Week 2 Fancy Face Conditional Execution Recursive Tree
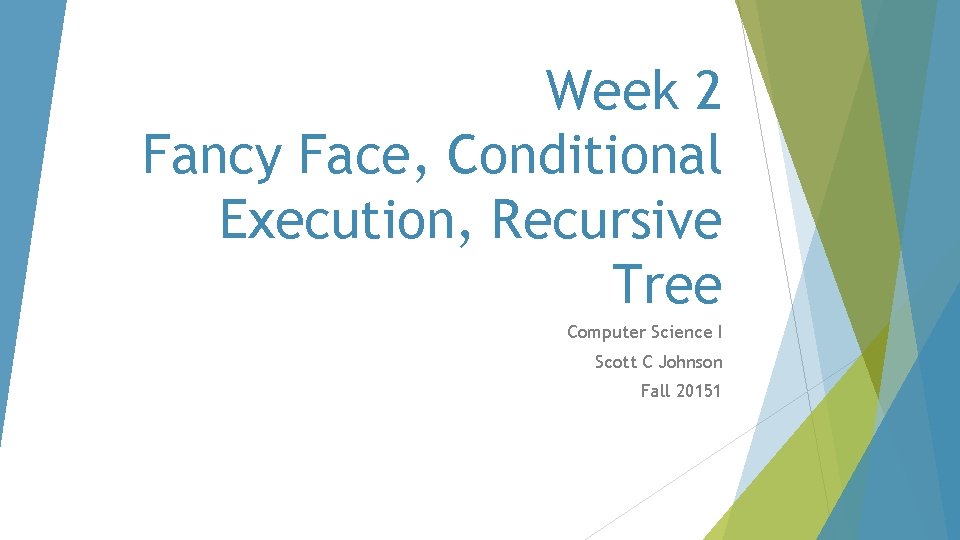
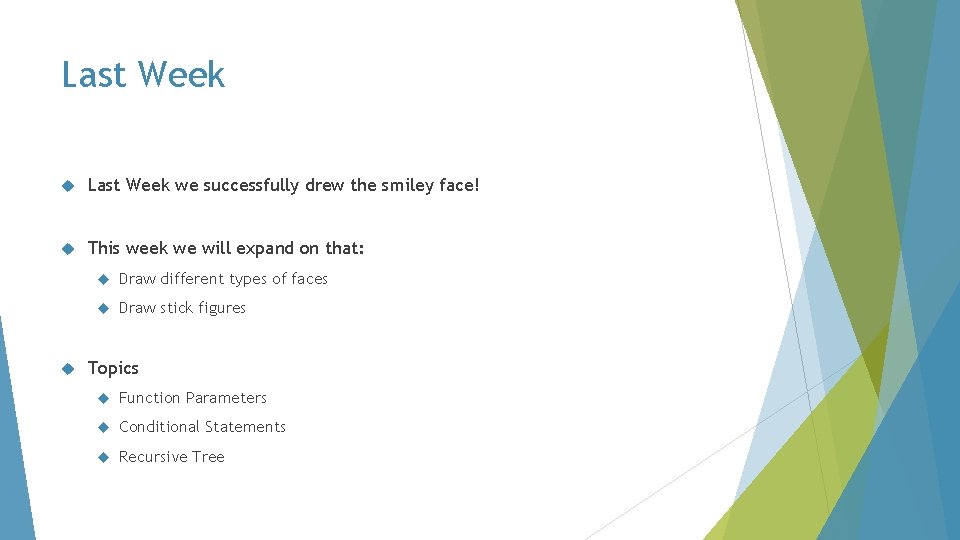
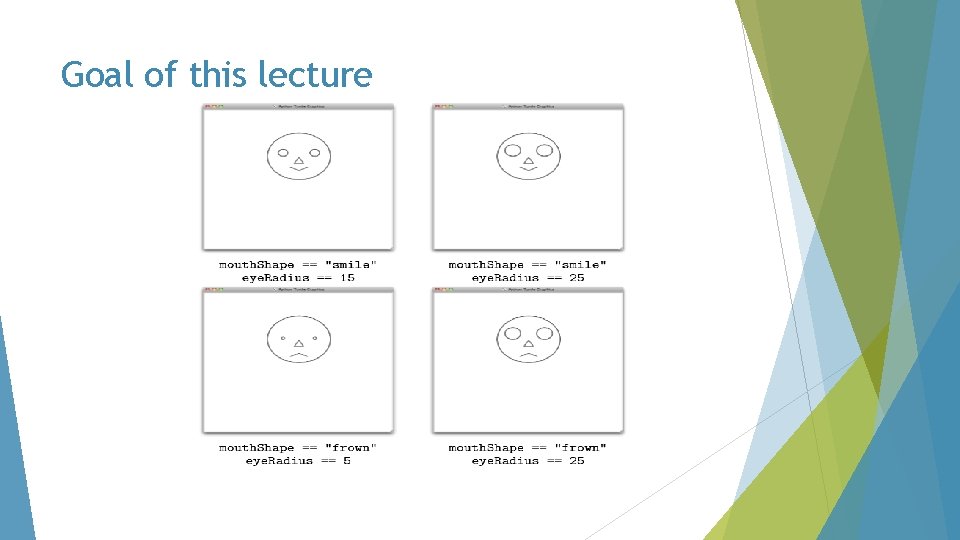
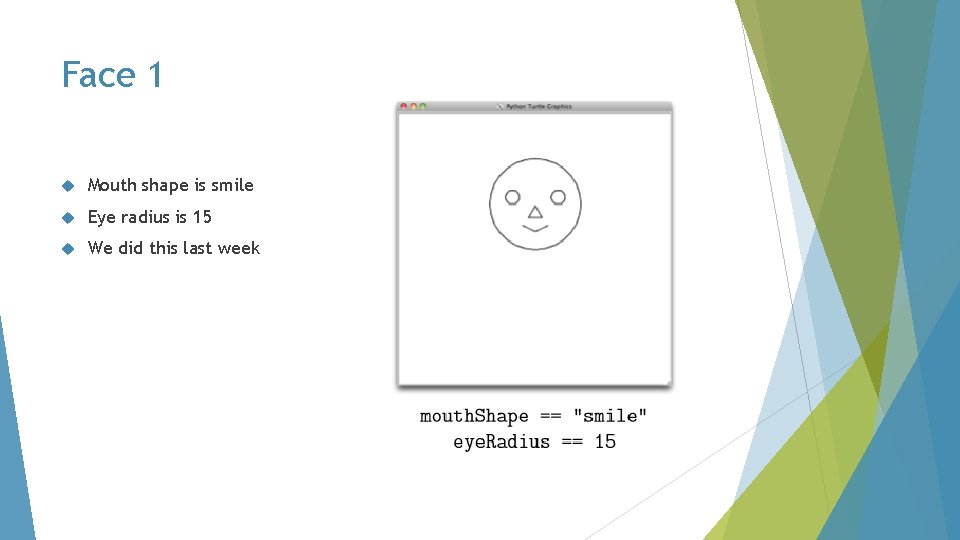
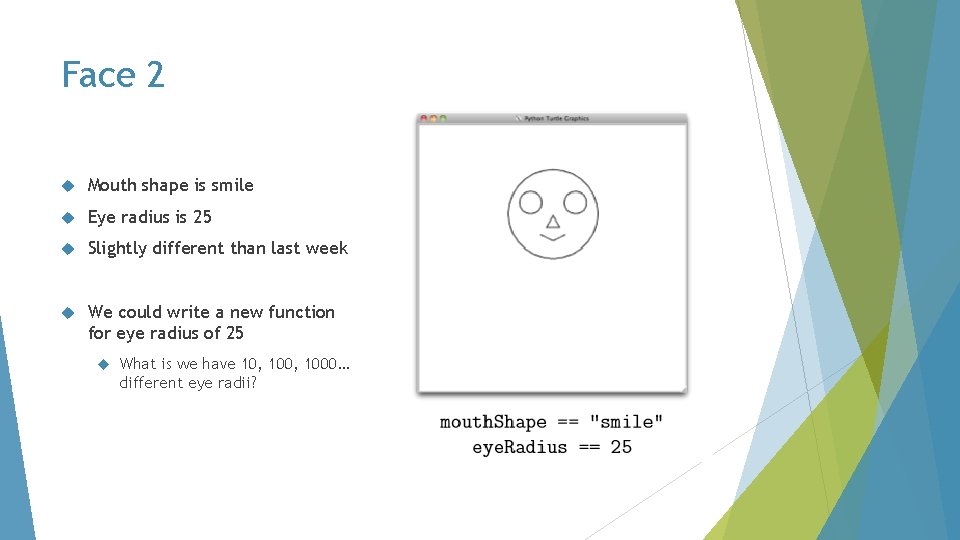
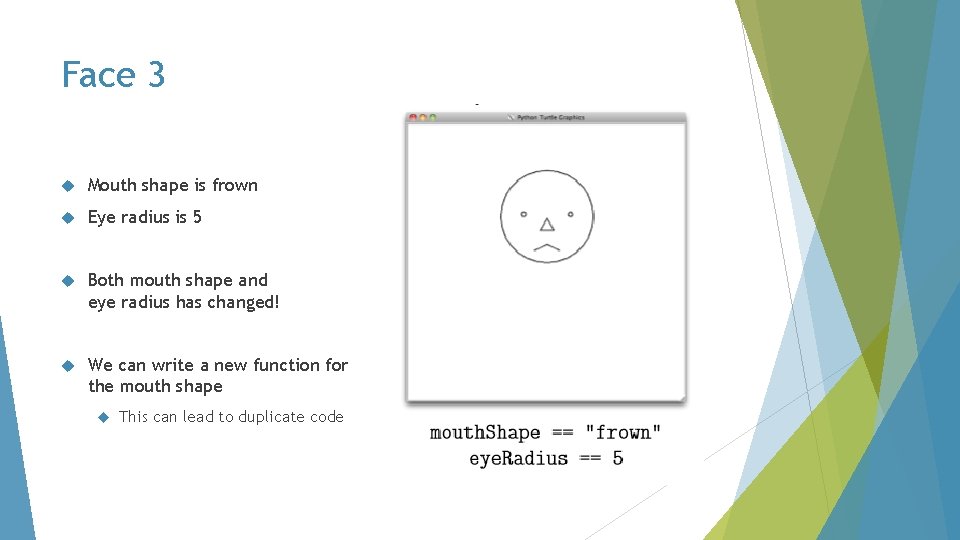
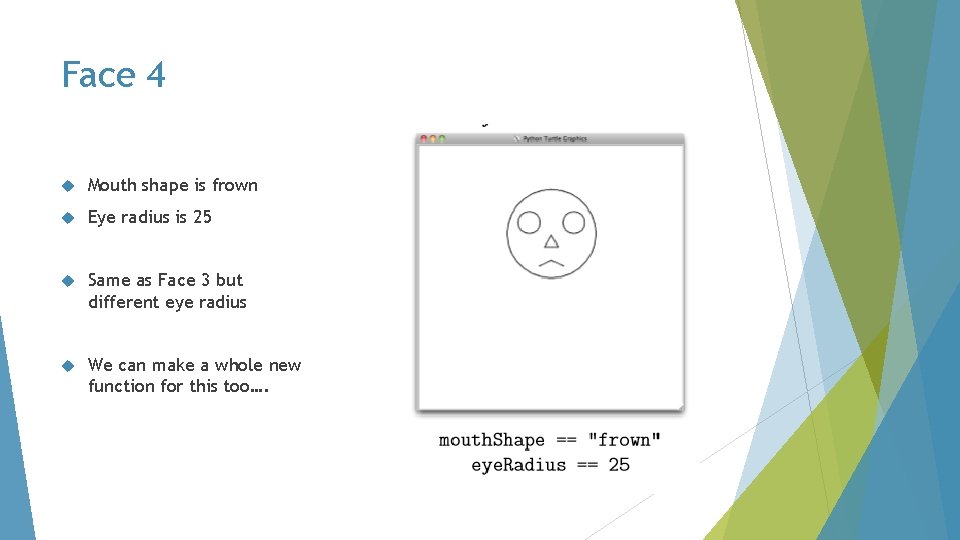
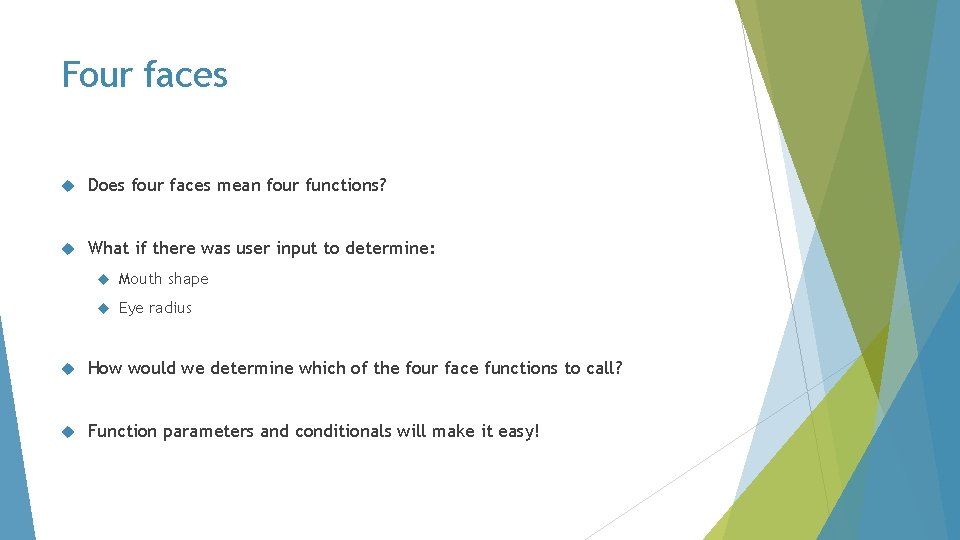
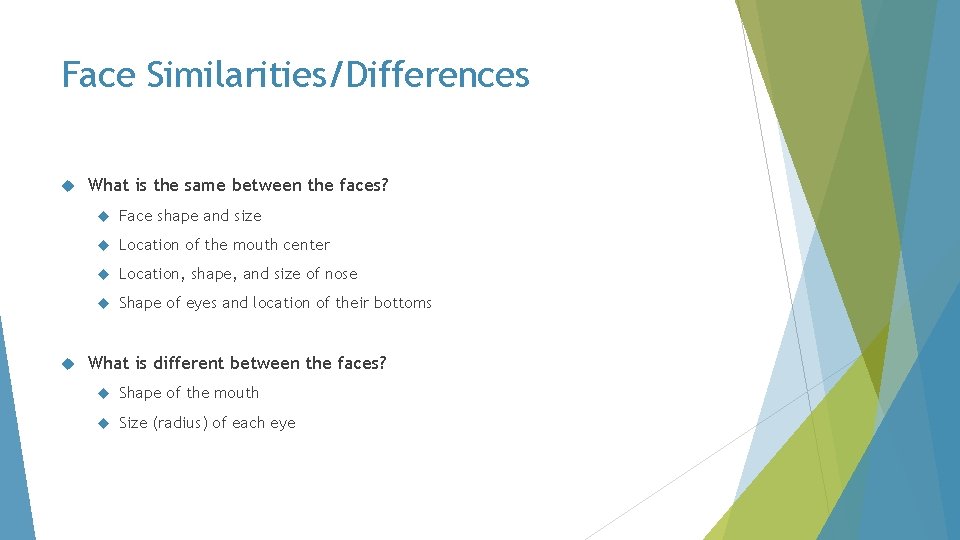
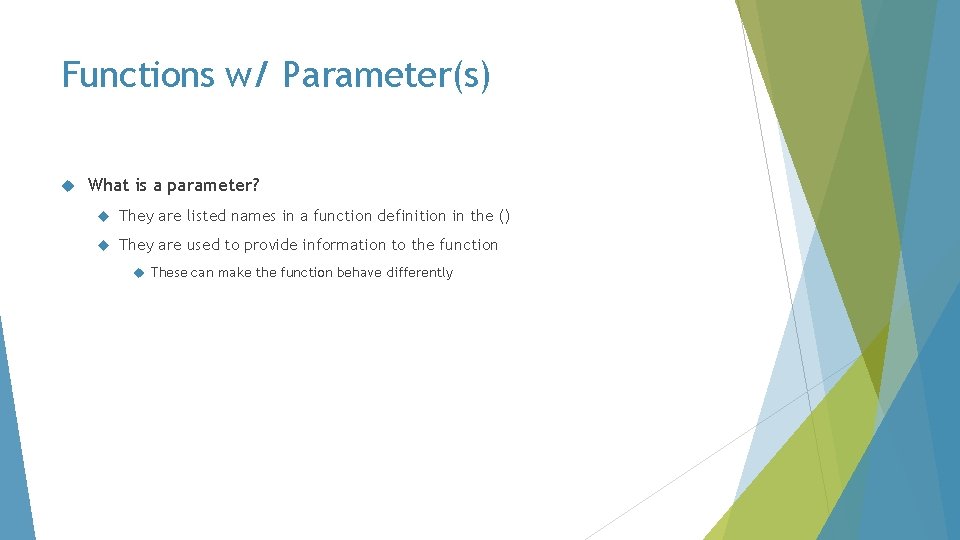
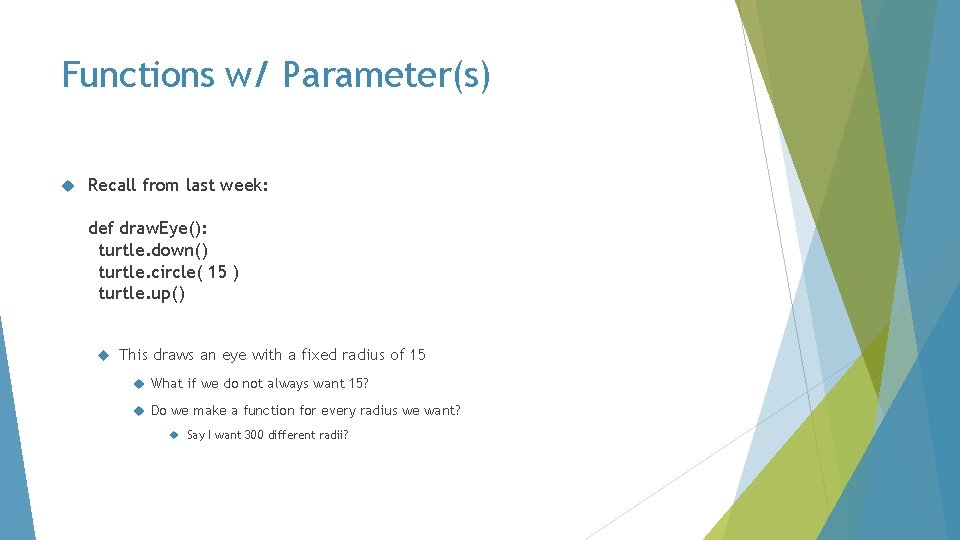
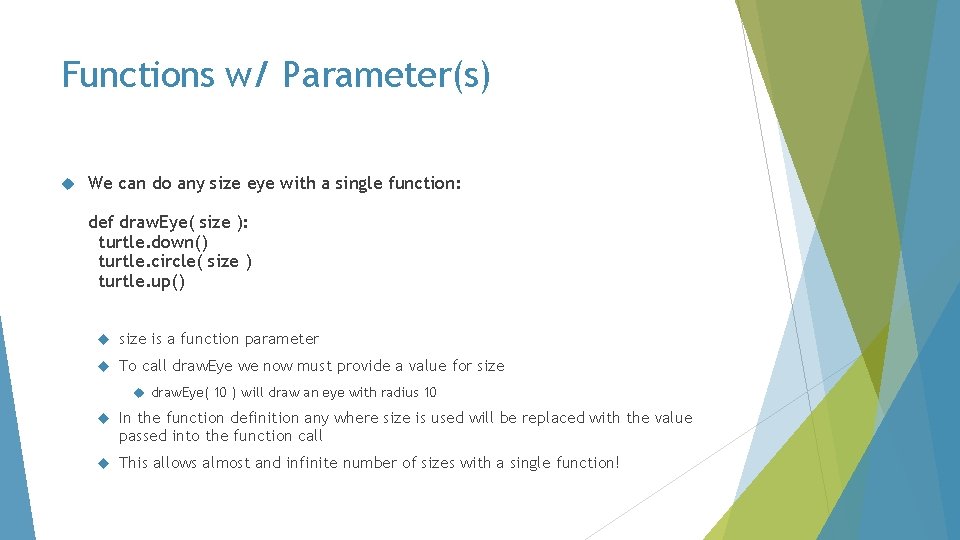
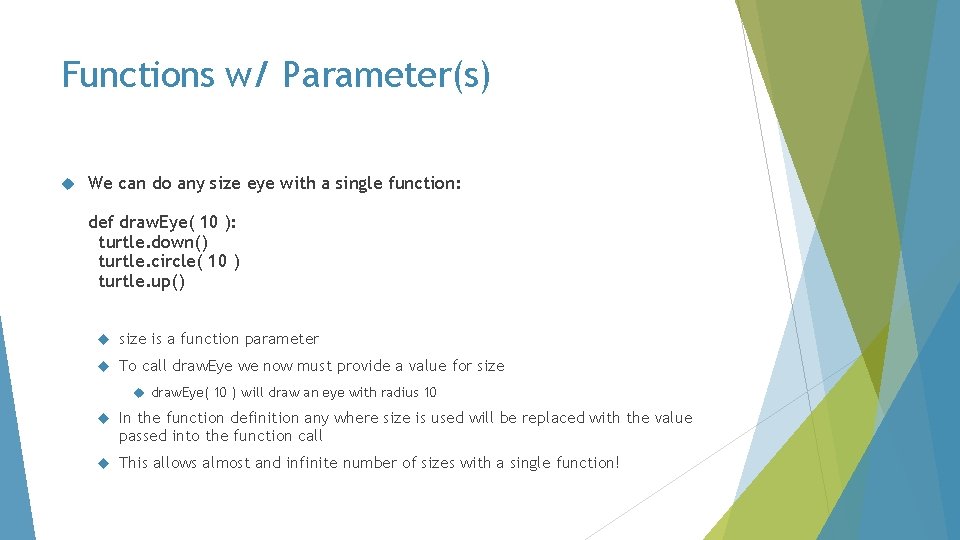
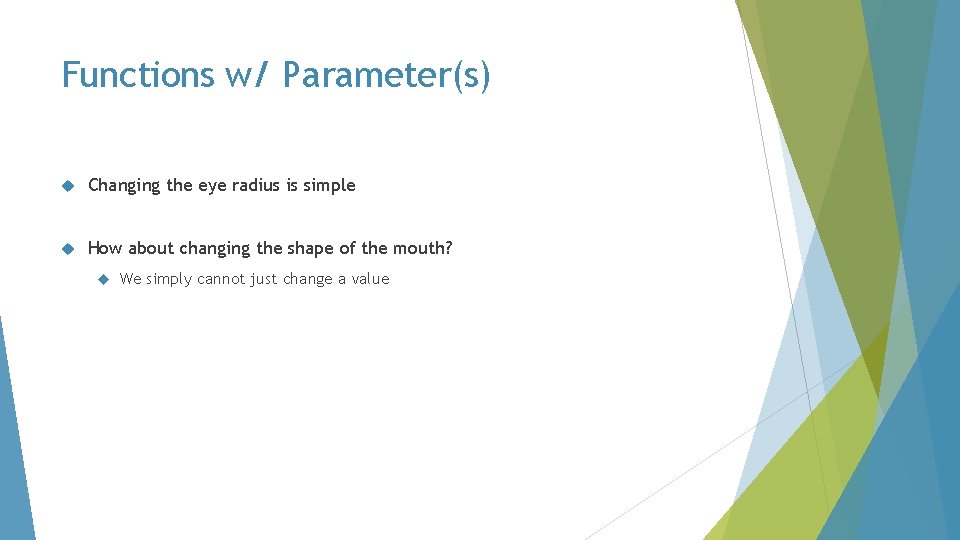
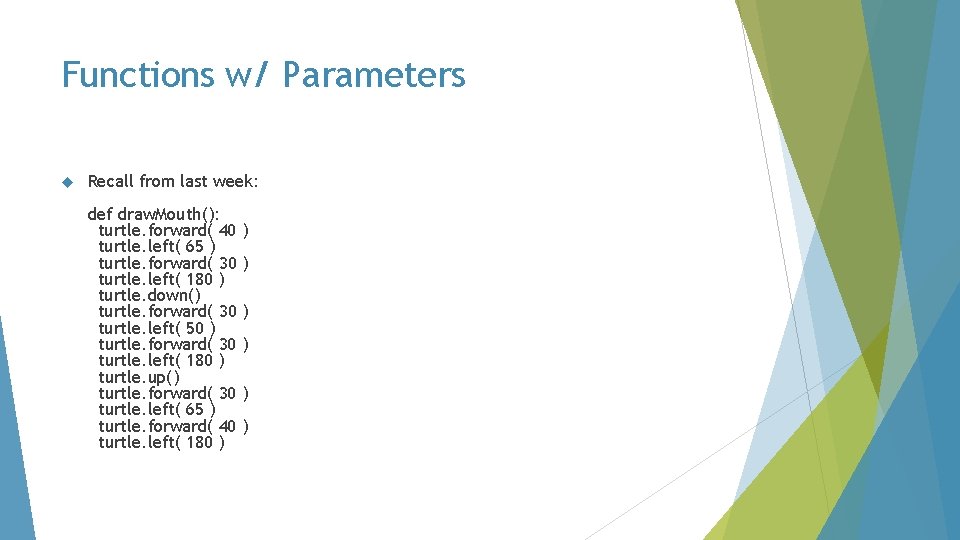
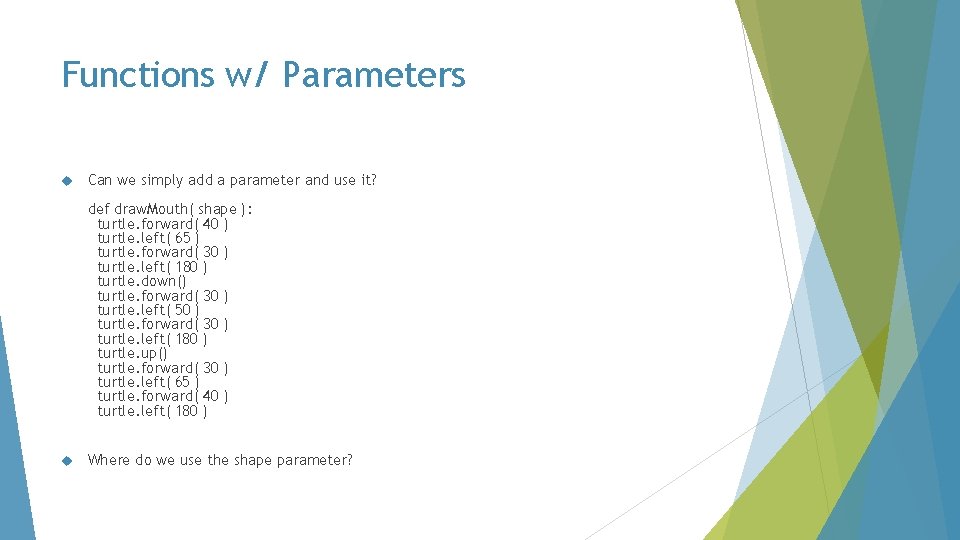
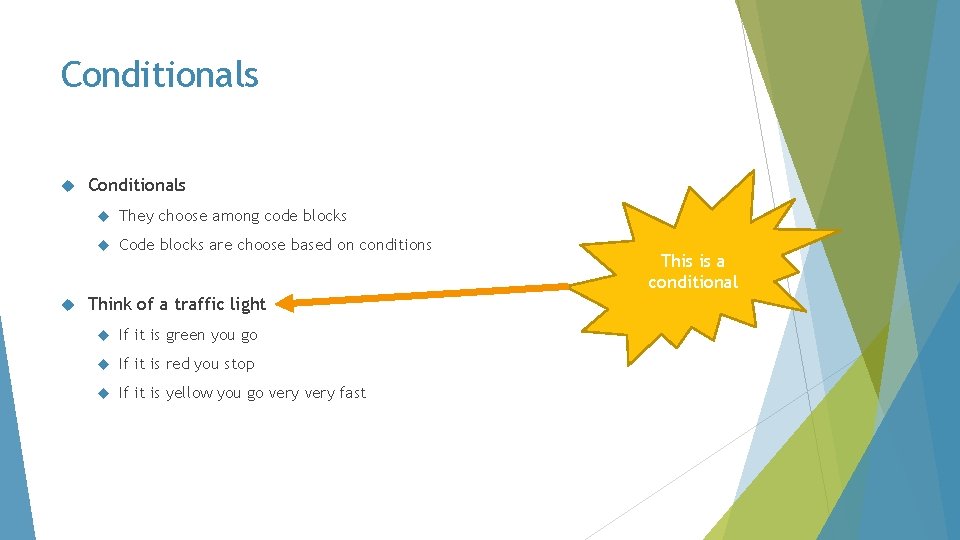
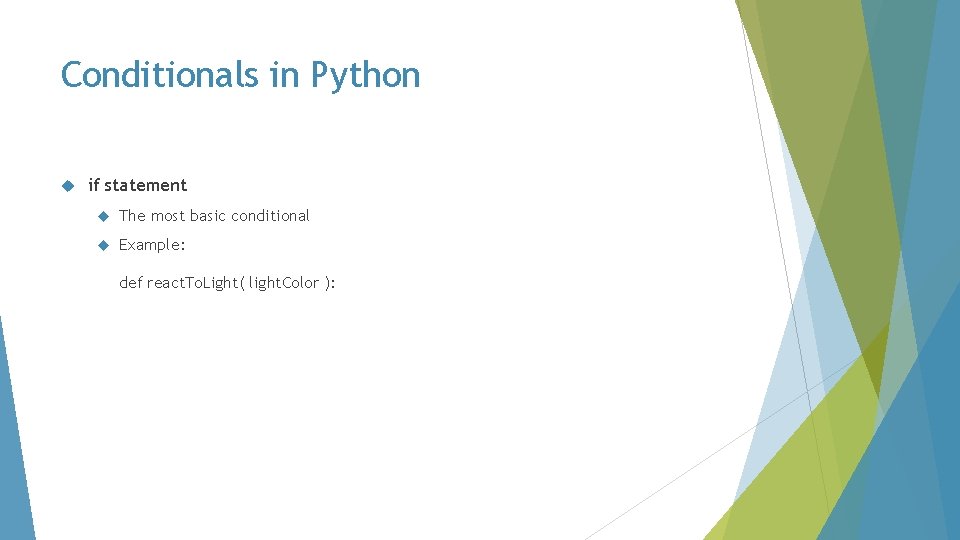
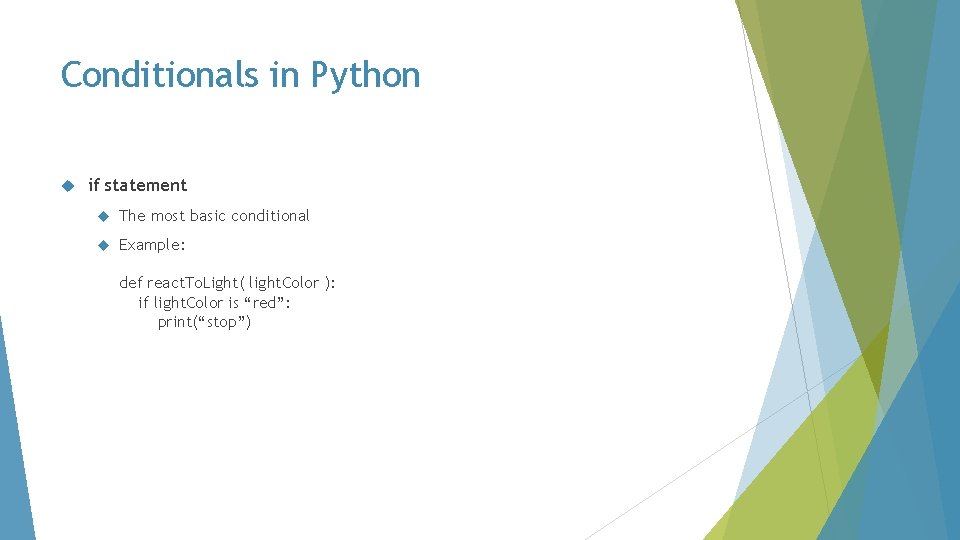
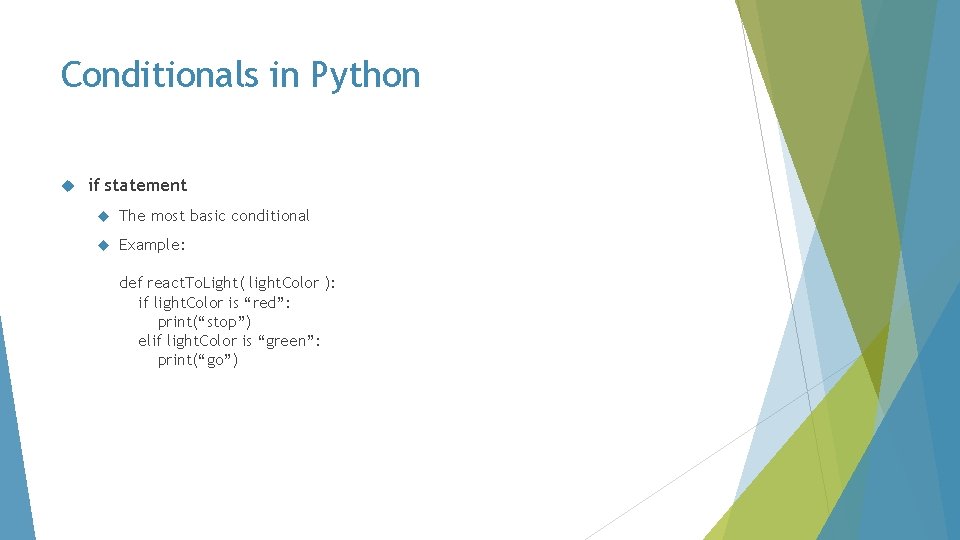
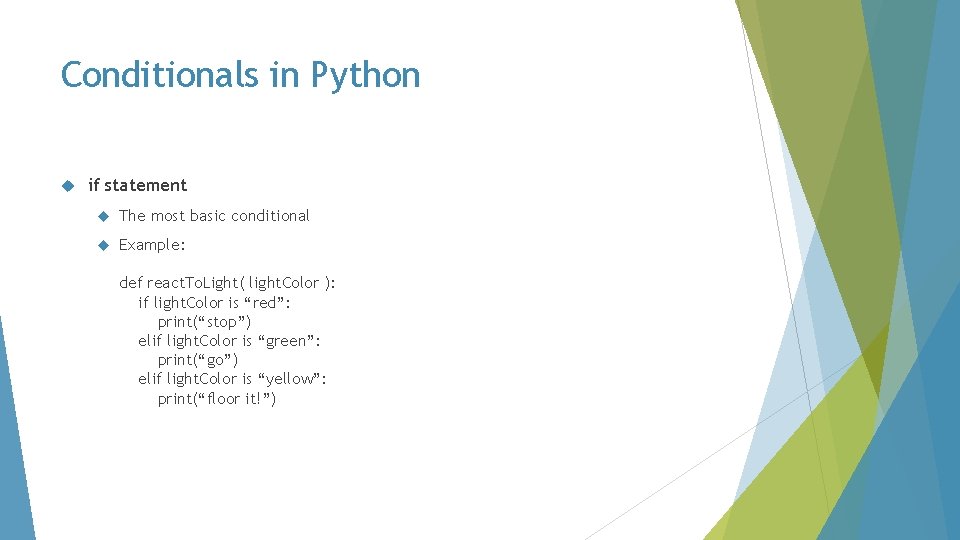
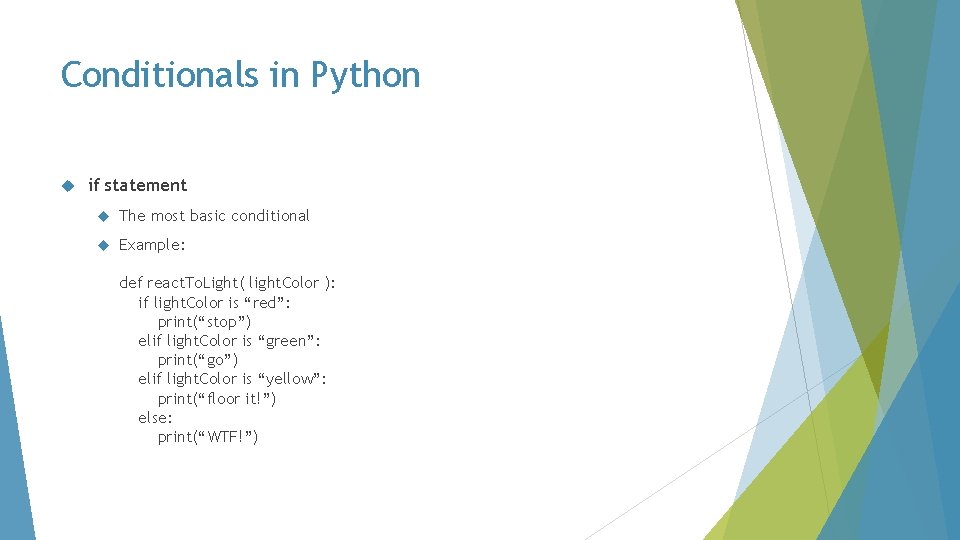
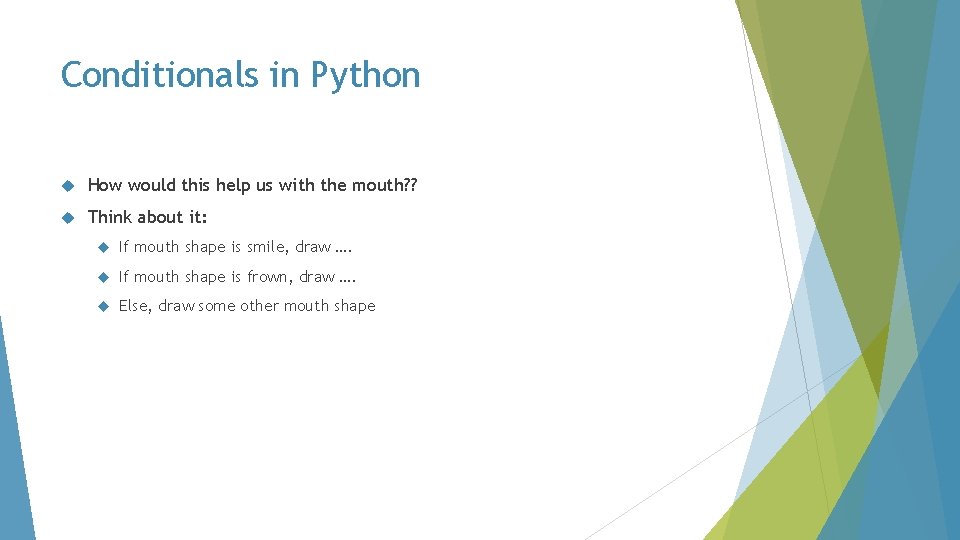
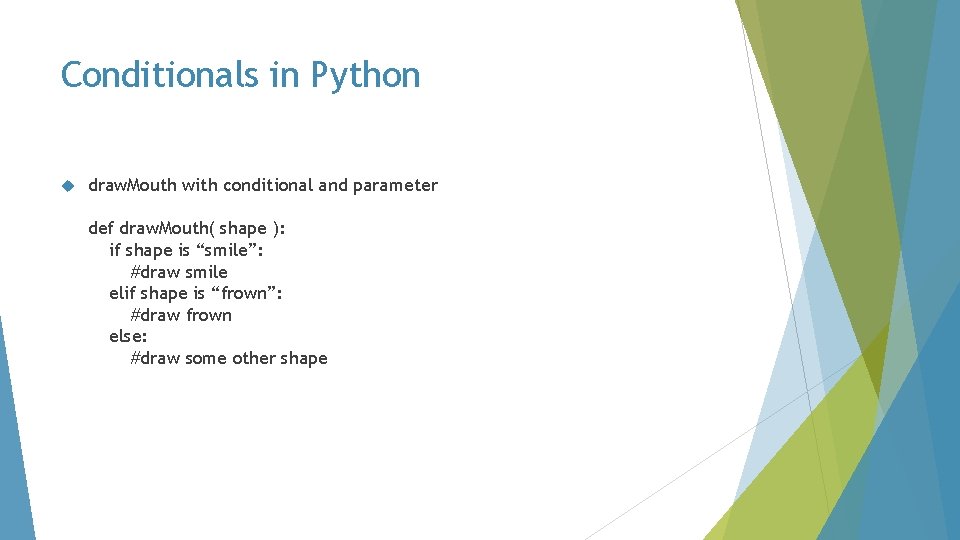
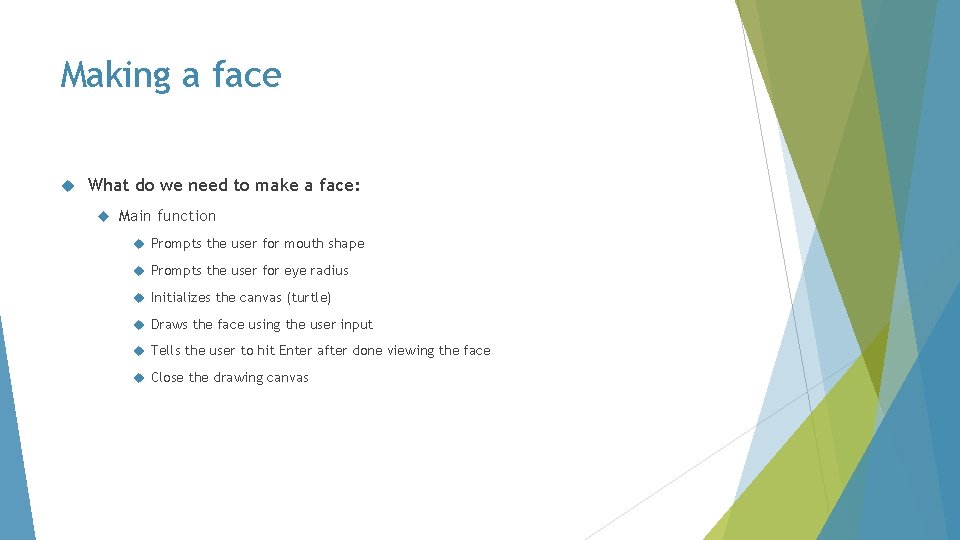
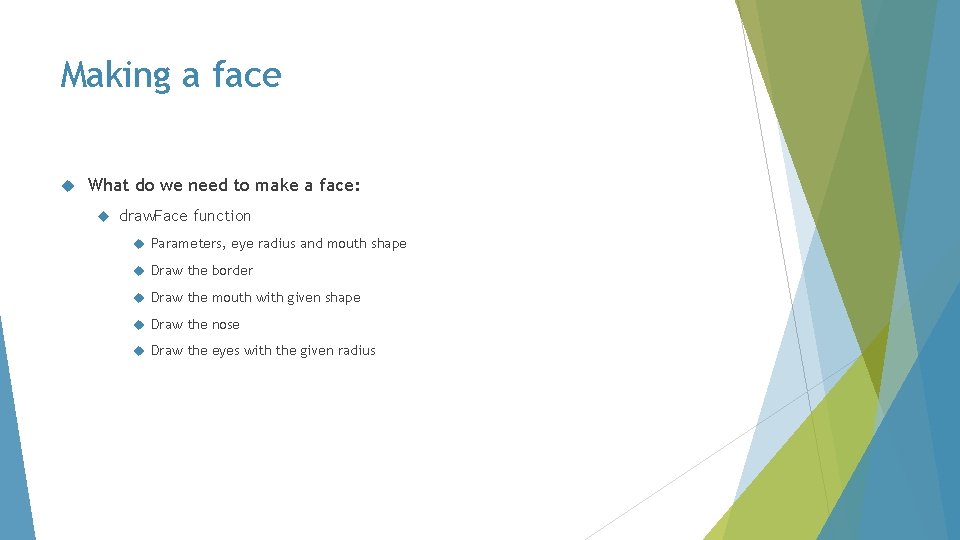
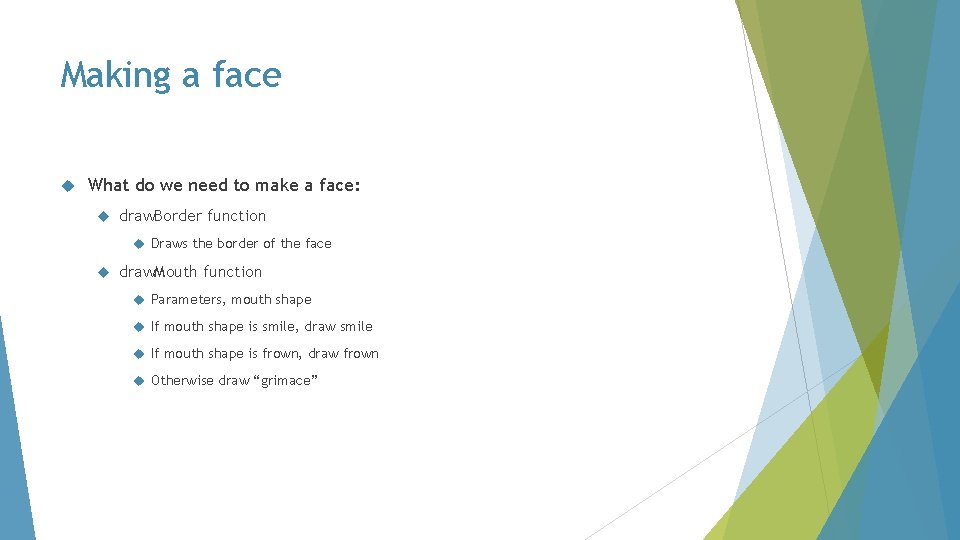
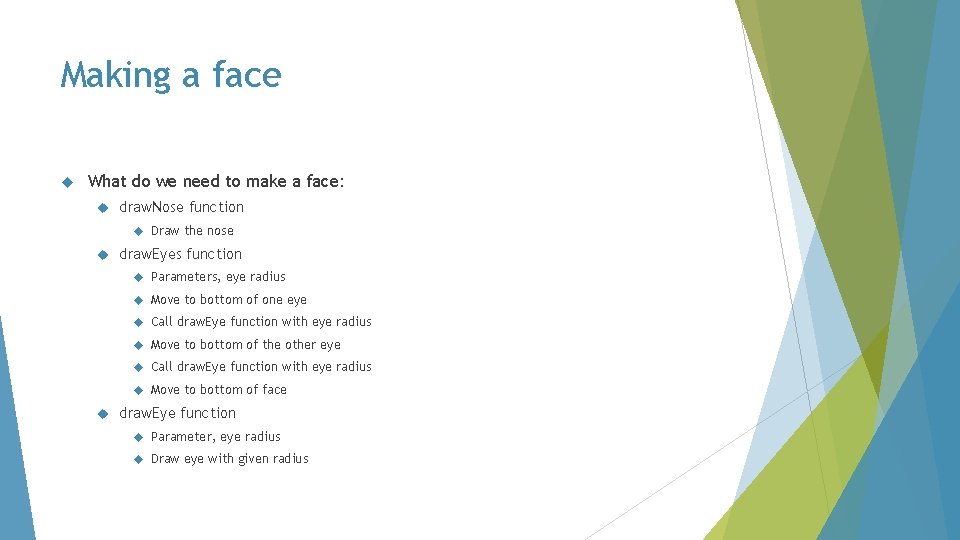
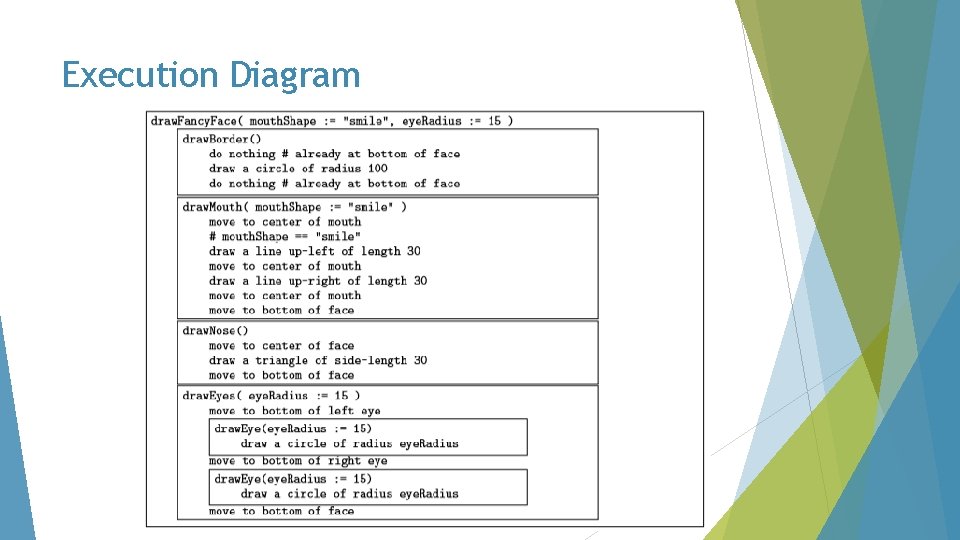
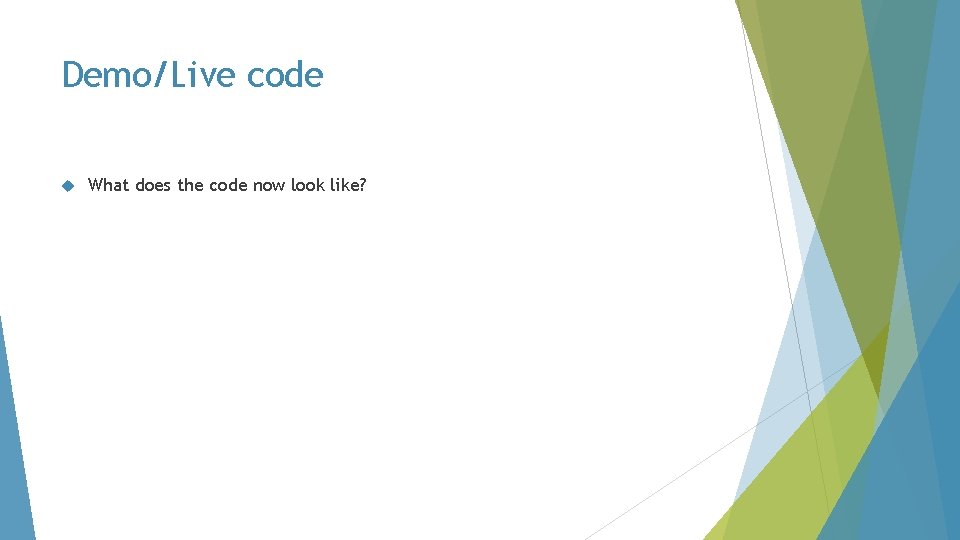
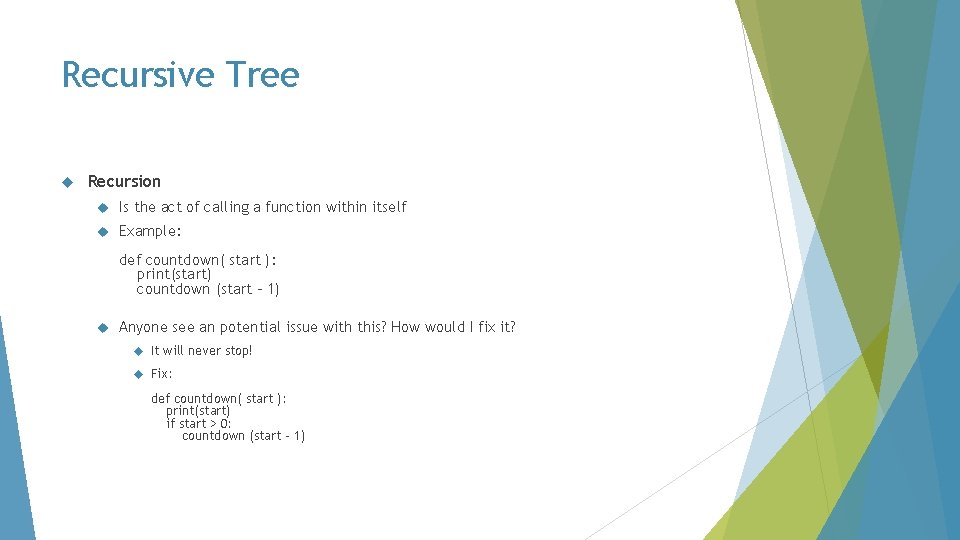
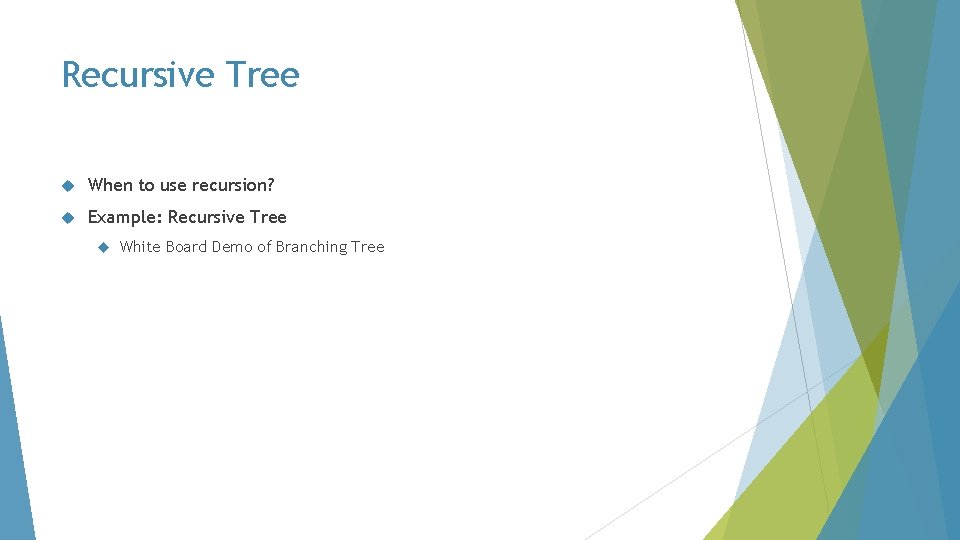
- Slides: 32
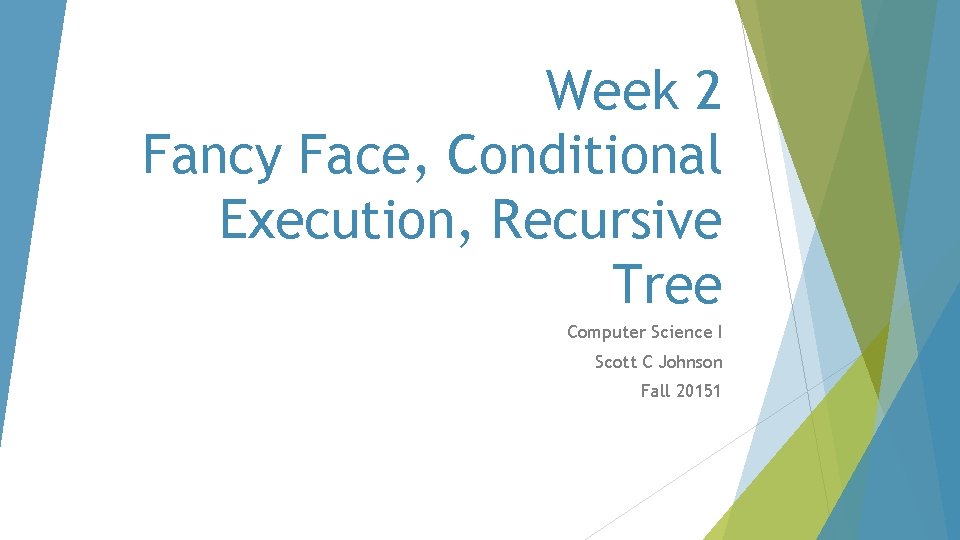
Week 2 Fancy Face, Conditional Execution, Recursive Tree Computer Science I Scott C Johnson Fall 20151
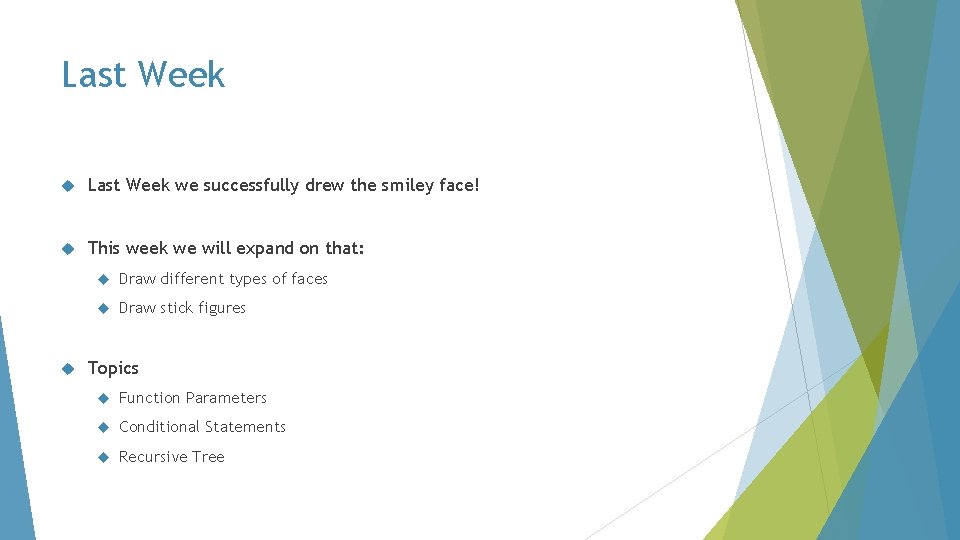
Last Week we successfully drew the smiley face! This week we will expand on that: Draw different types of faces Draw stick figures Topics Function Parameters Conditional Statements Recursive Tree
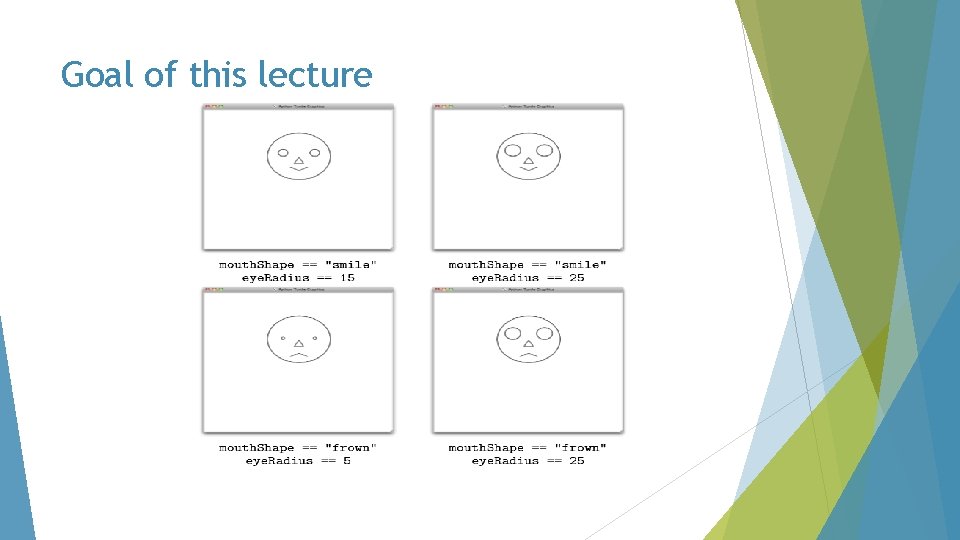
Goal of this lecture
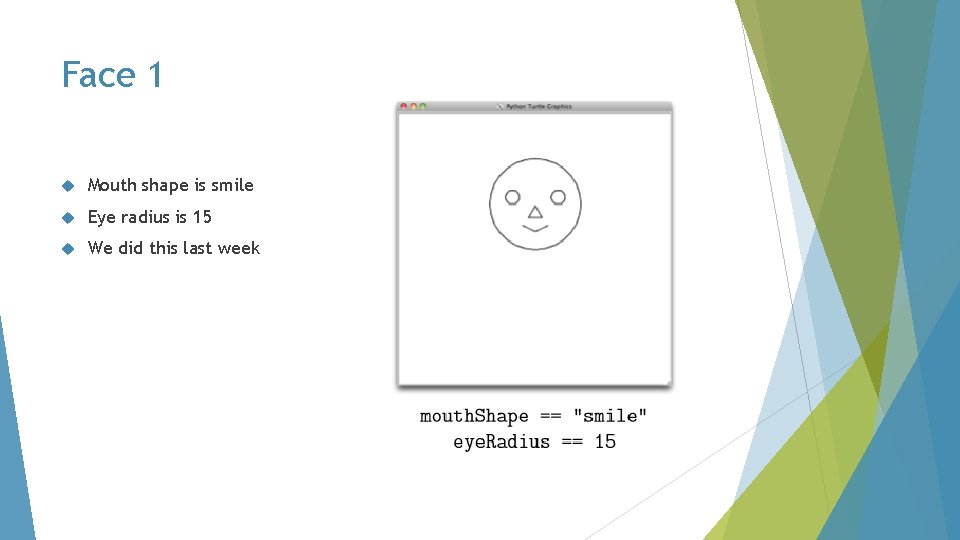
Face 1 Mouth shape is smile Eye radius is 15 We did this last week
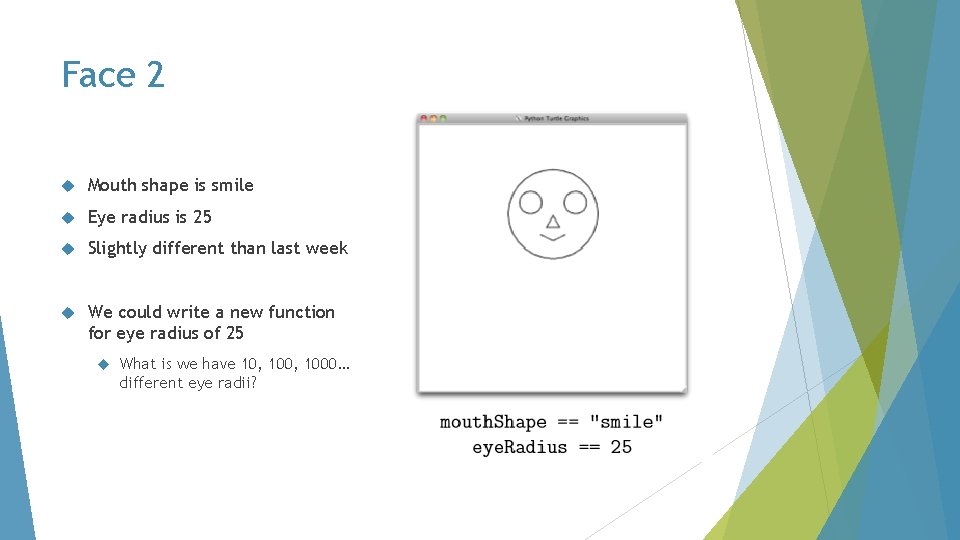
Face 2 Mouth shape is smile Eye radius is 25 Slightly different than last week We could write a new function for eye radius of 25 What is we have 10, 1000… different eye radii?
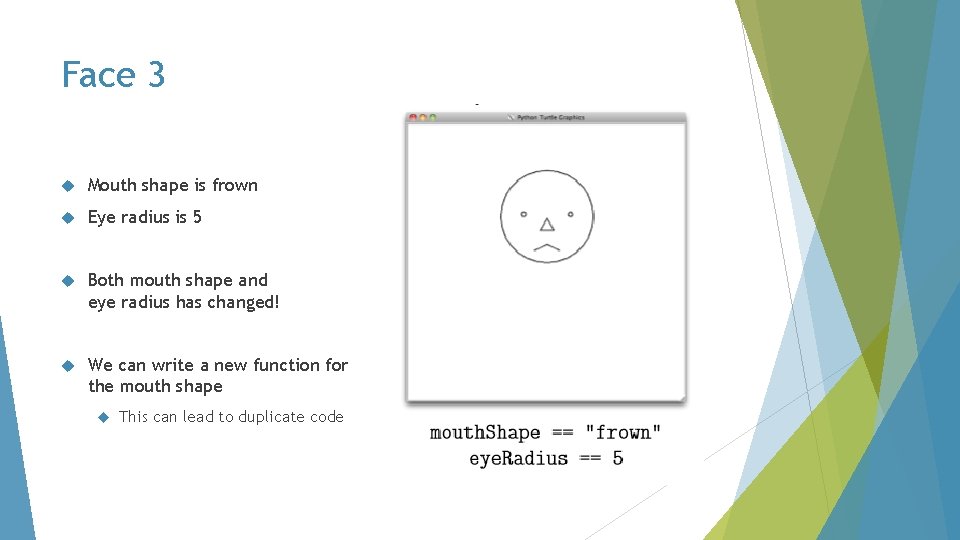
Face 3 Mouth shape is frown Eye radius is 5 Both mouth shape and eye radius has changed! We can write a new function for the mouth shape This can lead to duplicate code
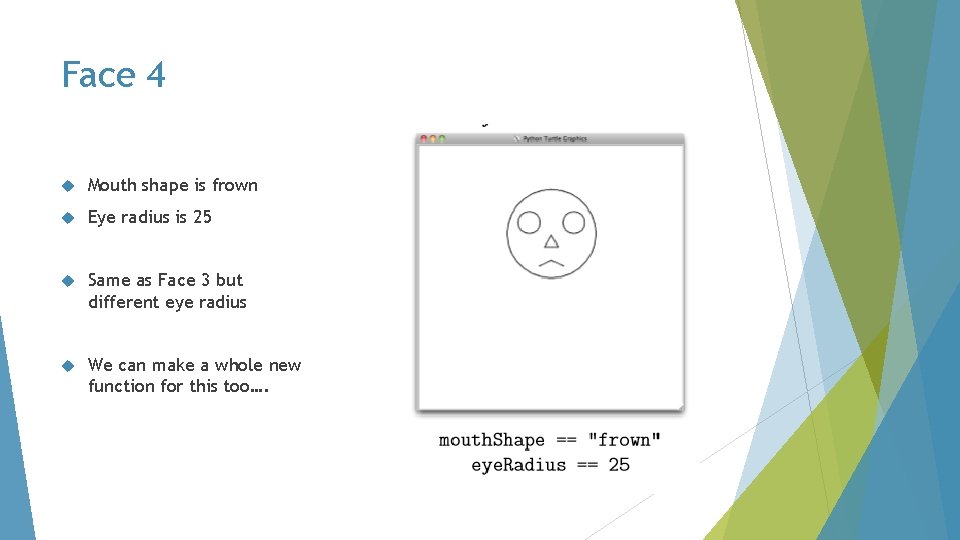
Face 4 Mouth shape is frown Eye radius is 25 Same as Face 3 but different eye radius We can make a whole new function for this too….
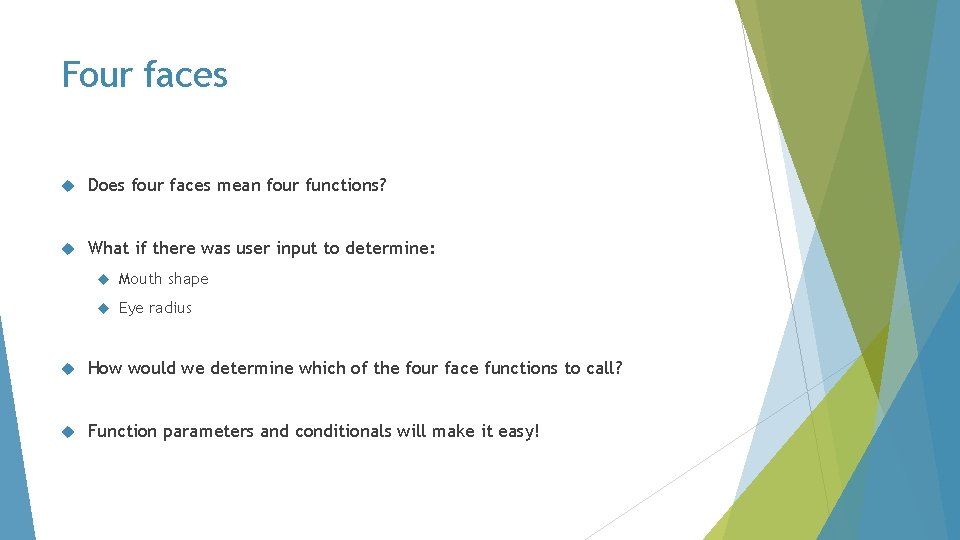
Four faces Does four faces mean four functions? What if there was user input to determine: Mouth shape Eye radius How would we determine which of the four face functions to call? Function parameters and conditionals will make it easy!
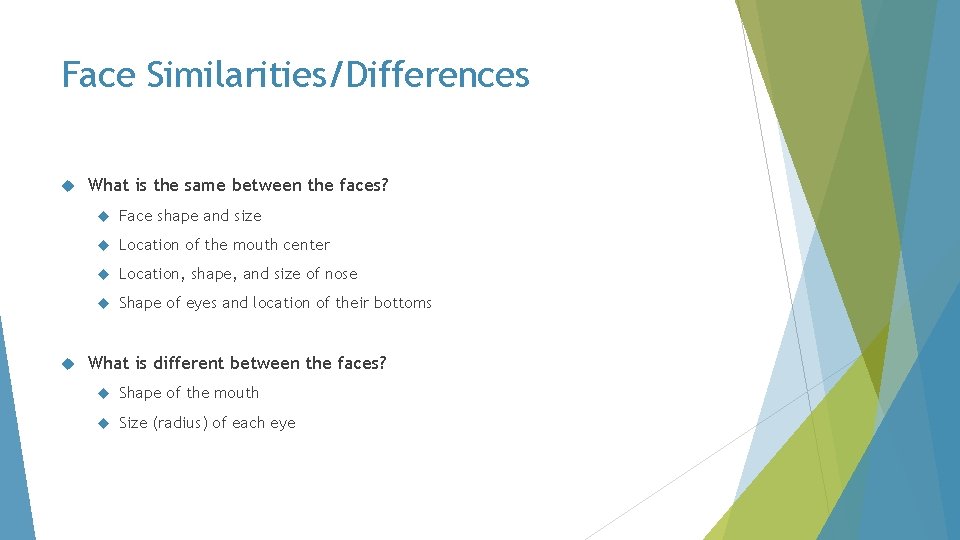
Face Similarities/Differences What is the same between the faces? Face shape and size Location of the mouth center Location, shape, and size of nose Shape of eyes and location of their bottoms What is different between the faces? Shape of the mouth Size (radius) of each eye
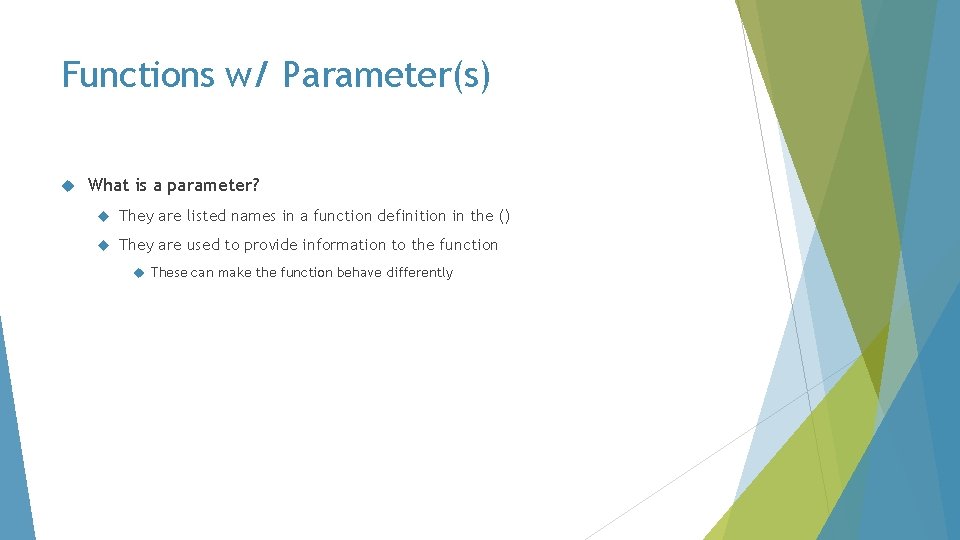
Functions w/ Parameter(s) What is a parameter? They are listed names in a function definition in the () They are used to provide information to the function These can make the function behave differently
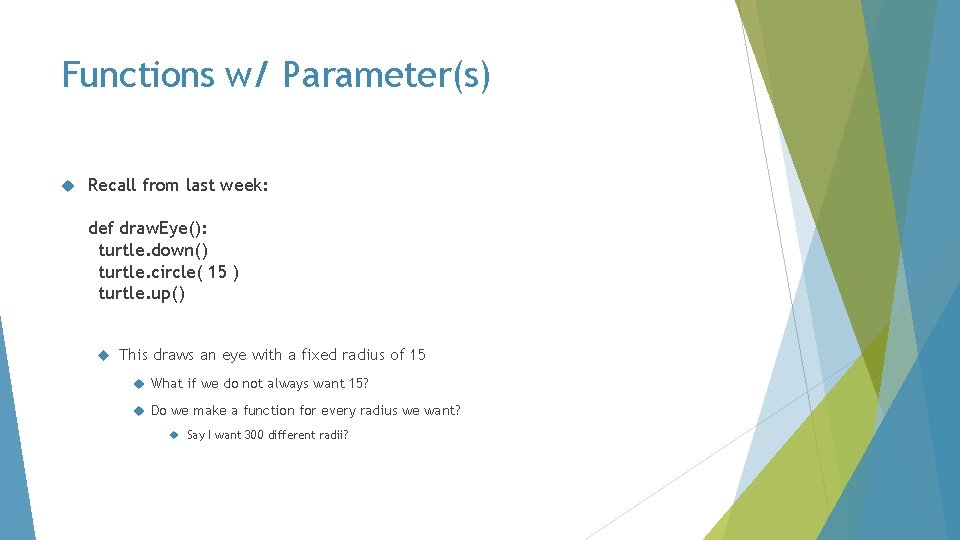
Functions w/ Parameter(s) Recall from last week: def draw. Eye(): turtle. down() turtle. circle( 15 ) turtle. up() This draws an eye with a fixed radius of 15 What if we do not always want 15? Do we make a function for every radius we want? Say I want 300 different radii?
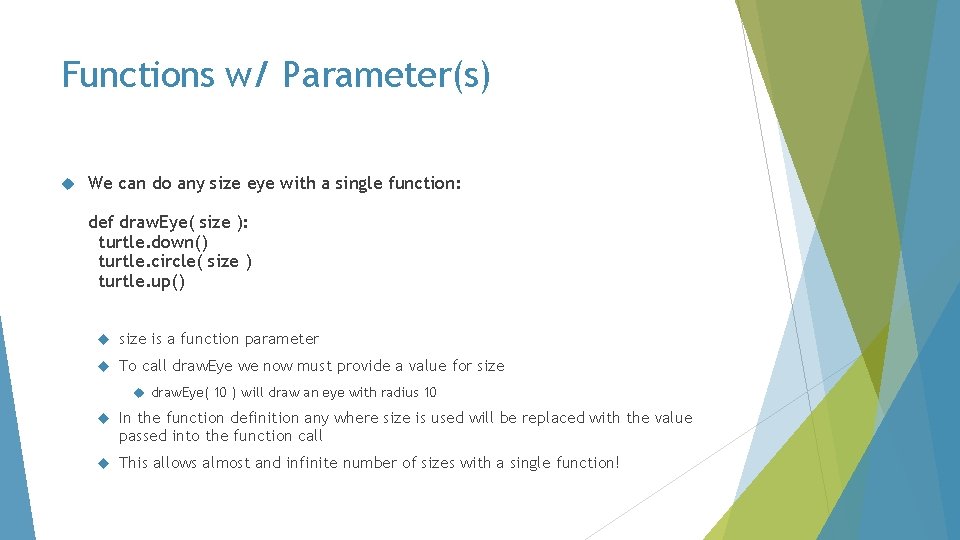
Functions w/ Parameter(s) We can do any size eye with a single function: def draw. Eye( size ): turtle. down() turtle. circle( size ) turtle. up() size is a function parameter To call draw. Eye we now must provide a value for size draw. Eye( 10 ) will draw an eye with radius 10 In the function definition any where size is used will be replaced with the value passed into the function call This allows almost and infinite number of sizes with a single function!
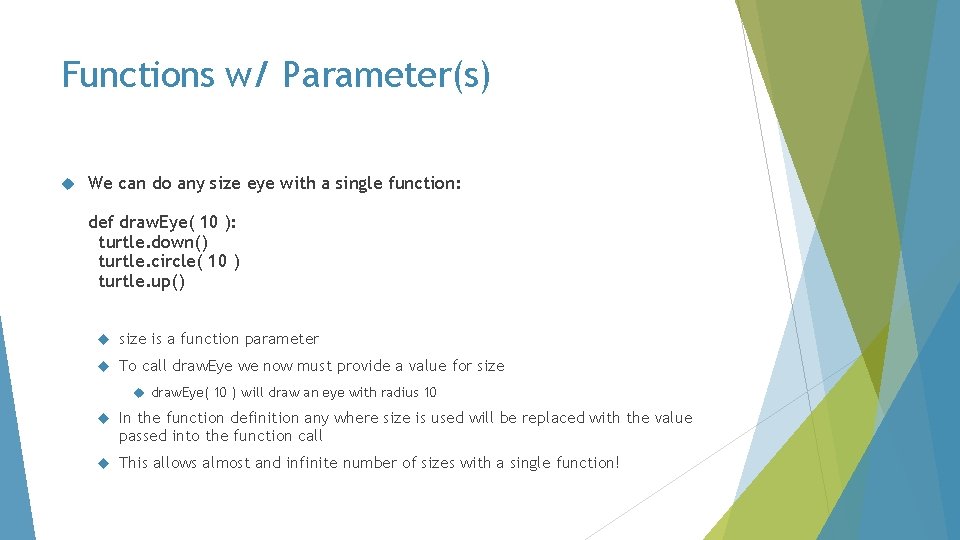
Functions w/ Parameter(s) We can do any size eye with a single function: def draw. Eye( 10 ): turtle. down() turtle. circle( 10 ) turtle. up() size is a function parameter To call draw. Eye we now must provide a value for size draw. Eye( 10 ) will draw an eye with radius 10 In the function definition any where size is used will be replaced with the value passed into the function call This allows almost and infinite number of sizes with a single function!
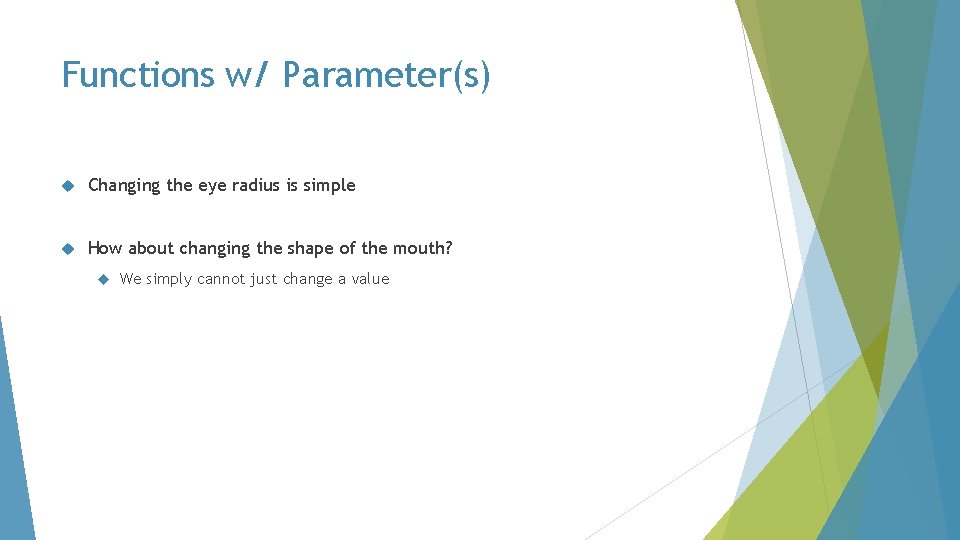
Functions w/ Parameter(s) Changing the eye radius is simple How about changing the shape of the mouth? We simply cannot just change a value
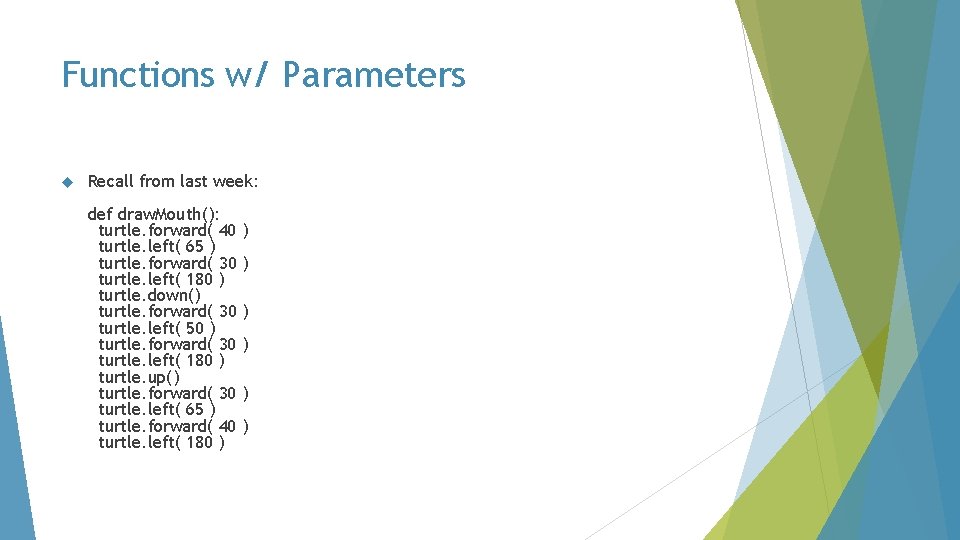
Functions w/ Parameters Recall from last week: def draw. Mouth(): turtle. forward( 40 turtle. left( 65 ) turtle. forward( 30 turtle. left( 180 ) turtle. down() turtle. forward( 30 turtle. left( 50 ) turtle. forward( 30 turtle. left( 180 ) turtle. up() turtle. forward( 30 turtle. left( 65 ) turtle. forward( 40 turtle. left( 180 ) ) ) )
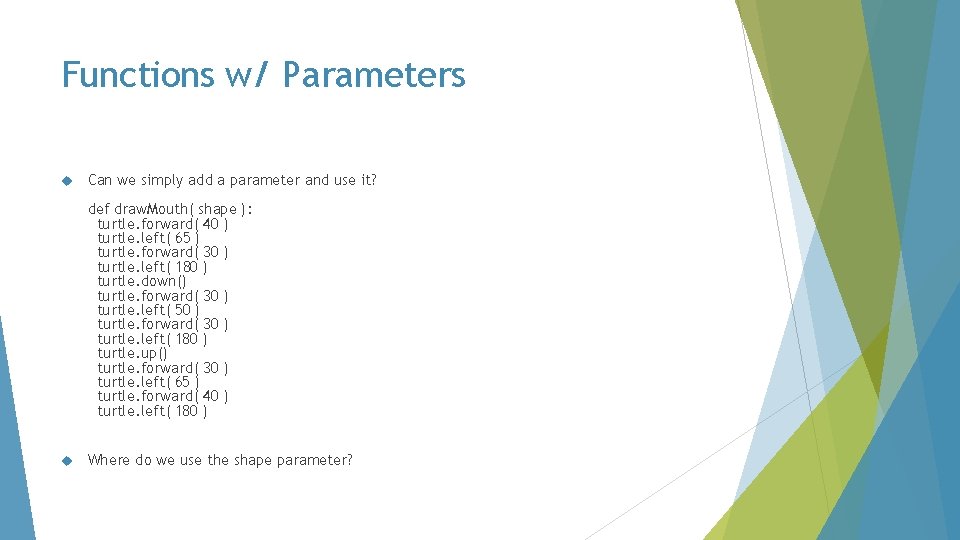
Functions w/ Parameters Can we simply add a parameter and use it? def draw. Mouth( shape ): turtle. forward( 40 ) turtle. left( 65 ) turtle. forward( 30 ) turtle. left( 180 ) turtle. down() turtle. forward( 30 ) turtle. left( 50 ) turtle. forward( 30 ) turtle. left( 180 ) turtle. up() turtle. forward( 30 ) turtle. left( 65 ) turtle. forward( 40 ) turtle. left( 180 ) Where do we use the shape parameter?
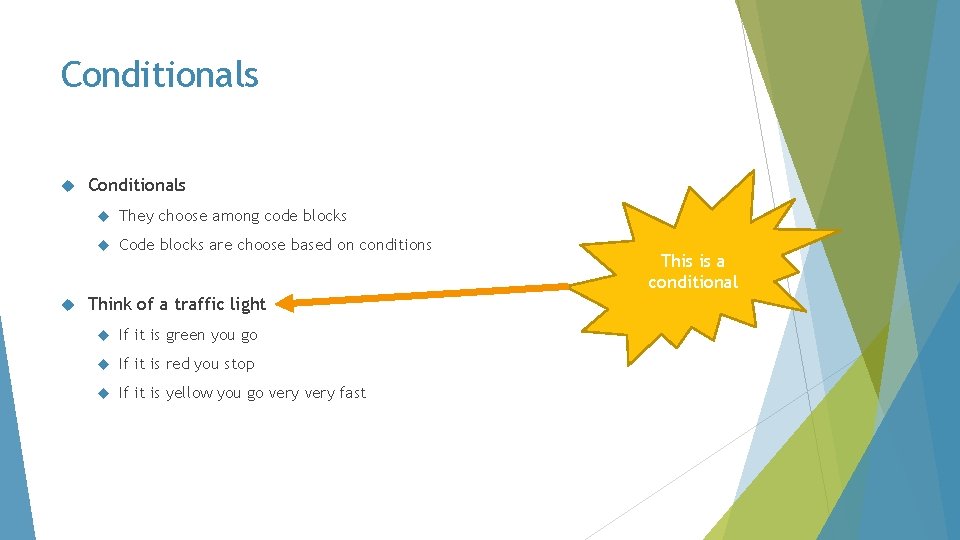
Conditionals They choose among code blocks Code blocks are choose based on conditions Think of a traffic light If it is green you go If it is red you stop If it is yellow you go very fast This is a conditional
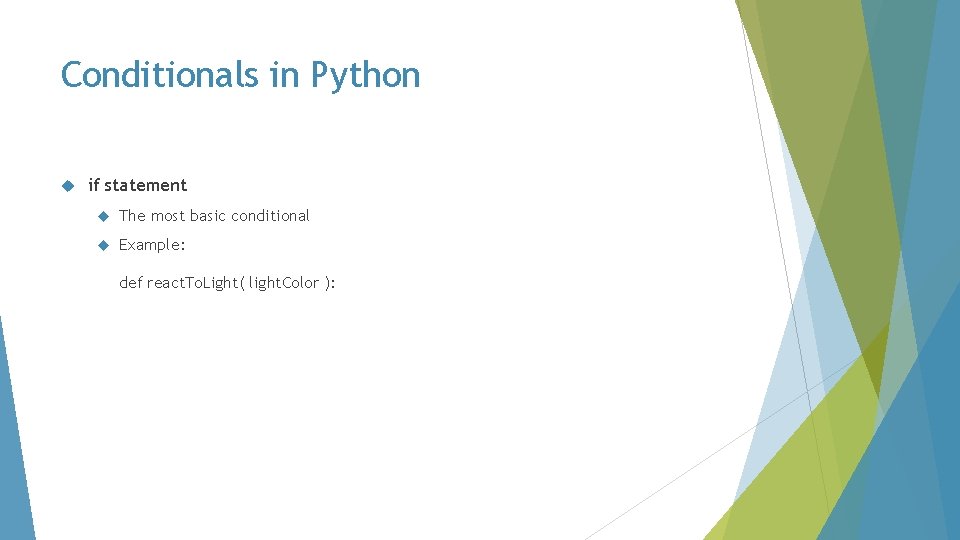
Conditionals in Python if statement The most basic conditional Example: def react. To. Light( light. Color ):
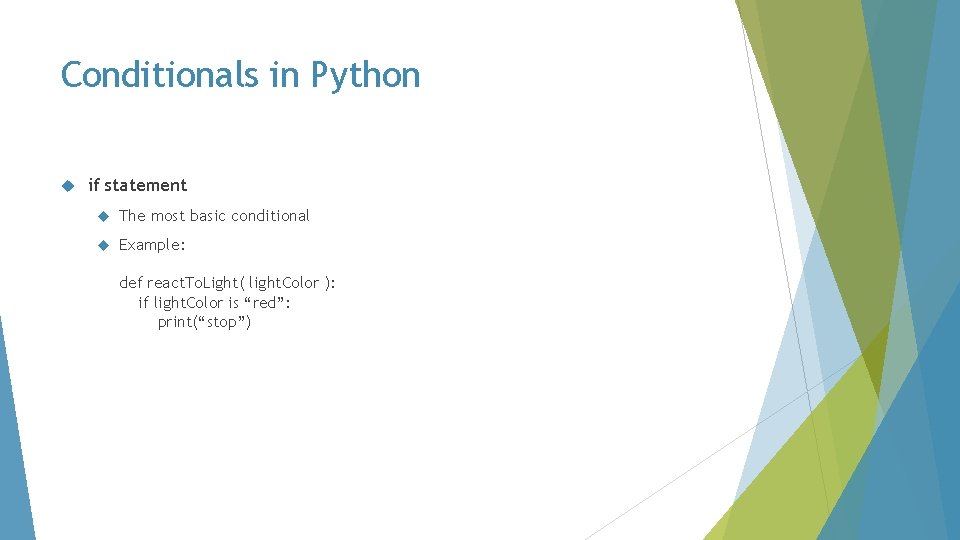
Conditionals in Python if statement The most basic conditional Example: def react. To. Light( light. Color ): if light. Color is “red”: print(“stop”)
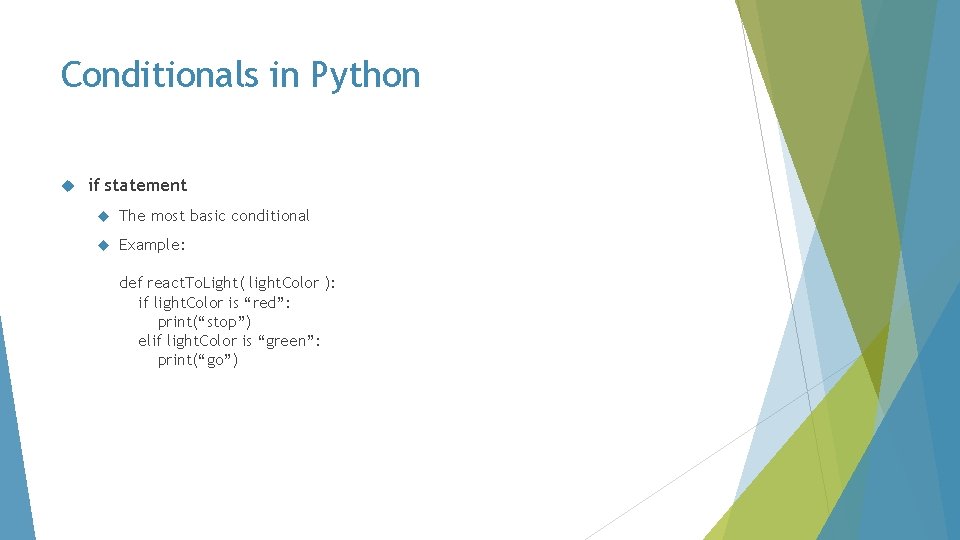
Conditionals in Python if statement The most basic conditional Example: def react. To. Light( light. Color ): if light. Color is “red”: print(“stop”) elif light. Color is “green”: print(“go”)
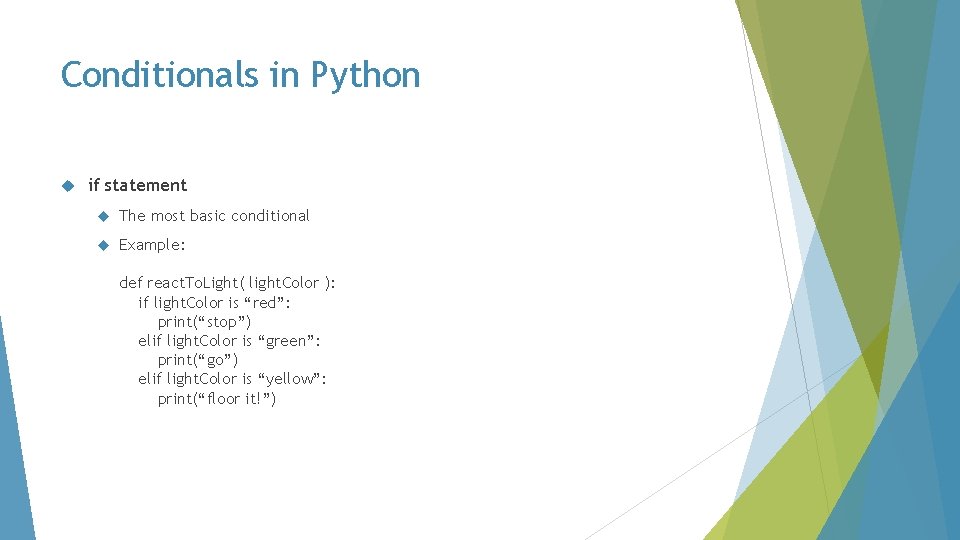
Conditionals in Python if statement The most basic conditional Example: def react. To. Light( light. Color ): if light. Color is “red”: print(“stop”) elif light. Color is “green”: print(“go”) elif light. Color is “yellow”: print(“floor it!”)
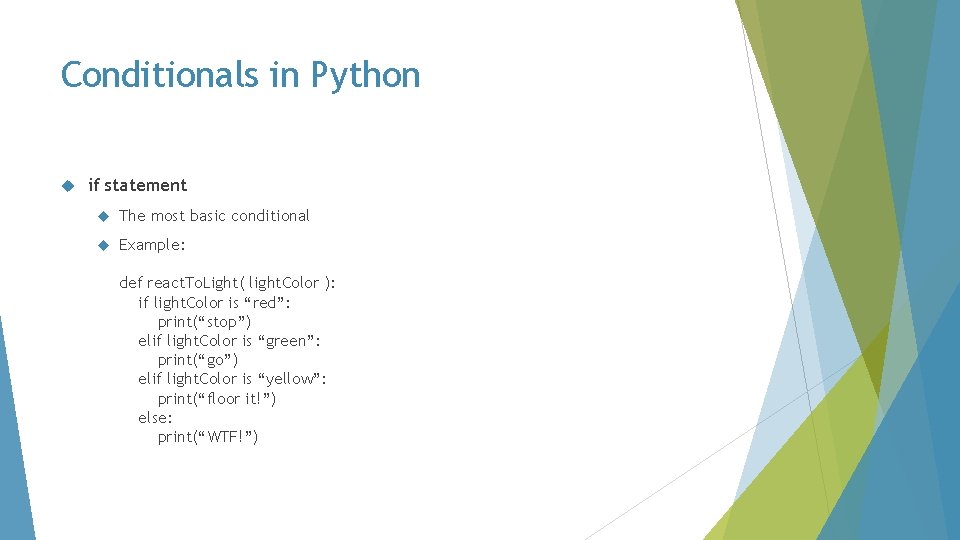
Conditionals in Python if statement The most basic conditional Example: def react. To. Light( light. Color ): if light. Color is “red”: print(“stop”) elif light. Color is “green”: print(“go”) elif light. Color is “yellow”: print(“floor it!”) else: print(“WTF!”)
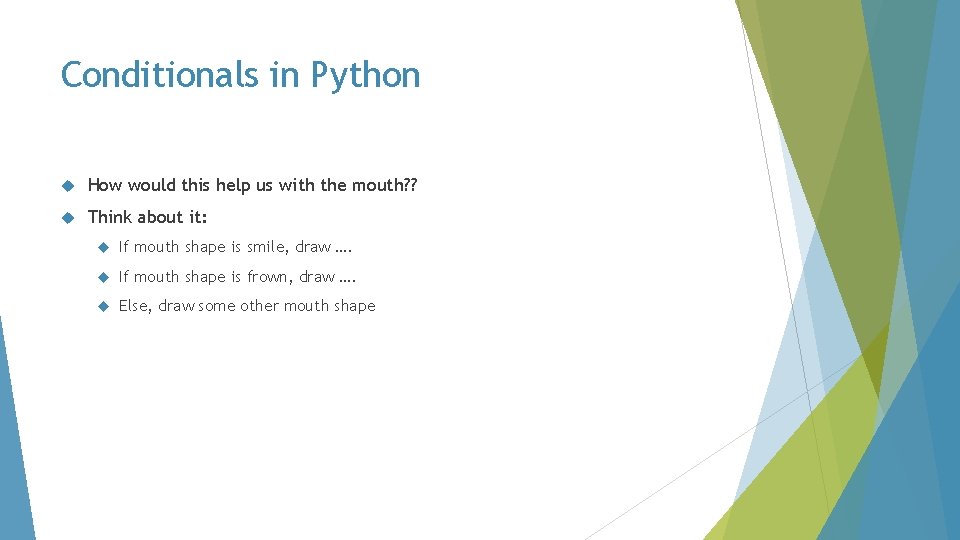
Conditionals in Python How would this help us with the mouth? ? Think about it: If mouth shape is smile, draw …. If mouth shape is frown, draw …. Else, draw some other mouth shape
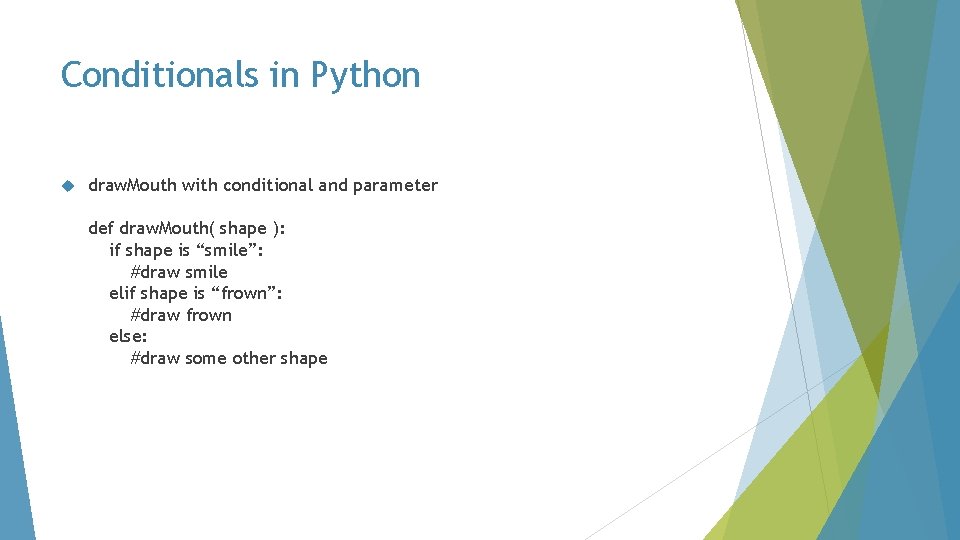
Conditionals in Python draw. Mouth with conditional and parameter def draw. Mouth( shape ): if shape is “smile”: #draw smile elif shape is “frown”: #draw frown else: #draw some other shape
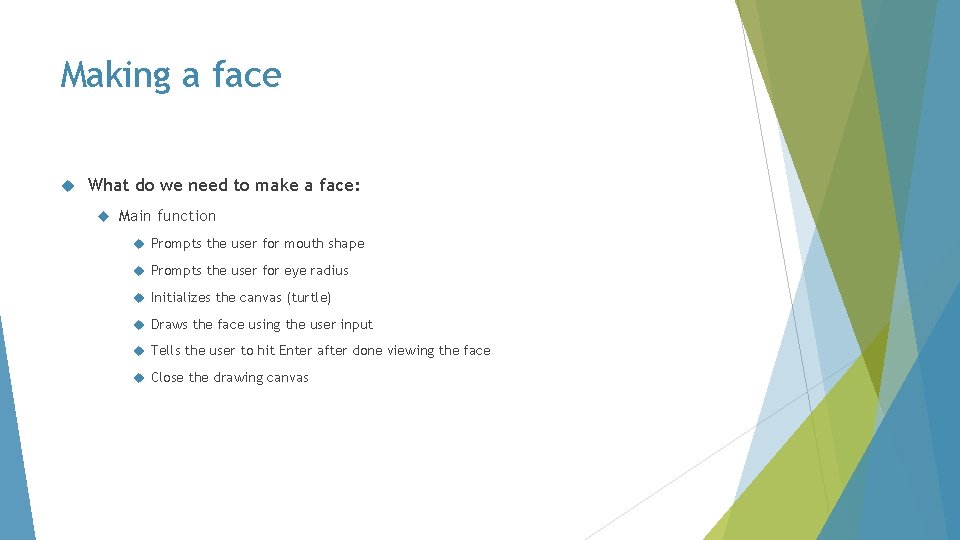
Making a face What do we need to make a face: Main function Prompts the user for mouth shape Prompts the user for eye radius Initializes the canvas (turtle) Draws the face using the user input Tells the user to hit Enter after done viewing the face Close the drawing canvas
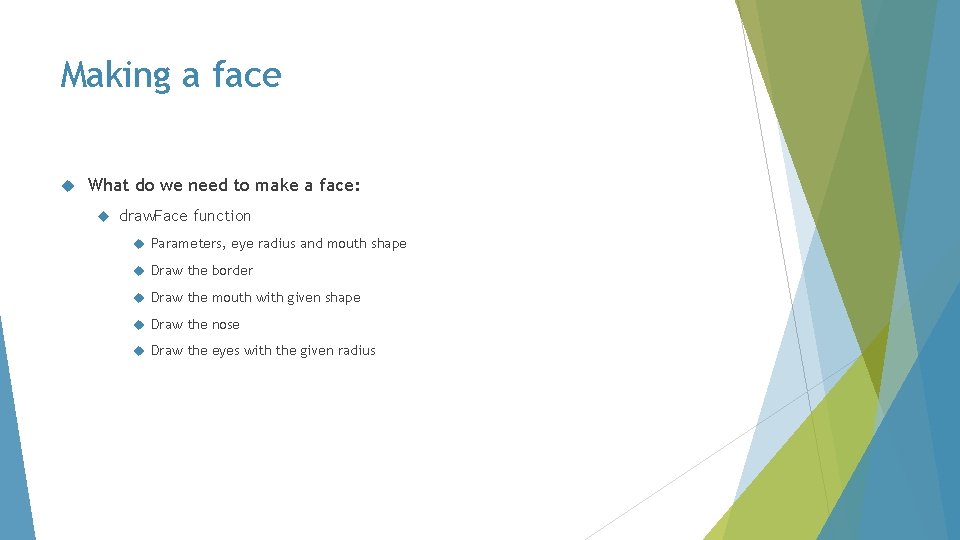
Making a face What do we need to make a face: draw. Face function Parameters, eye radius and mouth shape Draw the border Draw the mouth with given shape Draw the nose Draw the eyes with the given radius
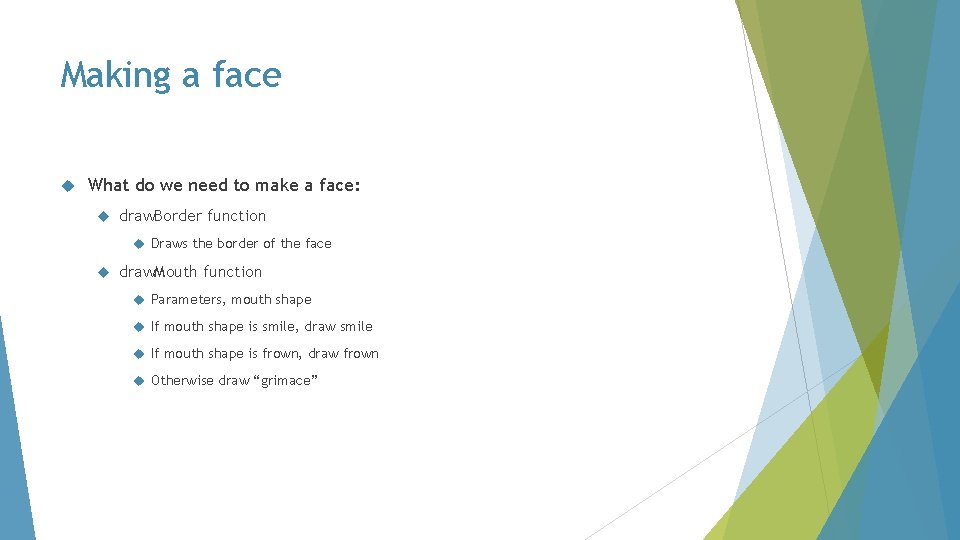
Making a face What do we need to make a face: draw. Border function Draws the border of the face draw. Mouth function Parameters, mouth shape If mouth shape is smile, draw smile If mouth shape is frown, draw frown Otherwise draw “grimace”
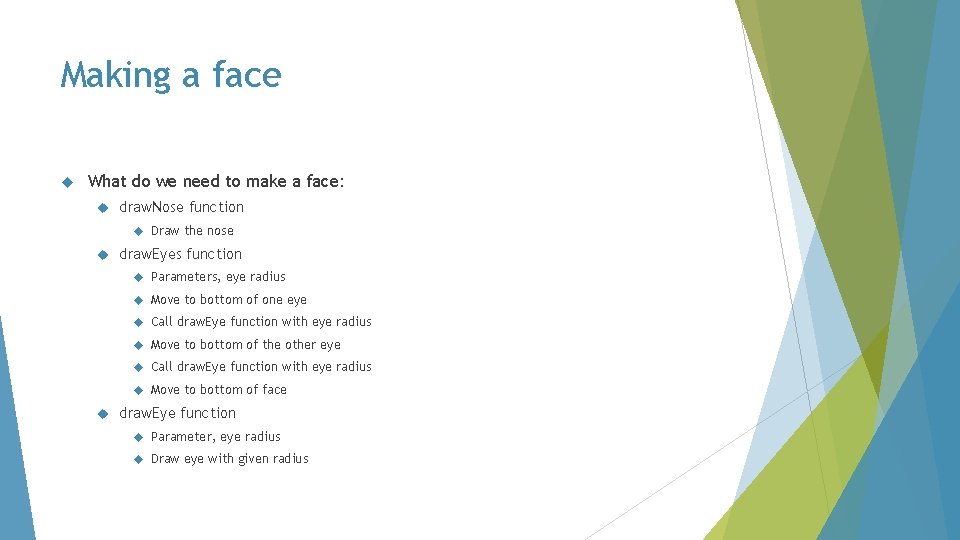
Making a face What do we need to make a face: draw. Nose function Draw the nose draw. Eyes function Parameters, eye radius Move to bottom of one eye Call draw. Eye function with eye radius Move to bottom of the other eye Call draw. Eye function with eye radius Move to bottom of face draw. Eye function Parameter, eye radius Draw eye with given radius
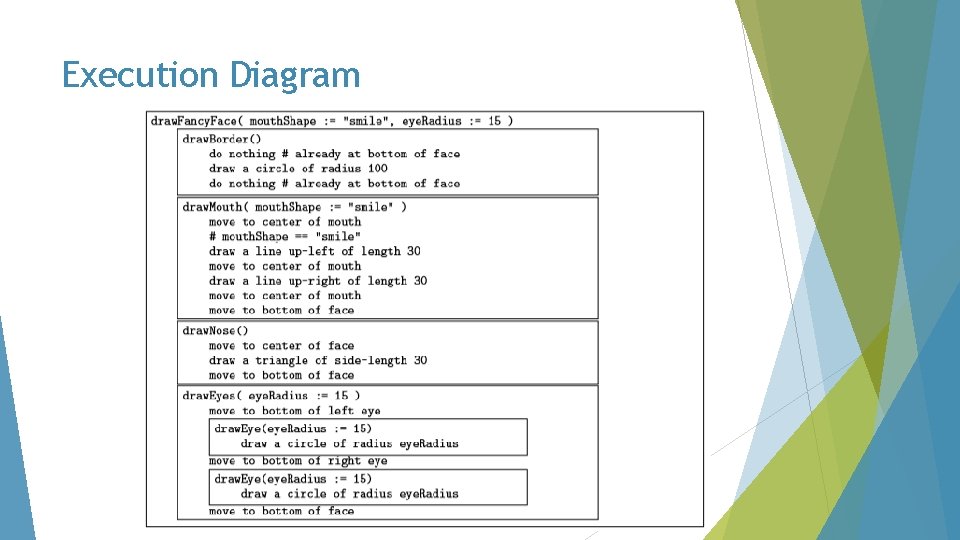
Execution Diagram
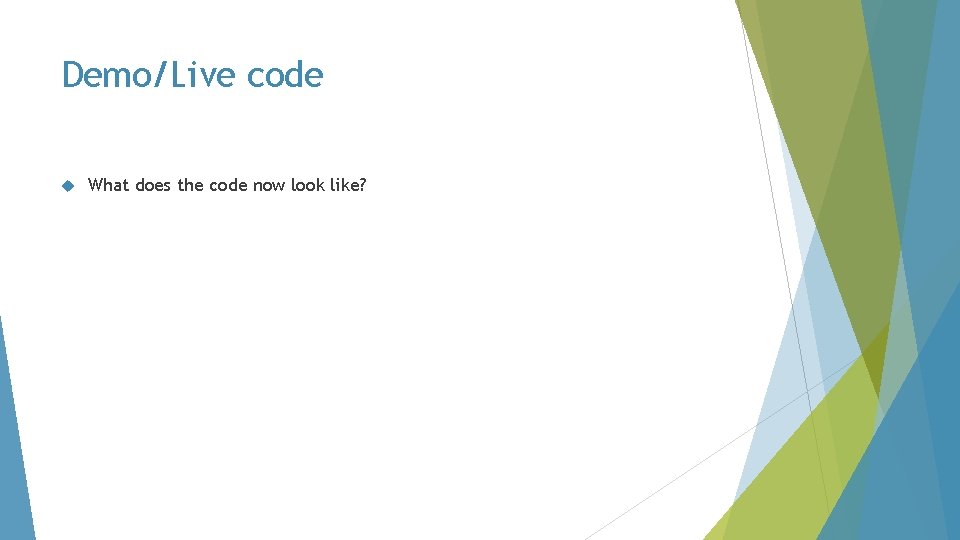
Demo/Live code What does the code now look like?
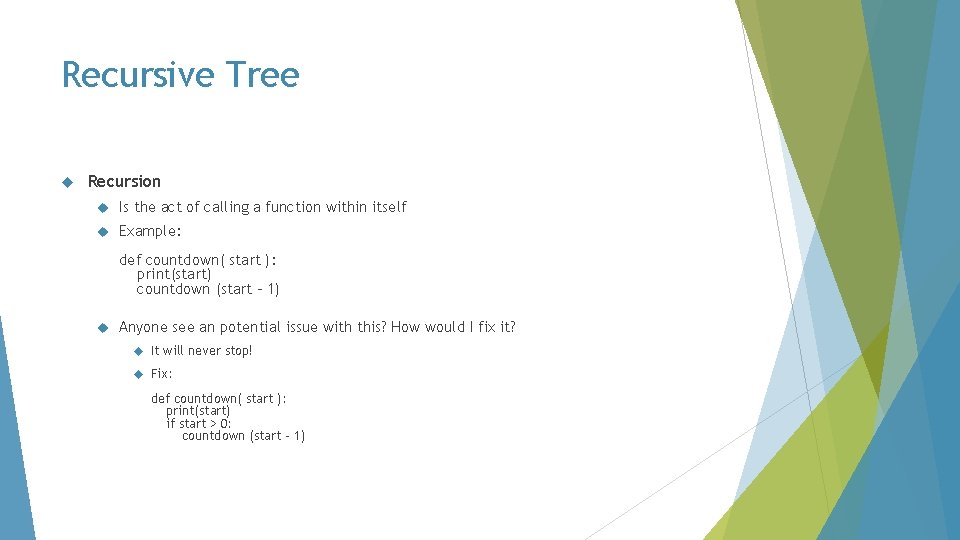
Recursive Tree Recursion Is the act of calling a function within itself Example: def countdown( start ): print(start) countdown (start – 1) Anyone see an potential issue with this? How would I fix it? It will never stop! Fix: def countdown( start ): print(start) if start > 0: countdown (start – 1)
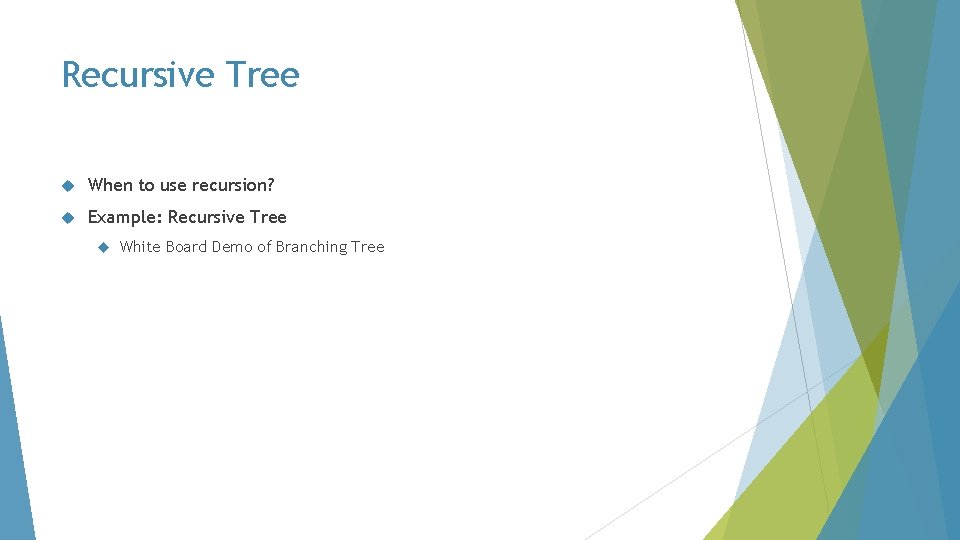
Recursive Tree When to use recursion? Example: Recursive Tree White Board Demo of Branching Tree
Fancy face definition
Summarize the general plan for non-recursive algorithms.
Week by week plans for documenting children's development
Comp550
How to read literature like a professor chapter 5
Do you fancy going for a drink
Us extra fancy
Ing
Fancy land
Her fancy was running riot along those days ahead of her
Politeness and interaction
Negative politeness examples
Hospice face to face template
2 elements of communication
Barbara cons
Negative face
I have one curved face and one flat face what am i
Example of a simile in romeo and juliet
Cis face and trans face
Myron b thompson academy
Face to face ecdl
First conditional grammar rules
Conditionals past perfect
Conditional probability tree diagram
Gene tree vs phylogenetic tree
Difference between plus tree and elite tree
Complete and full binary tree
Problem tree slides
Difference between general tree and binary tree
Winner tree loser tree
What are the advantages of a threaded binary tree?
Winner tree loser tree
Convert red black tree to 2-3-4