Mediator Pattern n n The Mediator pattern defines
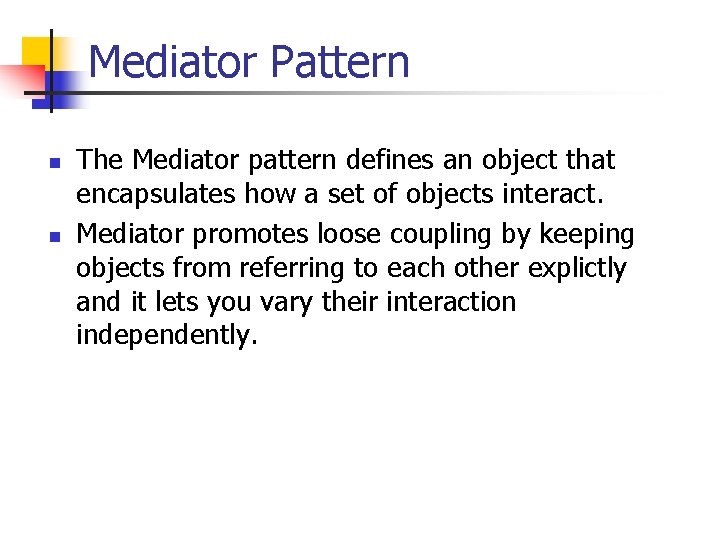
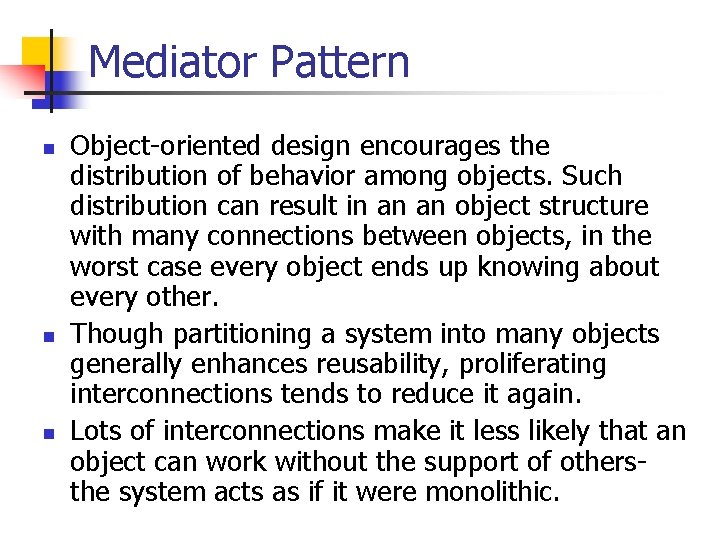
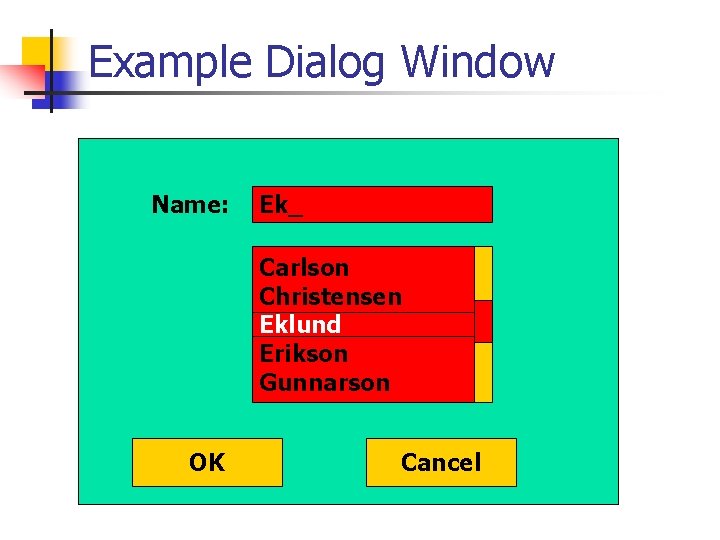
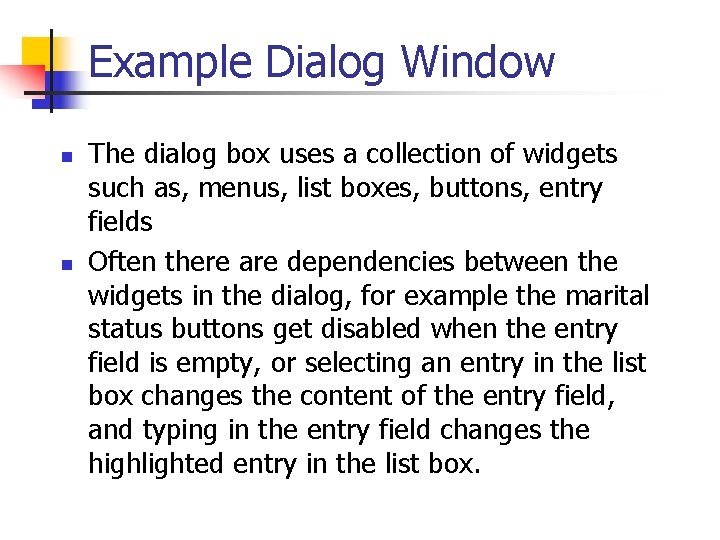
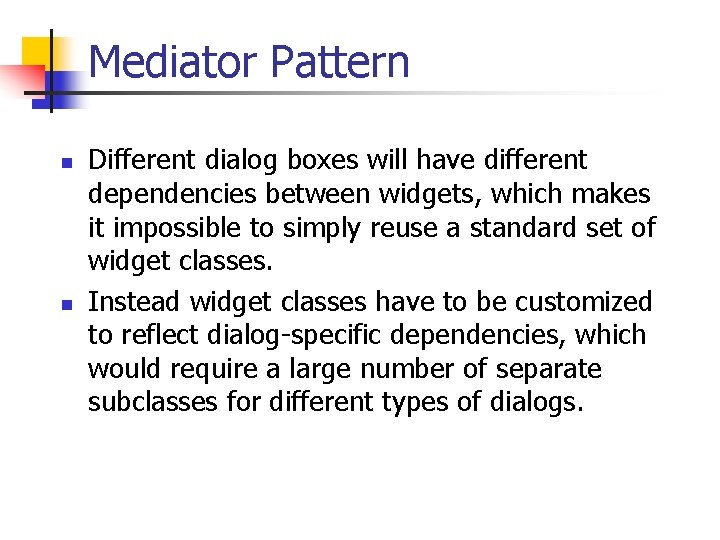
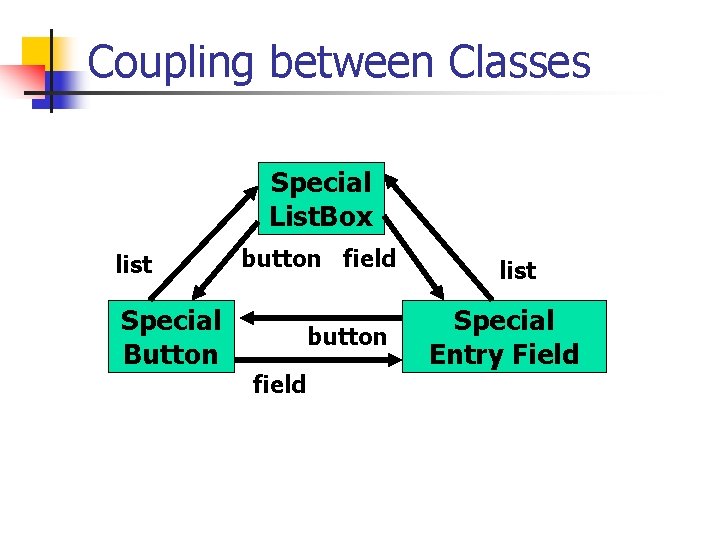
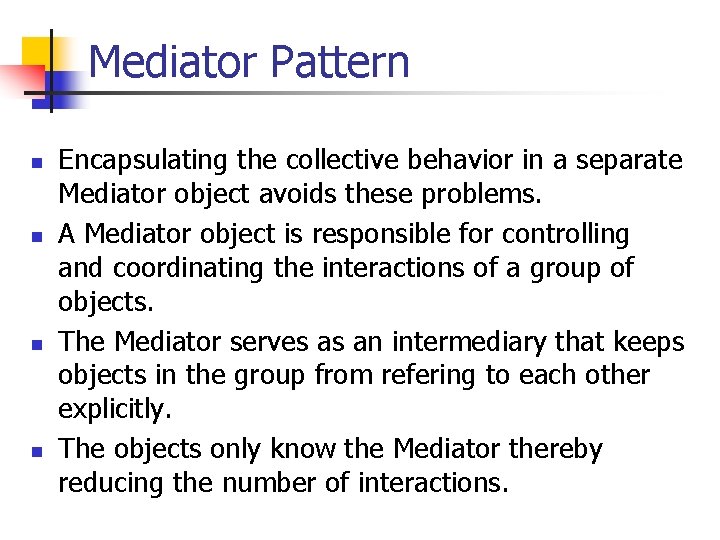
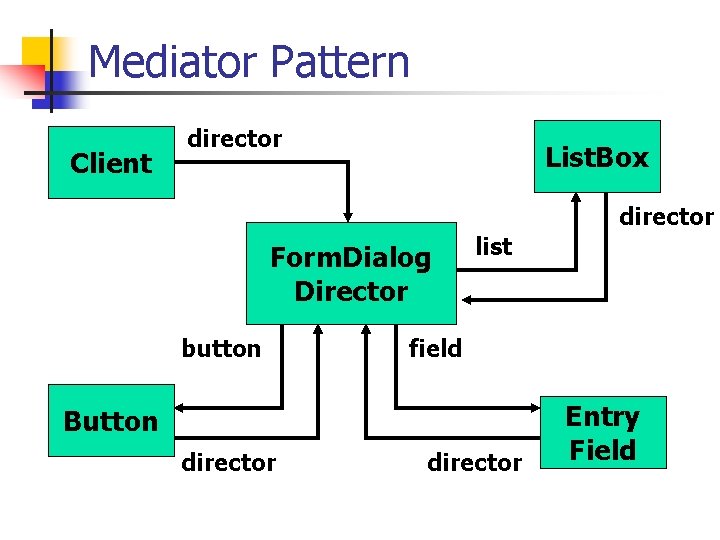
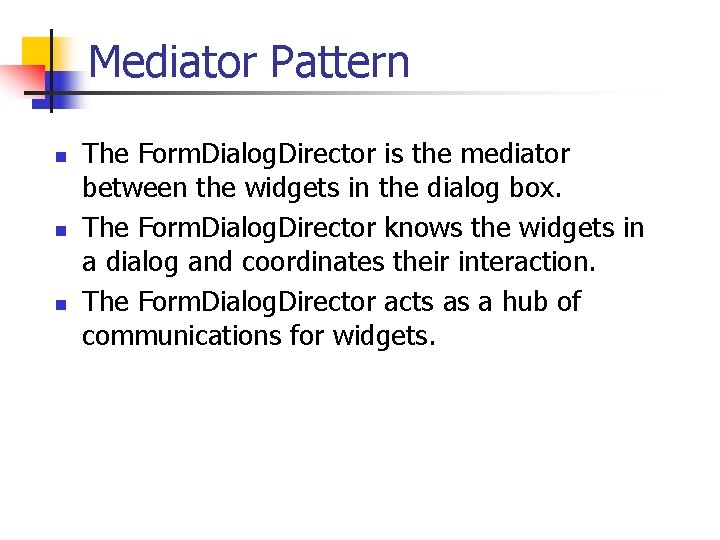
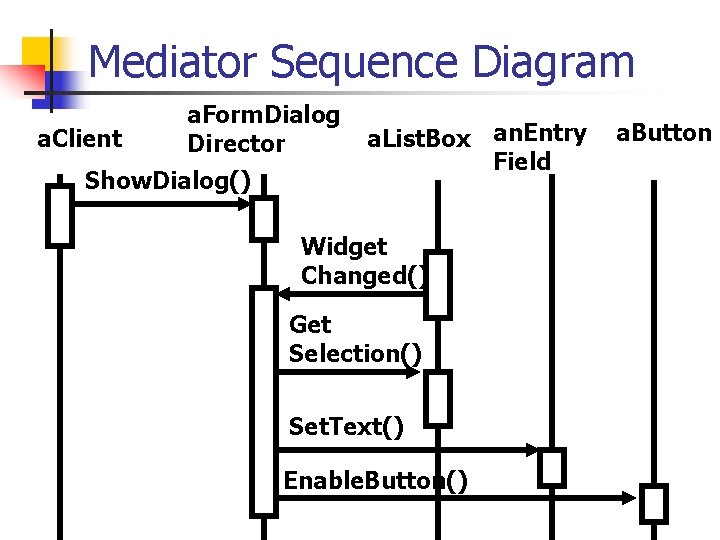
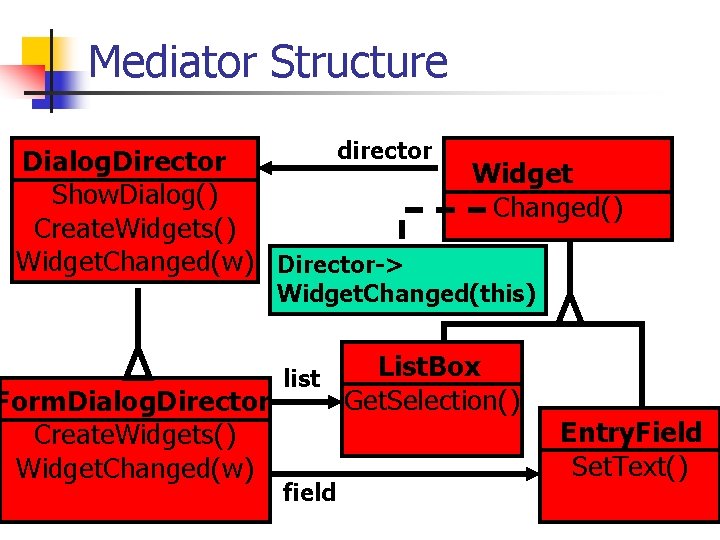
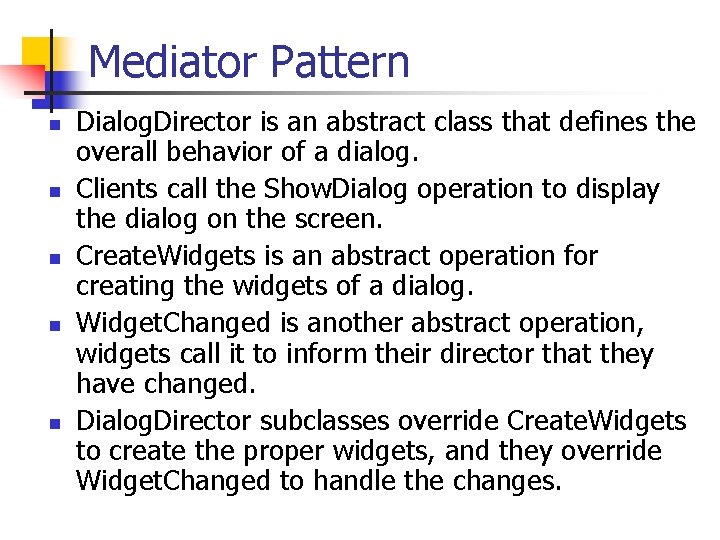
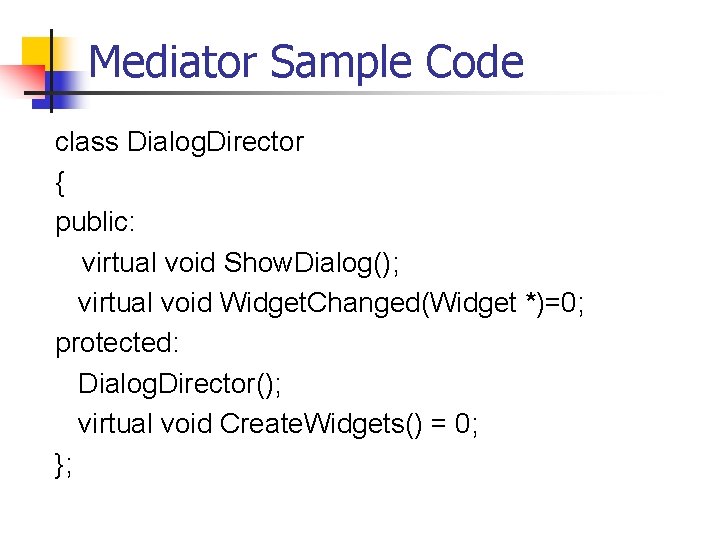
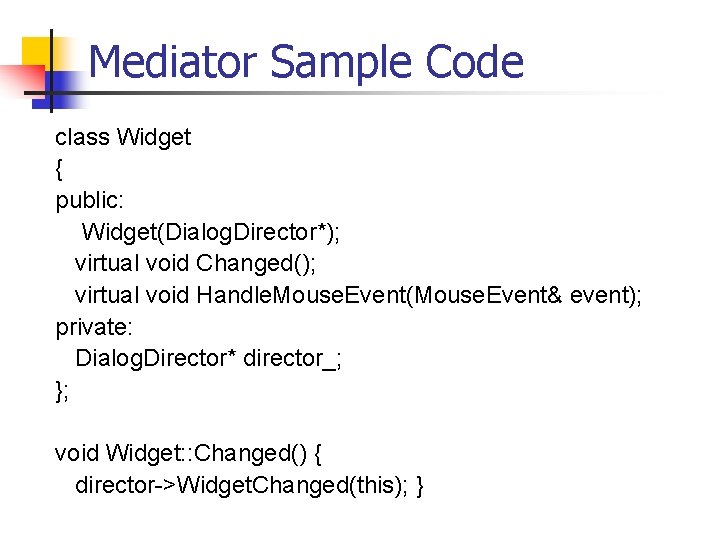
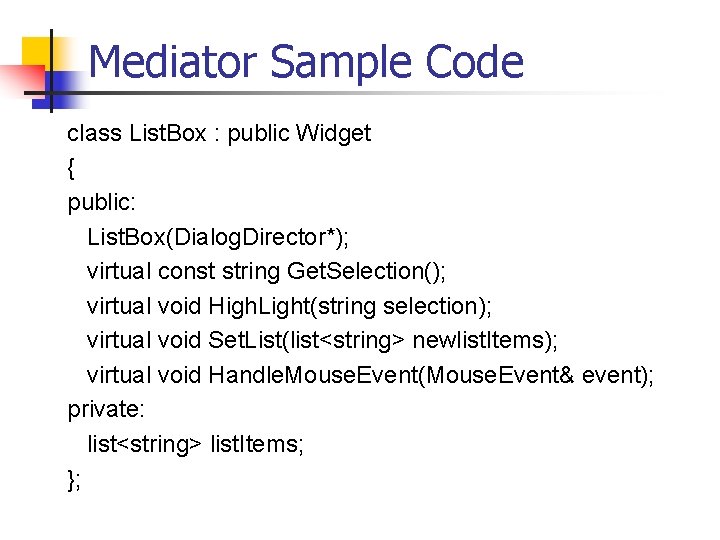
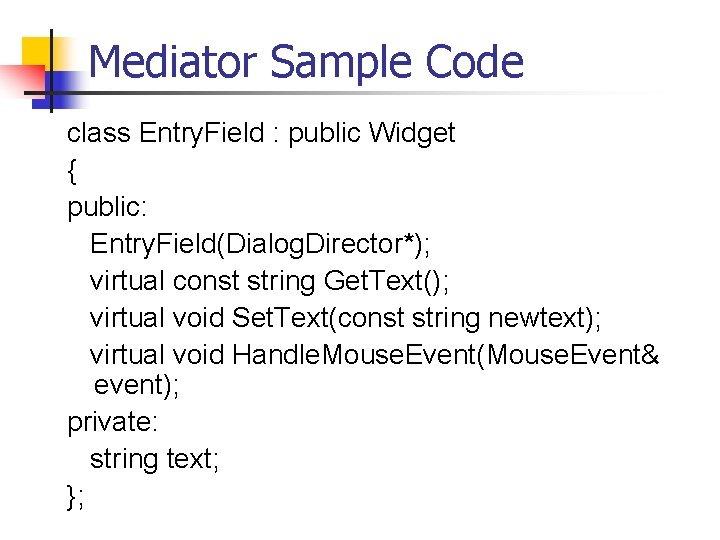
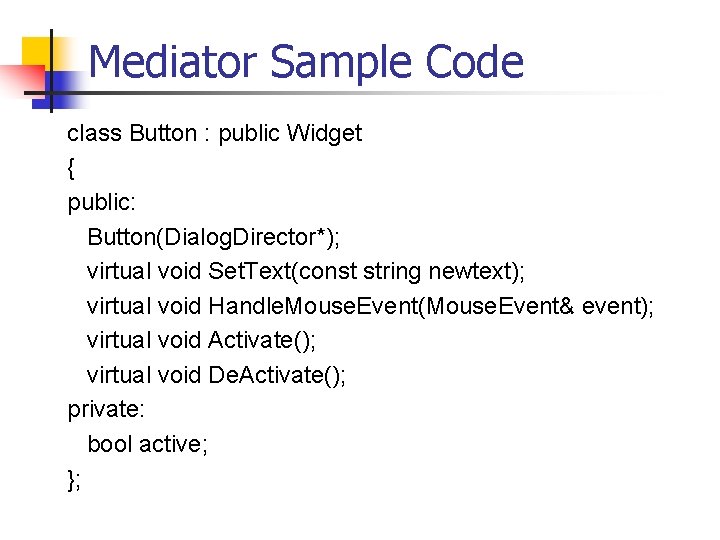
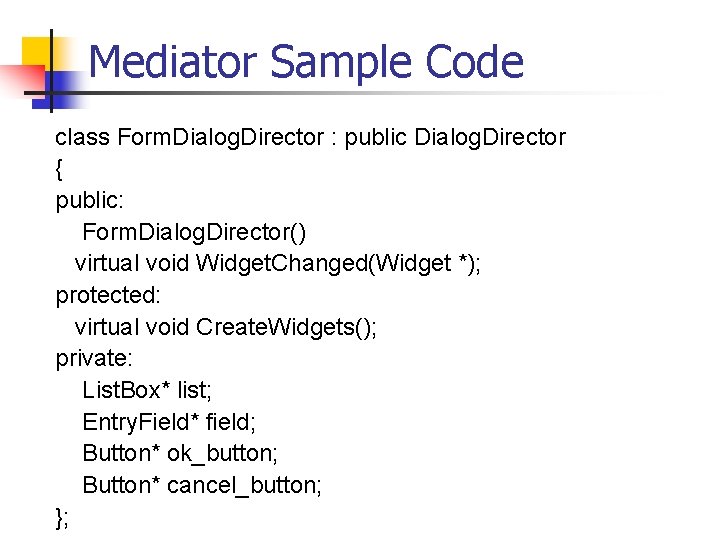
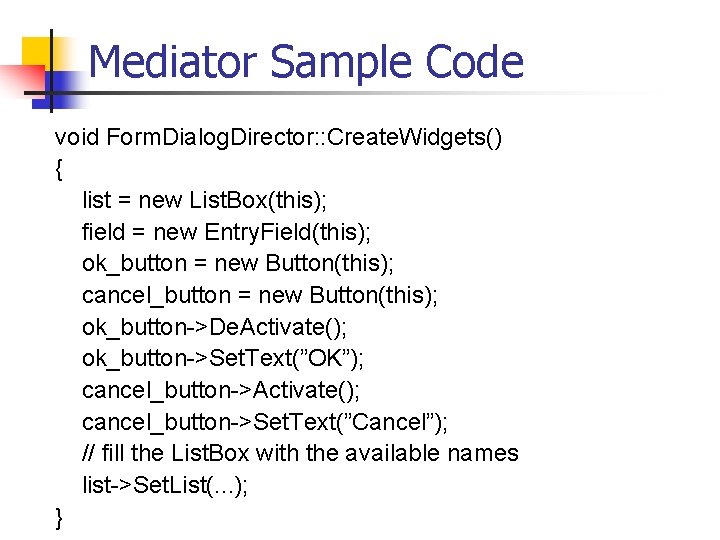
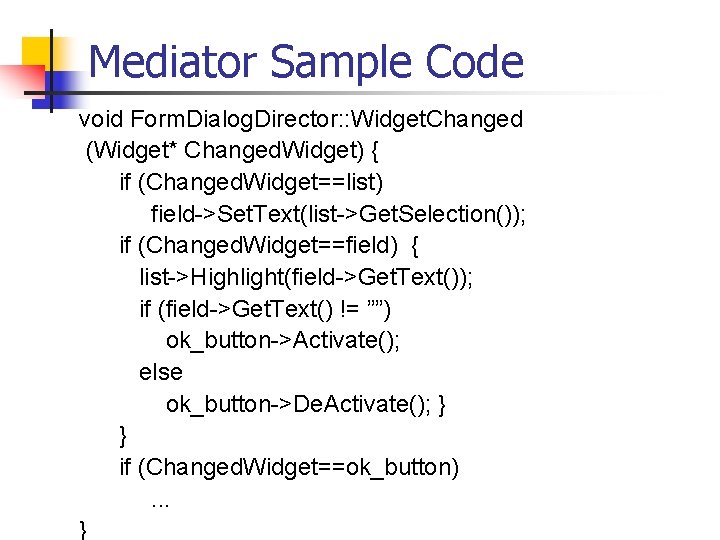
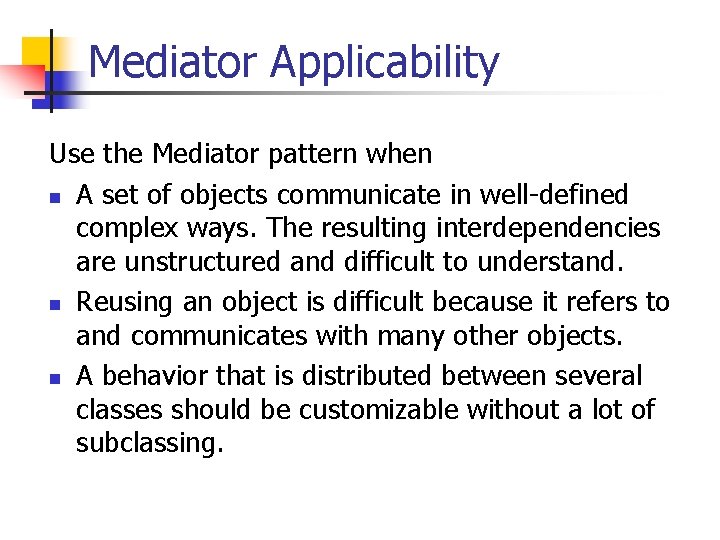
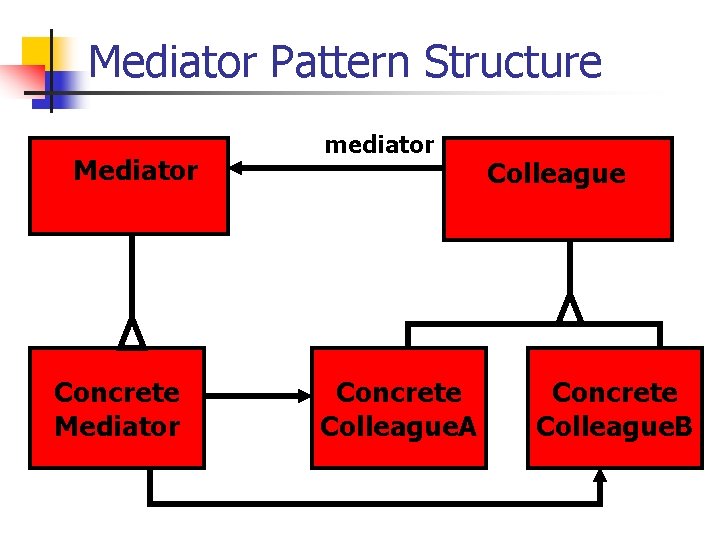
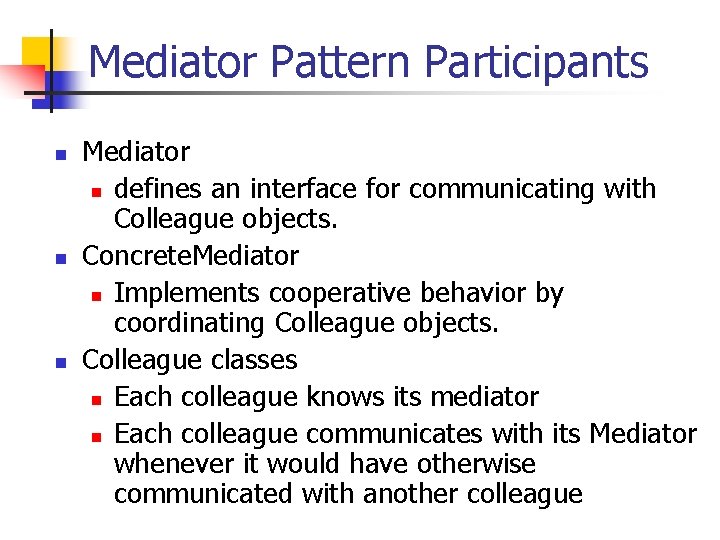
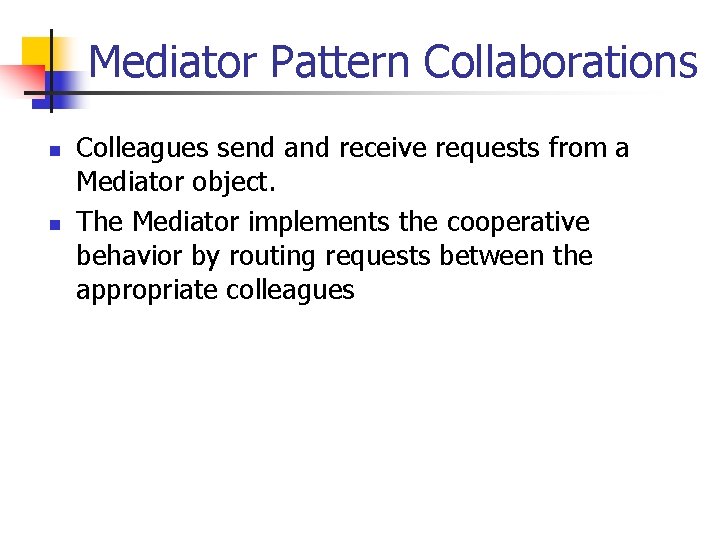
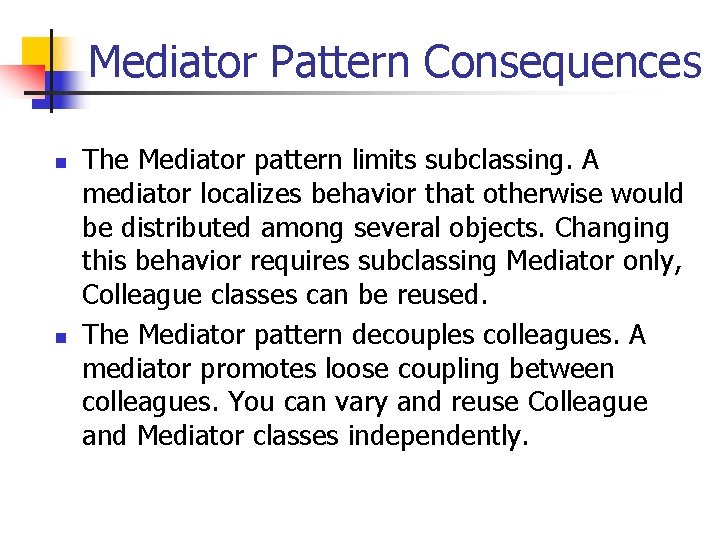
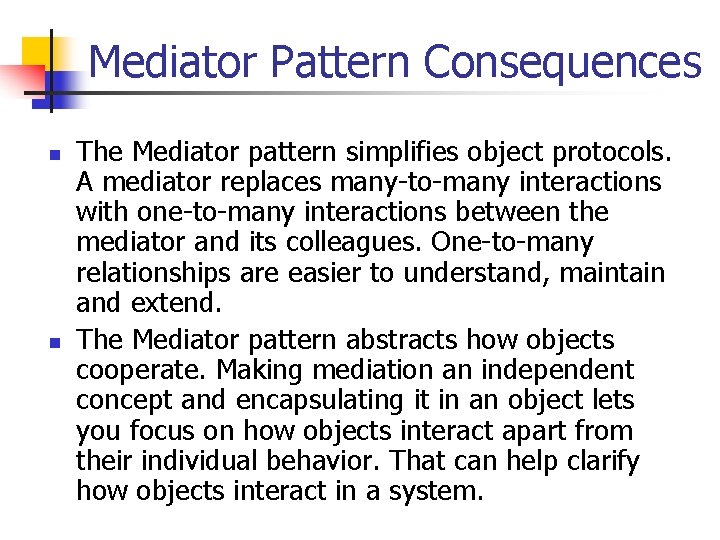
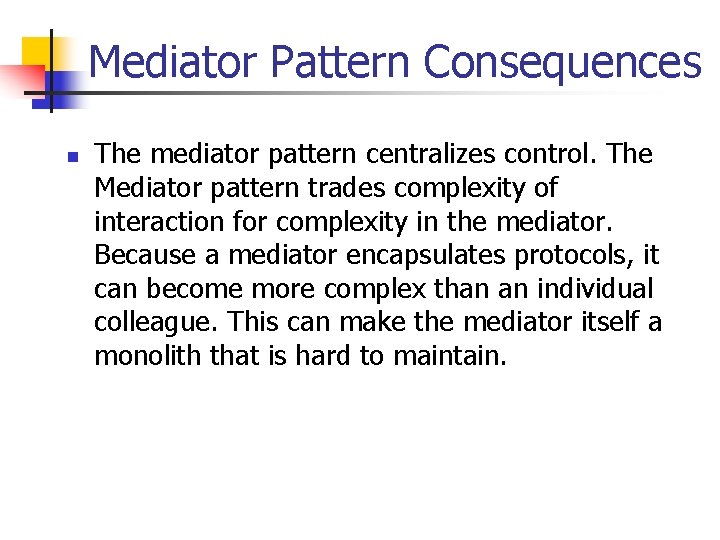
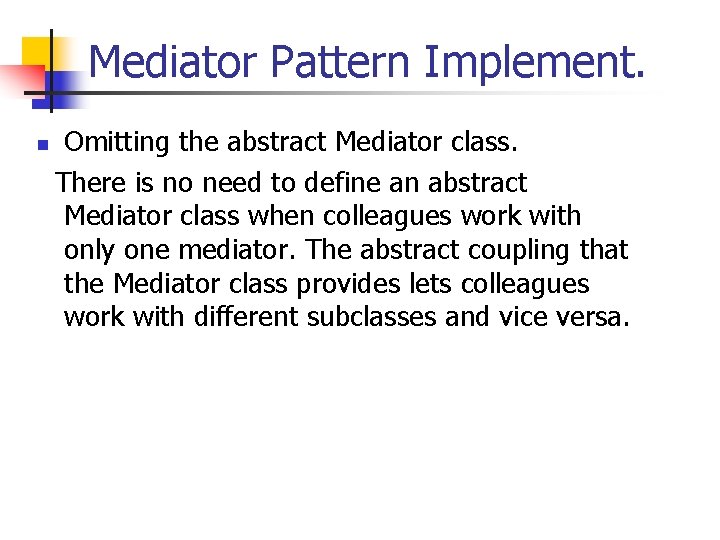
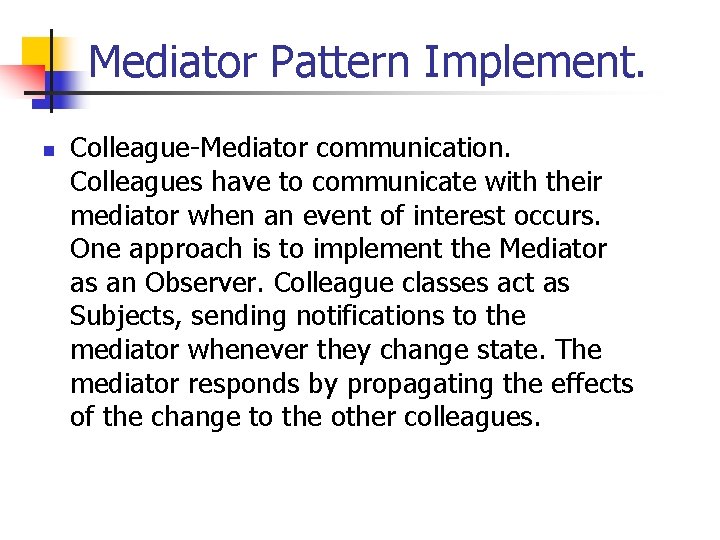
- Slides: 29
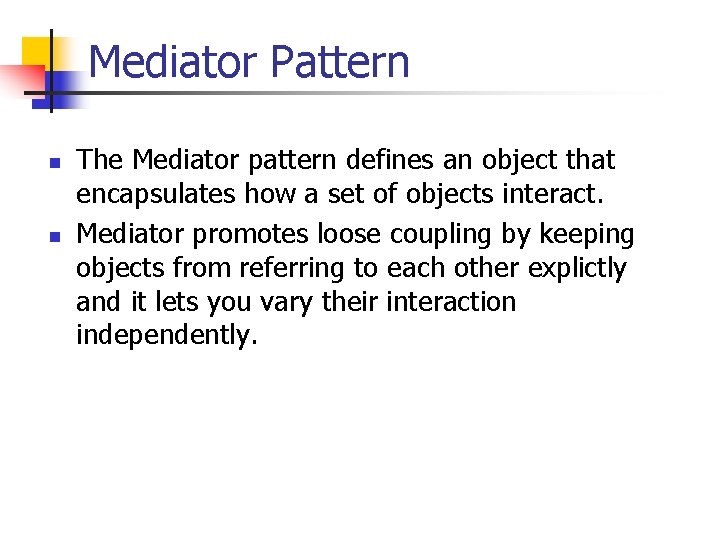
Mediator Pattern n n The Mediator pattern defines an object that encapsulates how a set of objects interact. Mediator promotes loose coupling by keeping objects from referring to each other explictly and it lets you vary their interaction independently.
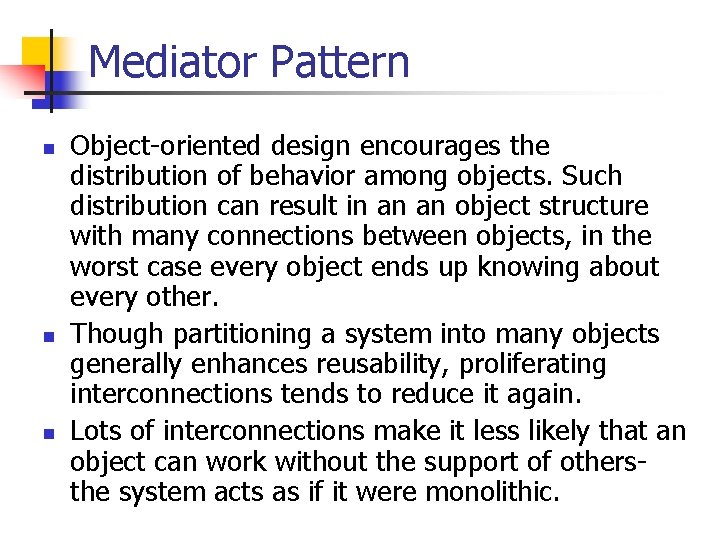
Mediator Pattern n Object-oriented design encourages the distribution of behavior among objects. Such distribution can result in an an object structure with many connections between objects, in the worst case every object ends up knowing about every other. Though partitioning a system into many objects generally enhances reusability, proliferating interconnections tends to reduce it again. Lots of interconnections make it less likely that an object can work without the support of othersthe system acts as if it were monolithic.
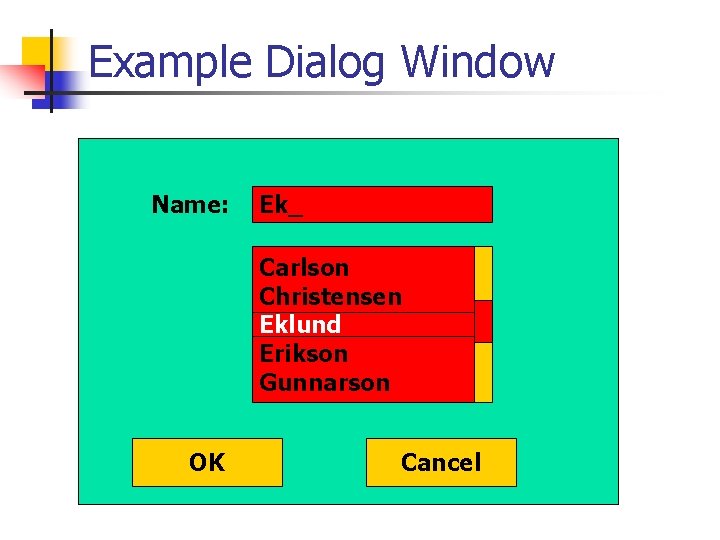
Example Dialog Window Name: Ek_ Carlson Christensen Eklund Erikson Gunnarson OK Cancel
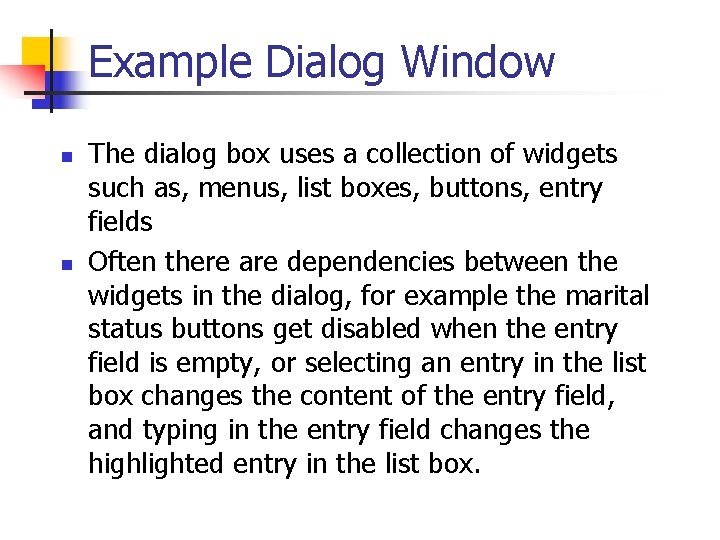
Example Dialog Window n n The dialog box uses a collection of widgets such as, menus, list boxes, buttons, entry fields Often there are dependencies between the widgets in the dialog, for example the marital status buttons get disabled when the entry field is empty, or selecting an entry in the list box changes the content of the entry field, and typing in the entry field changes the highlighted entry in the list box.
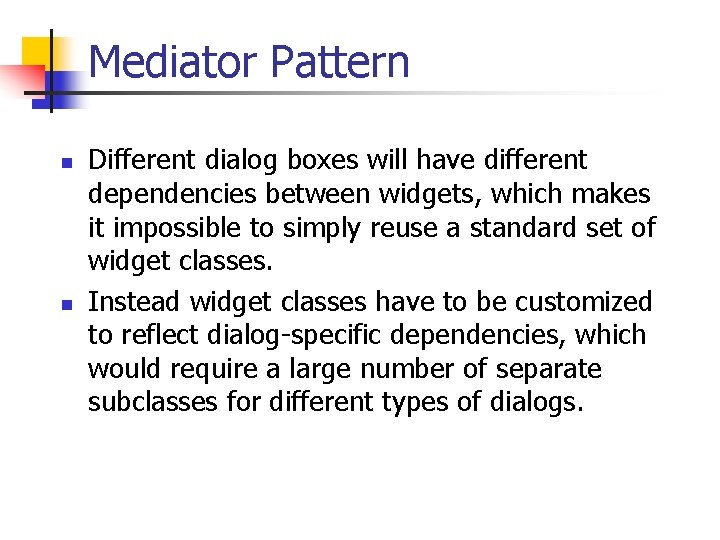
Mediator Pattern n n Different dialog boxes will have different dependencies between widgets, which makes it impossible to simply reuse a standard set of widget classes. Instead widget classes have to be customized to reflect dialog-specific dependencies, which would require a large number of separate subclasses for different types of dialogs.
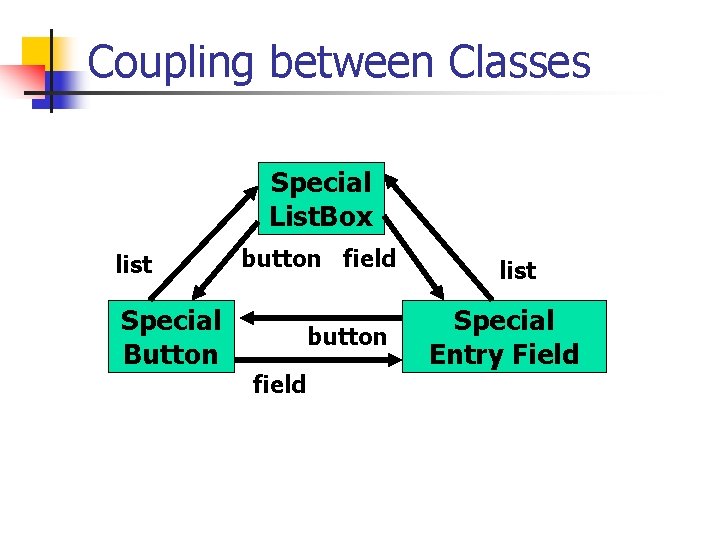
Coupling between Classes Special List. Box list Special Button button field list Special Entry Field
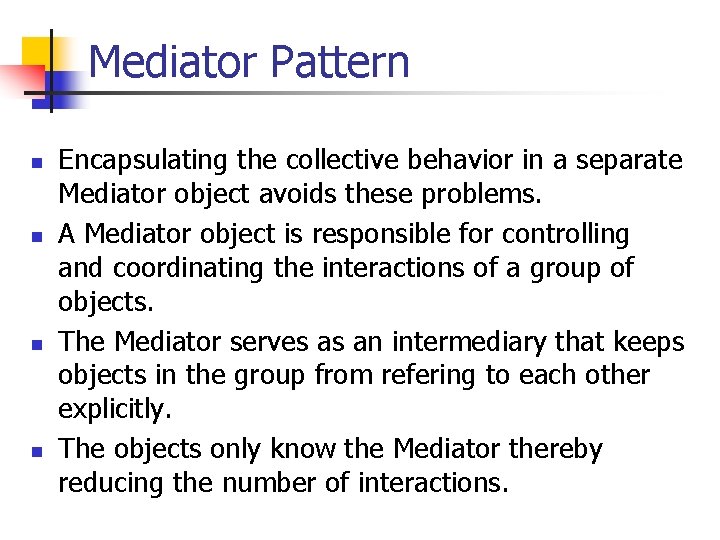
Mediator Pattern n n Encapsulating the collective behavior in a separate Mediator object avoids these problems. A Mediator object is responsible for controlling and coordinating the interactions of a group of objects. The Mediator serves as an intermediary that keeps objects in the group from refering to each other explicitly. The objects only know the Mediator thereby reducing the number of interactions.
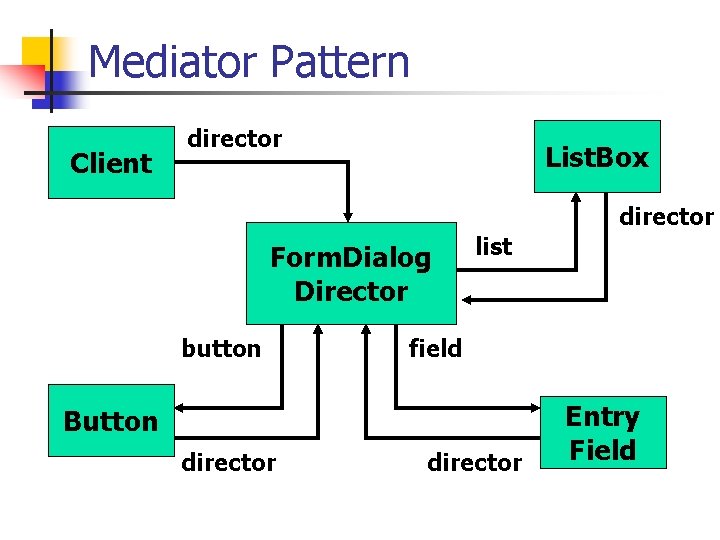
Mediator Pattern Client director List. Box director Form. Dialog Director button list field Button director Entry Field
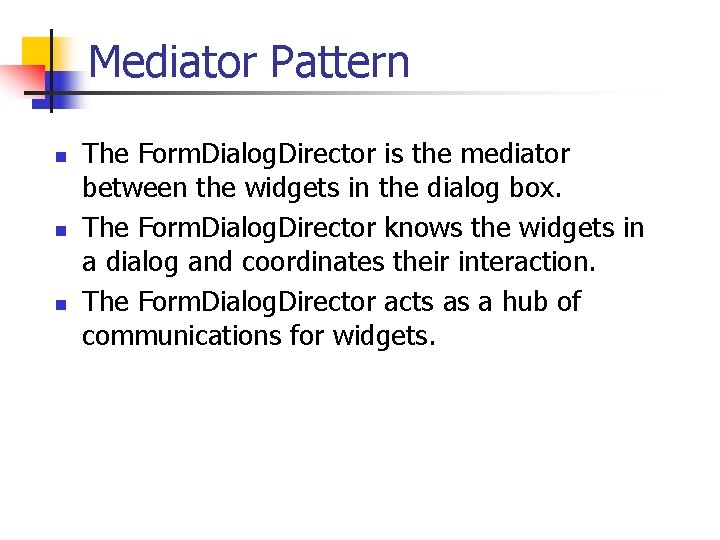
Mediator Pattern n The Form. Dialog. Director is the mediator between the widgets in the dialog box. The Form. Dialog. Director knows the widgets in a dialog and coordinates their interaction. The Form. Dialog. Director acts as a hub of communications for widgets.
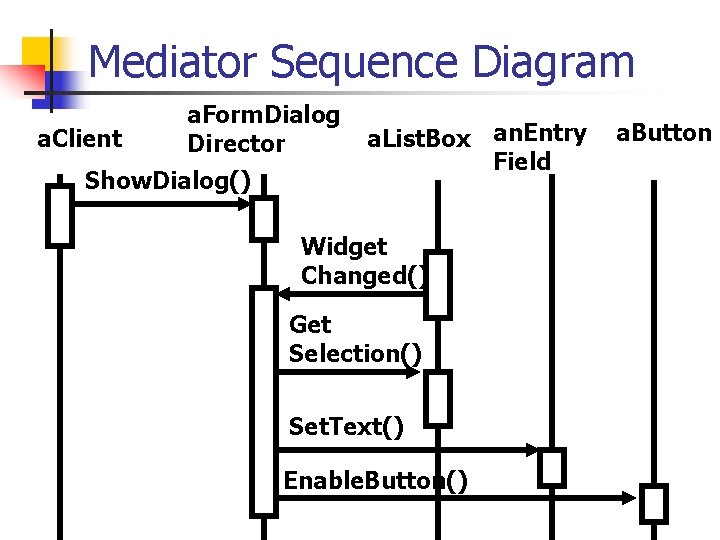
Mediator Sequence Diagram a. Client a. Form. Dialog Director Show. Dialog() a. List. Box an. Entry Field Widget Changed() Get Selection() Set. Text() Enable. Button() a. Button
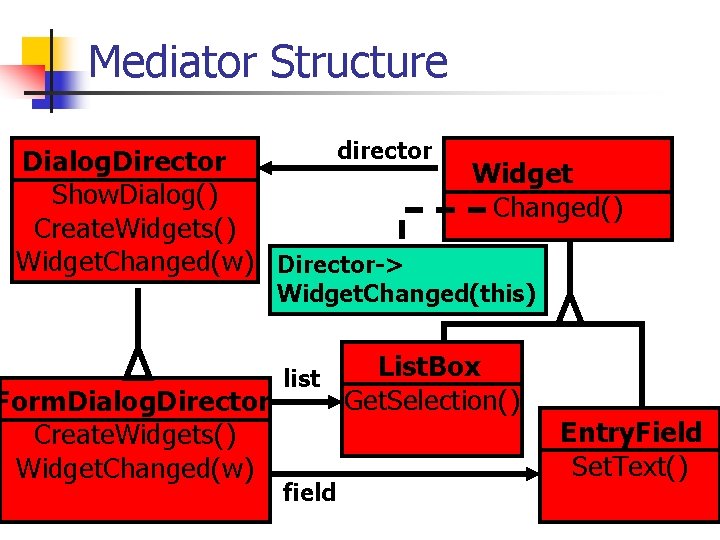
Mediator Structure director Dialog. Director Show. Dialog() Create. Widgets() Widget. Changed(w) Director-> Form. Dialog. Director Create. Widgets() Widget. Changed(w) Widget Changed() Widget. Changed(this) list field List. Box Get. Selection() Entry. Field Set. Text()
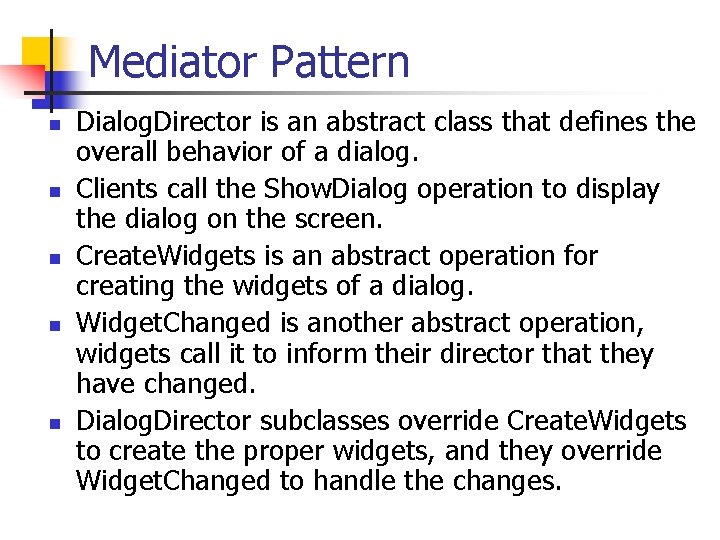
Mediator Pattern n n Dialog. Director is an abstract class that defines the overall behavior of a dialog. Clients call the Show. Dialog operation to display the dialog on the screen. Create. Widgets is an abstract operation for creating the widgets of a dialog. Widget. Changed is another abstract operation, widgets call it to inform their director that they have changed. Dialog. Director subclasses override Create. Widgets to create the proper widgets, and they override Widget. Changed to handle the changes.
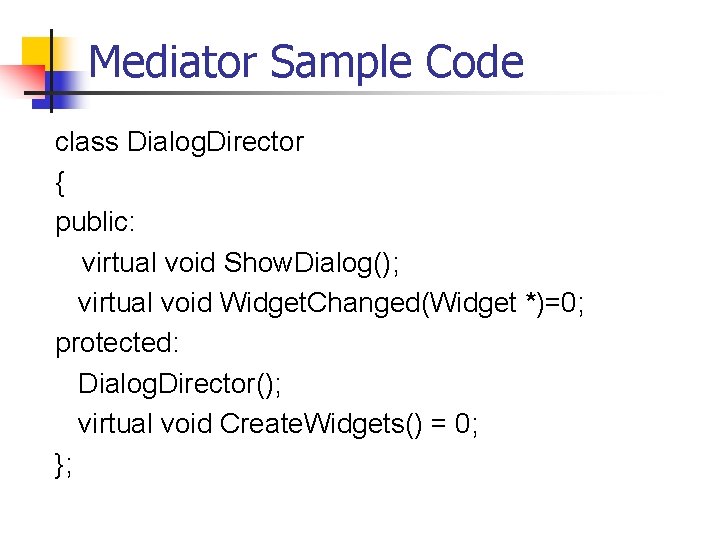
Mediator Sample Code class Dialog. Director { public: virtual void Show. Dialog(); virtual void Widget. Changed(Widget *)=0; protected: Dialog. Director(); virtual void Create. Widgets() = 0; };
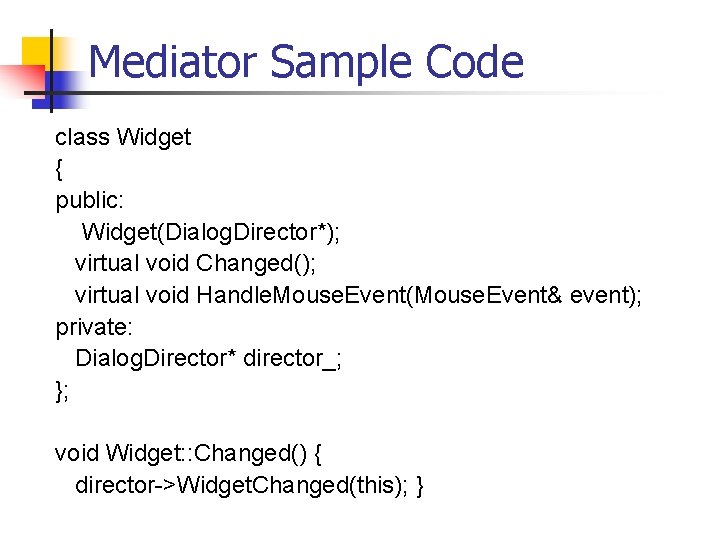
Mediator Sample Code class Widget { public: Widget(Dialog. Director*); virtual void Changed(); virtual void Handle. Mouse. Event(Mouse. Event& event); private: Dialog. Director* director_; }; void Widget: : Changed() { director->Widget. Changed(this); }
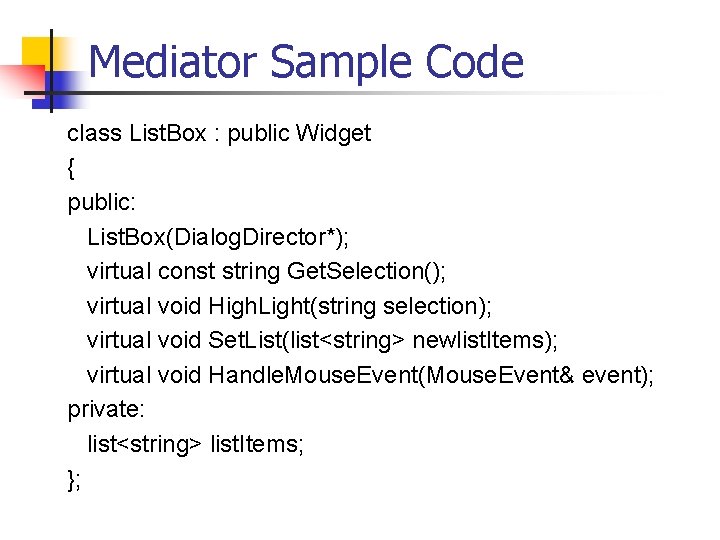
Mediator Sample Code class List. Box : public Widget { public: List. Box(Dialog. Director*); virtual const string Get. Selection(); virtual void High. Light(string selection); virtual void Set. List(list<string> newlist. Items); virtual void Handle. Mouse. Event(Mouse. Event& event); private: list<string> list. Items; };
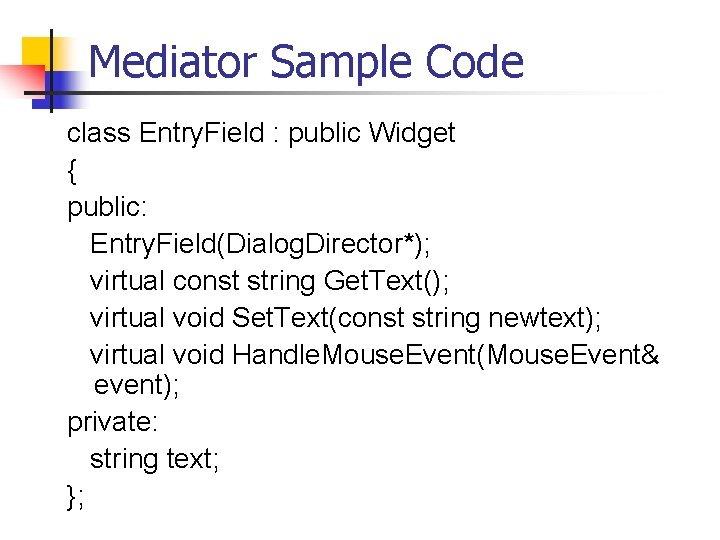
Mediator Sample Code class Entry. Field : public Widget { public: Entry. Field(Dialog. Director*); virtual const string Get. Text(); virtual void Set. Text(const string newtext); virtual void Handle. Mouse. Event(Mouse. Event& event); private: string text; };
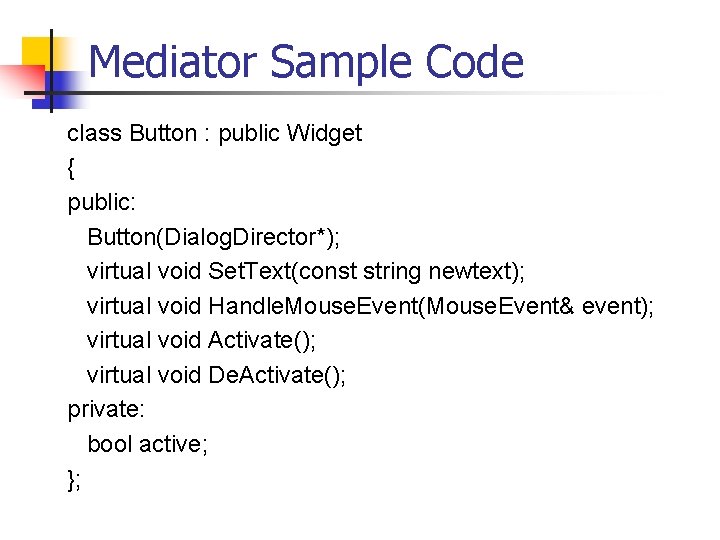
Mediator Sample Code class Button : public Widget { public: Button(Dialog. Director*); virtual void Set. Text(const string newtext); virtual void Handle. Mouse. Event(Mouse. Event& event); virtual void Activate(); virtual void De. Activate(); private: bool active; };
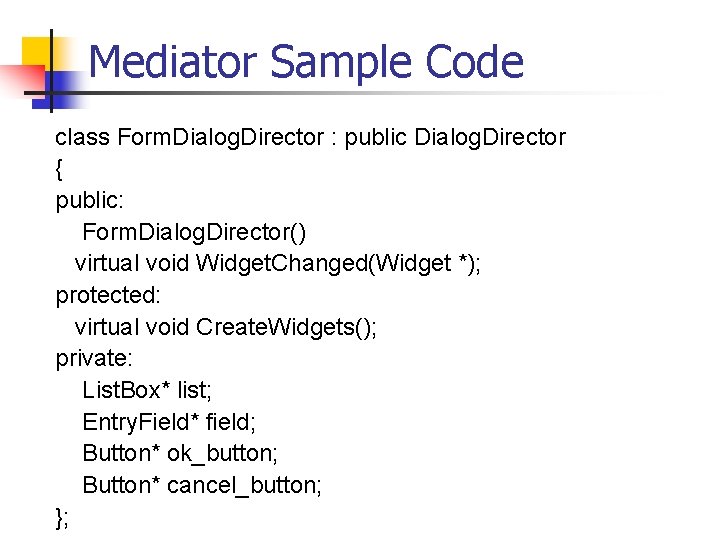
Mediator Sample Code class Form. Dialog. Director : public Dialog. Director { public: Form. Dialog. Director() virtual void Widget. Changed(Widget *); protected: virtual void Create. Widgets(); private: List. Box* list; Entry. Field* field; Button* ok_button; Button* cancel_button; };
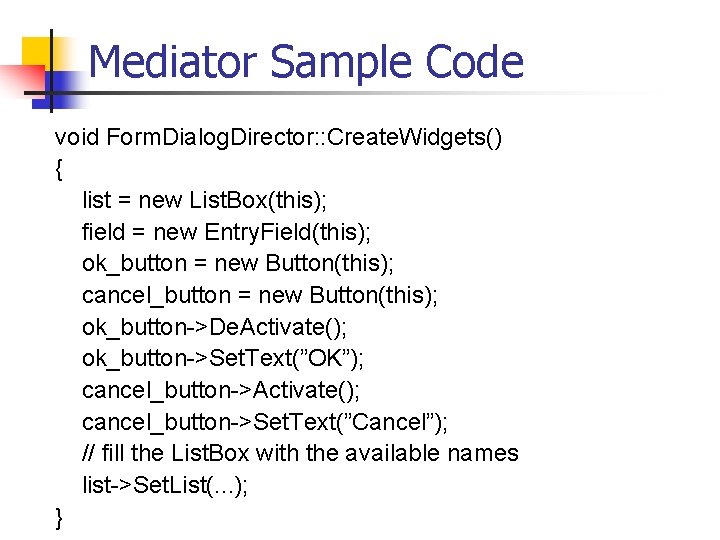
Mediator Sample Code void Form. Dialog. Director: : Create. Widgets() { list = new List. Box(this); field = new Entry. Field(this); ok_button = new Button(this); cancel_button = new Button(this); ok_button->De. Activate(); ok_button->Set. Text(”OK”); cancel_button->Activate(); cancel_button->Set. Text(”Cancel”); // fill the List. Box with the available names list->Set. List(. . . ); }
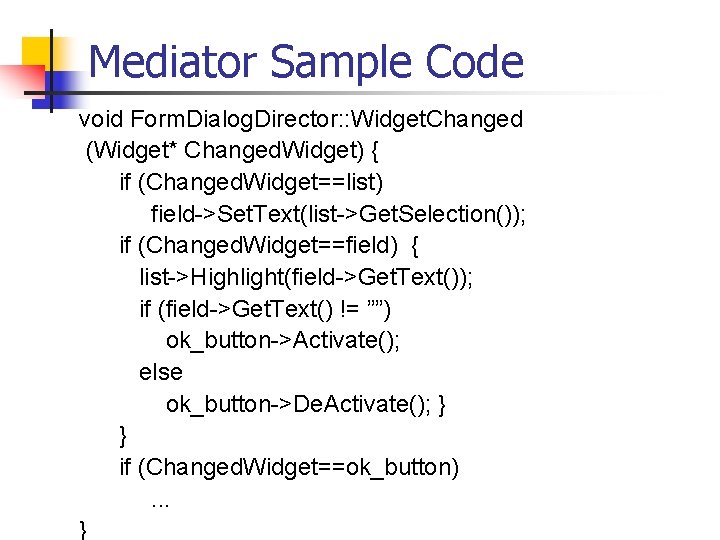
Mediator Sample Code void Form. Dialog. Director: : Widget. Changed (Widget* Changed. Widget) { if (Changed. Widget==list) field->Set. Text(list->Get. Selection()); if (Changed. Widget==field) { list->Highlight(field->Get. Text()); if (field->Get. Text() != ””) ok_button->Activate(); else ok_button->De. Activate(); } } if (Changed. Widget==ok_button). . . }
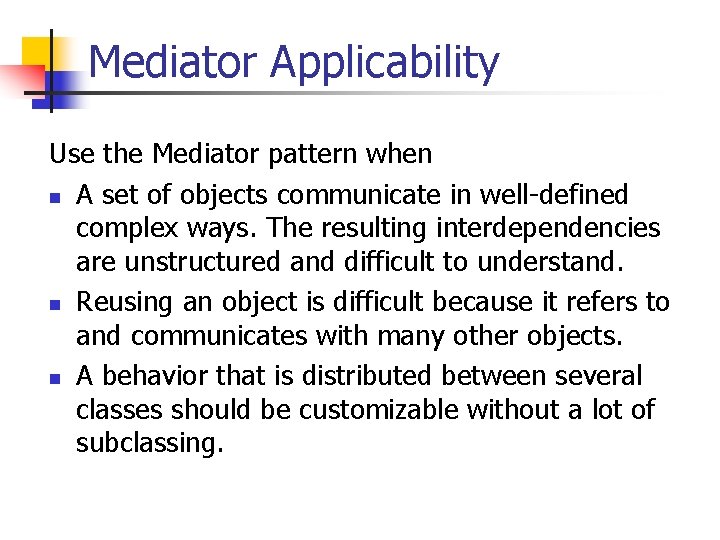
Mediator Applicability Use the Mediator pattern when n A set of objects communicate in well-defined complex ways. The resulting interdependencies are unstructured and difficult to understand. n Reusing an object is difficult because it refers to and communicates with many other objects. n A behavior that is distributed between several classes should be customizable without a lot of subclassing.
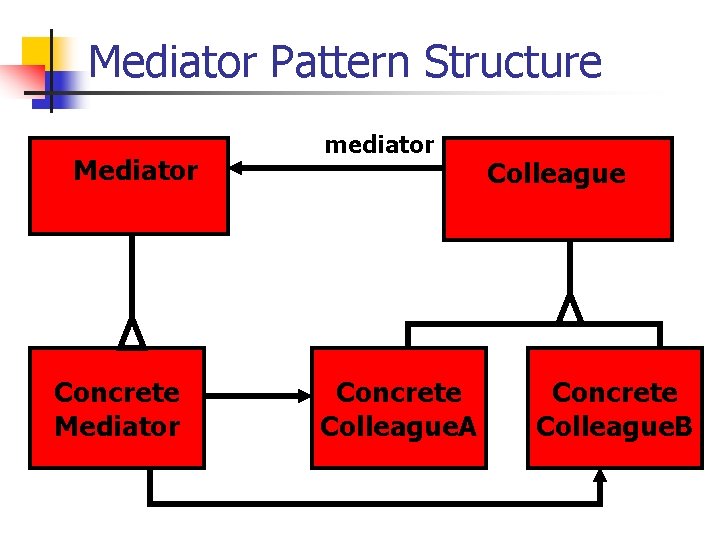
Mediator Pattern Structure Mediator Concrete Mediator mediator Concrete Colleague. A Colleague Concrete Colleague. B
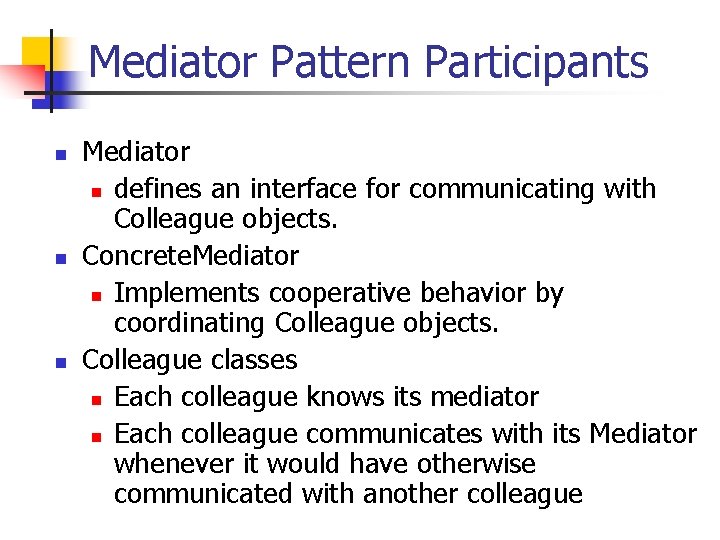
Mediator Pattern Participants n n n Mediator n defines an interface for communicating with Colleague objects. Concrete. Mediator n Implements cooperative behavior by coordinating Colleague objects. Colleague classes n Each colleague knows its mediator n Each colleague communicates with its Mediator whenever it would have otherwise communicated with another colleague
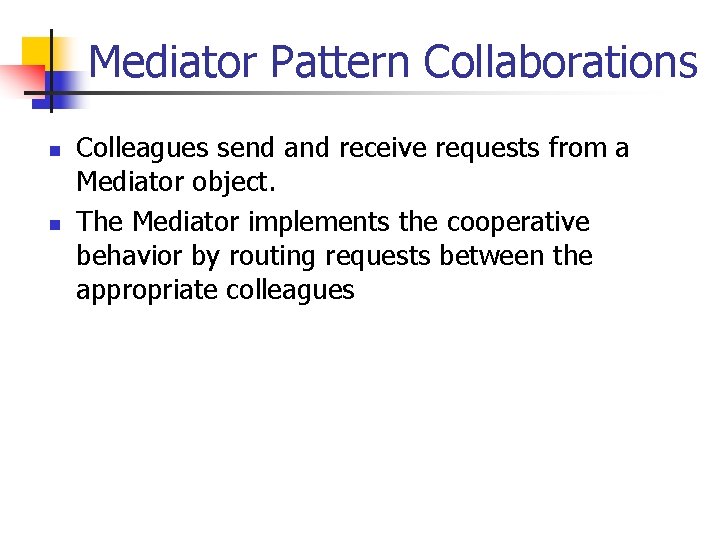
Mediator Pattern Collaborations n n Colleagues send and receive requests from a Mediator object. The Mediator implements the cooperative behavior by routing requests between the appropriate colleagues
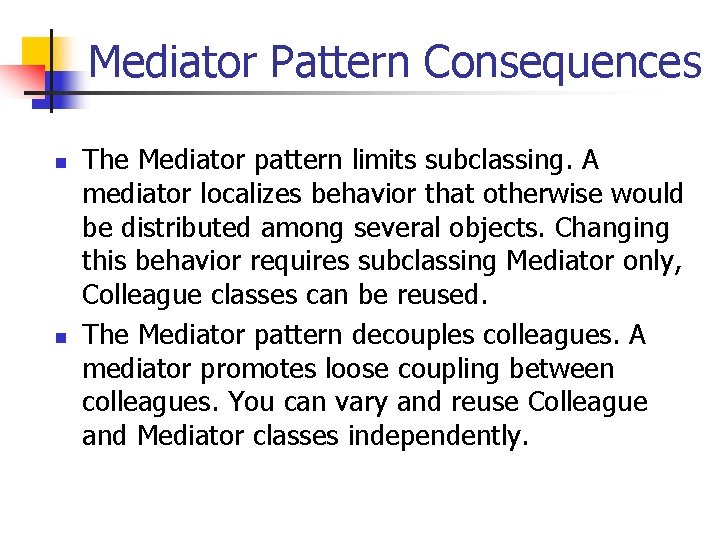
Mediator Pattern Consequences n n The Mediator pattern limits subclassing. A mediator localizes behavior that otherwise would be distributed among several objects. Changing this behavior requires subclassing Mediator only, Colleague classes can be reused. The Mediator pattern decouples colleagues. A mediator promotes loose coupling between colleagues. You can vary and reuse Colleague and Mediator classes independently.
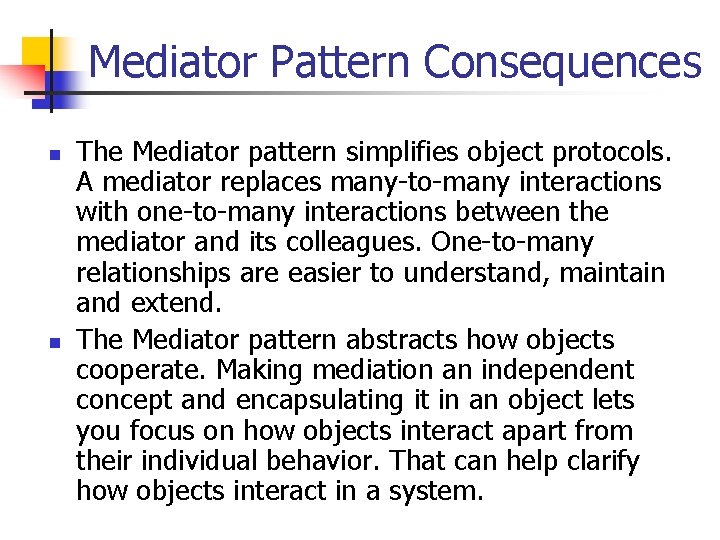
Mediator Pattern Consequences n n The Mediator pattern simplifies object protocols. A mediator replaces many-to-many interactions with one-to-many interactions between the mediator and its colleagues. One-to-many relationships are easier to understand, maintain and extend. The Mediator pattern abstracts how objects cooperate. Making mediation an independent concept and encapsulating it in an object lets you focus on how objects interact apart from their individual behavior. That can help clarify how objects interact in a system.
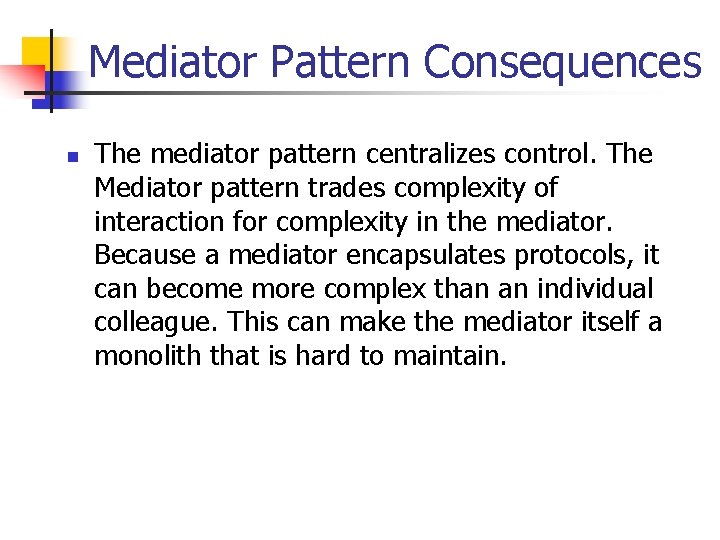
Mediator Pattern Consequences n The mediator pattern centralizes control. The Mediator pattern trades complexity of interaction for complexity in the mediator. Because a mediator encapsulates protocols, it can become more complex than an individual colleague. This can make the mediator itself a monolith that is hard to maintain.
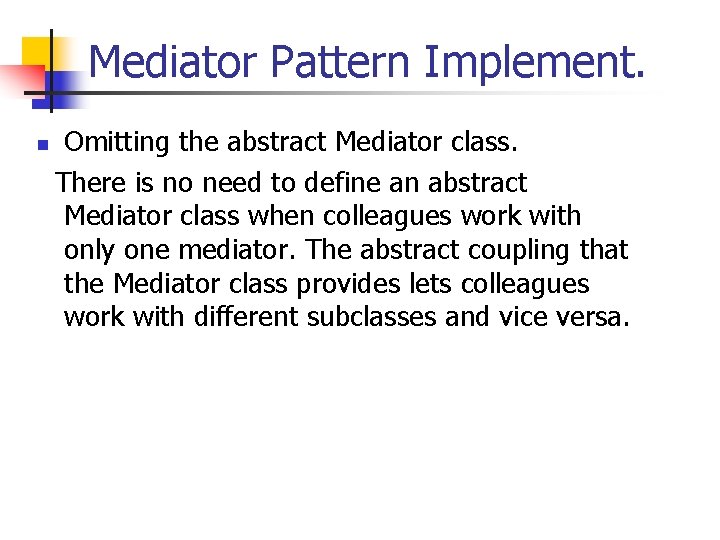
Mediator Pattern Implement. n Omitting the abstract Mediator class. There is no need to define an abstract Mediator class when colleagues work with only one mediator. The abstract coupling that the Mediator class provides lets colleagues work with different subclasses and vice versa.
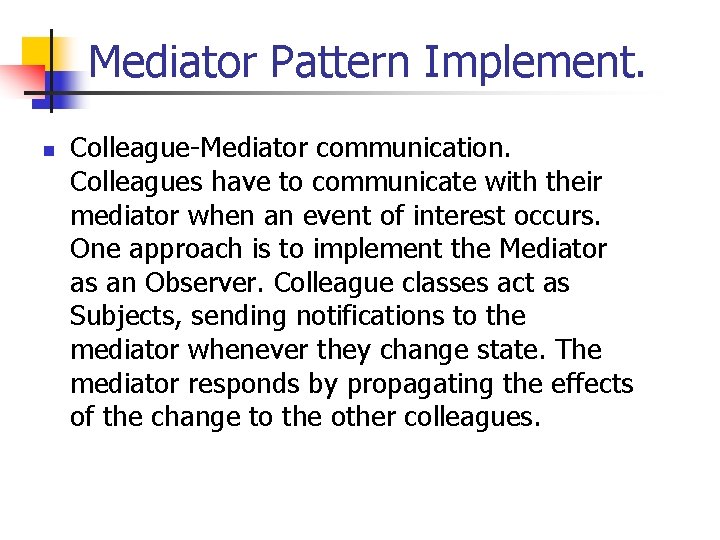
Mediator Pattern Implement. n Colleague-Mediator communication. Colleagues have to communicate with their mediator when an event of interest occurs. One approach is to implement the Mediator as an Observer. Colleague classes act as Subjects, sending notifications to the mediator whenever they change state. The mediator responds by propagating the effects of the change to the other colleagues.