Java Mail API Sending and Receiving Emails Mail
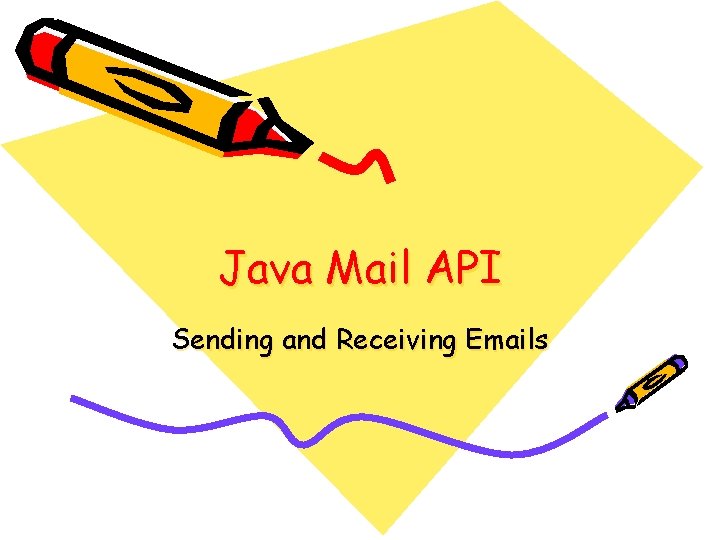
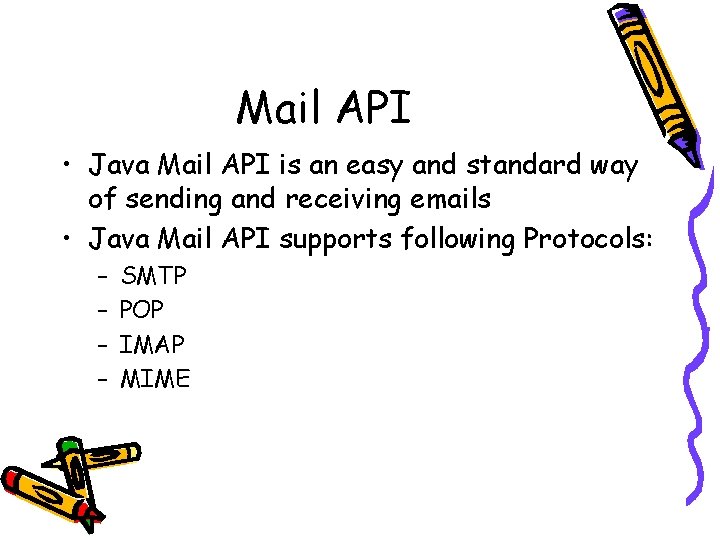
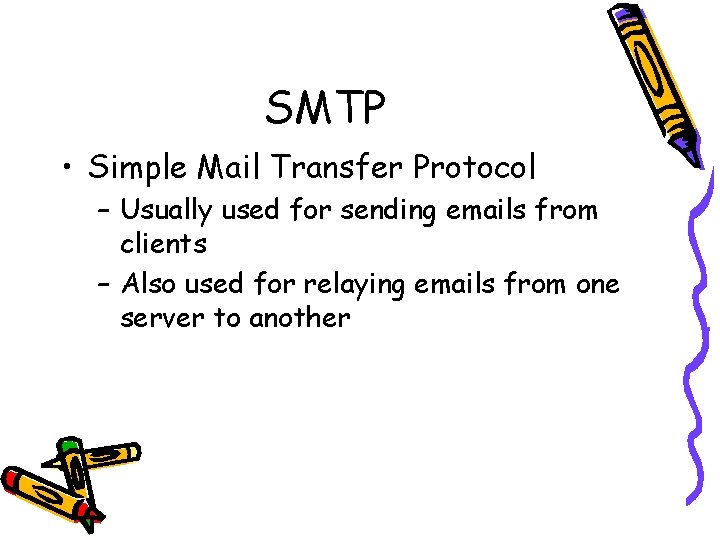
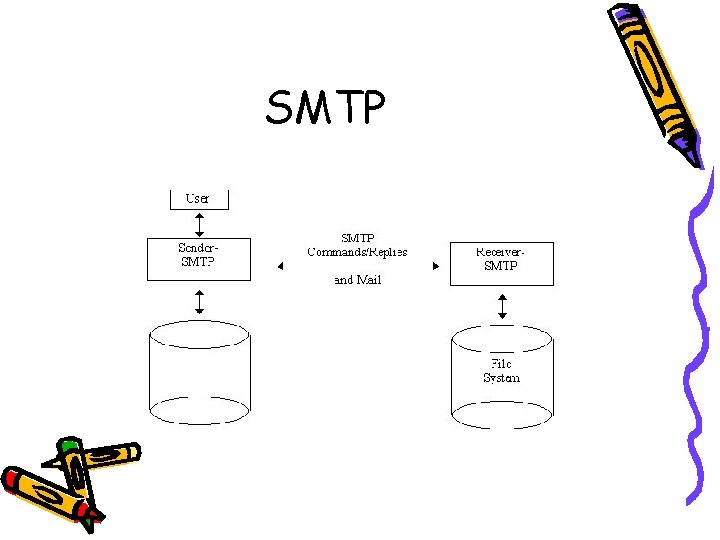
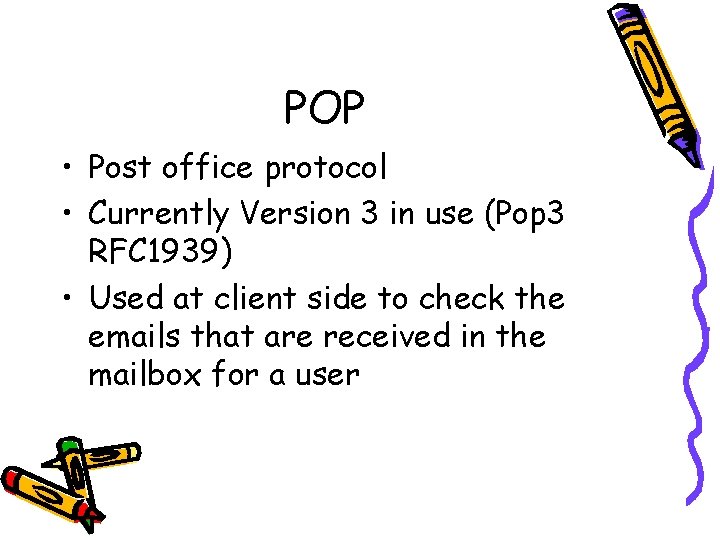
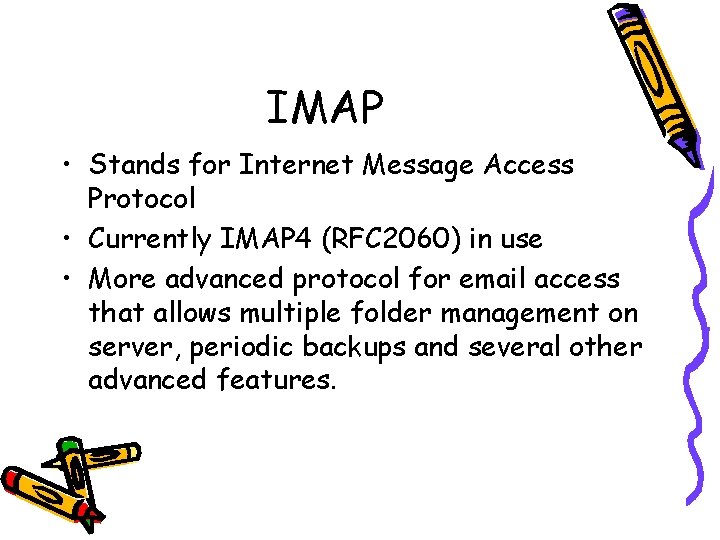
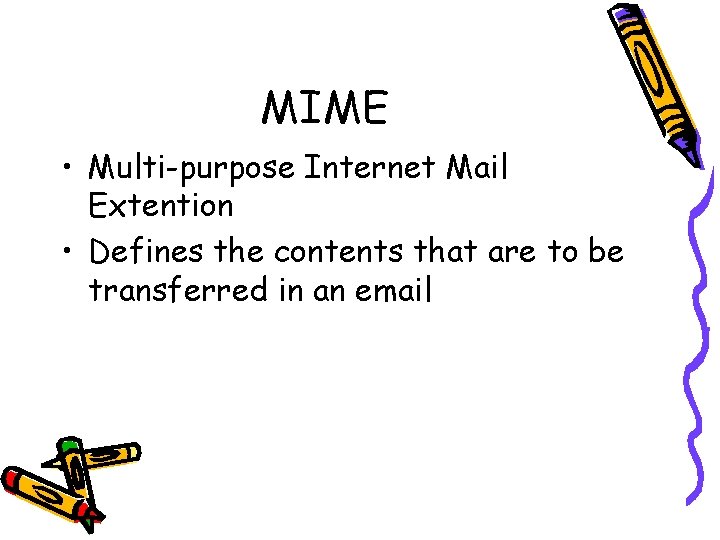
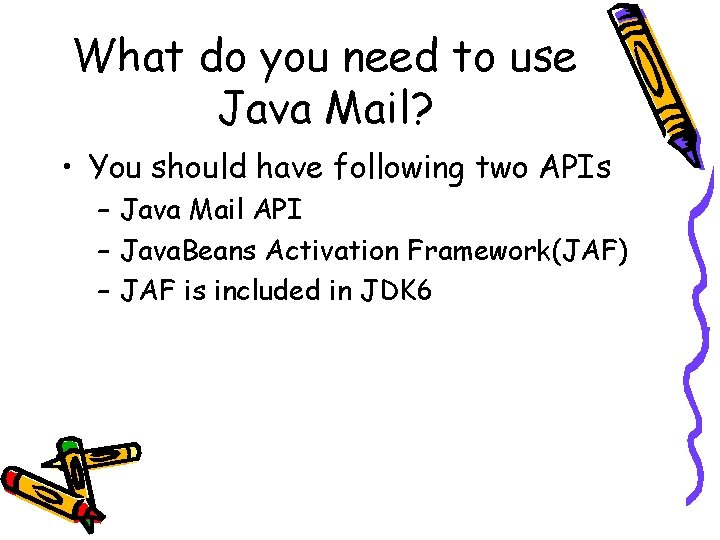
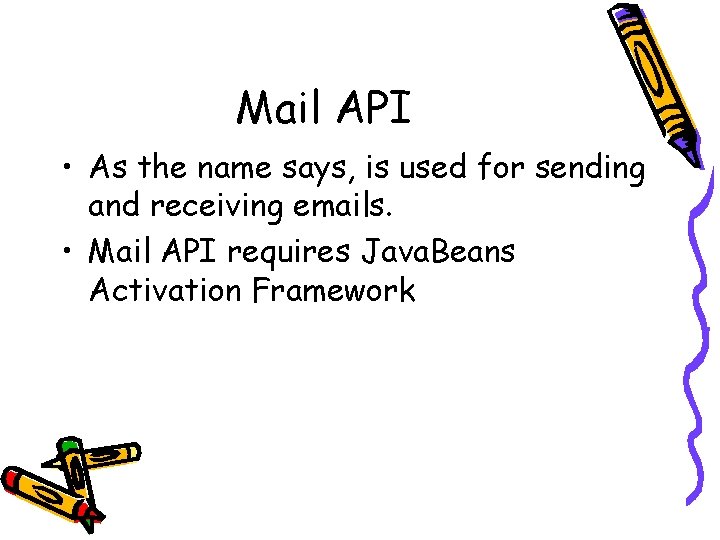
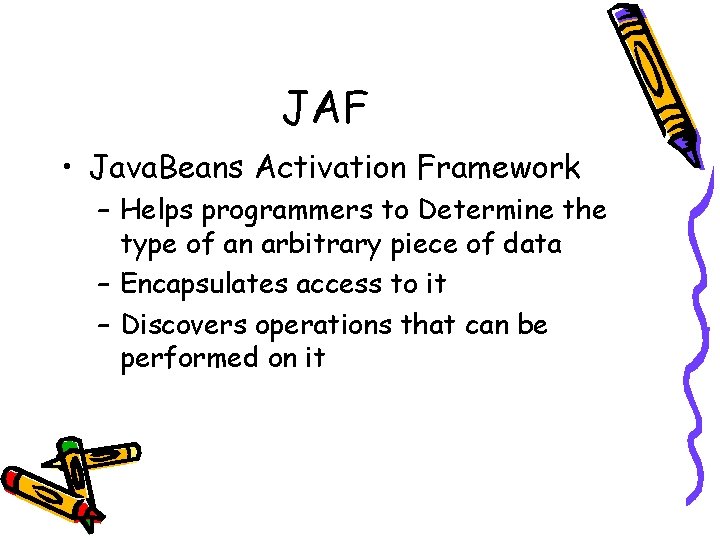
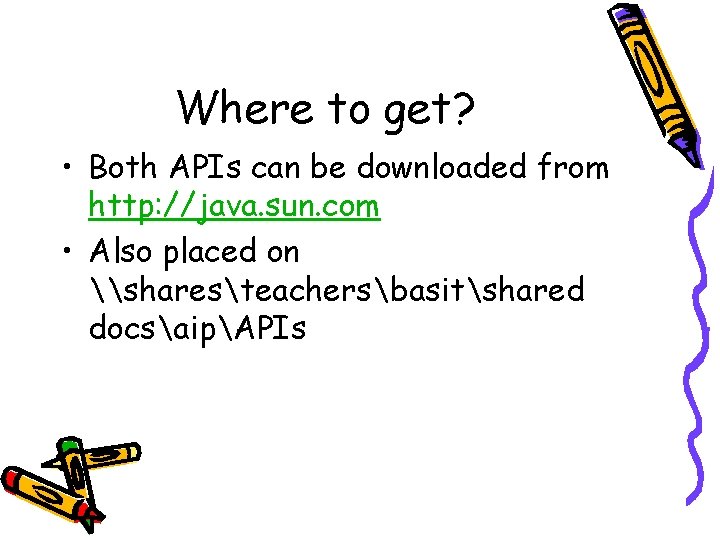
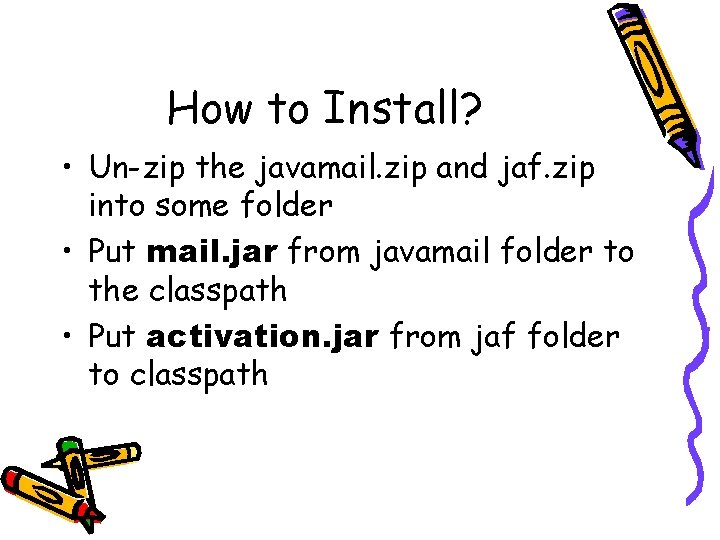
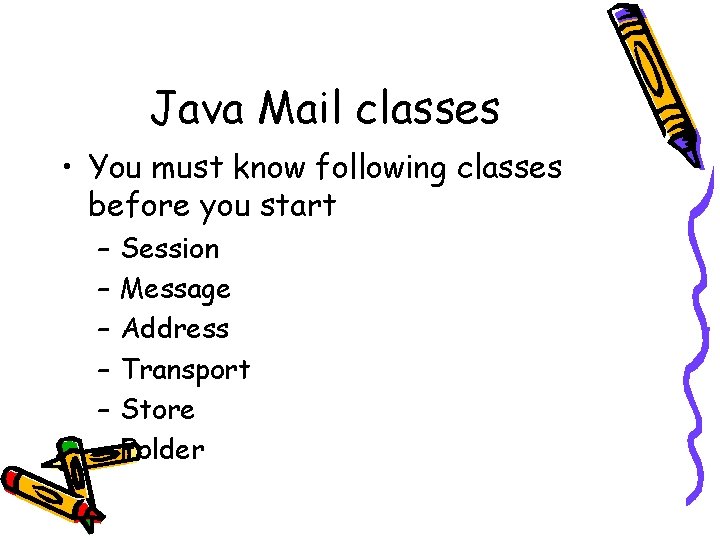
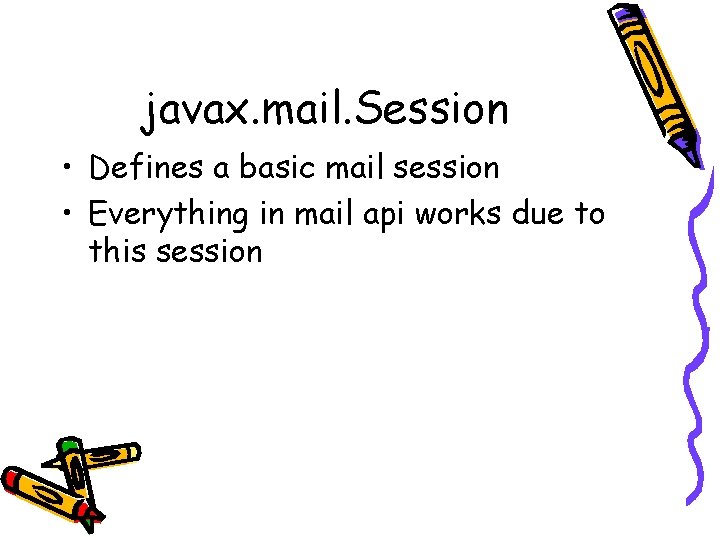
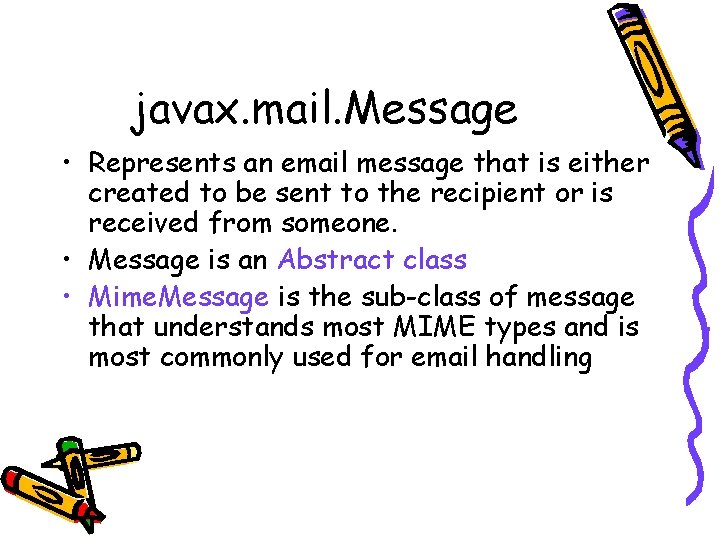
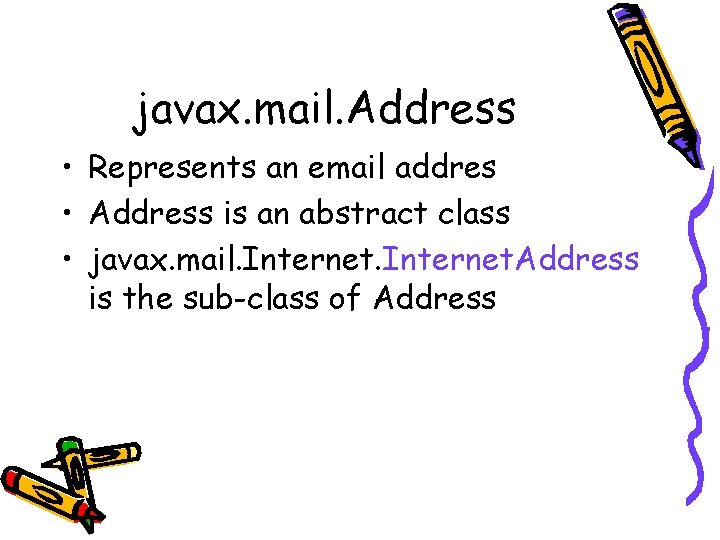
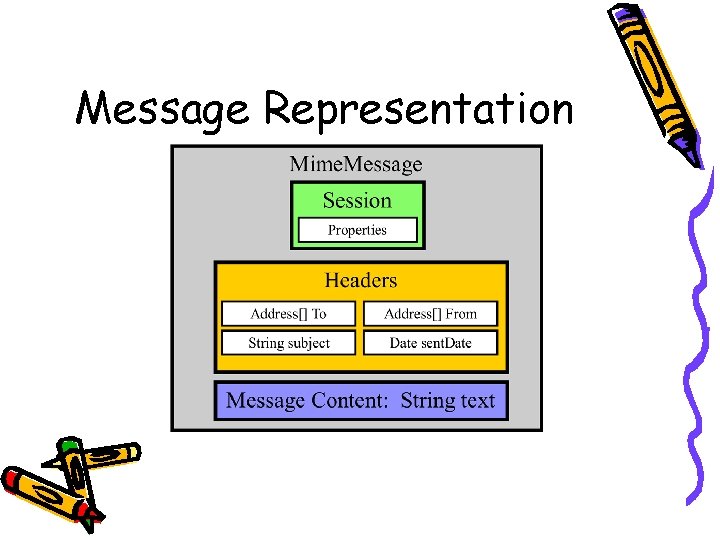
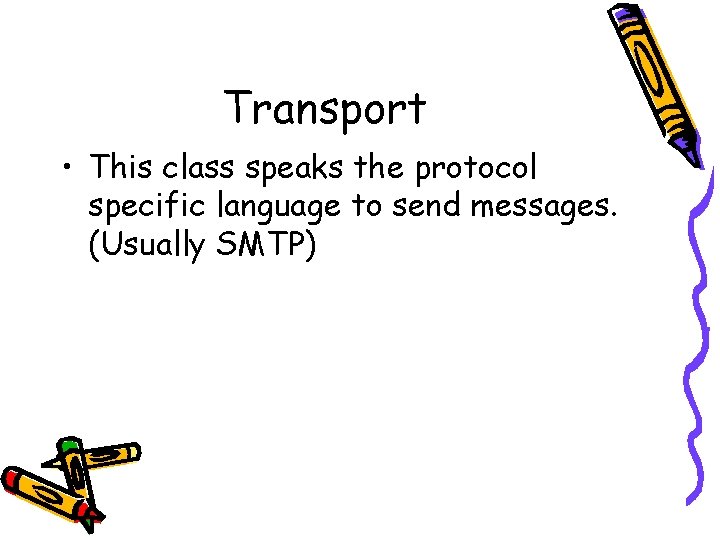
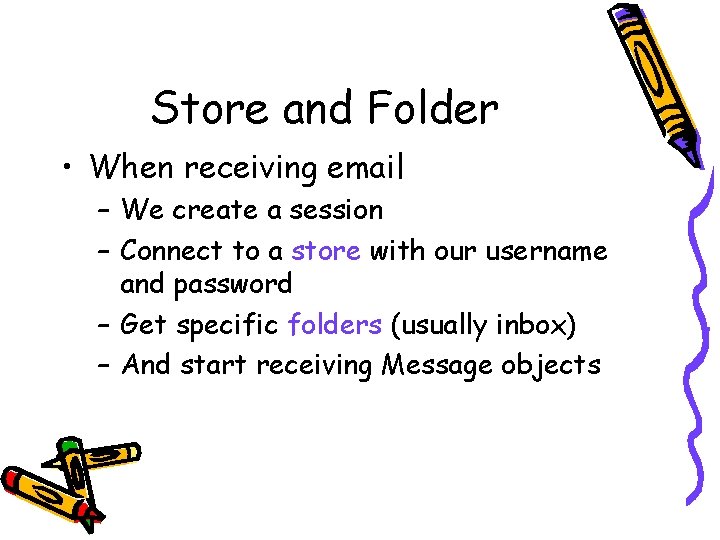
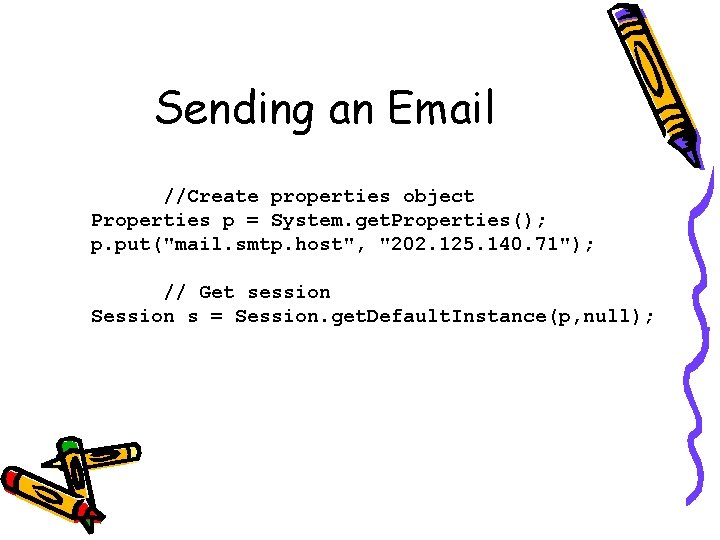
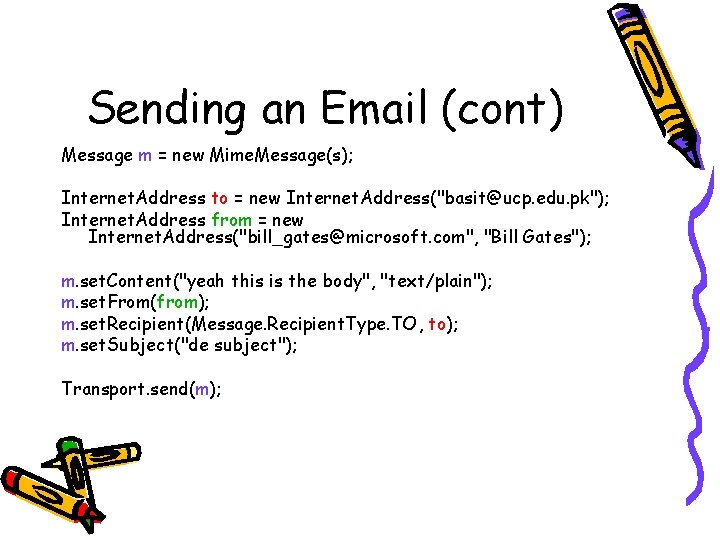
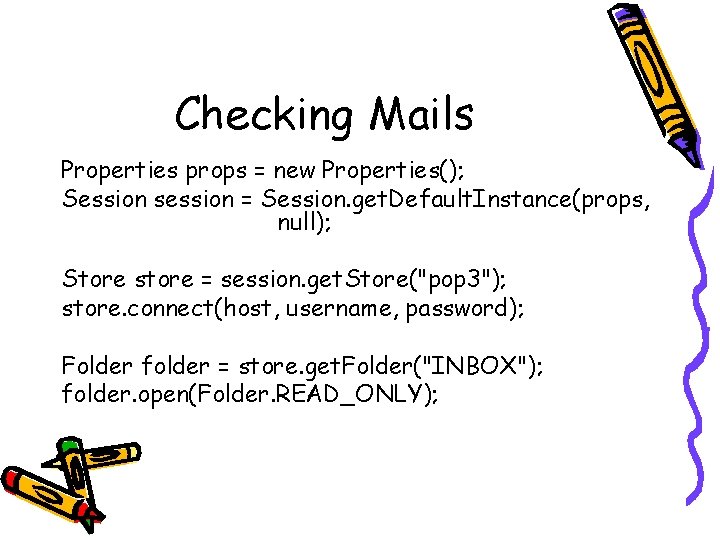
![Checking Mails(cont) Message message[] = folder. get. Messages(); for (int i=0, n=message. length; i<n; Checking Mails(cont) Message message[] = folder. get. Messages(); for (int i=0, n=message. length; i<n;](https://slidetodoc.com/presentation_image_h/05b3c22635f1d92a2f5800cdc98da524/image-23.jpg)
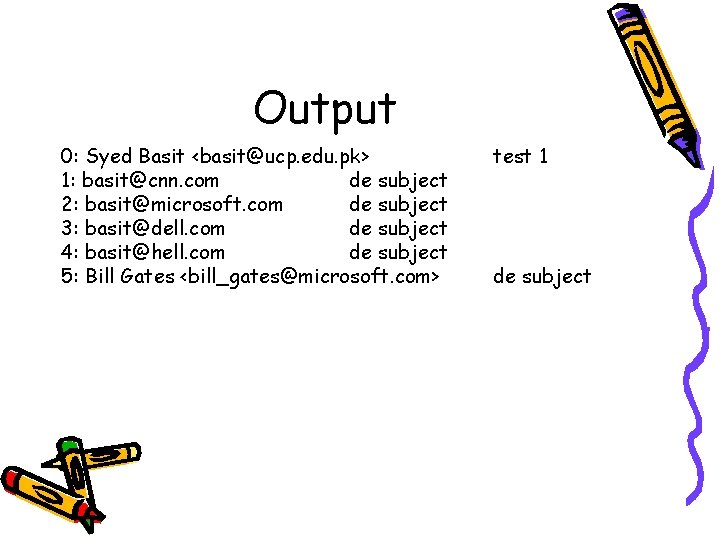
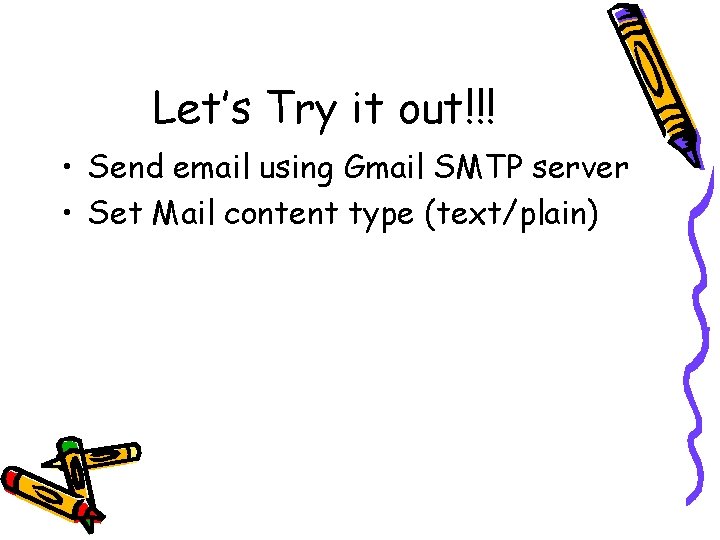
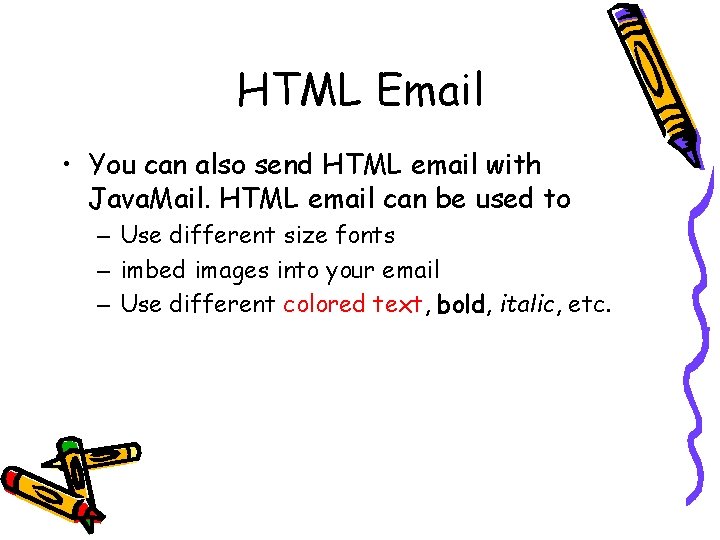
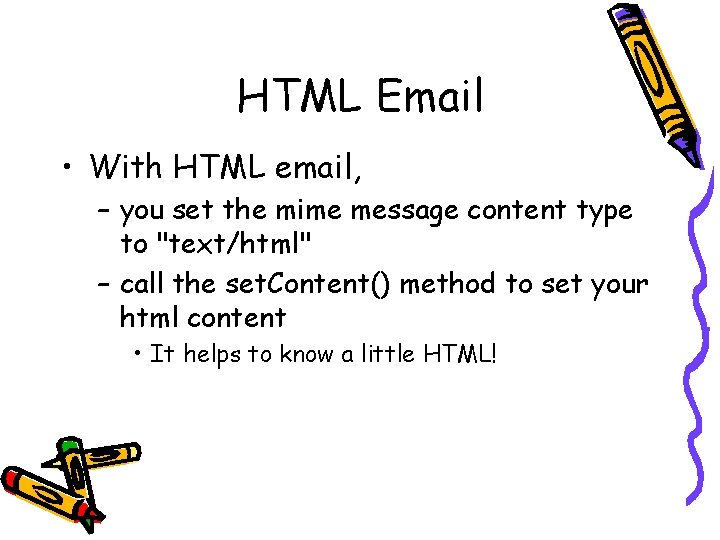
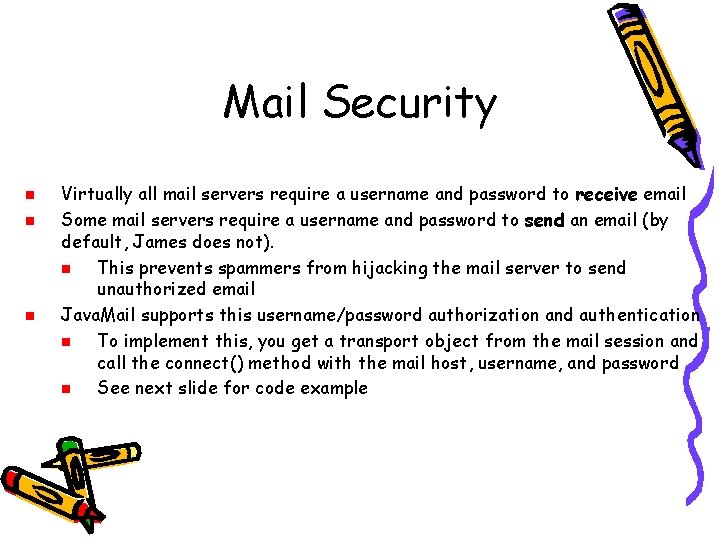
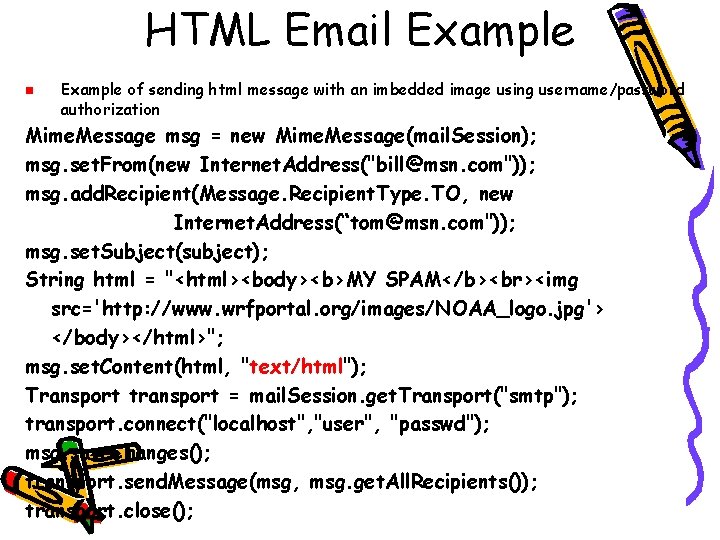
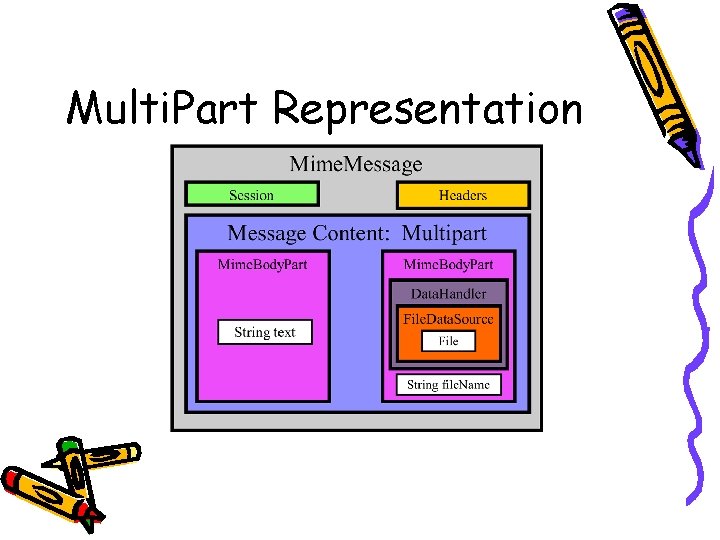
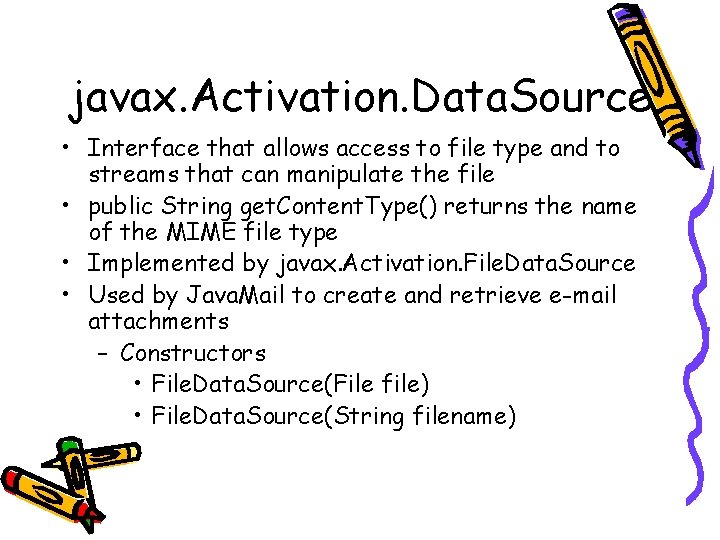
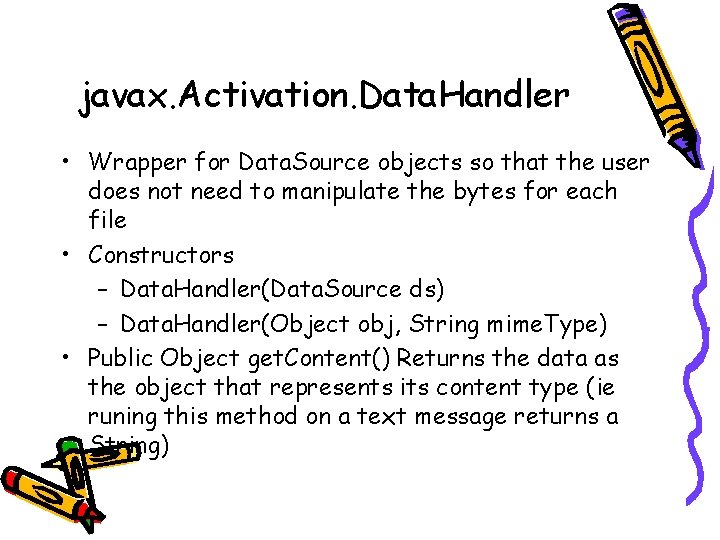
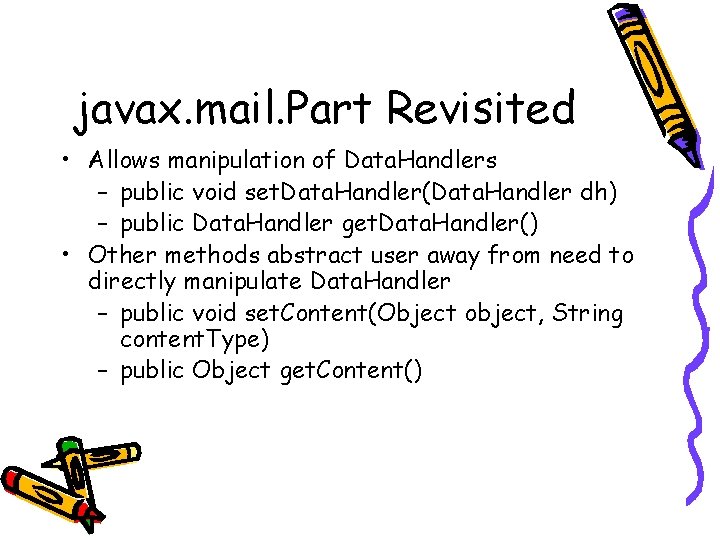
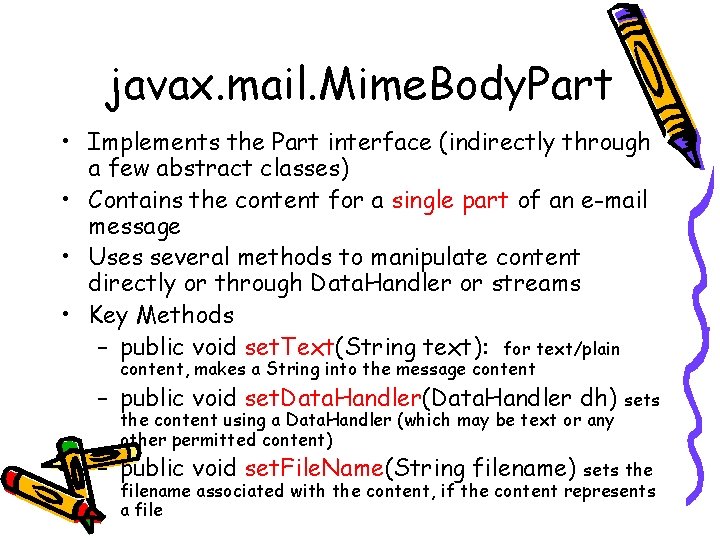
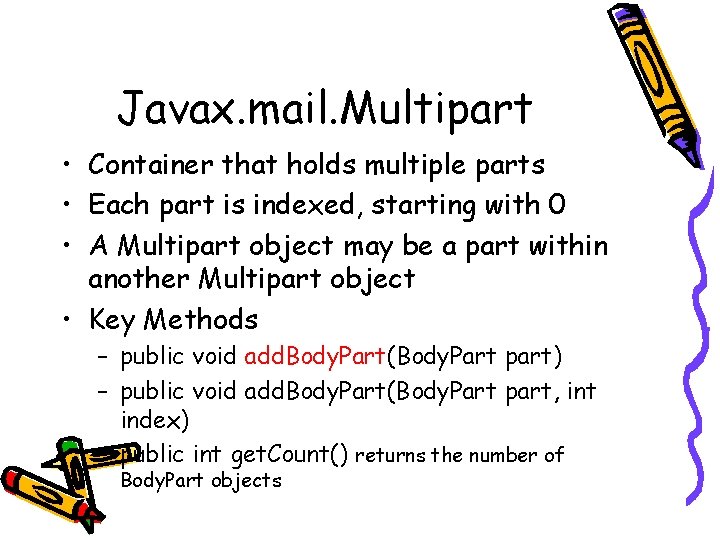
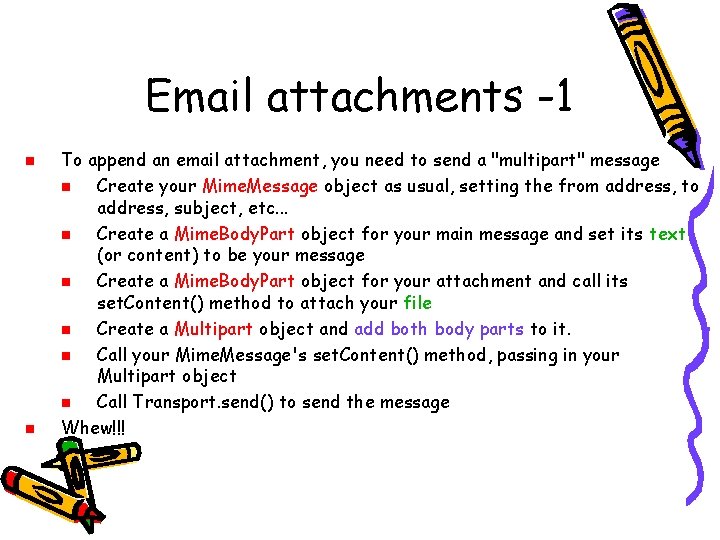
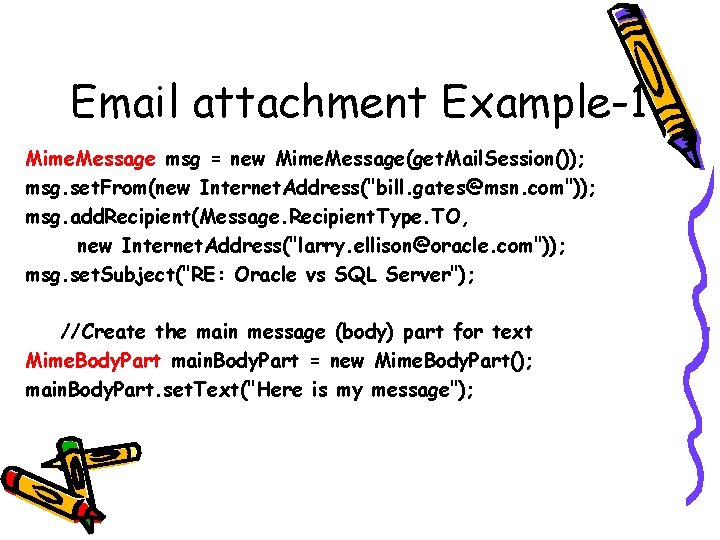
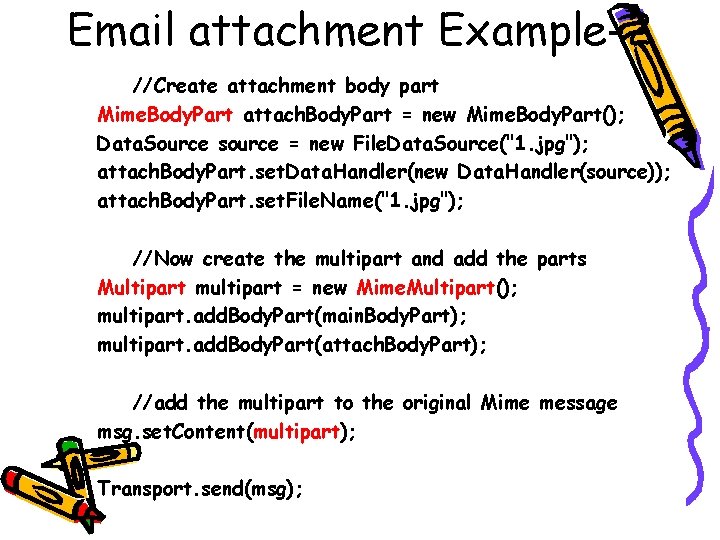
- Slides: 38
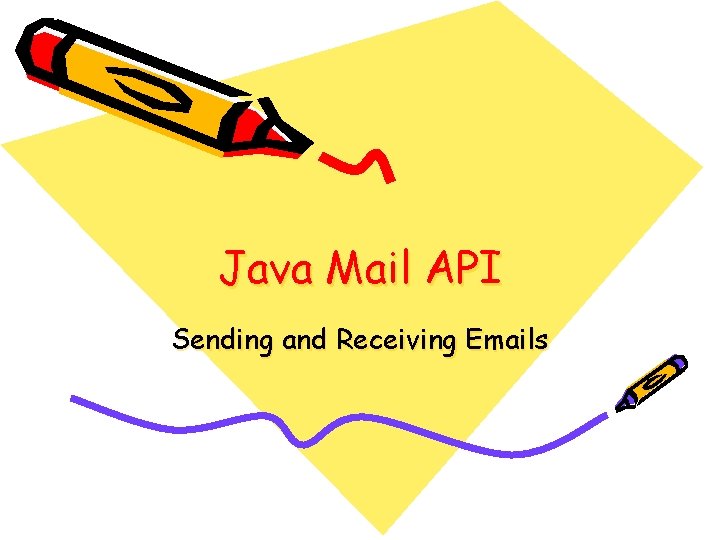
Java Mail API Sending and Receiving Emails
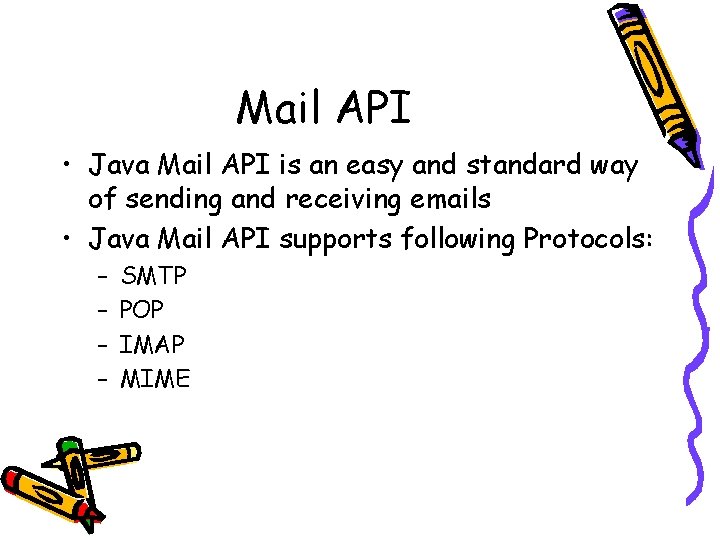
Mail API • Java Mail API is an easy and standard way of sending and receiving emails • Java Mail API supports following Protocols: – – SMTP POP IMAP MIME
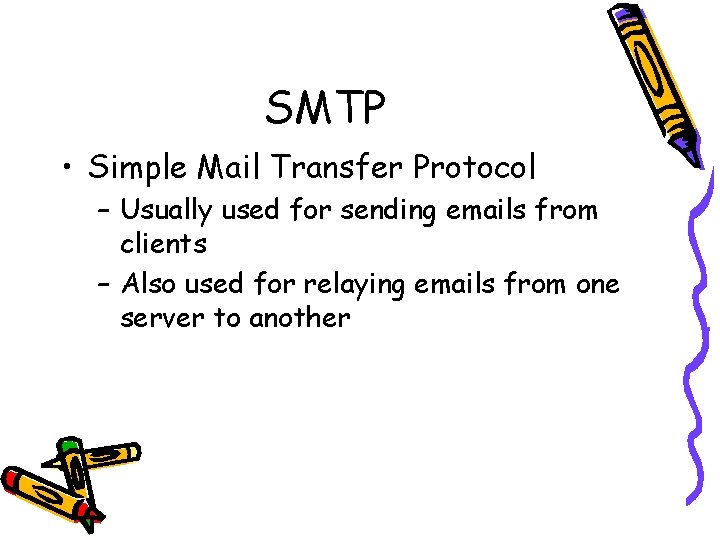
SMTP • Simple Mail Transfer Protocol – Usually used for sending emails from clients – Also used for relaying emails from one server to another
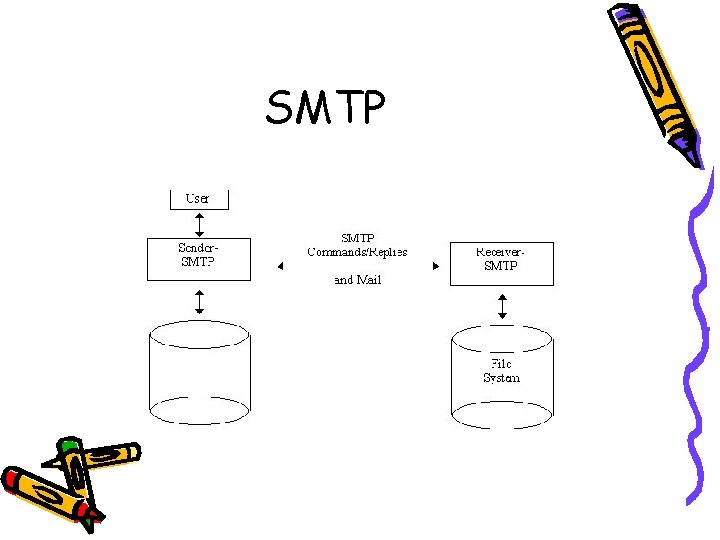
SMTP
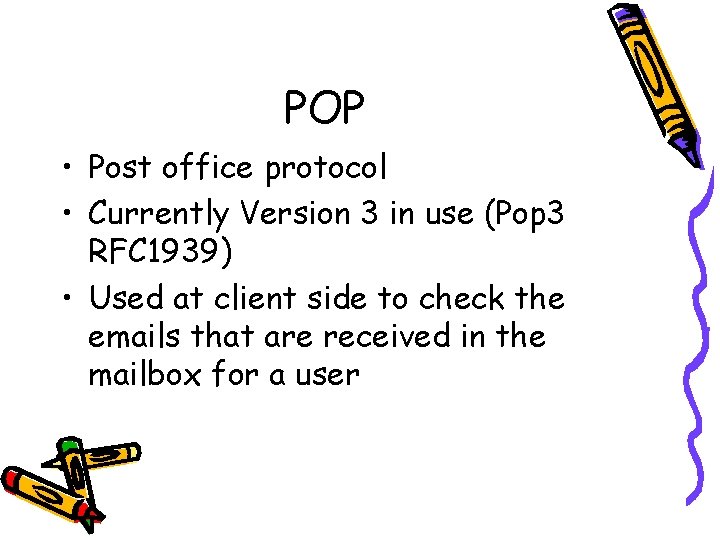
POP • Post office protocol • Currently Version 3 in use (Pop 3 RFC 1939) • Used at client side to check the emails that are received in the mailbox for a user
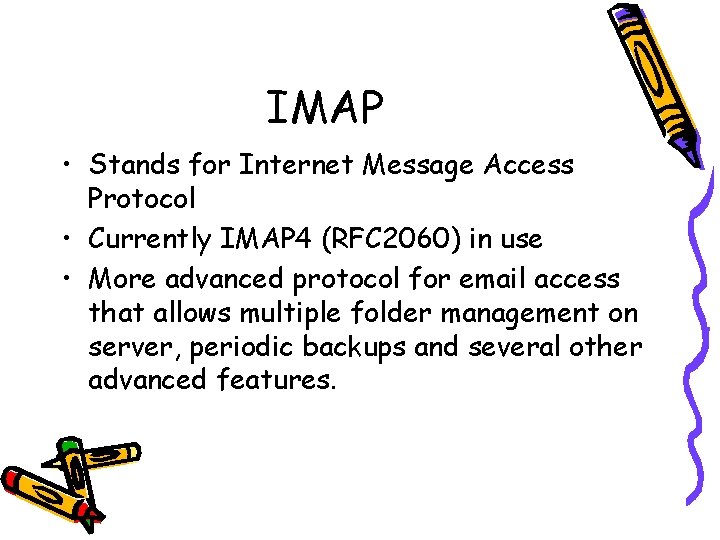
IMAP • Stands for Internet Message Access Protocol • Currently IMAP 4 (RFC 2060) in use • More advanced protocol for email access that allows multiple folder management on server, periodic backups and several other advanced features.
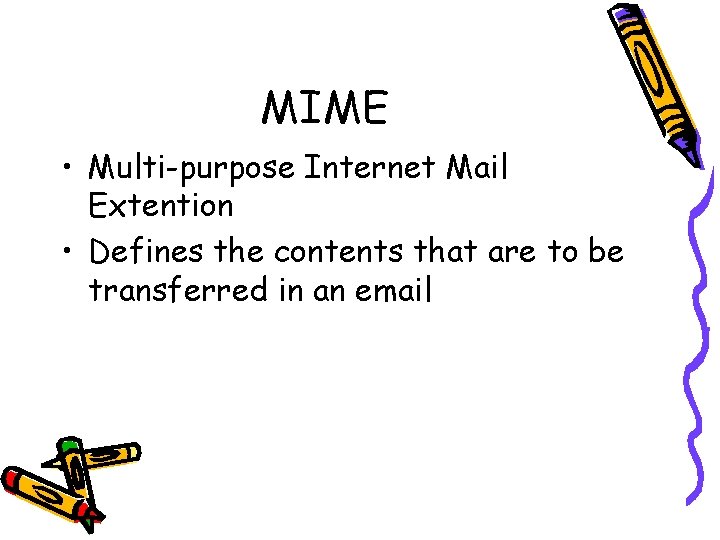
MIME • Multi-purpose Internet Mail Extention • Defines the contents that are to be transferred in an email
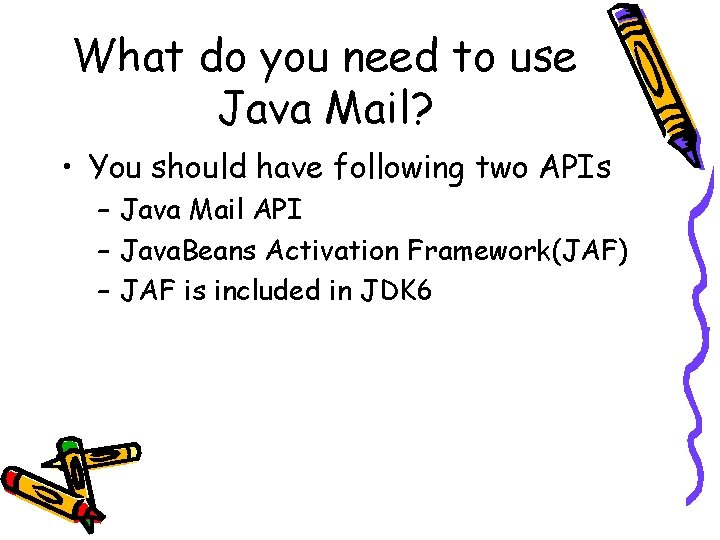
What do you need to use Java Mail? • You should have following two APIs – Java Mail API – Java. Beans Activation Framework(JAF) – JAF is included in JDK 6
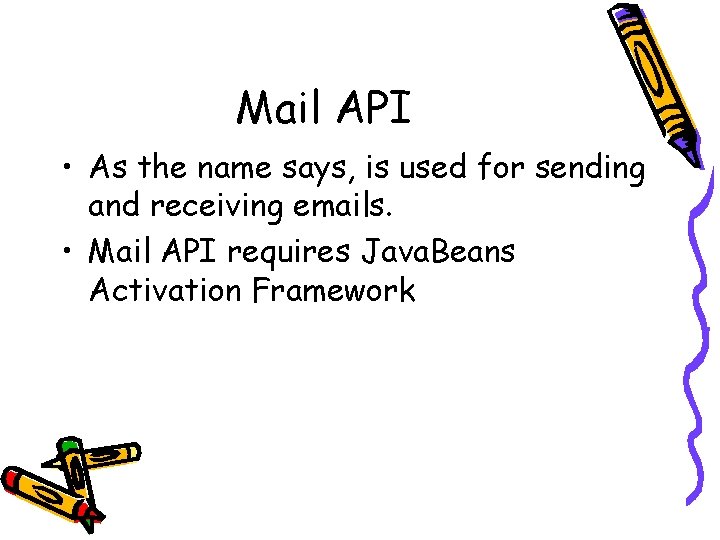
Mail API • As the name says, is used for sending and receiving emails. • Mail API requires Java. Beans Activation Framework
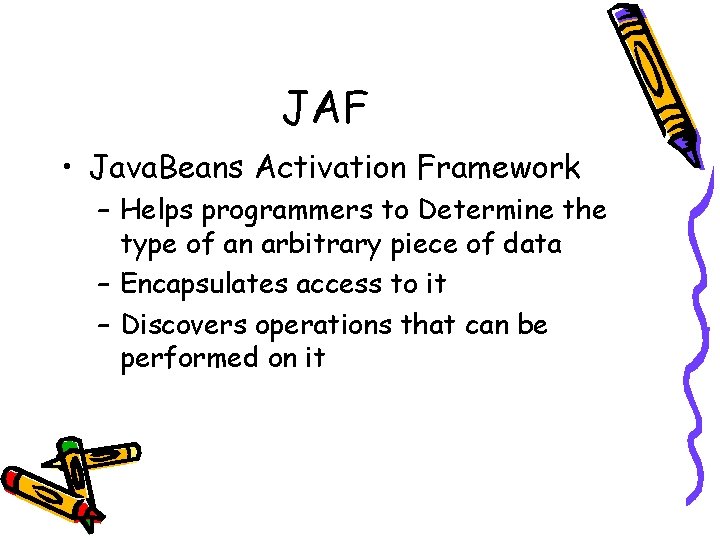
JAF • Java. Beans Activation Framework – Helps programmers to Determine the type of an arbitrary piece of data – Encapsulates access to it – Discovers operations that can be performed on it
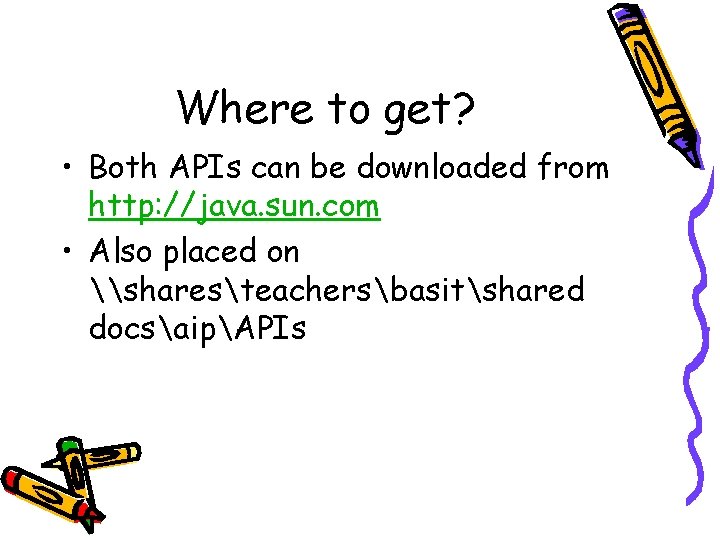
Where to get? • Both APIs can be downloaded from http: //java. sun. com • Also placed on \sharesteachersbasitshared docsaipAPIs
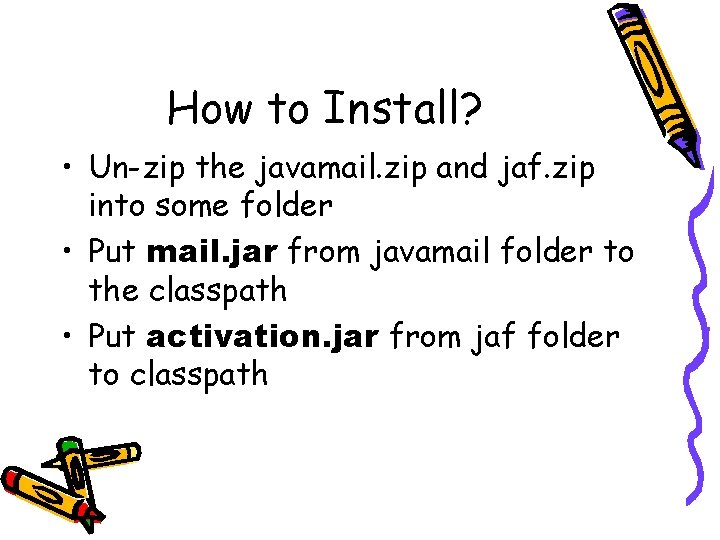
How to Install? • Un-zip the javamail. zip and jaf. zip into some folder • Put mail. jar from javamail folder to the classpath • Put activation. jar from jaf folder to classpath
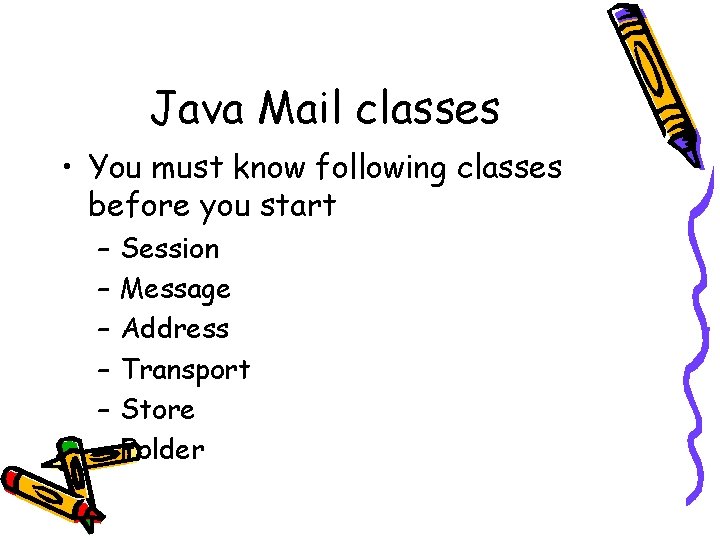
Java Mail classes • You must know following classes before you start – – – Session Message Address Transport Store Folder
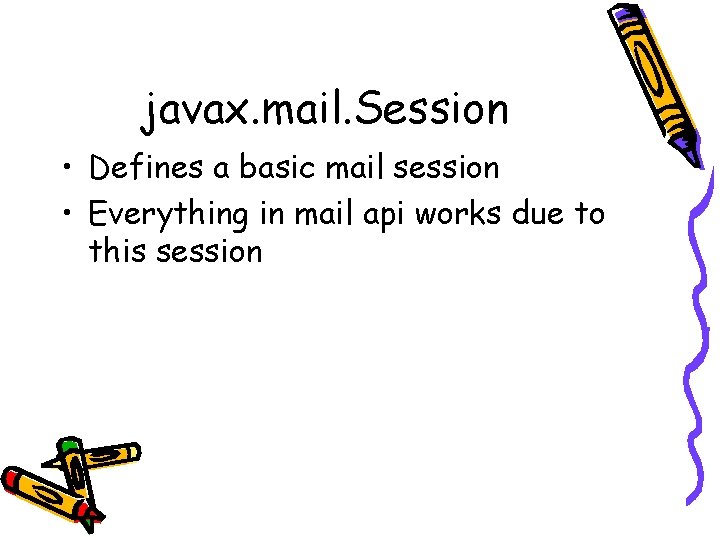
javax. mail. Session • Defines a basic mail session • Everything in mail api works due to this session
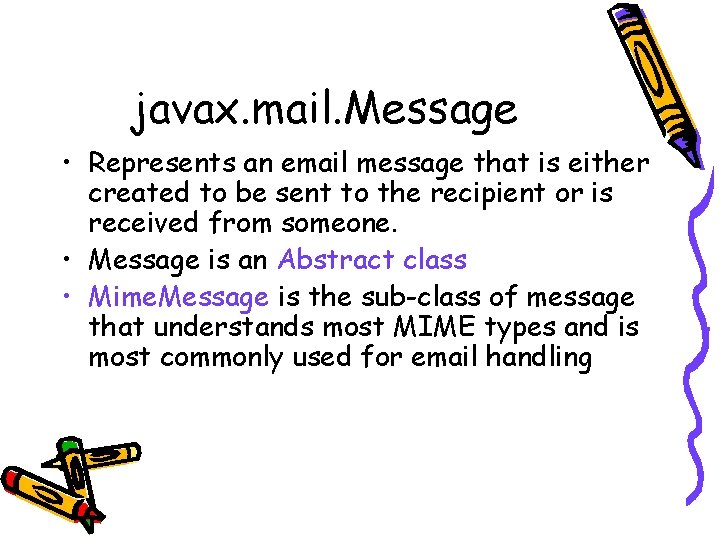
javax. mail. Message • Represents an email message that is either created to be sent to the recipient or is received from someone. • Message is an Abstract class • Mime. Message is the sub-class of message that understands most MIME types and is most commonly used for email handling
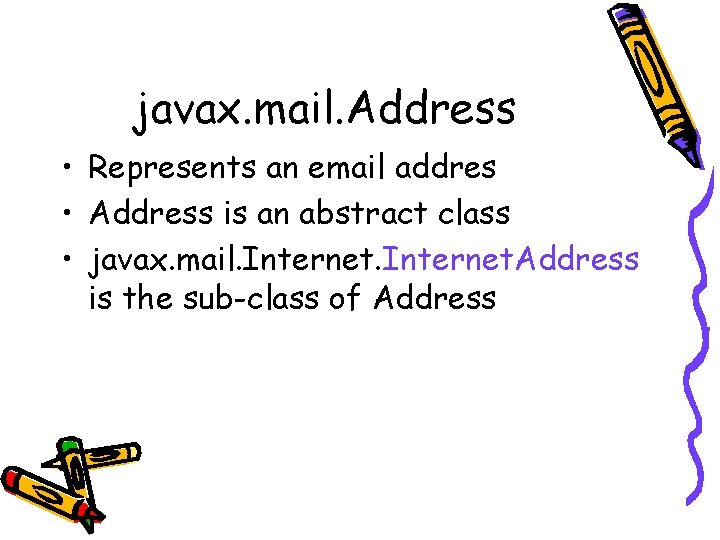
javax. mail. Address • Represents an email addres • Address is an abstract class • javax. mail. Internet. Address is the sub-class of Address
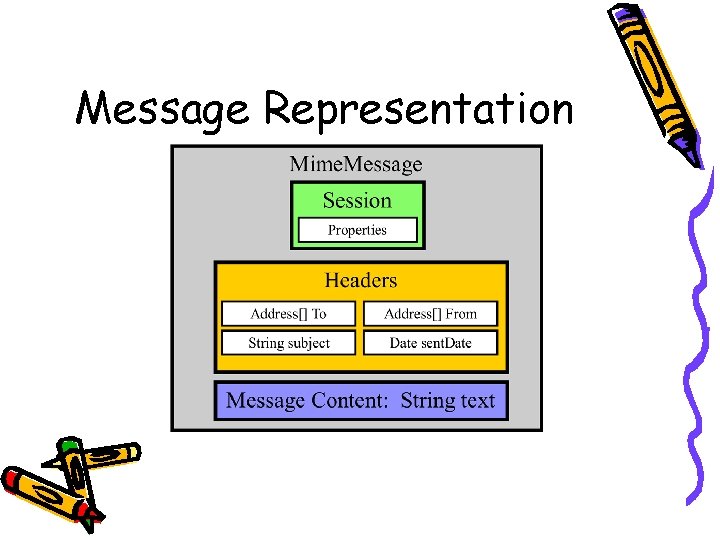
Message Representation
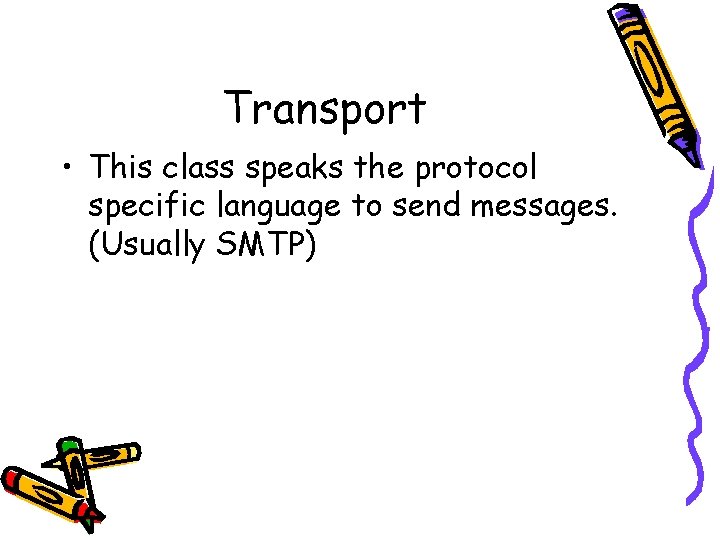
Transport • This class speaks the protocol specific language to send messages. (Usually SMTP)
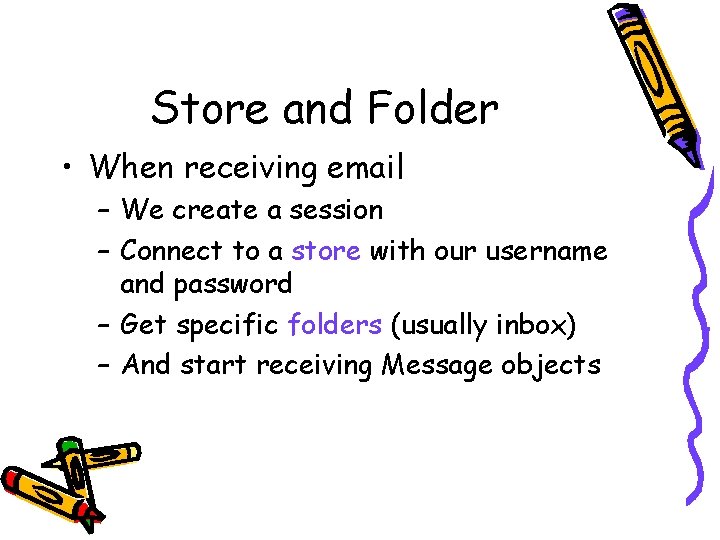
Store and Folder • When receiving email – We create a session – Connect to a store with our username and password – Get specific folders (usually inbox) – And start receiving Message objects
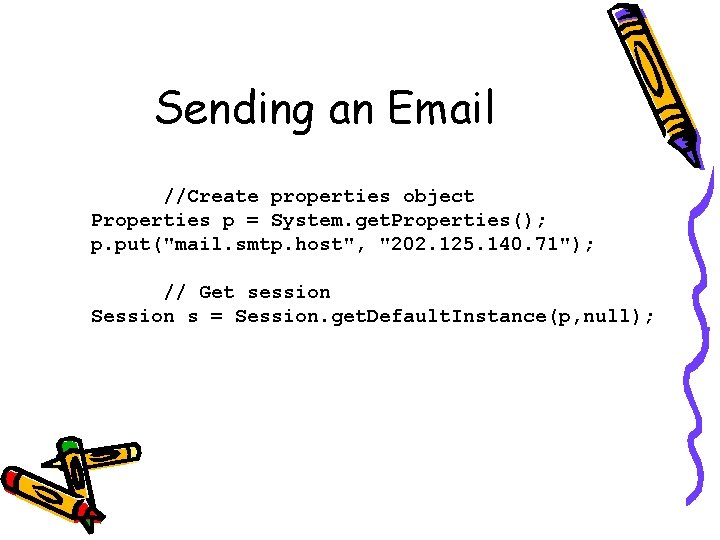
Sending an Email //Create properties object Properties p = System. get. Properties(); p. put("mail. smtp. host", "202. 125. 140. 71"); // Get session Session s = Session. get. Default. Instance(p, null);
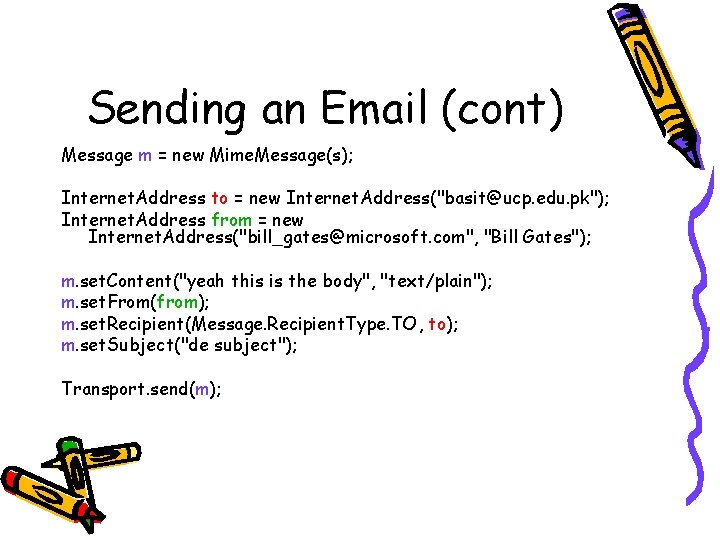
Sending an Email (cont) Message m = new Mime. Message(s); Internet. Address to = new Internet. Address("basit@ucp. edu. pk"); Internet. Address from = new Internet. Address("bill_gates@microsoft. com", "Bill Gates"); m. set. Content("yeah this is the body", "text/plain"); m. set. From(from); m. set. Recipient(Message. Recipient. Type. TO, to); m. set. Subject("de subject"); Transport. send(m);
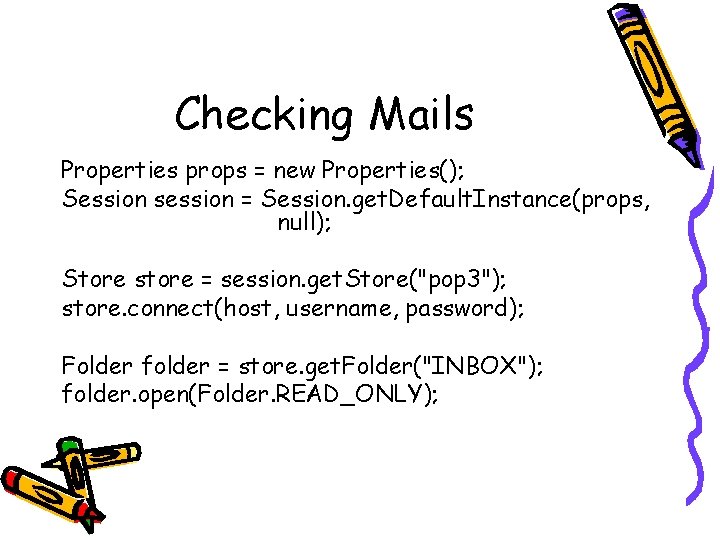
Checking Mails Properties props = new Properties(); Session session = Session. get. Default. Instance(props, null); Store store = session. get. Store("pop 3"); store. connect(host, username, password); Folder folder = store. get. Folder("INBOX"); folder. open(Folder. READ_ONLY);
![Checking Mailscont Message message folder get Messages for int i0 nmessage length in Checking Mails(cont) Message message[] = folder. get. Messages(); for (int i=0, n=message. length; i<n;](https://slidetodoc.com/presentation_image_h/05b3c22635f1d92a2f5800cdc98da524/image-23.jpg)
Checking Mails(cont) Message message[] = folder. get. Messages(); for (int i=0, n=message. length; i<n; i++) { System. out. println(i + ": " + message[i]. get. From()[0] + "tt" + message[i]. get. Subject()); } folder. close(false); store. close();
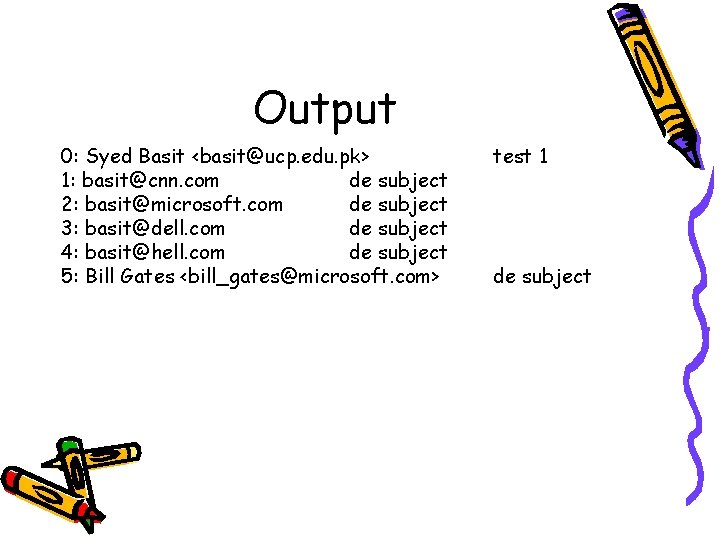
Output 0: Syed Basit <basit@ucp. edu. pk> 1: basit@cnn. com de subject 2: basit@microsoft. com de subject 3: basit@dell. com de subject 4: basit@hell. com de subject 5: Bill Gates <bill_gates@microsoft. com> test 1 de subject
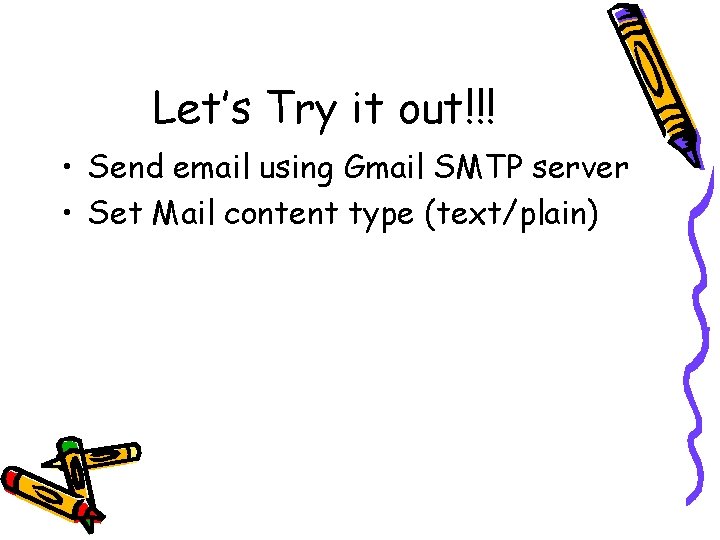
Let’s Try it out!!! • Send email using Gmail SMTP server • Set Mail content type (text/plain)
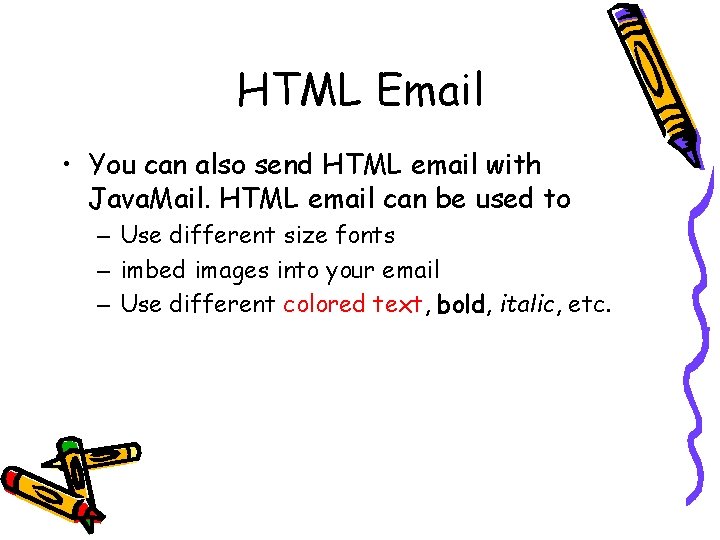
HTML Email • You can also send HTML email with Java. Mail. HTML email can be used to – Use different size fonts – imbed images into your email – Use different colored text, bold, italic, etc.
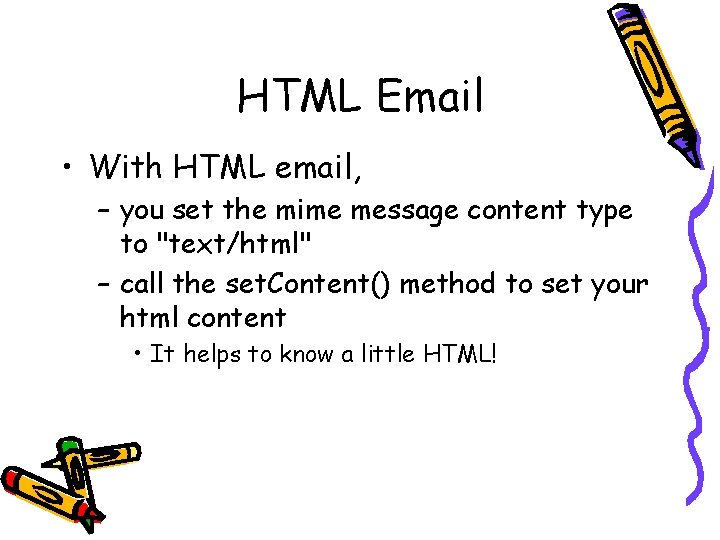
HTML Email • With HTML email, – you set the mime message content type to "text/html" – call the set. Content() method to set your html content • It helps to know a little HTML!
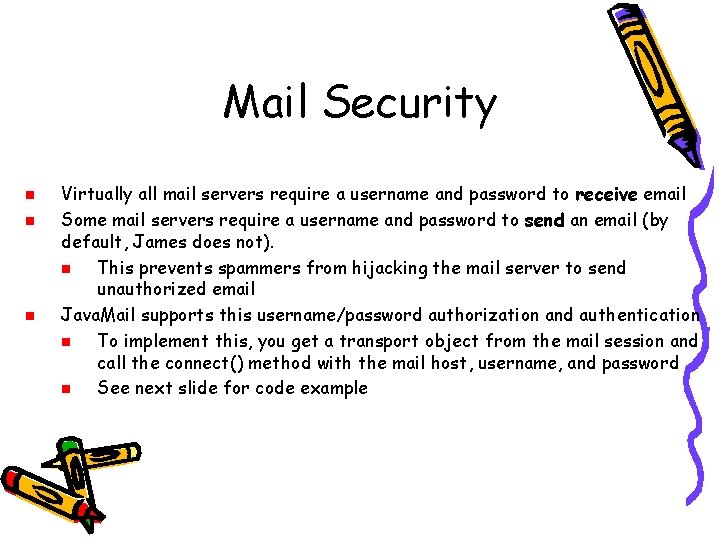
Mail Security n n n Virtually all mail servers require a username and password to receive email Some mail servers require a username and password to send an email (by default, James does not). n This prevents spammers from hijacking the mail server to send unauthorized email Java. Mail supports this username/password authorization and authentication n To implement this, you get a transport object from the mail session and call the connect() method with the mail host, username, and password n See next slide for code example
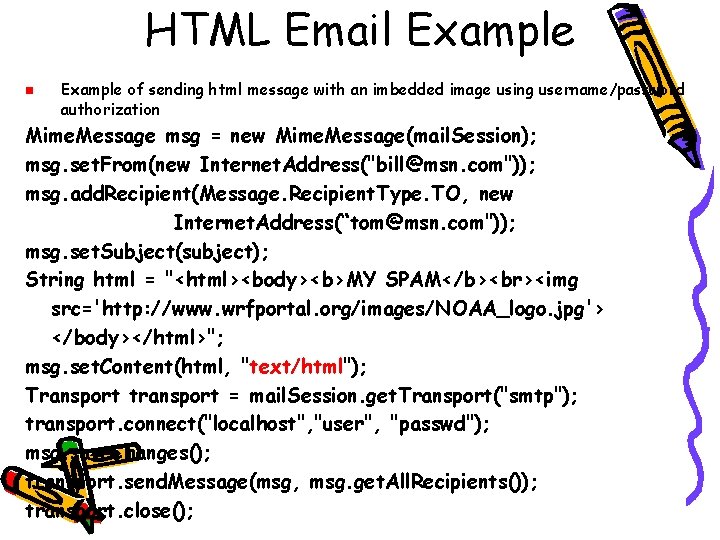
HTML Email Example n Example of sending html message with an imbedded image using username/password authorization Mime. Message msg = new Mime. Message(mail. Session); msg. set. From(new Internet. Address("bill@msn. com")); msg. add. Recipient(Message. Recipient. Type. TO, new Internet. Address(“tom@msn. com")); msg. set. Subject(subject); String html = "<html><body><b>MY SPAM</b> <img src='http: //www. wrfportal. org/images/NOAA_logo. jpg'> </body></html>"; msg. set. Content(html, "text/html"); Transport transport = mail. Session. get. Transport("smtp"); transport. connect("localhost", "user", "passwd"); msg. save. Changes(); transport. send. Message(msg, msg. get. All. Recipients()); transport. close();
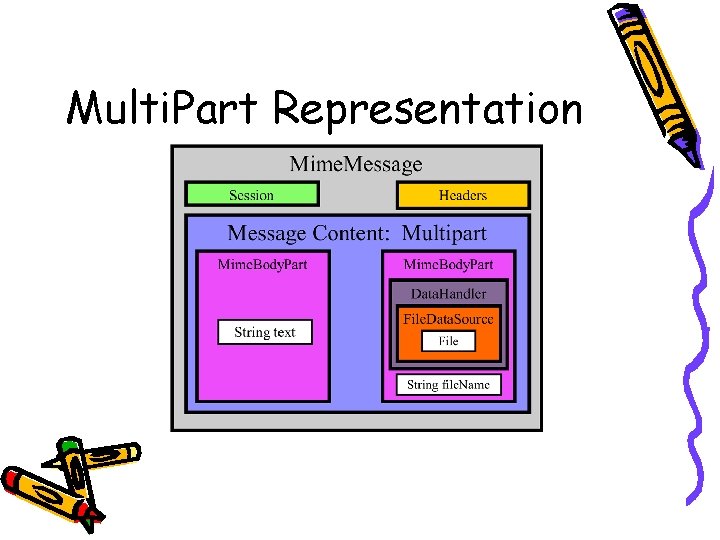
Multi. Part Representation
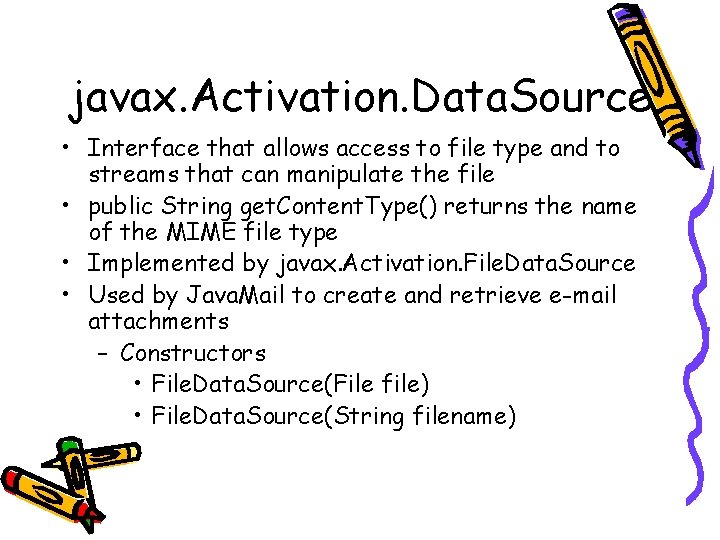
javax. Activation. Data. Source • Interface that allows access to file type and to streams that can manipulate the file • public String get. Content. Type() returns the name of the MIME file type • Implemented by javax. Activation. File. Data. Source • Used by Java. Mail to create and retrieve e-mail attachments – Constructors • File. Data. Source(File file) • File. Data. Source(String filename)
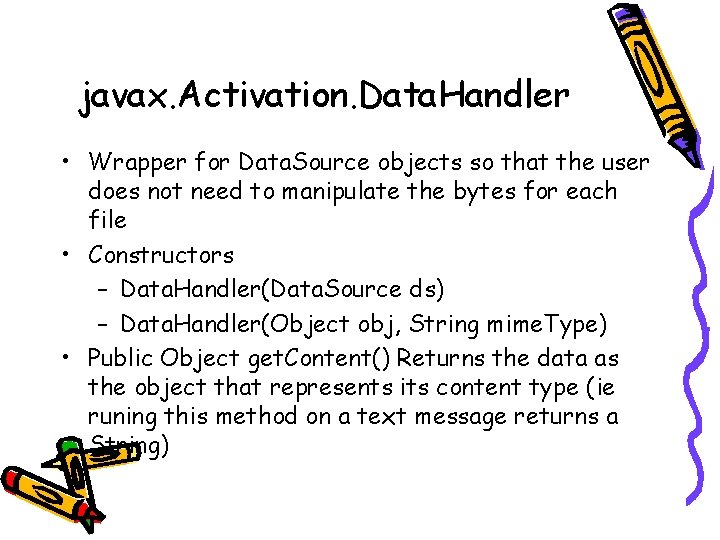
javax. Activation. Data. Handler • Wrapper for Data. Source objects so that the user does not need to manipulate the bytes for each file • Constructors – Data. Handler(Data. Source ds) – Data. Handler(Object obj, String mime. Type) • Public Object get. Content() Returns the data as the object that represents its content type (ie runing this method on a text message returns a String)
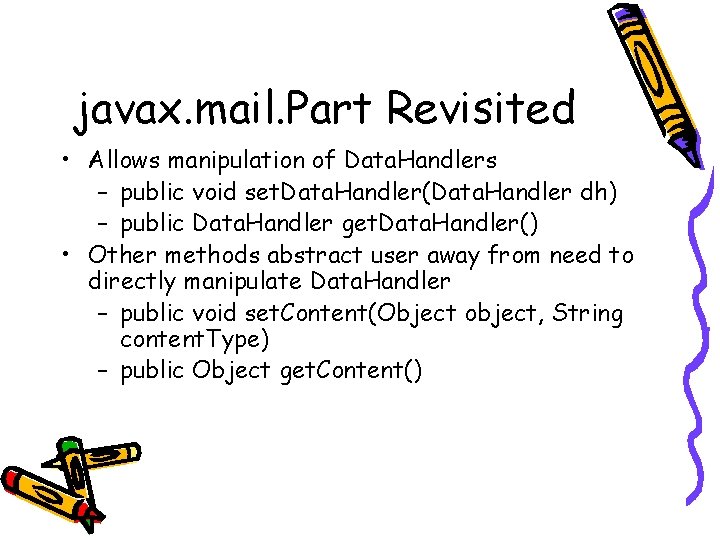
javax. mail. Part Revisited • Allows manipulation of Data. Handlers – public void set. Data. Handler(Data. Handler dh) – public Data. Handler get. Data. Handler() • Other methods abstract user away from need to directly manipulate Data. Handler – public void set. Content(Object object, String content. Type) – public Object get. Content()
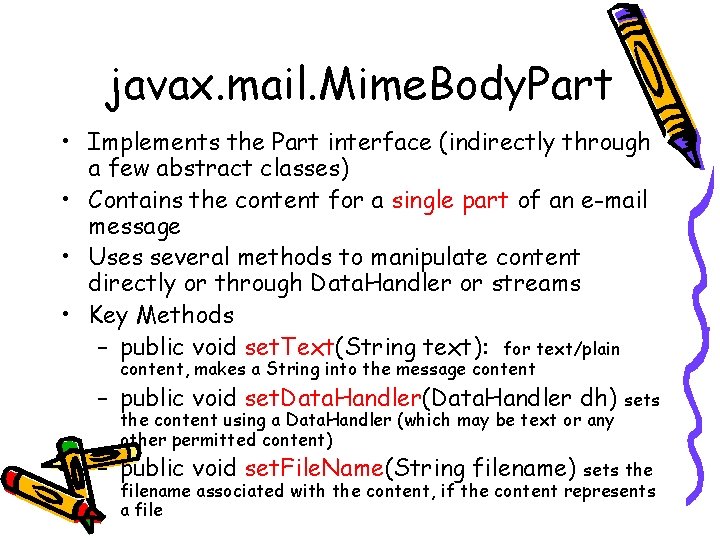
javax. mail. Mime. Body. Part • Implements the Part interface (indirectly through a few abstract classes) • Contains the content for a single part of an e-mail message • Uses several methods to manipulate content directly or through Data. Handler or streams • Key Methods – public void set. Text(String text): for text/plain content, makes a String into the message content – public void set. Data. Handler(Data. Handler dh) sets the content using a Data. Handler (which may be text or any other permitted content) – public void set. File. Name(String filename) sets the filename associated with the content, if the content represents a file
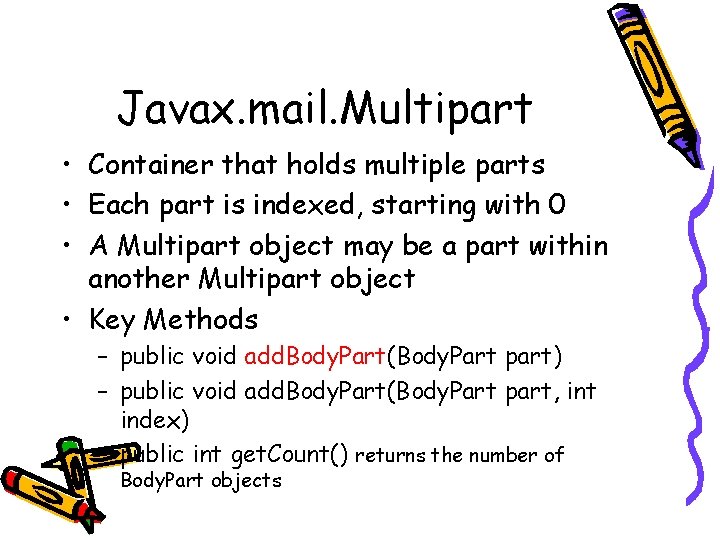
Javax. mail. Multipart • Container that holds multiple parts • Each part is indexed, starting with 0 • A Multipart object may be a part within another Multipart object • Key Methods – public void add. Body. Part(Body. Part part) – public void add. Body. Part(Body. Part part, int index) – public int get. Count() returns the number of Body. Part objects
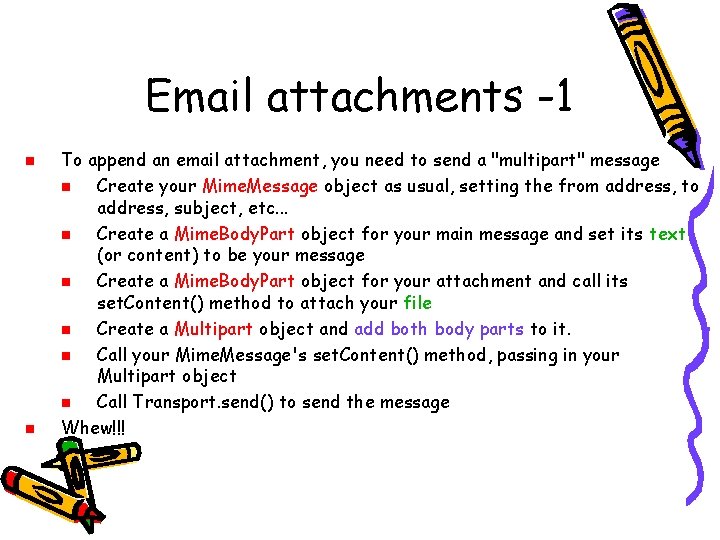
Email attachments -1 n n To append an email attachment, you need to send a "multipart" message n Create your Mime. Message object as usual, setting the from address, to address, subject, etc. . . n Create a Mime. Body. Part object for your main message and set its text (or content) to be your message n Create a Mime. Body. Part object for your attachment and call its set. Content() method to attach your file n Create a Multipart object and add both body parts to it. n Call your Mime. Message's set. Content() method, passing in your Multipart object n Call Transport. send() to send the message Whew!!!
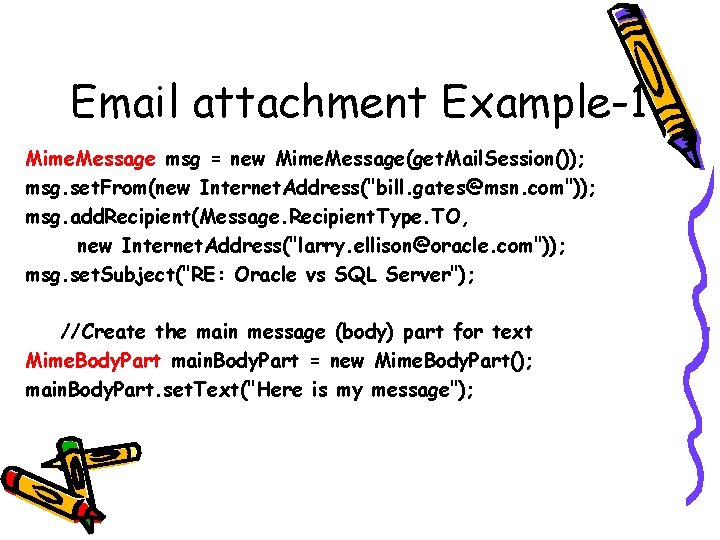
Email attachment Example-1 Mime. Message msg = new Mime. Message(get. Mail. Session()); msg. set. From(new Internet. Address("bill. gates@msn. com")); msg. add. Recipient(Message. Recipient. Type. TO, new Internet. Address("larry. ellison@oracle. com")); msg. set. Subject("RE: Oracle vs SQL Server"); //Create the main message (body) part for text Mime. Body. Part main. Body. Part = new Mime. Body. Part(); main. Body. Part. set. Text("Here is my message");
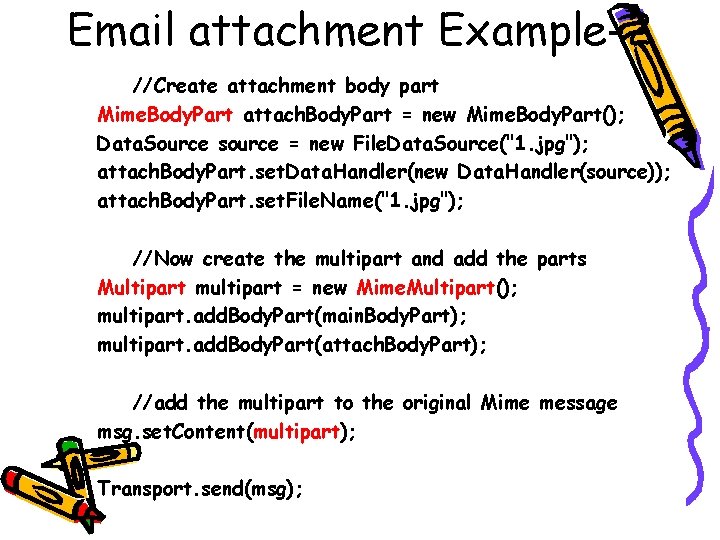
Email attachment Example-2 //Create attachment body part Mime. Body. Part attach. Body. Part = new Mime. Body. Part(); Data. Source source = new File. Data. Source("1. jpg"); attach. Body. Part. set. Data. Handler(new Data. Handler(source)); attach. Body. Part. set. File. Name("1. jpg"); //Now create the multipart and add the parts Multipart multipart = new Mime. Multipart(); multipart. add. Body. Part(main. Body. Part); multipart. add. Body. Part(attach. Body. Part); //add the multipart to the original Mime message msg. set. Content(multipart); Transport. send(msg);