Java Exception Handling errors using Javas exception handling
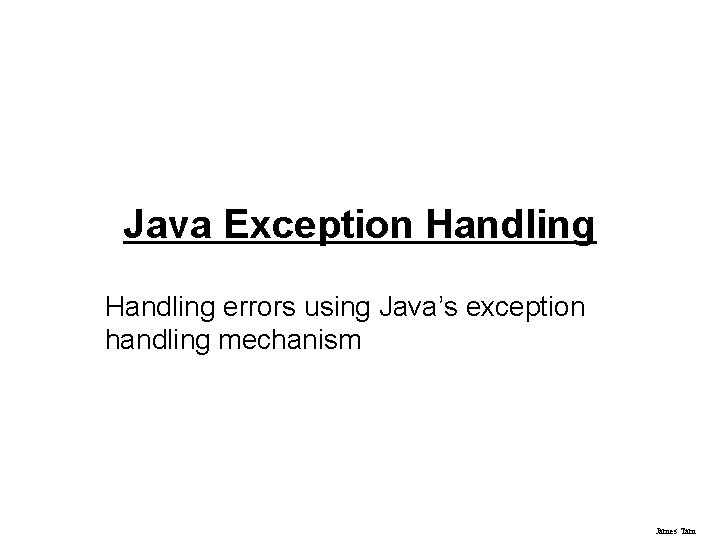
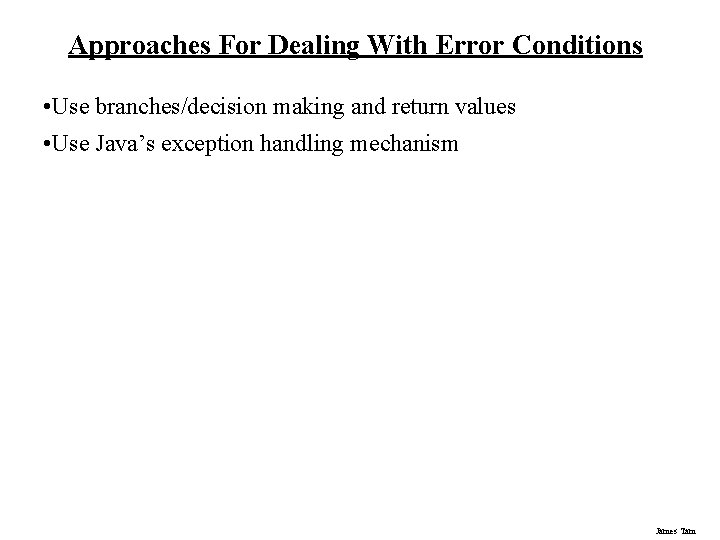
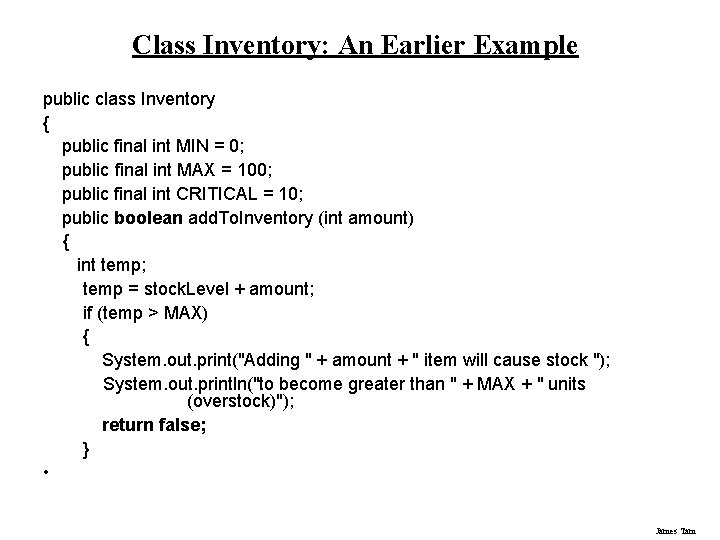
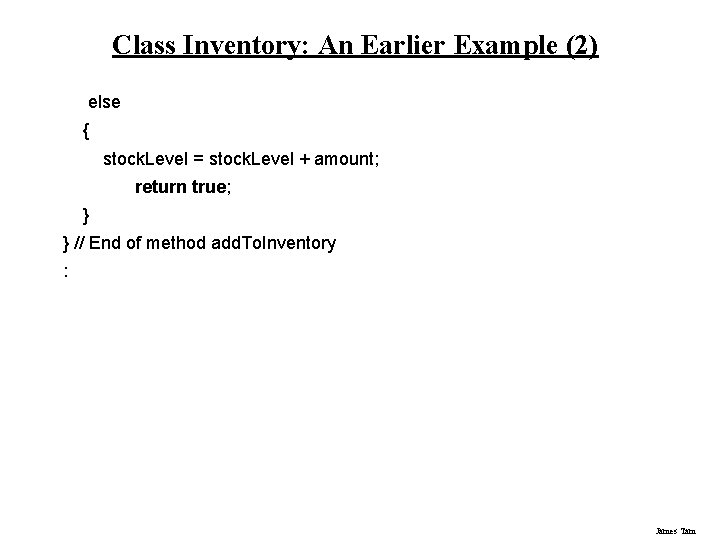
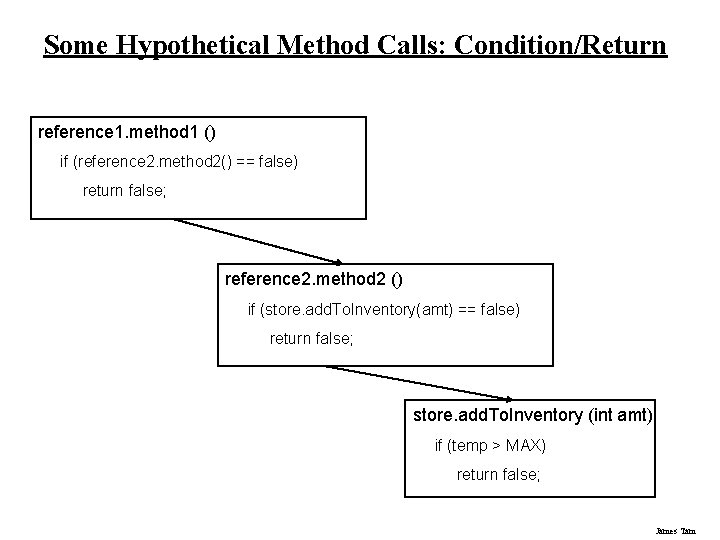
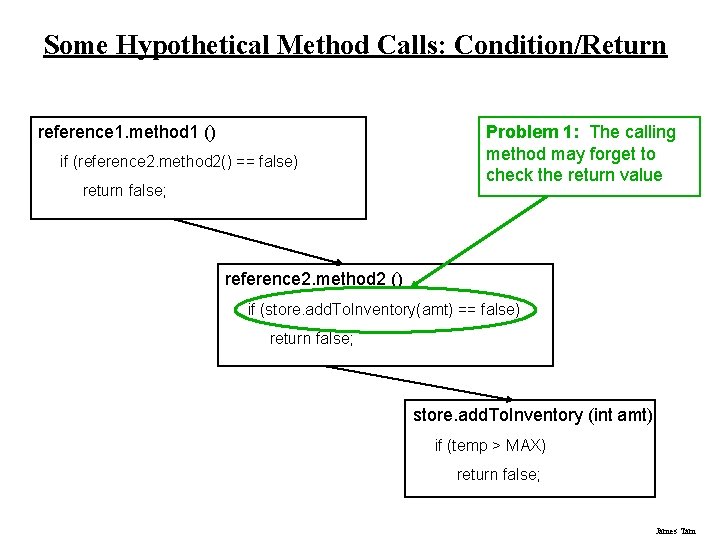
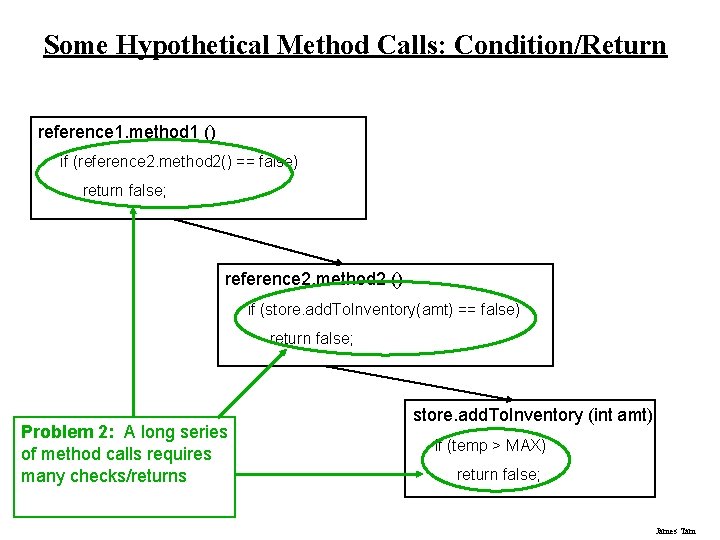
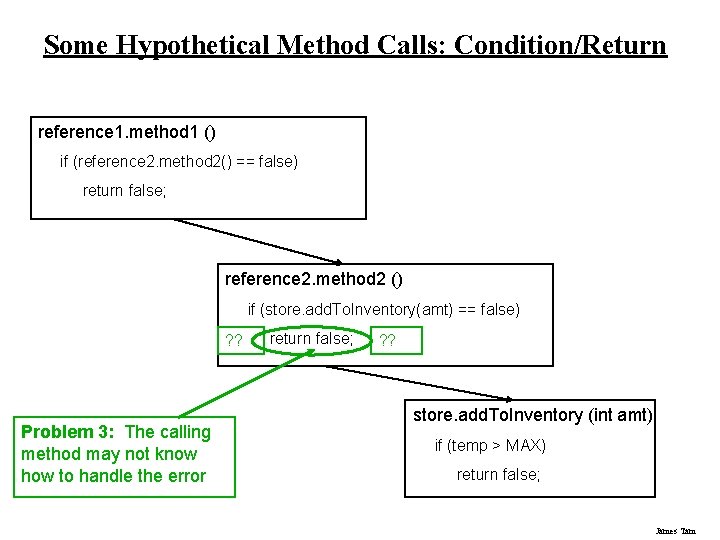
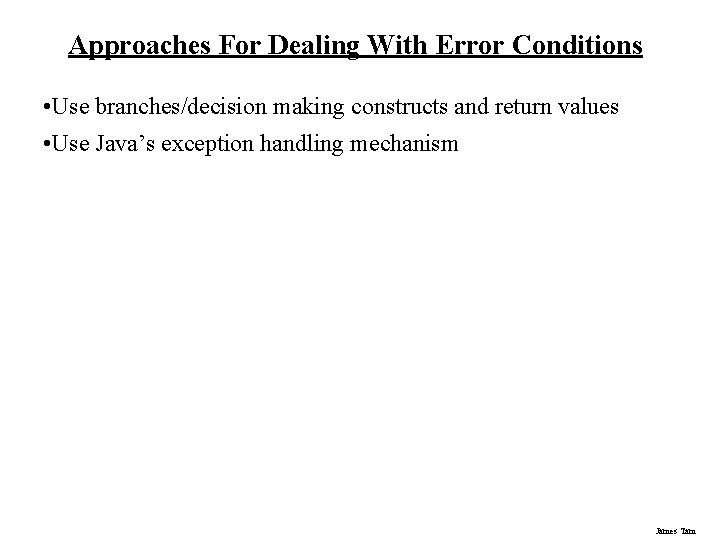
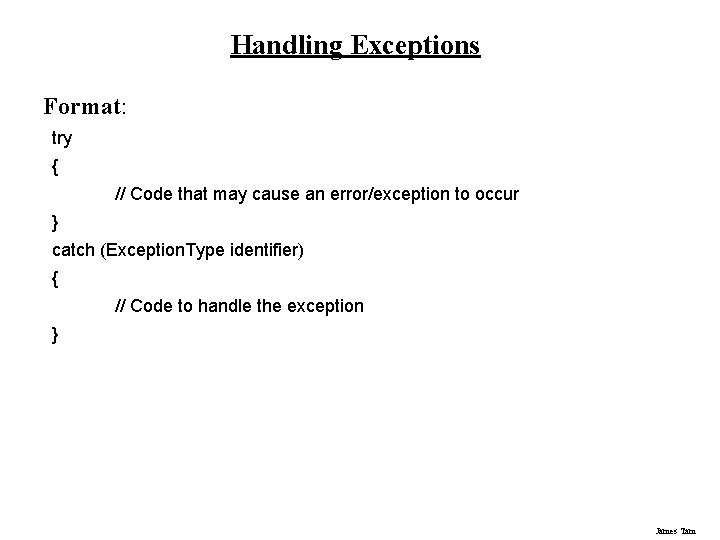
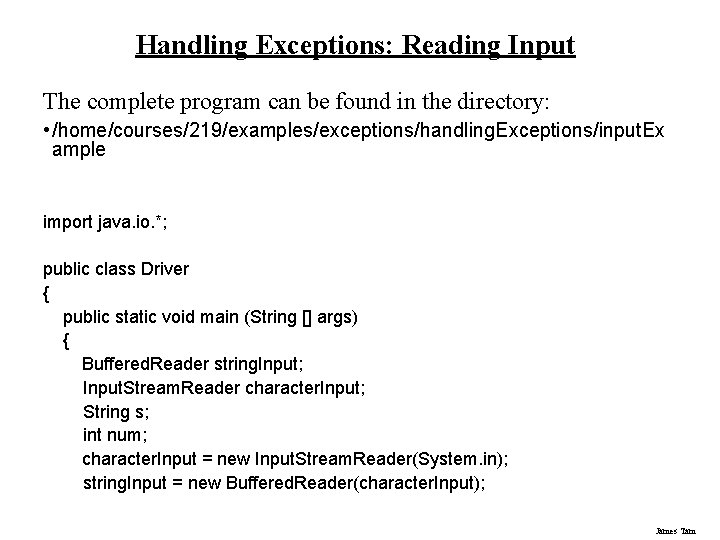
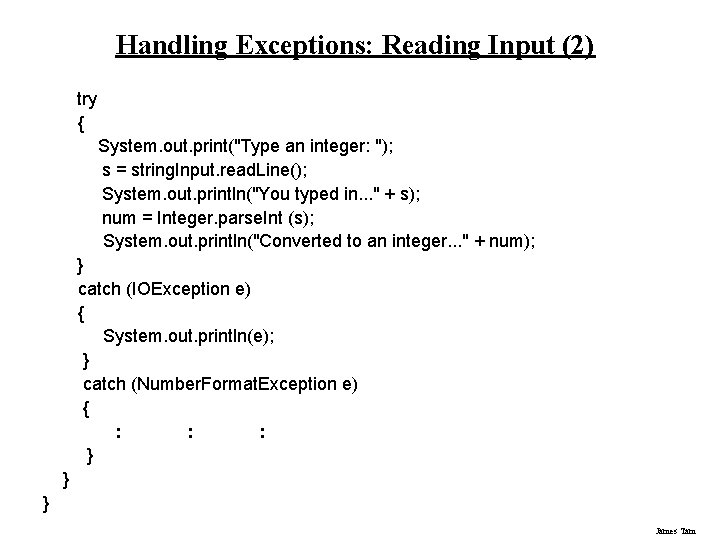
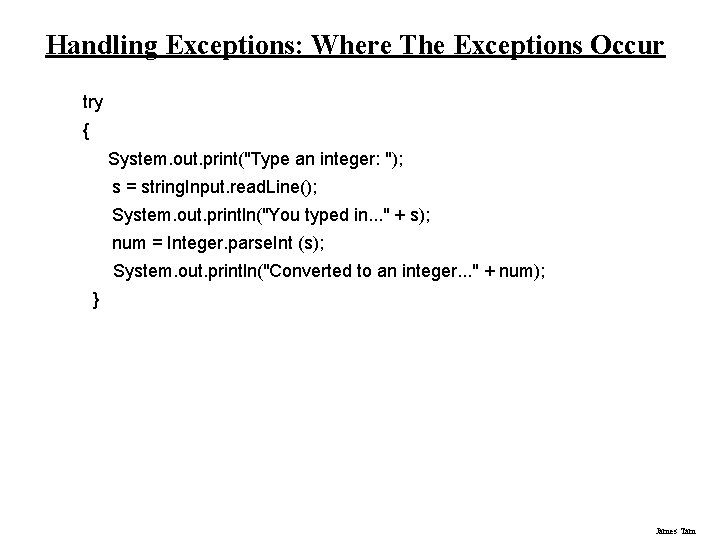
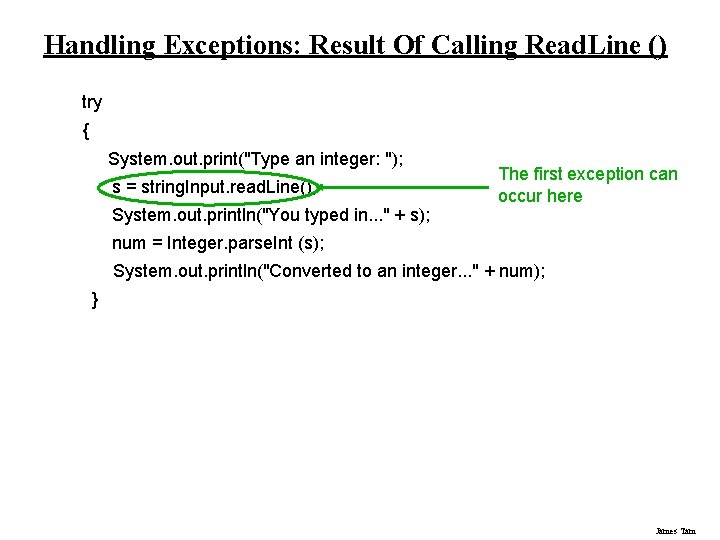
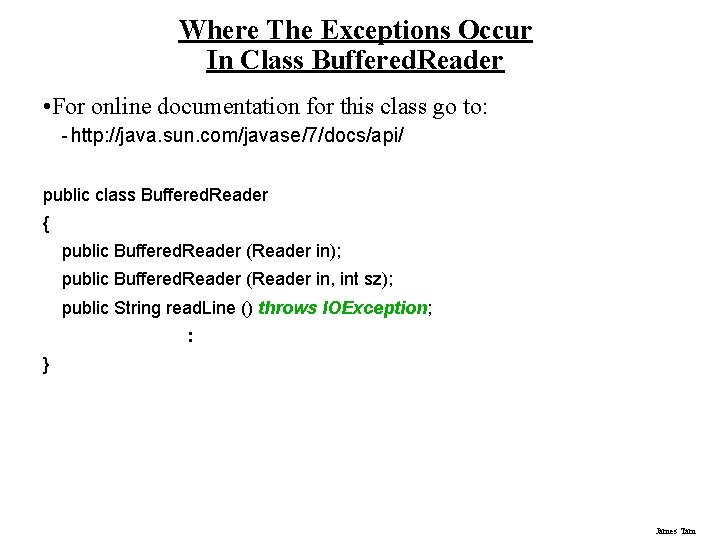
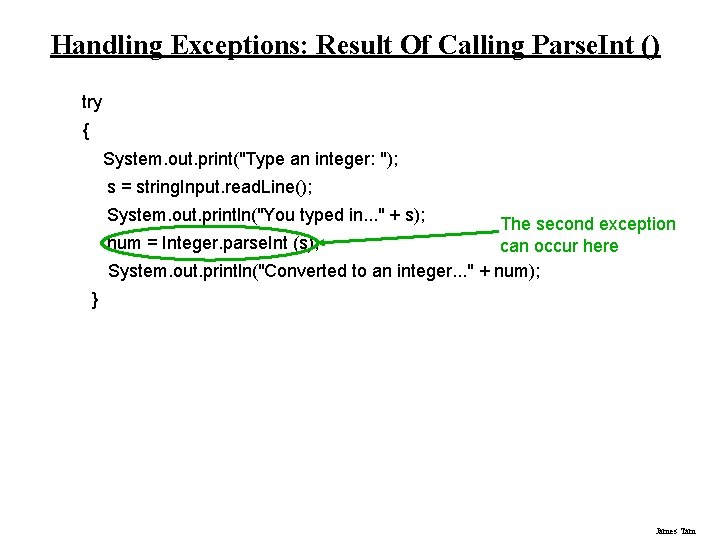
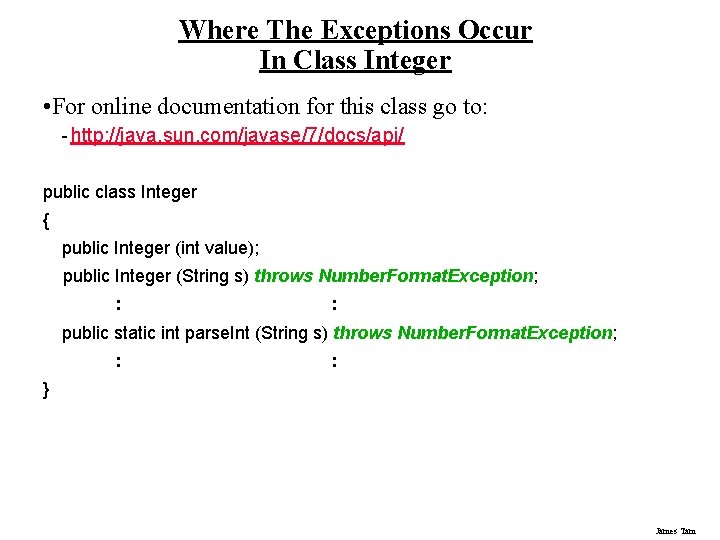
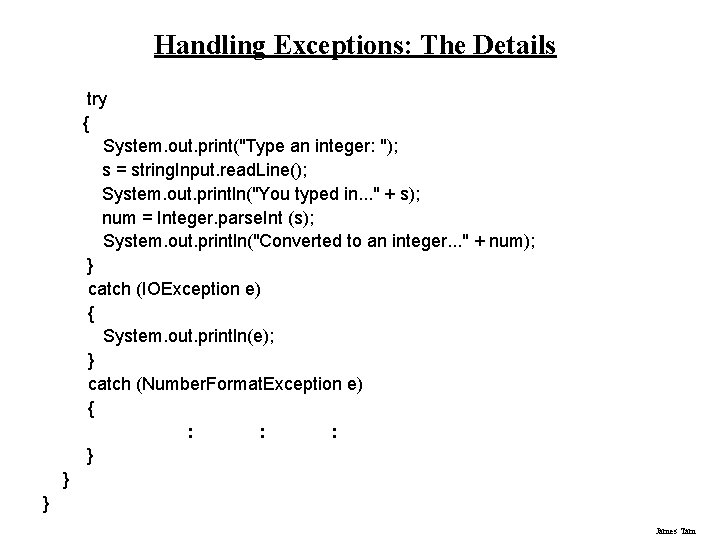
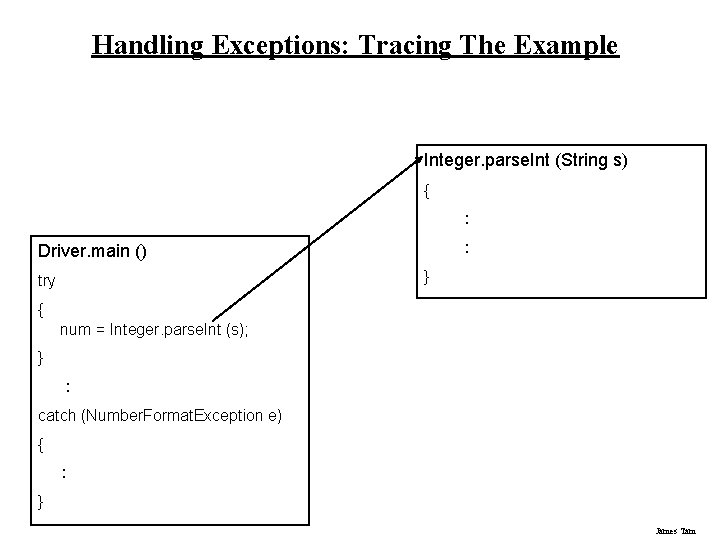
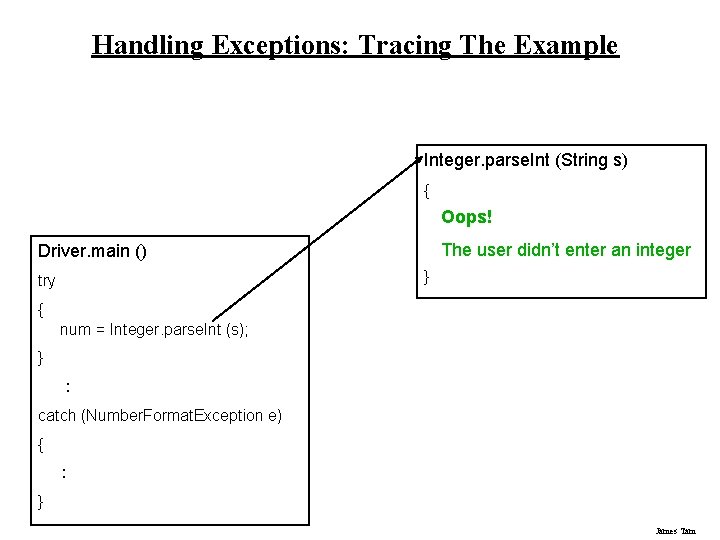
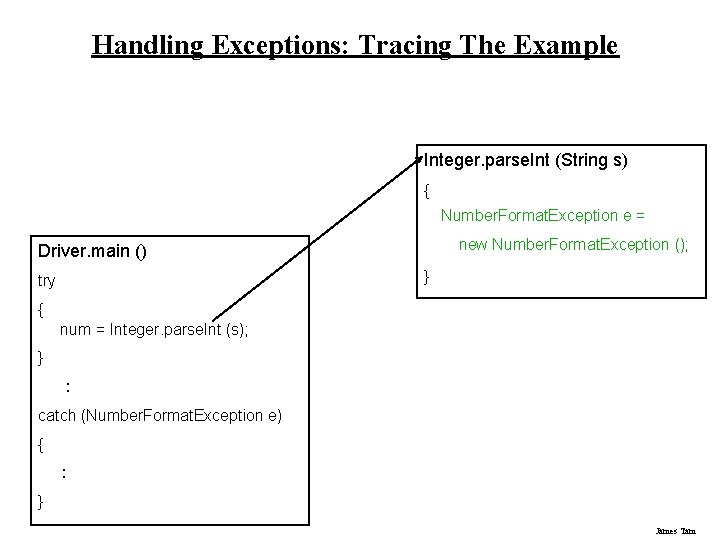
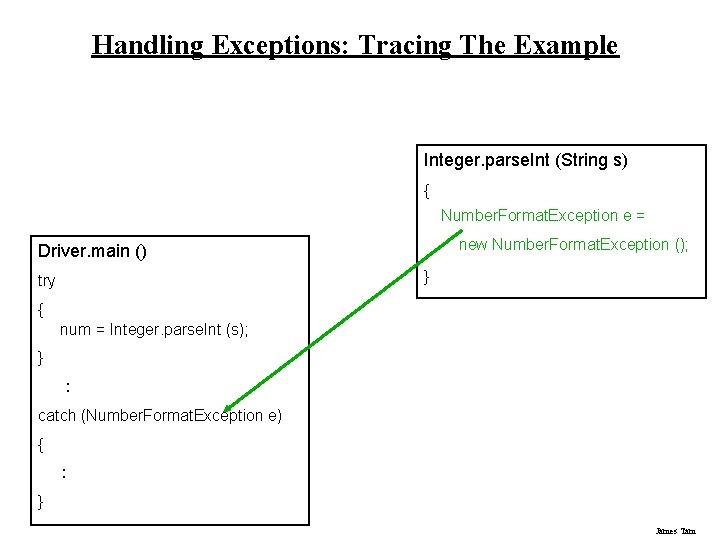
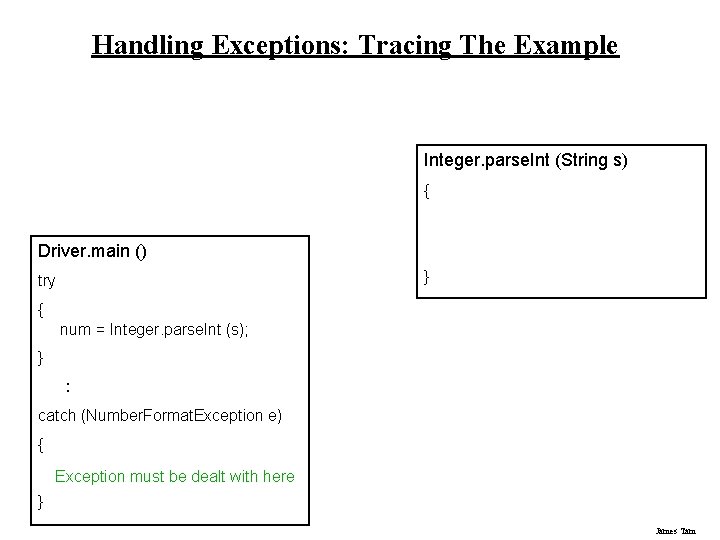
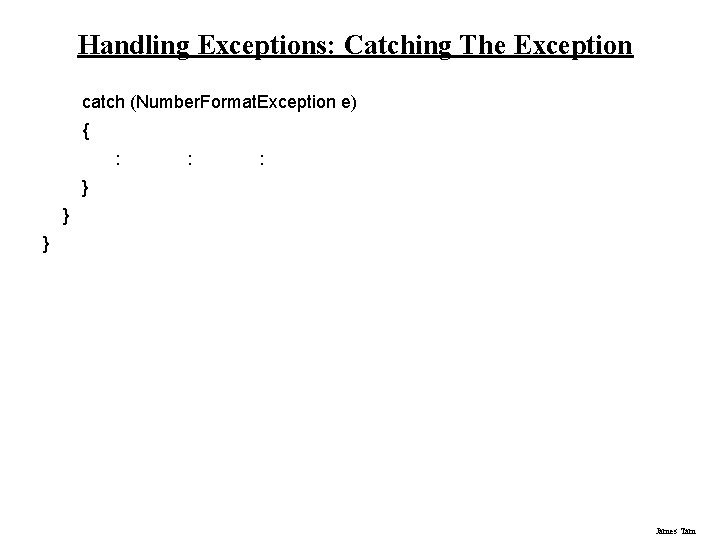
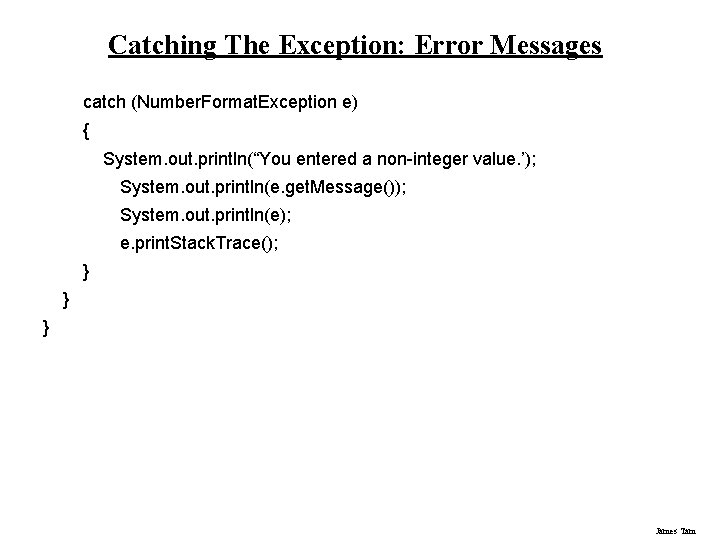
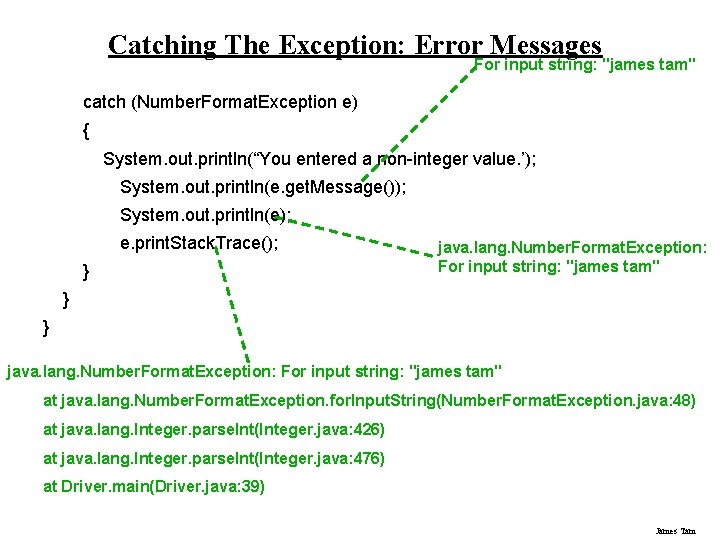
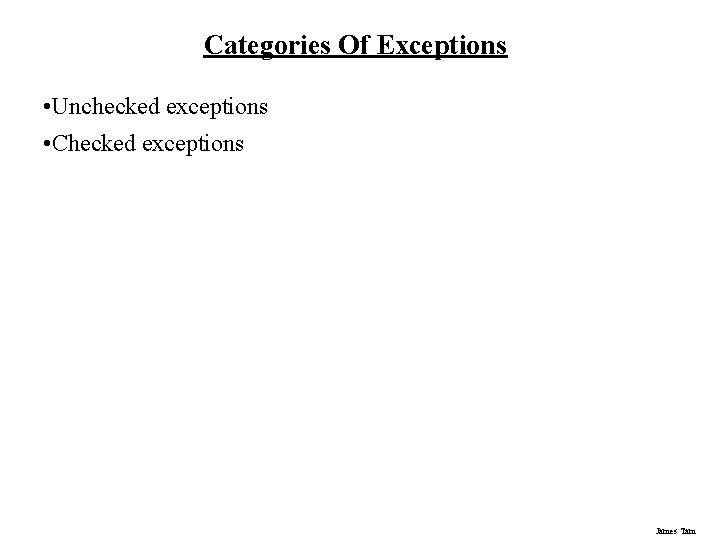
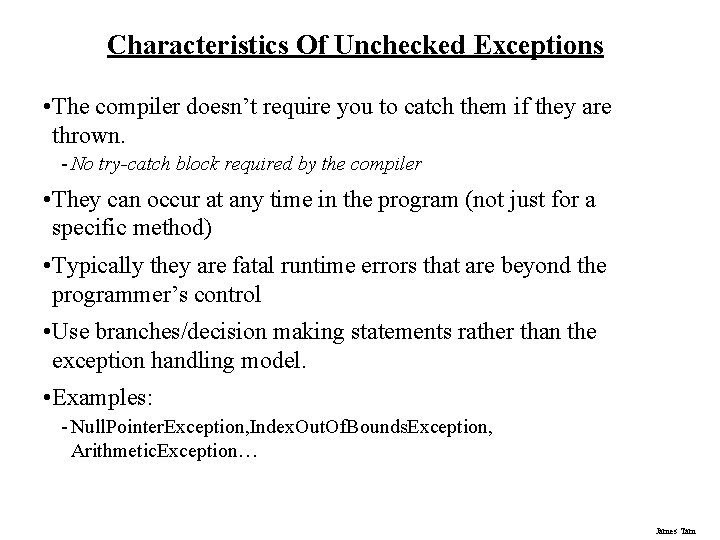
![Common Unchecked Exceptions: Null. Pointer. Exception int [] arr = null; arr[0] = 1; Common Unchecked Exceptions: Null. Pointer. Exception int [] arr = null; arr[0] = 1;](https://slidetodoc.com/presentation_image_h2/15f4f1d7fe6d7195bcb7ddb617836069/image-29.jpg)
![Common Unchecked Exceptions: Array. Index. Out. Of. Bounds. Exception int [] arr = null; Common Unchecked Exceptions: Array. Index. Out. Of. Bounds. Exception int [] arr = null;](https://slidetodoc.com/presentation_image_h2/15f4f1d7fe6d7195bcb7ddb617836069/image-30.jpg)
![Common Unchecked Exceptions: Arithmetic. Exceptions int [] arr = null; arr[0] = 1; arr Common Unchecked Exceptions: Arithmetic. Exceptions int [] arr = null; arr[0] = 1; arr](https://slidetodoc.com/presentation_image_h2/15f4f1d7fe6d7195bcb7ddb617836069/image-31.jpg)
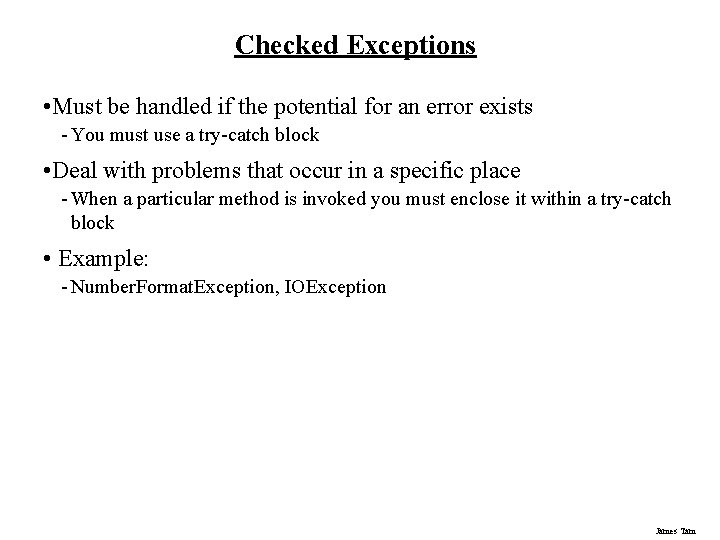
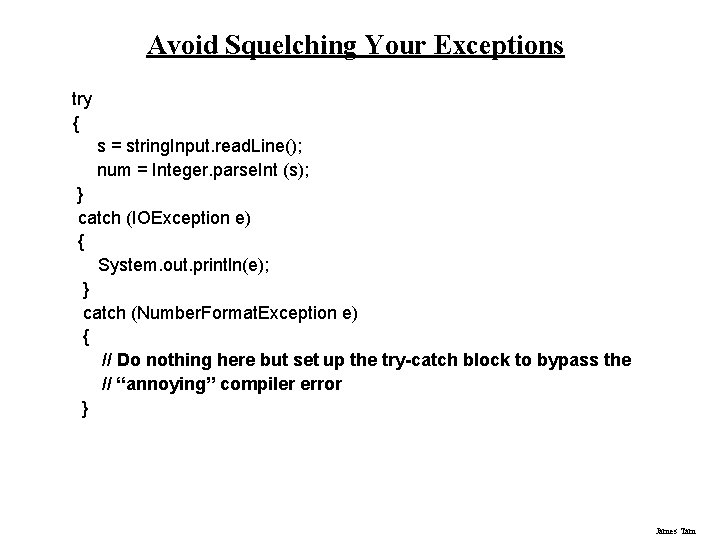
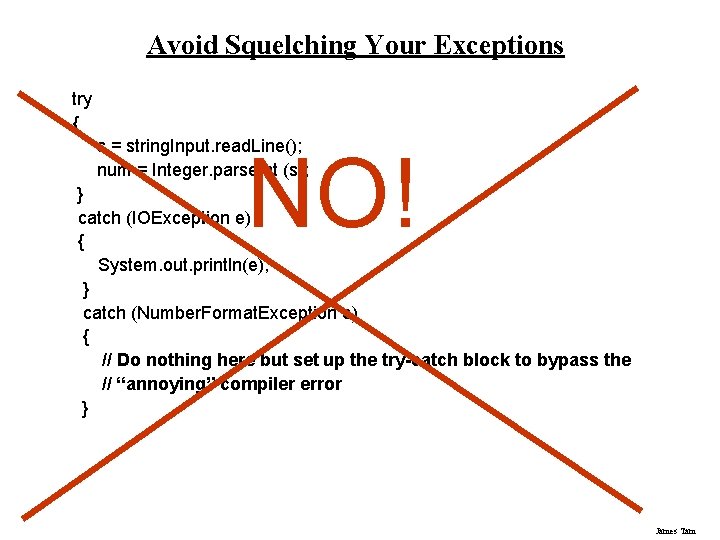
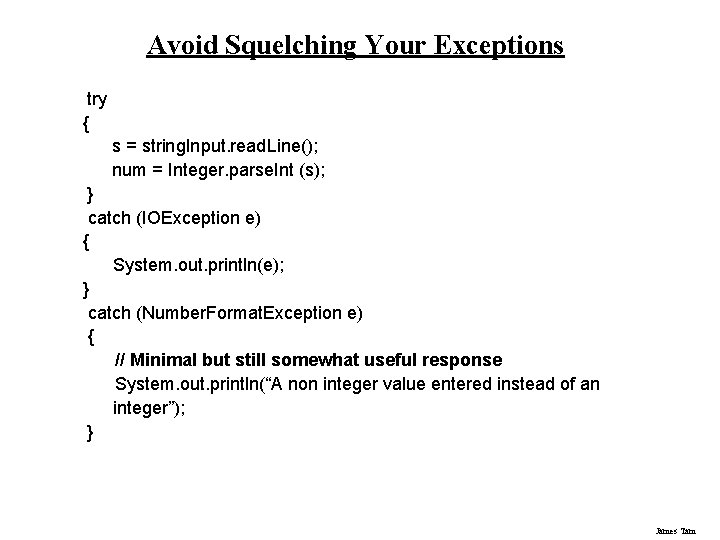
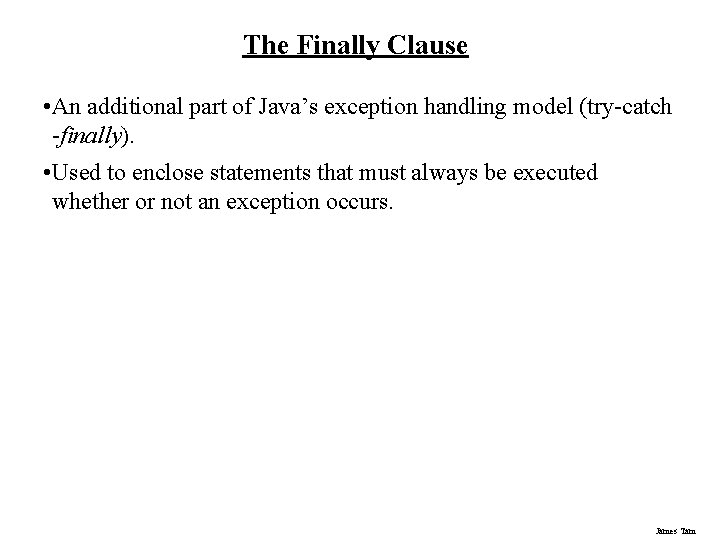
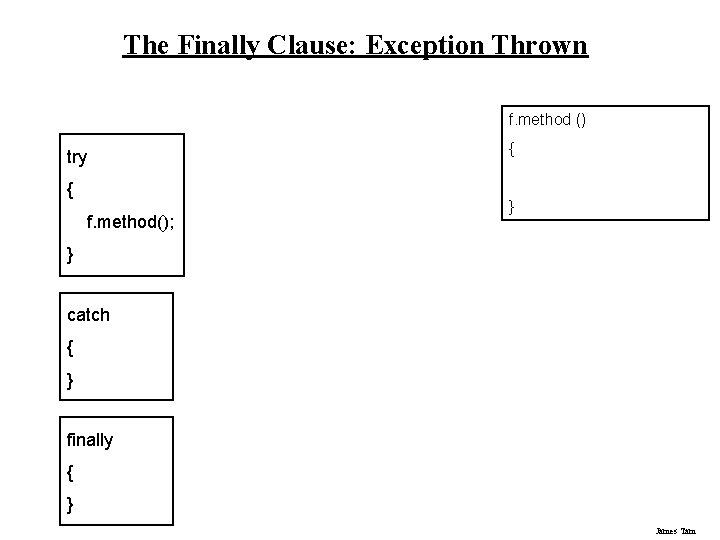
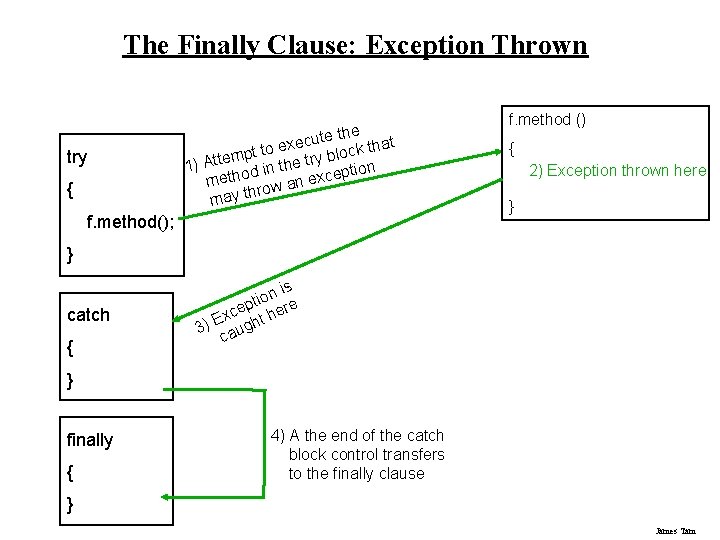
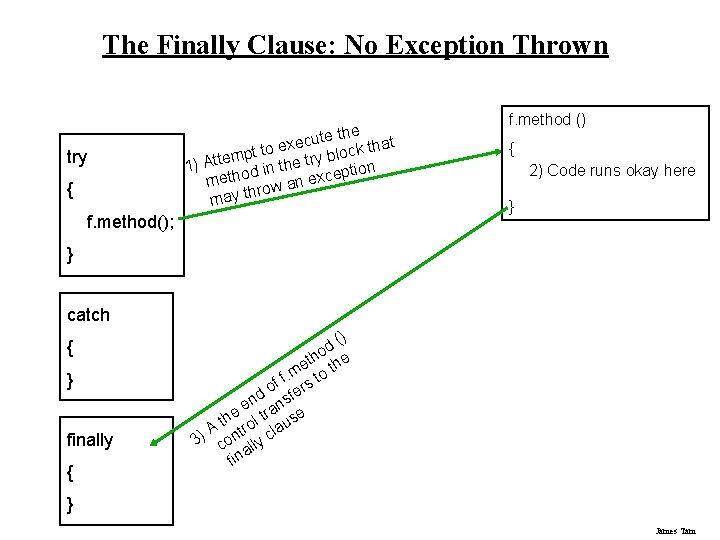
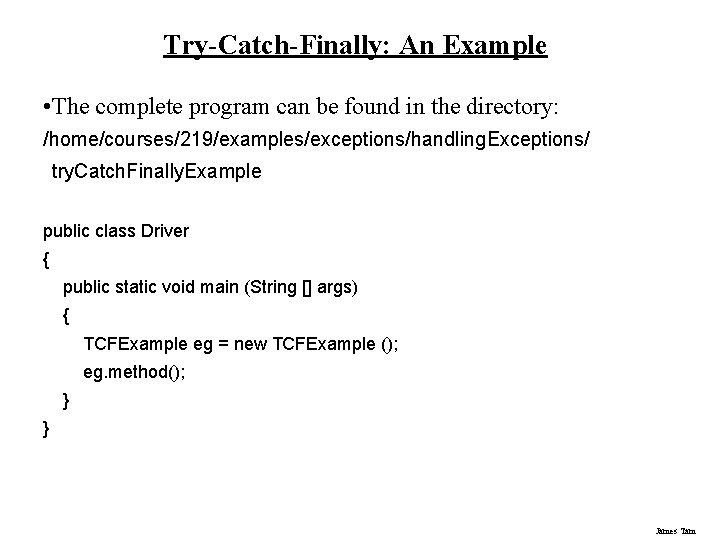
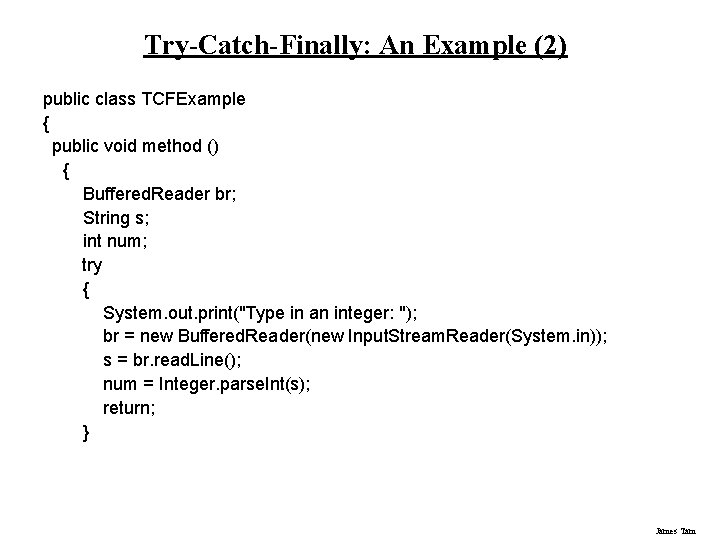
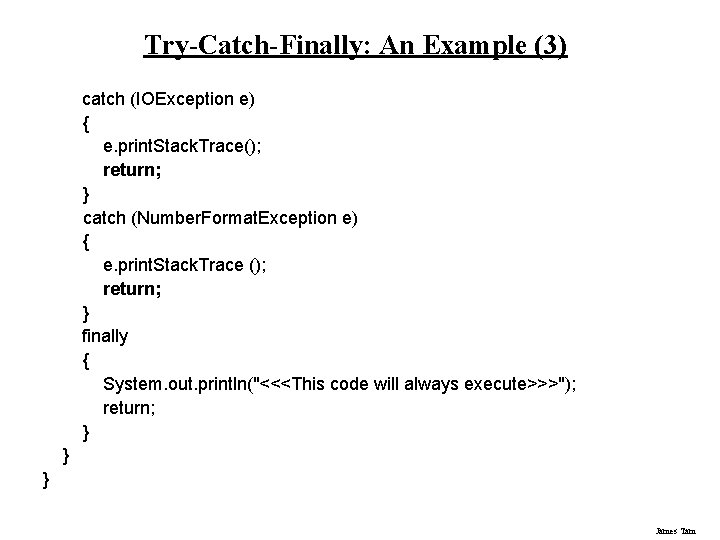
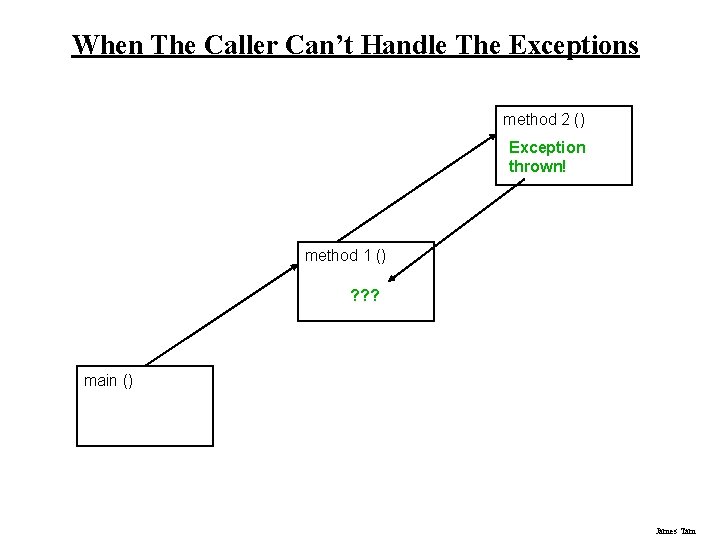
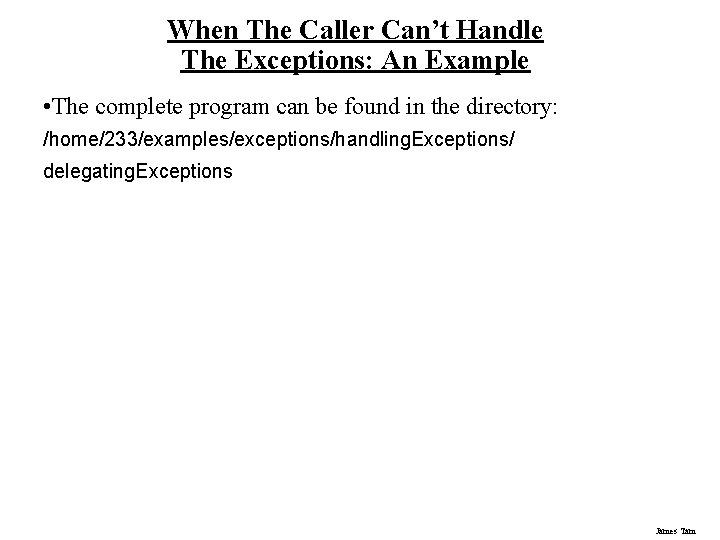
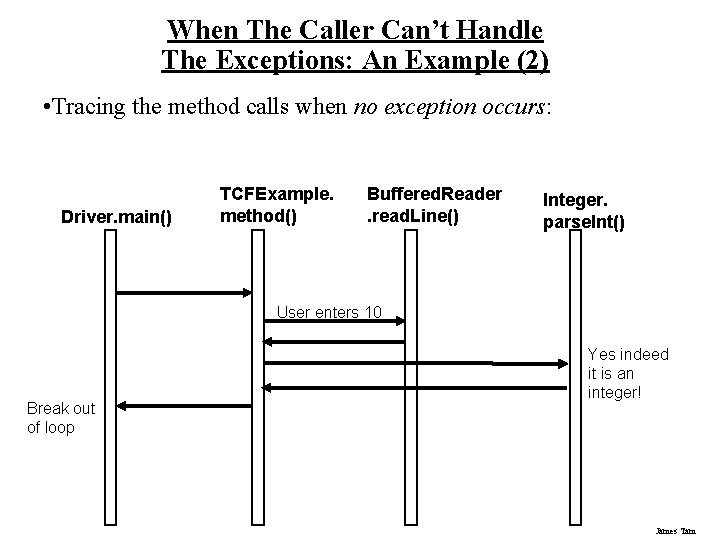
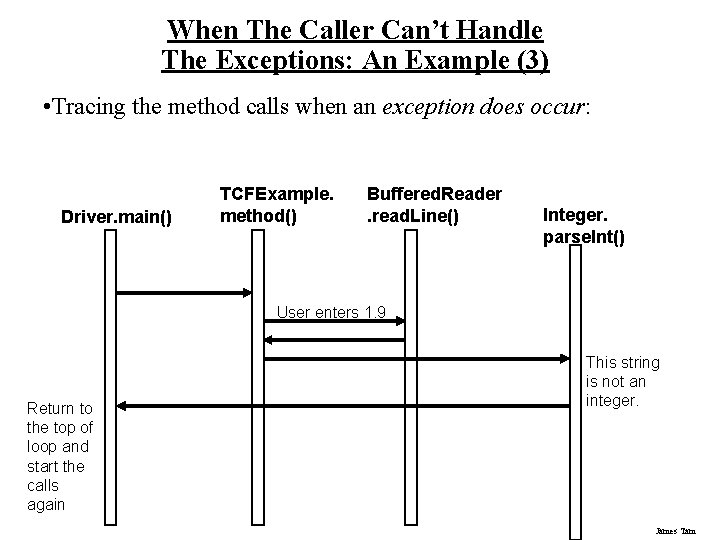
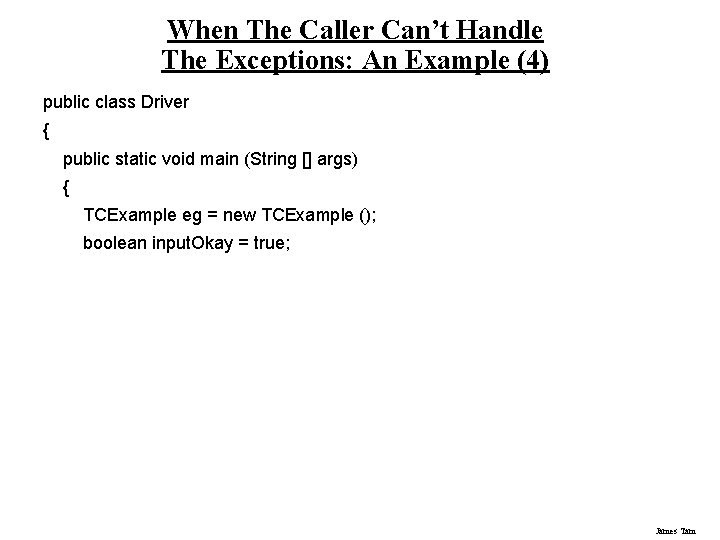
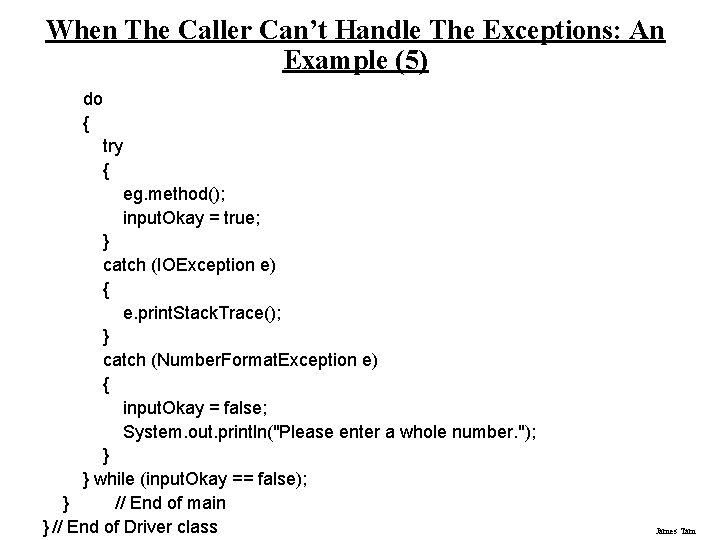
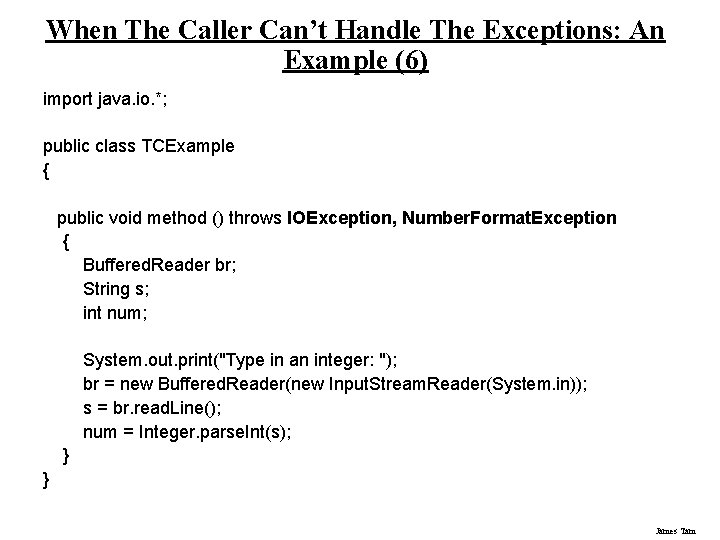
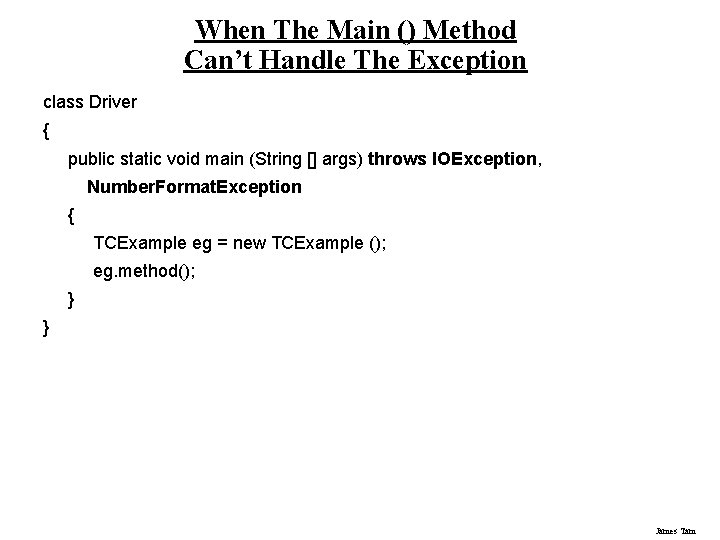
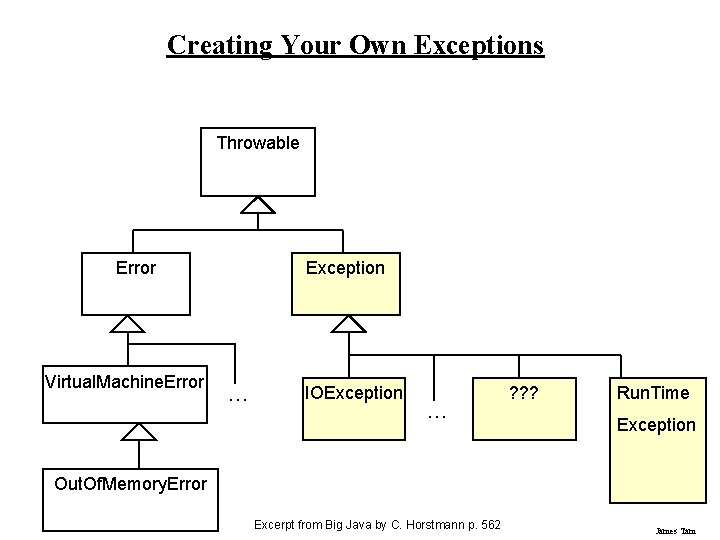
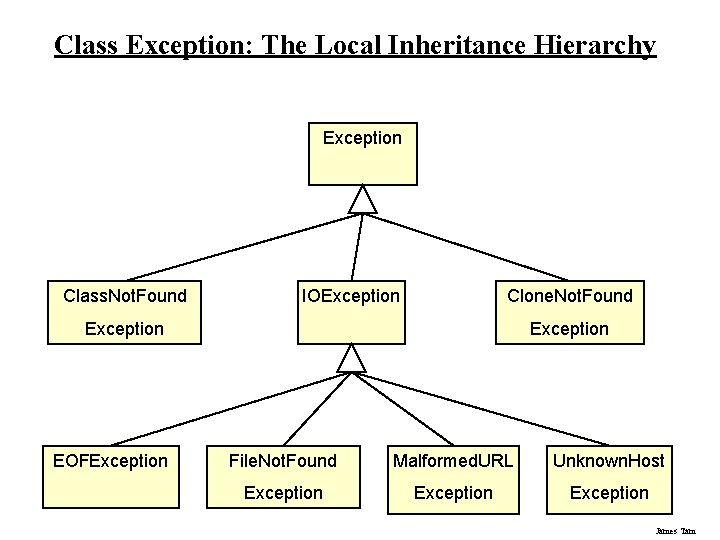
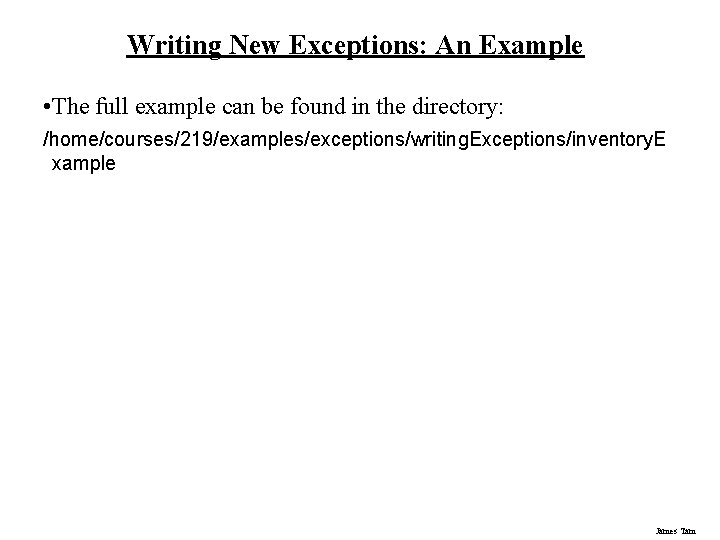
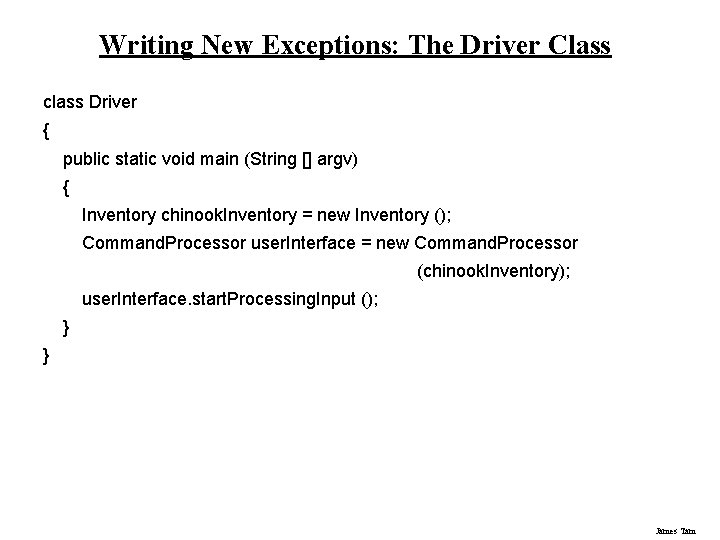
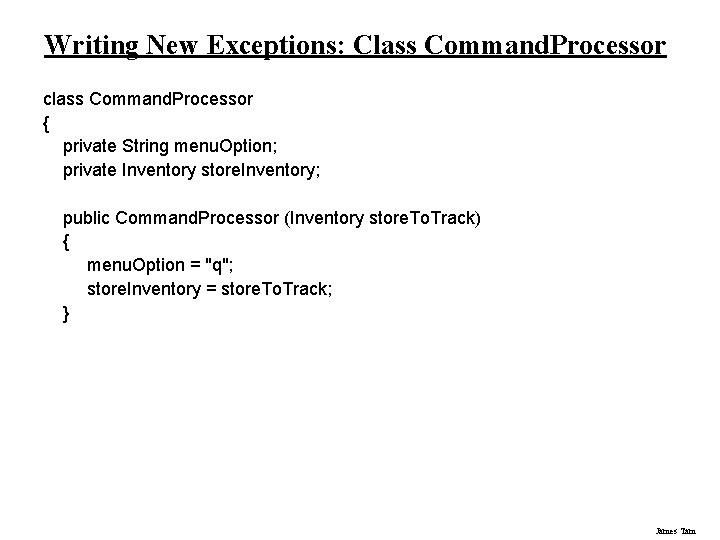
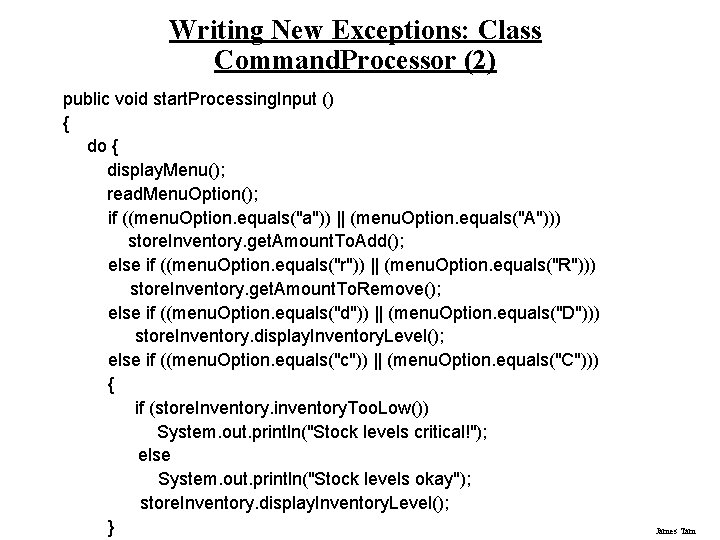
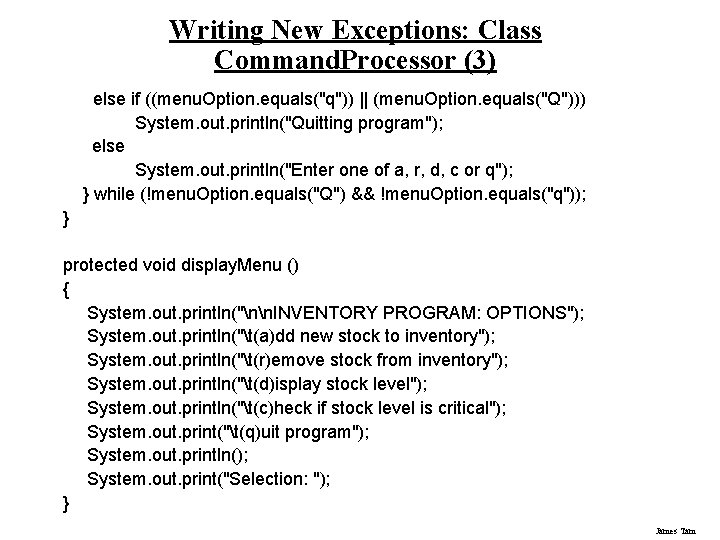
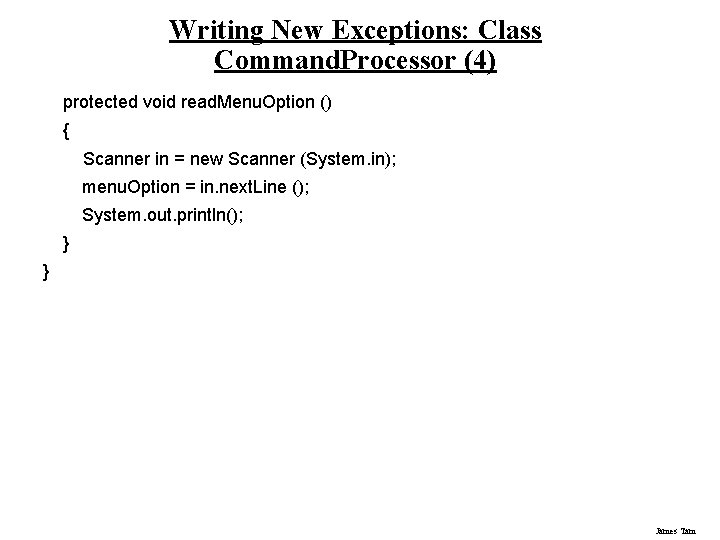
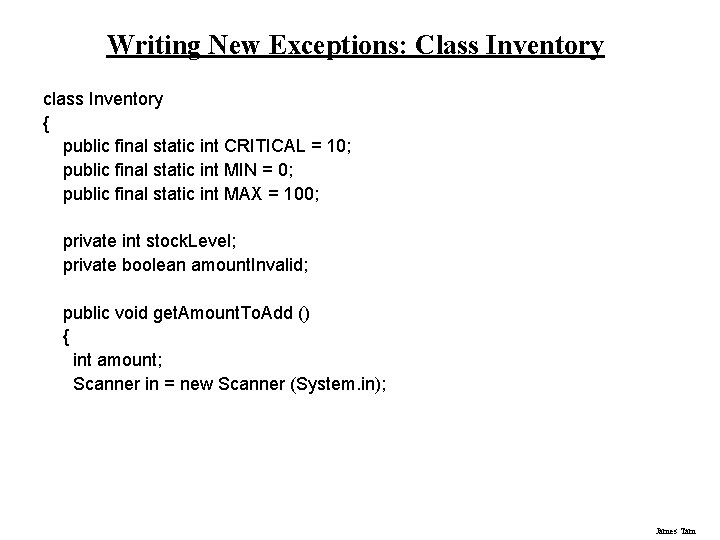
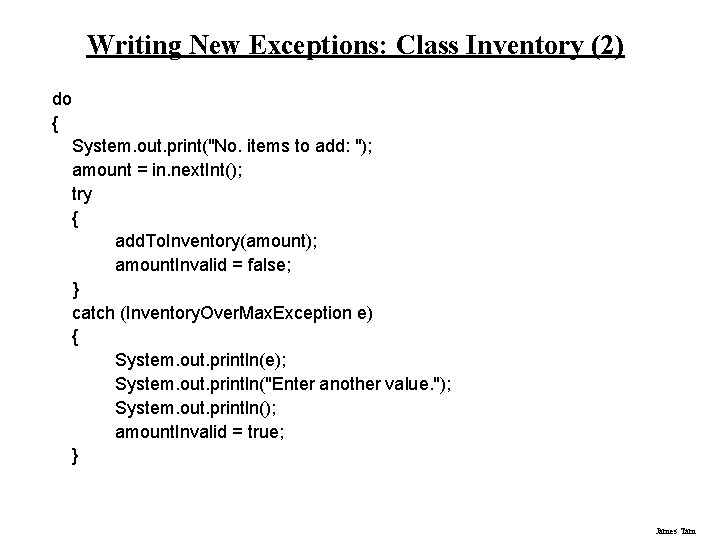
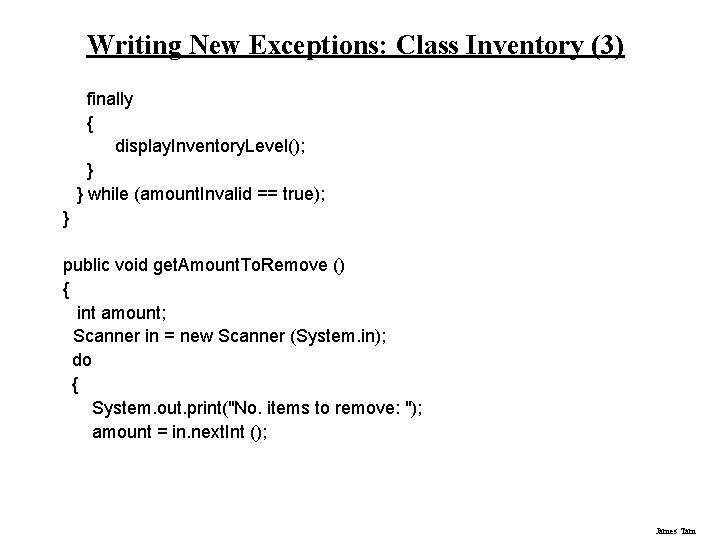
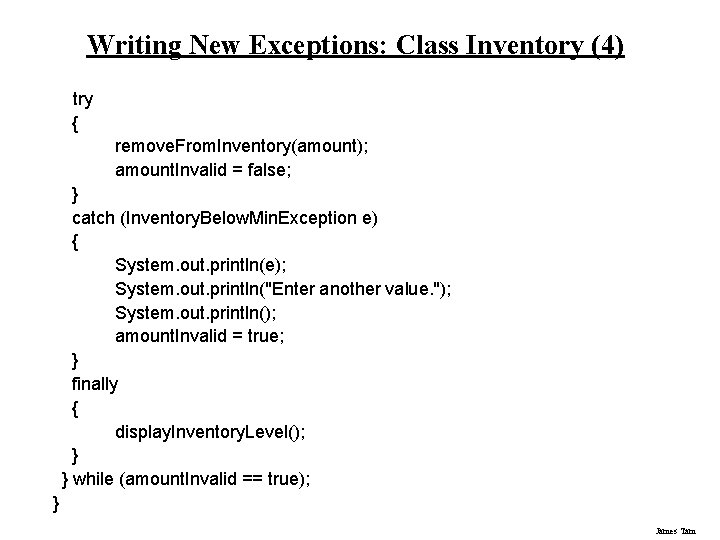
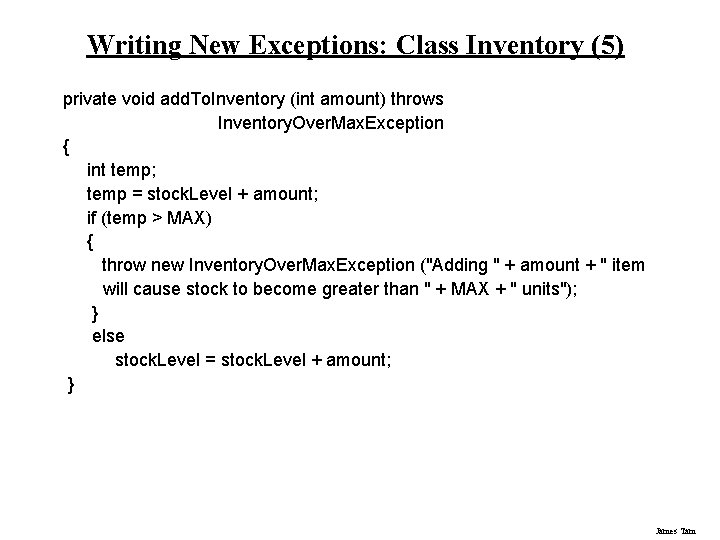
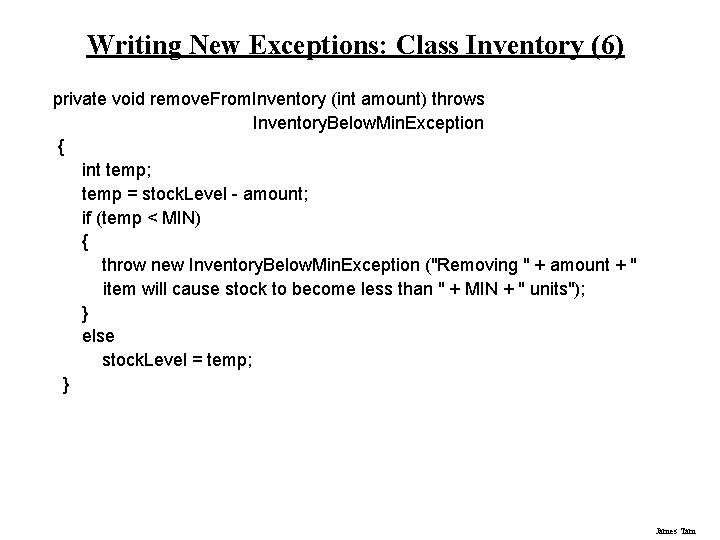
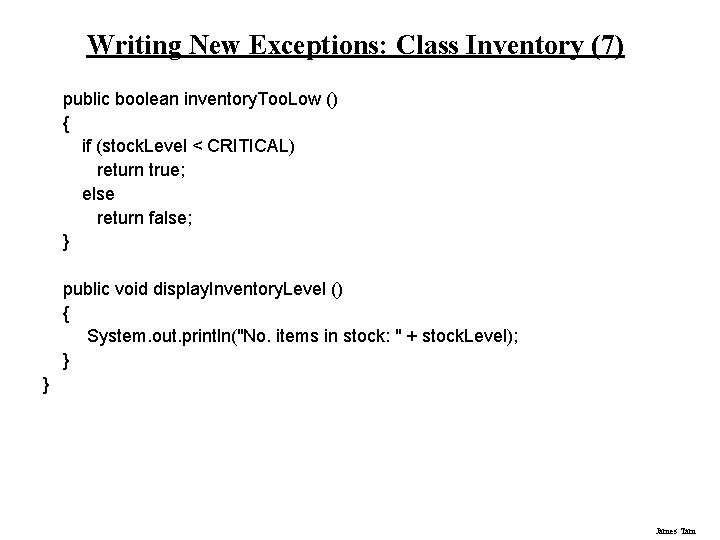
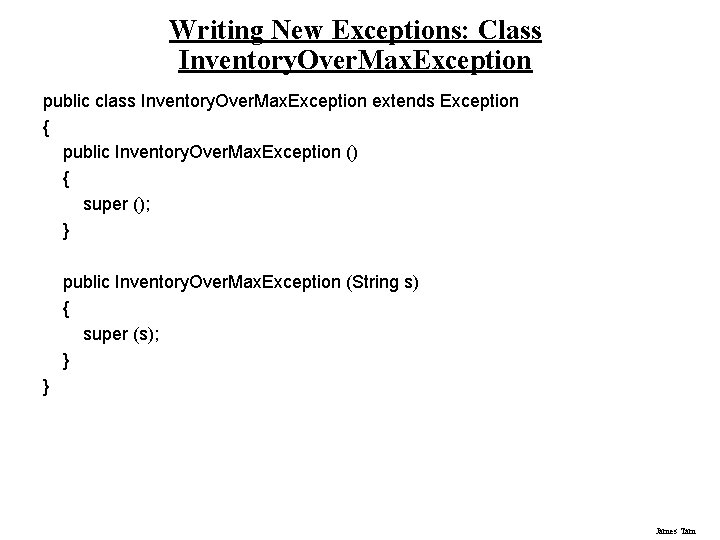
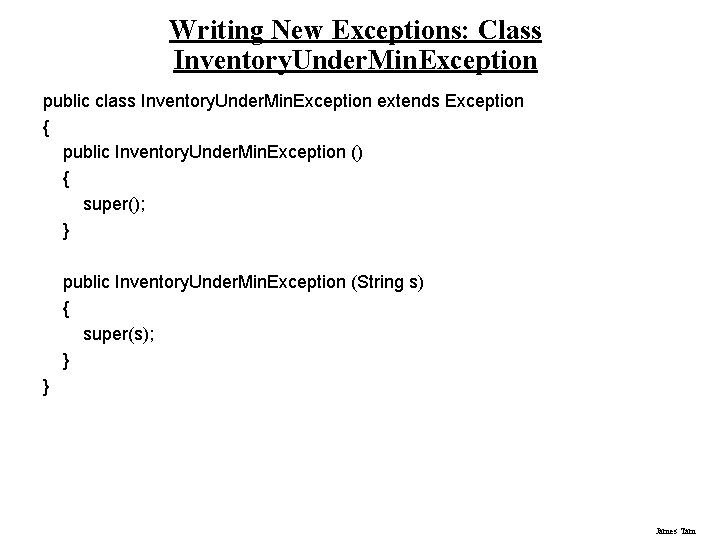
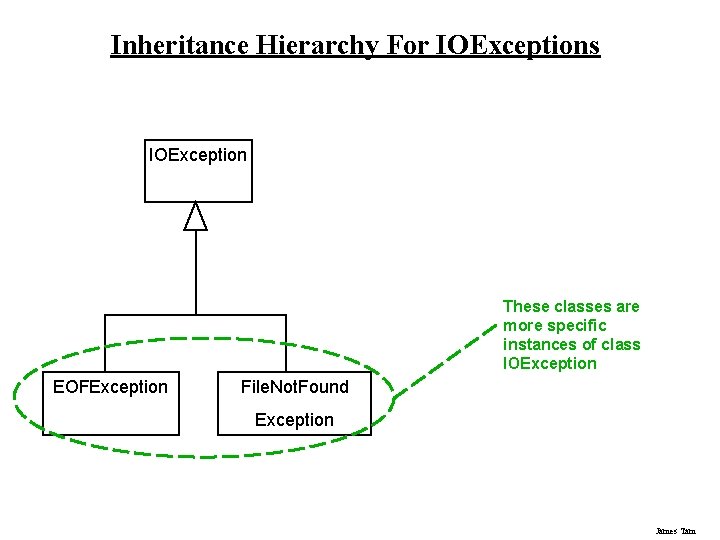
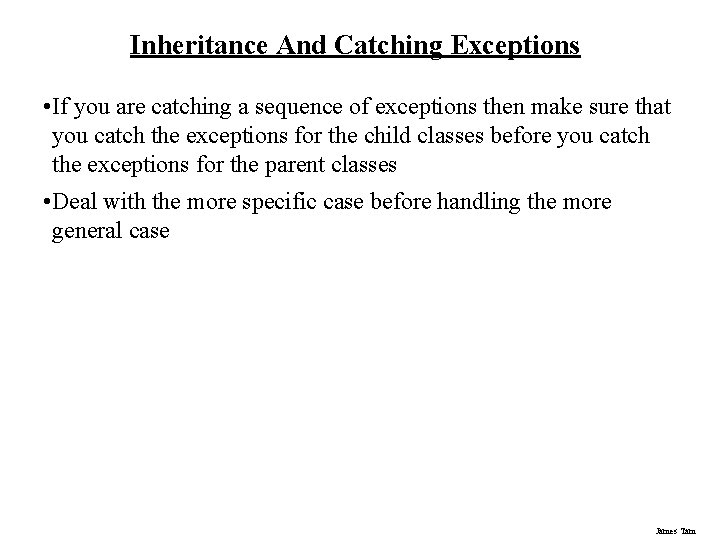
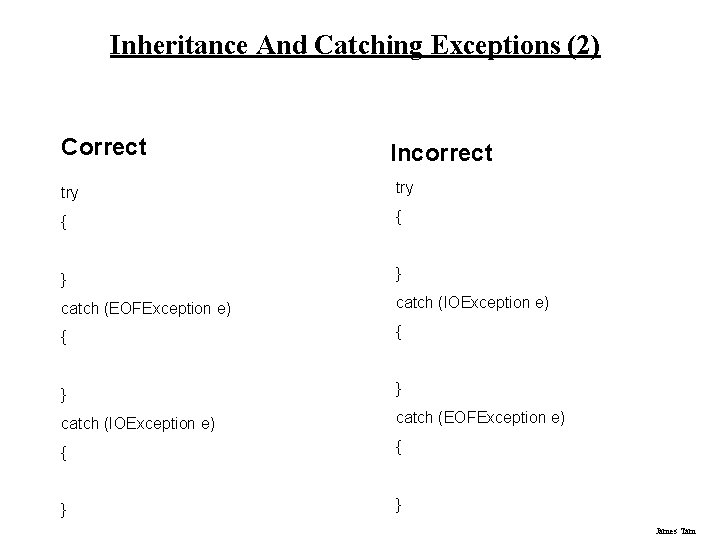
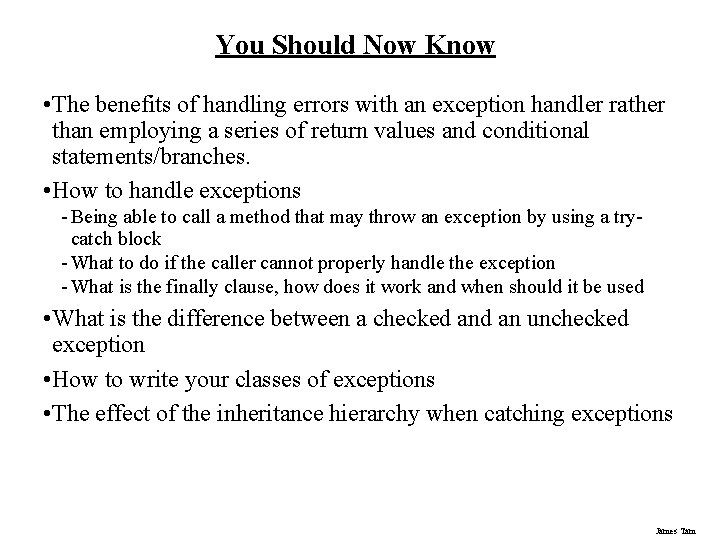
- Slides: 71
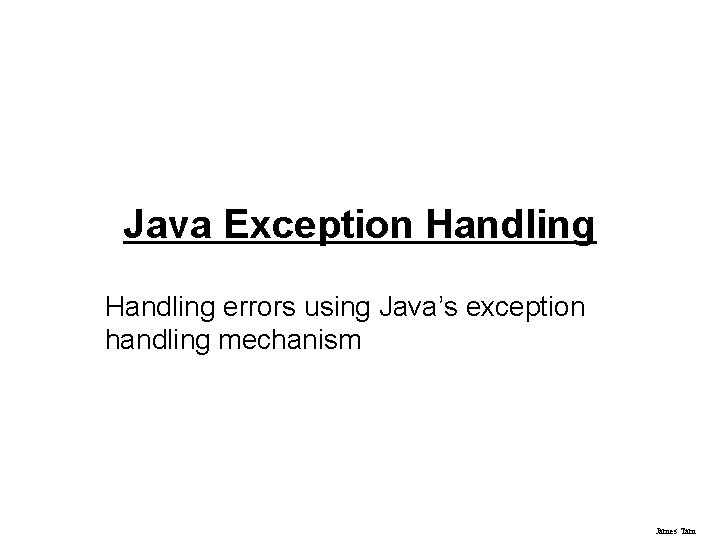
Java Exception Handling errors using Java’s exception handling mechanism James Tam
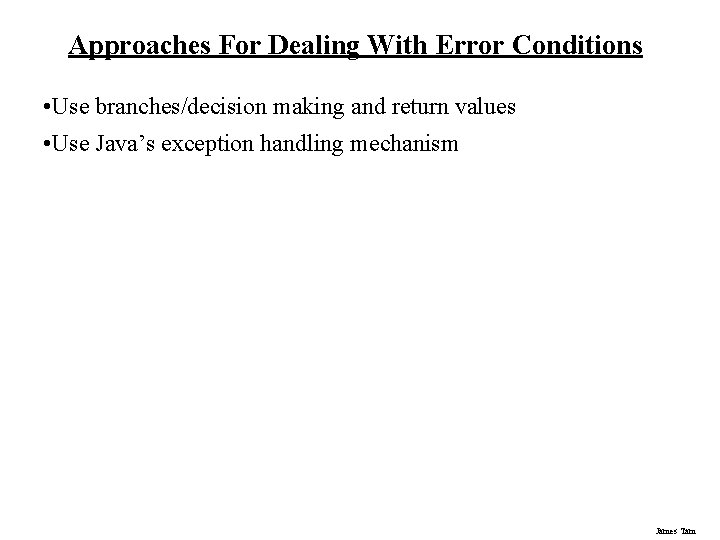
Approaches For Dealing With Error Conditions • Use branches/decision making and return values • Use Java’s exception handling mechanism James Tam
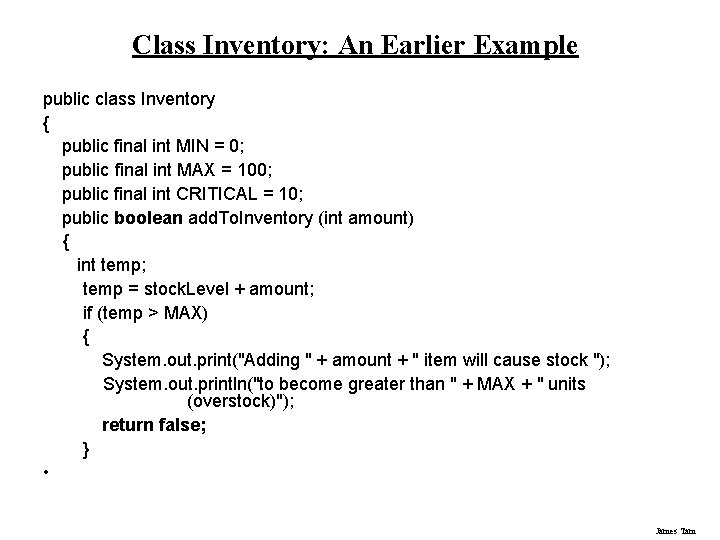
Class Inventory: An Earlier Example public class Inventory { public final int MIN = 0; public final int MAX = 100; public final int CRITICAL = 10; public boolean add. To. Inventory (int amount) { int temp; temp = stock. Level + amount; if (temp > MAX) { System. out. print("Adding " + amount + " item will cause stock "); System. out. println("to become greater than " + MAX + " units (overstock)"); return false; } • James Tam
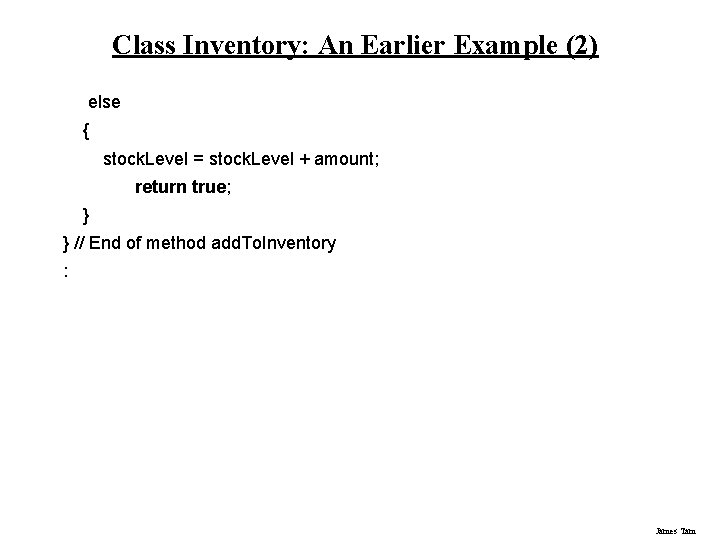
Class Inventory: An Earlier Example (2) else { stock. Level = stock. Level + amount; return true; } } // End of method add. To. Inventory : James Tam
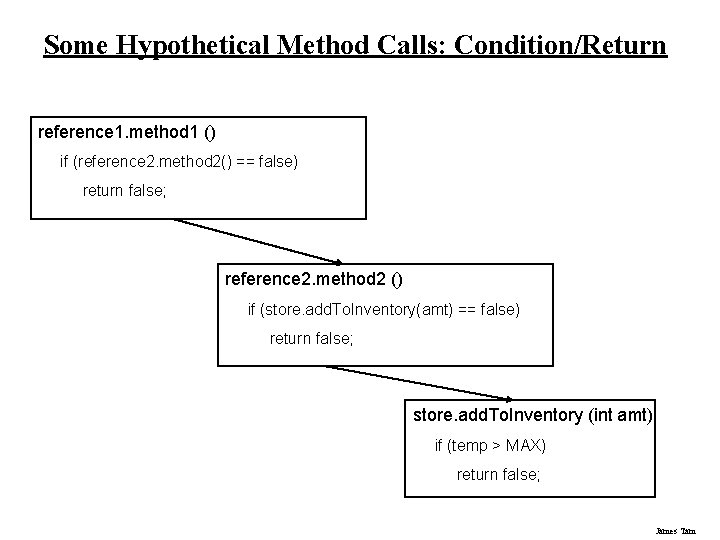
Some Hypothetical Method Calls: Condition/Return reference 1. method 1 () if (reference 2. method 2() == false) return false; reference 2. method 2 () if (store. add. To. Inventory(amt) == false) return false; store. add. To. Inventory (int amt) if (temp > MAX) return false; James Tam
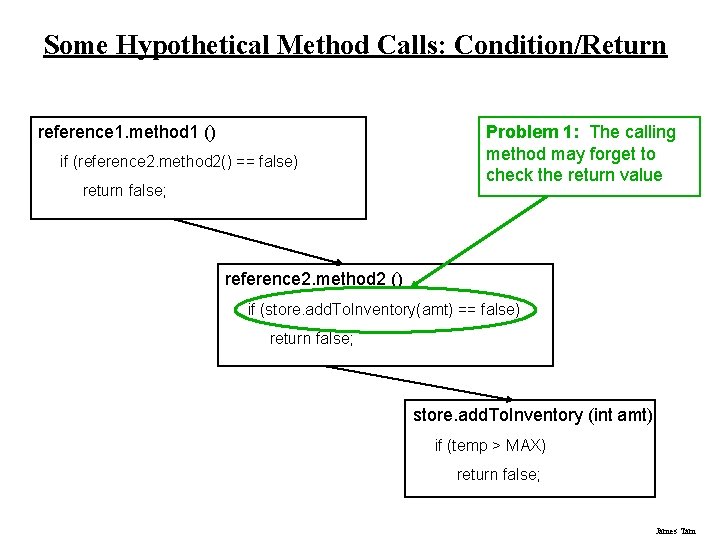
Some Hypothetical Method Calls: Condition/Return reference 1. method 1 () if (reference 2. method 2() == false) return false; Problem 1: The calling method may forget to check the return value reference 2. method 2 () if (store. add. To. Inventory(amt) == false) return false; store. add. To. Inventory (int amt) if (temp > MAX) return false; James Tam
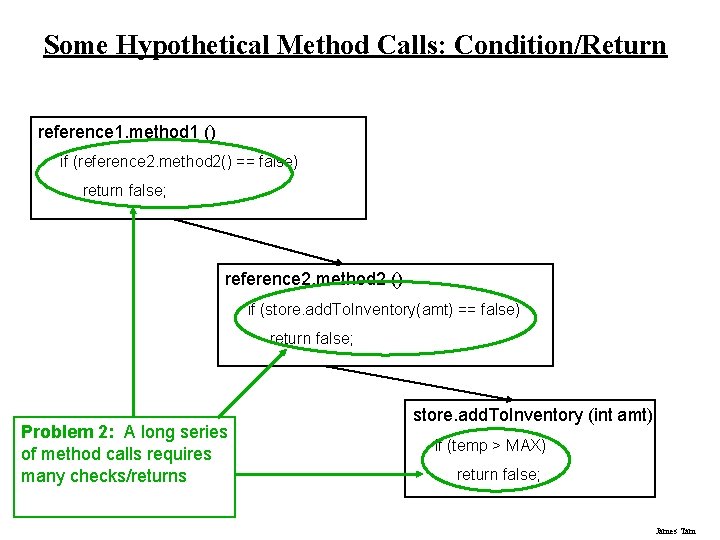
Some Hypothetical Method Calls: Condition/Return reference 1. method 1 () if (reference 2. method 2() == false) return false; reference 2. method 2 () if (store. add. To. Inventory(amt) == false) return false; Problem 2: A long series of method calls requires many checks/returns store. add. To. Inventory (int amt) if (temp > MAX) return false; James Tam
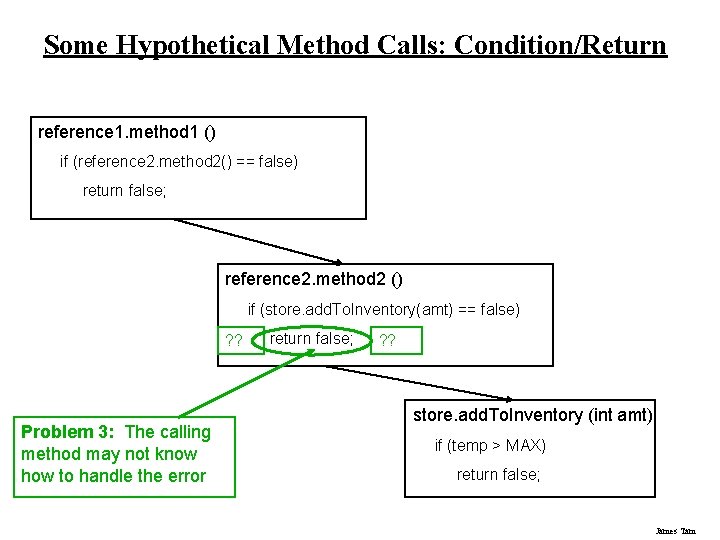
Some Hypothetical Method Calls: Condition/Return reference 1. method 1 () if (reference 2. method 2() == false) return false; reference 2. method 2 () if (store. add. To. Inventory(amt) == false) ? ? Problem 3: The calling method may not know how to handle the error return false; ? ? store. add. To. Inventory (int amt) if (temp > MAX) return false; James Tam
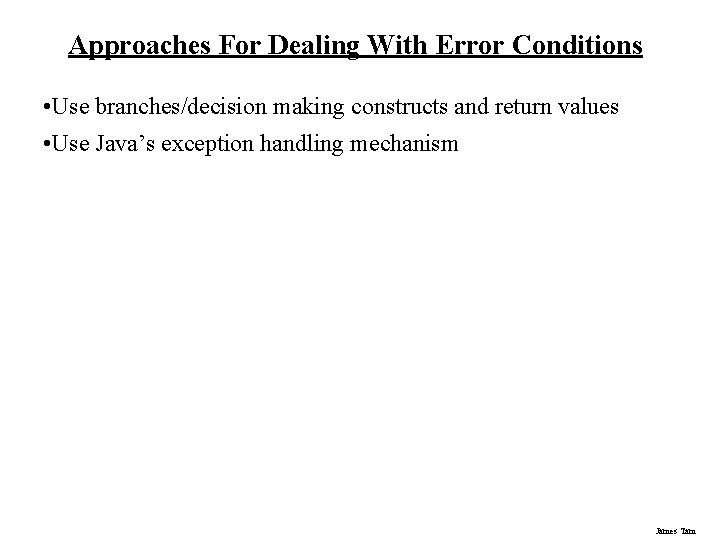
Approaches For Dealing With Error Conditions • Use branches/decision making constructs and return values • Use Java’s exception handling mechanism James Tam
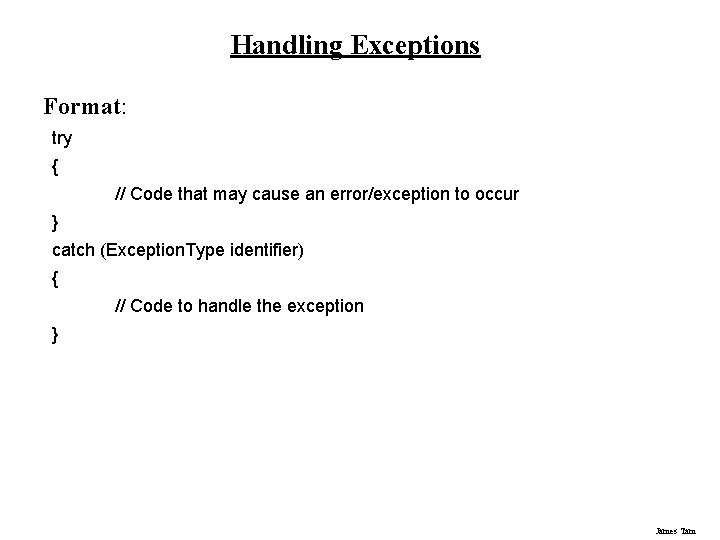
Handling Exceptions Format: try { // Code that may cause an error/exception to occur } catch (Exception. Type identifier) { // Code to handle the exception } James Tam
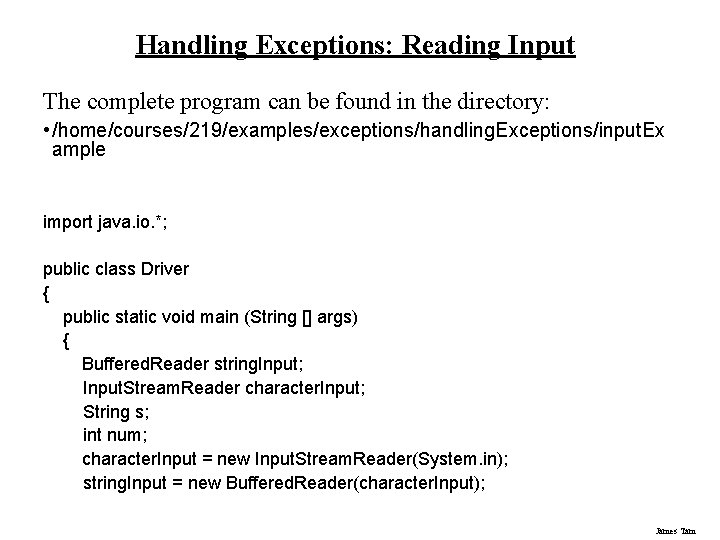
Handling Exceptions: Reading Input The complete program can be found in the directory: • /home/courses/219/examples/exceptions/handling. Exceptions/input. Ex ample import java. io. *; public class Driver { public static void main (String [] args) { Buffered. Reader string. Input; Input. Stream. Reader character. Input; String s; int num; character. Input = new Input. Stream. Reader(System. in); string. Input = new Buffered. Reader(character. Input); James Tam
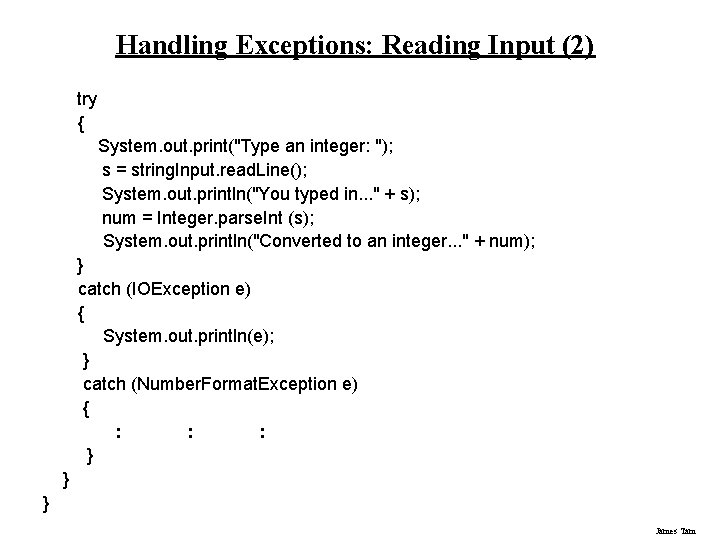
Handling Exceptions: Reading Input (2) try { System. out. print("Type an integer: "); s = string. Input. read. Line(); System. out. println("You typed in. . . " + s); num = Integer. parse. Int (s); System. out. println("Converted to an integer. . . " + num); } catch (IOException e) { System. out. println(e); } catch (Number. Format. Exception e) { : : : } } } James Tam
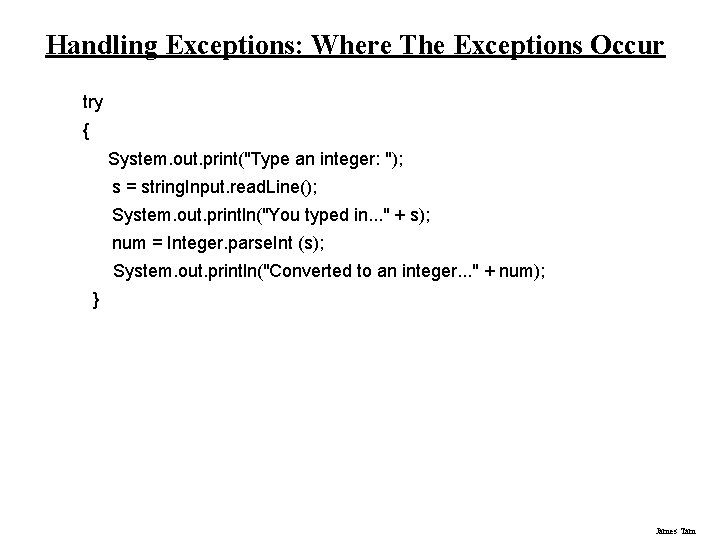
Handling Exceptions: Where The Exceptions Occur try { System. out. print("Type an integer: "); s = string. Input. read. Line(); System. out. println("You typed in. . . " + s); num = Integer. parse. Int (s); System. out. println("Converted to an integer. . . " + num); } James Tam
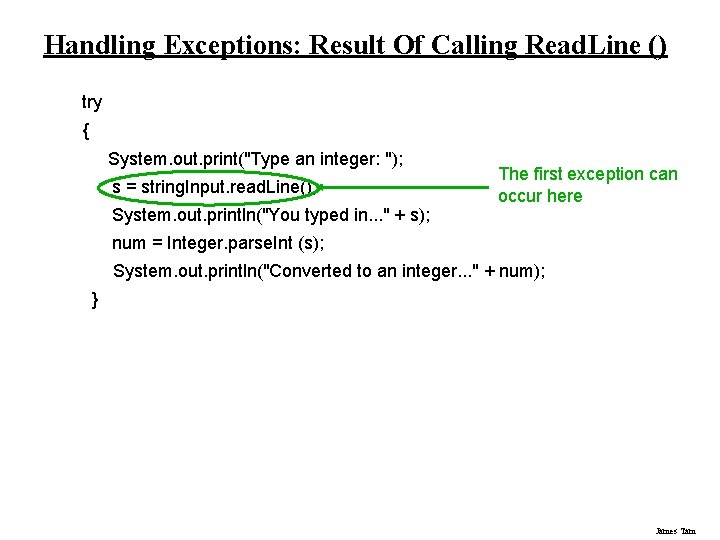
Handling Exceptions: Result Of Calling Read. Line () try { System. out. print("Type an integer: "); s = string. Input. read. Line(); System. out. println("You typed in. . . " + s); The first exception can occur here num = Integer. parse. Int (s); System. out. println("Converted to an integer. . . " + num); } James Tam
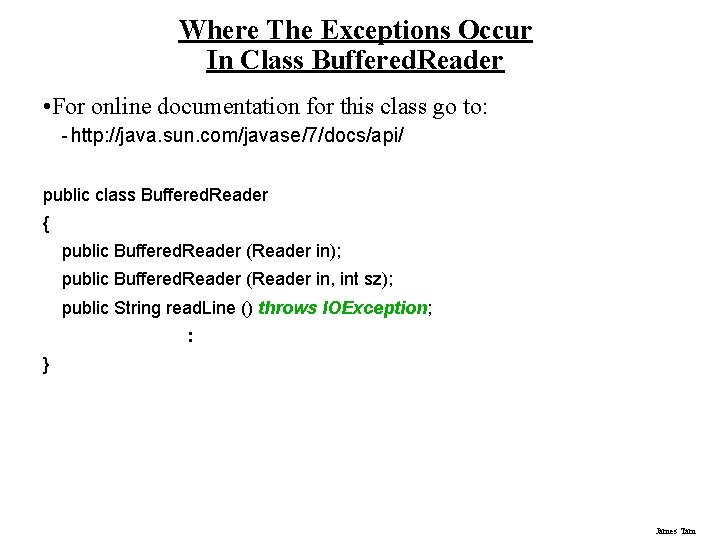
Where The Exceptions Occur In Class Buffered. Reader • For online documentation for this class go to: - http: //java. sun. com/javase/7/docs/api/ public class Buffered. Reader { public Buffered. Reader (Reader in); public Buffered. Reader (Reader in, int sz); public String read. Line () throws IOException; : } James Tam
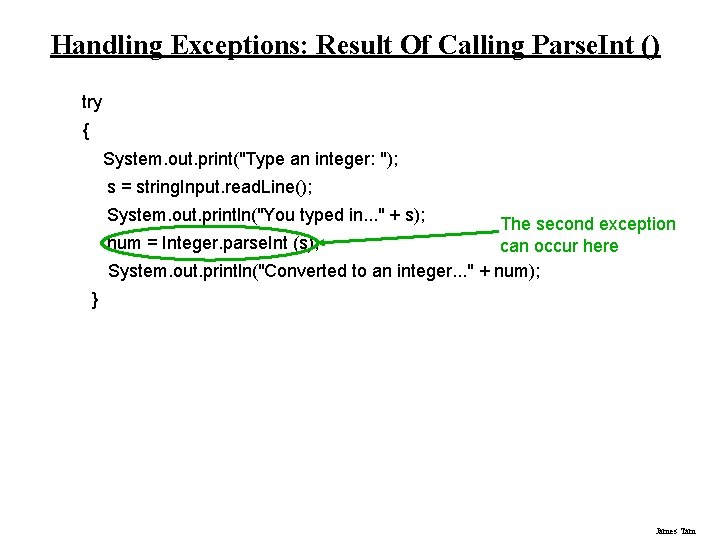
Handling Exceptions: Result Of Calling Parse. Int () try { System. out. print("Type an integer: "); s = string. Input. read. Line(); System. out. println("You typed in. . . " + s); The second exception num = Integer. parse. Int (s); can occur here System. out. println("Converted to an integer. . . " + num); } James Tam
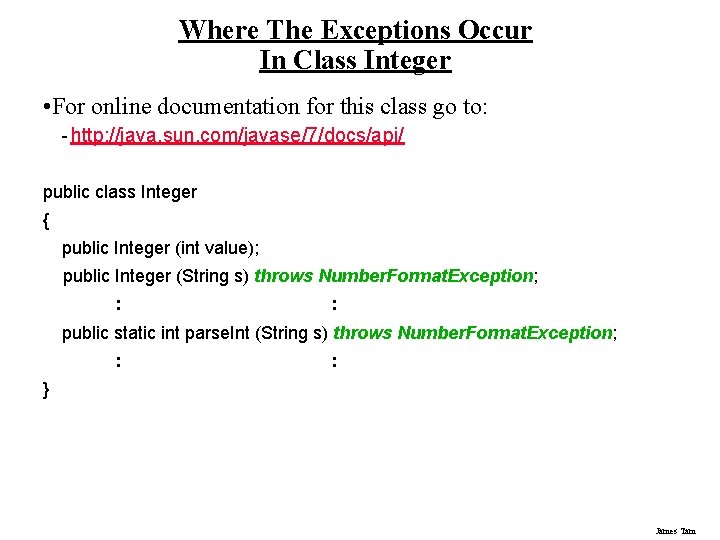
Where The Exceptions Occur In Class Integer • For online documentation for this class go to: - http: //java. sun. com/javase/7/docs/api/ public class Integer { public Integer (int value); public Integer (String s) throws Number. Format. Exception; : : public static int parse. Int (String s) throws Number. Format. Exception; : : } James Tam
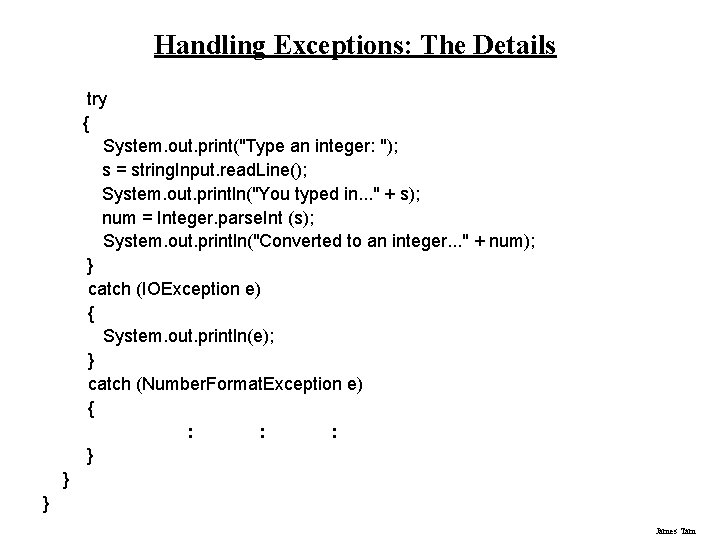
Handling Exceptions: The Details try { System. out. print("Type an integer: "); s = string. Input. read. Line(); System. out. println("You typed in. . . " + s); num = Integer. parse. Int (s); System. out. println("Converted to an integer. . . " + num); } catch (IOException e) { System. out. println(e); } catch (Number. Format. Exception e) { : : : } } } James Tam
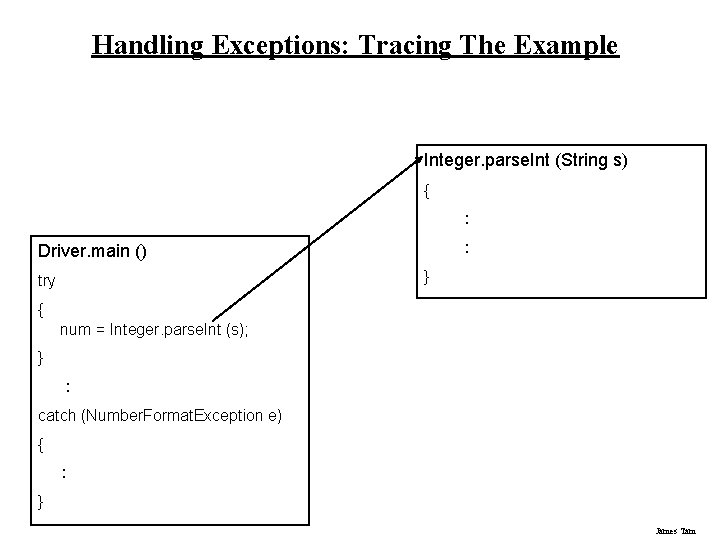
Handling Exceptions: Tracing The Example Integer. parse. Int (String s) { : : Driver. main () } try { num = Integer. parse. Int (s); } : catch (Number. Format. Exception e) { : } James Tam
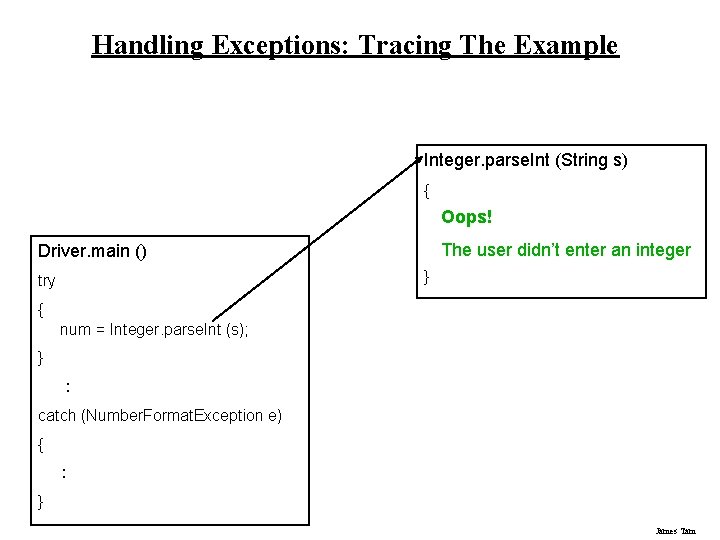
Handling Exceptions: Tracing The Example Integer. parse. Int (String s) { Oops! The user didn’t enter an integer Driver. main () } try { num = Integer. parse. Int (s); } : catch (Number. Format. Exception e) { : } James Tam
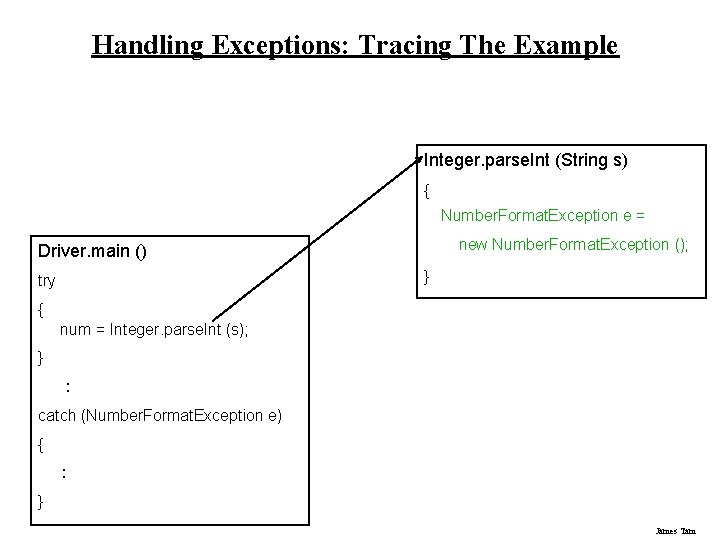
Handling Exceptions: Tracing The Example Integer. parse. Int (String s) { Number. Format. Exception e = new Number. Format. Exception (); Driver. main () } try { num = Integer. parse. Int (s); } : catch (Number. Format. Exception e) { : } James Tam
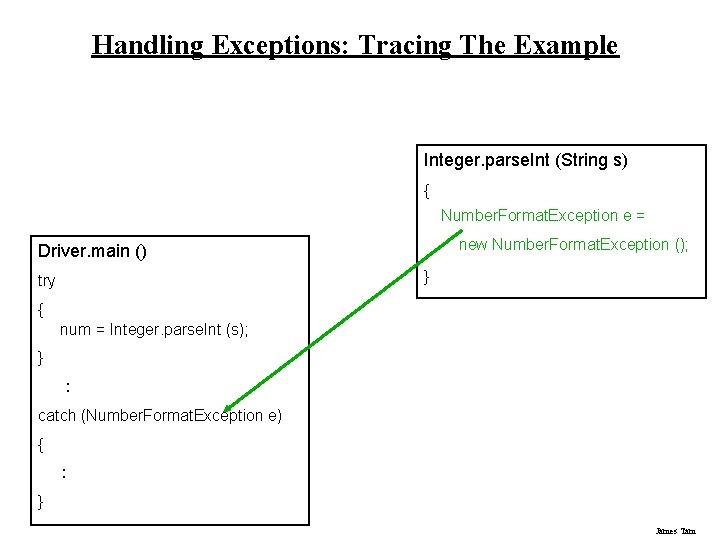
Handling Exceptions: Tracing The Example Integer. parse. Int (String s) { Number. Format. Exception e = new Number. Format. Exception (); Driver. main () } try { num = Integer. parse. Int (s); } : catch (Number. Format. Exception e) { : } James Tam
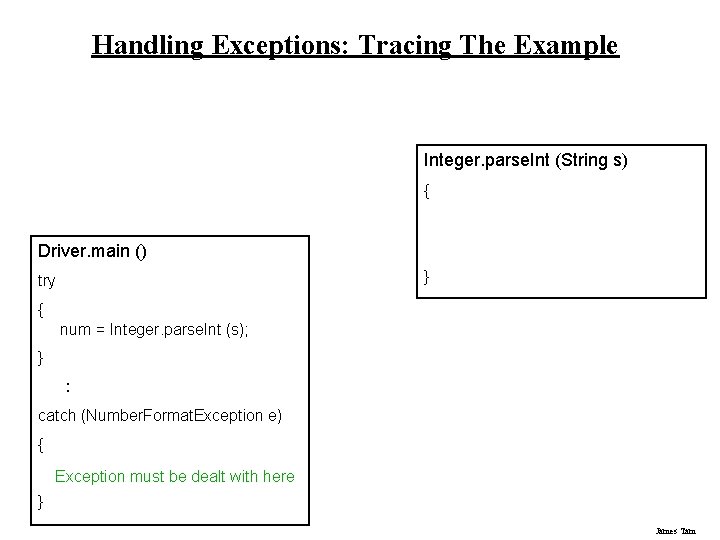
Handling Exceptions: Tracing The Example Integer. parse. Int (String s) { Driver. main () } try { num = Integer. parse. Int (s); } : catch (Number. Format. Exception e) { Exception must be dealt with here } James Tam
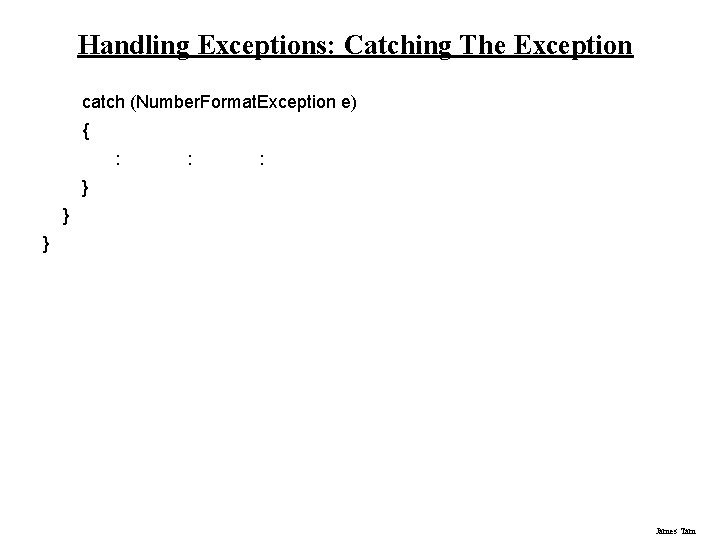
Handling Exceptions: Catching The Exception catch (Number. Format. Exception e) { : : : } } } James Tam
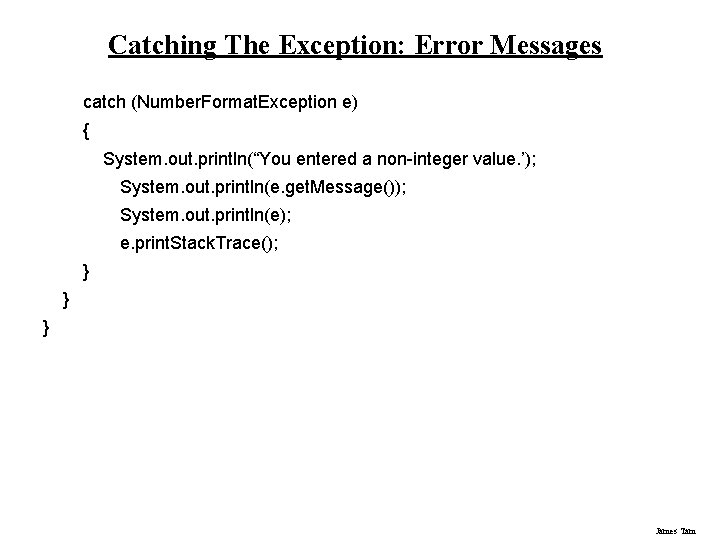
Catching The Exception: Error Messages catch (Number. Format. Exception e) { System. out. println(“You entered a non-integer value. ’); System. out. println(e. get. Message()); System. out. println(e); e. print. Stack. Trace(); } } } James Tam
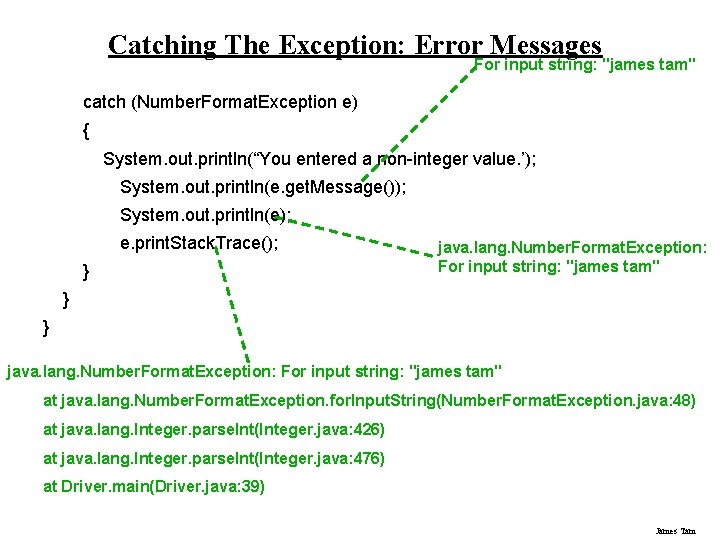
Catching The Exception: Error Messages For input string: "james tam" catch (Number. Format. Exception e) { System. out. println(“You entered a non-integer value. ’); System. out. println(e. get. Message()); System. out. println(e); e. print. Stack. Trace(); } java. lang. Number. Format. Exception: For input string: "james tam" } } java. lang. Number. Format. Exception: For input string: "james tam" at java. lang. Number. Format. Exception. for. Input. String(Number. Format. Exception. java: 48) at java. lang. Integer. parse. Int(Integer. java: 426) at java. lang. Integer. parse. Int(Integer. java: 476) at Driver. main(Driver. java: 39) James Tam
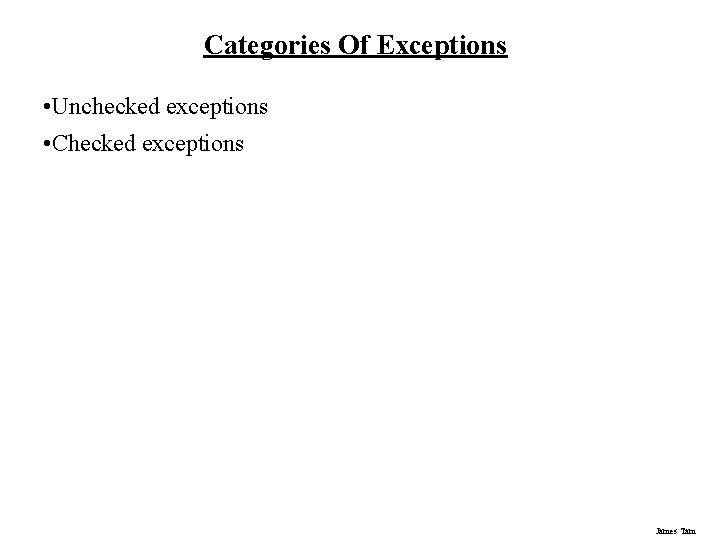
Categories Of Exceptions • Unchecked exceptions • Checked exceptions James Tam
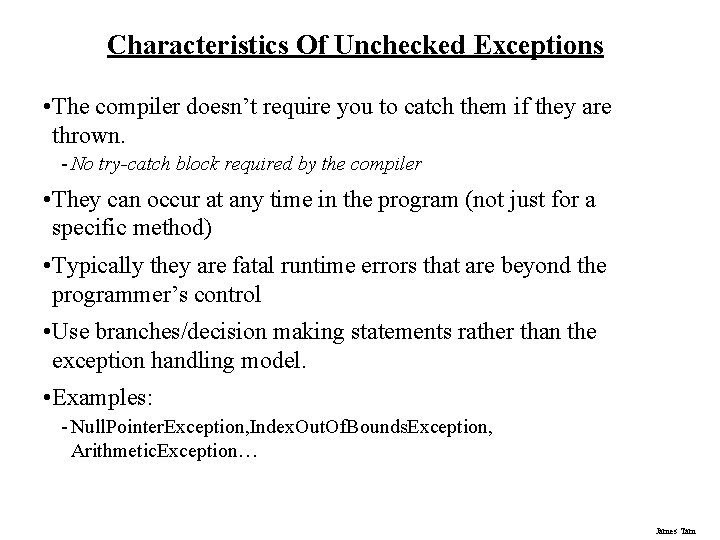
Characteristics Of Unchecked Exceptions • The compiler doesn’t require you to catch them if they are thrown. - No try-catch block required by the compiler • They can occur at any time in the program (not just for a specific method) • Typically they are fatal runtime errors that are beyond the programmer’s control • Use branches/decision making statements rather than the exception handling model. • Examples: - Null. Pointer. Exception, Index. Out. Of. Bounds. Exception, Arithmetic. Exception… James Tam
![Common Unchecked Exceptions Null Pointer Exception int arr null arr0 1 Common Unchecked Exceptions: Null. Pointer. Exception int [] arr = null; arr[0] = 1;](https://slidetodoc.com/presentation_image_h2/15f4f1d7fe6d7195bcb7ddb617836069/image-29.jpg)
Common Unchecked Exceptions: Null. Pointer. Exception int [] arr = null; arr[0] = 1; Null. Pointer. Exception arr = new int [4]; int i; for (i = 0; i <= 4; i++) arr[i] = i; arr[i-1] = arr[i-1] / 0; James Tam
![Common Unchecked Exceptions Array Index Out Of Bounds Exception int arr null Common Unchecked Exceptions: Array. Index. Out. Of. Bounds. Exception int [] arr = null;](https://slidetodoc.com/presentation_image_h2/15f4f1d7fe6d7195bcb7ddb617836069/image-30.jpg)
Common Unchecked Exceptions: Array. Index. Out. Of. Bounds. Exception int [] arr = null; arr[0] = 1; arr = new int [4]; int i; for (i = 0; i <= 4; i++) arr[i] = i; Array. Index. Out. Of. Bounds. Exception (when i = 4) arr[i-1] = arr[i-1] / 0; James Tam
![Common Unchecked Exceptions Arithmetic Exceptions int arr null arr0 1 arr Common Unchecked Exceptions: Arithmetic. Exceptions int [] arr = null; arr[0] = 1; arr](https://slidetodoc.com/presentation_image_h2/15f4f1d7fe6d7195bcb7ddb617836069/image-31.jpg)
Common Unchecked Exceptions: Arithmetic. Exceptions int [] arr = null; arr[0] = 1; arr = new int [4]; int i; for (i = 0; i <= 4; i++) arr[i] = i; arr[i-1] = arr[i-1] / 0; Arithmetic. Exception (Division by zero) James Tam
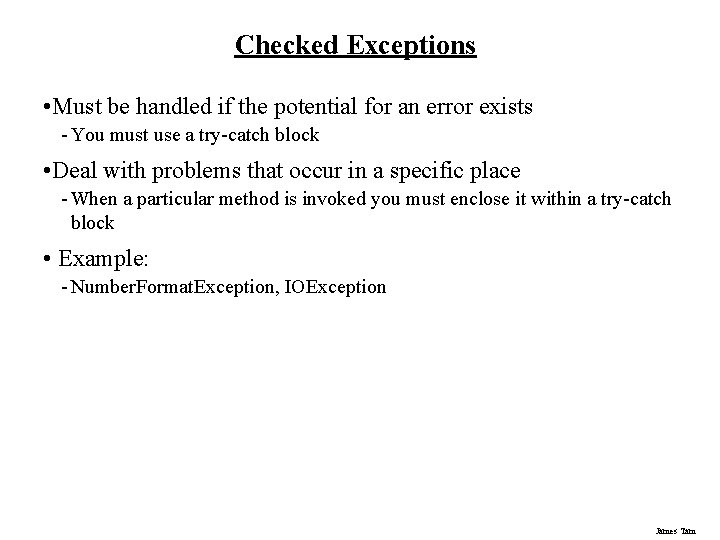
Checked Exceptions • Must be handled if the potential for an error exists - You must use a try-catch block • Deal with problems that occur in a specific place - When a particular method is invoked you must enclose it within a try-catch block • Example: - Number. Format. Exception, IOException James Tam
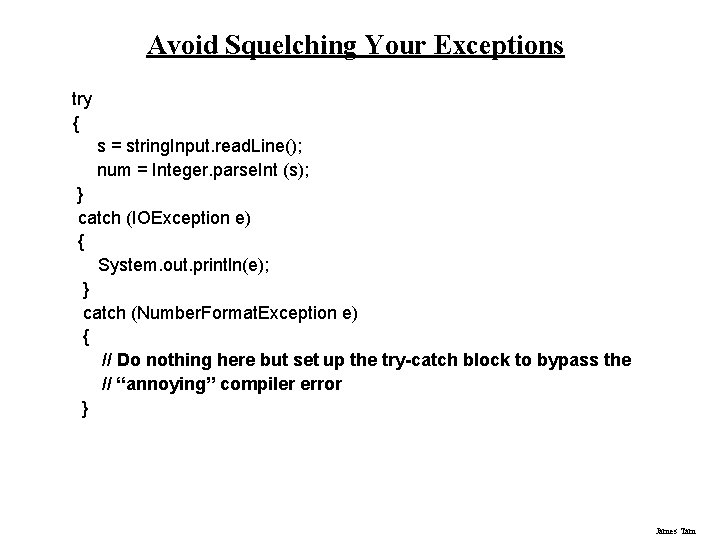
Avoid Squelching Your Exceptions try { s = string. Input. read. Line(); num = Integer. parse. Int (s); } catch (IOException e) { System. out. println(e); } catch (Number. Format. Exception e) { // Do nothing here but set up the try-catch block to bypass the // “annoying” compiler error } James Tam
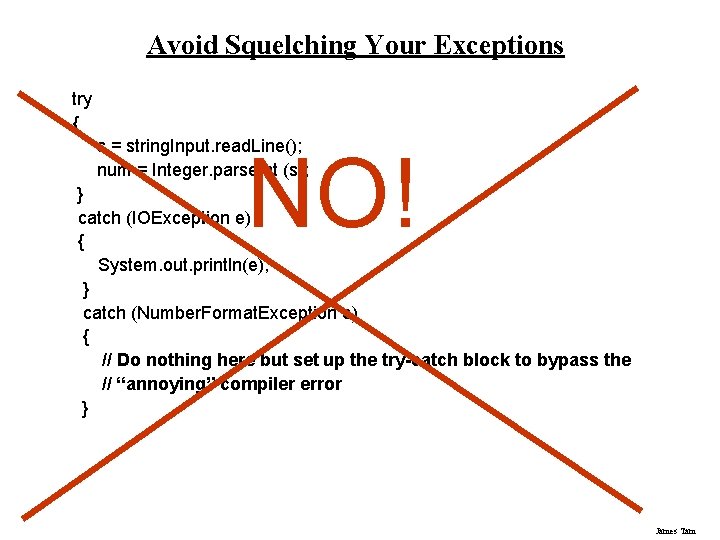
Avoid Squelching Your Exceptions try { s = string. Input. read. Line(); num = Integer. parse. Int (s); NO! } catch (IOException e) { System. out. println(e); } catch (Number. Format. Exception e) { // Do nothing here but set up the try-catch block to bypass the // “annoying” compiler error } James Tam
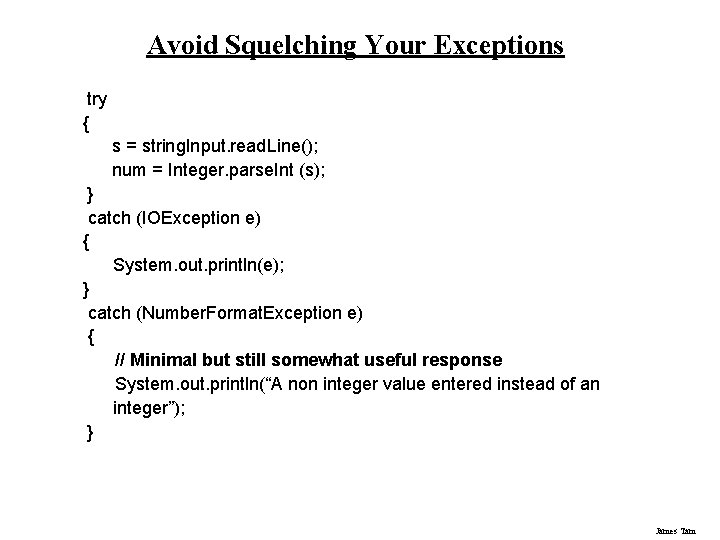
Avoid Squelching Your Exceptions try { s = string. Input. read. Line(); num = Integer. parse. Int (s); } catch (IOException e) { System. out. println(e); } catch (Number. Format. Exception e) { // Minimal but still somewhat useful response System. out. println(“A non integer value entered instead of an integer”); } James Tam
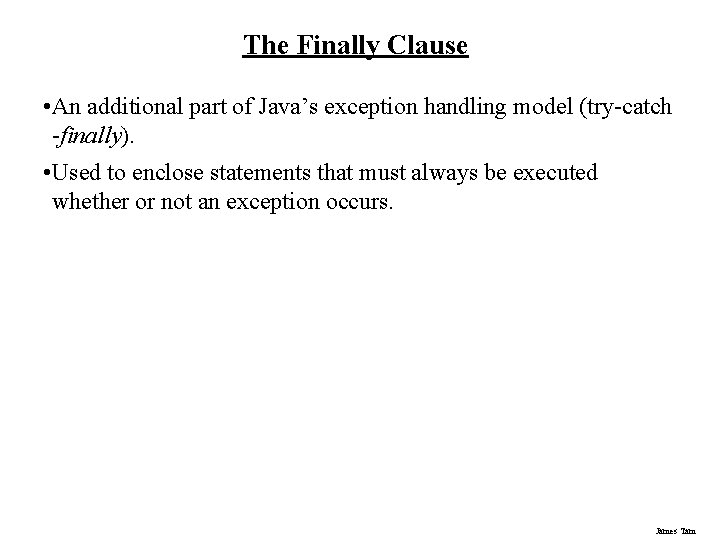
The Finally Clause • An additional part of Java’s exception handling model (try-catch -finally). • Used to enclose statements that must always be executed whether or not an exception occurs. James Tam
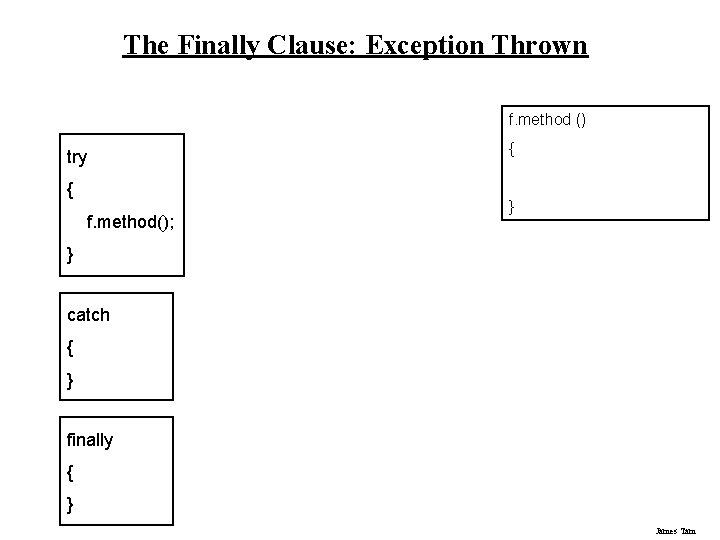
The Finally Clause: Exception Thrown f. method () try { f. method(); { } } catch { } finally { } James Tam
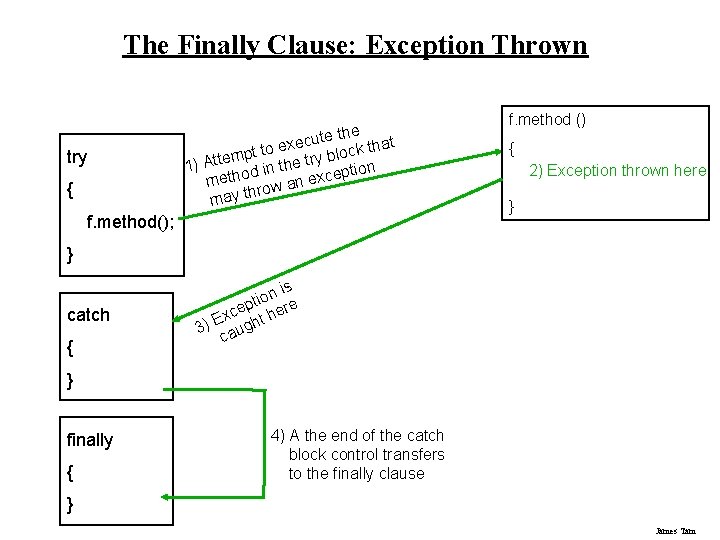
The Finally Clause: Exception Thrown try { te the at u c e x k th to e c t o p l b m y 1) Atte od in the tr eption meth ow an exc hr may t f. method(); f. method () { 2) Exception thrown here } } catch { n is o i t ep here c x t 3) E augh c } finally { 4) A the end of the catch block control transfers to the finally clause } James Tam
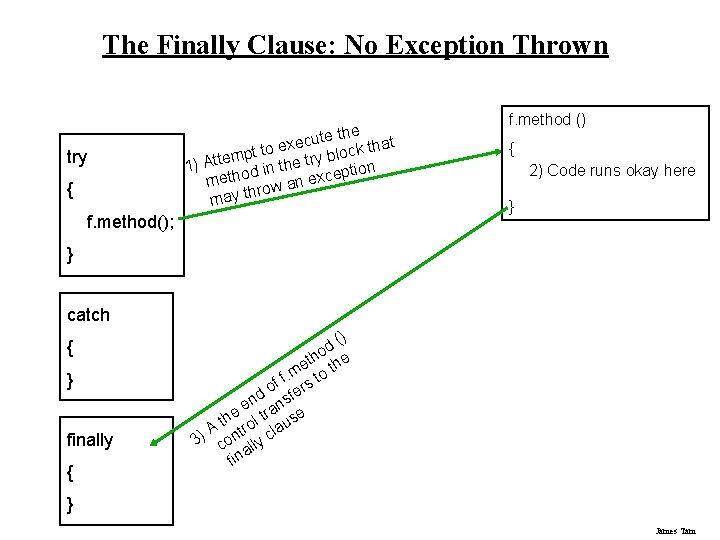
The Finally Clause: No Exception Thrown try { te the at u c e x k th to e c t o p l b m y 1) Atte od in the tr eption meth ow an exc hr may t f. method(); f. method () { 2) Code runs okay here } } catch { } finally { () d tho the e f. m s to f d o sfer n e ran e th rol t ause A t l 3) con lly c a fin } James Tam
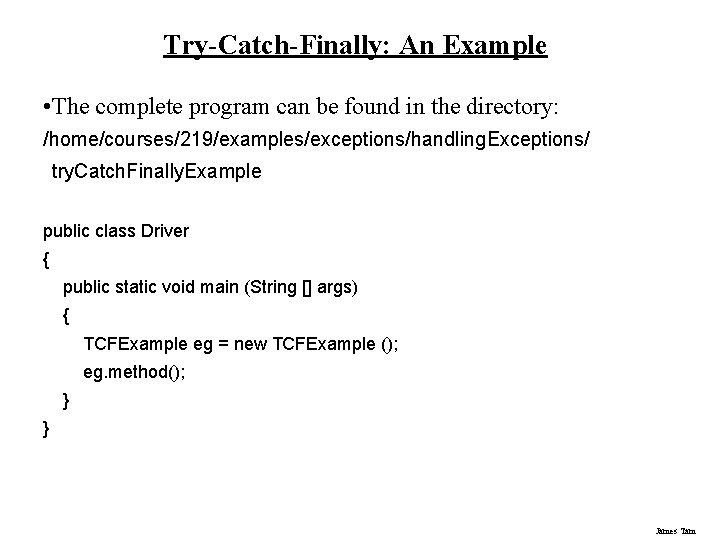
Try-Catch-Finally: An Example • The complete program can be found in the directory: /home/courses/219/examples/exceptions/handling. Exceptions/ try. Catch. Finally. Example public class Driver { public static void main (String [] args) { TCFExample eg = new TCFExample (); eg. method(); } } James Tam
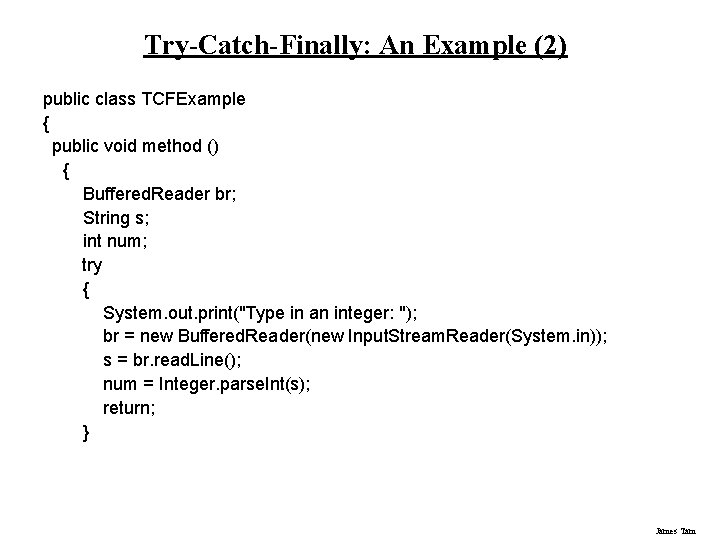
Try-Catch-Finally: An Example (2) public class TCFExample { public void method () { Buffered. Reader br; String s; int num; try { System. out. print("Type in an integer: "); br = new Buffered. Reader(new Input. Stream. Reader(System. in)); s = br. read. Line(); num = Integer. parse. Int(s); return; } James Tam
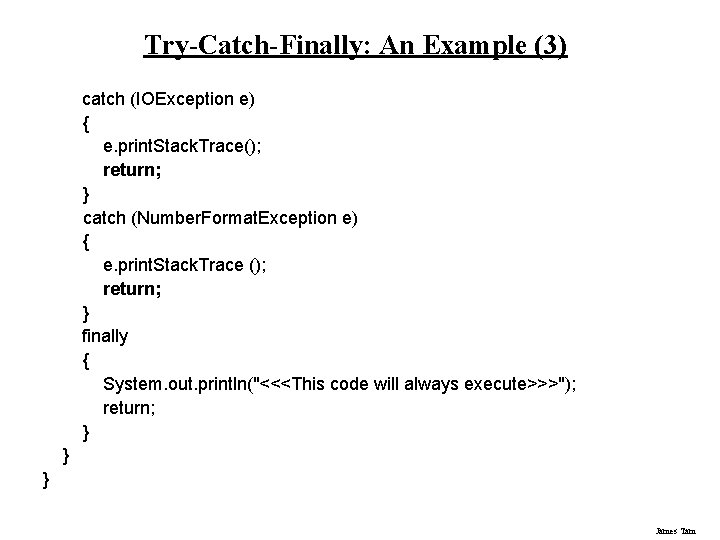
Try-Catch-Finally: An Example (3) catch (IOException e) { e. print. Stack. Trace(); return; } catch (Number. Format. Exception e) { e. print. Stack. Trace (); return; } finally { System. out. println("<<<This code will always execute>>>"); return; } } } James Tam
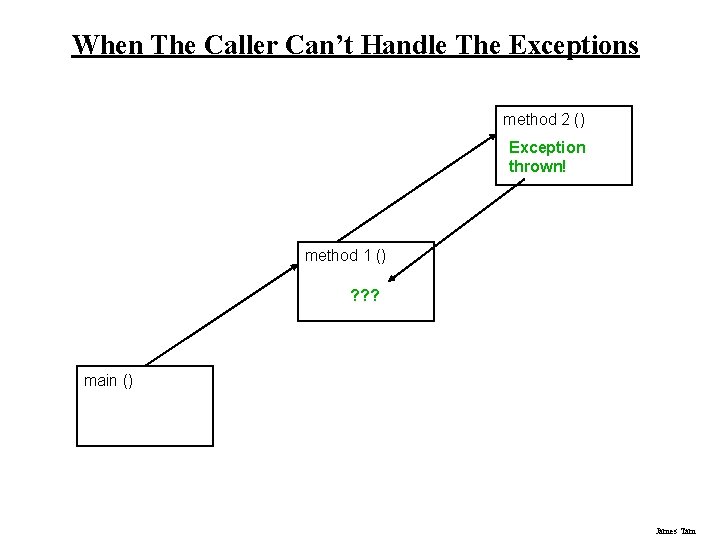
When The Caller Can’t Handle The Exceptions method 2 () Exception thrown! method 1 () ? ? ? main () James Tam
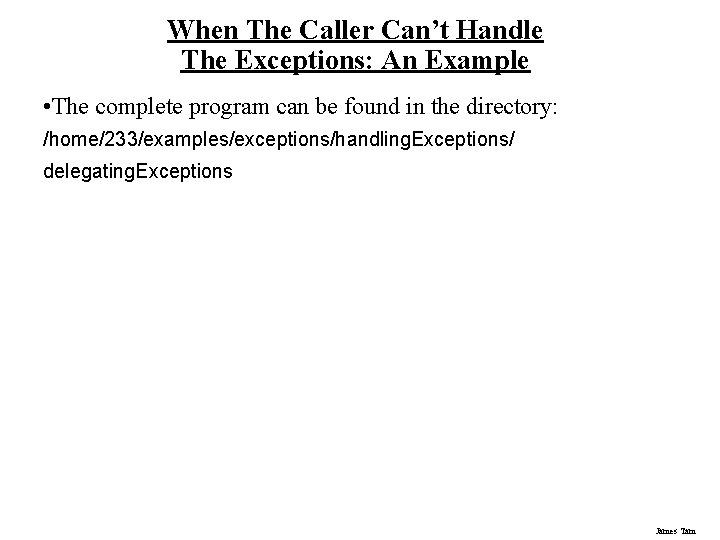
When The Caller Can’t Handle The Exceptions: An Example • The complete program can be found in the directory: /home/233/examples/exceptions/handling. Exceptions/ delegating. Exceptions James Tam
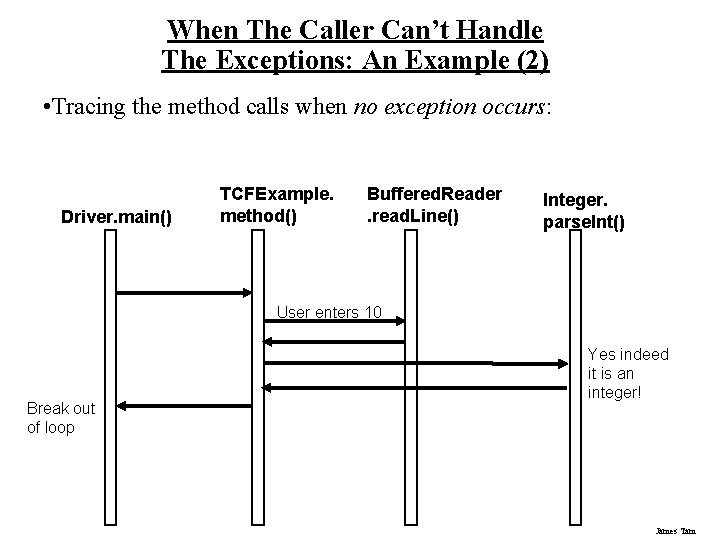
When The Caller Can’t Handle The Exceptions: An Example (2) • Tracing the method calls when no exception occurs: Driver. main() TCFExample. method() Buffered. Reader. read. Line() Integer. parse. Int() User enters 10 Break out of loop Yes indeed it is an integer! James Tam
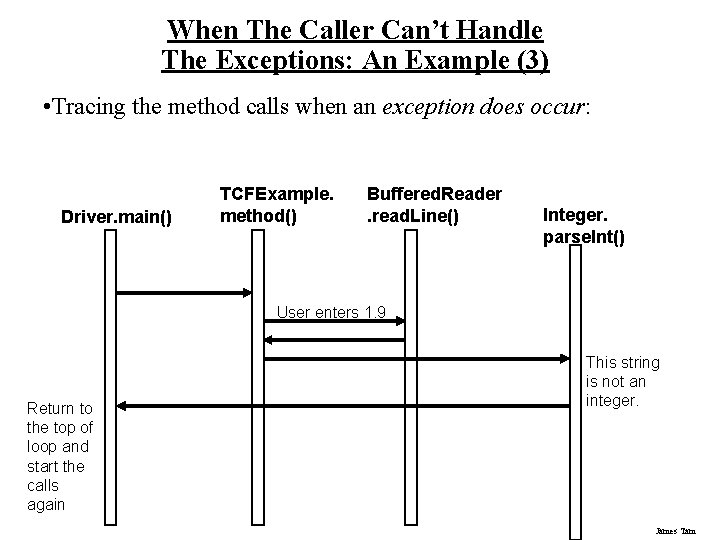
When The Caller Can’t Handle The Exceptions: An Example (3) • Tracing the method calls when an exception does occur: Driver. main() TCFExample. method() Buffered. Reader. read. Line() Integer. parse. Int() User enters 1. 9 Return to the top of loop and start the calls again This string is not an integer. James Tam
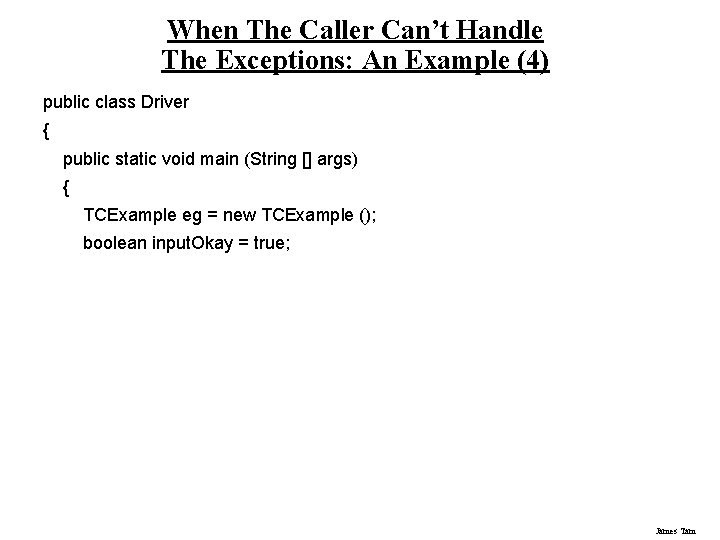
When The Caller Can’t Handle The Exceptions: An Example (4) public class Driver { public static void main (String [] args) { TCExample eg = new TCExample (); boolean input. Okay = true; James Tam
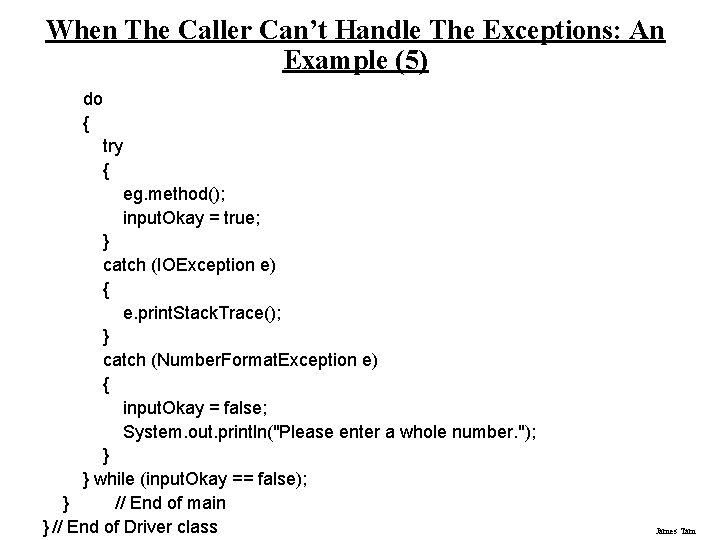
When The Caller Can’t Handle The Exceptions: An Example (5) do { try { eg. method(); input. Okay = true; } catch (IOException e) { e. print. Stack. Trace(); } catch (Number. Format. Exception e) { input. Okay = false; System. out. println("Please enter a whole number. "); } } while (input. Okay == false); } // End of main } // End of Driver class James Tam
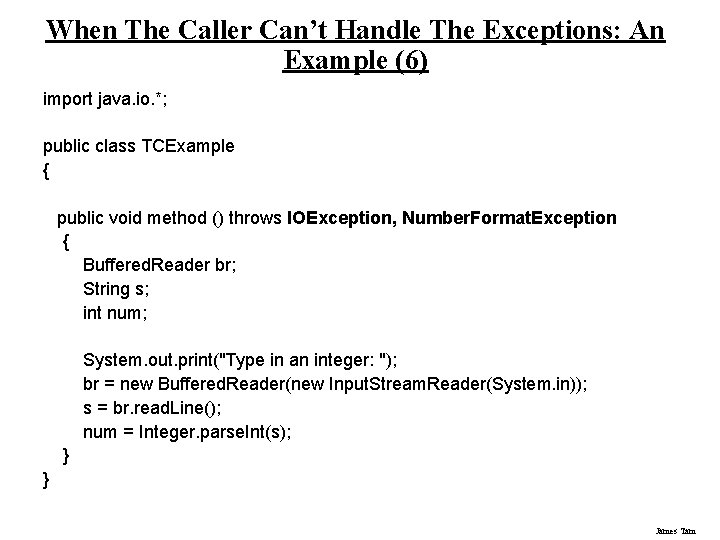
When The Caller Can’t Handle The Exceptions: An Example (6) import java. io. *; public class TCExample { public void method () throws IOException, Number. Format. Exception { Buffered. Reader br; String s; int num; System. out. print("Type in an integer: "); br = new Buffered. Reader(new Input. Stream. Reader(System. in)); s = br. read. Line(); num = Integer. parse. Int(s); } } James Tam
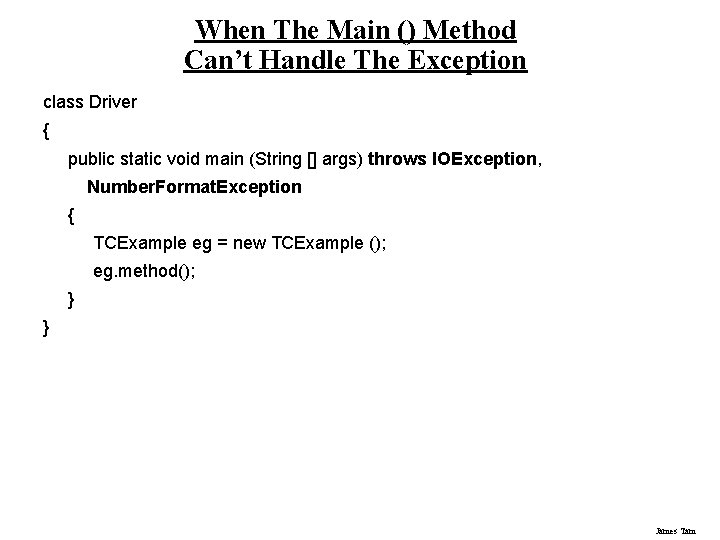
When The Main () Method Can’t Handle The Exception class Driver { public static void main (String [] args) throws IOException, Number. Format. Exception { TCExample eg = new TCExample (); eg. method(); } } James Tam
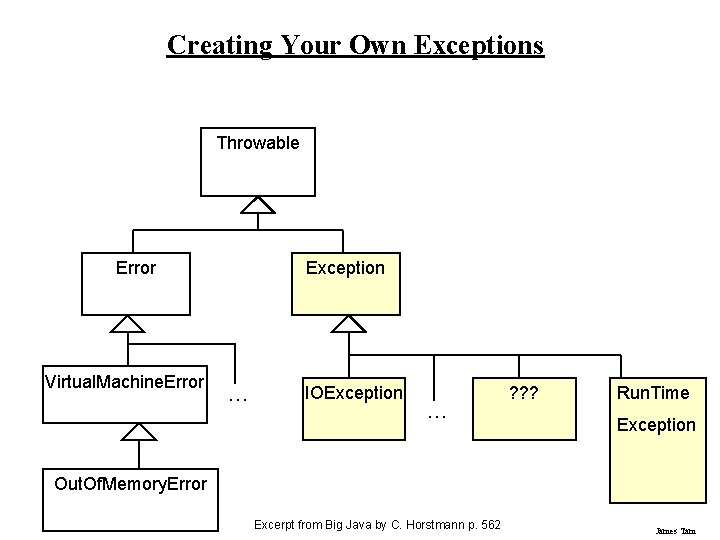
Creating Your Own Exceptions Throwable Error Virtual. Machine. Error Exception … IOException … ? ? ? Run. Time Exception Out. Of. Memory. Error Excerpt from Big Java by C. Horstmann p. 562 James Tam
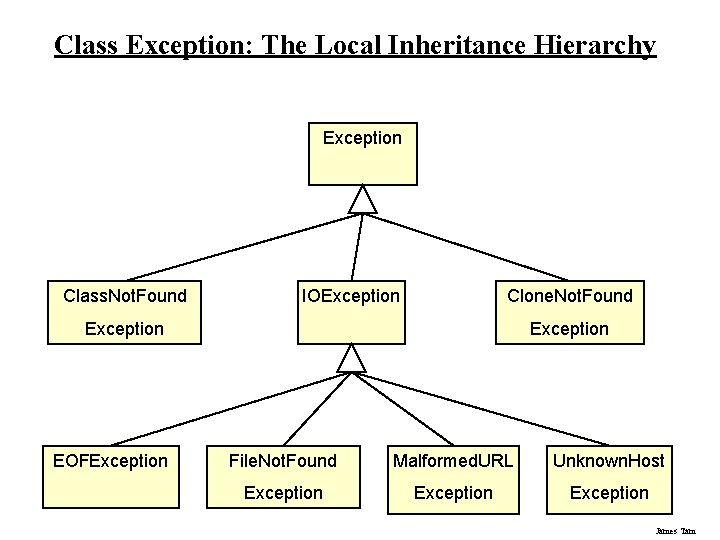
Class Exception: The Local Inheritance Hierarchy Exception Class. Not. Found IOException Clone. Not. Found Exception EOFException File. Not. Found Malformed. URL Unknown. Host Exception James Tam
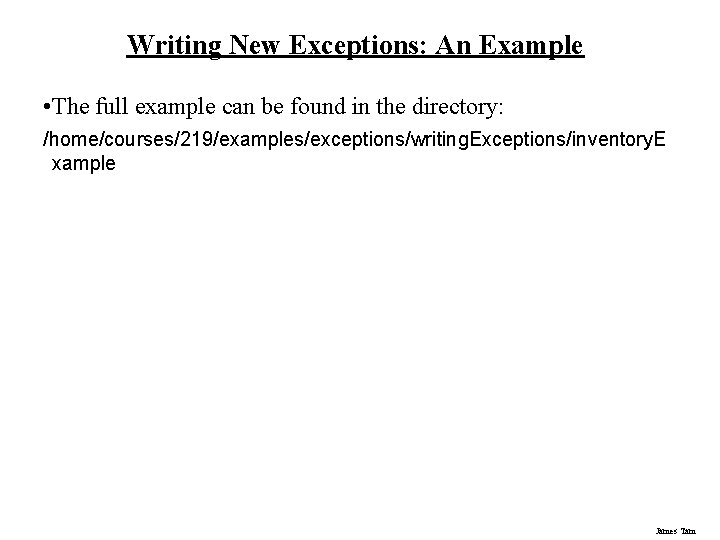
Writing New Exceptions: An Example • The full example can be found in the directory: /home/courses/219/examples/exceptions/writing. Exceptions/inventory. E xample James Tam
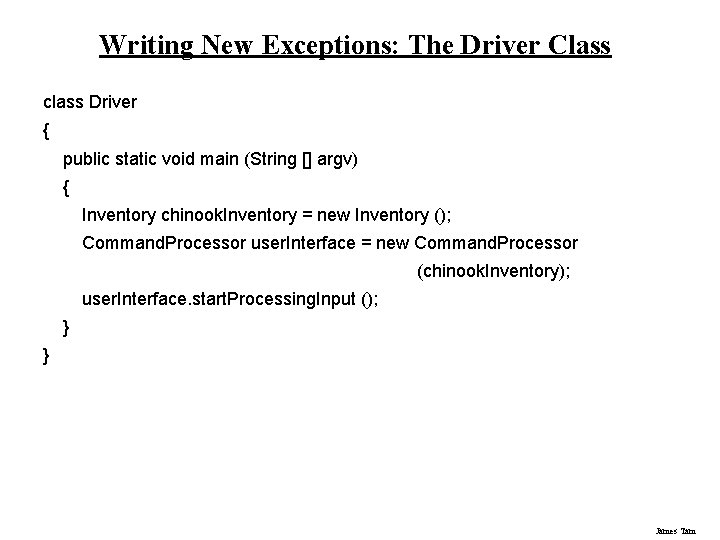
Writing New Exceptions: The Driver Class class Driver { public static void main (String [] argv) { Inventory chinook. Inventory = new Inventory (); Command. Processor user. Interface = new Command. Processor (chinook. Inventory); user. Interface. start. Processing. Input (); } } James Tam
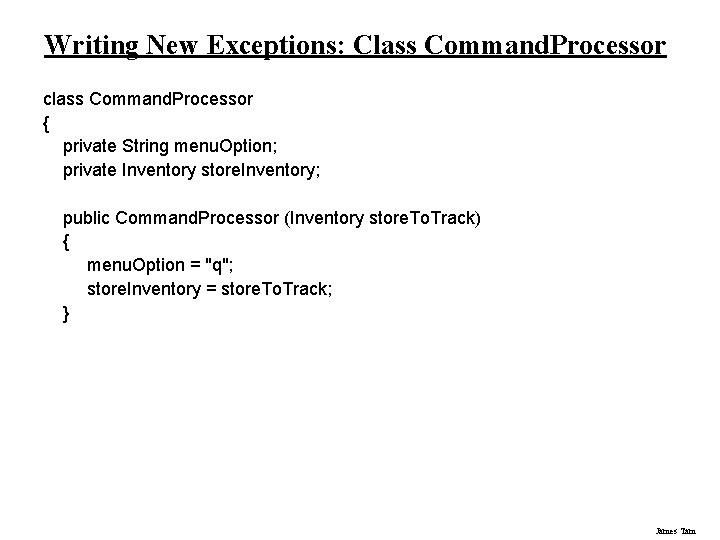
Writing New Exceptions: Class Command. Processor class Command. Processor { private String menu. Option; private Inventory store. Inventory; public Command. Processor (Inventory store. To. Track) { menu. Option = "q"; store. Inventory = store. To. Track; } James Tam
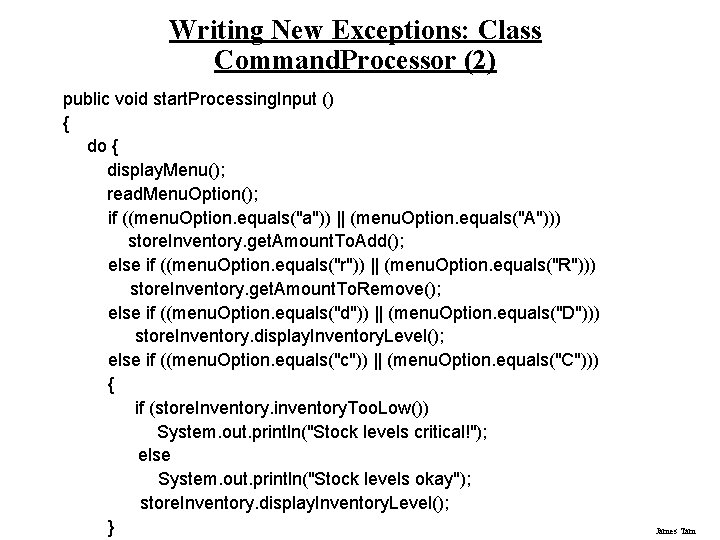
Writing New Exceptions: Class Command. Processor (2) public void start. Processing. Input () { do { display. Menu(); read. Menu. Option(); if ((menu. Option. equals("a")) || (menu. Option. equals("A"))) store. Inventory. get. Amount. To. Add(); else if ((menu. Option. equals("r")) || (menu. Option. equals("R"))) store. Inventory. get. Amount. To. Remove(); else if ((menu. Option. equals("d")) || (menu. Option. equals("D"))) store. Inventory. display. Inventory. Level(); else if ((menu. Option. equals("c")) || (menu. Option. equals("C"))) { if (store. Inventory. inventory. Too. Low()) System. out. println("Stock levels critical!"); else System. out. println("Stock levels okay"); store. Inventory. display. Inventory. Level(); } James Tam
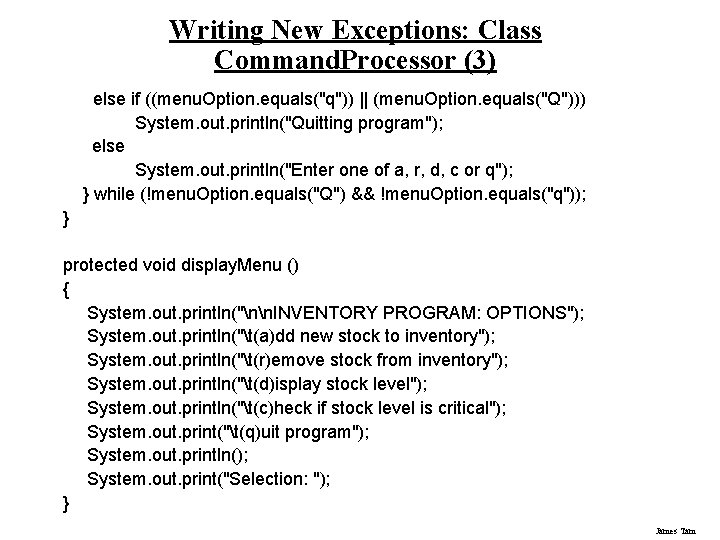
Writing New Exceptions: Class Command. Processor (3) else if ((menu. Option. equals("q")) || (menu. Option. equals("Q"))) System. out. println("Quitting program"); else System. out. println("Enter one of a, r, d, c or q"); } while (!menu. Option. equals("Q") && !menu. Option. equals("q")); } protected void display. Menu () { System. out. println("nn. INVENTORY PROGRAM: OPTIONS"); System. out. println("t(a)dd new stock to inventory"); System. out. println("t(r)emove stock from inventory"); System. out. println("t(d)isplay stock level"); System. out. println("t(c)heck if stock level is critical"); System. out. print("t(q)uit program"); System. out. println(); System. out. print("Selection: "); } James Tam
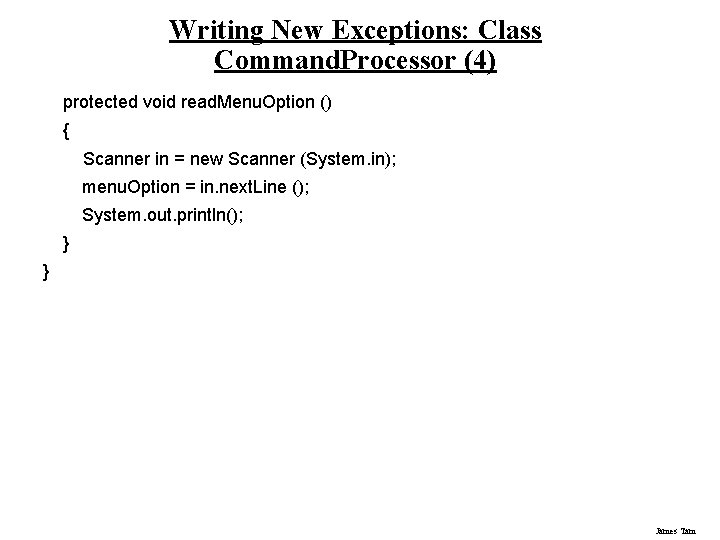
Writing New Exceptions: Class Command. Processor (4) protected void read. Menu. Option () { Scanner in = new Scanner (System. in); menu. Option = in. next. Line (); System. out. println(); } } James Tam
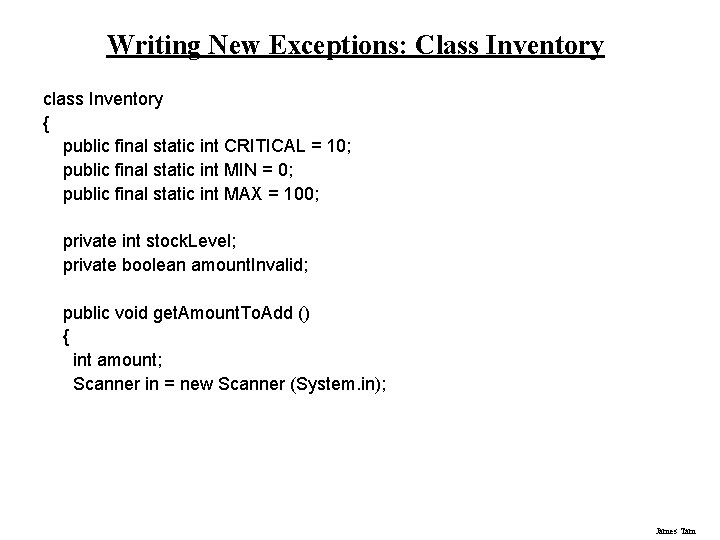
Writing New Exceptions: Class Inventory class Inventory { public final static int CRITICAL = 10; public final static int MIN = 0; public final static int MAX = 100; private int stock. Level; private boolean amount. Invalid; public void get. Amount. To. Add () { int amount; Scanner in = new Scanner (System. in); James Tam
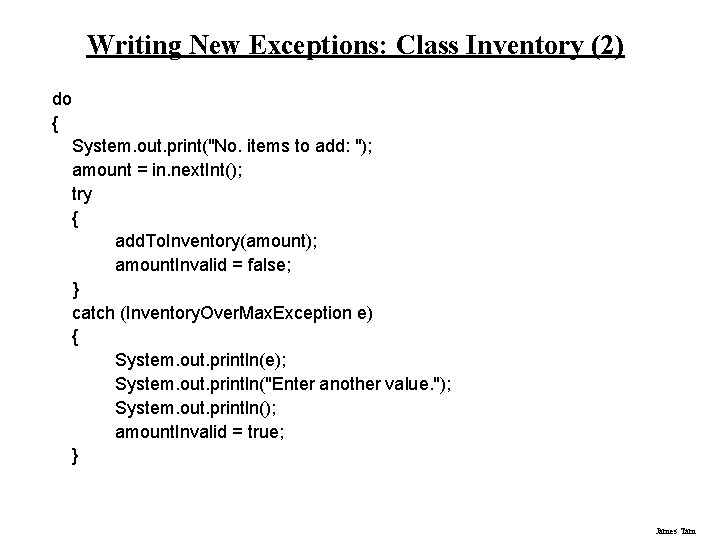
Writing New Exceptions: Class Inventory (2) do { System. out. print("No. items to add: "); amount = in. next. Int(); try { add. To. Inventory(amount); amount. Invalid = false; } catch (Inventory. Over. Max. Exception e) { System. out. println(e); System. out. println("Enter another value. "); System. out. println(); amount. Invalid = true; } James Tam
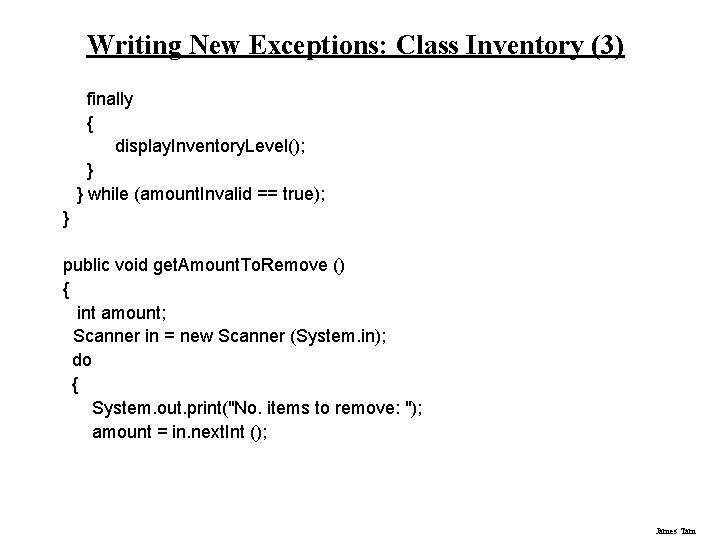
Writing New Exceptions: Class Inventory (3) finally { display. Inventory. Level(); } } while (amount. Invalid == true); } public void get. Amount. To. Remove () { int amount; Scanner in = new Scanner (System. in); do { System. out. print("No. items to remove: "); amount = in. next. Int (); James Tam
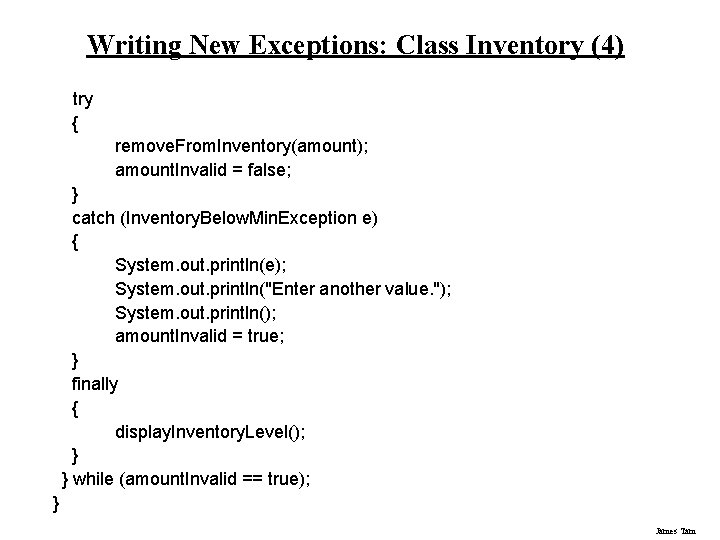
Writing New Exceptions: Class Inventory (4) try { remove. From. Inventory(amount); amount. Invalid = false; } catch (Inventory. Below. Min. Exception e) { System. out. println(e); System. out. println("Enter another value. "); System. out. println(); amount. Invalid = true; } finally { display. Inventory. Level(); } } while (amount. Invalid == true); } James Tam
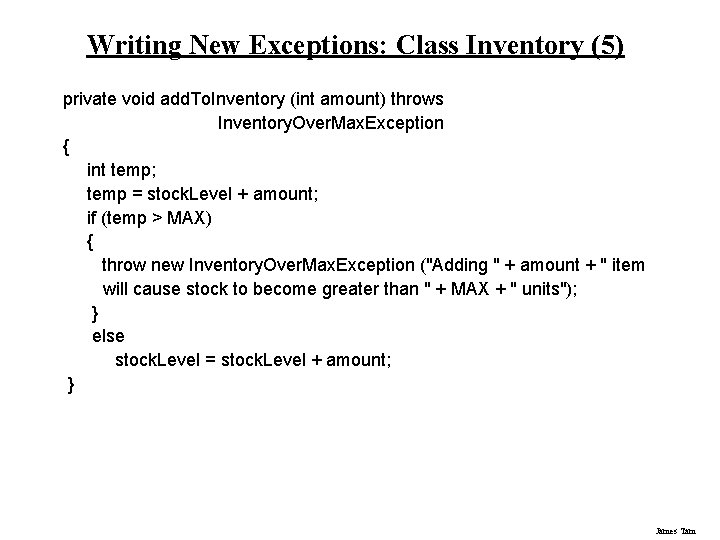
Writing New Exceptions: Class Inventory (5) private void add. To. Inventory (int amount) throws Inventory. Over. Max. Exception { int temp; temp = stock. Level + amount; if (temp > MAX) { throw new Inventory. Over. Max. Exception ("Adding " + amount + " item will cause stock to become greater than " + MAX + " units"); } else stock. Level = stock. Level + amount; } James Tam
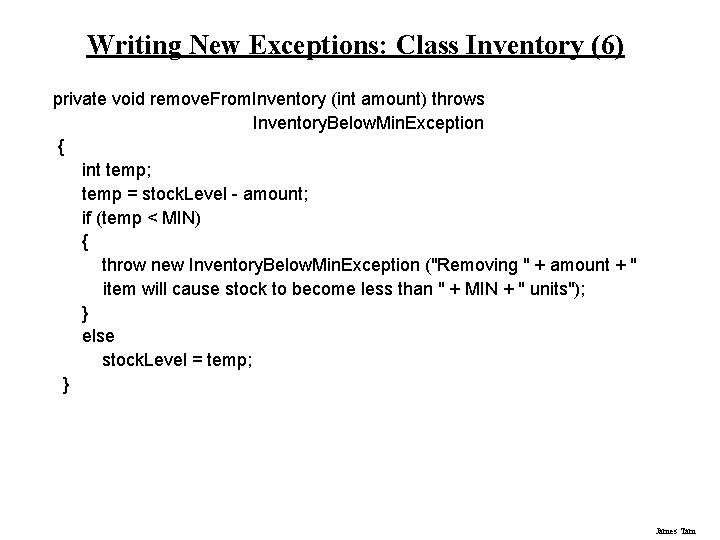
Writing New Exceptions: Class Inventory (6) private void remove. From. Inventory (int amount) throws Inventory. Below. Min. Exception { int temp; temp = stock. Level - amount; if (temp < MIN) { throw new Inventory. Below. Min. Exception ("Removing " + amount + " item will cause stock to become less than " + MIN + " units"); } else stock. Level = temp; } James Tam
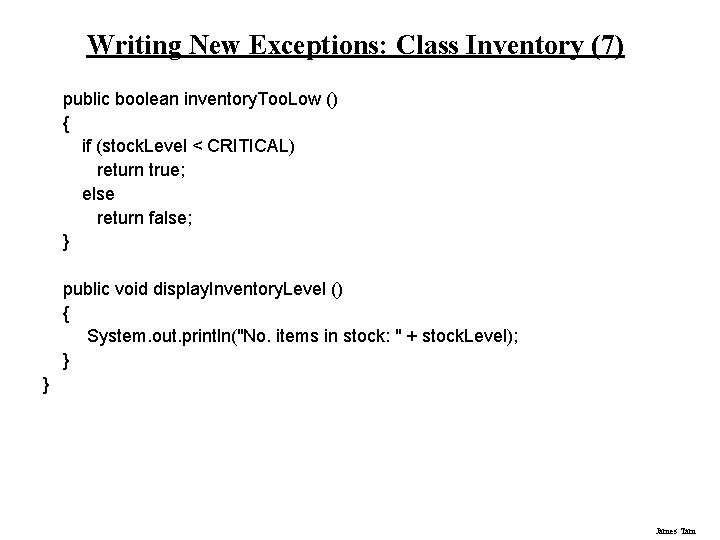
Writing New Exceptions: Class Inventory (7) public boolean inventory. Too. Low () { if (stock. Level < CRITICAL) return true; else return false; } public void display. Inventory. Level () { System. out. println("No. items in stock: " + stock. Level); } } James Tam
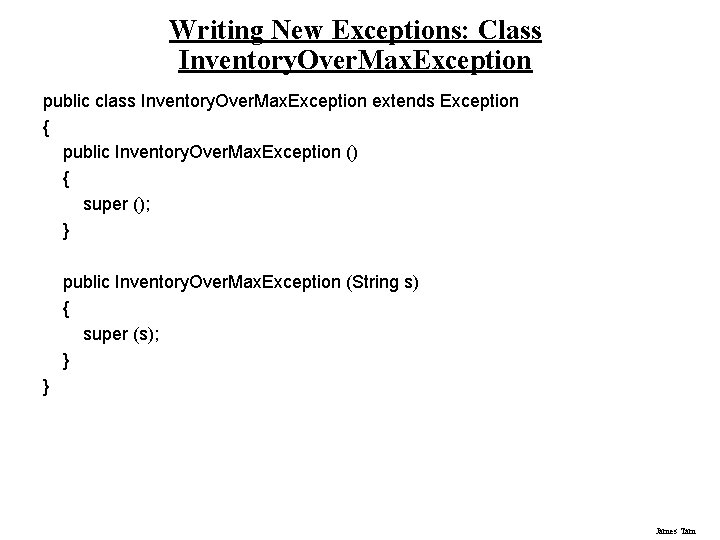
Writing New Exceptions: Class Inventory. Over. Max. Exception public class Inventory. Over. Max. Exception extends Exception { public Inventory. Over. Max. Exception () { super (); } public Inventory. Over. Max. Exception (String s) { super (s); } } James Tam
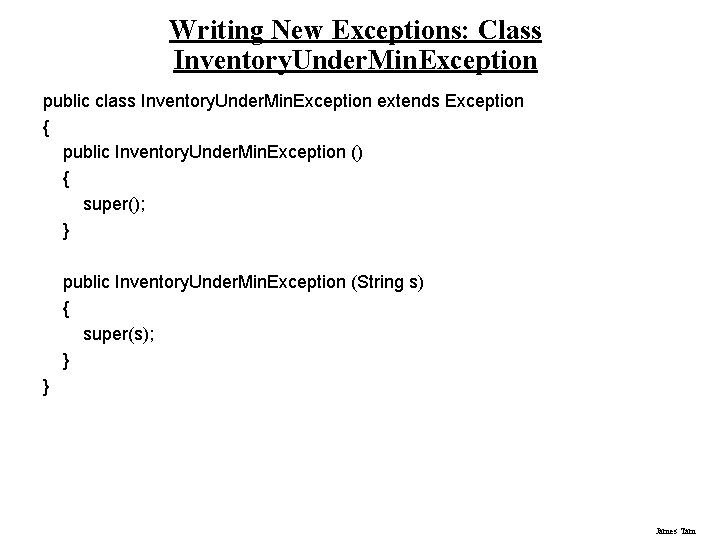
Writing New Exceptions: Class Inventory. Under. Min. Exception public class Inventory. Under. Min. Exception extends Exception { public Inventory. Under. Min. Exception () { super(); } public Inventory. Under. Min. Exception (String s) { super(s); } } James Tam
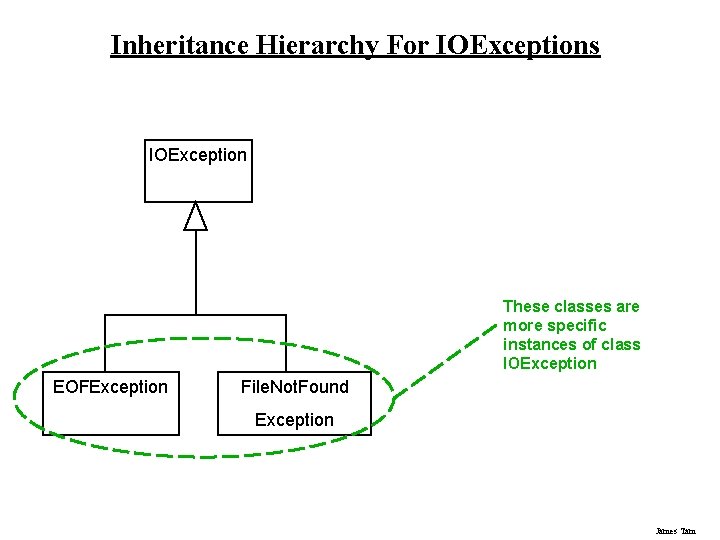
Inheritance Hierarchy For IOExceptions IOException These classes are more specific instances of class IOException EOFException File. Not. Found Exception James Tam
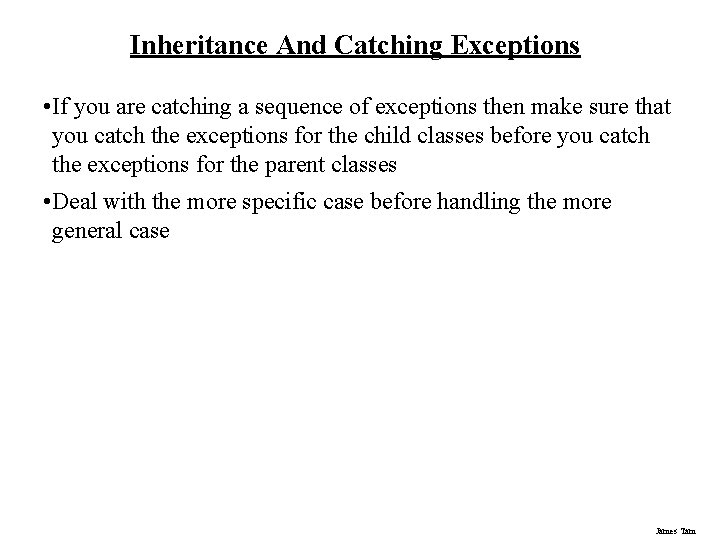
Inheritance And Catching Exceptions • If you are catching a sequence of exceptions then make sure that you catch the exceptions for the child classes before you catch the exceptions for the parent classes • Deal with the more specific case before handling the more general case James Tam
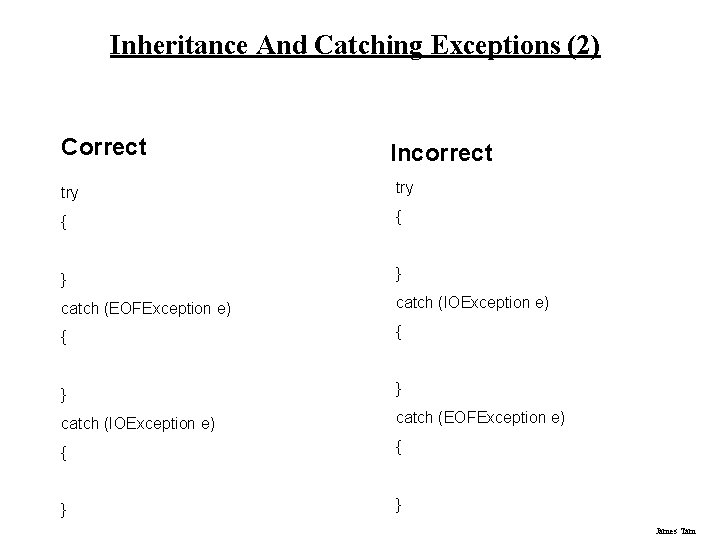
Inheritance And Catching Exceptions (2) Correct Incorrect try { { } } catch (EOFException e) catch (IOException e) { { } } catch (IOException e) catch (EOFException e) { { } } James Tam
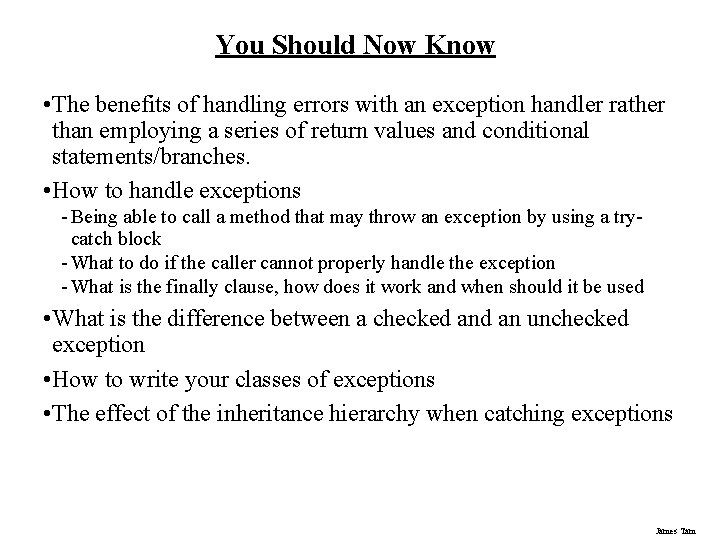
You Should Now Know • The benefits of handling errors with an exception handler rather than employing a series of return values and conditional statements/branches. • How to handle exceptions - Being able to call a method that may throw an exception by using a trycatch block - What to do if the caller cannot properly handle the exception - What is the finally clause, how does it work and when should it be used • What is the difference between a checked an unchecked exception • How to write your classes of exceptions • The effect of the inheritance hierarchy when catching exceptions James Tam
Switch case javas
Exception handling in java
Exception handling pada java
Exception handling pada java
Exception handling pl sql
Vb net error handling
Php exception handler
No irq handler for vector
Exception handling in ada
Php throw exception
Vb net error handling
Extends and implements difference
Java unchecked exceptions list
Exception vs error in java
Java exception hierarchy
Java exception error
Java unchecked exception
Java exception localized message
Java unchecked exception
Gui event handling
Java import java.util.*
Java import java.util.*
Java applet swing
Import java util
Java 讀檔
Java import java.util.*
Str2str 使い方
Import java.io.*
Public class
Java thread import
Perbedaan swing dan awt
Import java.awt.* import java.awt.event.*
Java interpreter
Ejb javatpoint
Payroll system using polymorphism in java
Automate java application
Java rmi example
Classic problems of synchronization
Java inheritance bank account example
Java definition
Data structures using java
Moving ball program in java using applet
Binary search tree java
Data structures using java
Send email using java
Android boot camp for developers using java
Android boot camp for developers using java
System.collections.generics
Defrost using internal heat is accomplished using
System argument exception
Public interface foo
Class a void foo() throws exception
Unt concur
Coccus
Runtimeexception extends exception
What is exception?
Explain the exceptions to mendel's law
Charting by exception pros and cons
Exception of koch postulates
Apa itu exception
Goodsalls rule
Exception
Usfws designated ports
Dbs311 assignment 2
No data found exception in oracle
Contoh model pie
Http data source exception
Liraglutide ramq
What is exception in computer architecture
A programmer can choose to ignore an unchecked exception.
Jenis assertion
Exception