Phase Implementation 1 Overview of Implementation phase Define
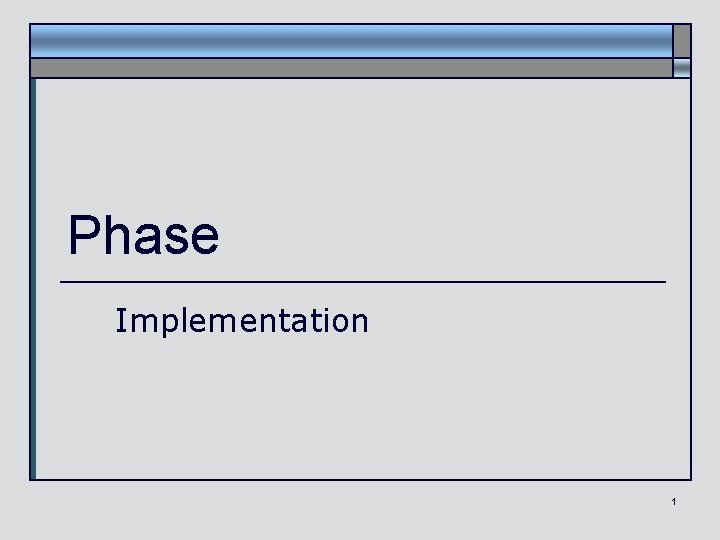
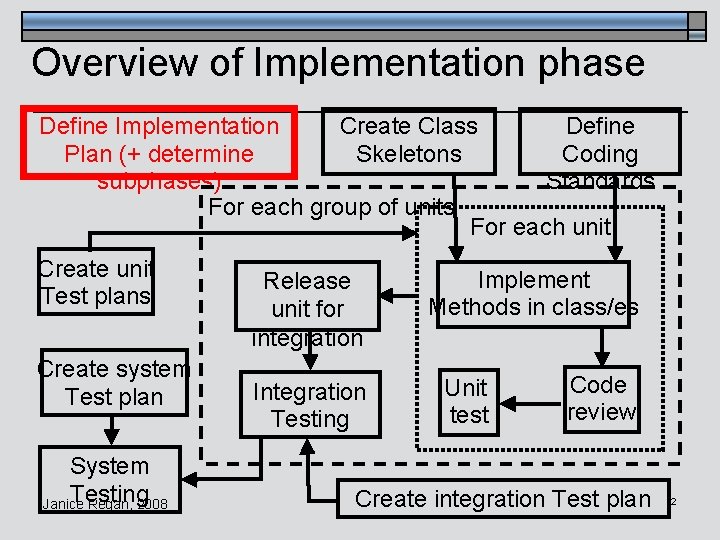
![Goal of Implementation Phase ] Represent software system such that it can be understood Goal of Implementation Phase ] Represent software system such that it can be understood](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-3.jpg)
![Overview of Implementation Phase ] Planning Implementation and testing ] Data persistence ] GUI Overview of Implementation Phase ] Planning Implementation and testing ] Data persistence ] GUI](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-4.jpg)
![Where are we starting from? ] From low level design we have a detailed Where are we starting from? ] From low level design we have a detailed](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-5.jpg)
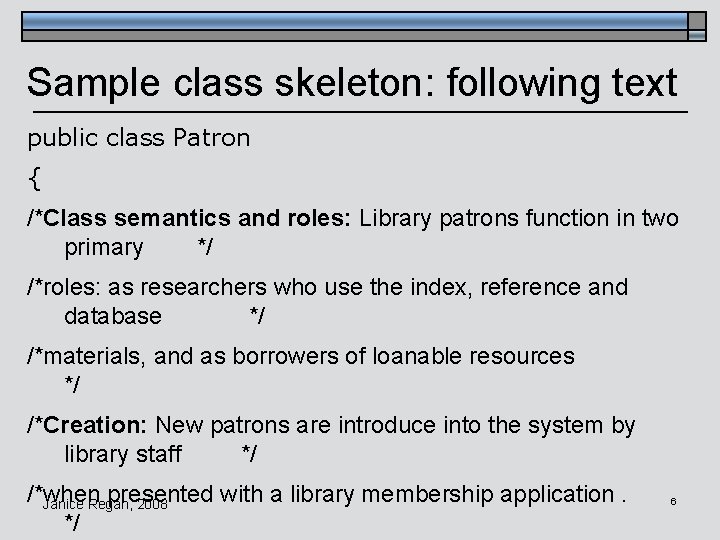
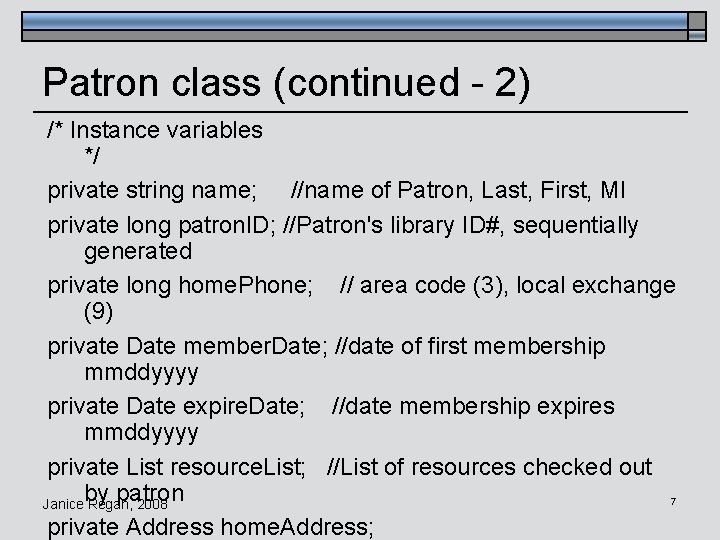
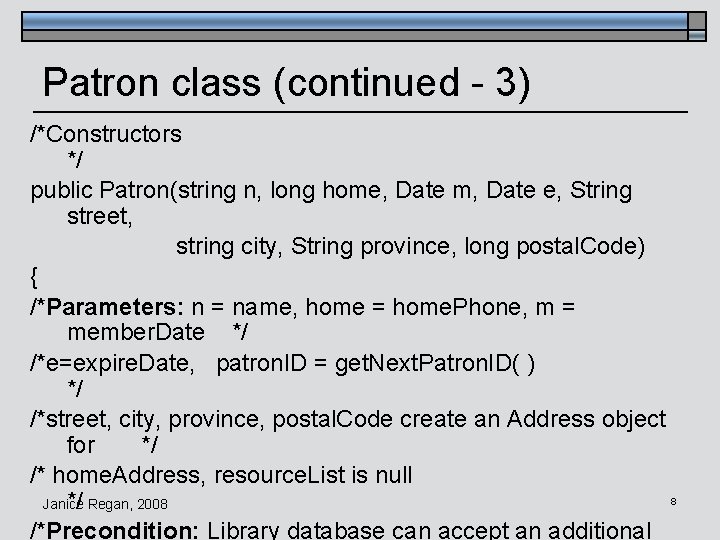
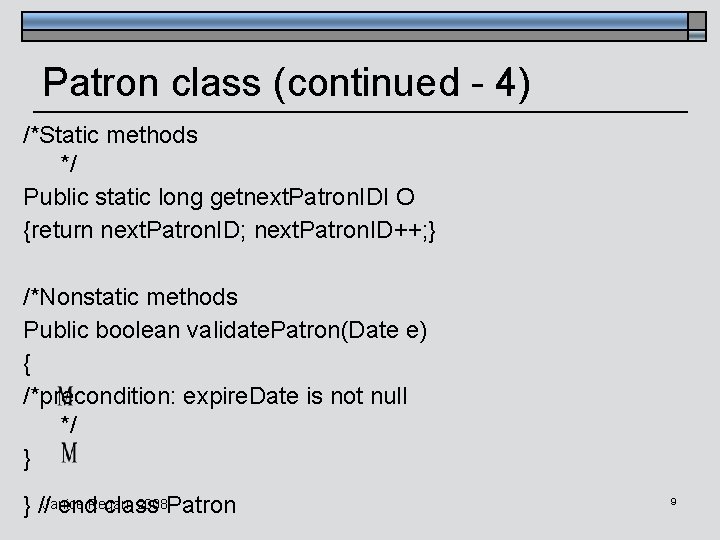
![Where are we starting from? ] (2) For previous phases we have u One Where are we starting from? ] (2) For previous phases we have u One](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-10.jpg)
![Planning the Implementation Phase ] Because software systems are complex, they must be built Planning the Implementation Phase ] Because software systems are complex, they must be built](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-11.jpg)
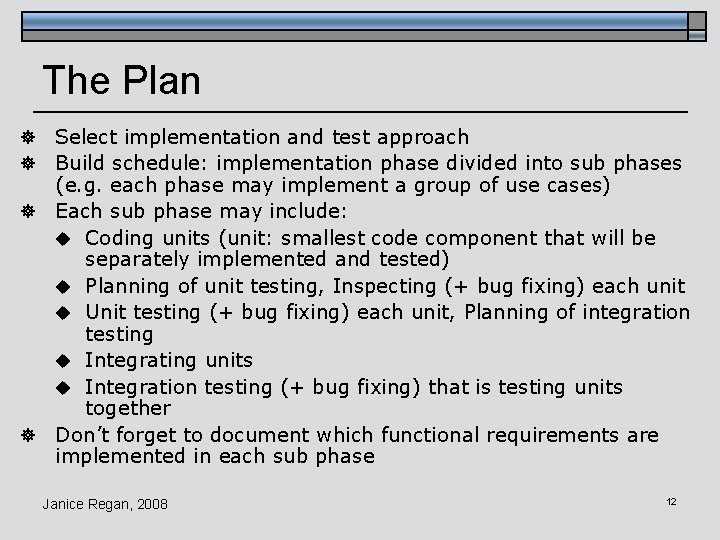
![Implementation Phase ] ] Linear software development process (waterfall) u Each phase of the Implementation Phase ] ] Linear software development process (waterfall) u Each phase of the](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-13.jpg)
![Implementation Approaches - 1 ] Big bang u Implement parts separately u Integrate and Implementation Approaches - 1 ] Big bang u Implement parts separately u Integrate and](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-14.jpg)
![Design of Real (read large) Systems ] Using the big bang approach, which may Design of Real (read large) Systems ] Using the big bang approach, which may](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-15.jpg)
![Implementation Approaches - 2 ] Top-down (in terms of execution flow) u In OO: Implementation Approaches - 2 ] Top-down (in terms of execution flow) u In OO:](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-16.jpg)
![Example of Top-Down: Step 1 ] Build the GUI ] All actions by other Example of Top-Down: Step 1 ] Build the GUI ] All actions by other](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-17.jpg)
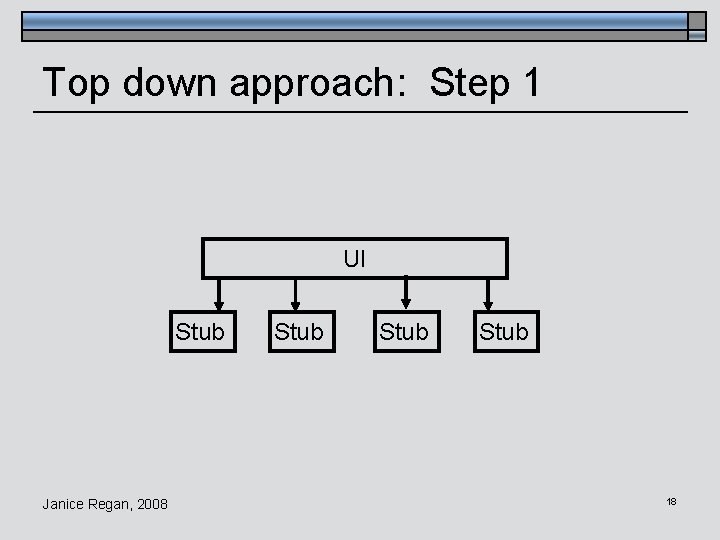
![Example of Top-Down: Step 2 ] Implement methods in the system directly called by Example of Top-Down: Step 2 ] Implement methods in the system directly called by](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-19.jpg)
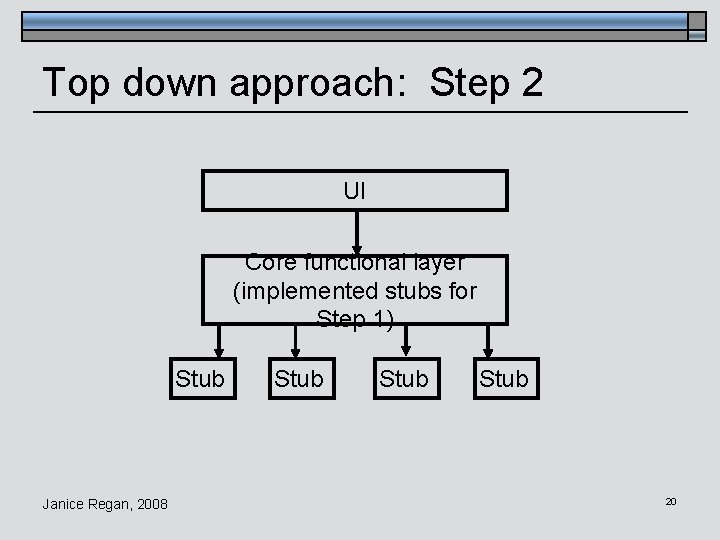
![Example of Top-Down: Step 3 ] Implement methods in the system directly called by Example of Top-Down: Step 3 ] Implement methods in the system directly called by](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-21.jpg)
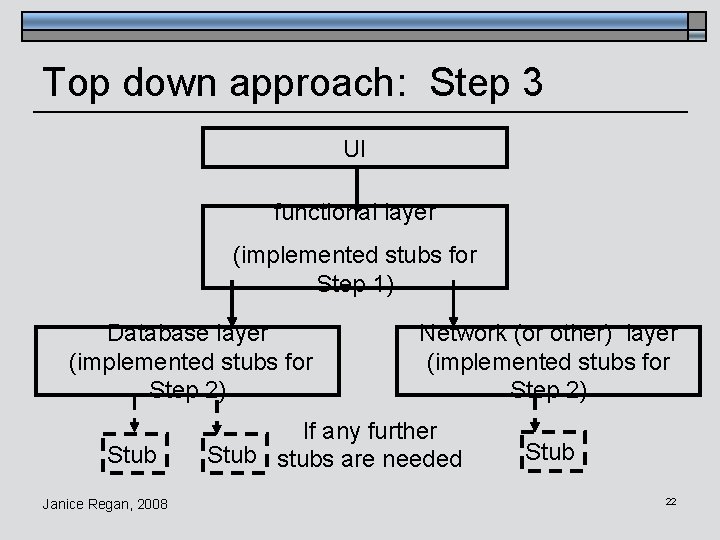
![Implementation Approaches - 3 ] Bottom-up u In OO: Start by implementing classes that Implementation Approaches - 3 ] Bottom-up u In OO: Start by implementing classes that](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-23.jpg)
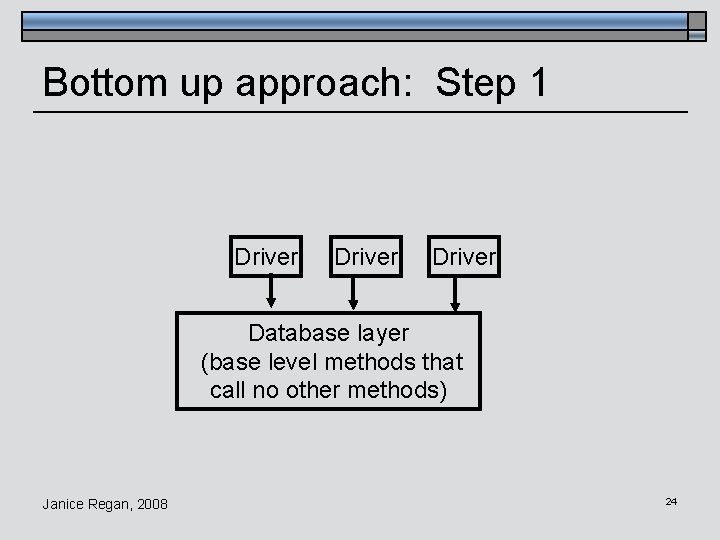
![Bottom up: example step 1 ] Implement all classes for the database layer ] Bottom up: example step 1 ] Implement all classes for the database layer ]](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-25.jpg)
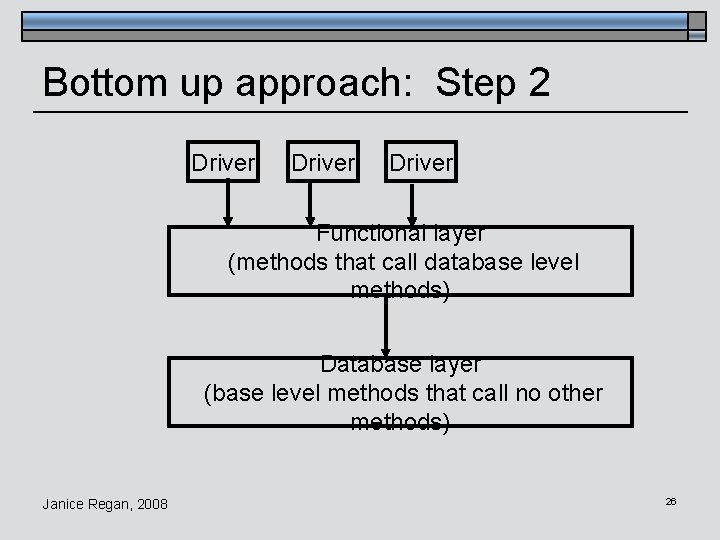
![Bottom up: example step 2 ] Implement all classes for the functional layer ] Bottom up: example step 2 ] Implement all classes for the functional layer ]](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-27.jpg)
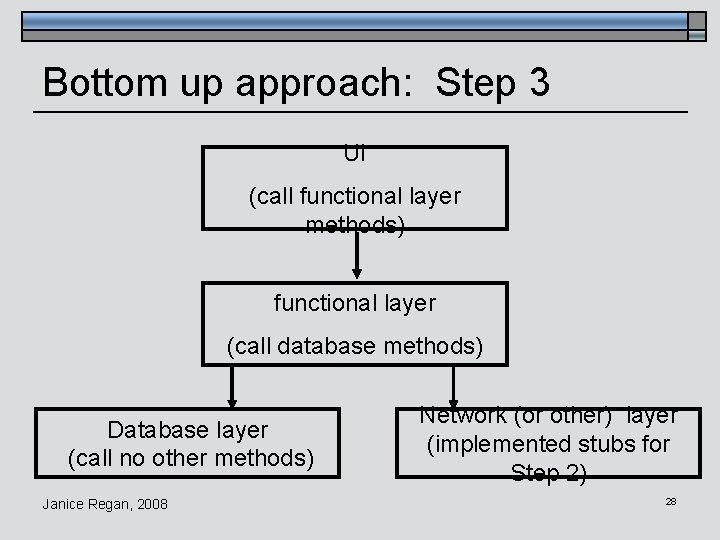
![Implementation Approaches Advantages and Disadvantages ] Advantages to both u Integration test by adding Implementation Approaches Advantages and Disadvantages ] Advantages to both u Integration test by adding](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-29.jpg)
![Implementation Approaches - 4 ] Threads u Implement and test a minimal set of Implementation Approaches - 4 ] Threads u Implement and test a minimal set of](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-30.jpg)
![LMS Implementation plan diagram ] Order based on importance of each use case as LMS Implementation plan diagram ] Order based on importance of each use case as](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-31.jpg)
![LMS Example: Thread phase 1 - 1 ] Implementing check. In. Resource and check. LMS Example: Thread phase 1 - 1 ] Implementing check. In. Resource and check.](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-32.jpg)
![LMS Example: Thread phase 1 - 2 ] Possible order in which to implement LMS Example: Thread phase 1 - 2 ] Possible order in which to implement](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-33.jpg)
![LMS Example: Thread phase 2 - 1 ] ] Implementing manage. Resource use case LMS Example: Thread phase 2 - 1 ] ] Implementing manage. Resource use case](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-34.jpg)
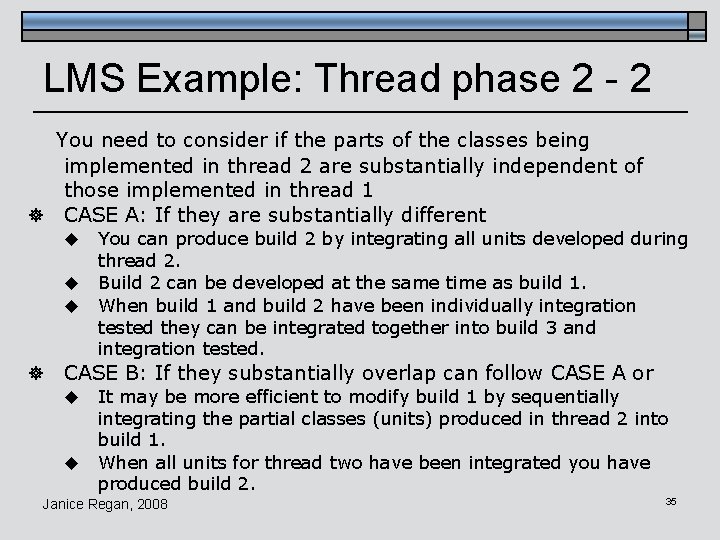
![LMS Example: Thread phase 2 - 3 ] ] ] Possible order in which LMS Example: Thread phase 2 - 3 ] ] ] Possible order in which](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-36.jpg)
![Implementation plan for class project ] We shall use thread implementation approach ] Determine Implementation plan for class project ] We shall use thread implementation approach ] Determine](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-37.jpg)
![Test Planning and Test Phases ] Unit Test Plan phase u Can be done Test Planning and Test Phases ] Unit Test Plan phase u Can be done](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-38.jpg)
![Document: Implementation/Test Plan ] To document your implementation plan, use u Implementation Plan Schedule Document: Implementation/Test Plan ] To document your implementation plan, use u Implementation Plan Schedule](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-39.jpg)
![For your term project: ] Use thread implementation approach ] Determine # of sub For your term project: ] Use thread implementation approach ] Determine # of sub](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-40.jpg)
![Evolutionary Software development ] Software system to be developed is divided into development subgoals Evolutionary Software development ] Software system to be developed is divided into development subgoals](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-41.jpg)
- Slides: 41
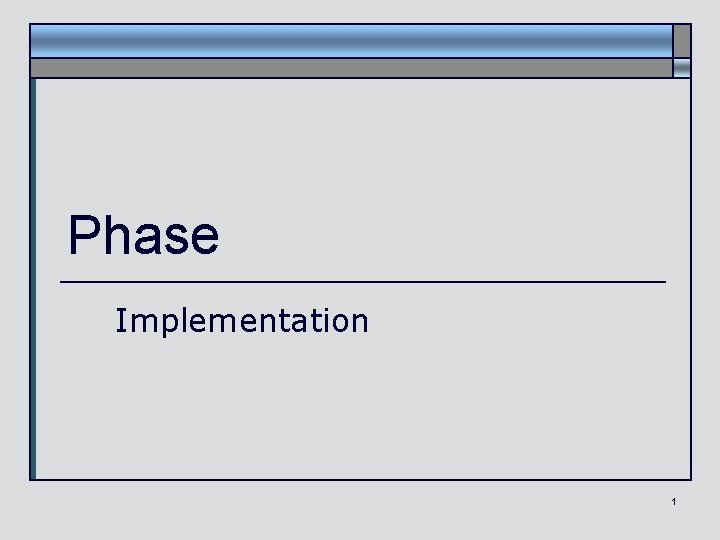
Phase Implementation 1
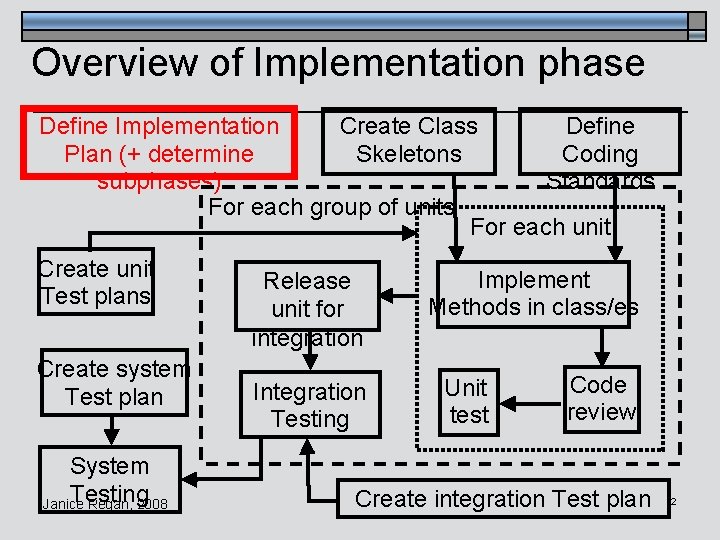
Overview of Implementation phase Define Implementation Create Class Define Plan (+ determine Skeletons Coding subphases) Standards For each group of units For each unit Create unit Test plans Create system Test plan System Testing Janice Regan, 2008 Release unit for integration Integration Testing Implement Methods in class/es Unit test Code review Create integration Test plan 2
![Goal of Implementation Phase Represent software system such that it can be understood Goal of Implementation Phase ] Represent software system such that it can be understood](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-3.jpg)
Goal of Implementation Phase ] Represent software system such that it can be understood by computer (audience) u Code design models using programming language u Software developers still part of our audience ] Must plan how u Order in which modules and classes are built u How the modules/classes are to be combined u How the system will be tested to assure it meets the requirements ] For our class project implementation phases will be defined on the basis of a group of use cases Janice Regan, 2008 3
![Overview of Implementation Phase Planning Implementation and testing Data persistence GUI Overview of Implementation Phase ] Planning Implementation and testing ] Data persistence ] GUI](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-4.jpg)
Overview of Implementation Phase ] Planning Implementation and testing ] Data persistence ] GUI ] Internal documentation: u Class skeletons u Code standard ( Programming style ) then u Unit test plan u Integration test plan u System test plan Janice Regan, 2008 4
![Where are we starting from From low level design we have a detailed Where are we starting from? ] From low level design we have a detailed](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-5.jpg)
Where are we starting from? ] From low level design we have a detailed class diagram. Each class on the diagram shows u u ] (1) Attributes: visibility, name, multiplicity, type [visibility] name [multiplicity] [: type] [= initial-value] [{property- -list. Of. Borrowed. Resources[1. . *] : List Name : String string}] Methods: visibility, name, parameters and their types, return type [visibility] name [(parameter-list)] [: return-type] +get. Due. Date(patron. Type: int, todays. Date: date): date get. Name(): String The information from each class can help us create a class skeleton Janice Regan, 2008 5
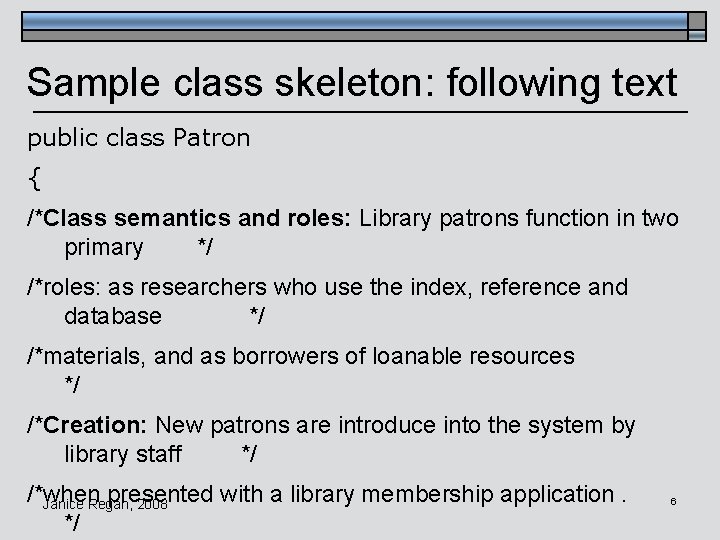
Sample class skeleton: following text public class Patron { /*Class semantics and roles: Library patrons function in two primary */ /*roles: as researchers who use the index, reference and database */ /*materials, and as borrowers of loanable resources */ /*Creation: New patrons are introduce into the system by library staff */ /*when presented with a library membership application. Janice Regan, 2008 */ 6
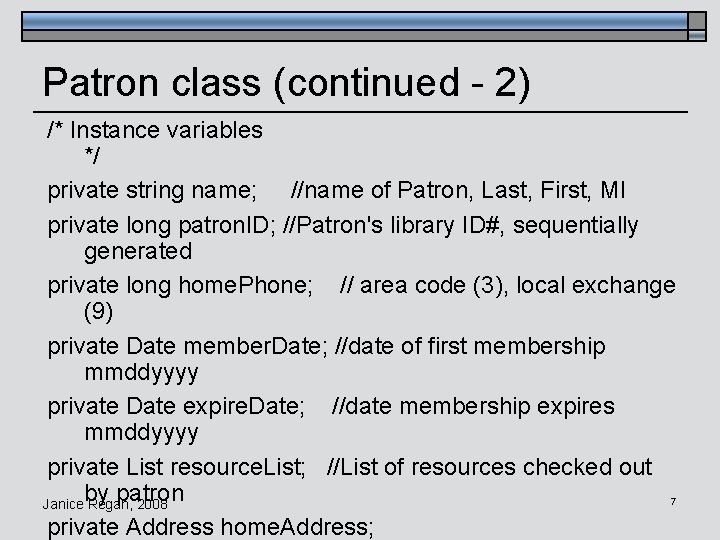
Patron class (continued - 2) /* Instance variables */ private string name; //name of Patron, Last, First, MI private long patron. ID; //Patron's library ID#, sequentially generated private long home. Phone; // area code (3), local exchange (9) private Date member. Date; //date of first membership mmddyyyy private Date expire. Date; //date membership expires mmddyyyy private List resource. List; //List of resources checked out by patron 7 Janice Regan, 2008 private Address home. Address;
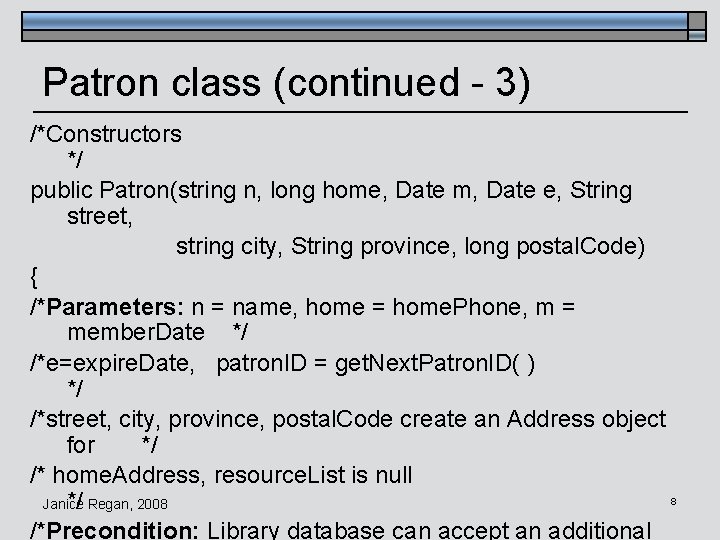
Patron class (continued - 3) /*Constructors */ public Patron(string n, long home, Date m, Date e, String street, string city, String province, long postal. Code) { /*Parameters: n = name, home = home. Phone, m = member. Date */ /*e=expire. Date, patron. ID = get. Next. Patron. ID( ) */ /*street, city, province, postal. Code create an Address object for */ /* home. Address, resource. List is null 8 */ Regan, 2008 Janice /*Precondition: Library database can accept an additional
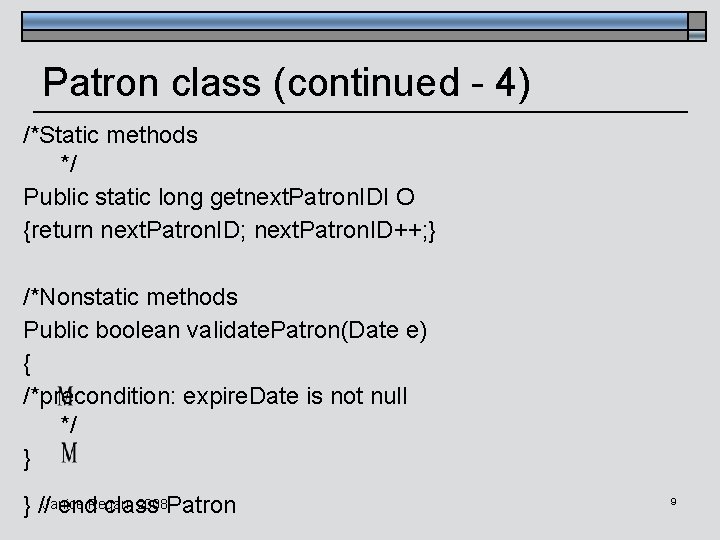
Patron class (continued - 4) /*Static methods */ Public static long getnext. Patron. IDI O {return next. Patron. ID; next. Patron. ID++; } /*Nonstatic methods Public boolean validate. Patron(Date e) { /*precondition: expire. Date is not null */ } 2008 Patron } //Janice end. Regan, class 9
![Where are we starting from 2 For previous phases we have u One Where are we starting from? ] (2) For previous phases we have u One](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-10.jpg)
Where are we starting from? ] (2) For previous phases we have u One class diagram showing the static properties of the system In practice one class diagram is often used for each subsystem making it easier to implement the subsystems independently u a series of interaction diagrams for separate use cases ] showing the dynamic behaviors of the system u A use case diagram showing the interrelation of the actors and use cases in the system u A list of core and less core requirements or prioritized requirements, cross referenced on the above diagrams The above information may help us to decide the order of subsystem or module development. But HOW? Janice Regan, 2008 10
![Planning the Implementation Phase Because software systems are complex they must be built Planning the Implementation Phase ] Because software systems are complex, they must be built](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-11.jpg)
Planning the Implementation Phase ] Because software systems are complex, they must be built of parts, which are first developed (implemented) independently then assembled (integrated) into “builds” u This also renders the implementation phase more efficient as parts can be implemented in parallel ] Planning of the Implementation Phase consists of planning implementation of parts and integration of parts into builds Janice Regan, 2008 11
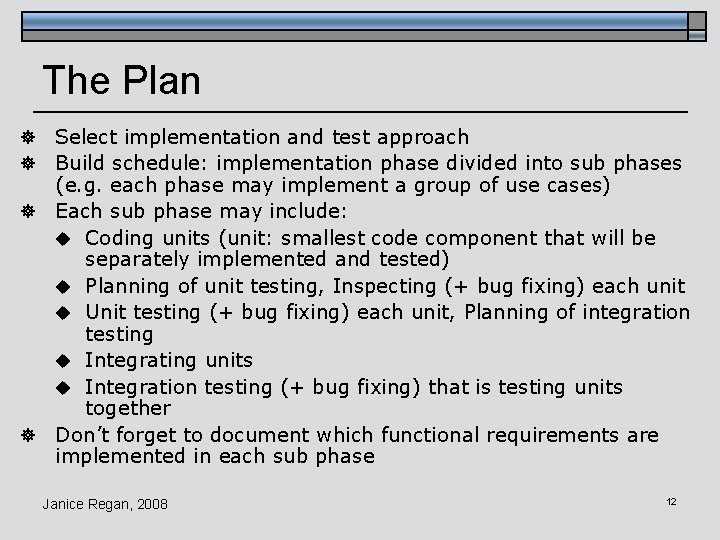
The Plan Select implementation and test approach Build schedule: implementation phase divided into sub phases (e. g. each phase may implement a group of use cases) ] Each sub phase may include: u Coding units (unit: smallest code component that will be separately implemented and tested) u Planning of unit testing, Inspecting (+ bug fixing) each unit u Unit testing (+ bug fixing) each unit, Planning of integration testing u Integrating units u Integration testing (+ bug fixing) that is testing units together ] Don’t forget to document which functional requirements are implemented in each sub phase ] ] Janice Regan, 2008 12
![Implementation Phase Linear software development process waterfall u Each phase of the Implementation Phase ] ] Linear software development process (waterfall) u Each phase of the](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-13.jpg)
Implementation Phase ] ] Linear software development process (waterfall) u Each phase of the development process is executed once u The whole software system is developed and tested Evolutionary Software development process u Some section of the software system is designed u This section is implemented and tested u These two steps are repeated for each successive section of the system. u Independent sections are integrated and integration tested u Sections may be composed of subsystems or of groups of use cases Janice Regan, 2008 13
![Implementation Approaches 1 Big bang u Implement parts separately u Integrate and Implementation Approaches - 1 ] Big bang u Implement parts separately u Integrate and](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-14.jpg)
Implementation Approaches - 1 ] Big bang u Implement parts separately u Integrate and test all at once u Simple u OK for small projects u Difficult to debug (difficult to know which module is the culprit) u User does not see product until very late in the development process u You end up with a single build of the system Janice Regan, 2008 14
![Design of Real read large Systems Using the big bang approach which may Design of Real (read large) Systems ] Using the big bang approach, which may](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-15.jpg)
Design of Real (read large) Systems ] Using the big bang approach, which may work for class assignments, will likely lead to chaos u Source of errors difficult to determine u Slow, debugging difficult u Impractical, difficult to coordinate the work of multiple programmers, testers, and, developers ] Must decide how to break down the system so it can be developed piece by piece in a predetermined order u Different programmers must be able to work on different pieces simultaneously u Want to provide ongoing feedback (showing progress) to the client Janice Regan, 2008 15
![Implementation Approaches 2 Topdown in terms of execution flow u In OO Implementation Approaches - 2 ] Top-down (in terms of execution flow) u In OO:](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-16.jpg)
Implementation Approaches - 2 ] Top-down (in terms of execution flow) u In OO: Start by implementing main method (where execution begins) u All classes instantiated by main( ) and all functions invoked by main( ) are implemented as stubs u Stub: “empty” class or method u Test main( ) by having it instantiating objects of its stub classes and by having it invoking stub methods Janice Regan, 2008 16
![Example of TopDown Step 1 Build the GUI All actions by other Example of Top-Down: Step 1 ] Build the GUI ] All actions by other](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-17.jpg)
Example of Top-Down: Step 1 ] Build the GUI ] All actions by other parts of the system requested by the GUI are implemented as “stub methods” u A stub method ] Has all arguments and return types of the final method Contains no code to perform the actions expected of the method Often will print a message indicating execution of the “stub method” has occurred (usually should include parameter values) Can use the list of messages the 'stub methods' produce when this build is run to compare with interaction diagrams to test 'flow' through the steps of a use case Janice Regan, 2008 17
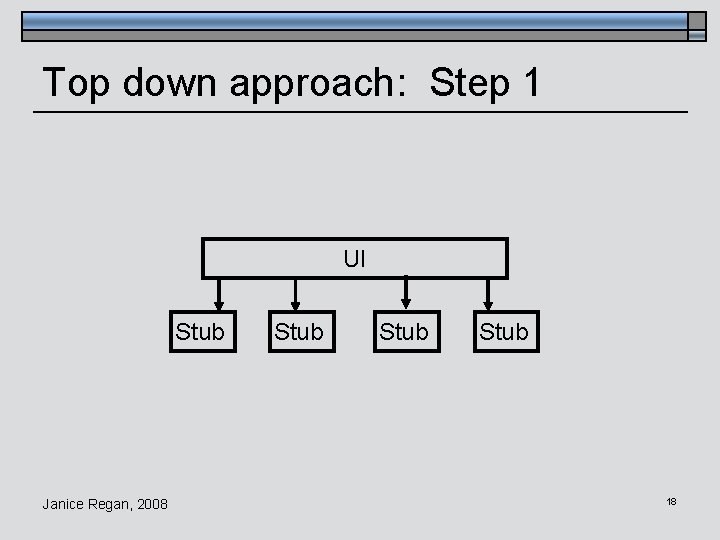
Top down approach: Step 1 UI Stub Janice Regan, 2008 Stub 18
![Example of TopDown Step 2 Implement methods in the system directly called by Example of Top-Down: Step 2 ] Implement methods in the system directly called by](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-19.jpg)
Example of Top-Down: Step 2 ] Implement methods in the system directly called by the GUI u Methods implemented as stubs in the Step 1 build are u u then implemented (sequentially or in parallel). For our project these would be methods implementing the core of the system Any methods called by these core that are not already implemented will be implemented as “stub methods” for our project the 'stub methods' at this level would probably be mostly DB interface classes Can use the list of messages the 'stub methods' produce when this build is run to compare with interaction diagrams to test 'flow' through the steps of a use case. Completeness and correctness of implemented method can now also be tested Janice Regan, 2008 19
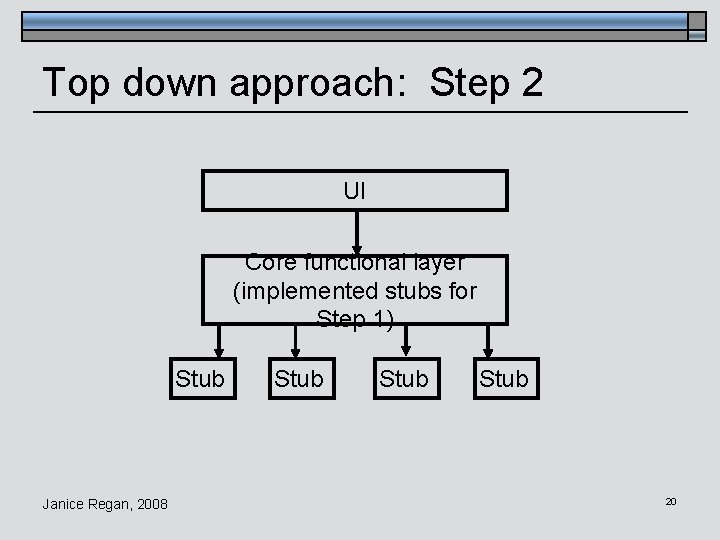
Top down approach: Step 2 UI Core functional layer (implemented stubs for Step 1) Stub Janice Regan, 2008 Stub 20
![Example of TopDown Step 3 Implement methods in the system directly called by Example of Top-Down: Step 3 ] Implement methods in the system directly called by](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-21.jpg)
Example of Top-Down: Step 3 ] Implement methods in the system directly called by any already implemented methods u Methods implemented as stubs in the Step 2 build are u u then implemented (sequentially or in parallel) Any methods called by the methods being implemented that are not already implemented are implemented as “stub methods” (If there are none this is our last iteration) for our project the methods implemented at this level would probably be mostly DB interface classes Can use the list of messages the 'stub methods' (if any) produce when this build is run to compare with interaction diagrams to test 'flow' through the steps of a use case. Completeness and correctness of implemented method can now also be tested Janice Regan, 2008 21
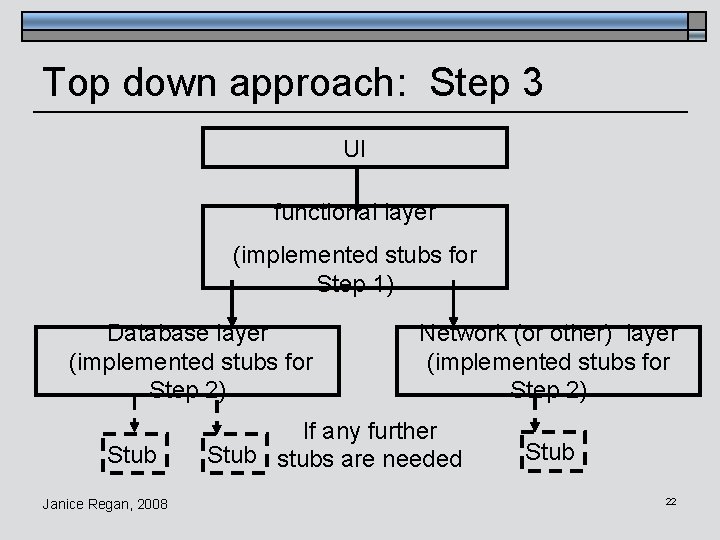
Top down approach: Step 3 UI functional layer (implemented stubs for Step 1) Database layer (implemented stubs for Step 2) Stub Janice Regan, 2008 Network (or other) layer (implemented stubs for Step 2) If any further Stub stubs are needed Stub 22
![Implementation Approaches 3 Bottomup u In OO Start by implementing classes that Implementation Approaches - 3 ] Bottom-up u In OO: Start by implementing classes that](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-23.jpg)
Implementation Approaches - 3 ] Bottom-up u In OO: Start by implementing classes that do not use other classes or parts of our software system (i. e. , classes that only use predefined classes) u Test using test drivers for each of these classes A unit test driver for a class implements the unit test cases planned for that class. It should Create several objects (different states) from the class Display these objects (attribute values) Invoke all methods of each instantiated object Janice Regan, 2008 23
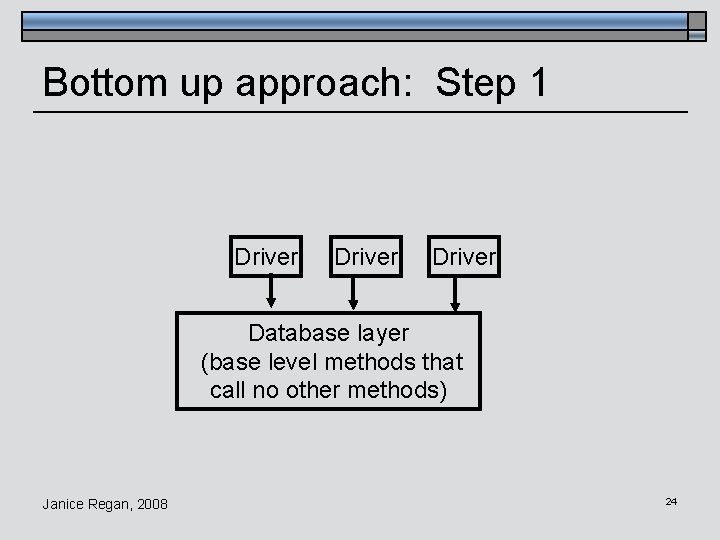
Bottom up approach: Step 1 Driver Database layer (base level methods that call no other methods) Janice Regan, 2008 24
![Bottom up example step 1 Implement all classes for the database layer Bottom up: example step 1 ] Implement all classes for the database layer ]](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-25.jpg)
Bottom up: example step 1 ] Implement all classes for the database layer ] Write a unit test plan for each class ] Write a test driver to implement the test plan ] Unit test each class ] Write an integration test plan to integrate each class into the DB layer ] Write test drivers for integration test ] Integration test the DB layer, tests whether all classes function properly together Janice Regan, 2008 25
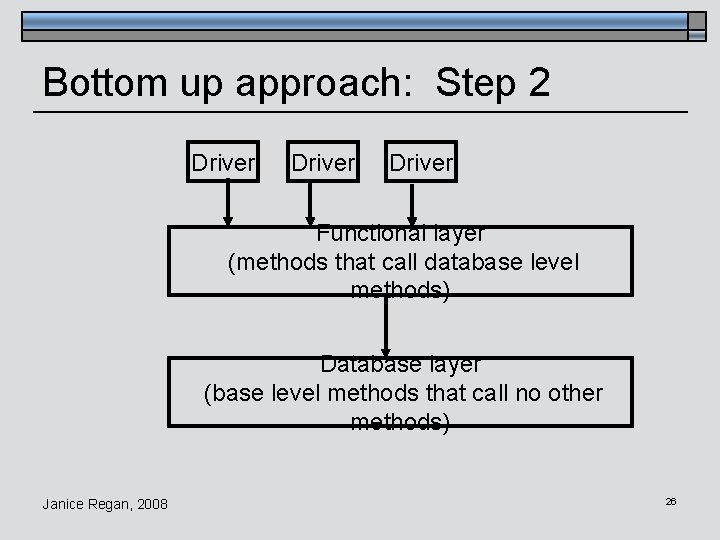
Bottom up approach: Step 2 Driver Functional layer (methods that call database level methods) Database layer (base level methods that call no other methods) Janice Regan, 2008 26
![Bottom up example step 2 Implement all classes for the functional layer Bottom up: example step 2 ] Implement all classes for the functional layer ]](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-27.jpg)
Bottom up: example step 2 ] Implement all classes for the functional layer ] Write a unit test plan for each class ] Write a test driver to implement the test plan ] Unit test each class ] Write an integration test plan to integrate each class into the functional layer ] Write test drivers for integration test of functional layer ] Integration test the functional layer, tests whether all classes in the functional layer and DB layer function properly together Janice Regan, 2008 27
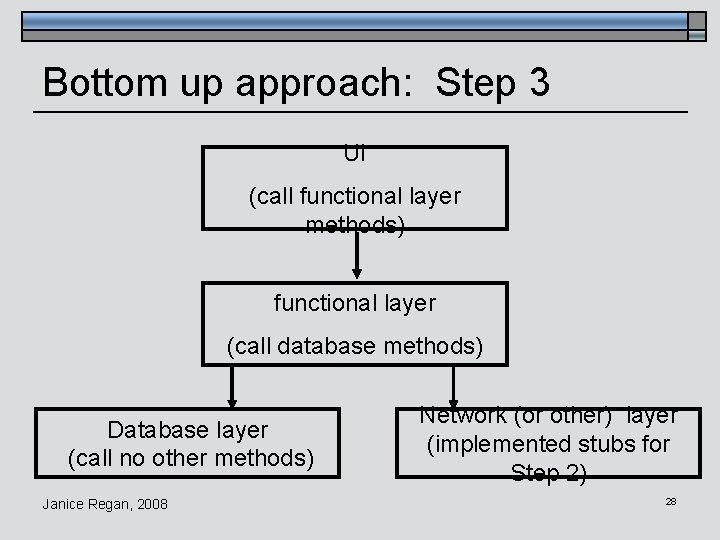
Bottom up approach: Step 3 UI (call functional layer methods) functional layer (call database methods) Database layer (call no other methods) Janice Regan, 2008 Network (or other) layer (implemented stubs for Step 2) 28
![Implementation Approaches Advantages and Disadvantages Advantages to both u Integration test by adding Implementation Approaches Advantages and Disadvantages ] Advantages to both u Integration test by adding](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-29.jpg)
Implementation Approaches Advantages and Disadvantages ] Advantages to both u Integration test by adding modules to previously debugged modules ] Advantages u Bottom up: Low-level (critical) modules built first, hence extensively tested u Top down: User interface is top-level module, hence built first. This is advantageous because UI eases testing u Top down; Stubs are easier to code than drivers ] Disadvantage to both u Stubs/drivers must be written Janice Regan, 2008 29
![Implementation Approaches 4 Threads u Implement and test a minimal set of Implementation Approaches - 4 ] Threads u Implement and test a minimal set of](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-30.jpg)
Implementation Approaches - 4 ] Threads u Implement and test a minimal set of classes implementing a function (thread = use case) e. g. : Manage. Resource: large thread, many closely related functions Add. Resource: small thread, single function u Advantage: partial software system ready for user consumption early (so early user feedback) u Disadvantage: order in which classes (units) are to be implemented not dictated by approach. Must establish which units are most important from the users point of view and start with them Janice Regan, 2008 30
![LMS Implementation plan diagram Order based on importance of each use case as LMS Implementation plan diagram ] Order based on importance of each use case as](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-31.jpg)
LMS Implementation plan diagram ] Order based on importance of each use case as expressed by the user/client gen. Form. Letter patron browse. Resource resourc e Overdue form letter request. Resource reserve. Resource manage. Resource check. Out. Resource manage. Patron Janice Regan, 2008 check. In. Resource Library staff Phase 1 Phase 2 Phase 3 Phase 4 31
![LMS Example Thread phase 1 1 Implementing check In Resource and check LMS Example: Thread phase 1 - 1 ] Implementing check. In. Resource and check.](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-32.jpg)
LMS Example: Thread phase 1 - 1 ] Implementing check. In. Resource and check. Out. Resource use cases during sub phase 1 ] In this sub phase, we need to implement the following units, then integrate them into build 1 u parts of classes in GUI subsystem u parts of classes such as Library. System in Library. Stuff subsystem u parts of classes such as Library. DB in DB subsystem u Parts of the Patron and Resource classes (and their children) in the Library. Stuff module Part of a class that has been implemented and tested as part of this sub phase some class subsystem Janice. GUI Regan, 2008 Library System Resource Patron Library. Stuff subsystem Part of a class that has been implemented and tested as part of this sub phase Library. DB DB subsystem 32
![LMS Example Thread phase 1 2 Possible order in which to implement LMS Example: Thread phase 1 - 2 ] Possible order in which to implement](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-33.jpg)
LMS Example: Thread phase 1 - 2 ] Possible order in which to implement the classes during sub phase 1: u GUI class(es) can be first implemented and unit tested u Resource class is then implemented and unit tested u Patron class is then implemented and unit tested u Finally, Library. System and Library. DB classes are implemented and unit tested ] Once unit tested, they can all be integrated into build 1 ] Build 1 can be tested (integration testing) by performing the check. In. Resource use case (function) and the check. Out. Resource use case Janice Regan, 2008 33
![LMS Example Thread phase 2 1 Implementing manage Resource use case LMS Example: Thread phase 2 - 1 ] ] Implementing manage. Resource use case](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-34.jpg)
LMS Example: Thread phase 2 - 1 ] ] Implementing manage. Resource use case during sub phase 2 In this sub phase, we need to implement the following units, then integrate the into build 2 u parts of classes in GUI subsystem u parts of classes such as Library. System in Library. Stuff subsystem u parts of classes such as Library. DB in DB subsystem u perhaps the entire Resource class (and its children) in the Library. Stuff module Part of a class that has been implemented and tested as part of this sub phase some class subsystem Janice. GUI Regan, 2008 Class that has been fully implemented and tested as part of this sub phase Library System Resource Library. Stuff subsystem Part of a class that has been implemented and tested as part of this sub phase Library. DB DB subsystem 34
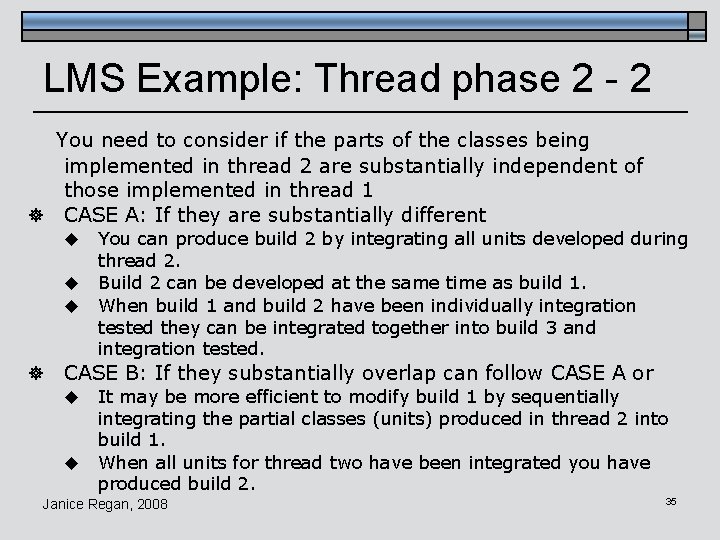
LMS Example: Thread phase 2 - 2 You need to consider if the parts of the classes being implemented in thread 2 are substantially independent of those implemented in thread 1 ] CASE A: If they are substantially different u u u ] You can produce build 2 by integrating all units developed during thread 2. Build 2 can be developed at the same time as build 1. When build 1 and build 2 have been individually integration tested they can be integrated together into build 3 and integration tested. CASE B: If they substantially overlap can follow CASE A or u u It may be more efficient to modify build 1 by sequentially integrating the partial classes (units) produced in thread 2 into build 1. When all units for thread two have been integrated you have produced build 2. Janice Regan, 2008 35
![LMS Example Thread phase 2 3 Possible order in which LMS Example: Thread phase 2 - 3 ] ] ] Possible order in which](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-36.jpg)
LMS Example: Thread phase 2 - 3 ] ] ] Possible order in which to implement the classes during sub phase 2: u GUI class(es) can be first implemented and unit tested u Resource class is then implemented and unit tested u Finally, Library. System and Library. DB classes are implemented and unit tested Once unit tested, they can all be integrated into a build This build (build 3 for CASE A, build 2 for CASE B) can be integration tested u CASE A build 2: by performing the Manage. Resource use case u CASE A build 3 and Case B build 2: by performing the check. In. Resource, the check. Out. Resource, and the manage. Resource use cases Janice Regan, 2008 36
![Implementation plan for class project We shall use thread implementation approach Determine Implementation plan for class project ] We shall use thread implementation approach ] Determine](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-37.jpg)
Implementation plan for class project ] We shall use thread implementation approach ] Determine # of sub phases and their content u This is done by considering core use cases versus non-core use cases and distributing the core use cases in the earlier sub phase(s) and the non-core use cases in the later sub phases (why such distribution? ) ] Build Implementation Plan Schedule by scheduling the sub phases Janice Regan, 2008 37
![Test Planning and Test Phases Unit Test Plan phase u Can be done Test Planning and Test Phases ] Unit Test Plan phase u Can be done](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-38.jpg)
Test Planning and Test Phases ] Unit Test Plan phase u Can be done as soon as you have detailed your classes (units) ] Integration Test Plan phase u Technically, can be done as soon as you have defined your subsystems or use cases (parts) ] Unit Test phase u Done after coding units, can be done in parallel with implementation phase ] Integration Test phase u Done as you are integrating parts, can overlap implementation phase (while coding further units) Janice Regan, 2008 38
![Document ImplementationTest Plan To document your implementation plan use u Implementation Plan Schedule Document: Implementation/Test Plan ] To document your implementation plan, use u Implementation Plan Schedule](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-39.jpg)
Document: Implementation/Test Plan ] To document your implementation plan, use u Implementation Plan Schedule the sub phases (coding, inspection, unit test plan, unit testing, integration test plan, integration test) using a Gantt Chart Add resource (name of assigned team member) to schedule Update the Gantt chart for your project! u Describe the content of each sub phase by using your Use Case Diagram (see Textbook, page 246, Deliverable 7. 2, note that the textbook use the term “phase”, but we use the term “sub phase”) Janice Regan, 2008 39
![For your term project Use thread implementation approach Determine of sub For your term project: ] Use thread implementation approach ] Determine # of sub](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-40.jpg)
For your term project: ] Use thread implementation approach ] Determine # of sub phases and their content u Consider core use cases vs non-core use cases Distribute core use cases between the early phases Distribute the non core use cases between the late phases u This way you will have a functional, if partially implemented project, after implementing the first phase or phases ] Build the Implementation plan schedule by scheduling the resulting sub phases u Be sure you take into account the components needed to test each phase, are the phases independent or sequential. Janice Regan, 2008 40
![Evolutionary Software development Software system to be developed is divided into development subgoals Evolutionary Software development ] Software system to be developed is divided into development subgoals](https://slidetodoc.com/presentation_image_h2/4d75e2cbbefd0cfe99da49b44c86e8f4/image-41.jpg)
Evolutionary Software development ] Software system to be developed is divided into development subgoals (phases) ] A sub goal is u One use case for each iteration u A group of tightly coupled used cases for each iteration ] In a pure evolutionary approach, each development sub goal is implemented by a full cycle through the development process, from requirements analysis to implementation and testing. Janice Regan, 2008 41
Mips implementation overview
Test implementation phase
Implementation phase meaning
The most creative and challenging phase of sdlc is
Normal phase vs reverse phase chromatography
Tswett pronunciation
Mobile phase and stationary phase
Stationary phase and mobile phase in hplc
Normal phase vs reverse phase chromatography
Line current and phase current
Adsorption chromatography
In a triangle connected source feeding a y connected load
Csce 441
Anovulatory cycle
3 sigma value
Overview of www
Maximo work order priority
Uml overview
Uml
Vertical retailer
Figure 12-1 provides an overview of the lymphatic vessels
Systemic artery
Texas public school finance overview
Walmart
Stylistic overview of architecture
Overview of sa/sd methodology
Spring framework overview
Nagios tactical overview
Market overview managed file transfer solutions
Sdn nfv overview
Sbic program overview
Sap pb00
Sap ariba overview
Safe overview
Rfid technology overview
Sots meaning in research
Perbedaan replikasi virus dna dan rna
Example of a project title
Overview of the major systemic arteries
Summary vs abstract
Solvency 2 pillar 2
Which of the following is a physical storage media