Exception Handling Exception HandlingFundamentals An exception is an
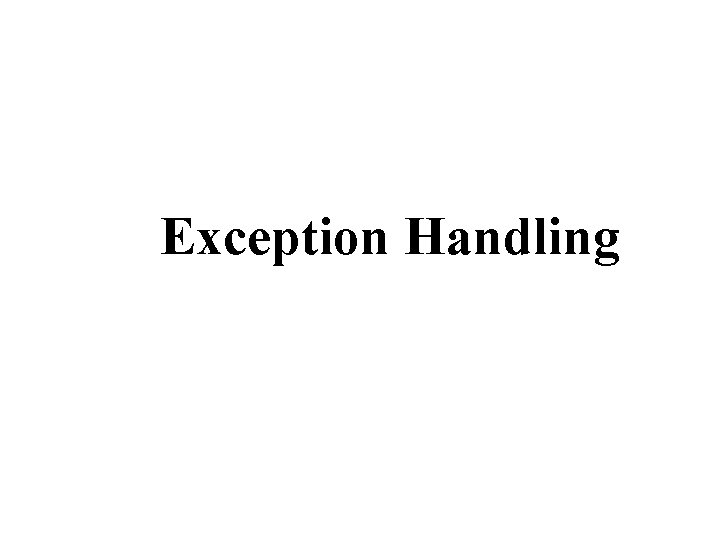
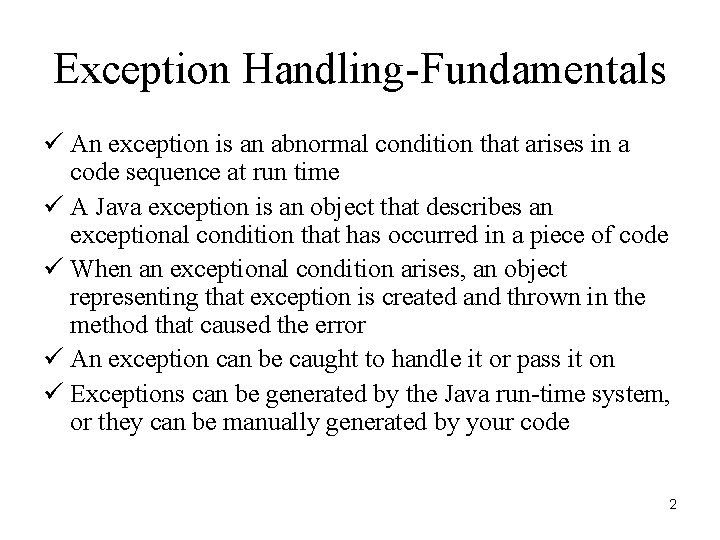
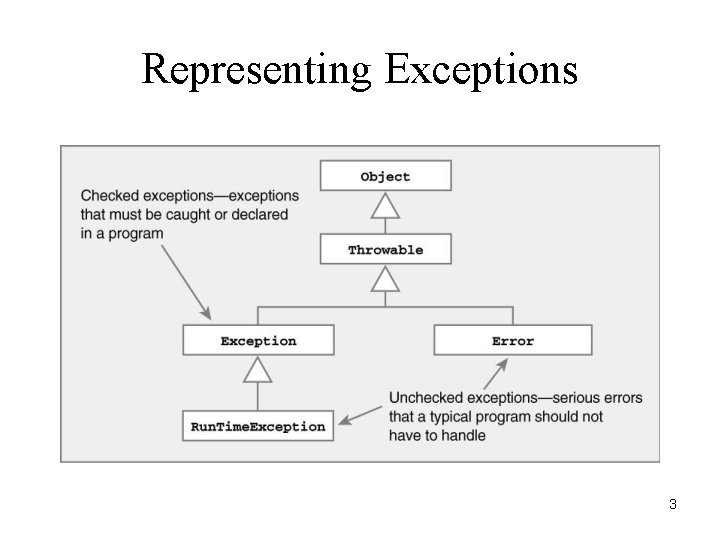
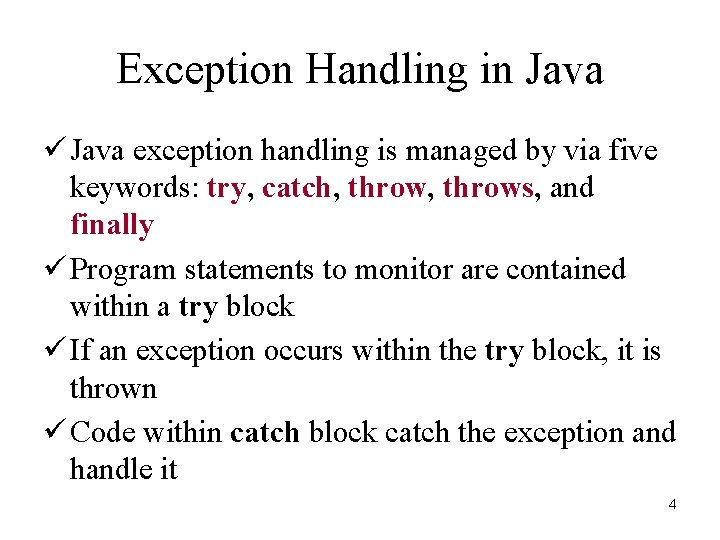
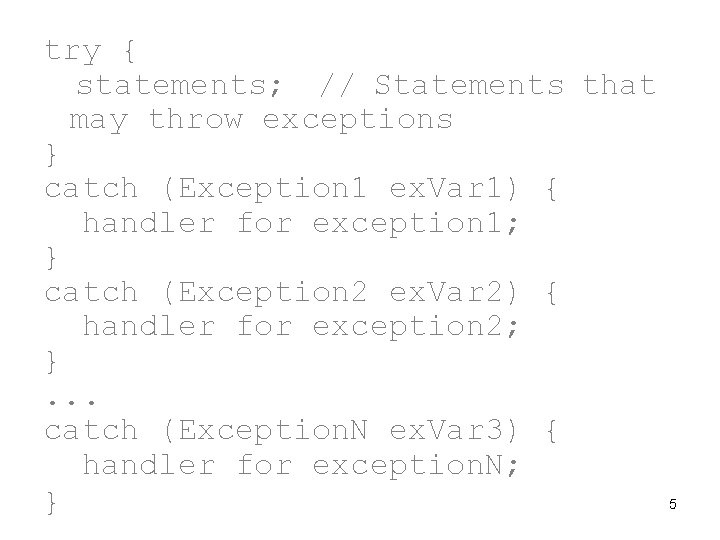
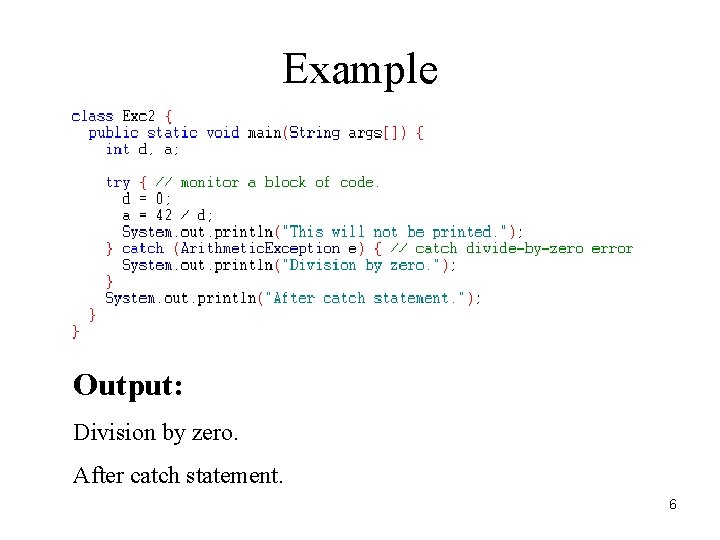
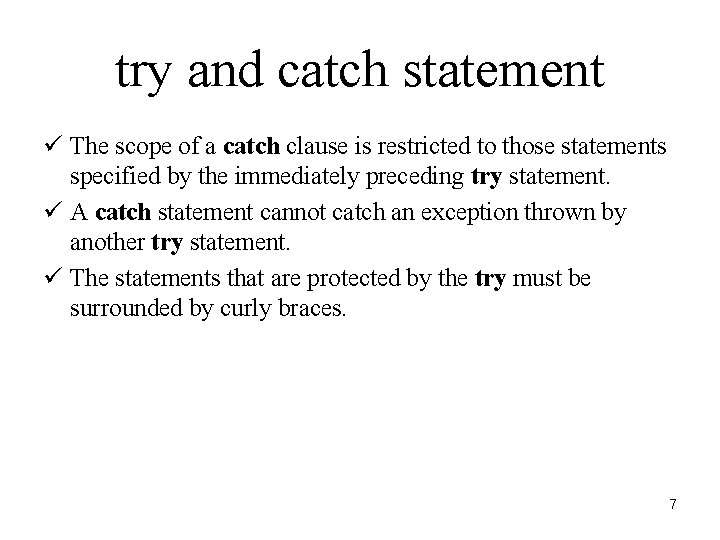
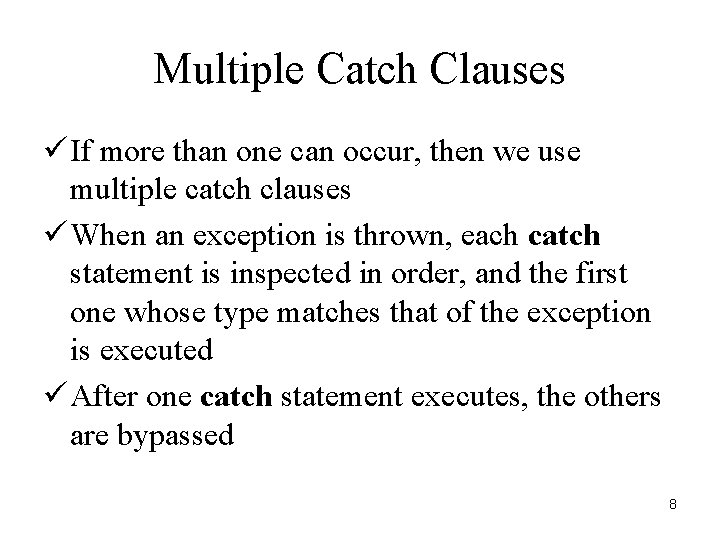
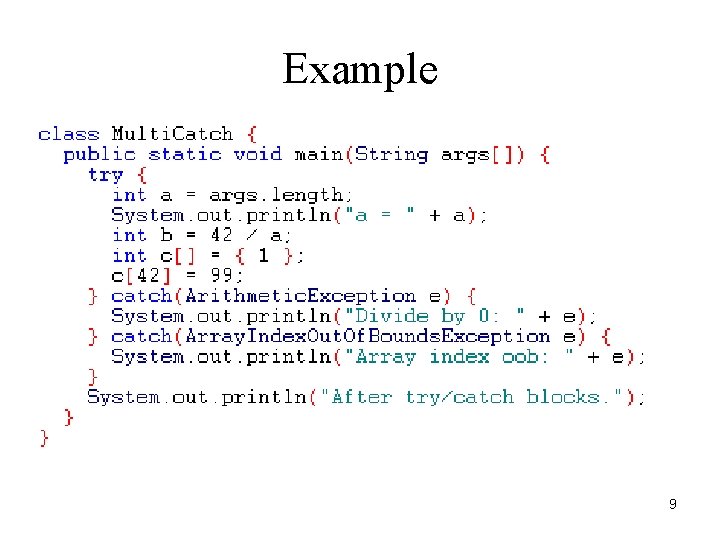
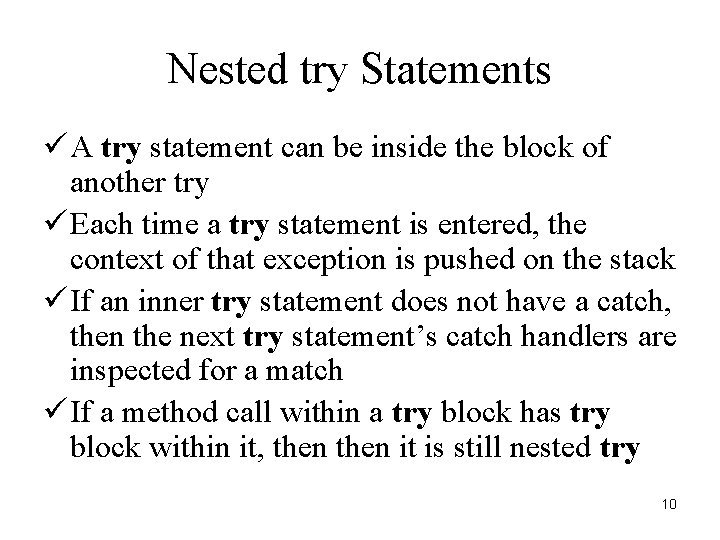
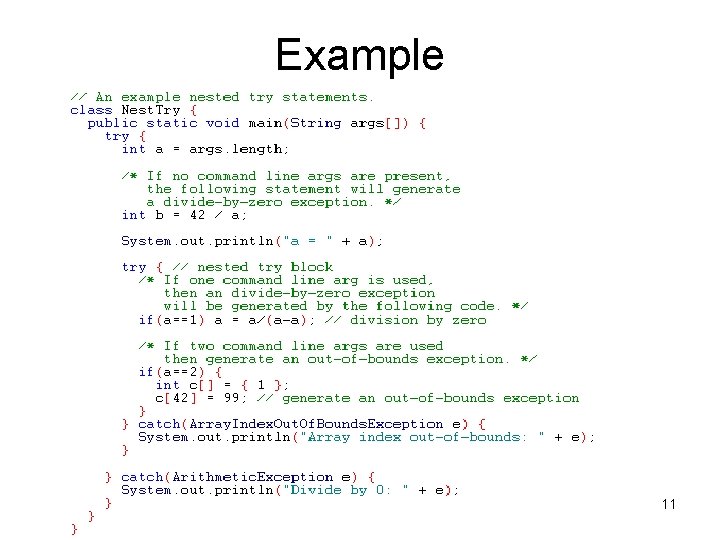
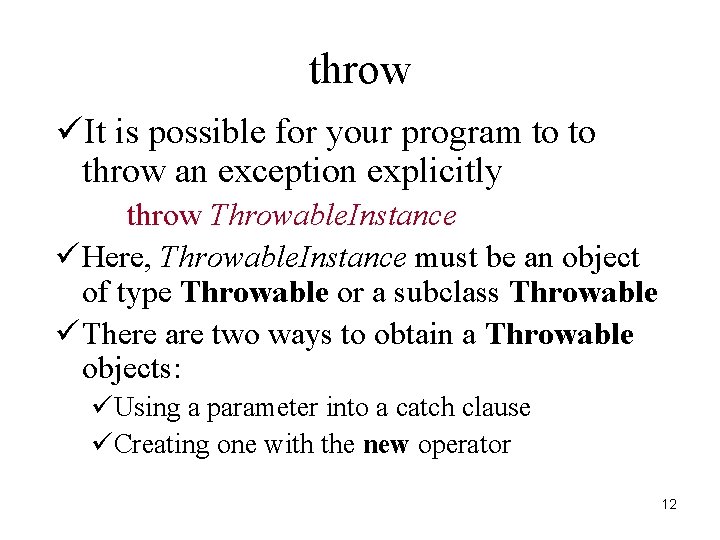
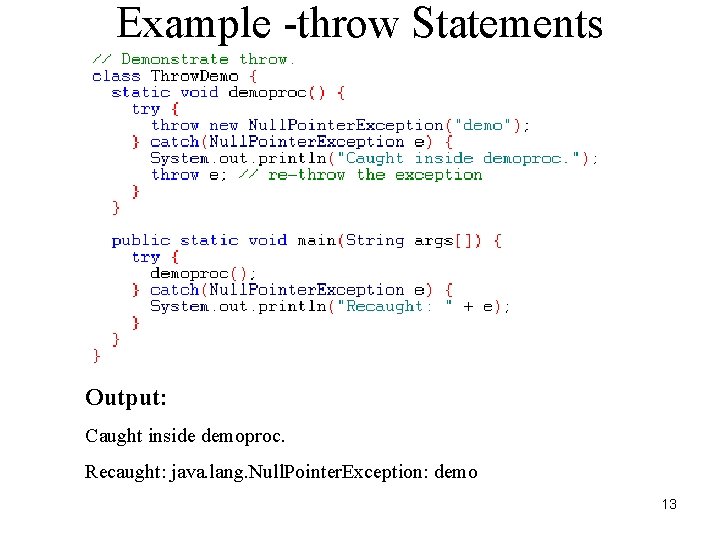
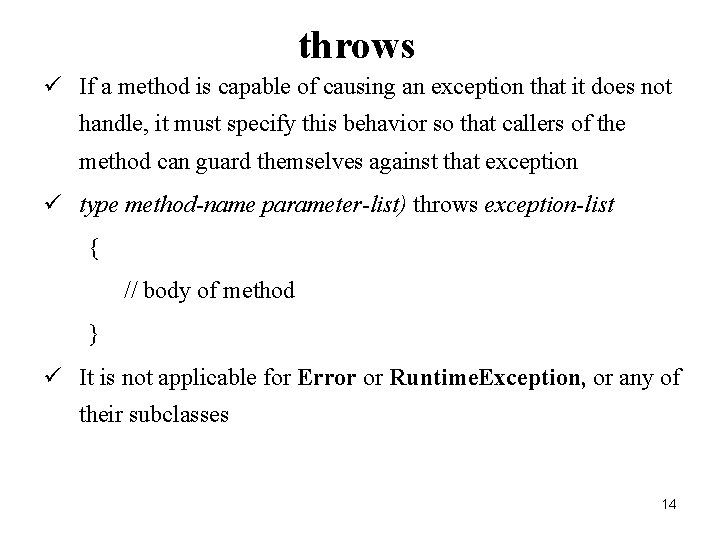
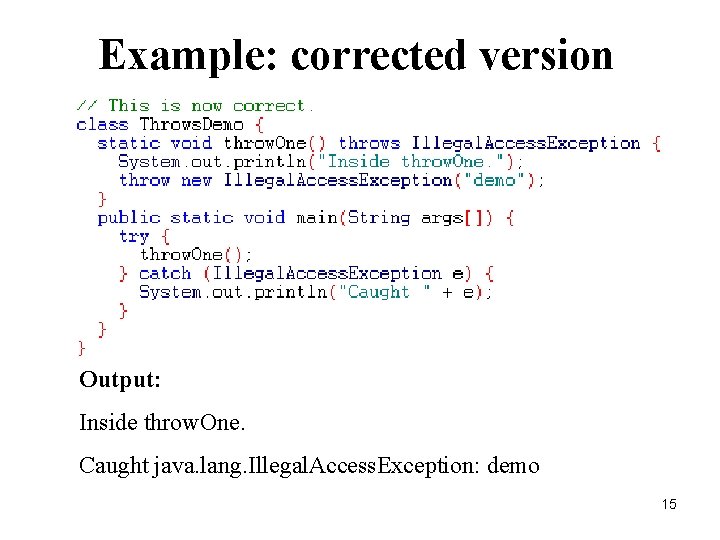
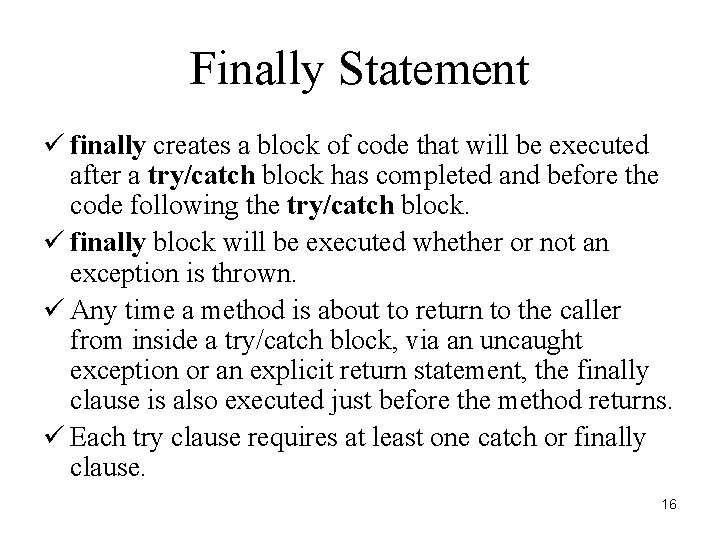
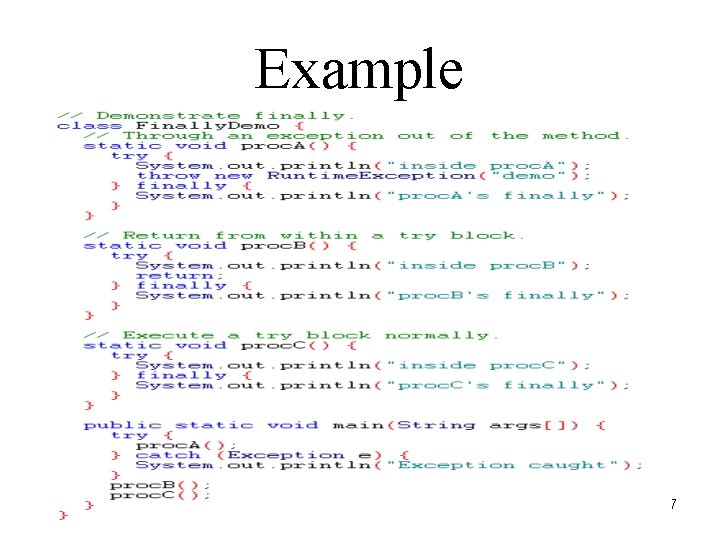
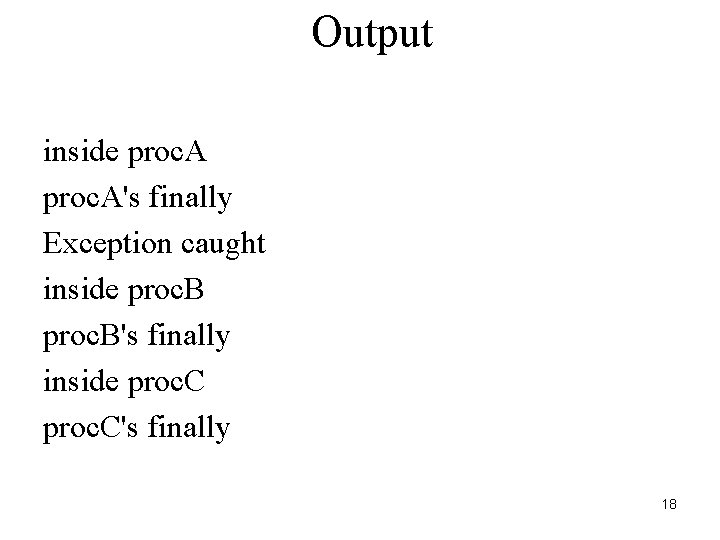
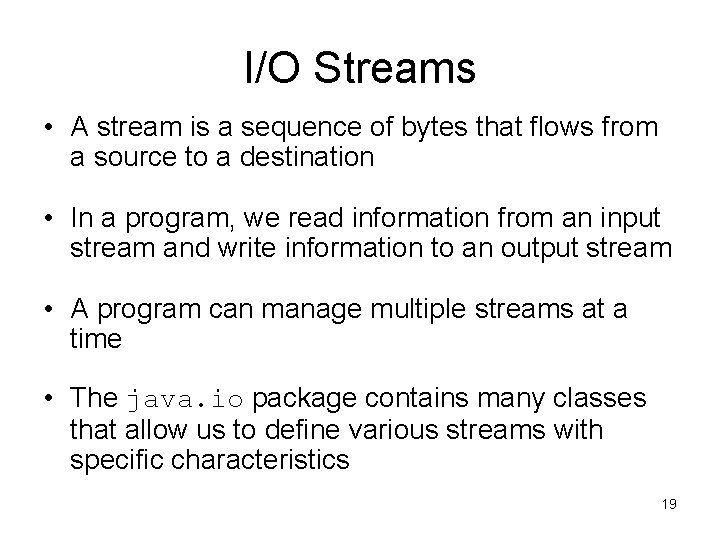
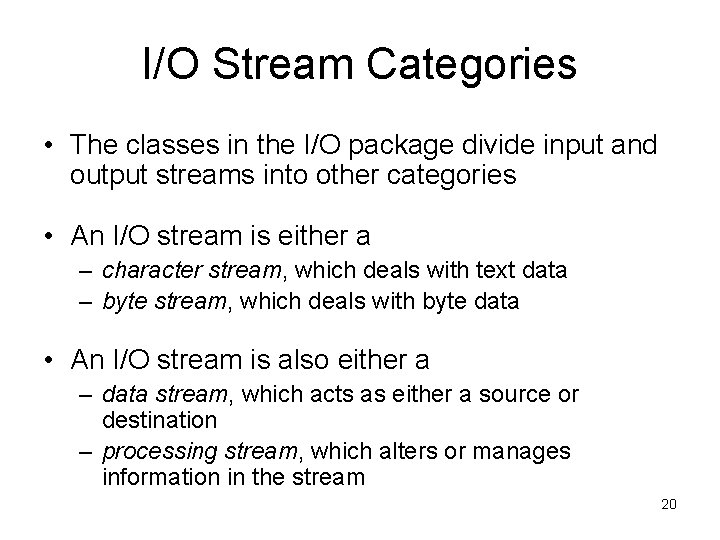
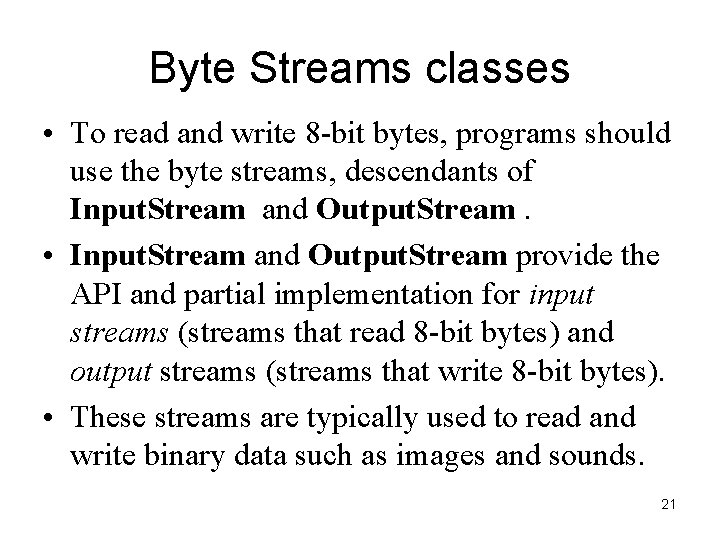
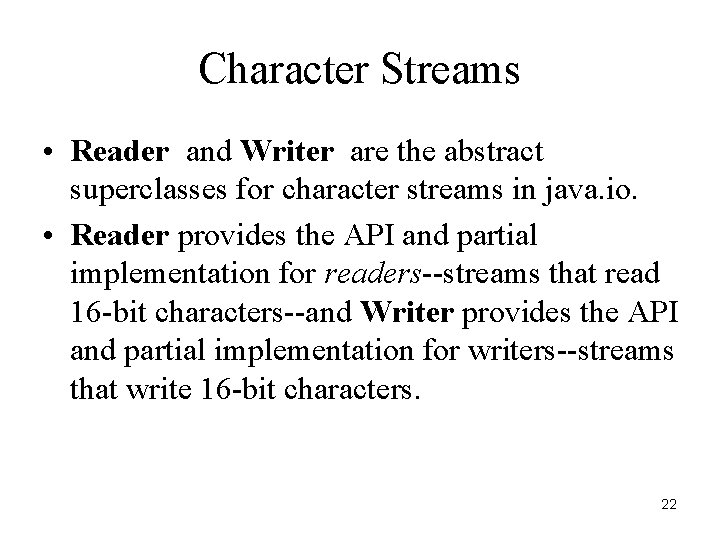
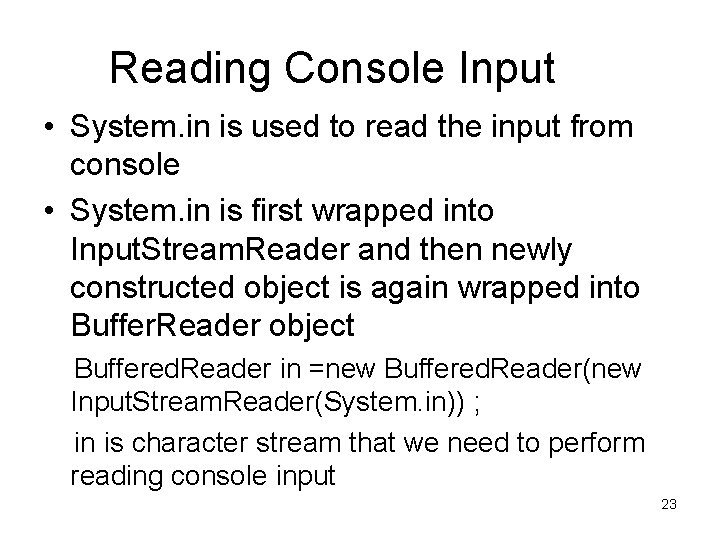
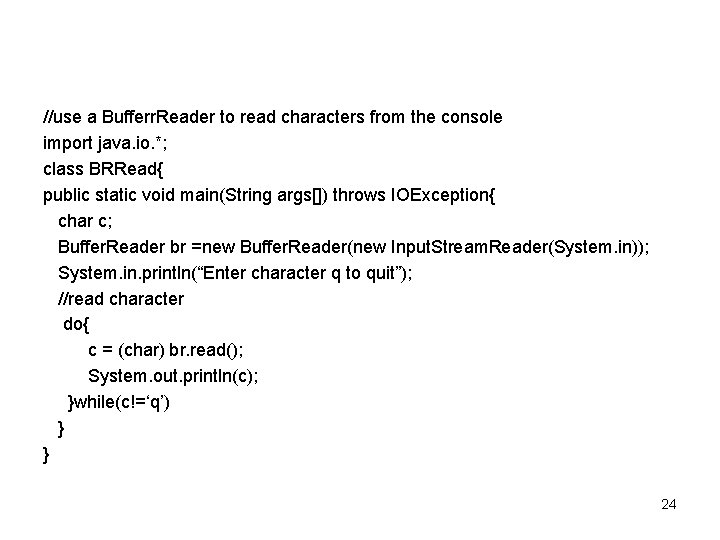
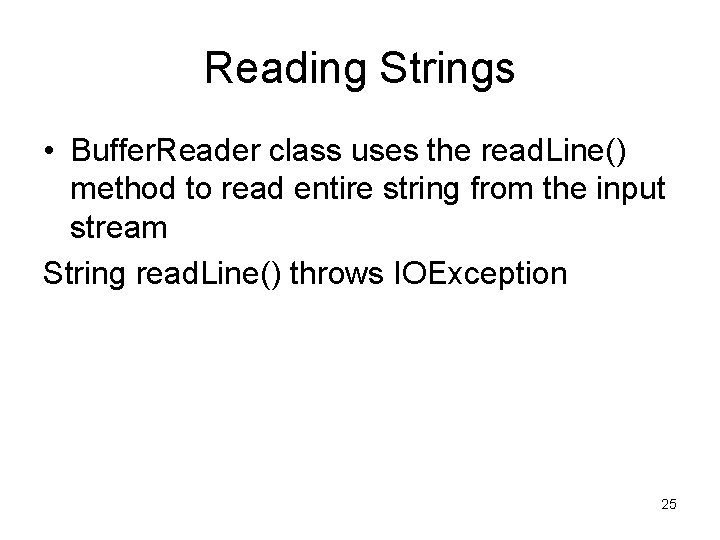
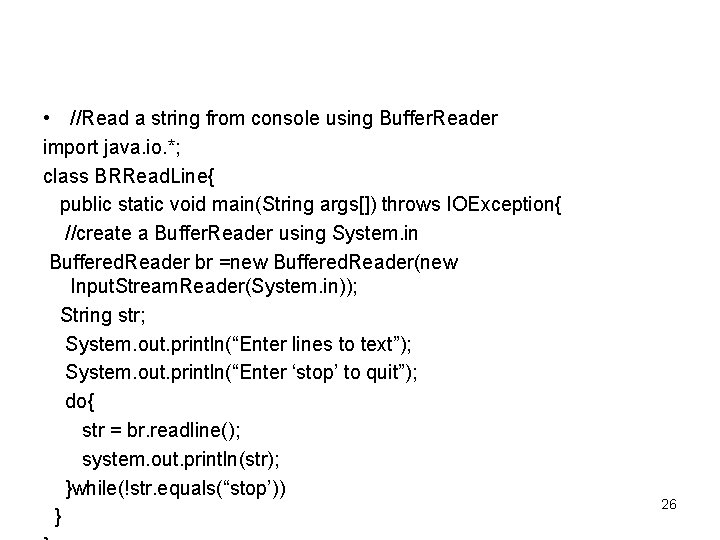
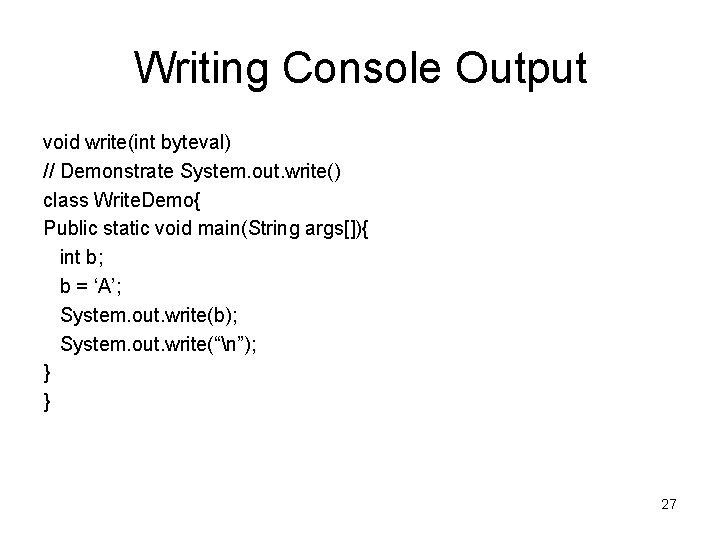
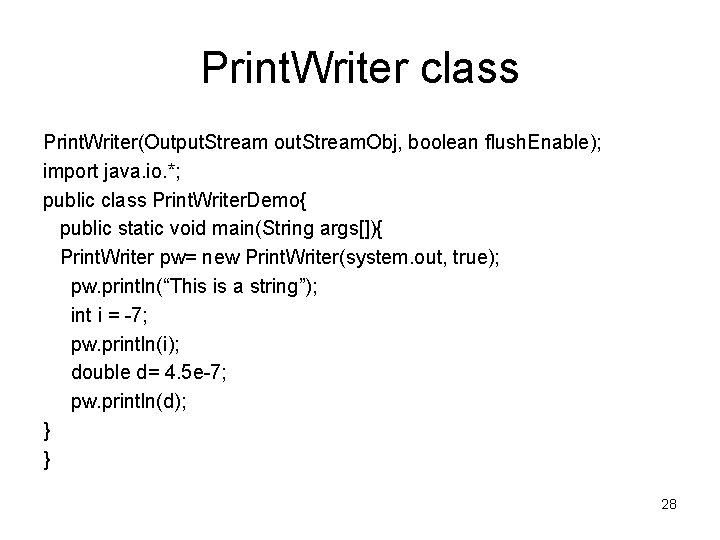
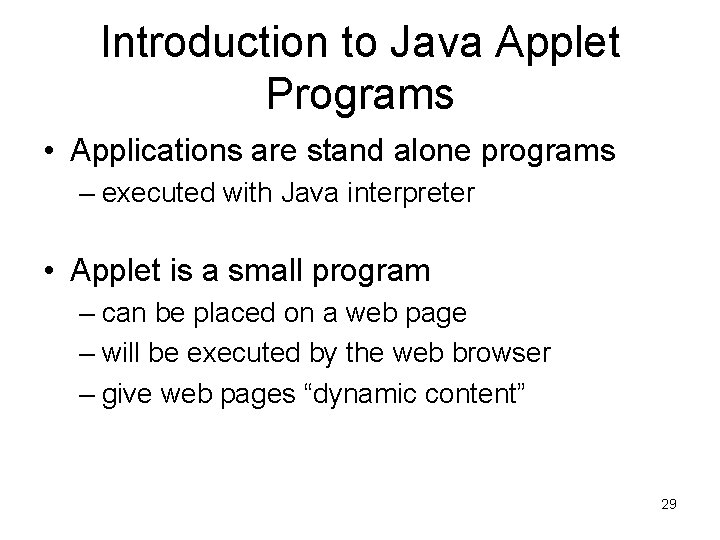
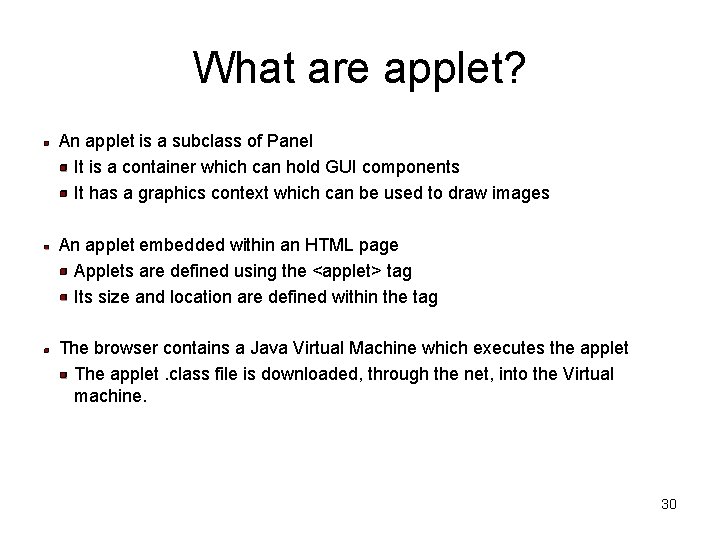
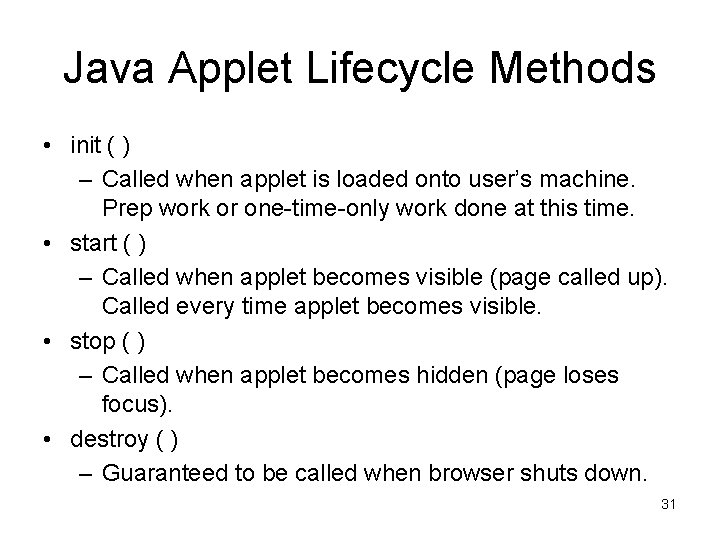
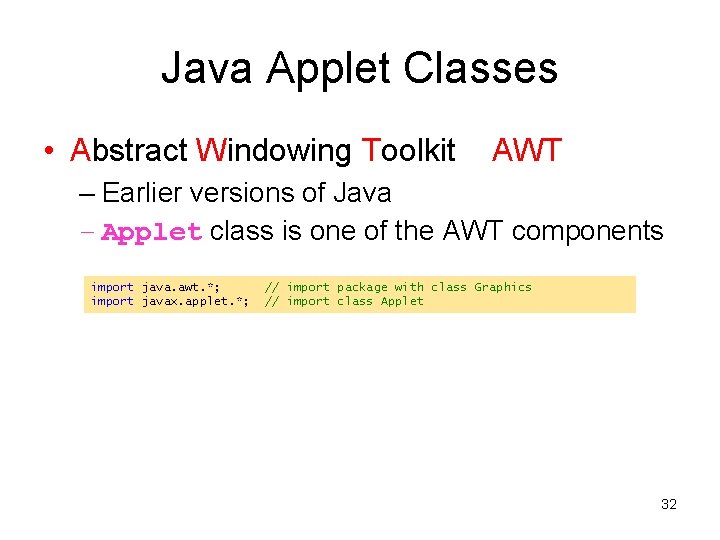
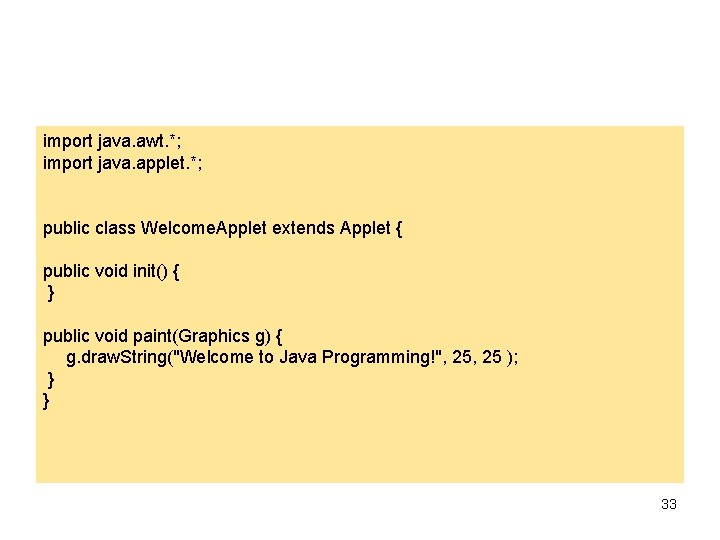
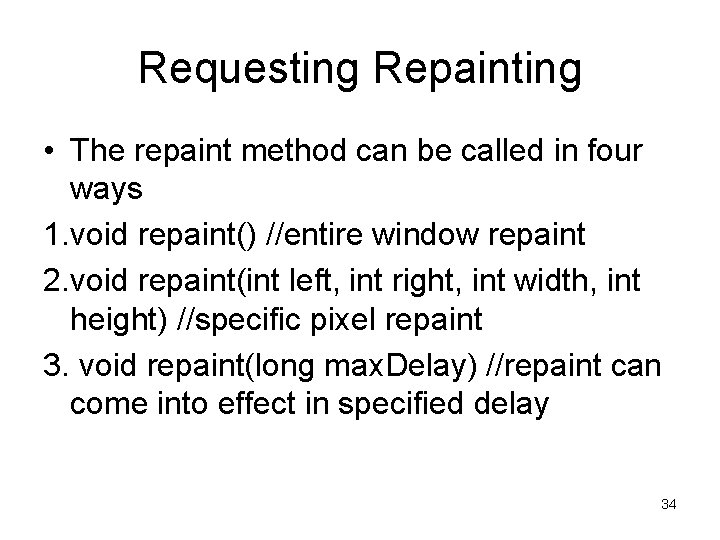
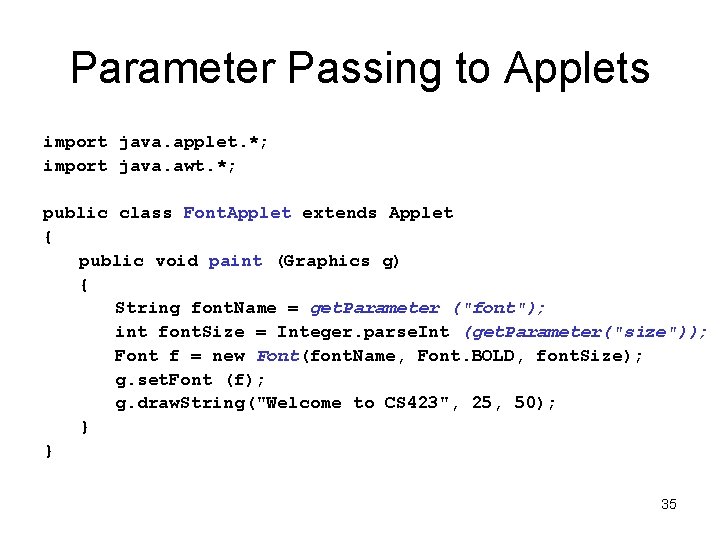
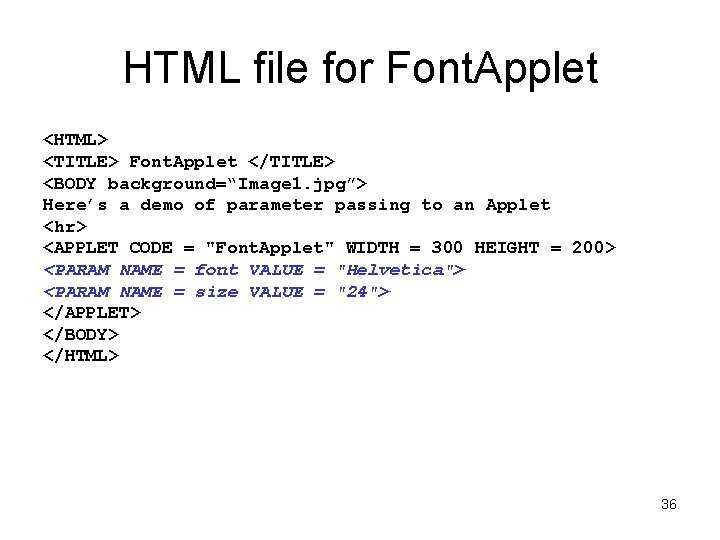
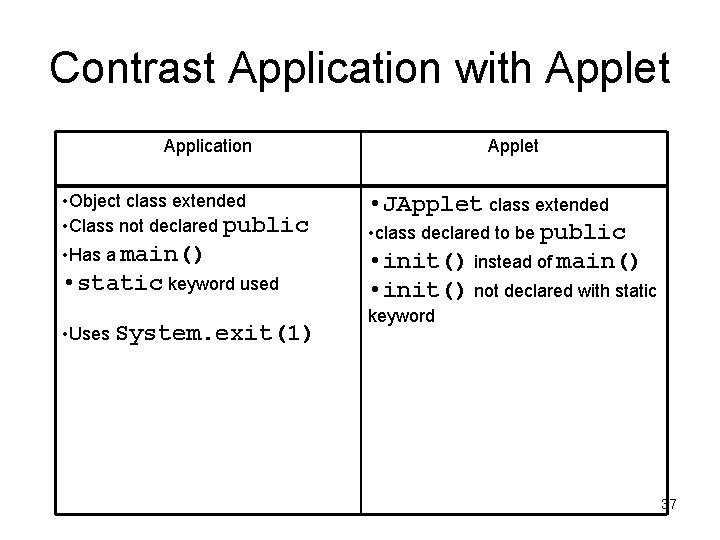
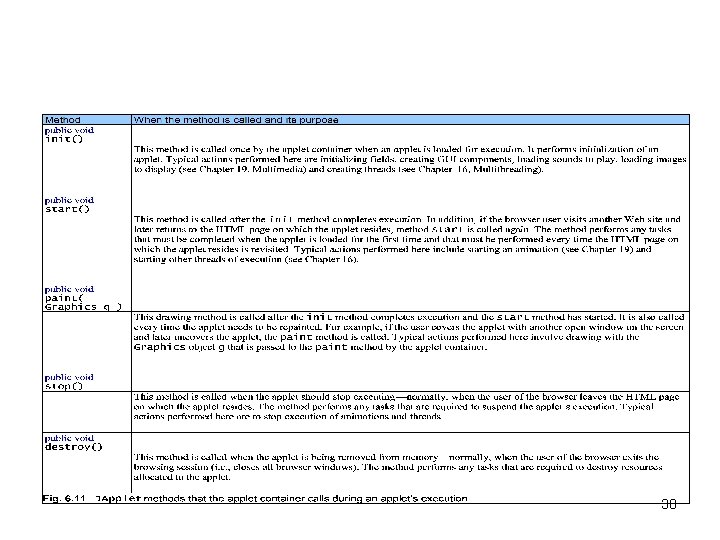
![Uncaught Exceptions class exc 0{ public static void main(String args[]) { int d=0; int Uncaught Exceptions class exc 0{ public static void main(String args[]) { int d=0; int](https://slidetodoc.com/presentation_image_h2/57f2df9395718cc70d5871cdfaaee5ac/image-39.jpg)
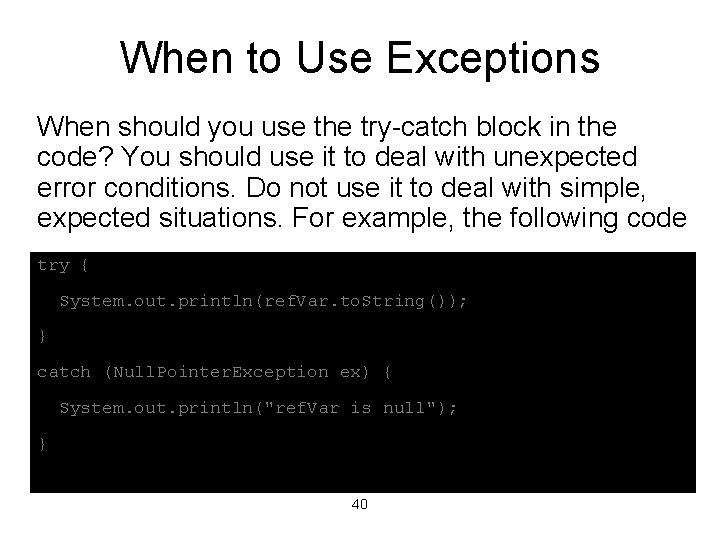
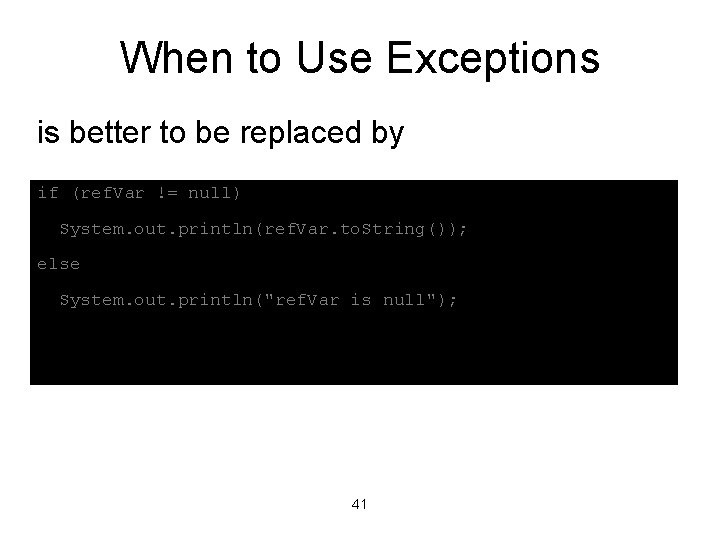
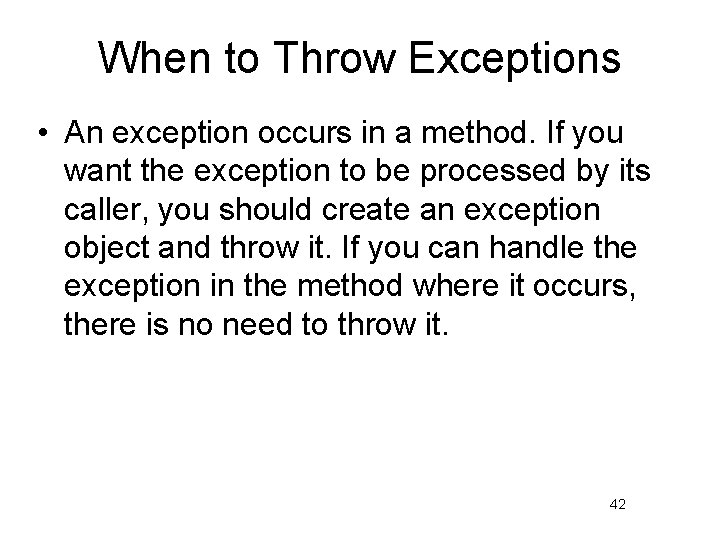
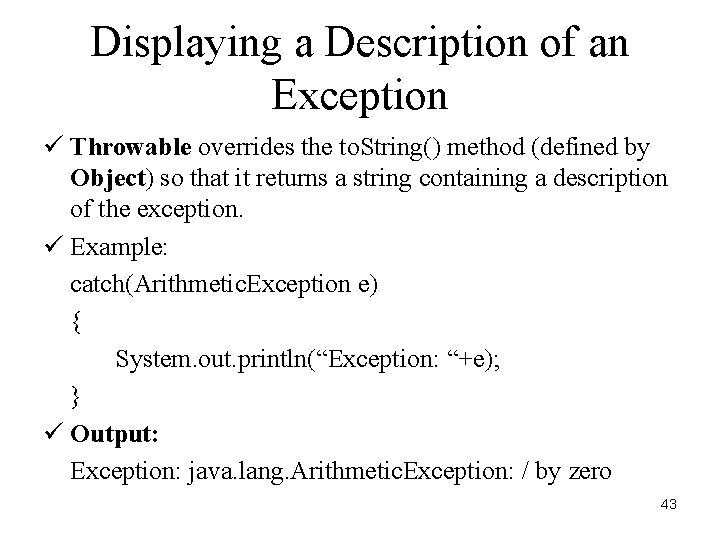
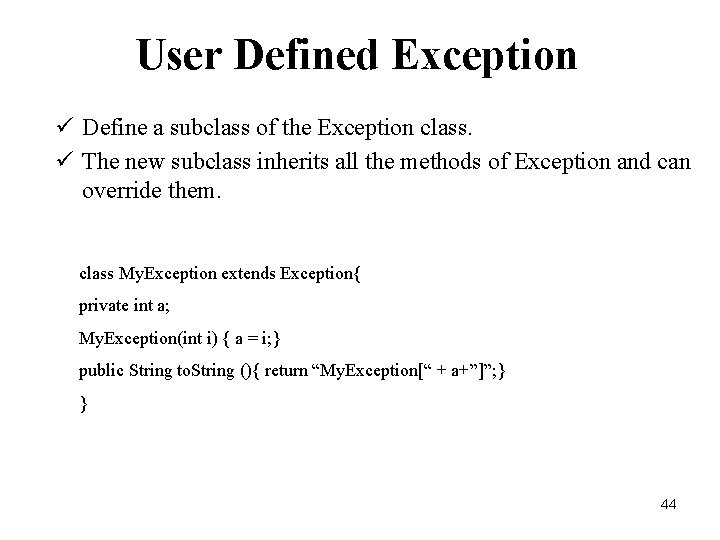
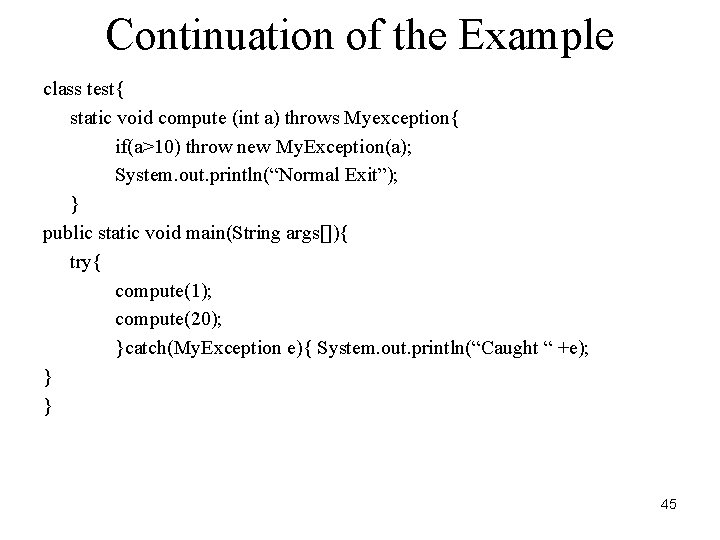
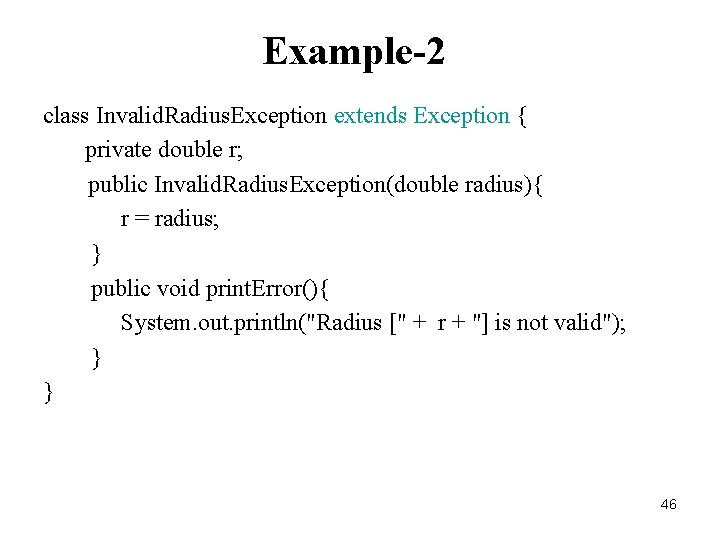
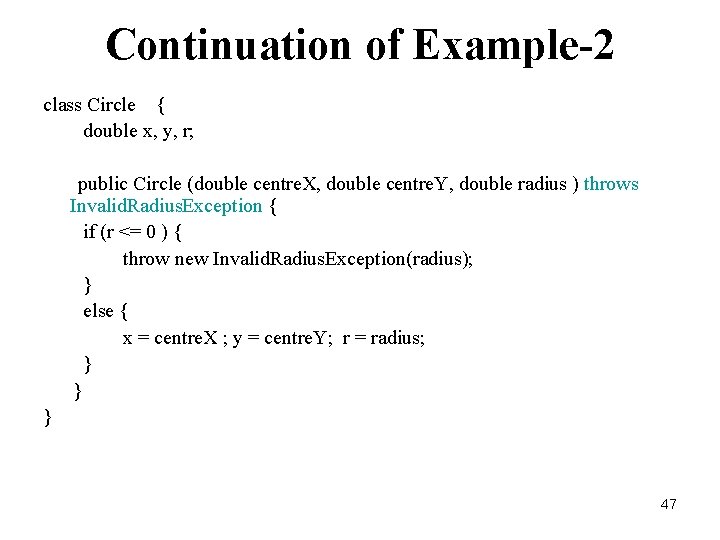
![Continuation of Example-2 class Circle. Test { public static void main(String[] args){ try{ Circle Continuation of Example-2 class Circle. Test { public static void main(String[] args){ try{ Circle](https://slidetodoc.com/presentation_image_h2/57f2df9395718cc70d5871cdfaaee5ac/image-48.jpg)
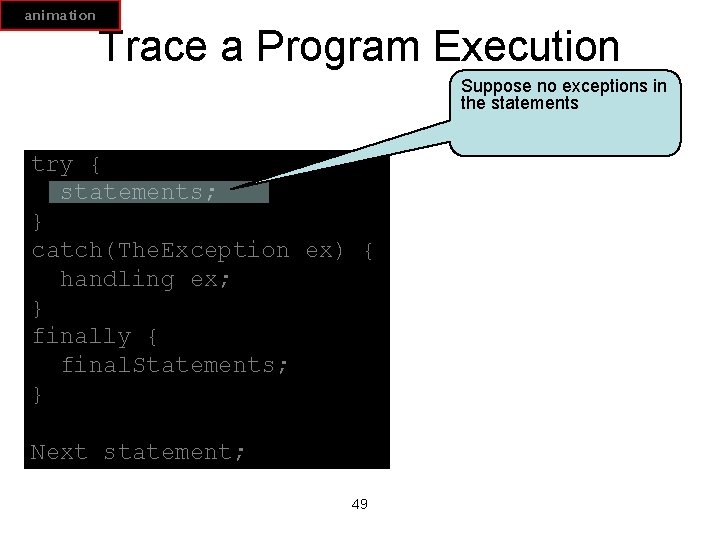
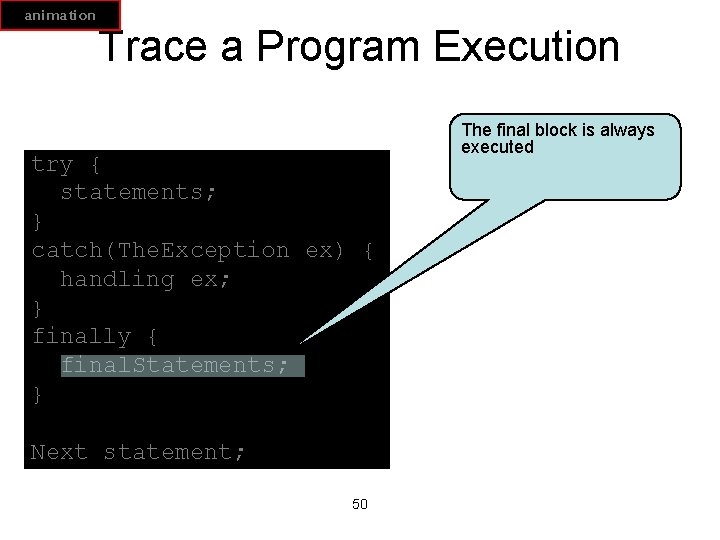
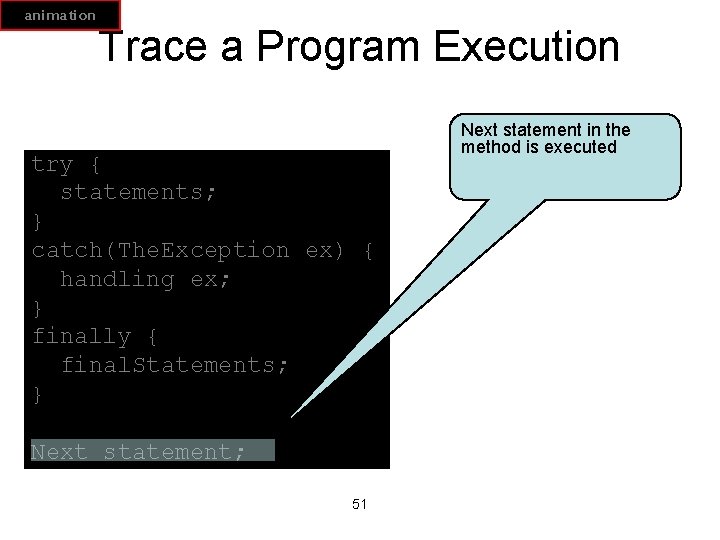
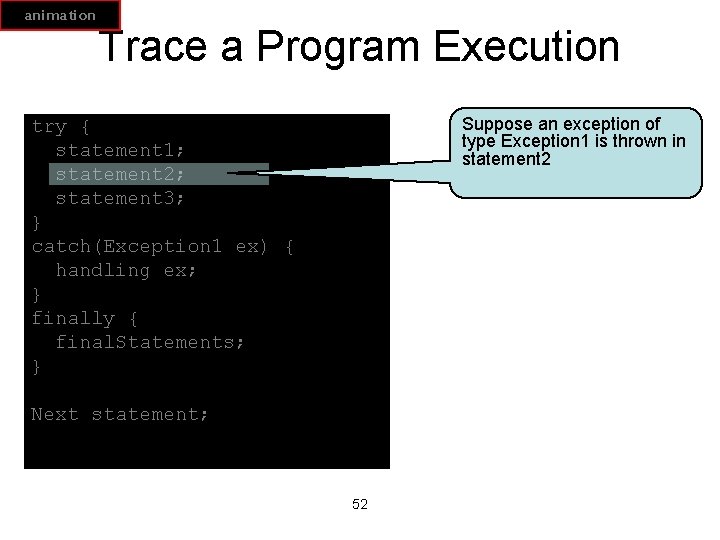
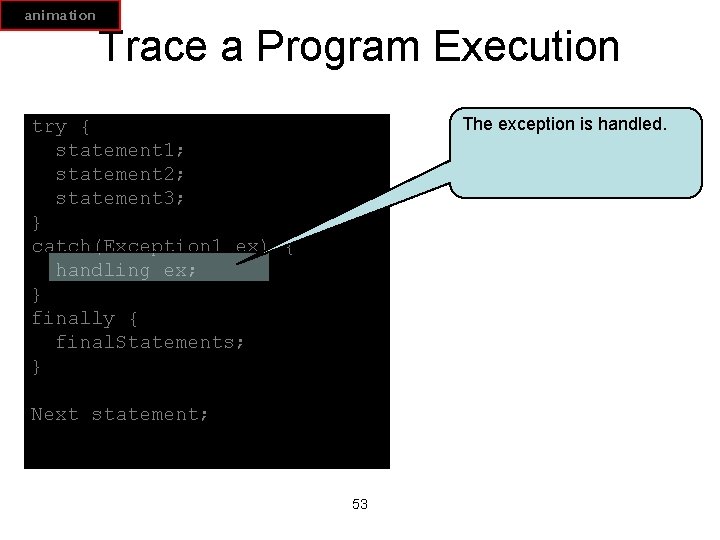
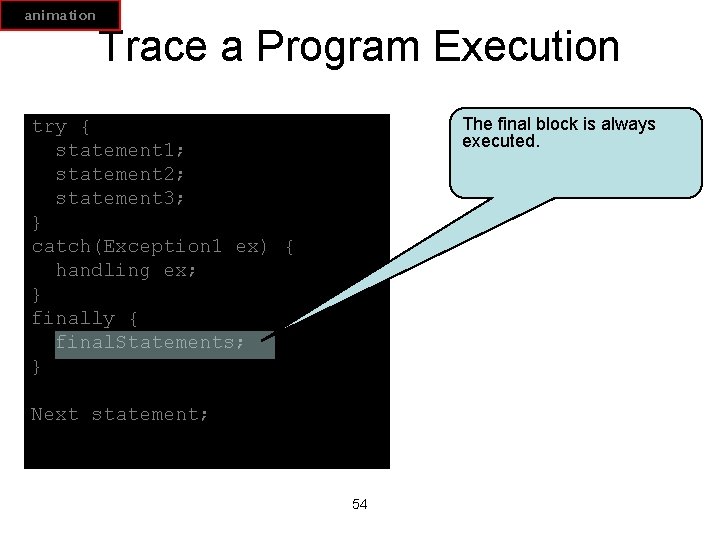
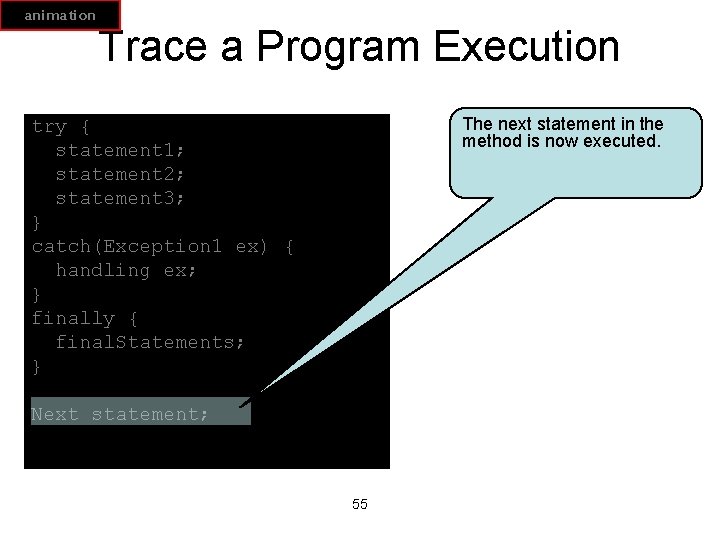
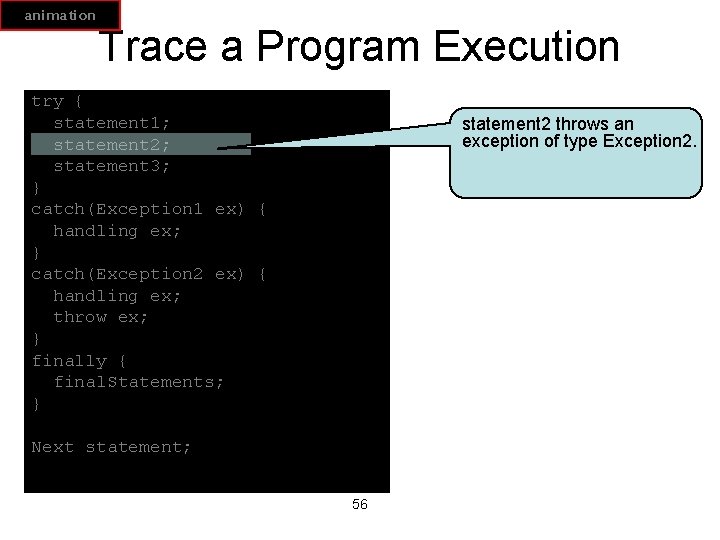
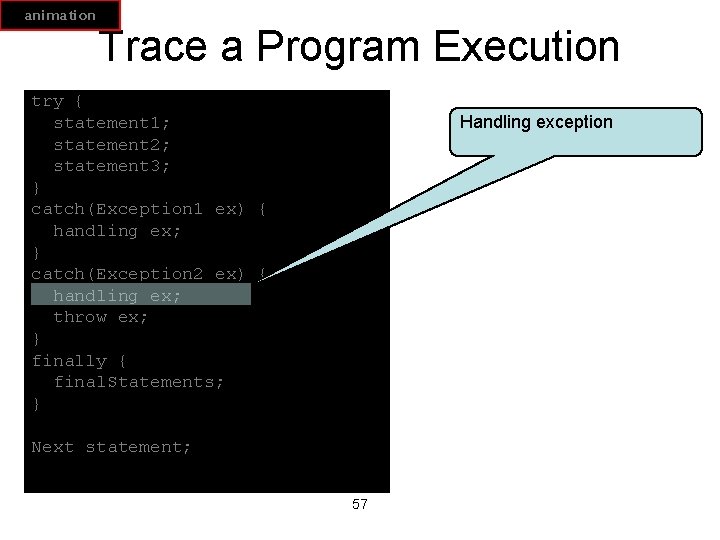
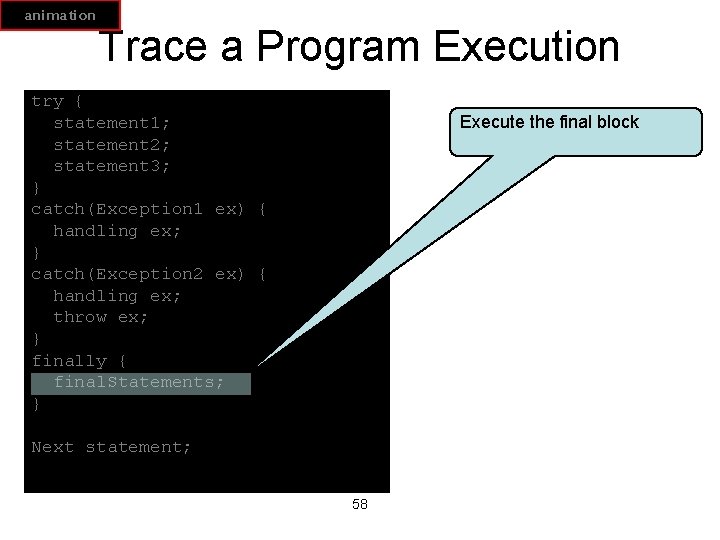
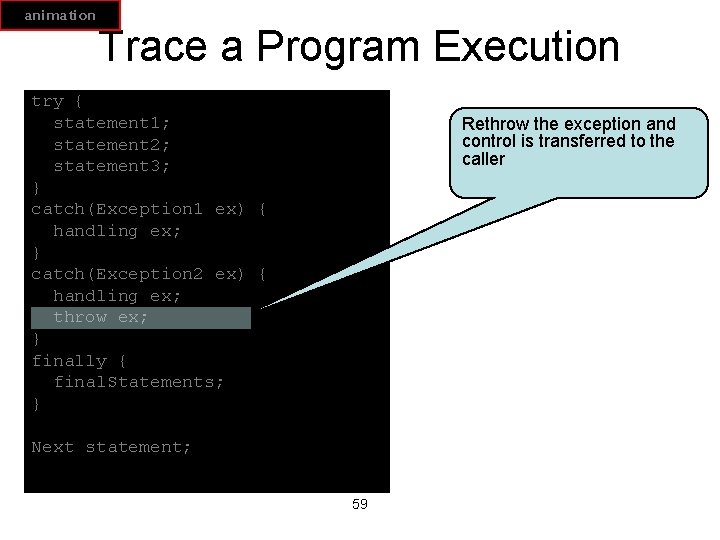
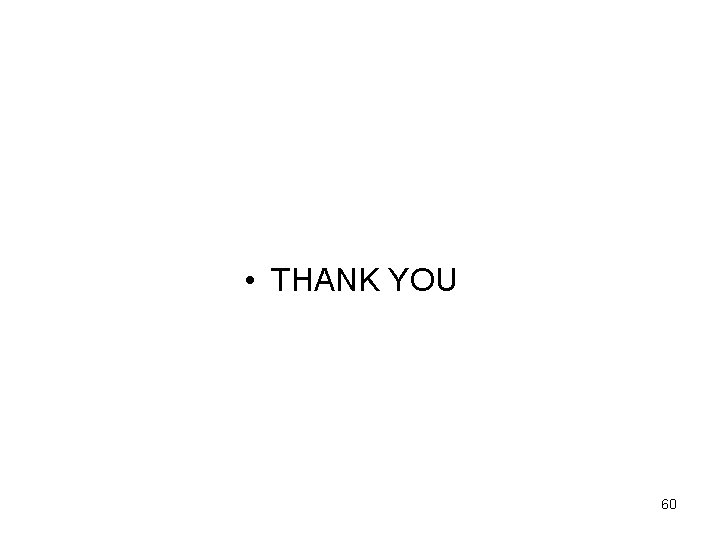
- Slides: 60
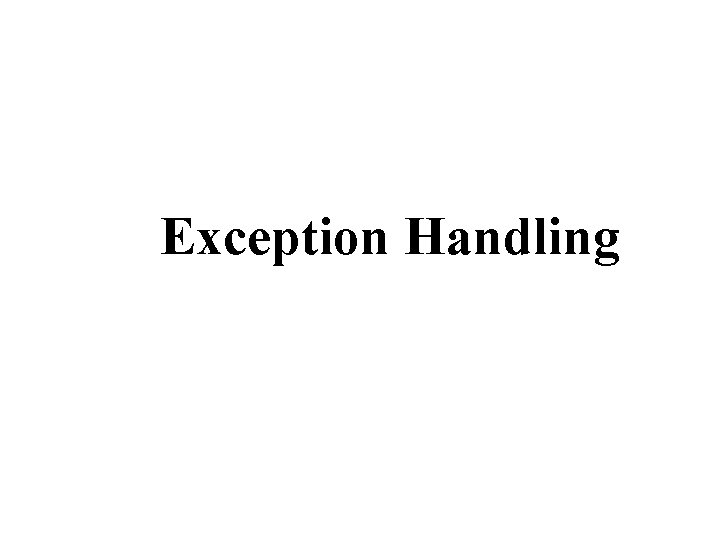
Exception Handling
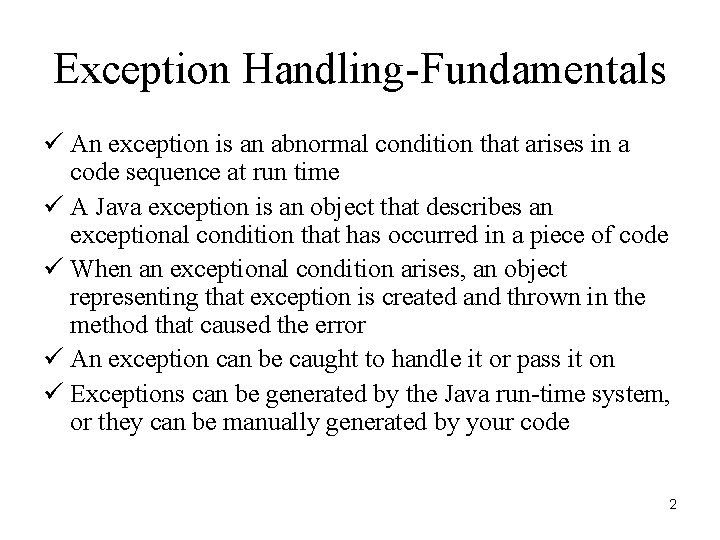
Exception Handling-Fundamentals ü An exception is an abnormal condition that arises in a code sequence at run time ü A Java exception is an object that describes an exceptional condition that has occurred in a piece of code ü When an exceptional condition arises, an object representing that exception is created and thrown in the method that caused the error ü An exception can be caught to handle it or pass it on ü Exceptions can be generated by the Java run-time system, or they can be manually generated by your code 2
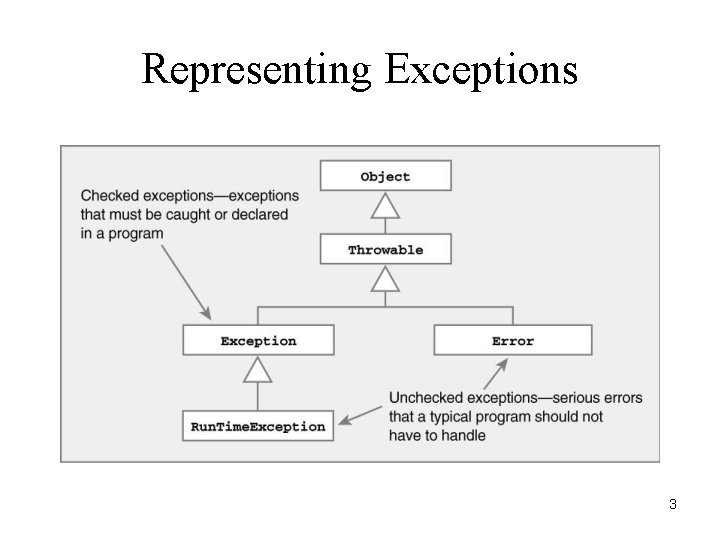
Representing Exceptions 3
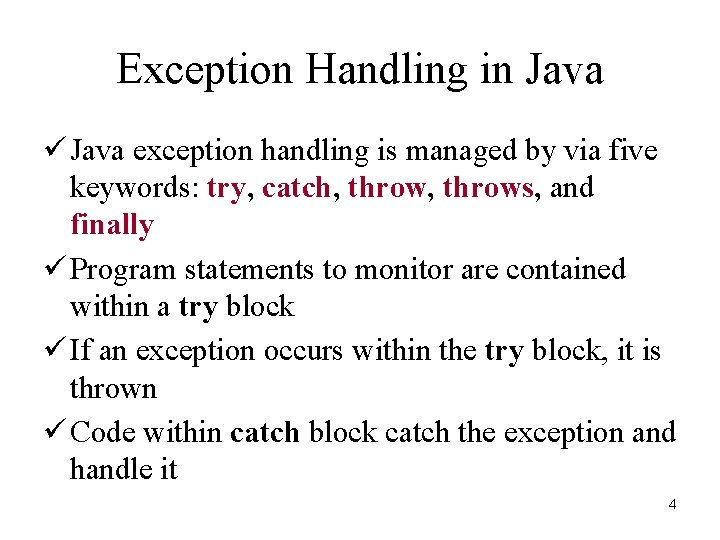
Exception Handling in Java ü Java exception handling is managed by via five keywords: try, catch, throws, and finally ü Program statements to monitor are contained within a try block ü If an exception occurs within the try block, it is thrown ü Code within catch block catch the exception and handle it 4
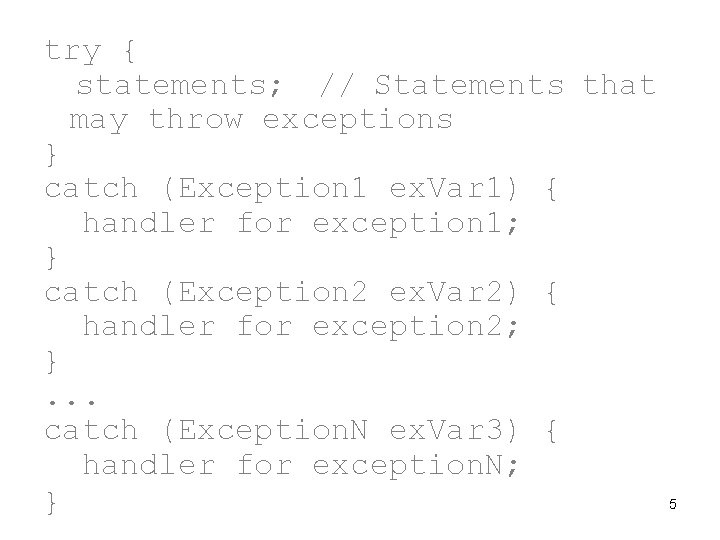
try { statements; // Statements that may throw exceptions } catch (Exception 1 ex. Var 1) { handler for exception 1; } catch (Exception 2 ex. Var 2) { handler for exception 2; }. . . catch (Exception. N ex. Var 3) { handler for exception. N; } 5
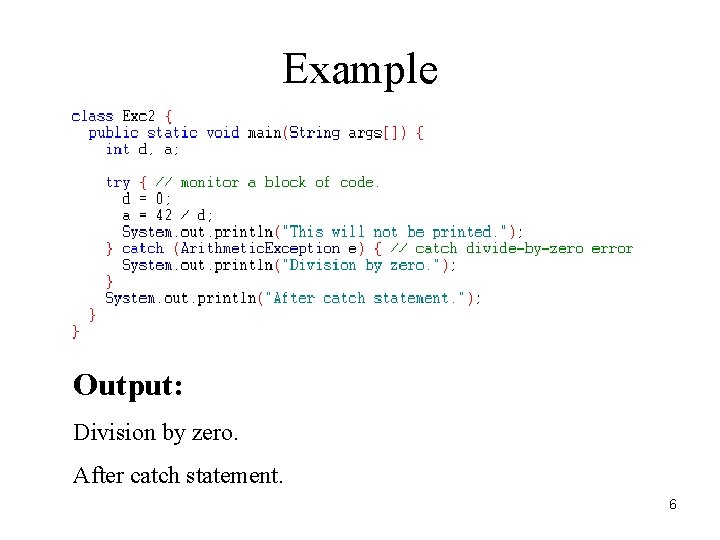
Example Output: Division by zero. After catch statement. 6
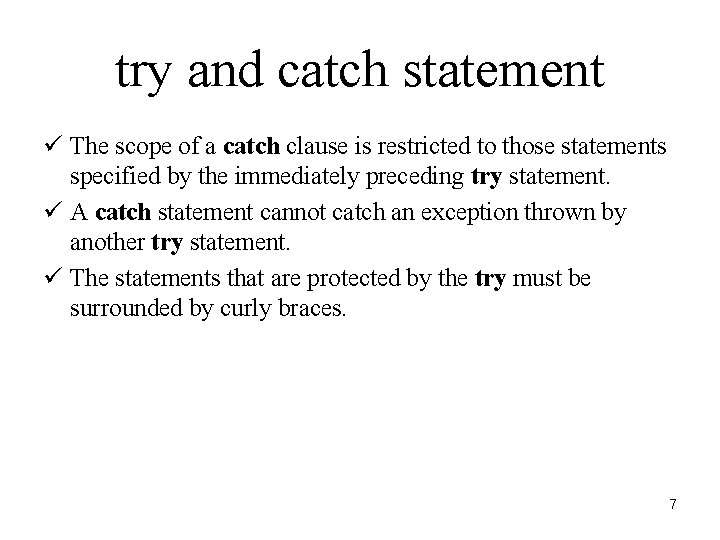
try and catch statement ü The scope of a catch clause is restricted to those statements specified by the immediately preceding try statement. ü A catch statement cannot catch an exception thrown by another try statement. ü The statements that are protected by the try must be surrounded by curly braces. 7
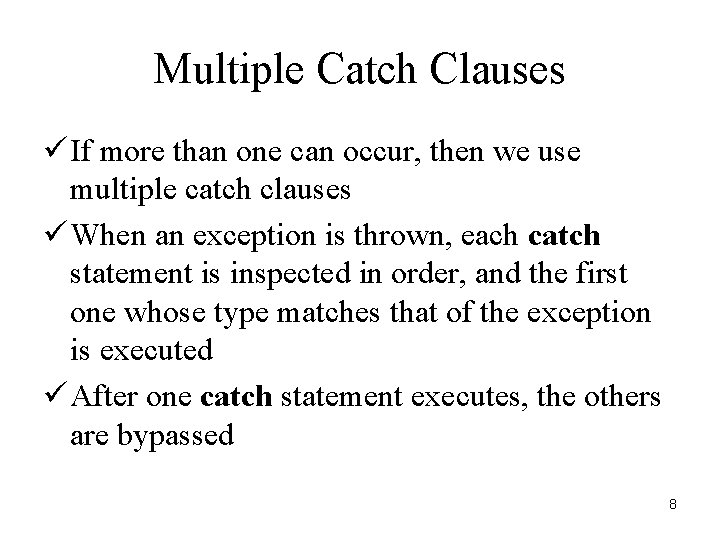
Multiple Catch Clauses ü If more than one can occur, then we use multiple catch clauses ü When an exception is thrown, each catch statement is inspected in order, and the first one whose type matches that of the exception is executed ü After one catch statement executes, the others are bypassed 8
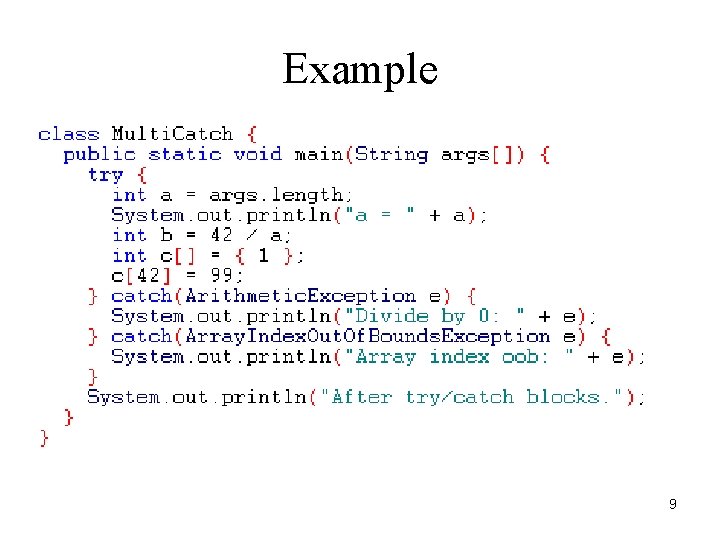
Example 9
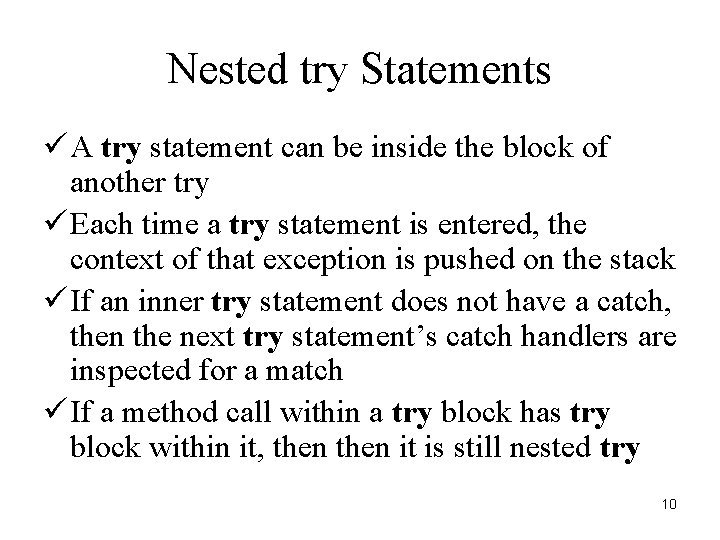
Nested try Statements ü A try statement can be inside the block of another try ü Each time a try statement is entered, the context of that exception is pushed on the stack ü If an inner try statement does not have a catch, then the next try statement’s catch handlers are inspected for a match ü If a method call within a try block has try block within it, then it is still nested try 10
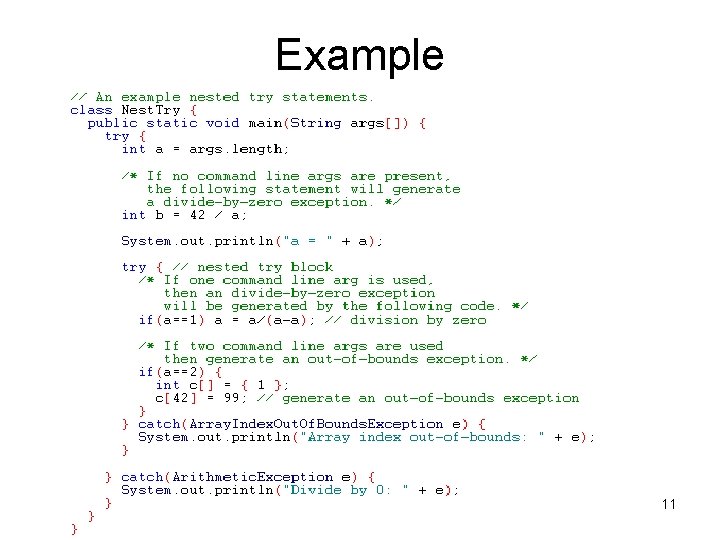
Example 11
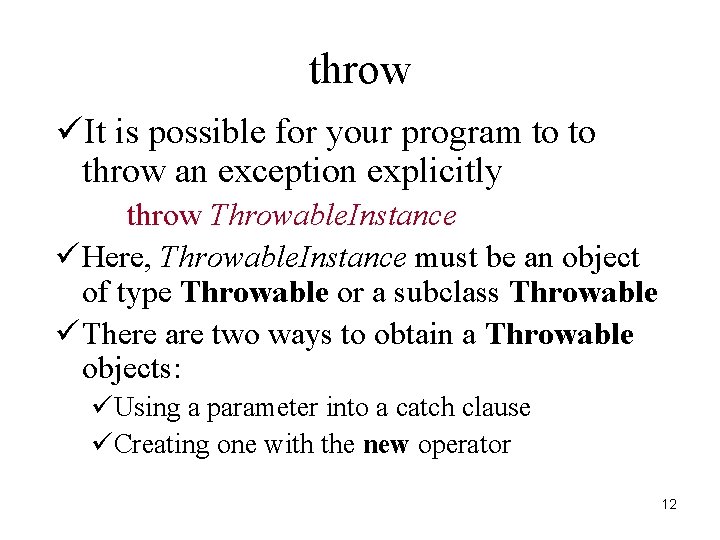
throw üIt is possible for your program to to throw an exception explicitly throw Throwable. Instance ü Here, Throwable. Instance must be an object of type Throwable or a subclass Throwable ü There are two ways to obtain a Throwable objects: üUsing a parameter into a catch clause üCreating one with the new operator 12
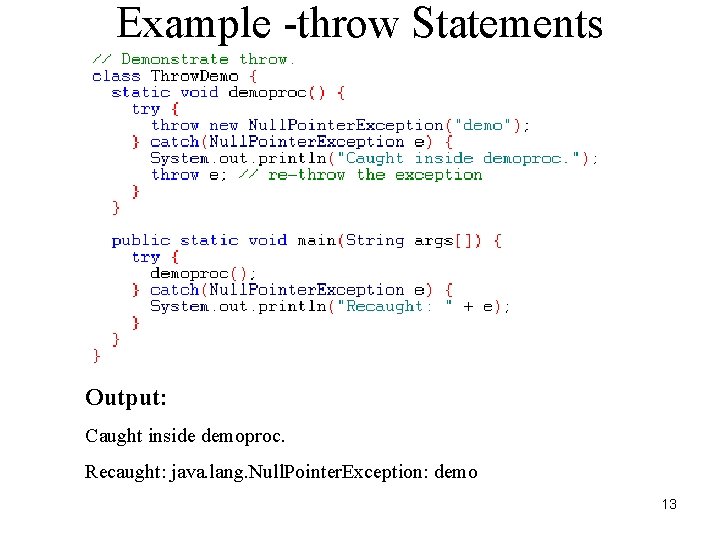
Example -throw Statements Output: Caught inside demoproc. Recaught: java. lang. Null. Pointer. Exception: demo 13
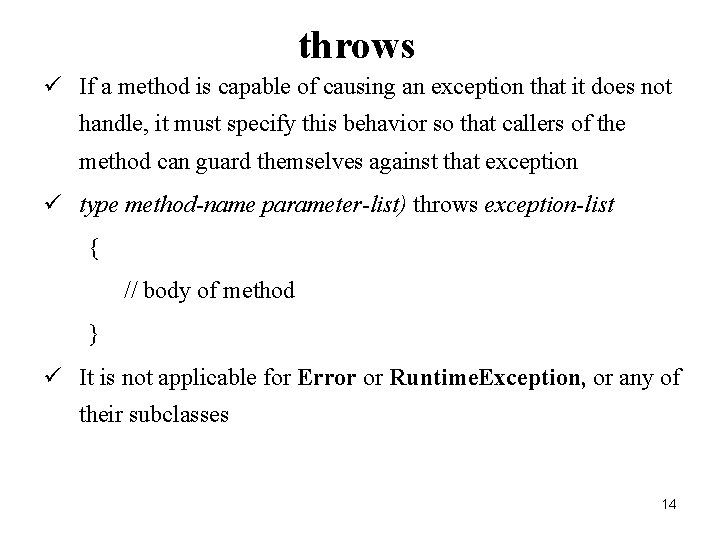
throws ü If a method is capable of causing an exception that it does not handle, it must specify this behavior so that callers of the method can guard themselves against that exception ü type method-name parameter-list) throws exception-list { // body of method } ü It is not applicable for Error or Runtime. Exception, or any of their subclasses 14
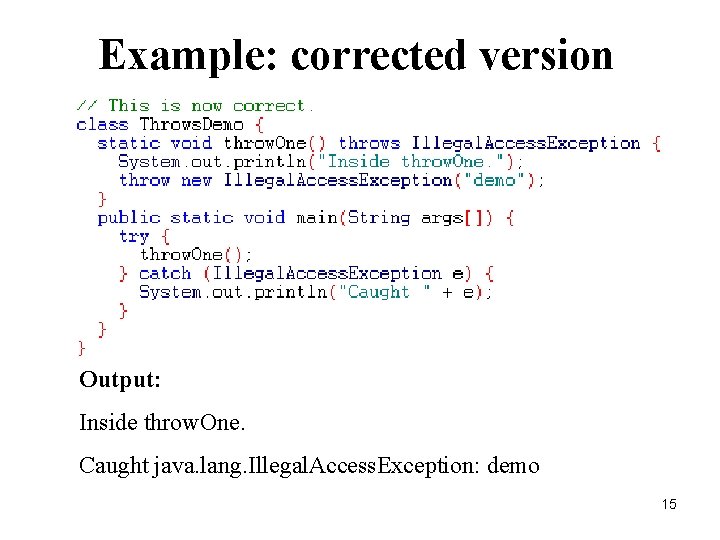
Example: corrected version Output: Inside throw. One. Caught java. lang. Illegal. Access. Exception: demo 15
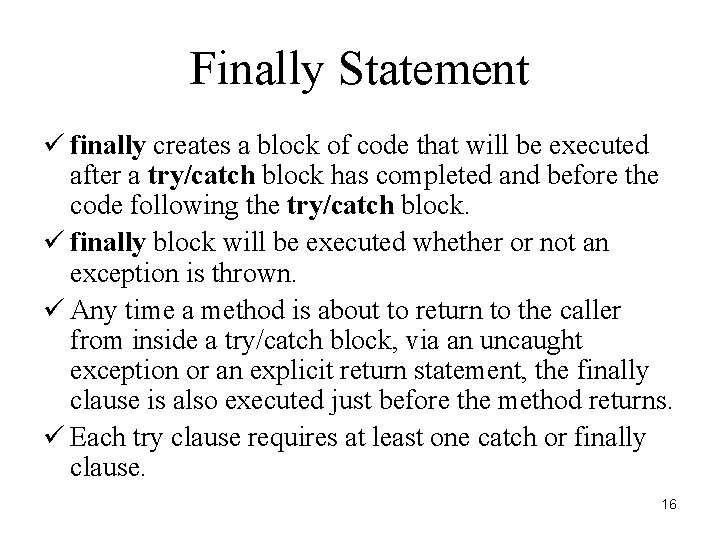
Finally Statement ü finally creates a block of code that will be executed after a try/catch block has completed and before the code following the try/catch block. ü finally block will be executed whether or not an exception is thrown. ü Any time a method is about to return to the caller from inside a try/catch block, via an uncaught exception or an explicit return statement, the finally clause is also executed just before the method returns. ü Each try clause requires at least one catch or finally clause. 16
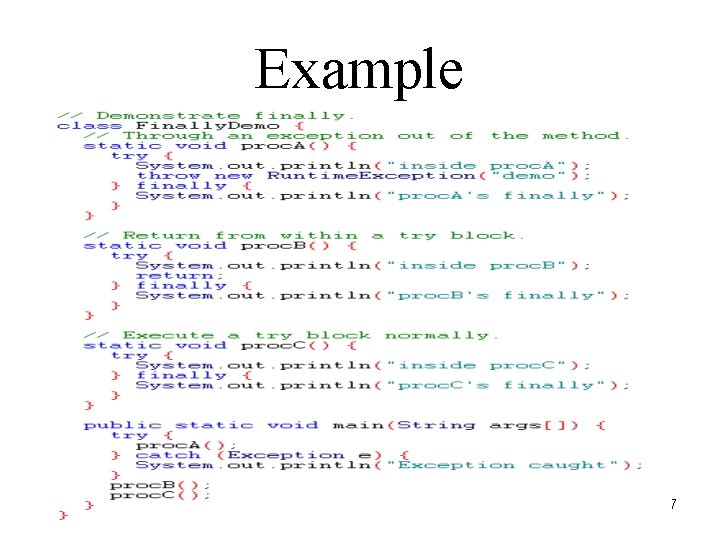
Example 17
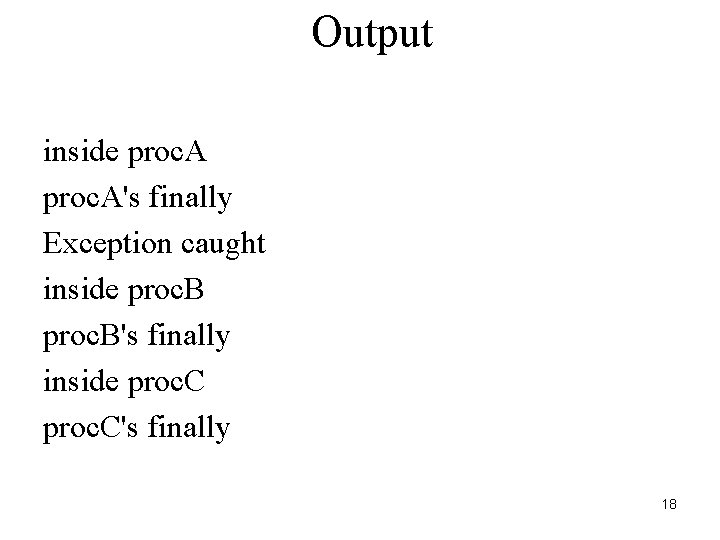
Output inside proc. A's finally Exception caught inside proc. B's finally inside proc. C's finally 18
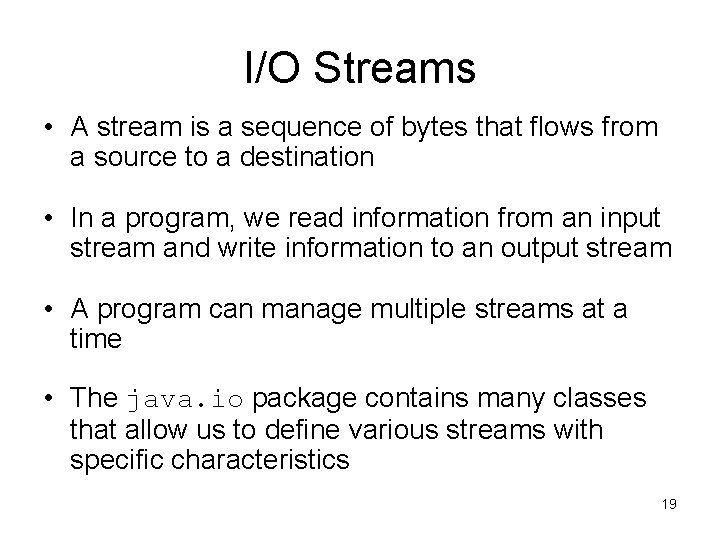
I/O Streams • A stream is a sequence of bytes that flows from a source to a destination • In a program, we read information from an input stream and write information to an output stream • A program can manage multiple streams at a time • The java. io package contains many classes that allow us to define various streams with specific characteristics 19
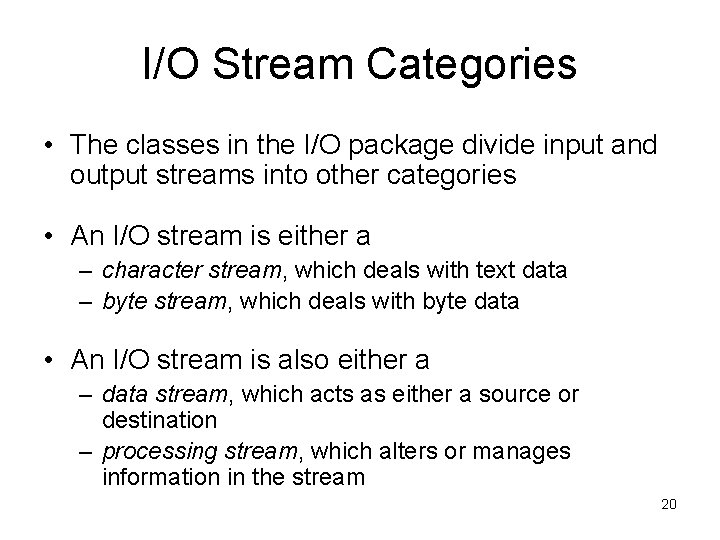
I/O Stream Categories • The classes in the I/O package divide input and output streams into other categories • An I/O stream is either a – character stream, which deals with text data – byte stream, which deals with byte data • An I/O stream is also either a – data stream, which acts as either a source or destination – processing stream, which alters or manages information in the stream 20
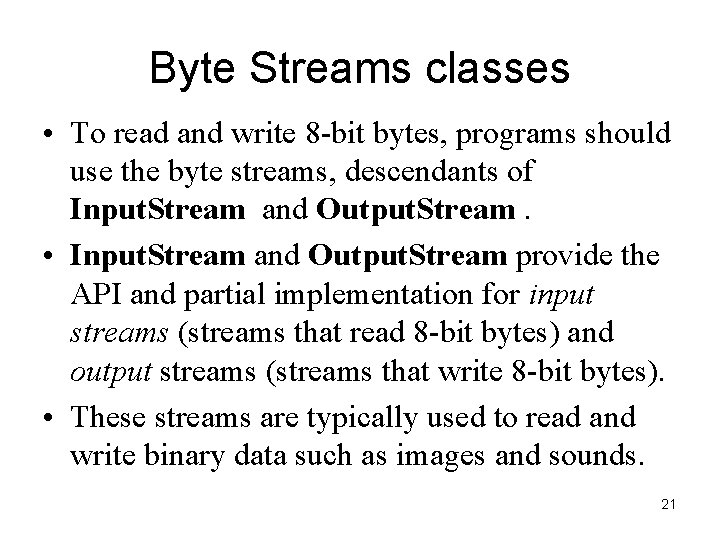
Byte Streams classes • To read and write 8 -bit bytes, programs should use the byte streams, descendants of Input. Stream and Output. Stream. • Input. Stream and Output. Stream provide the API and partial implementation for input streams (streams that read 8 -bit bytes) and output streams (streams that write 8 -bit bytes). • These streams are typically used to read and write binary data such as images and sounds. 21
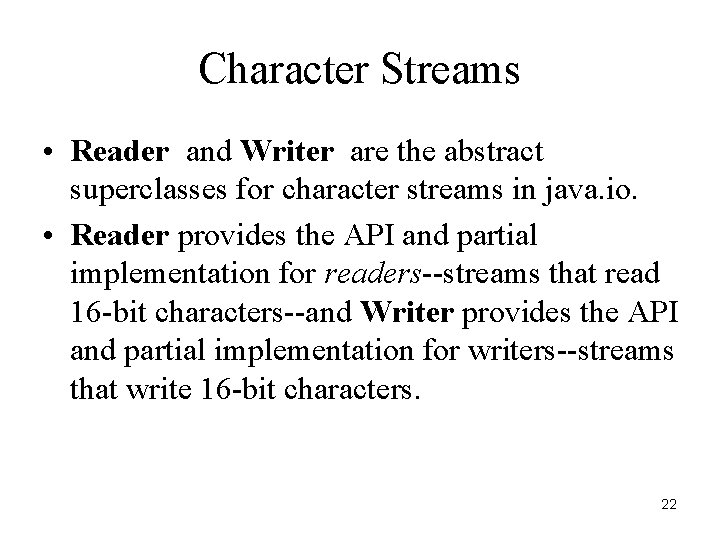
Character Streams • Reader and Writer are the abstract superclasses for character streams in java. io. • Reader provides the API and partial implementation for readers--streams that read 16 -bit characters--and Writer provides the API and partial implementation for writers--streams that write 16 -bit characters. 22
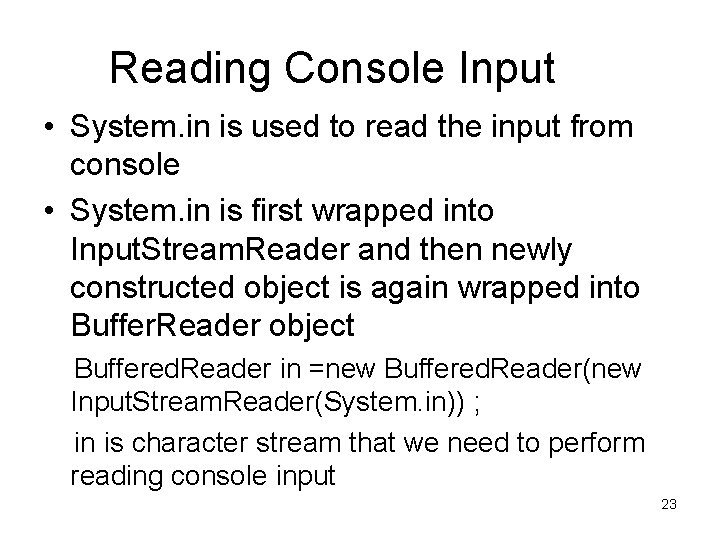
Reading Console Input • System. in is used to read the input from console • System. in is first wrapped into Input. Stream. Reader and then newly constructed object is again wrapped into Buffer. Reader object Buffered. Reader in =new Buffered. Reader(new Input. Stream. Reader(System. in)) ; in is character stream that we need to perform reading console input 23
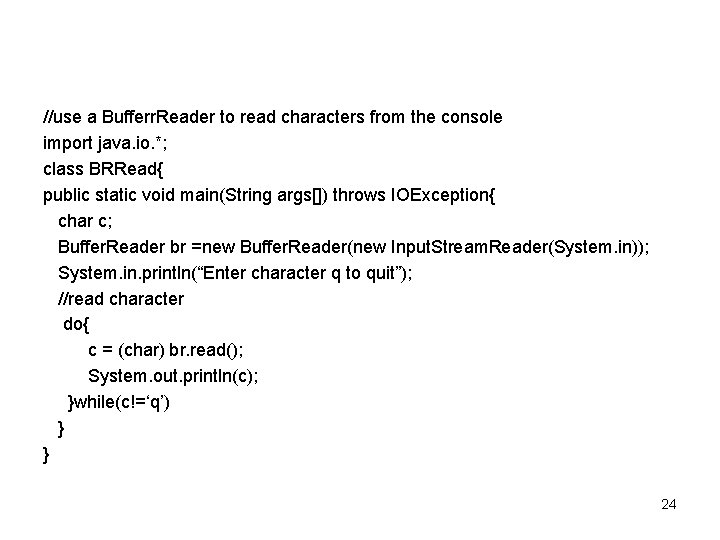
//use a Bufferr. Reader to read characters from the console import java. io. *; class BRRead{ public static void main(String args[]) throws IOException{ char c; Buffer. Reader br =new Buffer. Reader(new Input. Stream. Reader(System. in)); System. in. println(“Enter character q to quit”); //read character do{ c = (char) br. read(); System. out. println(c); }while(c!=‘q’) } } 24
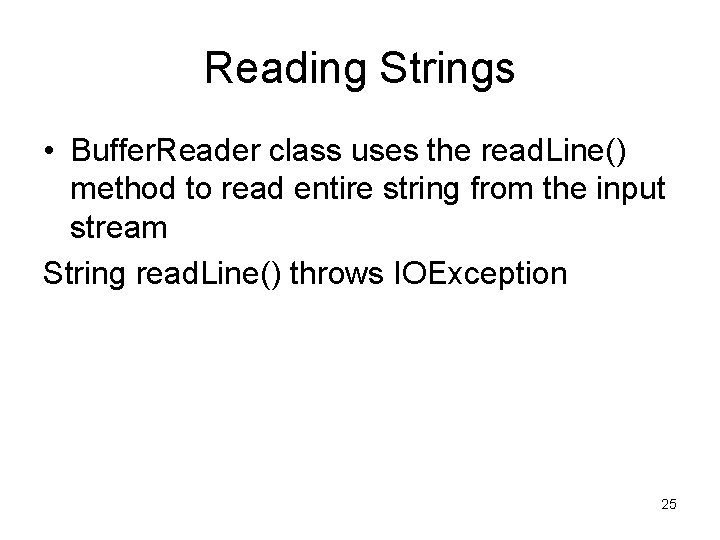
Reading Strings • Buffer. Reader class uses the read. Line() method to read entire string from the input stream String read. Line() throws IOException 25
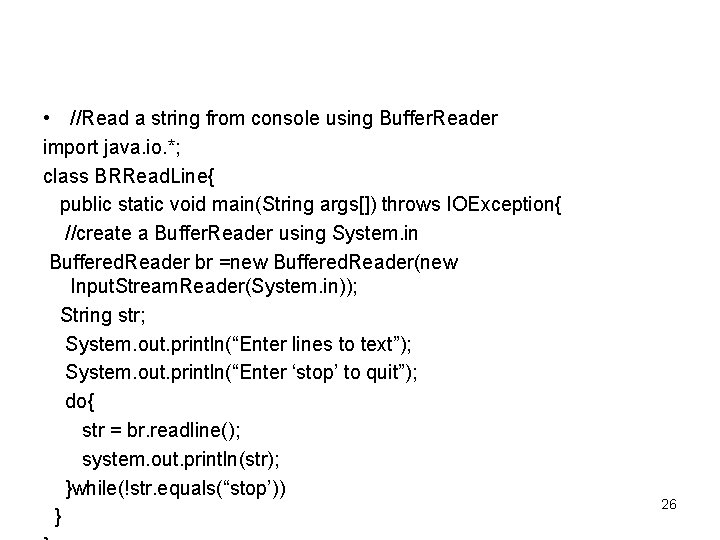
• //Read a string from console using Buffer. Reader import java. io. *; class BRRead. Line{ public static void main(String args[]) throws IOException{ //create a Buffer. Reader using System. in Buffered. Reader br =new Buffered. Reader(new Input. Stream. Reader(System. in)); String str; System. out. println(“Enter lines to text”); System. out. println(“Enter ‘stop’ to quit”); do{ str = br. readline(); system. out. println(str); }while(!str. equals(“stop’)) } 26
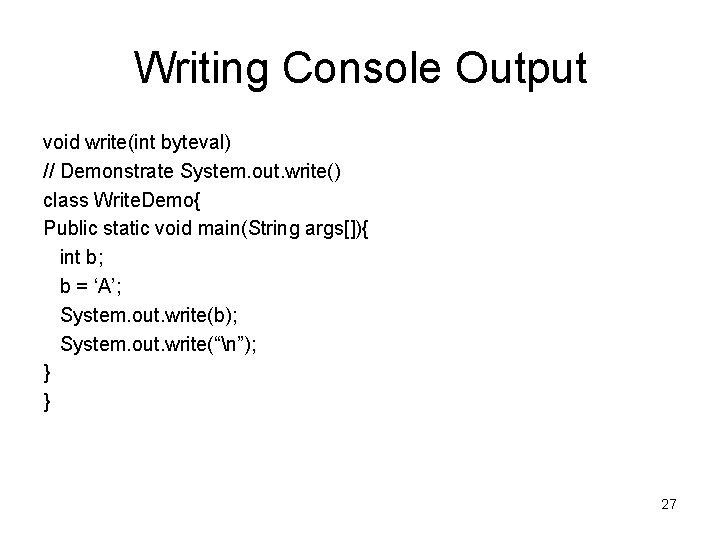
Writing Console Output void write(int byteval) // Demonstrate System. out. write() class Write. Demo{ Public static void main(String args[]){ int b; b = ‘A’; System. out. write(b); System. out. write(“n”); } } 27
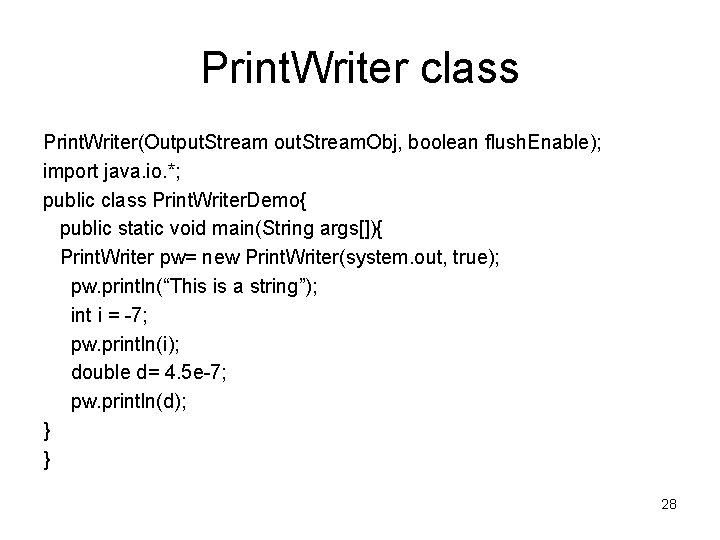
Print. Writer class Print. Writer(Output. Stream out. Stream. Obj, boolean flush. Enable); import java. io. *; public class Print. Writer. Demo{ public static void main(String args[]){ Print. Writer pw= new Print. Writer(system. out, true); pw. println(“This is a string”); int i = -7; pw. println(i); double d= 4. 5 e-7; pw. println(d); } } 28
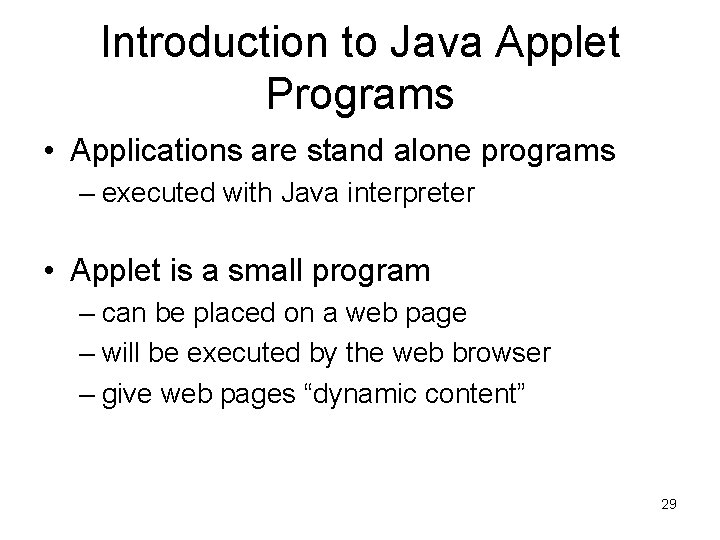
Introduction to Java Applet Programs • Applications are stand alone programs – executed with Java interpreter • Applet is a small program – can be placed on a web page – will be executed by the web browser – give web pages “dynamic content” 29
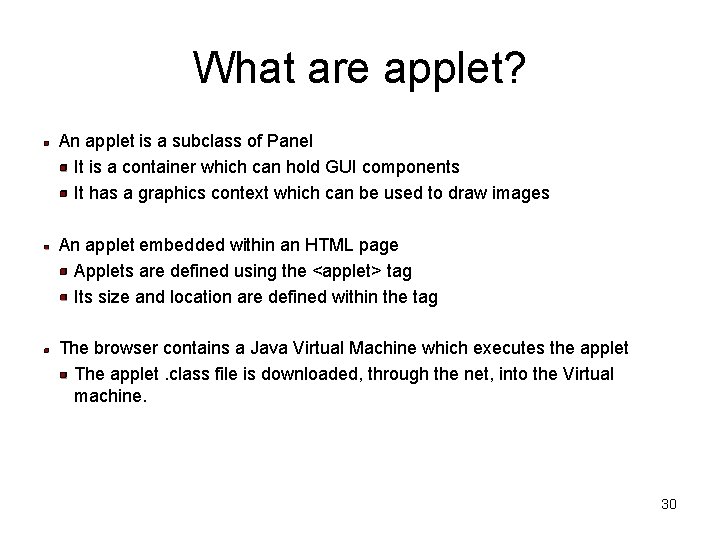
What are applet? An applet is a subclass of Panel It is a container which can hold GUI components It has a graphics context which can be used to draw images An applet embedded within an HTML page Applets are defined using the <applet> tag Its size and location are defined within the tag The browser contains a Java Virtual Machine which executes the applet The applet. class file is downloaded, through the net, into the Virtual machine. 30
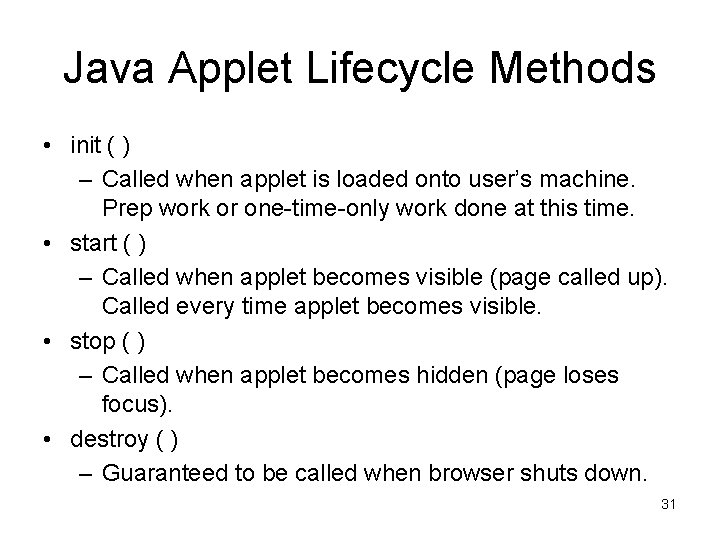
Java Applet Lifecycle Methods • init ( ) – Called when applet is loaded onto user’s machine. Prep work or one-time-only work done at this time. • start ( ) – Called when applet becomes visible (page called up). Called every time applet becomes visible. • stop ( ) – Called when applet becomes hidden (page loses focus). • destroy ( ) – Guaranteed to be called when browser shuts down. 31
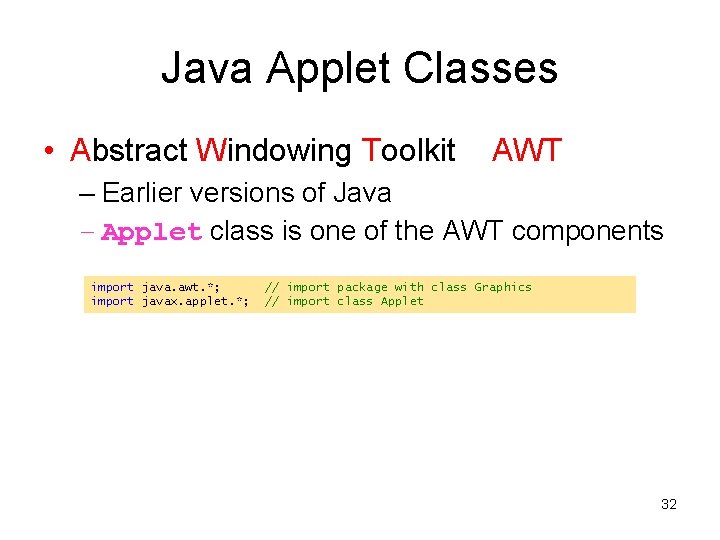
Java Applet Classes • Abstract Windowing Toolkit AWT – Earlier versions of Java – Applet class is one of the AWT components import java. awt. *; import javax. applet. *; // import package with class Graphics // import class Applet 32
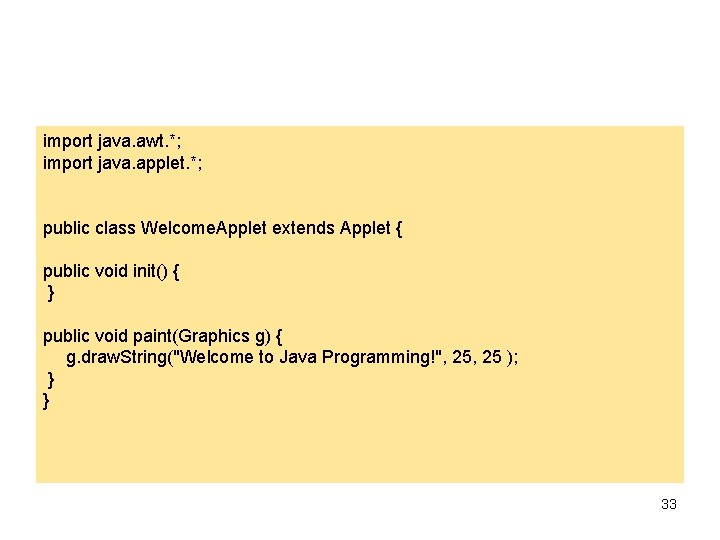
import java. awt. *; import java. applet. *; public class Welcome. Applet extends Applet { public void init() { } public void paint(Graphics g) { g. draw. String("Welcome to Java Programming!", 25 ); } } 33
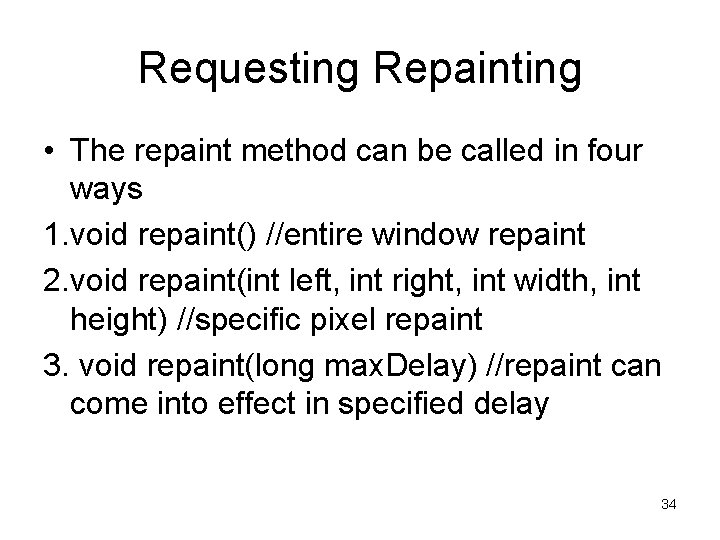
Requesting Repainting • The repaint method can be called in four ways 1. void repaint() //entire window repaint 2. void repaint(int left, int right, int width, int height) //specific pixel repaint 3. void repaint(long max. Delay) //repaint can come into effect in specified delay 34
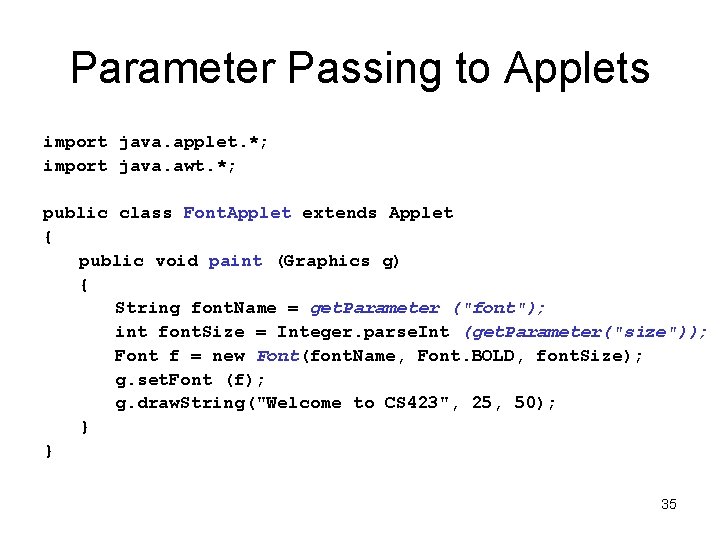
Parameter Passing to Applets import java. applet. *; import java. awt. *; public class Font. Applet extends Applet { public void paint (Graphics g) { String font. Name = get. Parameter ("font"); int font. Size = Integer. parse. Int (get. Parameter("size")); Font f = new Font(font. Name, Font. BOLD, font. Size); g. set. Font (f); g. draw. String("Welcome to CS 423", 25, 50); } } 35
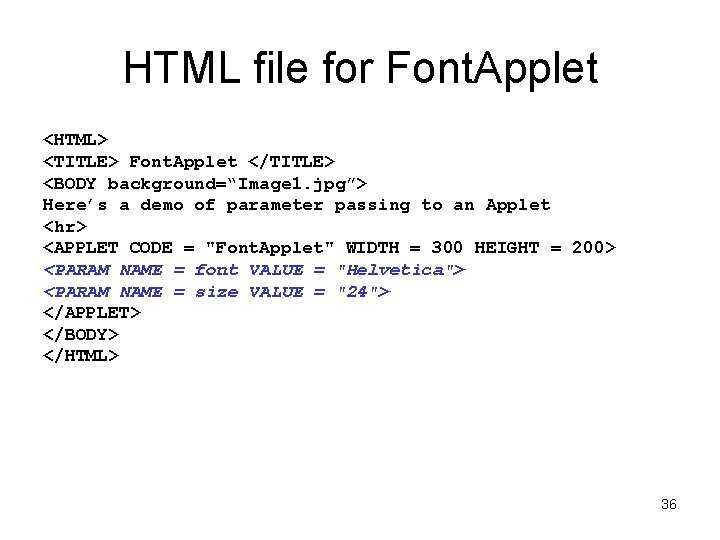
HTML file for Font. Applet <HTML> <TITLE> Font. Applet </TITLE> <BODY background=“Image 1. jpg”> Here’s a demo of parameter passing to an Applet <hr> <APPLET CODE = "Font. Applet" WIDTH = 300 HEIGHT = 200> <PARAM NAME = font VALUE = "Helvetica"> <PARAM NAME = size VALUE = "24"> </APPLET> </BODY> </HTML> 36
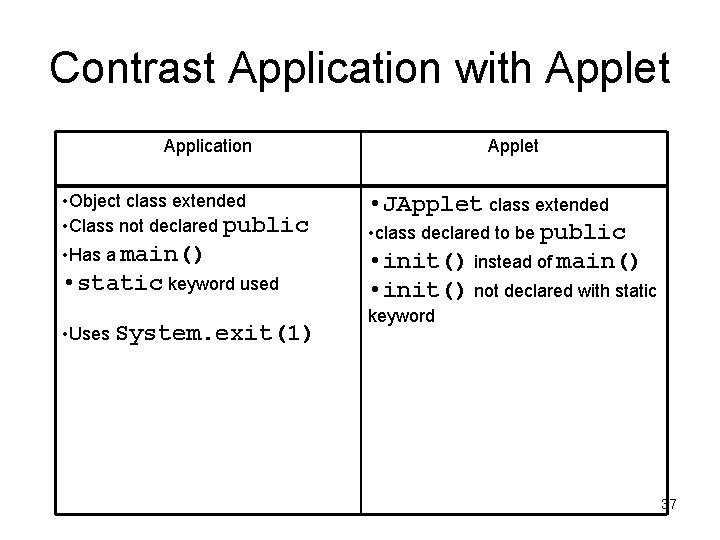
Contrast Application with Applet Application • Object class extended • Class not declared public • Has a main() • static keyword used • Uses System. exit(1) Applet • JApplet class extended • class declared to be public • init() instead of main() • init() not declared with static keyword 37
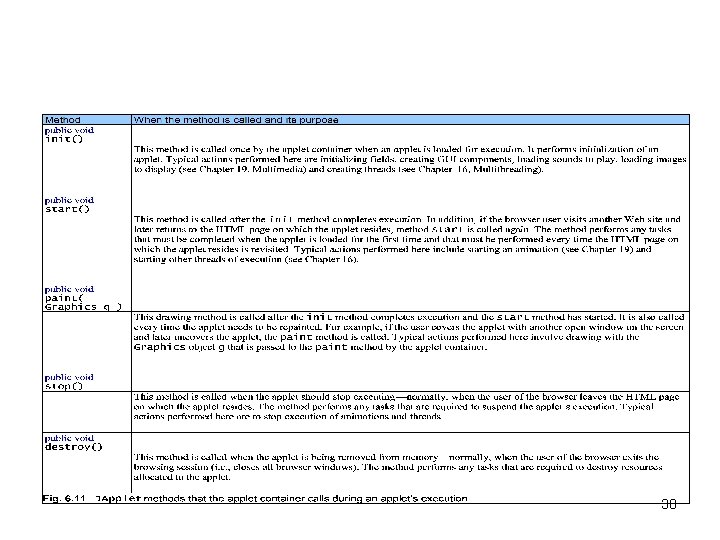
38
![Uncaught Exceptions class exc 0 public static void mainString args int d0 int Uncaught Exceptions class exc 0{ public static void main(String args[]) { int d=0; int](https://slidetodoc.com/presentation_image_h2/57f2df9395718cc70d5871cdfaaee5ac/image-39.jpg)
Uncaught Exceptions class exc 0{ public static void main(String args[]) { int d=0; int a=42/d; } } Output: java. lang. Arithmetic. Exception: / by zero ü A new exception object is constructed and then thrown. ü This exception is caught by the default handler provided by the java runtime system. ü The default handler displays a string describing the exception, prints the stack trace from the point at which the exception occurred and terminates the program. at exc 0. main(exc 0. java: 4) 39
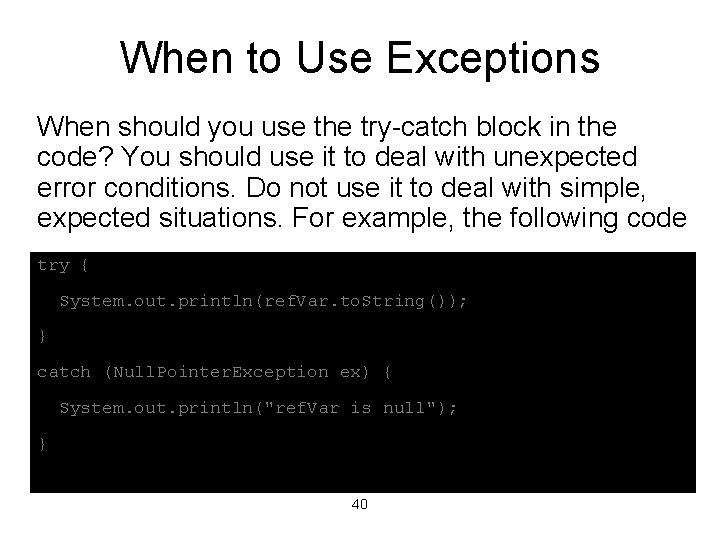
When to Use Exceptions When should you use the try-catch block in the code? You should use it to deal with unexpected error conditions. Do not use it to deal with simple, expected situations. For example, the following code try { System. out. println(ref. Var. to. String()); } catch (Null. Pointer. Exception ex) { System. out. println("ref. Var is null"); } 40
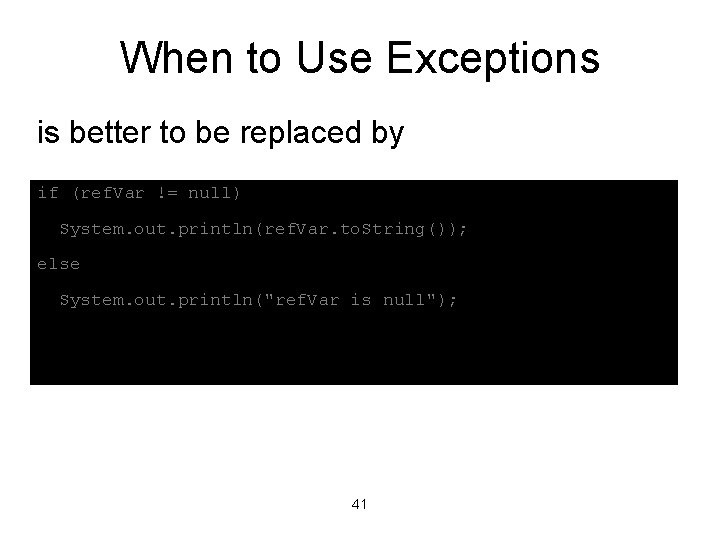
When to Use Exceptions is better to be replaced by if (ref. Var != null) System. out. println(ref. Var. to. String()); else System. out. println("ref. Var is null"); 41
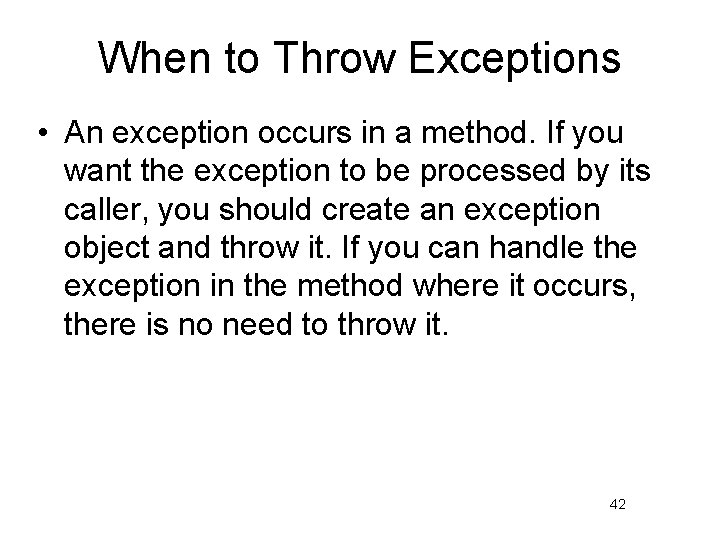
When to Throw Exceptions • An exception occurs in a method. If you want the exception to be processed by its caller, you should create an exception object and throw it. If you can handle the exception in the method where it occurs, there is no need to throw it. 42
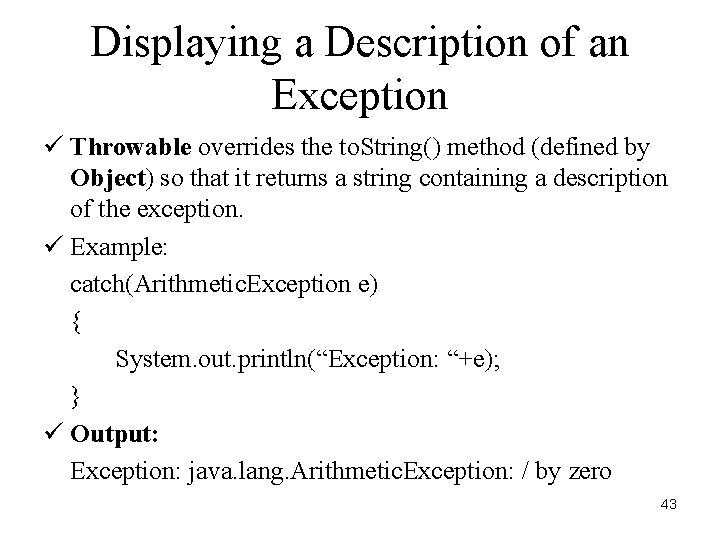
Displaying a Description of an Exception ü Throwable overrides the to. String() method (defined by Object) so that it returns a string containing a description of the exception. ü Example: catch(Arithmetic. Exception e) { System. out. println(“Exception: “+e); } ü Output: Exception: java. lang. Arithmetic. Exception: / by zero 43
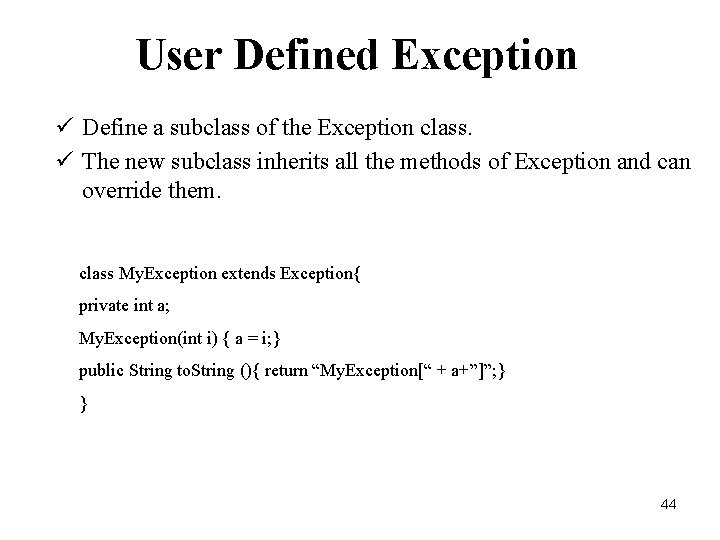
User Defined Exception ü Define a subclass of the Exception class. ü The new subclass inherits all the methods of Exception and can override them. class My. Exception extends Exception{ private int a; My. Exception(int i) { a = i; } public String to. String (){ return “My. Exception[“ + a+”]”; } } 44
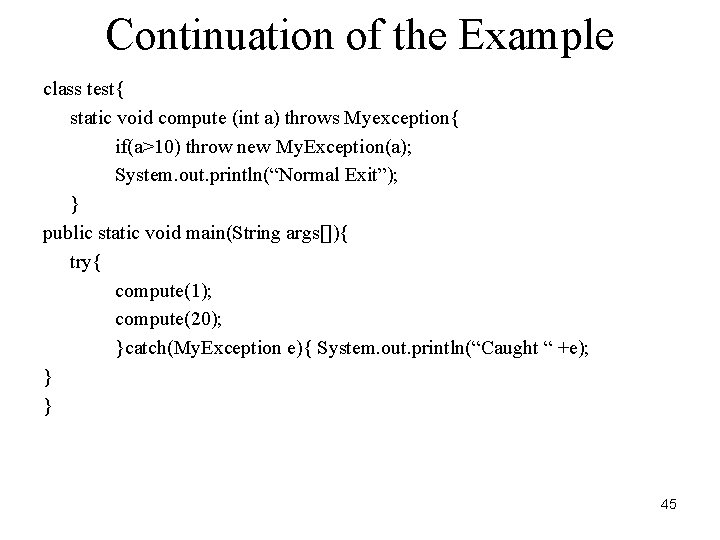
Continuation of the Example class test{ static void compute (int a) throws Myexception{ if(a>10) throw new My. Exception(a); System. out. println(“Normal Exit”); } public static void main(String args[]){ try{ compute(1); compute(20); }catch(My. Exception e){ System. out. println(“Caught “ +e); } } 45
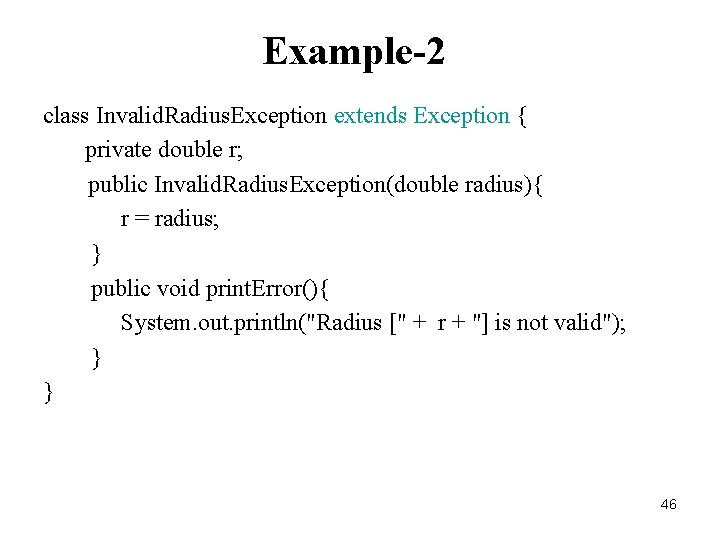
Example-2 class Invalid. Radius. Exception extends Exception { private double r; public Invalid. Radius. Exception(double radius){ r = radius; } public void print. Error(){ System. out. println("Radius [" + r + "] is not valid"); } } 46
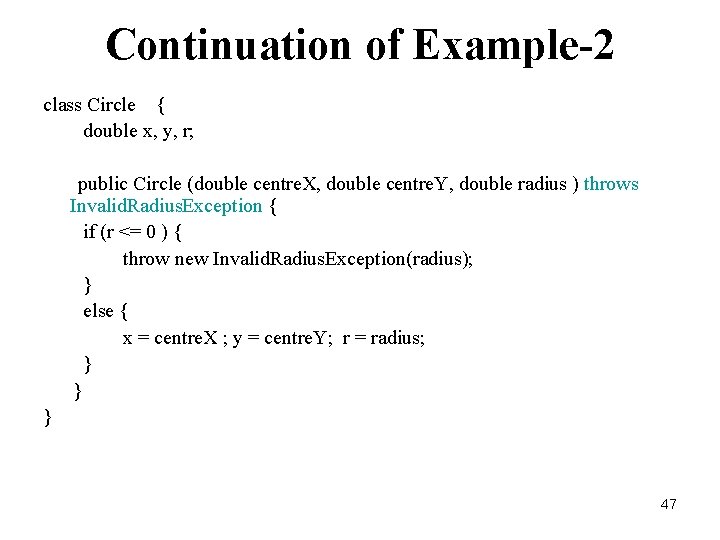
Continuation of Example-2 class Circle { double x, y, r; public Circle (double centre. X, double centre. Y, double radius ) throws Invalid. Radius. Exception { if (r <= 0 ) { throw new Invalid. Radius. Exception(radius); } else { x = centre. X ; y = centre. Y; r = radius; } } } 47
![Continuation of Example2 class Circle Test public static void mainString args try Circle Continuation of Example-2 class Circle. Test { public static void main(String[] args){ try{ Circle](https://slidetodoc.com/presentation_image_h2/57f2df9395718cc70d5871cdfaaee5ac/image-48.jpg)
Continuation of Example-2 class Circle. Test { public static void main(String[] args){ try{ Circle c 1 = new Circle(10, -1); System. out. println("Circle created"); } catch(Invalid. Radius. Exception e) { e. print. Error(); } } } 48
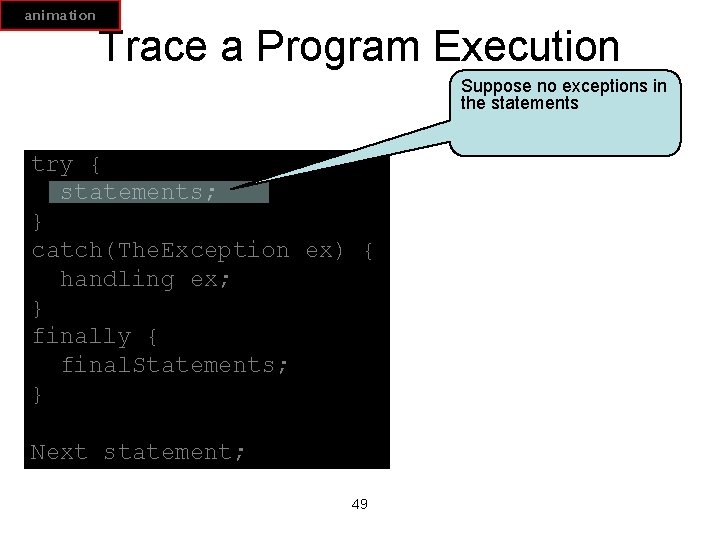
animation Trace a Program Execution Suppose no exceptions in the statements try { statements; } catch(The. Exception ex) { handling ex; } finally { final. Statements; } Next statement; 49
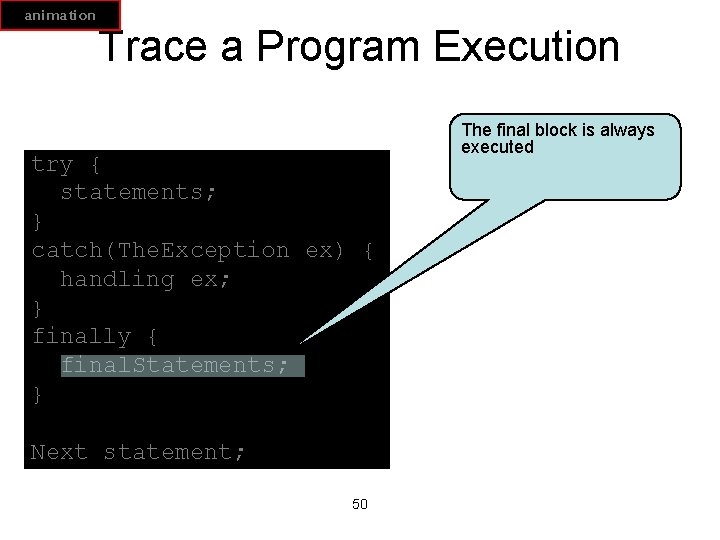
animation Trace a Program Execution try { statements; } catch(The. Exception ex) { handling ex; } finally { final. Statements; } Next statement; 50 The final block is always executed
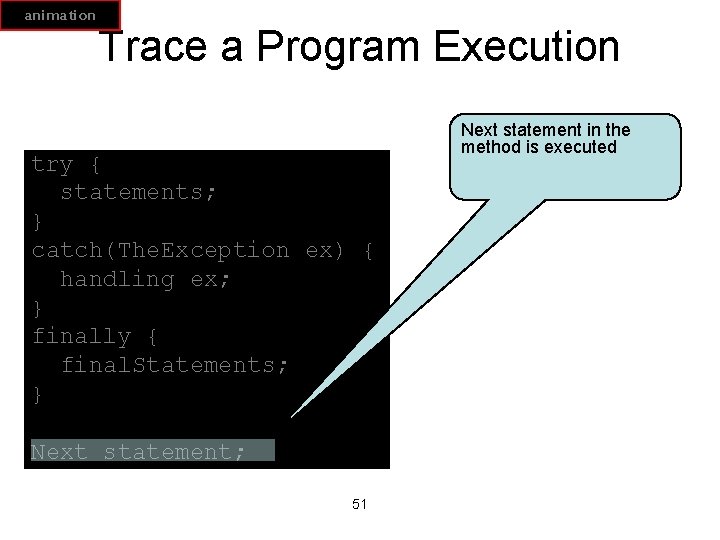
animation Trace a Program Execution try { statements; } catch(The. Exception ex) { handling ex; } finally { final. Statements; } Next statement; 51 Next statement in the method is executed
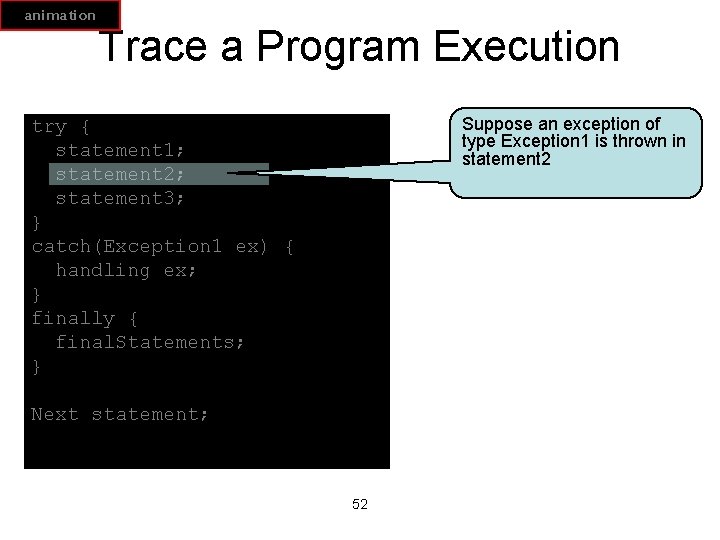
animation Trace a Program Execution Suppose an exception of type Exception 1 is thrown in statement 2 try { statement 1; statement 2; statement 3; } catch(Exception 1 ex) { handling ex; } finally { final. Statements; } Next statement; 52
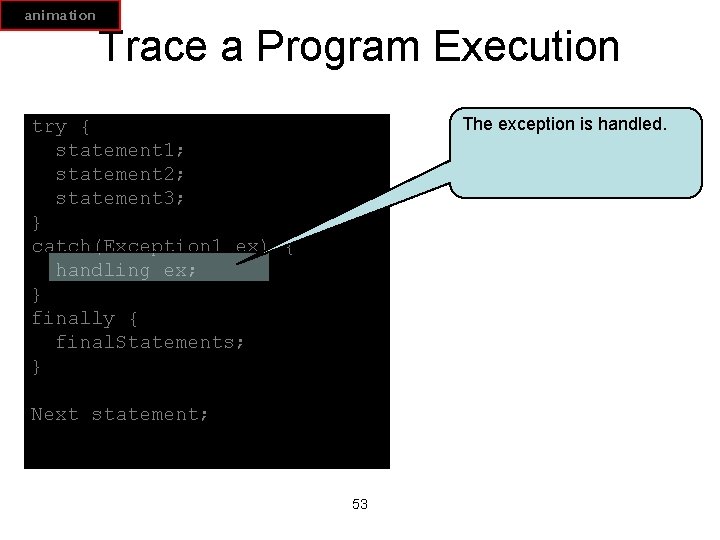
animation Trace a Program Execution The exception is handled. try { statement 1; statement 2; statement 3; } catch(Exception 1 ex) { handling ex; } finally { final. Statements; } Next statement; 53
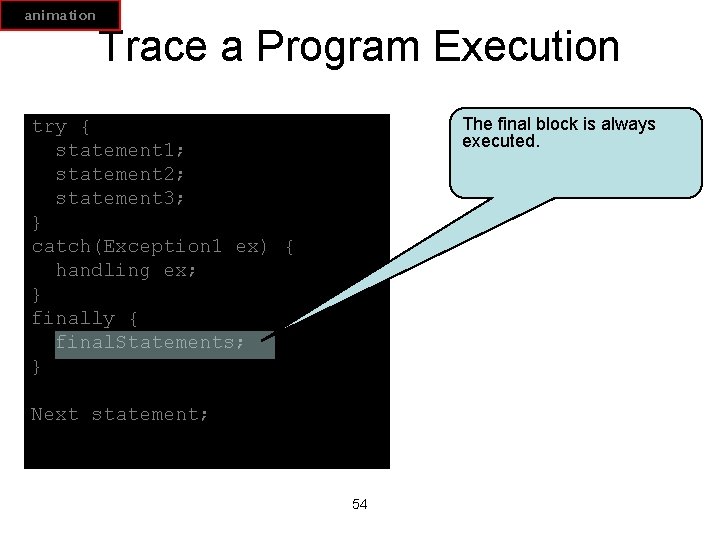
animation Trace a Program Execution The final block is always executed. try { statement 1; statement 2; statement 3; } catch(Exception 1 ex) { handling ex; } finally { final. Statements; } Next statement; 54
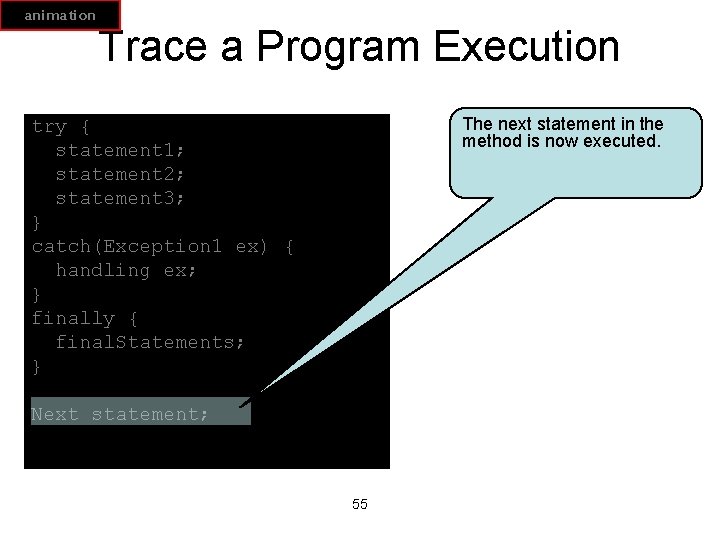
animation Trace a Program Execution The next statement in the method is now executed. try { statement 1; statement 2; statement 3; } catch(Exception 1 ex) { handling ex; } finally { final. Statements; } Next statement; 55
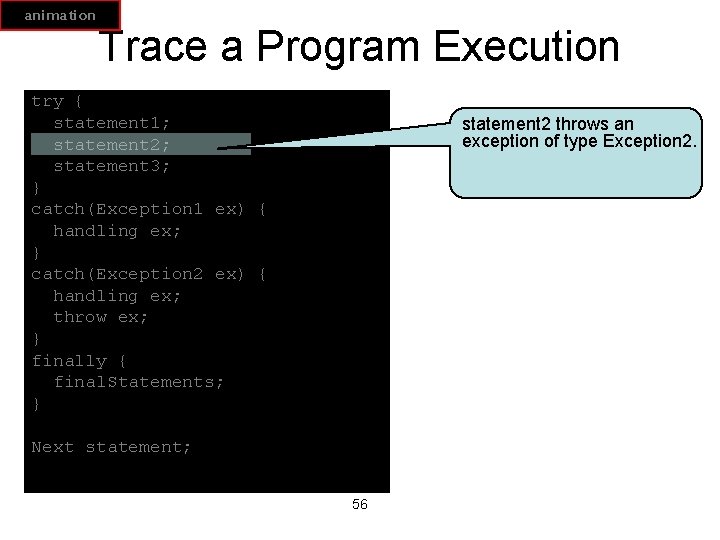
animation Trace a Program Execution try { statement 1; statement 2; statement 3; } catch(Exception 1 ex) { handling ex; } catch(Exception 2 ex) { handling ex; throw ex; } finally { final. Statements; } statement 2 throws an exception of type Exception 2. Next statement; 56
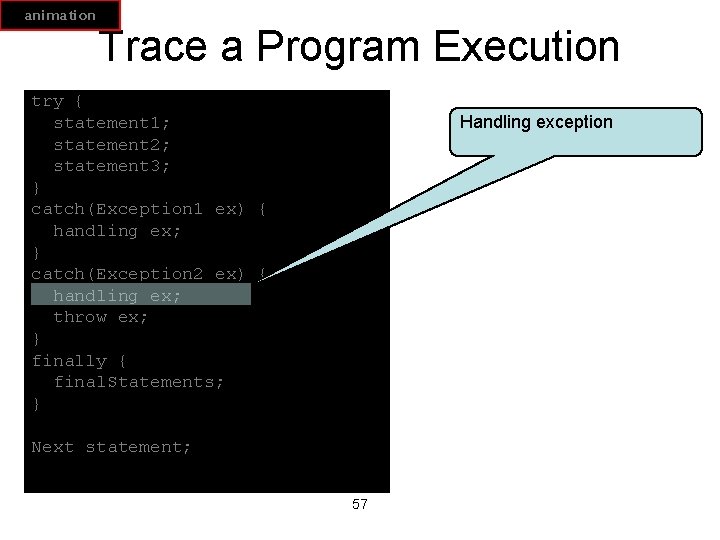
animation Trace a Program Execution try { statement 1; statement 2; statement 3; } catch(Exception 1 ex) { handling ex; } catch(Exception 2 ex) { handling ex; throw ex; } finally { final. Statements; } Handling exception Next statement; 57
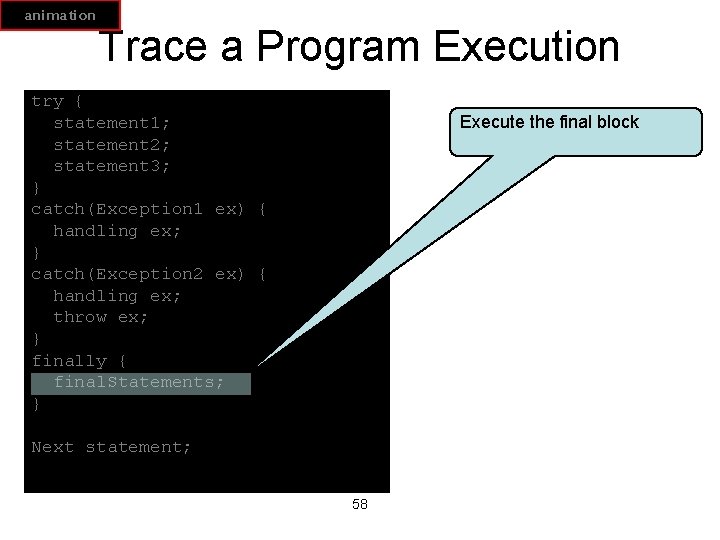
animation Trace a Program Execution try { statement 1; statement 2; statement 3; } catch(Exception 1 ex) { handling ex; } catch(Exception 2 ex) { handling ex; throw ex; } finally { final. Statements; } Execute the final block Next statement; 58
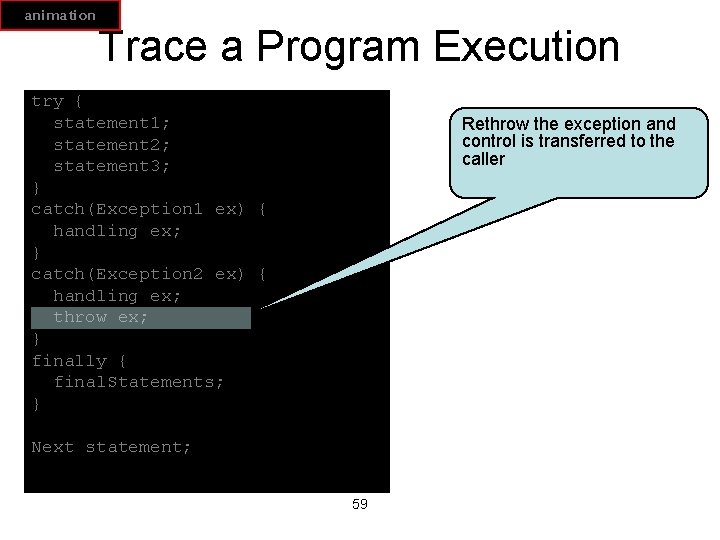
animation Trace a Program Execution try { statement 1; statement 2; statement 3; } catch(Exception 1 ex) { handling ex; } catch(Exception 2 ex) { handling ex; throw ex; } finally { final. Statements; } Rethrow the exception and control is transferred to the caller Next statement; 59
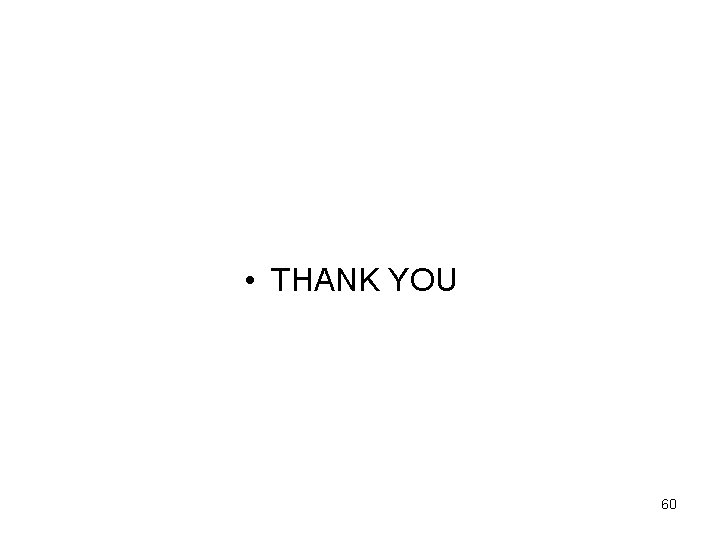
• THANK YOU 60
Exception handling pada java
Exception handling in vb.net
Exception handling in java
No irq handler for vector
Apa yang dimaksud dengan exception handling
Event handling in ada
Contoh error handling
Php exception
Php global exception handler
Vb.net error handling
Exception vs error in java
Pemrograman berorientasi objek
Robust programs
Tceq exception request
Liraglutide ramq
Exception of koch postulates
Phenotypic characteristics of bacteria
Public static void main
Fajans rules
An exception is a runtime error
Exception
Progress oriented record adalah
Usfws designated ports
Explain the exceptions to mendel's law
Java unchecked exception
Class a void foo() throws exception
What exception if any permits a private pilot
What is exception in computer architecture
Apa itu exception
Runtimeexception extends exception
Clien
Class not found exception
Bad superlative form
Thread class hierarchy in java
Java exception error
No data found exception in oracle
Exception class hierarchy in java
Java exception localized message
Tricky words phase 3
Class a void foo() throws exception
Friends exception
A programmer can choose to ignore an unchecked exception.
Goodsall rule exception
What is exception
R throw exception
Data migration strategiesê
Innerexception: system.io.directorynotfoundexception
Software interrupts
Http data source exception
No data found exception in oracle
Charting by exception pros and cons
Unt pcard exception form
Java unchecked exception
Invoice exception
Electronegativity rules
Apa yang dimaksud dengan exception
Epc register mips
Pl/i error handling
Concurre
Ten principles of material handling
Withoutexceptionhandling