Lecture No 11 Data Structures Dr Sohail Aslam
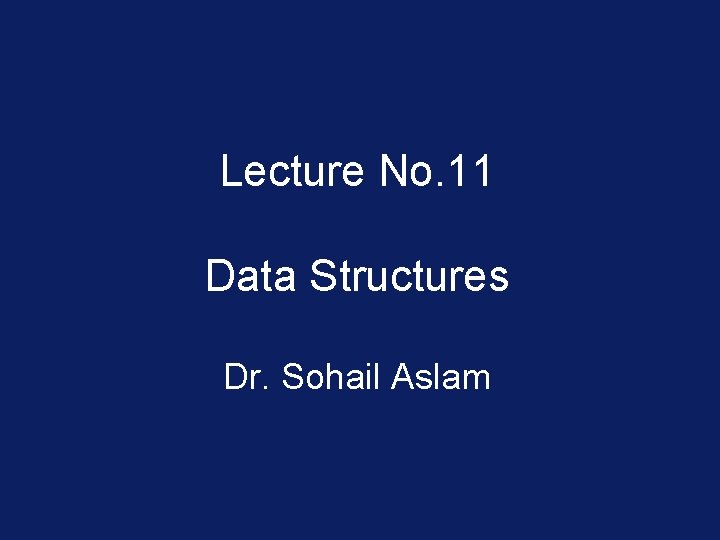
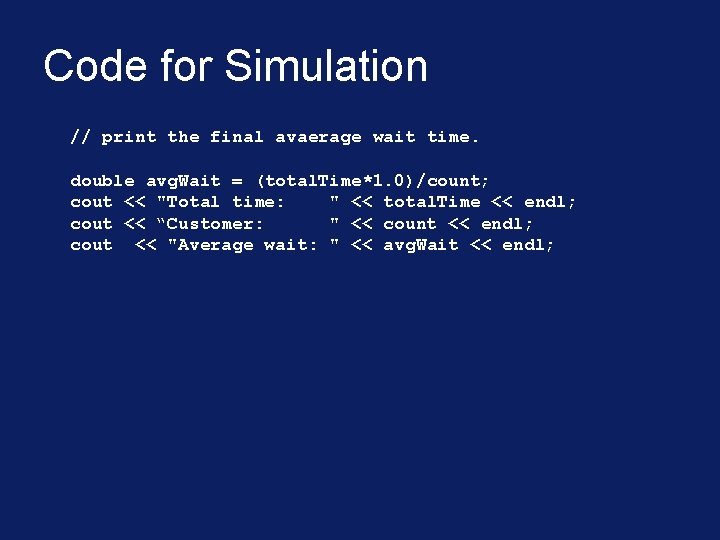
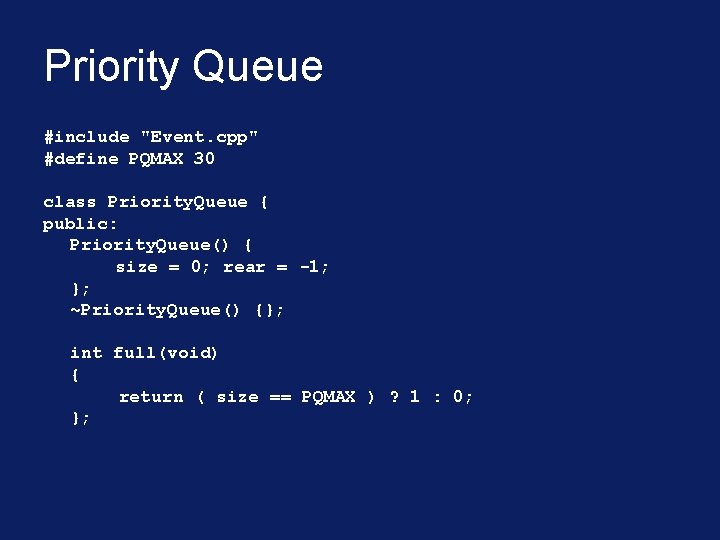
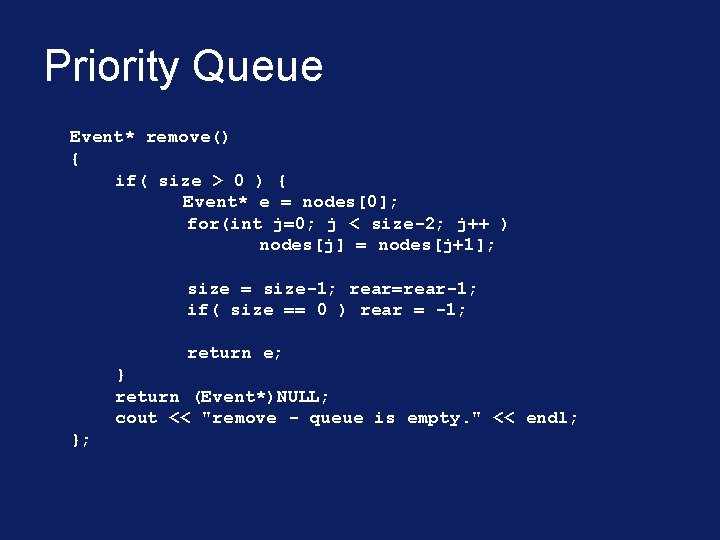
![Priority Queue int insert(Event* e) { if( !full() ) { rear = rear+1; nodes[rear] Priority Queue int insert(Event* e) { if( !full() ) { rear = rear+1; nodes[rear]](https://slidetodoc.com/presentation_image_h2/6d97fc572a6eb74dd0a322d6a63a8fe2/image-5.jpg)
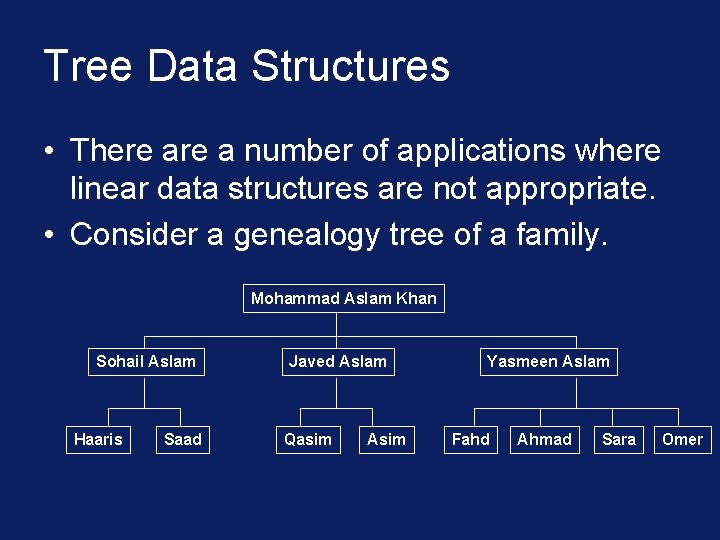
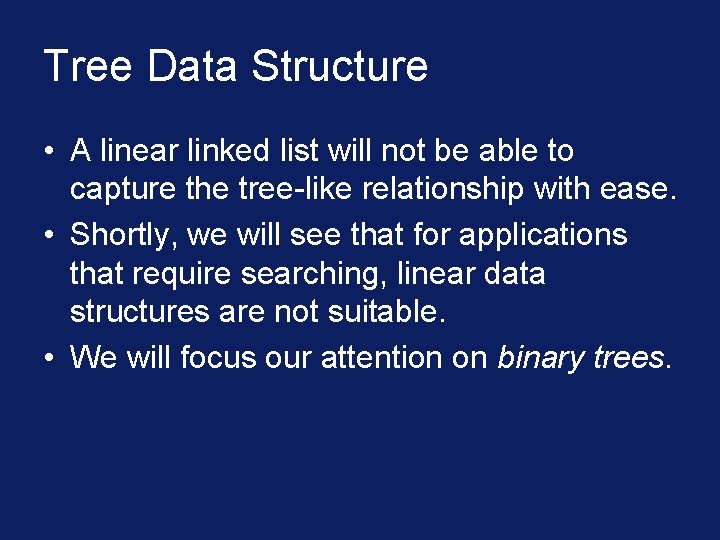
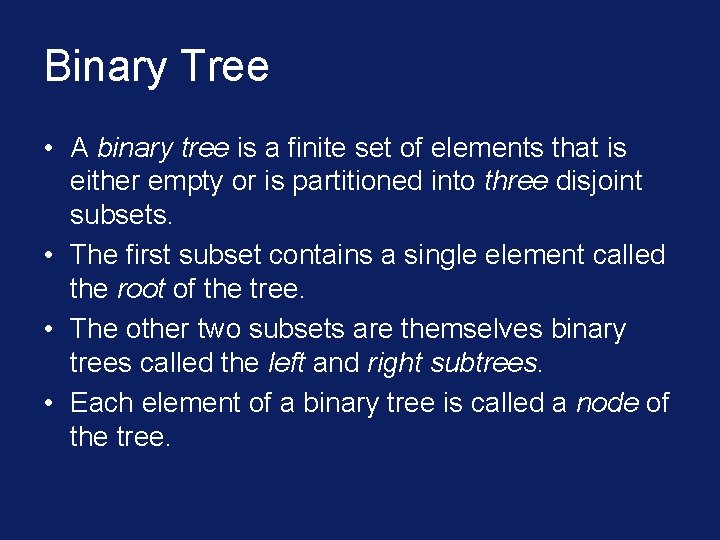
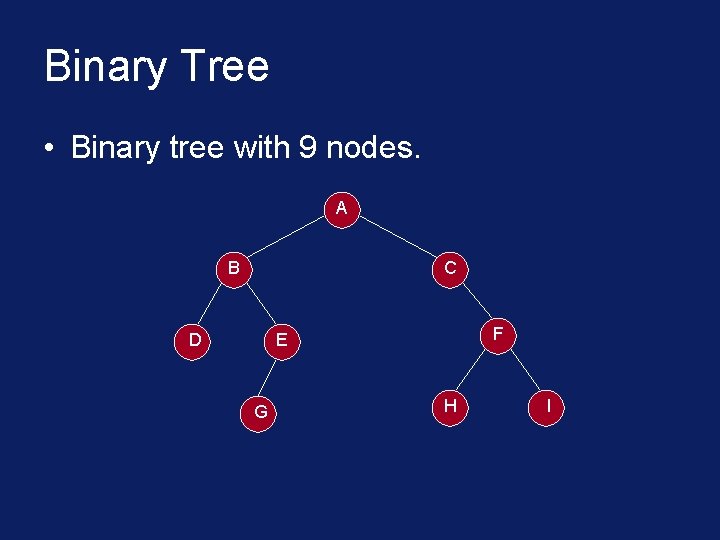
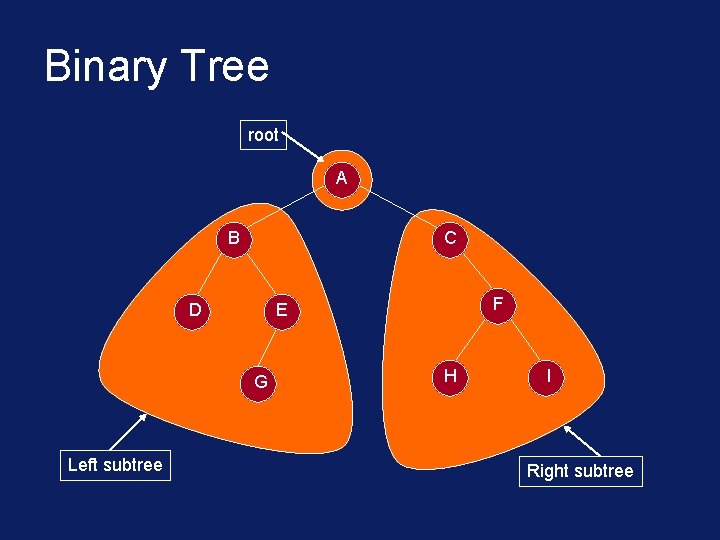
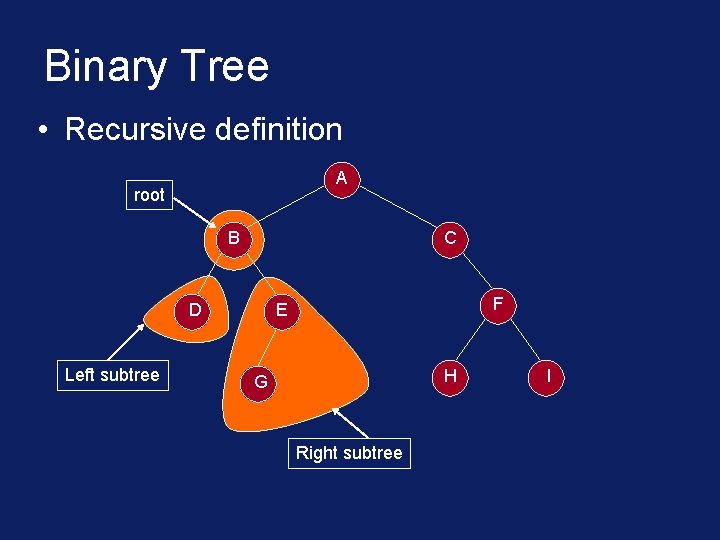
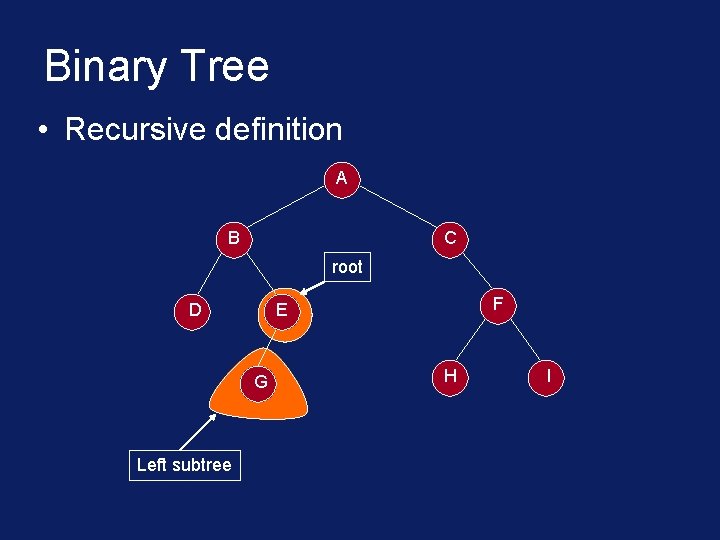
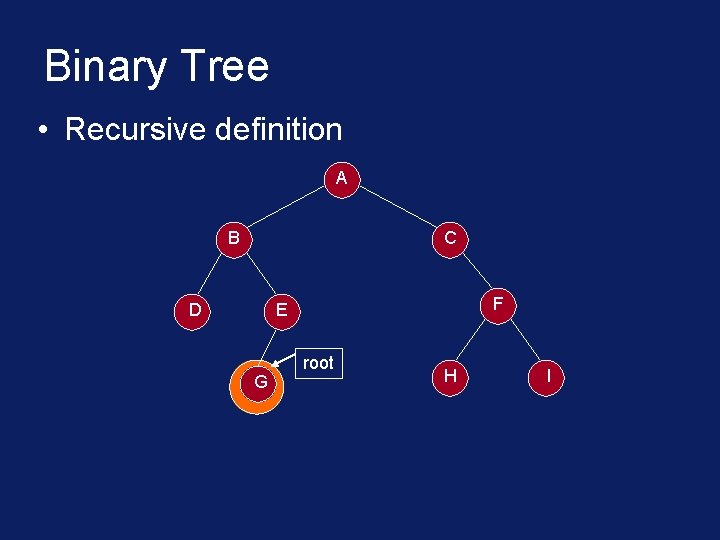
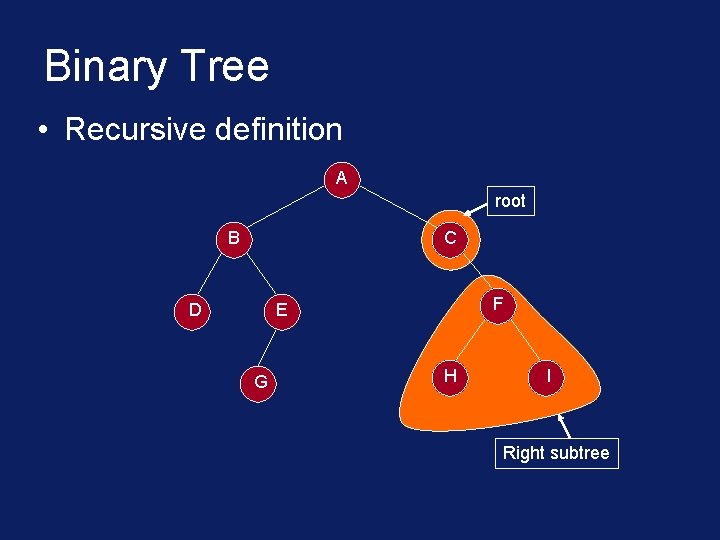
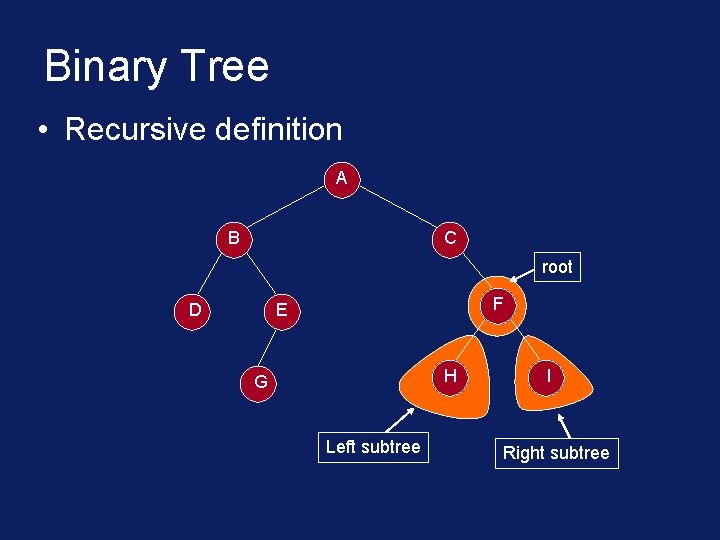
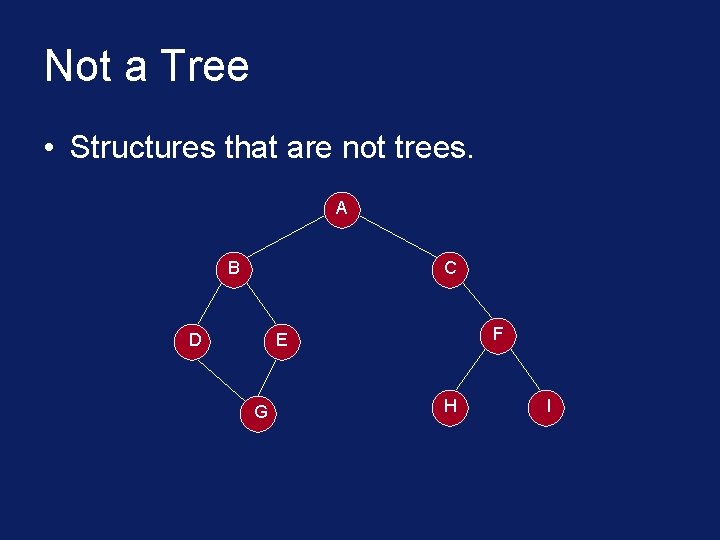
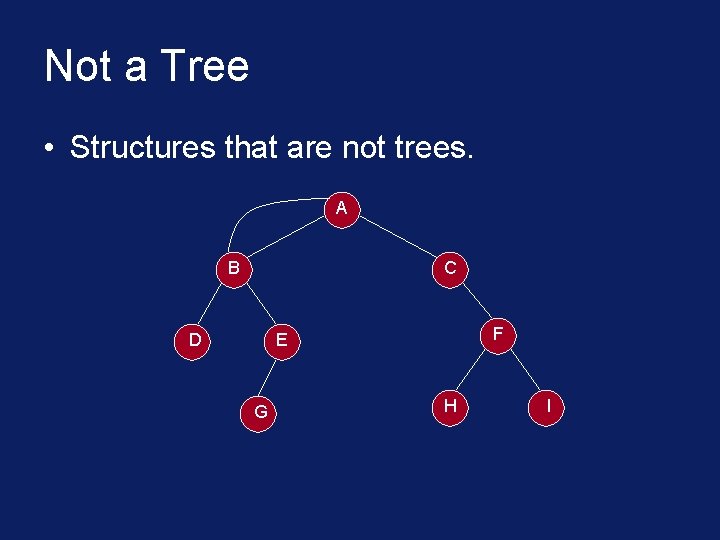
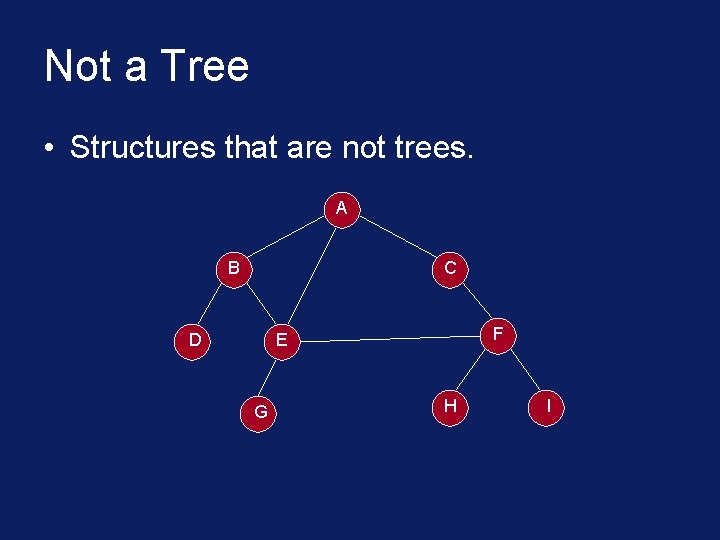
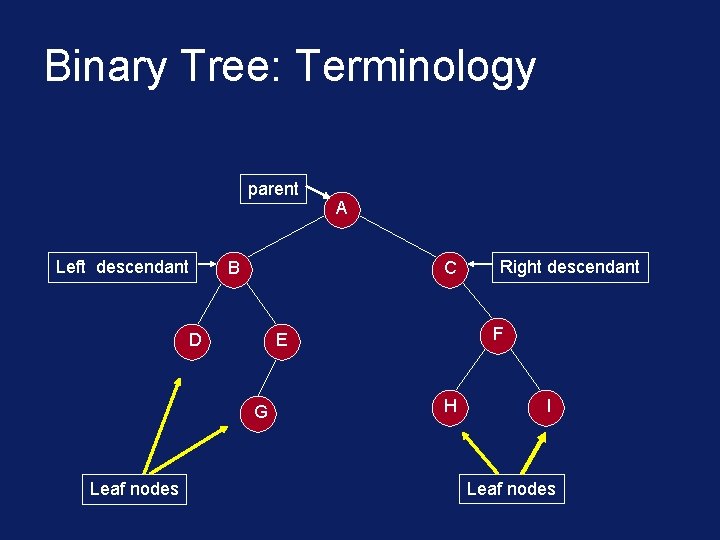
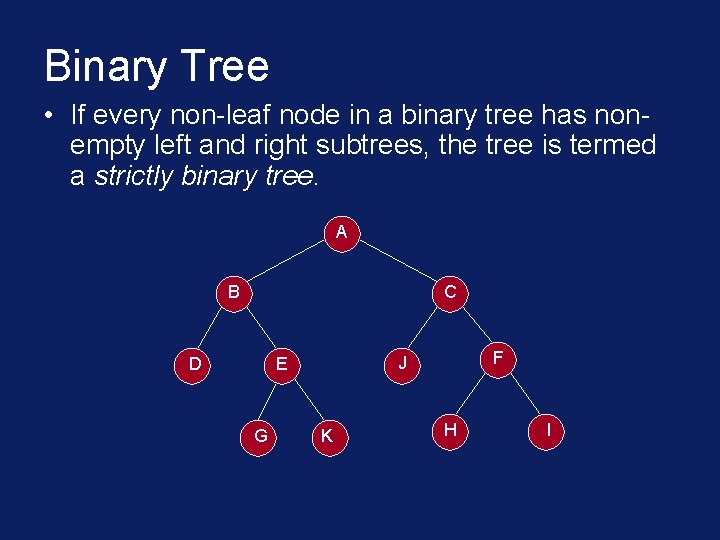
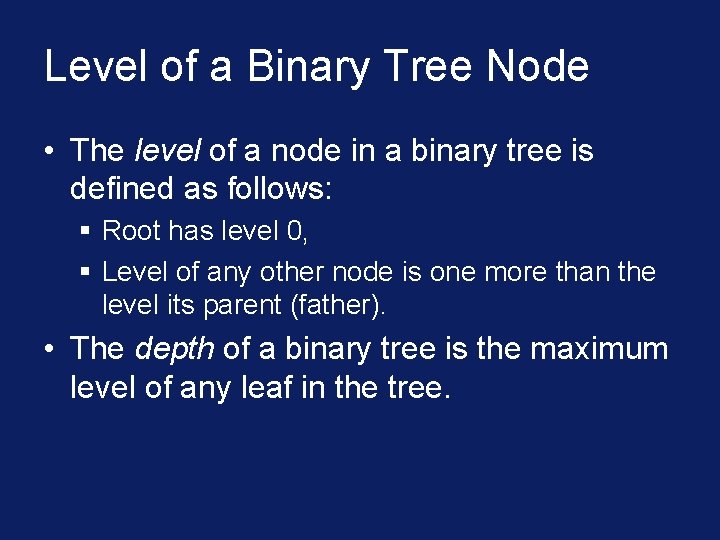
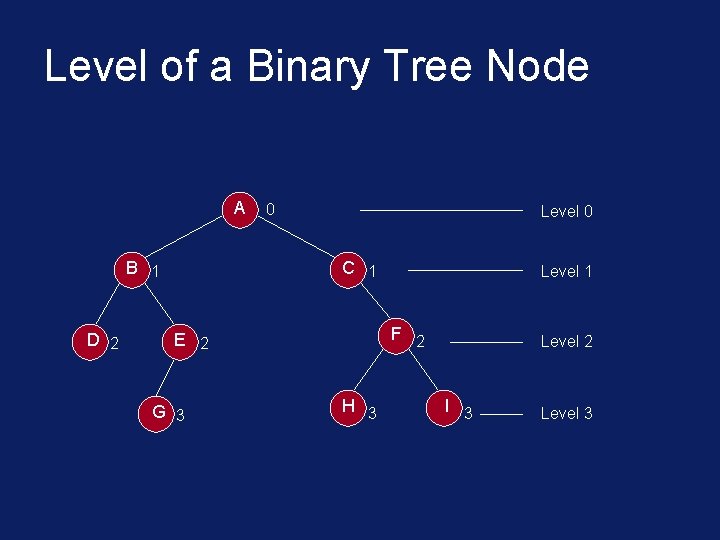
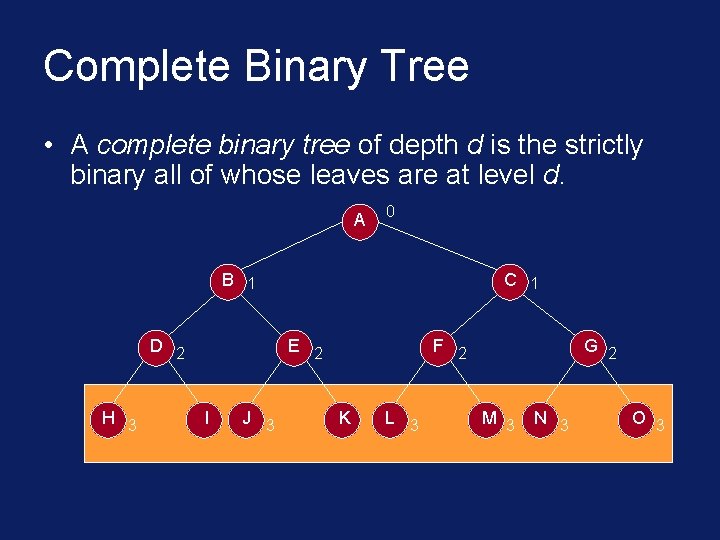
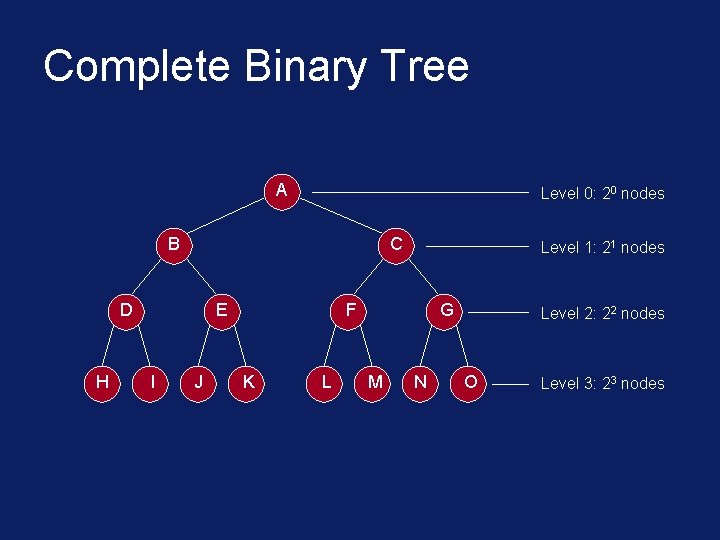
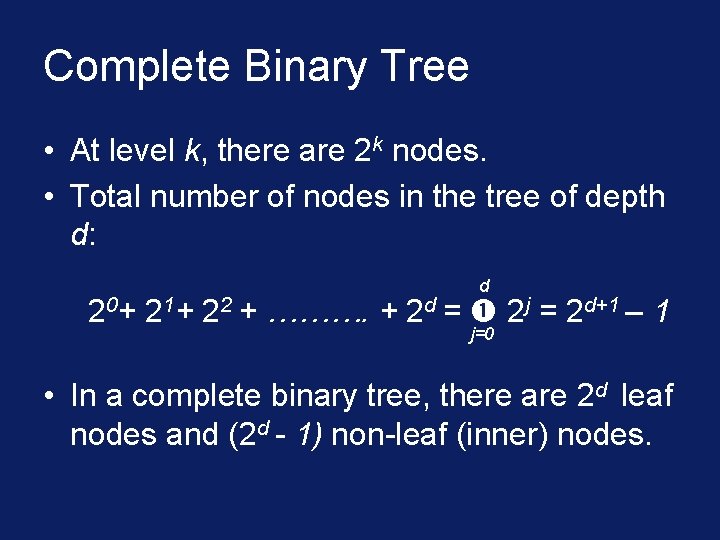
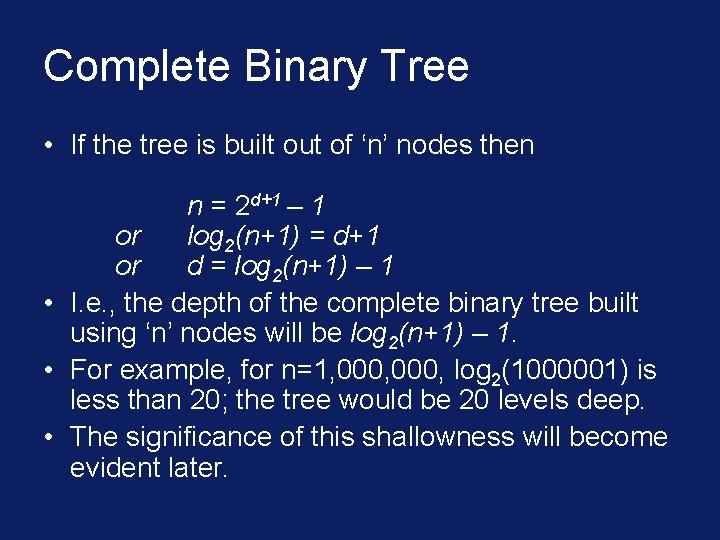
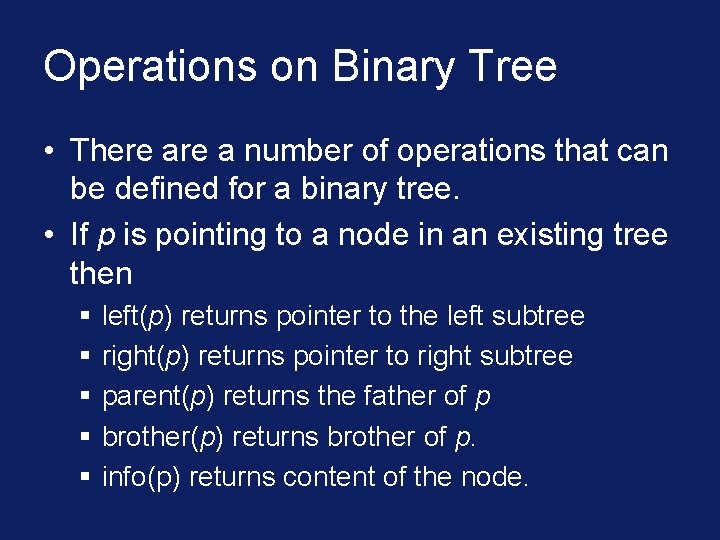
- Slides: 27
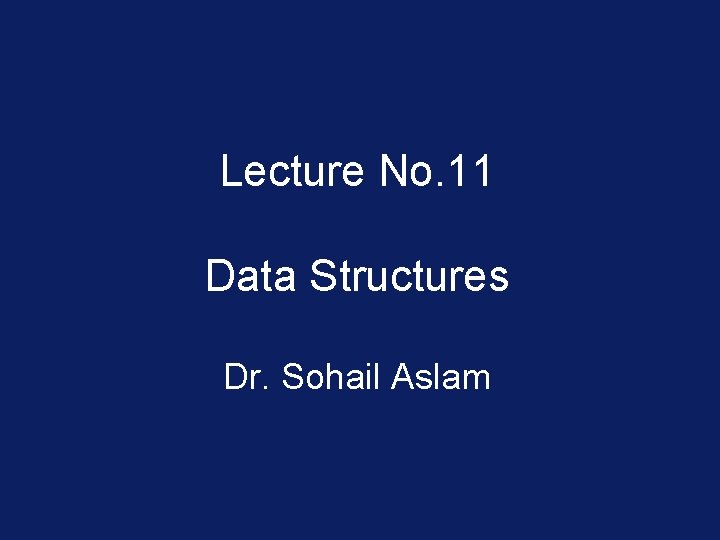
Lecture No. 11 Data Structures Dr. Sohail Aslam
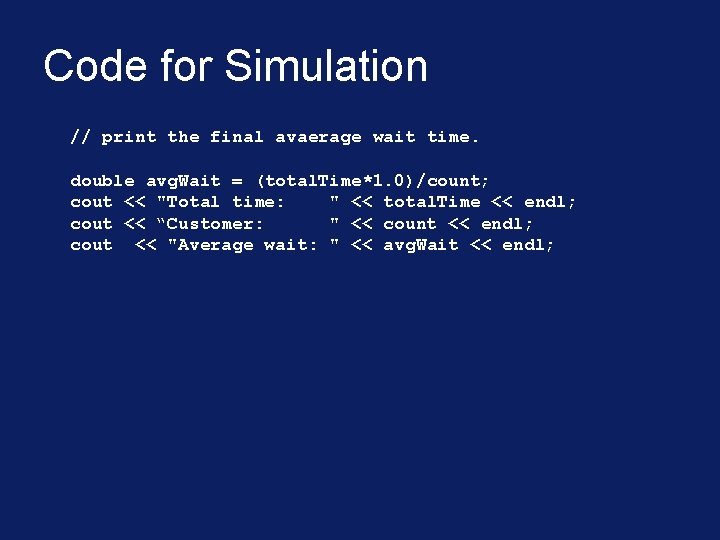
Code for Simulation // print the final avaerage wait time. double avg. Wait = (total. Time*1. 0)/count; cout << "Total time: " << total. Time << endl; cout << “Customer: " << count << endl; cout << "Average wait: " << avg. Wait << endl;
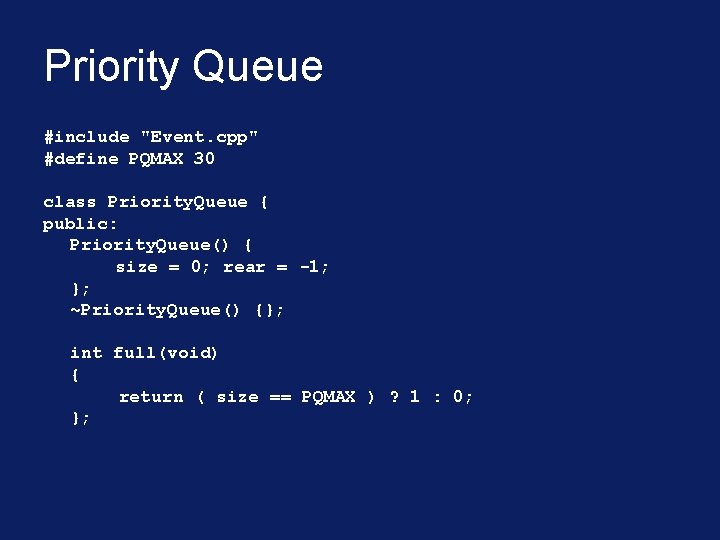
Priority Queue #include "Event. cpp" #define PQMAX 30 class Priority. Queue { public: Priority. Queue() { size = 0; rear = -1; }; ~Priority. Queue() {}; int full(void) { return ( size == PQMAX ) ? 1 : 0; };
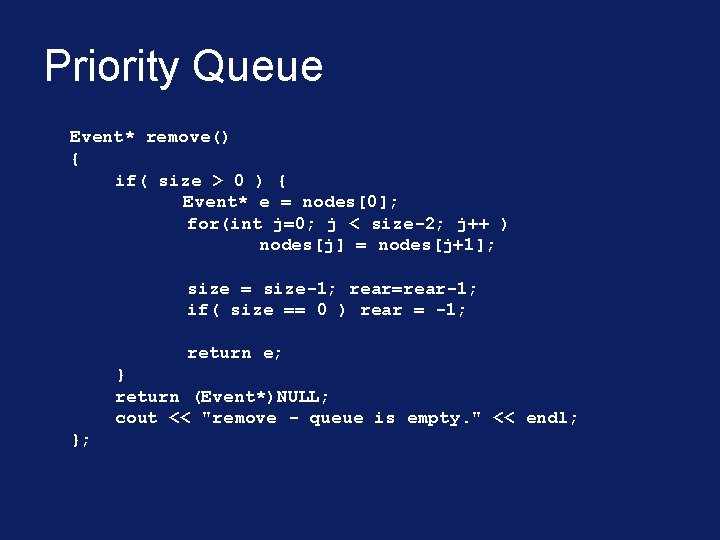
Priority Queue Event* remove() { if( size > 0 ) { Event* e = nodes[0]; for(int j=0; j < size-2; j++ ) nodes[j] = nodes[j+1]; size = size-1; rear=rear-1; if( size == 0 ) rear = -1; return e; } return (Event*)NULL; cout << "remove - queue is empty. " << endl; };
![Priority Queue int insertEvent e if full rear rear1 nodesrear Priority Queue int insert(Event* e) { if( !full() ) { rear = rear+1; nodes[rear]](https://slidetodoc.com/presentation_image_h2/6d97fc572a6eb74dd0a322d6a63a8fe2/image-5.jpg)
Priority Queue int insert(Event* e) { if( !full() ) { rear = rear+1; nodes[rear] = e; size = size + 1; sort. Elements(); // in ascending order return 1; } cout << "insert queue is full. " << endl; return 0; }; int length() { return size; }; };
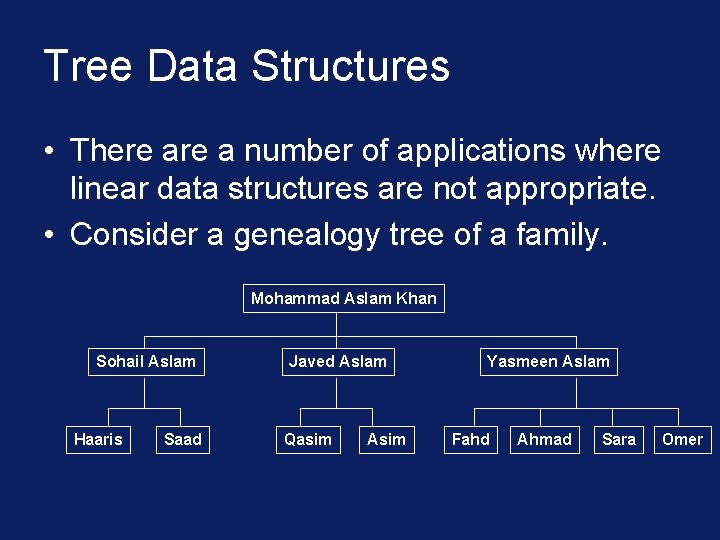
Tree Data Structures • There a number of applications where linear data structures are not appropriate. • Consider a genealogy tree of a family. Mohammad Aslam Khan Sohail Aslam Haaris Saad Javed Aslam Qasim Asim Yasmeen Aslam Fahd Ahmad Sara Omer
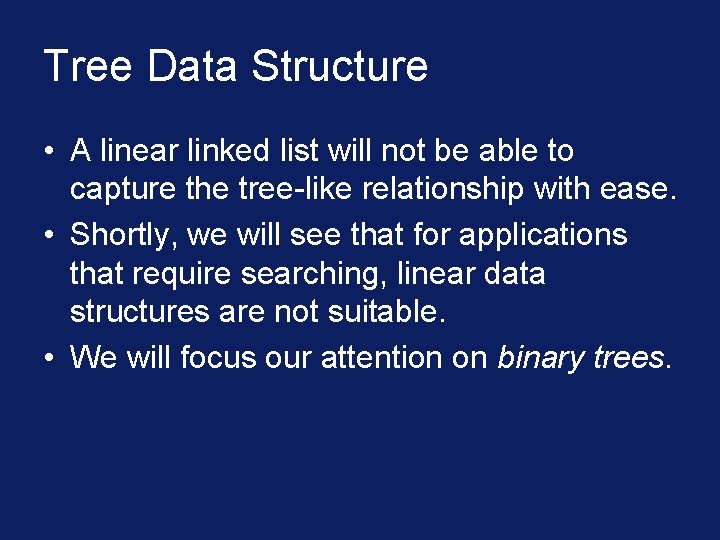
Tree Data Structure • A linear linked list will not be able to capture the tree-like relationship with ease. • Shortly, we will see that for applications that require searching, linear data structures are not suitable. • We will focus our attention on binary trees.
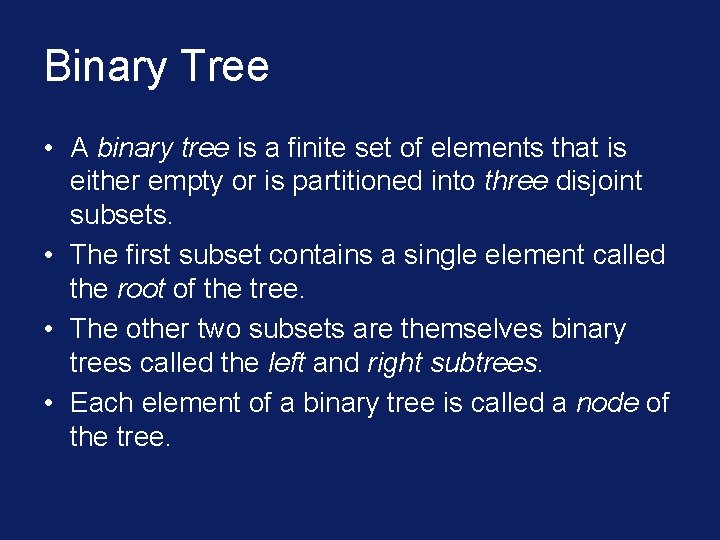
Binary Tree • A binary tree is a finite set of elements that is either empty or is partitioned into three disjoint subsets. • The first subset contains a single element called the root of the tree. • The other two subsets are themselves binary trees called the left and right subtrees. • Each element of a binary tree is called a node of the tree.
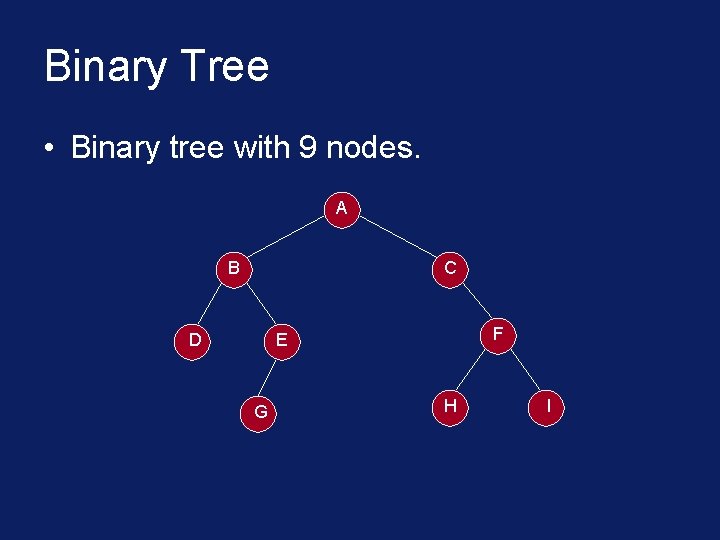
Binary Tree • Binary tree with 9 nodes. A B C D F E G H I
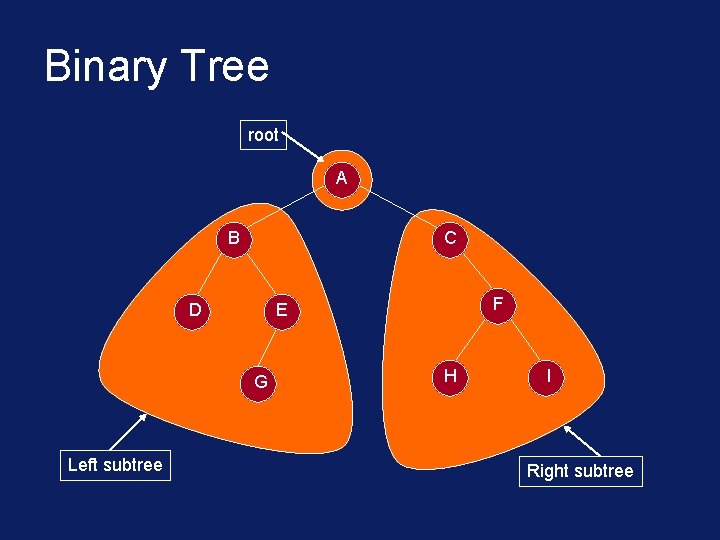
Binary Tree root A B C D G Left subtree F E H I Right subtree
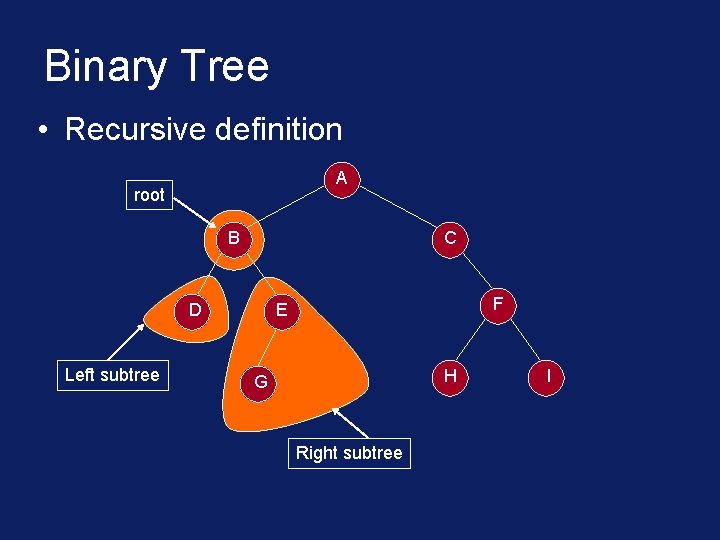
Binary Tree • Recursive definition A root B C D Left subtree F E H G Right subtree I
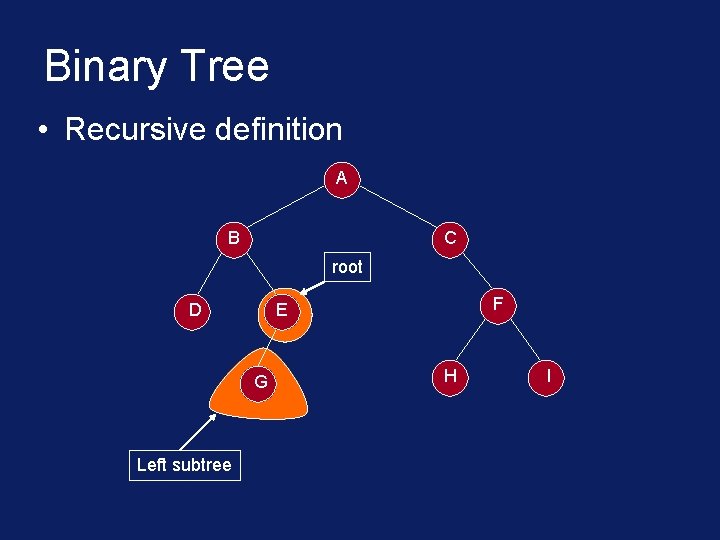
Binary Tree • Recursive definition A B C root D G Left subtree F E H I
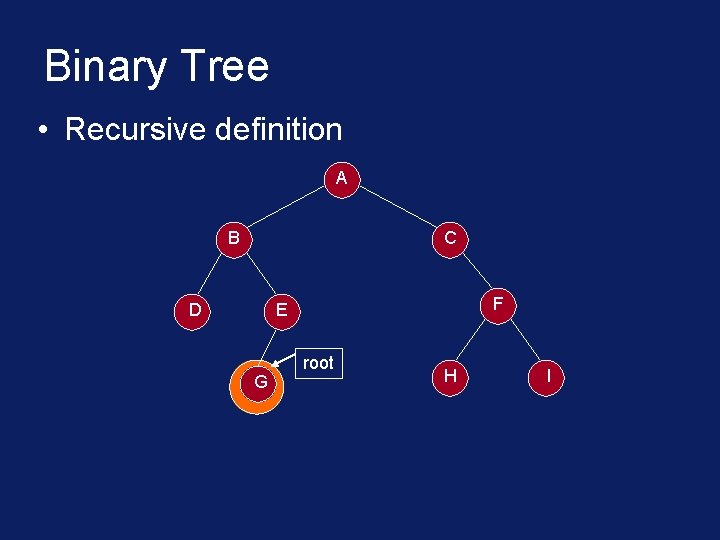
Binary Tree • Recursive definition A B C D F E G root H I
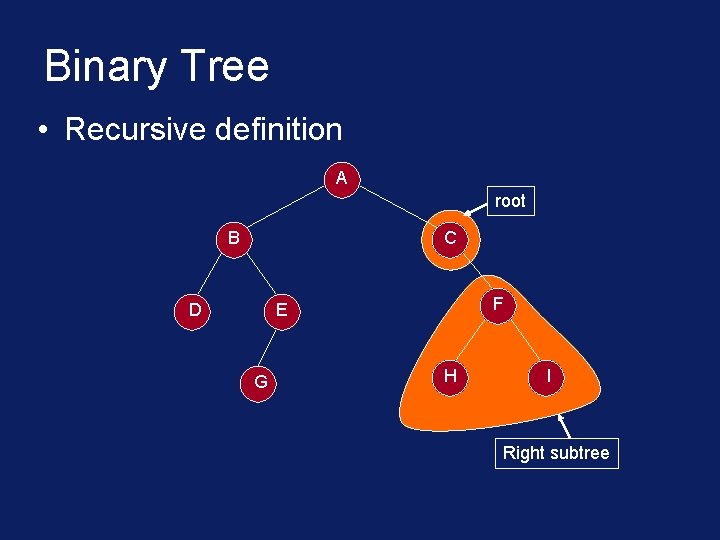
Binary Tree • Recursive definition A root B C D F E G H I Right subtree
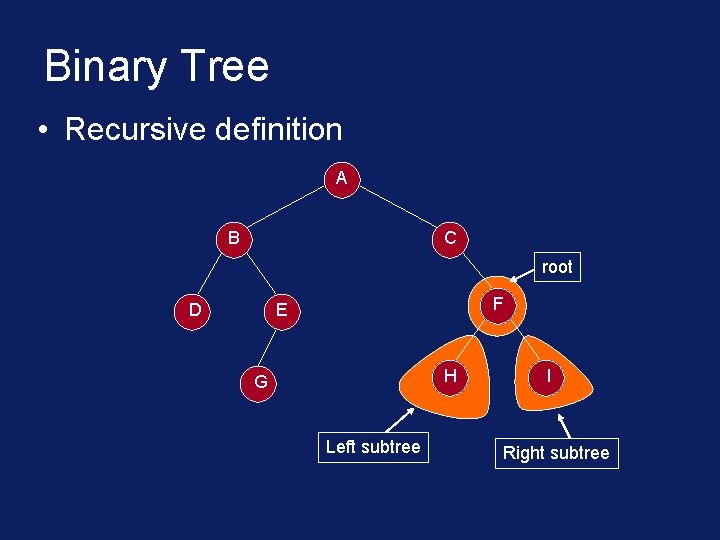
Binary Tree • Recursive definition A B C root D F E H G Left subtree I Right subtree
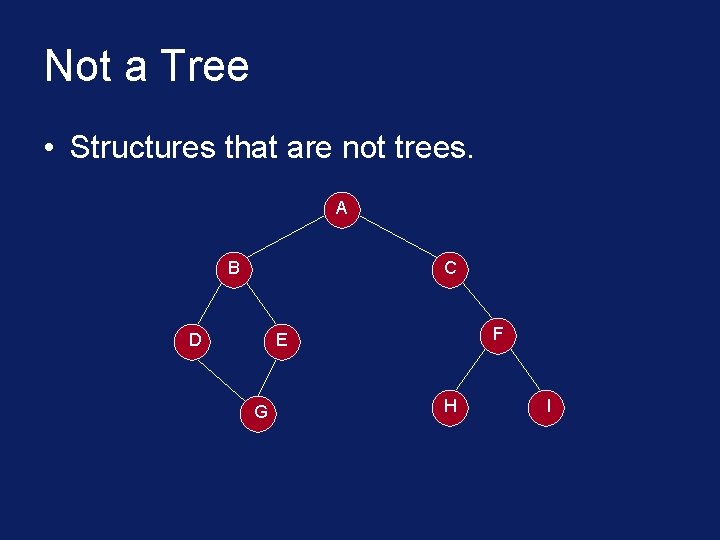
Not a Tree • Structures that are not trees. A B C D F E G H I
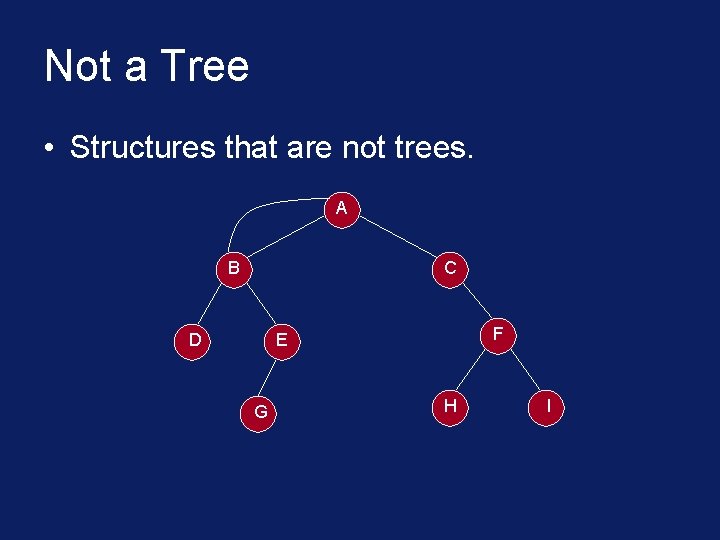
Not a Tree • Structures that are not trees. A B C D F E G H I
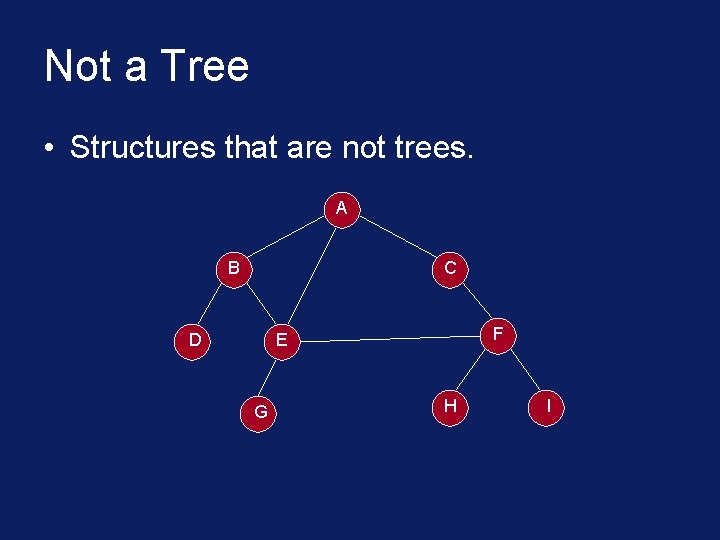
Not a Tree • Structures that are not trees. A B C D F E G H I
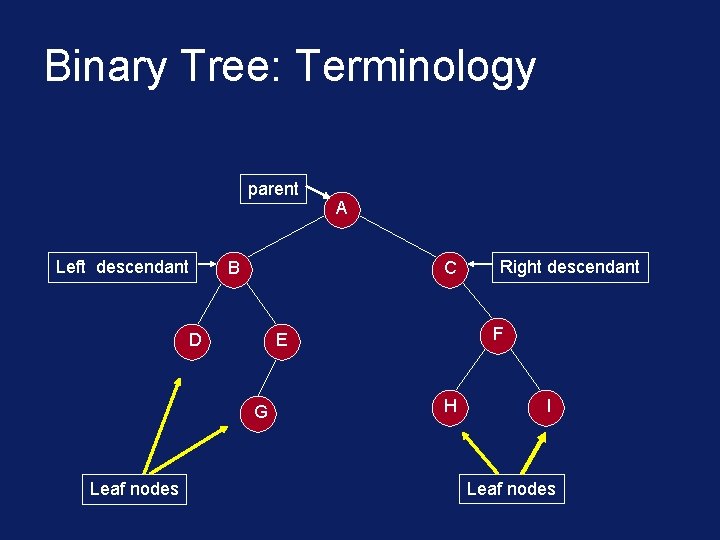
Binary Tree: Terminology parent Left descendant B C D Right descendant F E G Leaf nodes A H I Leaf nodes
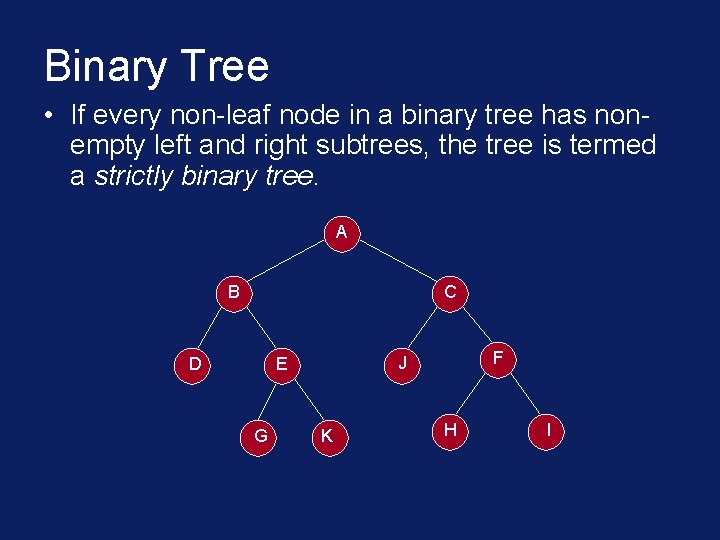
Binary Tree • If every non-leaf node in a binary tree has nonempty left and right subtrees, the tree is termed a strictly binary tree. A B C D G F J E K H I
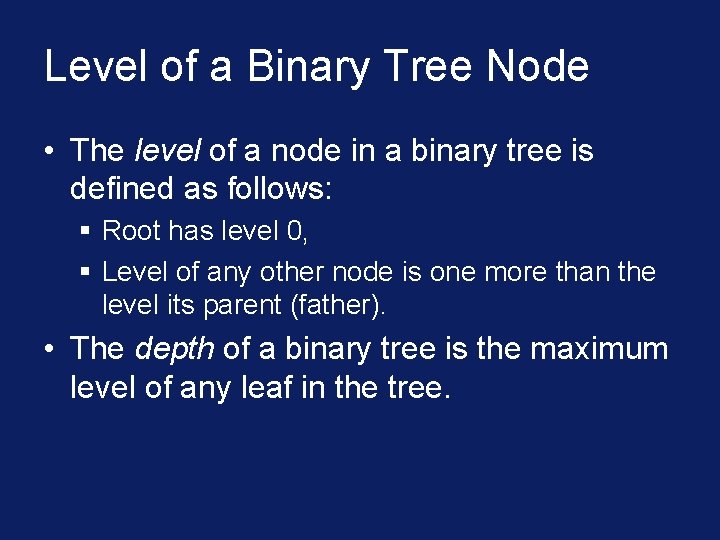
Level of a Binary Tree Node • The level of a node in a binary tree is defined as follows: § Root has level 0, § Level of any other node is one more than the level its parent (father). • The depth of a binary tree is the maximum level of any leaf in the tree.
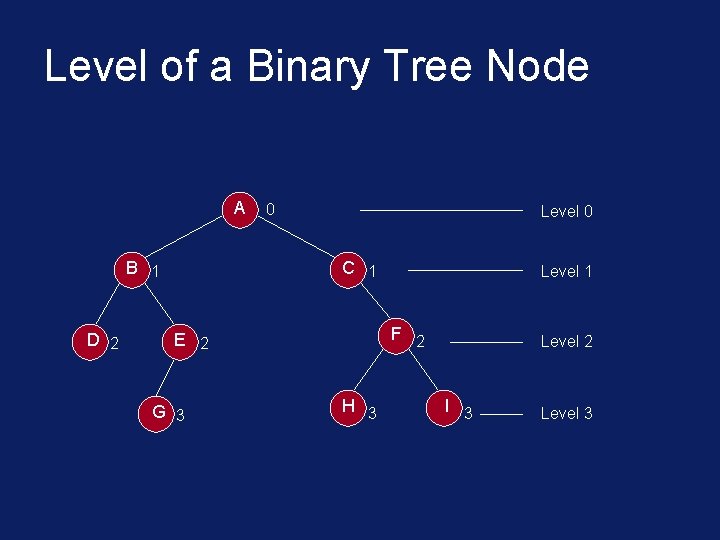
Level of a Binary Tree Node A B 1 D 2 0 Level 0 C 1 F 2 E 2 G 3 Level 1 H 3 Level 2 I 3 Level 3
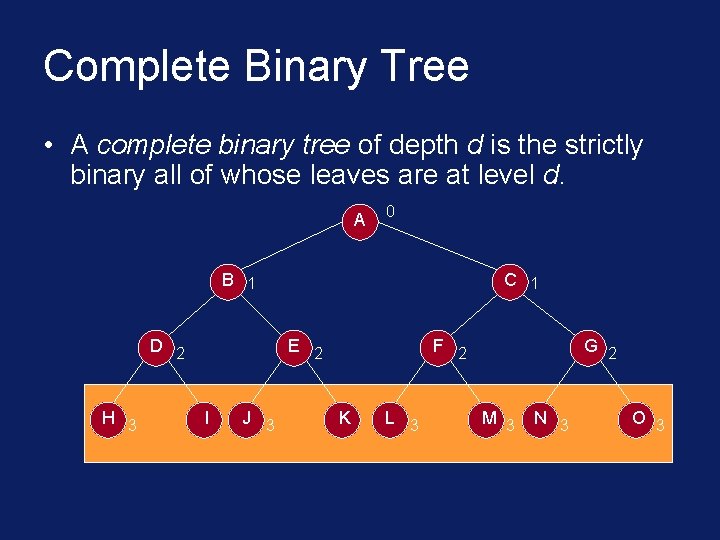
Complete Binary Tree • A complete binary tree of depth d is the strictly binary all of whose leaves are at level d. A 0 B 1 D 2 H 3 C 1 E 2 I J 3 F 2 K L 3 G 2 M 3 N 3 O 3
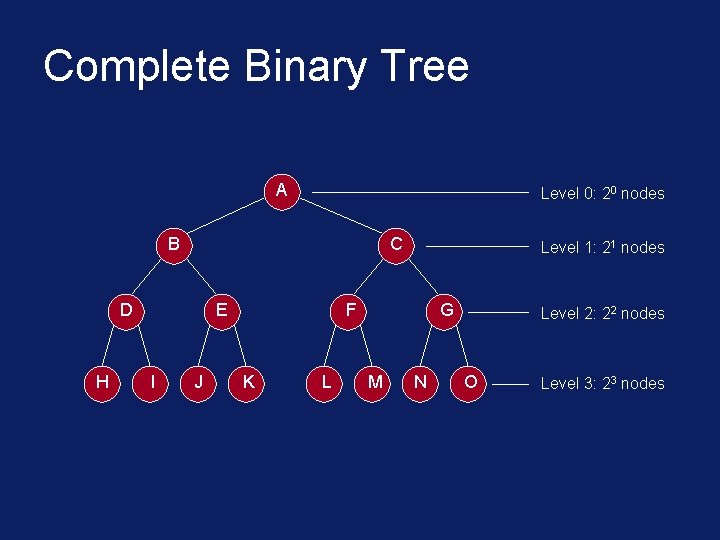
Complete Binary Tree A Level 0: 20 nodes B C D H E I J Level 1: 21 nodes F K L G M N Level 2: 22 nodes O Level 3: 23 nodes
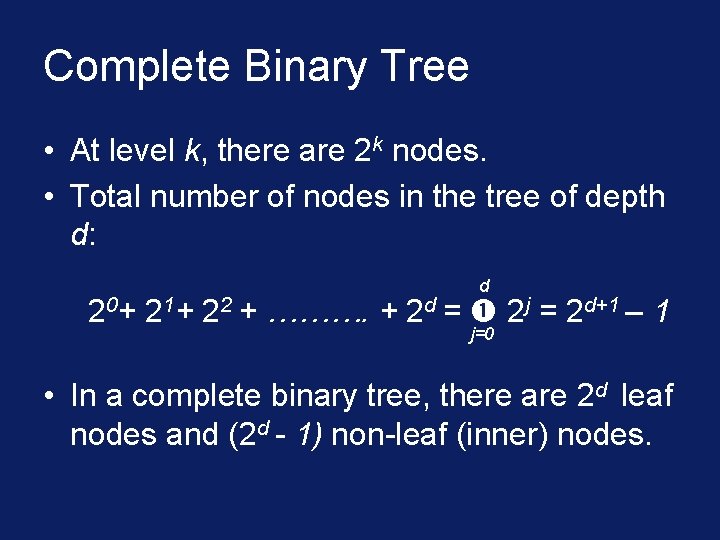
Complete Binary Tree • At level k, there are 2 k nodes. • Total number of nodes in the tree of depth d: d 20+ 21+ 22 + ………. + 2 d = 2 j = 2 d+1 – 1 j=0 • In a complete binary tree, there are 2 d leaf nodes and (2 d - 1) non-leaf (inner) nodes.
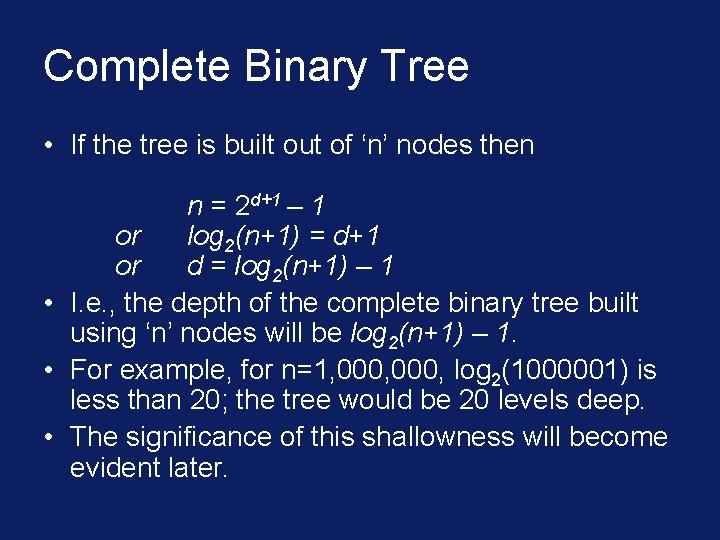
Complete Binary Tree • If the tree is built out of ‘n’ nodes then n = 2 d+1 – 1 or log 2(n+1) = d+1 or d = log 2(n+1) – 1 • I. e. , the depth of the complete binary tree built using ‘n’ nodes will be log 2(n+1) – 1. • For example, for n=1, 000, log 2(1000001) is less than 20; the tree would be 20 levels deep. • The significance of this shallowness will become evident later.
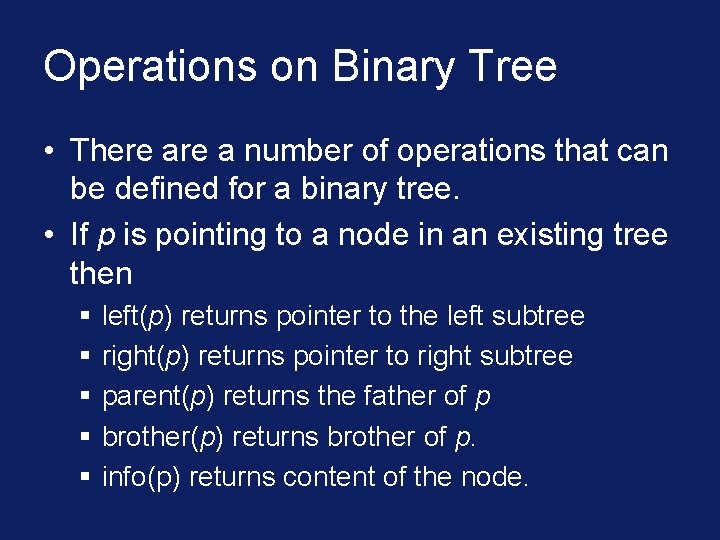
Operations on Binary Tree • There a number of operations that can be defined for a binary tree. • If p is pointing to a node in an existing tree then § § § left(p) returns pointer to the left subtree right(p) returns pointer to right subtree parent(p) returns the father of p brother(p) returns brother of p. info(p) returns content of the node.
Dr sohail aslam
Dr sohail lectures
Dian laundry
Aslam has to study four books
Hivnet
01:640:244 lecture notes - lecture 15: plat, idah, farad
Homologous structures definition
Exploratory data analysis lecture notes
Bayesian classification in data mining lecture notes
Data mining lecture notes
Data visualization lecture
Data mining lecture notes
Data mining lecture notes
Btechsmartclasses
R data structures
Oblivious data structures
Linux kernel data structures
Introduction to data structures
Introduction to data structures
Data structures and algorithms iit bombay
Esoteric data structures
Geometric data structures
Amit agarwal princeton
Data structures and algorithms tutorial
What is hadoop i/o
Explain single pass macro processor
Advanced data structures in java
Assembler data structures