Programming Languages and Compilers CS 421 Elsa L
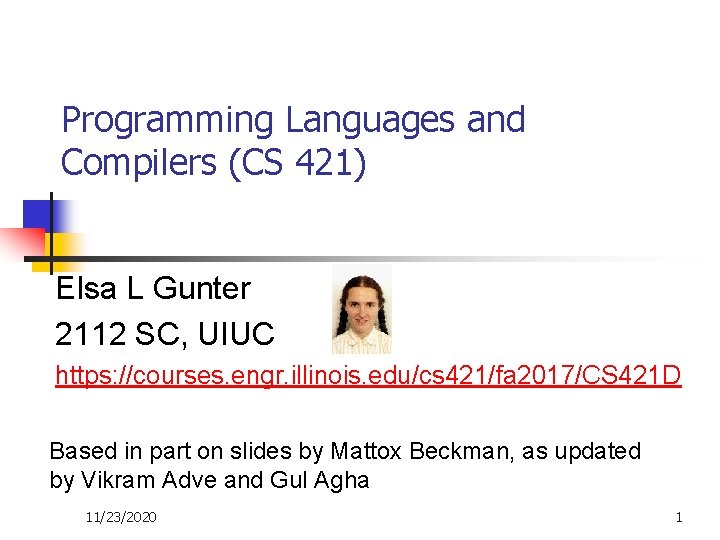
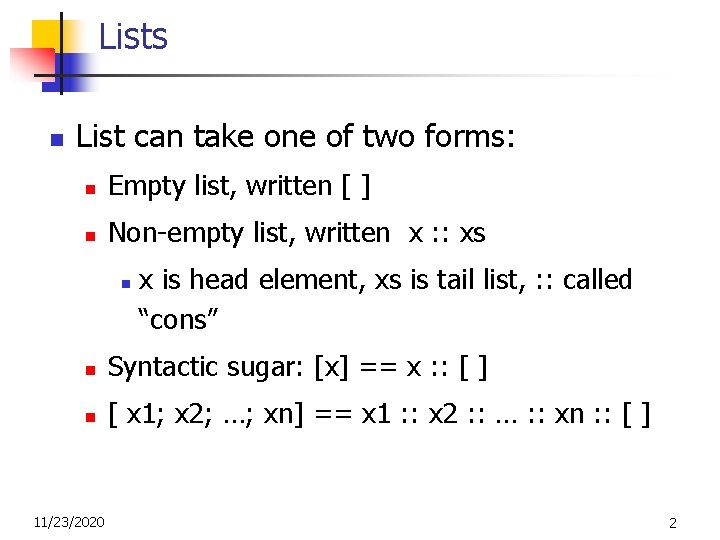
![Lists # let fib 5 = [8; 5; 3; 2; 1; 1]; ; val Lists # let fib 5 = [8; 5; 3; 2; 1; 1]; ; val](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-3.jpg)
![Lists are Homogeneous # let bad_list = [1; 3. 2; 7]; ; Characters 19 Lists are Homogeneous # let bad_list = [1; 3. 2; 7]; ; Characters 19](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-4.jpg)
![Question n Which one of these lists is invalid? 1. [2; 3; 4; 6] Question n Which one of these lists is invalid? 1. [2; 3; 4; 6]](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-5.jpg)
![Answer n Which one of these lists is invalid? 1. [2; 3; 4; 6] Answer n Which one of these lists is invalid? 1. [2; 3; 4; 6]](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-6.jpg)
![Functions Over Lists # let rec double_up list = match list with [ ] Functions Over Lists # let rec double_up list = match list with [ ]](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-7.jpg)
![Functions Over Lists # let silly = double_up ["hi"; "there"]; ; val silly : Functions Over Lists # let silly = double_up ["hi"; "there"]; ; val silly :](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-8.jpg)
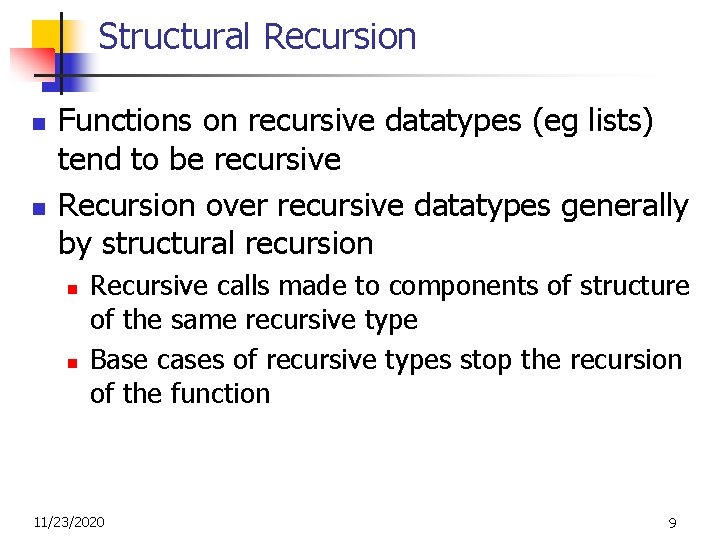
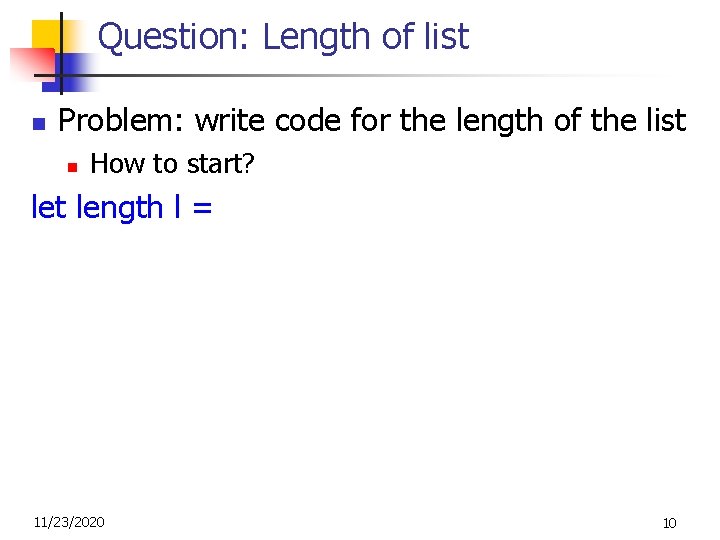
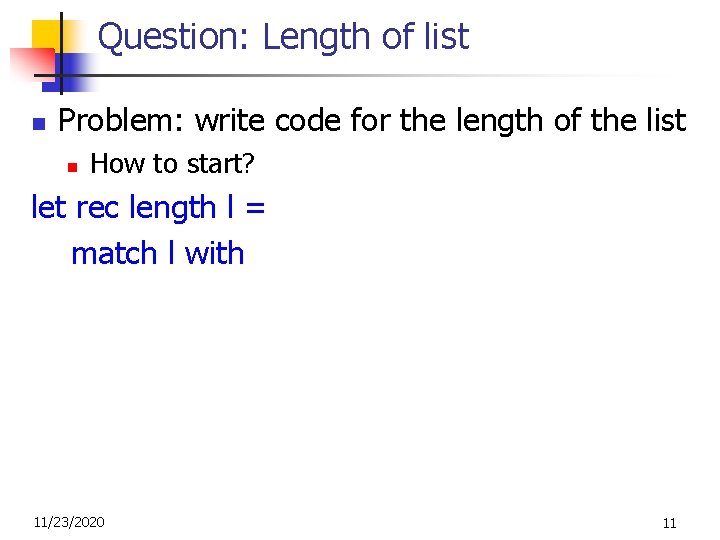
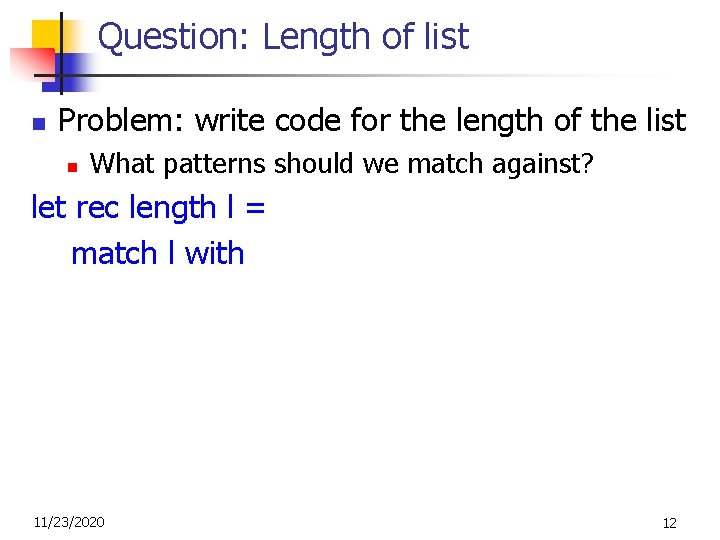
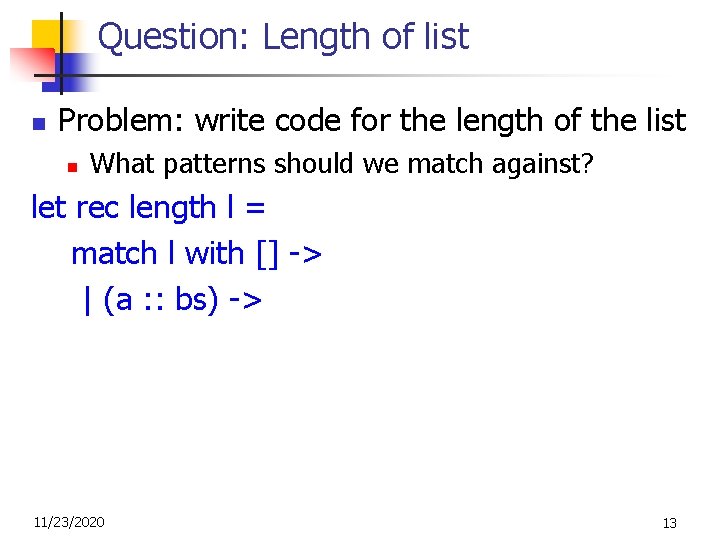
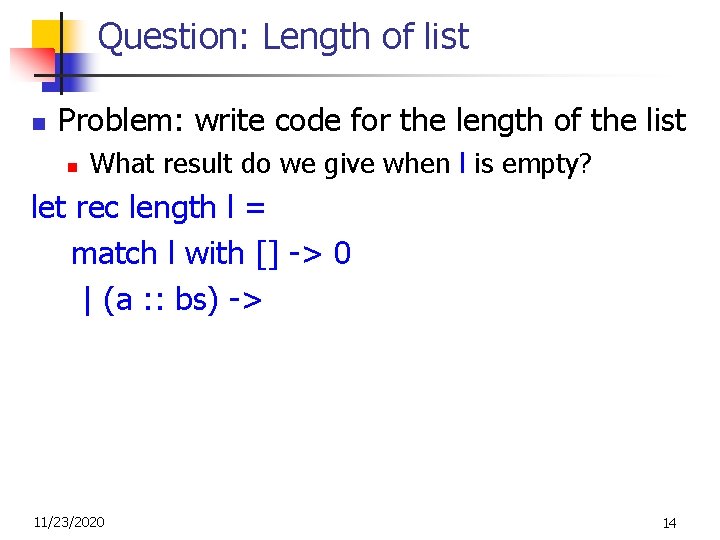
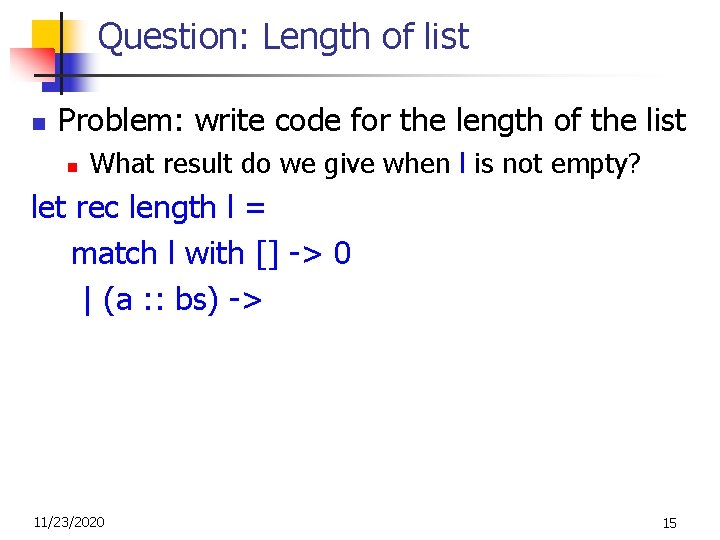
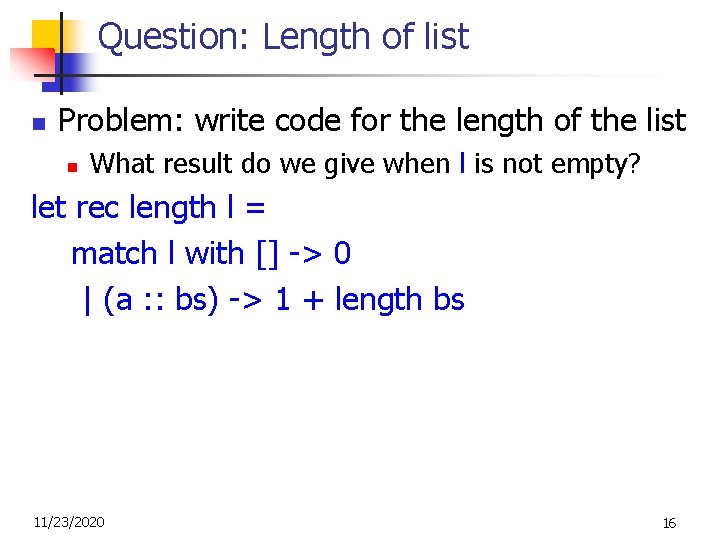
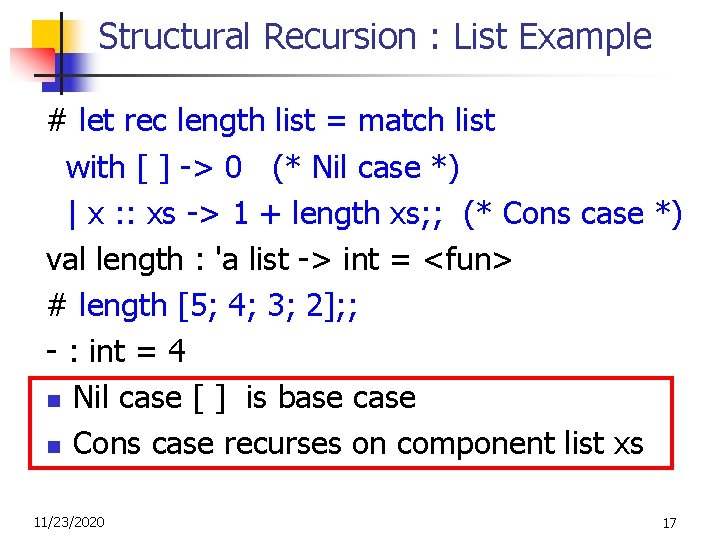
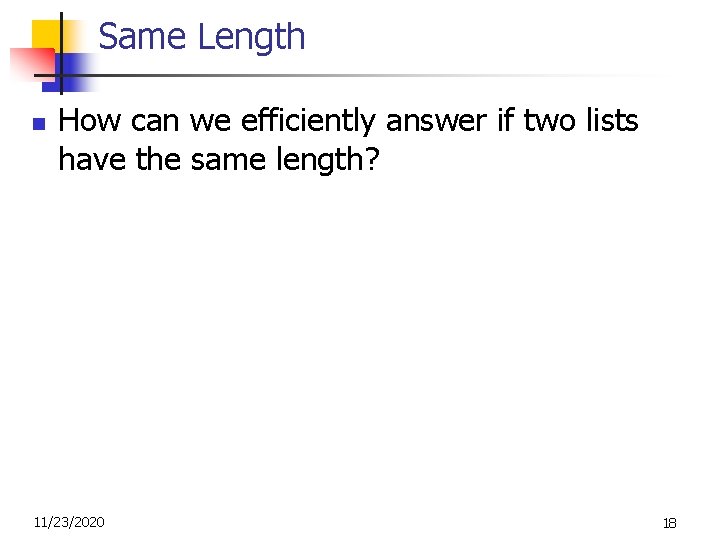
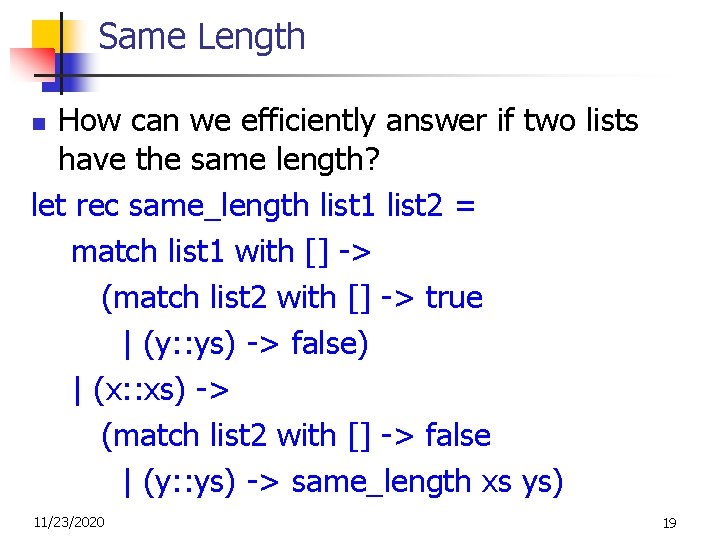
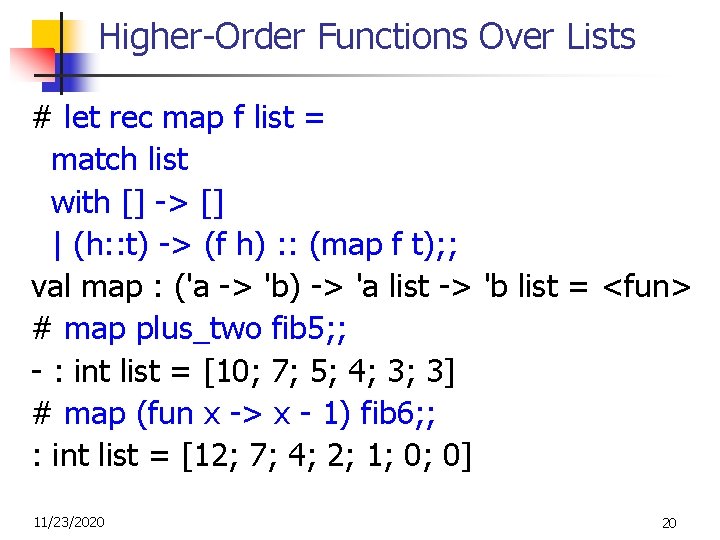
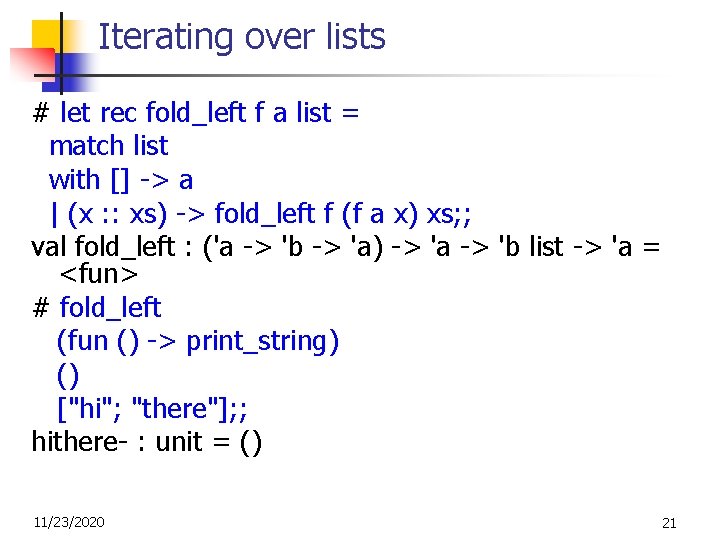
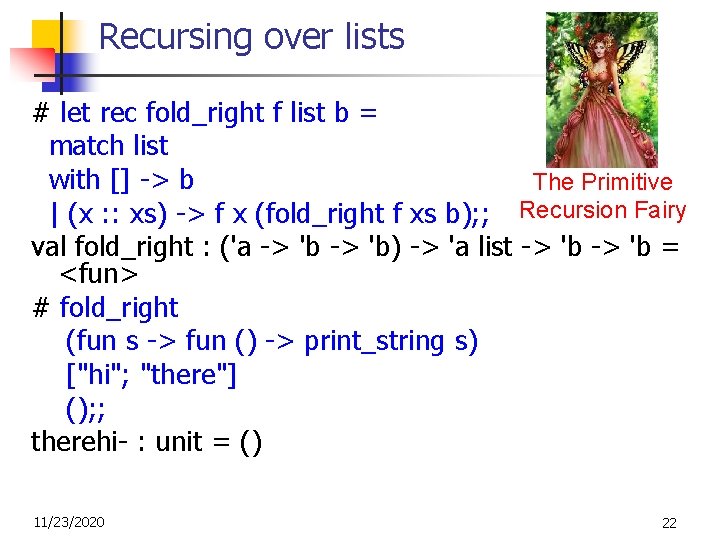
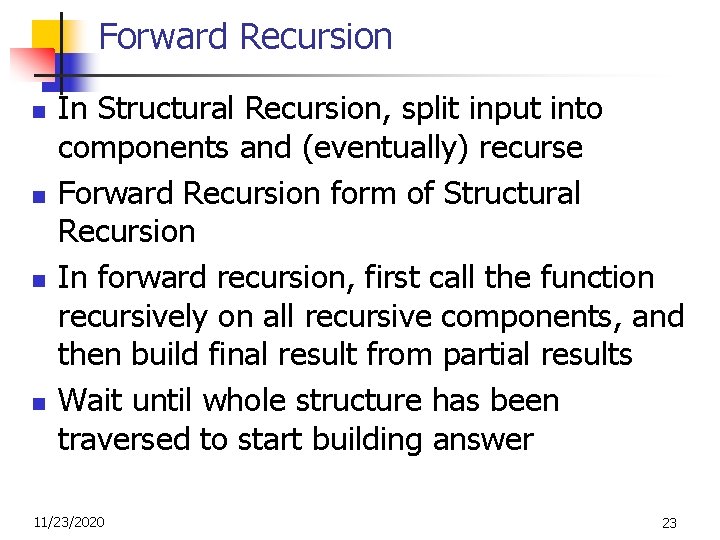
![Forward Recursion: Examples # let rec double_up list = match list with [ ] Forward Recursion: Examples # let rec double_up list = match list with [ ]](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-24.jpg)
![Forward Recursion: Examples # let rec double_up list = match list with [ ] Forward Recursion: Examples # let rec double_up list = match list with [ ]](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-25.jpg)
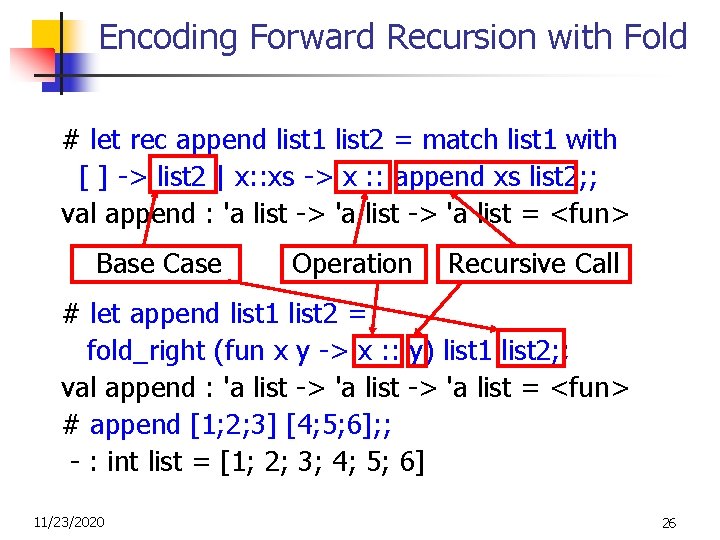
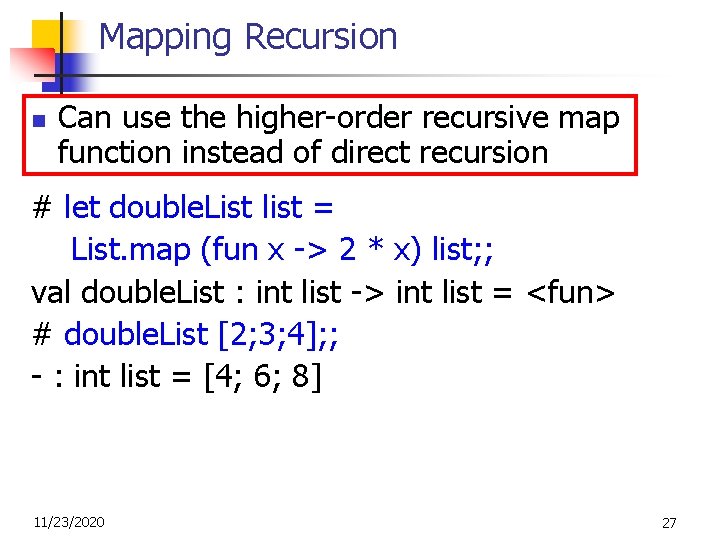
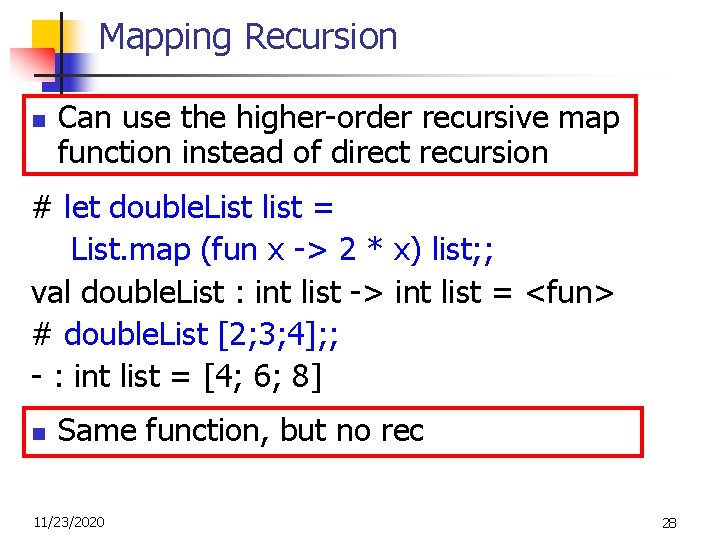
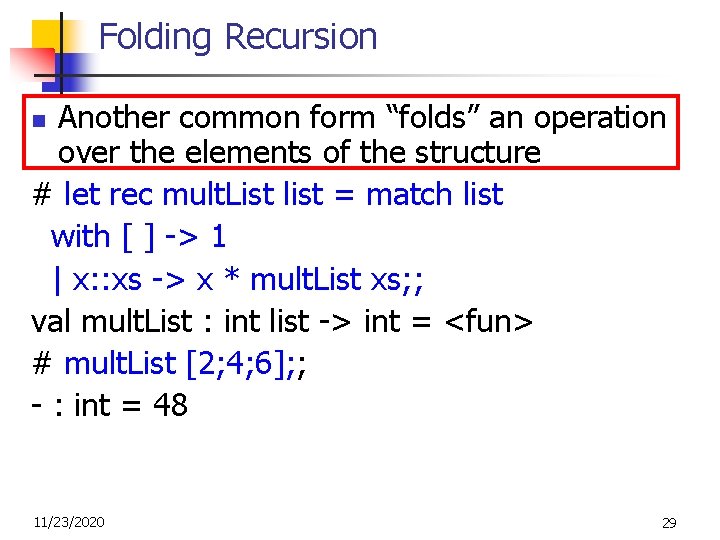
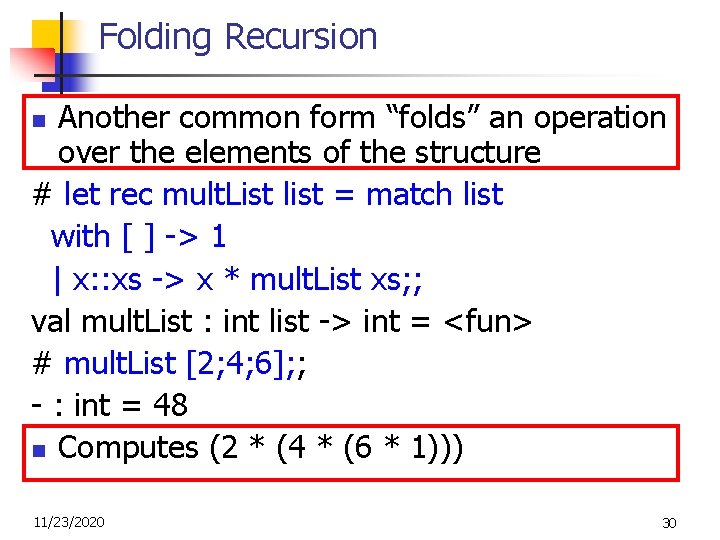
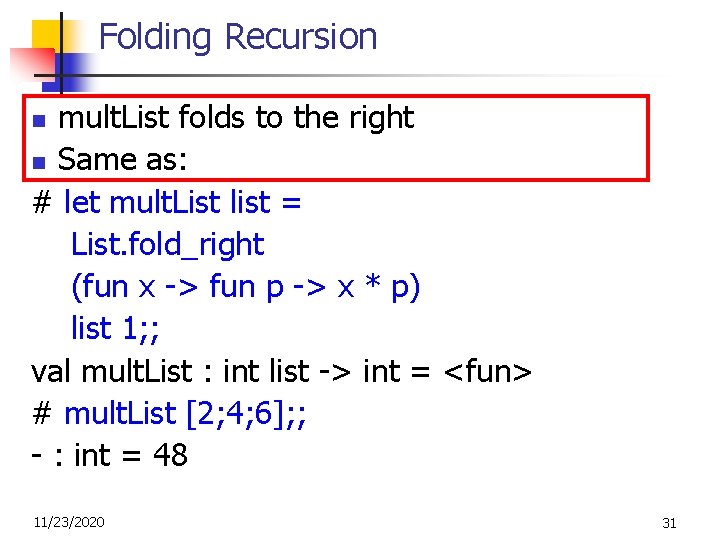
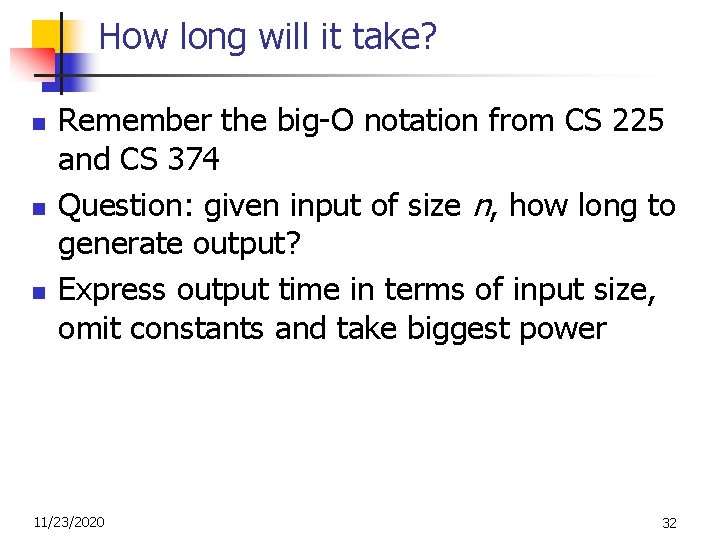
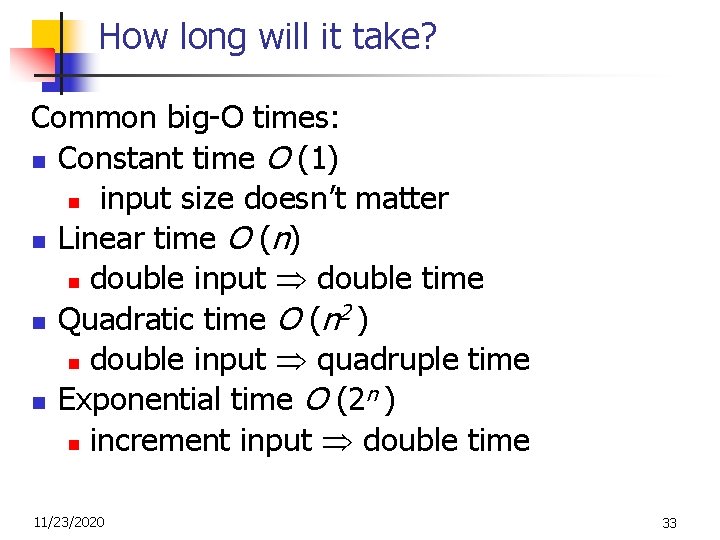
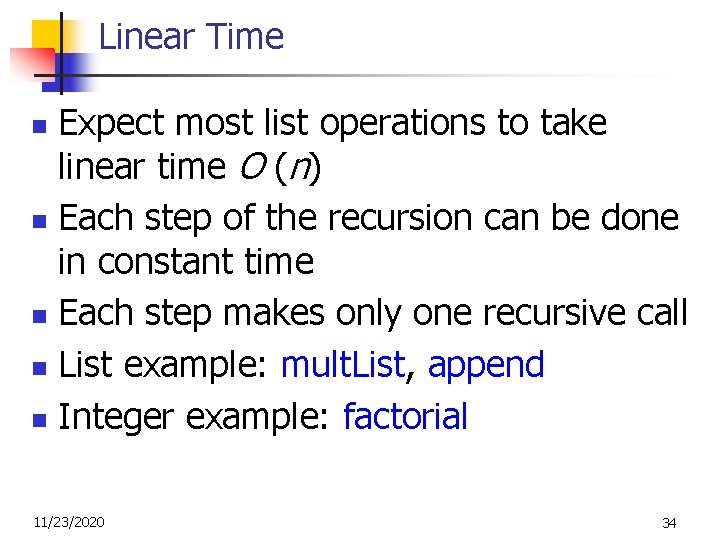
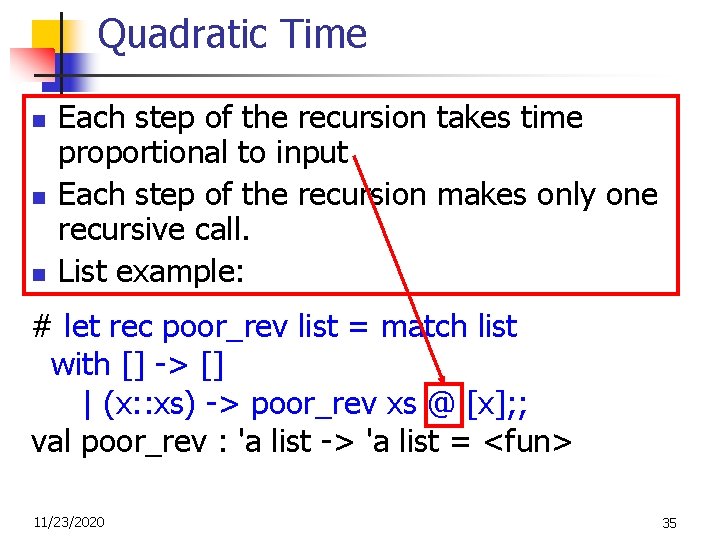
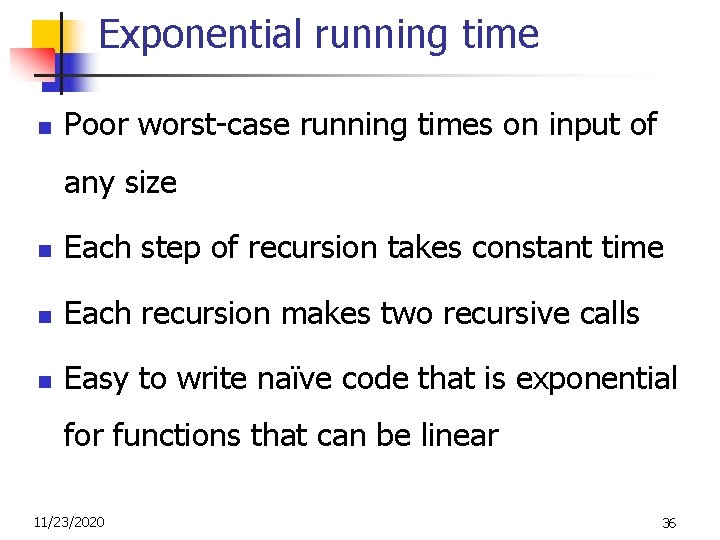
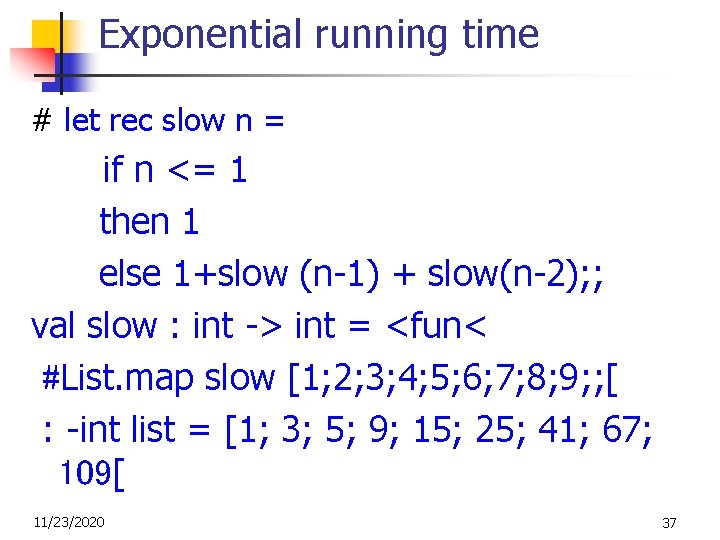
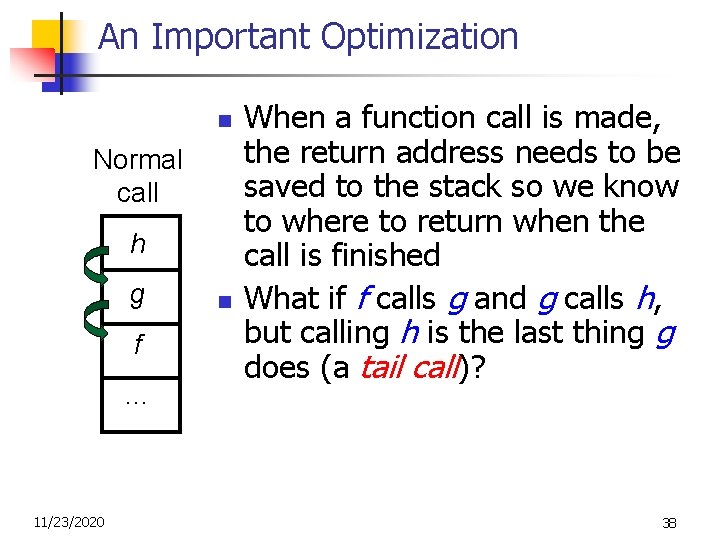
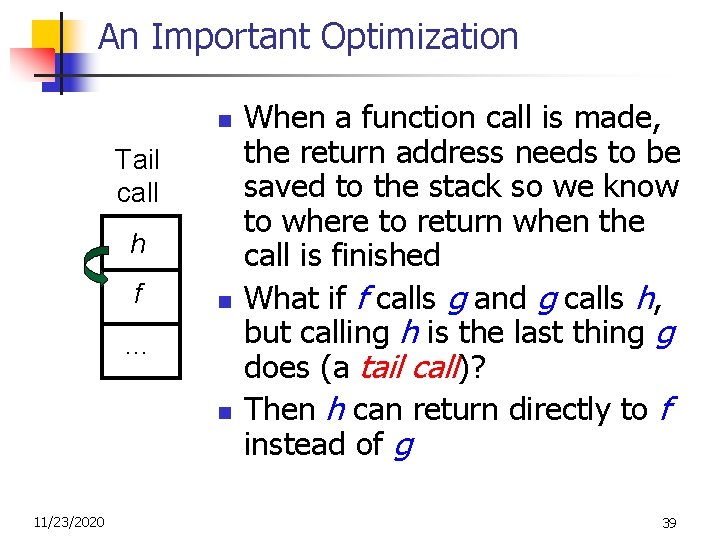
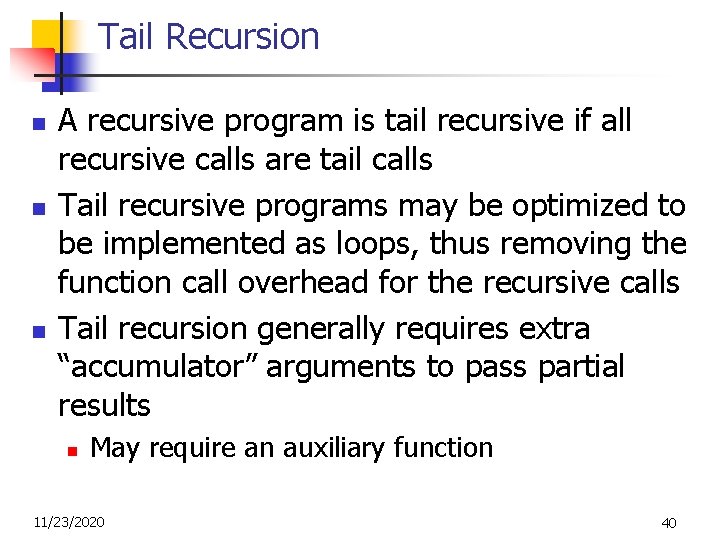
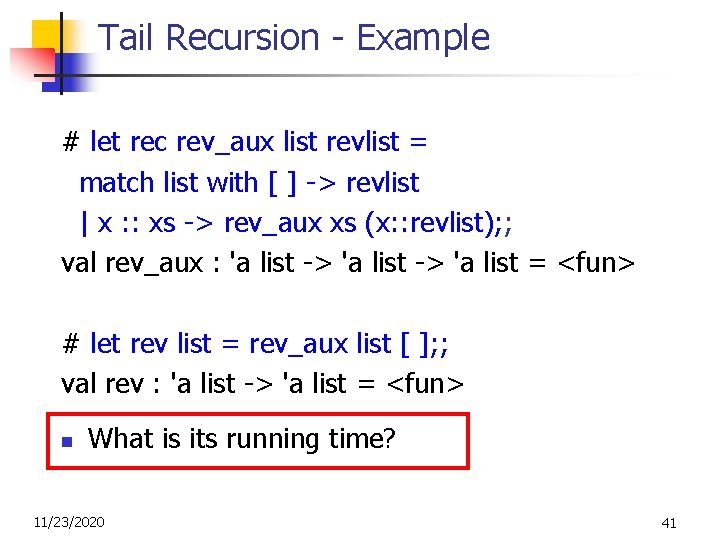
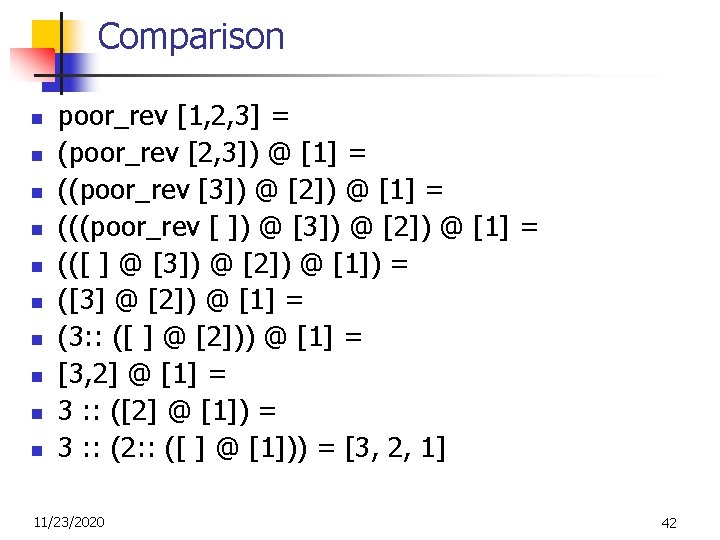
![Comparison n n rev [1, 2, 3] = rev_aux [1, 2, 3] [ ] Comparison n n rev [1, 2, 3] = rev_aux [1, 2, 3] [ ]](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-43.jpg)
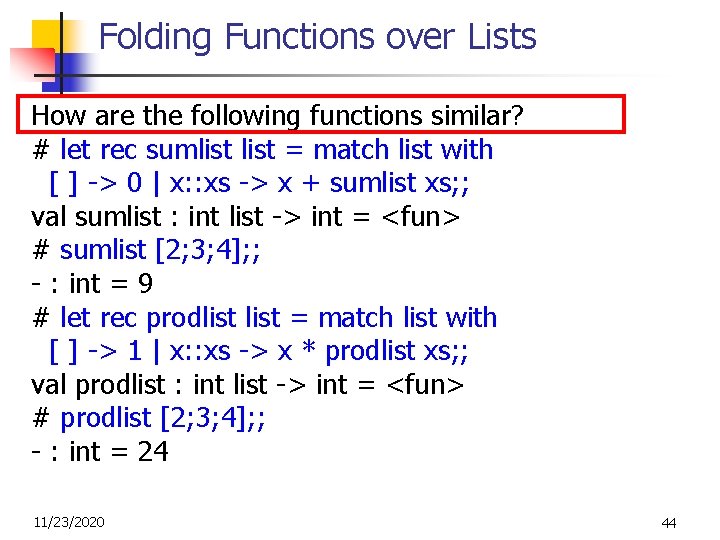
![Folding # let rec fold_left f a list = match list with [] -> Folding # let rec fold_left f a list = match list with [] ->](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-45.jpg)
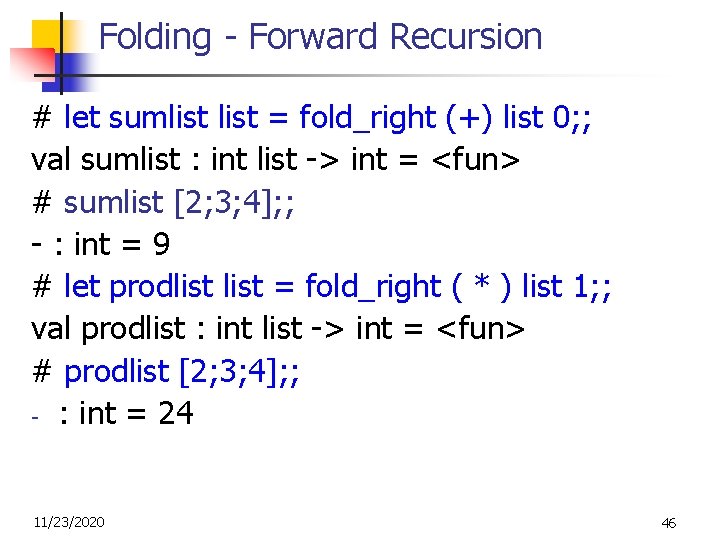
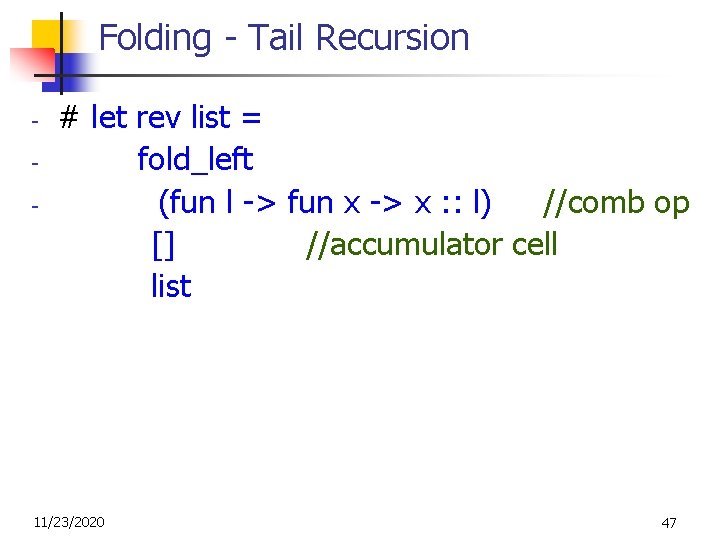
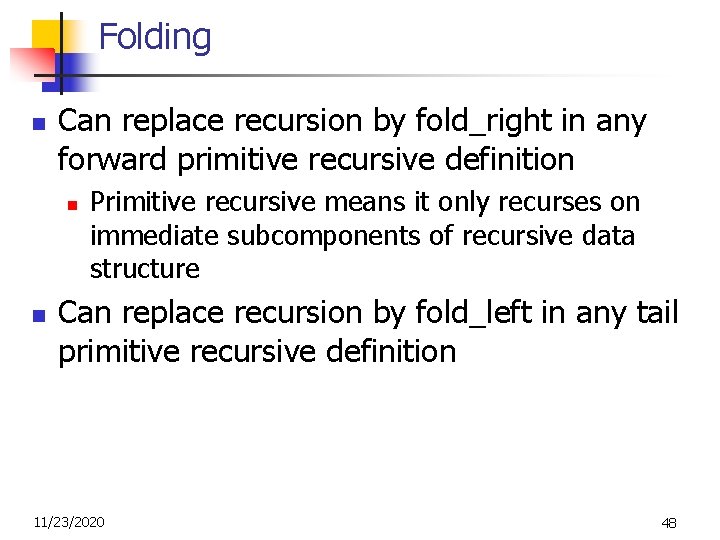
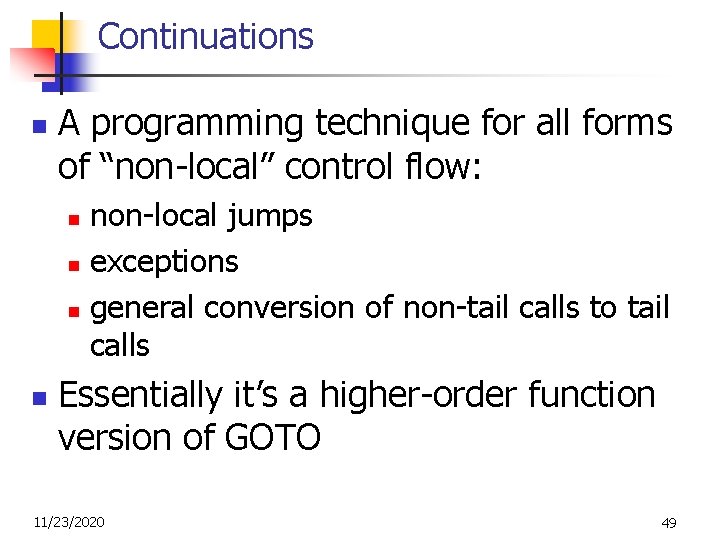
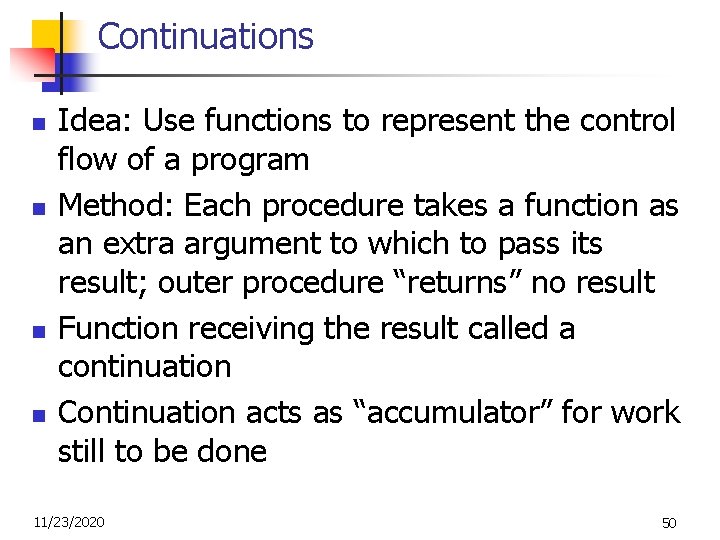
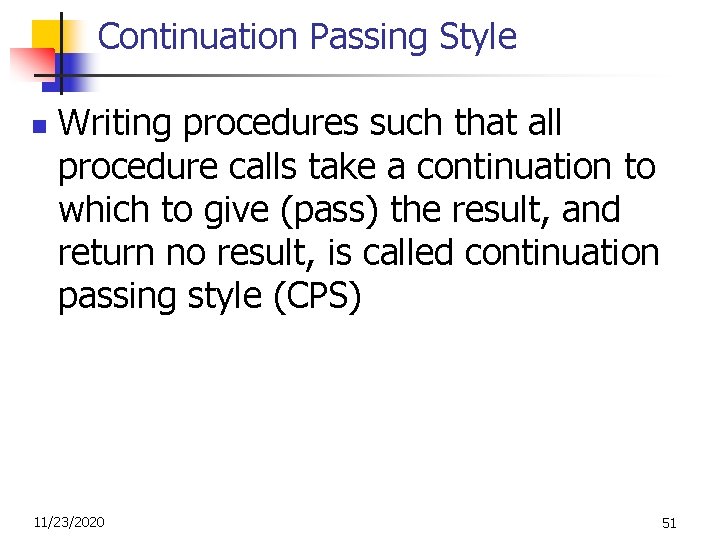
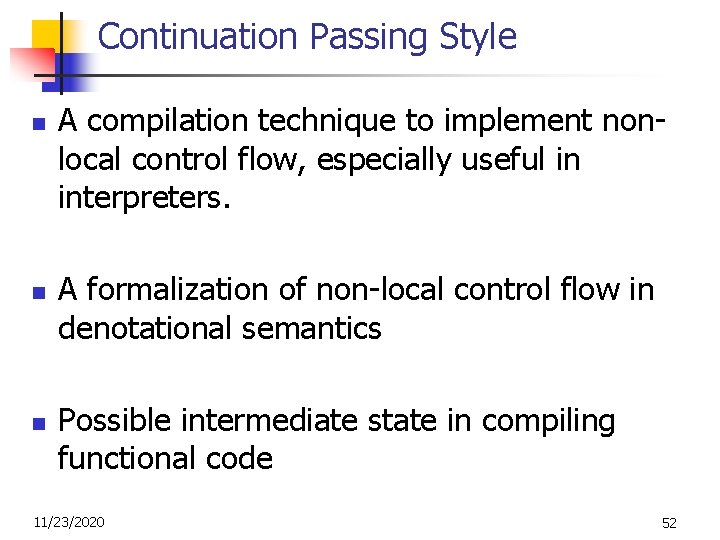
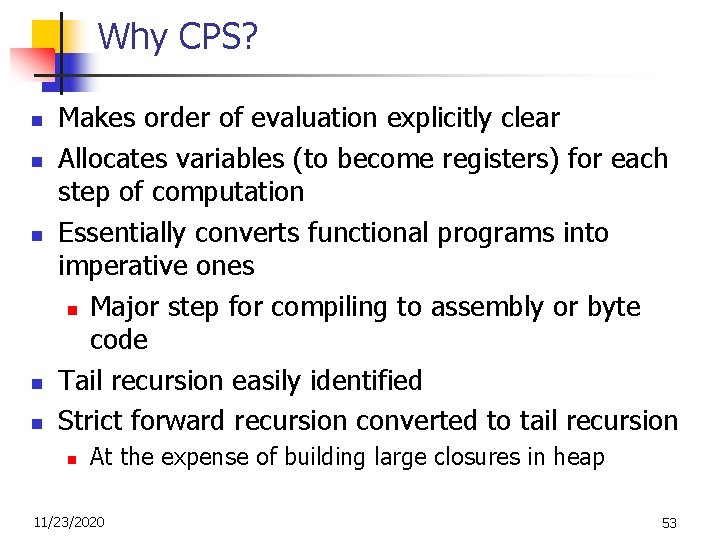
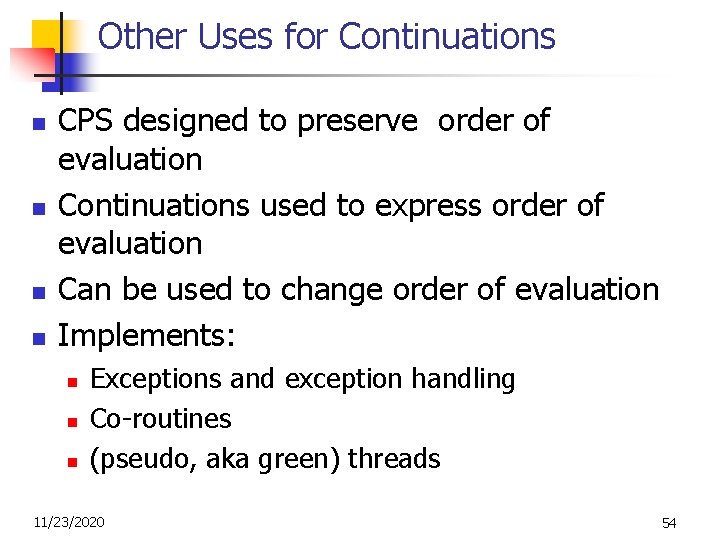
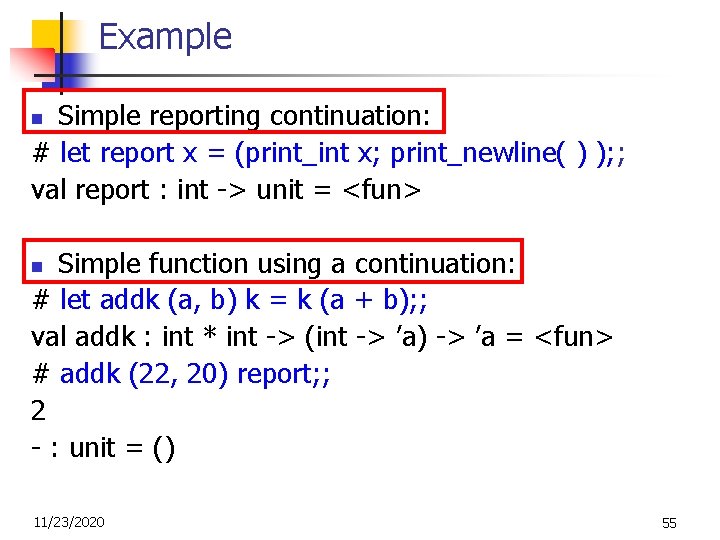
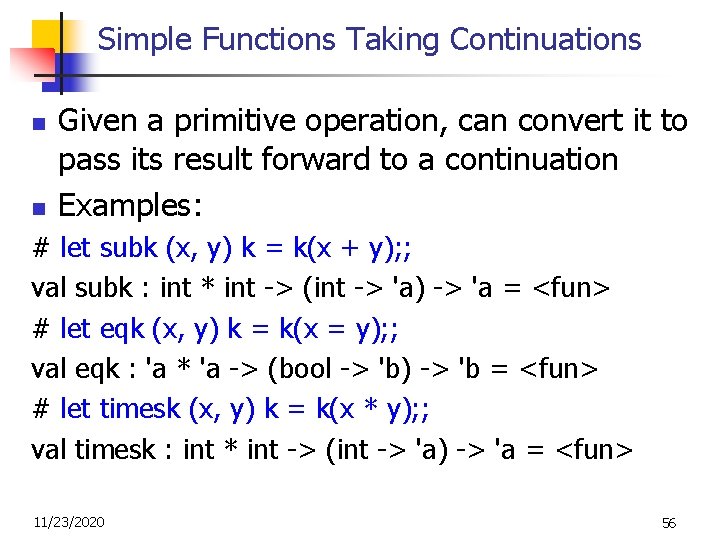
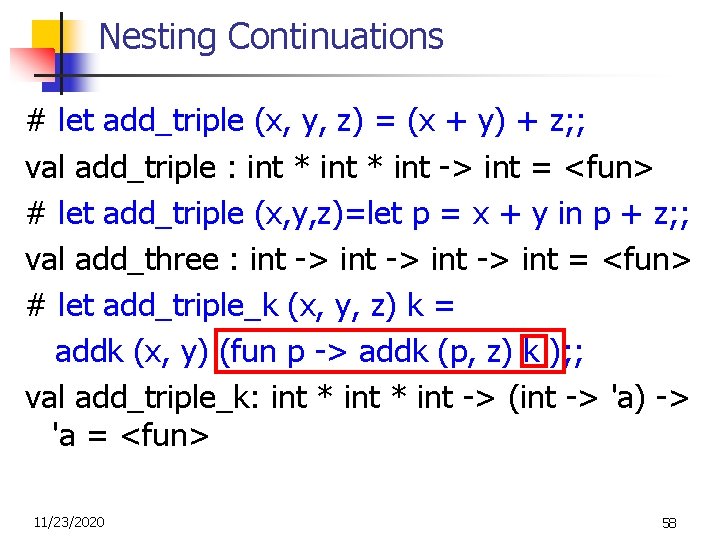
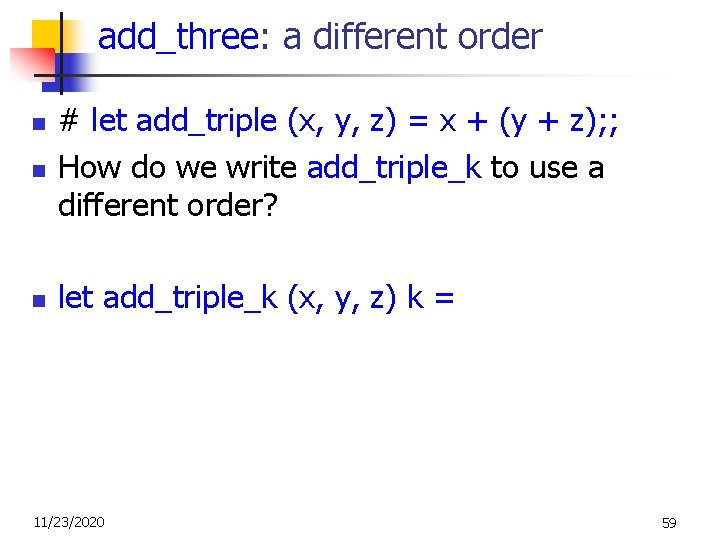
- Slides: 58
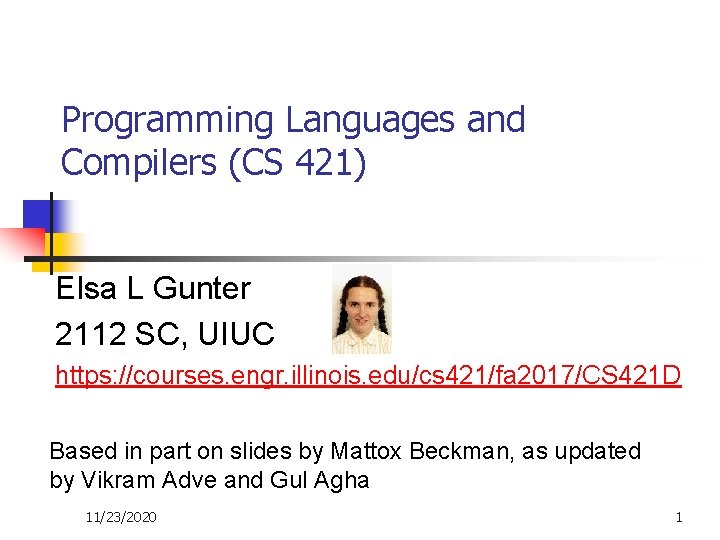
Programming Languages and Compilers (CS 421) Elsa L Gunter 2112 SC, UIUC https: //courses. engr. illinois. edu/cs 421/fa 2017/CS 421 D Based in part on slides by Mattox Beckman, as updated by Vikram Adve and Gul Agha 11/23/2020 1
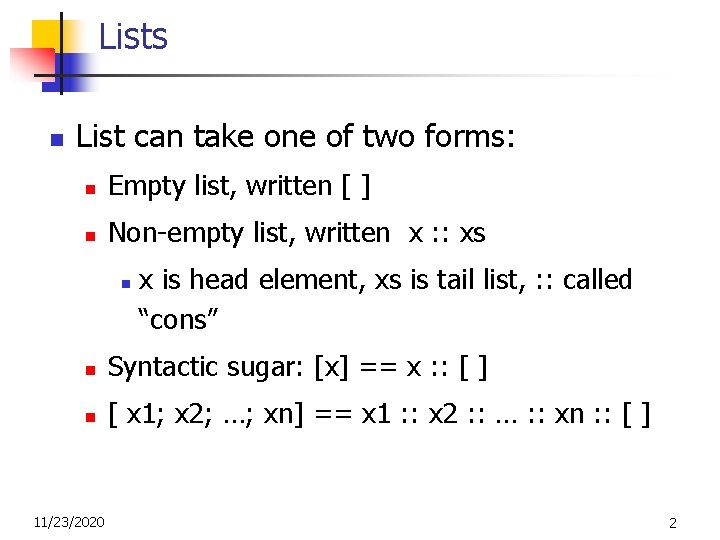
Lists n List can take one of two forms: n Empty list, written [ ] n Non-empty list, written x : : xs n x is head element, xs is tail list, : : called “cons” n Syntactic sugar: [x] == x : : [ ] n [ x 1; x 2; …; xn] == x 1 : : x 2 : : … : : xn : : [ ] 11/23/2020 2
![Lists let fib 5 8 5 3 2 1 1 val Lists # let fib 5 = [8; 5; 3; 2; 1; 1]; ; val](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-3.jpg)
Lists # let fib 5 = [8; 5; 3; 2; 1; 1]; ; val fib 5 : int list = [8; 5; 3; 2; 1; 1] # let fib 6 = 13 : : fib 5; ; val fib 6 : int list = [13; 8; 5; 3; 2; 1; 1] # (8: : 5: : 3: : 2: : 1: : [ ]) = fib 5; ; - : bool = true # fib 5 @ fib 6; ; - : int list = [8; 5; 3; 2; 1; 1; 13; 8; 5; 3; 2; 1; 1] 11/23/2020 3
![Lists are Homogeneous let badlist 1 3 2 7 Characters 19 Lists are Homogeneous # let bad_list = [1; 3. 2; 7]; ; Characters 19](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-4.jpg)
Lists are Homogeneous # let bad_list = [1; 3. 2; 7]; ; Characters 19 -22: let bad_list = [1; 3. 2; 7]; ; ^^^ This expression has type float but is here used with type int 11/23/2020 4
![Question n Which one of these lists is invalid 1 2 3 4 6 Question n Which one of these lists is invalid? 1. [2; 3; 4; 6]](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-5.jpg)
Question n Which one of these lists is invalid? 1. [2; 3; 4; 6] 2. [2, 3; 4, 5; 6, 7] 3. [(2. 3, 4); (3. 2, 5); (6, 7. 2)] 4. [[“hi”; “there”]; [“wahcha”]; [“doin”]] 11/23/2020 5
![Answer n Which one of these lists is invalid 1 2 3 4 6 Answer n Which one of these lists is invalid? 1. [2; 3; 4; 6]](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-6.jpg)
Answer n Which one of these lists is invalid? 1. [2; 3; 4; 6] 2. [2, 3; 4, 5; 6, 7] 3. [(2. 3, 4); (3. 2, 5); (6, 7. 2)] 4. [[“hi”; “there”]; [“wahcha”]; [“doin”]] § 3 is invalid because of last pair 11/23/2020 6
![Functions Over Lists let rec doubleup list match list with Functions Over Lists # let rec double_up list = match list with [ ]](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-7.jpg)
Functions Over Lists # let rec double_up list = match list with [ ] -> [ ] (* pattern before ->, expression after *) | (x : : xs) -> (x : : double_up xs); ; val double_up : 'a list -> 'a list = <fun> # let fib 5_2 = double_up fib 5; ; val fib 5_2 : int list = [8; 8; 5; 5; 3; 3; 2; 2; 1; 1] 11/23/2020 7
![Functions Over Lists let silly doubleup hi there val silly Functions Over Lists # let silly = double_up ["hi"; "there"]; ; val silly :](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-8.jpg)
Functions Over Lists # let silly = double_up ["hi"; "there"]; ; val silly : string list = ["hi"; "there"; "there"] # let rec poor_rev list = match list with [] -> [] | (x: : xs) -> poor_rev xs @ [x]; ; val poor_rev : 'a list -> 'a list = <fun> # poor_rev silly; ; - : string list = ["there"; "hi"; "hi"] 11/23/2020 8
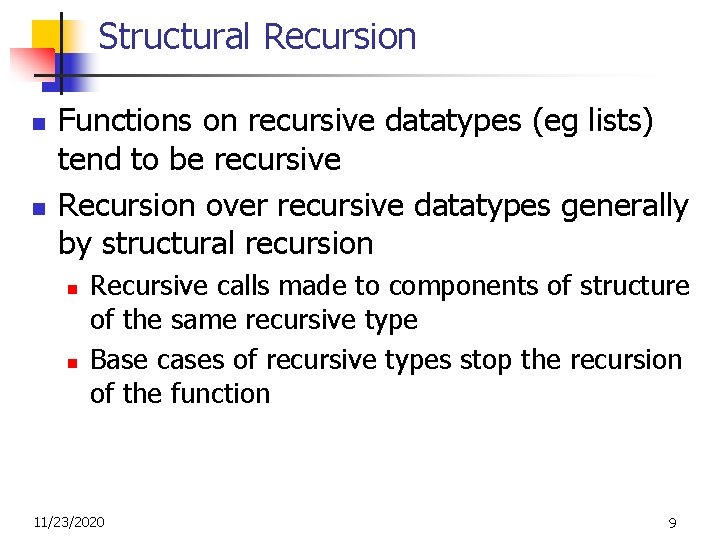
Structural Recursion n n Functions on recursive datatypes (eg lists) tend to be recursive Recursion over recursive datatypes generally by structural recursion n n Recursive calls made to components of structure of the same recursive type Base cases of recursive types stop the recursion of the function 11/23/2020 9
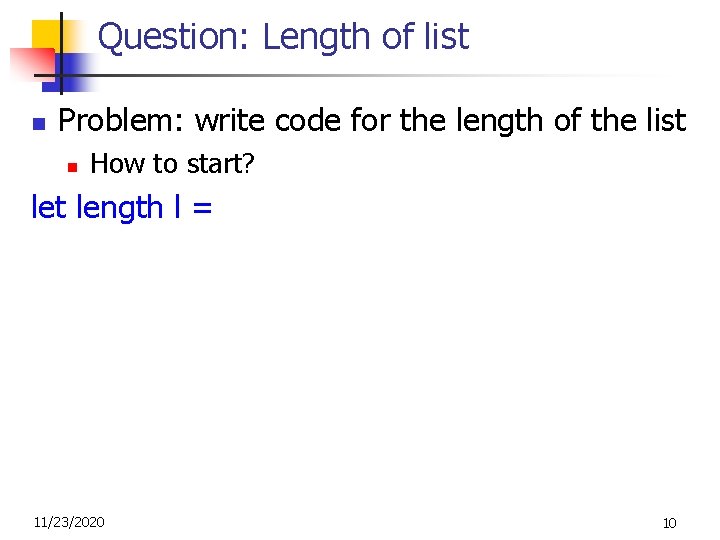
Question: Length of list n Problem: write code for the length of the list n How to start? let length l = 11/23/2020 10
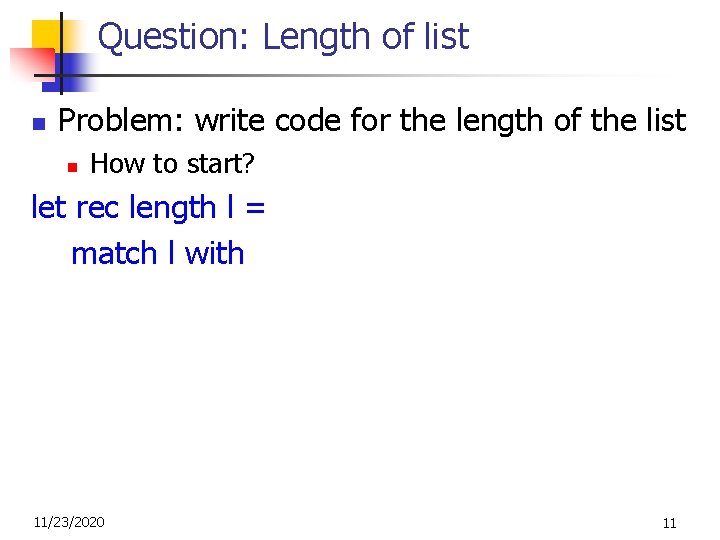
Question: Length of list n Problem: write code for the length of the list n How to start? let rec length l = match l with 11/23/2020 11
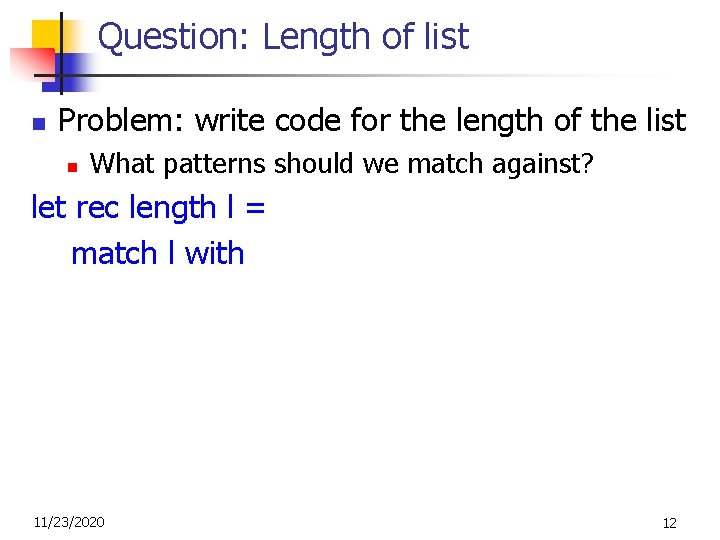
Question: Length of list n Problem: write code for the length of the list n What patterns should we match against? let rec length l = match l with 11/23/2020 12
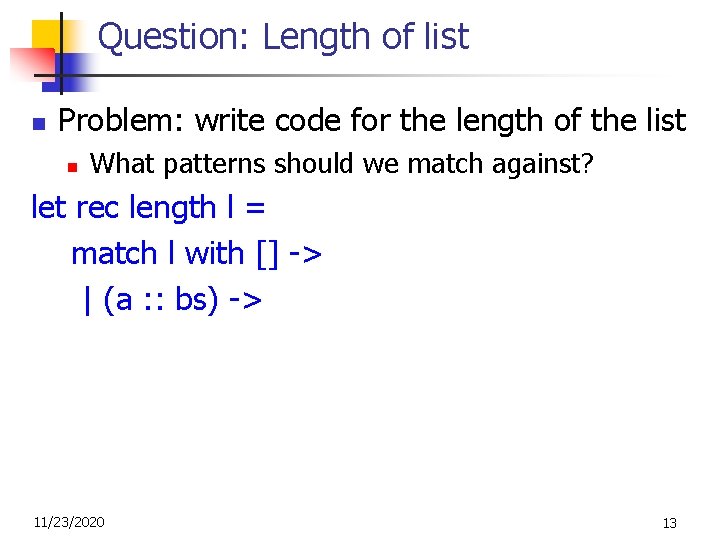
Question: Length of list n Problem: write code for the length of the list n What patterns should we match against? let rec length l = match l with [] -> | (a : : bs) -> 11/23/2020 13
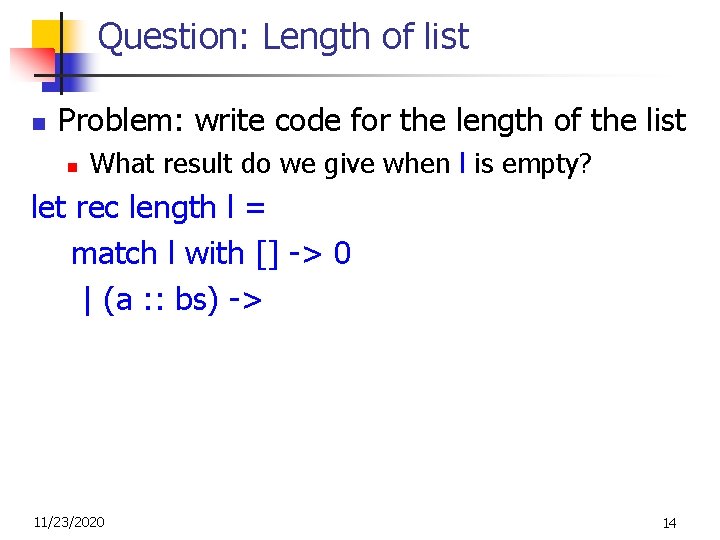
Question: Length of list n Problem: write code for the length of the list n What result do we give when l is empty? let rec length l = match l with [] -> 0 | (a : : bs) -> 11/23/2020 14
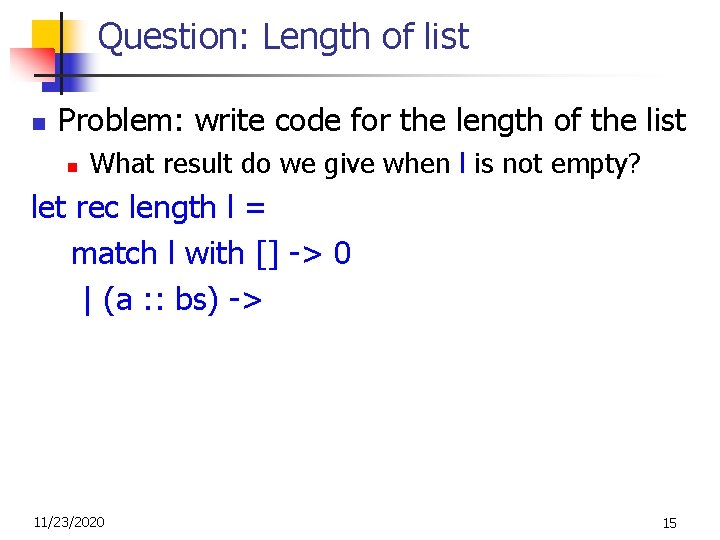
Question: Length of list n Problem: write code for the length of the list n What result do we give when l is not empty? let rec length l = match l with [] -> 0 | (a : : bs) -> 11/23/2020 15
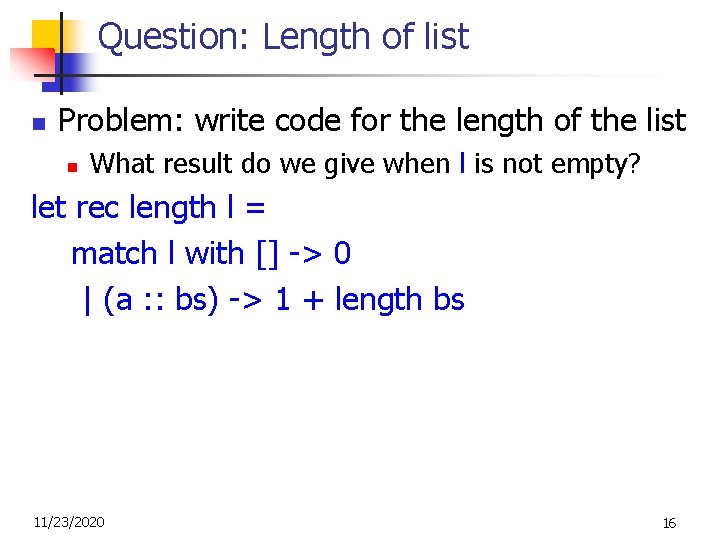
Question: Length of list n Problem: write code for the length of the list n What result do we give when l is not empty? let rec length l = match l with [] -> 0 | (a : : bs) -> 1 + length bs 11/23/2020 16
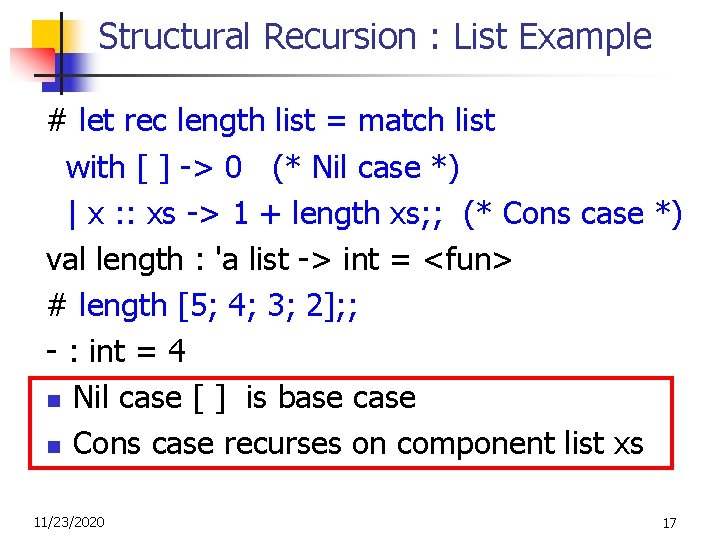
Structural Recursion : List Example # let rec length list = match list with [ ] -> 0 (* Nil case *) | x : : xs -> 1 + length xs; ; (* Cons case *) val length : 'a list -> int = <fun> # length [5; 4; 3; 2]; ; - : int = 4 n Nil case [ ] is base case n Cons case recurses on component list xs 11/23/2020 17
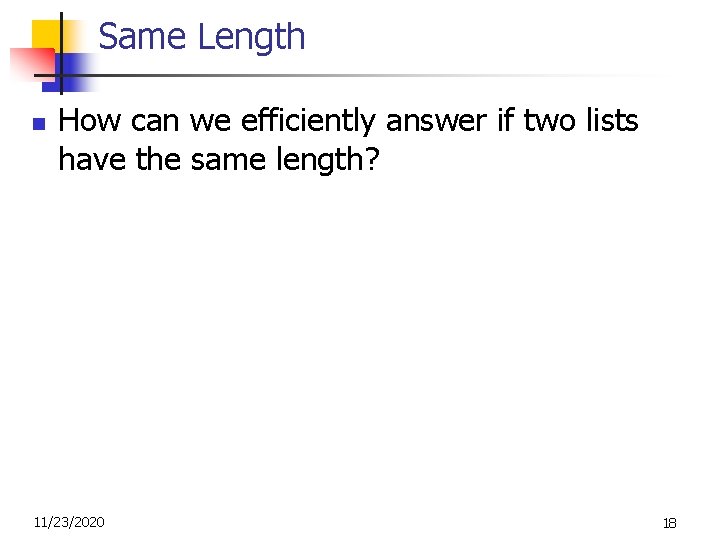
Same Length n How can we efficiently answer if two lists have the same length? 11/23/2020 18
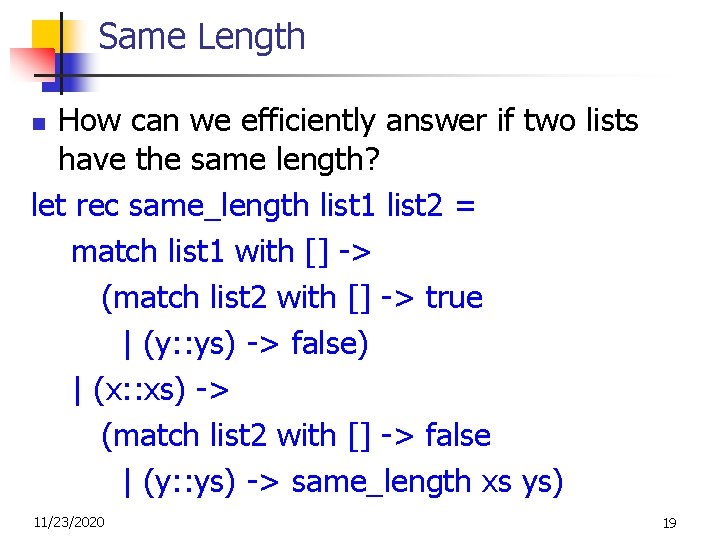
Same Length How can we efficiently answer if two lists have the same length? let rec same_length list 1 list 2 = match list 1 with [] -> (match list 2 with [] -> true | (y: : ys) -> false) | (x: : xs) -> (match list 2 with [] -> false | (y: : ys) -> same_length xs ys) n 11/23/2020 19
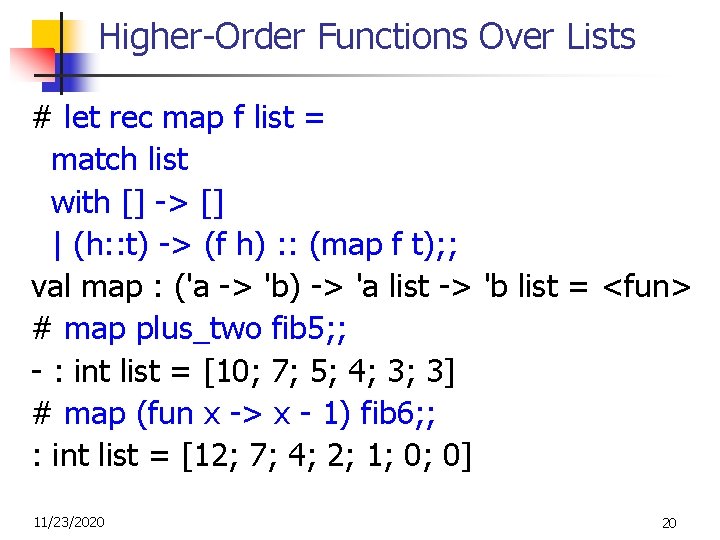
Higher-Order Functions Over Lists # let rec map f list = match list with [] -> [] | (h: : t) -> (f h) : : (map f t); ; val map : ('a -> 'b) -> 'a list -> 'b list = <fun> # map plus_two fib 5; ; - : int list = [10; 7; 5; 4; 3; 3] # map (fun x -> x - 1) fib 6; ; : int list = [12; 7; 4; 2; 1; 0; 0] 11/23/2020 20
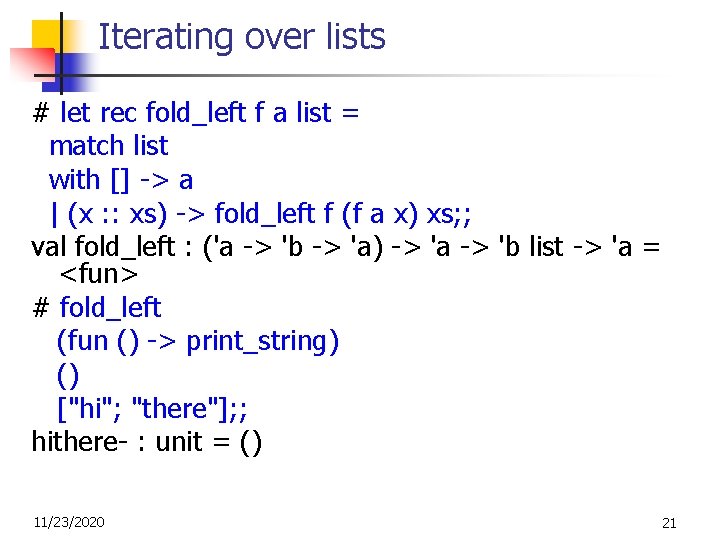
Iterating over lists # let rec fold_left f a list = match list with [] -> a | (x : : xs) -> fold_left f (f a x) xs; ; val fold_left : ('a -> 'b -> 'a) -> 'a -> 'b list -> 'a = <fun> # fold_left (fun () -> print_string) () ["hi"; "there"]; ; hithere- : unit = () 11/23/2020 21
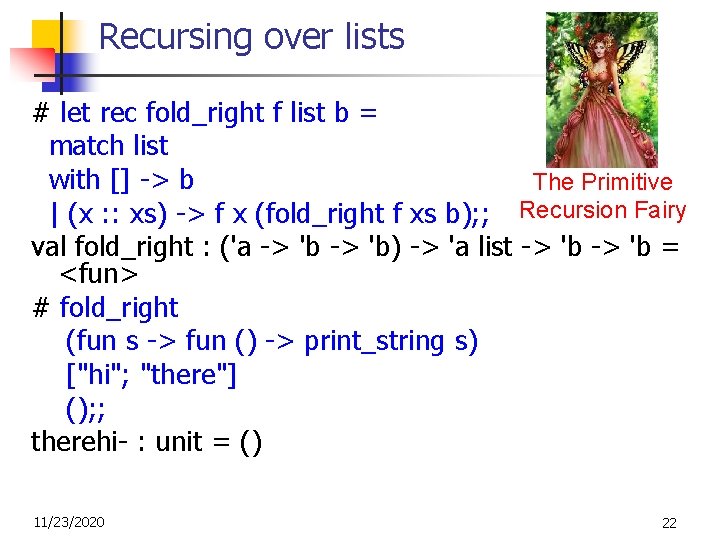
Recursing over lists # let rec fold_right f list b = match list with [] -> b The Primitive | (x : : xs) -> f x (fold_right f xs b); ; Recursion Fairy val fold_right : ('a -> 'b) -> 'a list -> 'b = <fun> # fold_right (fun s -> fun () -> print_string s) ["hi"; "there"] (); ; therehi- : unit = () 11/23/2020 22
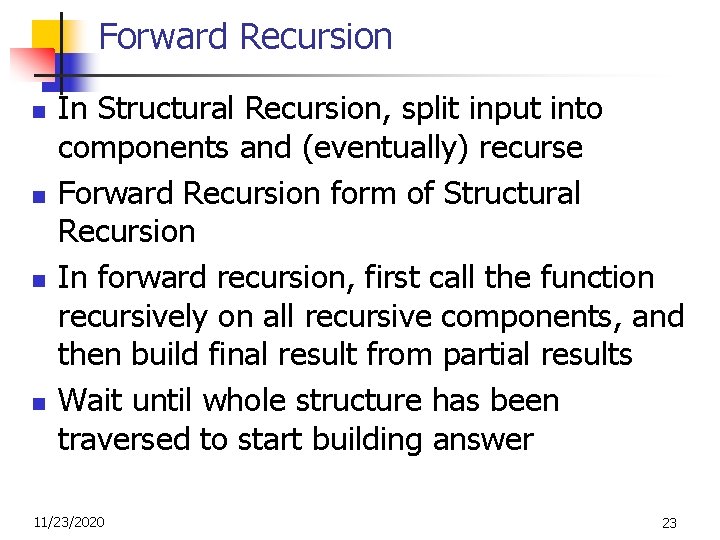
Forward Recursion n n In Structural Recursion, split input into components and (eventually) recurse Forward Recursion form of Structural Recursion In forward recursion, first call the function recursively on all recursive components, and then build final result from partial results Wait until whole structure has been traversed to start building answer 11/23/2020 23
![Forward Recursion Examples let rec doubleup list match list with Forward Recursion: Examples # let rec double_up list = match list with [ ]](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-24.jpg)
Forward Recursion: Examples # let rec double_up list = match list with [ ] -> [ ] | (x : : xs) -> (x : : double_up xs); ; val double_up : 'a list -> 'a list = <fun> # let rec poor_rev list = match list with [] -> [] | (x: : xs) -> poor_rev xs @ [x]; ; val poor_rev : 'a list -> 'a list = <fun> 11/23/2020 24
![Forward Recursion Examples let rec doubleup list match list with Forward Recursion: Examples # let rec double_up list = match list with [ ]](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-25.jpg)
Forward Recursion: Examples # let rec double_up list = match list with [ ] -> [ ] | (x : : xs) -> (x : : double_up xs); ; val double_up : 'a list -> 'a list = <fun> Base Case Operator Recursive Call # let rec poor_rev list = match list with [] -> [] | (x: : xs) -> poor_rev xs @ [x]; ; val poor_rev : 'a list -> 'a list = <fun> Base Case Operator Recursive Call 11/23/2020 25
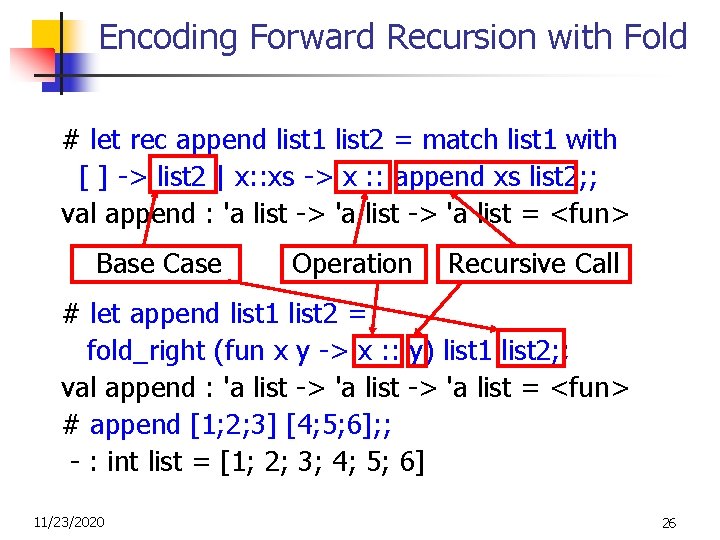
Encoding Forward Recursion with Fold # let rec append list 1 list 2 = match list 1 with [ ] -> list 2 | x: : xs -> x : : append xs list 2; ; val append : 'a list -> 'a list = <fun> Base Case Operation Recursive Call # let append list 1 list 2 = fold_right (fun x y -> x : : y) list 1 list 2; ; val append : 'a list -> 'a list = <fun> # append [1; 2; 3] [4; 5; 6]; ; - : int list = [1; 2; 3; 4; 5; 6] 11/23/2020 26
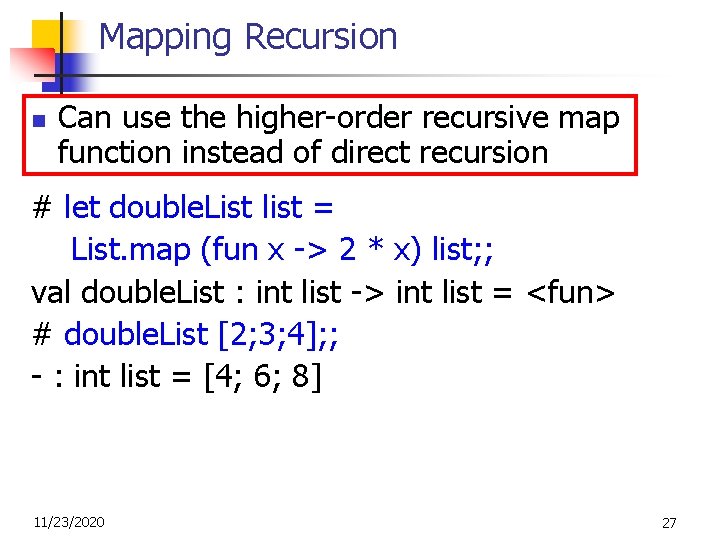
Mapping Recursion n Can use the higher-order recursive map function instead of direct recursion # let double. List list = List. map (fun x -> 2 * x) list; ; val double. List : int list -> int list = <fun> # double. List [2; 3; 4]; ; - : int list = [4; 6; 8] Same function, but no rec 11/23/2020 27
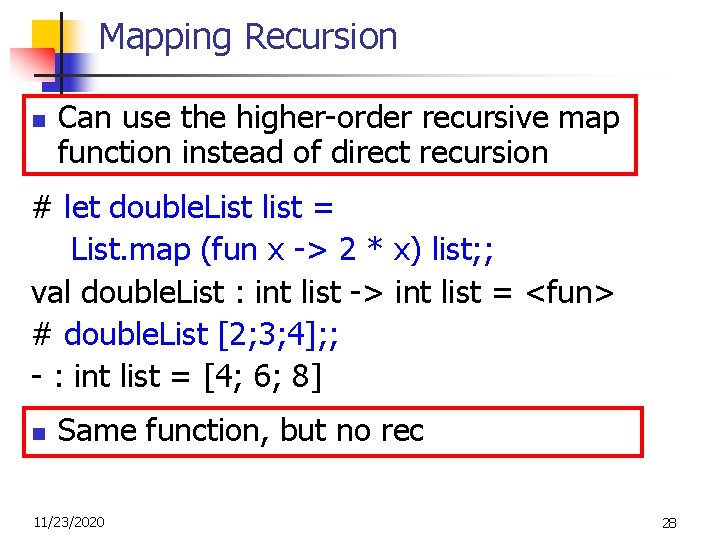
Mapping Recursion n Can use the higher-order recursive map function instead of direct recursion # let double. List list = List. map (fun x -> 2 * x) list; ; val double. List : int list -> int list = <fun> # double. List [2; 3; 4]; ; - : int list = [4; 6; 8] n Same function, but no rec 11/23/2020 28
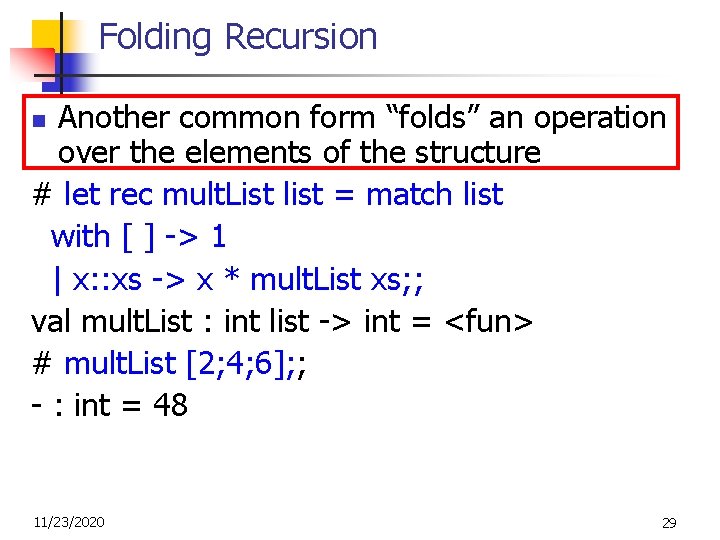
Folding Recursion Another common form “folds” an operation over the elements of the structure # let rec mult. List list = match list with [ ] -> 1 | x: : xs -> x * mult. List xs; ; val mult. List : int list -> int = <fun> # mult. List [2; 4; 6]; ; - : int = 48 n 11/23/2020 29
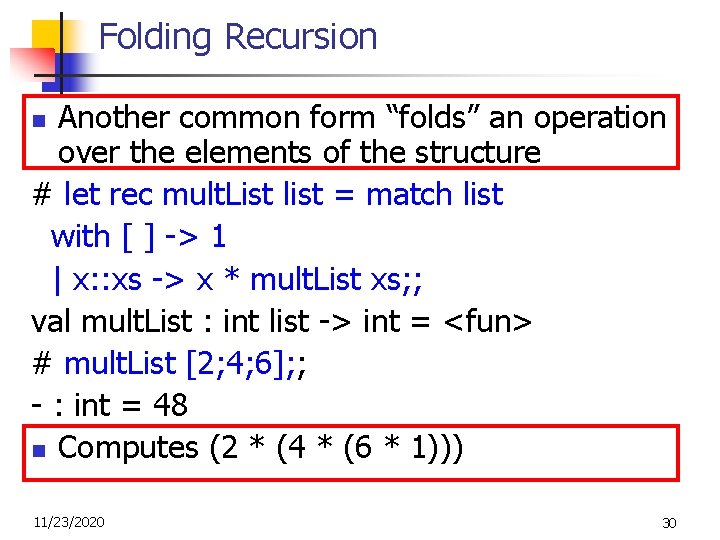
Folding Recursion Another common form “folds” an operation over the elements of the structure # let rec mult. List list = match list with [ ] -> 1 | x: : xs -> x * mult. List xs; ; val mult. List : int list -> int = <fun> # mult. List [2; 4; 6]; ; - : int = 48 n Computes (2 * (4 * (6 * 1))) n 11/23/2020 30
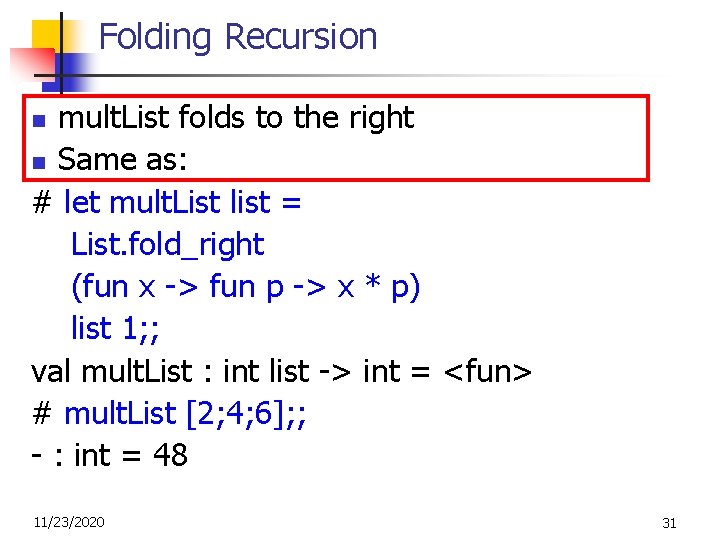
Folding Recursion mult. List folds to the right n Same as: # let mult. List list = List. fold_right (fun x -> fun p -> x * p) list 1; ; val mult. List : int list -> int = <fun> # mult. List [2; 4; 6]; ; - : int = 48 n 11/23/2020 31
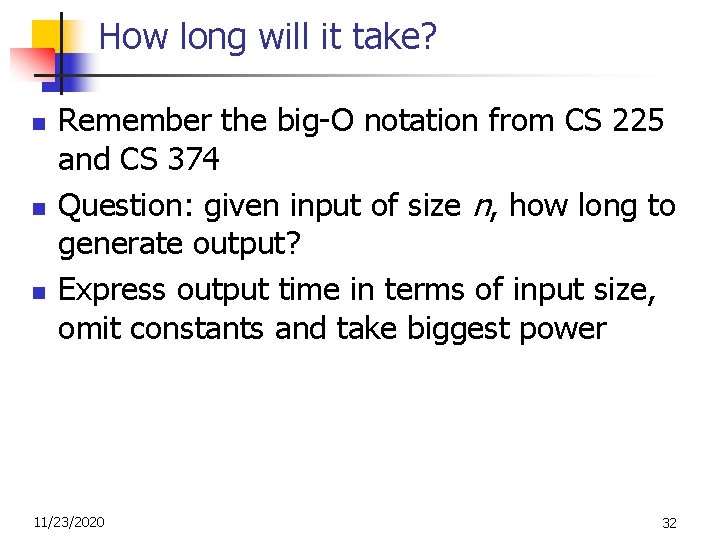
How long will it take? n n n Remember the big-O notation from CS 225 and CS 374 Question: given input of size n, how long to generate output? Express output time in terms of input size, omit constants and take biggest power 11/23/2020 32
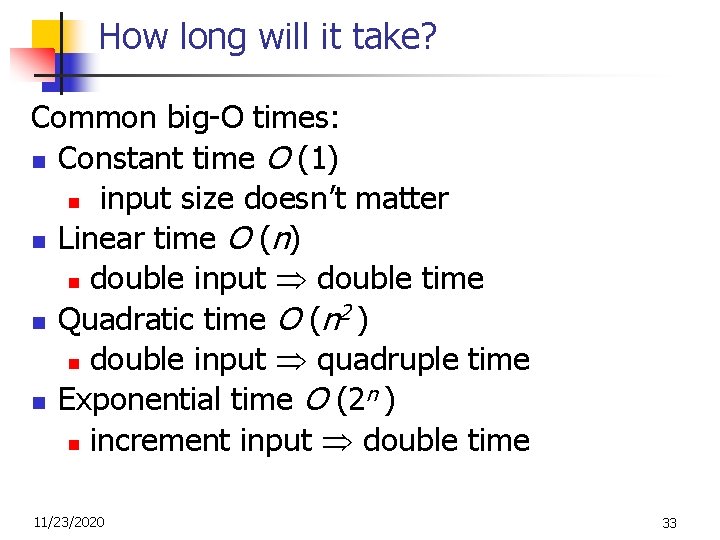
How long will it take? Common big-O times: n Constant time O (1) n input size doesn’t matter n Linear time O (n) n double input double time n Quadratic time O (n 2 ) n double input quadruple time n Exponential time O (2 n ) n increment input double time 11/23/2020 33
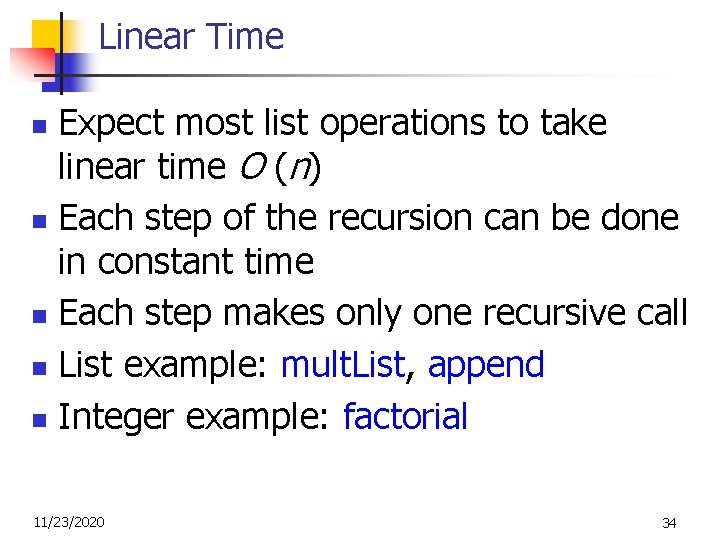
Linear Time Expect most list operations to take linear time O (n) n Each step of the recursion can be done in constant time n Each step makes only one recursive call n List example: mult. List, append n Integer example: factorial n 11/23/2020 34
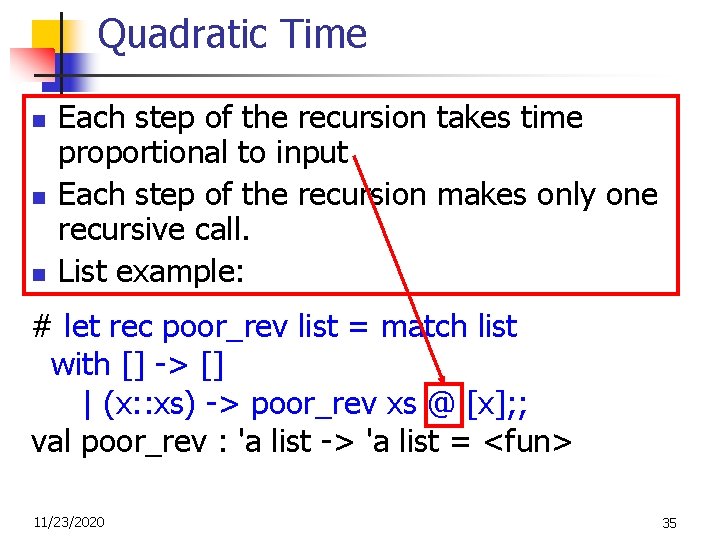
Quadratic Time n n n Each step of the recursion takes time proportional to input Each step of the recursion makes only one recursive call. List example: # let rec poor_rev list = match list with [] -> [] | (x: : xs) -> poor_rev xs @ [x]; ; val poor_rev : 'a list -> 'a list = <fun> 11/23/2020 35
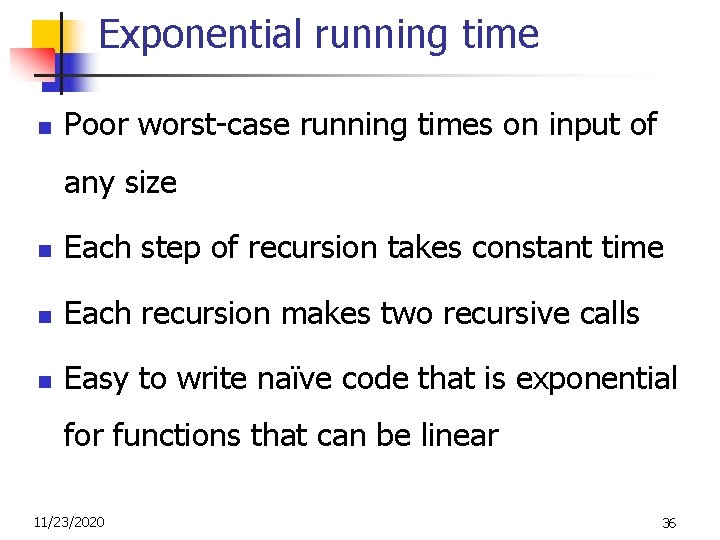
Exponential running time n Poor worst-case running times on input of any size n Each step of recursion takes constant time n Each recursion makes two recursive calls n Easy to write naïve code that is exponential for functions that can be linear 11/23/2020 36
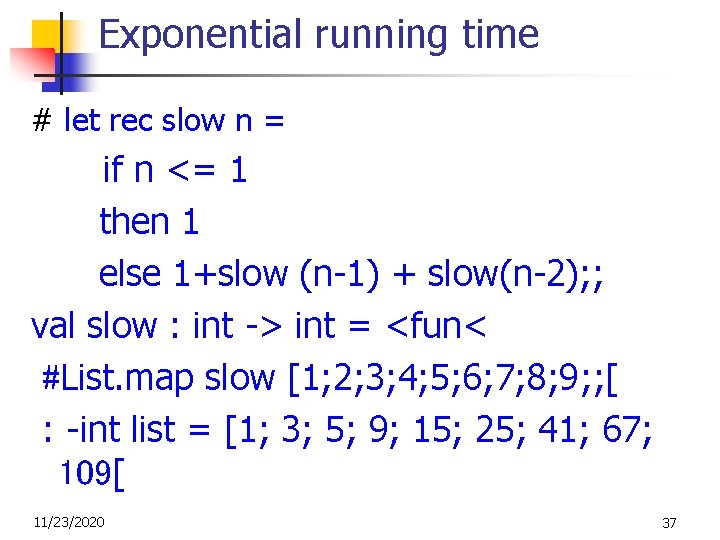
Exponential running time # let rec slow n = if n <= 1 then 1 else 1+slow (n-1) + slow(n-2); ; val slow : int -> int = <fun< #List. map slow [1; 2; 3; 4; 5; 6; 7; 8; 9; ; [ : -int list = [1; 3; 5; 9; 15; 25; 41; 67; 109[ 11/23/2020 37
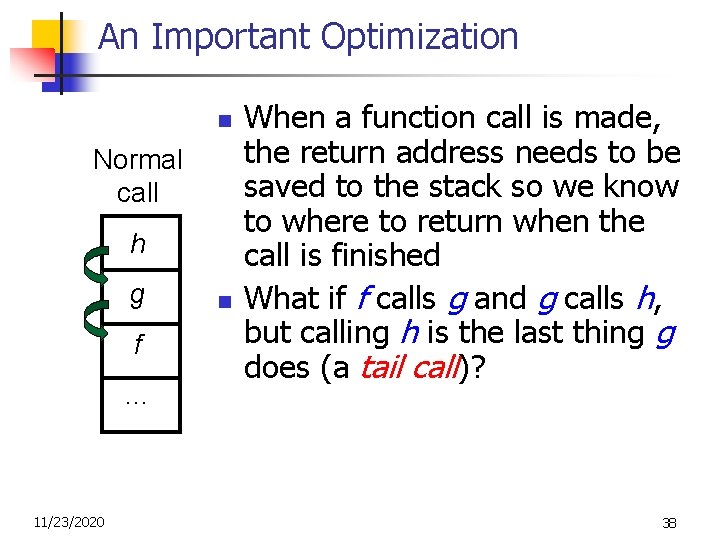
An Important Optimization n Normal call h g f … 11/23/2020 n When a function call is made, the return address needs to be saved to the stack so we know to where to return when the call is finished What if f calls g and g calls h, but calling h is the last thing g does (a tail call)? 38
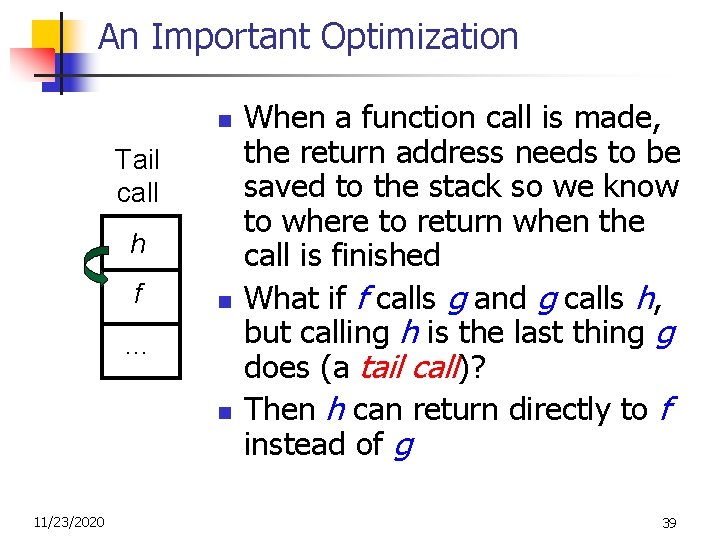
An Important Optimization n Tail call h f n … n 11/23/2020 When a function call is made, the return address needs to be saved to the stack so we know to where to return when the call is finished What if f calls g and g calls h, but calling h is the last thing g does (a tail call)? Then h can return directly to f instead of g 39
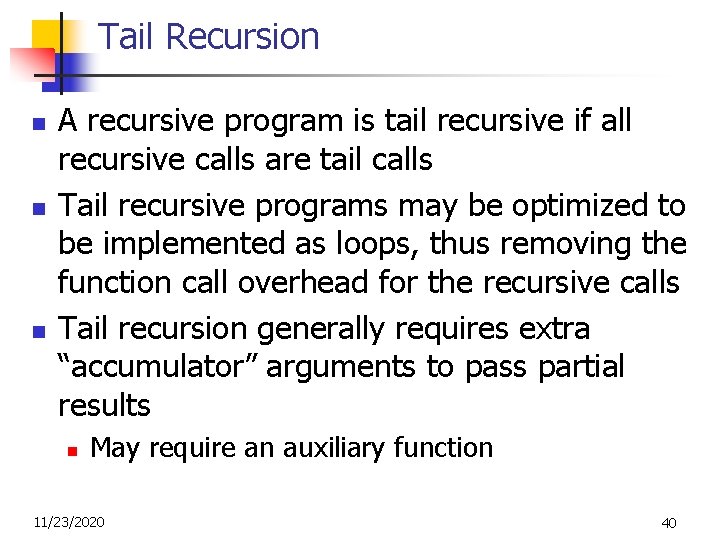
Tail Recursion n A recursive program is tail recursive if all recursive calls are tail calls Tail recursive programs may be optimized to be implemented as loops, thus removing the function call overhead for the recursive calls Tail recursion generally requires extra “accumulator” arguments to pass partial results n May require an auxiliary function 11/23/2020 40
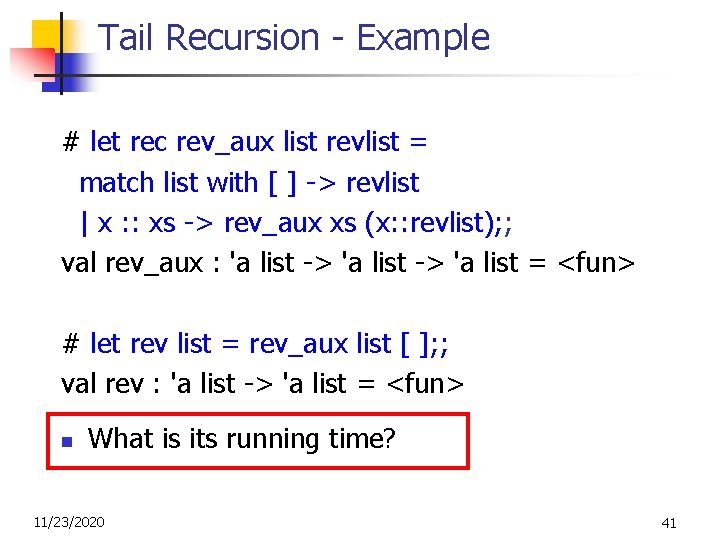
Tail Recursion - Example # let rec rev_aux list revlist = match list with [ ] -> revlist | x : : xs -> rev_aux xs (x: : revlist); ; val rev_aux : 'a list -> 'a list = <fun> # let rev list = rev_aux list [ ]; ; val rev : 'a list -> 'a list = <fun> n What is its running time? 11/23/2020 41
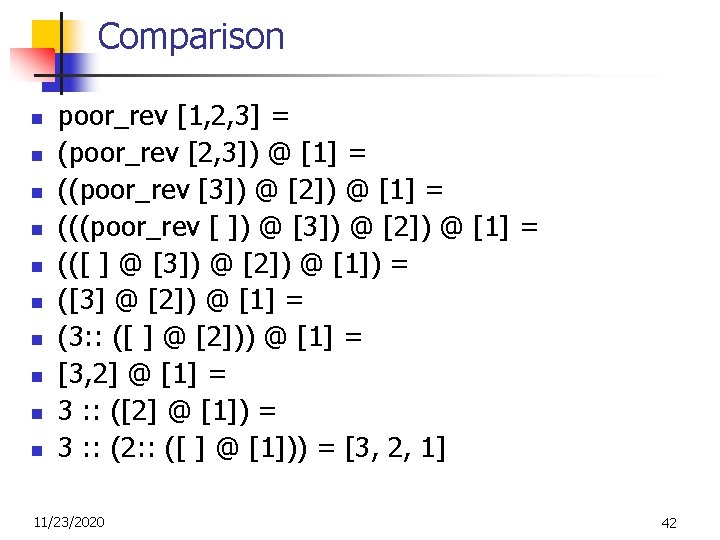
![Comparison n n rev 1 2 3 revaux 1 2 3 Comparison n n rev [1, 2, 3] = rev_aux [1, 2, 3] [ ]](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-43.jpg)
Comparison n n rev [1, 2, 3] = rev_aux [1, 2, 3] [ ] = rev_aux [2, 3] [1] = rev_aux [3] [2, 1] = rev_aux [ ] [3, 2, 1] = [3, 2, 1] 11/23/2020 43
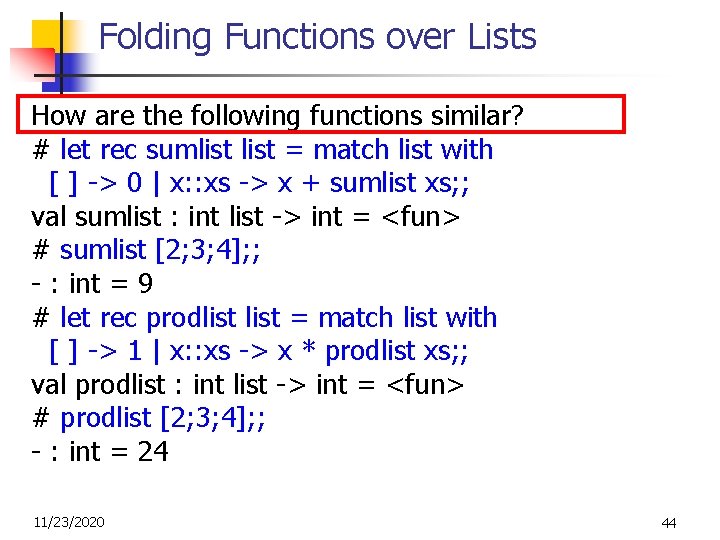
Folding Functions over Lists How are the following functions similar? # let rec sumlist = match list with [ ] -> 0 | x: : xs -> x + sumlist xs; ; val sumlist : int list -> int = <fun> # sumlist [2; 3; 4]; ; - : int = 9 # let rec prodlist = match list with [ ] -> 1 | x: : xs -> x * prodlist xs; ; val prodlist : int list -> int = <fun> # prodlist [2; 3; 4]; ; - : int = 24 11/23/2020 44
![Folding let rec foldleft f a list match list with Folding # let rec fold_left f a list = match list with [] ->](https://slidetodoc.com/presentation_image_h/8a2f186db16528e6f6f7e71b5a7d50d8/image-45.jpg)
Folding # let rec fold_left f a list = match list with [] -> a | (x : : xs) -> fold_left f (f a x) xs; ; val fold_left : ('a -> 'b -> 'a) -> 'a -> 'b list -> 'a = <fun> fold_left f a [x 1; x 2; …; xn] = f(…(f (f a x 1) x 2)…)xn # let rec fold_right f list b = match list with [ ] -> b | (x : : xs) -> f x (fold_right f xs b); ; val fold_right : ('a -> 'b) -> 'a list -> 'b = <fun> fold_right f [x 1; x 2; …; xn] b = f x 1(f x 2 (…(f xn b)…)) 11/23/2020 45
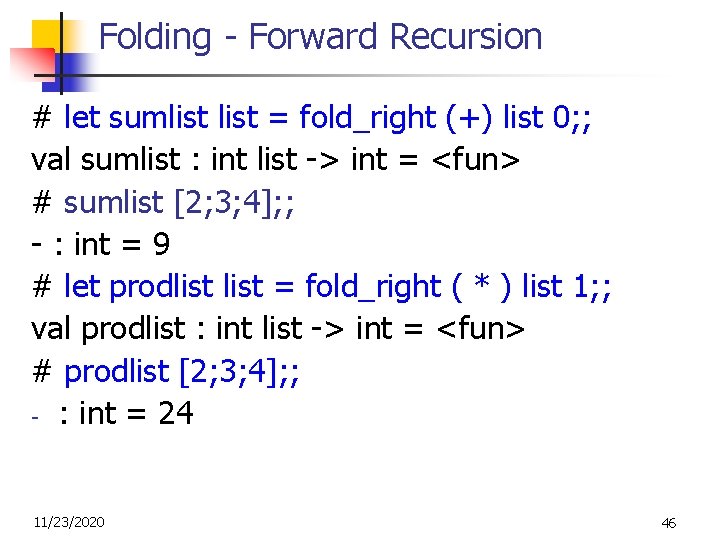
Folding - Forward Recursion # let sumlist = fold_right (+) list 0; ; val sumlist : int list -> int = <fun> # sumlist [2; 3; 4]; ; - : int = 9 # let prodlist = fold_right ( * ) list 1; ; val prodlist : int list -> int = <fun> # prodlist [2; 3; 4]; ; - : int = 24 11/23/2020 46
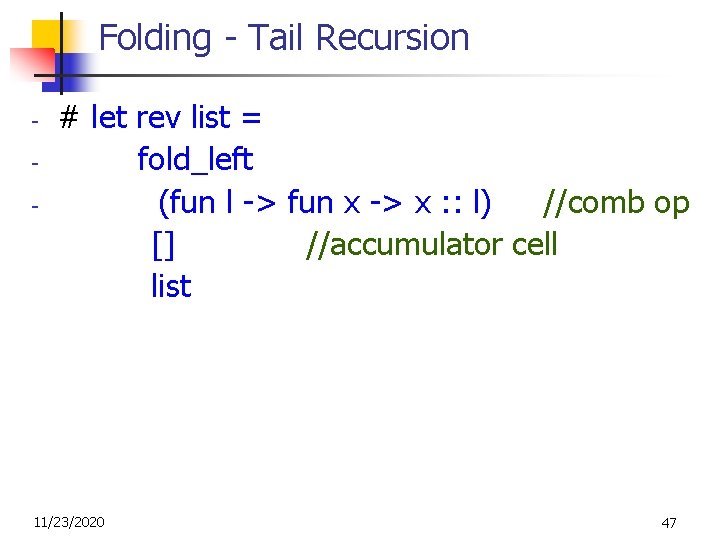
Folding - Tail Recursion - # let rev list = fold_left (fun l -> fun x -> x : : l) //comb op [] //accumulator cell list 11/23/2020 47
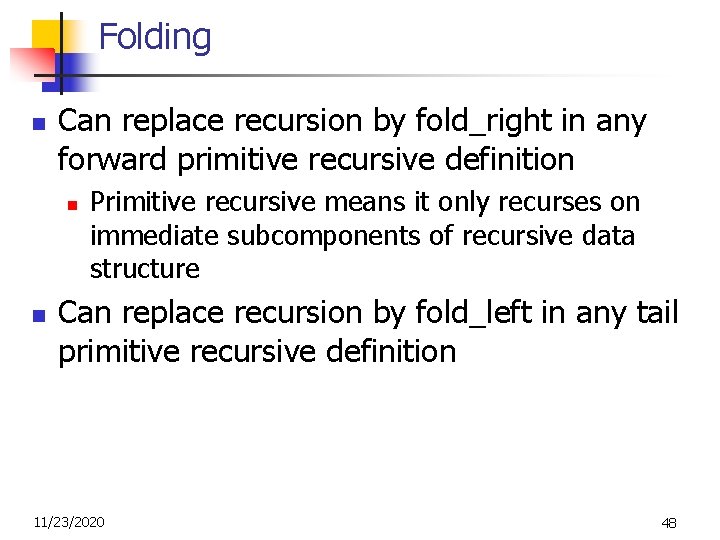
Folding n Can replace recursion by fold_right in any forward primitive recursive definition n n Primitive recursive means it only recurses on immediate subcomponents of recursive data structure Can replace recursion by fold_left in any tail primitive recursive definition 11/23/2020 48
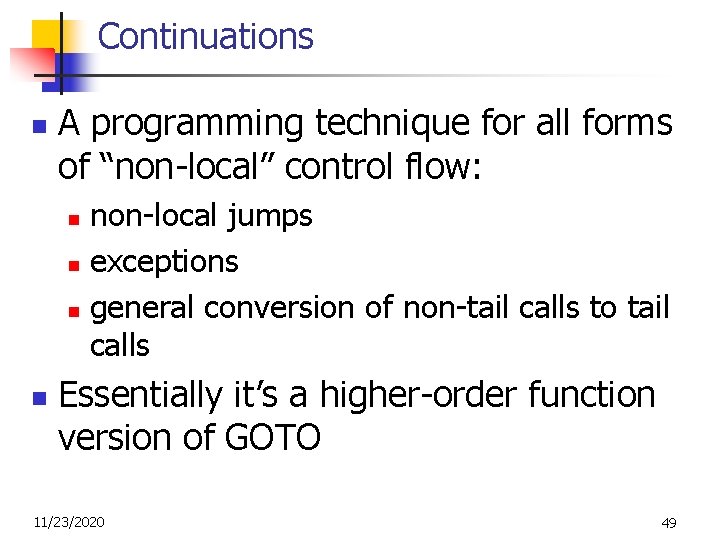
Continuations n A programming technique for all forms of “non-local” control flow: non-local jumps n exceptions n general conversion of non-tail calls to tail calls n n Essentially it’s a higher-order function version of GOTO 11/23/2020 49
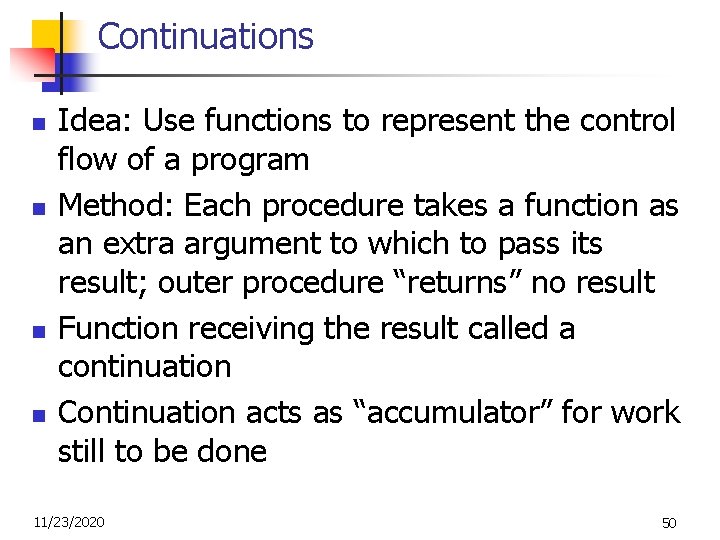
Continuations n n Idea: Use functions to represent the control flow of a program Method: Each procedure takes a function as an extra argument to which to pass its result; outer procedure “returns” no result Function receiving the result called a continuation Continuation acts as “accumulator” for work still to be done 11/23/2020 50
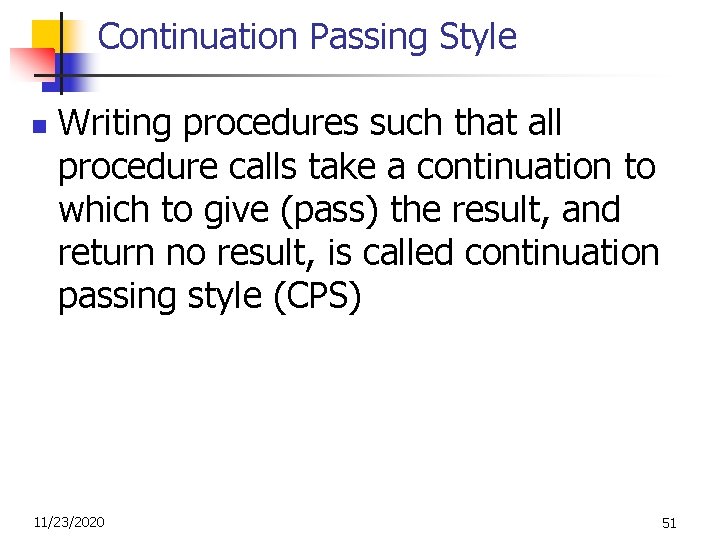
Continuation Passing Style n Writing procedures such that all procedure calls take a continuation to which to give (pass) the result, and return no result, is called continuation passing style (CPS) 11/23/2020 51
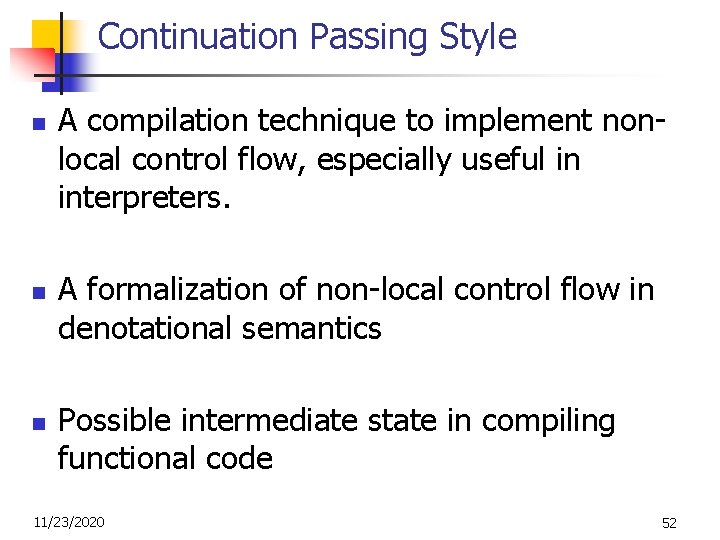
Continuation Passing Style n n n A compilation technique to implement nonlocal control flow, especially useful in interpreters. A formalization of non-local control flow in denotational semantics Possible intermediate state in compiling functional code 11/23/2020 52
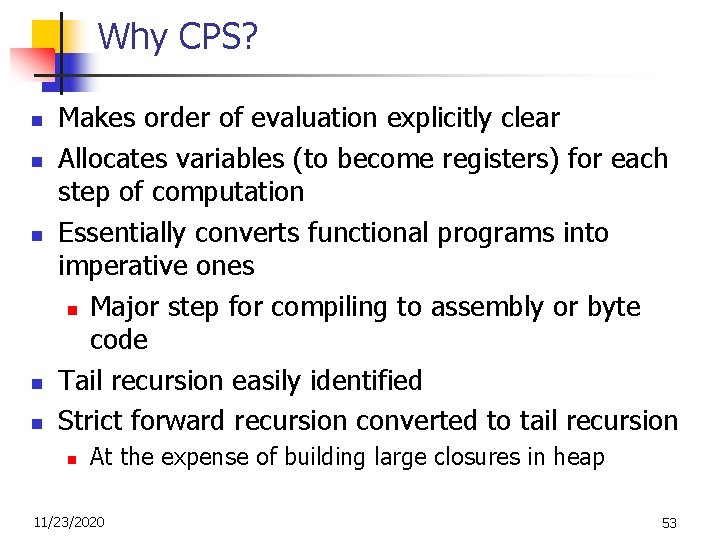
Why CPS? n n n Makes order of evaluation explicitly clear Allocates variables (to become registers) for each step of computation Essentially converts functional programs into imperative ones n Major step for compiling to assembly or byte code Tail recursion easily identified Strict forward recursion converted to tail recursion n At the expense of building large closures in heap 11/23/2020 53
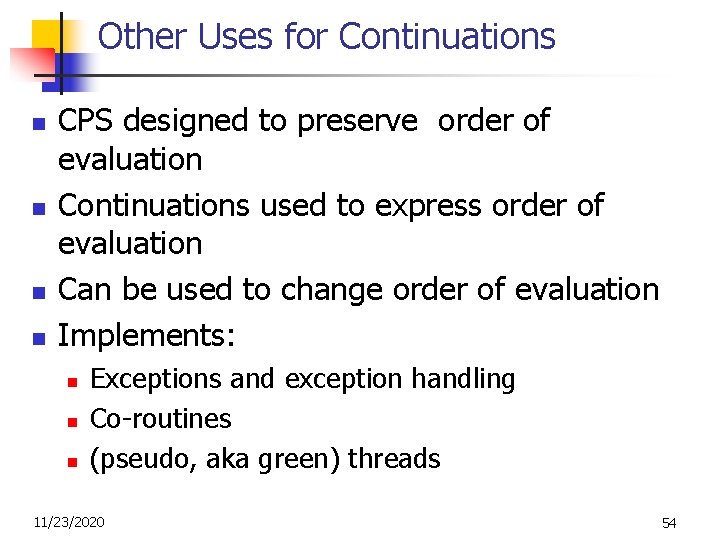
Other Uses for Continuations n n CPS designed to preserve order of evaluation Continuations used to express order of evaluation Can be used to change order of evaluation Implements: n n n Exceptions and exception handling Co-routines (pseudo, aka green) threads 11/23/2020 54
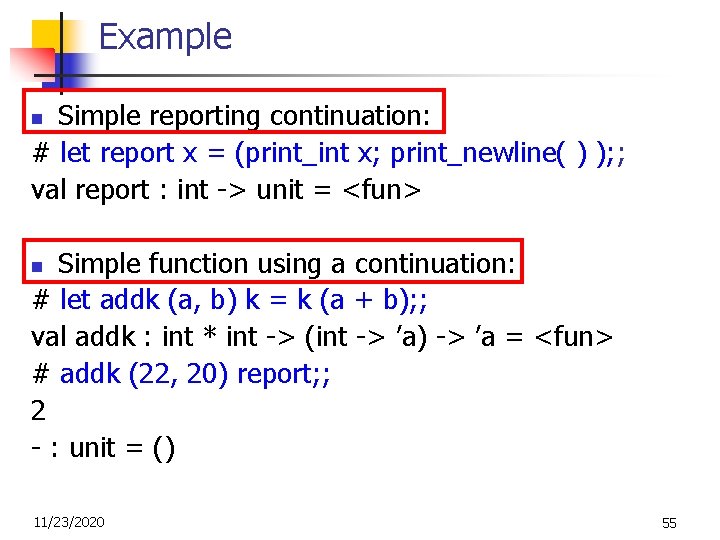
Example Simple reporting continuation: # let report x = (print_int x; print_newline( ) ); ; val report : int -> unit = <fun> n Simple function using a continuation: # let addk (a, b) k = k (a + b); ; val addk : int * int -> (int -> ’a) -> ’a = <fun> # addk (22, 20) report; ; 2 - : unit = () n 11/23/2020 55
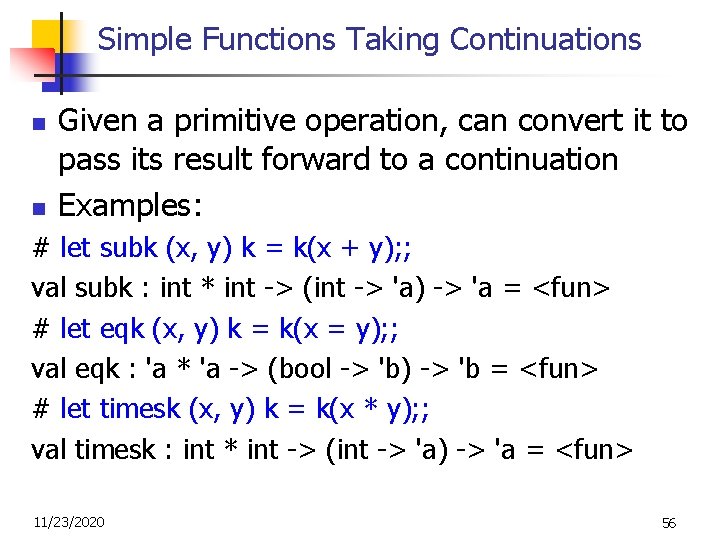
Simple Functions Taking Continuations n n Given a primitive operation, can convert it to pass its result forward to a continuation Examples: # let subk (x, y) k = k(x + y); ; val subk : int * int -> (int -> 'a) -> 'a = <fun> # let eqk (x, y) k = k(x = y); ; val eqk : 'a * 'a -> (bool -> 'b) -> 'b = <fun> # let timesk (x, y) k = k(x * y); ; val timesk : int * int -> (int -> 'a) -> 'a = <fun> 11/23/2020 56
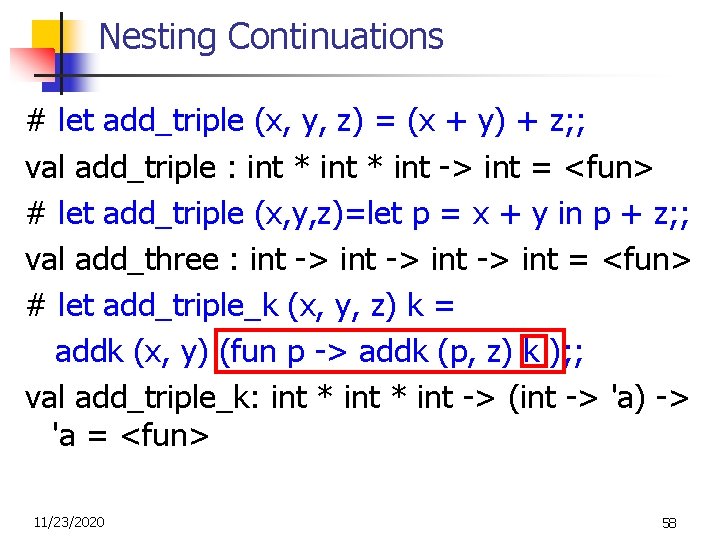
Nesting Continuations # let add_triple (x, y, z) = (x + y) + z; ; val add_triple : int * int -> int = <fun> # let add_triple (x, y, z)=let p = x + y in p + z; ; val add_three : int -> int = <fun> # let add_triple_k (x, y, z) k = addk (x, y) (fun p -> addk (p, z) k ); ; val add_triple_k: int * int -> (int -> 'a) -> 'a = <fun> 11/23/2020 58
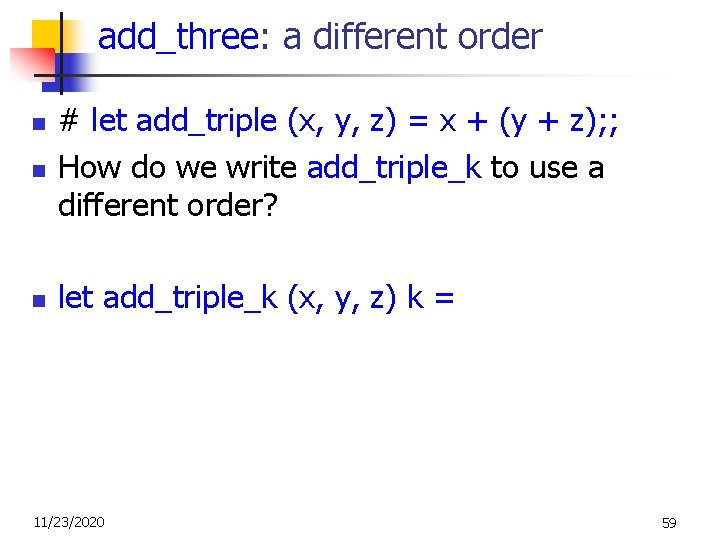
add_three: a different order n # let add_triple (x, y, z) = x + (y + z); ; How do we write add_triple_k to use a different order? n let add_triple_k (x, y, z) k = n 11/23/2020 59
Cs 421 uiuc
Cs 421 programming languages and compilers
What is an interpreter
Finding and understanding bugs in c compilers
Symbol table implementation in lex and yacc
Compiler
Real-time systems and programming languages
Advantages and disadvantages of programming languages
Real time programming language
Binarymove c++
Cousins of compiler
Compilers book
4 functions of a compiler
What is front end compiler
Multithreaded programming languages
Programming languages levels
Introduction to programming languages
Plc
Joey paquet
Comparative programming languages
Alternative programming languages
Types of programming languages
Transmission programming languages
Adam doupe cse 340
Integral data types
Xenia programming languages
Mainstream programming languages
Vineeth kashyap
Programming languages
Programming languages
Programming languages
Programming languages
Tiny programming language
Brief history of programming languages
Lisp_q
Low level language
If programming languages were cars
Reasons for studying concepts of programming languages
Cornell programming languages
Low level programming languages
Middle level programming languages
The art of programming language
Multimedia programming languages
Storage management in programming languages
421 could not create socket
4 2 1 fluid rule
Ist 421
[email protected]
Rule4ml
Estimated blood loss formula
4 2 1 fluid rule
Cse 421
Fwm 421
Fwm 421
Ist 421
Uiuc cs 421
Ist 421
Comp 421
Cmsc 421