Lecture 6 YACC and Syntax Directed Translation CS
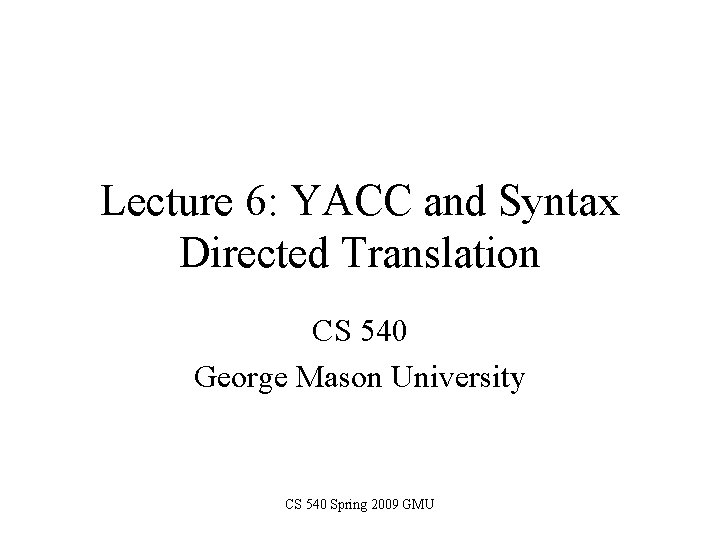
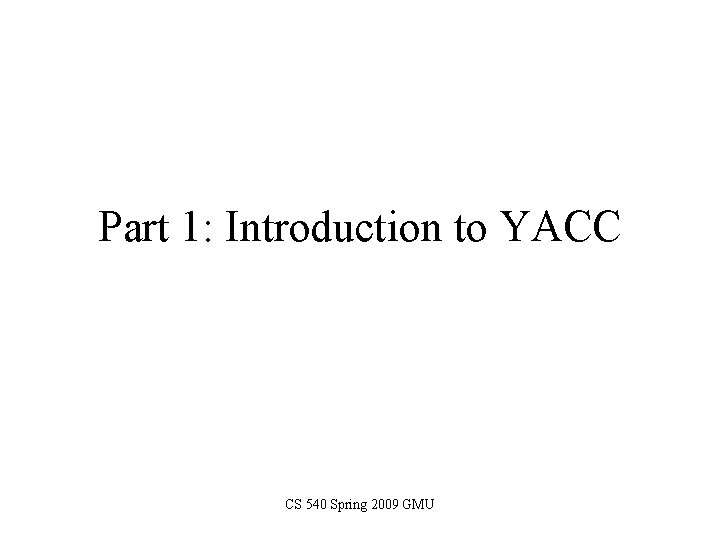
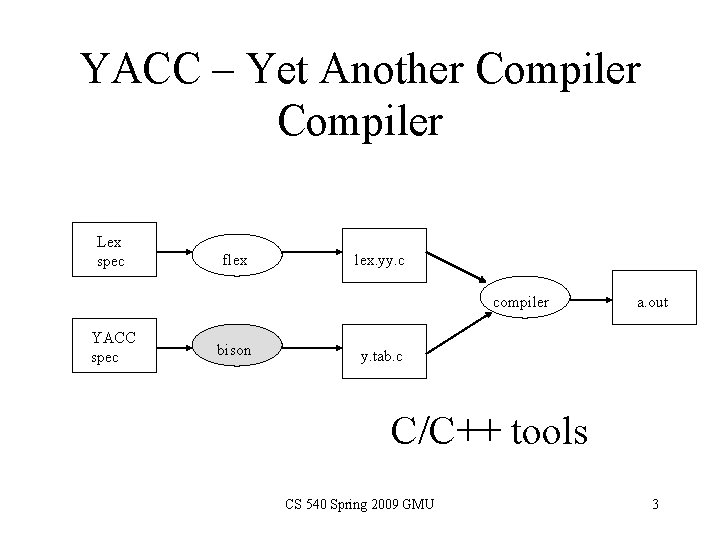
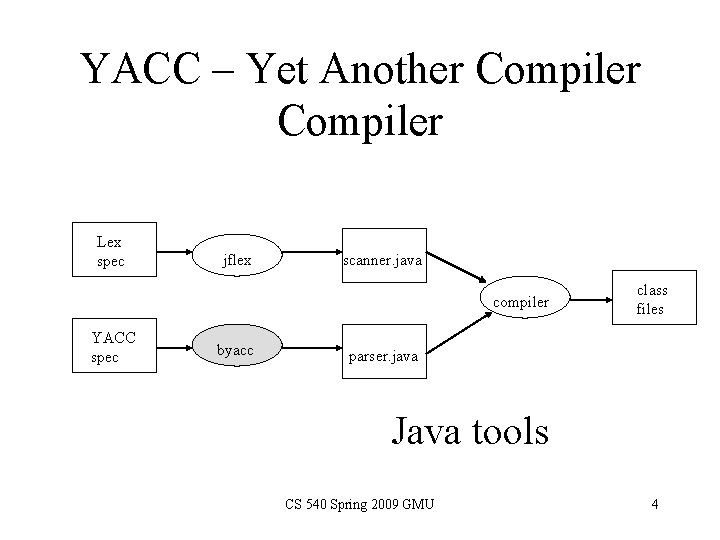
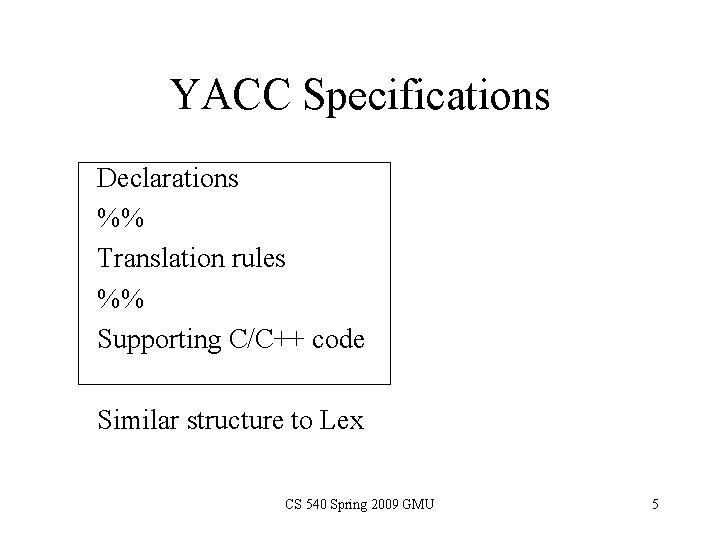
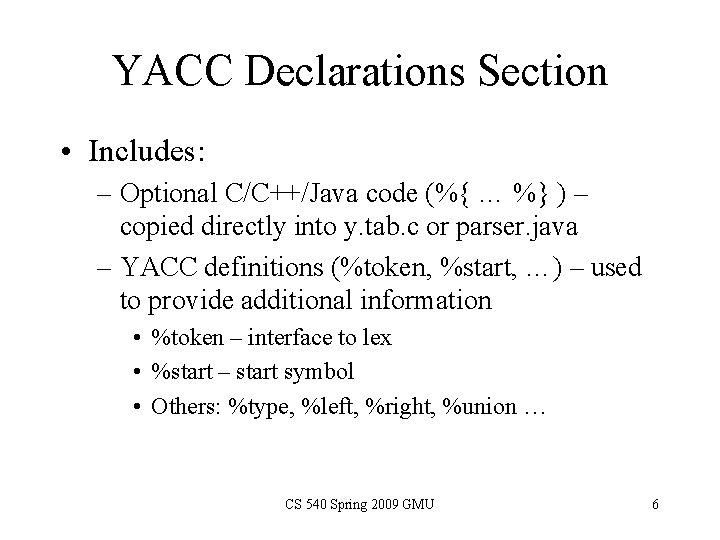
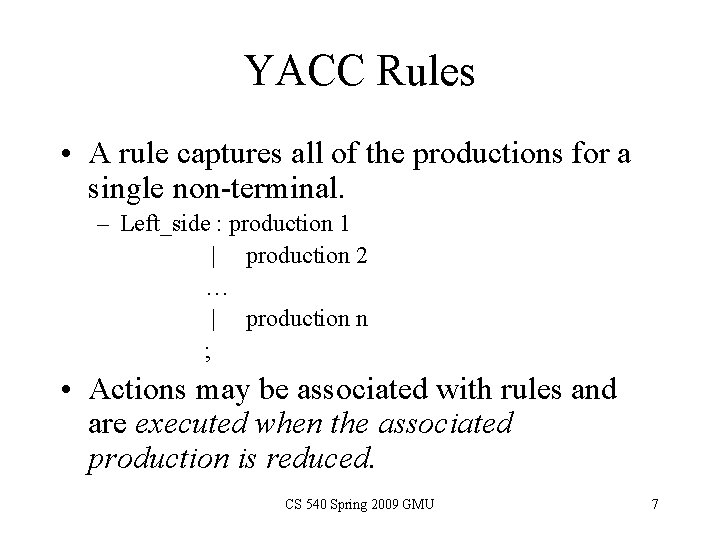
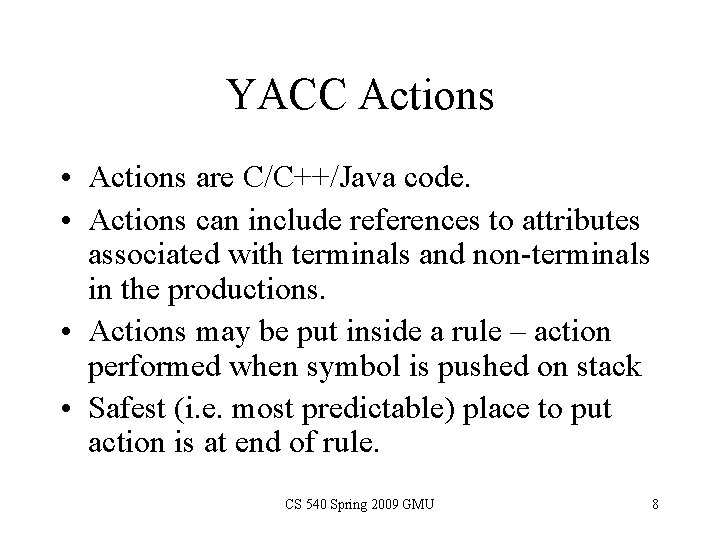
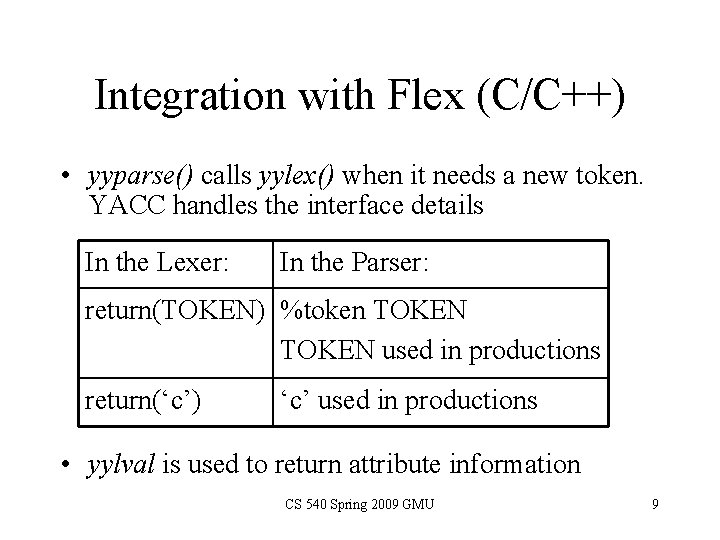
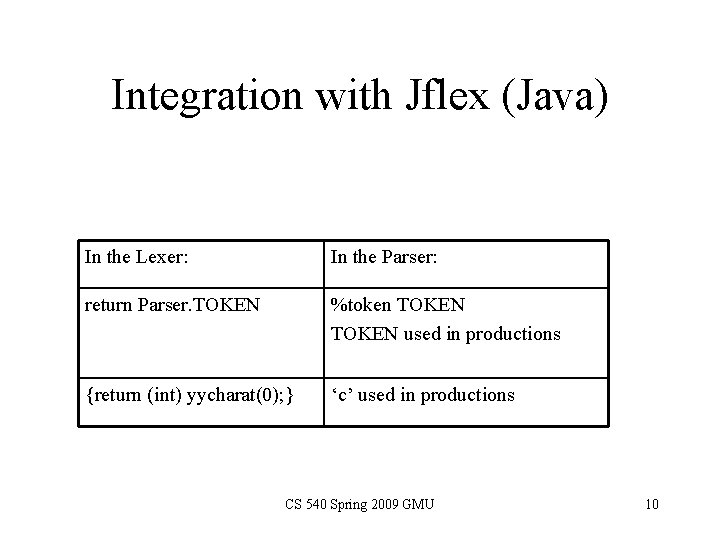
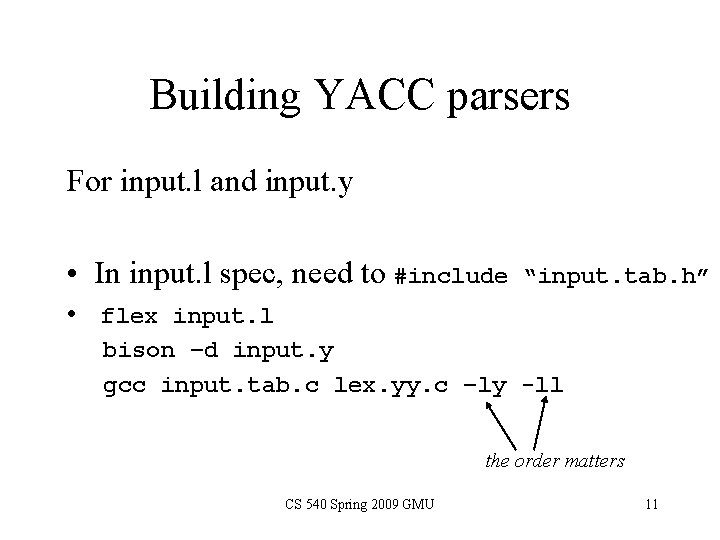
![Basic Lex/YACC example %{ #include “sample. tab. h” %} %% [a-z. A-Z]+ {return(NAME); } Basic Lex/YACC example %{ #include “sample. tab. h” %} %% [a-z. A-Z]+ {return(NAME); }](https://slidetodoc.com/presentation_image_h2/0d0f88dfb68db9c794658f8d7f762d86/image-12.jpg)
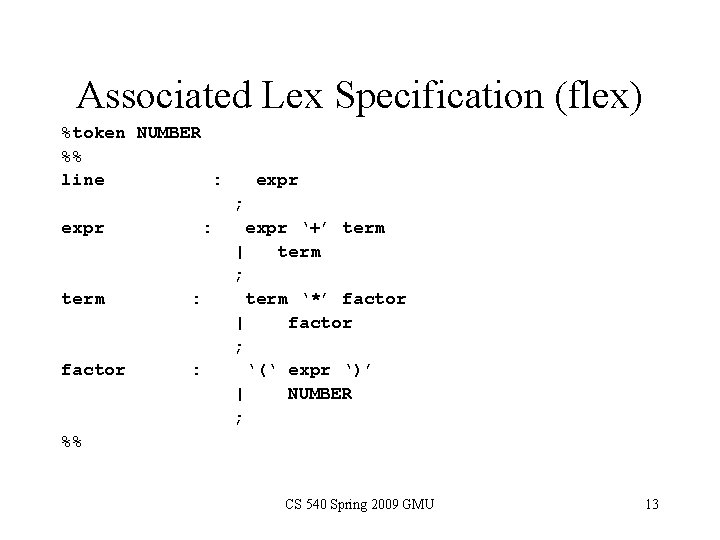
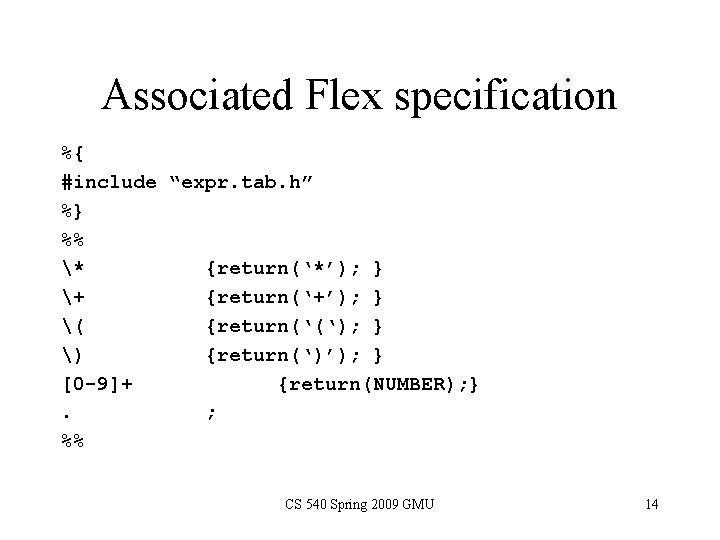
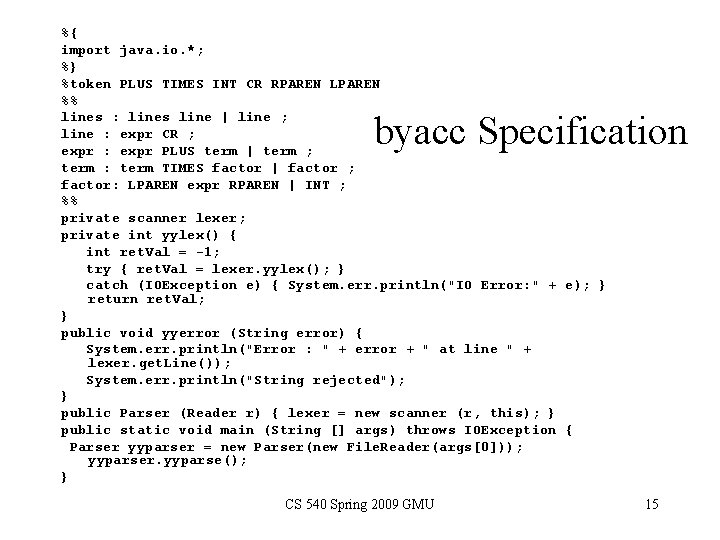
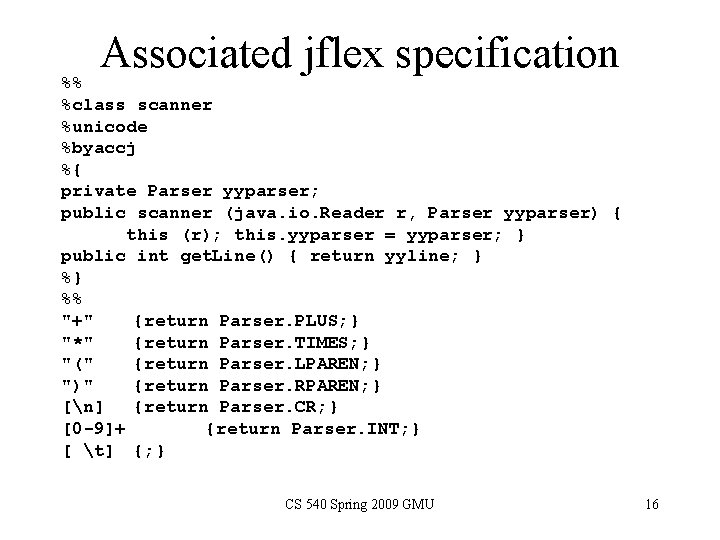
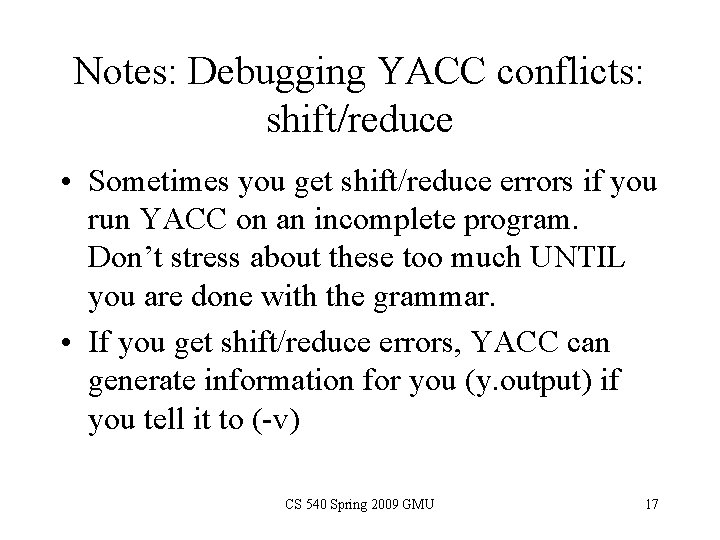
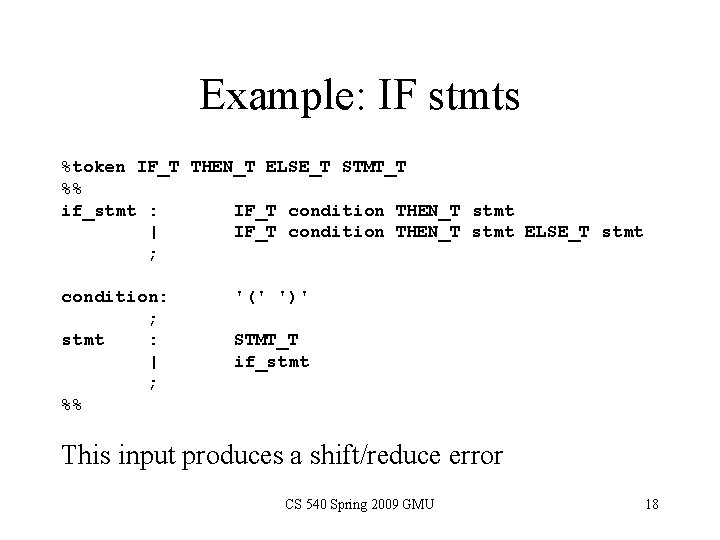
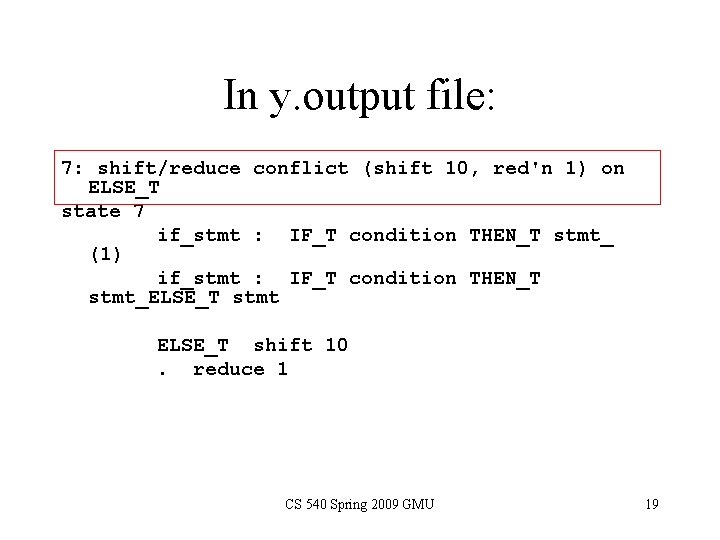
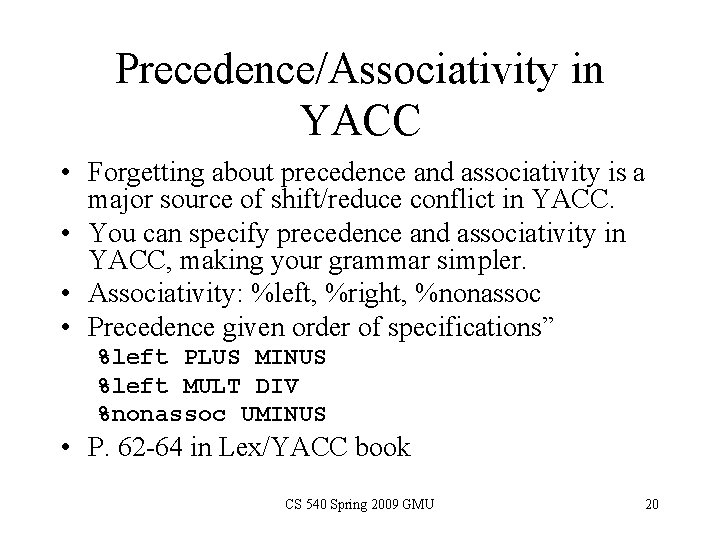
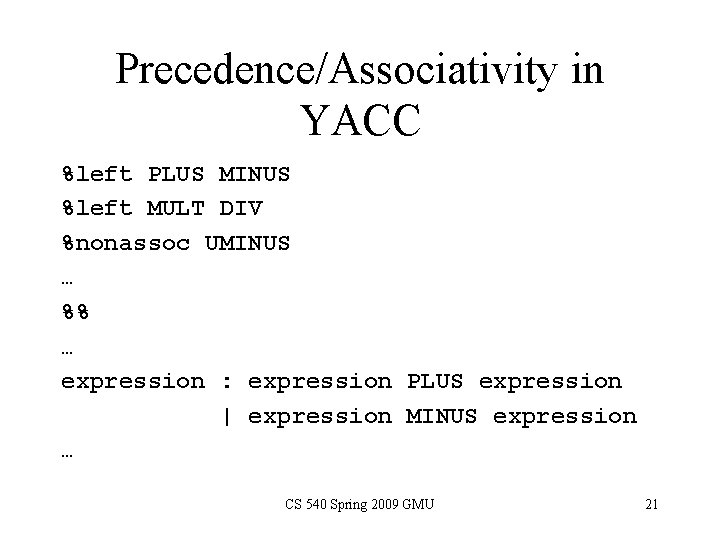
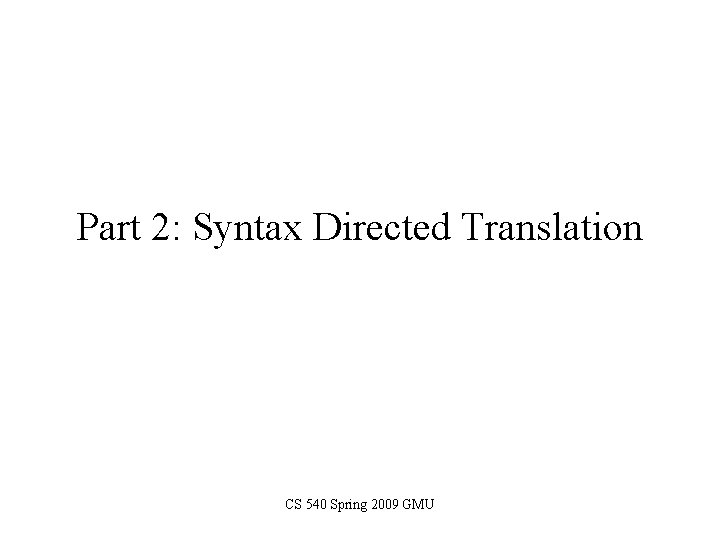
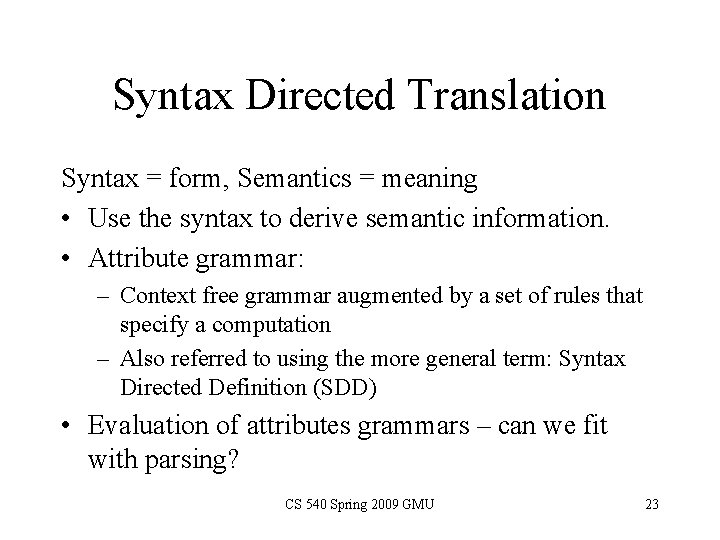
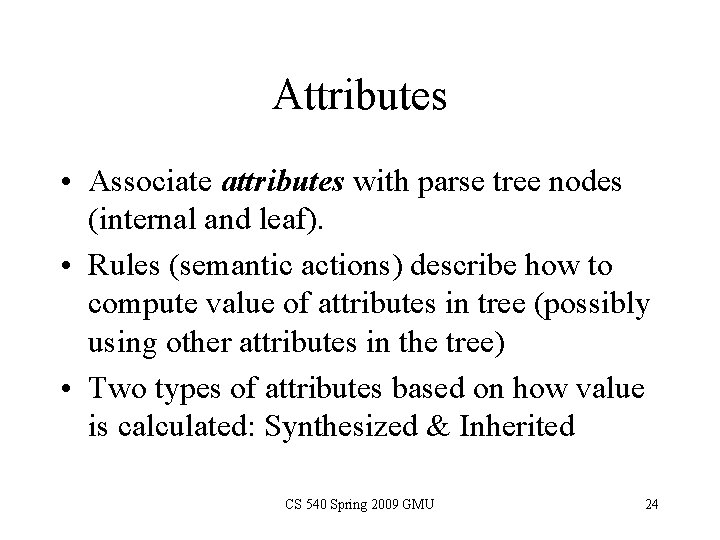
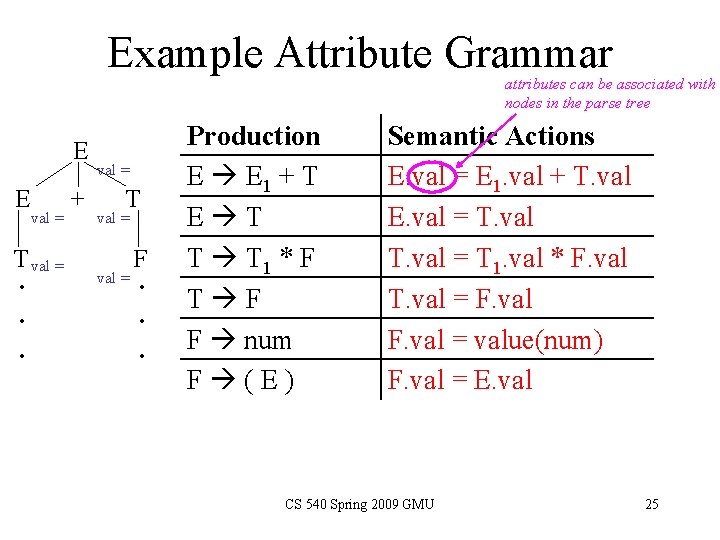
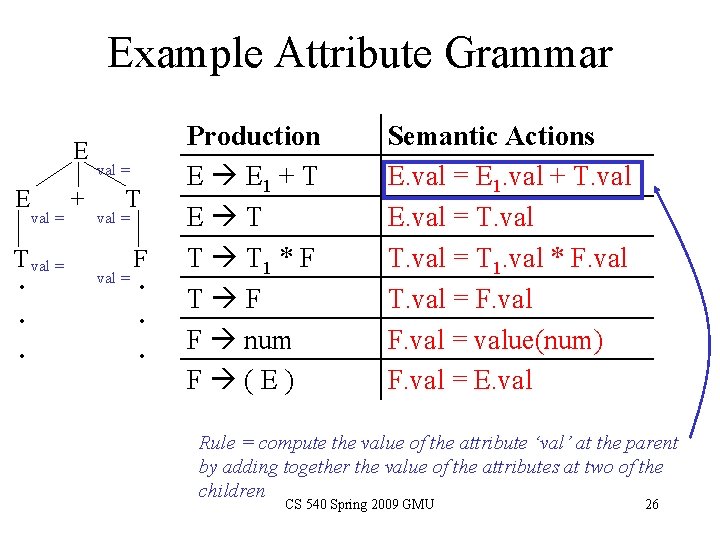
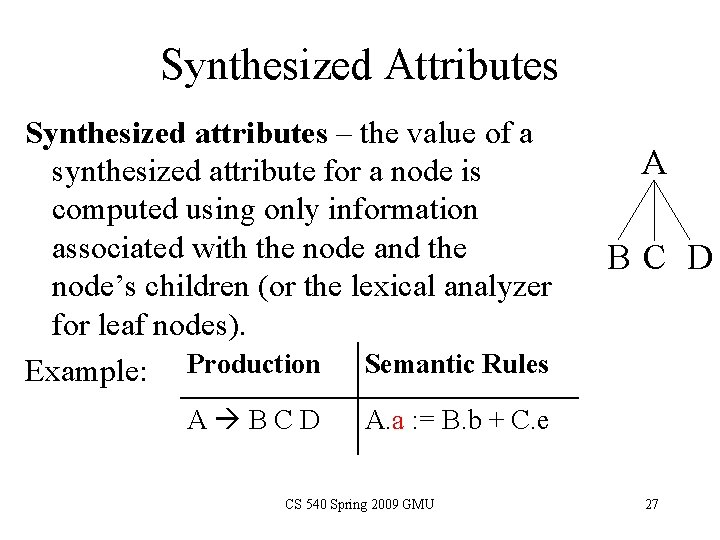
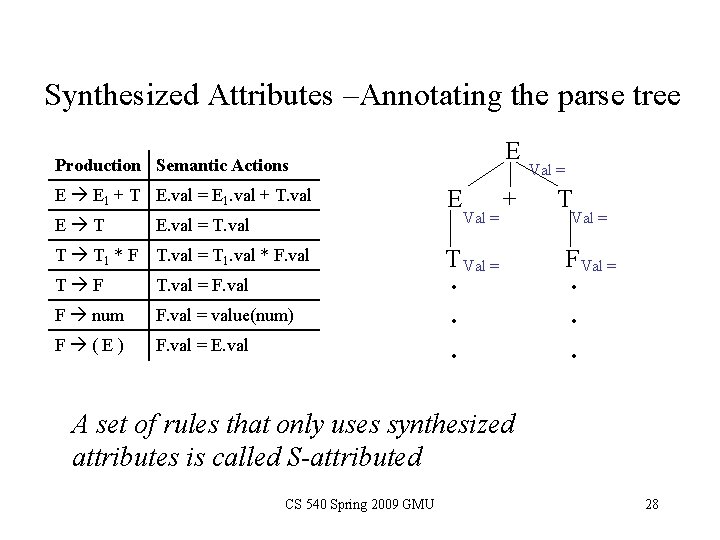
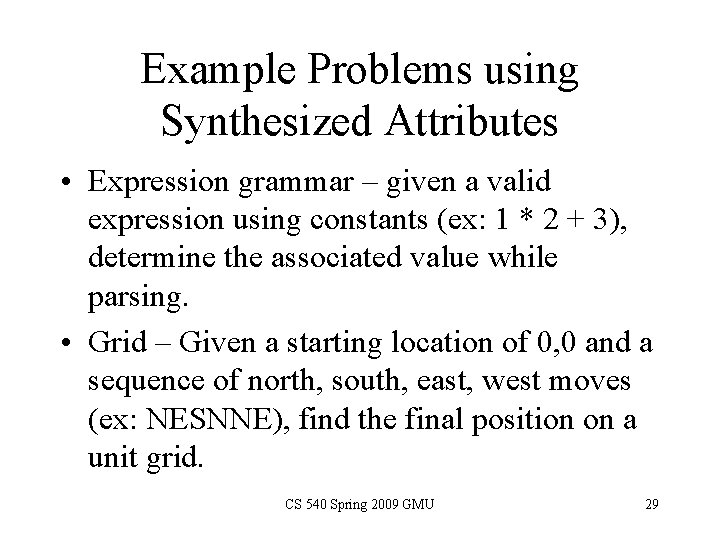
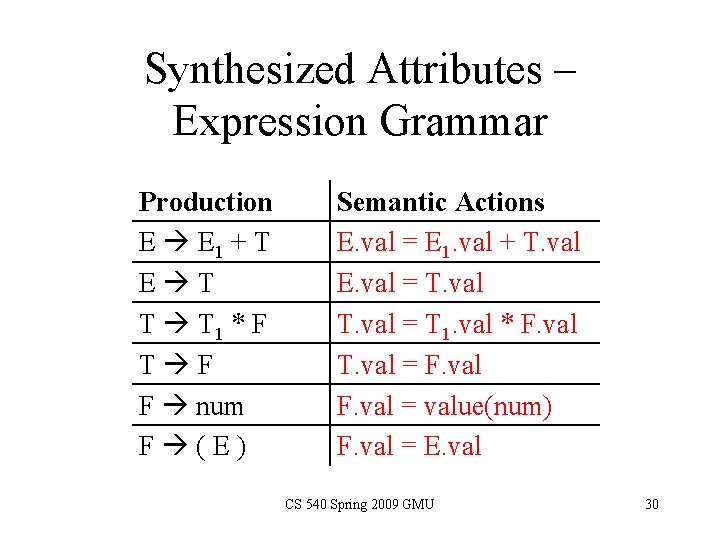
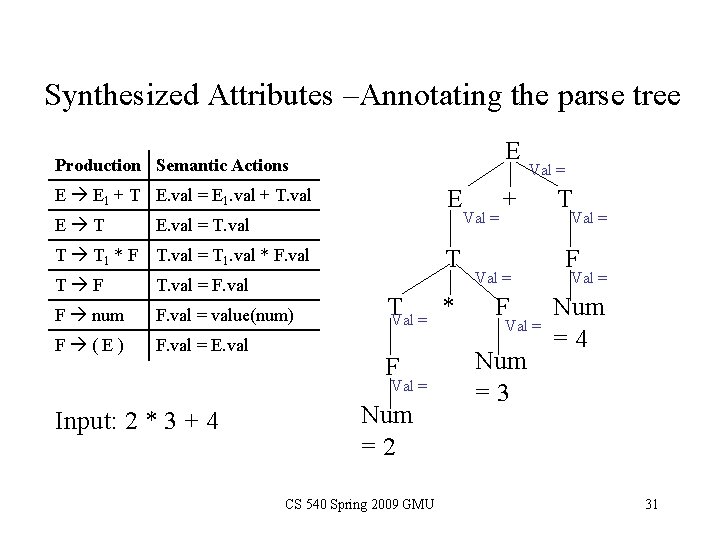
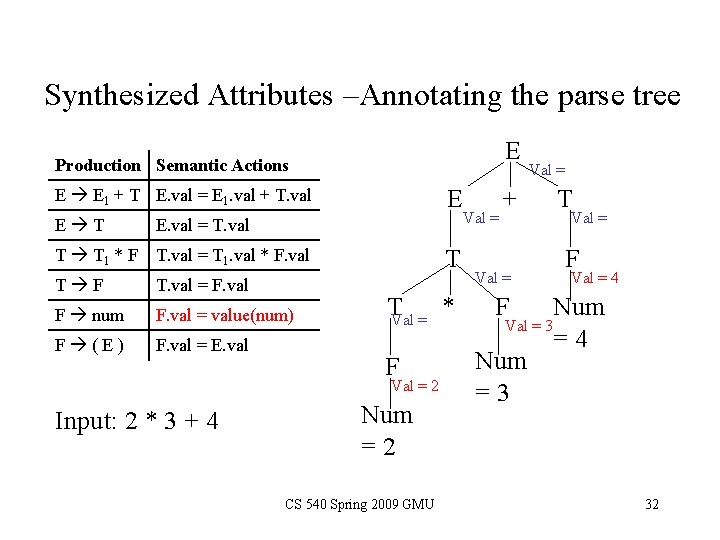
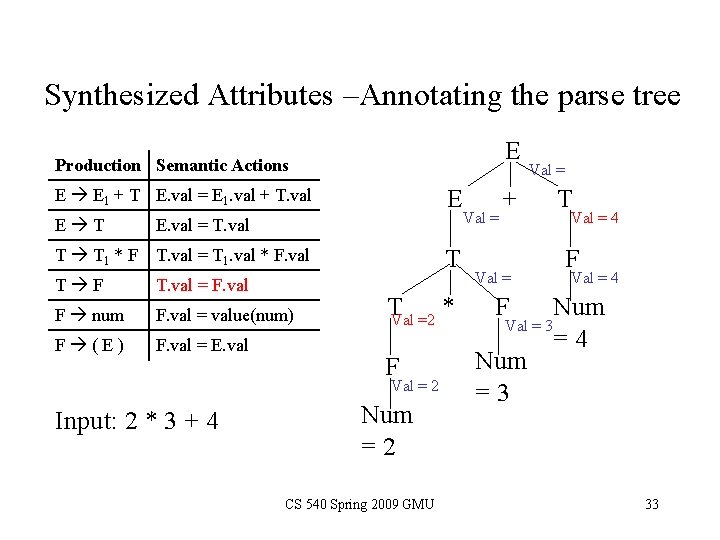
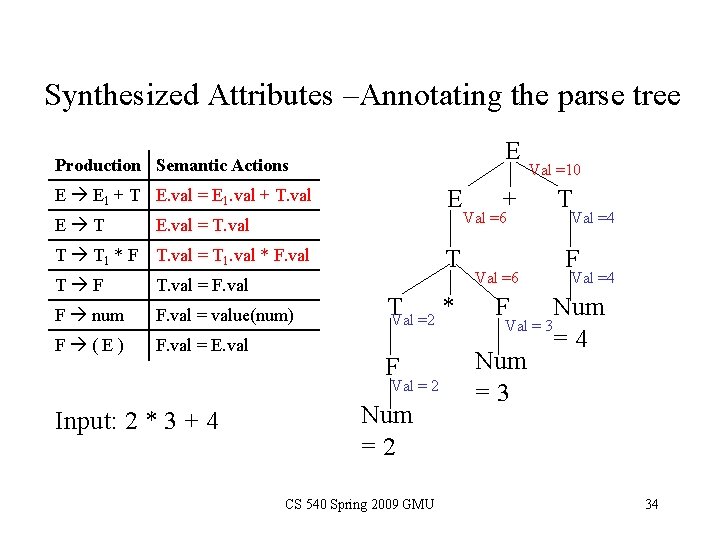
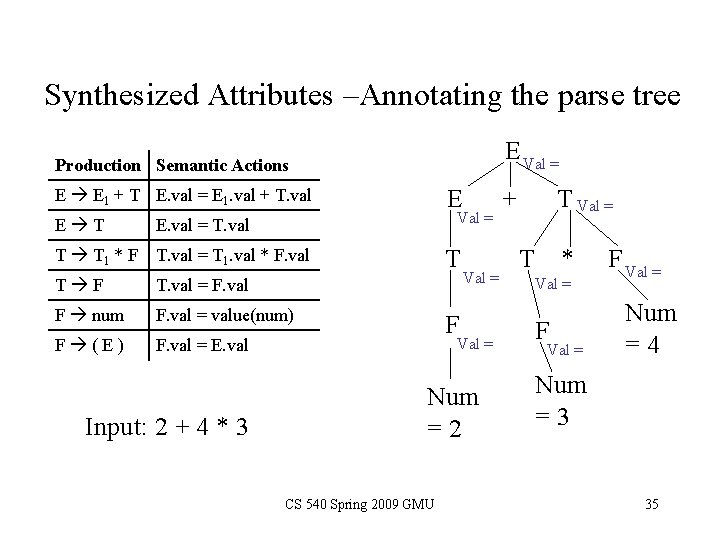
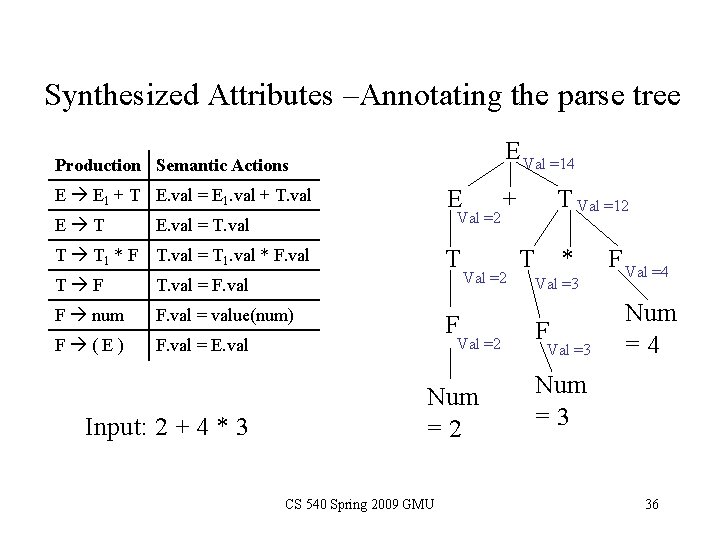
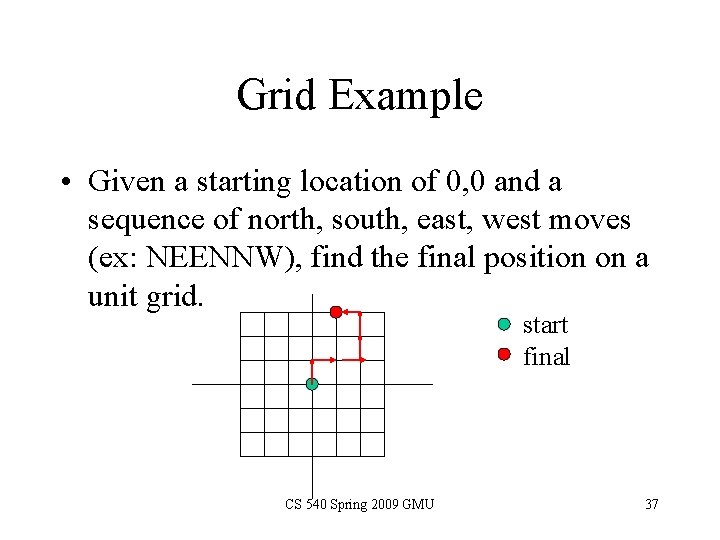
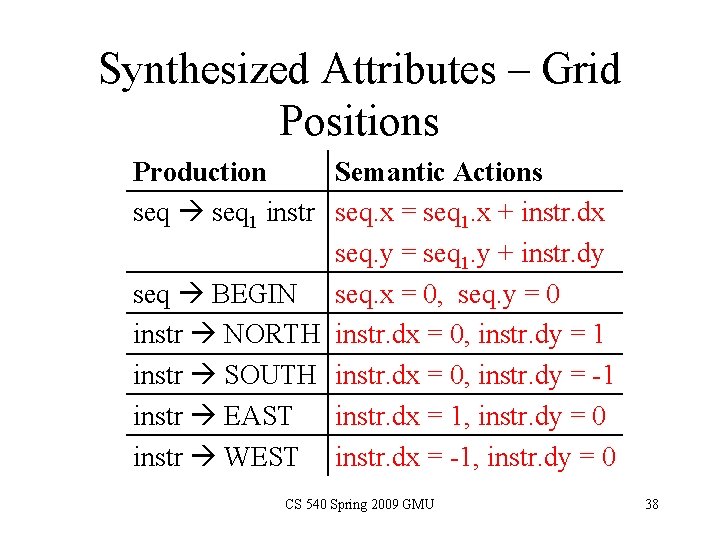
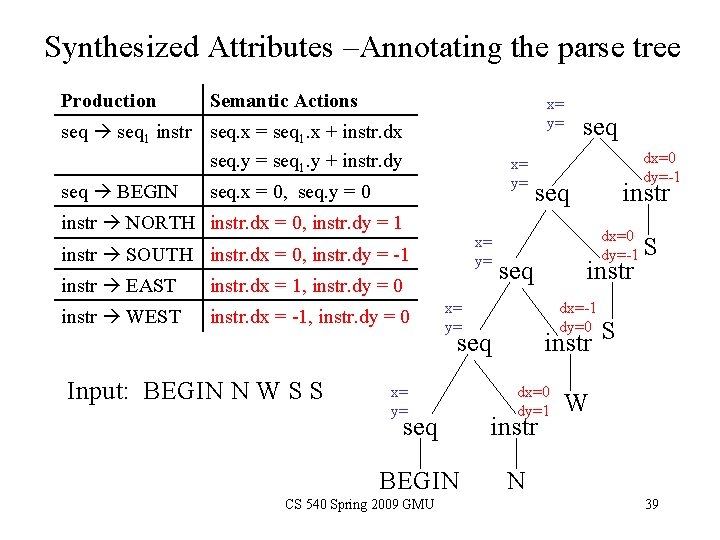
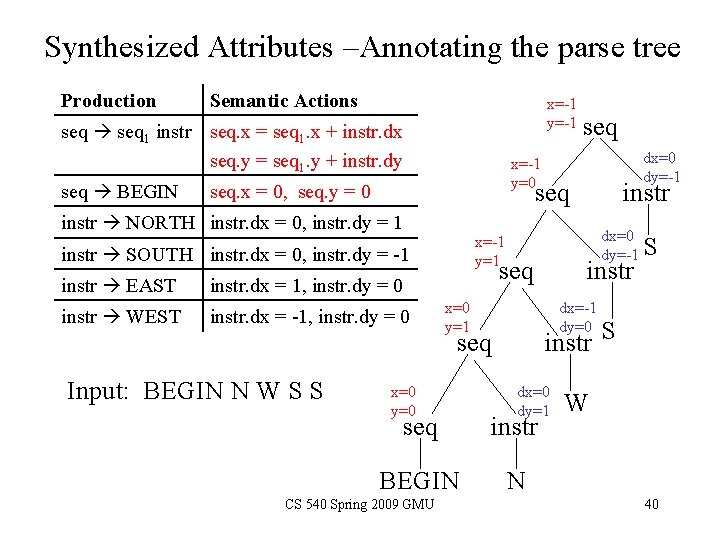
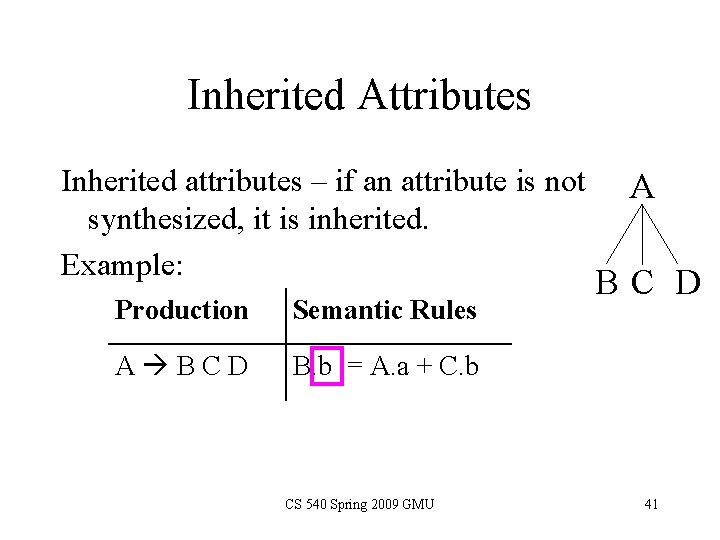
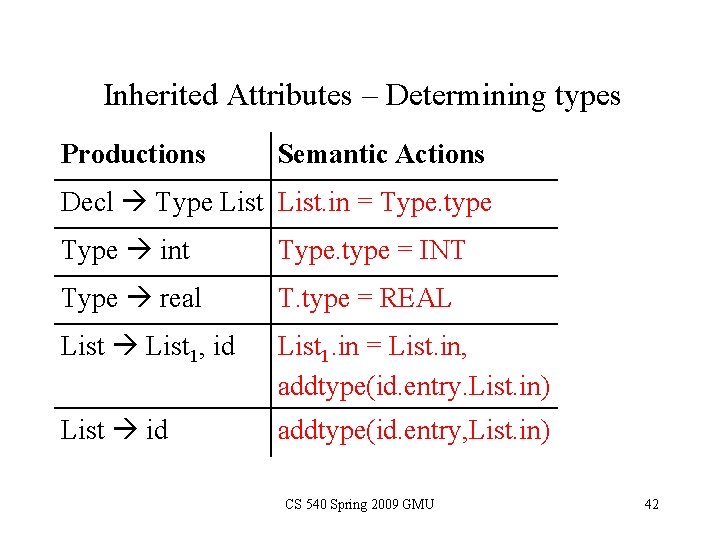
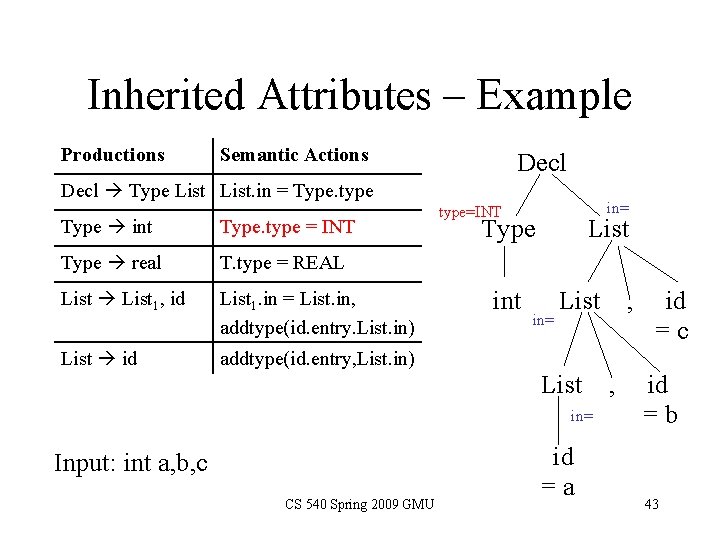
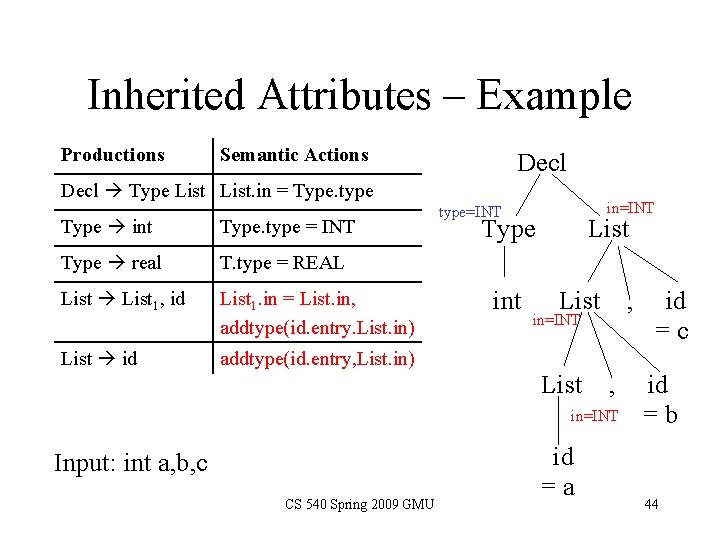
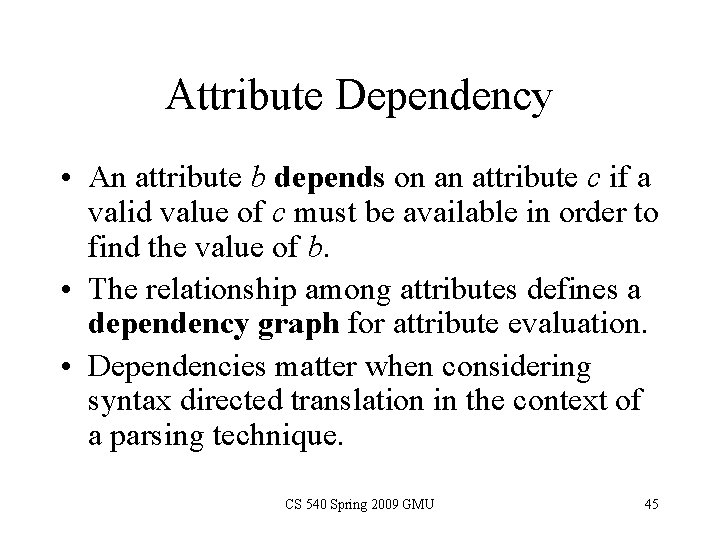
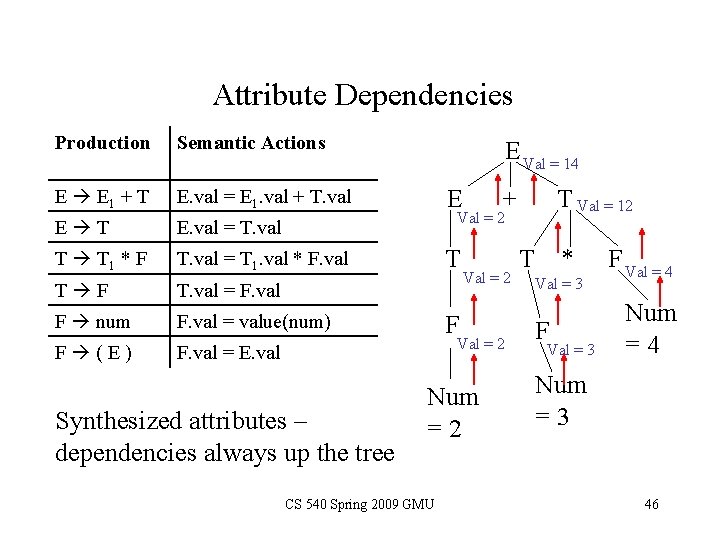
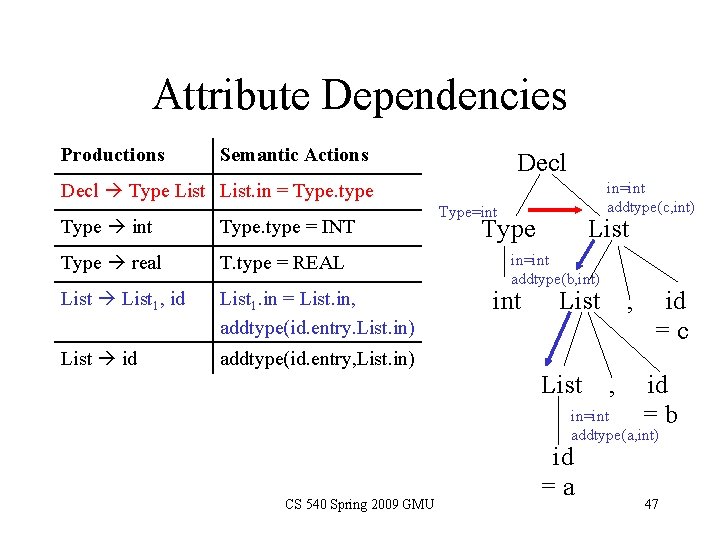
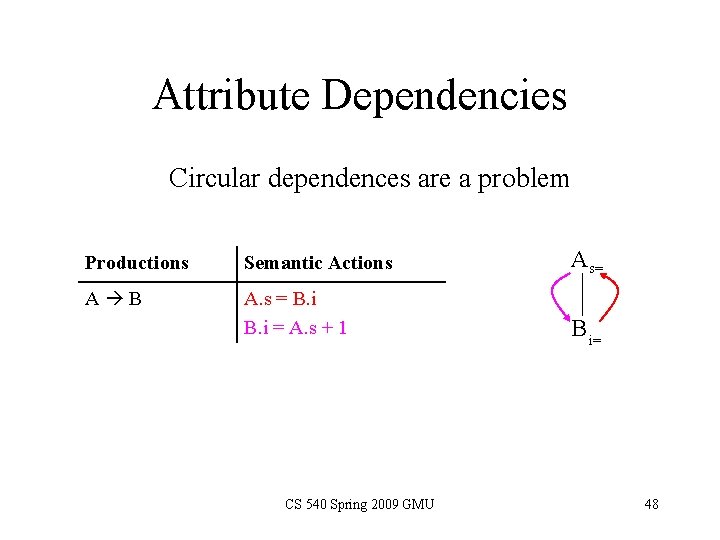
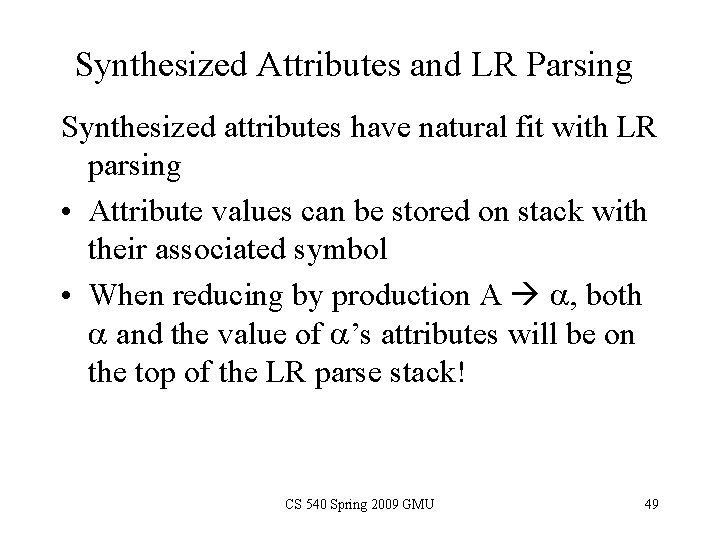
![Synthesized Attributes and LR Parsing Example Stack: $0[attr], a 1[attr], T 2[attr], b 5[attr], Synthesized Attributes and LR Parsing Example Stack: $0[attr], a 1[attr], T 2[attr], b 5[attr],](https://slidetodoc.com/presentation_image_h2/0d0f88dfb68db9c794658f8d7f762d86/image-50.jpg)
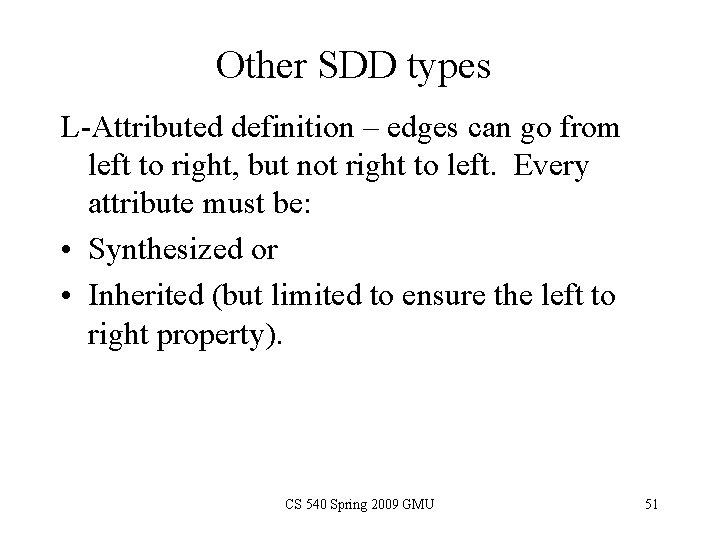
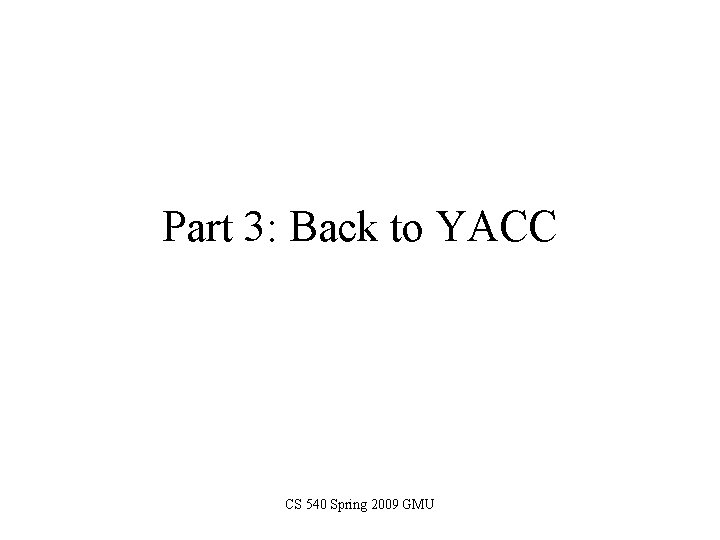
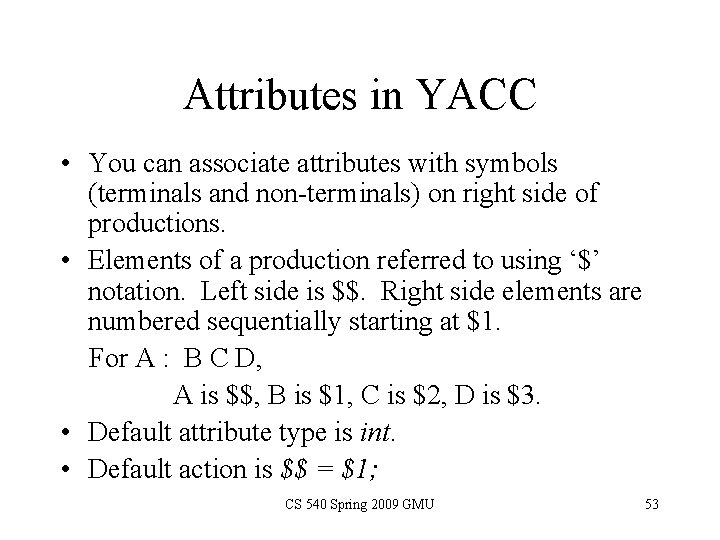
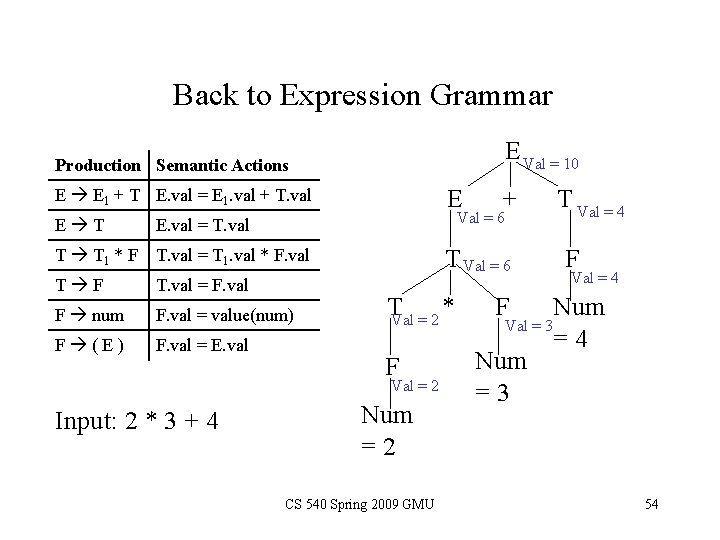
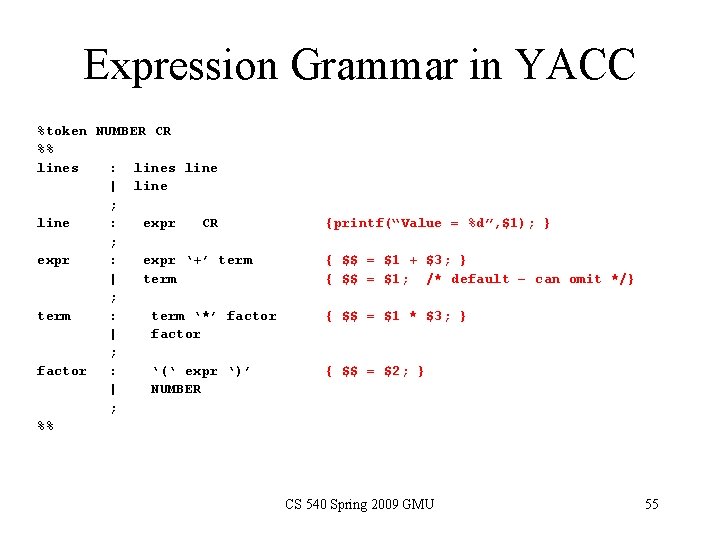
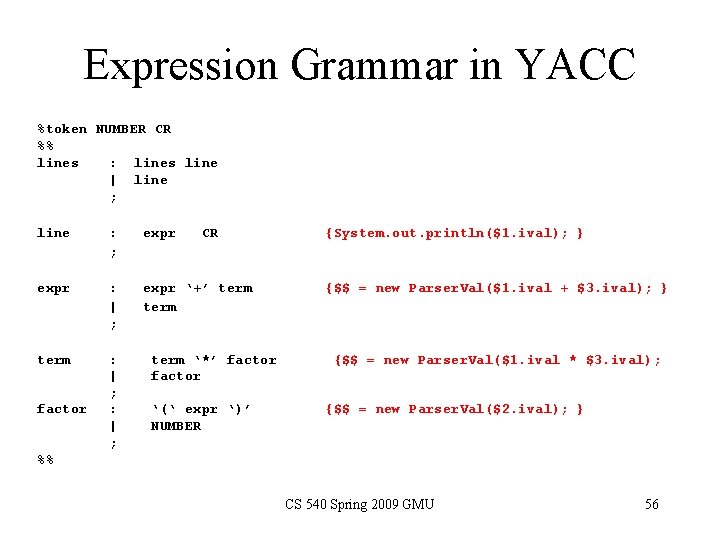
![Associated Lex Specification %% + * ( ) [0 -9]+ [n] [ t] %% Associated Lex Specification %% + * ( ) [0 -9]+ [n] [ t] %%](https://slidetodoc.com/presentation_image_h2/0d0f88dfb68db9c794658f8d7f762d86/image-57.jpg)
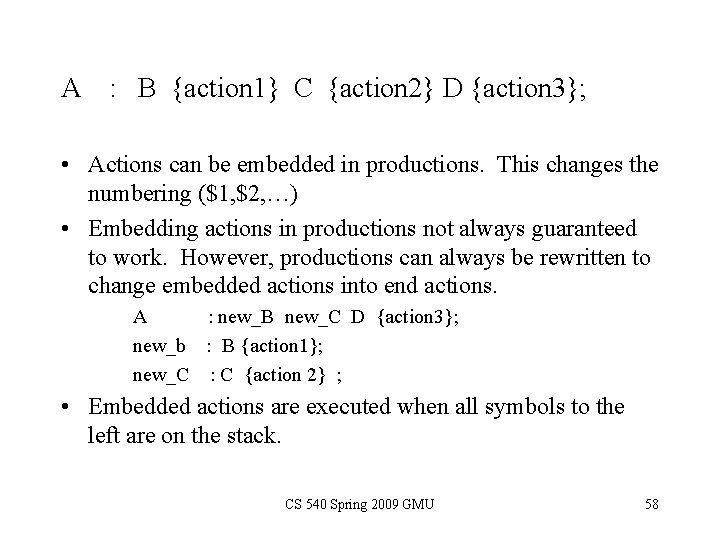
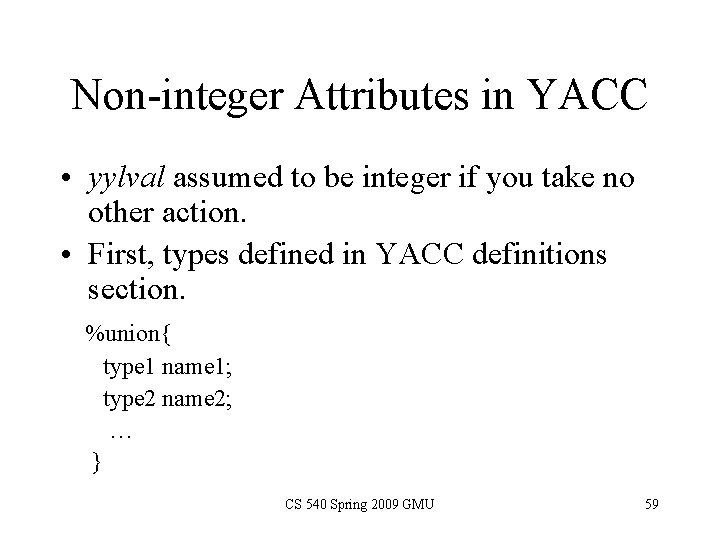
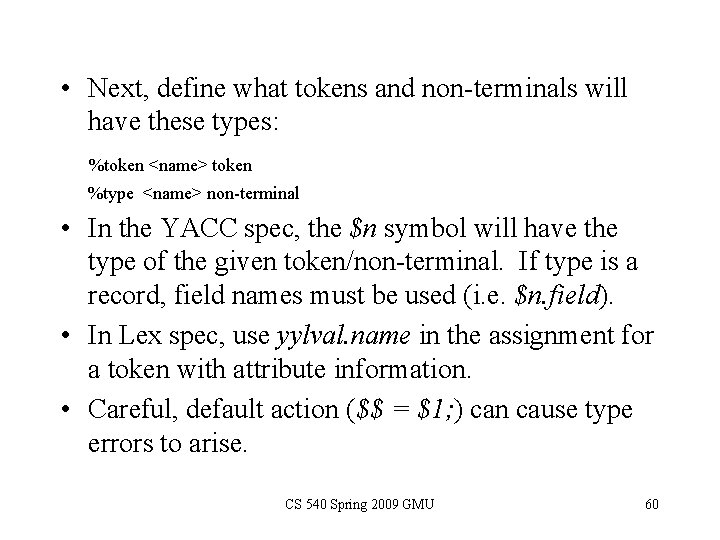
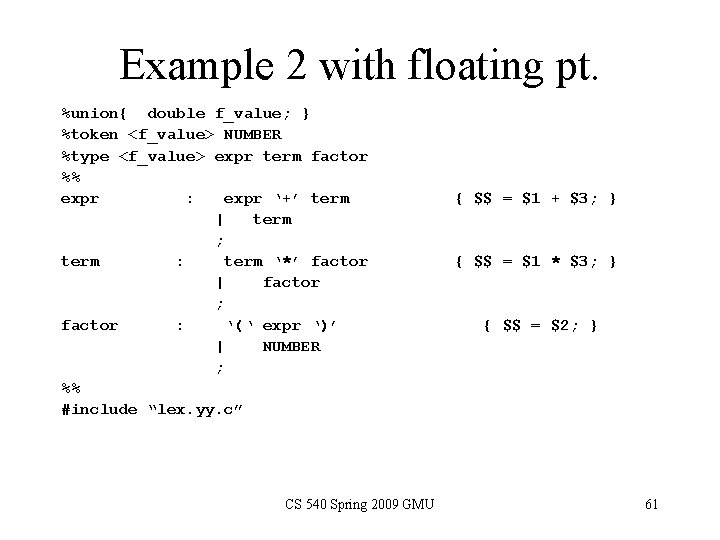
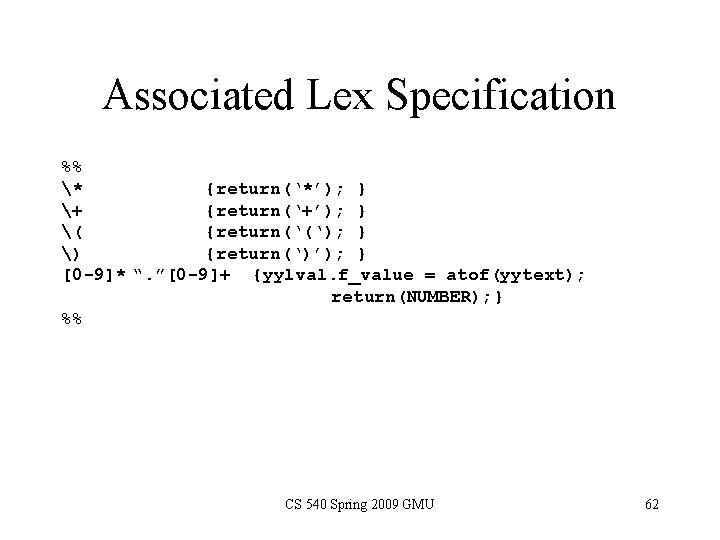
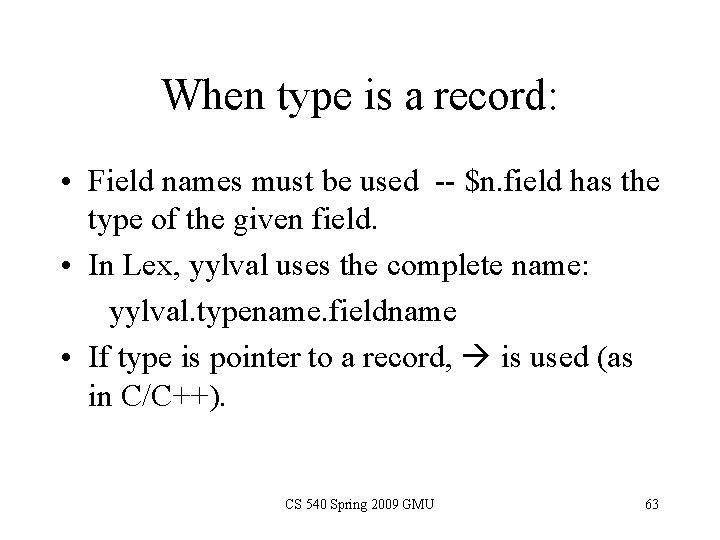
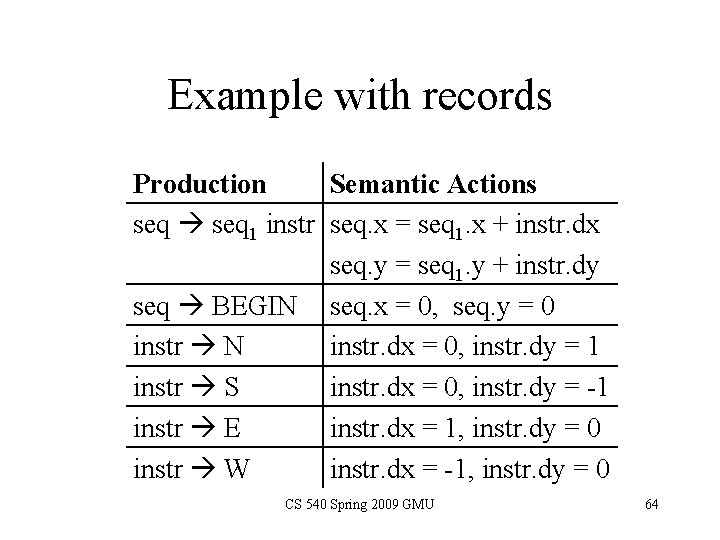
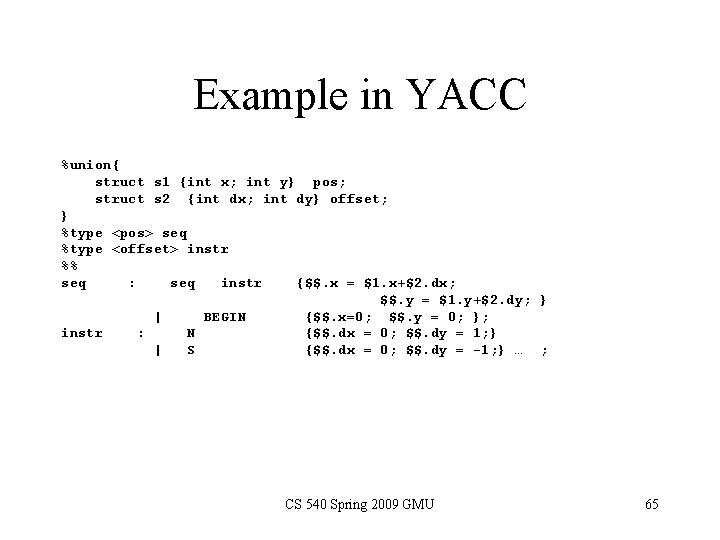
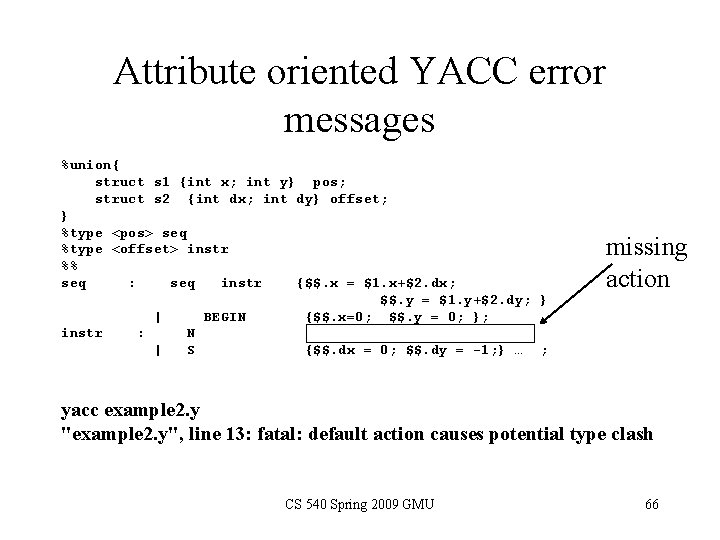
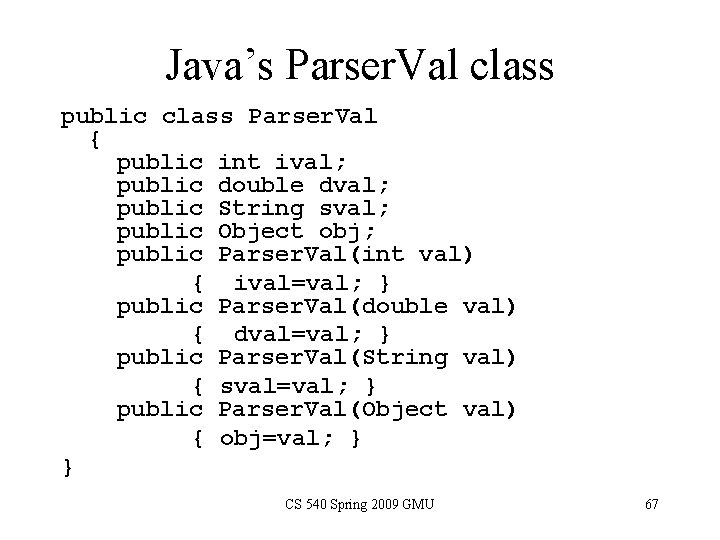
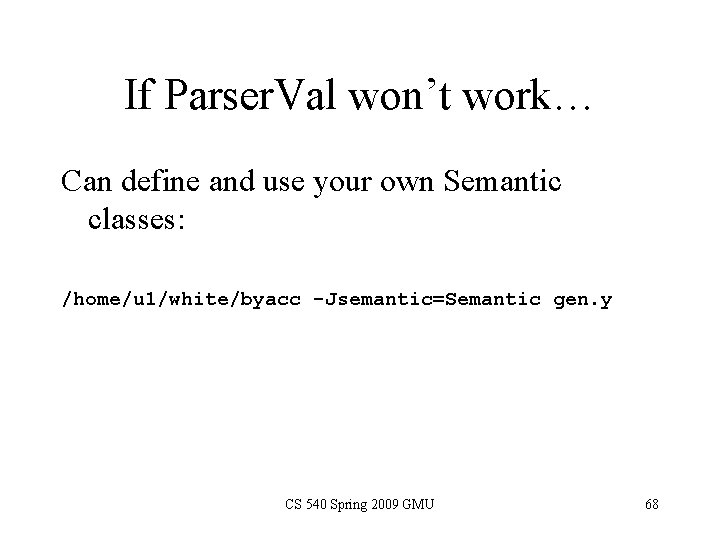
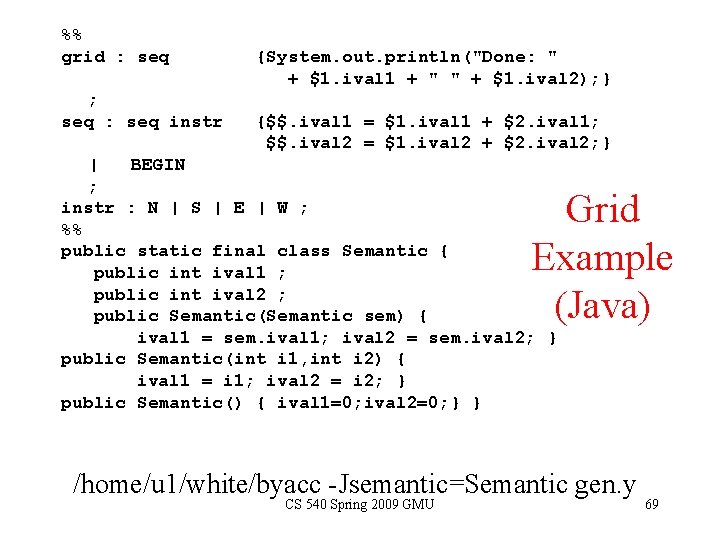
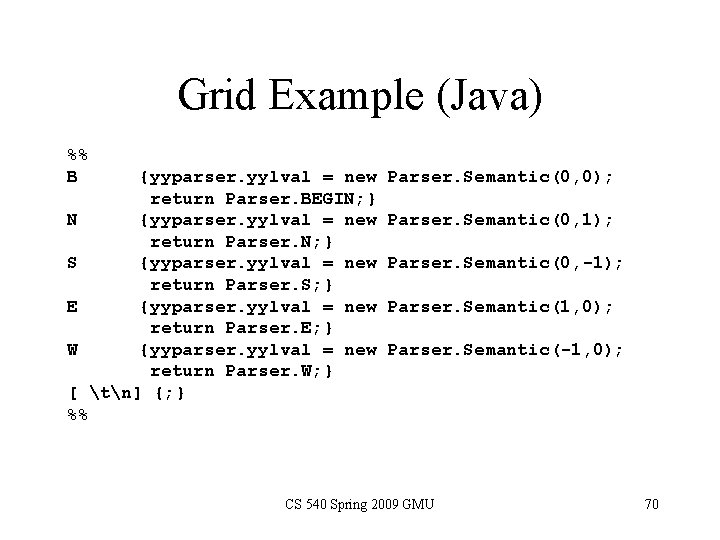
- Slides: 70
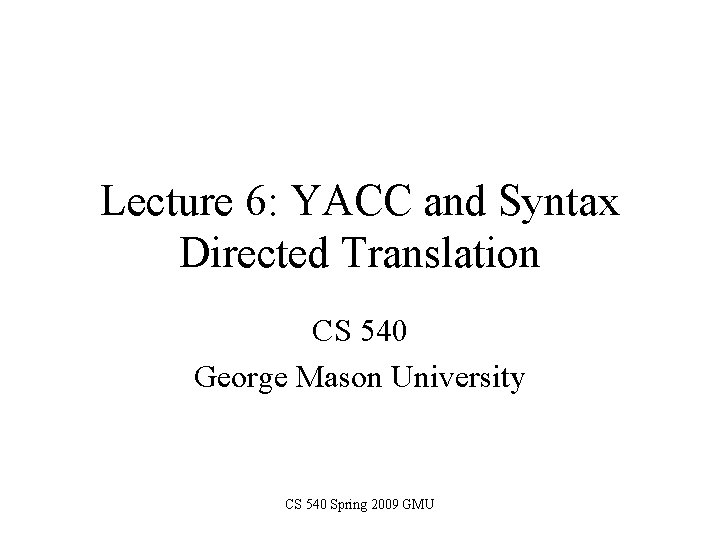
Lecture 6: YACC and Syntax Directed Translation CS 540 George Mason University CS 540 Spring 2009 GMU
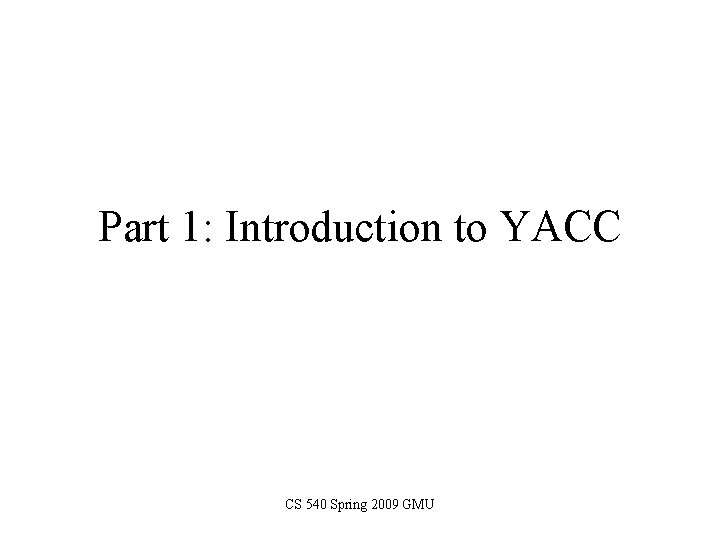
Part 1: Introduction to YACC CS 540 Spring 2009 GMU
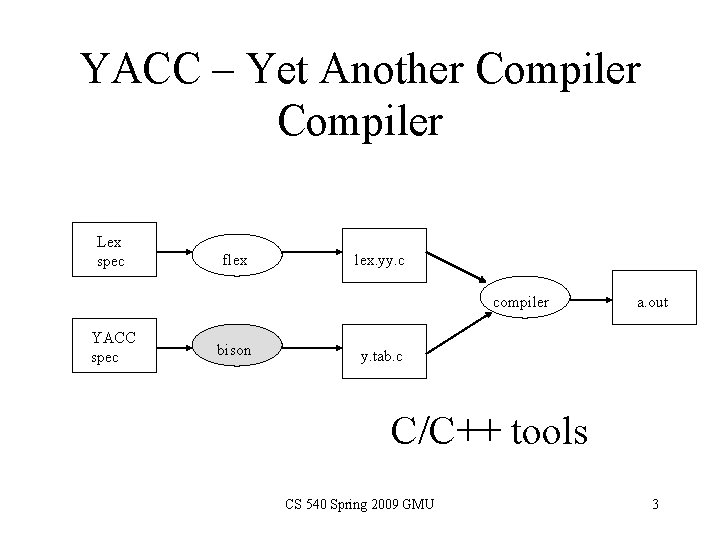
YACC – Yet Another Compiler Lex spec flex lex. yy. c compiler YACC spec bison a. out y. tab. c C/C++ tools CS 540 Spring 2009 GMU 3
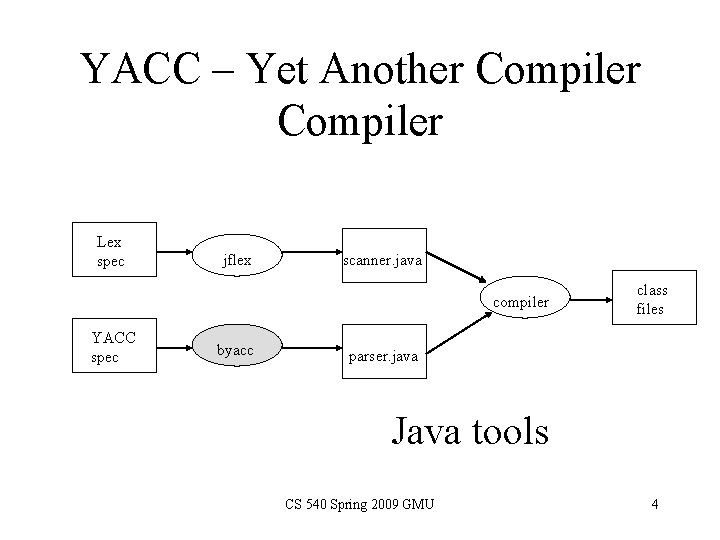
YACC – Yet Another Compiler Lex spec jflex scanner. java compiler YACC spec byacc class files parser. java Java tools CS 540 Spring 2009 GMU 4
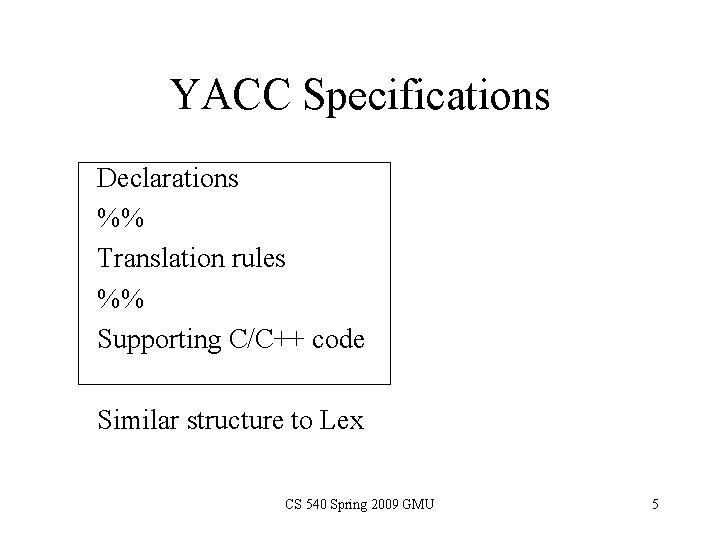
YACC Specifications Declarations %% Translation rules %% Supporting C/C++ code Similar structure to Lex CS 540 Spring 2009 GMU 5
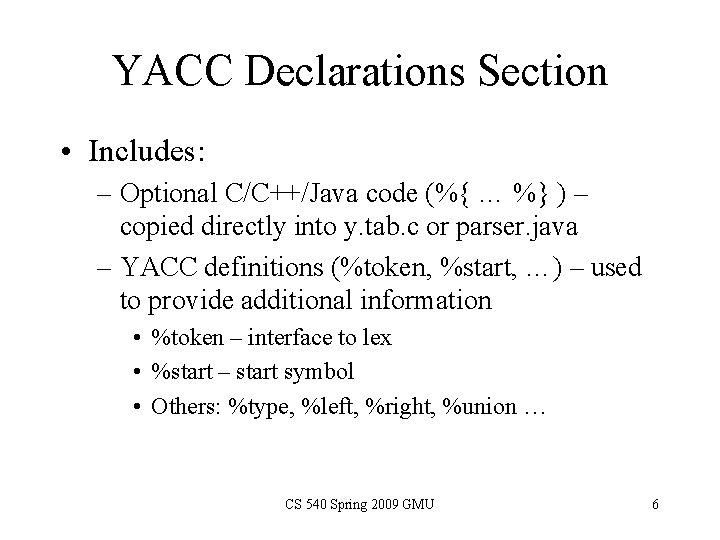
YACC Declarations Section • Includes: – Optional C/C++/Java code (%{ … %} ) – copied directly into y. tab. c or parser. java – YACC definitions (%token, %start, …) – used to provide additional information • %token – interface to lex • %start – start symbol • Others: %type, %left, %right, %union … CS 540 Spring 2009 GMU 6
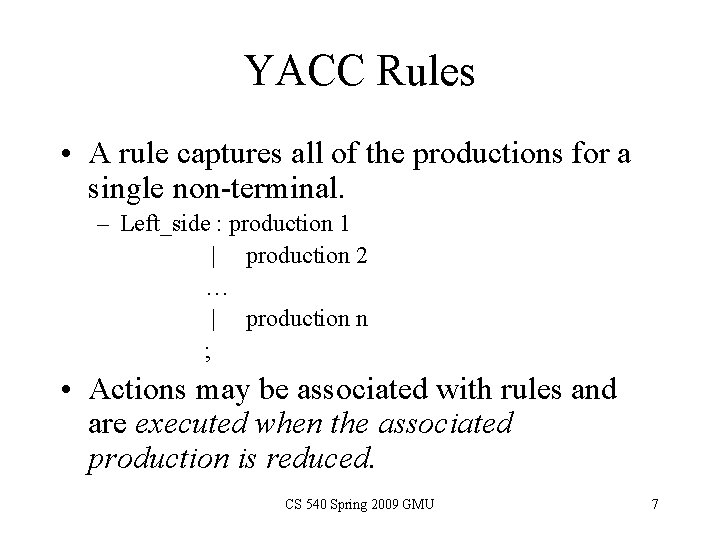
YACC Rules • A rule captures all of the productions for a single non-terminal. – Left_side : production 1 | production 2 … | production n ; • Actions may be associated with rules and are executed when the associated production is reduced. CS 540 Spring 2009 GMU 7
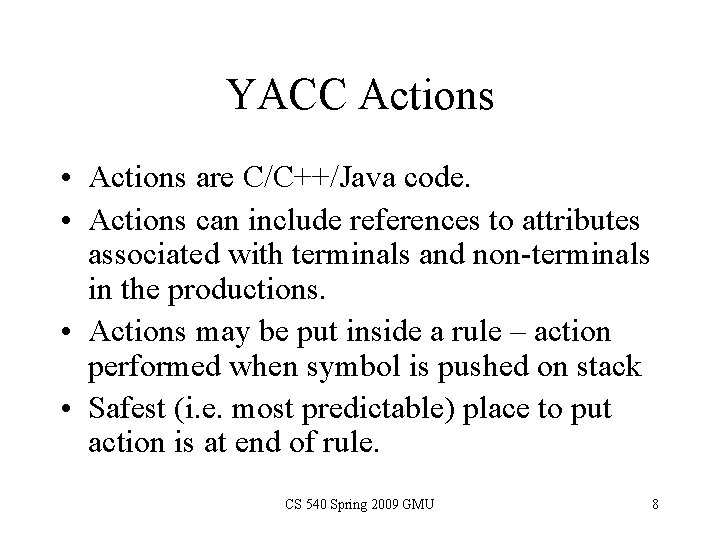
YACC Actions • Actions are C/C++/Java code. • Actions can include references to attributes associated with terminals and non-terminals in the productions. • Actions may be put inside a rule – action performed when symbol is pushed on stack • Safest (i. e. most predictable) place to put action is at end of rule. CS 540 Spring 2009 GMU 8
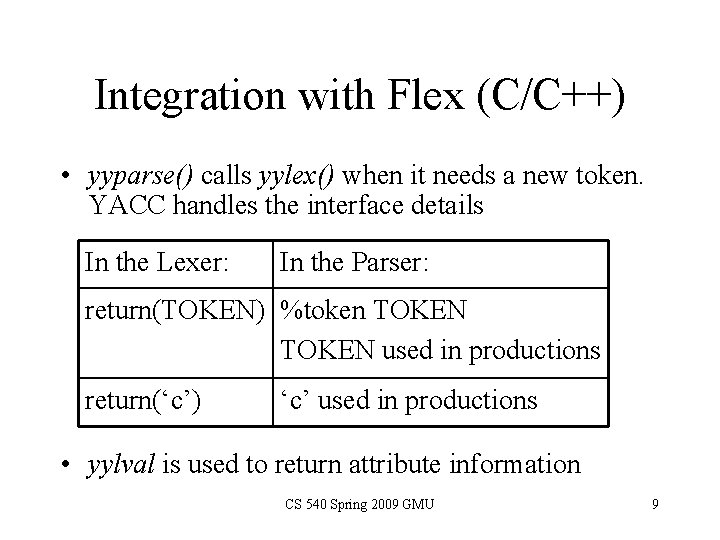
Integration with Flex (C/C++) • yyparse() calls yylex() when it needs a new token. YACC handles the interface details In the Lexer: In the Parser: return(TOKEN) %token TOKEN used in productions return(‘c’) ‘c’ used in productions • yylval is used to return attribute information CS 540 Spring 2009 GMU 9
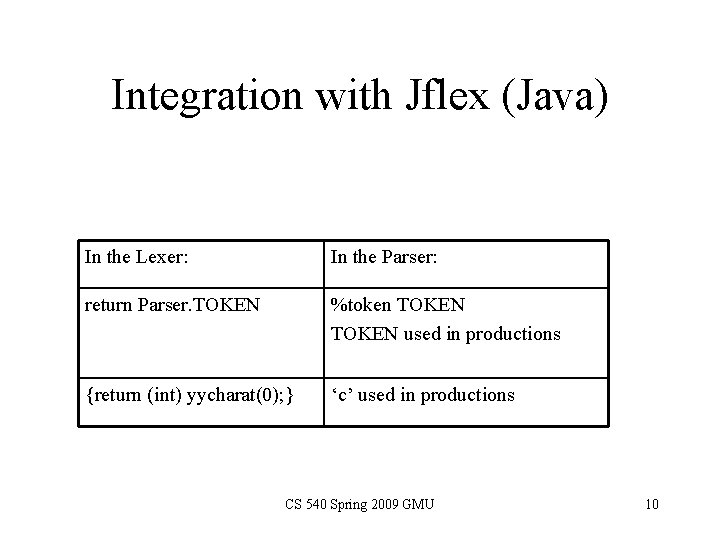
Integration with Jflex (Java) In the Lexer: In the Parser: return Parser. TOKEN %token TOKEN used in productions {return (int) yycharat(0); } ‘c’ used in productions CS 540 Spring 2009 GMU 10
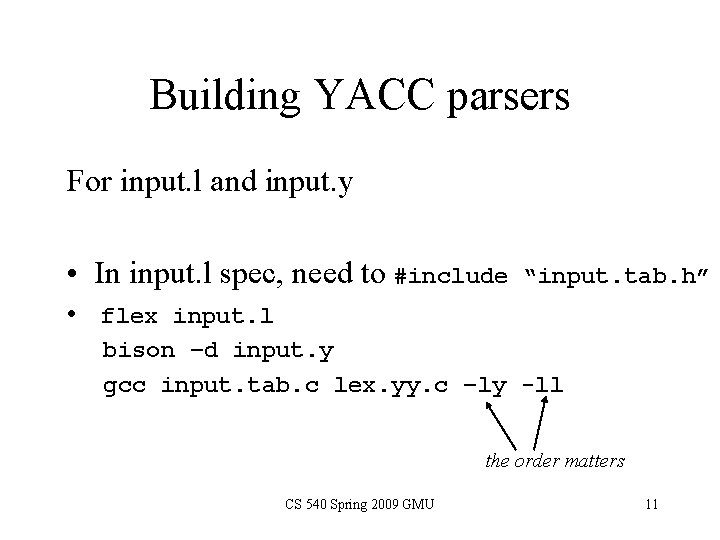
Building YACC parsers For input. l and input. y • In input. l spec, need to #include “input. tab. h” • flex input. l bison –d input. y gcc input. tab. c lex. yy. c –ly -ll the order matters CS 540 Spring 2009 GMU 11
![Basic LexYACC example include sample tab h az AZ returnNAME Basic Lex/YACC example %{ #include “sample. tab. h” %} %% [a-z. A-Z]+ {return(NAME); }](https://slidetodoc.com/presentation_image_h2/0d0f88dfb68db9c794658f8d7f762d86/image-12.jpg)
Basic Lex/YACC example %{ #include “sample. tab. h” %} %% [a-z. A-Z]+ {return(NAME); } [0 -9]{3}”-”[0 -9]{4} {return(NUMBER ); } [ nt] ; %% Lex (sample. l) %token NAME NUMBER %% file : file line | line ; line : NAME NUMBER ; %% YACC (sample. y) CS 540 Spring 2009 GMU 12
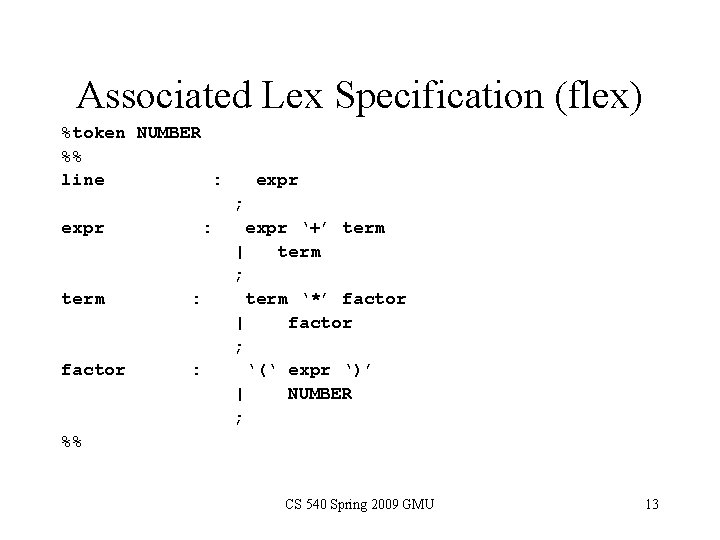
Associated Lex Specification (flex) %token NUMBER %% line : expr ; expr : term : factor : expr ‘+’ term | term ; term ‘*’ factor | factor ; ‘(‘ expr ‘)’ | NUMBER ; %% CS 540 Spring 2009 GMU 13
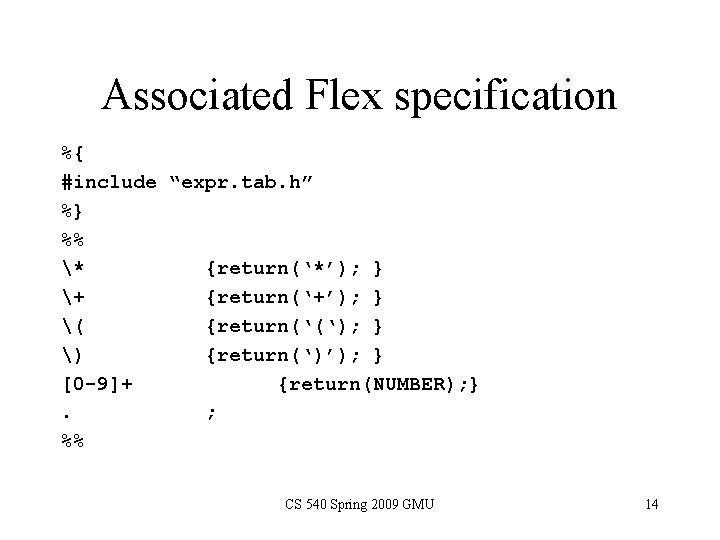
Associated Flex specification %{ #include “expr. tab. h” %} %% * {return(‘*’); } + {return(‘+’); } ( {return(‘(‘); } ) {return(‘)’); } [0 -9]+ {return(NUMBER); }. ; %% CS 540 Spring 2009 GMU 14
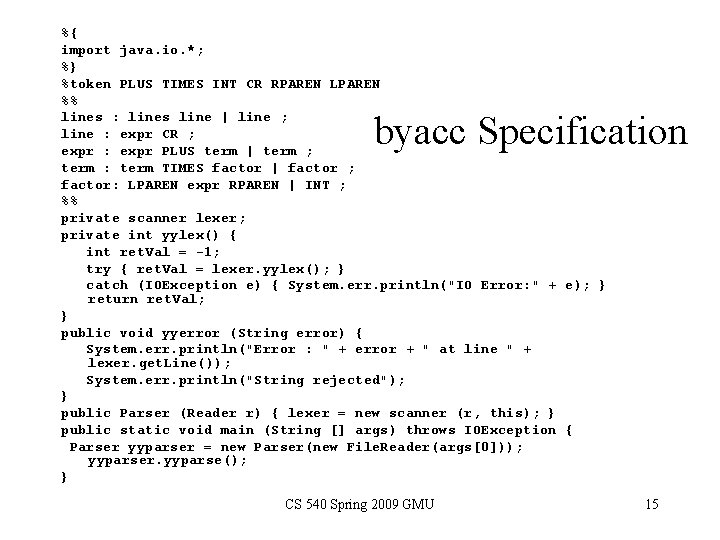
%{ import java. io. *; %} %token PLUS TIMES INT CR RPAREN LPAREN %% lines : lines line | line ; line : expr CR ; expr : expr PLUS term | term ; term : term TIMES factor | factor ; factor: LPAREN expr RPAREN | INT ; %% private scanner lexer; private int yylex() { int ret. Val = -1; try { ret. Val = lexer. yylex(); } catch (IOException e) { System. err. println("IO Error: " + e); } return ret. Val; } public void yyerror (String error) { System. err. println("Error : " + error + " at line " + lexer. get. Line()); System. err. println("String rejected"); } public Parser (Reader r) { lexer = new scanner (r, this); } public static void main (String [] args) throws IOException { Parser yyparser = new Parser(new File. Reader(args[0])); yyparser. yyparse(); } byacc Specification CS 540 Spring 2009 GMU 15
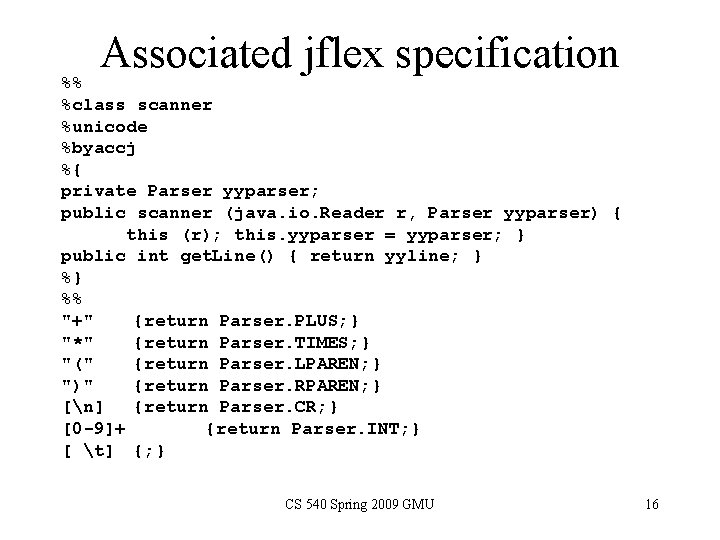
Associated jflex specification %% %class scanner %unicode %byaccj %{ private Parser yyparser; public scanner (java. io. Reader r, Parser yyparser) { this (r); this. yyparser = yyparser; } public int get. Line() { return yyline; } %} %% "+" {return Parser. PLUS; } "*" {return Parser. TIMES; } "(" {return Parser. LPAREN; } ")" {return Parser. RPAREN; } [n] {return Parser. CR; } [0 -9]+ {return Parser. INT; } [ t] {; } CS 540 Spring 2009 GMU 16
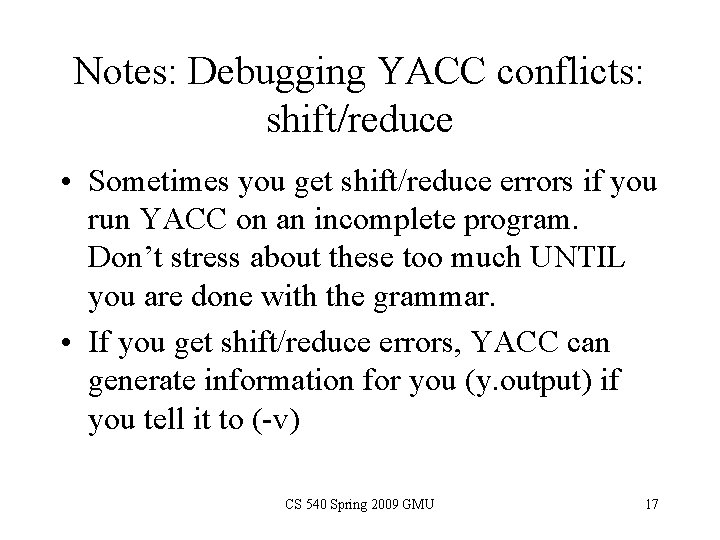
Notes: Debugging YACC conflicts: shift/reduce • Sometimes you get shift/reduce errors if you run YACC on an incomplete program. Don’t stress about these too much UNTIL you are done with the grammar. • If you get shift/reduce errors, YACC can generate information for you (y. output) if you tell it to (-v) CS 540 Spring 2009 GMU 17
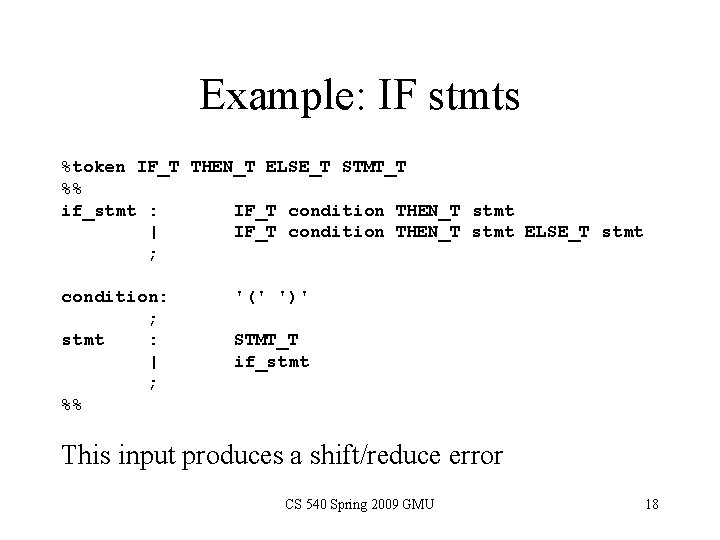
Example: IF stmts %token IF_T THEN_T ELSE_T STMT_T %% if_stmt : IF_T condition THEN_T stmt | IF_T condition THEN_T stmt ELSE_T stmt ; condition: ; stmt : | ; %% '(' ')' STMT_T if_stmt This input produces a shift/reduce error CS 540 Spring 2009 GMU 18
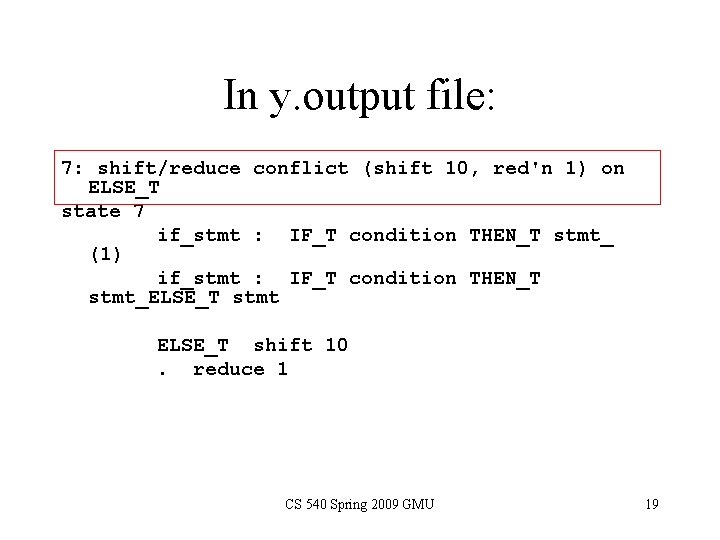
In y. output file: 7: shift/reduce conflict (shift 10, red'n 1) on ELSE_T state 7 if_stmt : IF_T condition THEN_T stmt_ (1) if_stmt : IF_T condition THEN_T stmt_ELSE_T stmt ELSE_T shift 10. reduce 1 CS 540 Spring 2009 GMU 19
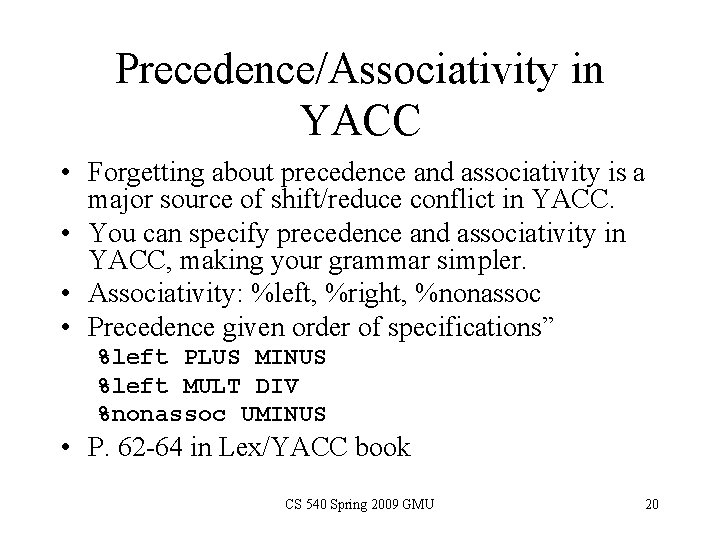
Precedence/Associativity in YACC • Forgetting about precedence and associativity is a major source of shift/reduce conflict in YACC. • You can specify precedence and associativity in YACC, making your grammar simpler. • Associativity: %left, %right, %nonassoc • Precedence given order of specifications” %left PLUS MINUS %left MULT DIV %nonassoc UMINUS • P. 62 -64 in Lex/YACC book CS 540 Spring 2009 GMU 20
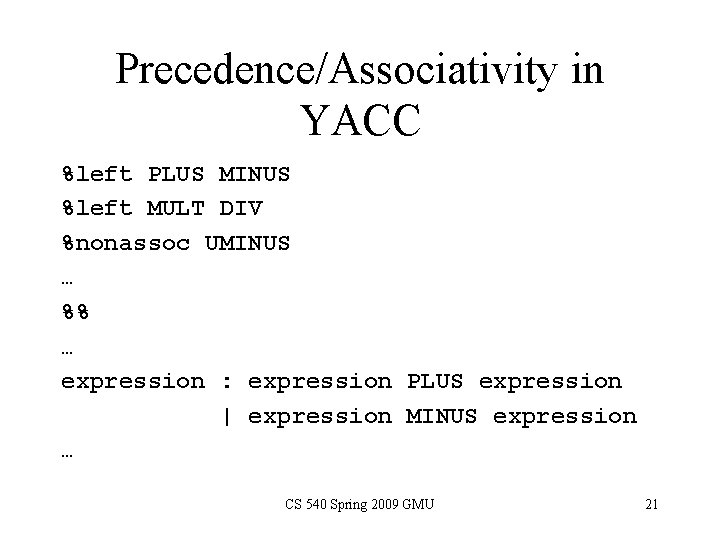
Precedence/Associativity in YACC %left PLUS MINUS %left MULT DIV %nonassoc UMINUS … %% … expression : expression PLUS expression | expression MINUS expression … CS 540 Spring 2009 GMU 21
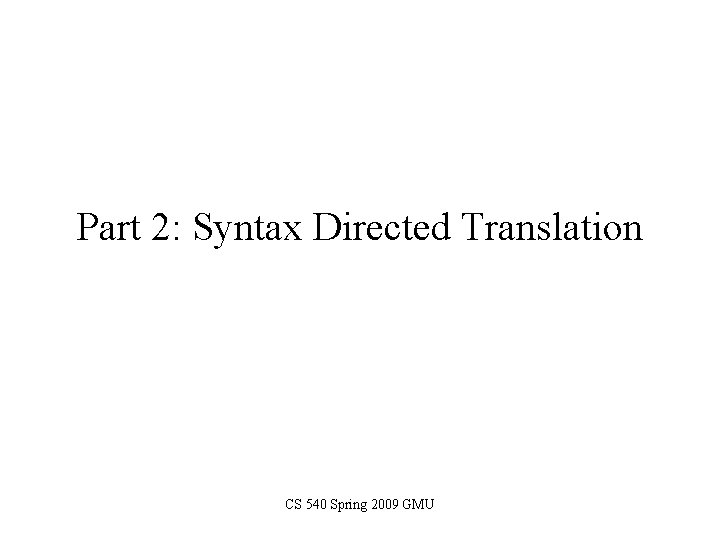
Part 2: Syntax Directed Translation CS 540 Spring 2009 GMU
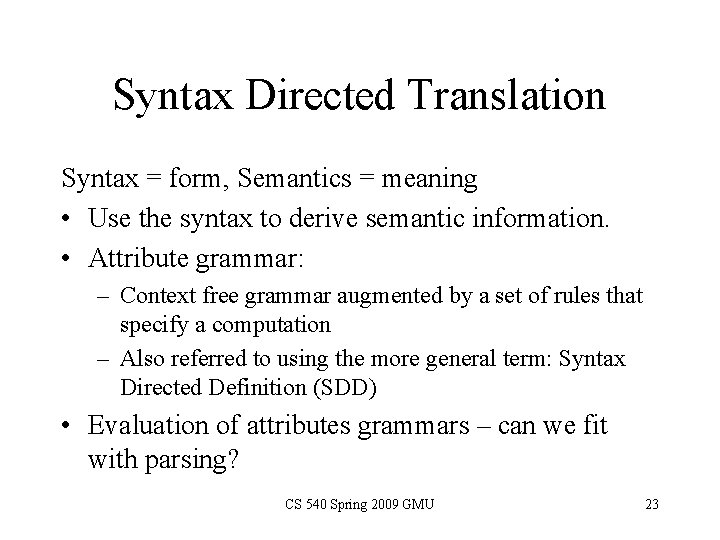
Syntax Directed Translation Syntax = form, Semantics = meaning • Use the syntax to derive semantic information. • Attribute grammar: – Context free grammar augmented by a set of rules that specify a computation – Also referred to using the more general term: Syntax Directed Definition (SDD) • Evaluation of attributes grammars – can we fit with parsing? CS 540 Spring 2009 GMU 23
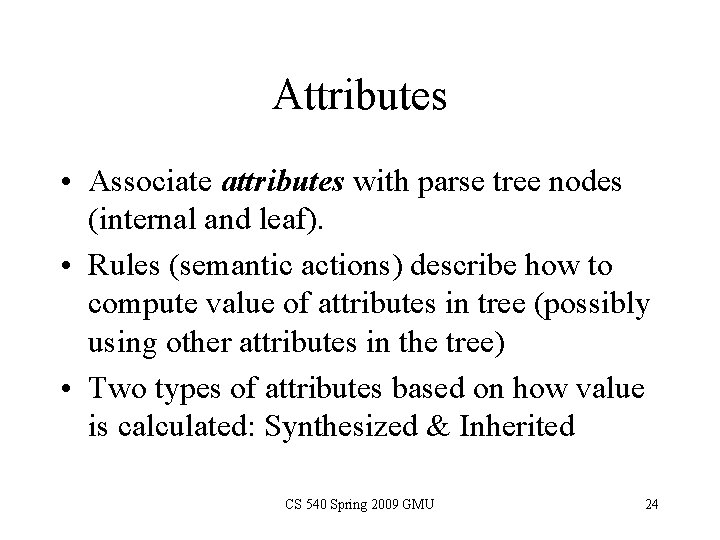
Attributes • Associate attributes with parse tree nodes (internal and leaf). • Rules (semantic actions) describe how to compute value of attributes in tree (possibly using other attributes in the tree) • Two types of attributes based on how value is calculated: Synthesized & Inherited CS 540 Spring 2009 GMU 24
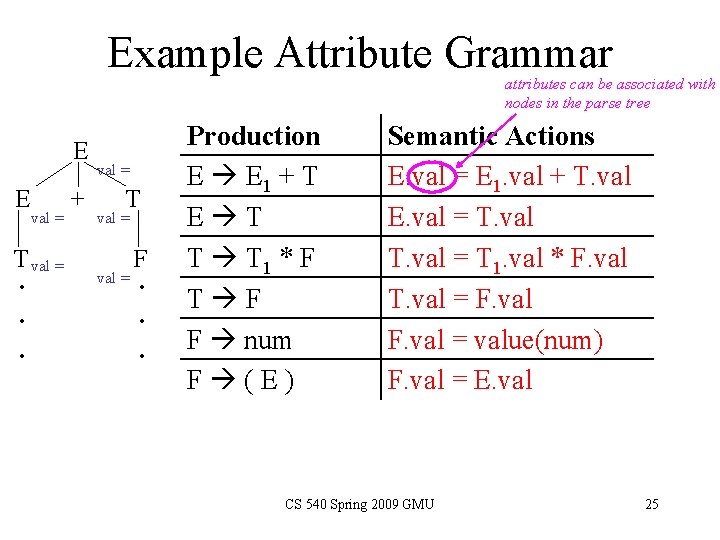
Example Attribute Grammar attributes can be associated with nodes in the parse tree E E val = T val = . . . + val = T val = F . . . Production E E 1 + T E T T T 1 * F T F F num F (E) Semantic Actions E. val = E 1. val + T. val E. val = T 1. val * F. val T. val = F. val = value(num) F. val = E. val CS 540 Spring 2009 GMU 25
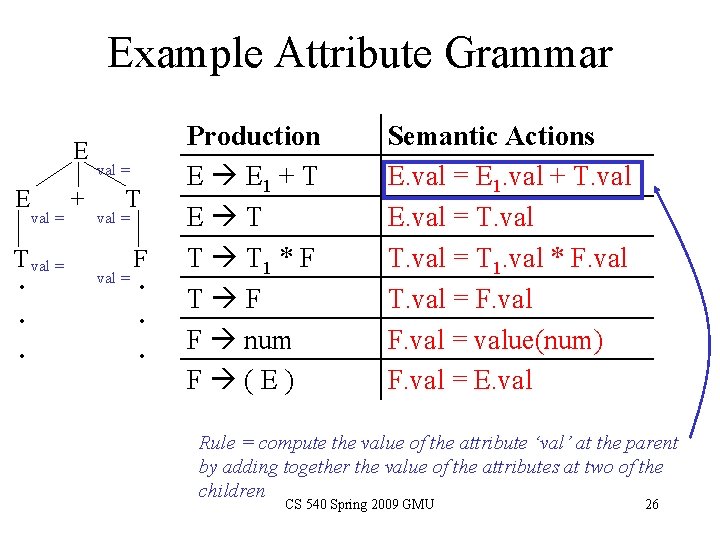
Example Attribute Grammar E E val = T val = . . . + val = T val = F . . . Production E E 1 + T E T T T 1 * F T F F num F (E) Semantic Actions E. val = E 1. val + T. val E. val = T 1. val * F. val T. val = F. val = value(num) F. val = E. val Rule = compute the value of the attribute ‘val’ at the parent by adding together the value of the attributes at two of the children CS 540 Spring 2009 GMU 26
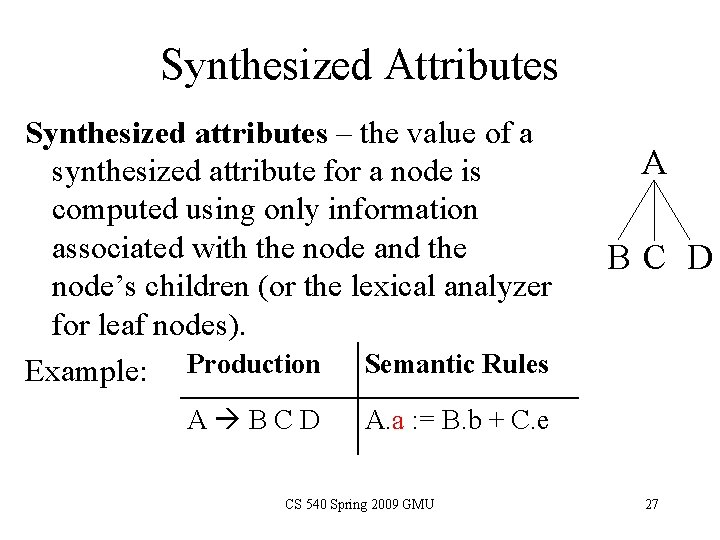
Synthesized Attributes Synthesized attributes – the value of a synthesized attribute for a node is computed using only information associated with the node and the node’s children (or the lexical analyzer for leaf nodes). Example: Production Semantic Rules A BCD A BC D A. a : = B. b + C. e CS 540 Spring 2009 GMU 27
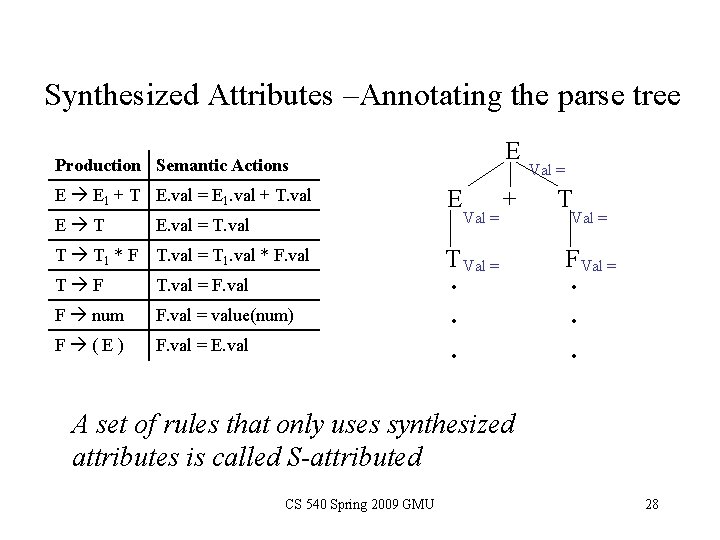
Synthesized Attributes –Annotating the parse tree E Production Semantic Actions E E 1 + T E. val = E 1. val + T. val E T E E. val = T. val T T 1 * F T. val = T 1. val * F. val T F T. val = F. val F num F. val = value(num) F (E) F. val = E. val Val = + T Val = . . . Val = T Val = FVal = . . . A set of rules that only uses synthesized attributes is called S-attributed CS 540 Spring 2009 GMU 28
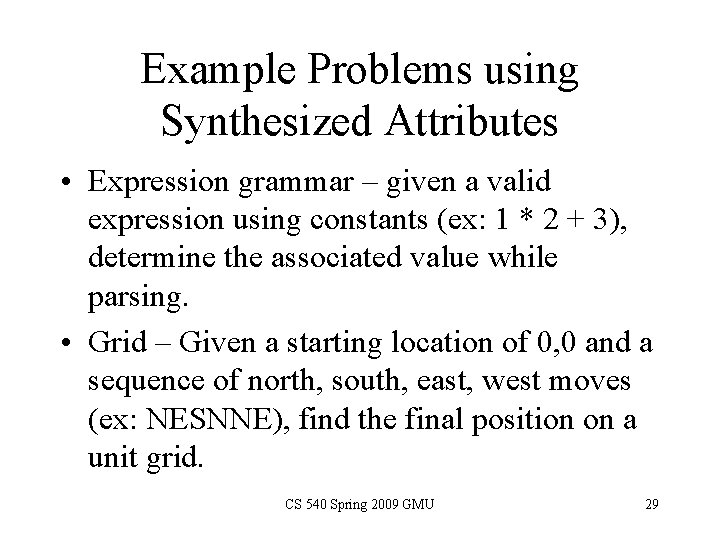
Example Problems using Synthesized Attributes • Expression grammar – given a valid expression using constants (ex: 1 * 2 + 3), determine the associated value while parsing. • Grid – Given a starting location of 0, 0 and a sequence of north, south, east, west moves (ex: NESNNE), find the final position on a unit grid. CS 540 Spring 2009 GMU 29
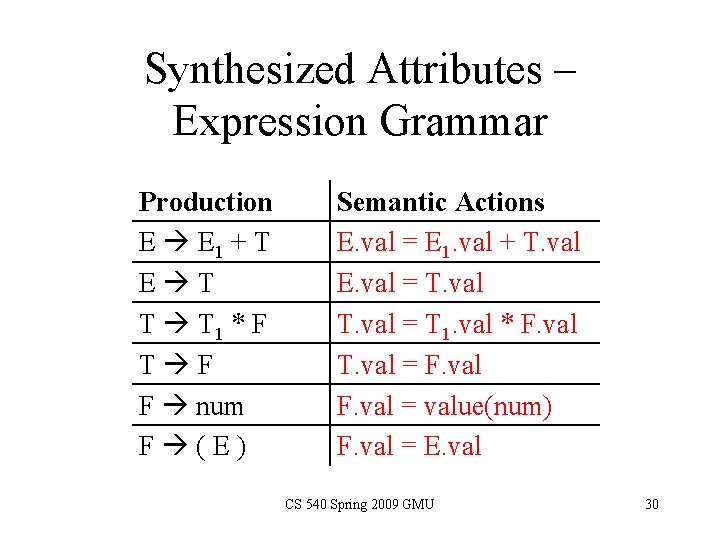
Synthesized Attributes – Expression Grammar Production E E 1 + T E T T T 1 * F T F F num F (E) Semantic Actions E. val = E 1. val + T. val E. val = T 1. val * F. val T. val = F. val = value(num) F. val = E. val CS 540 Spring 2009 GMU 30
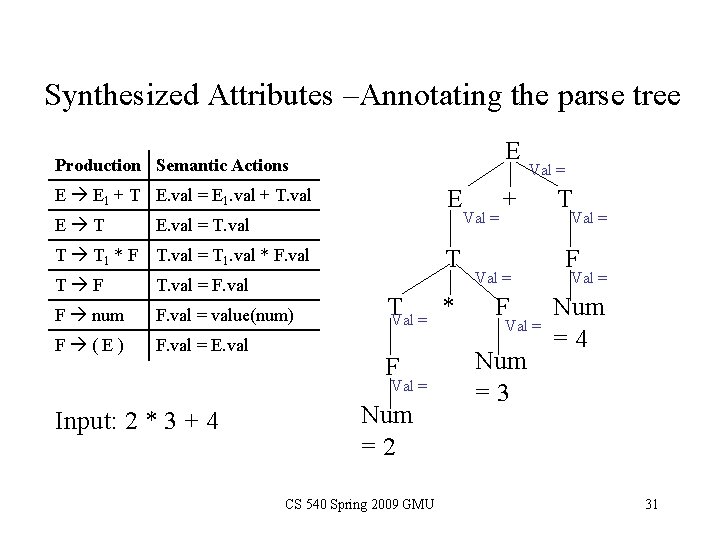
Synthesized Attributes –Annotating the parse tree E Production Semantic Actions E E E 1 + T E. val = E 1. val + T. val E T E. val = T. val T T T 1 * F T. val = T 1. val * F. val T F T. val = F. val F num F. val = value(num) F (E) F. val = E. val TVal = * F Val = Input: 2 * 3 + 4 Num =2 CS 540 Spring 2009 GMU Val = + Val = F Val = Num =3 T Val = F Val = Num =4 31
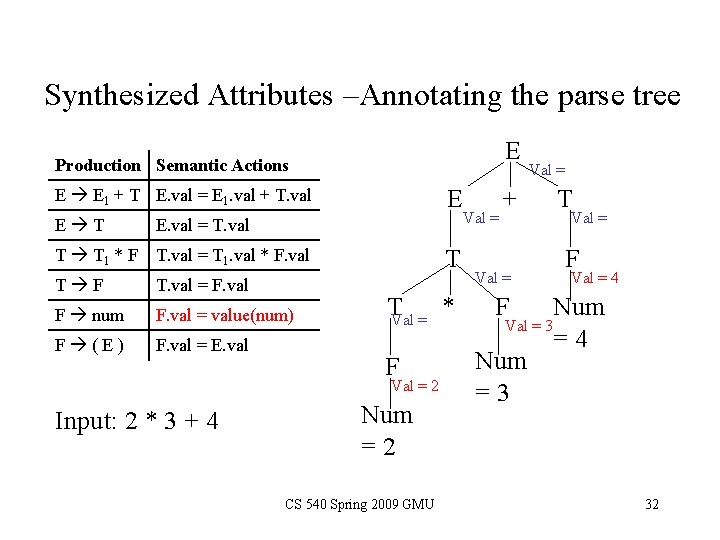
Synthesized Attributes –Annotating the parse tree E Production Semantic Actions E E E 1 + T E. val = E 1. val + T. val E T E. val = T. val T T T 1 * F T. val = T 1. val * F. val T F T. val = F. val F num F. val = value(num) F (E) F. val = E. val TVal = * F Val = 2 Input: 2 * 3 + 4 Num =2 CS 540 Spring 2009 GMU Val = + Val = T Val = F Val = 4 F Num Val = 3 =4 Num =3 32
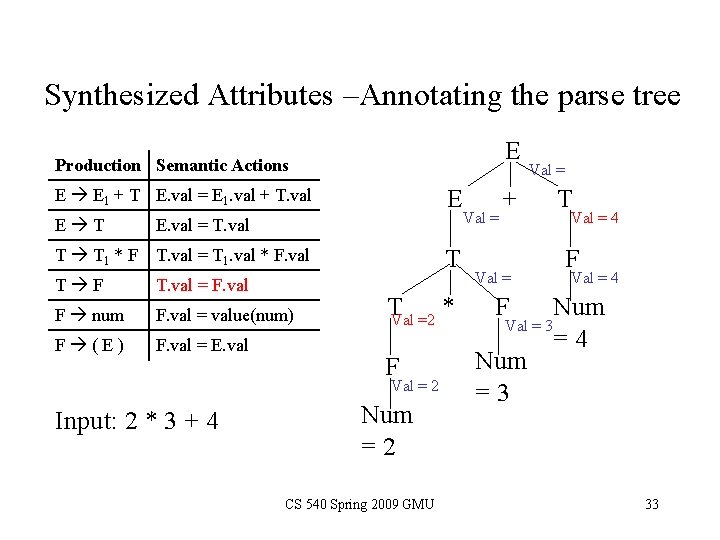
Synthesized Attributes –Annotating the parse tree E Production Semantic Actions E E E 1 + T E. val = E 1. val + T. val E T E. val = T. val T T T 1 * F T. val = T 1. val * F. val T F T. val = F. val F num F. val = value(num) F (E) F. val = E. val TVal =2 * F Val = 2 Input: 2 * 3 + 4 Num =2 CS 540 Spring 2009 GMU Val = + Val = T Val = 4 F Num Val = 3 =4 Num =3 33
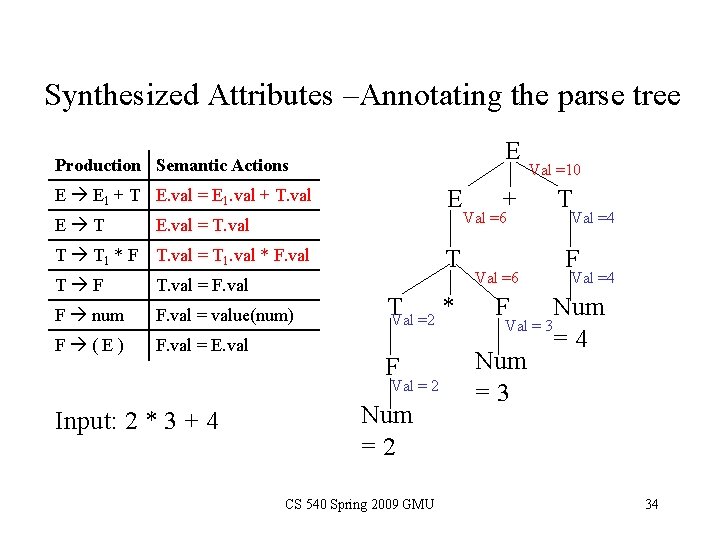
Synthesized Attributes –Annotating the parse tree E Production Semantic Actions E E E 1 + T E. val = E 1. val + T. val E T E. val = T. val T T T 1 * F T. val = T 1. val * F. val T F T. val = F. val F num F. val = value(num) F (E) F. val = E. val TVal =2 * F Val = 2 Input: 2 * 3 + 4 Num =2 CS 540 Spring 2009 GMU + Val =6 Val =10 T Val =4 F Num Val = 3 =4 Num =3 34
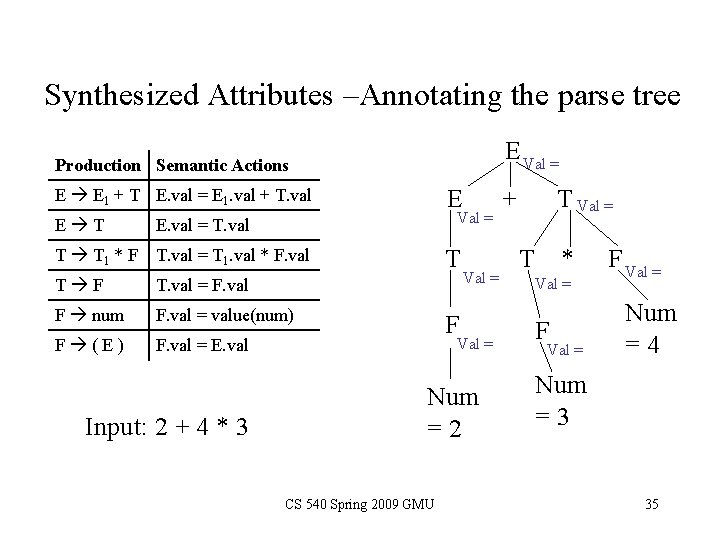
Synthesized Attributes –Annotating the parse tree E Val = Production Semantic Actions E E E 1 + T E. val = E 1. val + T. val E T Val = E. val = T. val T T T 1 * F T. val = T 1. val * F. val T F T. val = F. val F num F. val = value(num) F (E) F. val = E. val Input: 2 + 4 * 3 Val = F Val = Num =2 CS 540 Spring 2009 GMU + T Val = T * Val = F Val = Num =4 Num =3 35
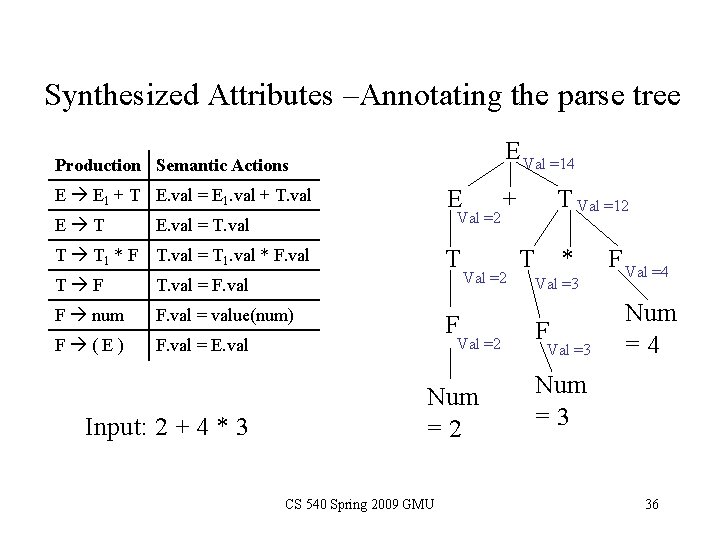
Synthesized Attributes –Annotating the parse tree E Val =14 Production Semantic Actions E E E 1 + T E. val = E 1. val + T. val E T Val =2 E. val = T. val T T T 1 * F T. val = T 1. val * F. val T F T. val = F. val F num F. val = value(num) F (E) F. val = E. val Input: 2 + 4 * 3 Val =2 F Val =2 Num =2 CS 540 Spring 2009 GMU + T Val =12 T * Val =3 F Val =4 Num =3 36
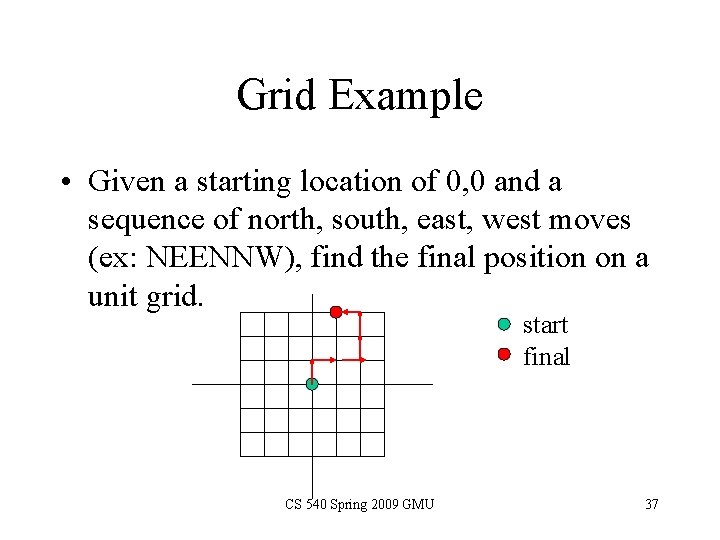
Grid Example • Given a starting location of 0, 0 and a sequence of north, south, east, west moves (ex: NEENNW), find the final position on a unit grid. start final CS 540 Spring 2009 GMU 37
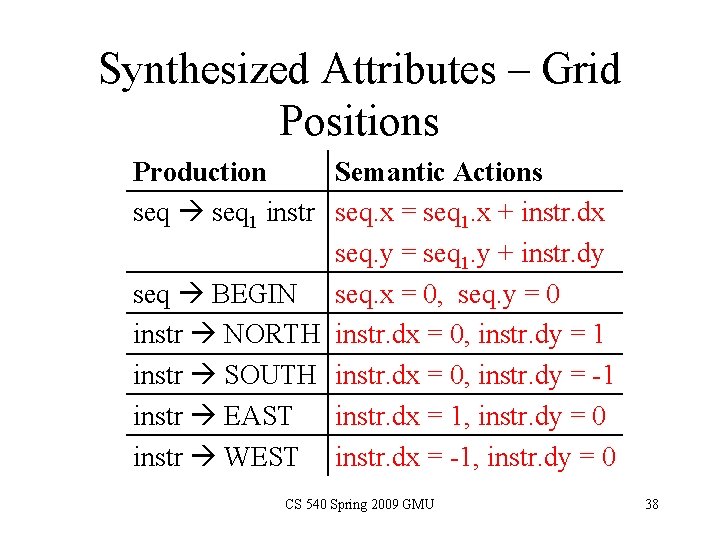
Synthesized Attributes – Grid Positions Production Semantic Actions seq 1 instr seq. x = seq 1. x + instr. dx seq. y = seq 1. y + instr. dy seq BEGIN seq. x = 0, seq. y = 0 instr NORTH instr. dx = 0, instr. dy = 1 instr SOUTH instr. dx = 0, instr. dy = -1 instr EAST instr. dx = 1, instr. dy = 0 instr WEST instr. dx = -1, instr. dy = 0 CS 540 Spring 2009 GMU 38
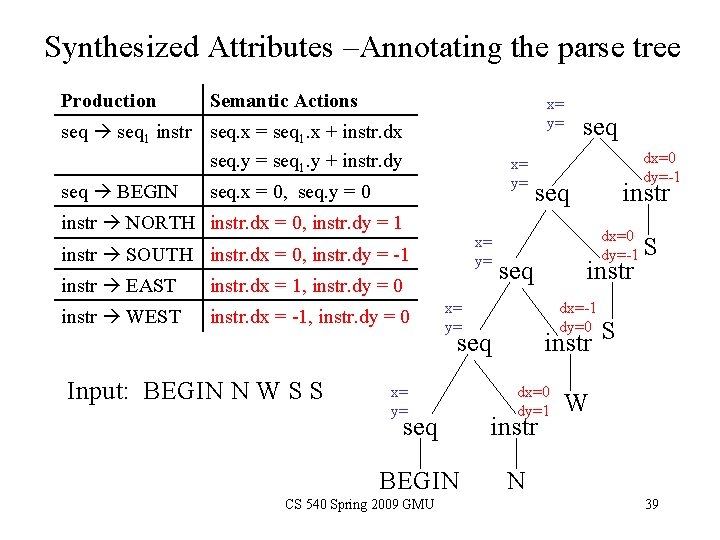
Synthesized Attributes –Annotating the parse tree Production Semantic Actions x= y= seq 1 instr seq. x = seq 1. x + instr. dx seq. y = seq 1. y + instr. dy seq BEGIN x= y= seq. x = 0, seq. y = 0 instr NORTH instr. dx = 0, instr. dy = 1 x= y= instr SOUTH instr. dx = 0, instr. dy = -1 instr EAST instr. dx = 1, instr. dy = 0 instr WEST instr. dx = -1, instr. dy = 0 instr S dx=-1 dy=0 instr S dx=0 dy=1 seq instr BEGIN N CS 540 Spring 2009 GMU instr dx=0 dy=-1 seq x= y= dx=0 dy=-1 seq Input: BEGIN N W S S seq W 39
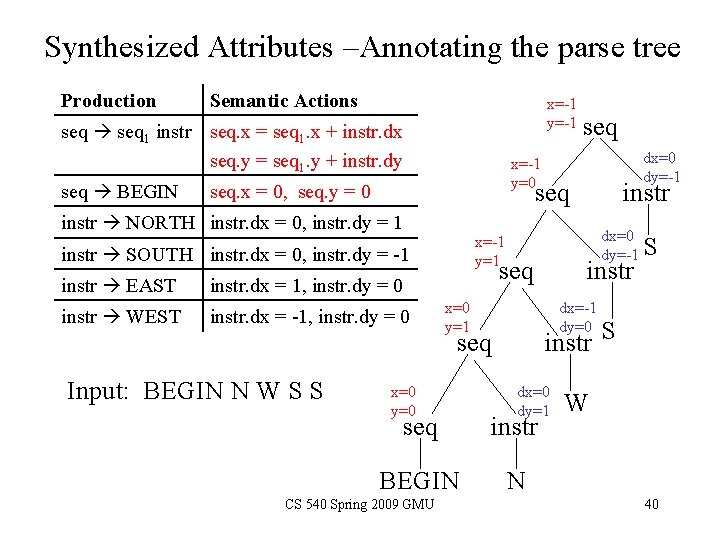
Synthesized Attributes –Annotating the parse tree Production Semantic Actions x=-1 y=-1 seq 1 instr seq. x = seq 1. x + instr. dx seq. y = seq 1. y + instr. dy seq BEGIN dx=0 dy=-1 x=-1 y=0 seq. x = 0, seq. y = 0 instr NORTH instr. dx = 0, instr. dy = 1 instr EAST instr. dx = 1, instr. dy = 0 instr WEST instr. dx = -1, instr. dy = 0 seq x=0 y=1 instr S dx=0 dy=1 seq instr BEGIN N CS 540 Spring 2009 GMU instr S dx=-1 dy=0 seq x=0 y=0 instr dx=0 dy=-1 x=-1 y=1 instr SOUTH instr. dx = 0, instr. dy = -1 Input: BEGIN N W S S seq W 40
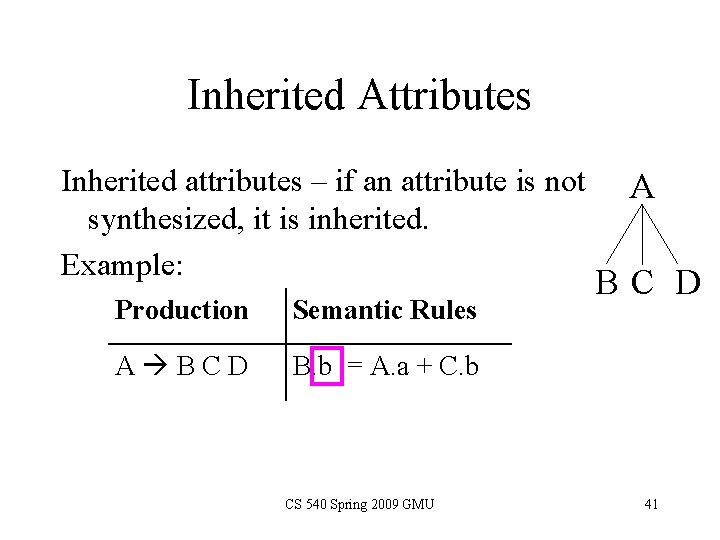
Inherited Attributes Inherited attributes – if an attribute is not synthesized, it is inherited. Example: Production Semantic Rules A BCD B. b : = A. a + C. b CS 540 Spring 2009 GMU A BC D 41
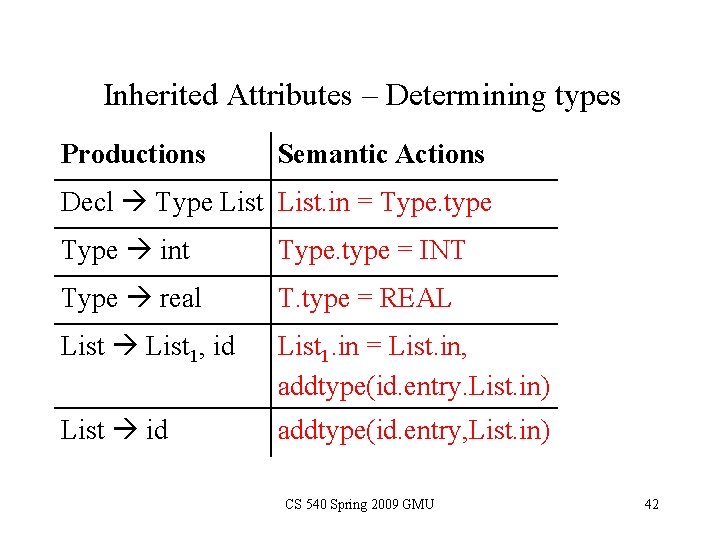
Inherited Attributes – Determining types Productions Semantic Actions Decl Type List. in = Type. type Type int Type. type = INT Type real T. type = REAL List 1, id List 1. in = List. in, addtype(id. entry. List. in) List id addtype(id. entry, List. in) CS 540 Spring 2009 GMU 42
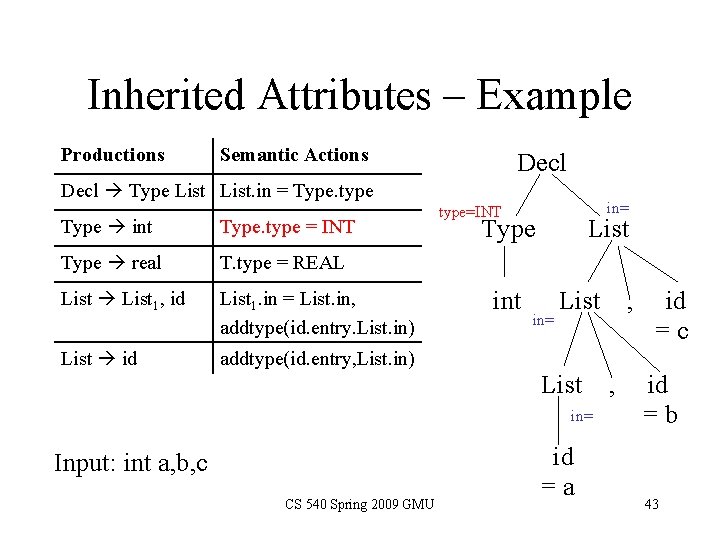
Inherited Attributes – Example Productions Semantic Actions Decl Type List. in = Type. type Type int Type. type = INT Type real T. type = REAL List 1, id List 1. in = List. in, addtype(id. entry. List. in) List id addtype(id. entry, List. in) in= type=INT Type int List in= Input: int a, b, c CS 540 Spring 2009 GMU id =a , , id =c id =b 43
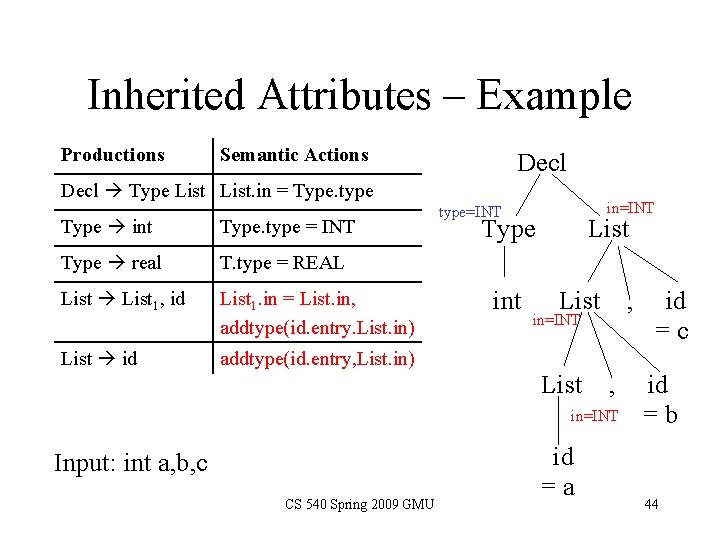
Inherited Attributes – Example Productions Semantic Actions Decl Type List. in = Type. type Type int Type. type = INT Type real T. type = REAL List 1, id List 1. in = List. in, addtype(id. entry. List. in) List id addtype(id. entry, List. in) in=INT type=INT Type int List , in=INT Input: int a, b, c CS 540 Spring 2009 GMU id =a id =c id =b 44
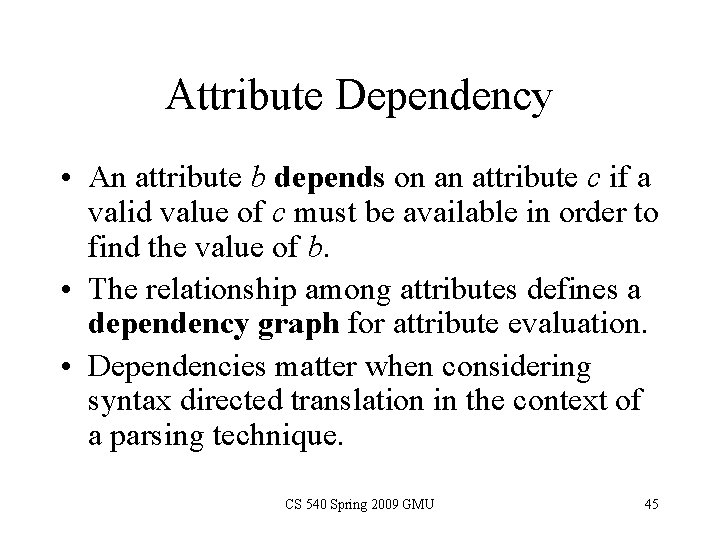
Attribute Dependency • An attribute b depends on an attribute c if a valid value of c must be available in order to find the value of b. • The relationship among attributes defines a dependency graph for attribute evaluation. • Dependencies matter when considering syntax directed translation in the context of a parsing technique. CS 540 Spring 2009 GMU 45
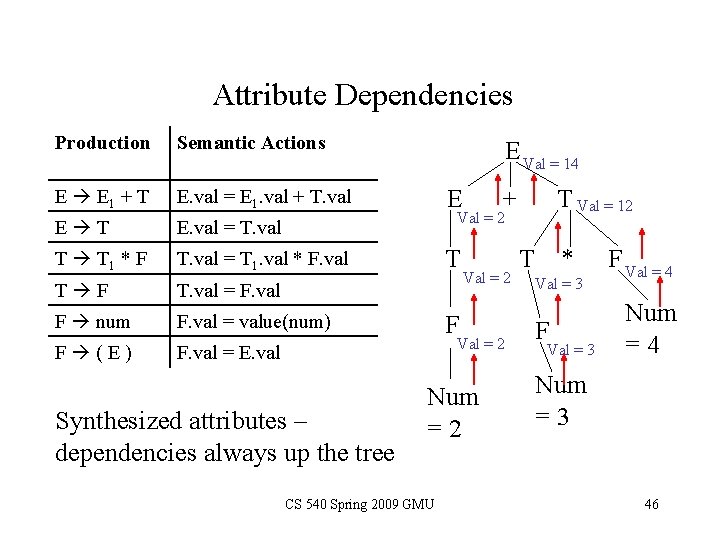
Attribute Dependencies Production Semantic Actions E E 1 + T E. val = E 1. val + T. val E T E. val = T. val T T 1 * F T. val = T 1. val * F. val T F T. val = F. val F num F. val = value(num) F (E) E Val = 14 E + Val = 2 T Val = 2 F. val = E. val Synthesized attributes – dependencies always up the tree T Val = 12 Num =2 CS 540 Spring 2009 GMU T * Val = 3 F Val = 4 Num =3 46
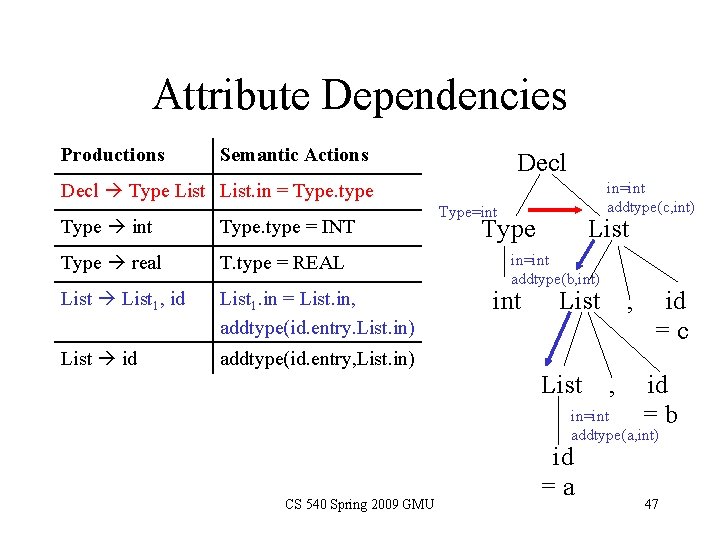
Attribute Dependencies Productions Semantic Actions Decl in=int addtype(c, int) Decl Type List. in = Type. type Type int Type. type = INT Type real T. type = REAL List 1, id List 1. in = List. in, addtype(id. entry. List. in) List id addtype(id. entry, List. in) Type=int Type List in=int addtype(b, int) int List , , id =c id =b in=int addtype(a, int) CS 540 Spring 2009 GMU id =a 47
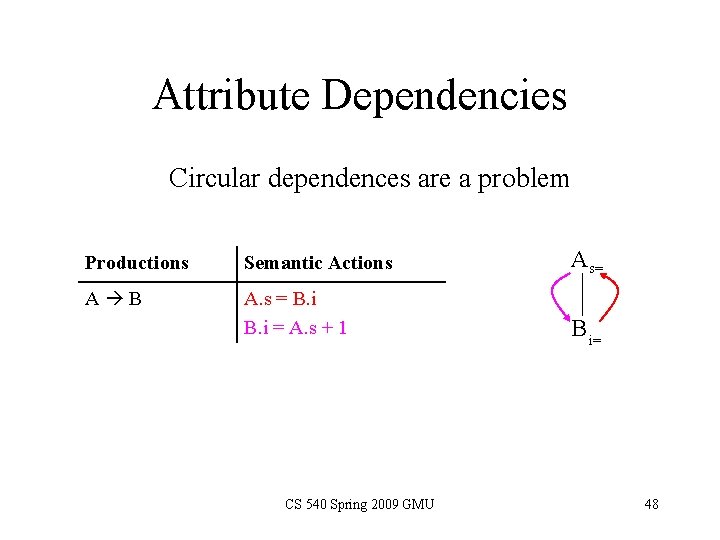
Attribute Dependencies Circular dependences are a problem Productions Semantic Actions A B A. s = B. i = A. s + 1 CS 540 Spring 2009 GMU As= B i= 48
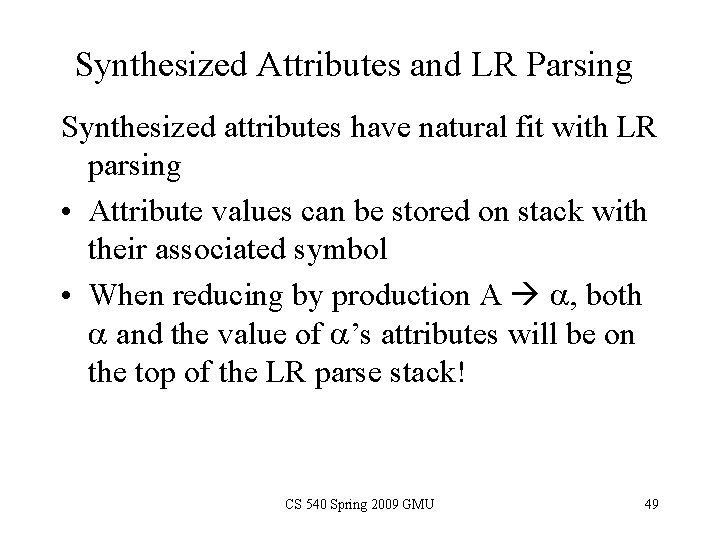
Synthesized Attributes and LR Parsing Synthesized attributes have natural fit with LR parsing • Attribute values can be stored on stack with their associated symbol • When reducing by production A a, both a and the value of a’s attributes will be on the top of the LR parse stack! CS 540 Spring 2009 GMU 49
![Synthesized Attributes and LR Parsing Example Stack 0attr a 1attr T 2attr b 5attr Synthesized Attributes and LR Parsing Example Stack: $0[attr], a 1[attr], T 2[attr], b 5[attr],](https://slidetodoc.com/presentation_image_h2/0d0f88dfb68db9c794658f8d7f762d86/image-50.jpg)
Synthesized Attributes and LR Parsing Example Stack: $0[attr], a 1[attr], T 2[attr], b 5[attr], c 8[attr] T Stack after T T b c: $0[attr], a 1[attr], T 2[attr’] a b b c T T a b CS 540 Spring 2009 GMU 50
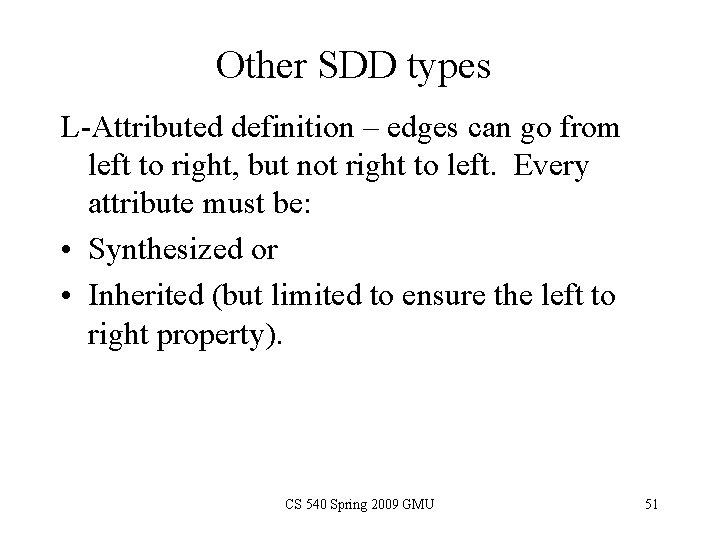
Other SDD types L-Attributed definition – edges can go from left to right, but not right to left. Every attribute must be: • Synthesized or • Inherited (but limited to ensure the left to right property). CS 540 Spring 2009 GMU 51
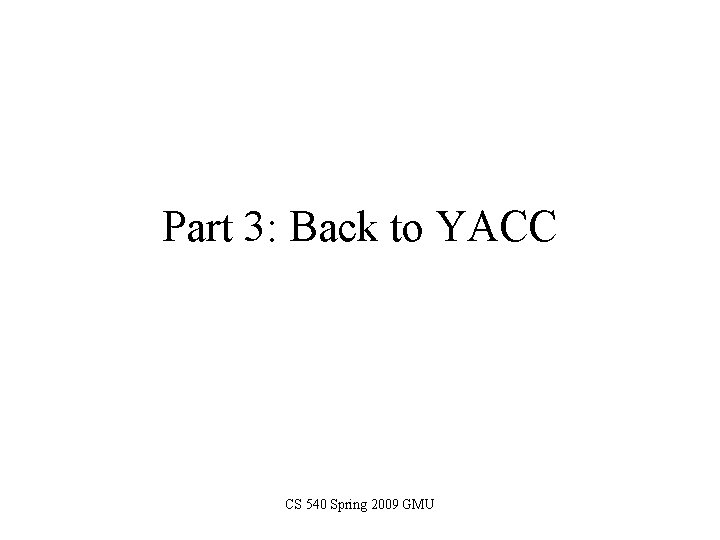
Part 3: Back to YACC CS 540 Spring 2009 GMU
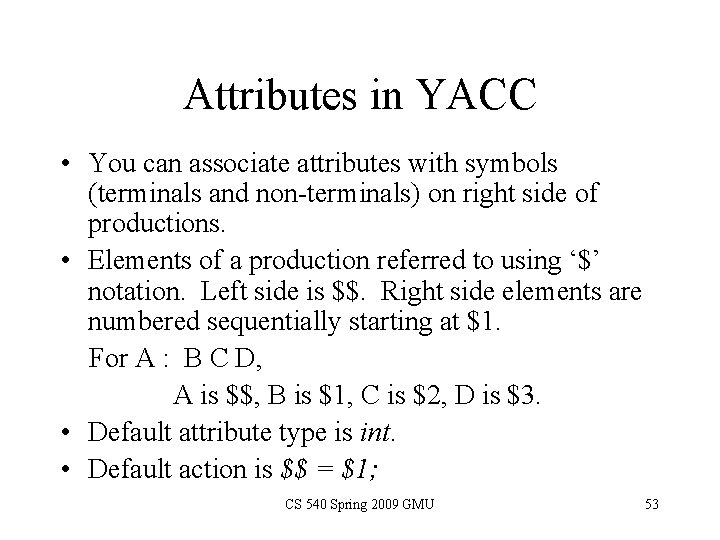
Attributes in YACC • You can associate attributes with symbols (terminals and non-terminals) on right side of productions. • Elements of a production referred to using ‘$’ notation. Left side is $$. Right side elements are numbered sequentially starting at $1. For A : B C D, A is $$, B is $1, C is $2, D is $3. • Default attribute type is int. • Default action is $$ = $1; CS 540 Spring 2009 GMU 53
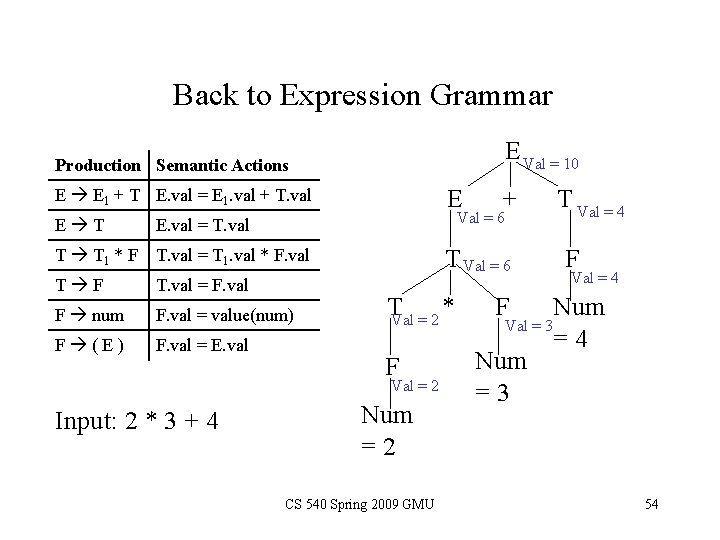
Back to Expression Grammar E Val = 10 Production Semantic Actions E E E 1 + T E. val = E 1. val + T. val E T Val = 6 E. val = T. val T Val = 6 T T 1 * F T. val = T 1. val * F. val T F T. val = F. val F num F. val = value(num) F (E) F. val = E. val TVal = 2 * F Val = 2 Input: 2 * 3 + 4 + Num =2 CS 540 Spring 2009 GMU T Val = 4 F Num Val = 3 =4 Num =3 54
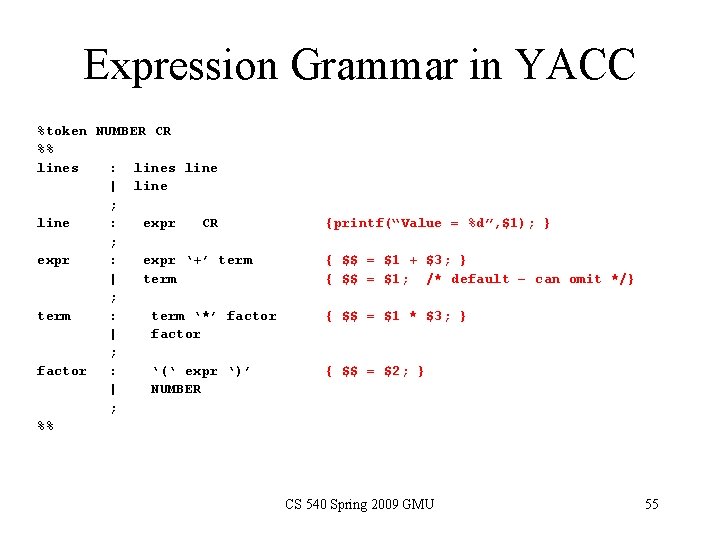
Expression Grammar in YACC %token NUMBER CR %% lines : lines line | line ; line : expr CR ; expr : expr ‘+’ term | term ; term : term ‘*’ factor | factor ; factor : ‘(‘ expr ‘)’ | NUMBER ; %% {printf(“Value = %d”, $1); } { $$ = $1 + $3; } { $$ = $1; /* default – can omit */} { $$ = $1 * $3; } { $$ = $2; } CS 540 Spring 2009 GMU 55
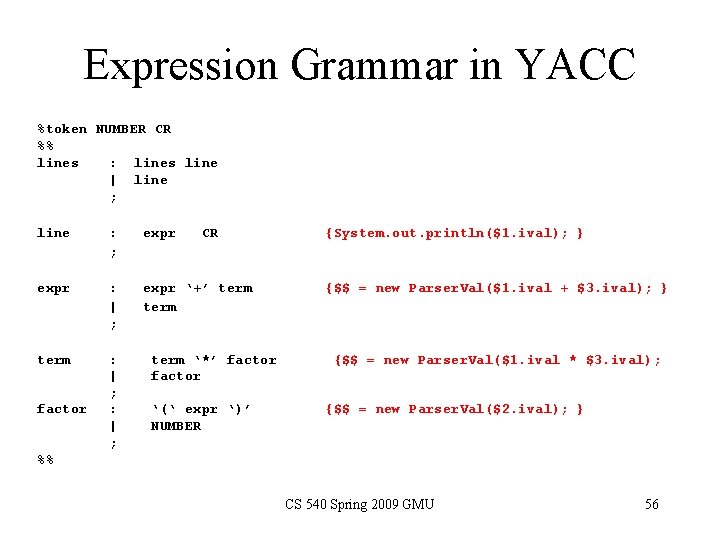
Expression Grammar in YACC %token NUMBER CR %% lines : lines line | line ; line : ; expr : | ; expr ‘+’ term : | ; factor CR term ‘*’ factor ‘(‘ expr ‘)’ NUMBER {System. out. println($1. ival); } {$$ = new Parser. Val($1. ival + $3. ival); } {$$ = new Parser. Val($1. ival * $3. ival); {$$ = new Parser. Val($2. ival); } %% CS 540 Spring 2009 GMU 56
![Associated Lex Specification 0 9 n t Associated Lex Specification %% + * ( ) [0 -9]+ [n] [ t] %%](https://slidetodoc.com/presentation_image_h2/0d0f88dfb68db9c794658f8d7f762d86/image-57.jpg)
Associated Lex Specification %% + * ( ) [0 -9]+ [n] [ t] %% {return(‘+’); } {return(‘*’); } {return(‘(’); } {return(‘)’); } {yylval = atoi(yytext); return(NUMBER); } {return(CR); } ; In Java: yyparser. yylval = new Parser. Val(Integer. parse. Int(yytext())); return Parser. INT; CS 540 Spring 2009 GMU 57
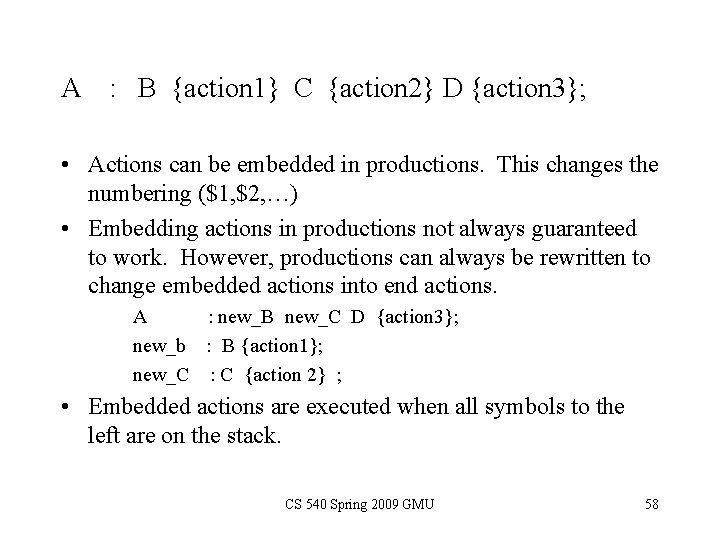
A : B {action 1} C {action 2} D {action 3}; • Actions can be embedded in productions. This changes the numbering ($1, $2, …) • Embedding actions in productions not always guaranteed to work. However, productions can always be rewritten to change embedded actions into end actions. A : new_B new_C D {action 3}; new_b : B {action 1}; new_C : C {action 2} ; • Embedded actions are executed when all symbols to the left are on the stack. CS 540 Spring 2009 GMU 58
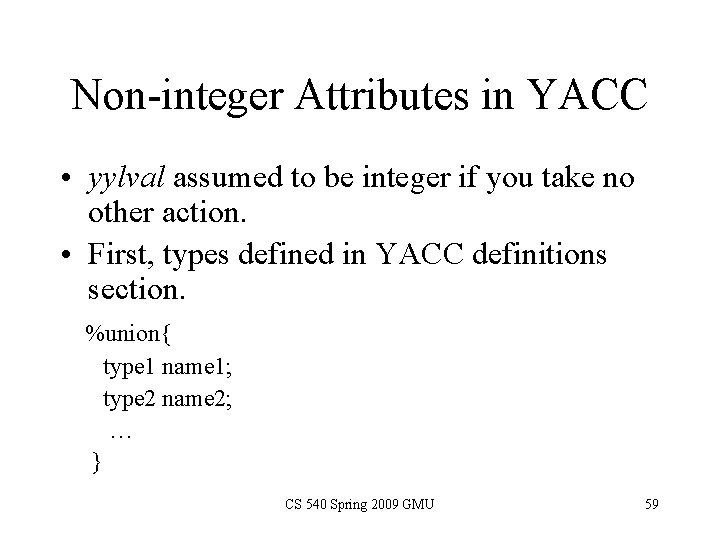
Non-integer Attributes in YACC • yylval assumed to be integer if you take no other action. • First, types defined in YACC definitions section. %union{ type 1 name 1; type 2 name 2; … } CS 540 Spring 2009 GMU 59
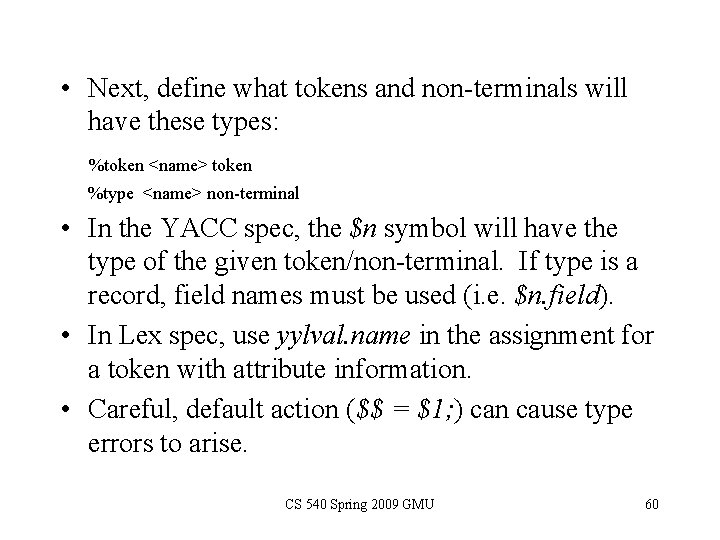
• Next, define what tokens and non-terminals will have these types: %token <name> token %type <name> non-terminal • In the YACC spec, the $n symbol will have the type of the given token/non-terminal. If type is a record, field names must be used (i. e. $n. field). • In Lex spec, use yylval. name in the assignment for a token with attribute information. • Careful, default action ($$ = $1; ) can cause type errors to arise. CS 540 Spring 2009 GMU 60
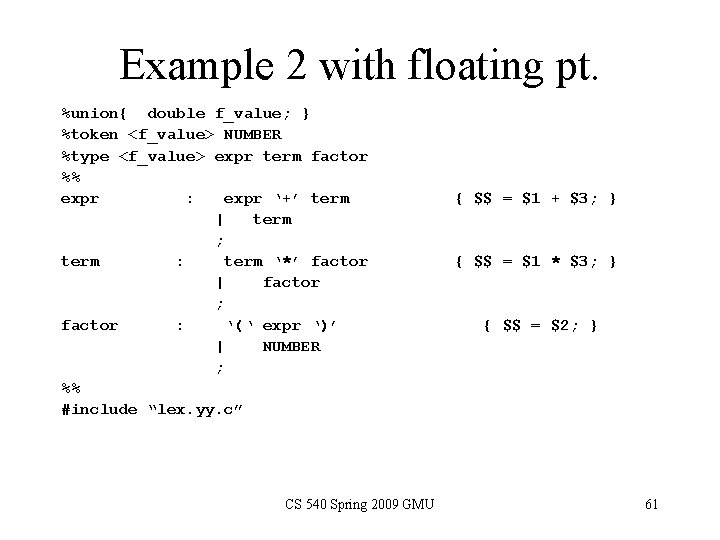
Example 2 with floating pt. %union{ double f_value; } %token <f_value> NUMBER %type <f_value> expr term factor %% expr : expr ‘+’ term | term ; term : term ‘*’ factor | factor ; factor : ‘(‘ expr ‘)’ | NUMBER ; %% #include “lex. yy. c” CS 540 Spring 2009 GMU { $$ = $1 + $3; } { $$ = $1 * $3; } { $$ = $2; } 61
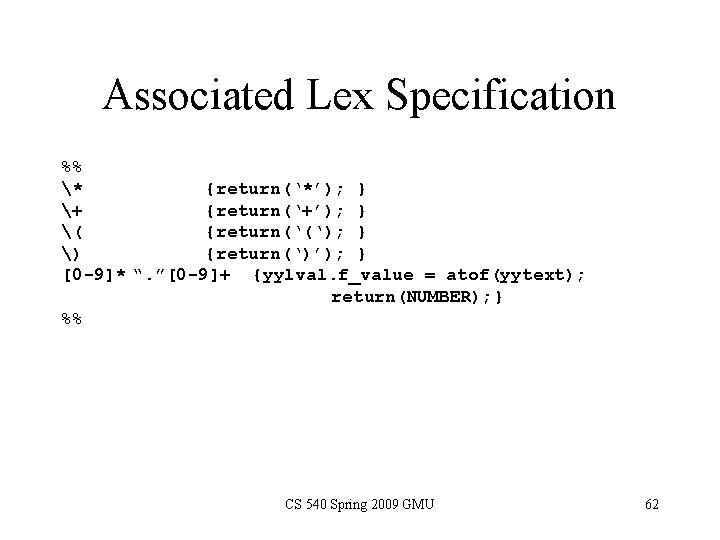
Associated Lex Specification %% * {return(‘*’); } + {return(‘+’); } ( {return(‘(‘); } ) {return(‘)’); } [0 -9]* “. ”[0 -9]+ {yylval. f_value = atof(yytext); return(NUMBER); } %% CS 540 Spring 2009 GMU 62
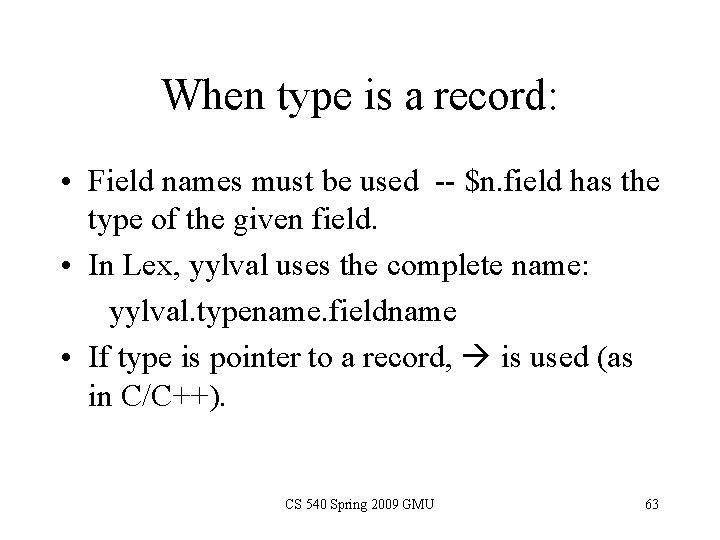
When type is a record: • Field names must be used -- $n. field has the type of the given field. • In Lex, yylval uses the complete name: yylval. typename. fieldname • If type is pointer to a record, is used (as in C/C++). CS 540 Spring 2009 GMU 63
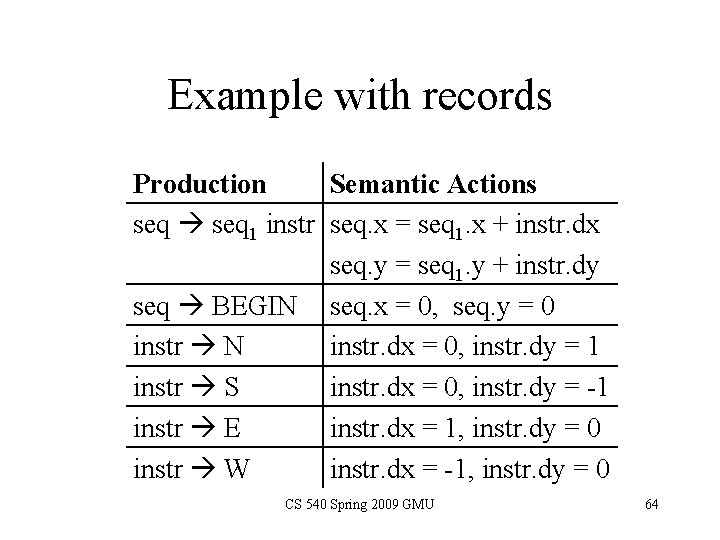
Example with records Production Semantic Actions seq 1 instr seq. x = seq 1. x + instr. dx seq. y = seq 1. y + instr. dy seq BEGIN seq. x = 0, seq. y = 0 instr N instr. dx = 0, instr. dy = 1 instr S instr. dx = 0, instr. dy = -1 instr E instr. dx = 1, instr. dy = 0 instr W instr. dx = -1, instr. dy = 0 CS 540 Spring 2009 GMU 64
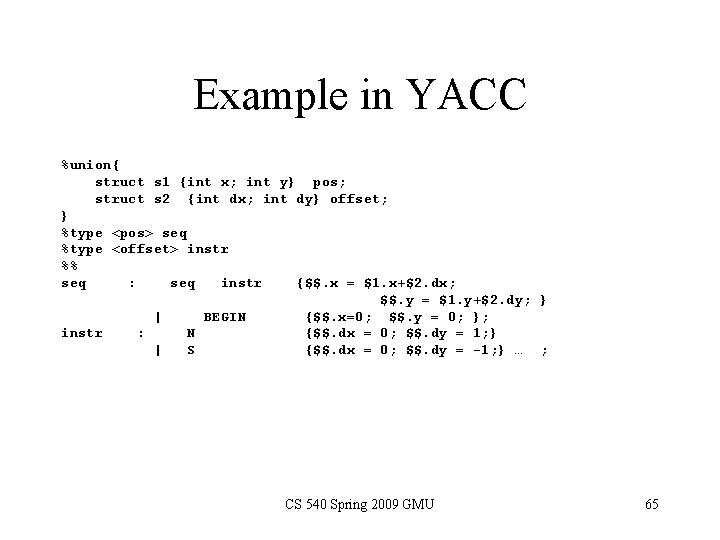
Example in YACC %union{ struct s 1 {int x; int y} pos; struct s 2 {int dx; int dy} offset; } %type <pos> seq %type <offset> instr %% seq : seq instr {$$. x = $1. x+$2. dx; $$. y = $1. y+$2. dy; } | BEGIN {$$. x=0; $$. y = 0; }; instr : N {$$. dx = 0; $$. dy = 1; } | S {$$. dx = 0; $$. dy = -1; } … ; CS 540 Spring 2009 GMU 65
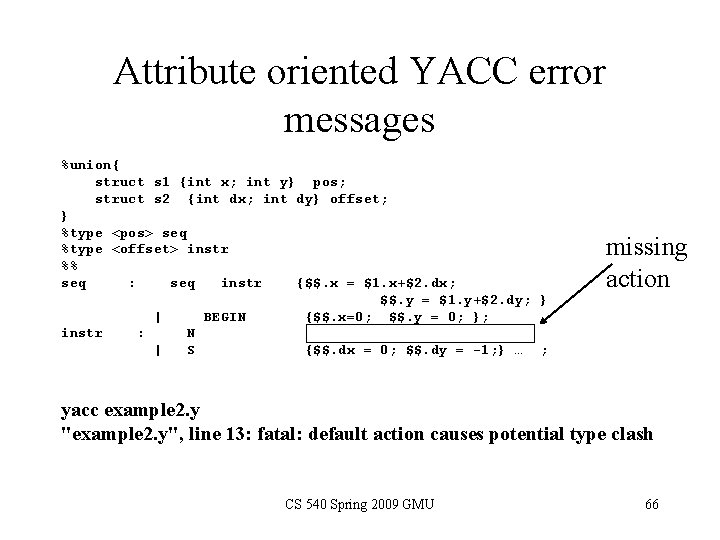
Attribute oriented YACC error messages %union{ struct s 1 {int x; int y} pos; struct s 2 {int dx; int dy} offset; } %type <pos> seq %type <offset> instr %% seq : seq instr {$$. x = $1. x+$2. dx; $$. y = $1. y+$2. dy; } | BEGIN {$$. x=0; $$. y = 0; }; instr : N | S {$$. dx = 0; $$. dy = -1; } … ; missing action yacc example 2. y "example 2. y", line 13: fatal: default action causes potential type clash CS 540 Spring 2009 GMU 66
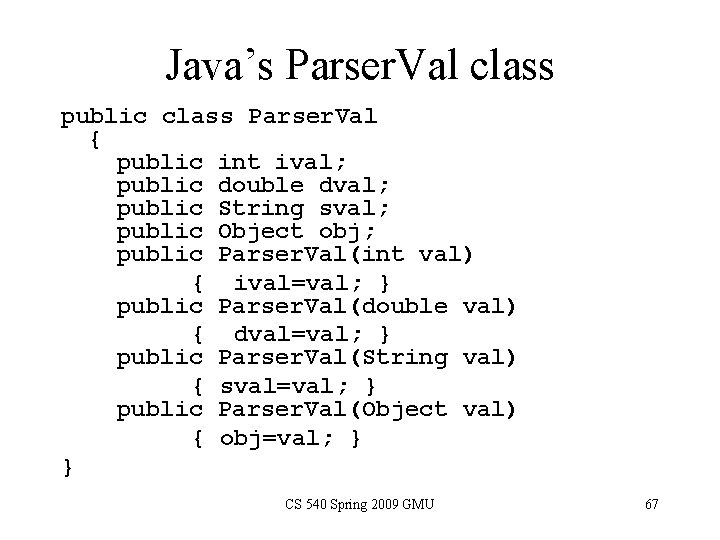
Java’s Parser. Val class public class Parser. Val { public int ival; public double dval; public String sval; public Object obj; public Parser. Val(int val) { ival=val; } public Parser. Val(double val) { dval=val; } public Parser. Val(String val) { sval=val; } public Parser. Val(Object val) { obj=val; } } CS 540 Spring 2009 GMU 67
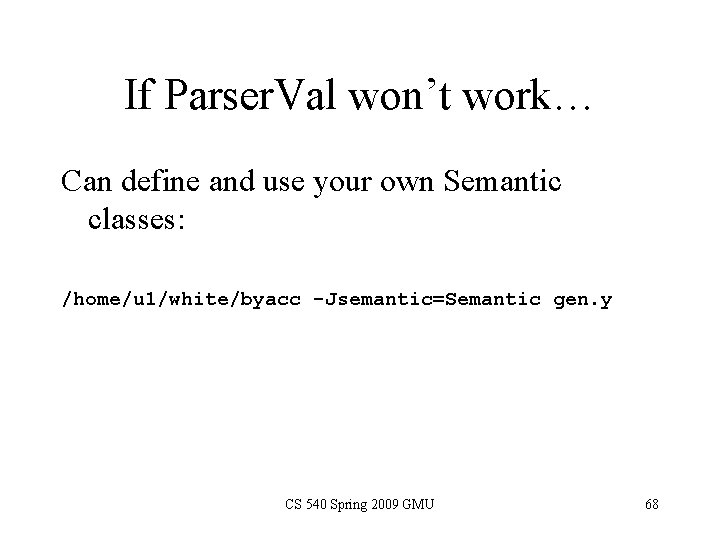
If Parser. Val won’t work… Can define and use your own Semantic classes: /home/u 1/white/byacc -Jsemantic=Semantic gen. y CS 540 Spring 2009 GMU 68
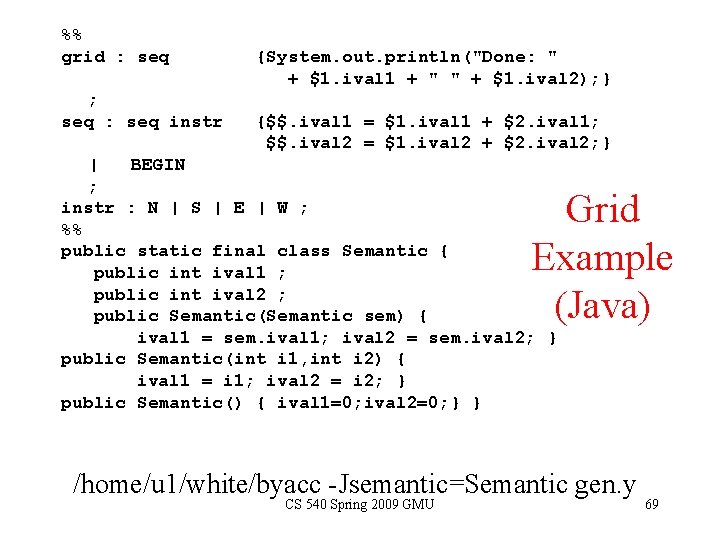
%% grid : seq ; seq : seq instr {System. out. println("Done: " + $1. ival 1 + " " + $1. ival 2); } {$$. ival 1 = $1. ival 1 + $2. ival 1; $$. ival 2 = $1. ival 2 + $2. ival 2; } | BEGIN ; instr : N | S | E | W ; %% public static final class Semantic { public int ival 1 ; public int ival 2 ; public Semantic(Semantic sem) { ival 1 = sem. ival 1; ival 2 = sem. ival 2; } public Semantic(int i 1, int i 2) { ival 1 = i 1; ival 2 = i 2; } public Semantic() { ival 1=0; ival 2=0; } } Grid Example (Java) /home/u 1/white/byacc -Jsemantic=Semantic gen. y CS 540 Spring 2009 GMU 69
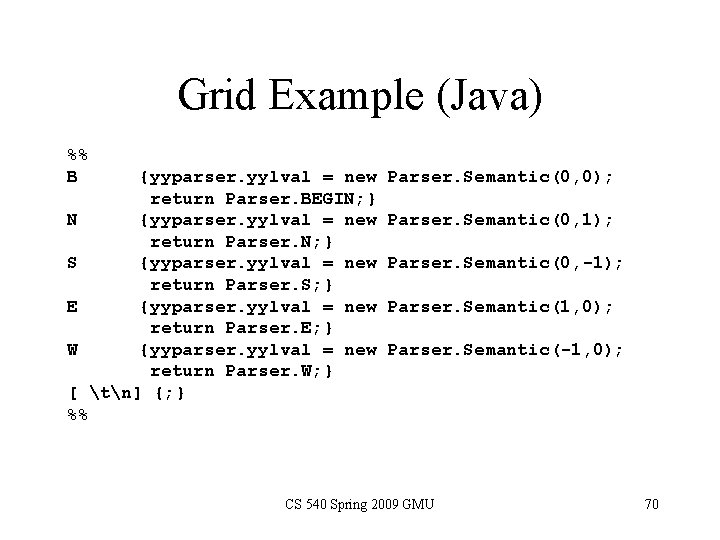
Grid Example (Java) %% B Parser. Semantic(0, 0); N Parser. Semantic(0, 1); S {yyparser. yylval = new return Parser. BEGIN; } {yyparser. yylval = new return Parser. S; } {yyparser. yylval = new return Parser. E; } {yyparser. yylval = new return Parser. W; } tn] {; } E W Parser. Semantic(0, -1); Parser. Semantic(1, 0); Parser. Semantic(-1, 0); [ %% CS 540 Spring 2009 GMU 70
Syntax directed definition
Sdt in compiler design
Syntax directed defination
Syntax directed definition
01:640:244 lecture notes - lecture 15: plat, idah, farad
Lex yacc tutorial
Lex yacc calculator
Symbol table implementation in lex and yacc
What is semantic translation?
Linear parent function
Translation lecture notes
Introduction to yacc
Yacc
Yacc program
Lexical analyzer 구현
Lex yacc example
Lex symbol
Yacc tutorial
What is yacc in compiler design
What is yacc
Lex & yacc
Yylval in yacc
Ntu lhs
Number translation using voice translation profiles
Semantic translation và communicative translation
Veterans directed home and community based care
Directed mutagenesis and protein engineering
Directed putaway and picking
Directed number
Directed paraphrasing
Veterans directed home and community based care
50 examples of direct and indirect speech exercises
How to find the unit vector
Child directed speech definition
Self directed search free
Professor john holland's self directed search
Romeo and juliet act 5 analysis
Compliant personality
Goal-directed personas
Metamorphic
Local markov assumption
Lvn scope
Self directed play
Adjacency matrix directed graph
The natural order
Sglib1
Confining pressure vs directed pressure
Goal-directed design
Direct motion specification in computer graphics
Affective play space
Positive number definition
Partition point formula
Directed line segments
Nrich maths
Directed number definition
Directed school laboratory sae
Self directed play
Interview questions for self-directed learning
Low and high uncertainty avoidance
Pseudograph
Force-directed algorithm
Working memory graphs
Directed number exercise
Define digraph
Surveillance detection routes
Directed number definition
Dari gambar diatas yang termasuk trail adalah
Oligonucleotide directed mutagenesis
Homework 4 partitioning a segment
An inner drive that directs behavior
Chapter 2 planning your career