Lecture J Exceptions Lecture J The Java API
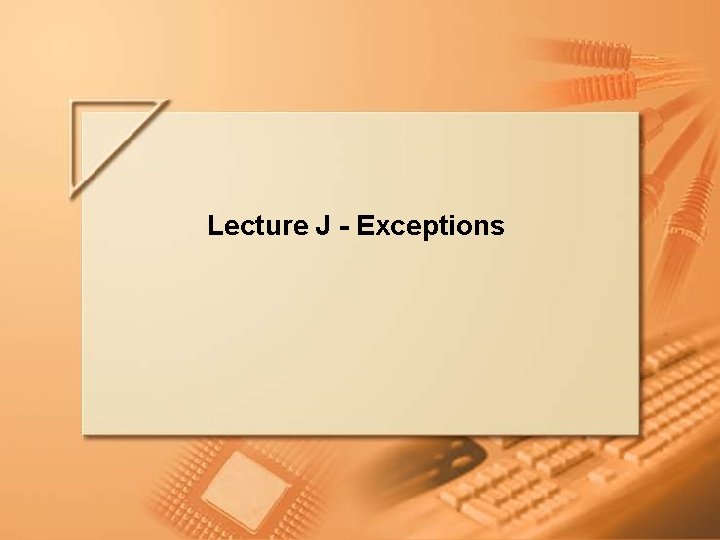
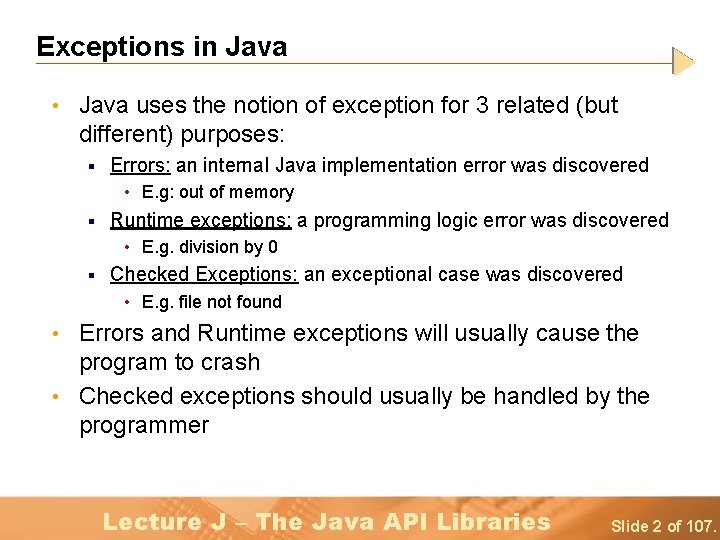
![Occurrence of a runtime exception public class Exception. Example { public static void main(String[] Occurrence of a runtime exception public class Exception. Example { public static void main(String[]](https://slidetodoc.com/presentation_image/212159855ef948c8e0094598c781b6a8/image-3.jpg)
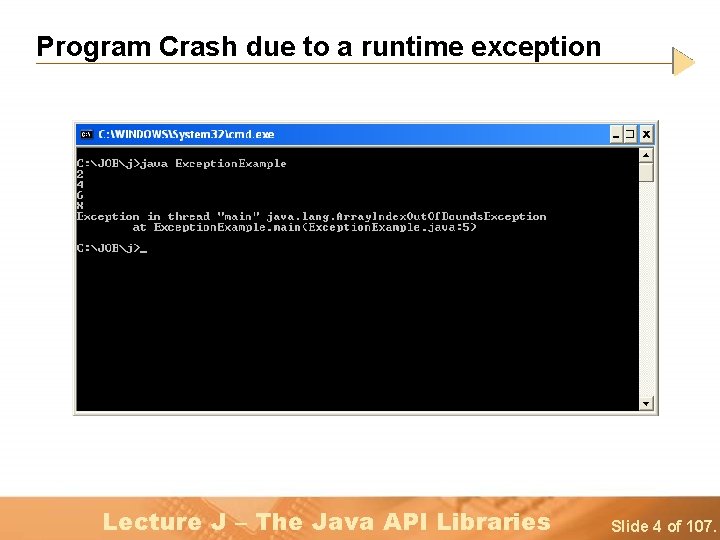
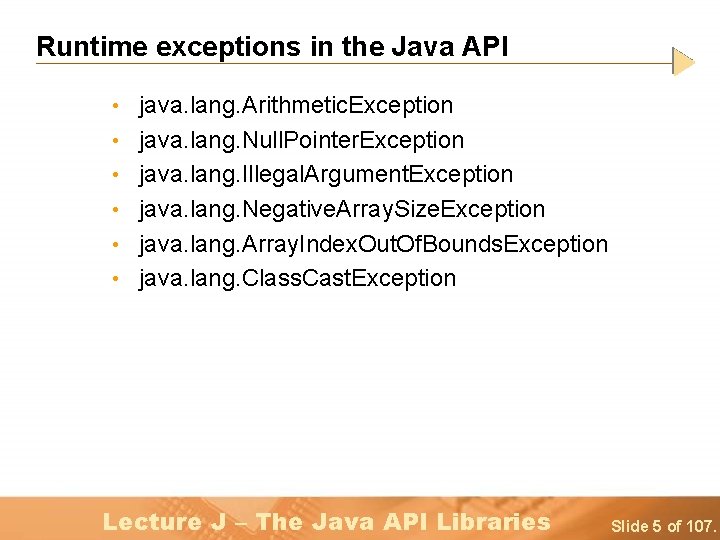
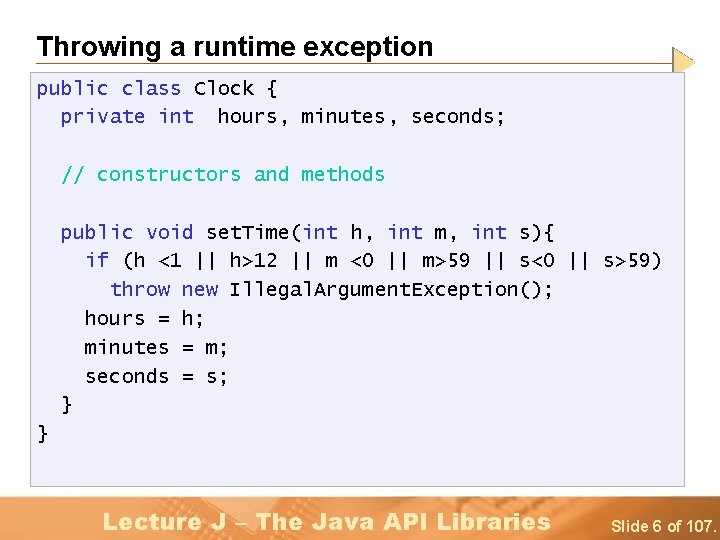
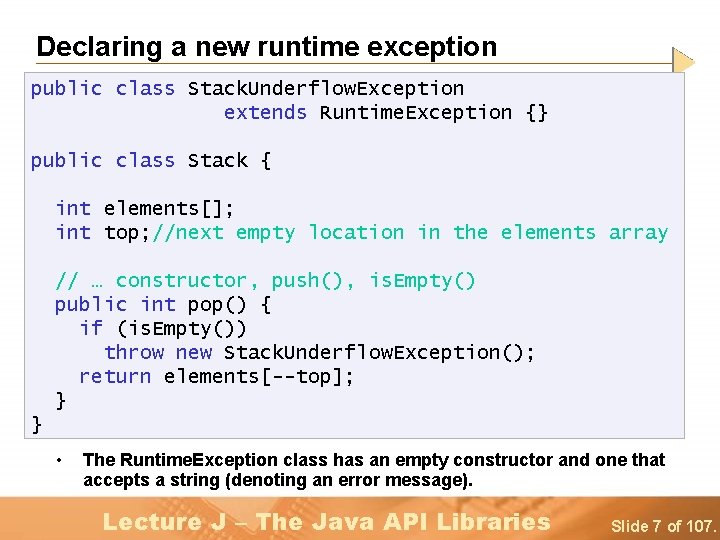
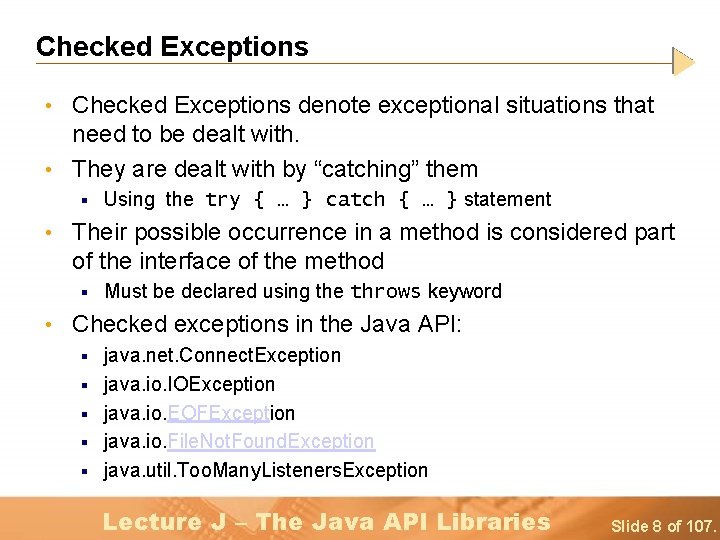
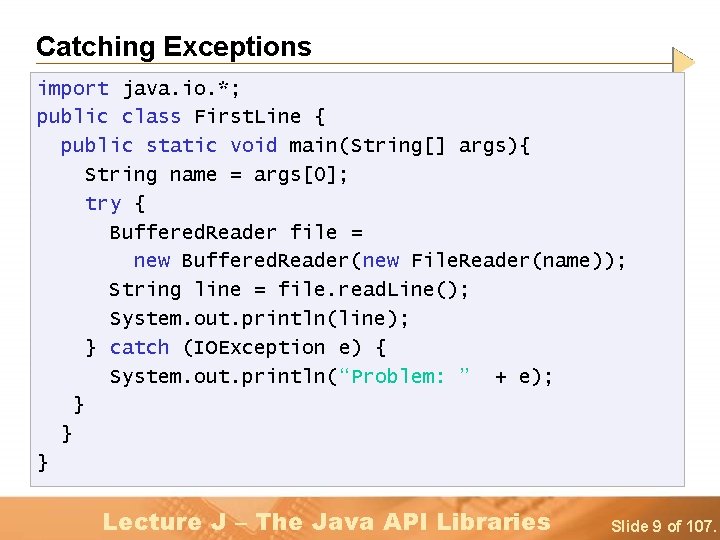
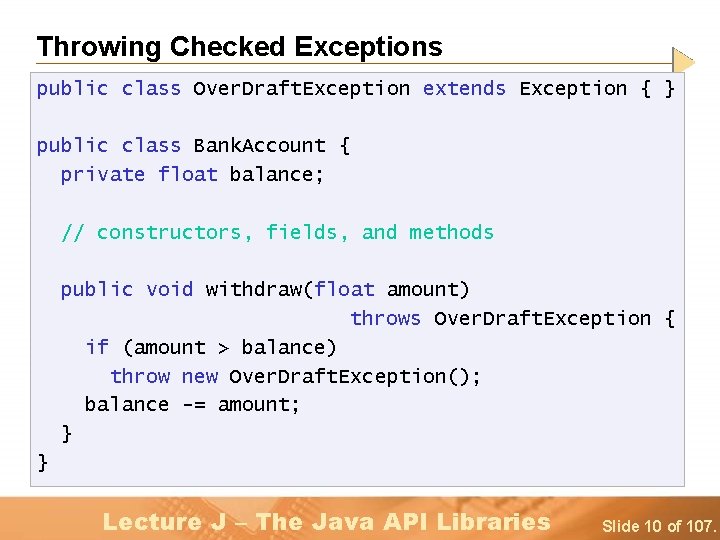
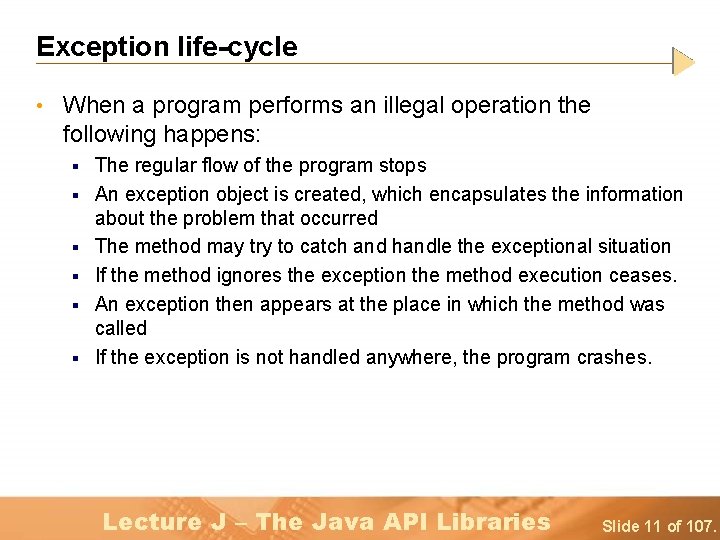
![Pumping up an exception public static void main(String[] args) { try { do. Withdraws(); Pumping up an exception public static void main(String[] args) { try { do. Withdraws();](https://slidetodoc.com/presentation_image/212159855ef948c8e0094598c781b6a8/image-12.jpg)
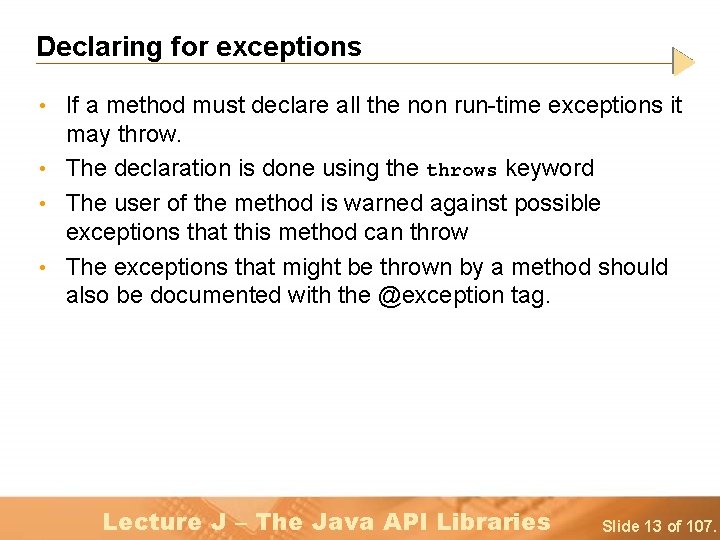
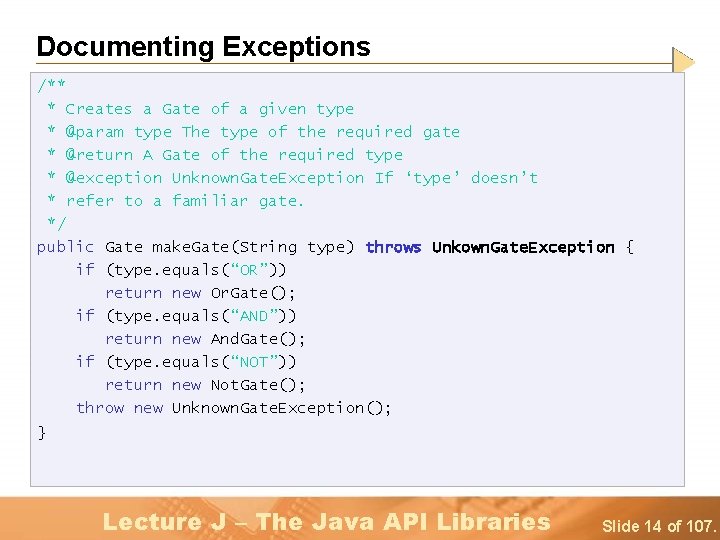
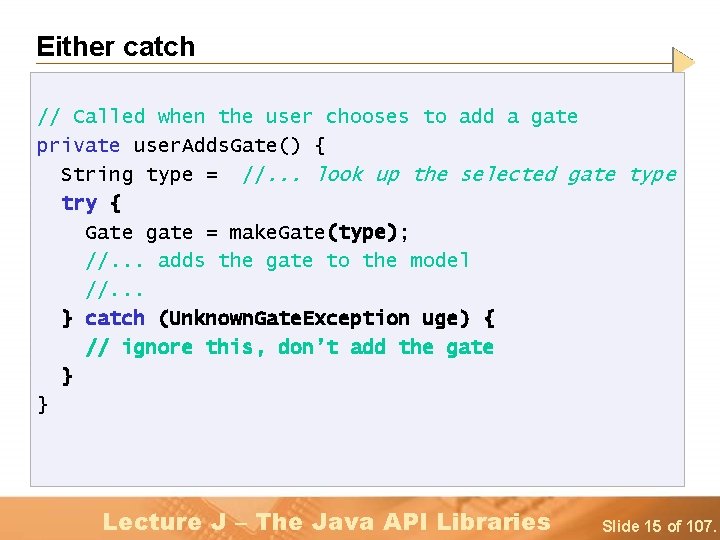
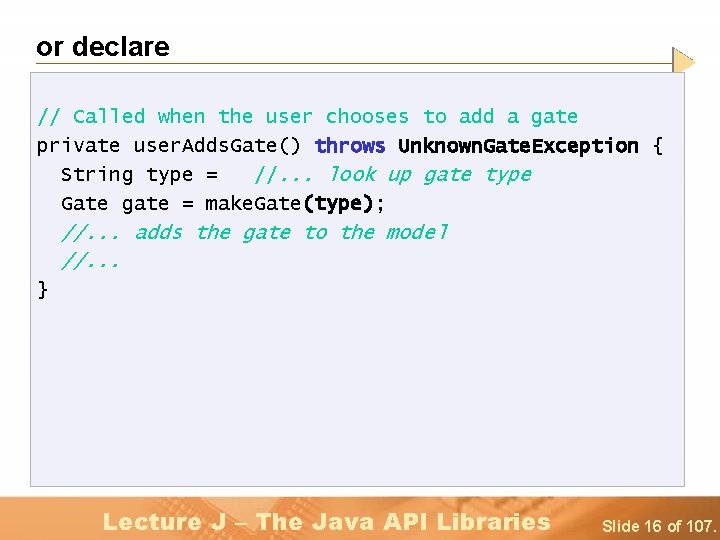
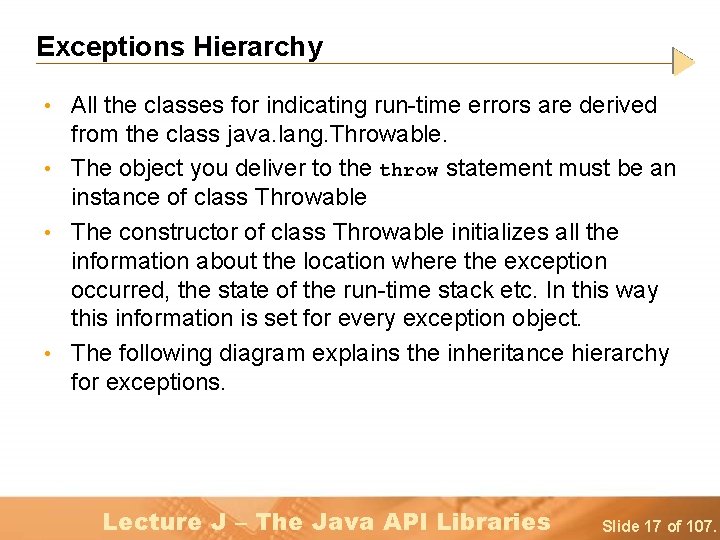
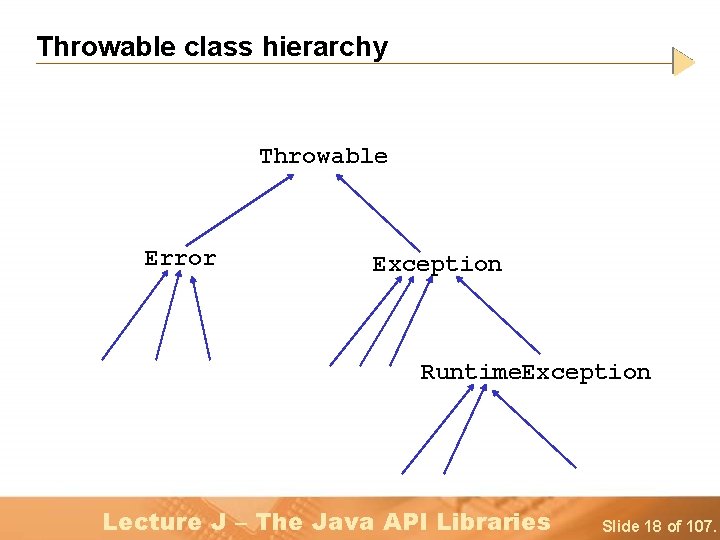
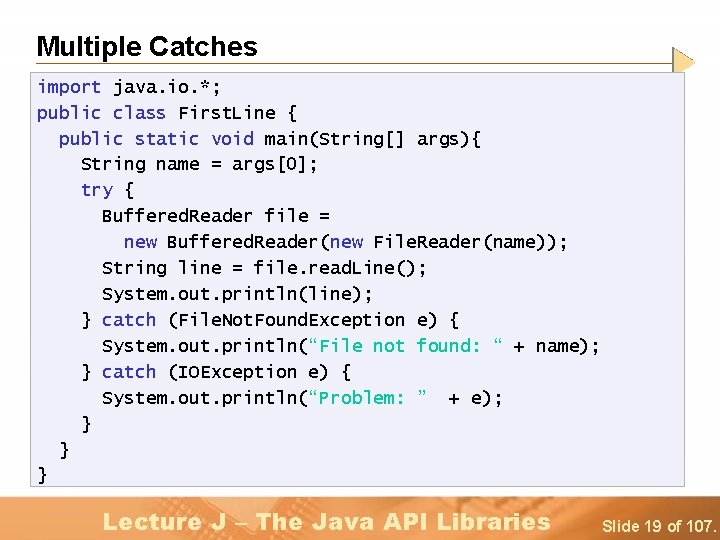
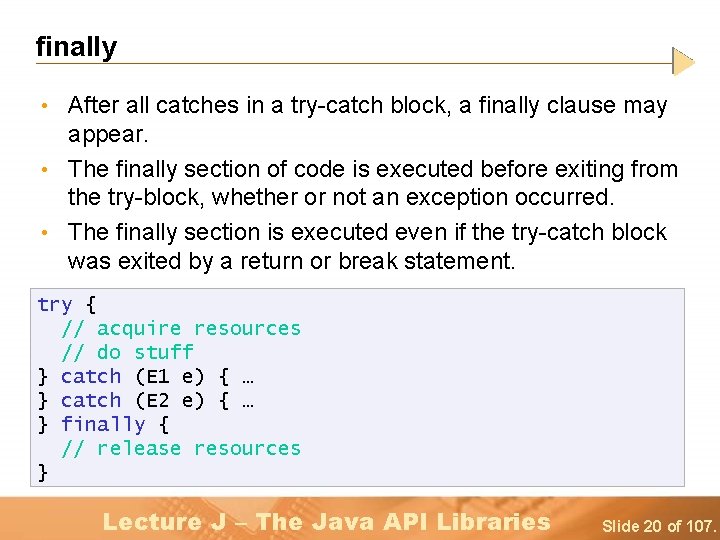
- Slides: 20
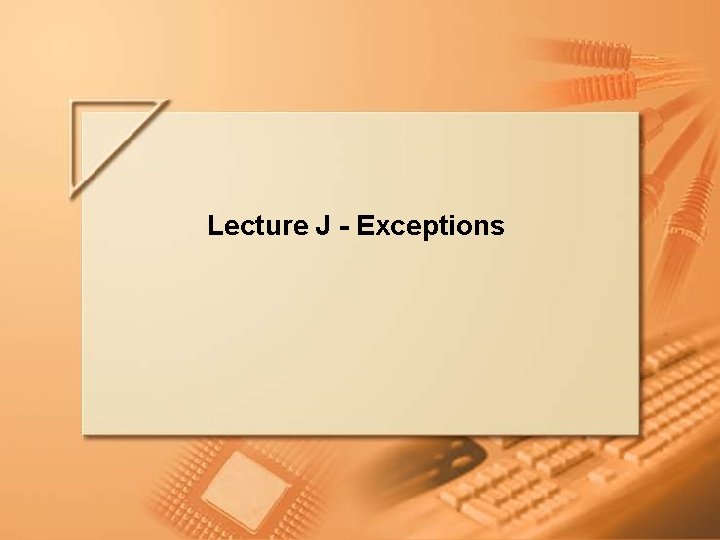
Lecture J - Exceptions Lecture J – The Java API Libraries Slide 1 of 107.
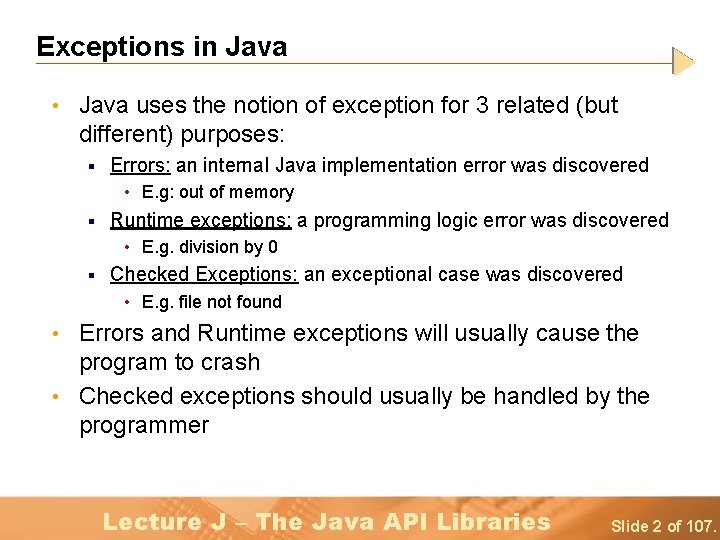
Exceptions in Java • Java uses the notion of exception for 3 related (but different) purposes: § Errors: an internal Java implementation error was discovered • E. g: out of memory § Runtime exceptions: a programming logic error was discovered • E. g. division by 0 § Checked Exceptions: an exceptional case was discovered • E. g. file not found • Errors and Runtime exceptions will usually cause the program to crash • Checked exceptions should usually be handled by the programmer Lecture J – The Java API Libraries Slide 2 of 107.
![Occurrence of a runtime exception public class Exception Example public static void mainString Occurrence of a runtime exception public class Exception. Example { public static void main(String[]](https://slidetodoc.com/presentation_image/212159855ef948c8e0094598c781b6a8/image-3.jpg)
Occurrence of a runtime exception public class Exception. Example { public static void main(String[] args) { int[] a = {2, 4, 6, 8}; for(int j = 0; j <= a. length ; j++) System. out. println(a[j]); } } Lecture J – The Java API Libraries Slide 3 of 107.
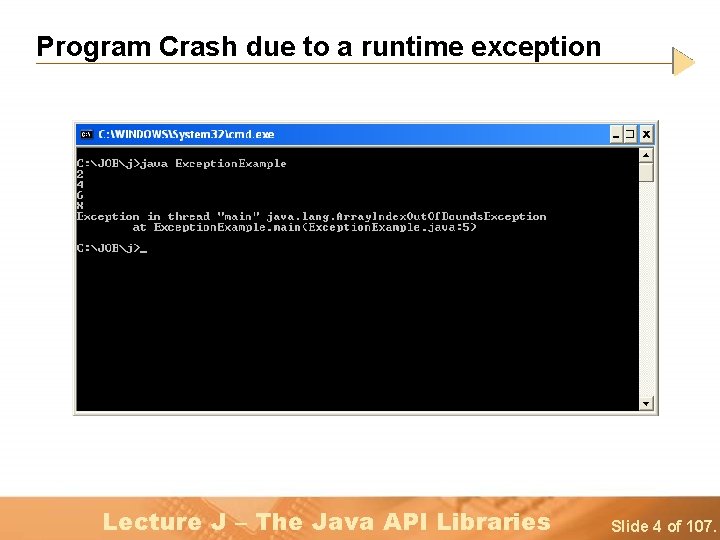
Program Crash due to a runtime exception Lecture J – The Java API Libraries Slide 4 of 107.
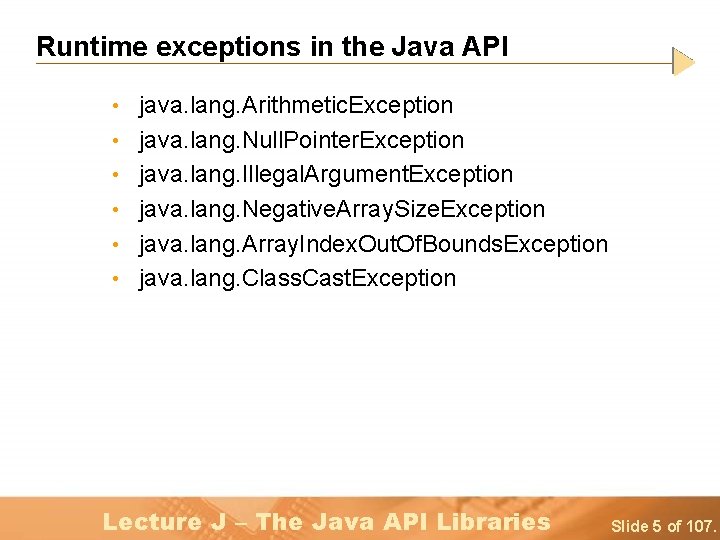
Runtime exceptions in the Java API • java. lang. Arithmetic. Exception • java. lang. Null. Pointer. Exception • java. lang. Illegal. Argument. Exception • java. lang. Negative. Array. Size. Exception • java. lang. Array. Index. Out. Of. Bounds. Exception • java. lang. Class. Cast. Exception Lecture J – The Java API Libraries Slide 5 of 107.
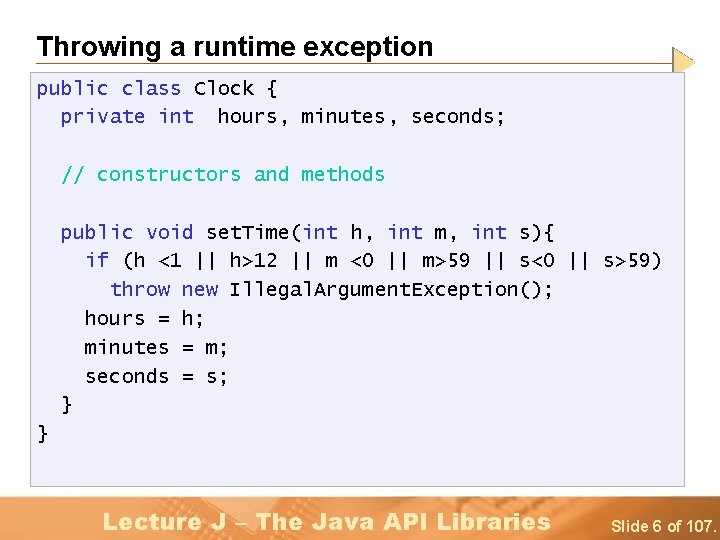
Throwing a runtime exception public class Clock { private int hours, minutes, seconds; // constructors and methods public void set. Time(int h, int m, int s){ if (h <1 || h>12 || m <0 || m>59 || s<0 || s>59) throw new Illegal. Argument. Exception(); hours = h; minutes = m; seconds = s; } } Lecture J – The Java API Libraries Slide 6 of 107.
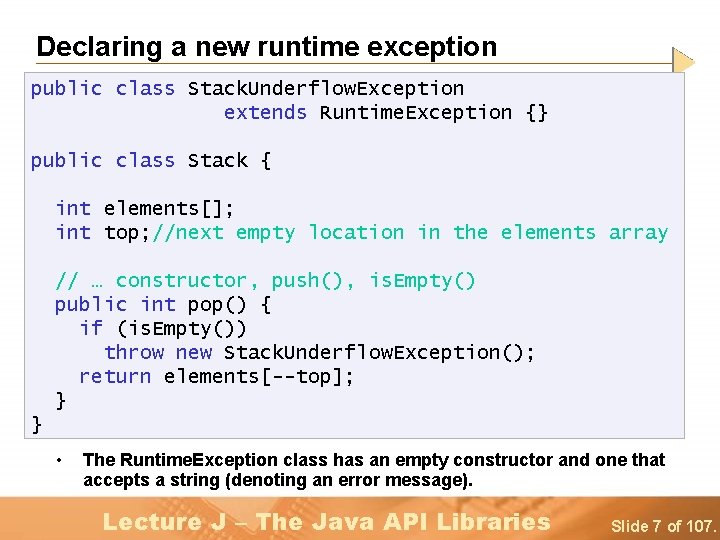
Declaring a new runtime exception public class Stack. Underflow. Exception extends Runtime. Exception {} public class Stack { int elements[]; int top; //next empty location in the elements array // … constructor, push(), is. Empty() public int pop() { if (is. Empty()) throw new Stack. Underflow. Exception(); return elements[--top]; } } • The Runtime. Exception class has an empty constructor and one that accepts a string (denoting an error message). Lecture J – The Java API Libraries Slide 7 of 107.
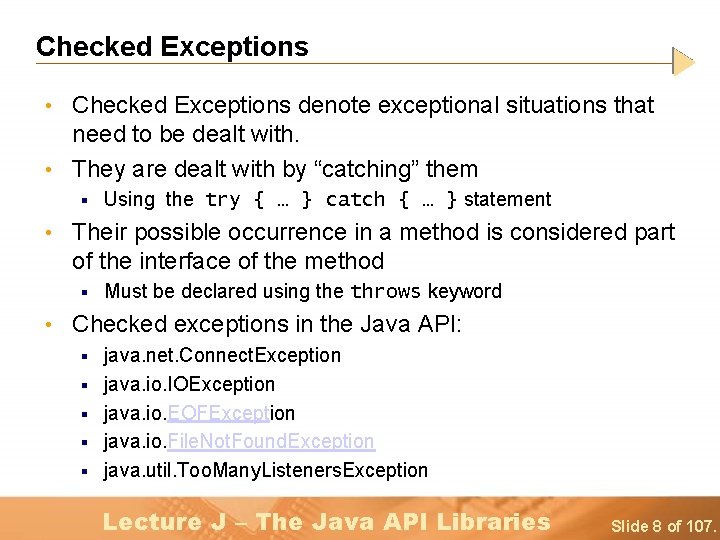
Checked Exceptions • Checked Exceptions denote exceptional situations that need to be dealt with. • They are dealt with by “catching” them § Using the try { … } catch { … } statement • Their possible occurrence in a method is considered part of the interface of the method § Must be declared using the throws keyword • Checked exceptions in the Java API: § § § java. net. Connect. Exception java. io. IOException java. io. EOFException java. io. File. Not. Found. Exception java. util. Too. Many. Listeners. Exception Lecture J – The Java API Libraries Slide 8 of 107.
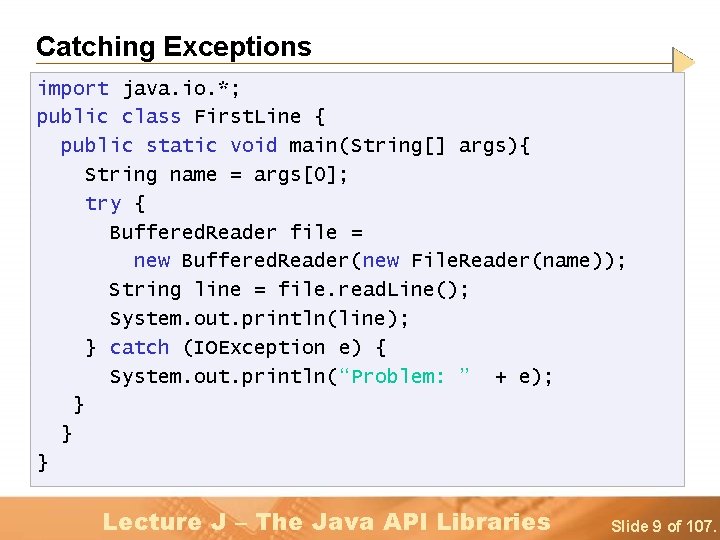
Catching Exceptions import java. io. *; public class First. Line { public static void main(String[] args){ String name = args[0]; try { Buffered. Reader file = new Buffered. Reader(new File. Reader(name)); String line = file. read. Line(); System. out. println(line); } catch (IOException e) { System. out. println(“Problem: ” + e); } } } Lecture J – The Java API Libraries Slide 9 of 107.
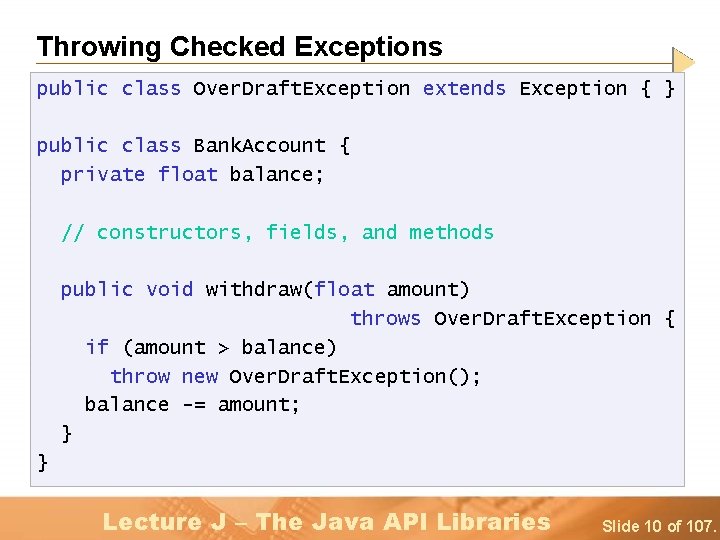
Throwing Checked Exceptions public class Over. Draft. Exception extends Exception { } public class Bank. Account { private float balance; // constructors, fields, and methods public void withdraw(float amount) throws Over. Draft. Exception { if (amount > balance) throw new Over. Draft. Exception(); balance -= amount; } } Lecture J – The Java API Libraries Slide 10 of 107.
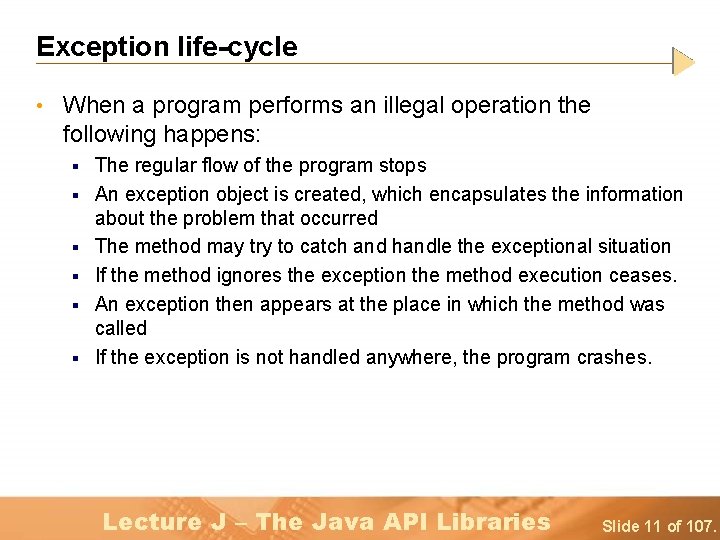
Exception life-cycle • When a program performs an illegal operation the following happens: § § § The regular flow of the program stops An exception object is created, which encapsulates the information about the problem that occurred The method may try to catch and handle the exceptional situation If the method ignores the exception the method execution ceases. An exception then appears at the place in which the method was called If the exception is not handled anywhere, the program crashes. Lecture J – The Java API Libraries Slide 11 of 107.
![Pumping up an exception public static void mainString args try do Withdraws Pumping up an exception public static void main(String[] args) { try { do. Withdraws();](https://slidetodoc.com/presentation_image/212159855ef948c8e0094598c781b6a8/image-12.jpg)
Pumping up an exception public static void main(String[] args) { try { do. Withdraws(); } catch (Over. Draft. Exception e) { call. Manager(); } } Overdraft! private static do. Withdraws() throws Over. Draft. Exception { // Get list of withdraw orders Hey, no one Catches this… for(/* iterate over withdraw orders */) bank. Account. withdraw(amount) I’ll crash the } method! Lecture J – The Java API Libraries Slide 12 of 107.
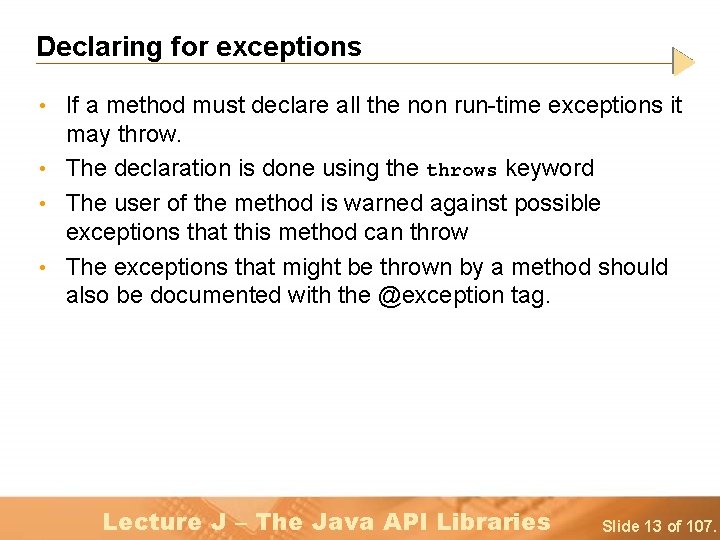
Declaring for exceptions • If a method must declare all the non run-time exceptions it may throw. • The declaration is done using the throws keyword • The user of the method is warned against possible exceptions that this method can throw • The exceptions that might be thrown by a method should also be documented with the @exception tag. Lecture J – The Java API Libraries Slide 13 of 107.
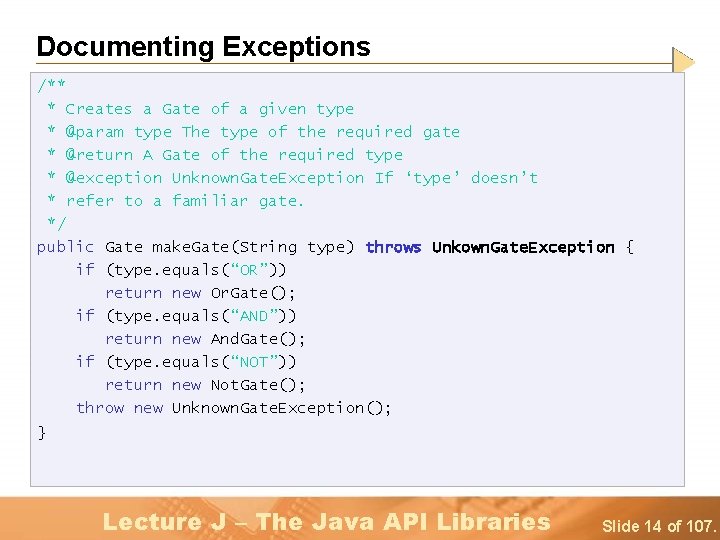
Documenting Exceptions /** * Creates a Gate of a given type * @param type The type of the required gate * @return A Gate of the required type * @exception Unknown. Gate. Exception If ‘type’ doesn’t * refer to a familiar gate. */ public Gate make. Gate(String type) throws Unkown. Gate. Exception { if (type. equals(“OR”)) return new Or. Gate(); if (type. equals(“AND”)) return new And. Gate(); if (type. equals(“NOT”)) return new Not. Gate(); throw new Unknown. Gate. Exception(); } Lecture J – The Java API Libraries Slide 14 of 107.
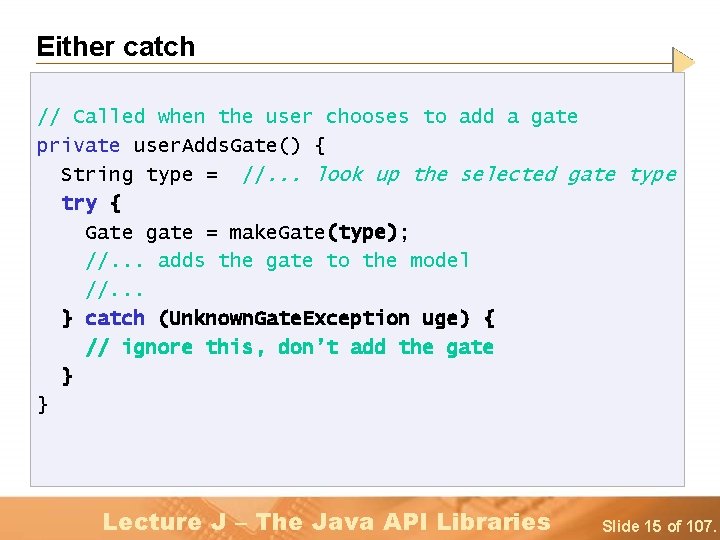
Either catch // Called when the user chooses to add a gate private user. Adds. Gate() { String type = //. . . look up the selected gate type try { Gate gate = make. Gate(type); //. . . adds the gate to the model //. . . } catch (Unknown. Gate. Exception uge) { // ignore this, don’t add the gate } } Lecture J – The Java API Libraries Slide 15 of 107.
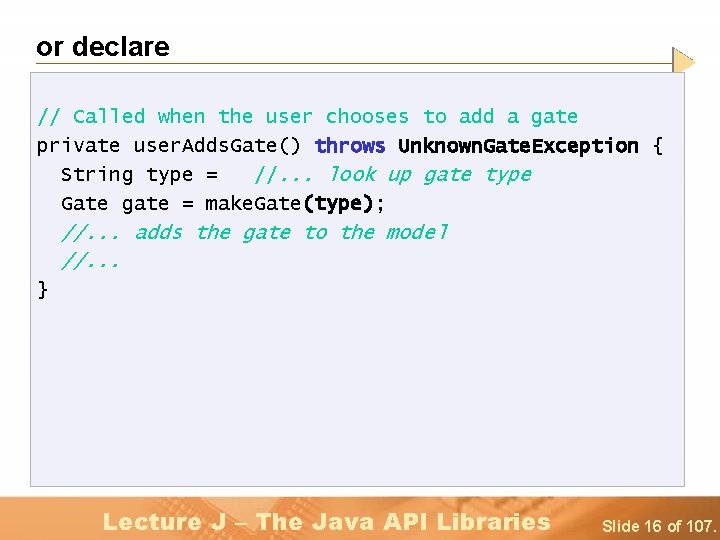
or declare // Called when the user chooses to add a gate private user. Adds. Gate() throws Unknown. Gate. Exception { String type = //. . . look up gate type Gate gate = make. Gate(type); //. . . adds the gate to the model //. . . } Lecture J – The Java API Libraries Slide 16 of 107.
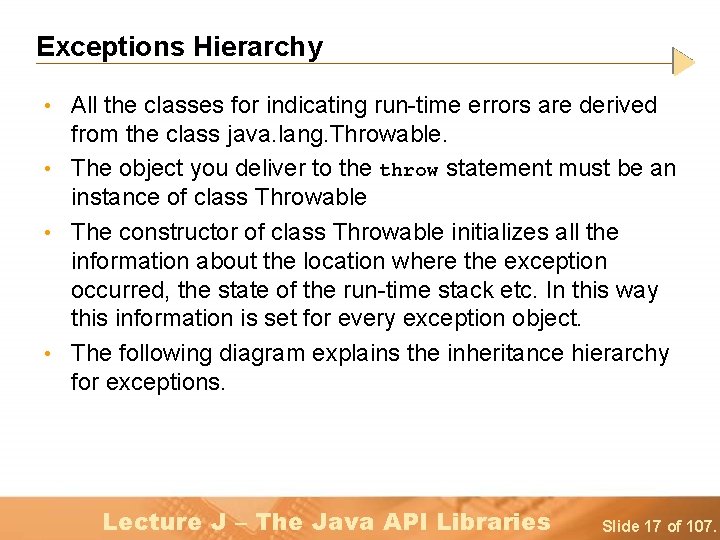
Exceptions Hierarchy • All the classes for indicating run-time errors are derived from the class java. lang. Throwable. • The object you deliver to the throw statement must be an instance of class Throwable • The constructor of class Throwable initializes all the information about the location where the exception occurred, the state of the run-time stack etc. In this way this information is set for every exception object. • The following diagram explains the inheritance hierarchy for exceptions. Lecture J – The Java API Libraries Slide 17 of 107.
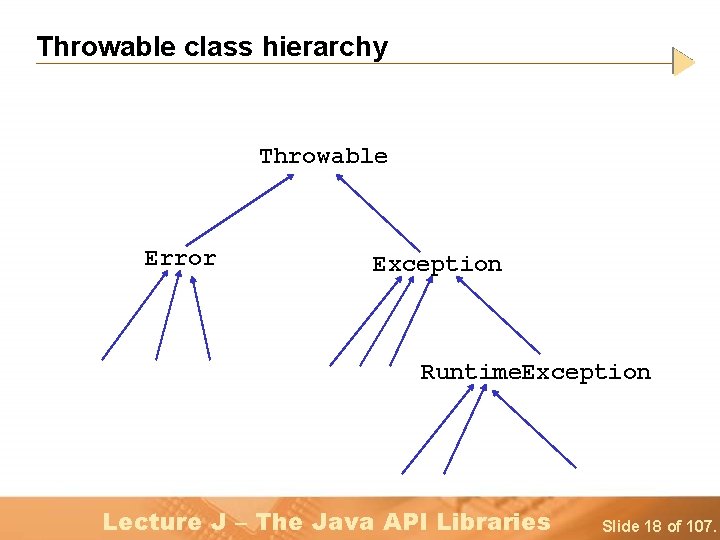
Throwable class hierarchy Throwable Error Exception Runtime. Exception Lecture J – The Java API Libraries Slide 18 of 107.
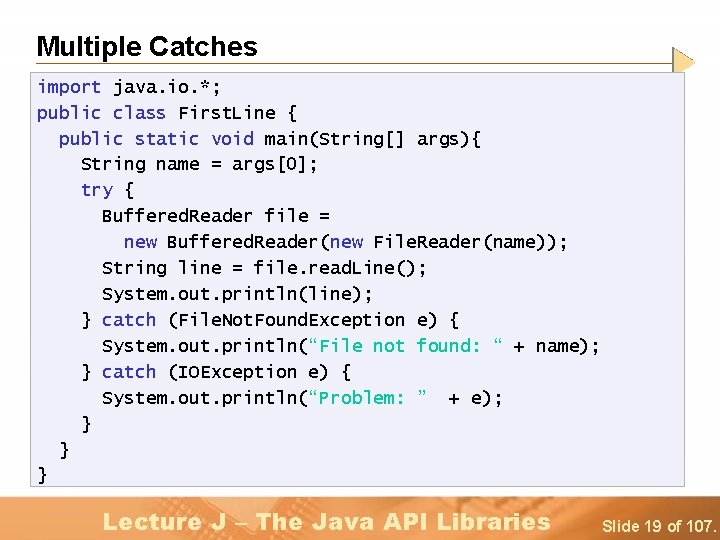
Multiple Catches import java. io. *; public class First. Line { public static void main(String[] args){ String name = args[0]; try { Buffered. Reader file = new Buffered. Reader(new File. Reader(name)); String line = file. read. Line(); System. out. println(line); } catch (File. Not. Found. Exception e) { System. out. println(“File not found: “ + name); } catch (IOException e) { System. out. println(“Problem: ” + e); } } } Lecture J – The Java API Libraries Slide 19 of 107.
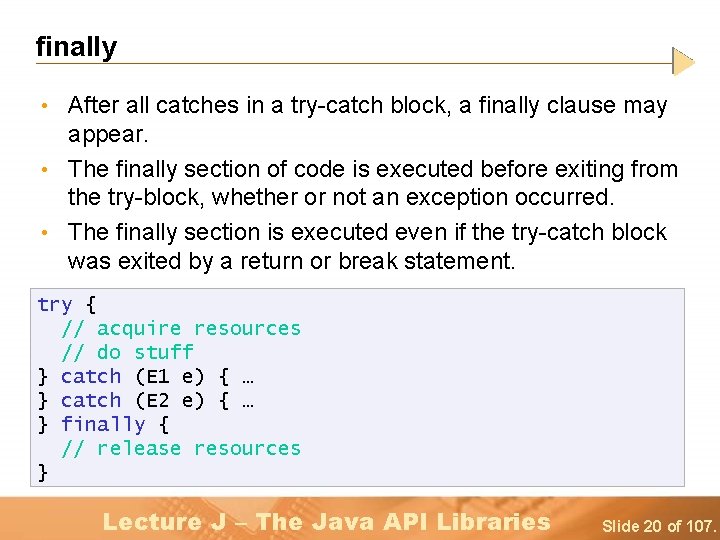
finally • After all catches in a try-catch block, a finally clause may appear. • The finally section of code is executed before exiting from the try-block, whether or not an exception occurred. • The finally section is executed even if the try-catch block was exited by a return or break statement. try { // acquire resources // do stuff } catch (E 1 e) { … } catch (E 2 e) { … } finally { // release resources } Lecture J – The Java API Libraries Slide 20 of 107.