Streams Formatted output cout right setw2 i setw8
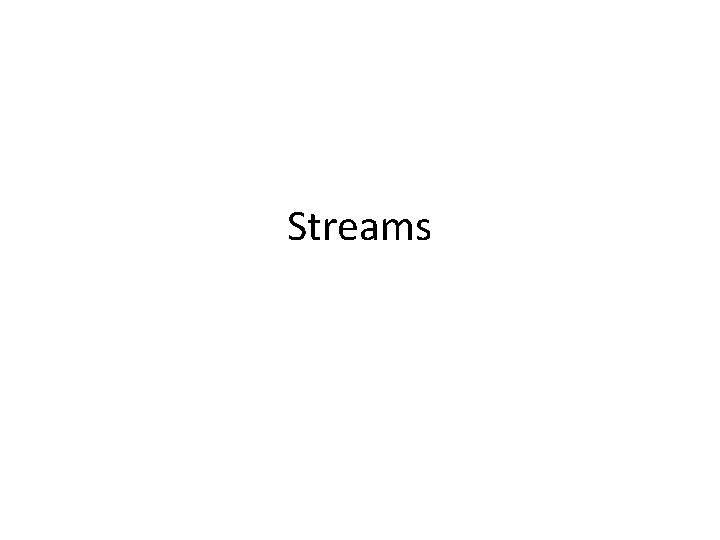
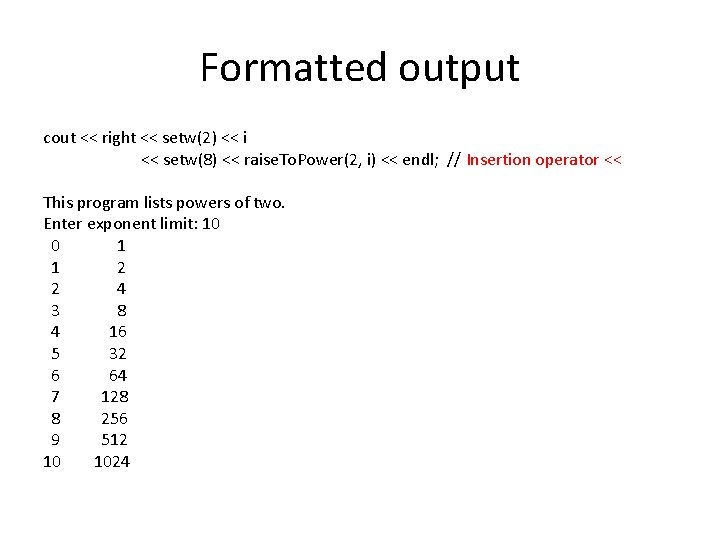
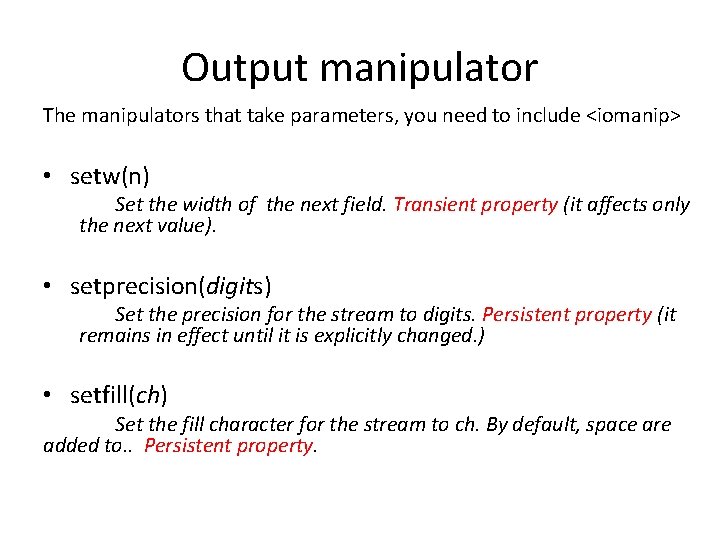
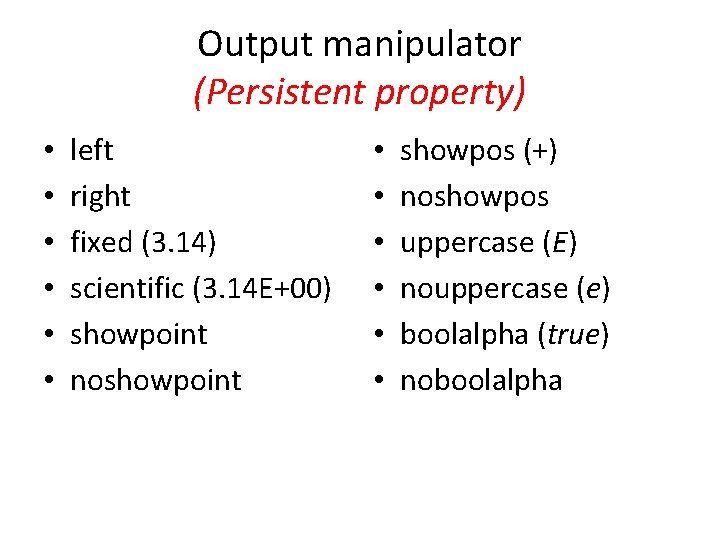
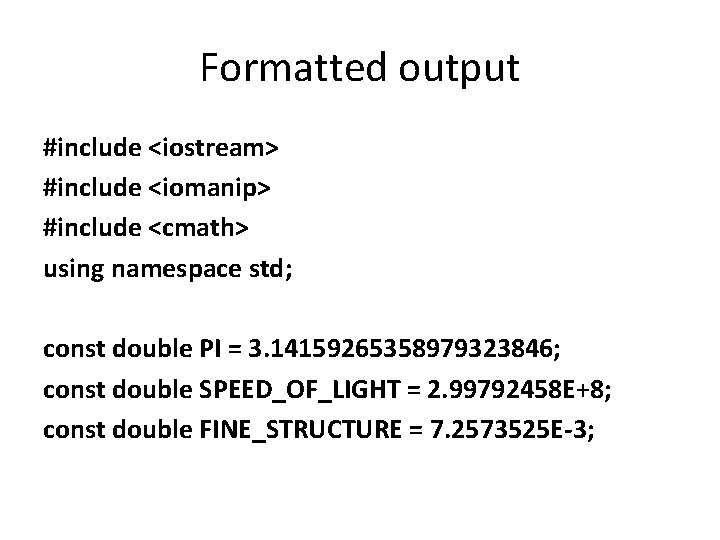
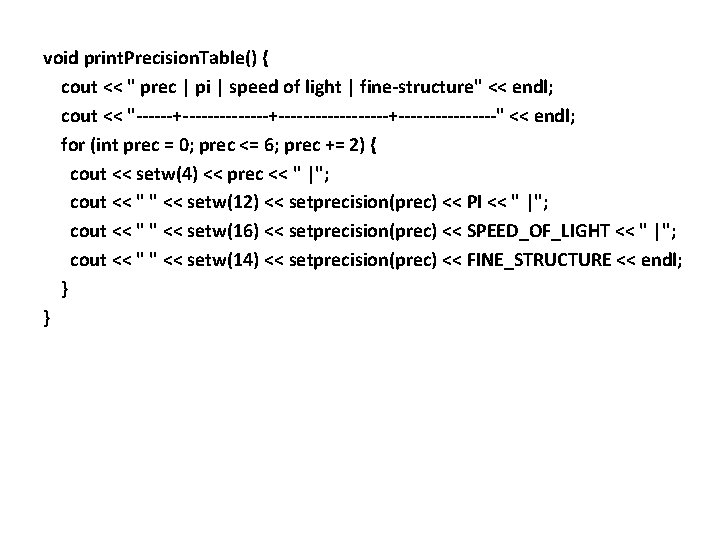
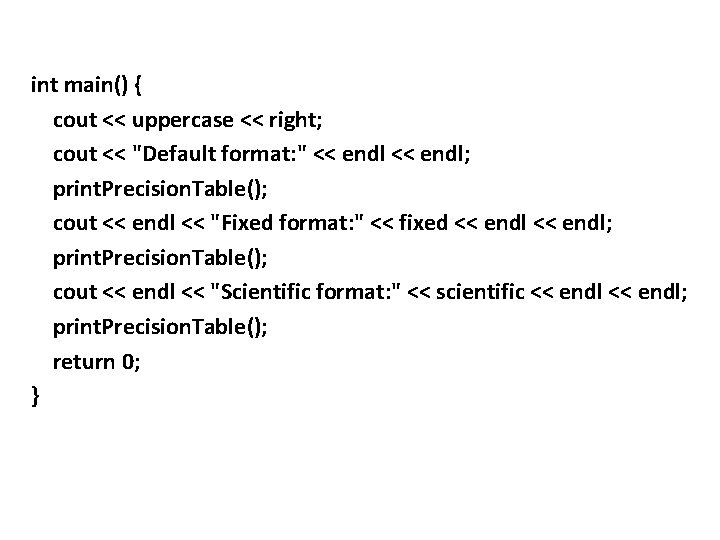
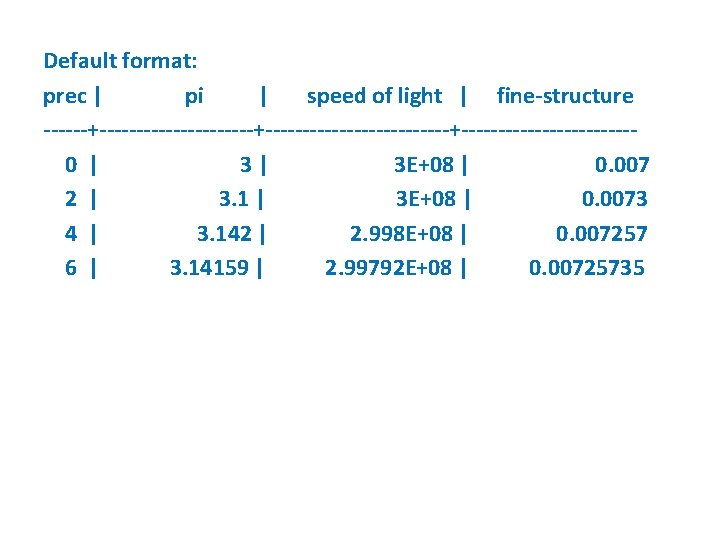
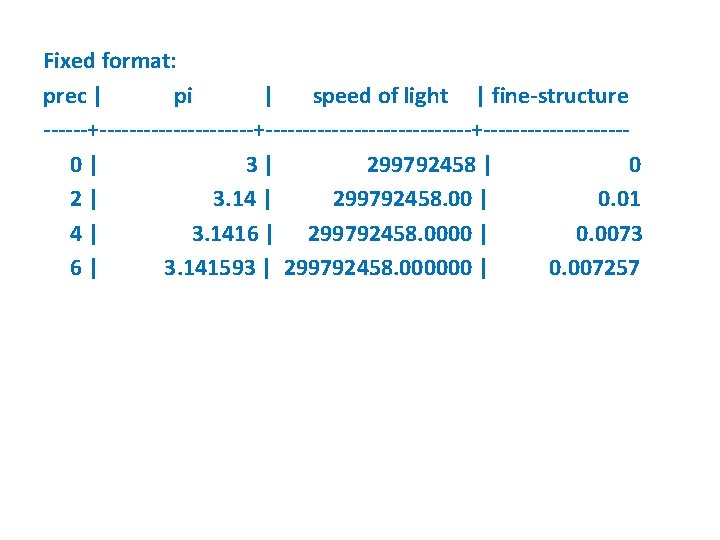
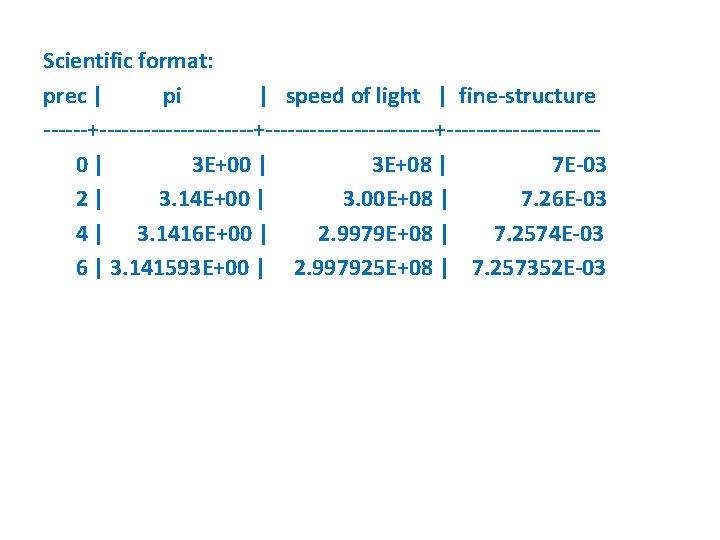
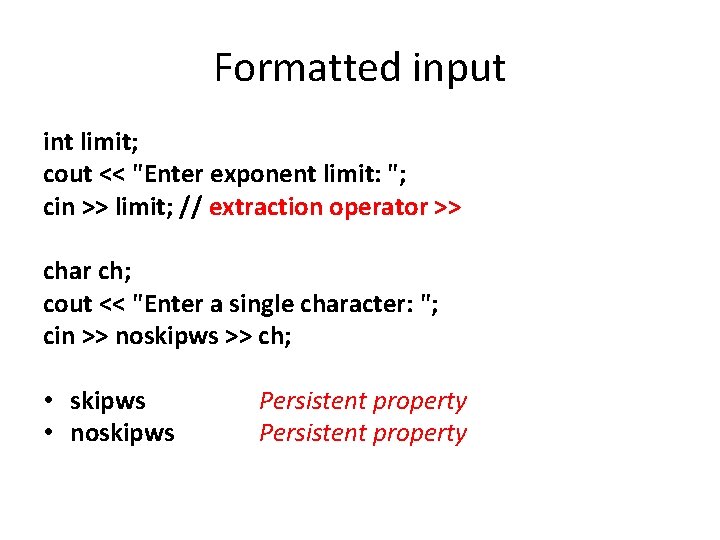
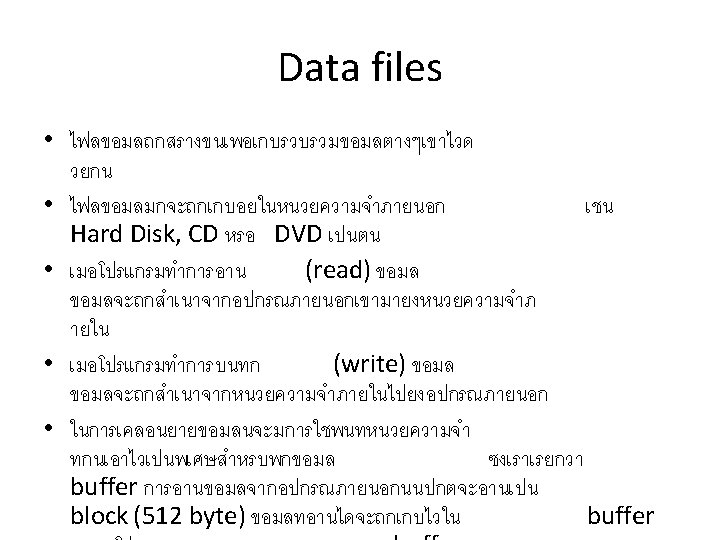
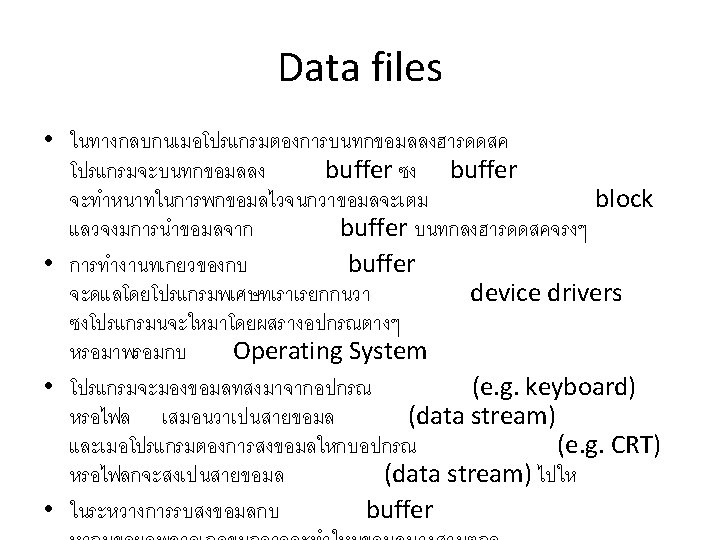
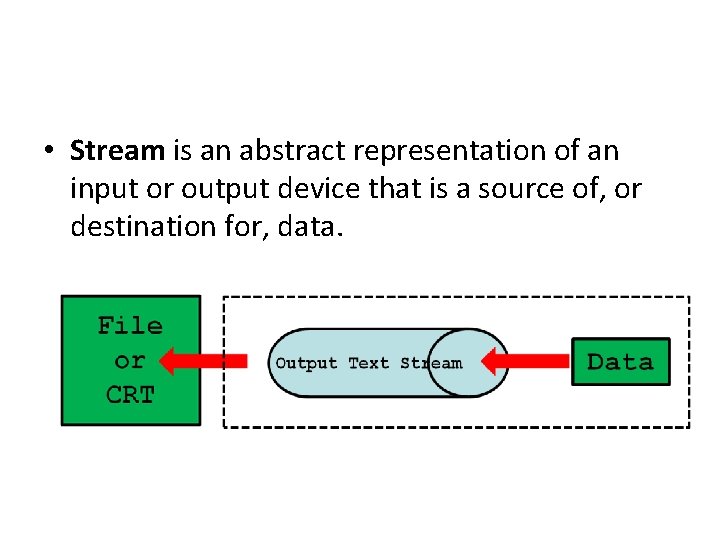
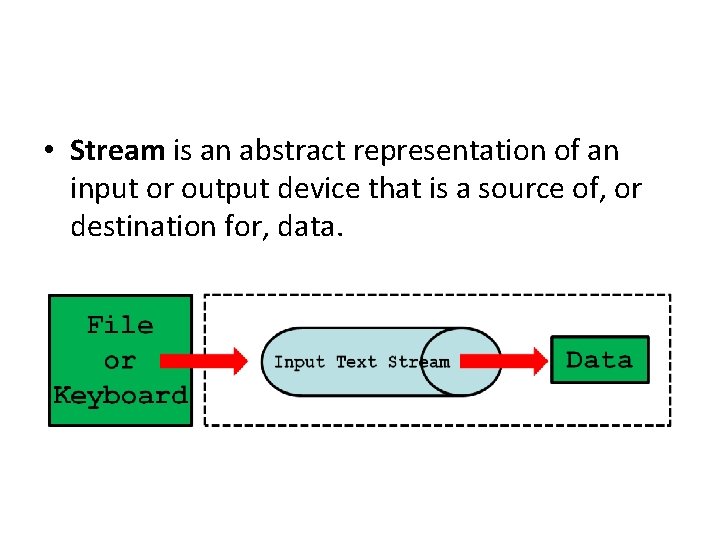
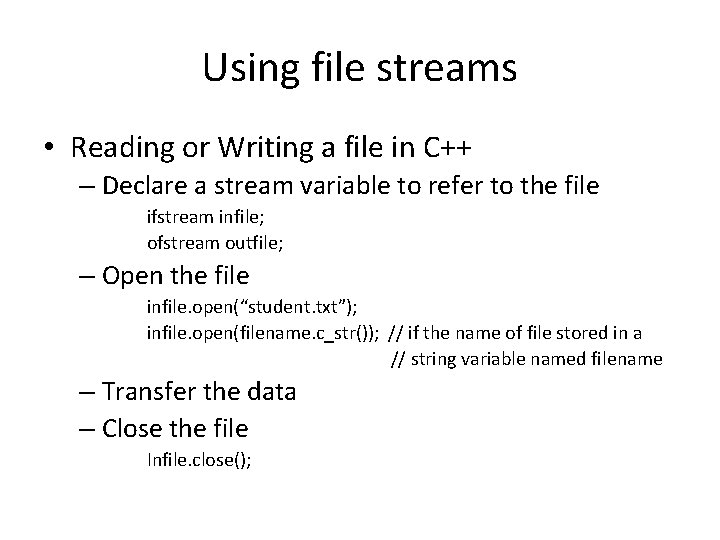
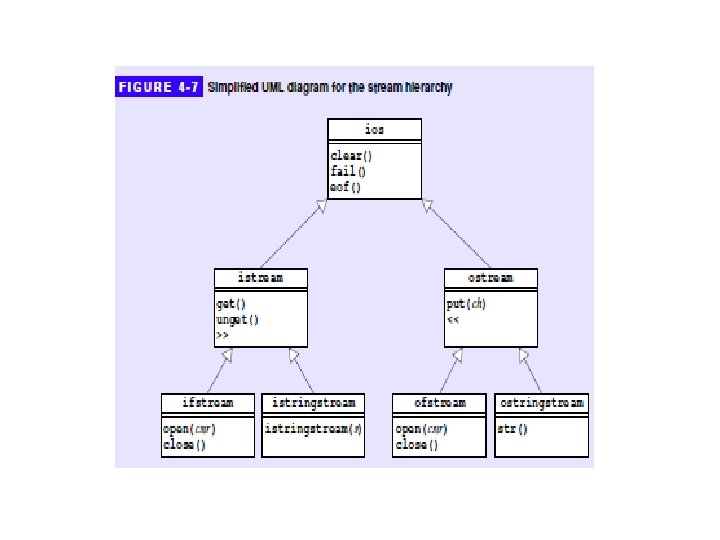
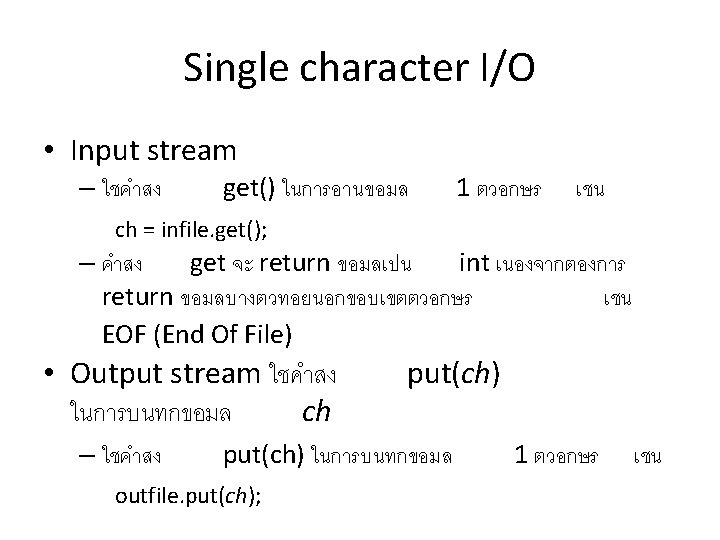
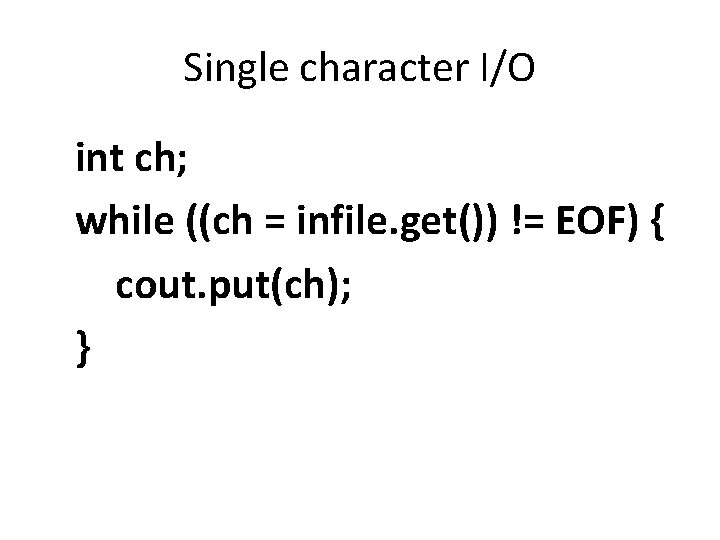
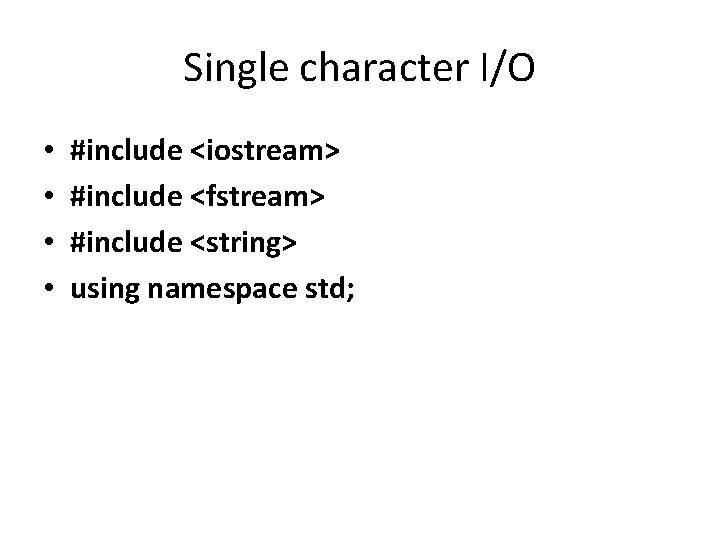
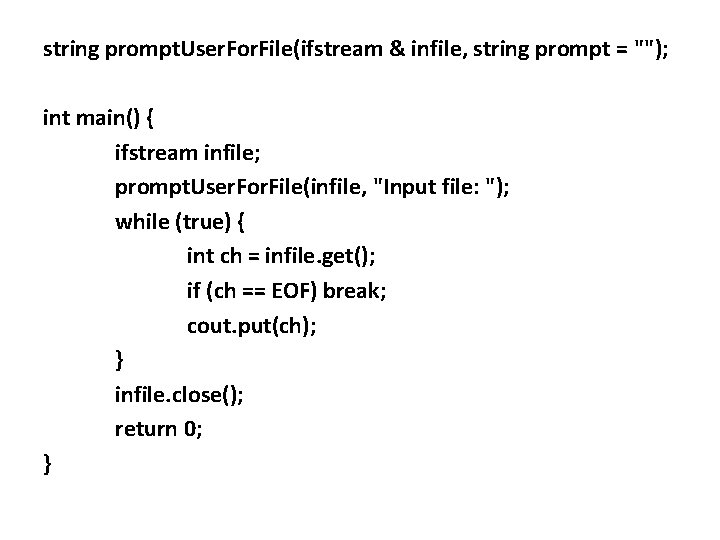
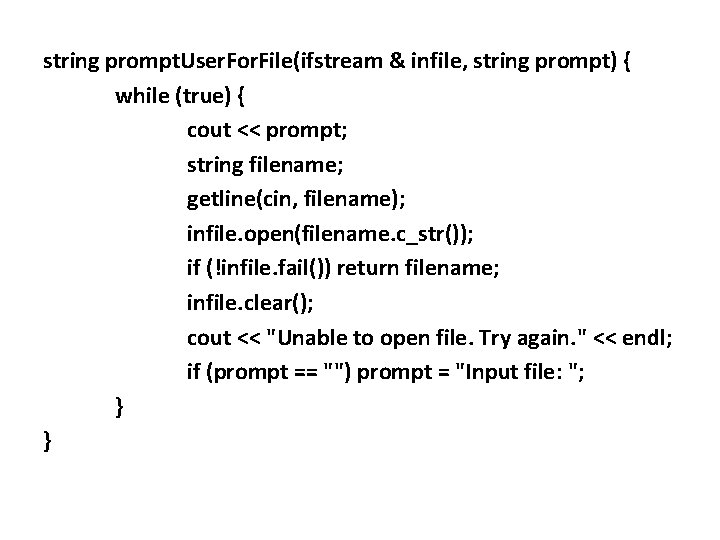
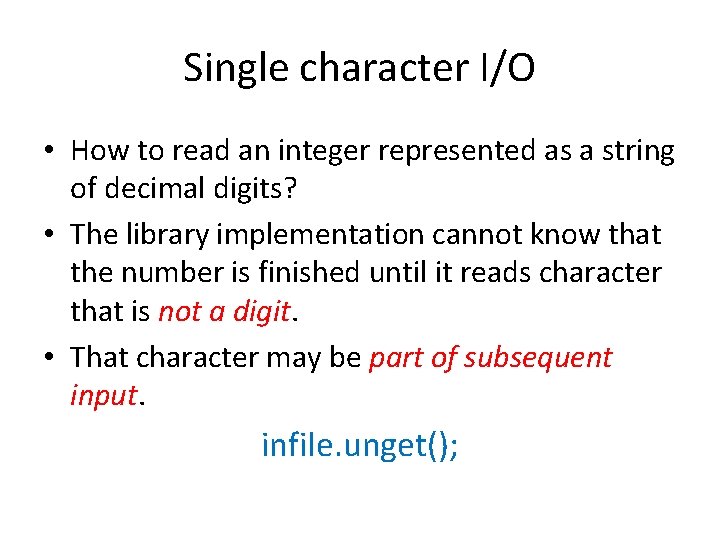
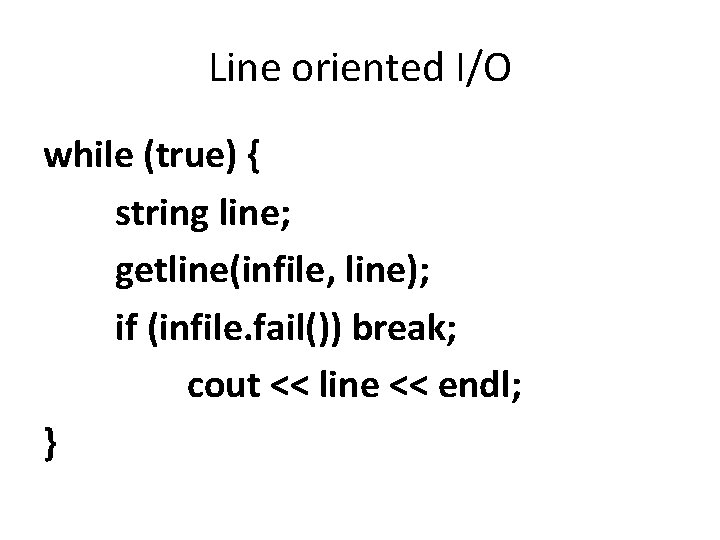
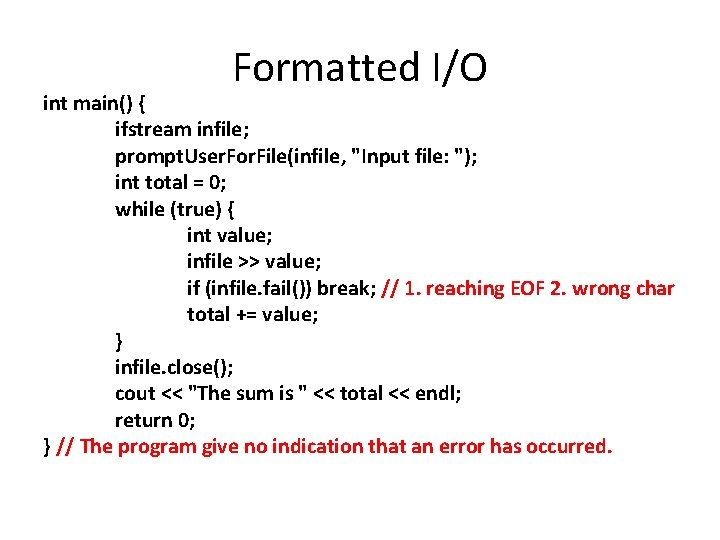
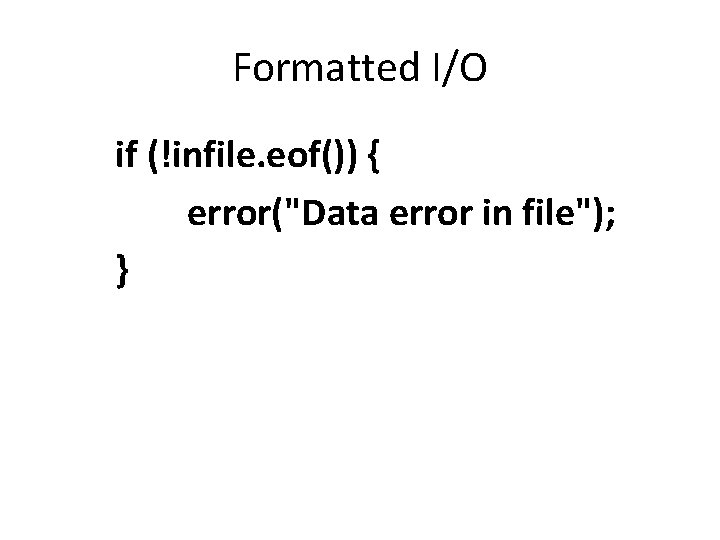
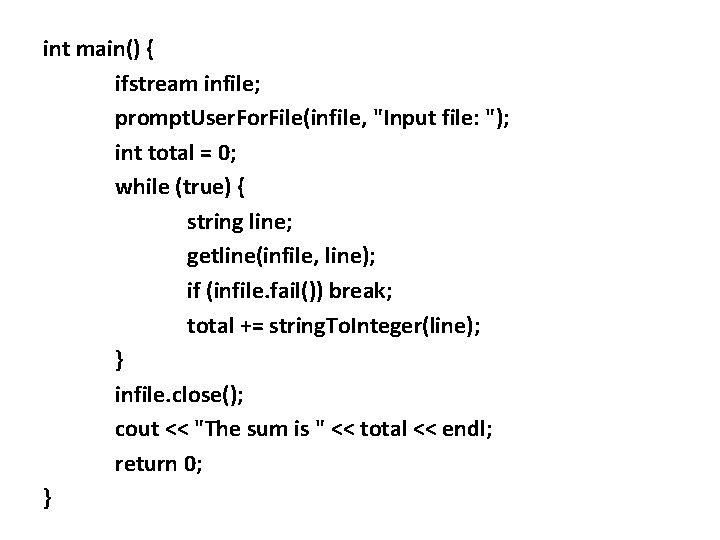
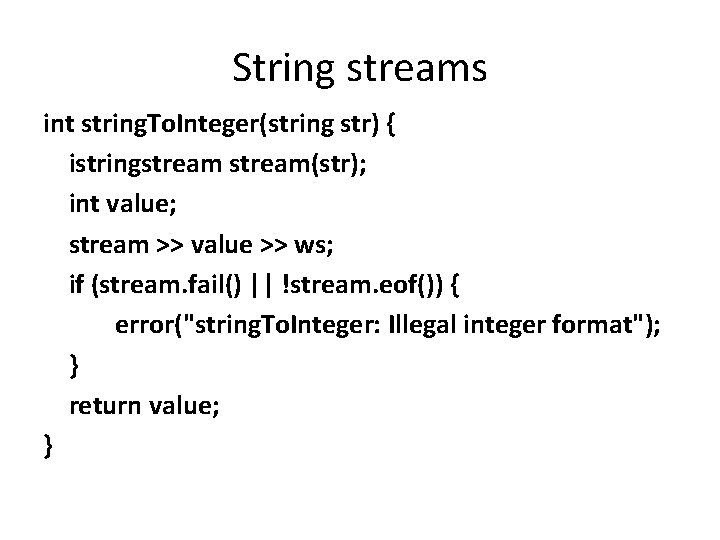
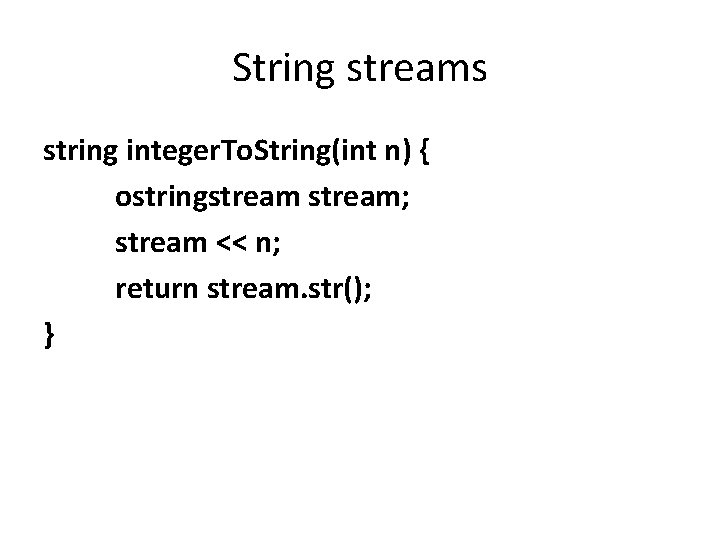
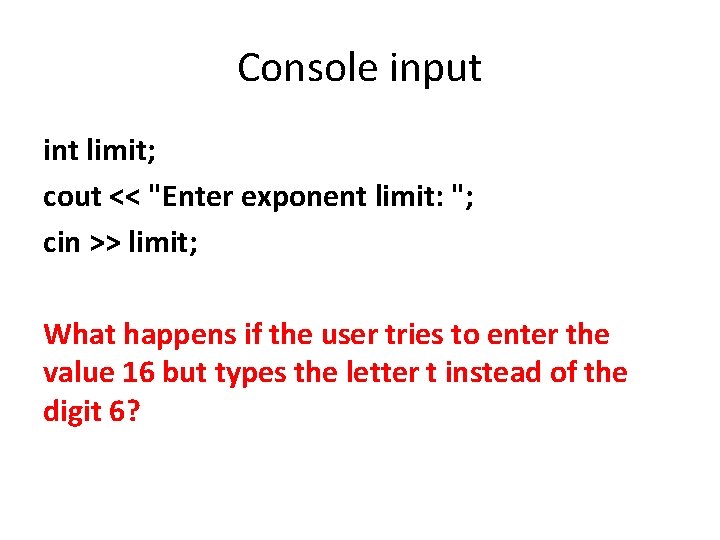
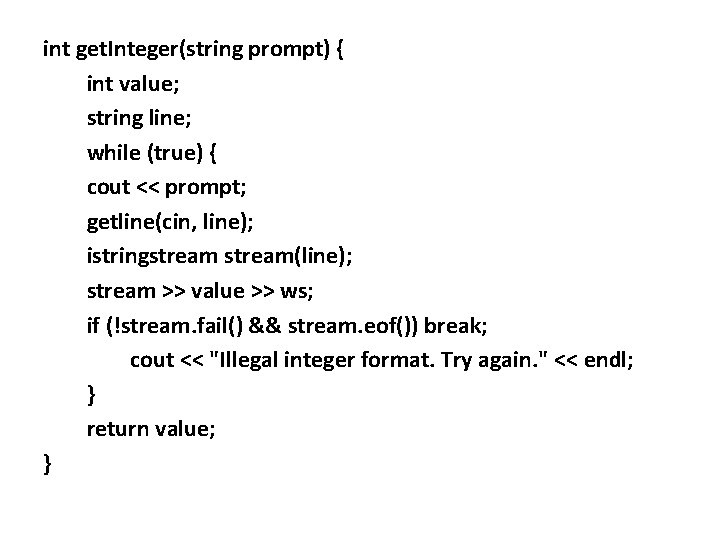
- Slides: 31
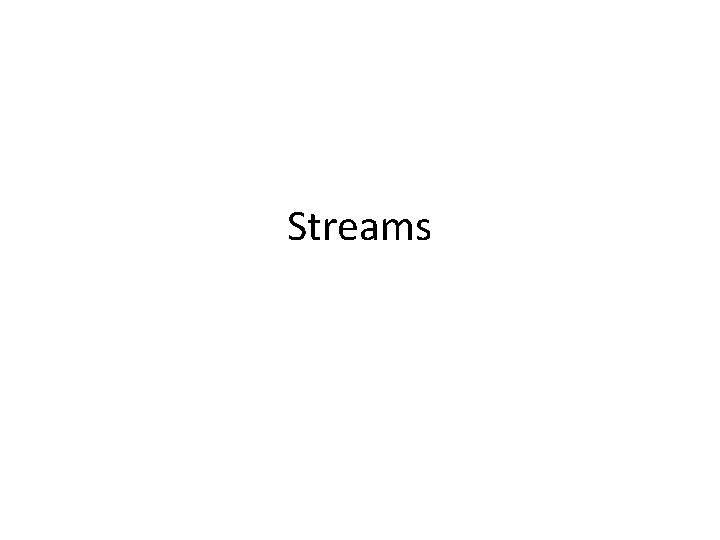
Streams
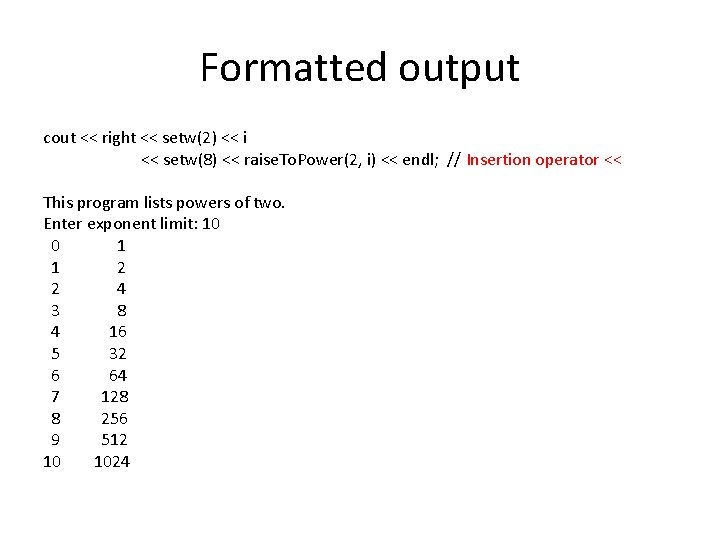
Formatted output cout << right << setw(2) << i << setw(8) << raise. To. Power(2, i) << endl; // Insertion operator << This program lists powers of two. Enter exponent limit: 10 0 1 1 2 2 4 3 8 4 16 5 32 6 64 7 128 8 256 9 512 10 1024
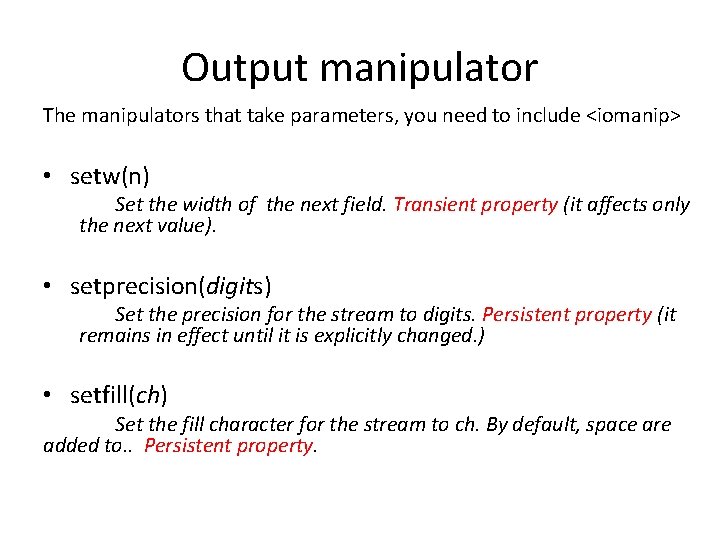
Output manipulator The manipulators that take parameters, you need to include <iomanip> • setw(n) Set the width of the next field. Transient property (it affects only the next value). • setprecision(digits) Set the precision for the stream to digits. Persistent property (it remains in effect until it is explicitly changed. ) • setfill(ch) Set the fill character for the stream to ch. By default, space are added to. . Persistent property.
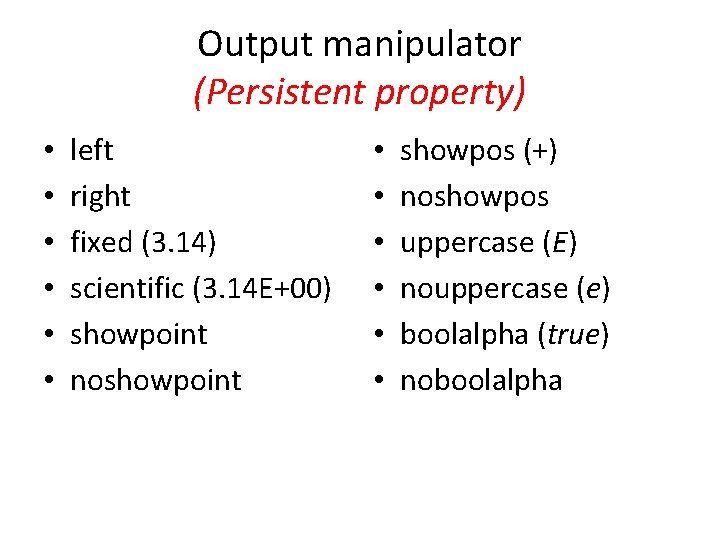
Output manipulator (Persistent property) • • • left right fixed (3. 14) scientific (3. 14 E+00) showpoint noshowpoint • • • showpos (+) noshowpos uppercase (E) nouppercase (e) boolalpha (true) noboolalpha
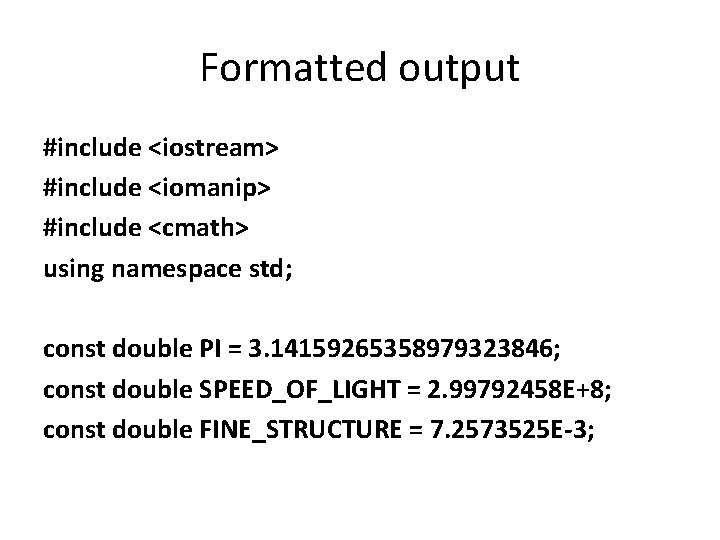
Formatted output #include <iostream> #include <iomanip> #include <cmath> using namespace std; const double PI = 3. 14159265358979323846; const double SPEED_OF_LIGHT = 2. 99792458 E+8; const double FINE_STRUCTURE = 7. 2573525 E-3;
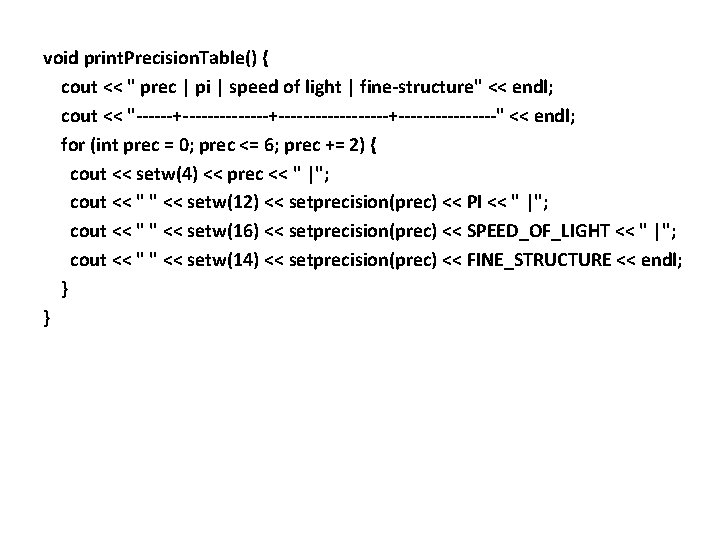
void print. Precision. Table() { cout << " prec | pi | speed of light | fine-structure" << endl; cout << "------+-------------+--------" << endl; for (int prec = 0; prec <= 6; prec += 2) { cout << setw(4) << prec << " |"; cout << " " << setw(12) << setprecision(prec) << PI << " |"; cout << " " << setw(16) << setprecision(prec) << SPEED_OF_LIGHT << " |"; cout << " " << setw(14) << setprecision(prec) << FINE_STRUCTURE << endl; } }
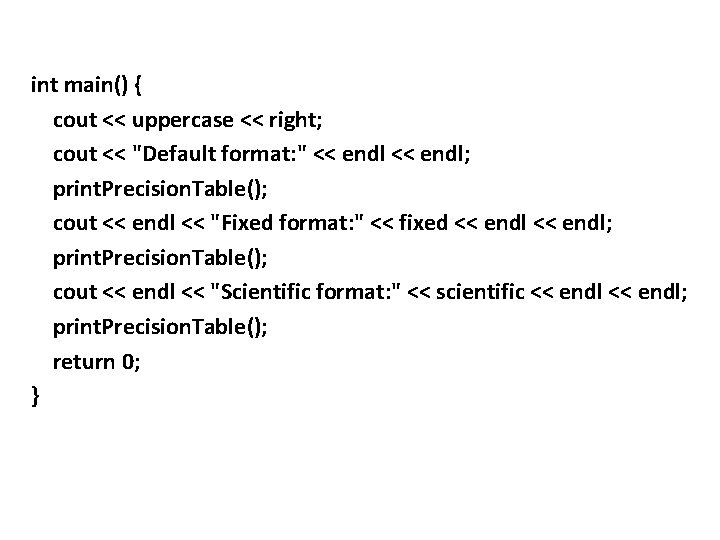
int main() { cout << uppercase << right; cout << "Default format: " << endl; print. Precision. Table(); cout << endl << "Fixed format: " << fixed << endl; print. Precision. Table(); cout << endl << "Scientific format: " << scientific << endl; print. Precision. Table(); return 0; }
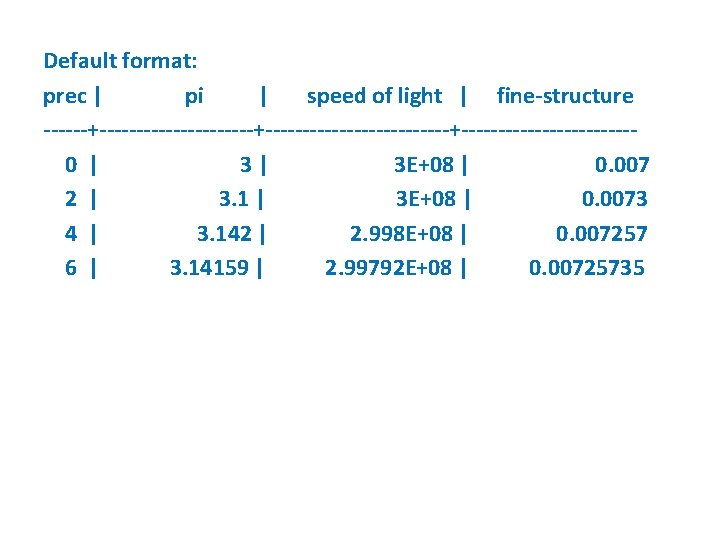
Default format: prec | pi | speed of light | fine-structure ------+--------------------+------------0 | 3| 3 E+08 | 0. 007 2 | 3. 1 | 3 E+08 | 0. 0073 4 | 3. 142 | 2. 998 E+08 | 0. 007257 6 | 3. 14159 | 2. 99792 E+08 | 0. 00725735
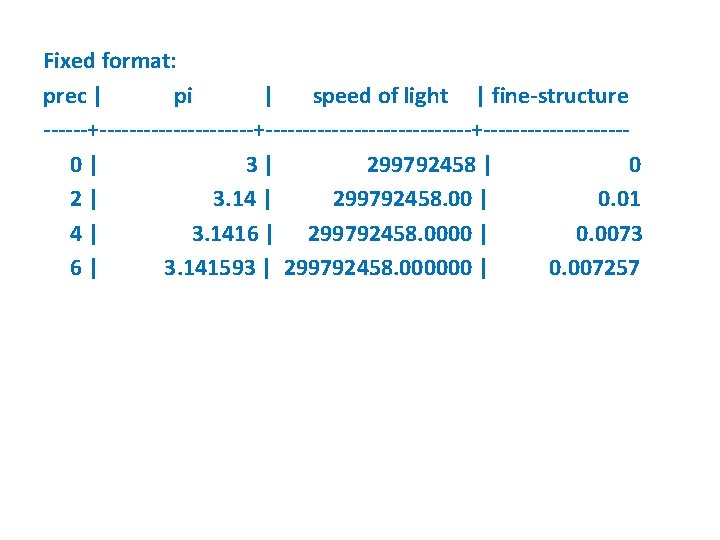
Fixed format: prec | pi | speed of light | fine-structure ------+----------------------+----------0| 3| 299792458 | 0 2| 3. 14 | 299792458. 00 | 0. 01 4| 3. 1416 | 299792458. 0000 | 0. 0073 6| 3. 141593 | 299792458. 000000 | 0. 007257
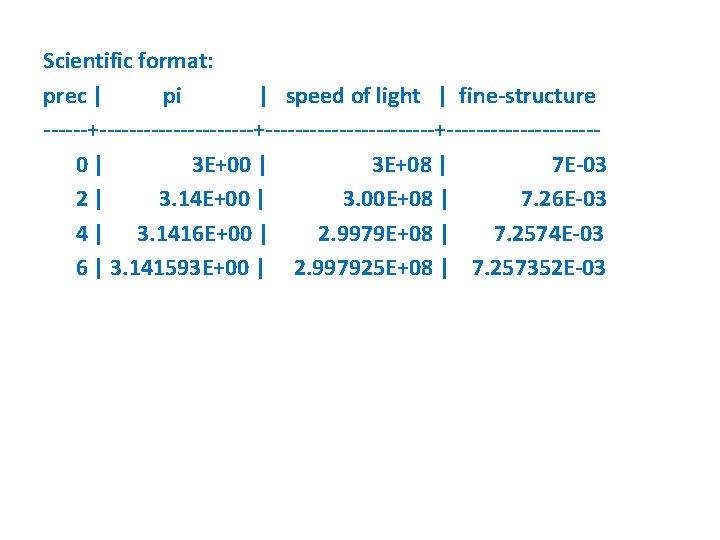
Scientific format: prec | pi | speed of light | fine-structure ------+-------------------+----------0| 3 E+00 | 3 E+08 | 7 E-03 2| 3. 14 E+00 | 3. 00 E+08 | 7. 26 E-03 4 | 3. 1416 E+00 | 2. 9979 E+08 | 7. 2574 E-03 6 | 3. 141593 E+00 | 2. 997925 E+08 | 7. 257352 E-03
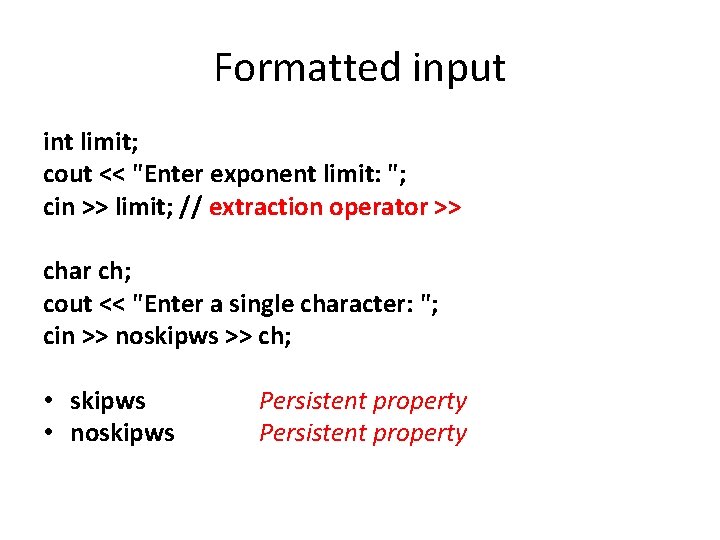
Formatted input int limit; cout << "Enter exponent limit: "; cin >> limit; // extraction operator >> char ch; cout << "Enter a single character: "; cin >> noskipws >> ch; • skipws • noskipws Persistent property
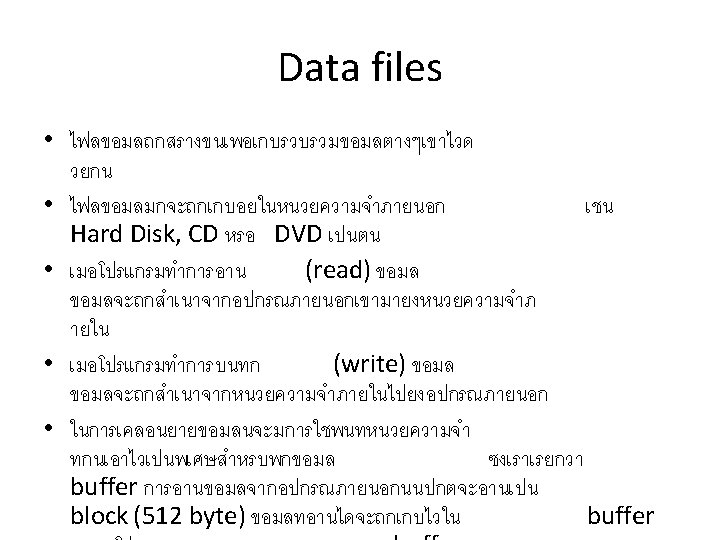
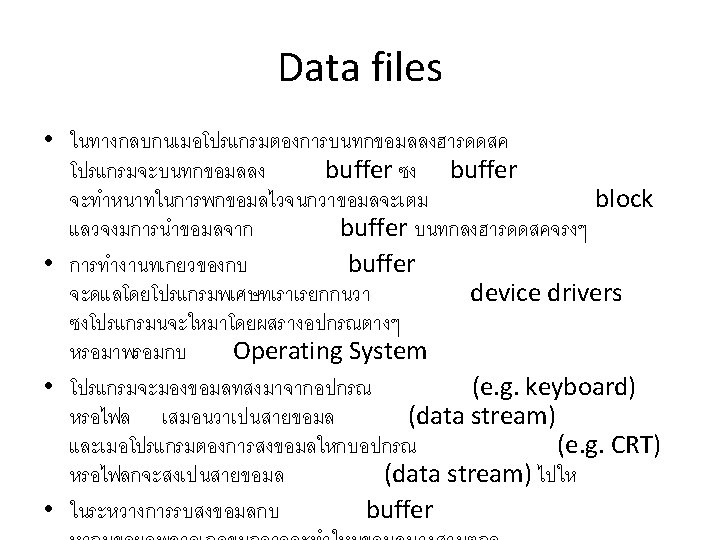
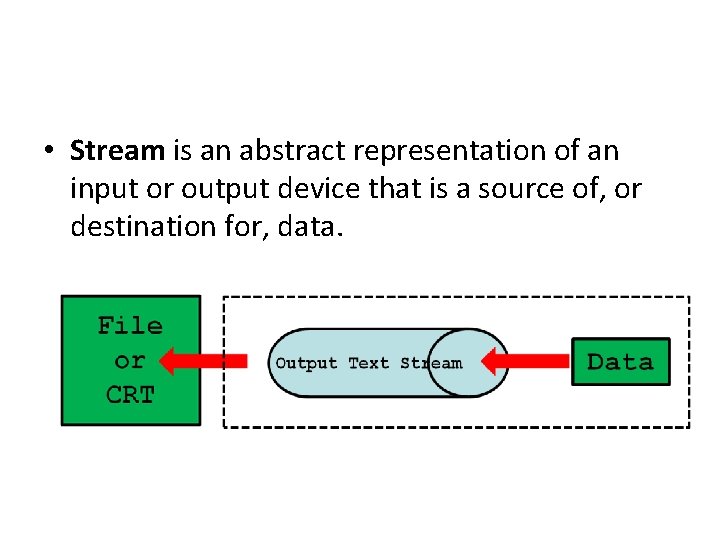
• Stream is an abstract representation of an input or output device that is a source of, or destination for, data.
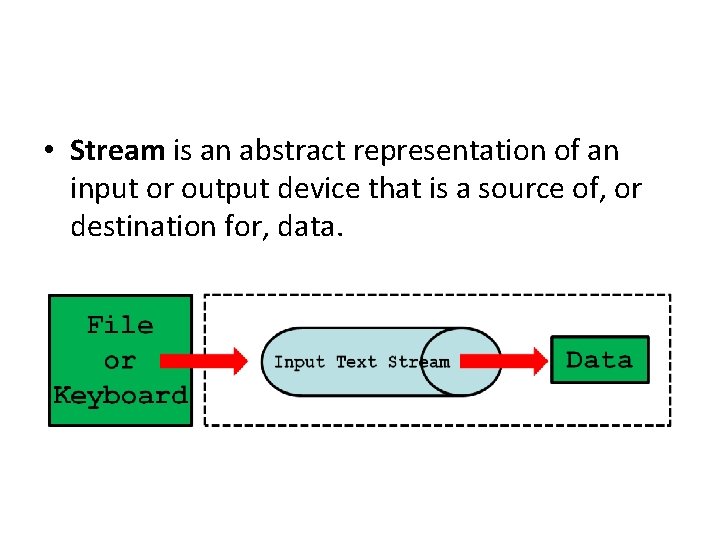
• Stream is an abstract representation of an input or output device that is a source of, or destination for, data.
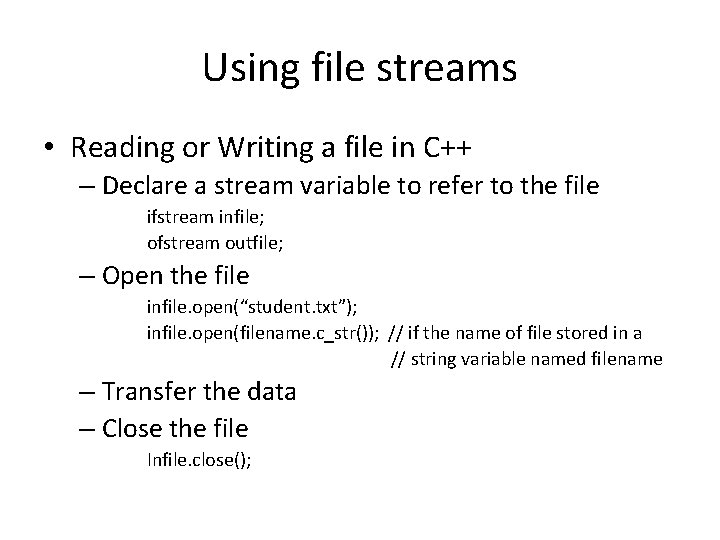
Using file streams • Reading or Writing a file in C++ – Declare a stream variable to refer to the file ifstream infile; ofstream outfile; – Open the file infile. open(“student. txt”); infile. open(filename. c_str()); // if the name of file stored in a // string variable named filename – Transfer the data – Close the file Infile. close();
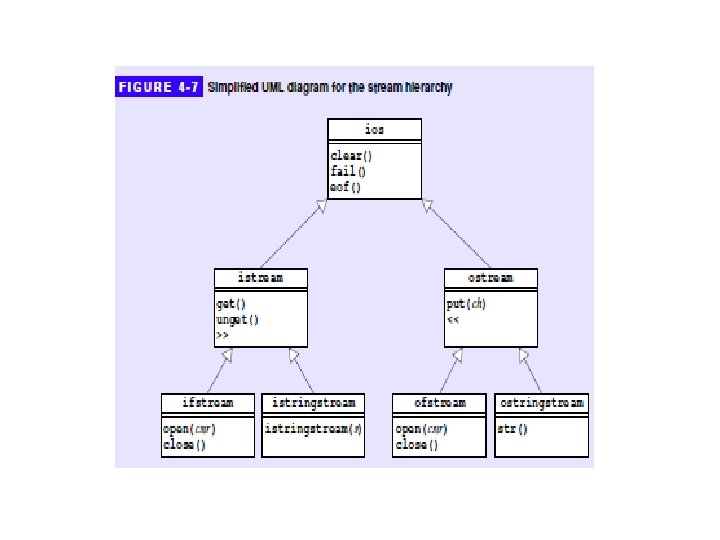
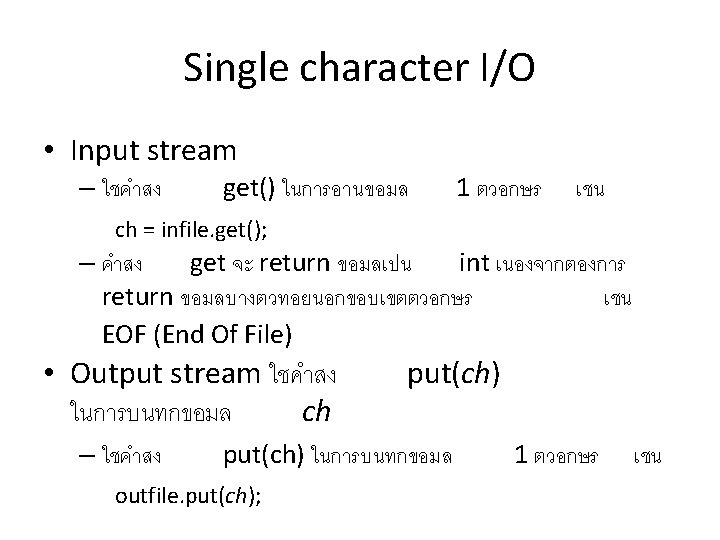
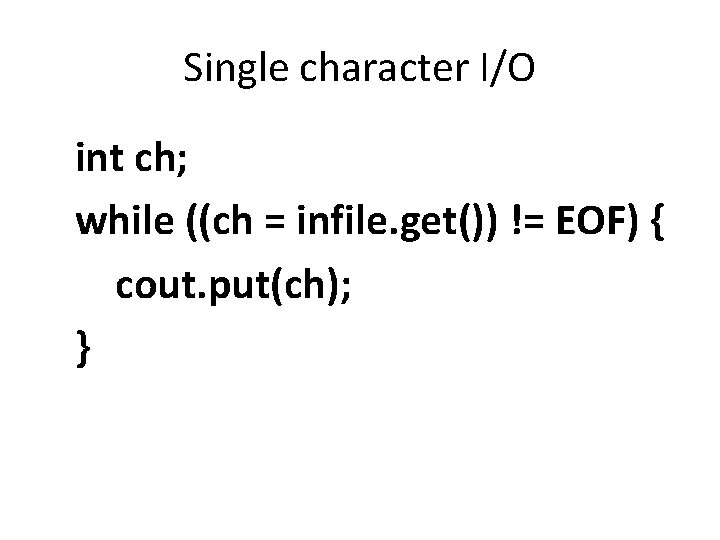
Single character I/O int ch; while ((ch = infile. get()) != EOF) { cout. put(ch); }
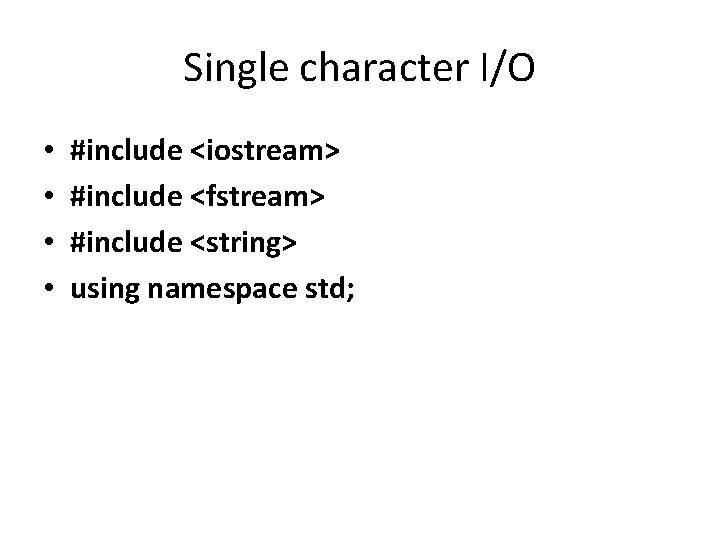
Single character I/O • • #include <iostream> #include <fstream> #include <string> using namespace std;
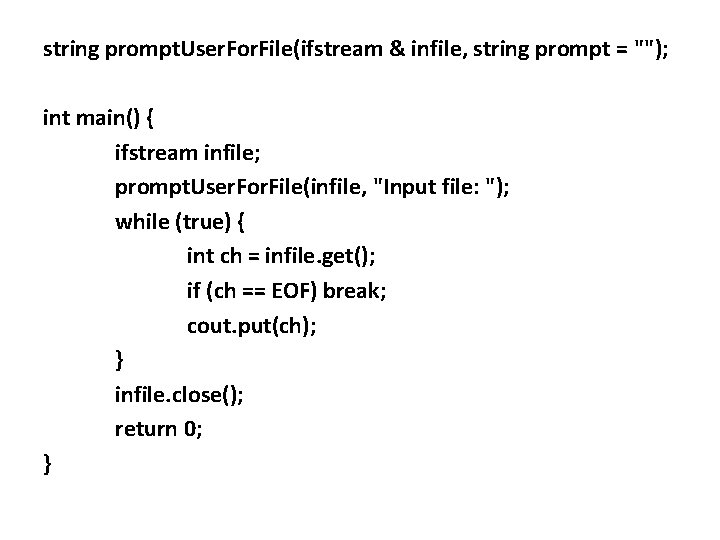
string prompt. User. For. File(ifstream & infile, string prompt = ""); int main() { ifstream infile; prompt. User. For. File(infile, "Input file: "); while (true) { int ch = infile. get(); if (ch == EOF) break; cout. put(ch); } infile. close(); return 0; }
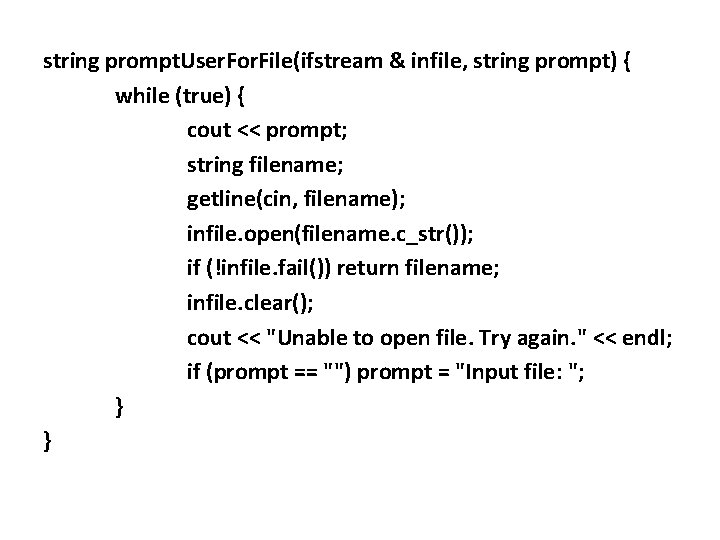
string prompt. User. For. File(ifstream & infile, string prompt) { while (true) { cout << prompt; string filename; getline(cin, filename); infile. open(filename. c_str()); if (!infile. fail()) return filename; infile. clear(); cout << "Unable to open file. Try again. " << endl; if (prompt == "") prompt = "Input file: "; } }
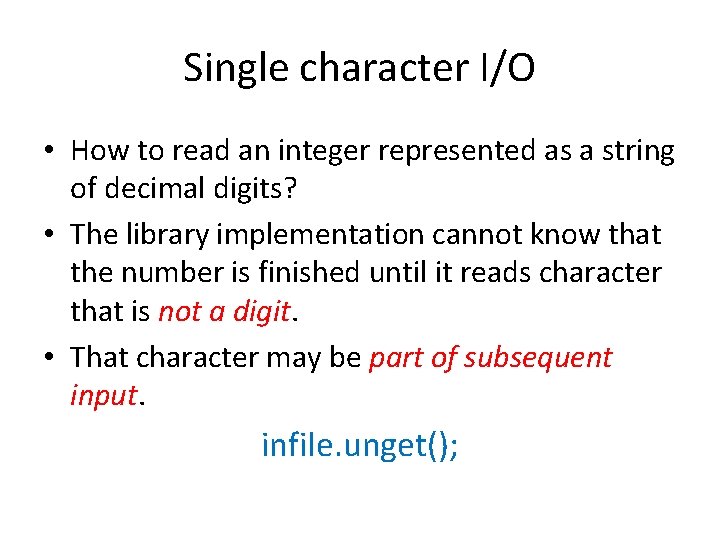
Single character I/O • How to read an integer represented as a string of decimal digits? • The library implementation cannot know that the number is finished until it reads character that is not a digit. • That character may be part of subsequent input. infile. unget();
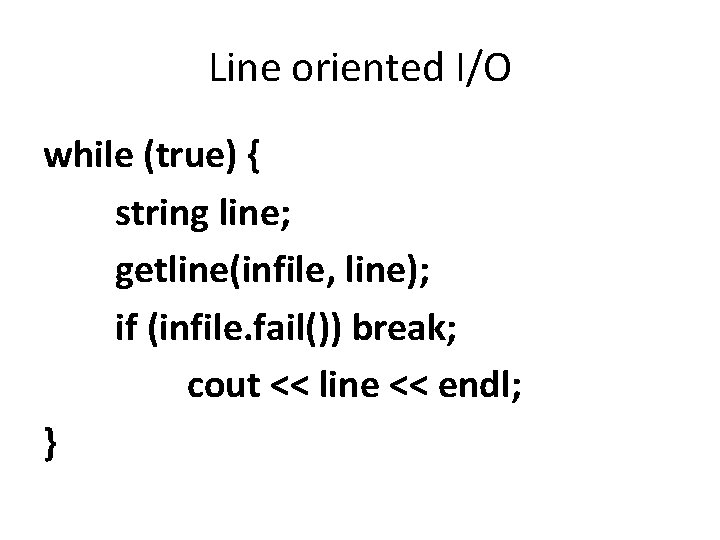
Line oriented I/O while (true) { string line; getline(infile, line); if (infile. fail()) break; cout << line << endl; }
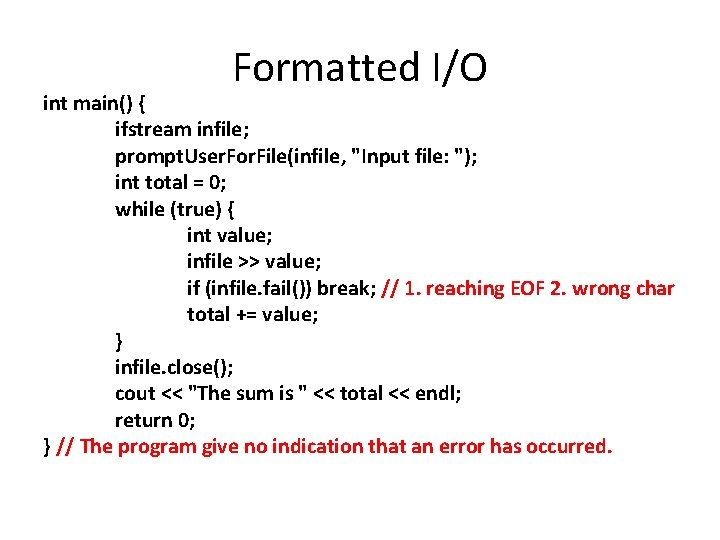
Formatted I/O int main() { ifstream infile; prompt. User. For. File(infile, "Input file: "); int total = 0; while (true) { int value; infile >> value; if (infile. fail()) break; // 1. reaching EOF 2. wrong char total += value; } infile. close(); cout << "The sum is " << total << endl; return 0; } // The program give no indication that an error has occurred.
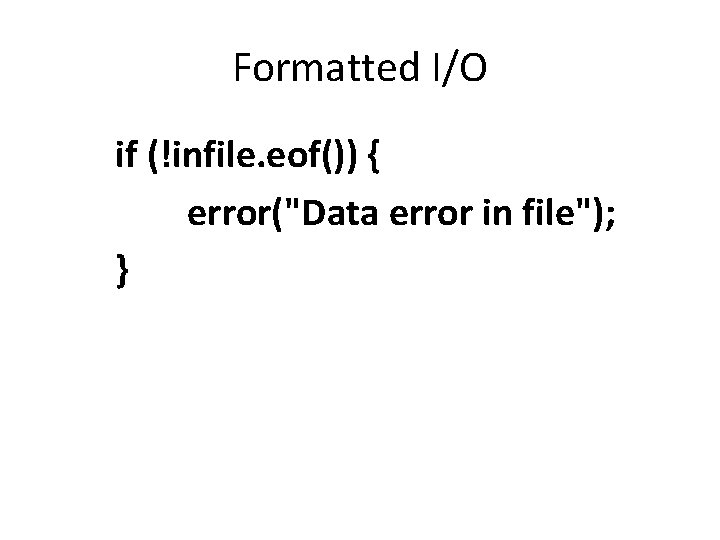
Formatted I/O if (!infile. eof()) { error("Data error in file"); }
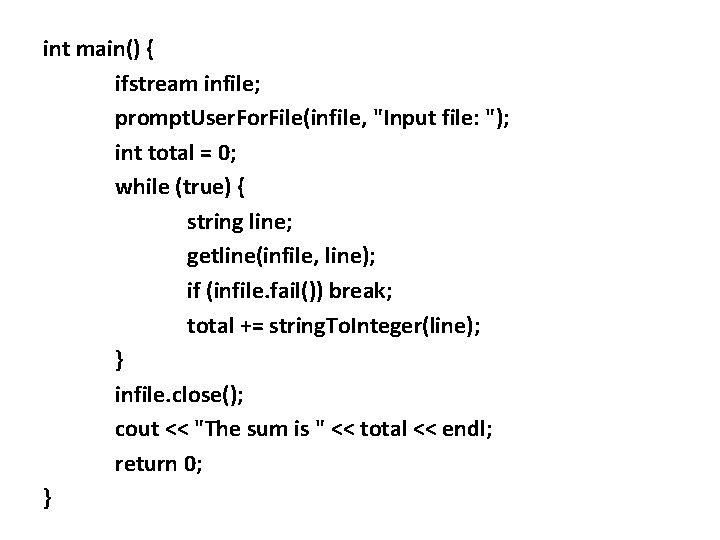
int main() { ifstream infile; prompt. User. For. File(infile, "Input file: "); int total = 0; while (true) { string line; getline(infile, line); if (infile. fail()) break; total += string. To. Integer(line); } infile. close(); cout << "The sum is " << total << endl; return 0; }
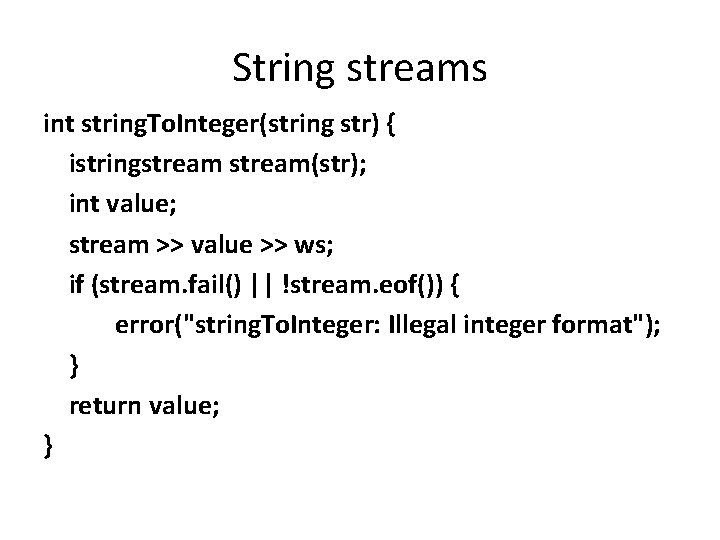
String streams int string. To. Integer(string str) { istringstream(str); int value; stream >> value >> ws; if (stream. fail() || !stream. eof()) { error("string. To. Integer: Illegal integer format"); } return value; }
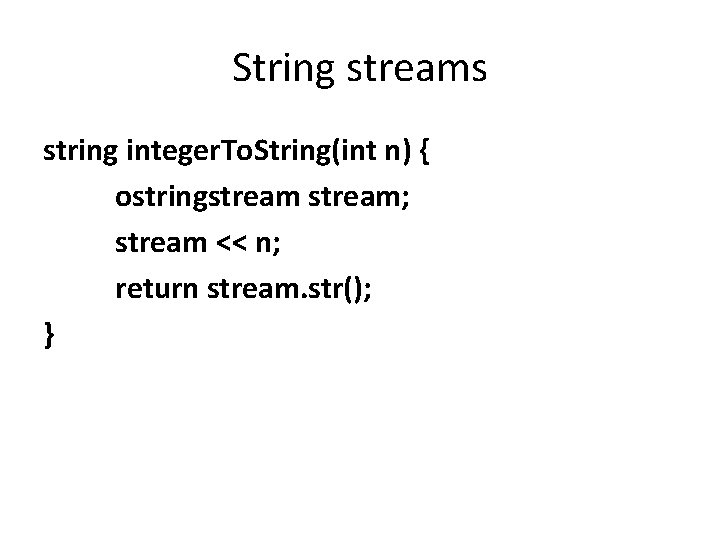
String streams string integer. To. String(int n) { ostringstream; stream << n; return stream. str(); }
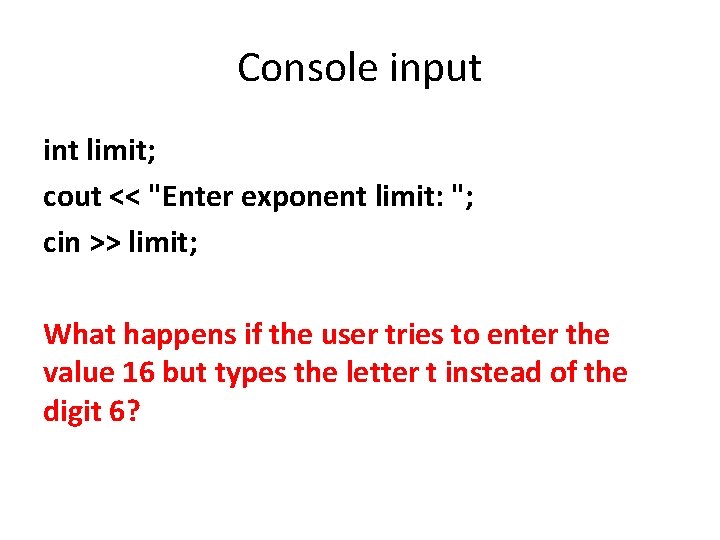
Console input int limit; cout << "Enter exponent limit: "; cin >> limit; What happens if the user tries to enter the value 16 but types the letter t instead of the digit 6?
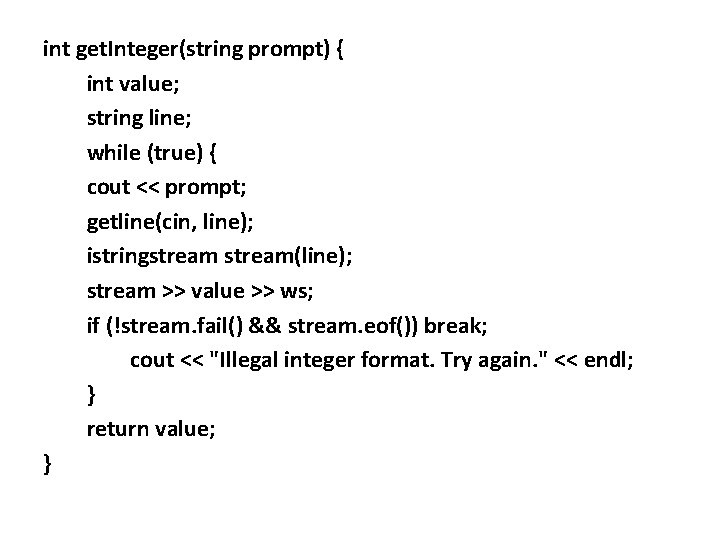
int get. Integer(string prompt) { int value; string line; while (true) { cout << prompt; getline(cin, line); istringstream(line); stream >> value >> ws; if (!stream. fail() && stream. eof()) break; cout << "Illegal integer format. Try again. " << endl; } return value; }
Right time right place right quantity right quality
Right product right place right time right price
The right man on the right place at the right time
Apa introduction example
Snmp model in computer networks
Cout setf ios fixed
Coût variable exemple
Fiche de coût standard
Std endl
Sai gon food
Cout cible
Cout.write("objectoriented programming", 6);
Coefficient imputation rationnelle
Iostream
Natexis banque populaire
Cout
Un facteur rare
Fiche de cout standard unitaire
Cout.write("objectoriented programming", 6);
Cout moyen pondéré du capital
Cout complet rationnel
Cout hors production
Display the address of intval using cout and intptr.
Streams in c++
Ecart de marge sur cout preetabli
Courbe cout marginal
Cout de renonciation croissant
Characout
Gestion de stock formule
Modificação
Types of jet streams
Cba streams