Chapter 10 IO Streams Input Streams Output Streams
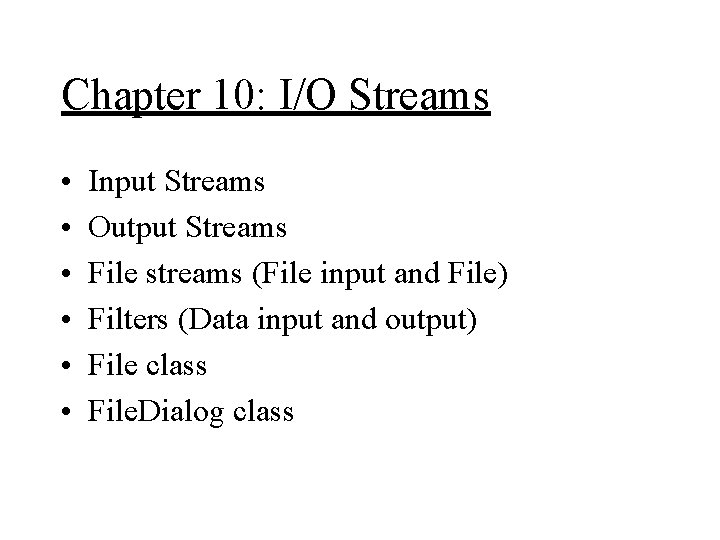
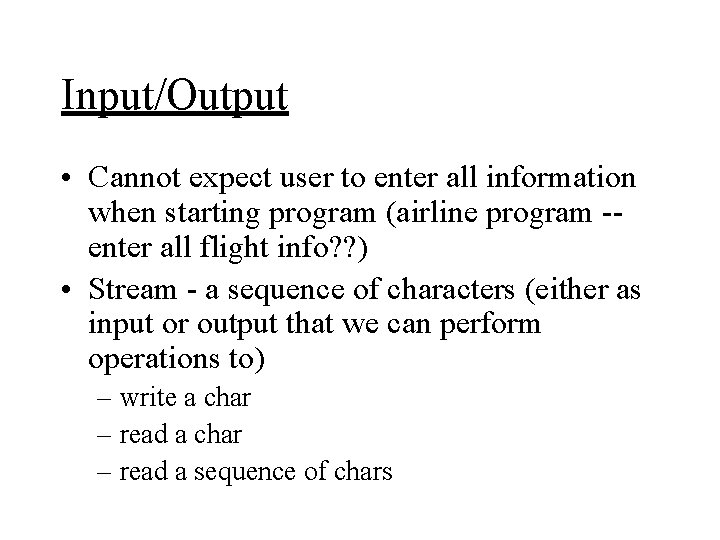
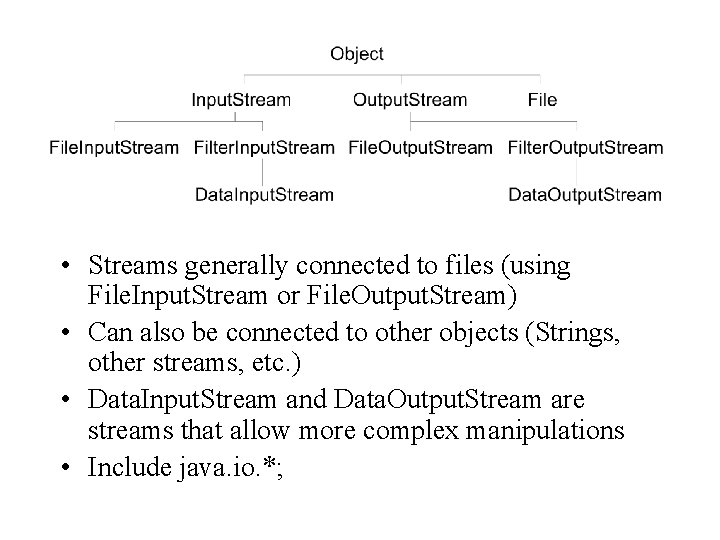
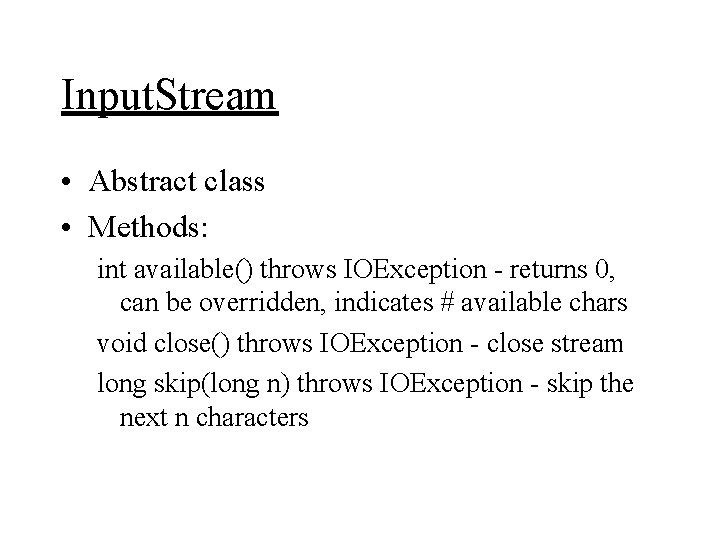
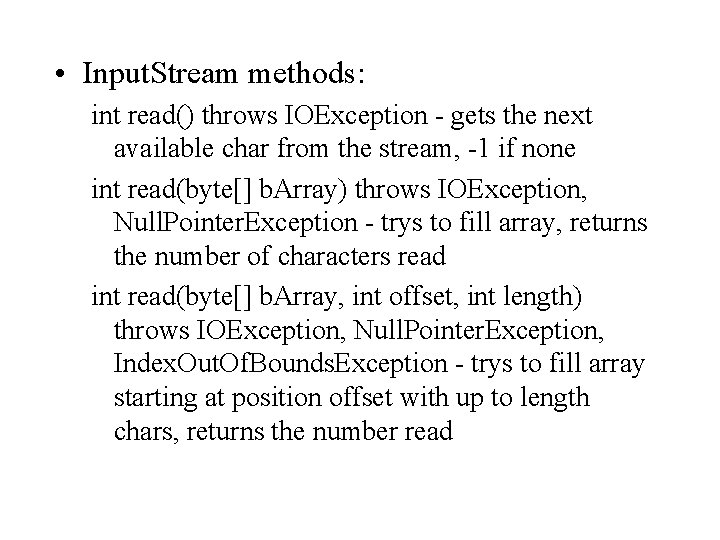
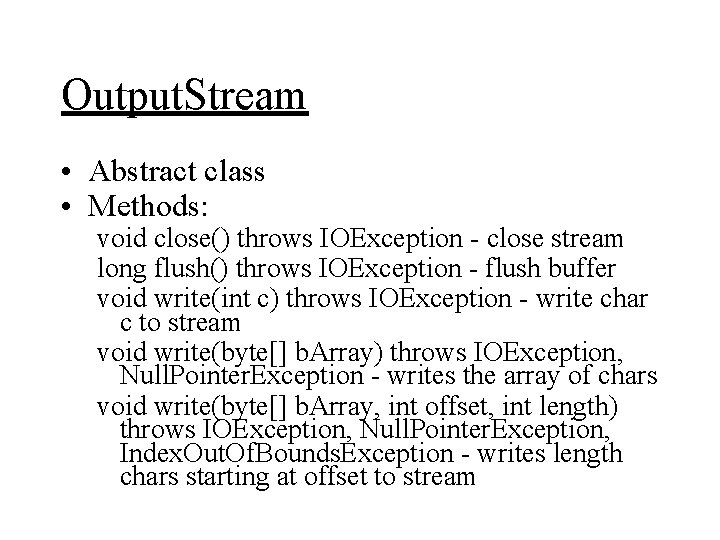
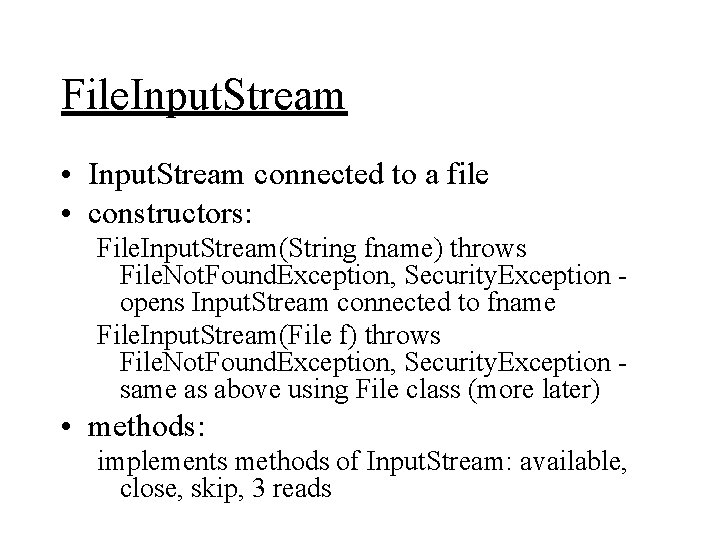
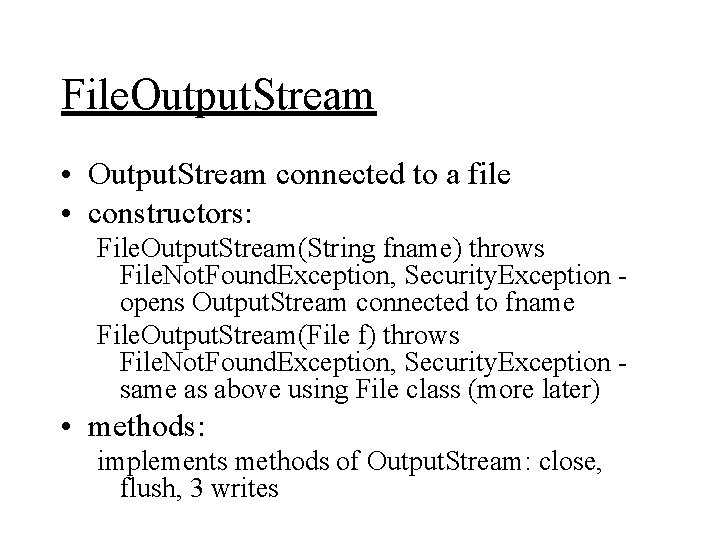
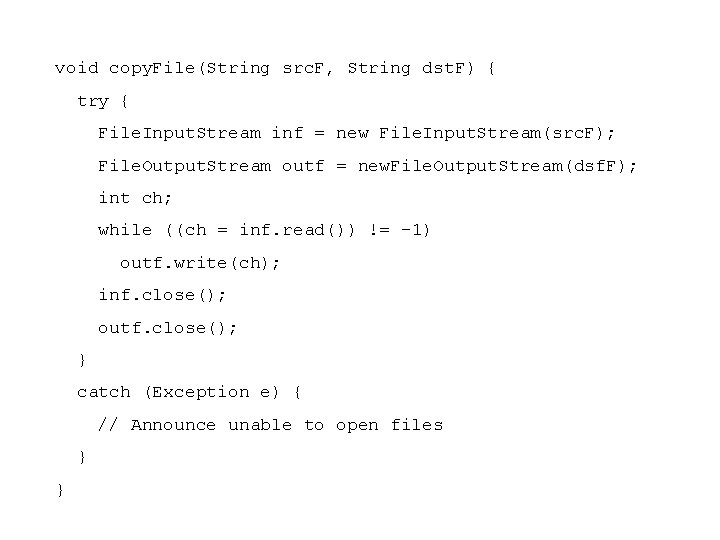
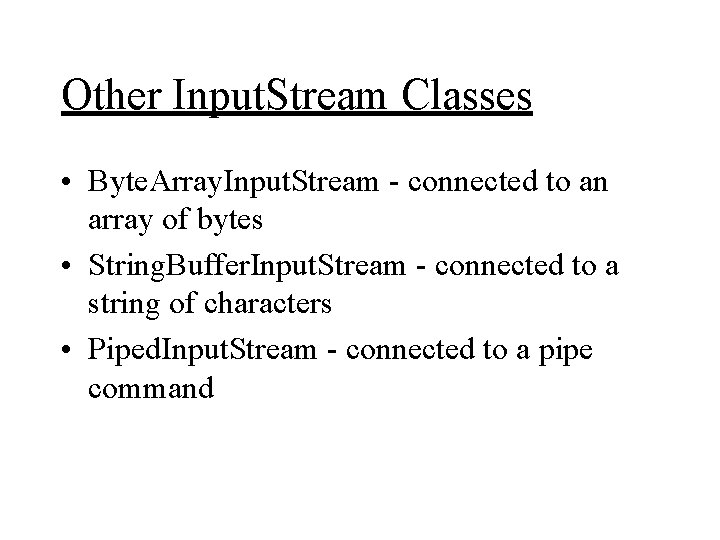
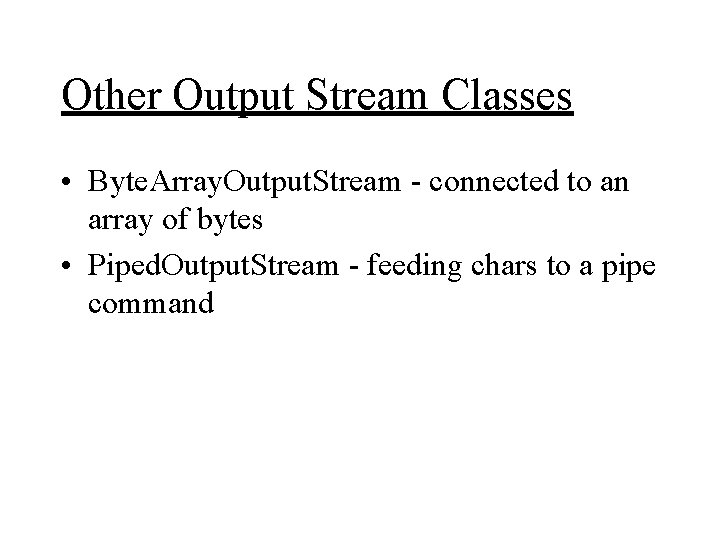
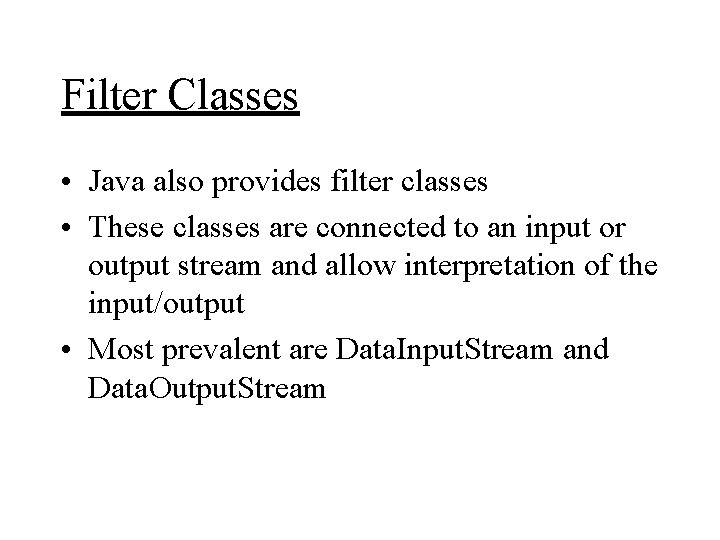
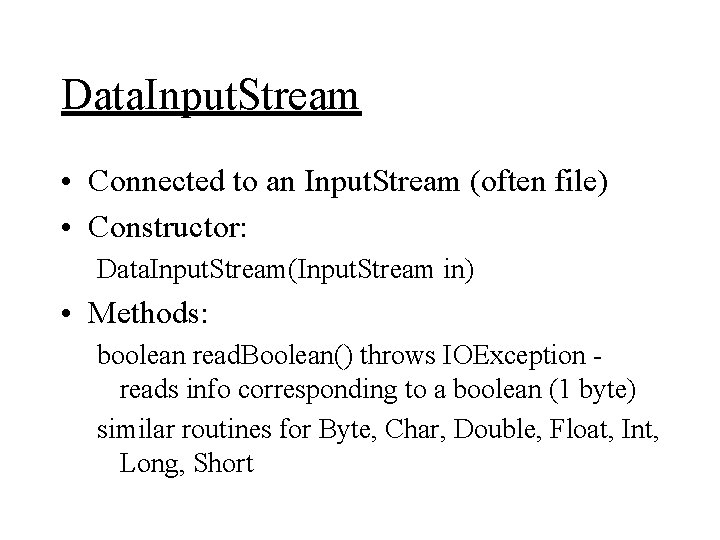
![Data. Input. Stream (cont) • More methods: int read. Fully(byte[] b. Array) throws IOException, Data. Input. Stream (cont) • More methods: int read. Fully(byte[] b. Array) throws IOException,](https://slidetodoc.com/presentation_image_h2/d2a32aa1cbaad6939d763c047e7275df/image-14.jpg)
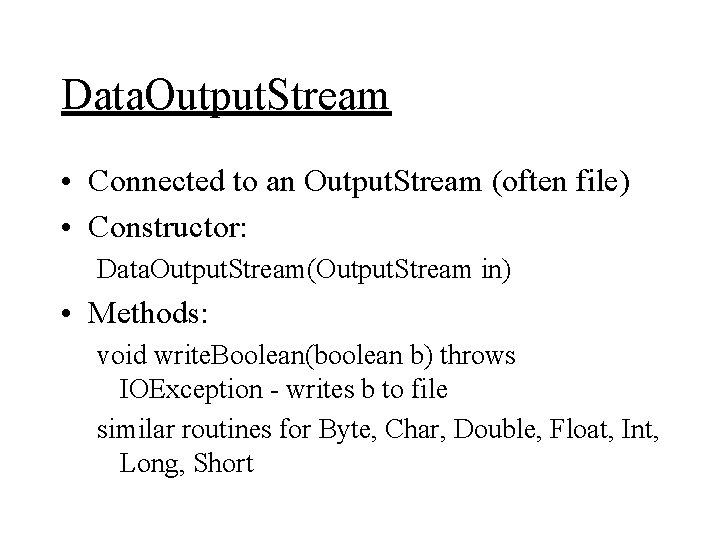
![Data. Output. Stream (cont) • More methods: void write. Bytes(byte[] barray) throws IOException - Data. Output. Stream (cont) • More methods: void write. Bytes(byte[] barray) throws IOException -](https://slidetodoc.com/presentation_image_h2/d2a32aa1cbaad6939d763c047e7275df/image-16.jpg)
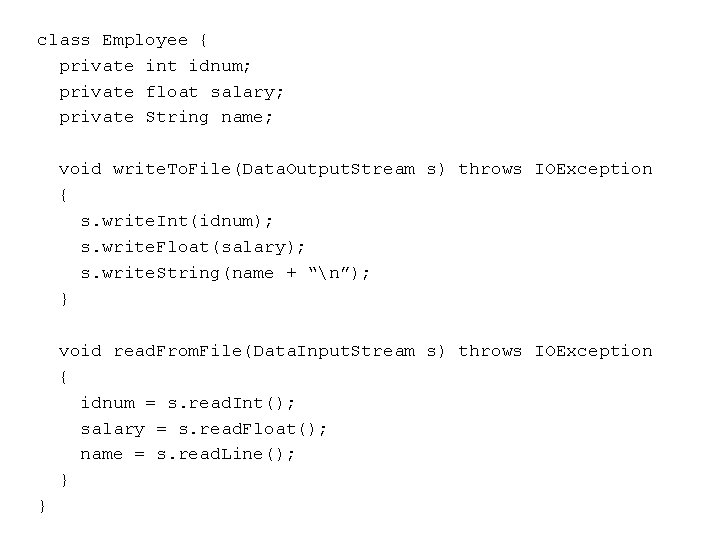
![void write. Employee. File(String fname, Employee[] bees, int num. Employees) { try { File. void write. Employee. File(String fname, Employee[] bees, int num. Employees) { try { File.](https://slidetodoc.com/presentation_image_h2/d2a32aa1cbaad6939d763c047e7275df/image-18.jpg)
![int read. Employee. File(String fname, Employee[] bees) { try { File. Input. Stream fis int read. Employee. File(String fname, Employee[] bees) { try { File. Input. Stream fis](https://slidetodoc.com/presentation_image_h2/d2a32aa1cbaad6939d763c047e7275df/image-19.jpg)
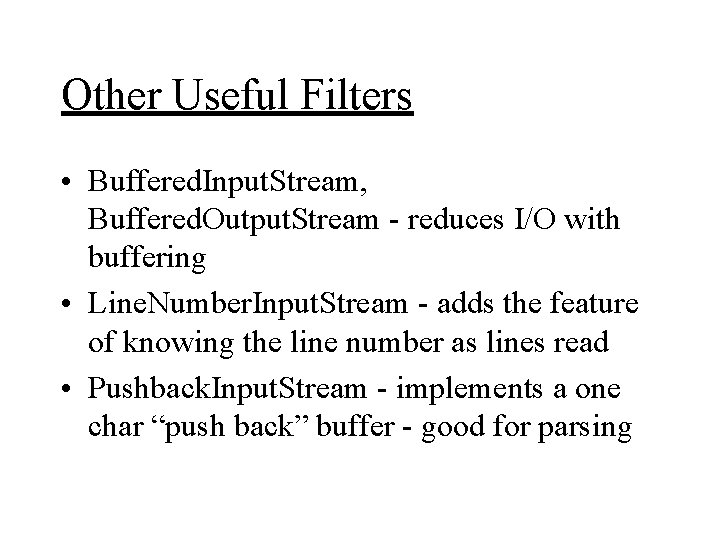
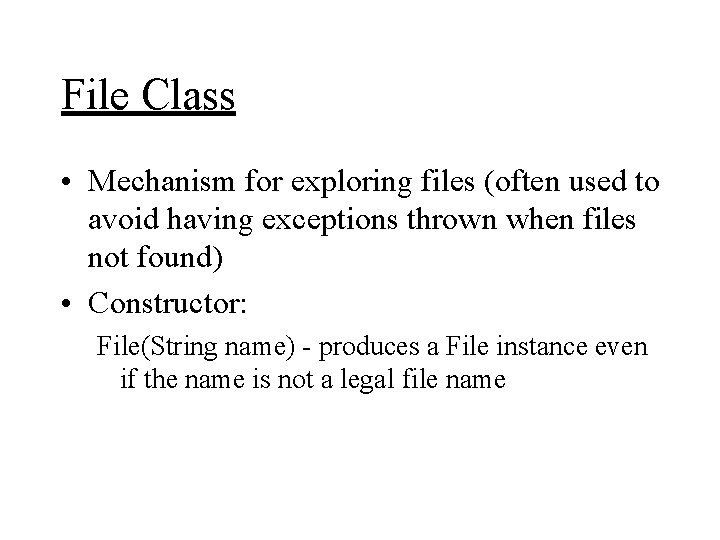
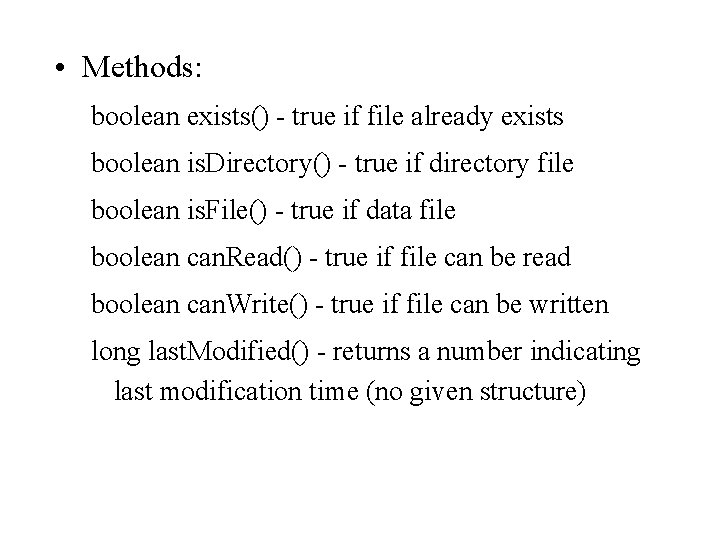
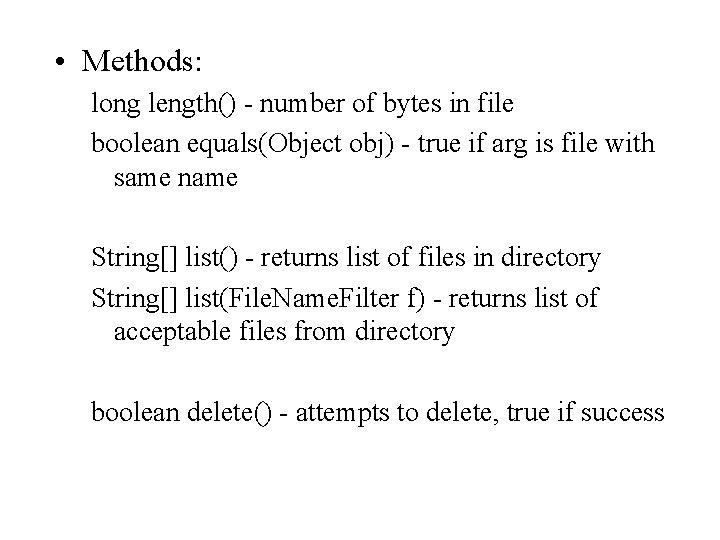
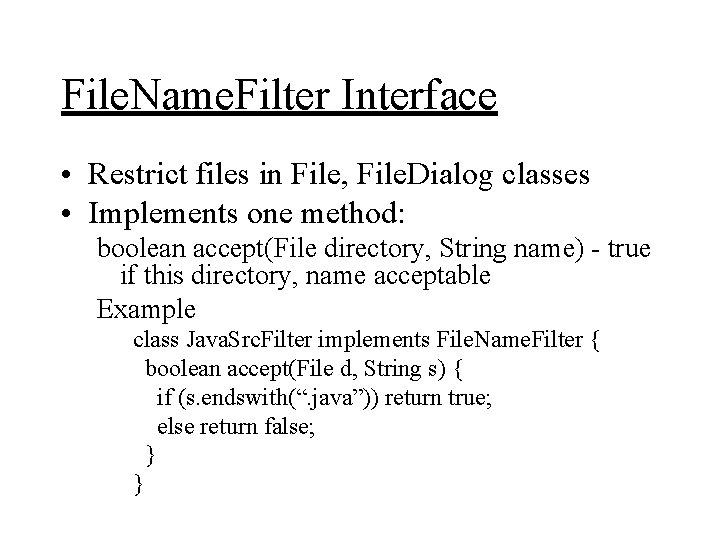
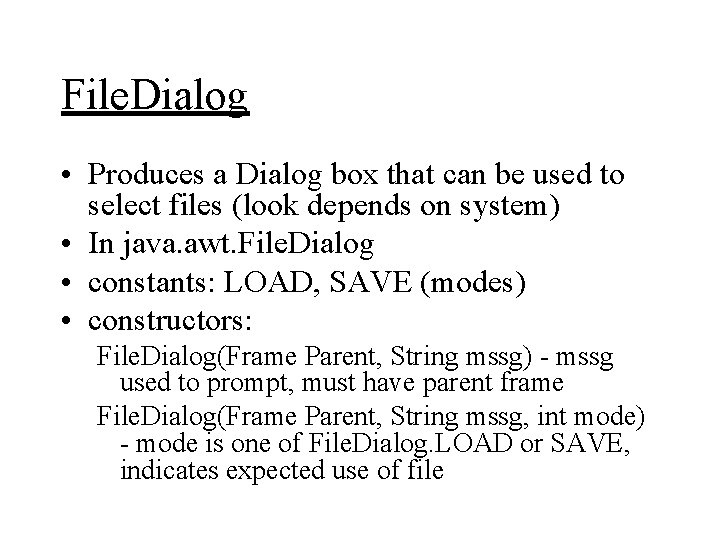
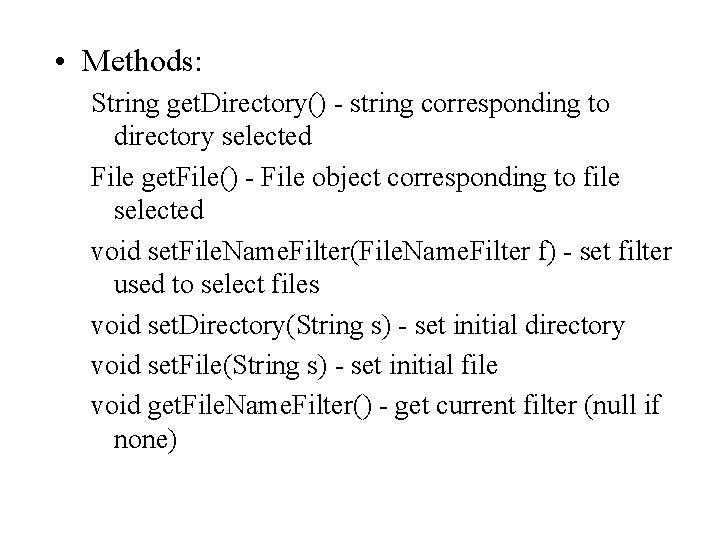
- Slides: 26
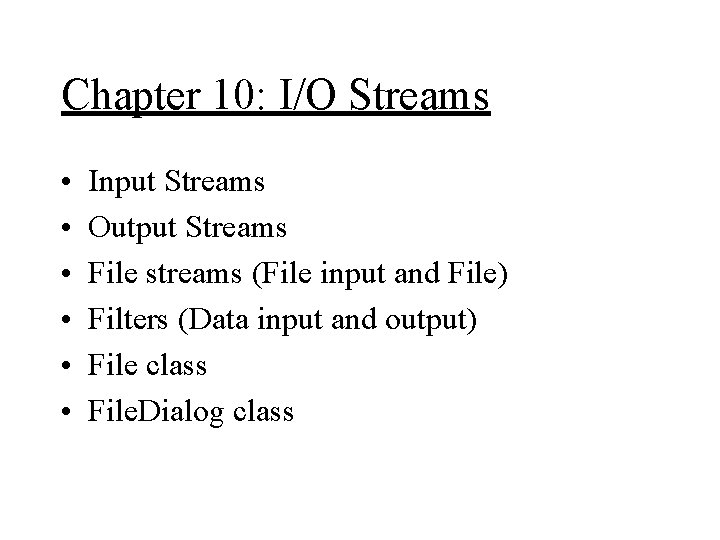
Chapter 10: I/O Streams • • • Input Streams Output Streams File streams (File input and File) Filters (Data input and output) File class File. Dialog class
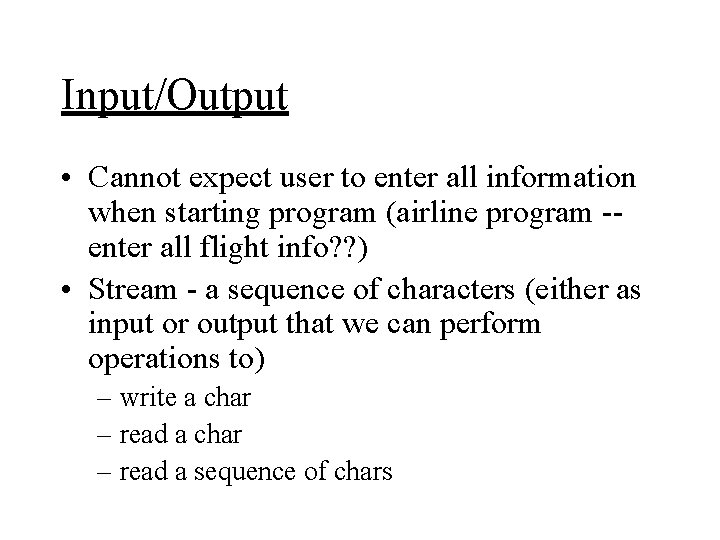
Input/Output • Cannot expect user to enter all information when starting program (airline program -enter all flight info? ? ) • Stream - a sequence of characters (either as input or output that we can perform operations to) – write a char – read a sequence of chars
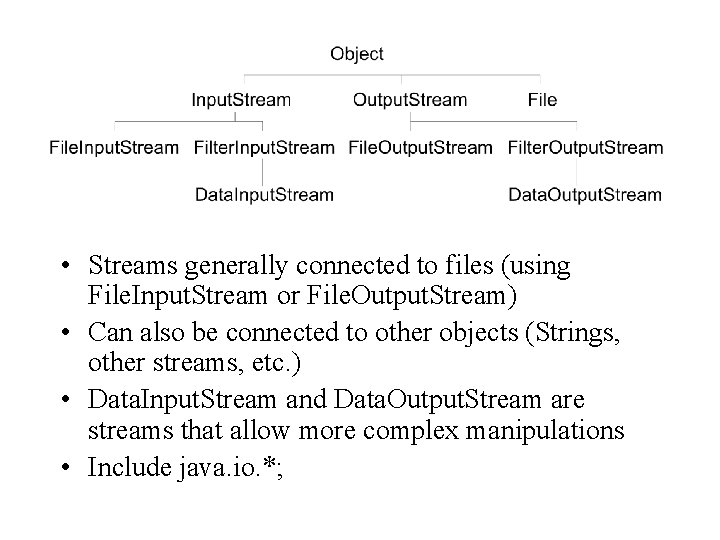
• Streams generally connected to files (using File. Input. Stream or File. Output. Stream) • Can also be connected to other objects (Strings, other streams, etc. ) • Data. Input. Stream and Data. Output. Stream are streams that allow more complex manipulations • Include java. io. *;
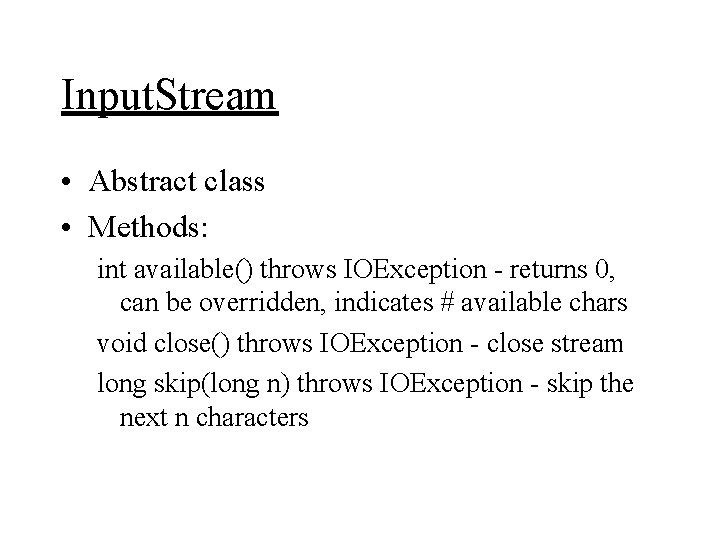
Input. Stream • Abstract class • Methods: int available() throws IOException - returns 0, can be overridden, indicates # available chars void close() throws IOException - close stream long skip(long n) throws IOException - skip the next n characters
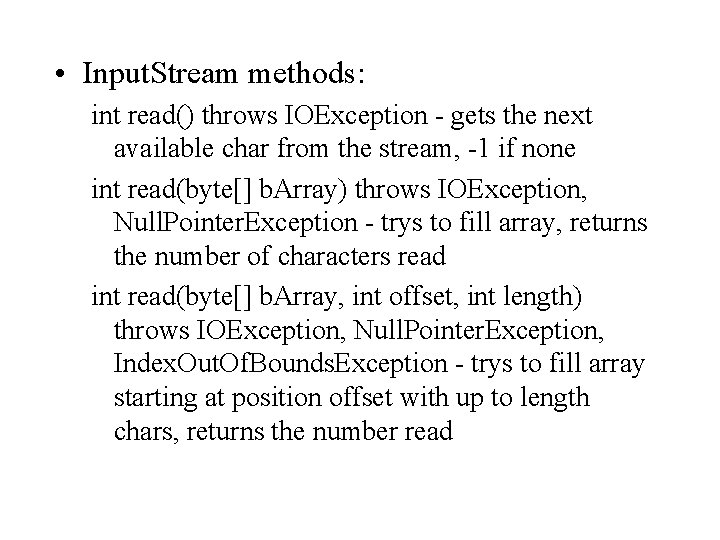
• Input. Stream methods: int read() throws IOException - gets the next available char from the stream, -1 if none int read(byte[] b. Array) throws IOException, Null. Pointer. Exception - trys to fill array, returns the number of characters read int read(byte[] b. Array, int offset, int length) throws IOException, Null. Pointer. Exception, Index. Out. Of. Bounds. Exception - trys to fill array starting at position offset with up to length chars, returns the number read
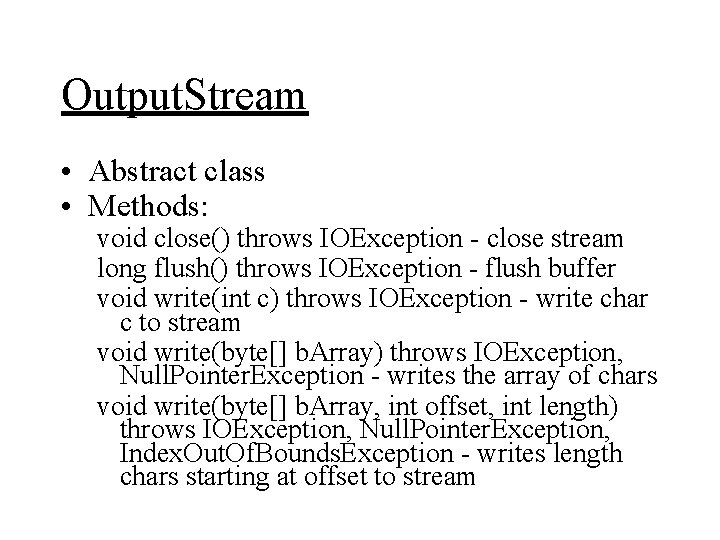
Output. Stream • Abstract class • Methods: void close() throws IOException - close stream long flush() throws IOException - flush buffer void write(int c) throws IOException - write char c to stream void write(byte[] b. Array) throws IOException, Null. Pointer. Exception - writes the array of chars void write(byte[] b. Array, int offset, int length) throws IOException, Null. Pointer. Exception, Index. Out. Of. Bounds. Exception - writes length chars starting at offset to stream
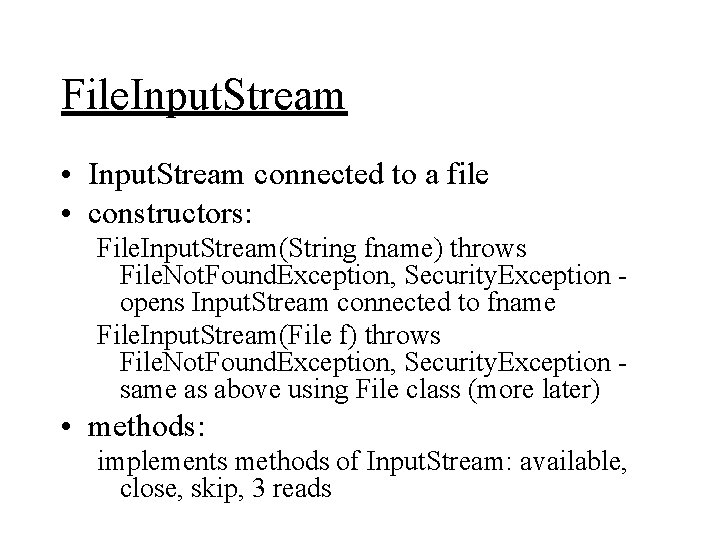
File. Input. Stream • Input. Stream connected to a file • constructors: File. Input. Stream(String fname) throws File. Not. Found. Exception, Security. Exception opens Input. Stream connected to fname File. Input. Stream(File f) throws File. Not. Found. Exception, Security. Exception same as above using File class (more later) • methods: implements methods of Input. Stream: available, close, skip, 3 reads
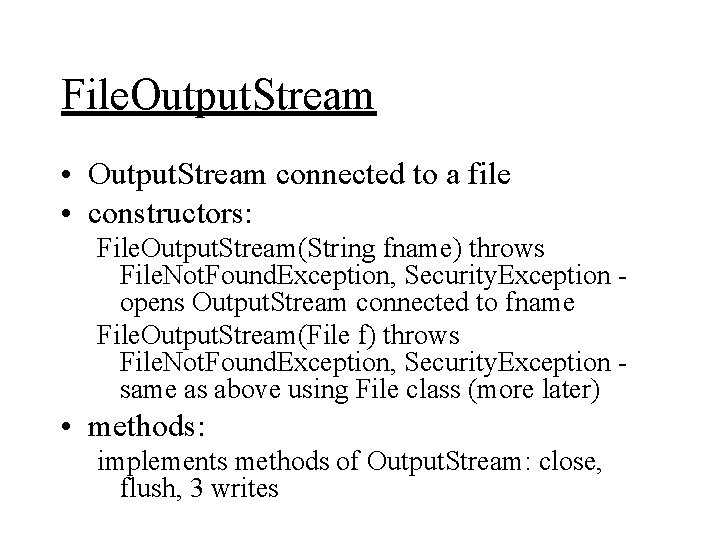
File. Output. Stream • Output. Stream connected to a file • constructors: File. Output. Stream(String fname) throws File. Not. Found. Exception, Security. Exception opens Output. Stream connected to fname File. Output. Stream(File f) throws File. Not. Found. Exception, Security. Exception same as above using File class (more later) • methods: implements methods of Output. Stream: close, flush, 3 writes
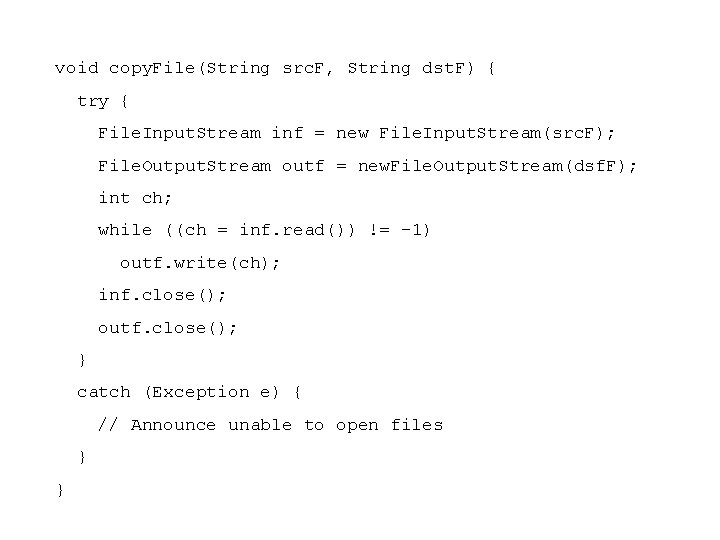
void copy. File(String src. F, String dst. F) { try { File. Input. Stream inf = new File. Input. Stream(src. F); File. Output. Stream outf = new. File. Output. Stream(dsf. F); int ch; while ((ch = inf. read()) != -1) outf. write(ch); inf. close(); outf. close(); } catch (Exception e) { // Announce unable to open files } }
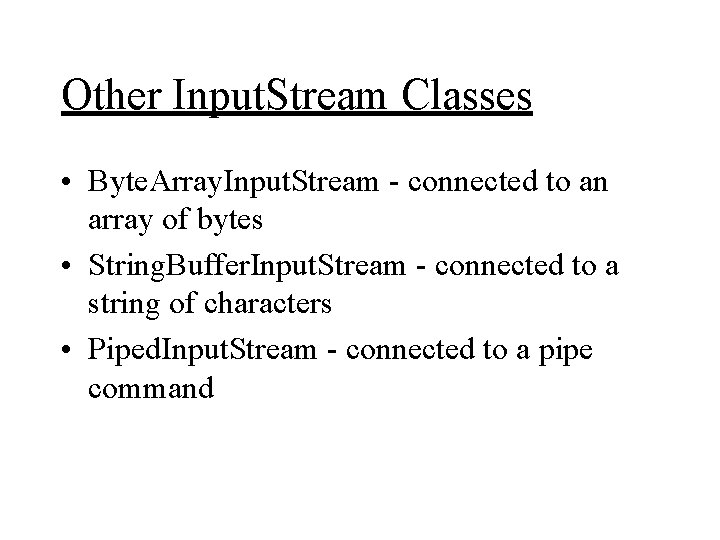
Other Input. Stream Classes • Byte. Array. Input. Stream - connected to an array of bytes • String. Buffer. Input. Stream - connected to a string of characters • Piped. Input. Stream - connected to a pipe command
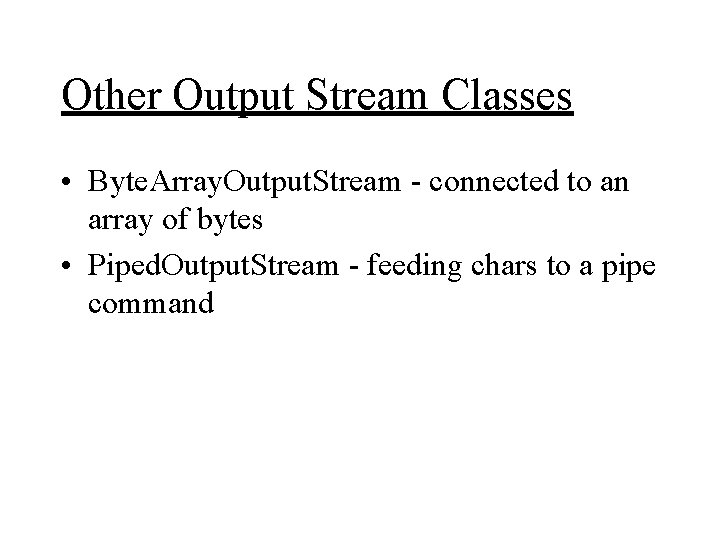
Other Output Stream Classes • Byte. Array. Output. Stream - connected to an array of bytes • Piped. Output. Stream - feeding chars to a pipe command
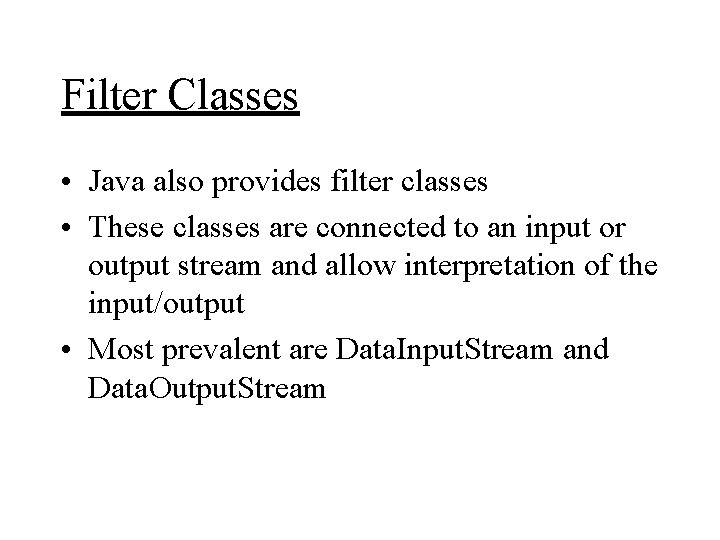
Filter Classes • Java also provides filter classes • These classes are connected to an input or output stream and allow interpretation of the input/output • Most prevalent are Data. Input. Stream and Data. Output. Stream
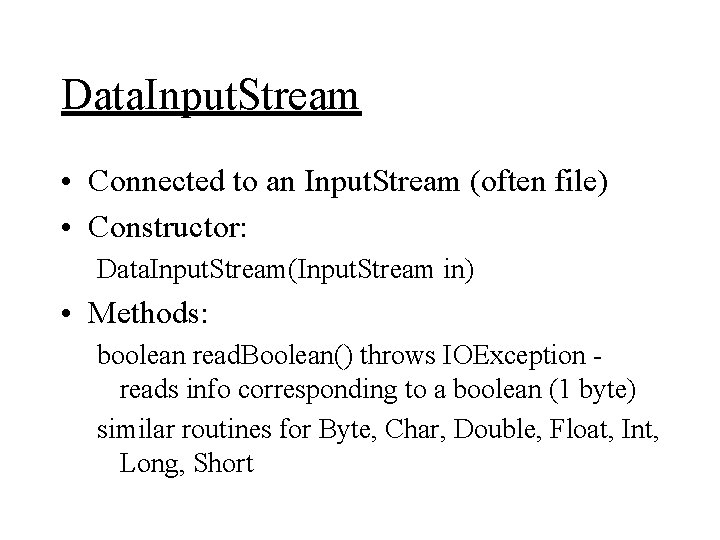
Data. Input. Stream • Connected to an Input. Stream (often file) • Constructor: Data. Input. Stream(Input. Stream in) • Methods: boolean read. Boolean() throws IOException reads info corresponding to a boolean (1 byte) similar routines for Byte, Char, Double, Float, Int, Long, Short
![Data Input Stream cont More methods int read Fullybyte b Array throws IOException Data. Input. Stream (cont) • More methods: int read. Fully(byte[] b. Array) throws IOException,](https://slidetodoc.com/presentation_image_h2/d2a32aa1cbaad6939d763c047e7275df/image-14.jpg)
Data. Input. Stream (cont) • More methods: int read. Fully(byte[] b. Array) throws IOException, Null. Pointer. Exception - read in array of chars stopping when full or at EOF String read. Line() throws IOException - read as a string the next sequence of chars until a newline encountered void skip. Bytes(int n) throws IOException - skip the next n bytes
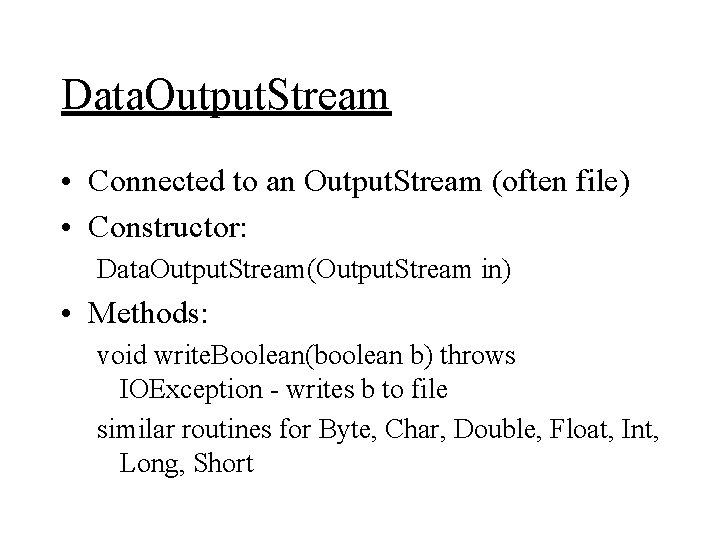
Data. Output. Stream • Connected to an Output. Stream (often file) • Constructor: Data. Output. Stream(Output. Stream in) • Methods: void write. Boolean(boolean b) throws IOException - writes b to file similar routines for Byte, Char, Double, Float, Int, Long, Short
![Data Output Stream cont More methods void write Bytesbyte barray throws IOException Data. Output. Stream (cont) • More methods: void write. Bytes(byte[] barray) throws IOException -](https://slidetodoc.com/presentation_image_h2/d2a32aa1cbaad6939d763c047e7275df/image-16.jpg)
Data. Output. Stream (cont) • More methods: void write. Bytes(byte[] barray) throws IOException - write array of bytes void write. Bytes(String s) throws IOException write string as array of bytes void write. Chars(String s) throws IOException similar to write. Bytes for string arg
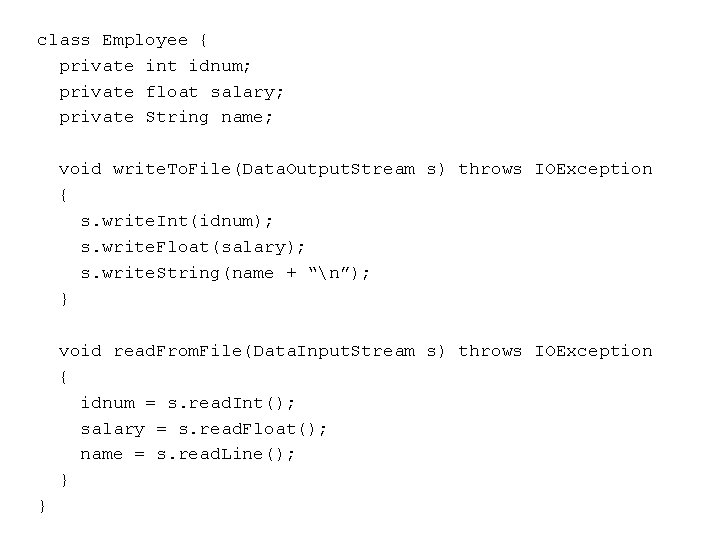
class Employee { private int idnum; private float salary; private String name; void write. To. File(Data. Output. Stream s) throws IOException { s. write. Int(idnum); s. write. Float(salary); s. write. String(name + “n”); } void read. From. File(Data. Input. Stream s) throws IOException { idnum = s. read. Int(); salary = s. read. Float(); name = s. read. Line(); } }
![void write Employee FileString fname Employee bees int num Employees try File void write. Employee. File(String fname, Employee[] bees, int num. Employees) { try { File.](https://slidetodoc.com/presentation_image_h2/d2a32aa1cbaad6939d763c047e7275df/image-18.jpg)
void write. Employee. File(String fname, Employee[] bees, int num. Employees) { try { File. Output. Stream fos = new File. Output. Stream(fname); Data. Output. Stream fstream = new Data. Output. Stream(fos); int e; fstream. write. Int(num. Employees); for (e = 0; e < num. Employees; e++) bees[e]. write. To. File(fstream); fstream. close(); } catch IOException { // report that something went wrong } }
![int read Employee FileString fname Employee bees try File Input Stream fis int read. Employee. File(String fname, Employee[] bees) { try { File. Input. Stream fis](https://slidetodoc.com/presentation_image_h2/d2a32aa1cbaad6939d763c047e7275df/image-19.jpg)
int read. Employee. File(String fname, Employee[] bees) { try { File. Input. Stream fis = new File. Input. Stream(fname); Data. Input. Stream fstream = new Data. Input. Stream(fis); int e; int num. Employees; num. Employees = fstream. read. Int(); for (e = 0; e < num. Employees; e++) bees[e]. read. From. File(fstream); fstream. close(); return num. Employees; } catch IOException { // report that something went wrong } }
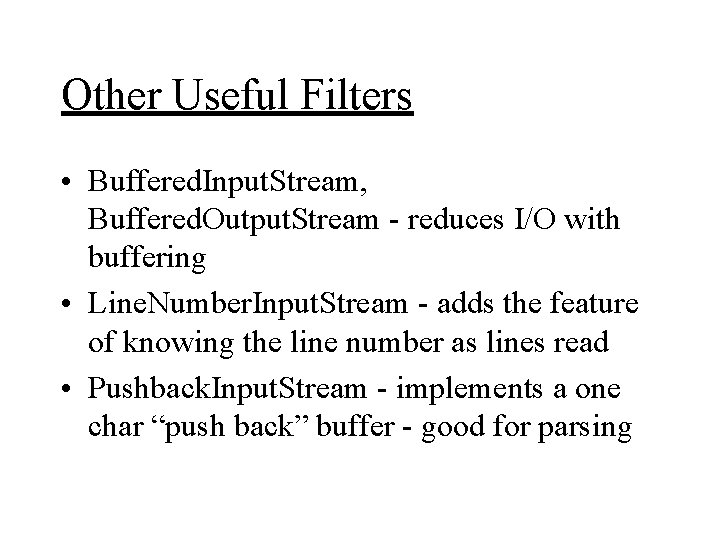
Other Useful Filters • Buffered. Input. Stream, Buffered. Output. Stream - reduces I/O with buffering • Line. Number. Input. Stream - adds the feature of knowing the line number as lines read • Pushback. Input. Stream - implements a one char “push back” buffer - good for parsing
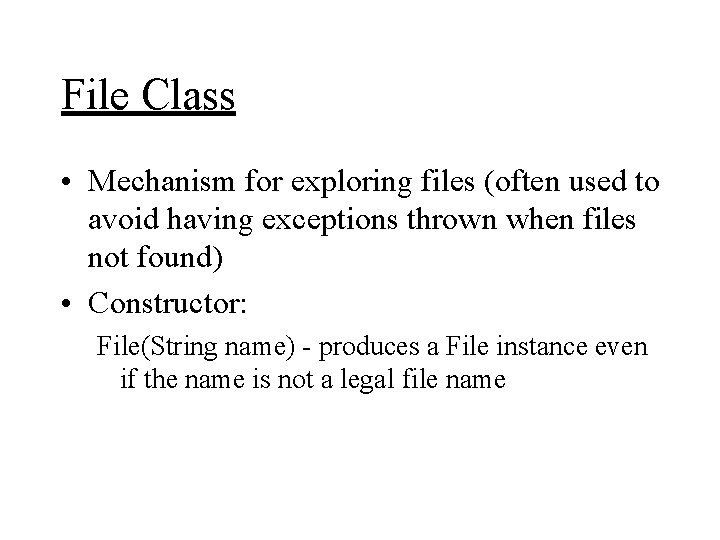
File Class • Mechanism for exploring files (often used to avoid having exceptions thrown when files not found) • Constructor: File(String name) - produces a File instance even if the name is not a legal file name
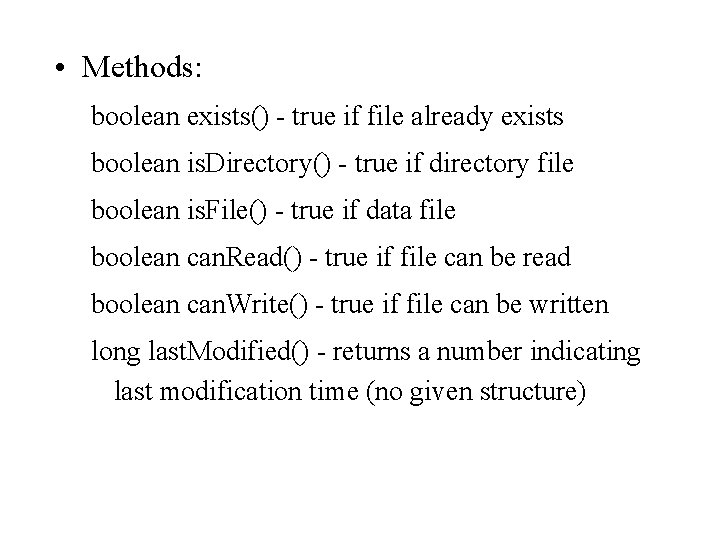
• Methods: boolean exists() - true if file already exists boolean is. Directory() - true if directory file boolean is. File() - true if data file boolean can. Read() - true if file can be read boolean can. Write() - true if file can be written long last. Modified() - returns a number indicating last modification time (no given structure)
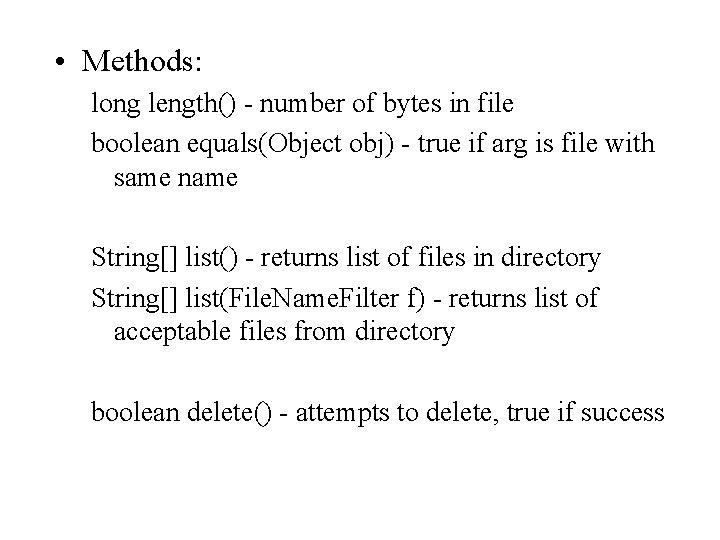
• Methods: long length() - number of bytes in file boolean equals(Object obj) - true if arg is file with same name String[] list() - returns list of files in directory String[] list(File. Name. Filter f) - returns list of acceptable files from directory boolean delete() - attempts to delete, true if success
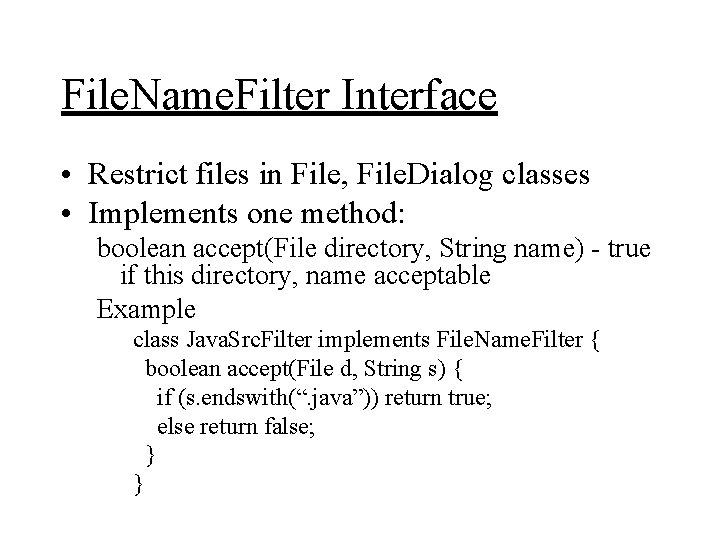
File. Name. Filter Interface • Restrict files in File, File. Dialog classes • Implements one method: boolean accept(File directory, String name) - true if this directory, name acceptable Example class Java. Src. Filter implements File. Name. Filter { boolean accept(File d, String s) { if (s. endswith(“. java”)) return true; else return false; } }
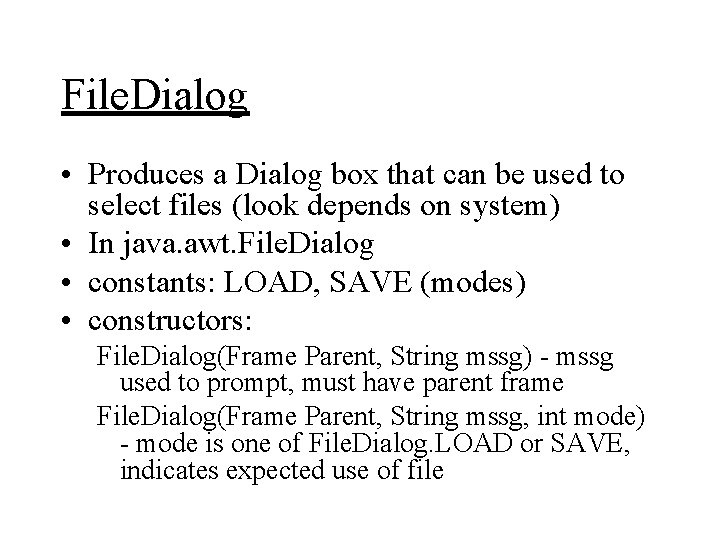
File. Dialog • Produces a Dialog box that can be used to select files (look depends on system) • In java. awt. File. Dialog • constants: LOAD, SAVE (modes) • constructors: File. Dialog(Frame Parent, String mssg) - mssg used to prompt, must have parent frame File. Dialog(Frame Parent, String mssg, int mode) - mode is one of File. Dialog. LOAD or SAVE, indicates expected use of file
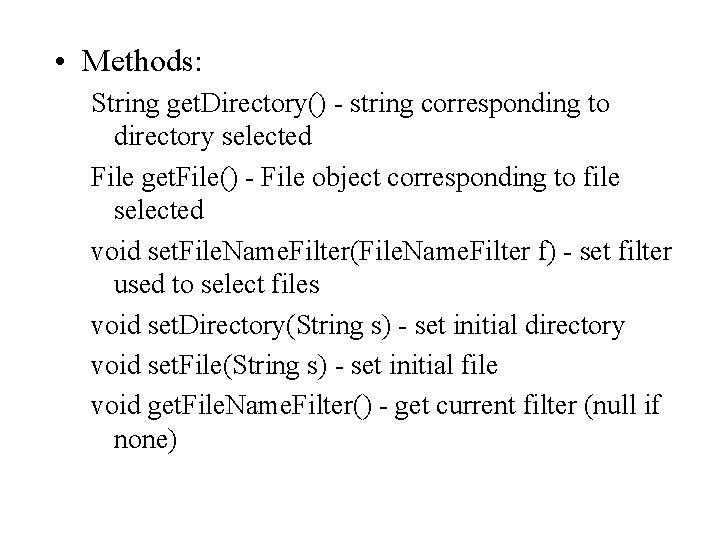
• Methods: String get. Directory() - string corresponding to directory selected File get. File() - File object corresponding to file selected void set. File. Name. Filter(File. Name. Filter f) - set filter used to select files void set. Directory(String s) - set initial directory void set. File(String s) - set initial file void get. File. Name. Filter() - get current filter (null if none)