COP 3804 INTERMEDIATE JAVA Data Structures Data Structures
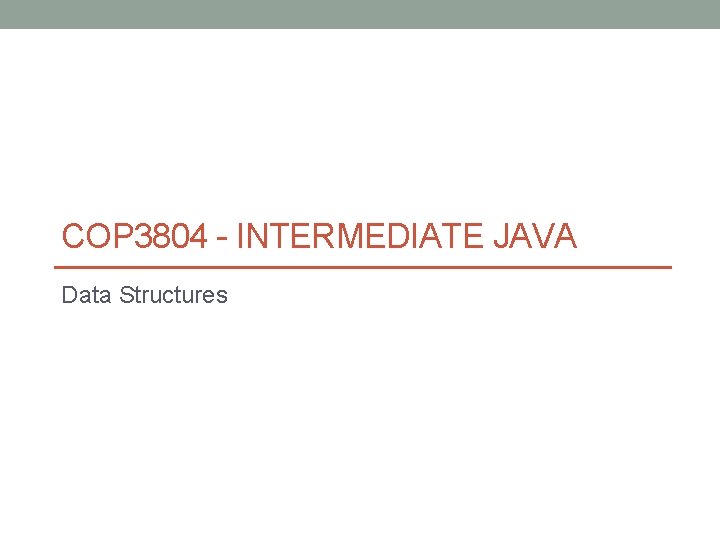
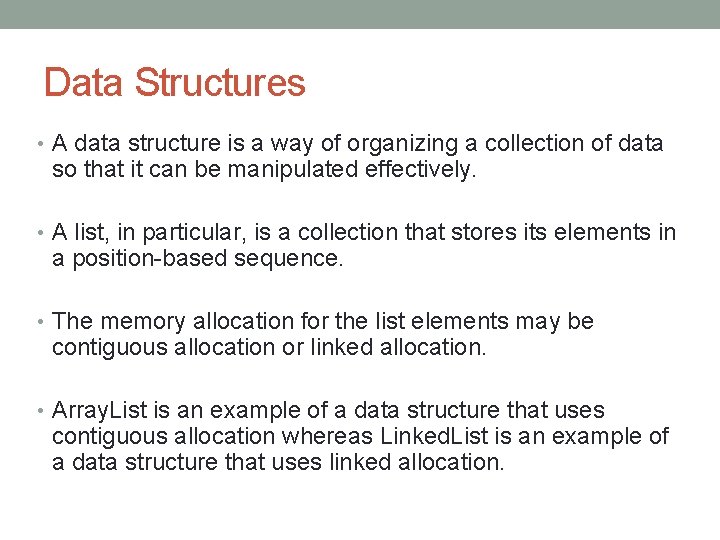
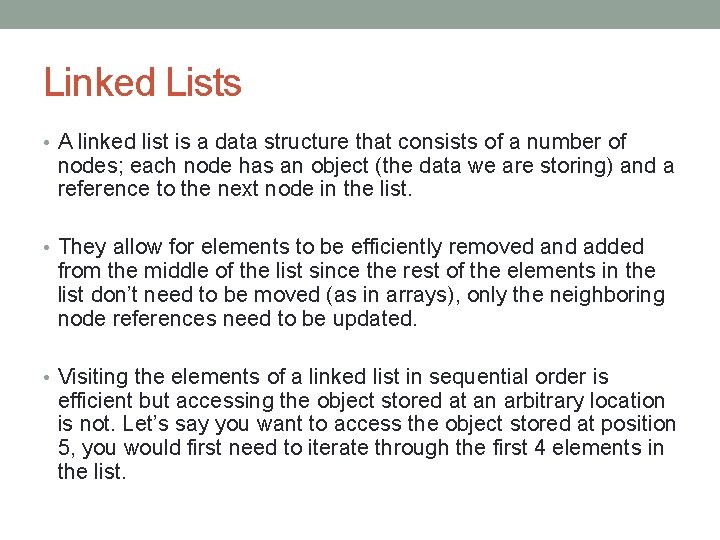
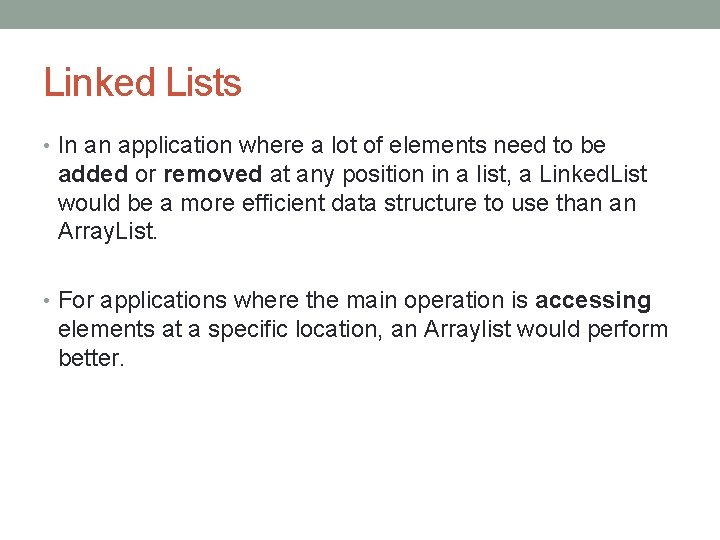
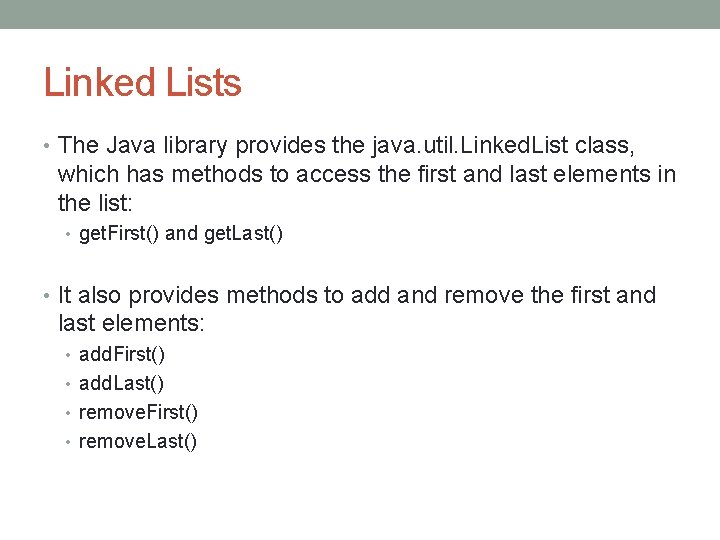
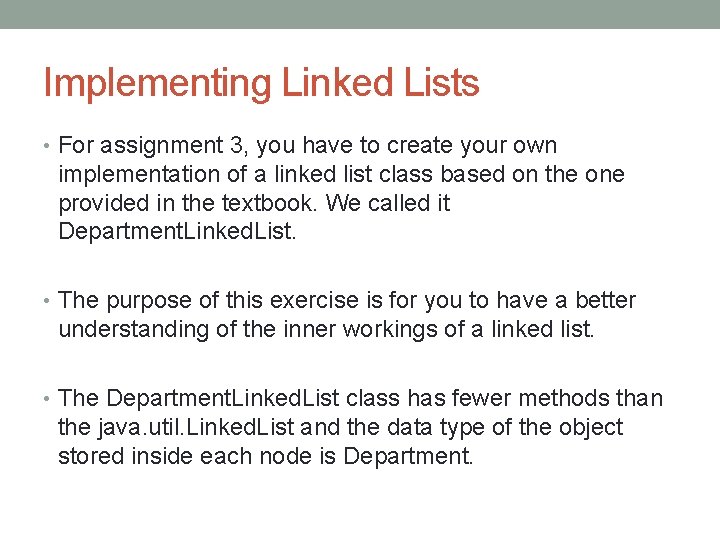
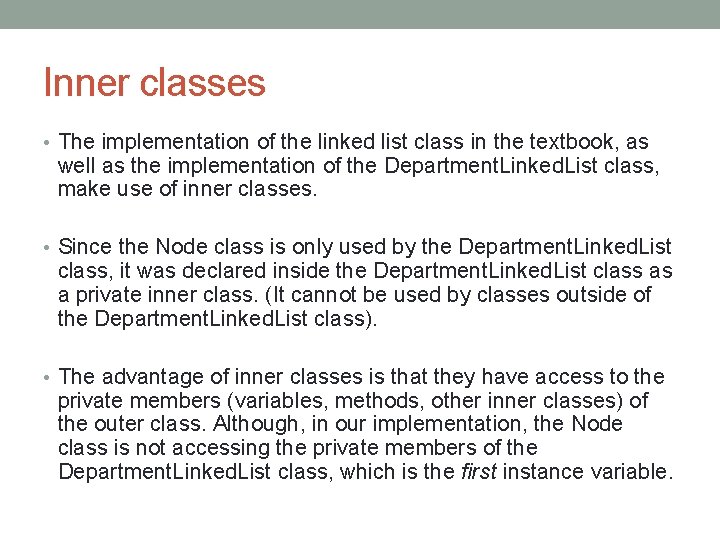
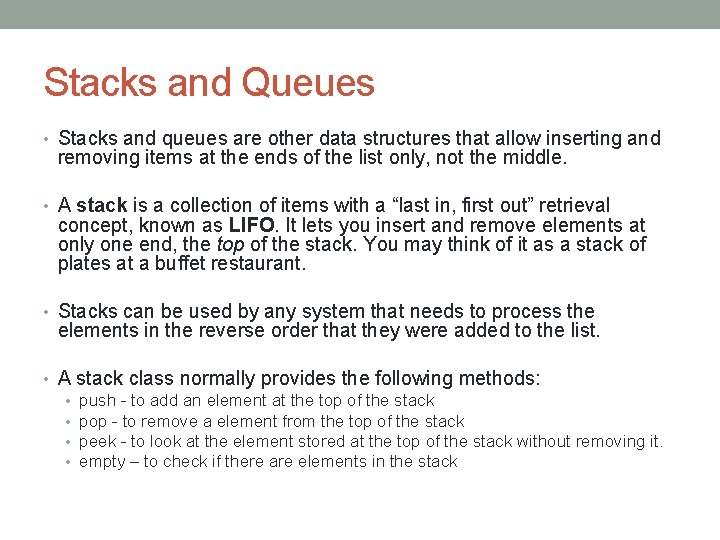
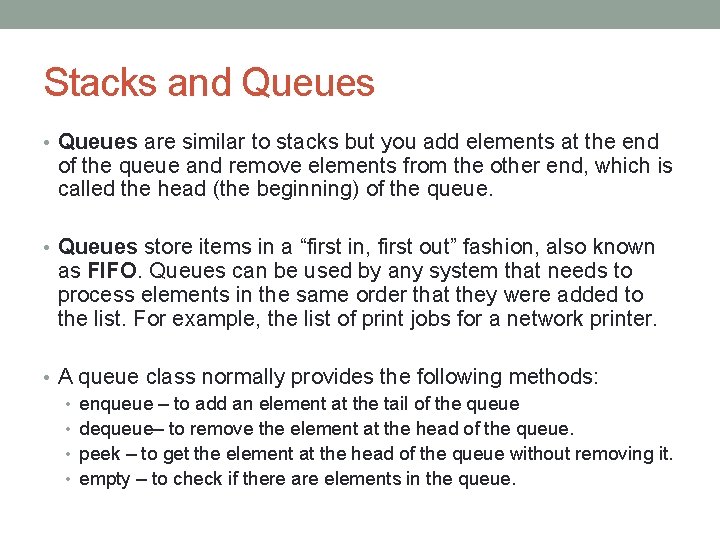
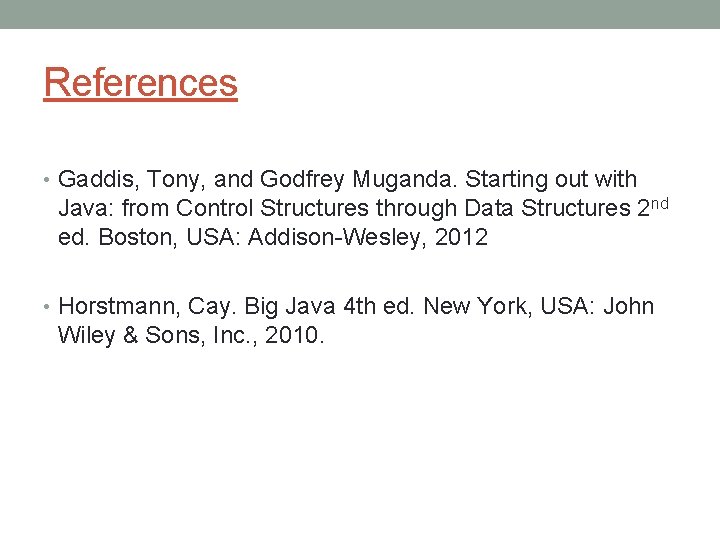
- Slides: 10
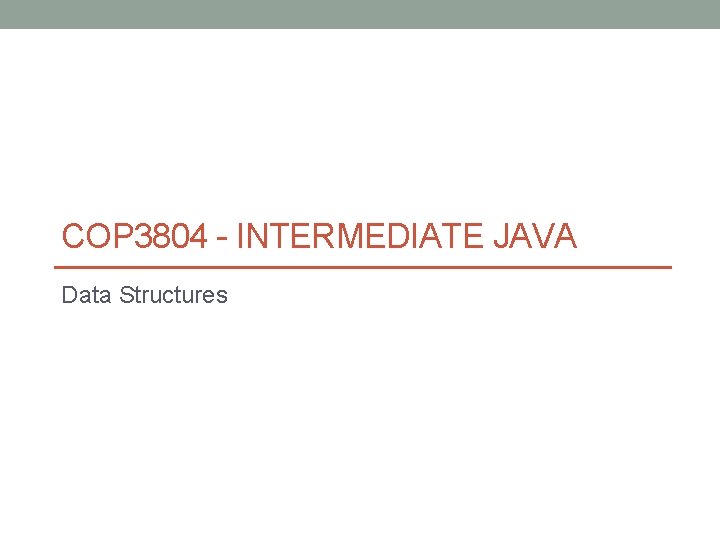
COP 3804 - INTERMEDIATE JAVA Data Structures
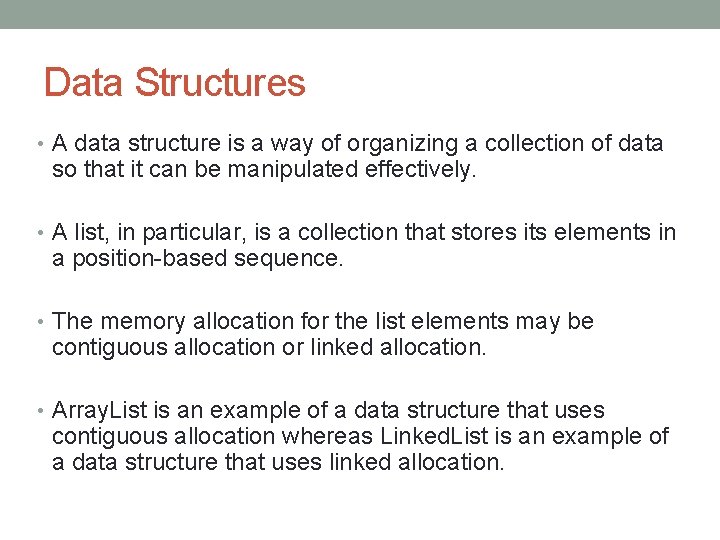
Data Structures • A data structure is a way of organizing a collection of data so that it can be manipulated effectively. • A list, in particular, is a collection that stores its elements in a position-based sequence. • The memory allocation for the list elements may be contiguous allocation or linked allocation. • Array. List is an example of a data structure that uses contiguous allocation whereas Linked. List is an example of a data structure that uses linked allocation.
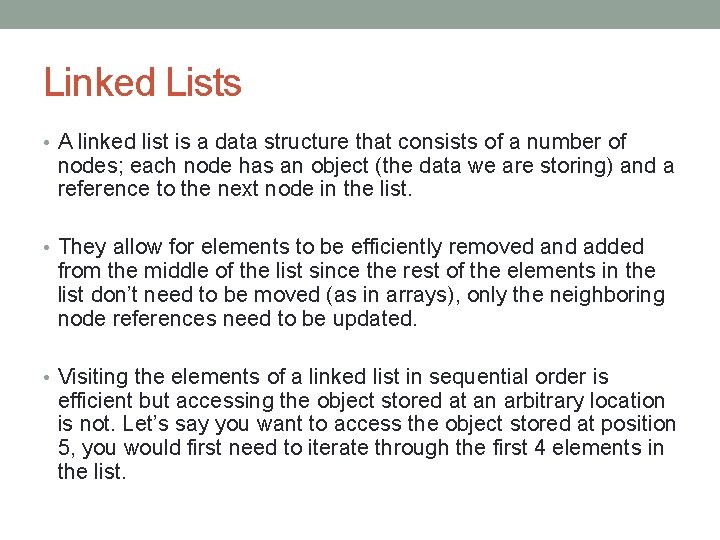
Linked Lists • A linked list is a data structure that consists of a number of nodes; each node has an object (the data we are storing) and a reference to the next node in the list. • They allow for elements to be efficiently removed and added from the middle of the list since the rest of the elements in the list don’t need to be moved (as in arrays), only the neighboring node references need to be updated. • Visiting the elements of a linked list in sequential order is efficient but accessing the object stored at an arbitrary location is not. Let’s say you want to access the object stored at position 5, you would first need to iterate through the first 4 elements in the list.
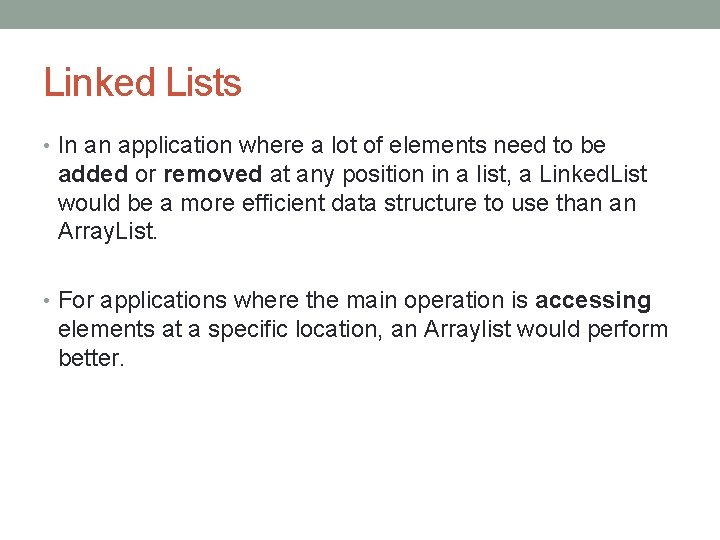
Linked Lists • In an application where a lot of elements need to be added or removed at any position in a list, a Linked. List would be a more efficient data structure to use than an Array. List. • For applications where the main operation is accessing elements at a specific location, an Arraylist would perform better.
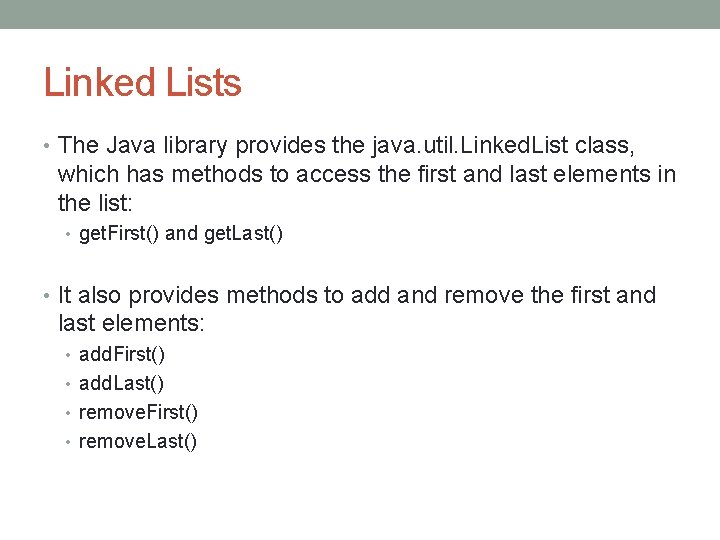
Linked Lists • The Java library provides the java. util. Linked. List class, which has methods to access the first and last elements in the list: • get. First() and get. Last() • It also provides methods to add and remove the first and last elements: • add. First() • add. Last() • remove. First() • remove. Last()
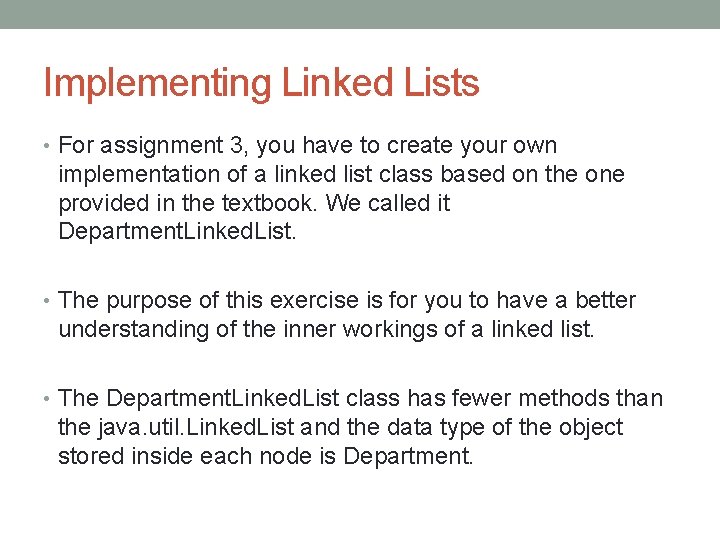
Implementing Linked Lists • For assignment 3, you have to create your own implementation of a linked list class based on the one provided in the textbook. We called it Department. Linked. List. • The purpose of this exercise is for you to have a better understanding of the inner workings of a linked list. • The Department. Linked. List class has fewer methods than the java. util. Linked. List and the data type of the object stored inside each node is Department.
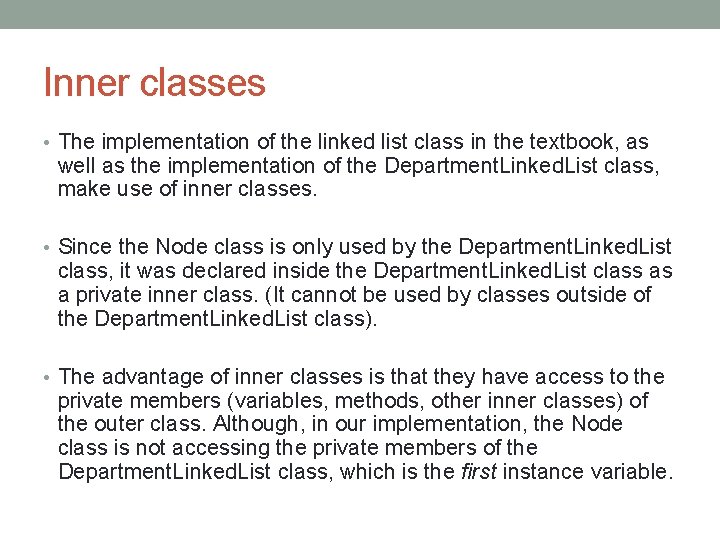
Inner classes • The implementation of the linked list class in the textbook, as well as the implementation of the Department. Linked. List class, make use of inner classes. • Since the Node class is only used by the Department. Linked. List class, it was declared inside the Department. Linked. List class as a private inner class. (It cannot be used by classes outside of the Department. Linked. List class). • The advantage of inner classes is that they have access to the private members (variables, methods, other inner classes) of the outer class. Although, in our implementation, the Node class is not accessing the private members of the Department. Linked. List class, which is the first instance variable.
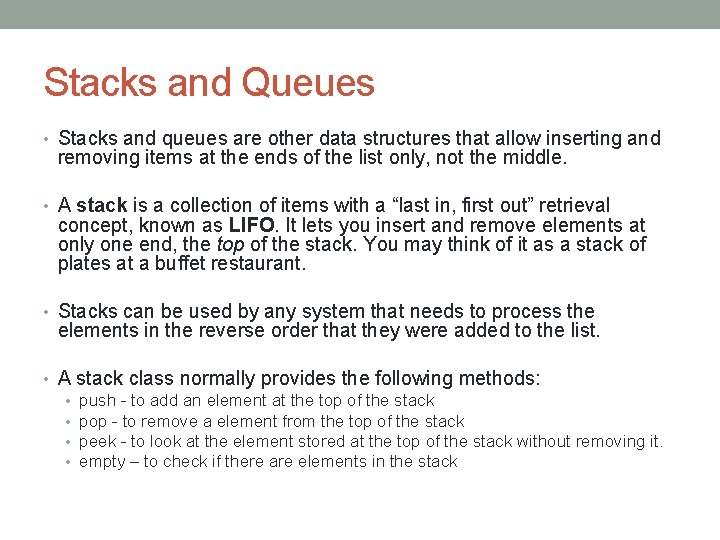
Stacks and Queues • Stacks and queues are other data structures that allow inserting and removing items at the ends of the list only, not the middle. • A stack is a collection of items with a “last in, first out” retrieval concept, known as LIFO. It lets you insert and remove elements at only one end, the top of the stack. You may think of it as a stack of plates at a buffet restaurant. • Stacks can be used by any system that needs to process the elements in the reverse order that they were added to the list. • A stack class normally provides the following methods: • push - to add an element at the top of the stack • pop - to remove a element from the top of the stack • peek - to look at the element stored at the top of the stack without removing it. • empty – to check if there are elements in the stack
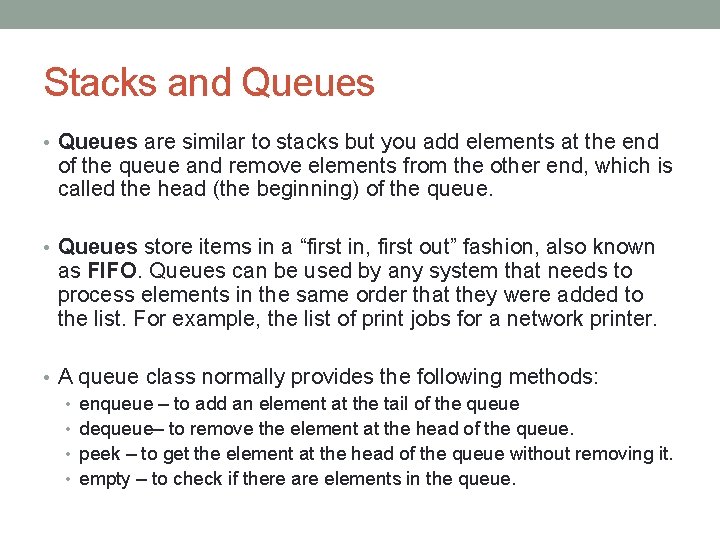
Stacks and Queues • Queues are similar to stacks but you add elements at the end of the queue and remove elements from the other end, which is called the head (the beginning) of the queue. • Queues store items in a “first in, first out” fashion, also known as FIFO. Queues can be used by any system that needs to process elements in the same order that they were added to the list. For example, the list of print jobs for a network printer. • A queue class normally provides the following methods: • enqueue – to add an element at the tail of the queue • dequeue– to remove the element at the head of the queue. • peek – to get the element at the head of the queue without removing it. • empty – to check if there are elements in the queue.
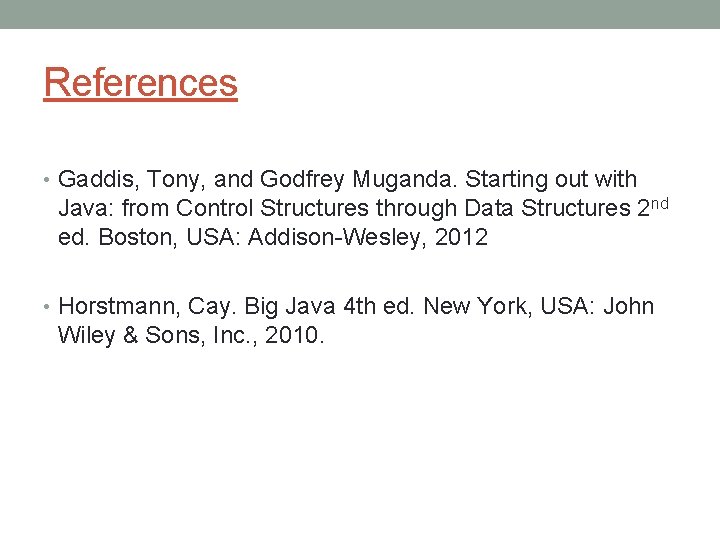
References • Gaddis, Tony, and Godfrey Muganda. Starting out with Java: from Control Structures through Data Structures 2 nd ed. Boston, USA: Addison-Wesley, 2012 • Horstmann, Cay. Big Java 4 th ed. New York, USA: John Wiley & Sons, Inc. , 2010.