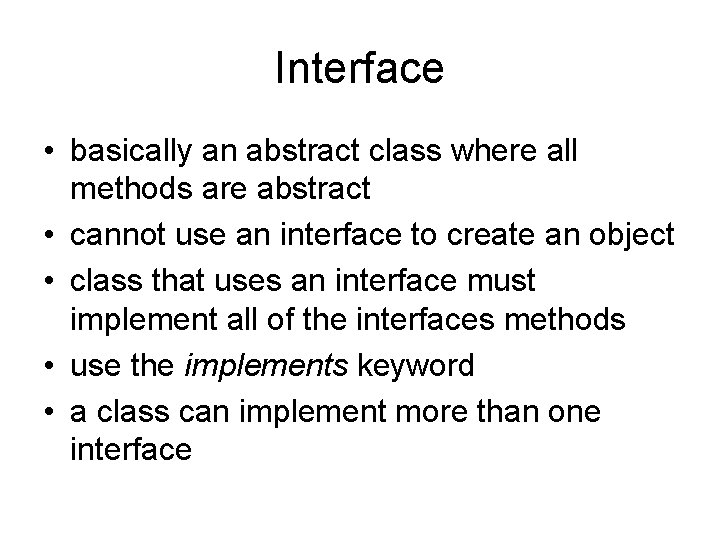
Interface • basically an abstract class where all methods are abstract • cannot use an interface to create an object • class that uses an interface must implement all of the interfaces methods • use the implements keyword • a class can implement more than one interface
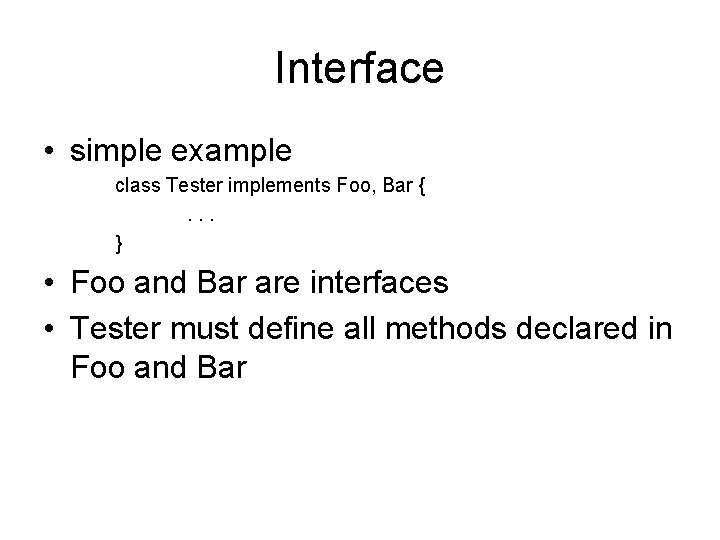
Interface • simple example class Tester implements Foo, Bar {. . . } • Foo and Bar are interfaces • Tester must define all methods declared in Foo and Bar
![Array Review Consecutive blocks of memory Creation int grades Array Review • • • Consecutive blocks of memory Creation: int [] grades =](https://slidetodoc.com/presentation_image/3b17d656250058ef7f6de5a3bfd78f5a/image-20.jpg)
Array Review • • • Consecutive blocks of memory Creation: int [] grades = new int[25]; __. length: holds size of array __. clone(): makes copy of array data out-of-bounds exception: trying to access data outside of array bounds generates an exception • Array size is fixed at creation
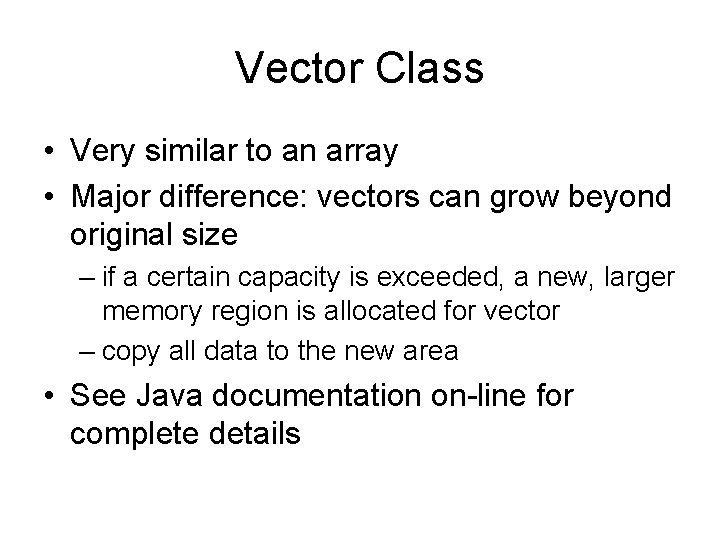
Vector Class • Very similar to an array • Major difference: vectors can grow beyond original size – if a certain capacity is exceeded, a new, larger memory region is allocated for vector – copy all data to the new area • See Java documentation on-line for complete details
Advanced data structures in java
Advanced programming in java
Advanced data structures in python
Data structures and abstractions with java
Java dynamic data structures
Singly linked list in data structure
Data structures in java
Data structures in java
Stacks in data structures
Binary search tree java
Data structures using java
Homologous structure
Sda hymnal 537
Cs537
Buddy system memory allocation
Cs 537
Cs 537
Cs 537
537-317-757
Passive advanced structures
Advanced design of steel structures notes
Dynamic programming bottom up