MACHINELEVEL PROGRAMMING III SWITCH STATEMENTS AND IA 32
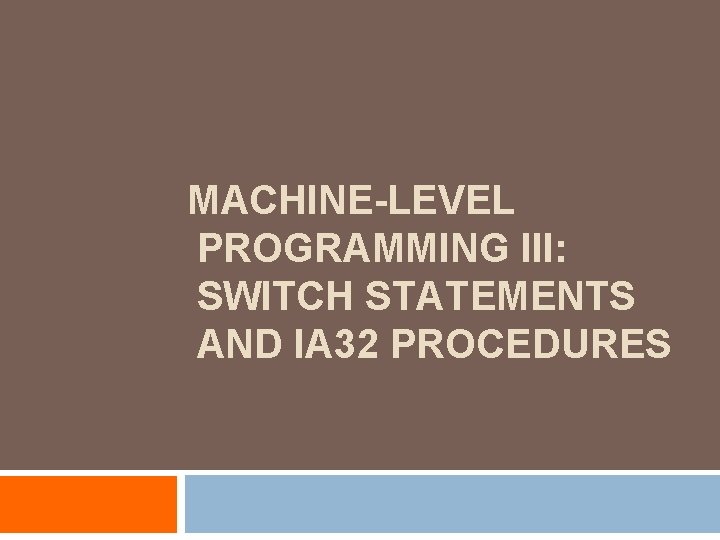
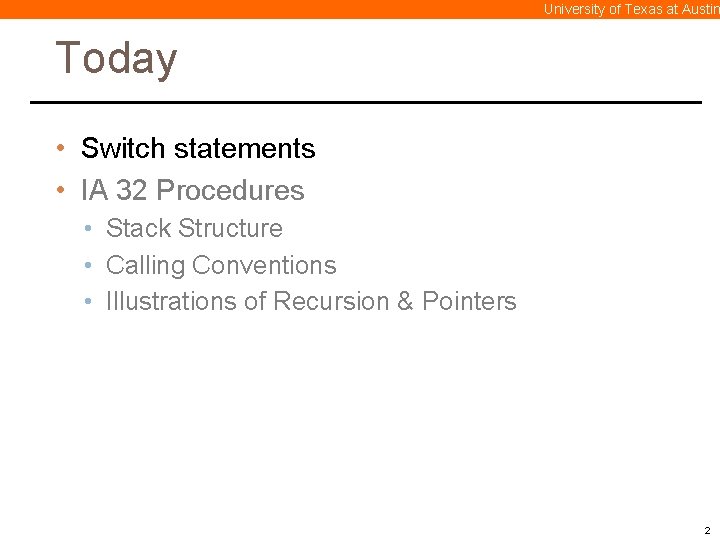
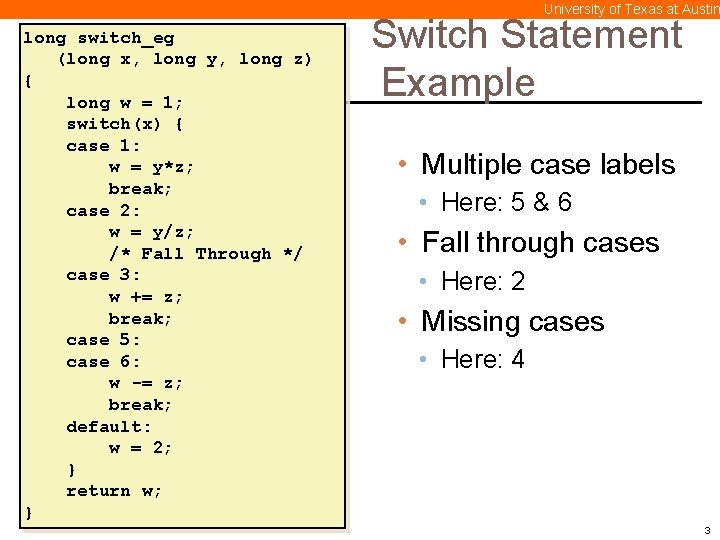
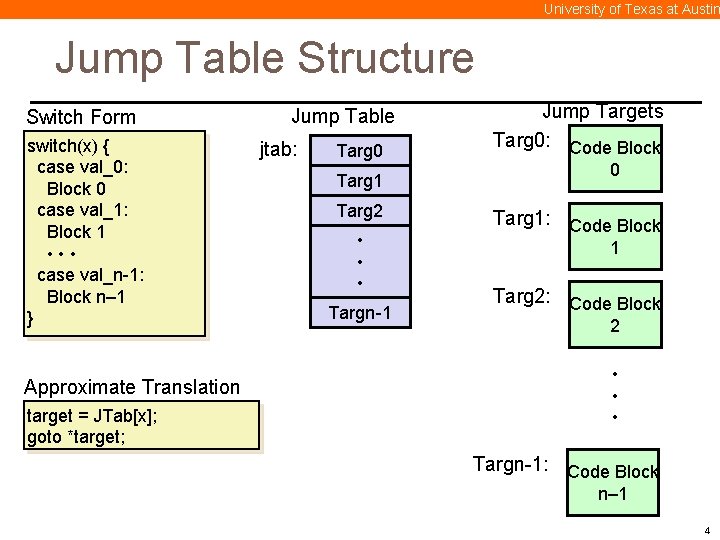
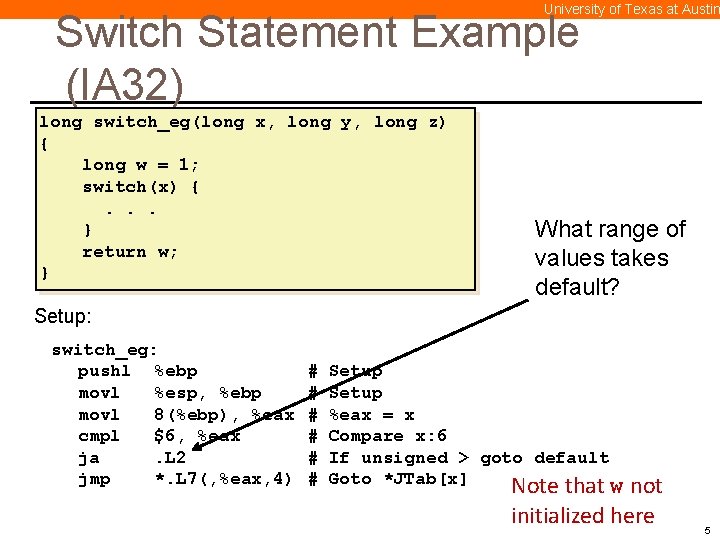
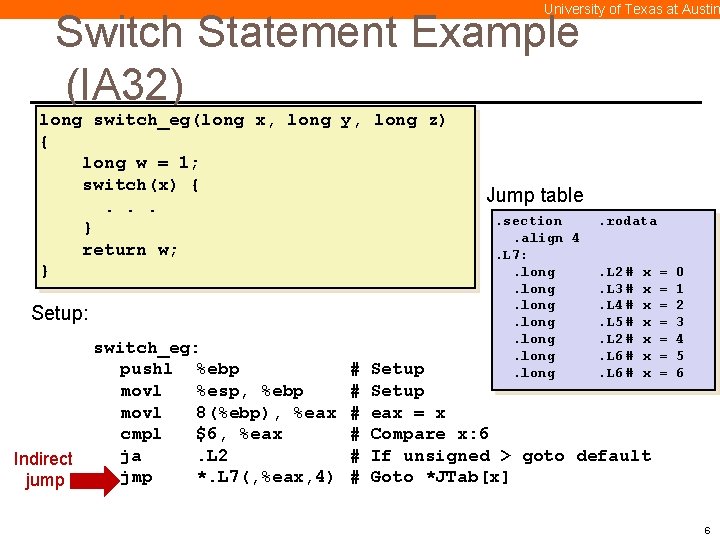
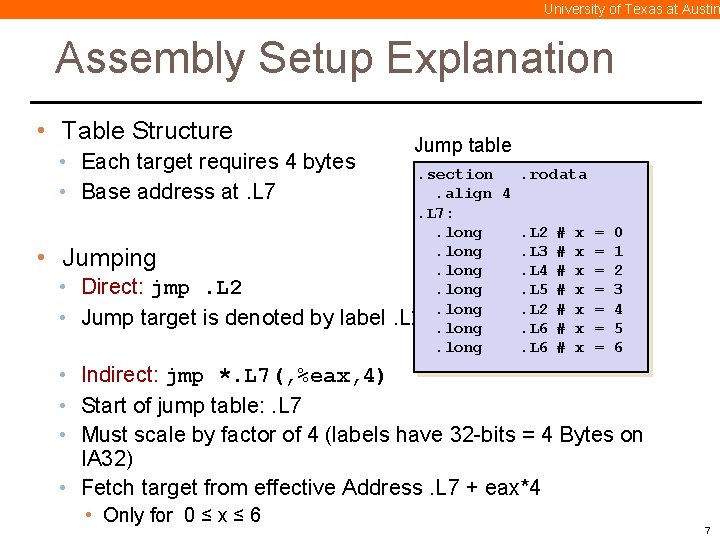
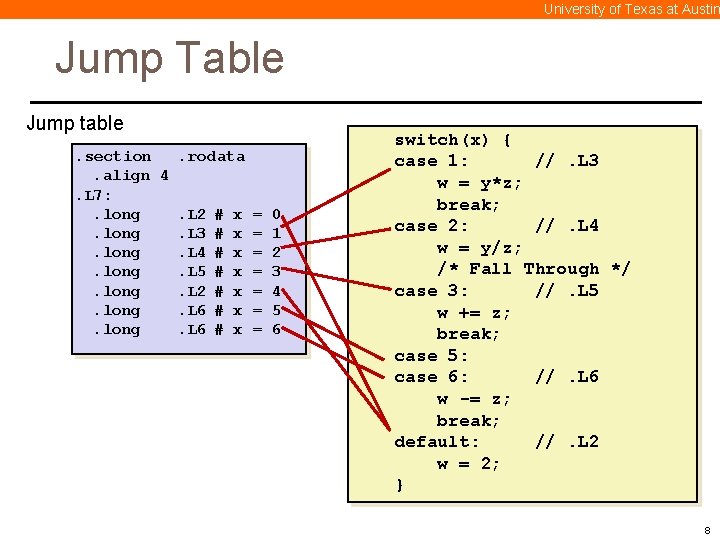
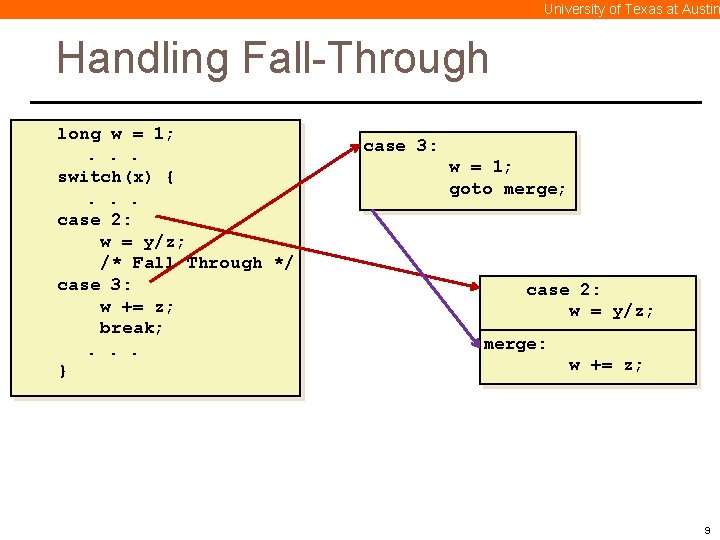
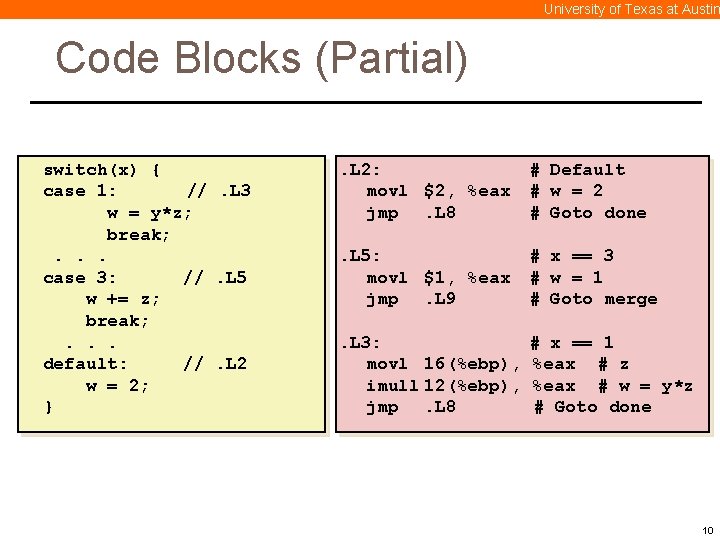
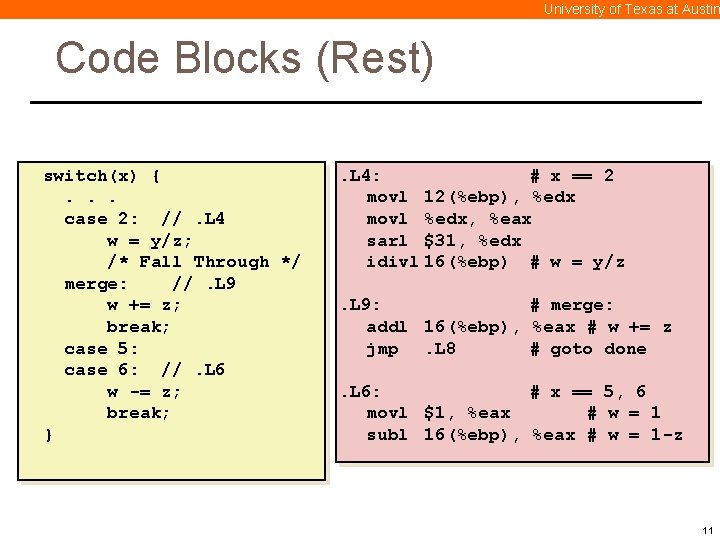
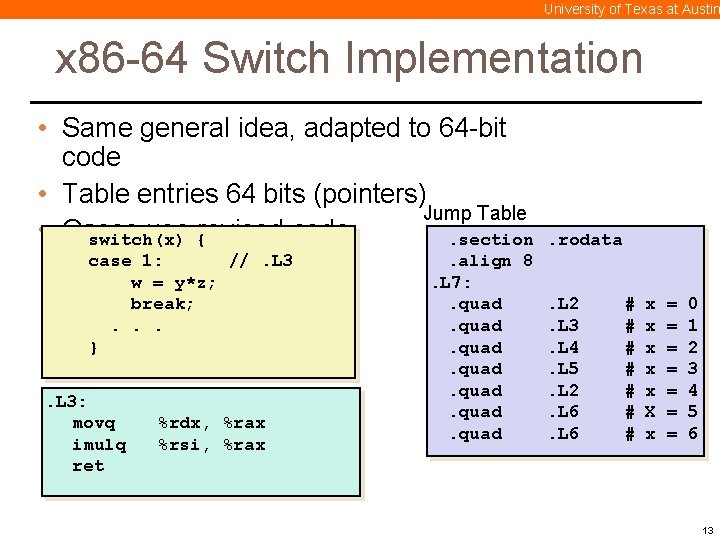
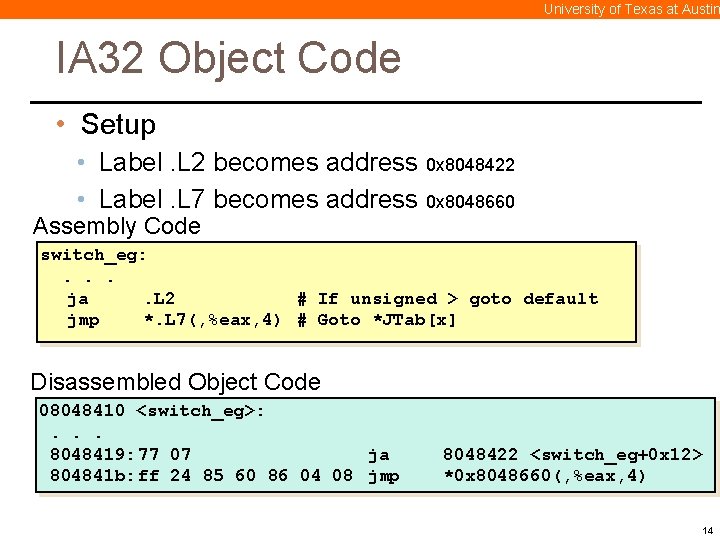
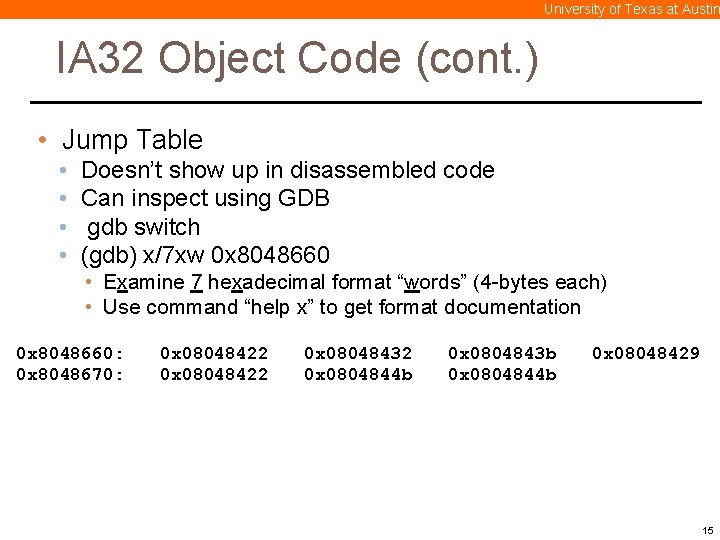
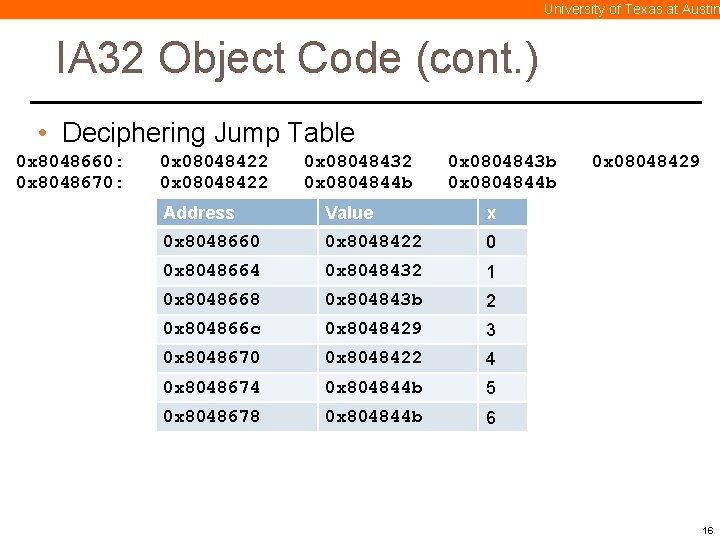
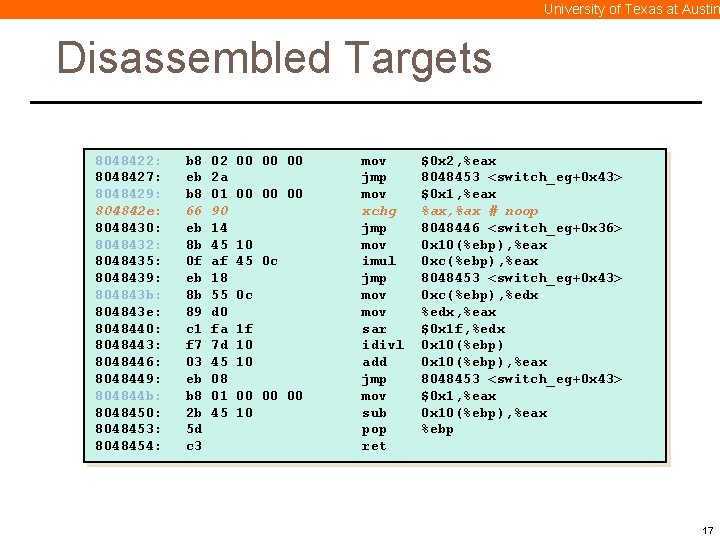
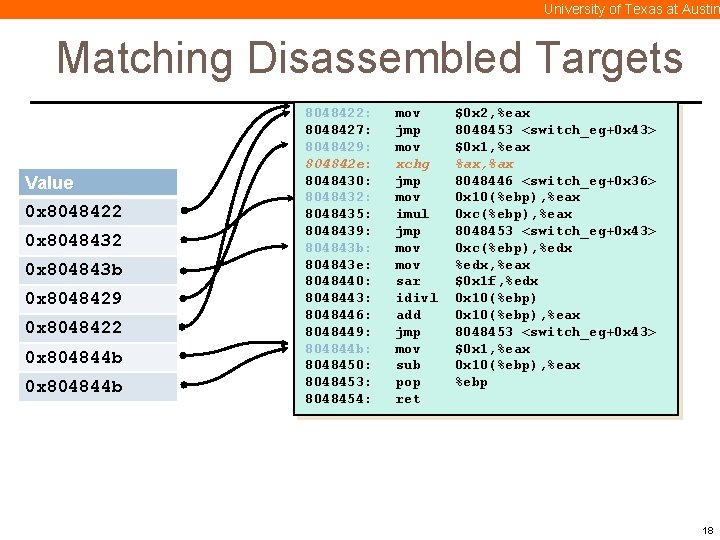
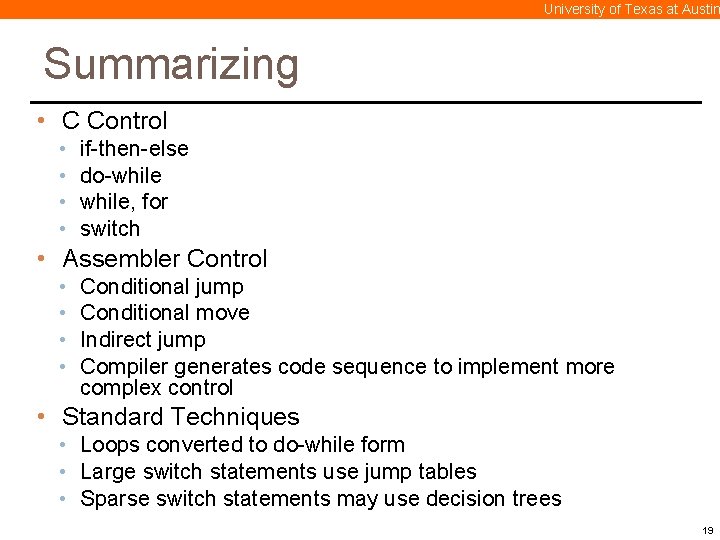
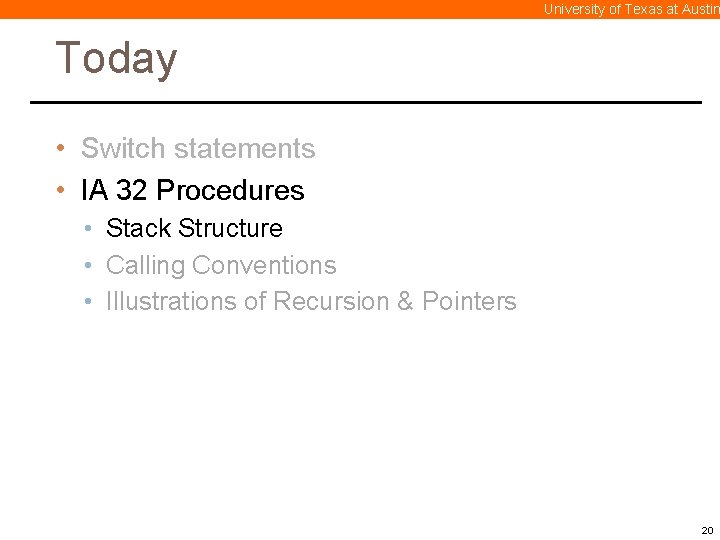
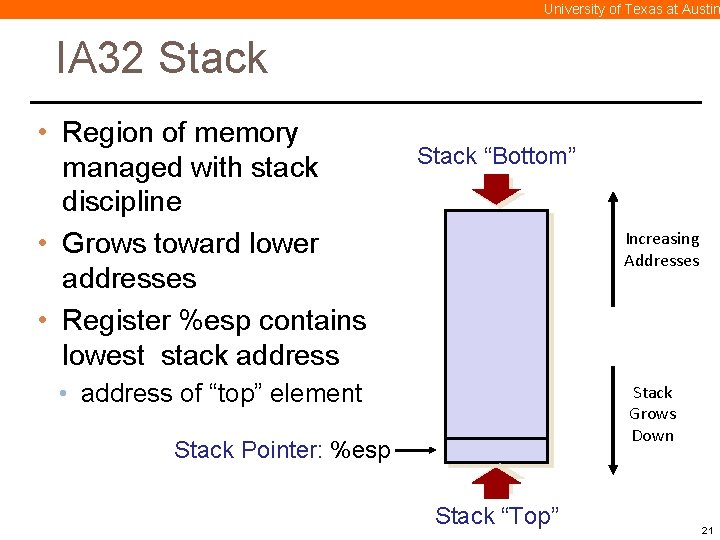
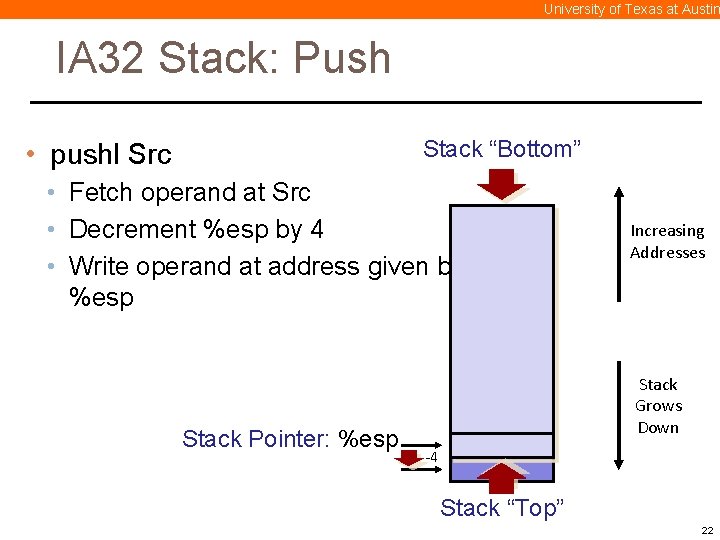
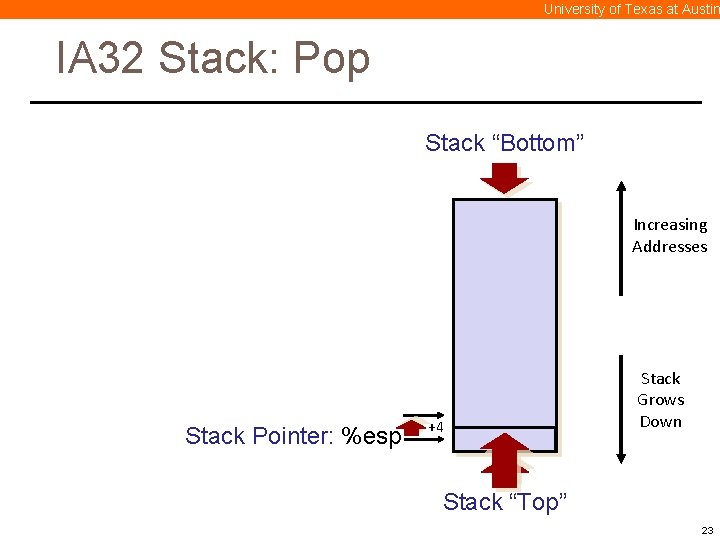
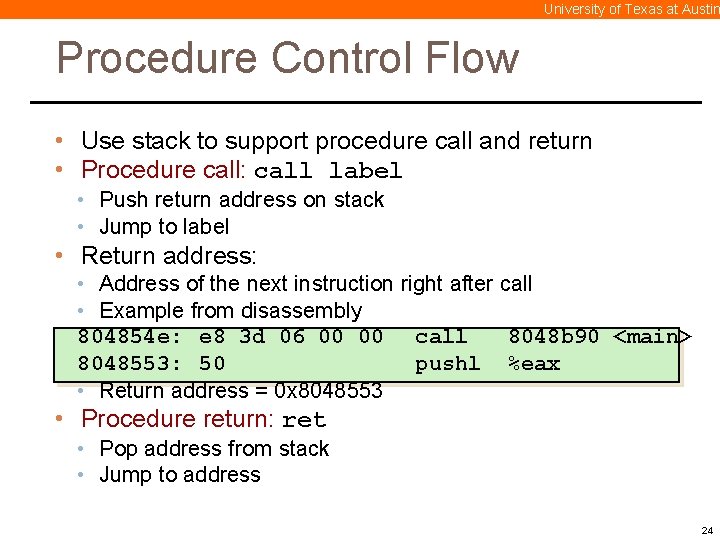
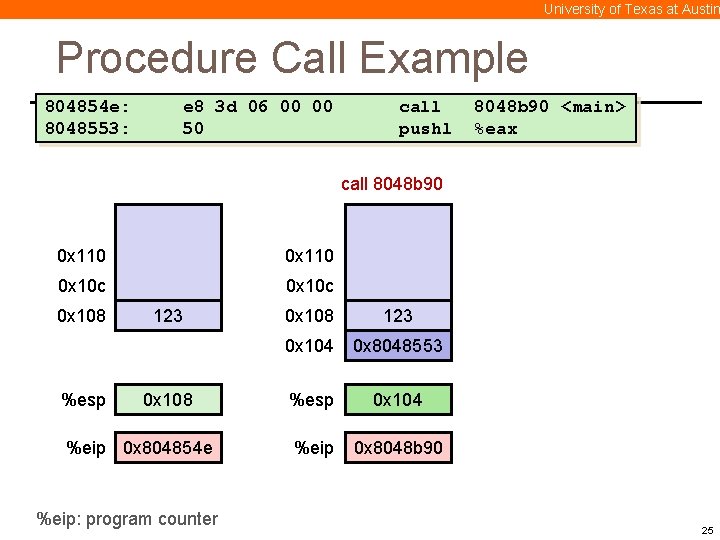
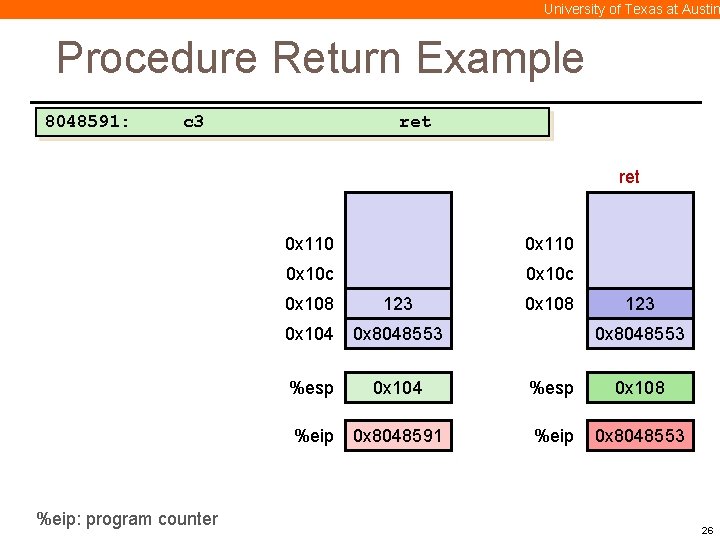
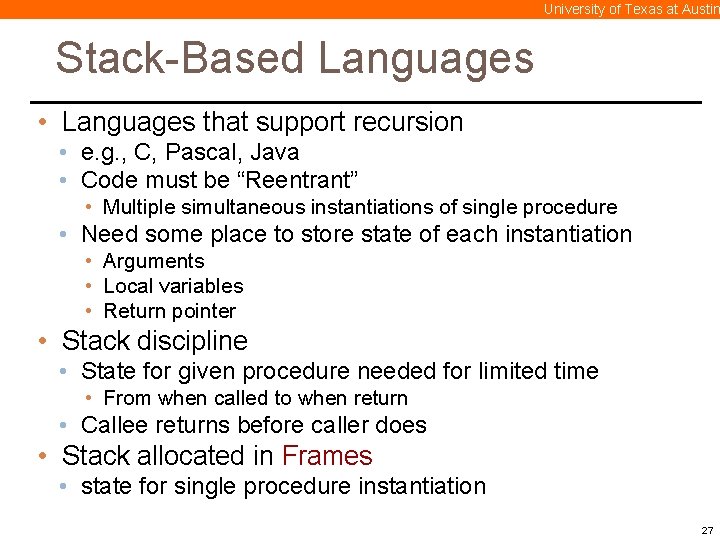
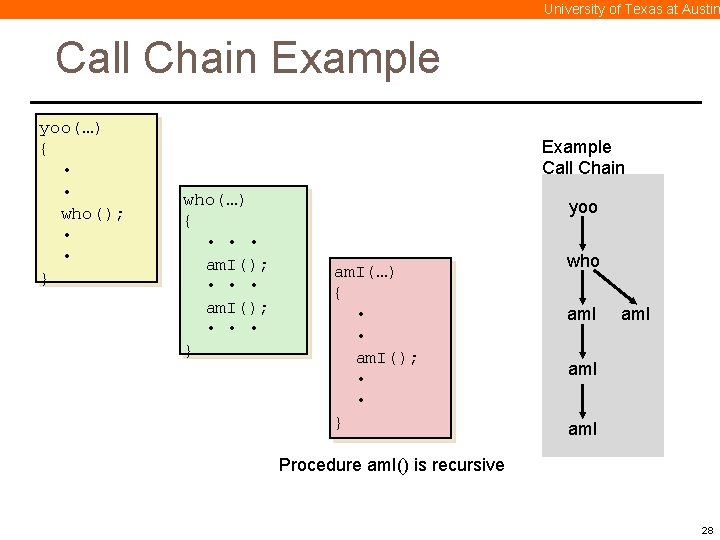
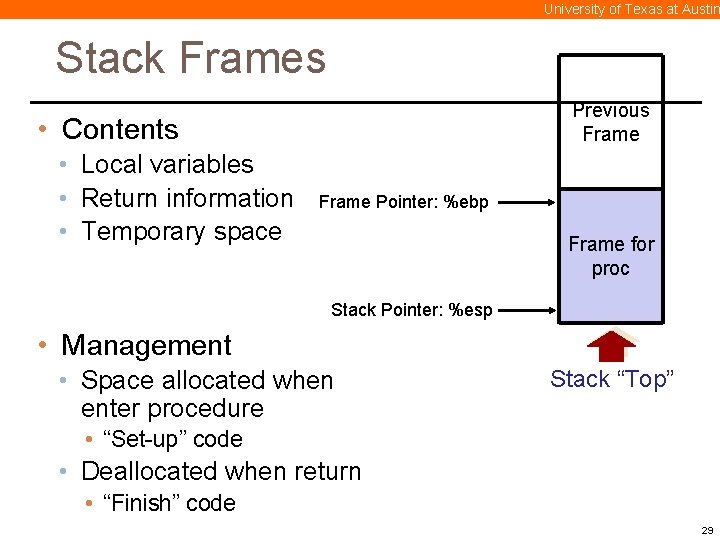
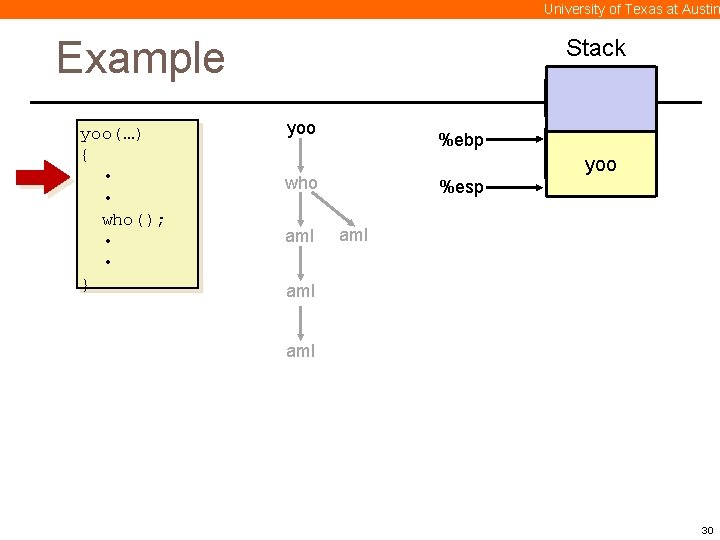
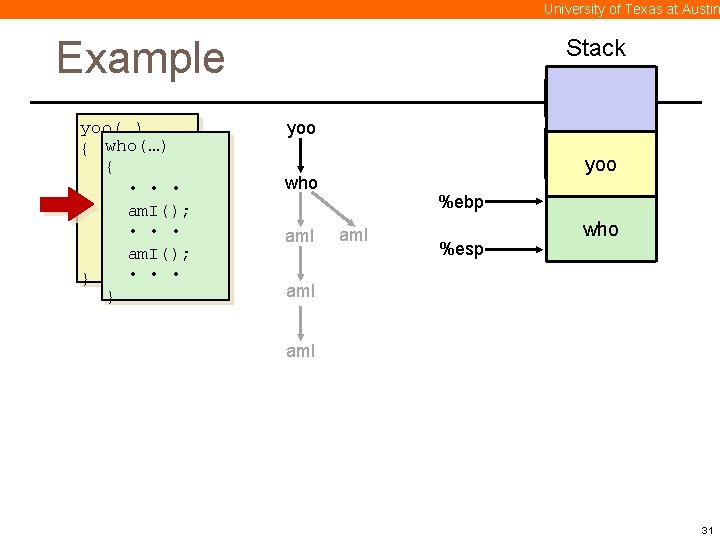
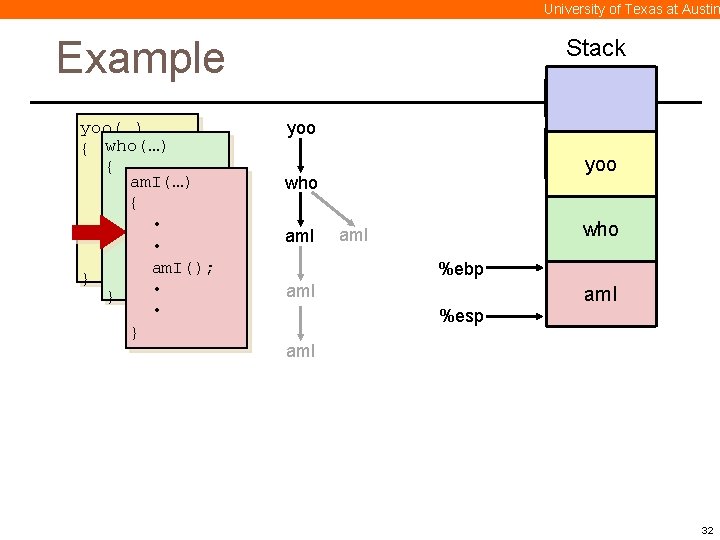
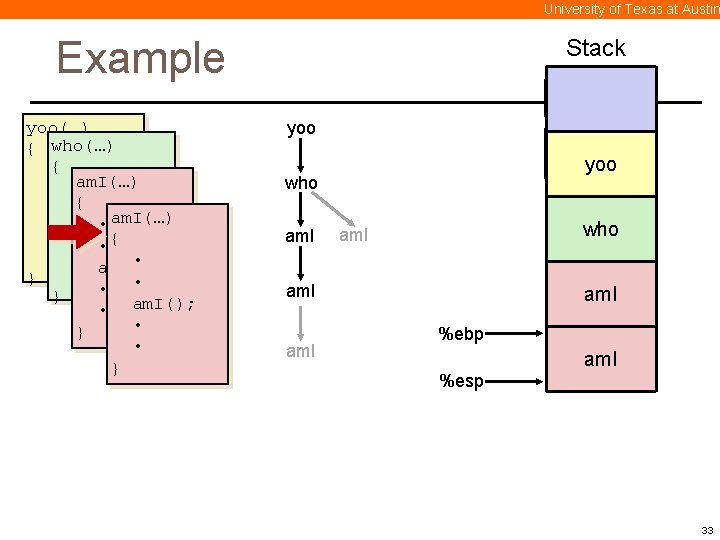
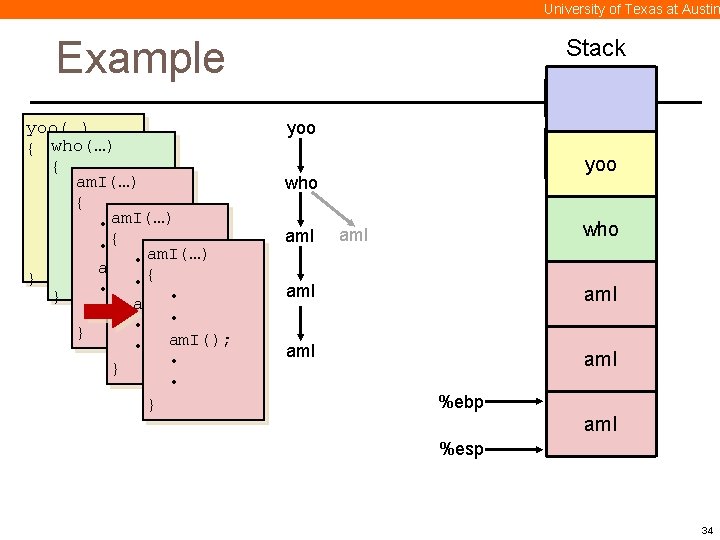
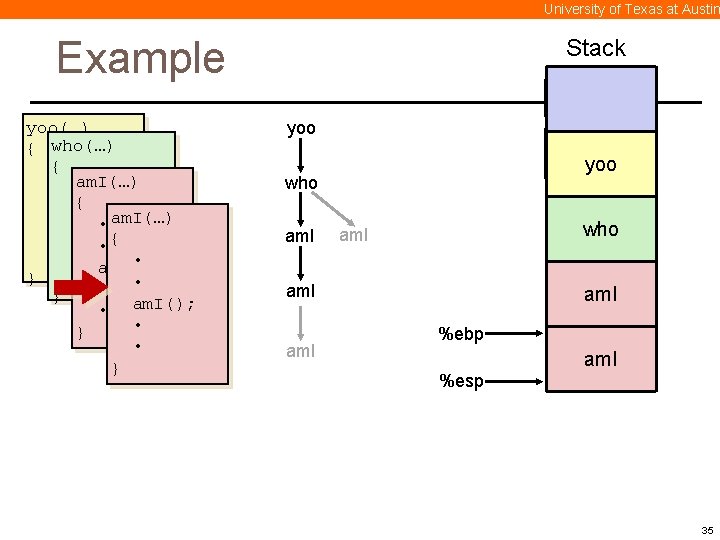
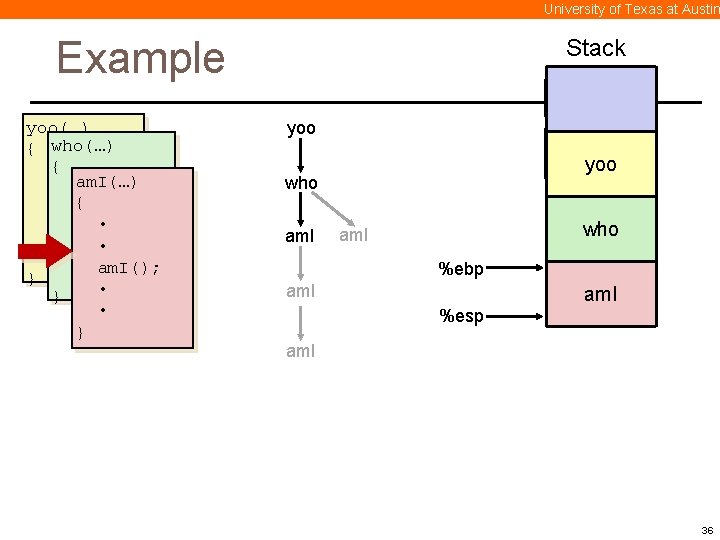
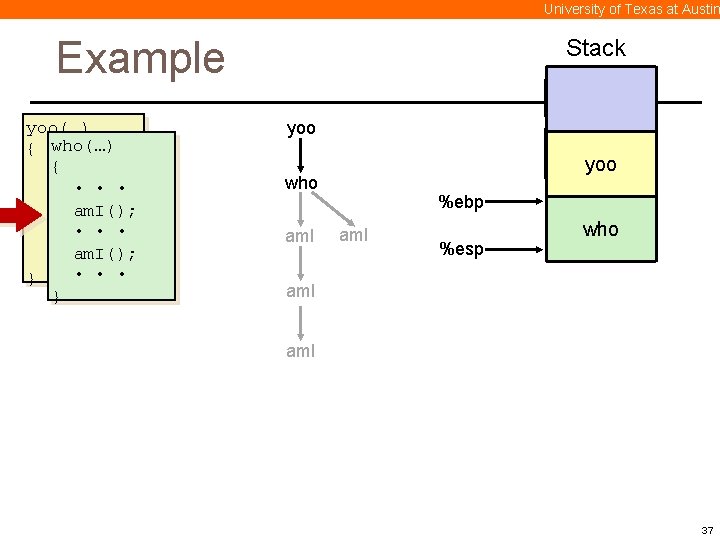
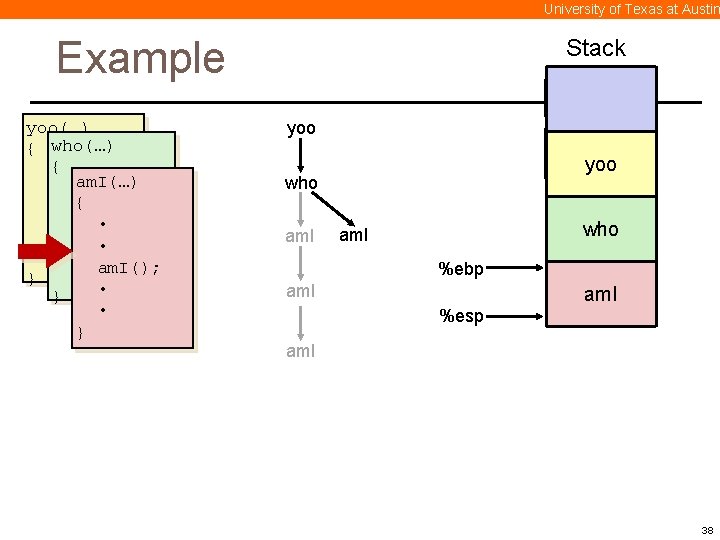
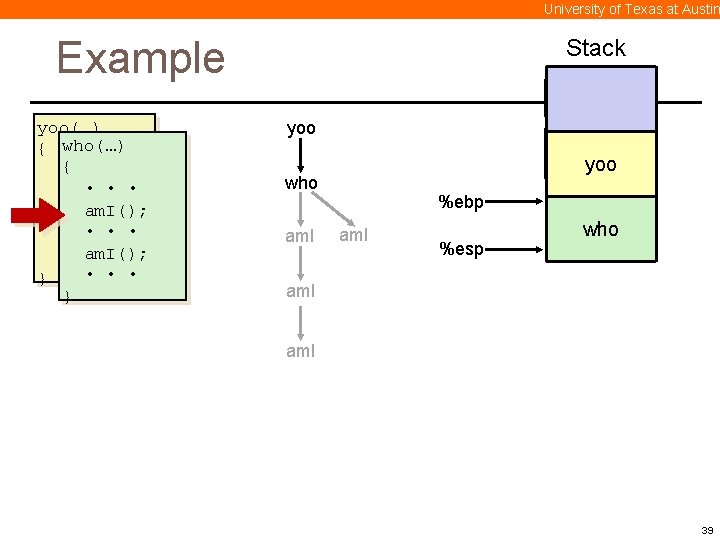
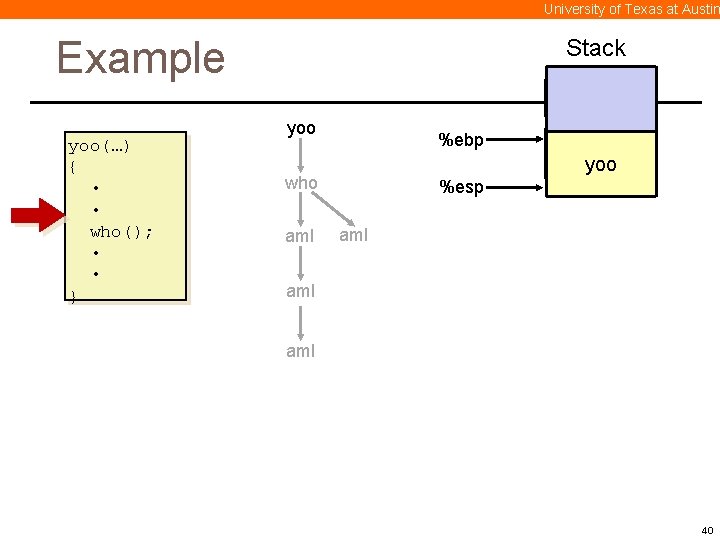
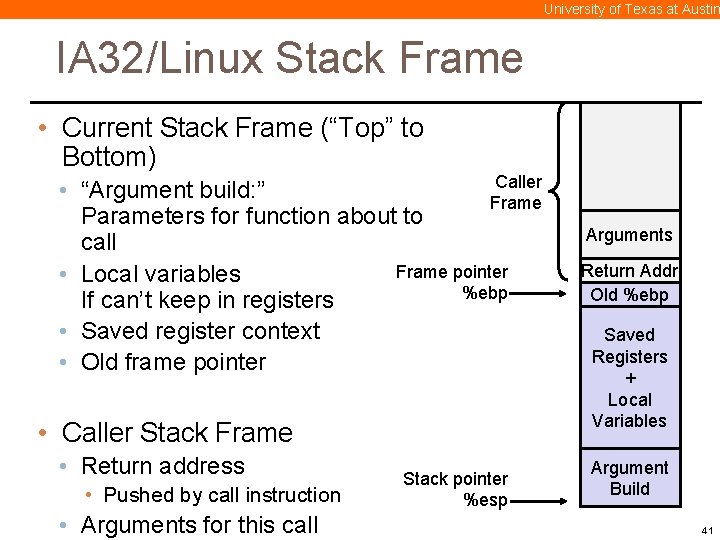
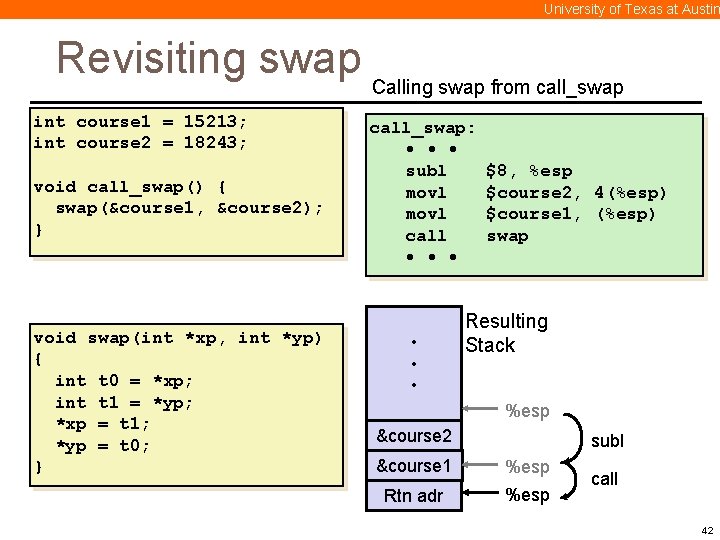
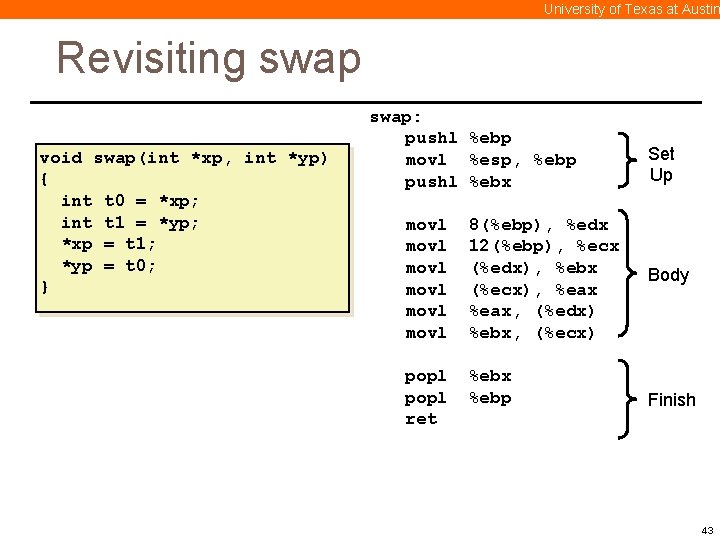
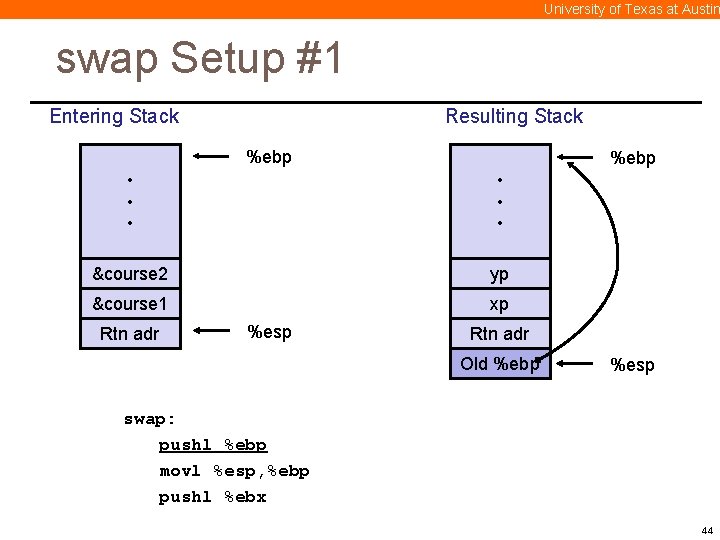
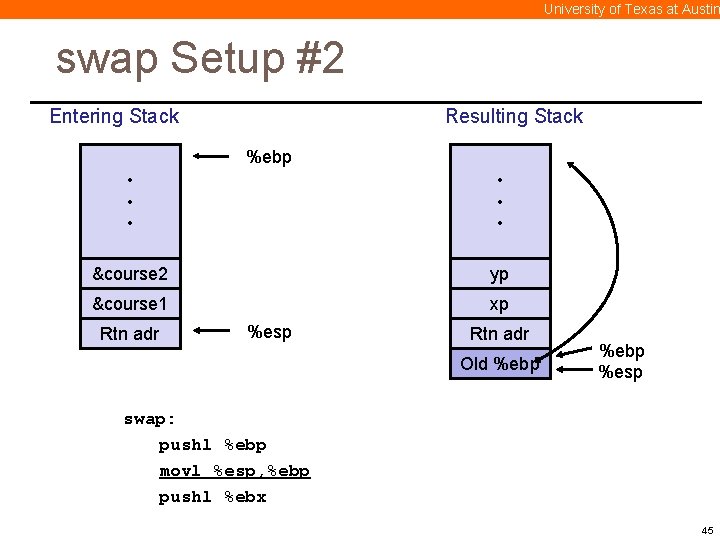
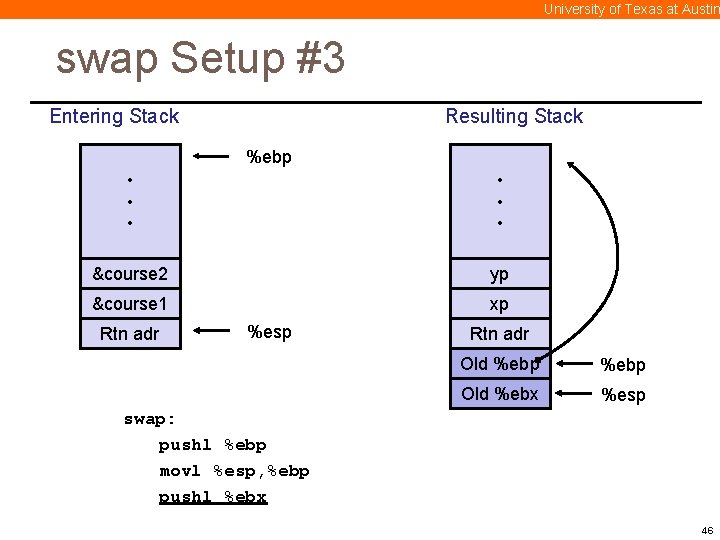
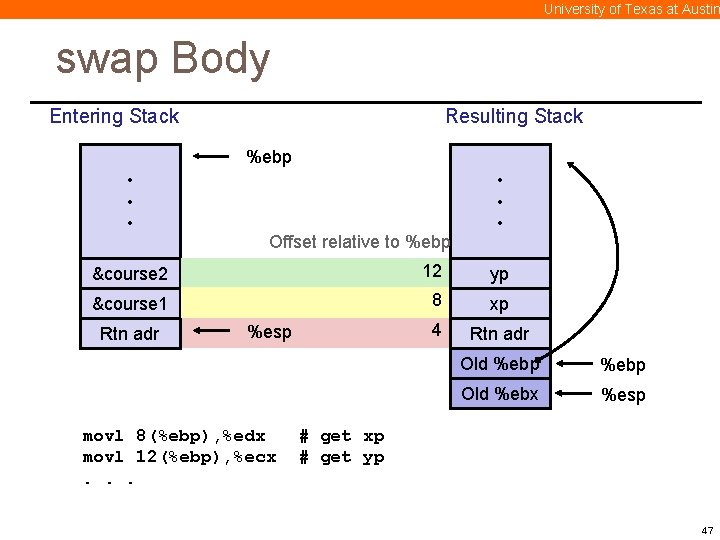
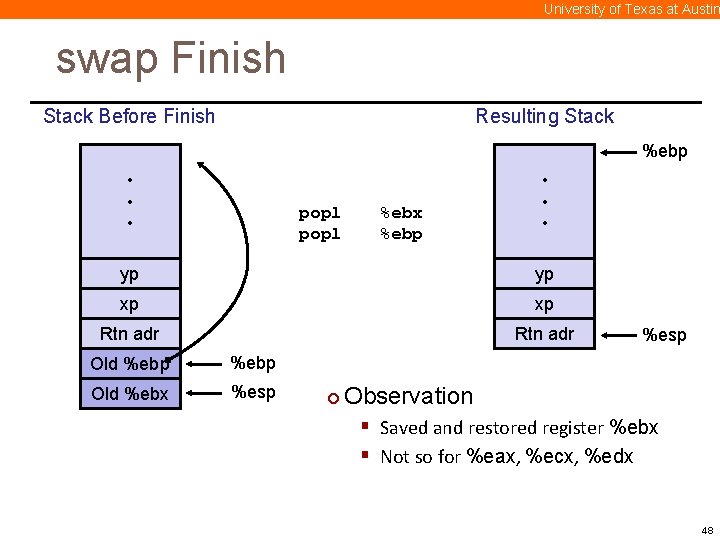
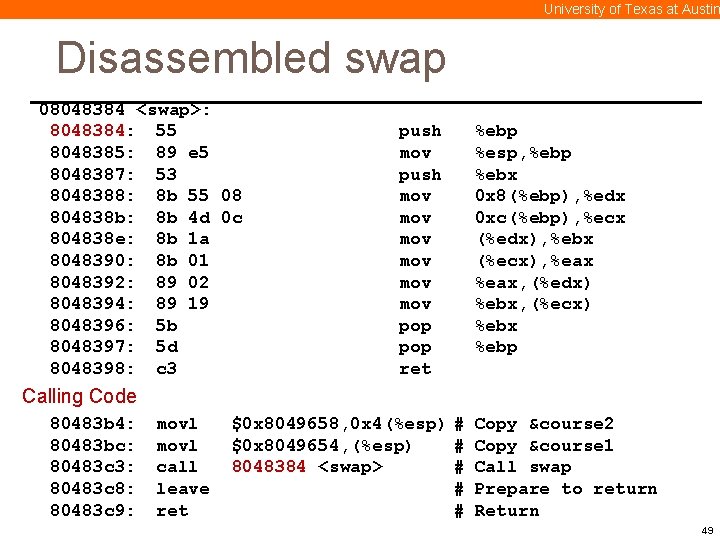
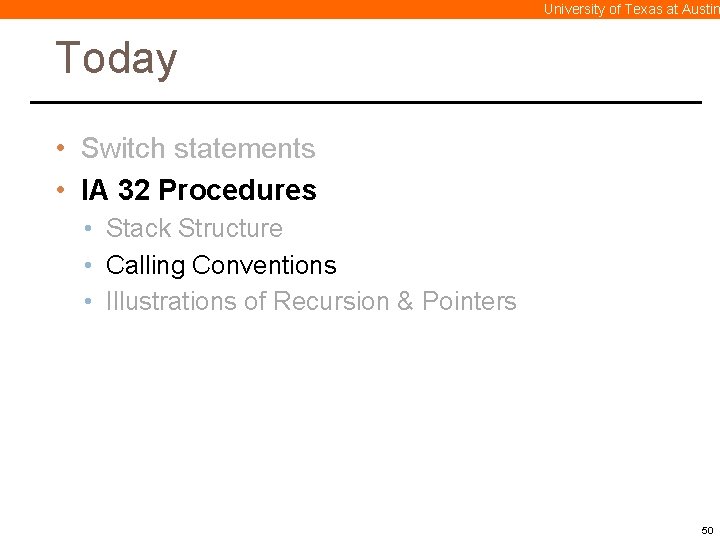
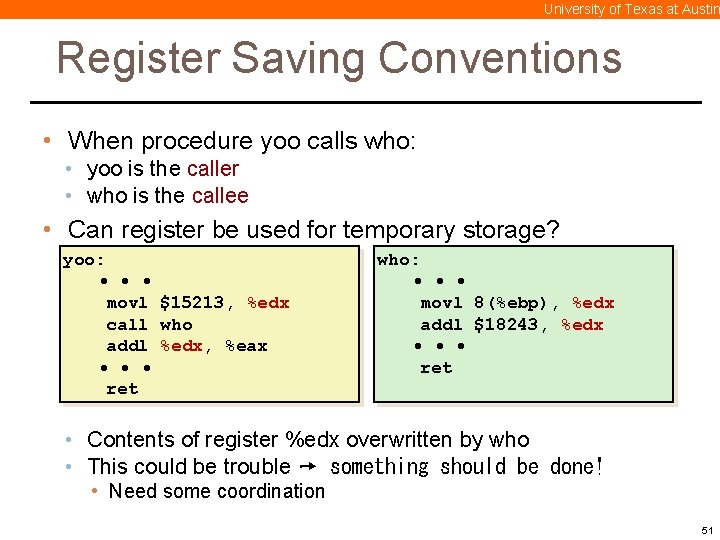
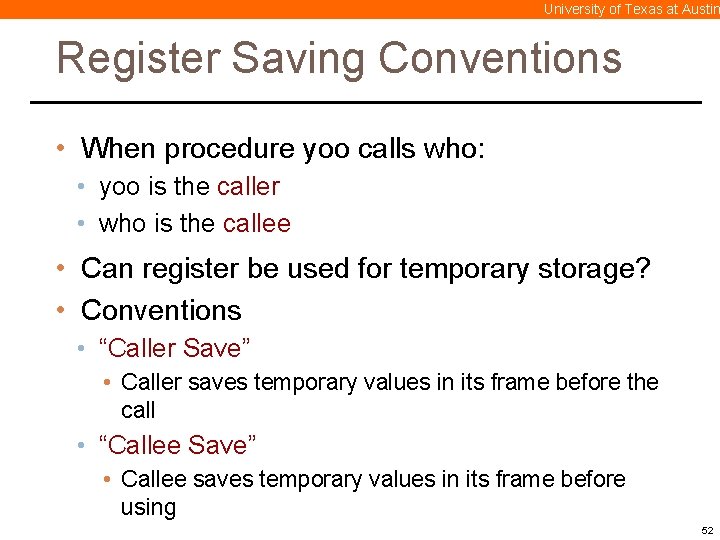
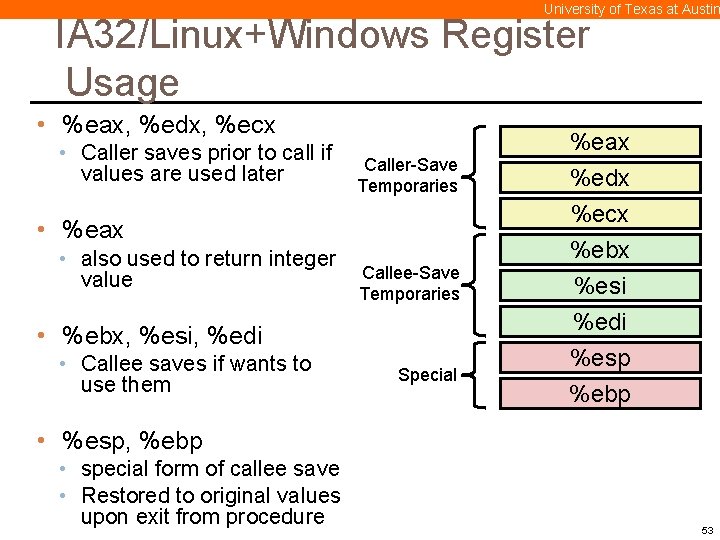
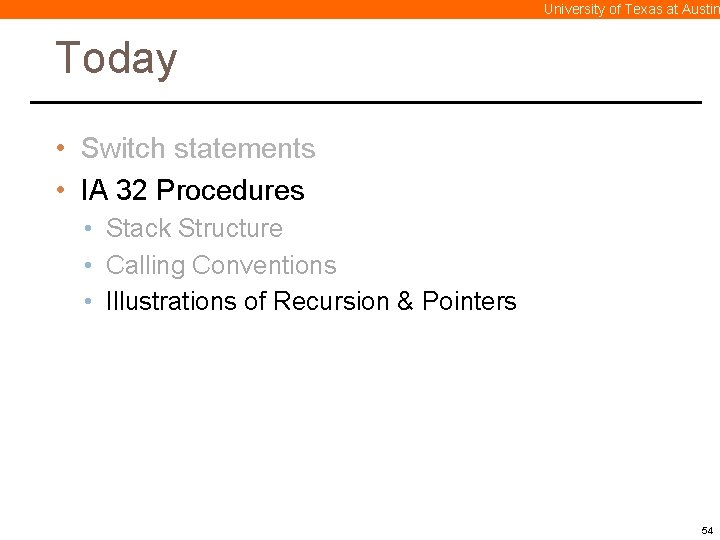
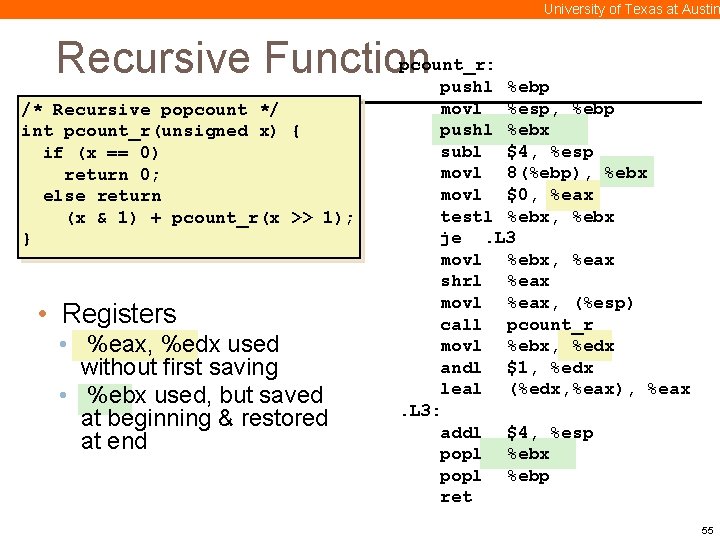
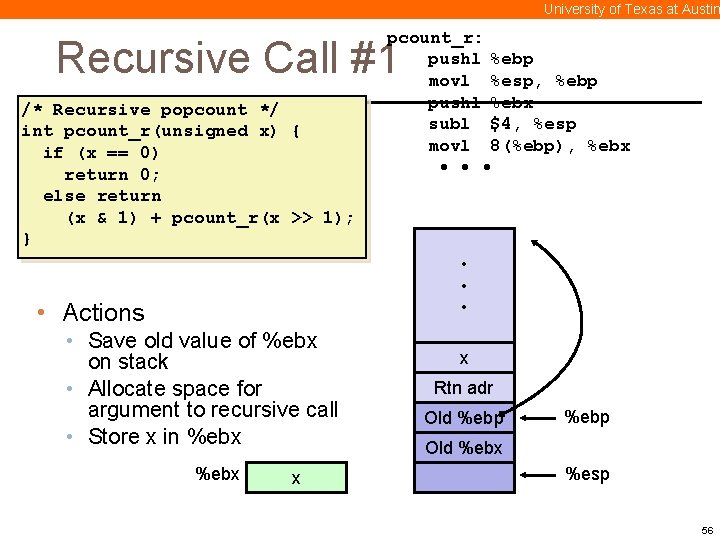
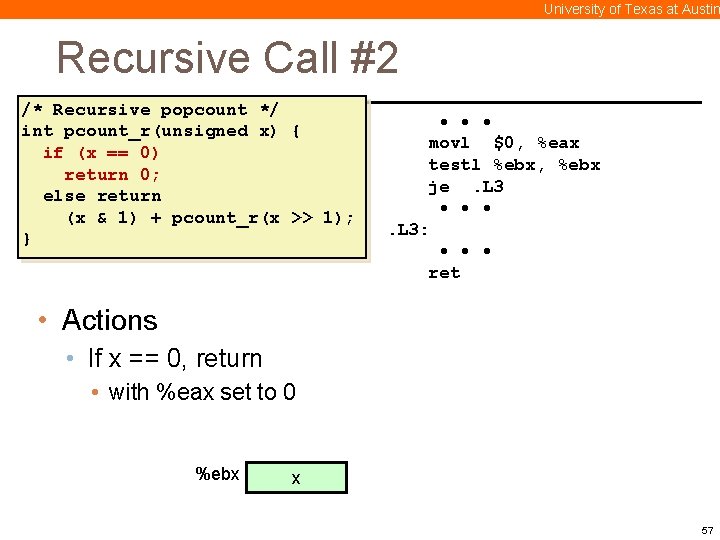
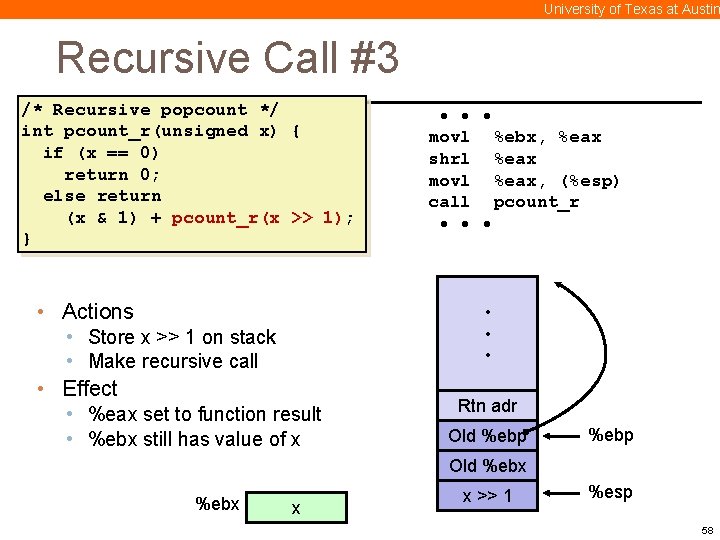
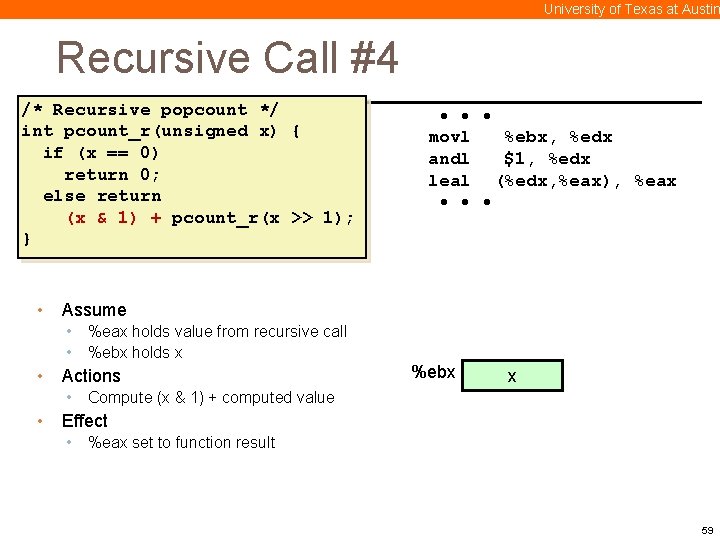
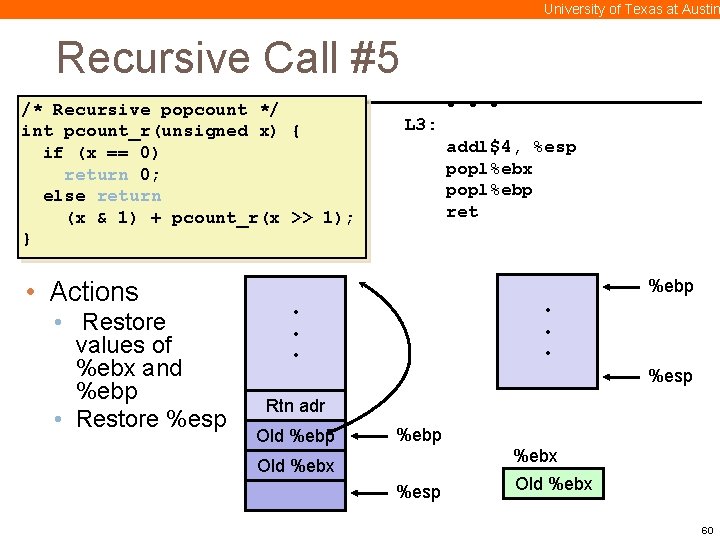
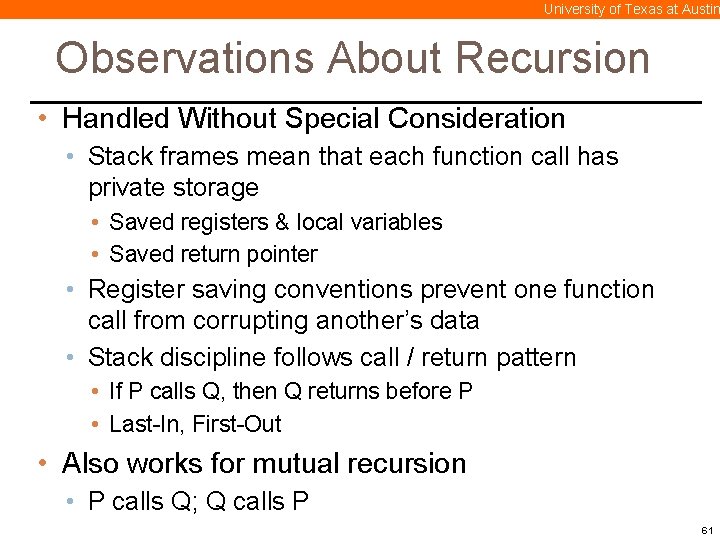
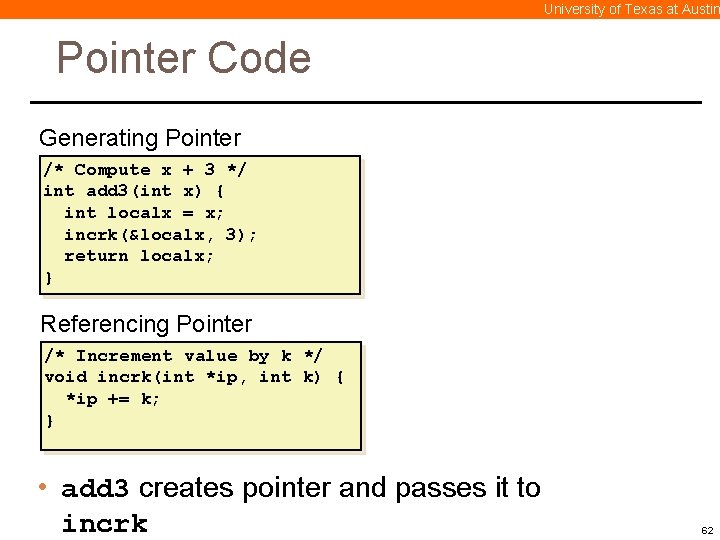
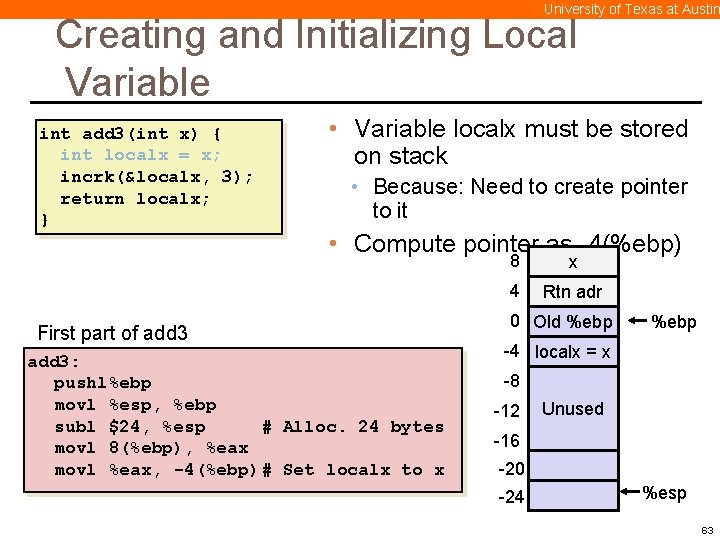
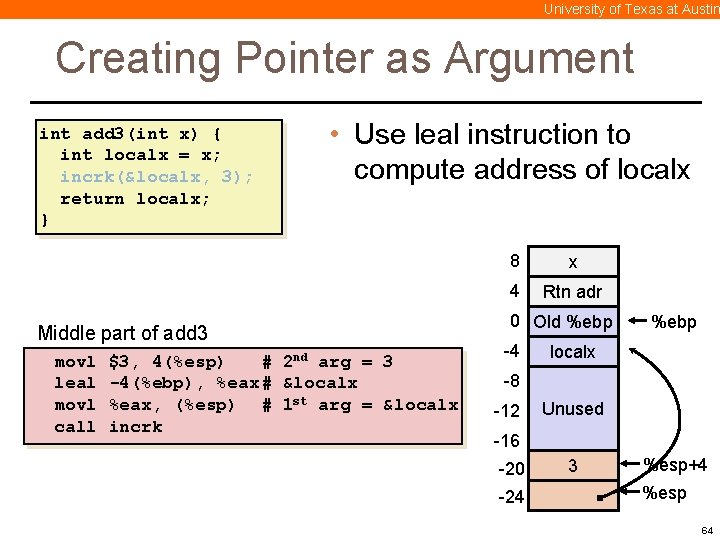
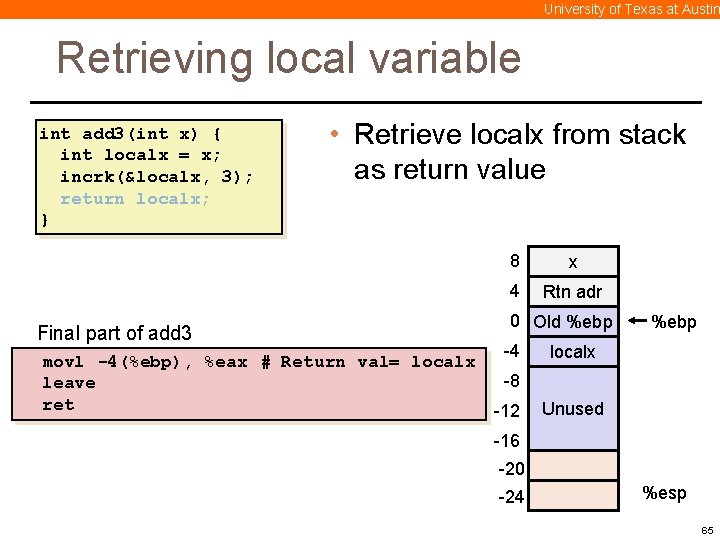
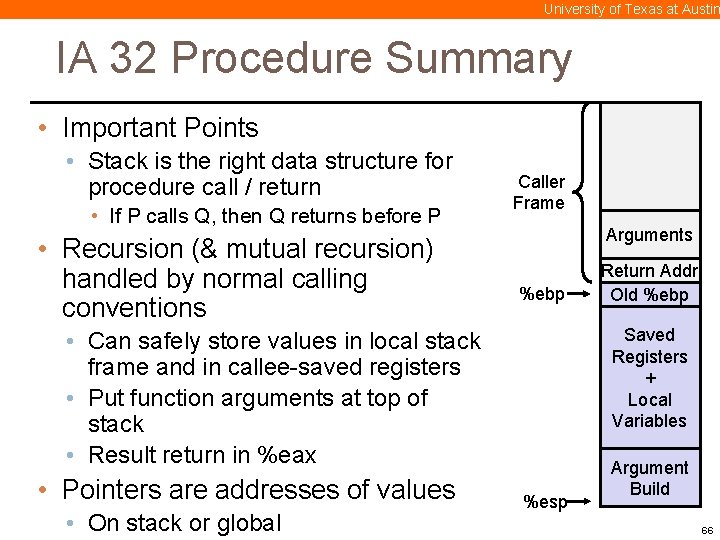
- Slides: 65
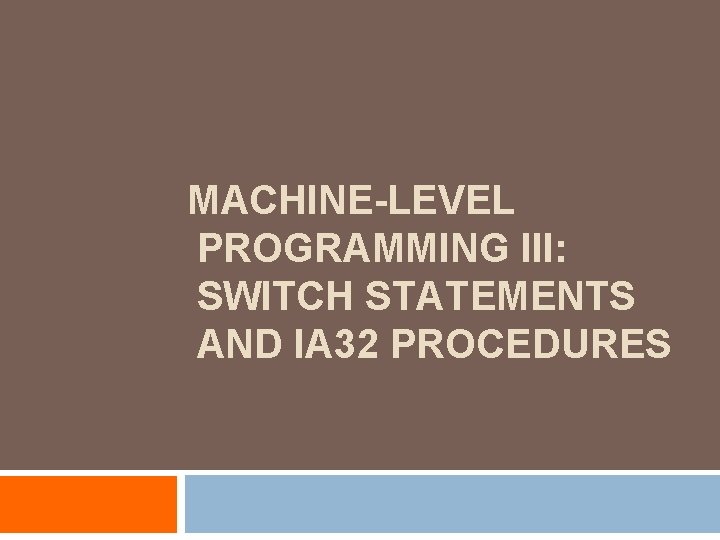
MACHINE-LEVEL PROGRAMMING III: SWITCH STATEMENTS AND IA 32 PROCEDURES
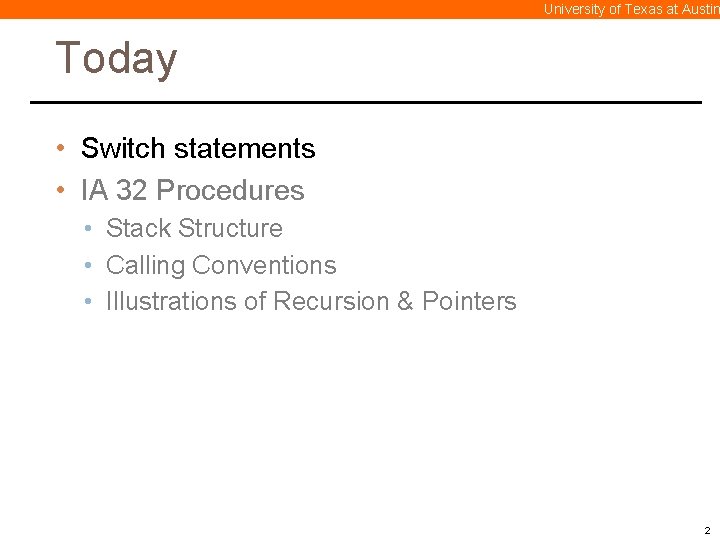
University of Texas at Austin Today • Switch statements • IA 32 Procedures • Stack Structure • Calling Conventions • Illustrations of Recursion & Pointers 2
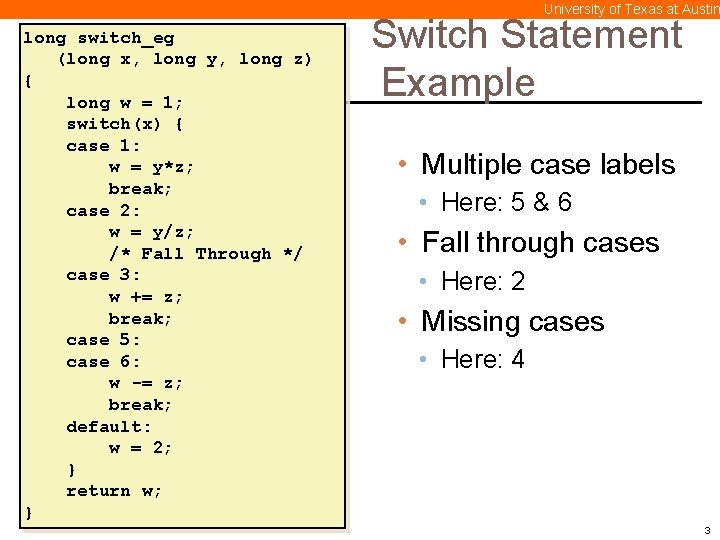
University of Texas at Austin long switch_eg (long x, long y, long z) { long w = 1; switch(x) { case 1: w = y*z; break; case 2: w = y/z; /* Fall Through */ case 3: w += z; break; case 5: case 6: w -= z; break; default: w = 2; } return w; } Switch Statement Example • Multiple case labels • Here: 5 & 6 • Fall through cases • Here: 2 • Missing cases • Here: 4 3
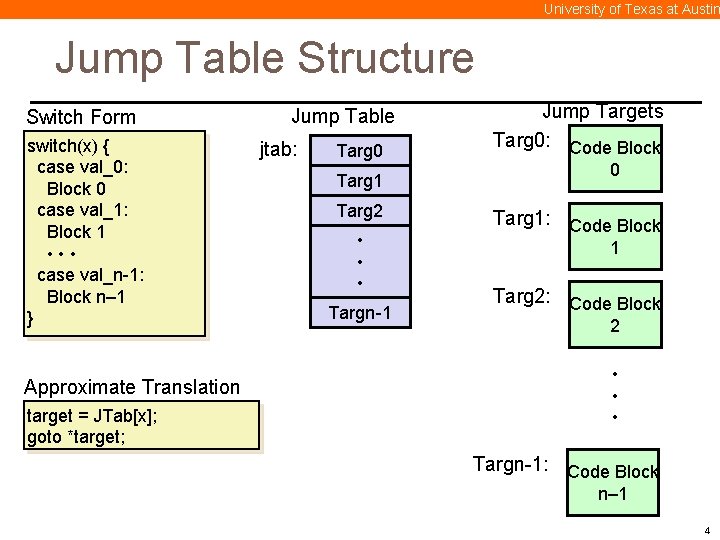
University of Texas at Austin Jump Table Structure Switch Form switch(x) { case val_0: Block 0 case val_1: Block 1 • • • case val_n-1: Block n– 1 } Approximate Translation target = JTab[x]; goto *target; Jump Table jtab: Targ 0 Targ 1 Targ 2 • • • Targn-1 Jump Targets Targ 0: Code Block 0 Targ 1: Code Block 1 Targ 2: Code Block 2 • • • Targn-1: Code Block n– 1 4
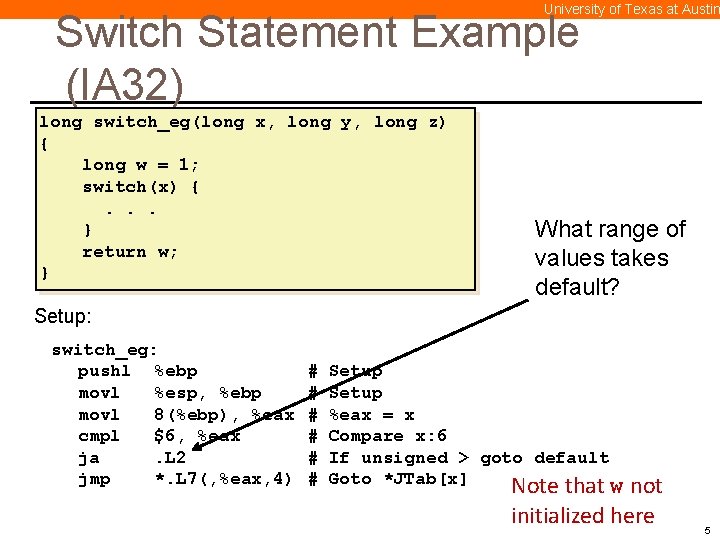
University of Texas at Austin Switch Statement Example (IA 32) long switch_eg(long x, long y, long z) { long w = 1; switch(x) {. . . } return w; } What range of values takes default? Setup: switch_eg: pushl %ebp movl %esp, %ebp movl 8(%ebp), %eax cmpl $6, %eax ja. L 2 jmp *. L 7(, %eax, 4) # # # Setup %eax = x Compare x: 6 If unsigned > goto default Goto *JTab[x] Note that w not initialized here 5
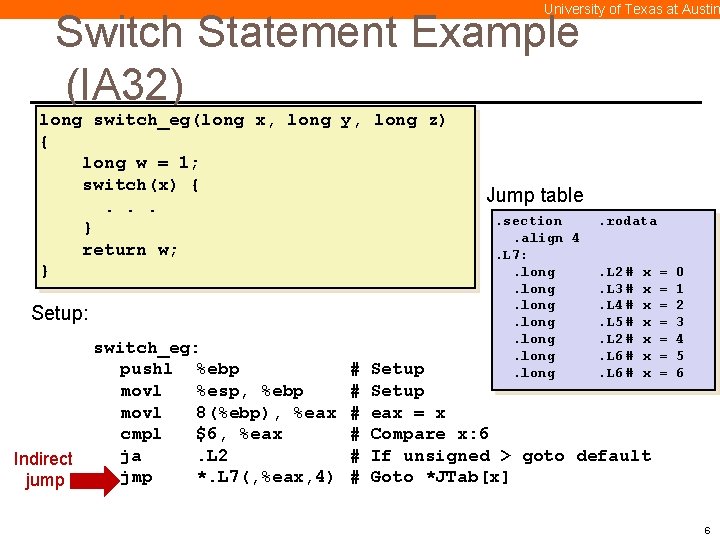
University of Texas at Austin Switch Statement Example (IA 32) long switch_eg(long x, long y, long z) { long w = 1; switch(x) {. . . } return w; } Setup: Indirect jump switch_eg: pushl %ebp movl %esp, %ebp movl 8(%ebp), %eax cmpl $6, %eax ja. L 2 jmp *. L 7(, %eax, 4) # # # Jump table. section. align 4. L 7: . long . rodata. L 2 #. L 3 #. L 4 #. L 5 #. L 2 #. L 6 # x x x x Setup eax = x Compare x: 6 If unsigned > goto default Goto *JTab[x] = = = = 0 1 2 3 4 5 6 6
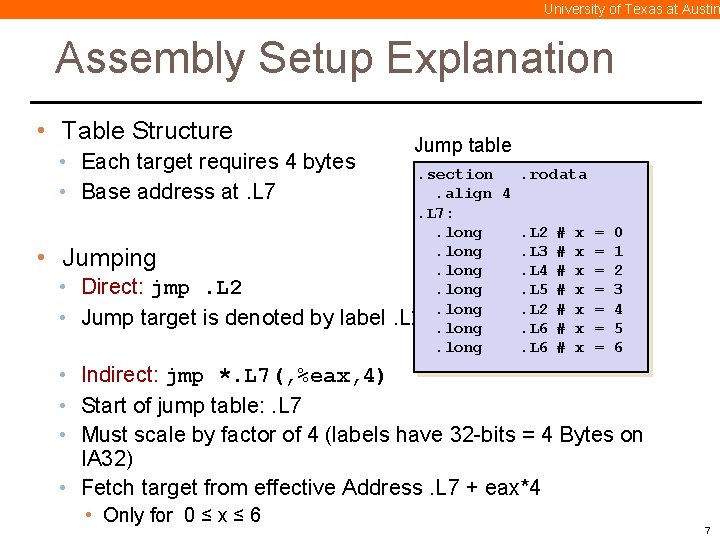
University of Texas at Austin Assembly Setup Explanation • Table Structure • Each target requires 4 bytes • Base address at. L 7 • Jumping • Direct: jmp. L 2 • Jump target is denoted by label Jump table. section. rodata. align 4. L 7: . long. L 2 # x = 0. long. L 3 # x = 1. long. L 4 # x = 2. long. L 5 # x = 3. long. L 2 # x = 4. L 2. long. L 6 # x = 5. long. L 6 # x = 6 • Indirect: jmp *. L 7(, %eax, 4) • Start of jump table: . L 7 • Must scale by factor of 4 (labels have 32 -bits = 4 Bytes on IA 32) • Fetch target from effective Address. L 7 + eax*4 • Only for 0 ≤ x ≤ 6 7
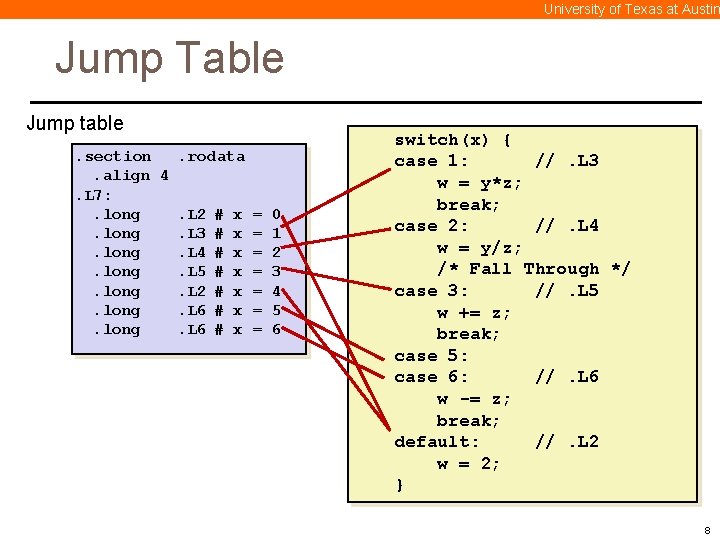
University of Texas at Austin Jump Table Jump table. section. rodata. align 4. L 7: . long. L 2 # x = 0. long. L 3 # x = 1. long. L 4 # x = 2. long. L 5 # x = 3. long. L 2 # x = 4. long. L 6 # x = 5. long. L 6 # x = 6 switch(x) { case 1: //. L 3 w = y*z; break; case 2: //. L 4 w = y/z; /* Fall Through */ case 3: //. L 5 w += z; break; case 5: case 6: //. L 6 w -= z; break; default: //. L 2 w = 2; } 8
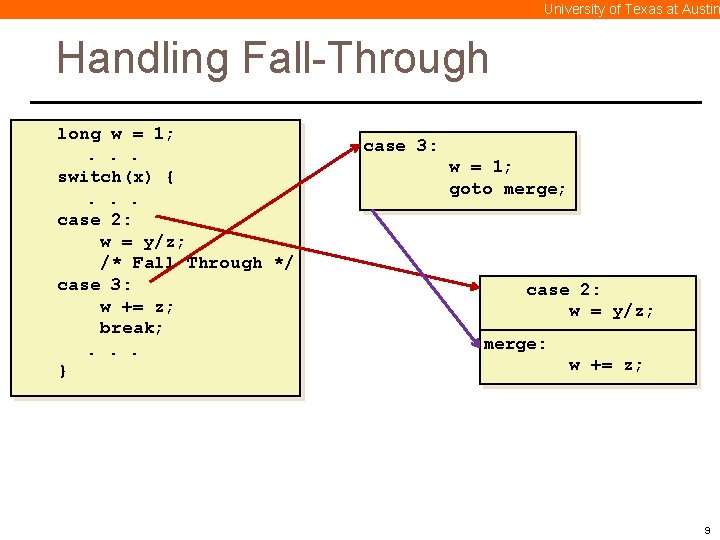
University of Texas at Austin Handling Fall-Through long w = 1; . . . switch(x) {. . . case 2: w = y/z; /* Fall Through */ case 3: w += z; break; . . . } case 3: w = 1; goto merge; case 2: w = y/z; merge: w += z; 9
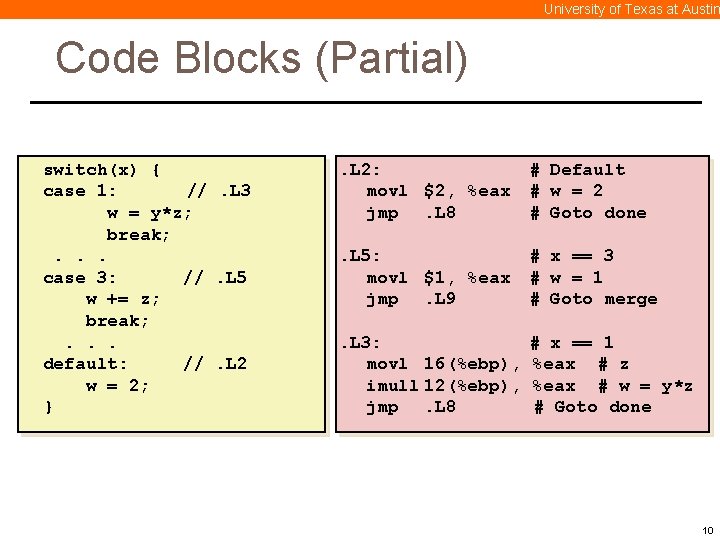
University of Texas at Austin Code Blocks (Partial) switch(x) { case 1: //. L 3 w = y*z; break; . . . case 3: //. L 5 w += z; break; . . . default: //. L 2 w = 2; } . L 2: # Default movl $2, %eax # w = 2 jmp. L 8 # Goto done. L 5: # x == 3 movl $1, %eax # w = 1 jmp. L 9 # Goto merge. L 3: movl 16(%ebp), imull 12(%ebp), jmp. L 8 # x == 1 %eax # z %eax # w = y*z # Goto done 10
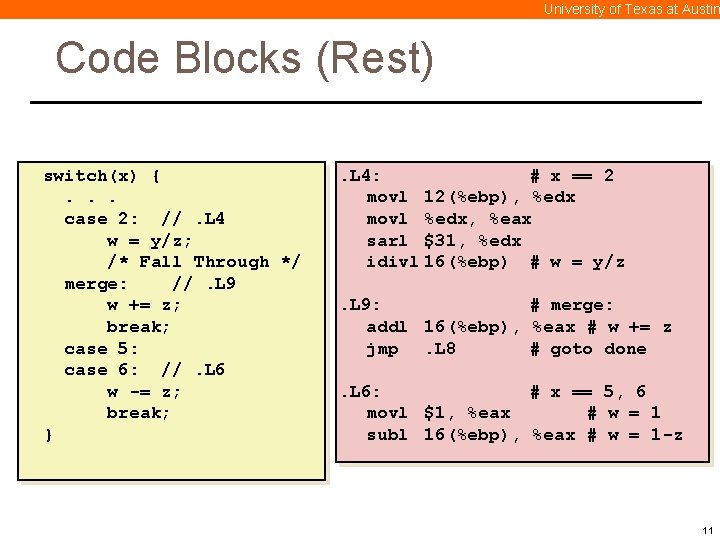
University of Texas at Austin Code Blocks (Rest) switch(x) {. . . case 2: //. L 4 w = y/z; /* Fall Through */ merge: //. L 9 w += z; break; case 5: case 6: //. L 6 w -= z; break; } . L 4: # x == 2 movl 12(%ebp), %edx movl %edx, %eax sarl $31, %edx idivl 16(%ebp) # w = y/z. L 9: # merge: addl 16(%ebp), %eax # w += z jmp. L 8 # goto done. L 6: # x == 5, 6 movl $1, %eax # w = 1 subl 16(%ebp), %eax # w = 1 -z 11
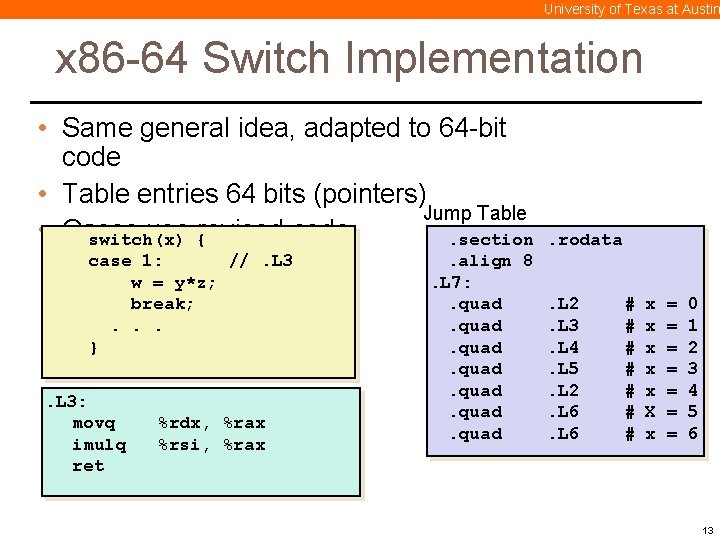
University of Texas at Austin x 86 -64 Switch Implementation • Same general idea, adapted to 64 -bit code • Table entries 64 bits (pointers) Jump Table • Cases use {revised code. section switch(x) case 1: //. L 3 w = y*z; break; . . . }. L 3: movq imulq ret %rdx, %rax %rsi, %rax . rodata . align 8. L 7: . quad. L 2. quad. L 3. quad. L 4. quad. L 5. quad. L 2. quad. L 6 # # # # x x x X x = = = = 0 1 2 3 4 5 6 13
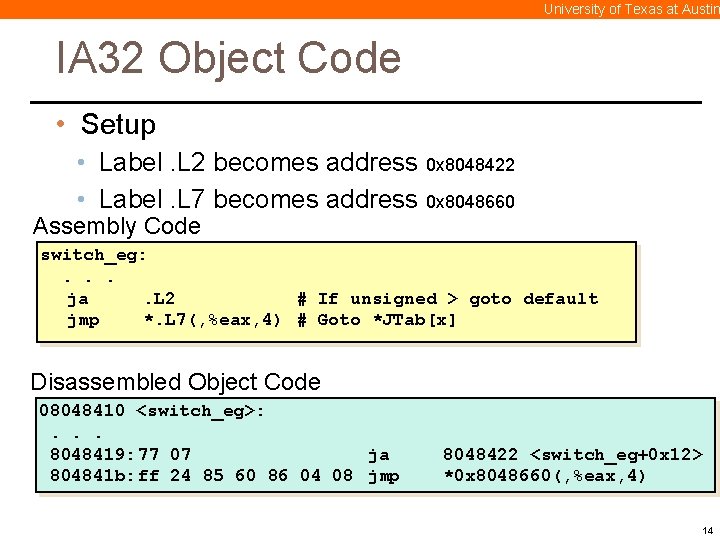
University of Texas at Austin IA 32 Object Code • Setup • Label. L 2 becomes address 0 x 8048422 • Label. L 7 becomes address 0 x 8048660 Assembly Code switch_eg: . . . ja. L 2 # If unsigned > goto default jmp *. L 7(, %eax, 4) # Goto *JTab[x] Disassembled Object Code 08048410 <switch_eg>: . . . 8048419: 77 07 ja 804841 b: ff 24 85 60 86 04 08 jmp 8048422 <switch_eg+0 x 12> *0 x 8048660(, %eax, 4) 14
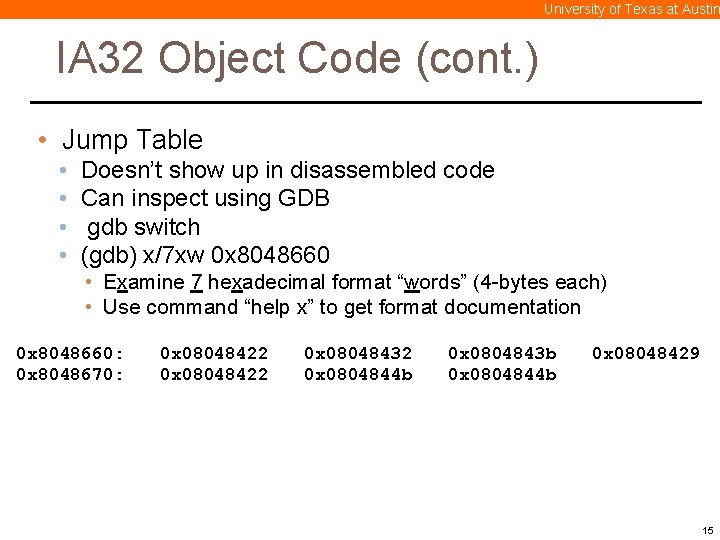
University of Texas at Austin IA 32 Object Code (cont. ) • Jump Table • • Doesn’t show up in disassembled code Can inspect using GDB gdb switch (gdb) x/7 xw 0 x 8048660 • Examine 7 hexadecimal format “words” (4 -bytes each) • Use command “help x” to get format documentation 0 x 8048660: 0 x 8048670: 0 x 08048422 0 x 08048432 0 x 0804844 b 0 x 0804843 b 0 x 0804844 b 0 x 08048429 15
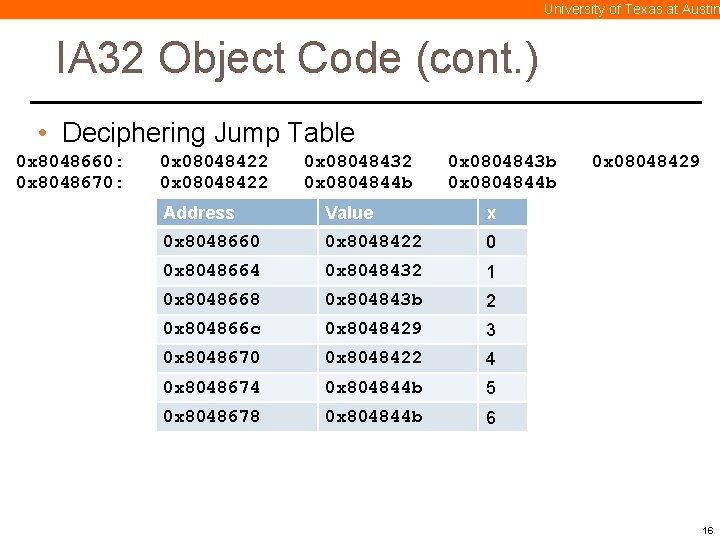
University of Texas at Austin IA 32 Object Code (cont. ) • Deciphering Jump Table 0 x 8048660: 0 x 8048670: 0 x 08048422 0 x 08048432 0 x 0804844 b 0 x 0804843 b 0 x 0804844 b Address Value x 0 x 8048660 0 x 8048422 0 0 x 8048664 0 x 8048432 1 0 x 8048668 0 x 804843 b 2 0 x 804866 c 0 x 8048429 3 0 x 8048670 0 x 8048422 4 0 x 8048674 0 x 804844 b 5 0 x 8048678 0 x 804844 b 6 0 x 08048429 16
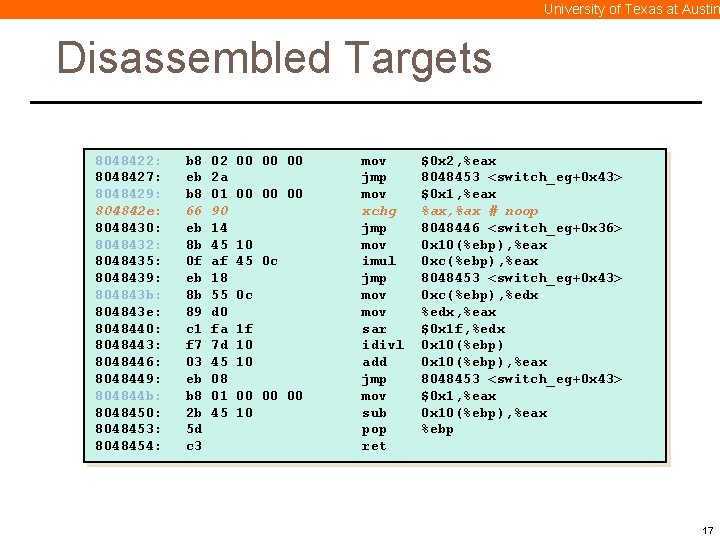
University of Texas at Austin Disassembled Targets 8048422: 8048427: 8048429: 804842 e: 8048430: 8048432: 8048435: 8048439: 804843 b: 804843 e: 8048440: 8048443: 8048446: 8048449: 804844 b: 8048450: 8048453: 8048454: b 8 eb b 8 66 eb 8 b 0 f eb 8 b 89 c 1 f 7 03 eb b 8 2 b 5 d c 3 02 2 a 01 90 14 45 af 18 55 d 0 fa 7 d 45 08 01 45 00 00 00 10 45 0 c 0 c 1 f 10 10 00 00 00 10 mov jmp mov xchg jmp mov imul jmp mov sar idivl add jmp mov sub pop ret $0 x 2, %eax 8048453 <switch_eg+0 x 43> $0 x 1, %eax %ax, %ax # noop 8048446 <switch_eg+0 x 36> 0 x 10(%ebp), %eax 0 xc(%ebp), %eax 8048453 <switch_eg+0 x 43> 0 xc(%ebp), %edx, %eax $0 x 1 f, %edx 0 x 10(%ebp), %eax 8048453 <switch_eg+0 x 43> $0 x 1, %eax 0 x 10(%ebp), %eax %ebp 17
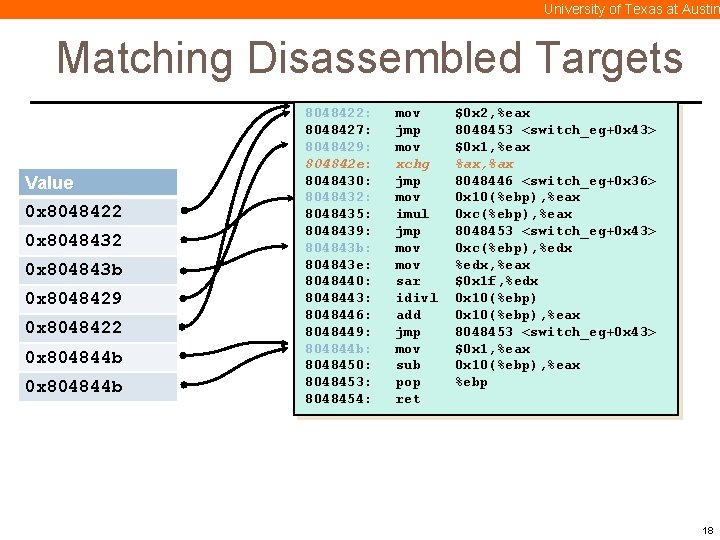
University of Texas at Austin Matching Disassembled Targets Value 0 x 8048422 0 x 804843 b 0 x 8048429 0 x 8048422 0 x 804844 b 8048422: 8048427: 8048429: 804842 e: 8048430: 8048432: 8048435: 8048439: 804843 b: 804843 e: 8048440: 8048443: 8048446: 8048449: 804844 b: 8048450: 8048453: 8048454: mov jmp mov xchg jmp mov imul jmp mov sar idivl add jmp mov sub pop ret $0 x 2, %eax 8048453 <switch_eg+0 x 43> $0 x 1, %eax %ax, %ax 8048446 <switch_eg+0 x 36> 0 x 10(%ebp), %eax 0 xc(%ebp), %eax 8048453 <switch_eg+0 x 43> 0 xc(%ebp), %edx, %eax $0 x 1 f, %edx 0 x 10(%ebp), %eax 8048453 <switch_eg+0 x 43> $0 x 1, %eax 0 x 10(%ebp), %eax %ebp 18
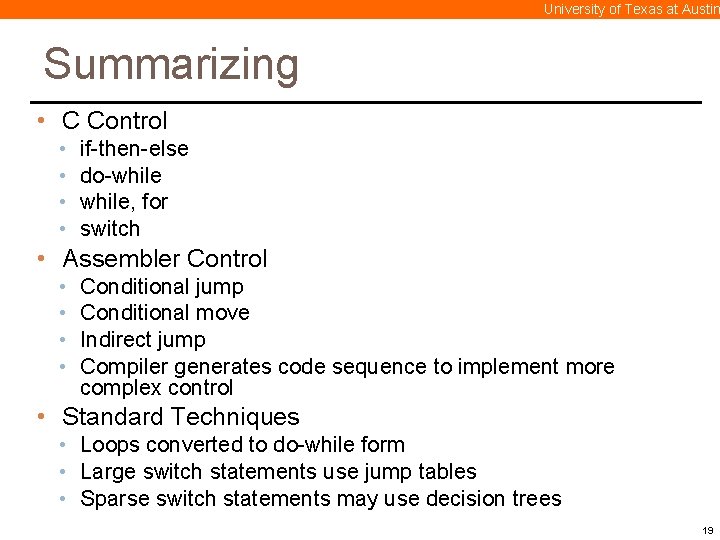
University of Texas at Austin Summarizing • C Control • • if-then-else do-while, for switch • Assembler Control • • Conditional jump Conditional move Indirect jump Compiler generates code sequence to implement more complex control • Standard Techniques • Loops converted to do-while form • Large switch statements use jump tables • Sparse switch statements may use decision trees 19
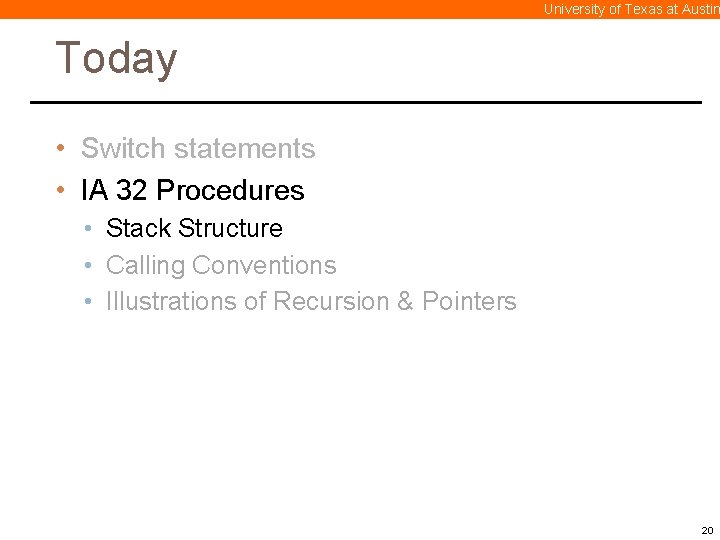
University of Texas at Austin Today • Switch statements • IA 32 Procedures • Stack Structure • Calling Conventions • Illustrations of Recursion & Pointers 20
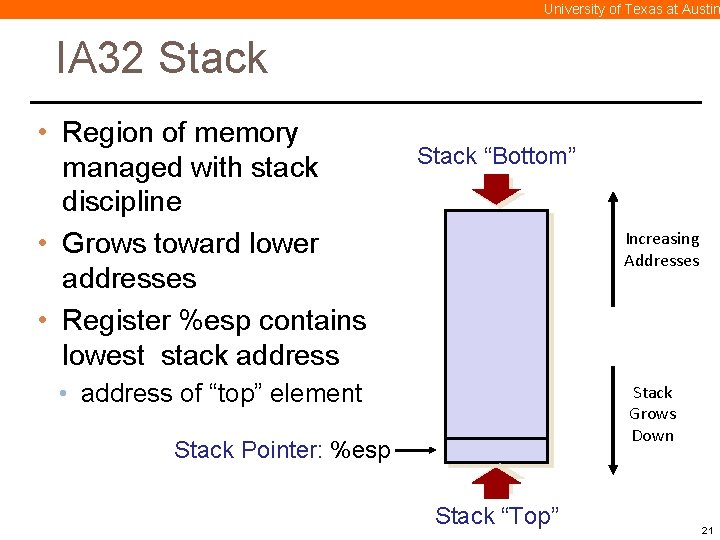
University of Texas at Austin IA 32 Stack • Region of memory managed with stack discipline • Grows toward lower addresses • Register %esp contains lowest stack address Stack “Bottom” Increasing Addresses • address of “top” element Stack Grows Down Stack Pointer: %esp Stack “Top” 21
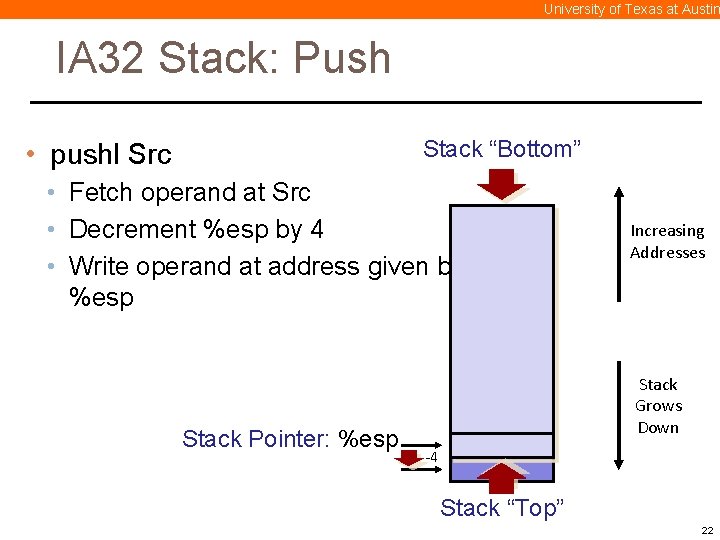
University of Texas at Austin IA 32 Stack: Push Stack “Bottom” • pushl Src • Fetch operand at Src • Decrement %esp by 4 • Write operand at address given by %esp Stack Pointer: %esp Increasing Addresses Stack Grows Down -4 Stack “Top” 22
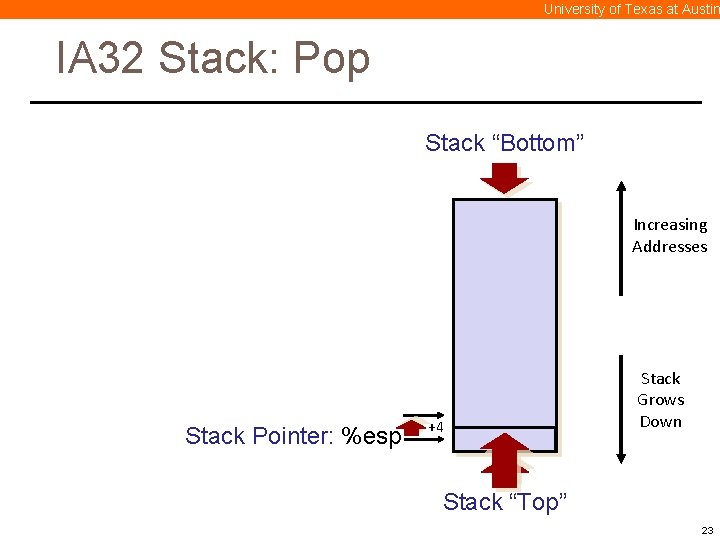
University of Texas at Austin IA 32 Stack: Pop Stack “Bottom” Increasing Addresses Stack Pointer: %esp +4 Stack Grows Down Stack “Top” 23
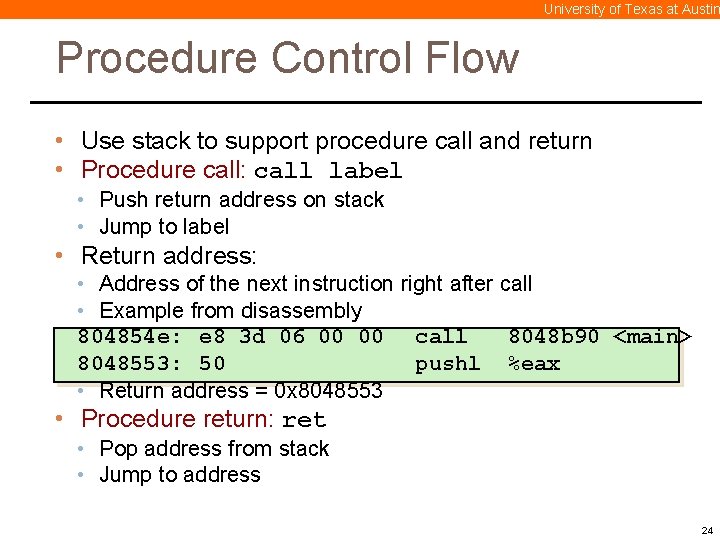
University of Texas at Austin Procedure Control Flow • Use stack to support procedure call and return • Procedure call: call label • Push return address on stack • Jump to label • Return address: • Address of the next instruction right after call • Example from disassembly 804854 e: e 8 3 d 06 00 00 call 8048 b 90 <main> 8048553: 50 pushl %eax • Return address = 0 x 8048553 • Procedure return: ret • Pop address from stack • Jump to address 24
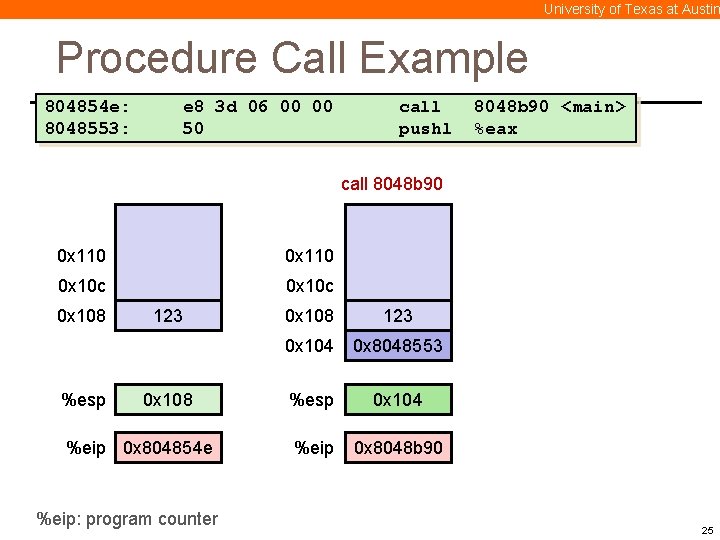
University of Texas at Austin Procedure Call Example 804854 e: 8048553: e 8 3 d 06 00 00 50 call pushl 8048 b 90 <main> %eax call 8048 b 90 0 x 110 0 x 10 c 0 x 108 %esp 123 0 x 108 %eip 0 x 804854 e %eip: program counter 0 x 108 123 0 x 104 0 x 8048553 %esp 0 x 104 %eip 0 x 8048 b 90 25
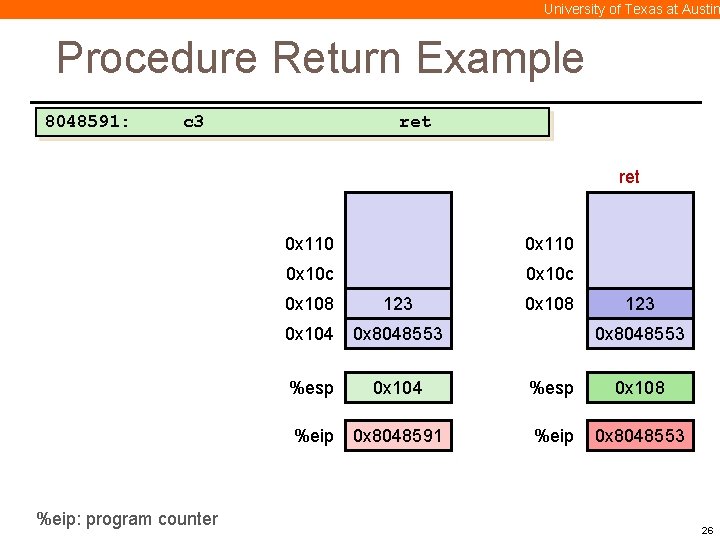
University of Texas at Austin Procedure Return Example 8048591: c 3 ret %eip: program counter 0 x 110 0 x 10 c 0 x 108 123 0 x 104 0 x 8048553 %esp 0 x 104 %esp 0 x 108 %eip 0 x 8048591 %eip 0 x 8048553 26
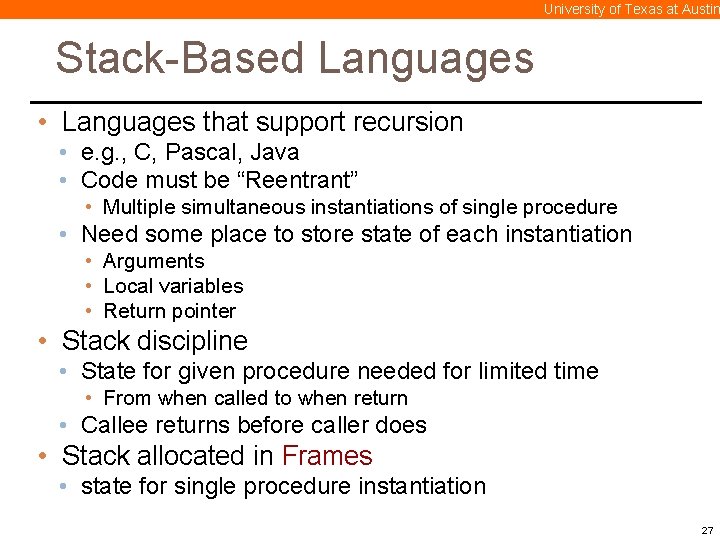
University of Texas at Austin Stack-Based Languages • Languages that support recursion • e. g. , C, Pascal, Java • Code must be “Reentrant” • Multiple simultaneous instantiations of single procedure • Need some place to store state of each instantiation • Arguments • Local variables • Return pointer • Stack discipline • State for given procedure needed for limited time • From when called to when return • Callee returns before caller does • Stack allocated in Frames • state for single procedure instantiation 27
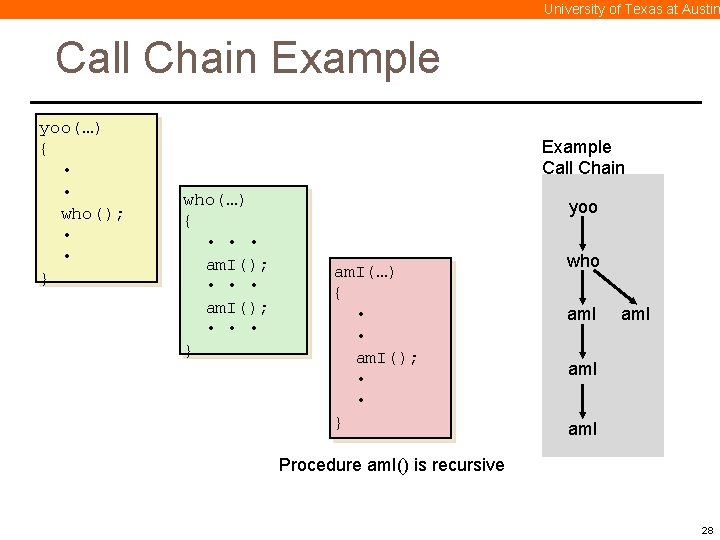
University of Texas at Austin Call Chain Example yoo(…) { • • who(); • • } Example Call Chain who(…) { • • • am. I(); • • • } yoo am. I(…) { • • am. I(); • • } who am. I Procedure am. I() is recursive 28
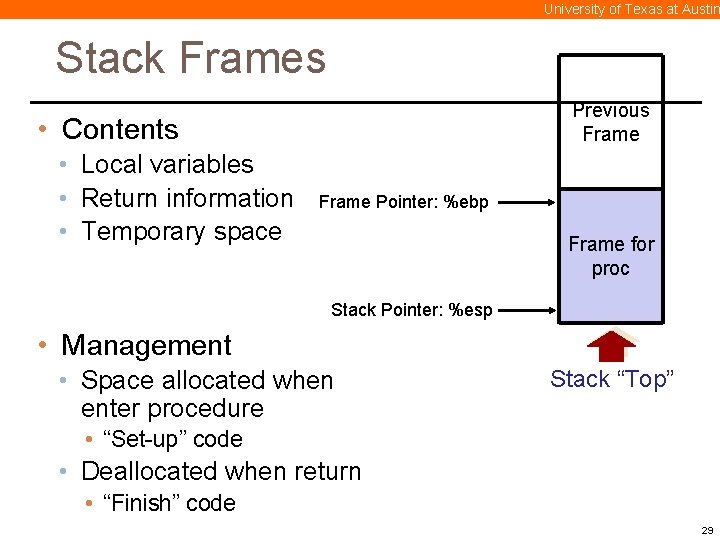
University of Texas at Austin Stack Frames Previous Frame • Contents • Local variables • Return information • Temporary space Frame Pointer: %ebp Frame for proc Stack Pointer: %esp • Management • Space allocated when enter procedure Stack “Top” • “Set-up” code • Deallocated when return • “Finish” code 29
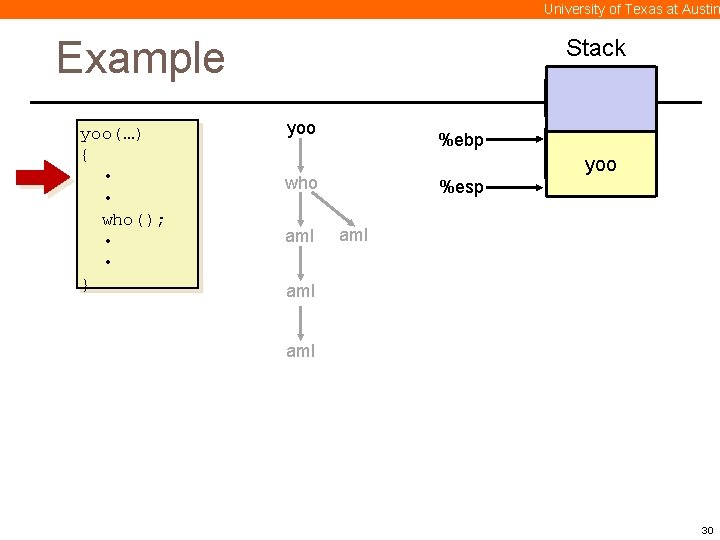
University of Texas at Austin Example yoo(…) { • • who(); • • } Stack yoo %ebp yoo who am. I %esp am. I 30
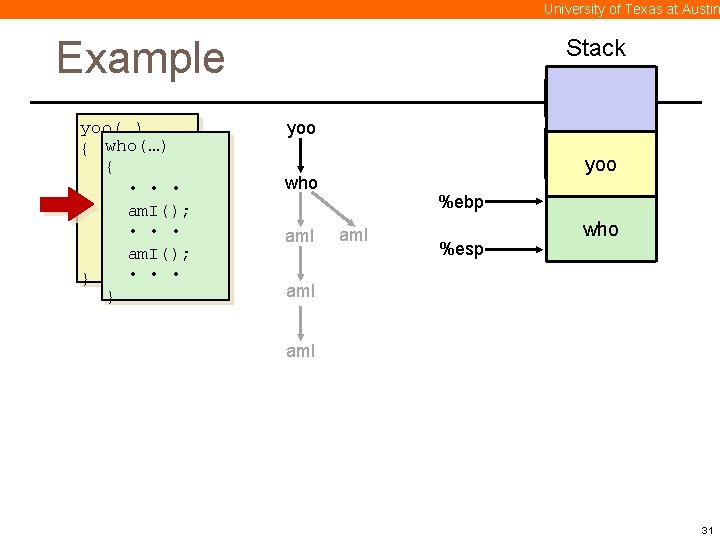
University of Texas at Austin Example yoo(…) { who(…) • { • • am. I(); who(); • • • am. I(); • • • } } Stack yoo yoo who am. I %ebp am. I %esp who am. I 31
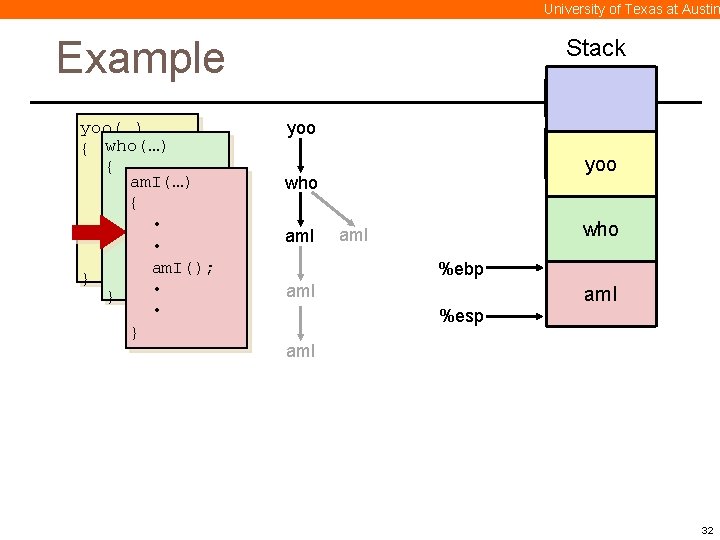
University of Texas at Austin Example yoo(…) { who(…) • { am. I(…) • • { am. I(); who(); • • am. I(); • } • } Stack yoo yoo who am. I %ebp am. I %esp am. I 32
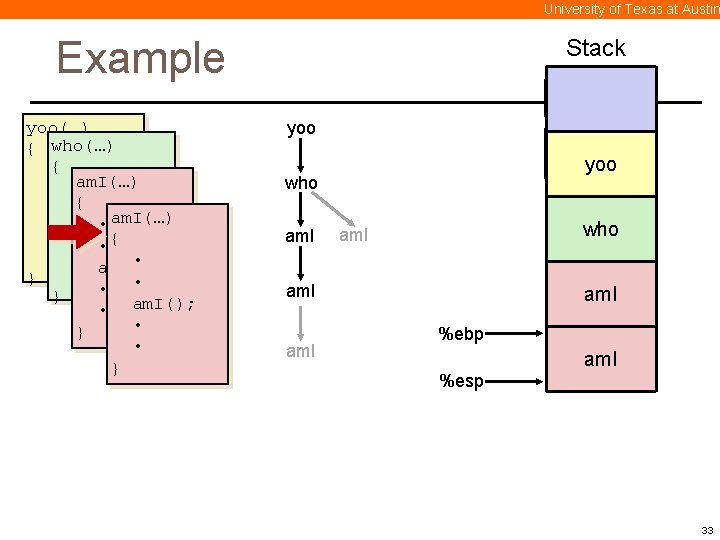
University of Texas at Austin Example yoo(…) { who(…) • { am. I(…) • • { am. I(); who(); am. I(…) • • { • • • am. I(); • • } • am. I(); • } Stack yoo yoo who am. I %ebp am. I %esp 33
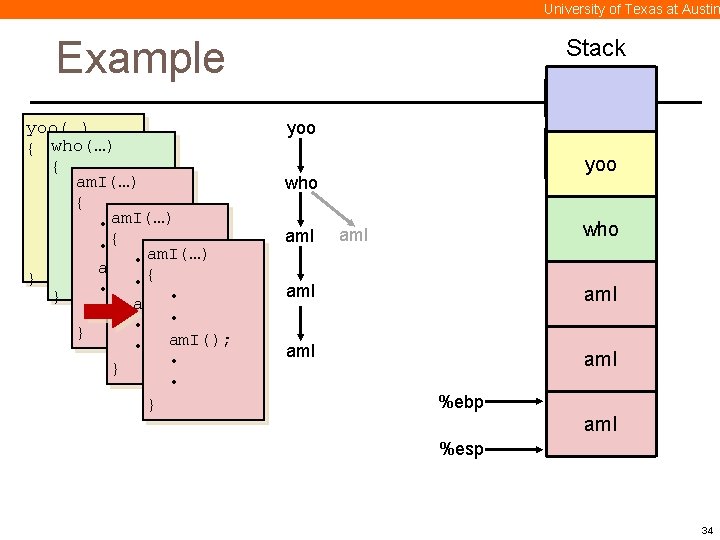
University of Texas at Austin Example yoo(…) { who(…) • { am. I(…) • • { am. I(); who(); am. I(…) • • { • • • am. I(); • am. I(…) • • am. I(); • • { } • am. I(); • • • } • am. I(); • } Stack yoo yoo who am. I %ebp am. I %esp 34
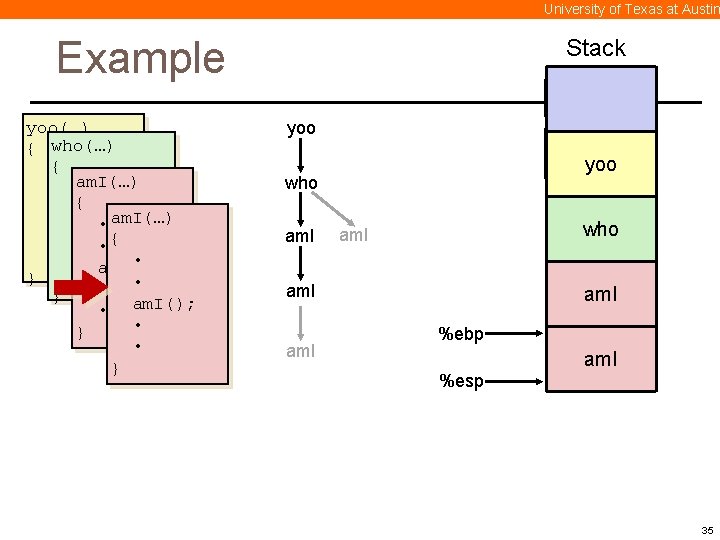
University of Texas at Austin Example yoo(…) { who(…) • { am. I(…) • • { am. I(); who(); am. I(…) • • { • • • am. I(); • • } • am. I(); • } Stack yoo yoo who am. I %ebp am. I %esp 35
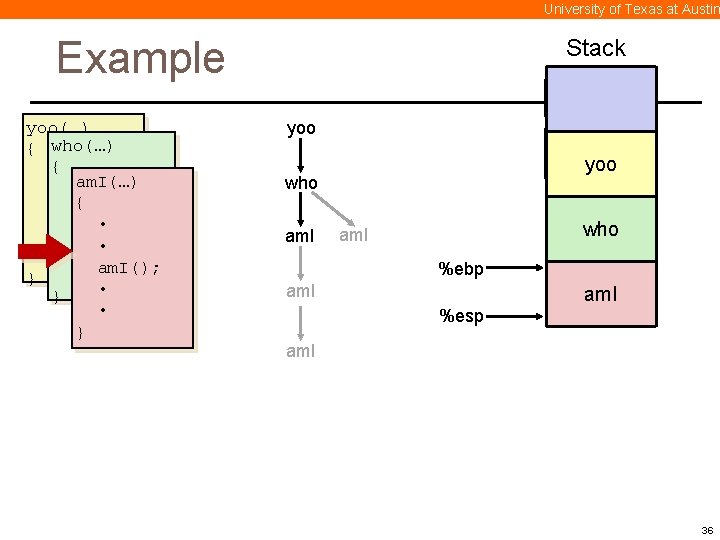
University of Texas at Austin Example yoo(…) { who(…) • { am. I(…) • • { am. I(); who(); • • am. I(); • } • } Stack yoo yoo who am. I %ebp am. I %esp am. I 36
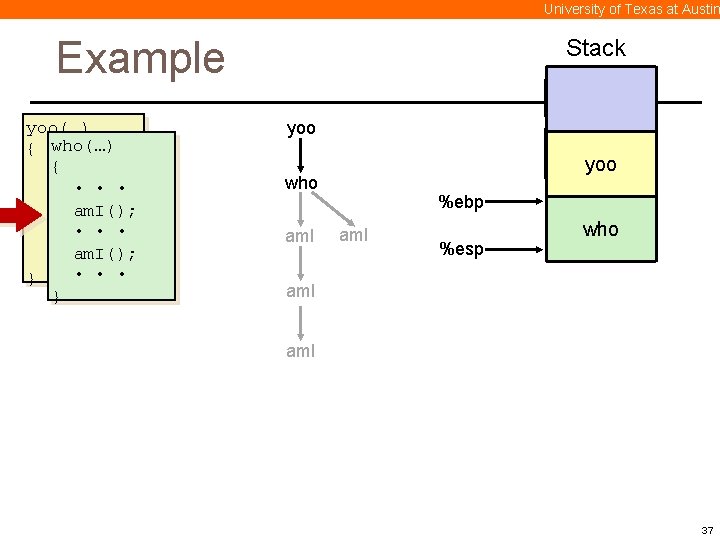
University of Texas at Austin Example yoo(…) { who(…) • { • • am. I(); who(); • • • am. I(); • • • } } Stack yoo yoo who am. I %ebp am. I %esp who am. I 37
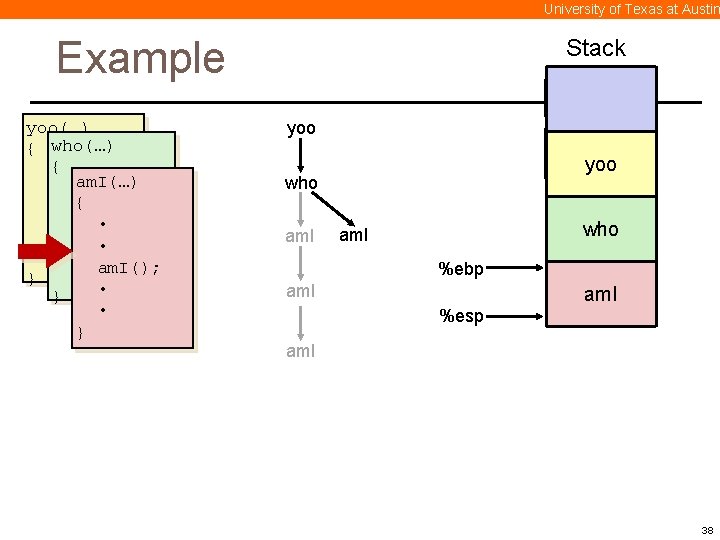
University of Texas at Austin Example yoo(…) { who(…) • { am. I(…) • • { am. I(); who(); • • am. I(); • } • } Stack yoo yoo who am. I %ebp am. I %esp am. I 38
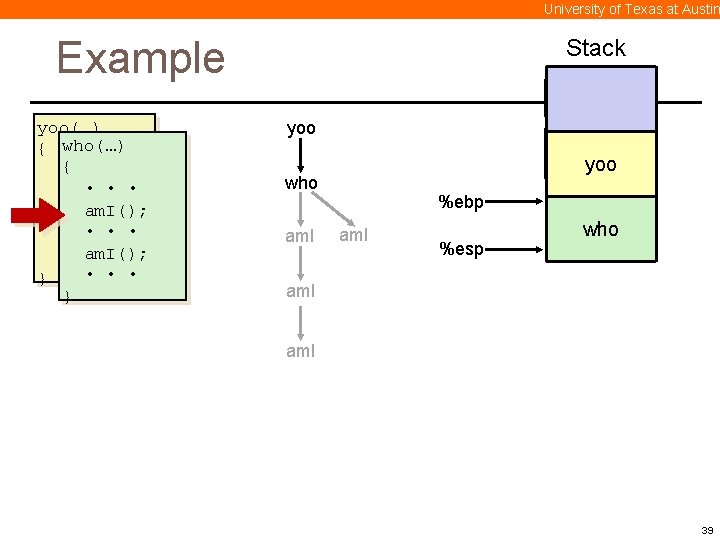
University of Texas at Austin Example yoo(…) { who(…) • { • • am. I(); who(); • • • am. I(); • • • } } Stack yoo yoo who am. I %ebp am. I %esp who am. I 39
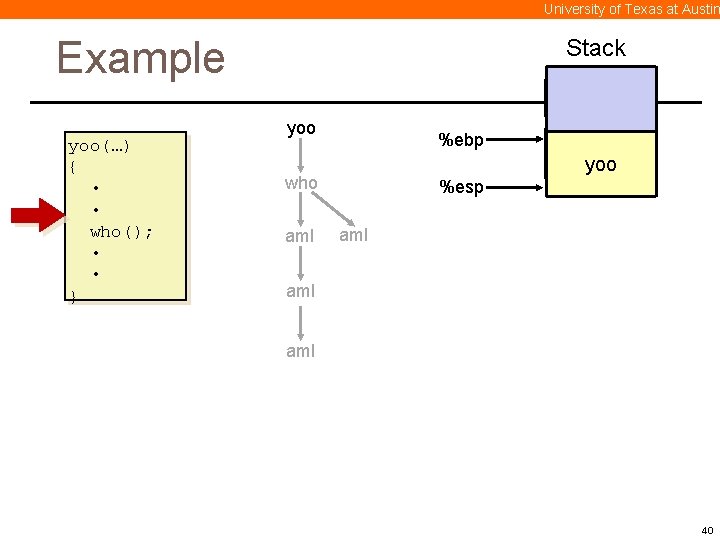
University of Texas at Austin Example yoo(…) { • • who(); • • } Stack yoo %ebp yoo who am. I %esp am. I 40
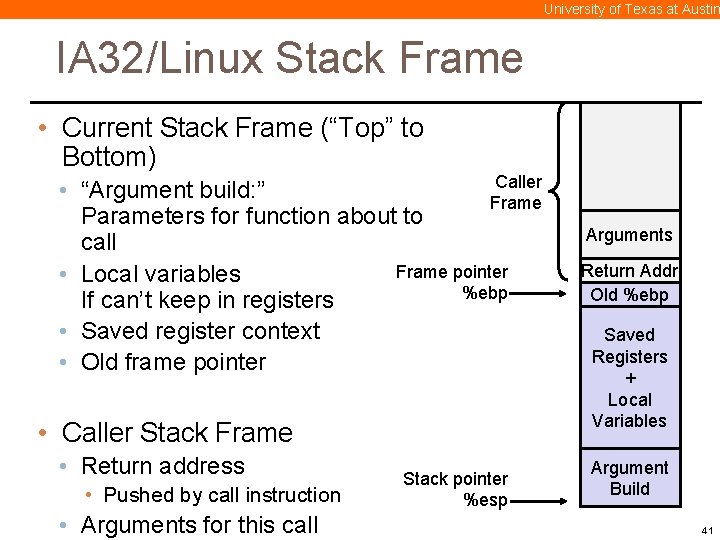
University of Texas at Austin IA 32/Linux Stack Frame • Current Stack Frame (“Top” to Bottom) Caller • “Argument build: ” Frame Parameters for function about to call Frame pointer • Local variables %ebp If can’t keep in registers • Saved register context • Old frame pointer • Caller Stack Frame • Return address • Pushed by call instruction • Arguments for this call Stack pointer %esp Arguments Return Addr Old %ebp Saved Registers + Local Variables Argument Build 41
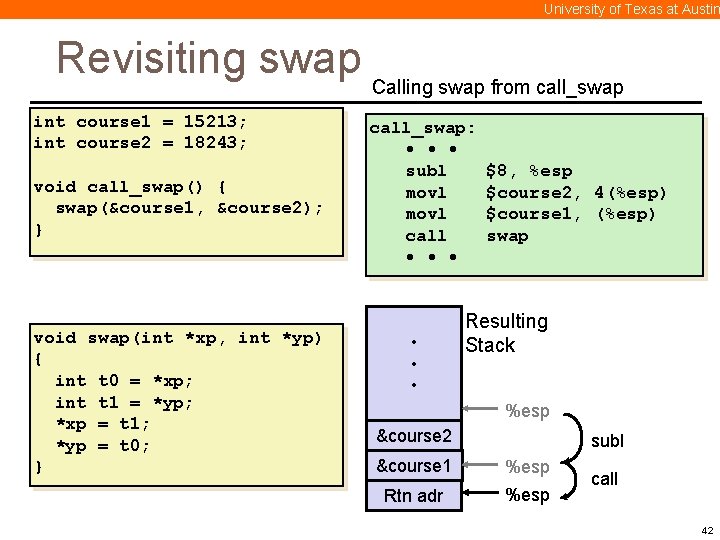
University of Texas at Austin Revisiting swap Calling swap from call_swap int course 1 = 15213; int course 2 = 18243; void call_swap() { swap(&course 1, &course 2); } void swap(int *xp, int *yp) { int t 0 = *xp; int t 1 = *yp; *xp = t 1; *yp = t 0; } call_swap: • • • subl movl call • • • $8, %esp $course 2, 4(%esp) $course 1, (%esp) swap Resulting Stack %esp &course 2 subl &course 1 %esp Rtn adr %esp call 42
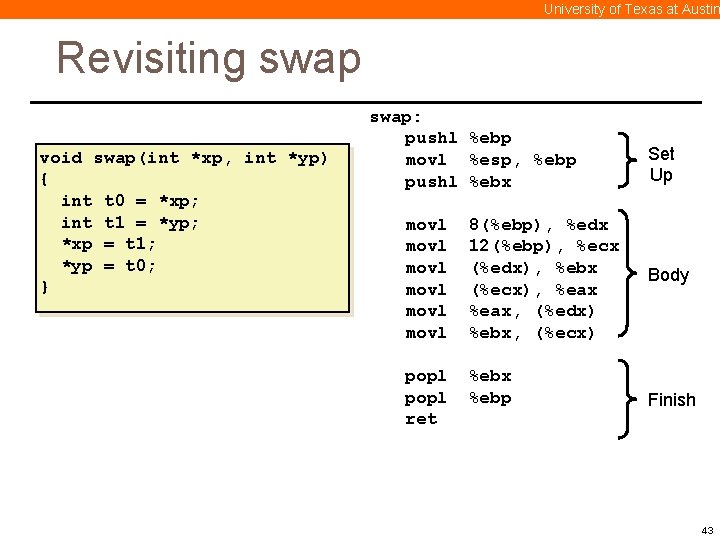
University of Texas at Austin Revisiting swap void swap(int *xp, int *yp) { int t 0 = *xp; int t 1 = *yp; *xp = t 1; *yp = t 0; } swap: pushl %ebp movl %esp, %ebp pushl %ebx movl movl 8(%ebp), %edx 12(%ebp), %ecx (%edx), %ebx (%ecx), %eax, (%edx) %ebx, (%ecx) popl ret %ebx %ebp Set Up Body Finish 43
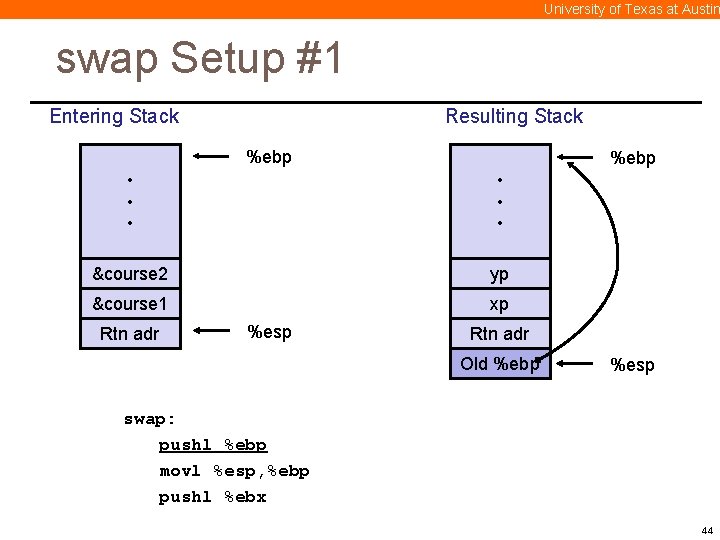
University of Texas at Austin swap Setup #1 Entering Stack Resulting Stack %ebp • • • &course 2 yp &course 1 xp Rtn adr %esp Rtn adr Old %ebp %esp swap: pushl %ebp movl %esp, %ebp pushl %ebx 44
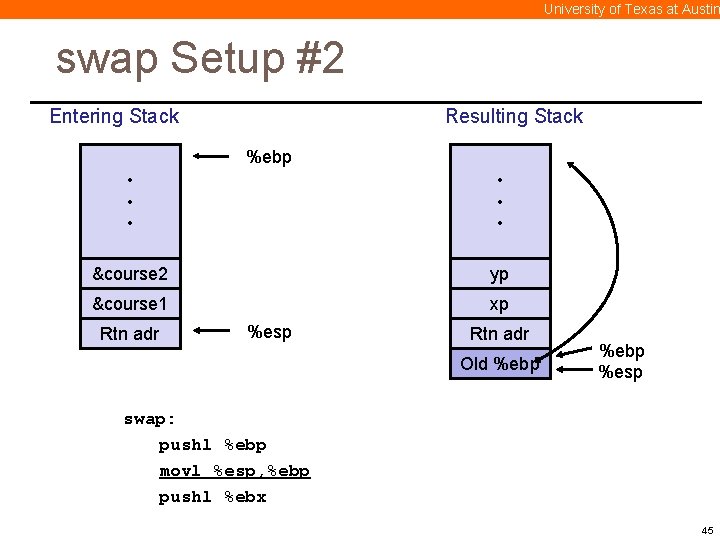
University of Texas at Austin swap Setup #2 Entering Stack Resulting Stack %ebp • • • &course 2 yp &course 1 xp Rtn adr %esp Rtn adr Old %ebp %esp swap: pushl %ebp movl %esp, %ebp pushl %ebx 45
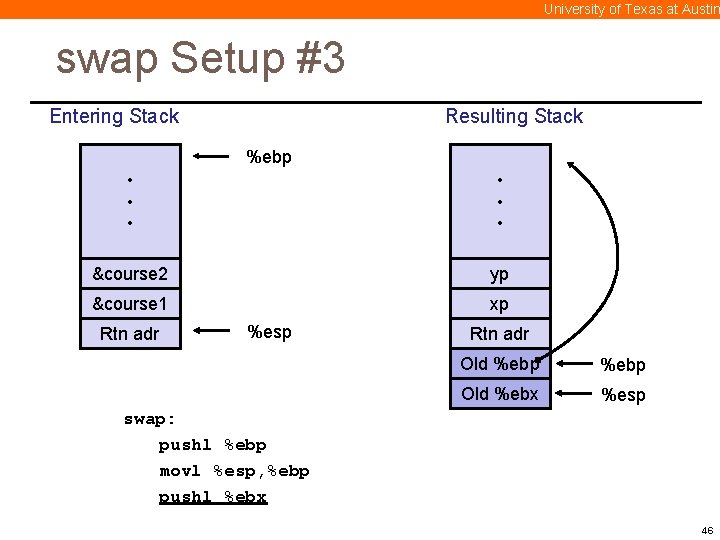
University of Texas at Austin swap Setup #3 Entering Stack Resulting Stack %ebp • • • &course 2 yp &course 1 xp Rtn adr %esp Rtn adr Old %ebp Old %ebx %esp swap: pushl %ebp movl %esp, %ebp pushl %ebx 46
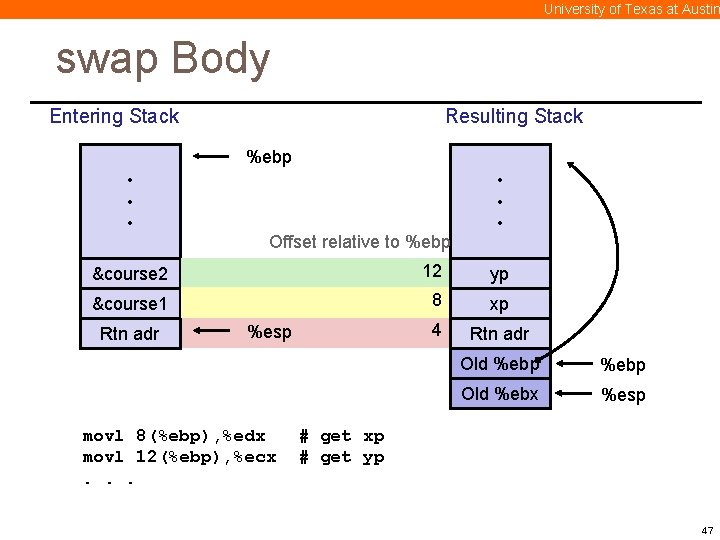
University of Texas at Austin swap Body Entering Stack Resulting Stack %ebp • • • Offset relative to %ebp • • • &course 2 12 yp &course 1 8 xp 4 Rtn adr %esp movl 8(%ebp), %edx movl 12(%ebp), %ecx. . . Old %ebp Old %ebx %esp # get xp # get yp 47
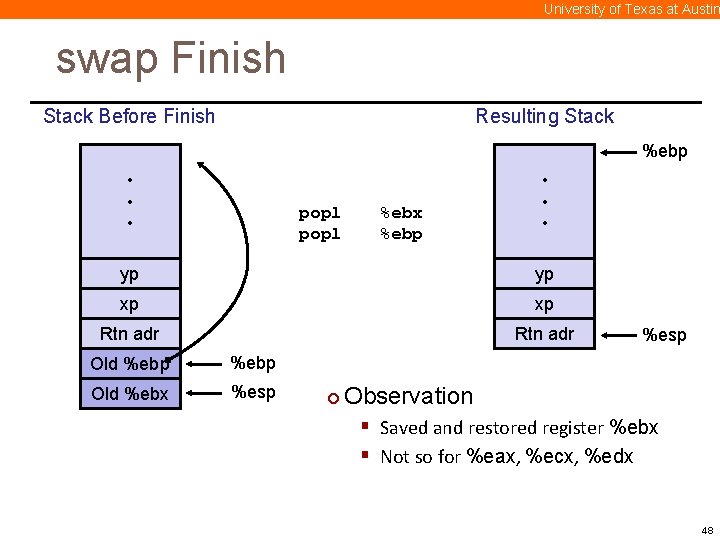
University of Texas at Austin swap Finish Stack Before Finish Resulting Stack %ebp • • • popl %ebx %ebp • • • yp yp xp xp Rtn adr Old %ebp Old %ebx %esp ¢ %esp Observation § Saved and restored register %ebx § Not so for %eax, %ecx, %edx 48
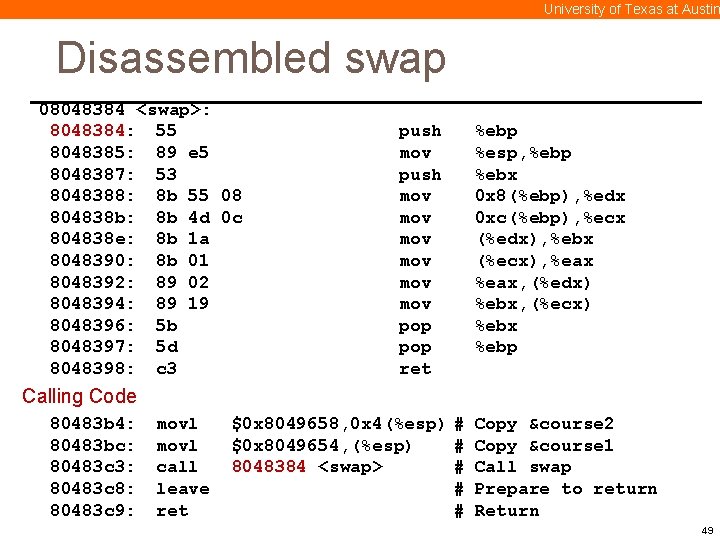
University of Texas at Austin Disassembled swap 08048384 <swap>: 8048384: 55 8048385: 89 e 5 8048387: 53 8048388: 8 b 55 08 804838 b: 8 b 4 d 0 c 804838 e: 8 b 1 a 8048390: 8 b 01 8048392: 89 02 8048394: 89 19 8048396: 5 b 8048397: 5 d 8048398: c 3 push mov mov pop ret %ebp %esp, %ebp %ebx 0 x 8(%ebp), %edx 0 xc(%ebp), %ecx (%edx), %ebx (%ecx), %eax, (%edx) %ebx, (%ecx) %ebx %ebp Calling Code 80483 b 4: 80483 bc: 80483 c 3: 80483 c 8: 80483 c 9: movl call leave ret $0 x 8049658, 0 x 4(%esp) # $0 x 8049654, (%esp) # 8048384 <swap> # # # Copy &course 2 Copy &course 1 Call swap Prepare to return Return 49
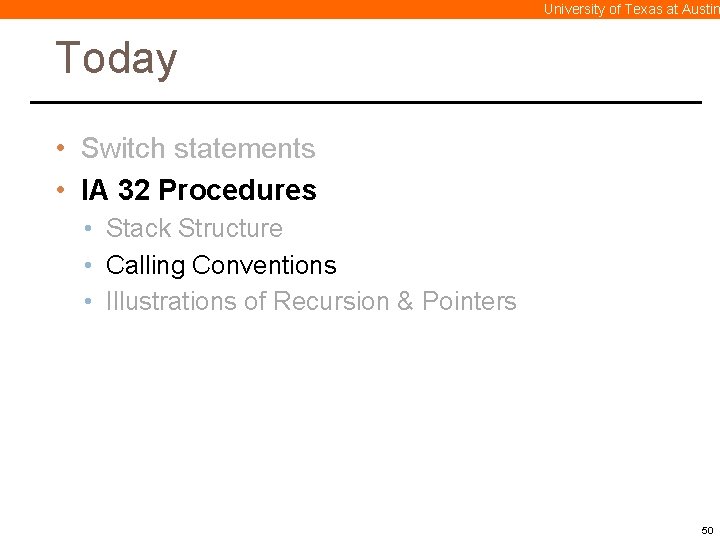
University of Texas at Austin Today • Switch statements • IA 32 Procedures • Stack Structure • Calling Conventions • Illustrations of Recursion & Pointers 50
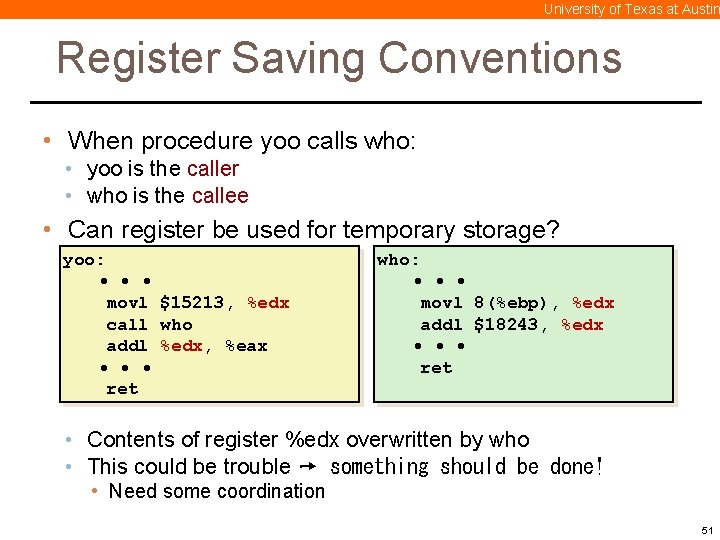
University of Texas at Austin Register Saving Conventions • When procedure yoo calls who: • yoo is the caller • who is the callee • Can register be used for temporary storage? yoo: • • • movl $15213, %edx call who addl %edx, %eax • • • ret who: • • • movl 8(%ebp), %edx addl $18243, %edx • • • ret • Contents of register %edx overwritten by who • This could be trouble ➙ something should be done! • Need some coordination 51
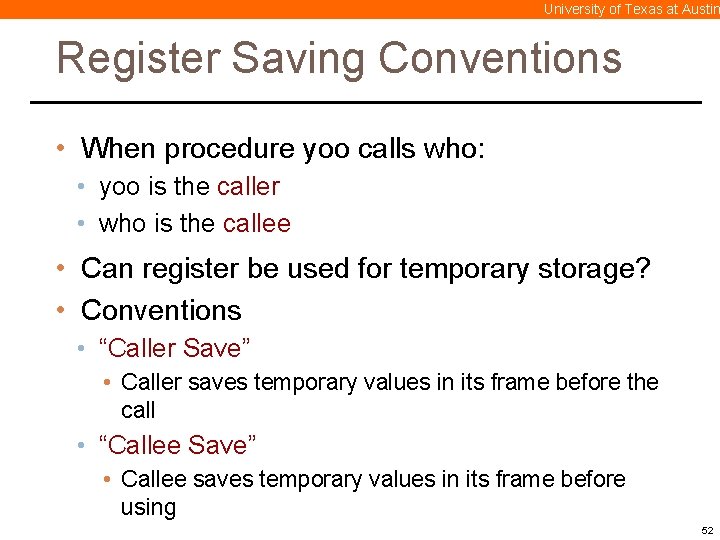
University of Texas at Austin Register Saving Conventions • When procedure yoo calls who: • yoo is the caller • who is the callee • Can register be used for temporary storage? • Conventions • “Caller Save” • Caller saves temporary values in its frame before the call • “Callee Save” • Callee saves temporary values in its frame before using 52
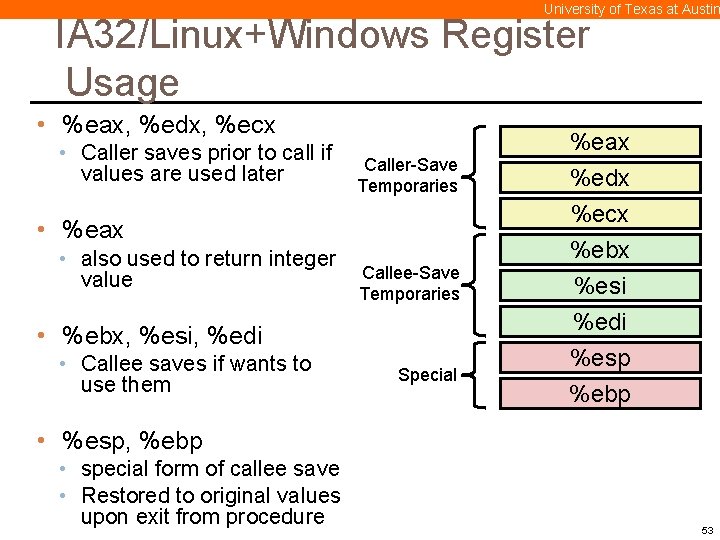
University of Texas at Austin IA 32/Linux+Windows Register Usage • %eax, %edx, %ecx • Caller saves prior to call if values are used later Caller-Save Temporaries • %eax • also used to return integer value Callee-Save Temporaries • %ebx, %esi, %edi • Callee saves if wants to use them Special %eax %edx %ecx %ebx %esi %edi %esp %ebp • %esp, %ebp • special form of callee save • Restored to original values upon exit from procedure 53
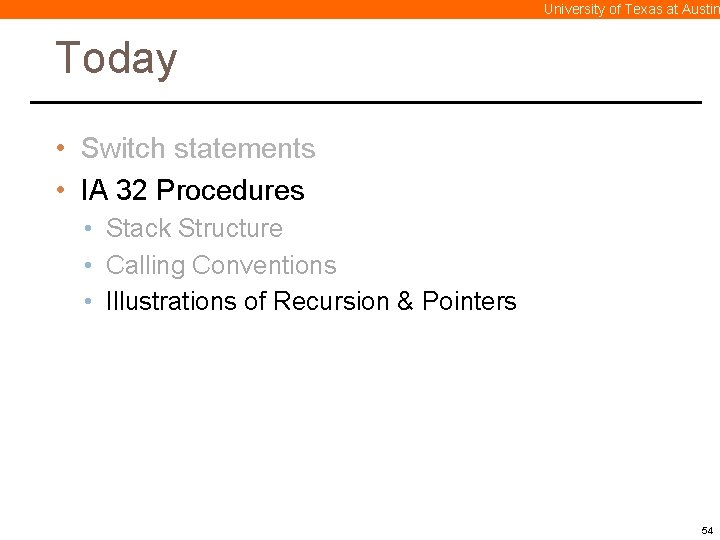
University of Texas at Austin Today • Switch statements • IA 32 Procedures • Stack Structure • Calling Conventions • Illustrations of Recursion & Pointers 54
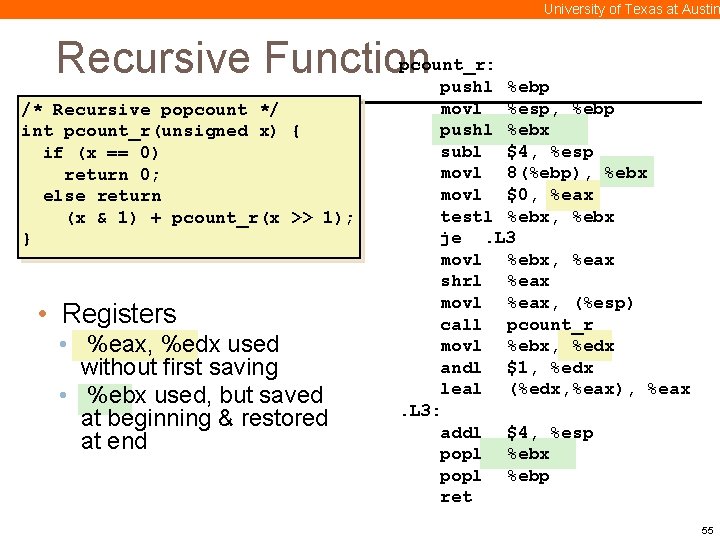
University of Texas at Austin pcount_r: Recursive Function pushl %ebp /* Recursive popcount */ int pcount_r(unsigned x) { if (x == 0) return 0; else return (x & 1) + pcount_r(x >> 1); } • Registers • %eax, %edx used without first saving • %ebx used, but saved at beginning & restored at end movl %esp, %ebp pushl %ebx subl $4, %esp movl 8(%ebp), %ebx movl $0, %eax testl %ebx, %ebx je. L 3 movl %ebx, %eax shrl %eax movl %eax, (%esp) call pcount_r movl %ebx, %edx andl $1, %edx leal (%edx, %eax), %eax. L 3: addl $4, %esp popl %ebx popl %ebp ret 55
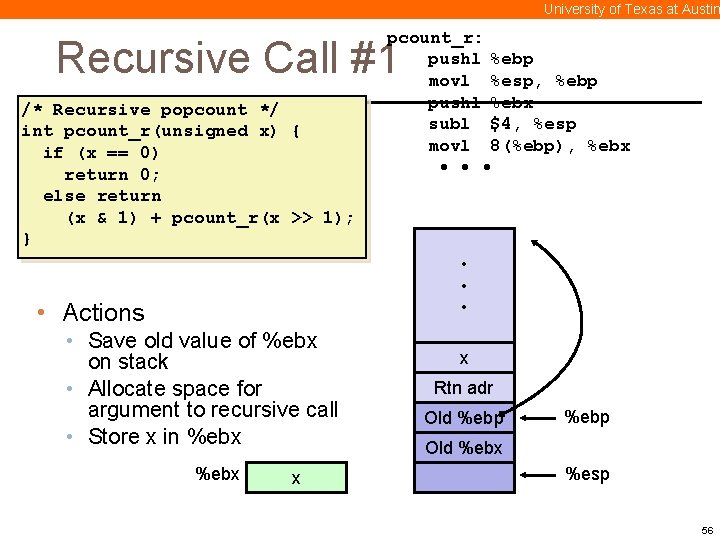
University of Texas at Austin pcount_r: pushl %ebp movl %esp, %ebp pushl %ebx subl $4, %esp movl 8(%ebp), %ebx • • • Recursive Call #1 /* Recursive popcount */ int pcount_r(unsigned x) { if (x == 0) return 0; else return (x & 1) + pcount_r(x >> 1); } • • Actions • Save old value of %ebx on stack • Allocate space for argument to recursive call • Store x in %ebx x x Rtn adr Old %ebp Old %ebx %esp 56
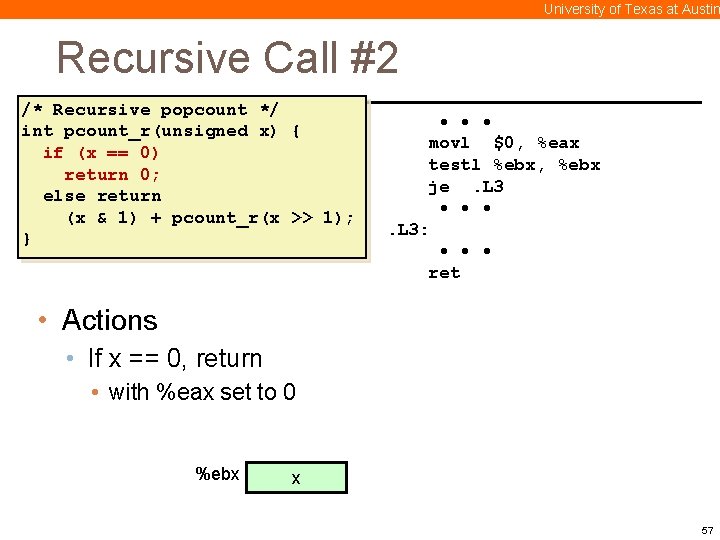
University of Texas at Austin Recursive Call #2 /* Recursive popcount */ int pcount_r(unsigned x) { if (x == 0) return 0; else return (x & 1) + pcount_r(x >> 1); } • • • movl $0, %eax testl %ebx, %ebx je. L 3 • • • . L 3: • • • ret • Actions • If x == 0, return • with %eax set to 0 %ebx x 57
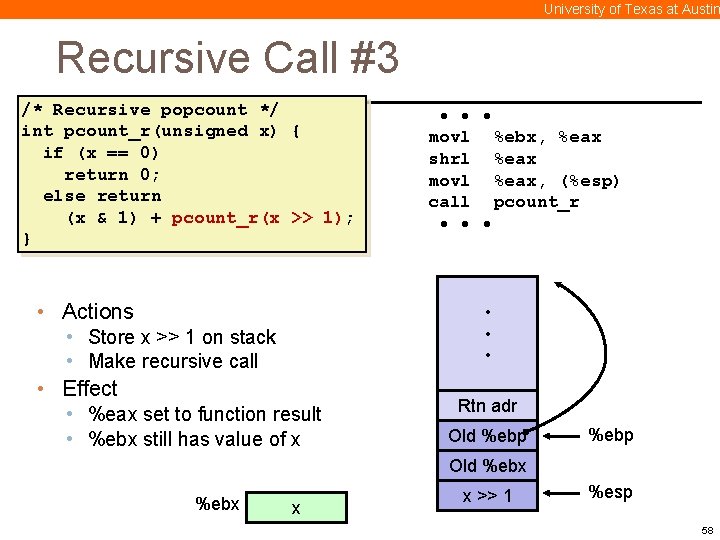
University of Texas at Austin Recursive Call #3 /* Recursive popcount */ int pcount_r(unsigned x) { if (x == 0) return 0; else return (x & 1) + pcount_r(x >> 1); } • Actions • • • movl %ebx, %eax shrl %eax movl %eax, (%esp) call pcount_r • • Store x >> 1 on stack • Make recursive call • Effect • %eax set to function result • %ebx still has value of x Rtn adr Old %ebp Old %ebx x x >> 1 %esp 58
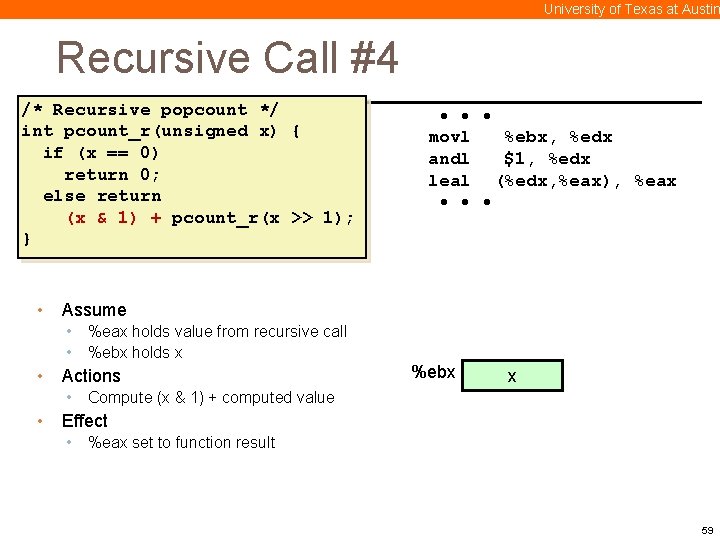
University of Texas at Austin Recursive Call #4 /* Recursive popcount */ int pcount_r(unsigned x) { if (x == 0) return 0; else return (x & 1) + pcount_r(x >> 1); } • • movl %ebx, %edx andl $1, %edx leal (%edx, %eax), %eax • • • Assume • %eax holds value from recursive call • %ebx holds x • Actions %ebx x • Compute (x & 1) + computed value • Effect • %eax set to function result 59
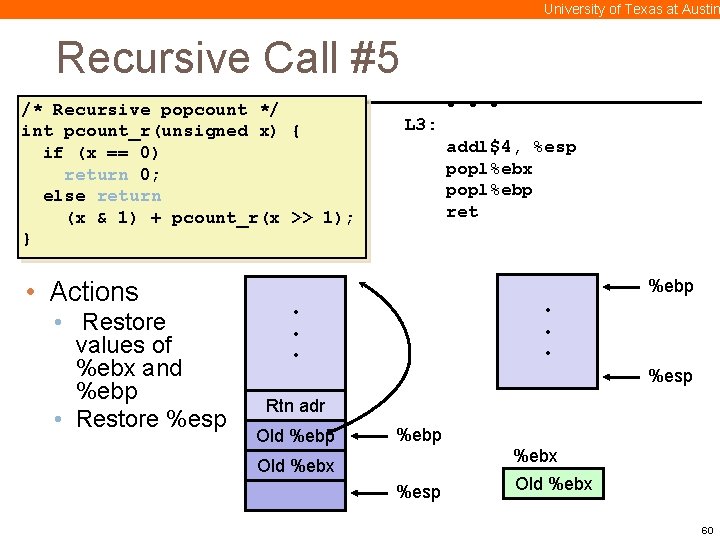
University of Texas at Austin Recursive Call #5 /* Recursive popcount */ int pcount_r(unsigned x) { if (x == 0) return 0; else return (x & 1) + pcount_r(x >> 1); } • Actions • Restore values of %ebx and %ebp • Restore %esp • • • L 3: addl$4, %esp popl%ebx popl%ebp ret %ebp • • • %esp Rtn adr Old %ebp %ebx Old %ebx %esp Old %ebx 60
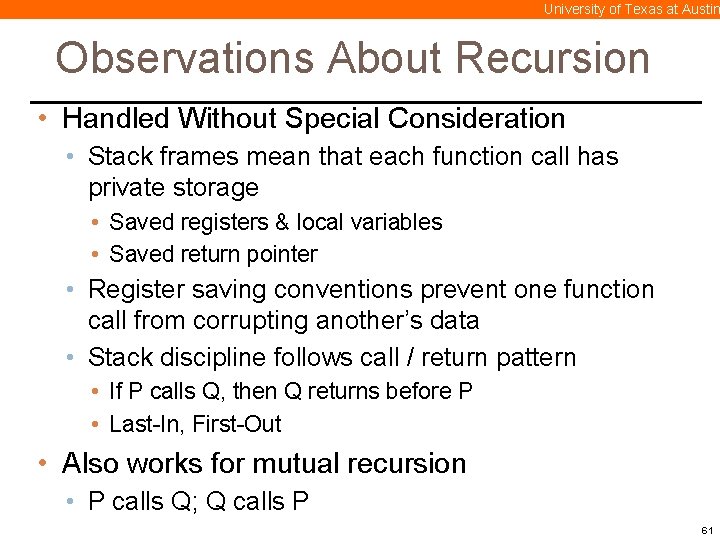
University of Texas at Austin Observations About Recursion • Handled Without Special Consideration • Stack frames mean that each function call has private storage • Saved registers & local variables • Saved return pointer • Register saving conventions prevent one function call from corrupting another’s data • Stack discipline follows call / return pattern • If P calls Q, then Q returns before P • Last-In, First-Out • Also works for mutual recursion • P calls Q; Q calls P 61
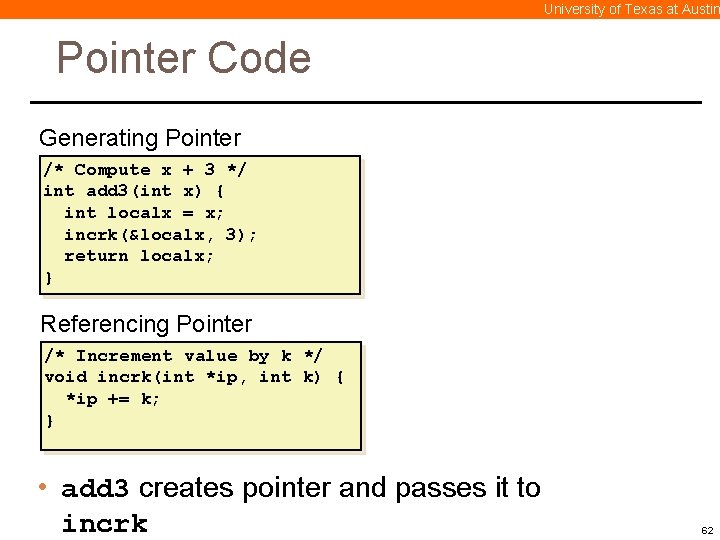
University of Texas at Austin Pointer Code Generating Pointer /* Compute x + 3 */ int add 3(int x) { int localx = x; incrk(&localx, 3); return localx; } Referencing Pointer /* Increment value by k */ void incrk(int *ip, int k) { *ip += k; } • add 3 creates pointer and passes it to incrk 62
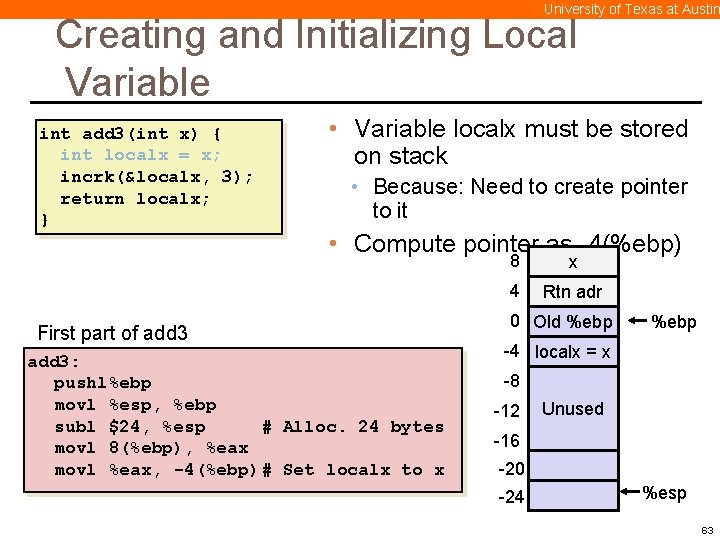
University of Texas at Austin Creating and Initializing Local Variable int add 3(int x) { int localx = x; incrk(&localx, 3); return localx; } • Variable localx must be stored on stack • Because: Need to create pointer to it • Compute pointer as -4(%ebp) First part of add 3: pushl%ebp movl %esp, %ebp subl $24, %esp # Alloc. 24 bytes movl 8(%ebp), %eax movl %eax, -4(%ebp)# Set localx to x 8 x 4 Rtn adr 0 Old %ebp -4 localx = x -8 -12 Unused -16 -20 -24 %esp 63
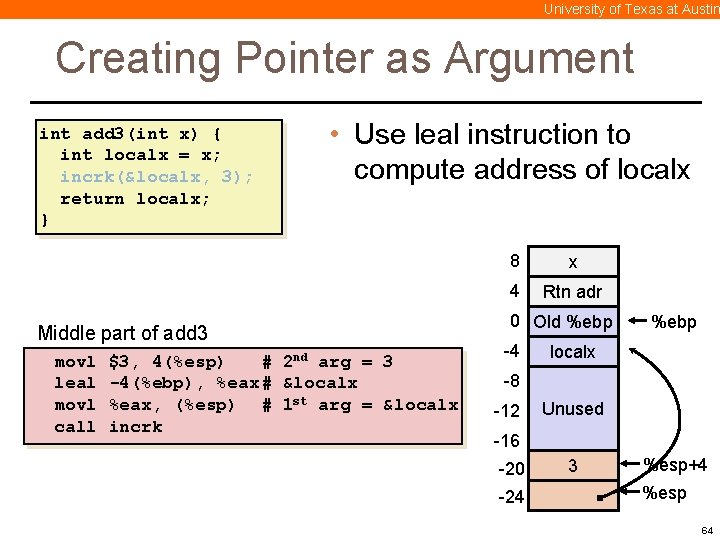
University of Texas at Austin Creating Pointer as Argument int add 3(int x) { int localx = x; incrk(&localx, 3); return localx; } • Use leal instruction to compute address of localx Middle part of add 3 movl leal movl call $3, 4(%esp) # 2 nd arg = 3 -4(%ebp), %eax# &localx %eax, (%esp) # 1 st arg = &localx incrk 8 x 4 Rtn adr 0 Old %ebp -4 %ebp localx -8 -12 Unused -16 -20 -24 3 %esp+4 %esp 64
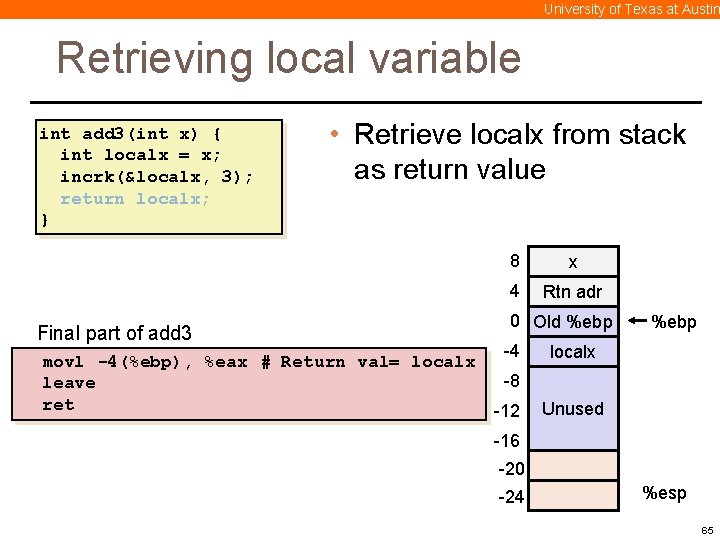
University of Texas at Austin Retrieving local variable int add 3(int x) { int localx = x; incrk(&localx, 3); return localx; } • Retrieve localx from stack as return value Final part of add 3 movl -4(%ebp), %eax # Return val= localx leave ret 8 x 4 Rtn adr 0 Old %ebp -4 %ebp localx -8 -12 Unused -16 -20 -24 %esp 65
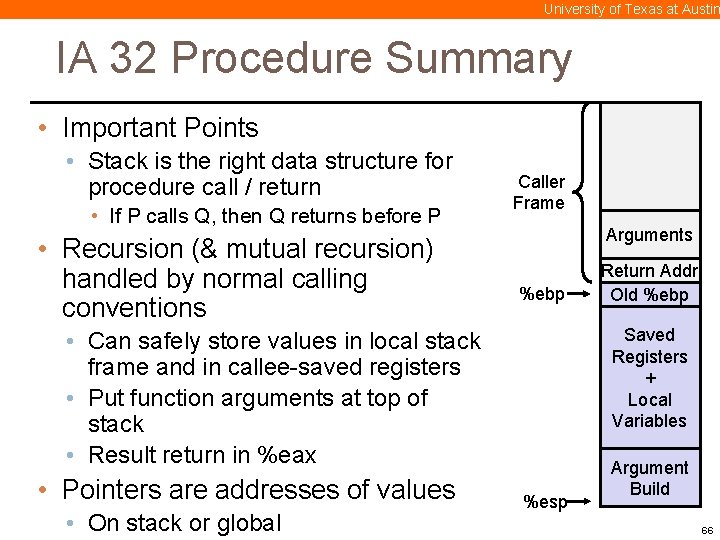
University of Texas at Austin IA 32 Procedure Summary • Important Points • Stack is the right data structure for procedure call / return • If P calls Q, then Q returns before P • Recursion (& mutual recursion) handled by normal calling conventions Caller Frame Arguments %ebp Saved Registers + Local Variables • Can safely store values in local stack frame and in callee-saved registers • Put function arguments at top of stack • Result return in %eax • Pointers are addresses of values • On stack or global Return Addr Old %ebp %esp Argument Build 66
The resources need to be reserved during the setup phase in
Go switch working principle
Hamlet act iii scene ii
What are switch statements in c
Are switch statements faster than if/else
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
Definition of system programming
Linear vs integer programming
Perbedaan linear programming dan integer programming
Conditional symbolic notation
Acd switch and ivr systems
Open and closed switch
Propaganda techniques emotional words
In a banyan switch for 8 inputs and 8 outputs we have
Matlab
Advantages and disadvantages of switch
Difference between tst and sts switch
Aluminum and iron iii oxide balanced equation
Differences between george washington and king george iii
Double replacement example
Looking for richard
John adams vs thomas jefferson venn diagram
The code of ethics for professional teachers
Romeo and juliet act 3 scene 3 summary
Act three romeo and juliet summary
What does the nurse give to romeo from juliet?
Hamlet act 3 events leading to climax
Digital network
Pole mounted voltage regulator
Difference between datagram and virtual circuit network
P type bjt
Getchar in c
Multiple selection statement in c
Terabit ethernet cable
Switch boot sequence
Switch structure
Switch statement c++
Switch framework
49p11-843
Switch merupakan struktur perulangan.
Apa yang disebut dengan determinate loop
Sleeve gastrectomy complications
G&w trident
Cisco 3750x warranty
Select case en visual basic ejemplos
Oki reed switch
Salt switch recycling
Www.dice-programming-etc.com
Prolog mobile
Compound light microscope parts
Ovs dpdk architecture
Nigeria central switch
Sami vibration switch
Trolley travel hand signal
Lectrotab switch wiring diagram
Juniper switch icon
Detent switch function
Kitchen simple machines
Switch 串接
Sentencias de control en visual basic
Bennett trim tab switch wiring diagram
Electric stove switch wiring diagram
Selector switch symbol plc
Command line arguments in unix
Switch 串接